repo
stringlengths 26
115
| file
stringlengths 54
212
| language
stringclasses 2
values | license
stringclasses 16
values | content
stringlengths 19
1.07M
|
---|---|---|---|---|
https://github.com/jgm/typst-hs | https://raw.githubusercontent.com/jgm/typst-hs/main/test/typ/compiler/closure-03.typ | typst | Other | // Capture environment.
#{
let mark = "!"
let greet = {
let hi = "Hi"
name => {
hi + ", " + name + mark
}
}
test(greet("Typst"), "Hi, Typst!")
// Changing the captured variable after the closure definition has no effect.
mark = "?"
test(greet("Typst"), "Hi, Typst!")
}
|
https://github.com/0x1B05/algorithm-journey | https://raw.githubusercontent.com/0x1B05/algorithm-journey/main/practice/note/content/数论.typ | typst | #import "../template.typ": *
#pagebreak()
= 数论
== 最大公约数 and 最小公倍数
证明辗转相除法就是证明关系: `gcd(a, b) = gcd(b, a % b)`
假设`a % b = r`,即需要证明的关系为:`gcd(a, b) = gcd(b, r)`
证明过程:
因为`a % b = r`,所以如下两个等式必然成立
1. `a = b * n + r`,n 为 0、1、2、3....中的一个整数
2. `r = a − b * n`,n 为 0、1、2、3....中的一个整数
假设 u 是 b 和 r 的公因子,则有: `b = x * u, r = y * u`; 把 b 和 r 带入 1 得到,`a = x * u * n + y * u = (x * n + y) * u`
这说明 : u 如果是 b 和 r 的公因子,那么 u 也是 a 的因子
假设 v 是 a 和 b 的公因子,则有: `a = s * v`, `b = t * v`
把 a 和 b 带入 2 得到,`r = s * v - t * v * n = (s - t * n) * v`
这说明 : v 如果是 a 和 b 的公因子,那么 v 也是 r 的因子
综上,a 和 b 的每一个公因子 也是 b 和 r 的一个公因子,反之亦然
所以,a 和 b 的全体公因子集合 = b 和 r 的全体公因子集合, 即 `gcd(a, b) = gcd(b, r)`
#code(caption: [gcd && lcm])[
```java
public static long gcd(long a, long b) {
return b == 0 ? a : gcd(b, a % b);
}
public static long lcm(long a, long b) {
return (long) a / gcd(a, b) * b;
}
```
]
=== 题目
==== #link("https://leetcode.cn/problems/nth-magical-number/")[ 第 N 个神奇数字 ]
一个正整数如果能被 a 或 b 整除,那么它是神奇的。
给定三个整数 n , a , b ,返回第 n 个神奇的数字。
#tip("Tip")[
因为答案可能很大,所以返回答案 对 10^9 + 7 取模后的值。
]
===== 解答
先估计答案范围, 一定 `1~min(a, b)` 之间
#code(caption: [解答])[
```java
public class Code02_NthMagicalNumber {
public static int nthMagicalNumber(int n, int a, int b) {
long lcm = lcm(a, b);
long ans = 0;
// l = 0
// r = (long) n * Math.min(a, b)
// l......r
for (long l = 0, r = (long) n * Math.min(a, b), m = 0; l <= r;) {
m = (l + r) / 2;
// 1....m
if (m / a + m / b - m / lcm >= n) {
ans = m;
r = m - 1;
} else {
l = m + 1;
}
}
return (int) (ans % 1000000007);
}
public static long gcd(long a, long b) {
return b == 0 ? a : gcd(b, a % b);
}
public static long lcm(long a, long b) {
return (long) a / gcd(a, b) * b;
}
}
```
]
== 同余原理
#code(caption: [模相同])[
```java
import java.math.BigInteger;
// 加法、减法、乘法的同余原理
// 不包括除法,因为除法必须求逆元,后续课讲述
public class Code03_SameMod {
// 为了测试
public static long random() {
return (long) (Math.random() * Long.MAX_VALUE);
}
// 计算 ((a + b) * (c - d) + (a * c - b * d)) % mod 的非负结果
public static int f1(long a, long b, long c, long d, int mod) {
BigInteger o1 = new BigInteger(String.valueOf(a)); // a
BigInteger o2 = new BigInteger(String.valueOf(b)); // b
BigInteger o3 = new BigInteger(String.valueOf(c)); // c
BigInteger o4 = new BigInteger(String.valueOf(d)); // d
BigInteger o5 = o1.add(o2); // a + b
BigInteger o6 = o3.subtract(o4); // c - d
BigInteger o7 = o1.multiply(o3); // a * c
BigInteger o8 = o2.multiply(o4); // b * d
BigInteger o9 = o5.multiply(o6); // (a + b) * (c - d)
BigInteger o10 = o7.subtract(o8); // (a * c - b * d)
BigInteger o11 = o9.add(o10); // ((a + b) * (c - d) + (a * c - b * d))
// ((a + b) * (c - d) + (a * c - b * d)) % mod
BigInteger o12 = o11.mod(new BigInteger(String.valueOf(mod)));
if (o12.signum() == -1) {
// 如果是负数那么+mod返回
return o12.add(new BigInteger(String.valueOf(mod))).intValue();
} else {
// 如果不是负数直接返回
return o12.intValue();
}
}
// 计算 ((a + b) * (c - d) + (a * c - b * d)) % mod 的非负结果
public static int f2(long a, long b, long c, long d, int mod) {
int o1 = (int) (a % mod); // a
int o2 = (int) (b % mod); // b
int o3 = (int) (c % mod); // c
int o4 = (int) (d % mod); // d
int o5 = (o1 + o2) % mod; // a + b
int o6 = (o3 - o4 + mod) % mod; // c - d
int o7 = (int) (((long) o1 * o3) % mod); // a * c
int o8 = (int) (((long) o2 * o4) % mod); // b * d
int o9 = (int) (((long) o5 * o6) % mod); // (a + b) * (c - d)
int o10 = (o7 - o8 + mod) % mod; // (a * c - b * d)
int ans = (o9 + o10) % mod; // ((a + b) * (c - d) + (a * c - b * d)) % mod
return ans;
}
public static void main(String[] args) {
System.out.println("测试开始");
int testTime = 100000;
int mod = 1000000007;
for (int i = 0; i < testTime; i++) {
long a = random();
long b = random();
long c = random();
long d = random();
if (f1(a, b, c, d, mod) != f2(a, b, c, d, mod)) {
System.out.println("出错了!");
}
}
System.out.println("测试结束");
System.out.println("===");
long a = random();
long b = random();
long c = random();
long d = random();
System.out.println("a : " + a);
System.out.println("b : " + b);
System.out.println("c : " + c);
System.out.println("d : " + d);
System.out.println("===");
System.out.println("f1 : " + f1(a, b, c, d, mod));
System.out.println("f2 : " + f2(a, b, c, d, mod));
}
}
```
]
总结:
- 加法`a+b -> (a+b)%MOD`
- 减法`a-b -> (MOD+a-b)%MOD`
- 乘法`a*b-> int x = ((long)a*b)%MOD`
|
|
https://github.com/kotfind/hse-se-2-notes | https://raw.githubusercontent.com/kotfind/hse-se-2-notes/master/algo/seminars/2024-09-09.typ | typst | = Введение
Семинарская оценка --- работа на семинаре, задачки на дом (до следующего
семинара)
Посещение не учитывается
== Проверка цикличности списка (алгоритм зайца и черепахи)
Черепаха прыгает на 1, заяц --- на два
Если заяц упирается в конец списка, то ацикличен
Если заяц и черепаха встретились, то цикличен
|
|
https://github.com/xrarch/books | https://raw.githubusercontent.com/xrarch/books/main/xrcomputerbook/titlepage.typ | typst | #page([
#image("xrcomputersystemshandbook.png", fit: "stretch")
], margin: (
top: 0cm,
bottom: 0cm,
left: 0cm,
right: 0cm,
))
#pagebreak()
*XR/station Project* \
*XR/computer Systems Handbook* \
_Revision 1.0, January 5, 2024_ |
|
https://github.com/whs/typst-govdoc | https://raw.githubusercontent.com/whs/typst-govdoc/master/README.md | markdown | # Thai Government Document with Typst (เขียนหนังสือราชการด้วยโปรแกรม Typst)
เพื่อเป็นการฝึกใช้งานระบบจัดหน้า [Typst](https://typst.app) ซึ่งเป็นซอฟต์แวร์ Open Source
จึงได้ทดลองจัดทำตามรูปแบบหนังสือราชการ และอัพโหลดไว้สำหรับผู้สนใจ
* [ตัวอย่างเอกสาร](govdoctest.pdf)
## คุณสมบัติ
สามารถจัดเนื้อหาในส่วนต่อไปนี้
* ชั้นความเร็ว
* ชั้นความลับ
* ตราครุฑ
* ที่
* ผู้ส่ง
* เรื่อง เรียน
* อ้างถึง
* สิ่งที่ส่งมาด้วย
* เนื้อความ
* ลงชื่อ
* ผู้ส่งแนบท้าย
ณ ขณะที่พิมพ์
* โปรแกรม Typst ยังไม่รองรับการตัดคำภาษาไทย (จะต้องแทรกเว้นวรรคเพื่อขึ้นบรรทัดใหม่ด้วยตนเอง)
* ไม่สามารถแทรกข้อความขึ้นหน้าใหม่ข้างท้ายได้อัตโนมัติ
* ไม่สามารถแปลงเลขไทยได้อัตโนมัติ (ต้องใช้คำสั่ง `thnum`)
* ไม่สามารถเว้นกั้นหน้าก่อนย่อหน้าแรกในเนื้อความอัตโนมัติ
## การใช้งาน
ณ ขณะนี้ยังไม่มีระบบมอดูลสำหรับ Typst จึงจะต้องคัดลอกไฟล์ไปเอง
1. คัดลอกไฟล์ <thai.typ>, <govdoc.typ>, <garuda.svg> ไปยังจุดที่ใช้งาน
2. เครื่องที่ใช้งานจะต้องติดตั้งโปรแกรม Typst และแบบอักษร TH Sarabun New (ขณะนี้ยังไม่สามารถติดตั้งบน Typst web application ได้ ต้องติดตั้งบนเครื่องคอมพิวเตอร์เท่านั้น)
3. สามารถดูตัวอย่างการใช้งานได้ที่ <govdoctest.typ>
### คำสั่ง
#### thnum
คำสั่ง `thnum` จะแปลงข้อมูลประเภทตัวเลขหรือ string ให้เป็นเลขไทย สามารถระบุข้อความอื่นๆ ที่ไม่ใช่ตัวเลขเข้ามาได้ แต่ไม่สามารถส่ง block เข้ามาได้
คำสั่งนี้จะไม่เติมเครื่องหมายจุลภาค (ลูกน้ำ) ในหลักพัน
**การใช้งาน**
```typst
เอกสารนี้มีจำนวน #thnum(1) หน้า
#thnum("วันที่ 1 มกราคม 2566")
```
#### gov
คำสั่ง `gov` เป็นการจัดหน้าเอกสารให้ตรงตามมาตรฐานของราชการ รวมถึงเรียกใช้คำสั่ง thai เพื่อเปลี่ยนแบบอักษร (ฟอนต์) อัตโนมัติ
**การใช้งาน**
```typst
#import "govdoc.typ": gov
#show: gov
```
#### govhead
คำสั่ง `govhead` จะแสดงส่วนขึ้นต้นของเอกสารตั้งแต่สิ่งที่ส่งมาด้วย ขึ้นไปจนถึงหัวเอกสาร
**การใช้งาน**
```typst
#import "govdoc.typ": thnum, govhead
#govhead(
secrecy: "ลับ", // ชั้นความลับ
urgency: "ด่วน", // ชั้นความเร็ว
id: thnum("ตย 1234/ 5678"),
address: [
หน่วยงาน \
ที่ตั้ง \
จังหวัด #thnum(10110)
],
date: thnum("1 มกราคม 2566"),
title: "ตัวอย่างเอกสาร", // เรื่อง
attention: "ผู้อ่าน", // เรียน
refer-to: "เอกสารก่อนหน้า", // อ้างถึง
attachments: ( // สิ่งที่ส่งมาด้วย ระบุเป็น array
"เอกสารแรก",
thnum("เอกสารที่ 2"),
// เพิ่มจำนวนได้ตามต้องการ
),
)
```
หากไม่ใช้งานในส่วนใด สามารถลบบรรทัดที่ส่งส่วนนั้นออกได้
#### govsign
คำสั่ง `govsign` จะแสดงส่วนลงท้ายของเอกสาร
**การใช้งาน**
```typst
#import "govdoc.typ": govsign
#govsign(
signoff: "คำลงท้าย",
name: "ชื่อ",
position: "ตำแหน่ง",
)
```
หากไม่ระบุ signoff จะกำหนดเป็น "ขอแสดงความนับถือ" โดยอัตโนมัติ
#### govsender
คำสั่ง `govsender` จะแสดงเนื้อหาหลังจากเว้นช่องไฟสำหรับที่อยู่ผู้ส่ง
**การใช้งาน**
```typst
#import "govdoc.typ": govsender
#govsender([
หน่วยงานผู้ส่ง \
ที่อยู่ \
โทร. #thnum("1111") \
โทรสาร #thnum("02 111 1111")
])
```
## ลิขสิทธิ์
ไม่สงวนลิขสิทธิ์
|
|
https://github.com/catppuccin/typst | https://raw.githubusercontent.com/catppuccin/typst/main/manual/template.typ | typst | MIT License | #import "../src/lib.typ": catppuccin, themes, get-palette
#import "@preview/codly:0.1.0": *
// The project function defines how your document looks.
// It takes your content and some metadata and formats it.
// Go ahead and customize it to your liking!
#let project(
title: "",
subtitle: "",
abstract: [],
authors: (),
url: none,
date: none,
version: none,
flavor: themes.mocha,
body,
) = {
let palette = get-palette(flavor)
// Set the document's basic properties.
set document(author: authors, title: title)
set page(numbering: "1", number-align: center)
// set text(font: "Linux Libertine", lang: "en")
set text(font: "Nunito", hyphenate: false, lang: "en")
show: catppuccin.with(flavor, code-block: true, code-syntax: true)
show heading.where(level: 1): set text(font: "Jellee")
show heading.where(level: 2): set text(font: "Jellee")
show heading.where(level: 1): it => block(smallcaps(it), below: 1em)
set heading(numbering: (..args) => if args.pos().len() <= 3 {
numbering("1.1.", ..args)
})
show figure.caption: set text(size: 0.8em, fill: palette.colors.subtext0.rgb)
show list: pad.with(x: 5%)
let ctp-blue = palette.colors.blue.rgb
show link: set text(fill: ctp-blue)
// show link: it => if type(it.element) != ref {
// underline
// }
// show ref: it => {
// set text(fill: ctp-blue)
// underline[#it]
// }
v(1fr)
// Title row.
align(center)[
#block(text(weight: 700, 1.75em, title, font: "Jellee"))
#block(text(1.0em, subtitle))
#v(1em, weak: true)
#let logo = sys.inputs.at("logo")
#image(logo, width: 4cm)
#v(1em, weak: true)
v#version #h(1.2cm) #date
#block(link(url))
#v(1.5em, weak: true)
]
// Author information.
pad(
top: 0.5em,
x: 2em,
grid(
columns: (1fr,) * calc.min(3, authors.len()),
gutter: 1em,
..authors.map(author => align(center, strong(author))),
),
)
v(1fr, weak: true)
// Abstract.
pad(
x: 3.8em,
top: 1em,
bottom: 1.1em,
align(center)[
#heading(outlined: false, numbering: none, text(0.85em, smallcaps[Abstract]))
#abstract
],
)
// Main body.
set par(justify: true)
v(1fr)
pad(x: 10%, outline(depth: 3, indent: auto))
v(1fr)
pagebreak()
show: codly-init
body
}
#let TeX = style(styles => {
set text(font: "Linux Libertine")
let e = measure(text(1em, "E"), styles)
let T = "T"
let E = text(1em, baseline: e.height / 4, "E")
let X = "X"
box(T + h(-0.1em) + E + h(-0.125em) + X)
})
#let LaTeX = style(styles => {
set text(font: "Linux Libertine")
let l = measure(text(1em, "L"), styles)
let a = measure(text(0.7em, "A"), styles)
let L = "L"
let A = text(0.7em, baseline: a.height - l.height, "A")
box(L + h(-0.3em) + A + h(-0.1em) + TeX)
})
#let to-title(string) = {
assert(string.len() > 0, message: "String is empty")
if string.len() == 1 {
return upper(string.at(0))
}
return upper(string.at(0)) + string.slice(1)
}
#let make-namespace(name: none, scope: (:), ..modules) = {
let contents = ""
for module in modules.pos() {
let mod = read("../src/" + module)
assert.ne(mod, none, message: "Module not found: " + module)
contents += mod + "\n"
}
assert.ne(modules, "", message: "Err: " + repr(modules))
assert.ne(name, none, message: "Namespace name is required")
return (
name: name,
scope: scope,
contents: contents,
)
}
|
https://github.com/EpicEricEE/typst-equate | https://raw.githubusercontent.com/EpicEricEE/typst-equate/master/tests/align-points/test.typ | typst | MIT License | #import "/src/lib.typ": equate
#set page(width: 8cm, height: auto, margin: 1em)
#show: equate
// Test re-implemented alignment algorithm.
$ a + b &= c \
&= d + e $
$ a + b &= c + d &= e + f \
g &= & + h $
$ a + b &= c + d &&= e + f \
g & &&= h $
$ a + b &= c \
d & &= e + f $
$ "text" & "text" \
& "text" $
// Cases below taken from Typst test suite.
$ "a" &= c \
&= c + 1 & "By definition" \
&= d + 100 + 1000 \
&= x & & "Even longer" \
$
$ & "right" \
"a very long line" \
"left" $
$ "right" \
"a very long line" \
"left" \ $
$ a &= b & quad c &= d \
e &= f & g &= h $
|
https://github.com/fabriceHategekimana/master | https://raw.githubusercontent.com/fabriceHategekimana/master/main/2_Etat_de_l_art/STLC.typ | typst | #import "@preview/simplebnf:0.1.0": *
#import "../src/module.typ" : *
=== Le lambda calcul simplement typé
Malgré le fait qu'il soit Turing-complet. Le lambda calcul simple manque de pas mal de fonctionnalités qui vont nous aider à représenter les tableaux multidimensionnels. L'une d'entre elle et la notion de type. C'est pourquoi nous introduisons le lambda calcul simplement typé.
En effet, le lambda calcul simplement typé est le prochain pas vers la construction de notre premier langage. Il est une extension du lambda calcul non typé où chaque expression est associée à un type. Ce typage introduit une structure supplémentaire qui aide à prévenir certaines formes d'erreurs computationnelles et à garantir des propriétés de sûreté dans les programmes.
#Definition[Syntaxe du lambda calcul simplement typé
$ #bnf(
Prod(
$t$,
delim: "term",
{
Or[$x$][_variable_]
Or[$lambda x: T.t$][_abstraction_]
Or[$t space t$][_application_]
},
),
Prod(
$v$,
delim: "value",
{
Or[$lambda x: T.t$][_abstraction value_]
},
),
Prod(
$T$,
delim: "types",
{
Or[$T → T$][_type of functions_]
},
),
Prod(
$Gamma$,
delim: "context",
{
Or[$emptyset$][_empty context_]
Or[$Gamma, "x:T"$][_term variable binding_]
},
),
) $
]
#Definition[Évaluation du lambda calcul simplement typé
$ #proof-tree(eval("E-APP1", $t_1 t_2 --> t_1p t_2$, $t_1 --> t_1p$)) $
$ #proof-tree(eval("E-APP2", $v_1 t_2 --> v_1 t_2p$, $t_2 --> t_2p$)) $
$ #proof-tree(eval("E-APPABS", $(lambda x . t_12) v_2 --> [x\/v_2] t_12$)) $
]
#Definition[Typage du lambda calcul simplement typé
$ #proof-tree(typing_c("T-VAR", "x : T", $ x:T in Gamma$)) $
$ #proof-tree((typing_c("T-ABS", $lambda x:T_1 . t_2 : T_1 -> T_2$))) $
$ #proof-tree(typing_c("T-APP", $t_1 t_2 : T_12 $, $Gamma tack.r : T_11 -> T_12$, $Gamma tack.r t_2 : T_11$)) $
]
Le lambda calculs simplement typé ajoute des types de base au choix et la définition de type de fonction. Le lambda calcul simplement typé est moins expressif que le lambda calcul classique mais offre plus de sécurité dans ses manipulations. On sera en mesure de faire des calculs plus sûrs dans notre langage, mais ce n'est pas encore suffisant pour assurer assez de sécurité pour la manipulation de tableaux multidimensionnels.
|
|
https://github.com/GabrielDTB/basalt-backlinks | https://raw.githubusercontent.com/GabrielDTB/basalt-backlinks/main/README.md | markdown | MIT License | A Typst package for generating and getting backlinks.
# Setup
```typ
#import "@preview/basalt-backlinks:0.1.0" as backlinks
#show link: backlinks.generate
```
# Usage
```typ
Here's some content I want to link to. <linktome>
#pagebreak()
#link(<linktome>)[I'm linking to the content.]
#pagebreak()
#link(<linktome>)[I'm also linking to the content!]
#pagebreak()
#context {
let backs = backlinks.get(<linktome>)
for (i, back) in backs.enumerate() [
#link(back.location())[
Backlink for \<linktome> (\##i)
]
]
}
```
|
https://github.com/leesum1/brilliant-cv | https://raw.githubusercontent.com/leesum1/brilliant-cv/master/cv.typ | typst | // Imports
#import "@preview/brilliant-cv:2.0.2": cv
#let metadata = toml("./metadata.toml")
#let importModules(modules, lang: metadata.language) = {
for module in modules {
include {
"modules_" + lang + "/" + module + ".typ"
}
}
}
#show: cv.with(
metadata,
profilePhoto: image("./src/github.png")
)
// #show "AXI4": [*AXI4*]
// #show "ICache": [*ICache*]
// #show "DCache": [*DCache*]
// #show "TLB": [*TLB*]
// #show "ysyx-SOC": {
// link("https://github.com/OSCPU/ysyxSoC/blob/ysyx4/ysyx/README.md")[*ysyx-SOC*]
// }
// #show "一生一芯":{
// link("https://ysyx.oscc.cc/project/intro.html")[一生一芯]
// }
// #show "LVGL" : {
// link("https://lvgl.io/")[*LVGL*]
// }
// #show "RT-Thread": {
// link("https://github.com/RT-Thread/rt-thread")[RT-Thread]
// }
// #show "Ibex": {
// link("https://github.com/lowRISC/ibex")[Ibex]
// }
// #show "riscv-tests": {
// link("https://github.com/riscv-software-src/riscv-tests")[riscv-tests]
// }
// #show "xmake": {
// link("https://github.com/xmake-io/xmake")[riscv-tests]
// }
#importModules((
"education",
"professional",
"projects",
// "pullrequst",
"certificates",
"skills",
))
|
|
https://github.com/typst/packages | https://raw.githubusercontent.com/typst/packages/main/packages/preview/unichar/0.1.0/ucd/block-10190.typ | typst | Apache License 2.0 | #let data = (
("ROMAN SEXTANS SIGN", "So", 0),
("ROMAN UNCIA SIGN", "So", 0),
("ROMAN SEMUNCIA SIGN", "So", 0),
("ROMAN SEXTULA SIGN", "So", 0),
("ROMAN DIMIDIA SEXTULA SIGN", "So", 0),
("ROMAN SILIQUA SIGN", "So", 0),
("ROMAN DENARIUS SIGN", "So", 0),
("ROMAN QUINARIUS SIGN", "So", 0),
("ROMAN SESTERTIUS SIGN", "So", 0),
("ROMAN DUPONDIUS SIGN", "So", 0),
("ROMAN AS SIGN", "So", 0),
("ROMAN CENTURIAL SIGN", "So", 0),
("ASCIA SYMBOL", "So", 0),
(),
(),
(),
("GREEK SYMBOL TAU RHO", "So", 0),
)
|
https://github.com/GeorgeDong32/GD-Typst-Templates | https://raw.githubusercontent.com/GeorgeDong32/GD-Typst-Templates/main/examples/example-for-homework.typ | typst | Apache License 2.0 | #import "../templates/homework.typ": *
#show: homework.with(
title: "作业一",
subject: "Typst作业编写课程",
name: "张三三",
stdid: "11223344",
)
= 作业第一部分
#lorem(50)
== 第一题
#booktab(
width: 100%,
aligns: (left + horizon, center, right + bottom),
columns: (1fr, 1fr, 1fr),
caption: [`booktab` 示例],
[左对齐], [居中], [右对齐],
[4], [5], [6],
[7], [8], [9],
[10], [$ (n(n+1)) / 2 $], [11],
)
== 第二题
=== 第一小问
$ sum_(k=0)^n k
&= 1 + ... + n \
&= (n(n+1)) / 2 $
=== 第二小问
```python
import plotly.express as px
import pandas as pd
df_seeds = pd.read_csv('./seeds.csv')
df_flights = pd.read_csv('./flights.csv')
df_seeds.head()
df_flights.head()
px.line(df_seeds, x='kernel_length', y='groove_length' )
px.scatter(df_seeds, x='kernel_length', y='asymmetry_coefficient', color='species', title='seed data', marginal_x='violin', marginal_y='histogram')
px.histogram(df_seeds, x='kernel_length', color='species', title='seed data')
px.box(df_seeds, x='species', y='kernel_length', color='species', title='seed data')
px.scatter_3d(df_seeds, x='kernel_length', y='kernel_width', z='groove_length', color='species', title='seed data')
```
= 作业第二部分
3.1 设 $cal(V)$ 和 $cal(W)$ 是两 Hilbert 空间,${ket(v_j)}$ 和 ${ket(w_j)}$ 分别为 $cal(V)$ 和 $cal(W)$ 的一组正交模基。设 $display(T_A: sum_(j = 1)^n c_j ket(v_j) arrow.r sum_(j=1)^n c_j sum_(i=1)^m a_(i j) ket(w_j))$,证明 $T_A$ 是线性算子。
证明:对于 $forall k in NN, 0 < k <= n$,可以取一组 $display(c_j = cases(
1 "," j = k,
0 "," j != k
))$,由上述定义,可得到:
$ T_A (sum_(j = 1)^n c_j ket(v_j)) &= T_A (c_k ket(v_j)) = T_A (ket(v_j)) \
&= sum_(j=1)^n c_j sum_(i=1)^m a_(i j) ket(w_j) = c_k sum_(i=1)^m a_(i j) ket(w_j) = sum_(i=1)^m a_(i j) ket(w_j) $
则对于任意的一组 $c_j$,可以得到:
$ T_A (sum_(j = 1)^n c_j ket(v_j)) = sum_(j=1)^n c_j sum_(i=1)^m a_(i j) ket(w_j) = sum_(j=1)^n c_j T_A (ket(v_j)) $
即 $T_A$ 是线性算子。 |
https://github.com/QuadnucYard/crossregex-typ | https://raw.githubusercontent.com/QuadnucYard/crossregex-typ/main/src/regex.typ | typst | MIT License | #let regex-plugin = plugin("crossregex.wasm")
// we use a plugin because typst regex does not support back-ref
#let regex-match(re, str) = {
let r = regex-plugin.regex_match(bytes(re), bytes(str))
r.at(0) > 0
}
|
https://github.com/The-Notebookinator/notebookinator | https://raw.githubusercontent.com/The-Notebookinator/notebookinator/main/themes/linear/linear.typ | typst | The Unlicense | #import "rules.typ": rules
#import "entries.typ": cover, frontmatter-entry, body-entry, appendix-entry
#import "format.typ": set-border, set-heading
#import "components/components.typ"
#import "colors.typ": *
#import "/utils.typ"
#let linear-theme = utils.make-theme(
// Global show rules
rules: rules,
cover: cover,
// Entry pages
frontmatter-entry: frontmatter-entry,
body-entry: body-entry,
appendix-entry: appendix-entry,
)
|
https://github.com/SWATEngineering/Docs | https://raw.githubusercontent.com/SWATEngineering/Docs/main/src/2_RTB/LetteraDiPresentazioneRTB/content.typ | typst | MIT License | #import "functions.typ": glossary, team
/*************************************/
/* INSERIRE SOTTO IL CONTENUTO */
/*************************************/
E<NAME> Vardanega e Cardin,
#v(25pt)
con la presente il gruppo #team esprime la propria intenzione di candidarsi alla revisione di avanzamento _Requirements & Technology Baseline (RTB)_ per il capitolato
#align(
center,
strong("InnovaCity")
)
proposto dall'azienda _SyncLab_.
All'interno del seguente #underline(link("https://swatengineering.github.io/","link")), sotto la sezione _2_RTB_, è possibile trovare tutta la documentazione richiesta, in particolare:
- _AnalisiDeiRequisiti_1.0.pdf_;
- _Glossario_1.0.pdf_;
- _NormeDiProgetto_1.0.pdf_;
- _PianoDiProgetto_1.0.pdf_;
- _PianoDiQualifica_1.0.pdf_;
oltre a tutti i _Verbali Esterni_ ed _Interni_ divisi nelle relative categorie.
Viene inoltre fornito il _Proof of Concept_.
#team si assume l'impegno di completare il progetto entro il 08/04/2024, preventivando costi sempre per un importo totale di *11,070€*.
L'importo rimane invariato rispetto a quanto preventivato in sede di candidatura, nonostante il team abbia dovuto modificare la ripartizione delle ore produttive a disposizione per ruolo; in particolare, si è deciso di allocare più ore al ruolo di Amministratore, e marginalmente anche ai ruoli di Responsabile e Verificatore. Ne risulta dunque un nuovo preventivo con lo stesso numero di ore e lo stesso costo di partenza.
#v(50pt)
<NAME>,
#team. |
https://github.com/qlaush/template_thesis_stuttgart_imvt | https://raw.githubusercontent.com/qlaush/template_thesis_stuttgart_imvt/main/content/proposal.typ | typst | MIT License | #import "/utils/todo.typ": TODO
#TODO(color: red)[ // Remove this block
Before you start with your thesis, have a look at our guides on Confluence!
#link("https://confluence.ase.in.tum.de/display/EduResStud/How+to+thesis")
]
#set heading(numbering: none)
= Abstract
#TODO[ // Remove this block
*Abstract*
- Provide a brief summary of the proposed work
- What is the main content, the main contribution?
- What is your methodology? How do you proceed?
]
#set heading(numbering: "1.1")
= Introduction
#TODO[ // Remove this block
*Introduction*
- Introduce the reader to the general setting
- What is the environment?
- What are the tools in use?
]
= Problem
#TODO[ // Remove this block
*Problem description*
- What is/are the problem(s)?
- Identify the actors and use these to describe how the problem negatively influences them.
- Do not present solutions or alternatives yet!
- Present the negative consequences in detail
]
= Motivation
#TODO[ // Remove this block
*Thesis Motivation*
- Outline why it is important to solve the problem
- Again use the actors to present your solution, but don’t be to specific
- Be visionary!
- If applicable, motivate with existing research, previous work
]
#pagebreak(weak: true)
= Objective
#TODO[ // Remove this block
*Thesis Objective*
- What are the main goals of your thesis?
]
= Schedule
#TODO[ // Remove this block
*Thesis Schedule*
- When will the thesis start
- Create a rough plan for your thesis (separate the time in sprints with a length of 2-4 weeks)
- Each sprint should contain several work items - Again keep it high-level and make to keep your plan realistic
- Make sure the work-items are measurable and deliverable
- No writing related tasks! (e.g. ”Write Analysis Chapter”)
]
|
https://github.com/polarkac/MTG-Stories | https://raw.githubusercontent.com/polarkac/MTG-Stories/master/stories/011_Journey%20into%20Nyx.typ | typst | #import "@local/mtgset:0.1.0": conf
#show: doc => conf("Journey into Nyx", doc)
#include "./011 - Journey into Nyx/001_Ajani, Mentor of Heroes.typ"
#include "./011 - Journey into Nyx/002_Desperate Stand.typ"
#include "./011 - Journey into Nyx/003_Dreams of the City.typ"
#include "./011 - Journey into Nyx/004_Thank the Gods.typ"
#include "./011 - Journey into Nyx/005_The Path or the Horizon.typ"
#include "./011 - Journey into Nyx/006_Kruphix's Insight.typ"
|
|
https://github.com/cyx2015s/PhyLabReportTemplateTypst | https://raw.githubusercontent.com/cyx2015s/PhyLabReportTemplateTypst/main/phylab.typ | typst | #let phylab(
name: "实验名称",
instructor: "指导教师",
class: "班级",
author: "姓名",
author-id: "学号",
date: datetime.today(),
week: 1,
am-pm: "上午",
body
) = {
let 小初 = 36pt
let 三号 = 16pt
let 四号 = 14pt
let 小四 = 12pt
// 设置文档元数据
set document(title: author + "-"+ name, author: author)
// 设置字体
set text(font: "SimSun", size: 四号)
show raw: set text(font: ("Consolas", "SimSun"), size: 10pt)
show heading.where(level: 1): set heading(numbering: "一、")
show heading.where(level: 1): set text(stroke: 0.03em)
// 设置纸张大小与页边距
set page(paper: "a4", margin: (top: 2.54cm, bottom: 2.54cm, left: 1.91cm, right: 1.91cm), numbering: (..args) => {
let ind = args.pos().at(0)
if ind > 1 {
"第" + str(args.pos().at(0) - 1) + "页"
}
})
// 封面部分开始
set text(stroke: 0.03em, size: 三号)
set align(center)
set box(stroke: (bottom: 1pt), inset: (bottom: 20%, left: -10%, right: -10%))
v(1fr)
text("普通物理学实验Ⅱ\n电子实验报告\n ", size: 小初)
v(1fr)
[#box("实验名称:", stroke: none) #box(name, width: 8cm)]
v(1cm)
[#box("指导教师:", stroke: none) #box(instructor, width: 8cm)]
set text(size: 四号)
v(.9fr)
[#box("班级:", stroke: none) #box(class, width: 9.4cm)]
v(.1cm)
[#box("姓名:", stroke: none) #box(author, width: 9.4cm)]
v(.1cm)
[#box("学号:", stroke: none) #box(author-id, width: 9.4cm)]
v(0.5fr)
[#box("实验日期:", stroke: none) #box(str(date.year()), width: 3em) #box("年", stroke: none) #box(str(date.month()), width: 1.5em) #box("月", stroke: none) #box(str(date.day()), width: 1.5em) #box("日", stroke: none) #h(1cm) #box("星期", stroke: none) #box("一二三四五六日".clusters().at(date.weekday() - 1), width: 1em) #box(am-pm, stroke: none, width: 2em)]
v(0.5fr)
set text(stroke: none)
align(center, text("浙江大学物理实验教学中心", size: 四号))
set align(left)
set text(size: 小四)
v(1fr)
// 封面部分结束
pagebreak()
body
}
|
|
https://github.com/jgm/typst-hs | https://raw.githubusercontent.com/jgm/typst-hs/main/test/typ/text/edge-01.typ | typst | Other | // Error: 21-23 expected "ascender", "cap-height", "x-height", "baseline", "descender", or length, found array
#set text(top-edge: ())
|
https://github.com/Myriad-Dreamin/typst.ts | https://raw.githubusercontent.com/Myriad-Dreamin/typst.ts/main/crates/conversion/typst2vec/README.md | markdown | Apache License 2.0 | # reflexo-typst2vec
Convert typst items to vector format.
The vector format is abstracted from SVG and doesn't depend on typst.
- See [Typst.ts](https://github.com/Myriad-Dreamin/typst.ts)
- See [Vector Format](https://github.com/Myriad-Dreamin/typst.ts/blob/main/docs/proposals/8-vector-representation-for-rendering.typ)
|
https://github.com/TypstApp-team/typst | https://raw.githubusercontent.com/TypstApp-team/typst/master/tests/typ/visualize/gradient-text-decorations.typ | typst | Apache License 2.0 | // Tests gradients on text decorations.
---
#set text(fill: gradient.linear(red, blue))
Hello #underline[World]! \
Hello #overline[World]! \
Hello #strike[World]! \
|
https://github.com/Le-foucheur/dotfile | https://raw.githubusercontent.com/Le-foucheur/dotfile/main/.config/Code/User/History/4f8ef55/0UjW.typ | typst | #import "/template.typ": *
#import "/transposition.typ": *
#import "@preview/drafting:0.2.0": *
#import "@preview/vartable:0.1.0": *
#show: template
<NAME>
#align(center, text(20pt)[Maths : ES 1])
#set-page-properties()
= Groupe \
\
=== \
Comme $Q$ est non commutatif, alors $Q$ est nécessairement non monogène
=== \
$soietn( x , Q ) tq x !=e != e’$,\
On sait que $o(x) divides card Q = 8$\
Ainsi $x = 1 ou x = 2 ou x = 4 ou x = 8$\
Or comme $Q$ n'est pas monogène $x != 8$\
Si $o(x) = 1$, alors $x = x ^ 1 = e$. Absurde, donc $x != 1$\
Et par unicité de $e’$, $o(x) != 2$\
#undermath[Ainsi $o(x) = 4$]
#QED
=== #h(-5.4mm) a.\
Comme $a in Q et e’ in Q$, alors comme $Q$ est un groupe, alors $e’a in Q$,\
Or prouvons par contraposé que $a != e et a != e’$, Ainsi prouvons que $a = e ou a= e’ => a = e’ a e’$\
- supposons que $a = e$, alors :
$ e’ a e’ = (e’)^2 = e = a $
- supposons que $a = e’$, alors :
$ e’ a e’ = (e’)^2 e’ = e’ = a $
Donc par contraposé et par hypotèse de l'exercice, $a != e et a != e’$ \
Et donc comme $a != e donc e’a != e’ et$ comme $a != e’ donc e’a != (e’)^2 = e $\
et donc d'après la question précédente #undermath[$o(e’a) = 4$]
#QED
==== \
$ (a’a)^2 = (e’a e’a)^2 = (e’a)^4 = e $
#undermath[Donc $o(a’a) = 2 ou o(a’a) = 1$]
#QED
==== \
- Si $o(a’a) = 1$, alors
$
a’a = e &donc e’a e’a donc (e’a)^2 = e\
&donc o(e’a) = 2 donc e’a = e’ "(par unicité)"
&donc a = e "Absurde !!"
$
#undermath[Donc $o(a’a) != 1$]
#pagebreak()
- Si $o(a’a) = 2$, alors par unicité de $e’$
$
a’a = e’ &donc e’a e’a = e’ \
&donc a e’ a = e donc cases(a e’ = a^(-1), e’a = a^(-1)) \
&donc a e’ = e’a donc a = e’ a e’ = a’ "Absurde !!"
$
#undermath[Donc $o(a’a) != 2$]\
\
Ce qui est en contradiction avec la sous-question précédente, et donc Absurde !!\
#undermath[Donc $exists.not a in Q, a != e’a e’ <==> forall a in Q, a = e’ a e’$]\
Ce qui reviens a dire que $e’$ commute avec tout éléments de $Q$
#QED
=== #h(-5.4mm) a.\
#let ep = $e’$
Soit $frak(Q) = {e,j,k,l,e’,j e’, k e’,l e’}$\
Comme $card Q = card frak(Q)$, alors il suffis de montrer que un des deux ensemble contient le second\
On a que les éléments $e, j, k et e’ in Q$, et comme $Q$ est un groupe\
alors les éléments $l = j k, j ep, k ep et l ep in Q$\
#undermath[Ainsi $Q = frak(Q)$]
#QED
==== \
Soit $x in {j, k, l}$, comme $o(j) = o(k) = o(l) = 4$, alors $o(x) = 4$\
donc $x^4 = e donc (x^2)^2 = e donc o(x^2) = 2$, par unicité de $ep$, $x^2 = ep$\
#undermath[Donc $j^2 = k^2 = l^2 = ep$]
#QED
#grid(
columns: 2,
column-gutter: 1cm,
table(
align: center + horizon,
columns: 9,
$*$,$e$,$j$,$k$,$l$,$ep$,$j ep$,$k ep$,$l ep$,
$e$,$e$,$j$,$k$,$l$,$ep$,$j ep$,$k ep$,$l ep$,
$j$,$j$,$ep$,$l$,$k ep$,$j ep$,$e$,$l ep$,$k$,
$k$,$k$,$l ep$,$ep$,$j$,$k ep$,$l$,$e$,$j ep$,
$l$,$l$,$$,$j ep$,$ep$,$l ep$,$$,$j$,$e$,
$ep$,$ep$,$j ep$,$k ep$,$l ep$,$e$,$j$,$k$,$l$,
$j ep$,$j ep$,$e$,$l ep$,$k$,$j$,$ep$,$l$,$k ep$,
$k ep$,$k ep$,$l$,$e$,$j ep$,$k$,$l ep$,$ep$,$j$,
$l ep$,$l ep$,$$,$j$,$e$,$l$,$$,$j ep$,$ep$,
),
[
- $l k j = j k k j = (ep)^2 = e$ ($i$)\
- $(ep)^2 = e <==> ep = (ep)^(-1)$
- par ($i$) : $l^2 k j = k j ep = l <=> k j = l ep$
- $j k = l <=> j k l = ep <=> k l ep = j ep <=> k l = j $
]
) |
|
https://github.com/jbro/supernote-templates | https://raw.githubusercontent.com/jbro/supernote-templates/main/include/elements.typ | typst | The Unlicense |
#let titled-box(contents, title: "title", text-size: 12pt,) = box(stroke: 2pt + gray, inset: 6pt, radius: 5pt, width: 100%,
[
#v(2pt)
#text(size: text-size, [#place(top+left, [#v(-1.2em) #box(fill: white, inset: 1pt, title)])])
#v(2pt)
#contents
]
)
#let task-lines(count) = {
let line = (mark: rotate(30deg, text(fill: gray, "")), line: [#line(start: (0em, 1em), length: 100%)])
[
#grid(columns: (1em, 1fr),
..for _ in range(1, count) {
(line.mark, line.line, v(1em), "")
},
[#line.mark], [#line.line],
)
#v(1pt)
]
}
#let note-lines(lines) = block(
[
#set text(size: 10pt)
#v(0.5em)
#for _ in range(lines) {
line(start: (0em, 1em), stroke: (paint: black, dash: "dotted"), length: 99%)
}
#v(1pt)
]
)
#let week-box() ={
let mark = box(text(fill: gray, rotate(30deg, "")))
titled-box(title: "Week",
[
#set align(right)
#v(-2pt)
#grid(columns: 2,
[
#set text(size: 24pt, fill: gray)
#set align(center+horizon)
#h(16pt)
],
[
#set text(size: 8pt)
#set align(left)
#mark mon #mark tue #mark wed\
#mark thu #mark fri
],
)
]
)
}
|
https://github.com/RaphGL/ElectronicsFromBasics | https://raw.githubusercontent.com/RaphGL/ElectronicsFromBasics/main/DC/chap3/chap3.typ | typst | Other | == Electrical Safety
#include "1_importance_of_electrical_safety.typ"
#include "2_physiological_effects_of_electricity.typ"
#include "3_shock_current_path.typ"
#include "4_ohms_law_again.typ"
#include "5_safe_practices.typ"
#include "6_emergency_response.typ"
#include "7_common_hazards.typ"
#include "8_safe_circuit_design.typ"
#include "9_safe_meter_usage.typ"
#include "10_electric_shock_data.typ"
|
https://github.com/0x1B05/english | https://raw.githubusercontent.com/0x1B05/english/main/cnn10/content/20240521.typ | typst | #import "../template.typ": *
#pagebreak()
= 20240521
== Start
What's up, everybody? It's your boy Coy here. Time to #strike[a few hours ...]#underline[*fuel* our minds] this terrific Tuesday, but man, what a #underline[*bittersweet*] week! Summertime #underline[is almost _upon us_, and that is awesome] But it's also the last week of the show before summer break. Definitely you have some #strike[plans]#underline[some things planned] for #underline[us over] the summer though. Some more #underline[on that] to come.
== Iran's president death
All right, let's get #underline[to] your news. In Iran, the country's army chief is ordering an *investigation into* a deadly helicopter crash that has shaken the nation. Iran's president, <NAME>, and several other officials were killed after their helicopter crashed in #strike[the]#underline[a] remote mountain region in northwestern part of the country. There was #strike[a] heavy fog in the area at the time of crash. Raisi was #strike[the]#underline[a] political #underline[*hard-liner*]. He was *voted into power* in 2021, #underline[easily] beating the competition, the former president, #strike[has signed]#underline[<NAME>], who was more #underline[of a *moderate*]. Raisi *earned* 18 million of nearly 29 million votes that were *cast*. Iran's considered #strike[in his .. public]#underline[an *Islamic* republic] or #underline[a *theocracy*], meaning that the religious figure runs the country. In #strike[the runs]#underline[Iran's] case, that religious leader is #underline[*supreme* leader, <NAME> an Islamic] *fundamentalist*. Raisi, as president, was *second in command*. His death comes at #strike[the]#underline[a] tense moment for the entire Middle #strike[list]#underline[East]. #strike[There]#underline[It] was only weeks ago that Iran#strike['s] launched a #underline[full-scale drone] a #strike[mis-attack]#underline[missile attack] #strike[in]#underline[on] Israel. That was retaliation, they said, for #strike[the]#underline[an] #strike[Israel]#underline[Israeli] strike #strike[for ..]#underline[on a *diplomatic*] complex in Syria that killed several Iranian officials. CNN's Ivan Watson shows how the people #underline[of Iran are] reacting and what's next for the country.
Iran in the state of mourning, #underline[*commemorating*] the shocking death of Iranian president <NAME>. He was killed along with #strike[four]#underline[the Foreign] Minister and seven other officials #strike[in ..]#underline[and crew] members. When their helicopter crashed in remote mountains Sunday in the northwestern of the country. During the *frantic* hours#strike[, the]#underline[when] rescuers were searching for the missing president, the most powerful figure in the Iranian political system, Supreme Leader <NAME> declared the government#strike['s] stable and strong.
Inside Iran, highly #underline[*polarized*] reactions to the sudden death of a leader. According the Iranian *constitution*, the little-known #strike[advice]#underline[vice] president, <NAME>, has now become #strike[in term]#underline[*interim*] president, #strike[heading]#underline[paving] the way for #underline[elections] to be #underline[held] #underline[with]in 50 days.
Messages of #underline[*condolence* are pouring in] from *longtime* #strike[ally]#underline[allies], like Syria, as well as Russia, with launches Iranian Shahed #underline[*drones*] against the cities in Ukraine.
Also #strike[public ... is lost]#underline[*publicly* mourning Raisi's loss], Iranian#underline[-backed militant] group#underline[s] such as Yemen's Houthis, Hamas and Lebanon's Hezbollah. Meanwhile, _few tears likely to be #strike[shared]#underline[*shed*]_ #strike[like] Iran's #underline[*sworn*] enemy Israel. The two countries' #underline[long-*simmering* shadow war] exploded into direct, #underline[tit-for-tat, long-range strikes just last month.]
How #strike[Iran's]#underline[the Islamic] Republic deals with this deadly crash may #strike[sudden]#underline[set the] stage for #strike[the]#underline[a] much bigger future challenge, the question of succession for ..., the country's 85-year-old supreme leader.
=== words, phrases and sentences
==== words
- _bittersweet_
- _hard-liner_
- _cast_
- _theocracy_
- _fundamentalist_
- _diplomatic_
- _commemorate_
- _frantic_
- _polarized_
- _constitution_
- _interim_
- _condolence_
- _publicly_
- _simmer_
==== phrases
- _fuel our minds_
- _be voted into power_
==== sentences
- _Summertime is almost upon us._
- _Few tears likely to be shed Iran's sworn enemy Israel._
=== 回译
==== 原文
What's up, everybody? It's your boy Coy here. Time to fuel our minds this terrific Tuesday, but man, what a bittersweet week! Summertime is almost upon us, and that is awesome But it's also the last week of the show before summer break. Definitely you have some some things planned for us over the summer though. Some more on that to come.
All right, let's get to your news. In Iran, the country's army chief is ordering an investigation into a deadly helicopter crash that has shaken the nation. Iran's president, <NAME>, and several other officials were killed after their helicopter crashed in a remote mountain region in northwestern part of the country. There was heavy fog in the area at the time of crash. Raisi was a political hard-liner. He was voted into power in 2021, easily beating the competition, the former president, <NAME>, who was more of a moderate. Raisi earned 18 million of nearly 29 million votes that were cast. Iran's considered an Islamic republic or a theocracy, meaning that the religious figure runs the country. In Iran's case, that religious leader is supreme leader, <NAME> an Islamic fundamentalist. Raisi, as president, was second in command. His death comes at a tense moment for the entire Middle East. It was only weeks ago that Iran launched a full-scale drone a missile attack on Israel. That was retaliation, they said, for an Israeli strike on a diplomatic complex in Syria that killed several Iranian officials. CNN's Ivan Watson shows how the people of Iran are reacting and what's next for the country.
Iran in the state of mourning, commemorating the shocking death of Iranian president <NAME>. He was killed along with the Foreign Minister and seven other officials and crew members. When their helicopter crashed in remote mountains Sunday in the northwestern of the country. During the frantic hourswhen rescuers were searching for the missing president, the most powerful figure in the Iranian political system, Supreme Leader <NAME> declared the government stable and strong.
Inside Iran, highly polarized reactions to the sudden death of a leader. According the Iranian constitution, the little-known vice president, <NAME>, has now become interim president, paving the way for elections to be held within 50 days.
Messages of condolence are pouring in from longtime allies, like Syria, as well as Russia, with launches Iranian Shahed drones against the cities in Ukraine.
Also publicly mourning Raisi's loss, Iranian-backed militant groups such as Yemen's Houthis, Hamas and Lebanon's Hezbollah. Meanwhile, few tears likely to be shed Iran's sworn enemy Israel. The two countries' long-simmering shadow war exploded into direct, tit-for-tat, long-range strikes just last month.
How the Islamic Republic deals with this deadly crash may set the stage for a much bigger future challenge, the question of succession for..., the country's 85-year-old supreme leader.
==== 参考翻译
大家好!Coy在这里,带着我们一起度过美好的星期二。不过,这也是让人百感交集的一周!夏天即将来临,这很棒,但这也是夏季休假前的最后一周节目。不过,我们在夏天还有一些计划,后续会有更多信息。
好了,让我们来看今天的新闻。在伊朗,军队总司令下令调查一起致命的直升机坠毁事件,这起事故震惊了全国。伊朗总统Ebrahim Raisi及其他几位官员在他们的直升机坠毁于该国西北部的偏远山区后遇难。事故发生时,现场有浓雾。Raisi是一个政治强硬派,2021年他轻松击败竞争对手、前总统Hassan Rouhani当选,总共获得了近2900万张选票中的1800万票。伊朗被视为一个伊斯兰共和国或神权国家,宗教领袖掌控国家。在伊朗,这位宗教领袖是最高领袖Ayatollah <NAME>i,一名伊斯兰原教旨主义者。作为总统的Raisi是国家的二号人物。他的去世正值整个中东地区紧张之际。几周前,伊朗对以色列发动了大规模无人机和导弹袭击,称这是对以色列在叙利亚的一个外交设施的袭击的报复,那次袭击造成几名伊朗官员死亡。CNN的<NAME>将向大家展示伊朗人民的反应以及该国的下一步举措。
伊朗正处于哀悼状态,纪念伊朗总统<NAME>的意外去世。他与外交部长及其他七名官员和机组成员在直升机周日在该国西北部偏远山区坠毁时丧生。在搜救人员紧张寻找失踪总统的几小时内,伊朗政治体系中最有权力的人物、最高领袖Ayatollah Khamenei宣布政府稳定且强大。
在伊朗国内,对领导人突然去世的反应高度两极化。根据伊朗宪法,鲜为人知的副总统<NAME>现已成为临时总统,为在50天内举行选举铺平了道路。
来自长期盟友如叙利亚以及俄罗斯的慰问信息纷纷涌入,俄罗斯对乌克兰城市发射伊朗Shahed无人机。同样公开哀悼Raisi去世的还有伊朗支持的武装组织,如也门的胡塞武装、哈马斯和黎巴嫩的真主党。与此同时,伊朗的宿敌以色列可能不会流太多眼泪。两国长期以来的影子战争上个月爆发为直接的针锋相对的远程打击。
伊朗如何处理这起致命的坠机事件可能会为未来的一个更大挑战奠定基础,那就是85岁的最高领袖的继任问题。
==== 1st
|
|
https://github.com/tingerrr/anti-matter | https://raw.githubusercontent.com/tingerrr/anti-matter/main/docs/manual.typ | typst | MIT License | #import "@preview/tidy:0.1.0"
#import "template.typ": project
#let package = toml("/typst.toml").package
#show: project.with(
package: package,
date: datetime.today().display(),
abstract: [
This packages automatically numbers the front and back matter of your document separately
from the main content. This is commonly used for books and theses.
]
)
#let codeblocks(body) = {
show raw.where(block: true): set block(
width: 100%,
fill: gray.lighten(50%),
radius: 5pt,
inset: 5pt
)
show "{{version}}": package.version
body
}
#show heading.where(level: 1): it => pagebreak(weak: true) + it
#outline(
indent: auto,
target: heading.where(outlined: true).before(<api-ref>, inclusive: true),
)
= Introduction
#[
#show: codeblocks
A document like this:
#raw(
block: true,
lang: "typst",
read("/example/main.typ").replace(
regex("/src/lib.typ"),
"@preview/anti-matter:{{version}}",
),
)
Would generate an outline like this:
#block(fill: gray.lighten(50%), radius: 5pt, inset: 5pt, image("/example/example.png"))
The front matter (in this case the outlines) are numbered using `"I"`, the content starts new at
`"1"` and the back matter (glossary, acknowledgement, etc) are numbered `"I"` again, continuing
from where the front matter left off.
]
= How it works & caveats
#[
#show: codeblocks
`anti-matter` keeps track of its own inner and outer counter, which are updated in the header
of a page. Numbering at a given location is resolved by inspecting where this location is
between the given fences and applying the expected numbering to it. Both `page.header` and
`outline.entry` need some special care if you wish to configure them. While `page.header` can
simply be set in `anti-matter`, if you want to set it somewhere else you need to ensure that the
counters are stepped. Likewise `outline.entry` or anything that displays page numbers for
elements needs to get the page number from `anti-matter`.
== Numbering
Numbering is done as usual, with a string or function, or `none`. If the numbering is set to
`none` then the counter is not stepped. Patterns and functions receive the current and total
value. Which means that `"1 / 1"` will display `"3 / 5"` on the third out of five pages. Because
`none` skips stepping it can be used to easily add a title page beforehand, without having to
reset the page counter.
```typst
#import "@preview/anti-matter:{{version}}": anti-matter, fence, set-numbering
#show: anti-matter(numbering: ("I", numbering.with("1 / 1"), none))
#set-numbering(none)
#align(center + horizon)[Title]
#pagebreak()
#set-numbering("I")
// page numbering starts at "I"
// ...
```
== Fences
For `anti-matter` to know in which part of the document it is, it needs exactly 2 fences, these
must be placed on the last page of the front matter and the last page of the main content. Make
sure to put them before your page breaks, otherwise they'll be pushed onto the next page. Fences
are placed with `fence()`.
```typst
#import "@preview/anti-matter:{{version}}": anti-matter, fence
#show: anti-matter
// front matter
#lorem(1000)
#fence()
// content
#lorem(1000)
#fence()
// back matter
#lorem(1000)
```
== Page header
`anti-matter` uses the page header to step its own counters. If you want to adjust the page
header sometime after the `anti-matter` show rule, you have to add `step()` before it.
```typst
#import "@preview/hydra:0.2.0": hydra
#import "@preview/anti-matter:{{version}}": anti-matter, step
#show: anti-matter
// ...
#set page(header: step() + hydra())
// ...
```
== Outline entries and querying
By default `outline` will use the regular page counter to resolve the page number. If you want
to configure the appearance of `outline` but still get the correct page numbers use
`page-number` with the element location.
```typst
#import "@preview/anti-matter:{{version}}": anti-matter, page-number
#show: anti-matter
// render your own outline style while retaining the correct page numbering for queried elements
#show outline.entry: it => {
it.body
box(width: 1fr, it.fill)
page-number(loc: it.element.location())
}
// ...
```
The same logic applies to other things where elemnts are queried and display their page number.
]
= API-Reference <api-ref>
#let mods = (
(`anti-matter`, "/src/lib.typ", [
The public and stable library API intended for regular use.
]),
(`core`, "/src/core.typ", [
The core API, used for querying internal state, public, but not stable.
]),
(`rules`, "/src/rules.typ", [
Show and set rules which are applied in `anti-matter`, provides default versions to turn of
rule.
]),
)
#for (title, path, descr) in mods [
== #title
#descr
#tidy.show-module(tidy.parse-module(read(path)), style: tidy.styles.default)
#pagebreak(weak: true)
]
|
https://github.com/duskmoon314/THU_AMA | https://raw.githubusercontent.com/duskmoon314/THU_AMA/main/docs/ch2/4-群在集合上的作用.typ | typst | Creative Commons Attribution 4.0 International | #import "/book.typ": *
#show: thmrules
#show: book-page.with(title: "群在集合上的作用")
= 群在集合上的作用
== 群对集合的作用
#example()[
设$X = { 1 , 2 , dots.h.c , n } , S_n$对$X$可产生作用$lr((G lt.eq S_n))$:$forall g in G , forall x in X , g lr((x))$为$g$在$X$上的作用
]
#definition()[
设$G$为群,$Omega$是一个集合,若$forall g in G$对应$Omega$上对一个变换$g lr((x))$使得$forall x in Omega$均有
+ $e lr((x)) = x$ (#strong[单位变换])
+ $lr((g_1 g_2)) lr((x)) = g_1 lr((g_2 lr((x))))$ (#strong[运算相容])
则称$G$在$Omega$上定义了一个#strong[群作用],$Omega$称为一个#strong[$G$-集合]
]
#remark()[
+ $Omega$可为有限集,也可为无限集
+ 由$g$作为群元素是可逆的,$g$作为变换必为可逆变换(双射)
+ 等价定义$G arrow.r^sigma { Omega upright("的变换群(置换群)") }$的一个同态
#definition("等价定义")[
若存在$G times Omega arrow.r Omega , lr((g , x)) arrow.r.bar g lr((x))$使得
+ $e lr((x)) = x$
+ $lr((g_1 g_2)) lr((x)) = g_1 lr((g_2 lr((x))))$
则称$G$在$Omega$上定义了一个群作用,$Omega$称为一个$G$-集合
]
]
#example()[
+ 左乘作用:设$Omega = G$,定义$G$对$Omega = G$的作用$g lr((x)) eq.delta g x$
验证这是群作用:$e lr((x)) = e x = x , lr((g_1 g_2)) lr((x)) = g_1 g_2 x = g_1 lr((g_2 lr((x))))$
右乘:$g lr((x)) eq.delta x g$,则$lr((g_1 g_2)) lr((x)) = x g_1 g_2 eq.not g_1 lr((g_2 lr((x)))) = g_1 lr((x g_2)) = x g_2 g_1$,故不是群作用
右乘逆 $g lr((x)) eq.delta x g^(- 1)$ 为群作用
+ 共轭作用:设$Omega = G$,定义$G$对$Omega = G$的作用$g lr((x)) eq.delta g x g^(- 1) , forall g , x in G$
验证:$e lr((x)) = e x e^(- 1) = x , g_1 g_2 lr((x)) = lr((g_1 g_2)) x lr((g_1 g_2))^(- 1) = g_1 g_2 x g_2^(- 1) g_1^(- 1) = g_1 lr((g_2 lr((x))))$
+ 子群共轭作用:$Omega = cal(A) = { H \| H lt.eq G } , g lr((H)) eq.delta g H g^(- 1)$
+ 陪集左乘作用:$Omega = lr((G \/ H))_L , g lr((a H)) eq.delta lr((g a)) H$
+ 陪集右乘作用:$Omega = lr((G \/ H))_R , g lr((H a)) eq.delta H lr((a g^(- 1)))$
]
== 轨道与稳定子群
#definition()[
设$Omega$为一个$G$-集合,取定$x in Omega$,则$Omega_x := { g lr((x)) \| g in G }$称为$x$在$G$作用下的一个#strong[轨道(orbit)],$x$称为此轨道的一个代表元
]
#property()[
+ 同轨道的关系是$Omega$中的等价关系
自反性 $x = e lr((x))$, 对称性 $y = g lr((x)) arrow.r.double x = g^(- 1) lr((x))$, 传递性 $y = g lr((x)) , z = g_1 lr((y)) arrow.r.double z = g_1 lr((g lr((x)))) = g_1 g lr((x))$
+ 等价类就是轨道$Omega_x$,对集合$Omega$产生划分$Omega = union.sq.big_(x in Omega) Omega_x arrow.r.double lr(|Omega|) = sum_(x in Omega) lr(|Omega_x|)$
+ $y in Omega_x arrow.l.r.double Omega_x = Omega_y$ (代表元公平)要么$Omega_x sect Omega_y = nothing$,要么$Omega_x = Omega_y$
]
#definition()[
设$Omega$为一个$G$-集合,$g in G , x in Omega$,若$g lr((x)) = x$,则称$x$是$g$的一个#strong[不动点(fix point)]。而集合 $ upright("stab")_G x = G_x := { g in G \| g lr((x)) = x } subset G $ 称为$x$的#strong[稳定子群(稳定化子, stabilizer)]
]
#property()[
$G_x lt.eq G$
]
#proof()[
$e in G_x$,故$G_x eq.not nothing$。
$forall g_1 , g_2 in G_x$,有$g_1 lr((x)) = x = g_2 lr((x)) , g_1^(- 1) lr((x)) = g_1^(- 1) lr((g_1 lr((x)))) = lr((g_1^(- 1) g_1)) lr((x)) = e lr((x)) = x arrow.r.double g_1^(- 1) in G_x$
$g_1 g_2 lr((x)) = g_1 lr((g_2 lr((x)))) = g_1 lr((x)) = x arrow.r.double g_1 g_2 in G_x$
故$G_x lt.eq G$
]
#example()[
+ 左乘作用:$Omega = G , g lr((x)) = g x , Omega_x = { g lr((x)) |g in G } = { g x| g in G } = G = Omega$,只有一个轨道,称为可迁作用。$G_x = { g in G \| g lr((x)) = g x = x } = { e }$ (且此时 $lr(|Omega_x|) lr(|G_x|) = lr(|G|)$)
+ 右乘作用:略
+ 共轭作用:$Omega = G , g lr((x)) = g x g^(- 1) , Omega_x = { g x g^(- 1)
|g in G } = K_x,
G_x = { g in G| g x g^(- 1) = x } = { g in G \| g x = x g } = C_G lr((x))$ (且此时 $lr(|K_x|) = lr([G : C lr((x))]) = lr(|G|) \/ lr(|C lr((x))|) arrow.r.double lr(|Omega_x|) lr(|G_x|) = lr(|G|)$)
+ 子群共轭作用:$Omega = cal(A) , g lr((H)) = g H g^(- 1) , Omega_H = { g H g^(- 1)
|g in G } = K_H,
G_H = { g in G| g H g^(- 1) = H } = { g in G \| g H = H g } = N_G lr((H))$ (且此时 $lr(|K_H|) = lr([G : N lr((H))]) = lr(|G|) \/ lr(|N lr((H))|) arrow.r.double lr(|Omega_H|) lr(|G_H|) = lr(|G|)$)
+ 陪集作用:$Omega = lr((G \/ H))_L , g lr((a H)) = lr((g a)) H , Omega_H = { g lr((H)) = g H |g in G } = lr((G \/ H))_L, G_H = { g in G| g lr((H)) = H } = H$ (且此时 $lr(|lr((G \/ H))_L|) = lr([G : H]) = lr(|G|) \/ lr(|H|) arrow.r.double lr(|Omega_H|) lr(|G_H|) = lr(|G|)$)
]
#property()[
+ 轨道公式$|Omega_x| = [G: "stab" x]$
+ $|G| = |Omega_x| |"stab"x|, |Omega| = sum_{x in Omega}[G: "stab" x]$(代表元求和)
+ $"stab" g(x) = g("stab" x)g^(-1)$ (同一轨道上元素的稳定子群互相共轭)
]
#proof()[
+ 作对应关系 $Omega_x arrow.r lr((G \/ G_x))_L , g lr((x)) arrow.r.bar g G_x$,验证映射、单射、满射
+ 由1和Lagrange定理及$Omega$单轨道划分可得
+ $forall h in G_(g lr((x))) arrow.r.double h lr((g lr((x)))) = g lr((x)) arrow.r.double g^(- 1) h g lr((x)) = x arrow.r.double g^(- 1) h g in G_x arrow.r.double h in g G_x g^(- 1) arrow.r.double$ 左 $subset.eq$ 右
反之,$forall tilde(h) in g G_x g^(- 1) arrow.r.double g^(- 1) tilde(h) g in G_x arrow.r.double g^(- 1) tilde(h) g lr((x)) = x arrow.r.double tilde(h) lr((g lr((x)))) = g lr((x)) arrow.r.double tilde(h) in G_(g lr((x))) arrow.r.double$ 右 $subset.eq$ 左
综上,$g G_x g^(- 1) = G_(g lr((x)))$
]
#example()[
确定正四面体的旋转群(旋转后重合)
]
#solution()[
取定定点A,保持其不动的旋转有3个,即$lr(|G_A|) = 3$
总存在旋转将A转到其他顶点,即$lr(|Omega_A|) = 4$
$lr(|G|) = lr(|Omega_A|) dot.op lr(|G_A|) = 12 , G lt.eq S_4$
对应 $lr((1)) , lr((123)) , lr((132)) , lr((124)) , lr((142)) , lr((134)) , lr((143)) , lr((234)) , lr((243)) , lr((12)) lr((34)) , lr((13)) lr((24)) , lr((14)) lr((23))$
$G = A_4 lt.tri.eq S_4$
]
== Burnside 引理
一般的群作用会用多少个轨道?
#theorem("Burnside 引理")[
设有限群$G$作用在有限集$Omega$上,记$N$为$Omega$在$G$的作用下的轨道数,$chi lr((g))$表示群元素$g$在$Omega$中的不动点个数,则有$N = 1 / lr(|G|) sum_(g in G) chi lr((g))$
]
$G_x = {g in G |g lr((x)) = x}, chi lr((g)) = \# {x in Omega| g lr((x)) = x}$
#proof()[
设$Omega = { a_1 , dots.h.c , a_n } , G = { g_1 , dots.h.c , g_m }$
令$E_(i j) = D lr((g_i , g_j)) eq.delta cases(delim: "{", 1 & g_i lr((a_j)) = a_j, 0 & g_i lr((a_j)) eq.not a_j)$
$K = lr((E_(i j)))_(1 lt.eq i lt.eq m , 1 lt.eq j lt.eq n) in M_(m times n) {0 , 1}$
$chi lr((g_i))$为矩阵$K$的第$i$行的行和,$\# G_(a_j)$为第$j$列的列和
因而$sum_(a in Omega) lr(|G_a|) = sum_(g in G) chi lr((g))$
设$Omega$的全体轨道为$Omega^(lr((1))) , dots.h.c , Omega^(lr((N)))$,上式左侧化为$sum_(k = 1)^N sum_(a in Omega^(lr((k)))) lr(|G_a|) = sum_(k = 1)^N lr(|Omega^(lr((k)))|) lr(|G_a|) = sum_(k = 1)^N lr(|G|)$
] |
https://github.com/Student-Smart-Printing-Service-HCMUT/ssps-docs | https://raw.githubusercontent.com/Student-Smart-Printing-Service-HCMUT/ssps-docs/main/contents/categories/task4/4.conclude.typ | typst | Apache License 2.0 | #{include "./4.1.typ"}
#{include "./4.2.typ"}
#{include "./4.3.typ"} |
https://github.com/LDemetrios/Typst4k | https://raw.githubusercontent.com/LDemetrios/Typst4k/master/src/test/resources/suite/layout/flow/footnote.typ | typst | // Test footnotes.
--- footnote-basic ---
#footnote[Hi]
--- footnote-space-collapsing ---
// Test space collapsing before footnote.
A#footnote[A] \
A #footnote[A]
--- footnote-nested ---
// Currently, numbers a bit out of order if a nested footnote ends up in the
// same frame as another one. :(
First \
Second #footnote[A, #footnote[B, #footnote[C]]]
Third #footnote[D, #footnote[E]] \
Fourth #footnote[F]
--- footnote-entry ---
// Test customization.
#show footnote: set text(red)
#show footnote.entry: set text(8pt, style: "italic")
#set footnote.entry(
indent: 0pt,
gap: 0.6em,
clearance: 0.3em,
separator: repeat[.],
)
Beautiful footnotes. #footnote[Wonderful, aren't they?]
--- footnote-break-across-pages ---
#set page(height: 200pt)
#lines(2)
#footnote[ // 1
I
#footnote[II ...] // 2
]
#lines(6)
#footnote[III: #lines(8, "1")] // 3
#lines(6)
#footnote[IV: #lines(15, "1")] // 4
#lines(6)
#footnote[V] // 5
--- footnote-break-across-pages-block ---
#set page(height: 100pt)
#block[
#lines(3) #footnote(lines(6, "1"))
#footnote[Y]
#footnote[Z]
]
--- footnote-break-across-pages-float ---
#set page(height: 180pt)
#lines(5)
#place(
bottom,
float: true,
rect(height: 50pt, width: 100%, {
footnote(lines(6, "1"))
footnote(lines(2, "I"))
})
)
#lines(5)
--- footnote-break-across-pages-nested ---
#set page(height: 120pt)
#block[
#lines(4)
#footnote[
#lines(6, "1")
#footnote(lines(3, "I"))
]
]
--- footnote-in-columns ---
#set page(height: 120pt, columns: 2)
#place(
top + center,
float: true,
scope: "parent",
clearance: 12pt,
strong[Title],
)
#lines(3)
#footnote(lines(4, "1"))
#lines(2)
#footnote(lines(2, "1"))
--- footnote-in-list ---
#set page(height: 120pt)
- A #footnote[a]
- B #footnote[b]
- C #footnote[c]
- D #footnote[d]
- E #footnote[e]
- F #footnote[f]
- G #footnote[g]
--- footnote-block-at-end ---
#set page(height: 50pt)
A
#block(footnote[hello])
--- footnote-float-priority ---
#set page(height: 100pt)
#lines(3)
#place(
top,
float: true,
rect(height: 40pt)
)
#block[
V
#footnote[1]
#footnote[2]
#footnote[3]
#footnote[4]
]
#lines(5)
--- footnote-in-caption ---
// Test footnote in caption.
Read the docs #footnote[https://typst.app/docs]!
#figure(
image("/assets/images/graph.png", width: 70%),
caption: [
A graph #footnote[A _graph_ is a structure with nodes and edges.]
]
)
More #footnote[just for ...] footnotes #footnote[... testing. :)]
--- footnote-in-place ---
A
#place(top + right, footnote[A])
#figure(
placement: bottom,
caption: footnote[B],
rect(),
)
--- footnote-duplicate ---
// Test duplicate footnotes.
#let lang = footnote[Languages.]
#let nums = footnote[Numbers.]
/ "Hello": A word #lang
/ "123": A number #nums
- "Hello" #lang
- "123" #nums
+ "Hello" #lang
+ "123" #nums
#table(
columns: 2,
[Hello], [A word #lang],
[123], [A number #nums],
)
--- footnote-invariant ---
// Ensure that a footnote and the first line of its entry
// always end up on the same page.
#set page(height: 120pt)
#lines(5)
A #footnote(lines(6, "1"))
--- footnote-ref ---
// Test references to footnotes.
A footnote #footnote[Hi]<fn> \
A reference to it @fn
--- footnote-self-ref ---
// Error: 2-16 footnote cannot reference itself
#footnote(<fn>) <fn>
--- footnote-ref-multiple ---
// Multiple footnotes are refs
First #footnote[A]<fn1> \
Second #footnote[B]<fn2> \
First ref @fn1 \
Third #footnote[C] \
Fourth #footnote[D]<fn4> \
Fourth ref @fn4 \
Second ref @fn2 \
Second ref again @fn2
--- footnote-ref-forward ---
// Forward reference
Usage @fn \
Definition #footnote[Hi]<fn>
--- footnote-ref-in-footnote ---
// Footnote ref in footnote
#footnote[Reference to next @fn]
#footnote[Reference to myself @fn]<fn>
#footnote[Reference to previous @fn]
--- footnote-styling ---
// Styling
#show footnote: text.with(fill: red)
Real #footnote[...]<fn> \
Ref @fn
--- footnote-ref-call ---
// Footnote call with label
#footnote(<fn>)
#footnote[Hi]<fn>
#ref(<fn>)
#footnote(<fn>)
--- footnote-in-table ---
// Test footnotes in tables. When the table spans multiple pages, the footnotes
// will all be after the table, but it shouldn't create any empty pages.
#set page(height: 100pt)
= Tables
#table(
columns: 2,
[Hello footnote #footnote[This is a footnote.]],
[This is more text],
[This cell
#footnote[This footnote is not on the same page]
breaks over multiple pages.],
image("/assets/images/tiger.jpg"),
)
#table(
columns: 3,
..range(1, 10)
.map(numbering.with("a"))
.map(v => upper(v) + footnote(v))
)
--- footnote-multiple-in-one-line ---
#set page(height: 100pt)
#v(50pt)
A #footnote[a]
B #footnote[b]
--- issue-1433-footnote-in-list ---
// Test that footnotes in lists do not produce extraneous page breaks. The list
// layout itself does not currently react to the footnotes layout, weakening the
// "footnote and its entry are on the same page" invariant somewhat, but at
// least there shouldn't be extra page breaks.
#set page(height: 100pt)
#block(height: 50pt, width: 100%, fill: aqua)
- #footnote[1]
- #footnote[2]
--- issue-footnotes-skip-first-page ---
// In this issue, we would get an empty page at the beginning because footnote
// layout didn't properly check for in_last.
#set page(height: 50pt)
#footnote[A]
#footnote[B]
--- issue-4454-footnote-ref-numbering ---
// Test that footnote references are numbered correctly.
A #footnote(numbering: "*")[B]<fn>, C @fn, D @fn, E @fn.
|
|
https://github.com/HarryLuoo/sp24 | https://raw.githubusercontent.com/HarryLuoo/sp24/main/431/notes/part1.typ | typst |
#set math.equation(numbering:"(1)")
= Sample Spaces, collection of events, probability measure
- Sample space $Omega$: set of all possible outcomes of an experiment. Comes in n-tuples where n represents number of repeated trials.
- Collection of events $cal(F) $: subset of state space to which we assign a probability.
- Probability measure: function that assigns a probability to each event.$P: F -> RR$.
- Range is $[0,1]$.
- Axioms
- $P(Omega) = 1 "and" P(nothing)=0$
- For pairwise disjoint events $A_1, A_2, ...$, \ $P(A_1 union A_2 union ...) = P(A_1) + P(A_2) + ...$
#line(length:100%)
= Sampling
== Uniform sampling
If the sample space $Omega$ has finitely many elements and each outcome is equally likely, then for any event $A subset Omega$ we have
$
P(A) = (\# A)/(\# Omega)
$ where \# means the "cardinality" of the set.
- uniform sampling: each outcome is equally likely
- Binomial coeff $
binom(n,k) = n!/(k!(n-k)!)
$
== Sampling with Replacement, order matters
- ex: sample K distinct marked balls from N balls in a box, *with* Replacement
$
Omega = {1,2,3,...,N}^K\
||Omega|| = N^K\
P("none of the balls is marked 1")= (N-1)^K/N^K
$
- ex: sample K distinct marked balls from N balls in a box, *without* Replacement
$
Omega = {(i_1, i_2, ..., i_K) | i_1, ..., i_K in {1,2,...,N}, "distinct"\
||Omega|| = binom(N-1,K)\
P("none of the balls is marked 1")= binom(N-1,K)/binom(N,K)=(N-K)/N
$
== Order
- order matters: $A_n^k=(n!)/(n-k)!$
- order doesn't matter: $binom(n,k)=C_n^k=(n!)/(k!(n-k)!)$
#line(length:100%)
= Infinite Sample Spaces
== discrete <sec.discreteSampleSpace>
$ Omega = {infinity, 1, 2, ...} $
== continuous
$
P([a',b'])=("length of" [a',b'])/("length of"[a,b])\
"single point, or sets of points:" P({x})=P(union_(i=1)^infinity {x_i})=0 \
$
- Complements: $P(A)=1-P(A^C)$
#line(length:100%)
= Conditioinal Probability, Law of Total Prob., Bayes' Theorem, Independence
== Conditional prob.
$
P(A|B)=(|A sect B|)/(|B|) => P(A B)= P(B)P(A|B)
$ \ (new sample space is B, total number of outcomes is $A sect B$)
== Law of total probability:
Given partitions $
B_1, B_2, ...$ of $Omega$, $
P(A)=sum_i P(A|B_i)P(B_i)
$
== Bayes' Theorem:
Given events A, B, P(A) and P(B) >0,$
P(B_i|A)=(P(A|B_i)P(B_i))/P(A)\
$
Considering the law of total prob., the generalized form, when $B_i$ are partitions, is given as: $
P(B_i|A)=(P(A|B_i)P(B_i))/(sum_j P(A|B_j)P(B_j))
$
== Independence:
$
P(A B)=P(A)P(B) <=> P(B|A)=P(B)
$\
Note: By virtue of conventions, we write $A sect B$ as $A B$ in Probability.\
If A,B,C,D are independent, it follows that $P(A B C D)=P(A)P(B)P(C)P(D)$; however, the inverse is not always true.
- Independence of Random Variables (messy as hell...)
Given 2 random variables $
X_1 in {x_11, x_12, x_13,..., x_(1m)}\
X_2 in {x_21, x_22, x_23,..., x_(2n)}\
"Random variables X_1 and X_2 are independent" <=> \
P(X_1=x_(1i), X_2=x_(2j))=P(X_1=x_(1i))P(X_2=x_(2j))\
$
Need to check n*m equations to verify independence.
== Conditional Independence:
For events $A_1,A_2,...,A_n, B$, any set of events in A: $A_(i 1),A_(i 2),A_(i 3)$, they are conditionally independent given B if $
P(A_(i 1) A_(i 2)A_(i 3)|B)=P(A_(i 1)|B)* P(A_(i 2)|B)* P(A_(i 3)|B)
$
= Independent Trials, Distributions
== Bernoulli dirtribution:
a single trial, with success probability p, and failure probability 1-p. Prameter being the success probability.
$
X~"Ber"(p) => P(X=x)=p^x*(1-p)^(1-x), x in {0,1}
$
== Binomial Distribution:
multiple independent Bernoulli trials, with success probability p, and failure probability 1-p. Parameters being the number of trials $n$ and the success probability $p$.
$
X~"Bin"(n,p) => P(X=k)=binom(n,k)p^k*(1-p)^(n-k), k in {0,1,...,n}
$
== Geometric distribution:
multiple independent Bernoulli trials with success probability $p$, while stoping the experiment at the first success.
$
X~"Geom"(p)=p*(1-p)^(k-1), k in {1,2,...}
$
== Hypergeometric distribution:
There are N objects of type A, and $N_A- N$ objects of type B. Pick n objects without replacement. Denote number of A objects we picked as k. Parameters are $N, N_A, n$.
$
P(X=k)= (binom(N_A,k)binom(N-N_A,n-k))/(binom(N,n))\ "choose k from N_A, choose n-k from N-N_A, divide by total number of ways to choose n from N"
$
|
|
https://github.com/EstebanMunoz/typst-template-informe | https://raw.githubusercontent.com/EstebanMunoz/typst-template-informe/main/template/main.typ | typst | MIT No Attribution | #import "@local/fcfm-informe:0.1.0": conf, subfigures, today
// Parámetros para la configuración del documento. Descomentar aquellas que se quieran usar
#let document-params = (
// "include-title-page": false,
"title": "Título del documento",
"subject": "Tema del documento",
// "course-name": "",
// "course-code": "",
"students": ("<NAME>",),
// "teachers": ("",),
// "auxiliaries": ("",),
// "assistants": ("",),
// "semester": "Otoño 2024",
"due-date": today,
"place": "Santiago de Chile",
"university": "Universidad de Chile",
"faculty": "Facultad de Ciencias Físicas y Matemáticas",
"department": "Departamento de Ingeniería Eléctrica",
"logo": box(height: 1.57cm, image("assets/logos/die.svg")),
)
// Aplicación de la configuración del documento
#show: doc => conf(..document-params, doc)
// Índices de contenidos
#{
set page(numbering: "i")
outline(title: "Índice de Contenidos")
pagebreak()
outline(title: "Índice de Figuras", target: figure.where(kind: image))
outline(title: "Índice de Tablas", target: figure.where(kind: table))
outline(title: "Índice de Códigos", target: figure.where(kind: raw))
}
#counter(page).update(1)
////////// COMIENZO DEL DOCUMENTO //////////
= Sección importante
#lorem(200)
== Subsección importante
#lorem(33)
#subfigures(
columns: 2,
label: <fig:rects>,
caption: "Figura importante",
[#figure(
caption: "Un rectángulo muy importante",
rect(fill: blue)
) <fig:blue-rect>],
[#figure(
caption: "Rectángulo menos importante",
rect(fill: yellow)
) <fig:yellow-rect>]
)
#lorem(7) According to @fig:blue-rect, is known that #lorem(23) The main objective of @fig:rects is to show the importance of #lorem(40)
#pagebreak()
= Experimentos
== Experimento 1
One way to prove the above statement, is by means of the results of the following computational experiment:
#figure(caption: "Very important experiment",
```python
# This code replicates the important experiment. It has the same
# exact parameters of the results exposed.
print("Hello World!")
```
) <code:experiment>
#pagebreak()
= Resultados
The results of the experiment shown in @code:experiment, can be found in the below table #footnote[More experiments are needed to validate the results.]:
#figure(
caption: "The experiment results",
table(
columns: 3,
table.header(
[Experiment],
[N° experiments],
[Metrics \[Accuracy\]],
),
[Hello World],
[1], [92.1],
[Actual experiment],
[0], [0]
)
) <table:results>
#pagebreak()
= Análisis de resultados
The results shown in @table:results, one can understand that the importance of the experiment resides in #lorem(40)
#lorem(100)
#pagebreak()
= Conclusión
#lorem(134)
#lorem(101)
Por lo tanto, se concluye que se cumplieron los objetivos
|
https://github.com/cu1ch3n/karenda | https://raw.githubusercontent.com/cu1ch3n/karenda/main/lib.typ | typst | #import "nord.typ": *
#import "date.typ": *
#let karenda(year: datetime.today().year(), begin-weekday: 1, body) = {
let dates-by-month-week = get-dates(year, begin-weekday)
let left-margin = 15pt
let left-margin-content = 60pt
let top-margin = 20pt
let top-margin-content = 50pt
let radius = 5pt
let inner-inset = (x: 6pt, y: 5pt)
let outer-inset = (x: 4pt, y: 5pt)
let font-size = 12pt
let text-color = nord0
let text-color-light = nord4
let stroke-color = nord8
let panel-background-color = nord5
let panel-background-color-active = nord4
let month-calender-week-num-column-width = font-size * 2
let month-calender-column-width = 70pt
let month-calender-weekday-column-height = font-size * 2
let month-calender-day-num-row-height = 15pt
let month-calender-text-field-row-height = 45pt
let month-calender-columns = (month-calender-week-num-column-width,) + (month-calender-column-width,) * 7
let month-calender-rows = n => (month-calender-weekday-column-height,) + (
month-calender-day-num-row-height,
month-calender-text-field-row-height,
) * n
let month-calender-width = month-calender-week-num-column-width + month-calender-column-width * 7
let notes-left-margin = month-calender-width + left-margin-content + 20pt
let notes-top-margin = top-margin-content + 10pt
let notes-width = 230pt
let monthly-goals-height = 150pt
let notes-height = 150pt
let stroke = (thickness: 1pt, paint: stroke-color)
let text-field-pattern = pattern(size: (10pt, 10pt))[
#place(line(start: (0%, 0%), end: (0%, 100%), stroke: stroke))
#place(line(start: (0%, 0%), end: (100%, 0%), stroke: stroke))
]
let place-label(l) = {
place(left + top, [\ #label(l)])
}
let place-big-year(year) = {
place(left + top, dx: left-margin, dy: top-margin, {
text([#year], fill: text-color, size: font-size * 2)
})
}
let month-panel-button(month, fill) = link(label(month-short-text.at(month)), box(
fill: fill,
inset: inner-inset,
radius: radius,
{ month-short-text.at(month) },
))
let place-month-panel(highlight-month) = {
place(
left + horizon,
dx: left-margin,
{
block(fill: panel-background-color, inset: outer-inset, radius: radius, {
set align(center)
for month in range(12) {
if month == highlight-month {
month-panel-button(month, panel-background-color-active)
} else {
month-panel-button(month, none)
}
linebreak()
}
})
},
)
}
let place-big-month(month) = {
place(left + top, dx: left-margin-content, dy: top-margin, {
text(
[#h(font-size * 2) #month-long-text.at(month)],
fill: text-color,
size: font-size * 2,
)
})
}
let month-calender-header = table.header(..(([],) + (weekday-names * 2).slice(begin-weekday - 1, count: 7)))
let month-calender(num-weeks, data) = {
return table(
columns: month-calender-columns,
rows: month-calender-rows(num-weeks),
align: (x, y) => {
if x == 0 or y == 0 or calc.rem(y, 2) == 1 {
center + horizon
} else {
left + top
}
},
stroke: (x, y) => {
if y > 0 {
(right: stroke)
}
if x > 0 and calc.rem(y, 2) == 0 {
(bottom: stroke)
}
},
month-calender-header,
..data,
)
}
let place-notes = {
place(left + top, dx: notes-left-margin, dy: notes-top-margin, {
text([Monthly Goals], fill: text-color, size: font-size * 1.2)
v(-.5em)
rect(
fill: text-field-pattern,
width: notes-width,
height: monthly-goals-height,
stroke: 1pt + text-color-light,
)
text([Notes], fill: text-color, size: font-size * 1.2)
v(-.5em)
rect(
fill: text-field-pattern,
width: notes-width,
height: notes-height,
stroke: 1pt + text-color-light,
)
})
}
let get-month-calender(month, dates-by-week) = {
let result = ()
for (i, week) in dates-by-week {
result.push(table.cell(rowspan: 2, [#(i + 1)]))
for day in week {
result.push(table.cell({
if day.month() - 1 == month {
text(fill: text-color)[#day.day()]
} else {
text(fill: text-color-light)[#day.day()]
}
}))
}
for _ in week {
result.push[]
}
}
return result
}
let month-pages = {
for month in range(12) {
let dates-by-week = dates-by-month-week.at(month)
let data = get-month-calender(month, dates-by-week)
set page(background: {
place-label(month-short-text.at(month))
place-big-year(year)
place-month-panel(month)
place-big-month(month)
place-notes
})
month-calender(dates-by-week.len(), data)
pagebreak(weak: true)
}
}
set page(
paper: "presentation-16-9",
margin: (left: left-margin-content, top: top-margin-content, bottom: 0pt),
fill: nord6,
)
set text(font: "<NAME>", size: font-size, fill: text-color)
month-pages
} |
|
https://github.com/Kasci/LiturgicalBooks | https://raw.githubusercontent.com/Kasci/LiturgicalBooks/master/tmp.typ | typst | Kňaz: Sláva svätej, jednopodstatnej, životodarnej a nedeliteľnej Trojici v každom čase, teraz i vždycky, i na veky vekov. (ak nie je kňaz, prednášaj: Pane Ježišu Kriste, Bože náš, pre modlitby našich svätých otcov, zmiluj sa nad nami.)
Ľud: Amen.
Sláva na výsostiach Bohu a na zemi pokoj ľuďom dobrej vôle. (3x)
Pane, otvor moje pery, a moje ústa budú ohlasovať tvoju slávu. (2x)
Šesťžalmie
Potom prednášame šesťžalmie, ale z praktických či pastoračných dôvodov je možné modliť sa iba jeden zo žalmov – ak je radový hlas 1. – Ž 3, 2. hlas – Ž 37, 3. hlas – Ž 62, 4. hlas – Ž 87, 5. hlas – Ž 102, 6., 7., 8. hlas – Ž 142.
Žalm3
Pane, jak mnoho je tých, čo ma sužujú! \* Mnohí povstávajú proti mne. – Mnohí o mne hovoria: \* „Boh mu nepomáha.“ – Ale ty, Pane, si môj ochranca, \* moja sláva, čo mi hlavu vztyčuje. – Hlasne som volal k Pánovi \* a on mi odpovedal zo svojho svätého vrchu. – A ja som sa uložil na odpočinok a usnul som. \* Prebudil som sa, lebo Pán ma udržuje. – Nebudem sa báť tisícov ľudí, čo ma obkľučujú. \* Povstaň, Pane; zachráň ma, Bože môj. – Veď ty si udrel mojich nepriateľov po tvári \* a hriešnikom si zuby vylámal. – Pane, ty si naša spása. \* Na tvoj ľud nech zostúpi tvoje požehnanie. – A ja som sa uložil na odpočinok a usnul som. \* Prebudil som sa, lebo Pán ma udržuje.
Žalm 37
Nekarhaj ma, Pane, vo svojom rozhorčení \* a netrestaj ma vo svojom hneve, – lebo tvoje šípy utkveli vo mne, \* dopadla na mňa tvoja ruka. – Pre tvoje rozhorčenie niet na mojom tele zdravého miesta, \* pre môj hriech nemajú pokoj moje kosti. – Hriechy mi prerástli nad hlavu \* a ťažia ma príliš sťa veľké bremeno. – Rany mi zapáchajú a hnisajú \* pre moju nerozumnosť. – Zohnutý som a veľmi skľúčený, \* smutne sa vlečiem celý deň. – Bedrá mi spaľuje horúčka \* a moje telo je nezdravé. – Nevládny som a celý dobitý, \* v kvílení srdca nariekam. – Pane, ty poznáš každú moju túžbu; \* ani moje vzdychy nie sú skryté pred tebou. – Srdce mi búcha, sila ma opúšťa \* i svetlo v očiach mi hasne. – Priatelia moji a moji známi odvracajú sa odo mňa pre moju biedu; \* aj moji príbuzní sa ma stránia. – Tí, čo mi číhajú na život, nastavujú mi osídla, \* a tí, čo mi stroja záhubu, rozchyrujú o mne výmysly a deň čo deň vymýšľajú úklady. – Ale ja som sťa hluchý, čo nečuje, \* ako nemý, čo neotvára ústa. – Podobám sa človekovi, čo nepočuje \* a čo nevie obvinenie vyvrátiť. – Pane, pretože v teba dúfam, \* ty ma vyslyšíš, Pane, Bože môj. – A tak hovorím: „Nech sa už neradujú nado mnou; \* a keď sa potknem, nech sa nevystatujú nado mňa.“ – Ja, pravda, už takmer padám \* a na svoju bolesť myslím ustavične. – Preto vyznávam svoju vinu \* a pre svoj hriech sa trápim. – Moji nepriatelia sú živí a stále mocnejší, \* ba ešte pribudlo tých, čo ma nenávidia neprávom. – Za dobro sa mi odplácajú zlom a tupia ma za to, \* že som konal dobre. – Neopúšťaj ma, Pane; \* Bože môj, nevzďaľuj sa odo mňa. – Ponáhľaj sa mi na pomoc, \* Pane, moja spása.– Neopúšťaj ma, Pane; \* Bože môj, nevzďaľuj sa odo mňa. – Ponáhľaj sa mi na pomoc, \* Pane, moja spása.
Žalm 62
Bože, ty si môj Boh, \* už od úsvitu sa viniem k tebe. – Za tebou prahne moja duša, \* za tebou túži moje telo; – ako vyschnutá, pustá zem bez vody, \* tak ťa túžim uzrieť vo svätyni a vidieť tvoju moc a slávu. – Veď tvoja milosť je lepšia než život; \* moje pery budú ťa oslavovať. – Celý život ťa chcem velebiť \* a v tvojom mene dvíhať svoje ruky k modlitbe. – Sťa na bohatej hostine sa nasýti moja duša \* a moje ústa ťa budú chváliť jasavými perami. – Na svojom lôžku myslím na teba, \* o tebe rozjímam hneď za rána. – Lebo ty si mi pomáhal \* a pod ochranou tvojich krídel budem plesať. – Moja duša sa vinie k tebe, \* ujímaš sa ma svojou pravicou. – Tí však, čo chcú môj život zahubiť, \* zostúpia do hlbín zeme; – vydaní budú meču napospas, \* stanú sa korisťou šakalov. – Kráľ sa však bude tešiť v Bohu, \* chváliť sa budú všetci, ktorí prisahajú na neho, lebo budú umlčané ústa klamárov. – O tebe rozjímam hneď za rána. Lebo ty si mi pomáhal \* a pod ochranou tvojich krídel budem plesať.
(toto Sláva i teraz... prednášame iba ak sa modlíme všetky žalmy)
Sláva Otcu i Synu i Svätému Duchu. I teraz i vždycky i na veky vekov. Amen.
Aleluja, aleluja, aleluja, sláva tebe, Bože. (3x) (bez poklôn)
Pane, zmiluj sa. (3x)
Sláva, i teraz:
Žalm 87
Pane, ty Boh mojej spásy, \* dňom i nocou volám k tebe. – Kiež prenikne k tebe moja modlitba, \* nakloň svoj sluch k mojej prosbe. – Moja duša je plná utrpenia \* a môj život sa priblížil k ríši smrti. – Už ma počítajú k tým, čo zostupujú do hrobu, \* majú ma za človeka, ktorému niet pomoci. – Moje lôžko je medzi mŕtvymi, \* som ako tí, čo padli a odpočívajú v hroboch, – na ktorých už nepamätáš, \* lebo sa vymanili z tvojej náruče. – Hádžeš ma do hlbokej priepasti, \* do temravy a tône smrti. – Doľahlo na mňa tvoje rozhorčenie, \* svojimi prívalmi si ma zaplavil. – Odohnal si mi známych a zošklivil si ma pred nimi. \* Uväznený som a vyjsť nemôžem, aj zrak mi slabne od zármutku. – K tebe, Pane, volám deň čo deň \* a k tebe ruky vystieram. – Či mŕtvym budeš robiť zázraky? \* A vari ľudské tône vstanú ťa chváliť? – Či v hrobe bude dakto rozprávať o tvojej dobrote \* a na mieste zániku o tvojej vernosti? – Či sa v ríši tmy bude hovoriť o tvojich zázrakoch \* a v krajine zabudnutia o tvojej spravodlivosti? – Ale ja, Pane, volám k tebe, \* včasráno prichádza k tebe moja modlitba. – Prečo ma, Pane, odháňaš? \* Prečo predo mnou skrývaš svoju tvár? – Biedny som a umieram od svojej mladosti, \* vyčerpaný znášam tvoje hrôzy. – Cezo mňa sa tvoj hnev prevalil \* a zlomili ma tvoje hrozby. – Deň čo deň ma obkľučujú ako záplava \* a zvierajú ma zovšadiaľ. – Priateľov aj rodinu si odohnal odo mňa, \* len tma je mi dôverníkom. – Pane, ty Boh mojej spásy, \* dňom i nocou volám k tebe. – Kiež prenikne k tebe moja modlitba, \* nakloň svoj sluch k mojej prosbe.
Žalm 102
Dobroreč, duša moja, Pánovi \* a celé moje vnútro jeho menu svätému. – Dobroreč, duša moja, Pánovi \* a nezabúdaj na jeho dobrodenia. – Veď on ti odpúšťa všetky neprávosti, \* on lieči všetky tvoje neduhy; – on vykupuje tvoj život zo záhuby, \* on ťa venčí milosrdenstvom a milosťou; – on naplňuje dobrodeniami tvoje roky, \* preto sa ti mladosť obnovuje ako orlovi. – Pán koná spravodlivo \* a prisudzuje právo všetkým utláčaným. – Mojžišovi zjavil svoje cesty \* a synom Izraela svoje skutky. – Milostivý a milosrdný je Pán, \* zhovievavý a dobrotivý nesmierne. – Nevyčíta nám ustavične naše chyby \* ani sa nehnevá naveky. – Nezaobchodí s nami podľa našich hriechov \* ani nám neodpláca podľa našich neprávostí. – Lebo ako vysoko je nebo od zeme, \* také veľké je jeho zľutovanie voči tým, čo sa ho boja. – Ako je vzdialený východ od západu, \* tak vzďaľuje od nás našu neprávosť. – Ako sa otec zmilúva nad deťmi, \* tak sa Pán zmilúva nad tými, čo sa ho boja. – Veď on dobre vie, z čoho sme stvorení; \* pamätá, že sme iba prach. – Ako tráva sú dni človeka, \* odkvitá sťa poľný kvet. – Ledva ho vietor oveje, už ho niet, \* nezostane po ňom ani stopa. – No milosrdenstvo Pánovo je od večnosti až na večnosť \* voči tým, čo sa ho boja, – a jeho spravodlivosť chráni ich detné deti, \* tie, čo zachovávajú jeho zmluvu, čo pamätajú na jeho prikázania a plnia ich. – Pán si pripravil trón v nebesiach; \* kraľuje a panuje nad všetkými. – Dobrorečte Pánovi, všetci jeho anjeli, \* udatní hrdinovia, čo počúvate jeho slová a plníte jeho príkazy. – Dobrorečte Pánovi, všetky jeho zástupy, \* jeho služobníci, čo jeho vôľu plníte. – Dobrorečte Pánovi, všetky jeho diela, všade, kde on panuje. \* Dobroreč, duša moja, Pánovi.– Všade, kde on panuje. \* Dobroreč, duša moja, Pánovi.
Žalm 142
Pane, vyslyš moju modlitbu, pre svoju vernosť vypočuj moju úpenlivú prosbu, \* pre svoju spravodlivosť ma vyslyš. – A svojho služobníka na súd nevolaj, \* veď nik, kým žije, nie je spravodlivý pred tebou. – Nepriateľ ma prenasleduje, zráža ma k zemi, \* do temnôt ma vrhá ako dávno mŕtveho. – Duch sa mi zmieta v úzkostiach; \* v hrudi mi srdce meravie. – Spomínam si na uplynulé dni, o všetkých tvojich skutkoch rozmýšľam \* a uvažujem o dielach tvojich rúk. – Vystieram k tebe ruky, \* za tebou dychtím ako vyprahnutá zem. – Rýchle ma vyslyš, Pane, \* lebo už klesám na duchu. – Neskrývaj predo mnou svoju tvár, \* aby som nebol ako tí, čo zostupujú do hrobu. – Včasráno mi daj pocítiť, že si sa zmiloval nado mnou, \* lebo sa spolieham na teba. – Ukáž mi cestu, po ktorej mám kráčať, \* veď svoju dušu dvíham k tebe. – Pred nepriateľmi ma zachráň; \* Pane, k tebe sa utiekam. – Nauč ma plniť tvoju vôľu, lebo ty si môj Boh; \* na správnu cestu nech ma vedie tvoj dobrý duch. – Pre svoje meno, Pane, zachováš ma nažive; \* pretože si spravodlivý, vyveď ma z úzkosti. – Vo svojom milosrdenstve znič mojich nepriateľov, \* zahub všetkých, čo ma sužujú, veď ja som tvoj služobník. – Pre svoju spravodlivosť ma vyslyš \* a svojho služobníka na súd nevolaj. – Pre svoju spravodlivosť ma vyslyš \* a svojho služobníka na súd nevolaj. – Na správnu cestu \* nech ma vedie tvoj dobrý duch.
Sláva, i teraz:
Aleluja, aleluja, aleluja, sláva tebe, Bože. (3x)
(Ak je prítomný kňaz, alebo diakon, prednáša veľkú ekténiu. Ak nie, berieme
Pane, zmiluj sa. (12x)
Sláva, i teraz: )
Diakon: Modlime sa v pokoji k Pánovi.
Ľud: Pane, zmiluj sa. (po každej prosbe)
Za pokoj zhora a za spásu našich duší modlime sa k Pánovi.
Za mier na celom svete, za blaho svätých Božích cirkví a za zjednotenie všetkých modlime sa k Pánovi.
Za tento svätý chrám a za tých, čo doň vstupujú s vierou, nábožnosťou a s Božou bázňou, modlime sa k Pánovi.
Za veľkňaza všeobecnej Cirkvi, nášho Svätého Otca (povie meno), rímskeho pápeža, modlime sa k Pánovi.
Za nášho najosvietenejšieho otca arcibiskupa a metropolitu (povie meno), za nášho bohumilého otca biskupa (povie meno), za ctihodných kňazov a diakonov v Kristovi, za všetko duchovenstvo a ľud modlime sa k Pánovi.
Za tých, čo spravujú a ochraňujú našu krajinu, modlime sa k Pánovi.
Za toto mesto (alebo Za túto obec alebo Za tento svätý dom ), za všetky mestá, obce, krajiny a za tých, ktorí v nich podľa viery žijú, modlime sa k Pánovi.
Za priaznivé počasie, hojnosť plodov zeme a za pokojné časy modlime sa k Pánovi.
Za cestujúcich, chorých, trpiacich, zajatých a za ich záchranu modlime sa k Pánovi.
Za oslobodenie od všetkého nášho zármutku, hnevu a núdze modlime sa k Pánovi.
Zastaň sa a spas nás, zmiluj sa a zachráň nás, Bože, svojou milosťou.
Presvätú, prečistú, preblahoslavenú a slávnu Vládkyňu našu, Bohorodičku Máriu, vždy Pannu, i všetkých svätých spomínajúc, sami seba, druh druha i celý náš život Kristu Bohu oddajme.
Ľud: Tebe, Pane.
Kňaz: Lebo tebe patrí všetka sláva, česť a poklona, Otcu i Synu, i Svätému Duchu, teraz i vždycky, i na veky vekov.
Ľud: Amen.
Kňaz: Aleluja, aleluja, aleluja. (ľud opakuje po každom verši na aktuálny hlas oktoicha)
Verš: Svojou dušou dychtím po tebe v noci, Bože, lebo tvoje prikázania osvecujú celú zem.
Verš: Učte sa spravodlivosti, obyvatelia zeme.
Verš: Závisť zničí ľudí nepolepšiteľných.
Verš: Potrestal si zlých, Pane, potrestal si mocných zeme.
Trojičné piesne, 8. hlas
Svoje srdcia dvíhame k nebesám, \* a tak napodobňujeme anjelské šíky. \* Padnime s bázňou pred Sudcom \* a spievajme víťaznú chválu: \* Svätý, svätý, svätý si, Bože náš, \* na príhovor svätých apoštolov a svätého Mikuláša \*\* zmiluj sa nad nami.
Sláva:
Cherubíni ťa nemôžu vidieť, \* lietajú a s radosťou spievajú trojsvätým hlasom božskú pieseň. \* S nimi aj my ti spievame: \* Svätý, svätý, svätý si, Bože náš, \* na príhovor všetkých svojich svätých, zmiluj sa nad nami.
I teraz:
Skľúčení množstvom svojich hriechov \* nemôžeme ani len vzhliadnuť k nebeským výšinám, \* ale svoju dušu i telo skláňame pred tebou \* a s anjelmi ti spievame pieseň: \* Svätý, svätý, svätý si, Bože náš, \* na príhovor Bohorodičky, zmiluj sa nad nami.
1. katizma
Pane, zmiluj sa. (trikrát)
Sláva, i teraz:
Žalm 55
Zmiluj sa nado mnou, Bože, lebo ma prenasleduje človek, \* každodenne ma napáda a utláča. – Moji nepriatelia ma prenasledujú deň čo deň \* a veľa je tých, čo bojujú proti mne, Najvyšší. – Kedykoľvek ma popadne strach, \* spolieham sa na teba. – Na Boha, ktorého slovo velebím, \* na Boha sa ja spolieham – a nebojím sa: \* veď čože mi môže urobiť človek? – Dennodenne mi spôsobujú škodu, \* myslia len na to, ako mi zle urobiť. – Podnecujú spory, stroja úklady, \* idú mi v pätách a sliedia. – Ako mne číhali na život, tak im odplať neprávosť, \* Bože, v hneve zraz pohanov až k zemi. – Ty vieš, koľko ráz som musel utekať: \* pozbieraj moje slzy do svojich nádob; či nie sú zapísané v tvojich účtoch? – Vtedy moji nepriatelia ustúpia, ešte v ten deň, keď budem volať: \* áno, viem, že ty si môj Boh. – Na Boha, ktorého slovo velebím, \* na Pána, ktorého slovo velebím, – na Boha sa ja spolieham \* a nebojím sa: veď čože mi môže urobiť človek? – Bože, ešte mám splniť sľuby, čo som tebe urobil; \* obety chvály ti prinesiem, – lebo ty si mi život zachránil pred smrťou a nohy pred pádom, \* aby som kráčal pred Bohom vo svetle žijúcich.
Žalm 56
Zmiluj sa, Bože, nado mnou, zmiluj sa nado mnou, \* lebo sa k tebe utiekam; – v tieni tvojich krídel nachodím útočisko, \* kým sa nepominú nástrahy. – Volám k Bohu, k Najvyššiemu, \* k Bohu, ktorý mi preukazuje dobro. – On zošle pomoc z neba a zachráni ma; \* zahanbí tých, čo ma týrajú. Boh zošle svoju milosť a pravdu. – Som akoby uprostred levíčat, \* čo požierajú ľudí. – Ich zuby sú ako oštepy a šípy, \* ich jazyk sťa nabrúsený meč. – Bože, vznes sa nad nebesia \* a tvoja sláva nech je nad celou zemou. – Mojim nohám nastavili osídlo, \* až sa mi skormútila duša; – predo mnou vykopali jamu, \* no sami do nej padli. – Ochotné je moje srdce, Bože, ochotné je moje srdce; \* budem ti spievať a hrať. – Prebuď sa, duša moja, \* prebuď sa, harfa a citara, chcem zobudiť zornicu. – Budem ťa, Pane, velebiť medzi pohanmi \* a zaspievam ti žalmy medzi národmi. – Lebo až po nebesia siaha tvoje milosrdenstvo \* a tvoja vernosť až po oblaky. – Bože, vznes sa nad nebesia \* a tvoja sláva nech je nad celou zemou.
Žalm 57
Vari vy, mocnári, naozaj hlásate spravodlivosť, \* vari správne súdite ľudských synov? – Naopak, v srdci páchate neprávosti, \* vaše ruky pripravujú násilie na zemi. – Hriešnici sú na scestí už od lona matky, \* od materského života blúdia klamári. – Jed v nich podobá sa jedu hadiemu, \* jedu hluchej vretenice, čo si uši zapcháva, – ktorá nepočúva hlas zaklínačov, \* hlas skúseného čarodeja. – Bože, vylám im zuby v ústach, \* rozdrv čeľusť levov, Pane. – Nech sa rozplynú sťa voda, čo steká, \* nech vyschnú ako zdeptaná tráva. – Nech sa pominú ako slimák, čo sa v hlien rozteká, \* ako nedonosený plod ženy, ktorý slnko neuzrie. – Prv ako vaše hrnce pocítia oheň z bodliaka, \* zaživa ich strávi ako oheň hnevu. – Spravodlivý sa poteší, keď pomstu uvidí, \* umyje si nohy v krvi hriešnika. – A ľudia povedia: „Naozaj je odmena pre spravodlivého, \* naozaj je Boh, čo súdi na zemi.
Sláva Otcu i Synu i Svätému Duchu. I teraz i vždycky i na veky vekov. Amen.
Aleluja, aleluja, aleluja, sláva tebe, Bože. (3x)
Pane, zmiluj sa. (3x)
Sláva, i teraz:
Žalm 58
Vytrhni ma, Bože, z moci mojich nepriateľov, \* chráň ma pred tými, čo povstávajú proti mne. – Vytrhni ma z rúk zločincov \* a zachráň pred krvilačníkmi. – Pozri, úklady robia na môj život \* a surovo sa vrhajú na mňa. – Pane, neťaží ma ani priestupok, ani hriech; \* nedopustil som sa neprávosti, – a predsa sa zbiehajú a na mňa chystajú. \* Hor’ sa, poď mi v ústrety a pozri. – Ty, Pane, Bože mocností, Boh Izraela, \* precitni a potrestaj všetkých pohanov, nemaj zľutovanie nad tými, čo, vierolomne konajú. – Na večer sa vracajú, zavýjajú ako psy \* a pobehujú okolo mesta. – Hľa, čo chrlia ich ústa, \* meč majú na perách: „Ktože to počuje?” – Ty sa im však smeješ, Pane; \* vysmievaš sa všetkým pohanom. – Záštita moja, čakám na teba; \* lebo ty, Bože, si moja ochrana. – So mnou je Boh, jeho láska ma predchádza. \* Boh dá, že svojimi nepriateľmi budem môcť pohrdnúť. – Nepobi ich, aby môj ľud nezabudol; \* rozožeň ich svojou mocou a zraz ich k zemi, Pane, môj ochranca. – Pre hriech ich úst, pre reč ich perí nech sa chytia do svojej pýchy; \* pre kliatbu a lož, ktorú vyriekli. – Skoncuj s nimi v rozhorčení, skoncuj a nebude ich; \* a spoznajú, že Boh panuje v Jakubovi a až po kraj zeme. – Na večer sa vracajú, zavýjajú ako psy \* a pobehujú okolo mesta. – Túlajú sa za pokrmom \* a ak sa nenasýtia, skuvíňajú. – Ja však budem oslavovať tvoju moc \* a z tvojho milosrdenstva sa tešiť od rána, – lebo ty si sa mi stal oporou \* a útočiskom v deň môjho súženia. – Tebe, záštita moja, chcem spievať, \* lebo ty, Bože, si môj ochranca, môj Boh, moje milosrdenstvo.
Žalm 59
Bože, ty si nás odvrhol, ty si nás rozohnal; \* rozhneval si sa, ale opäť sa k nám obráť. – Zatriasol si zemou, rozštiepil si ju, \* ale zahoj jej trhliny, lebo sa chveje. – Tvrdú skúšku si zoslal na svoj ľud, \* napojil si nás vínom závratu. – Tým, čo sa ťa boja, dal si znamenie, \* aby utiekli pred lukom. – Aby sa vyslobodili tí, ktorých miluješ; \* zachráň nás svojou pravicou a vyslyš nás. – Vo svojej svätyni Boh povedal: \* „S radosťou rozdelím Sichem a rozmeriam sukotské údolie. – Môj je Galaád a môj je Manasses; \* Efraim je prilba mojej hlavy. – Júda je moje žezlo vladárske, \* Moab je nádrž, v ktorej sa kúpavam. – Idumea sa mi stane podnožkou, \* nad Filištínskom víťazne zajasám.” – Kto ma privedie do opevneného mesta \* a kto ma odprevadí až do Idumey? – Kto iný, ako ty, Bože, čo si nás odvrhol? \* A prečo už, Bože, nekráčaš na čele našich vojsk? – Pomôž nám dostať sa z útlaku, \* pretože ľudská pomoc nestačí. – S Bohom budeme udatní, \* on našich utláčateľov pošliape.
Žalm 60
Vypočuj, Bože, moju vrúcnu prosbu, \* všimni si moju modlitbu. – Od konca zeme volám k tebe, keď sa mi srdce chveje úzkosťou, \* priveď ma na nedostupné bralo. – Ty si moja nádej \* a bašta pred nepriateľom. – V tvojom stánku chcem prebývať naveky \* a skrývať sa pod ochranou tvojich krídel. – Veď ty si, Bože môj, vypočul moju modlitbu, \* dal si mi dedičstvo tých, čo si ctia tvoje meno. – Kráľovi pridaj k jeho dňom ďalšie \* a jeho roky nech trvajú z pokolenia na pokolenie. – Pred Božou tvárou nech tróni večne \* a nech ho chráni milosť a vernosť. – Tak budem naveky ospevovať tvoje meno \* a plniť svoj sľub deň čo deň.
Sláva Otcu i Synu i Svätému Duchu. I teraz i vždycky i na veky vekov. Amen.
Aleluja, aleluja, aleluja, sláva tebe, Bože. (3x)
Pane, zmiluj sa. (3x)
Sláva, i teraz:
Žalm 61
Iba v Bohu spočiň, duša moja, \* lebo od neho mi prichádza spása. – Iba on je moje útočisko a moja spása, \* moja opora, nezakolíšem sa nikdy viac. – Dokedy chcete napádať človeka, dokedy ho chcete všetci krušiť \* ako stenu, čo sa nakláňa, ako múr, čo sa váľa? – Stroja sa zvrhnúť ho z popredného miesta, \* v klamaní majú záľubu, – dobrorečia svojimi ústami, \* no v srdci zlorečia. – Iba v Bohu spočiň, duša moja, \* lebo len on mi dáva nádej, – iba on je moje útočisko a moja spása, \* moja opora, nezakolíšem sa. – V Bohu je moja spása i sláva; \* Boh je moja sila a v Bohu je moje útočisko. – Dúfajte v neho, ľudia, v každom čase, pred ním si srdce otvorte; \* Boh je naše útočisko. – Veď iba klam a mam sú potomci Adama, \* ľudia sú iba preludom. – Keby si stali na váhu, \* dohromady sú ľahší ako para. – Nespoliehajte sa na násilie a neprepadnite zbojstvu; \* ak vám pribúda bohatstva, neoddávajte mu srdce. – Raz prehovoril Boh, \* počul som toto dvoje: že Boh je mocný – a ty, Pane, milostivý; \* že ty každému odplácaš podľa jeho skutkov.
Žalm 62
Bože, ty si môj Boh, \* už od úsvitu sa viniem k tebe. – Za tebou prahne moja duša, \* za tebou túži moje telo; – ako vyschnutá, pustá zem bez vody, \* tak ťa túžim uzrieť vo svätyni a vidieť tvoju moc a slávu. – Veď tvoja milosť je lepšia než život; \* moje pery budú ťa oslavovať. – Celý život ťa chcem velebiť \* a v tvojom mene dvíhať svoje ruky k modlitbe. – Sťa na bohatej hostine sa nasýti moja duša \* a moje ústa ťa budú chváliť jasavými perami. – Na svojom lôžku myslím na teba, \* o tebe rozjímam hneď za rána. – Lebo ty si mi pomáhal \* a pod ochranou tvojich krídel budem plesať. – Moja duša sa vinie k tebe, \* ujímaš sa ma svojou pravicou. – Tí však, čo chcú môj život zahubiť, \* zostúpia do hlbín zeme; – vydaní budú meču napospas, \* stanú sa korisťou šakalov. – Kráľ sa však bude tešiť v Bohu, \* chváliť sa budú všetci, ktorí prisahajú na neho, lebo budú umlčané ústa klamárov.
Žalm 63
Počuj, Bože, môj hlasitý nárek; \* ochraňuj mi život pred strašným nepriateľom. – Bráň ma pred zberbou ničomníkov, \* pred tlupou zločincov. – Jazyk si brúsia ako meč, ako šípy hádžu jedovaté slová, \* aby nevinného zasiahli z úkrytu. – Znezrady a smelo doňho strieľajú, \* 6 utvrdzujú sa v zločinnom zámere. – Radia sa, ako zastrieť osídla, \* a vravia si: „Ktože ich zbadá?” – Vymýšľajú neprávosť a čo vymyslia, aj dovŕšia. \* Hlbočina je človek a jeho srdce priepasť. – Lež Boh ich zasiahol svojimi šípmi; \* zrazu ich pokryli rany, – vlastný jazyk sa im stal nešťastím. \* Všetci, čo ich vidia, kývajú hlavou, – strach zachváti každého človeka; \* i budú hlásať Božie skutky a chápať jeho diela. – Spravodlivý sa teší v Pánovi a spolieha sa na neho, \* a jasajú všetci, čo majú srdce úprimné.
Sláva Otcu i Synu i Svätému Duchu. I teraz i vždycky i na veky vekov. Amen.
Aleluja, aleluja, aleluja, sláva tebe, Bože. (3x)
Pane, zmiluj sa. (3x)
Sláva, i teraz:
1. Sedalen, 8. hlas
Ako svetlá žiariace vo svete \* a pomocníci našej spásy ste vy, apoštoli. \* My sme sedeli vo tme a tôni hriechu, \* ale vy ste nám ukázali svetlo slávy. \* Premohli ste klamstvá zlého ducha, \* hlásali ste Najsvätejšiu Trojicu v jednom Bohu. \* Preto vás aj my prosíme: \* Proste za nás Krista Boha, \* aby nám odpustil všetky previnenia.
Sláva i teraz, 8. hlas
Neodolal som mnohým pokušeniam, \* ktoré na mňa číhali od viditeľných i neviditeľných vrahov. \* Moje vnútro je znečistené mnohými vinami. \* Preto ako k istej zástankyni a ochrane \* prichádzam k tebe, najčistejšia Bohorodička. \* Prihováraj sa za mňa u Boha, \* ktorý bez spolupôsobenia muža prijal z teba telo. \* Pros ho, aby mi odpustil všetky poklesky, \* lebo s vierou si uctievam pamiatku jeho vtelenia a narodenia.
Pane, zmiluj sa. (trikrát)
Sláva, i teraz:
2. Sedalen, 8. hlas
Dnes sa splnili slová prorokov, \* lebo po celej zemi sa rozlieha hlas svätých apoštolov. \* Oni hlásali večné živé Slovo, \* jeho učením ožiarili všetky národy. \* Aj my si ich uctievame oslavnými piesňami, \* lebo sa za nás prihovárajú u Krista, \* aby sme spolu s nimi mohli byť večne blažení.
Sláva i teraz, 8. hlas
Zástankyňa veriacich, Bohorodička, \* radosť zarmútených, \* veľká potecha plačúcich, \* z tvojho presvätého lona nadprirodzene Narodeného \* neustále pros za nás spolu so svätými apoštolmi, \* aby sme v čase súdu boli oslobodení od krutého odsúdenia.
Pane, zmiluj sa. (trikrát)
Sláva, i teraz:
Žalm 50
Zmiluj sa, Bože, nado mnou pre svoje milosrdenstvo \* a pre svoje veľké zľutovanie znič moju neprávosť. – Úplne zmy zo mňa moju vinu \* a očisť ma od hriechu. – Vedomý som si svojej neprávosti \* a svoj hriech mám stále pred sebou. – Proti tebe, proti tebe samému som sa prehrešil \* a urobil som, čo je v tvojich očiach zlé, – aby si sa ukázal spravodlivý vo svojom výroku \* a nestranný vo svojom súde. – Naozaj som sa v neprávosti narodil \* a hriešneho ma počala moja mať. – Ty naozaj máš záľubu v srdci úprimnom \* a v samote mi múdrosť zjavuješ. – Pokrop ma yzopom a zasa budem čistý; \* umy ma a budem belší ako sneh. – Daj, aby som počul radosť a veselosť, \* a zaplesajú kosti, ktoré si rozdrvil. – Odvráť svoju tvár od mojich hriechov \* a zotri všetky moje viny. – Bože, stvor vo mne srdce čisté \* a v mojom vnútri obnov ducha pevného. – Neodvrhuj ma spred svojej tváre \* a neodnímaj mi svojho Ducha Svätého. – Navráť mi radosť z tvojej spásy \* a posilni ma duchom veľkej ochoty. – Poučím blúdiacich o tvojich cestách \* a hriešnici sa k tebe obrátia. – Bože, Boh mojej spásy, zbav ma škvrny krvipreliatia \* a môj jazyk zajasá nad tvojou spravodlivosťou. – Pane, otvor moje pery \* a moje ústa budú ohlasovať tvoju slávu. – Veď ty nemáš záľubu v obete \* ani žertvu neprijmeš odo mňa. – Obetou Bohu milou je duch skrúšený; \* Bože, ty nepohŕdaš srdcom skrúšeným a poníženým. – Buď dobrotivý, Pane, a milosrdný voči Sionu, \* vybuduj múry Jeruzalema. – Potom prijmeš náležité obety, obetné dary a žertvy; \* potom položia na tvoj oltár obetné zvieratá.
Kánon, 6. hlas
Pieseň 1.
Irmos
Pomocník a Zástanca, \* stal sa mojou záchranou. \* On je môj Boh, budem ho oslavovať, \* Boh môjho otca, budem ho vyvyšovať, lebo sa veľmi preslávil.\* ~~(2x)\*~~
Verš: Zmiluj sa nado mnou, Bože, zmiluj sa nado mnou.
Odkiaľ začnem oplakávať skutky svojho úbohého života? Akýže položím základ, Kriste, pre terajšie trúchlenie? Ale ty mi daruj, ako milosrdný, odpustenie hriechov.
Kráčaj, úbohá duša, so svojím telom k Tvorcovi všetkého. Vyznaj sa a zotrvaj ďaleko od predošlej nerozumnosti; Prinášaj Bohu v pokání slzy.
Prvostvoreného Adama prečin som prekonal. Ocitol som sa nahý bez Boha, bez večného kráľovstva a radosti; pre moje hriechy.
Verš: Zmiluj sa nado mnou, Bože, zmiluj sa nado mnou.
Beda mi, úbohá duša! Prečo si napodobnila prvú Evu? Okúsila si zlo a trpko si sa zranila, siahla si na strom a trúfalo ochutnala nerozumný pokrm.
Miesto skutočnej Evy povstala vo mne Eva pomyselná, telesné pokušenie z vášne;sľubuje pôžitok, no stále ochutnáva z horkého nápoja.
Právom bol Adam vyhnaný z raja, lebo nezachoval jediný tvoj príkaz, Spasiteľ. Čo potom čaká mňa, keď stále odmietam tvoje slová života?
Prešiel som si Kainovou úkladnou vraždou: Prebudil som telesnosť a stal som sa vrahom svedomia duše; tomu som ubližoval svojimi zlými skutkami.
Ježišu, Ábelovu spravodlivosť som nenapodobnil. Dar tebe príjemný nikdy som nepriniesol, a ani Bohom určené skutky, ani čistú obeť, ani bezúhonný život.
Ako Kain, tak aj my, úbohá duša, Tvorcovi všetkého nečisté skutky, poškvrnenú obeť a neužitočný život vedno sme priniesli, a preto sme boli odsúdení.
Hlinu si ako Hrnčiar oživil a vložil do mojej podstaty, mäso a kosti, dych a život. Ale, Tvorca môj, Vykupiteľ a Sudca, prijmi ma, kajúceho.
Vyznávam ti, Spasiteľ, hriechy, ktoré som vykonal; mojej duše a môjho tela rany, ktoré mi zvnútra
smrtiace pokušenia zločinne spôsobili.
Hoci som zhrešil, Spasiteľ, viem, že miluješ človeka. Súcitne ho vychovávaš a vrúcne sa zmilúvaš. Keď vidíš jeho slzy, bežíš v ústrety ako otec a voláš márnotratného.
Ležím, Spasiteľ, pred tvojimi bránami, aspoň v starobe, neodvrhni ma naprázdno do podsvetia, ale ešte pred koncom daruj mi, ako Milujúci človeka, odpustenie hriechov.
Vydaný do rúk zbojníkov – to som ja, v zajatí svojich pokušení. Celý som od nich teraz dobitý, som plný rán; ale ty sám sa ma zastaň, Kriste Spasiteľu, a uzdrav ma.
Kňaz ma zďaleka videl a obišiel, rovnako aj levita: Uzrel ma nahého v nešťastí, a odvrátil zrak. Ale ty, ktorý si z Márie zažiaril Ježišu, zastaň sa a zľutuj sa nado mnou.
Baránok Boží, ty snímaš hriechy všetkých, sním zo mňa ťažké bremeno, ťažobu hriechov; A ako milosrdný mi daruj slzy skrúšenosti.
Je čas pokánia, prichádzam k tebe, svojmu Stvoriteľovi, sním zo mňa ťažké bremeno, ťažobu hriechov. A ako milosrdný mi daruj slzy skrúšenosti.
Neštíť sa ma, Spasiteľ, neodháňaj ma od svojej tváre. Sním zo mňa ťažké bremeno, ťažobu hriechov A ako milosrdný mi daruj odpustenie hriechov.
Moje dobrovoľné hriechy, i tie nedobrovoľné, Spasiteľu, zjavné i tajné; vedomé a všetky nevedomé; odpusť ako Boh, očisť ma a zachráň.
Od mladosti, Kriste, prestupoval som tvoje prikázania. Ovládnutý vášňami nedbanlivo v pohodlí premárnil som život. Preto ti volám, Spasiteľu: aspoň na poslednú chvíľu ma zachráň.
Svoje bohatstvo, Spasiteľ, márnotratne som premrhal; som ako púšť bez ovocia zbožnosti, a hladný volám: Otče zľutovania, poď mi v ústrety a zľutuj sa.
Padám pred tebou, Ježišu, zhrešil som proti tebe, očisť ma. Sním zo mňa ťažké bremeno, ťažobu hriechov. A ako milosrdný Boh, prijmi ma, kajúceho.
Nevolaj ma na súd, nepredkladaj tam moje skutky. Ty skúmaš slová a naprávaš myšlienky, vo svojom zľutovaní prehliadni moje hanebnosti a zachráň ma, Všemocný.
Verš: Prepodobná matka Mária, pros Boha za nás.
Daruj mi žiarivú milosť zhora, z Božej prozreteľnosti; milosť uniknúť zatemneniu vášňami, a horlivo ospevovať povzbudivý príbeh tvojho života, Mária.
Verš: Prepodobná matka Mária, pros Boha za nás.
Priklonila si sa ku Kristovým božským zákonom, pristúpila si k nemu; zanechala si neodolateľnú náklonnosť k pôžitkom a zbožne každej cnosti postupne si sa učila.
Verš: Prepodobný otec Andrej, pros Boha za nás.
Svojimi modlitbami, Andrej, osloboď nás od hanebných vášní. S vierou a láskou ťa ospevujeme, slávny, a prosíme ťa teraz, daj nám účasť na Kristovom kráľovstve.
Sláva:
Nadprirodzená Trojica, ako Jednej sa ti klaniame. Sním zo mňa ťažké bremeno, ťažobu hriechov, a ako milosrdná mi daruj slzy dojatia.
I teraz:
Bohorodička, nádej a záštita tých, čo ťa ospevujú. Sním zo mňa ťažké bremeno, ťažobu hriechov, a ako čistá Vládkyňa, prijmi ma, kajúceho.
Katavasia: Pomocník a Zástanca, \* stal sa mojou záchranou. \* On je môj Boh, budem ho oslavovať, \* Boh môjho otca, budem ho vyvyšovať, lebo sa veľmi preslávil.
Pieseň 2a.
Irmos
Pozorne vnímaj, nebo, ja prehovorím: budem ospevovať Krista, ktorý z Panny v tele prišiel k nám. (2x)
Verš: Zmiluj sa nado mnou, Bože, zmiluj sa nado mnou.
Pozorne vnímaj, nebo, ja prehovorím; zem, počuj hlas, ktorý sa kajá pred Bohom a ospevuje ho.
Všimni si ma, Bože, môj Spasiteľ, svojím láskavým okom, a prijmi moje vrúcne vyznanie.
Zhrešil som viac než všetci ľudia, ja sám som zhrešil proti tebe, ale zľutuj sa ako Boh, Spasiteľ, nad svojím dielom.
Búrka hrôz ma obklopuje, milosrdný Pane; ale ako k Petrovi, aj ku mne vystri ruku.
Slzy smilnice, Milosrdný, predkladám aj ja; očisť ma, Spasiteľu svojím milosrdenstvom.
Zatemnil som duševnú krásu pôžitkami vášní, celú myseľ som úplne premenil na prach.
Zodral som si teraz svoj prvotný odev, ktorý mi utkal Tvorca na počiatku, a odvtedy ležím nahý.
Obliekol som si roztrhané rúcho, ktoré mi utkal Had svojou radou, a hanbím sa.
Vzhliadol som na nádheru Záhrady a moja myseľ sa dala oklamať. Odvtedy ležím nahý a hanbím sa.
Nakladali na môj chrbát všetci vládcovia vášní, rozmnožovali vo mne svoje bezprávie. Stratil som prvostvorenú krásu a svoju dôstojnosť; teraz ležím nahý a hanbím sa.
Aj mne hriech ušil kožený odev, a zvliekol mi pôvodné, Bohom utkané šaty.
Pokrytý som odevom hanby ako figovým lístím, na usvedčenie mojich nespútaných vášní.
Odel som si potupné rúcho, hanebne zakrvavené počas života vášní a pôžitkov.
Poškvrnil som rúcho svojho tela, pošpinil som, to, čo je na obraz, Spasiteľ, i na podobu.
Upadol som do trápenia vášní, do hmotného rozkladu, a nepriateľ ma odvtedy až doteraz potupuje.
Lásku k veciam, život pre majetok, to som v nezdržanlivosti, Spasiteľ, uprednostnil a teraz si nesiem ťažké bremeno.
Skrášlil som vzhľad tela rozmanitými závojmi necudných pokušení; a tým sa odsudzujem.
Vonkajšie usilovné skrášľovanie bolo mi jedinou starosťou, prehliadal som pritom vnútornú svätyňu na Boží obraz.
Dal som tvár ohavnostiam svojich vášní; náklonnosťou k pôžitkom zničil som krásu svojej mysle.
Pochoval som krásu prvotného obrazu svojimi vášňami, Spasiteľ. Ty ako kedysi drachmu, hľadaj ju a nájdi.
Zhrešil som; ako smilnica ťa volám, ja sám som proti tebe zhrešil. Prijmi ako myro, Spasiteľ, aj moje slzy.
Pošmykol som sa na smilstve ako Dávid, a poškvrnil som sa. Ale obmy aj mňa, Spasiteľ, mojimi slzami.
Očisť, ako mýtnik ťa volám: Spasiteľ, očisť ma, lebo nik z potomkov Adama nezhrešil proti tebe viac ako ja.
Nemám slzy, nemám pokánie, a ani ľútosť. Ty sám mi ich, Spasiteľ, daruj ako Boh.
Svoju bránu nezavri predo mnou v ten deň, Pane, Pane, ale otvor mi ju, keď sa ti kajám.
Miluješ človeka a chceš, aby všetci boli spasení, ty ma zavolaj a prijmi ma, ako Dobrotivý, keď sa kajám.
Počuj vzdychanie mojej duše a z mojich očí prijmi kvapky sĺz, Spasiteľ, a zachráň ma.
Verš: Presvätá Bohorodička, zachráň nás!
Prečistá Bohorodička Panna, jediná všeospevovaná, horlivo sa modli, aby sme boli zachránení.
Pieseň 2b.
Irmos
Hľaďte, hľaďte, ja som Boh. Zoslal som mannu ako dážď a vodu z kameňa nechal som kedysi vytrysknúť na púšti pre svoj ľud; jedinou pravicou a svojou mocou.
Verš: Zmiluj sa nado mnou, Bože, zmiluj sa nado mnou.
Hľaďte, hľaďte, ja som Boh. Počúvaj, duša moja, Pána, ktorý volá, a vzdiaľ sa od predošlých hriechov; boj sa ho ako neúplatného, ako Sudcu a Boha.
Kohože si napodobnila, mnohohriešna duša? Iba prvotného Kaina a povestného Lamecha, keď si kameňovala telo zlými skutkami; a zabila si rozum nerozumnými žiadosťami.
Prešla si si, duša, všetkým, čo bolo pred Zákonom,ale nenapodobnila si Seta, nenasledovala si Enosa, ani Henocha a jeho vzatie Bohom, a ani Noeho; ostávaš ochudobnená o životy spravodlivých.
Ty sama si spustila vodopády hnevu svojho Boha, duša moja, a zatopila si ako zem celé svoje telo, i svoje skutky a život; ostala si mimo zachraňujúcej archy.
„Zabil som muža, ktorý ma zranil“ – tak hovoril – „i mládenca, ktorý ma udrel“, tak Lámech volal s plačom. A ty sa nechveješ, keď si pošpinila telo, duša moja, a myseľ poškvrnila?!
Prečo som súperil s Lámechom, dávnym vrahom? Dušu ako muža, rozum ako mládenca; ako svojho brata telo som zabil; ako vrah Kain,pre náklonnosť k pôžitkom.
Zaumienila si si, ó duša, postaviť vežu, a svojimi žiadosťami založiť pevnosť. Ešteže Tvorca zadržal tvoje zámery a zboril k zemi tvoje chytráctva.
Som dobitý, som zranený, hľa, nepriateľské strely! Tie mi zranili dušu a telo. Hľa, moje rany, vredy, zatemnenie zraku, volajú pod údermi mojich svojvoľných vášní.
Pán kedysi ako dážď zoslal „oheň od Pána“, a spálil prerastenú neprávosť Sodomčanov. Aj ty si zažala oheň gehenský, v ktorom máš byť, duša, kruto spálená.
Spoznajte a hľaďte, že ja som Boh, skúmam srdcia a skúšam myšlienky, odhaľujem skutky a spaľujem hriechy; hájim právo siroty, poníženého a chudobného.
Verš: Prepodobná matka Mária, pros Boha za nás.
Dvíhala si svoje ruky k milosrdnému Bohu, Mária, ponorená v priepasti zla. On, tak ako k Petrovi, ľudomilne vystrel aj k tebe svoju ochrannú ruku; nadovšetko sa usiloval o tvoj návrat.
Verš: Prepodobná matka Mária, pros Boha za nás.
So všetkou horlivosťou a láskou si bežala ku Kristovi, pôvodnú hriešnu cestu si zavrhla. Sýtila si sa v neprístupných pustatinách a v čistote si plnila jeho božské prikázania.
Verš: Prepodobný otec Andrej, pros Boha za nás.
Hľaďme, hľaďme, duša, na ľudomilnosť Boha a Vládcu. Preto skôr, než príde koniec, padnime pred ním a volajme v slzách: Na príhovor Andreja, Spasiteľ, zmiluj sa nad nami.
Sláva:
Bezpočiatočná, nestvorená Trojica, nedeliteľné Jedno! Kajám sa, prijmi ma, zhrešil som, zachráň ma. Som tvoje dielo, neprehliadni ma, ale ušetri a vysloboď ma z ohňa odsúdenia.
I teraz:
Prečistá Vládkyňa, Bohorodička, nádej k tebe sa utiekajúcich, prístav búrkou zmietaných. Milosrdný, Tvorca, tvoj Syn, nech je milosrdný aj voči mne; pre tvoje modlitby.
Katavasia: Hľaďte, hľaďte, ja som Boh. Zoslal som mannu ako dážď a vodu z kameňa nechal som kedysi vytrysknúť na púšti pre svoj ľud; jedinou pravicou a svojou mocou.
Pieseň 3a.
Irmos
Na nehybnom kameni svojich prikázaní, Kriste, upevni moje zmýšľanie. (2x)
Verš: Zmiluj sa nado mnou, Bože, zmiluj sa nado mnou.
„Oheň od Pána“ kedysi Pán zoslal ako dážď, a sodomskú zem tak vtedy dávno spálil.
Na vrchu sa zachráň, duša, ako tamten Lót, hľadaj útočisko v Segore.
Utekaj, duša, pred vznietením, utekaj pred Sodomským požiarom, utekaj pred spálením božským plameňom.
Vyznávam sa ti, Spasiteľ, nadmieru som zhrešil pred tebou; ale prepáč a odpusť mi ako súcitný
Ja sám som proti tebe zhrešil, zhrešil som viac ako všetci, Kriste, Spasiteľu, neprehliadni ma.
Ty si Dobrý Pastier, hľadaj ma ako baránka, ako zablúdeného, neprehliadni ma.
Ty si potešenie, Ježišu, ty si môj Tvorca, v tebe, Spasiteľ, budem ospravedlnený.
Verš: Presvätá Trojica, Bože náš, zmiluj sa nad nami.
Ó, Trojica, Jeden, Bože! Zachráň nás od klamu, pokušení a nástrah.
Verš: Presvätá Bohorodička, zachráň nás!
Raduj sa, Lono, ktoré prijalo Boha, raduj sa, Pánov prestol, raduj sa, Matka nášho Života.
Pieseň 3b.
Irmos
Upevni Pane na kameni svojich prikázaní moje rozochvené srdce; lebo ty jediný si Svätý a Pán.
Verš: Zmiluj sa nado mnou, Bože, zmiluj sa nado mnou.
Našiel som prameň života teba, Premožiteľa smrti, a zo srdca ťa volám, na poslednú chvíľu: zhrešil som, očisť ma a zachráň.
Z čias Noeho, Spasiteľ, iba rozpustilých ľudí som napodobnil, a tým som získal aj ich odsúdenie vo vodách potopy.
Zhrešil som, Pane, zhrešil som pred tebou, očisť ma. Lebo niet tak hriešneho medzi ľuďmi, koho by som hriechmi neprekonal.
Dávneho Chama, otcovraha, napodobnila si, duša. Nezakryla si hanbu blížneho, neustúpila si s odvráteným zrakom.
Semovo požehnanie nezdedila si, úbohá duša, ani rozsiahly majetok ako Jafet nevlastníš v zemi odpustenia.
Vyjdi zo zeme Haran – z hriechu, duša moja, choď do zeme, kde pramení neporušenosť večného života, a ktorú zdedil Abrahám.
Počula si o Abrahámovi, duša moja, ako kedysi zanechal zem svojich otcov a stal sa prisťahovalcom; jeho voľbu napodobni.
Pri Mamreho dube patriarcha anjelov pohostil a tak získal v starobe predchuť prísľubu.
Novú obetu Izáka spoznala si, úbohá duša moja, tajomný celopal Pánovi; napodobni jeho voľbu.
Počula si o Izmaelovi, že bol vyhnaný ako dieťa otrokyne; vytriezvi, duša moja, a hľaď, nech neskončíš rovnako, pre svoju chlípnosť.
Dávnu Agaru Egypťanku napodobnila si, duša. Zvolila si si otroctvo a porodila si nového Izmaela – samoľúbosť.
Jakubov rebrík spoznala si, duša moja, siahajúci od zeme po nebo. Prečo si ho nevyužila na pevný vzostup v zbožnosti?
Božieho kňaza, osamoteného kráľa, obraz Kristovho života vo svete, medzi ľuďmi napodobňuj.
Nestaň sa soľným stĺpom, duša, neobracaj sa späť! Nech ťa odstraší príklad Sodomy! Zachráň sa hore v Segore.
Ako Lót pred požiarom, ujdi, duša moja, pred hriechom! Ujdi od Sodomy a Gomory, ujdi pred plameňom každej nerozumnej túžby.
Zmiluj sa, Pane, volám k tebe: zmiluj sa nado mnou, keď prídeš so svojimi anjelmi dať odplatu každému podľa jeho skutkov.
Neodmietni, Vládca, prosby tých, čo ťa ospevujú. Zľutuj sa, Milujúci človeka, a prosiacim s vierou daruj odpustenie .
Verš: Prepodobná matka Mária, pros Boha za nás.
Obklopila ma búrka a vlnobitie hriechov, matka; Ty sama ma teraz zachráň a zaveď do prístavu božského pokánia.
Verš: Prepodobná matka Mária, pros Boha za nás.
Prosebnú modlitbu prines aj teraz, Prepodobná, svojím príhovorom k súcitnej Bohorodičke; a otvor mi svoju bránu k Bohu.
Verš: Prepodobný otec Andrej, pros Boha za nás.
Svojimi modlitbami daruj aj mne odpustenie vín, ó, Andrej, predsedajúci Kréte, veď ty si pokánia najlepší znalec.
Sláva:
Jedna, jednoduchá, nestvorená, bezpočiatočná prirodzenosť, v Trojici hypostáz ospevovaná. Zachráň nás, ktorí sa s vierou klaniame tvojej moci.
I teraz:
Z Otca bez času, Syna v čase, Božia Rodička, bez účasti muža si porodila, dojčila – a, aký nezvyčajný zázrak!, Pannou si zostala.
Katavasia: Upevni Pane na kameni svojich prikázaní moje rozochvené srdce; lebo ty jediný si Svätý a Pán.
(Ak je prítomný kňaz, alebo diakon, prednáša malú ekténiu. Ak nie, berieme
Pane, zmiluj sa. (3x)
Sláva, i teraz: )
Znova a znova modlime sa v pokoji k Pánovi.
Ľud: Pane, zmiluj sa.
Zastaň sa a spas nás, zmiluj sa a zachráň nás, Bože, svojou milosťou.
Ľud: Pane, zmiluj sa.
Presvätú, prečistú, preblahoslavenú a slávnu Vládkyňu našu, Bohorodičku Máriu, vždy Pannu, i všetkých svätých spomínajúc, sami seba, druh druha i celý náš život Kristu Bohu oddajme.
Ľud: Tebe, Pane.
Kňaz: Lebo ty si náš Boh a my ti vzdávame slávu, Otcu i Synu, i Svätému Duchu, teraz i vždycky, i na veky vekov.
Ľud: Amen.
Sedalen, 8. hlas, podoben Voskrésl jesí iz mértvych
Pochodne Božej žiary, \* Spasiteľovi apoštoli, \* svieťte nám v temnote života; \* Nech teraz príkladne kráčame ako vo dne, \* a vo svetle zdržanlivosti unikáme pred nočnými vášňami, \*\* aby sme v radosti uzreli svetlé Kristove strasti.
Sláva, podoben Poveľínnoje tájno:
Bohom vybraná dvanástka apoštolov, \* prines teraz prosbu Kristovi, \* aby prešli bojiskom pôstu všetci \* v skrúšení konajúci modlitby \* a horlivo získavajúci čnosti. \* Nech takto napredujeme k videniu slávneho vzkriesenia Krista Boha, \*\* a vzdáme mu slávu a chválu.
I teraz, rovnaký podoben:
Neopísateľného Boha, Syna a Slovo, \* ktorý sa nad ľudské chápanie z teba narodil, \* pros, Bohorodička, spolu s apoštolmi, \* aby daroval svetu v čistote pokoj, \* a nám odpustil hriechy, \* skôr než príde koniec; \* aby svojich služobníkov urobil hodnými nebeského kráľovstva, \*\* pre svoju bezhraničnú dobrotu.
Trojpieseň – Triodion (bez poklôn)
Irmos
Počul som, Pane, tajomstvo tvojej prozreteľnosti; spoznal som tvoje diela a oslávil som tvoje božstvo.
Verš: Svätí apoštoli, proste Boha za nás.
Zdržanlivo žili osvietení Kristovi apoštoli, teraz nám čas zdržanlivosti stišujú božským príhovorom.
Dvanásťstrunná lýra zanôtila spásonosnú pieseň; božský chór učeníkov umlčal nápevy zloby.
Dažďom Ducha napojili ste všetko pod slnkom, zahnali ste, preblažení, súš mnohobožstva.
Verš: Presvätá Bohorodička, zachráň nás!
Bol som ponížený, zachráň ma, vysoko som o sebe zmýšľal; veď ty si porodila toho, ktorý povýšil poníženú prirodzenosť, prečistá Panna.
Irmos:
Pane, počul som tvoje tajomstvo a preľakol som sa, spoznal som tvoje diela a oslávil som tvoju moc, Vládca.
Verš: Svätí apoštoli, proste Boha za nás.
Prečestný zbor apoštolov, chválime ťa; modli sa a pros Tvorcu všetkých, aby sa zmiloval nad nami.
Stali ste sa roľníkmi, apoštoli Krista, a celý svet obrábate Božím slovom; prinášajte Mu ovocie v každom čase.
Stali ste sa vinohradom, ktorý si Kristus vskutku zamiloval, lebo prameň duchovného vína otvorili ste svetu, apoštoli.
Sláva:
Najvyššia, jednej podoby, najmocnejšia, Trojica svätá, Otec, Slovo a Duch Svätý; Boh, Svetlo a Život, chráň svoje stádo.
I teraz:
Raduj sa, ohnivý prestol, raduj sa, svietnik nosiaci Sviecu, raduj sa, vrch posvätenia, archa života, sieň nadovšetko svätá.
Ďalej už s poklonami
Pieseň 4.
Irmos:
Prorok počul o tvojom príchode a preľakol sa; že sa chceš narodiť z Panny a zjaviť sa ľuďom, a vravel: Počul som zvesť o tebe a preľakol som sa. Sláva tvojej moci. (2x)
Verš: Zmiluj sa nado mnou, Bože, zmiluj sa nado mnou.
Neprehliadaj svoje diela, neopúšťaj svoje stvorenie, spravodlivý Sudca. Hoci ja sám som ľudsky zhrešil viac ako všetci ľudia, Milujúci človeka, ty ako Pán všetkých, máš moc odpúšťať hriechy.
Blíži sa koniec, duša, blíži sa a ty nedbáš, ani sa nepripravuješ. Čas sa kráti, vstaň, Sudca sa blíži k dverám. Ako sen, ako kvet, tak sa čas života míňa – prečo sa nadarmo rozptyľujeme?
Vzpriam sa, duša moja, a svoje skutky, ktoré si konala, posudzuj; postav ich pred svoju tvár a vyroň kvapky sĺz. Statočne priznaj skutky a myšlienky Kristovi – a získaj ospravedlnenie.
V živote niet hriechu, ani skutku, ani zla, ktorými by som ja, Spasiteľ, nezhrešil, rozumom, slovom či rozhodnutím. Návrhom, súhlasom aj skutkom som zhrešil, ako dosiaľ nik iný.
Preto ma potrestalo, preto ma odsúdilo, mňa úbohého, moje svedomie, najneoblomnejšie na svete. Sudca môj, vyslobodil si ma, poznáš ma, ušetri, vysloboď a zachráň svojho služobníka.
Rebrík, ktorý kedysi uzrel najväčší z patriarchov, duša moja, ukazuje vzostup konania a výstup poznania. Ak teda chceš skutkami, v poznaní a nazeraní odteraz žiť, nechaj sa obnoviť.
Denný pot v núdzi znášal patriarcha. Mráz noci znášal, denne nahrádzal stratené. Pásol, namáhal sa a pracoval, aby obe svoje ženy získal.
Dve ženy, to pochop ako konanie a poznanie v duchovnom nazeraní. Liu – mnohodetnú, ako konanie, a Ráchel – námahou obťaženú, ako poznanie. Veď bez námahy sa ani konanie ani nazeranie, duša, nenapravia.
Bdej, duša moja! Vynikni ako najväčší z patriarchov, aby si získala konanie s poznaním, aby si sa stala umom vidiacim Boha. Tak v nazeraní dosiahneš nezachádzajúci oblak a staneš sa úspešným kupcom.
Dvanásť patriarchov najväčší z patriarchov splodil. Tajomne ti tým postavil rebrík, aby si skutkami stúpala nahor, duša moja. Deti ako stupne, priečky ako výstup, všemúdro ustanovil.
Znenávideného Ezaua nasledovala si, duša, prenechala si tomu, čo ťa udrel pätou, prvenstvo dávnej krásy a prišla si o otcovské požehnanie.
Dvakrát si sa pošmykla, úbohá, v konaní aj poznaní. Preto sa teraz kajaj!
Ezaua nazvali Edom, pre jeho krajnú náruživosť voči ženám. Stále roznecovaný nezdržanlivosťou a poškvrňovaný pôžitkami, dostal meno Edom, čo znamená rozpálenie duše milujúcej hriech.
O Jóbovi na hnojisku počula si, duša moja, že bol ospravedlnený. Jeho statočnosti si sa nevyrovnala, nemala si pevné odhodlanie vo všetkom, čo si spoznala, videla a okúsila; prejavila si sa ako netrpezlivá.
Kedysi bol na tróne, teraz je na hnojisku pokrytý vredmi. Mnohodetný a slávny zrazu je bez detí a bez domova. Palác na hnojisko a perly na rany sa zmenili.
Kráľovskou dôstojnosťou, diadémom a purpurom odetý; mnohomajetný človek, spravodlivý, prekypujúci bohatstvom a stádami. Náhle bohatstvo, slávu a kráľovstvo stratil a schudobnel.
Hoci bol spravodlivý, bezúhonný nad všetkých, neunikol pred úkladmi ľstivého a jeho sieťami. A ty, úbohá duša, milujúca hriech, čo urobíš, ak sa čosi nečakané stane a teba zasiahne?
Telo sa mi poškvrnilo, duch sa zašpinil, celý som pokrytý vredmi. Ale ty ako lekár, Kriste, oboje uzdrav mojím pokáním. Umy, očisť a vyper, učiň ich Spasiteľ čistejšími než sneh.
Svoje telo a krv, Ukrižovaný, dal si za všetkých, Slovo. Telo, aby si ma obnovil, krv, aby si ma obmyl. Ducha si odovzdal, aby si ma priviedol, Kriste, k svojmu Otcovi.
Uskutočnil si spásu uprostred zeme, Milosrdný, aby sme sa zachránili. Dobrovoľne si sa dal rozpäť na kríži, otvoril sa zavretý raj. Horné i dolné tvorstvo, i všetky národy, zachránené sa ti klaňajú.
Nech sa mi stane kúpeľom krv z tvojho boku, a tiež nápojom. Z neho pramení voda odpustenia, aby som bol dvojako očistený – pomazaním aj pitím. / Lebo pomazanie i nápoj, Slovo, sú tvoje životodarné slová.
Prišiel som o sieň, prišiel som aj o svadbu spolu s hostinou. Lampa zhasla bez oleja, sieň zamkli, kým som spal. Večeru zjedli, a mňa, s nohami a rukami v putách vyhodili von.
Cirkev čašu získala – tvoj životodarný bok. Z neho nám pramení dvojaký prúd odpustenia a poznania. Ako obraz starého a nového, spolu obidvoch zákonov, Spasiteľ náš.
Čas môjho života krátky je, naplnený bolesťou a zlobou. Ale prijmi ma v pokání a pozvi ma k poznaniu, aby som sa nestal ziskom ani pokrmom nepriateľa; ty sám, Spasiteľ, zľutuj sa nado mnou.
Teraz sa vystatujem, krutý som v srdci, bezdôvodne a márne. Neodsúď ma spolu s farizejom, ale daj mi skôr pokoru mýtnika, jediný Milosrdný, spravodlivý Sudca, a pripočítaj ma k nemu.
Zhrešil som a potupil som nádobu svojho tela; viem to, Milosrdný. Ale prijmi ma v pokání a pozvi ma k poznaniu, aby som sa nestal ziskom ani pokrmom nepriateľa; ty sám, Spasiteľ, zľutuj sa nado mnou.
Stal som sa sám sebe modlou, vášňami som svojej duši škodil. Ale prijmi ma v pokání a pozvi ma k poznaniu, aby som sa nestal ziskom ani pokrmom nepriateľa; ty sám, Spasiteľ, zľutuj sa nado mnou.
Nepočúval som tvoj hlas, neposlúchal som tvoje Písmo, Zákonodarca. Ale prijmi ma v pokání a pozvi ma k poznaniu, aby som sa nestal ziskom ani pokrmom nepriateľa; ty sám, Spasiteľ, zľutuj sa nado mnou.
Verš: Prepodobná matka Mária, pros Boha za nás.
Netelesné bytosti v tele si prekonala, Prepodobná, a prijala si skutočne veľkú milosť pred Bohom; zastávaj sa tých, čo si ťa verne ctia. Preto ťa prosíme, zo všetkých nástrah svojimi modlitbami nás vysloboď.
Verš: Prepodobná matka Mária, pros Boha za nás.
Zostúpila si do hlbín veľkých neprístojností, ale neudržali ťa. Rozbehla si sa s najlepším zámerom, obdivuhodne, k najvyššej cnosti zjavných skutkov. /Anjelský stav, Mária, si prekvapila.
Verš: Prepodobný otec Andrej, pros Boha za nás.
Andrej, chvála Otcov, neprestávaj prosiť vo svojich modlitbách, stojac pred Božskou Trojicou, nech nás zbaví múk, nás, ktorí k tebe s láskou ako božskému zástancovi voláme, ozdoba Kréty.
Sláva:
Nedeliteľný v prirodzenosti, nezliaty v osobách, nazývam ťa Bohom, jediné božstvo v Trojici, jediná vláda na spoločnom tróne. Spievam ti veľkú pieseň, ktorá sa na výsostiach trojnásobne spieva.
I teraz:
Rodíš a zároveň si uchovávaš panenstvo, ostávaš v oboch prípadoch prirodzenosťou Panna. Narodený obnovuje zákony prirodzenosti, počalo lono, ktoré ešte nerodilo. Kde Boh chce, podriaďuje sa prirodzený poriadok, lebo on koná tak, ako chce.
Katavasia: Prorok počul o tvojom príchode a preľakol sa; že sa chceš narodiť z Panny a zjaviť sa ľuďom, a vravel: Počul som zvesť o tebe a preľakol som sa. Sláva tvojej moci.
Pieseň 5.
Irmos:
Od noci bdiem, Milujúci človeka, osvieť ma, prosím, usmerni aj mňa k svojim príkazom a nauč ma, Spasiteľ, konať tvoju vôľu. (2x)
Verš: Zmiluj sa nado mnou, Bože, zmiluj sa nado mnou.
Ako v noci som neustále žil svoj život; stala sa mi totiž tmou a hustou hmlou noc hriechu. Ale urob zo mňa syna svetla, Spasiteľ.
Napodobnil som Rubena, ja úbohý, strojil som úklady ničomnosti a bezprávia, proti najvyššiemu Bohu. Poškvrnil som svoje lôžko tak, ako on otcovo.
Vyznávam sa ti, <NAME>ľu: zhrešil som; zhrešil som, ako kedysi bratia, čo predali Jozefa; plod čistoty a rozvážnosti.
Súrodenci zviazali spravodlivú dušu; nechali predať do otroctva ľúbezného, ako predobraz Pána. Ty tiež si sa, duša, celá zapredala svojou zlobou.
Spravodlivého Jozefa, rozvážny um napodobňuj, úbohá, neskúsená duša, a nepoškvrňuj sa nerozumnými žiadosťami; nekonaj stále bezprávie.
Hoci Jozef kedysi prebýval v jame, Vládca, Pane, bolo to predobrazom tvojho pohrebu a vzkriesenia. Či aj ja niekedy podobné dokážem tebe priniesť?
Počula si o Mojžišovom košíku, duša, vodami, vlnami rieky unášanom; ako v podpalubí takto kedysi utekal pred krutým výkonom faraónovho rozsudku.
Počula si o babiciach, ktoré kedysi zabíjali každého nedospelého muža, teda skutky striedmosti, úbohá duša; teraz ako veľký Mojžiš saj Múdrosť.
Ako veľký Mojžiš, egyptskú myseľ len si zranila, úbohá duša ale nezabila; povedz, ako sa chceš teraz usídliť do púšte žiadostí v pokání?
V púšti sa usídlil veľký Mojžiš; choď teda a nasleduj jeho život, duša, aby ti bolo dané aj duchovné nazeranie na Božie zjavenie v kríku.
Mojžišovu palicu predstav si, duša, ako udiera more a tuhnú hlbiny pred obrazom božského Kríža. S ním môžeš aj ty konať veľké veci.
Áron priniesol Bohu oheň, bezúhonný a poctivý. Ale Ofni a Fines, - ako aj ty, duša! – prinášali od Boha vzdialený, život poškvrnený.
Oťažel som, Pane, ako krutý faraón svojím zmýšľaním; ako Jannes a Jambres dušou a telom. Zaplavilo mi rozum; ale ty mi pomôž.
Znečistil som sa od bahna, ja úbohý mysľou; umy ma, Vládca, kúpeľom mojich sĺz, prosím ťa. Odev môjho tela učiň bielym ako sneh.
Keď skúmam svoje skutky, Spasiteľ, vidím seba samého, ako prekonávam hriechmi každého človeka. Lebo uvažujem s poznaním, nezhrešil som z nevedomosti.
Ušetri, Pane, ušetri svoje stvorenie. Zhrešil som, prepáč mi, lebo v prirodzenosti čistý len ty si jediný a nik iný okrem teba nie je bez škvrny.
Ty si Boh a kvôli mne vzal si na seba môj obraz; vykonal si zázraky, uzdravil si malomocných, ochrnutého si vzpriamil, zastavil si žene krvotok, Spasiteľ, po dotyku rúcha.
Napodobni ženu s krvotokom, úbohá duša, pribehni a uchop lem Kristovho odevu, nech ťa zbaví rán a počuješ jeho slová: „Tvoja viera ťa zachránila!”
K zemi zhrbenú ženu napodobňuj, duša, poď a padni k Ježišovým nohám, nech ťa narovná, aby si kráčala priamo po Pánových cestách.
Hoci si, Vládca, hlbokou studňou, daruj mi tečúcu vodu zo svojich prečistých prameňov; nech ako Samaritánka pijem a nie som smädný. Lebo z teba pramenia prúdy života.
Nech sa mi stanú ako Siloe moje slzy, Vládca, Pane, nech si aj ja umyjem zreničky srdca, aby som duchovne videl teba, predvečné Svetlo.
Verš: Prepodobná matka Mária, pros Boha za nás.
Prekypujúca nesmiernou túžbou chcela si sa pokloniť stromu Života, a bolo ti to dané. Urob aj mňa hodným získať slávu zhora.
Verš: Prepodobná matka Mária, pros Boha za nás.
Prekročila si vody Jordánu a našla si odpočinutie bez bolesti, keď si ušla od telesných pôžitkov. Aj nás od nich osloboď svojimi modlitbami, Prepodobná.
Verš: Prepodobný otec Andrej, pros Boha za nás.
Ako výnimočného pastiera, múdry Andrej, a ako vyvoleného, s veľkou láskou a bázňou prosím, na tvoj príhovor, nech dosiahnem spásu a večný život.
Sláva:
Oslavujeme ťa, Trojica, jediného Boha: svätý, svätý, svätý si, Otče, Synu a Duchu, jednoduché Bytie, Jeden, tebe sa ustavične klaniame.
I teraz:
Matka a Panna, bez muža, neporušená, skrze teba do mojej zemitosti obliekol sa Boh, Tvorca vekov; a zjednotil so sebou prirodzenosť človeka.
Katavasia: Od noci bdiem, Milujúci človeka, osvieť ma, prosím, usmerni aj mňa k svojim príkazom a nauč ma, Spasiteľ, konať tvoju vôľu.
Pieseň 6.
Irmos:
Volal som z celého svojho srdca k milosrdnému Bohu; On ma začul z najhlbšieho podsvetia a pozdvihol z rozkladu môj život. (2x)
Verš: Zmiluj sa nado mnou, Bože, zmiluj sa nado mnou.
Slzy mojich očí, Spasiteľ, a vzdychanie z hlbín prinášam ti s čistým úmyslom a v srdci volám: Bože, zhrešil som proti tebe, očisť ma.
Odklonila si sa, duša, od svojho Pána, ako Dátan a Abíram. Ale „Ušetri ma!“, volaj z najhlbšieho podsvetia aby ťa priepasť zeme nezakryla.
Ako jalovica vzdorovitá, duša, napodobnila si Efraima. Ako srna z osídel zachráň si život, daj svojej mysli krídla skutkami a nazeraním.
Nech nás ruka Mojžišova ubezpečí, duša, že Boh môže malomocný život obieliť a očistiť. Nezúfaj nad sebou, hoci tiež si malomocná.
Vlny mojich hriechov, Spasiteľ, ako v Červenom mori, vracajú sa späť a náhle ma zakryli, ako kedysi Egypťanov a ich veliteľov.
Nerozumné ciele si, duša, sledovala, ako kedysi Izrael. Pred božskou mannou si totiž dala nerozumne prednosť pôžitkárskemu obžerstvu vášní.
Studne kanaánskeho zmýšľania uprednostnila si, duša, pred skalným prameňom, z ktorého Múdrosť akoby čašou nalieva prúdy bohoslovia.
Bravčové mäso, plné kotly a egyptskú stravu, pred stravou nebeskou uprednostnila si, duša moja, ako kedysi nerozumný ľud na púšti.
Keď Mojžiš, tvoj služobník, udrel obrazne skalu palicou, naznačil predobraz tvojho životodarného boku. Z neho všetci čerpáme nápoj života, Spasiteľ.
Skúmaj, duša, a pozeraj ako <NAME>, hľaď ako vyzerá zem zasľúbená. Usídli sa v nej vernosťou Zákonu.
Vstaň a napadni, ako <NAME>, telesné vášne; a ako Gabaonitov ľstivé pokušenia; vždy ich premáhaj.
Prejdi cez pominuteľnú prirodzenosť času, ako kedysi Archa, a zem prevezmi do vlastníctva podľa zasľúbenia, duša; tak prikazuje Boh.
Ako si zachránil volajúceho Petra, príď mi v ústrety a zachráň ma, Spasiteľ, vystri svoju ruku, osloboď ma od šelmy; a vyveď ma z hlbiny hriechu.
Poznám ťa ako pokojný prístav, Vládca, Vládca Kriste, Z bezodných hlbín hriechu a od beznádeje, ponáhľaj sa ma zachrániť.
To ja som tá kráľovská drachma, Spasiteľ, ktorú si kedysi stratil. Zažni však pochodeň, svojho Predchodcu, Slovo, hľadaj a nájdi svoj obraz.
Verš: Prepodobná matka Mária, pros Boha za nás.
Aby si uhasila plameň vášní, ronila si kvapky sĺz, Mária, neustále v duši rozpaľovaná. Tú milosť daruj aj mne, tvojmu služobníkovi.
Verš: Prepodobná matka Mária, pros Boha za nás.
Získala si nebeskú slobodu od vášní dôkladným pozemským životom, matka. Preto pros, na tvoj príhovor, za oslobodenie od vášní tých, čo ťa ospevujú.
Verš: Prepodobný otec Andrej, pros Boha za nás.
Ty si Krétsky pastier a predstaviteľ, zástanca sveta. S vedomím toho utiekam sa k tebe, Andrej, a volám ťa: vytiahni ma, otče, z hlbiny hriechu.
Sláva:
Som Trojica, jednoduchá, nedeliteľná, rozlíšená v osobách; Som však aj Jedno, v prirodzenosti zjednotené – tak hovorí Otec, i Syn i Božský Duch.
I teraz:
Tvoje lono zrodilo nám Boha, ktorý prijal náš obraz. Jeho, Tvorcu všetkých pros, Bohorodička, nech nás na tvoj príhovor ospravedlní.
Katavasia: Volal som z celého svojho srdca k milosrdnému Bohu; On ma začul z najhlbšieho podsvetia a pozdvihol z rozkladu môj život.
(Ak je prítomný kňaz, alebo diakon, prednáša malú ekténiu. Ak nie, berieme
Pane, zmiluj sa. (3x)
Sláva, i teraz: )
Znova a znova modlime sa v pokoji k Pánovi.
Ľud: Pane, zmiluj sa.
Zastaň sa a spas nás, zmiluj sa a zachráň nás, Bože, svojou milosťou.
Ľud: Pane, zmiluj sa.
Presvätú, prečistú, preblahoslavenú a slávnu Vládkyňu našu, Bohorodičku Máriu, vždy Pannu, i všetkých svätých spomínajúc, sami seba, druh druha i celý náš život Kristu Bohu oddajme.
Ľud: Tebe, Pane.
Kňaz: Lebo ty si Kráľ pokoja a Spasiteľ našich duší a my ti vzdávame slávu, Otcu i Synu, i Svätému Duchu, teraz i vždycky, i na veky vekov.
Ľud: Amen.
Kondák, 6. hlas
Duša moja, duša moja, vstaň! Prečo spíš? \* Blíži sa koniec a ty sa máš prečo znepokojovať. \* Preber sa teda, nech ťa ušetrí Kristus Boh; \*\*on je všade a všetko napĺňa.
Ikos
Keď videl Kristovu liečebňu otvorenú, ako z nej Adamovi pramení zdravie, diabol trpel a bol zranený, vo svojej biede nariekal, a svojim druhom volal: Ako si poradím s Máriiným synom? Zabíja ma ten Betlehemčan; on je všade a všetko napĺňa.
Blaženstvá
Hlas 6, s poklonami:
Pane, spomeň si na nás, keď prídeš do svojho kráľovstva.
Zločinca, ktorý ti, Kriste, volal na kríži: “Spomeň si na mňa,” urobil si obyvateľom raja. Jeho pokánia učiň hodným aj mňa, nehodného.
Blažení chudobní v duchu, lebo ich je nebeské kráľovstvo.
Počula si o Manuovi, duša moja, ako sa mu kedysi zjavil Boh a z neplodnej vtedy prijal plod prisľúbenia. Napodobňuj jeho zbožnosť.
Blažení plačúci, lebo oni budú potešení.
Vyrovnala si sa Samsonovej nedbanlivosti, nechala si si ostrihať slávu tvojich skutkov; pre lásku k pôžitkom, duša, prenechala si nepriateľom, rozvážny a blažený život.
Blažení tichí, lebo oni budú dedičmi zeme.
Kedysi osľou čeľusťou porazil cudzincov, ale teraz sa ocitol v zajatí vášnivého pôžitkárstva. Ale utekaj, duša moja, pred takým príkladom, konaním a slabosťou.
Blažení hladní a smädní po spravodlivosti, lebo oni budú nasýtení.
Barak a Jefte, vojvodcovia, sudcovia Izraela, požívali úctu, a s nimi aj odvážna Debora. Opri sa o ich šľachetnosť, duša, a aj ty sa vzchop a upevni.
Blažení milosrdní, lebo oni dosiahnu milosrdenstvo.
Spoznala si, duša moja, odvahu Jahely, ktorá sa postarala o záchranu, keď kedysi prebodla Sísara ostrým drevom – počuješ to! – tým sa ti predobrazuje Kríž.
Blažení čistého srdca, lebo oni uvidia Boha.
Obetuj, duša, pochvalnú žertvu, prines skutky ako dcéru, čistejšiu než tá Jefteho. Zabi ako obeť svojmu Pánovi telesné vášne.
Blažení tí, čo šíria pokoj, lebo ich budú volať Božími synmi.
Pomysli na Gedeonovo rúno, duša moja, prijmi rosu z neba, zohni sa ako pes a pi vodu zo Zákona prameniacu pod váhou napísaného.
Blažení prenasledovaní pre spravodlivosť, lebo ich je nebeské kráľovstvo.
Odsúdenie kňaza Héliho, dostalo sa aj tebe, duša moja; z nedostatku rozumu si nadobudla vášne, podobne ako on deti, aby konali neprávosti.
Blažení ste, keď vás budú pre mňa potupovať a prenasledovať a všetko zlé na vás nepravdivo hovoriť.
V knihe S<NAME> rovným dielom svoju ženu dvanástim pokoleniam rozdelil, duša moja; aby obžaloval zločin, Benjamínovu vinu.
Radujte sa a jasajte, lebo máte hojnú odmenu v nebi.
S láskou k Múdrosti sa Anna modlila, pohybovala perami na chválu, ale jej hlas počuť nebolo. A hoci bola neplodná, porodila syna hodného tej modlitby.
Pane, spomeň si na nás, keď prídeš do svojho kráľovstva.
Medzi sudcov sa zaradilo dieťa Anny, veľký Samuel, vychovávaný v Ramataime, v Pánovom dome. Jeho nasleduj, duša moja, a skôr než iných, súď vlastné skutky.
Vládca, spomeň si na nás, keď prídeš do svojho kráľovstva.
Dávida, vyvoleného za kráľa, kráľovsky pomazali, z rohu božského myra. Ty však, duša moja, ak túžiš po kráľovstve zhora, pomaž sa myrom sĺz.
Svätý, spomeň si na nás, keď prídeš do svojho kráľovstva.
Zmiluj sa nad svojím stvorením, Milosrdný, zľutuj sa nad dielom svojich rúk. Ušetri všetkých, ktorí zhrešili, a aj mňa, ktorý som viac než ostatní prehliadal tvoje prikázania.
Sláva:
Bezpočiatočný, Rodenie a Pochádzanie; rodiacemu Otcovi sa klaniam; zrodeného Syna oslavujem, ospevujem vyžarujúceho spolu s Otcom a Synom – Svätého Ducha.
I teraz:
Tvojmu nadprirodzenému pôrodu sa klaniame, slávu tvojho dieťaťa medzi prirodzenosti nedelíme, Božia rodička. On je totiž jeden v osobe, ale vyznávame ho v dvojici prirodzeností.
Pieseň 7.
Irmos
Zhrešili sme, porušili sme zákon, konali sme neprávosti pred tebou. Ani sme nezachovali, ani sme nekonali to, čo si nám prikázal. Ale nezriekni sa nás navždy, Bože otcov. (2x)
Verš: Zmiluj sa nado mnou, Bože, zmiluj sa nado mnou.
Zhrešil som, previnil som sa, odvrhol som tvoje prikázanie. Lebo hriechmi som sa nechal zviesť a priložil som ďalšie rany k svojim podliatinám. Ale ty sám sa zmiluj nado mnou ako milosrdný, Bože otcov.
Tajnosti svojho srdca vyznal som pred tebou, mojím Sudcom. Hľaď na moje poníženie, hľaď na moje trápenie, všimni si teraz môj súdny spor. Ty sám sa zmiluj nado mnou ako milosrdný, Bože otcov.
Saul kedysi, keď stratil oslíčatá svojho otca, duša, nečakane našiel pomazanie za kráľa. Ty však dávaj pozor, nepozabudni sa, svoje dobytčie žiadosti neuprednostňuj pred Kristovým kráľovstvom.
Dávid, praotec, kedysi dvojnásobne zhrešil, duša moja, ale zasiahol ho šíp smilstva, premohla ho kopija trestu za vraždu. A ty sama pre najťažšie skutky strádaš, pre vedomé náklonnosti.
Dávid kedysi spojil s neprávosťou neprávosť, k vražde pridal smilstvo, no hneď na to dvojité pokánie prejavil. A ty sama, duša, to najhoršie si vykonala a nekajala si sa pred Bohom.
Dávid kedysi zostavil, ako na ikone zobrazil, pieseň, ktorou pomenoval svoje skutky, a volal: Zmiluj sa nado mnou! Zhrešil som totiž proti tebe samému, Bohu všetkých; ty sám ma očisť.
Keď Archu prevážali na voze, vtedy istý Oza, len sa jej dotkol, keď sa dobytča prevracalo, a okúsil Boží hnev. Od jeho trúfalosti utekaj, duša, dôstojne si cti to, čo je Božie.
Počula si o Absolónovi, ako sa postavil proti prirodzenosti? Spoznala si jeho hanebné skutky, ktorými poškvrnil lôžko otca Dávida. A ty si nasledovala jeho vášnivé a pôžitkárske hnutia.
Podriadila si svoju nezotročenú dôstojnosť svojmu telu. Našla si iného Achitofela, nepriateľa, duša, a priľnula si k jeho radám. Ale sám Kristus ich rozptýlil, aby si sa napriek všetkému zachránila.
Zázračný Šalamún, hoci plný daru múdrosti, predsa aj to, čo je zlé pred Bohom, kedysi vykonal a od neho odstúpil. A ty si sa mu svojím zlorečeným životom, duša, pripodobnila.
Vlečený pôžitkami svojich vášní sa poškvrňoval. Beda! – z ctiteľa múdrosti je ctiteľ smilníc, odcudzený Bohu. A ty si ho nasledovala mysľou, duša, poškvrnenými pôžitkárskymi vášňami.
Nasledovala si Roboama, ktorý neposlúchol otcovskú radu, zároveň aj najhoršieho služobníka Jeroboama, dávneho odpadlíka, duša; Vyhni sa ich napodobňovaniu a volaj k Bohu: Zhrešil som, zľutuj sa nado mnou!
Nasledovala si Achaba, jeho vinu za krv, duša moja, beda mi! Stala si sa príbytkom telesných škvŕn a hanebnou nádobou vášní. Ale zo svojej hĺbky povzdychni a vyznaj Bohu svoje hriechy.
Eliáš kedysi spálil dve Jezabeline päťdesiatky, a predtým hanebných prorokov pobil na svedectvo proti Achabovi. Stráň sa nasledovania tých dvoch, duša, a vzchop sa.
Zatvorilo sa ti nebo, duša, a hlad Boží na teba doľahol, keď si sa nepodriadila, ako kedysi Achab, slovám Tesbana Eliáša. Ty však napodobni Sarepťanku a nakŕm prorokovu dušu.
Zhromaždila si Manasesove viny svojím rozhodnutím, keď si ako modly postavila vášne a rozmnožila si, duša, ohavnosť. Ty však vrúcne napodobni aj jeho pokánie a získaj skrúšenosť.
Padám pred tebou a prinášam ti miesto sĺz svoje slová: Zhrešil som viac než smilnica, a konal som neprávosti ako nik iný na zemi. Ale zľutuj sa, Vládca, nad svojím stvorením a privolaj ma späť.
Tvoj obraz som pochoval, porušil som tvoje prikázanie. Potemnela všetka krása a vášňe uhasili svetlo, Spasiteľ. Ale zľutuj sa a daruj mi – ako spieva Dávid – radostné jasanie.
Obráť sa, kajaj sa, odhaľ, čo je skryté. Povedz vševidiacemu Bohu: Ty poznáš moje tajnosti, jediný Spasiteľ. ale ty sám sa zmiluj nado mnou – ako spieva Dávid – podľa svojho milosrdenstva.
Moje dni sa minuli ako sen po prebudení. Preto ako Ezechiáš slzím na svojom lôžku, nech sa mi pridá k rokom života. Lenže aký Izaiáš zastane pred tebou, duša, ak len nie Boh všetkých?
Verš: Prepodobná matka Mária, pros Boha za nás.
Volala si k prečistej Božej matke; najprv si však odhodila zúrivosť dorážajúcich vášní, a zahanbila si útočiaceho nepriateľa. Daruj teraz pomoc v trápení aj mne, svojmu služobníkovi.
Verš: Prepodobná matka Mária, pros Boha za nás.
Zamilovala si si Krista, zatúžila si po ňom, pre neho si sužovala telo, prepodobná; Pros ho teraz za služobníkov, nech je milosrdný k nám všetkým, nech daruje pokojné podmienky tým, ktorí si ho ctia.
Verš:Prepodobný otec Andrej, pros Boha za nás.
Na kameni viery upevni ma, otče, svojimi modlitbami. Ohraď ma Božou bázňou, a daruj mi teraz pokánie, Andrej. Prosím ťa, vysloboď ma zo siete nepriateľov, ktorí ma prenasledujú.
Sláva:
Trojica jednoduchá, nedeliteľná, jednopodstatná, jediná prirodzenosť. Svetlá a Svetlo, Sväté Tri a jediná Svätosť – tak ospevujeme Boha, Trojicu. Ty však ospevuj a oslavuj Život a Životy, duša, Boha všetkých.
I teraz:
Ospevujeme ťa, dobrorečíme ti, klaniame sa ti, Božia rodička; lebo si porodila z nedeliteľnej Trojice jediného Krista, Boha. Ty sama si nám na zemi, otvorila nebesá.
Katavasia: Zhrešili sme, porušili sme zákon, konali sme neprávosti pred tebou. Ani sme nezachovali, ani sme nekonali to, čo si nám prikázal. Ale nezriekni sa nás navždy, Bože otcov.
Trojpieseň – Triodion (bez poklôn)
Irmos:
Bezpočiatočného Kráľa Slávy, pred ktorým sa chvejú nebeské mocnosti: ospevujte, kňazi, vyvyšujte, ľudia, po všetky veky.
Verš: Svätí apoštoli, proste Boha za nás.
Ako uhlíky nehmotného ohňa, spáľte moje hmotné vášne; a zažnite vo mne teraz túžbu po Božskej láske, apoštoli.
Poľnice Slova ľúbozvučné! Uctime si ich, vďaka nim padli hradby nepriateľa nepevné; oni vztýčili bašty božského poznania.
Domýšľavosť mojej vášnivej duše, rozdrvte! Veď ste chrámy a stĺpy nepriateľa rozdrvili; apoštoli Pánovi, posvätené chrámy.
Verš: Presvätá Bohorodička, zachráň nás.
Pojala si Nepojateľného prirodzenosťou, nosila si Nosiaceho všetko, dojčila si, Čistá, Sýtiaceho tvorstvo, Krista, Darcu života.
Verš: Svätí apoštoli, proste Boha za nás.
Staviteľským umením Ducha vybudovali ste celú cirkev, Kristovi apoštoli, dobrorečte v nej Kristovi naveky.
Zatrúbili na poľnicu učenia, apoštoli zvrhli nadol všetku modlársku lesť; vyvyšujúc Krista po všetky veky.
Apoštoli, dobre ste sa usídlili; dozorcovia sveta a nebies obyvatelia, vysloboďte z bied tých, čo vás ustavične chvália.
Sláva:
Trojslnečná, všesvetlá Božia vláda, jednomyseľná, jednoprestolná prirodzenosť; Otče všemocný, Synu a božský Duchu, ospevujem ťa naveky.
I teraz:
Ako čestný a najvyšší prestol neprestajne ospevujme, ľudia, Božiu Matku jedinú po pôrode matku aj pannu.
Ďalej už s poklonami
Pieseň 8.
Irmos:
Nebeské vojská Ho oslavujú a chvejú sa pred ním cherubíni a serafíni; všetko, čo dýcha, celé tvorstvo, ospevuj ho, dobroreč mu a vyvyšuj ho po všetky veky. (2x)
Verš: Zmiluj sa nado mnou, Bože, zmiluj sa nado mnou.
Zhrešil som, Spasiteľ, zmiluj sa, pozdvihni môj um k obráteniu. Prijmi kajúceho, zľutuj sa nad volajúcim: Zhrešil som pred tebou, zachráň ma! Previnil som sa, zmiluj sa nado mnou!
Vozataj Eliáš vystúpil vozom čností až na nebesá, vzniesol sa kedysi ponad pozemské veci. Ty, duša moja, o jeho výstupe uvažuj.
Jordánsky prúd kedysi Eliášovým plášťom Elizeus oddelil na dve strany. /Ty však, duša moja, nemáš účasť na tomto dare, pre nezdržanlivosť.
Keď Elizeus kedysi prijal Eliášov plášť, získal tým dvojnásobný dar od Boha. /Ty však, duša moja, nemáš účasť na tomto dare, pre nezdržanlivosť.
Sunamitka kedysi spravodlivého pohostila, ó duša, s dobrým úmyslom. Ty si však neubytovala ani cudzinca ani pútnika. Preto ťa zo Siene vyhodia von s plačom.
Giéziho poškvrnené zmýšľanie stále napodobňuješ, úbohá duša. Jeho chamtivosť odlož aspoň v starobe, unikaj pred gehenským ohňom, vzdiaľ sa od svojej zloby.
Ty si Oziáša napodobnila, duša, a jeho malomocenstvo dvojmo si získala. Neprístojne totiž zmýšľaš a neprávosti konáš. Zanechaj to, čo máš, a ponáhľaj sa k pokániu.
O Ninivčanoch počula si, duša, ako sa kajali pred Bohom vo vrecovine a popole. Nenasledovala si ich, ale ukázala si sa horšia ako všetci, ktorí pred Zákonom aj po Zákone zhrešili.
Počula si, duša, ako Jeremiáš v blatovej jame s nárekom volal na Sionské mesto a hľadal slzy; nasleduj jeho trúchlivý život a zachrániš sa.
Jonáš do Taršíša ušiel, keď predvídal obrátenie Ninivčanov. Spoznal totiž ako prorok Božiu dobrotivosť, a preto horlil, aby proroctvo nezlyhalo.
Počula si o Danielovi v jame, ako zavrel tlamy zverov, duša. Spoznala si, ako mládenci s Azariášom vierou uhasili horiaci plameň pece.
Zo Starého zákona všetko predložil som ti, duša, ako príklad: napodobni spravodlivých bohumilé skutky a naopak, utekaj od hriechov tých skazených.
Spravodlivý sudca, Spasiteľ, zmiluj sa, zbav ma ohňa a hrozieb, ktoré mám na súde právom podstúpiť. Prepáč mi, na poslednú chvíľu, pre čnosti a pokánie.
Ako zločinec volám k tebe: Spomeň si na mňa. Ako Peter trpko plačem: Prepáč mi, Spasiteľ. Volám ako mýtnik, roním slzy ako smilnica. Prijmi môj nárek, ako kedysi od Kanaánčanky.
Uzdrav, Spasiteľ, hnilobu mojej úbohej duše, ty jediný lekár. Prilož mi náplasť, olej a víno – skutky pokánia, skrúšenosť a slzy.
Kanaánčanku aj ja napodobňujem, zmiluj sa, volám, Synu Dávidov. Dotýkam sa lemu rúcha ako žena s krvotokom, plačem ako Marta a Mária nad Lazárom.
Sklenicu sĺz, Spasiteľ, ako myro lejem ti na hlavu, volám ťa ako smilnica, hľadám zmilovanie. Prinášam ti modlitbu a prosím, nech sa mi dostane odpustenie. Hoci nik nezhrešil proti tebe tak, ako ja, predsa len prijmi aj mňa, dobrotivý Spasiteľ; V bázni sa kajám a s láskou volám: Proti tebe samému som zhrešil, zmiluj sa nado mnou, Milosrdný.
Ušetri, Spasiteľ, svoje stvorenie, a hľadaj ako pastier to, čo sa stratilo. Zblúdenú ovečku vytrhni vlkovi, postaraj sa o mňa v stáde svojich oviec.
Keď zasadneš, Sudca, ako dobrotivý, a zjavíš svoju hrozivú slávu, Spasiteľ, ó, aká hrôza nastane! – keď sa rozpáli pec a všetci sa budú báť tvojho prísneho súdu.
Verš: Prepodobná matka Mária, pros Boha za nás.
Matka nezapadajúceho Svetla osvietila ťa a z temnoty vášní oslobodila. Vošla si tak do milosti Ducha, preto daj svetlo, Mária, tým, čo ťa verne zvelebujú.
Verš: Prepodobná matka Mária, pros Boha za nás.
Nový, vskutku Boží zázrak uzrel Zosima v tebe, Matka, a žasol. Videl totiž anjela v tele, a hrôzou celkom preniknutý ospevoval Krista naveky.
Verš: Prepodobný otec Andrej, pros Boha za nás.
Ty máš odvahu pred Pánom, <NAME>, čestná ozdoba, prosím, modli sa za uvoľnenie pút neprávosti; teraz na tvoj príhovor, Učiteľ, Sláva prepodobných.
Verš: Zvelebujeme Otca i Syna i Svätého Ducha, Pána.
Bezpočiatočný Otče, spolubezpočiatočný Synu, dobrotivý Utešiteľu, priamy Duchu. Pôvodca Božieho Slova, Slovo bezpočiatočného Otca, živý a tvoriaci Duch; Trojica a Jedno, zmiluj sa nado mnou.
I teraz:
Ako z purpurovej farby, Prečistá, utkal sa duchovný plášť – telo Emanuela, vo vnútri tvojho lona. Preto ťa ako Bohorodičku pravdivo uctievame.
Verš: Chválime, dobrorečíme a klaniame sa Pánovi, ospevujeme a vyvyšujeme ho po všetky veky.
Katavasia: Nebeské vojská Ho oslavujú a chvejú sa pred ním cherubíni a serafíni; všetko, čo dýcha, celé tvorstvo, ospevuj ho, dobroreč mu a vyvyšuj ho po všetky veky.
Diakon: Bohorodičku a Matku Svetla piesňami zvelebujme.
Velebí moja duša Pána \* a môj duch jasá v Bohu, mojom Spasiteľovi.
Po každom verši spievame:
Čestnejšia si ako cherubíni \* a neporovnateľne slávnejšia ako serafíni, \* bez porušenia si porodila Boha Slovo, \* opravdivá Bohorodička, velebíme ťa.
Lebo zhliadol na poníženosť svojej služobnice, \* hľa, od tejto chvíle blahoslaviť ma budú všetky pokolenia.
Lebo veľké veci mi urobil ten, ktorý je mocný, a sväté je jeho meno. \* A jeho milosrdenstvo z pokolenia na pokolenie s tými, čo sa ho boja.
Ukázal silu svojho ramena, \* rozptýlil tých, čo v srdci pyšne zmýšľajú.
Mocnárov zosadil z trónov a povýšil ponížených. \* Hladných nakŕmil dobrotami a bohatých prepustil naprázdno.
Ujal sa Izraela, svojho služobníka, lebo pamätá na svoje milosrdenstvo, \* ako sľúbil našim otcom, Abrahámovi a jeho potomstvu naveky.
Trojpieseň – Triodion (bez poklôn)
Irmos:
Nadovšetko ako Bohorodičku ťa vyznávame; ty si nás zachránila, Panna čistá; s beztelesnými zbormi ťa velebíme.
Verš: Svätí apoštoli, proste Boha za nás.
Stali ste sa prameňmi spásonosnej vody; zvlažte, apoštoli, moju dušu, roztavenú hriešnym smädom.
Plavím sa v hlbinách záhuby, už som celkom zatopený – svojou pravicou ma ako Petra, Pane, zachráň.
Ste ako soľ chutného učenia; hnilobu mojej mysle vysušte a tmu nevedomosti odožeňte.
Verš: Presvätá Bohorodička, zachráň nás.
Radosť si porodila, daruj mi plač, ktorým božskú útechu, Vládkyňa, v nadchádzajúcom dni dokážem nájsť.
Iný irmos:
Teba, prostrednicu medzi nebom a zemou, všetky pokolenia velebia. Telom sa totiž v tebe, Panna, usídlila plnosť Božstva.
Verš: Svätí apoštoli, proste Boha za nás.
Preslávený zbor apoštolov, velebíme ťa piesňami; odháňate poblúdenie a stali ste sa žiarivými svetlami sveta.
Svojou sieťou dobrej zvesti ulovili ste duchovné ryby, prinášajte ich vždy Kristovi ako odmenu, blažení apoštoli.
Vo vašich modlitbách k Bohu pamätajte na nás, apoštoli; s láskou vás ospevujeme a prosíme, zbavte nás každého pokušenia.
Sláva:
Teba, trojhypostázne Jedno, Otče, Synu s Duchom, jediného, jednopodstatného Boha, ospevujeme; Trojica jednomocná a bezpočiatočná.
I teraz:
Teba, Rodičku a Pannu, vo všetkých pokoleniach velebíme, lebo ty si nás oslobodila spod kliatby, Radosť si nám totiž porodila – Pána.
Ďalej už s poklonami
Pieseň 9.
Irmos
Po panenskom počatí neopísateľný pôrod, z Matky bez muža neporušiteľný plod, Božie narodenie, obnovuje prirodzenosť. Preto ťa vo všetkých pokoleniach ako Matku, Božiu nevestu, pravoverne velebíme. (2x)
Verš: Zmiluj sa nado mnou, Bože, zmiluj sa nado mnou.
Myseľ je zranená, telo zoslablo, ochorel duch, slovo stratilo moc, život sa umŕtvil, koniec je pred dverami. Úbohá moja duša, čo urobíš, keď príde Sudca skúmať tvoje veci?
Priblížil som ti Mojžišov Pôvod sveta, duša, a všetko, čo vložil do Zákona – Písmo, ktoré ti hovorí o spravodlivých a nespravodlivých. Z nich, duša, si tých druhých nasledovala, a nie prvých, a pred Bohom si zhrešila.
Zákon je bez sily, ďaleko je Dobrá zvesť, celé Písmo nechávaš nepovšimnuté; ochabli Proroci a každé spravodlivé slovo. Tvoje rany, duša, sa rozmnožili, keď nebolo lekára, ktorý by ťa liečil.
Z nového Písma ponúknem ti príklady ktoré ťa uvedú, duša, do skrúšenosti; len nasleduj spravodlivých, a odvráť sa od hriešnych. Získaj Kristovo zmilovanie modlitbami a pôstom, čistotou a bezúhonnosťou.
Kristus sa stal človekom a k pokániu pozval zločincov so smilnicami; kajaj sa, duša! Už sa otvorili dvere kráľovstva, a pred tebou sa ho zmocňujú farizeji, mýtnici a cudzoložníci – ktorí sa kajajú.
Kristus sa stal človekom, pripodobnil sa mi telom, a všetko, čo patrí k prirodzenosti, podľa svojej vôle naplnil– okrem hriechu. Prednaznačil ti, duša, vzor a obraz svojho zostúpenia.
Kristus spasil mágov, zvolal pastierov, množstvo mládencov sa stalo mučeníkmi; oslávil starcov i staré vdovy. A ty si nenasledovala, duša, ani ich skutky ani život. Ale beda ti, keď budeš súdená.
Pán sa postil štyridsať dní na púšti a potom vyhladol, prejavil sa ako človek. –Nie že zlenivieš, duša! Keď bude do teba dorážať nepriateľ, od modlitby a pôstu – – tvojich nôh, nech sa odrazí.
Kristus bol pokúšaný, diabol pokúšal, ponúkal kamene, aby sa stali chlebmi; vyviedol ho na vrch, aby videl všetky kráľovstvá sveta v jednom okamihu. Boj sa, duša, jeho vábenia! Buď triezva a v každej hodine sa modli k Bohu.
Hrdlička milujúca púšť, Hlas volajúceho zvestoval; Kristov svietnik, pokánie ohlasoval; Herodes však konal neprávosť aj s Herodiadou. Hľaď, duša moja, aby si neuviazla v sieti neprávosti, ale zvítaj sa s pokáním.
V púšti sa usídlil predchodca Milosti, a celá Judea a Samária, keď o tom počuli, bežali, vyznávali sa zo svojich hriechov, a horlivo sa dávali krstiť. A ty si ich nenasledovala, duša!
Úctyhodné manželstvo a nepoškvrnené lôžko! Obidve kedysi Kristus požehnal, keď v tele jedol a v Káne na svadbe z vody urobil víno, čím ukázal prvý zázrak; aby si sa ty, duša, zmenila.
Kristus ochrnutého uzdravil a on s lôžkom odišiel, zosnulého mládenca prebudil, i dieťa vdovy, aj sluhu stotníka, Ukázal sa Samaritánke a službu v Duchu, duša, dal ti tak za vzor.
Pán uzdravil ženu s krvotokom, keď sa dotkla lemu rúcha, očistil malomocných; slepých a chromých osvietil a vzpriamil; /hluchých a nemých, i nadol zhrbených, slovom vyliečil. Aby si sa ty, úbohá duša, zachránila.
Uzdravoval choroby, chudobným hlásal dobrú zvesť; <NAME> liečil skrivených, jedol s mýtnikmi, rozprával sa s hriešnikmi. Jairovej dcére zosnulú dušu vrátil dotykom ruky.
Mýtnik sa zachránil, smilnica našla miernosť, ale farizej chválil sa a bol odsúdený. Prvý totiž volal: "očisť ma", tá druhá: "zmiluj sa nado mnou", tento sa však vyvyšoval: “Bože, ďakujem ti” a ďalšie nerozumné slová.
Zachej bol mýtnik, a predsa sa zachránil, ale farizej Šimon sa pohoršil; smilnica dostala slobodu odpustenia – od toho, ktorý má moc odpúšťať hriechy. Ponáhľaj sa, duša, nasleduj ju.
Nenapodobnila si smilnicu, úbohá duša moja; ona vzala nádobu s myrom v slzách natierala Spasiteľove nohy, a vlasmi utrela toho, ktorý jej dávnych hriechov rukopis roztrhal.
Boli mestá, ktorým priniesol Kristus blahozvesť, a zistila si, duša moja, že predsa boli zavrhnuté. Boj sa tohto príkladu, nech nie si ako tí, ktorých k Sodomčanom Vládca prirovnal, a až do podsvetia odsúdil.
Nezaostaň, duša moja, kvôli beznádeji! O Kanaánčanke, o jej viere si počula – skrze ňu bola jej dcéra uzdravená Božím slovom. "Synu Dávidov, zachráň aj mňa!" – volaj takto z hlbín srdca, tak ako ona, Kristovi.
Buď milosrdný a zachráň ma, <NAME>, zmiluj sa; ty si posadnutých slovom uzdravil, láskavým hlasom ako zločincovi mi povedz: Amen, hovorím ti, budeš so mnou v raji, keď prídem do svojej slávy.
Zločinec ťa ohováral, zločinec ti dobrorečil, obidvaja spolu s tebou viseli na kríži. Ale Dobrotivý, tak ako vernému zločincovi, ktorý ťa spoznal ako Boha, aj mne otvor brány tvojho slávneho kráľovstva.
Tvorstvo sa triaslo, keď videlo, ako ťa križujú, vrchy a kamene od strachu sa rozpadali; Aj zem sa spolu chvela,a podsvetie sa obnažilo. Potemnelo svetlo počas dňa, keď hľadelo na teba, Ježišu, pribitého na kríž.
Plody hodné pokánia u mňa nehľadaj, lebo sa moja sila vo mne vyčerpala; daruj mi srdce neustále skrúšené, a duchovnú chudobu, aby som ti ich priniesol ako príjemnú obetu, jediný Spasiteľ.
Sudca môj a Znalec môj; keď znovu prídeš s anjelmi súdiť celý svet, svojím láskavým okom zhliadni na mňa, ušetri ma a zľutuj sa, Ježišu, nado mnou, lebo som zhrešil viac ako celý ľudský rod.
Verš: Prepodobná matka Mária, pros Boha za nás.
Svojím neobyčajným životom udivila si všetky anjelské stavy a ľudské zbory; žila si nehmotne a prekonala si prirodzenosť. Vďaka tomu si vstúpila do Jordánu, Mária, a akoby nehmotný, tak si ho prebrodila.
Verš: Prepodobná matka Mária, pros Boha za nás.
Nakloň Stvoriteľa k milosrdenstvu voči tým, čo ťa chvália, prepodobná matka, nech sme zbavení zloby a trápení, ktoré dorážajú zo všetkých strán. Aby sme oslobodení od nástrah ustavične velebili Pána, ktorý ťa oslávil.
Verš: Prepodobný otec Andrej, pros Boha za nás.
Ctihodný Andrej, trojblažený otče, <NAME>, neprestávaj sa modliť za tých, čo ťa ospevujú; aby sme boli oslobodení všetci, ktorí si verne ctíme tvoju pamiatku, od hnevu, trápenia, porušenia a od nadmiery hriechov.
Sláva:
Jednopodstatná Trojica, Jeden v troch hypostázach, ospevujeme ťa: /oslavujeme Otca, velebíme Syna a klaniame sa Duchu, jedinej prirodzenosti, pravému Bohu, Životu a živému nekonečnému kráľovstvu.
I teraz:
Ochraňuj svoje mesto, Prečistá Božia rodička, lebo ak s tebou verne vládne, v tebe sa aj upevní, a skrze teba bude víťaziť. Zaženie každý útok, zajme vojsko a ukončí sa poroba.
Katavasia: Po panenskom počatí neopísateľný pôrod, z Matky bez muža neporušiteľný plod, Božie narodenie, obnovuje prirodzenosť. Preto ťa vo všetkých pokoleniach ako Matku, Božiu nevestu, pravoverne velebíme.
Dôstojné je velebiť teba, Bohorodička, \* vždy blažená a nepoškvrnená Matka nášho Boha. \* Čestnejšia si ako cherubíni \* a neporovnateľne slávnejšia ako serafíni, \* bez porušenia si porodila Boha Slovo, \* opravdivá Bohorodička, velebíme ťa.
(Ak je prítomný kňaz, alebo diakon, prednáša malú ekténiu. Ak nie, berieme
Pane, zmiluj sa. (3x)
Sláva, i teraz: )
Znova a znova modlime sa v pokoji k Pánovi.
Ľud: Pane, zmiluj sa.
Zastaň sa a spas nás, zmiluj sa a zachráň nás, Bože, svojou milosťou.
Ľud: Pane, zmiluj sa.
Presvätú, prečistú, preblahoslavenú a slávnu Vládkyňu našu, Bohorodičku Máriu, vždy Pannu, i všetkých svätých spomínajúc, sami seba, druh druha i celý náš život Kristu Bohu oddajme.
Ľud: Tebe, Pane.
Kňaz: Lebo teba chvália všetky nebeské mocnosti a my ti vzdávame slávu, Otcu i Synu, i Svätému Duchu, teraz i vždycky, i na veky vekov.
Ľud: Amen.
Svitilen
Ty si Svetlom, Kriste, preto ma osvieť sebou na príhovor tvojich svätých apoštolov a svätiteľa Mikuláša a spas ma.
Sláva: Ty si Svetlom, Kriste,\* preto ma osvieť sebou na príhovor všetkých tvojich svätých a spas ma.
I teraz: Ty si Svetlom, Kriste,\* preto ma osvieť sebou na príhovor Bohorodičky a spas ma.
Žalm 148
Chváľte Pána z nebies, \* chváľte ho na výsostiach. – Chváľte ho, všetci jeho anjeli; \* chváľte ho, všetky nebeské mocnosti; – chváľte ho, slnko a mesiac; \* chváľte ho, všetky hviezdy žiarivé; – chváľte ho, nebies nebesia \* a všetky vody nad oblohou. – Nech chvália meno Pánovo, \* lebo na jeho rozkaz boli stvorené. – Založil ich navždy a naveky; \* vydal zákon, ktorý nepominie. – Chváľ Pána, tvorstvo pozemské: \* obludy morské a všetky hlbiny, – oheň, kamenec, sneh a dym, \* víchrica, čo jeho slovo poslúcha, – vrchy a všetky pahorky, \* ovocné stromy a všetky cédre, – divá zver a všetok dobytok, \* plazy a okrídlené vtáctvo. – Králi zeme a všetky národy, \* kniežatá a všetci zemskí sudcovia, – mládenci a panny, \* starci a junáci – nech chvália meno Pánovo, \* lebo iba jeho meno je vznešené. – Jeho veleba prevyšuje zem i nebesia \* a svojmu ľudu dáva veľkú moc. – Je chválou všetkým svojim svätým, \* synom Izraela, ľudu, ktorý je mu blízky.
Žalm 149
Spievajte Pánovi pieseň novú; \* jeho chvála nech znie v zhromaždení svätých. – Nech sa teší Izrael zo svojho Stvoriteľa, \* synovia Siona nech jasajú nad svojím kráľom. – Nech oslavujú jeho meno tancom, \* nech mu hrajú na bubne a na citare. – Lebo Pán miluje svoj ľud \* a tichých venčí víťazstvom. – Nech svätí jasajú v sláve, \* nech sa veselia na svojich lôžkach. – Oslavu Boha nech majú na perách \* a v rukách meč dvojsečný, – aby sa pomstili na pohanoch \* a potrestali národy, – aby ich kráľom nasadili putá \* a ich veľmožom železné okovy. Aby ich súdili podľa písaného práva, \* všetkým jeho verným to bude na slávu.
Žalm 150
Chváľte Pána v jeho svätyni! \* Chváľte ho na jeho vznešenej oblohe! Chváľte ho za jeho činy mohutné, \* chváľte ho za jeho nesmiernu velebnosť! Chváľte ho zvukom poľnice, \* chváľte ho harfou a citarou! Chváľte ho bubnom a tancom, \* chváľte ho lýrou a flautou! Chváľte ho zvučnými cimbalmi, chváľte ho jasavými cimbalmi, \* všetko, čo dýcha, nech chváli Pána.
Kňaz: Tebe patrí veleba, Pane, Bože náš, a my ti vzdávame slávu, Otcu i Synu, i Svätému Duchu, teraz i vždycky, i na veky vekov.
Ľud: Amen.
Kňaz: Sláva tebe, ktorý si nám zjavil svetlo.
Ľud: Sláva Bohu na nebi a mier na zemi. V ľuďoch dobrá vôľa. Chválime ťa, velebíme ťa. Klaniame sa ti, oslavujeme ťa. Dobrorečíme ti pre nesmiernu tvoju slávu, Pane a kráľu nebeský, Bože, Otče, všemohúci Vládca, Pane náš a jednorodený Synu, Ježišu Kriste, i Svätý Duchu, Pane Bože. Baránku Boží, Synu Otca. Ty, čo snímaš hriechy sveta, zmiluj sa nad nami. Ty, čo snímaš hriechy sveta, prijmi naše modlitby. Ty, čo sedíš po pravici Otcovej, zmiluj sa nad nami. Len ty sám si svätý, ty jediný si Pán, Jež<NAME> v sláve Boha Otca. Amen. Každý deň ťa budeme velebiť a tvoje meno chváliť navždy a naveky. Pane, bol si naše útočisko z pokolenia na pokolenie. Riekol som: Pane, zmiluj sa nado mnou a uzdrav moju dušu, lebo som sa prehrešil proti tebe. Pane, k tebe sa utiekam. Nauč ma plniť tvoju vôľu, lebo ty si môj Boh. Veď u teba je zdroj života a v tvojom svetle uzrieme svetlo. Svoju milosť daj tým, čo ťa poznajú.
Potom:
Ráč nás, Pane, v tento deň zachrániť od hriechu. Velebíme ťa, Pane, Bože otcov našich. Chválime a oslavujeme tvoje meno naveky. Amen. Preukáž nám, Pane, svoje milosrdenstvo, lebo dúfame v teba. Blahoslavený si, Pane, nauč nás svoje pravdy. Blahoslavený si, Vládca, daj nám porozumieť svojim pravdám. Blahoslavený si, Svätý, osvieť nás svojimi pravdami. Pane, tvoje milosrdenstvo je večné, nepohŕdaj dielom svojich rúk. Tebe patrí chvála, tebe patrí pieseň. Tebe, Otcu i Synu, i Svätému Duchu, patrí sláva teraz i vždycky, i na veky vekov. Amen.
(Ak je prítomný kňaz, alebo diakon, prednáša túto ekténiu. Ak nie, berieme
Pane, zmiluj sa. (12x)
Sláva, i teraz: )
Vykonajme našu rannú modlitbu k Pánovi.
Ľud: Pane, zmiluj sa.
Zastaň sa a spas nás, zmiluj sa a zachráň nás, Bože, svojou milosťou.
Ľud: Pane, zmiluj sa.
Celé ráno prežité v dokonalosti, svätosti, pokoji a bez hriechu prosme si od Pána.
Po každej prosbe ľud odpovedá: Daruj nám, Pane.
Anjela pokoja, verného sprievodcu a ochrancu našej duše i tela prosme si od Pána.
Odpustenie a oslobodenie z našich hriechov a previnení, prosme si od Pána.
Dobro a úžitok pre naše duše a pokoj pre svet prosme si od Pána.
Aby sme zostávajúce dni nášho života prežili v pokoji a kajúcnosti, prosme si od Pána.
Pokojné kresťanské dovŕšenie nášho života, bez bolesti a zahanbenia, dobrú odpoveď na prísnom Kristovom súde prosme si od Pána.
Presvätú, prečistú, preblahoslavenú a slávnu Vládkyňu našu, Bohorodičku Máriu, vždy Pannu, i všetkých svätých spomínajúc, sami seba, druh druha i celý náš život Kristu Bohu oddajme.
Ľud: Tebe, Pane.
Kňaz: Lebo ty si Boh dobrý a láskavý k ľuďom a my ti vzdávame slávu, Otcu i Synu, i Svätému Duchu, teraz i vždycky, i na veky vekov.
Ľud: Amen.
Kňaz: Pokoj všetkým!
Ľud: I tvojmu duchu.
Diakon: Skloňte si hlavy pred Pánom.
Ľud: Pred tebou, Pane.
A kňaz hovorí potichu túto modlitbu:
Najsvätejší Pane, ktorý žiješ na výsostiach a svojím vševidiacim okom sleduješ každú bytosť, pred tebou sme sklonili svoju dušu i telo. A prosíme ťa, Svätý svätých: požehnaj nás svojou neviditeľnou rukou zo svojho svätého príbytku. Vo svojej dobrote a láske odpusť nám všetky poklesky vedomé i nevedomé. A našej duši i telu udeľ potrebné milosti.
Kňaz: Lebo ty nás miluješ a chrániš, Bože náš, tebe, Otcu i Synu, i Svätému Duchu, vzdávame slávu teraz i vždycky, i na veky vekov.
Ľud: Amen.
Veršové slohy, 8. hlas
Na cesty lúpežníkov vpadla si, duša moja, \* kruto ťa zraňujú tvoje vlastné hriechy, \* keď si sa vzdala nerozumným nepriateľom. \* Ale máš ešte čas, preto v skrúšenosti volaj: \* Nádej beznádejných, život zúfajúcich, \*\* Spasiteľ, pomôž mi vstať a zachráň ma.
Verš: RRáno sme sa nasýtili tvojím milosrdenstvom, \* jasali sme a tešili sme sa po všetky naše dni. \* Tešili sme sa za dni, keď si nás ponižoval, \* za roky, keď sme videli zlo. \* Pozri na svojich sluhov a na svoje diela a veď ich synov.
Na cesty lúpežníkov vpadla si, duša moja, \* kruto ťa zraňujú tvoje vlastné hriechy, \* keď si sa vzdala nerozumným nepriateľom. \* Ale máš ešte čas, preto v skrúšenosti volaj: \* Nádej beznádejných, život zúfajúcich, \*\* Spasiteľ, pomôž mi vstať a zachráň ma.
Verš: Nech je ž<NAME>, nášho Boha, nad nami, diela našich rúk riaď nad nami, dielo našich rúk riaď.
Pancier viery správne ste si obliekli, \* znakom kríža ste sa ozbrojili, \* ako pripravení vojaci ste sa prejavili, \* mučiteľom ste statočne vzdorovali \* a úklady diabla ste rozdrvili. \* Stali ste sa víťazmi a zaslúžili ste si vence. \*\* Proste Krista za nás, aby zachránil naše duše.
Sláva i teraz, 8. hlas
Prijmi volanie svojich služobníkov, \* prečistá Bohorodička, Panna, \* a bez prestania sa modli \* za odpustenie našich hriechov \*\* a za dar pokoja pre nás.
Potom:
Dobré je oslavovať Pána \* a ospevovať tvoje meno, Najvyšší. \* Za rána zvestovať tvoju dobrotu a tvoju vernosť celú noc. (dvakrát)
Po skončení slôh:
Teraz prepustíš, Pane, svojho služobníka podľa svojho slova v pokoji, lebo moje oči uvideli tvoju spásu, ktorú si pripravil pred tvárou všetkých národov. Svetlo na osvietenie pohanov a slávu Izraela, tvojho ľudu.
Svätý Bože, Svätý Silný, Svätý Nesmrteľný, zmiluj sa nad nami. (3x)
Sláva Otcu i Synu i Svätému Duchu.
I teraz i vždycky i na veky vekov. Amen.
Presvätá Trojica, zmiluj sa nad nami. \* Pane, očisť nás od našich hriechov. \* Vládca, odpusť nám naše neprávosti, \* Svätý, príď a uzdrav naše slabosti pre svoje meno.
Pane, zmiluj sa. (3x)
Sláva Otcu i Synu i Svätému Duchu.
I teraz i vždycky i na veky vekov. Amen.
Otče náš, ktorý si na nebesiach, \* posväť sa meno tvoje, \* príď kráľovstvo tvoje, \* buď vôľa tvoja ako v nebi, tak i na zemi. \* Chlieb náš každodenný daj nám dnes \* a odpusť nám naše viny, \* ako i my odpúšťame svojim vinníkom, \* a neuveď nás do pokušenia, \* ale zbav nás Zlého.
Kňaz: Lebo tvoje je kráľovstvo a moc i sláva, Otca i Syna i Svätého Ducha, teraz i vždycky i na veky vekov.
Ľud: Amen.
Tropár, 7. hlas
Stojíme v chráme tvojej slávy, \* a myslíme, že stojíme v nebesiach, \* Bohorodička, brána nebeská, \* otvor nám dvere svojho milosrdenstva.
Pane, zmiluj sa. (40-krát) Pane, požehnaj.
Kňaz: Požehnaný je Kristus, náš Boh, v každom čase, teraz i vždycky, i na veky vekov. (ak sa nemodlí kňaz, hovoríme: Pane Ježišu Kriste, Bože náš, pre modlitby našich svätých otcov zmiluj sa nad nami.)
Ľud: Amen.
Kňaz: Nebeský Kráľu, posilni tých, čo spravujú a ochraňujú našu krajinu, utvrď vieru, skroť nepriateľov, upokoj svet, ochraňuj toto mesto (alebo túto obec, alebo tento svätý dom) ; našich zosnulých otcov, bratov a sestry uveď do príbytkov spravodlivých a nás, ktorí robíme pokánie a vyznávame svoje hriechy, prijmi, a zmiluj sa nad nami, lebo si dobrý a miluješ nás.
Ľud: Amen. Pane, zmiluj sa. (trikrát)
Sláva, i teraz:
Čestnejšia si ako cherubíni \* a neporovnateľne slávnejšia ako serafíni, \* bez porušenia si porodila Boha Slovo, \* opravdivá Bohorodička, velebíme ťa.
(Ak niet kňaza, toto zvolanie vynechávame:)V mene Pánovom požehnaj, otče.
Kňaz: Pane Ježišu Kriste, Bože náš, pre modlitby našich svätých otcov zmiluj sa nad nami.
Ľud: Amen.
Modlitba svätého Efréma Sýrskeho
Pane a Vládca môjho života, odním odo mňa ducha znechutenosti, nedbalosti, mocibažnosti a prázdnych rečí. (veľká poklona)
Daruj mne, svojmu služobníkovi, ducha miernosti, poníženosti, trpezlivosti a lásky. (veľká poklona)
Áno, Pane a Kráľu, daj, aby som videl vlastné prehrešenia a neposudzoval svojho brata, lebo ty si požehnaný na veky vekov. Amen. (veľká poklona)
Potom nasleduje dvanásť malých poklôn, pričom pri každej poklone hovoríme:
Bože, buď milostivý mne hriešnemu. (malá poklona)
Bože, očisť moje hriechy a zmiluj sa nado mnou. (malá poklona)
Mnoho ráz som zhrešil, Pane, odpusť mi. (malá poklona)
Pane a Vládca môjho života, odním odo mňa ducha znechutenosti, nedbalosti, mocibažnosti a prázdnych rečí.
Daruj mne, svojmu služobníkovi, ducha miernosti, poníženosti, trpezlivosti a lásky.
Áno, Pane a Kráľu, daj, aby som videl vlastné prehrešenia a neposudzoval svojho brata, lebo ty si požehnaný na veky vekov. Amen. (veľká poklona)
Ak sa modlí kňaz, zvoláva: Sláva tebe, <NAME>, naša nádej, sláva tebe.
Ľud: Sláva Otcu i Synu, i Svätému Duchu. I teraz, i vždycky, i na veky vekov. Amen. Pane, zmiluj sa. (3x) Požehnaj.
Kňaz: Kristus, náš pravý Boh, na prosby svojej prečistej Matky, svätých, slávnych a všechválnych apoštolov, nášho otca svätého Mikuláša, lycijsko-myrského divotvorcu, nášho prepodobného otca Benedikta a všetkých svätých, nech sa nad nami zmiluje a spasí nás, lebo je dobrý a miluje nás.
Ľud: Amen.
Ak sa nemodlí kňaz, berieme: Dôstojné je: Sláva, i teraz: Pane, zmiluj sa (3x) Pane, požehnaj. Pane Ježišu Kriste, Bože náš, pre modlitby našich svätých otcov zmiluj sa nad nami. Amen. |
|
https://github.com/PauKaifler/typst-template-dhbw | https://raw.githubusercontent.com/PauKaifler/typst-template-dhbw/main/example.typ | typst | Apache License 2.0 | #import "template.typ": template
#import "@preview/glossarium:0.4.1": make-glossary, print-glossary, gls, glspl
#set document(
title: "Development of an LLM-Based Pet Entertainment System",
author: "<NAME>",
)
#set text(lang: "en")
#show: make-glossary
#show: template.with(
article-kind: "Bachelorarbeit",
author: (
matriculation-number: "1208964",
major: "Informatik",
course-code: "TIX20",
),
company: (
name: "Example Corporation",
city: "91719 Heidenheim",
logo: image("company-logo.png"),
supervisor: "<NAME>",
),
dhbw: (
location: "Ravensburg",
reviewer: "Dr. <NAME>",
),
signature: (
location: "Heidenheim",
date: datetime.today(),
),
deadline: datetime(day: 25, month: 9, year: 2023),
handling-period: "12 Wochen",
restriction-note: true,
abstract: [
In this thesis, I present the design and implementation of a novel system to occupy pets' time.
Using an integrated automatic speech recognition model, the animals' sounds are converted into a text format.
Said text is then read by a pretrained large language model, which will then generate a response.
Finally, the answer is synthesized and played back using a common loudspeaker.
While my hamster and goldfish did not try to communicate with the system, my Chihuahua made heavy use of it.
As such, the contributions of this thesis can greatly increase the enjoyment of animals staying at home.
],
acronyms: print-glossary((
(key: "asr", short: "ASR", long: "automatic speech recognition"),
(key: "llm", short: "LLM", long: "large language model"),
)),
)
#bibliography("refs.bib", style: "ieee")
|
https://github.com/GZTimeWalker/GZ-Typst-Templates | https://raw.githubusercontent.com/GZTimeWalker/GZ-Typst-Templates/main/functions/fonts.typ | typst | MIT License | #let line_height = 1em
#let fonts = (
serif: ("Source Han Serif SC", "Source Han Serif"),
sans: ("Source Han Sans SC", "Source Han Sans"),
monospace: ("JetBrains Mono", "Consolas"),
)
#let textbf(it) = block(text(font: fonts.sans, weight: "semibold", it))
#let textit(it) = block(text(style: "italic", it))
|
https://github.com/dadn-dream-home/documents | https://raw.githubusercontent.com/dadn-dream-home/documents/main/contents/07-thiet-ke-kien-truc/1-backend/index.typ | typst | == Mô hình MVC ở Backend
Lược đồ các class ở backend được biểu diễn ở @fig:backend-architecture.
#figure(
image("backend-architecture.drawio.svg", width: 100%),
caption: "Lược đồ các class ở backend"
) <fig:backend-architecture>
Các class này tạo thành mô hình MVC thành phần:
/ `VerbNounHandler`: là các class chứa các method tiếp nhận các request từ client.
Các method này được sinh tự động từ các file `*.proto` do `gRPC`. Các class
đóng vai trò là view. Các class này sẽ gọi các controller khác để xử lý và
trả về kết quả cho client.
/ `Server`: là class chứa các method để khởi tạo server và điều phối các
controller. Class này đóng vai trò là view. \
Class này sử dụng design pattern *Mediator*, là đầu mối để tất cả các
handler và controller giao tiếp với nhau.
/ `Repository`: là class có nhiệm vụ truy xuất dữ liệu từ database. Class này đóng
vai trò là model.
/ `Feed`, `Config`, `Activity`, `Notification`: là các class model, được tạo bởi file
`*.proto` do `gRPC` sinh ra.
/ `DatabaseHooker`: là class controller, có nhiệm vụ lắng nghe các thay đổi
ngay từ database và cập nhật cho những class cần. \
Class này sử dụng design pattern *Observer*, để gửi các cập nhật cho các
class cần thiết khác.
/ `AutomaticScheduler`: là class controller, có nhiệm vụ chạy các task định kỳ
là bật tắt thiết bị theo lịch.
/ `FeedValueLogger`#footnote([Tên class _đáng lẽ_ nên là `*Notifier`, tên class hiện
tại mang tính tàn tích lịch sử.]): là class controller, có nhiệm vụ lắng nghe các giá trị
cảm biến để sinh ra thông báo.
/ `MQTTSubscriber`: là class controller, có nhiệm vụ lắng nghe các giá trị từ
MQTT và lưu vào database, cũng như nhận các yêu cầu publish MQTT. \
Class này sử dụng design pattern *Adaptor*, cung cấp API dễ sử dụng hơn class
`mqtt.Client` do thư viện cung cấp. |
|
https://github.com/jgm/typst-hs | https://raw.githubusercontent.com/jgm/typst-hs/main/test/typ/compiler/dict-07.typ | typst | Other | // Error: 17-20 duplicate key: a
#(a: 1, "b": 2, "a": 3)
|
https://github.com/typst/packages | https://raw.githubusercontent.com/typst/packages/main/packages/preview/unichar/0.1.0/ucd/block-2C80.typ | typst | Apache License 2.0 | #let data = (
("COPTIC CAPITAL LETTER ALFA", "Lu", 0),
("COPTIC SMALL LETTER ALFA", "Ll", 0),
("COPTIC CAPITAL LETTER VIDA", "Lu", 0),
("COPTIC SMALL LETTER VIDA", "Ll", 0),
("COPTIC CAPITAL LETTER GAMMA", "Lu", 0),
("COPTIC SMALL LETTER GAMMA", "Ll", 0),
("COPTIC CAPITAL LETTER DALDA", "Lu", 0),
("COPTIC SMALL LETTER DALDA", "Ll", 0),
("COPTIC CAPITAL LETTER EIE", "Lu", 0),
("COPTIC SMALL LETTER EIE", "Ll", 0),
("COPTIC CAPITAL LETTER SOU", "Lu", 0),
("COPTIC SMALL LETTER SOU", "Ll", 0),
("COPTIC CAPITAL LETTER ZATA", "Lu", 0),
("COPTIC SMALL LETTER ZATA", "Ll", 0),
("COPTIC CAPITAL LETTER HATE", "Lu", 0),
("COPTIC SMALL LETTER HATE", "Ll", 0),
("COPTIC CAPITAL LETTER THETHE", "Lu", 0),
("COPTIC SMALL LETTER THETHE", "Ll", 0),
("COPTIC CAPITAL LETTER IAUDA", "Lu", 0),
("COPTIC SMALL LETTER IAUDA", "Ll", 0),
("COPTIC CAPITAL LETTER KAPA", "Lu", 0),
("COPTIC SMALL LETTER KAPA", "Ll", 0),
("COPTIC CAPITAL LETTER LAULA", "Lu", 0),
("COPTIC SMALL LETTER LAULA", "Ll", 0),
("COPTIC CAPITAL LETTER MI", "Lu", 0),
("COPTIC SMALL LETTER MI", "Ll", 0),
("COPTIC CAPITAL LETTER NI", "Lu", 0),
("COPTIC SMALL LETTER NI", "Ll", 0),
("COPTIC CAPITAL LETTER KSI", "Lu", 0),
("COPTIC SMALL LETTER KSI", "Ll", 0),
("COPTIC CAPITAL LETTER O", "Lu", 0),
("COPTIC SMALL LETTER O", "Ll", 0),
("COPTIC CAPITAL LETTER PI", "Lu", 0),
("COPTIC SMALL LETTER PI", "Ll", 0),
("COPTIC CAPITAL LETTER RO", "Lu", 0),
("COPTIC SMALL LETTER RO", "Ll", 0),
("COPTIC CAPITAL LETTER SIMA", "Lu", 0),
("COPTIC SMALL LETTER SIMA", "Ll", 0),
("COPTIC CAPITAL LETTER TAU", "Lu", 0),
("COPTIC SMALL LETTER TAU", "Ll", 0),
("COPTIC CAPITAL LETTER UA", "Lu", 0),
("COPTIC SMALL LETTER UA", "Ll", 0),
("COPTIC CAPITAL LETTER FI", "Lu", 0),
("COPTIC SMALL LETTER FI", "Ll", 0),
("COPTIC CAPITAL LETTER KHI", "Lu", 0),
("COPTIC SMALL LETTER KHI", "Ll", 0),
("COPTIC CAPITAL LETTER PSI", "Lu", 0),
("COPTIC SMALL LETTER PSI", "Ll", 0),
("COPTIC CAPITAL LETTER OOU", "Lu", 0),
("COPTIC SMALL LETTER OOU", "Ll", 0),
("COPTIC CAPITAL LETTER DIALECT-P ALEF", "Lu", 0),
("COPTIC SMALL LETTER DIALECT-P ALEF", "Ll", 0),
("COPTIC CAPITAL LETTER OLD COPTIC AIN", "Lu", 0),
("COPTIC SMALL LETTER OLD COPTIC AIN", "Ll", 0),
("COPTIC CAPITAL LETTER CRYPTOGRAMMIC EIE", "Lu", 0),
("COPTIC SMALL LETTER CRYPTOGRAMMIC EIE", "Ll", 0),
("COPTIC CAPITAL LETTER DIALECT-P KAPA", "Lu", 0),
("COPTIC SMALL LETTER DIALECT-P KAPA", "Ll", 0),
("COPTIC CAPITAL LETTER DIALECT-P NI", "Lu", 0),
("COPTIC SMALL LETTER DIALECT-P NI", "Ll", 0),
("COPTIC CAPITAL LETTER CRYPTOGRAMMIC NI", "Lu", 0),
("COPTIC SMALL LETTER CRYPTOGRAMMIC NI", "Ll", 0),
("COPTIC CAPITAL LETTER OLD COPTIC OOU", "Lu", 0),
("COPTIC SMALL LETTER OLD COPTIC OOU", "Ll", 0),
("COPTIC CAPITAL LETTER SAMPI", "Lu", 0),
("COPTIC SMALL LETTER SAMPI", "Ll", 0),
("COPTIC CAPITAL LETTER CROSSED SHEI", "Lu", 0),
("COPTIC SMALL LETTER CROSSED SHEI", "Ll", 0),
("COPTIC CAPITAL LETTER OLD COPTIC SHEI", "Lu", 0),
("COPTIC SMALL LETTER OLD COPTIC SHEI", "Ll", 0),
("COPTIC CAPITAL LETTER OLD COPTIC ESH", "Lu", 0),
("COPTIC SMALL LETTER OLD COPTIC ESH", "Ll", 0),
("COPTIC CAPITAL LETTER AKHMIMIC KHEI", "Lu", 0),
("COPTIC SMALL LETTER AKHMIMIC KHEI", "Ll", 0),
("COPTIC CAPITAL LETTER DIALECT-P HORI", "Lu", 0),
("COPTIC SMALL LETTER DIALECT-P HORI", "Ll", 0),
("COPTIC CAPITAL LETTER OLD COPTIC HORI", "Lu", 0),
("COPTIC SMALL LETTER OLD COPTIC HORI", "Ll", 0),
("COPTIC CAPITAL LETTER OLD COPTIC HA", "Lu", 0),
("COPTIC SMALL LETTER OLD COPTIC HA", "Ll", 0),
("COPTIC CAPITAL LETTER L-SHAPED HA", "Lu", 0),
("COPTIC SMALL LETTER L-SHAPED HA", "Ll", 0),
("COPTIC CAPITAL LETTER OLD COPTIC HEI", "Lu", 0),
("COPTIC SMALL LETTER OLD COPTIC HEI", "Ll", 0),
("COPTIC CAPITAL LETTER OLD COPTIC HAT", "Lu", 0),
("COPTIC SMALL LETTER OLD COPTIC HAT", "Ll", 0),
("COPTIC CAPITAL LETTER OLD COPTIC GANGIA", "Lu", 0),
("COPTIC SMALL LETTER OLD COPTIC GANGIA", "Ll", 0),
("COPTIC CAPITAL LETTER OLD COPTIC DJA", "Lu", 0),
("COPTIC SMALL LETTER OLD COPTIC DJA", "Ll", 0),
("COPTIC CAPITAL LETTER OLD COPTIC SHIMA", "Lu", 0),
("COPTIC SMALL LETTER OLD COPTIC SHIMA", "Ll", 0),
("COPTIC CAPITAL LETTER OLD NUBIAN SHIMA", "Lu", 0),
("COPTIC SMALL LETTER OLD NUBIAN SHIMA", "Ll", 0),
("COPTIC CAPITAL LETTER OLD NUBIAN NGI", "Lu", 0),
("COPTIC SMALL LETTER OLD NUBIAN NGI", "Ll", 0),
("COPTIC CAPITAL LETTER OLD NUBIAN NYI", "Lu", 0),
("COPTIC SMALL LETTER OLD NUBIAN NYI", "Ll", 0),
("COPTIC CAPITAL LETTER OLD NUBIAN WAU", "Lu", 0),
("COPTIC SMALL LETTER OLD NUBIAN WAU", "Ll", 0),
("COPTIC SYMBOL KAI", "Ll", 0),
("COPTIC SYMBOL MI RO", "So", 0),
("COPTIC SYMBOL PI RO", "So", 0),
("COPTIC SYMBOL STAUROS", "So", 0),
("COPTIC SYMBOL TAU RO", "So", 0),
("COPTIC SYMBOL KHI RO", "So", 0),
("COPTIC SYMBOL SHIMA SIMA", "So", 0),
("COPTIC CAPITAL LETTER CRYPTOGRAMMIC SHEI", "Lu", 0),
("COPTIC SMALL LETTER CRYPTOGRAMMIC SHEI", "Ll", 0),
("COPTIC CAPITAL LETTER CRYPTOGRAMMIC GANGIA", "Lu", 0),
("COPTIC SMALL LETTER CRYPTOGRAMMIC GANGIA", "Ll", 0),
("COPTIC COMBINING NI ABOVE", "Mn", 230),
("COPTIC COMBINING SPIRITUS ASPER", "Mn", 230),
("COPTIC COMBINING SPIRITUS LENIS", "Mn", 230),
("COPTIC CAPITAL LETTER BOHAIRIC KHEI", "Lu", 0),
("COPTIC SMALL LETTER BOHAIRIC KHEI", "Ll", 0),
(),
(),
(),
(),
(),
("COPTIC OLD NUBIAN FULL STOP", "Po", 0),
("COPTIC OLD NUBIAN DIRECT QUESTION MARK", "Po", 0),
("COPTIC OLD NUBIAN INDIRECT QUESTION MARK", "Po", 0),
("COPTIC OLD NUBIAN VERSE DIVIDER", "Po", 0),
("COPTIC FRACTION ONE HALF", "No", 0),
("COPTIC FULL STOP", "Po", 0),
("COPTIC MORPHOLOGICAL DIVIDER", "Po", 0),
)
|
https://github.com/ysthakur/PHYS121-Notes | https://raw.githubusercontent.com/ysthakur/PHYS121-Notes/main/Notes/Ch12.typ | typst | MIT License | #import "@preview/gentle-clues:0.3.0": note, important
= Chapter 12: Thermal Properties of Matter
== The Atomic Model of Matter
/ Gas: System in which each particle moves freely through space until it occasionally collides with another particle or the wall
/ Liquid: Weak bonds permit motion while keeping the particles close together
/ Solid: Particles are connected by stiff spring-like bonds. Solids have a definite shape and can be compressed only slightly
==== Atomic Mass and Atomic Mass Number
/ Atomic mass number: $A$ is the sum of the number of protons and neutrons in an atom
/ Atomic mass: The atomic mass scale is established by defining the mass of Carbon-12 to be exactly 12 u
/ Atomic mass unit: $1 u = 1.66 times 10^(-27)$
/ Molecular mass: Sum of the atomic masses of the atoms that form the molecule
==== Moles
/ Mole: 1 mole (abbr. mol) is $6.02 times 10^23$ basic particles
This is *Avogadro's number* ($N_A$). $N_A$ has units $"mol"^(-1)$
The number $n$ of moles in a substance containing $N$ basic particles is $N/N_A$
The basic particle depends on the substance:
- *Monatomic gas* means that the basic particles are atoms, e.g., helium
- *Diatomic gas* means that the basic particle is a two-atom diatomic molecule, e.g., $upright(O)_2$
/ Molar mass: Molar mass ($M_"mol"$) is mass in grams of 1 mol of substance:
$ n = (M "(in grams)")/M_"mol" $
=== Pressure
- Particles in a gas move around in a container, they sometimes bounce off the walls, creating a force on the walls
- The collisions with the wall create a force perpendicular to the wall
/ Pressure: The pressure of a gas is the ratio of the force to the area
$ p = F/A $
SI unit for pressure is pascal (Pa), equal to $N/m^2$
/ Standard atmosphere: Pressure from atmosphere at sea level: 1 atm = 101,300 Pa = 14.7 psi
The net pressure force is exterted only *where there is a pressure difference* between the two sides of a surface
$ F_"net" = F_2 - F_1 = p_2A - p_1A = A(p_2 - p_1) = A Delta p $
$Delta p$ is gauge pressure
/ Vacuum: Enclosed space with $p << 1 "atm"$
/ Perfect vacuum: Vacuum with $p = 0 "Pa"$, but impossible to remove every molecule
/ Absolute pressure: $p = F/A$
/ Gauge pressure: $p_g$ is the *difference* between the absolute pressure and the atmospheric pressure
== Ideal Gas Law
Version with $N$ as number of molecules:
$ p V = N k_upright(B) T$
Version with $n$ as number of moles:
$ p V = n R T $
- $p$ is absolute pressure
- $V$ is volume of sample ($upright(m)^3$)
- $n$ is number of moles in the sample/container of gas
- $R$ is *gas constant* ($R = N_upright(A) k_upright(B) = 8.31 upright(J/"mol" dot K)$)
- $T$ is temperature in Kelvin
== Ideal Gas Processes
*Ideal gas processes* have the following properties:
- Quantity of gas is fixed
- Well-defined initial state. Initial values of pressure, volume, and
temperature written as $p_i$, $V_i$, $T_i$
- Well-defined final state where pressure, volume, and temperature are $p_f$, $V_f$, and $T_f$
Initial and final states related by:
$ (p_f V_f)/T_f = (p_i V_i)/T_i $
$p$ and $V$ can be in any units since they appear on both sides,
but $T$ must be in Kelvin since it needs to start at 0.
=== $p V$ Diagrams
In a $p V$ diagram, each point on the graph represents a single, unique state of the gas
Assuming that $n$ is known for a sealed container, we can find $T$ from the ideal-gas law
since we know $p$ and $V$
#align(center)[#image("../Images/12-pV-Diagram.png", height: 50%)]
=== Constant-Volume Processes
/ Isochoric: An isochoric process is a *constant-volume process*
Warming a gas will raise its pressure without changing its volume. This is an example of a constant-volume process
A constant-volume process appears on a $p V$ diagram as a vertical line.
#align(center)[#image("../Images/12-Constant-Volume.png", width: 50%)]
=== Constant-Pressure Processes
/ Isobaric: An isobaric process is a *constant-pressure process*
A constant-pressure process appears on a $p V$ diagram as a horizontal line.
For example, you can heat up a container so that the temperature increases,
making the pressure increase with it, but the volume wouldn't increase.
#align(center)[#image("../Images/12-Constant-Pressure.png")]
=== Constant-Temperature Processes
/ Isothermal: An isothermal process is a *constant-temperature process*
#align(center)[#image("../Images/12-Constant-Temperature-Piston.png", width: 50%)]
/ Isotherm: A graph of an isothermal process
The location of the isotherm depends on temperature.
The direction along the isotherm depends on the process.
#align(center)[#image("../Images/12-Constant-Temperature-pV.png")]
=== Thermodynamics of Ideal-Gas Processes
- Heat and work are just two different ways to add energy to a system
- When gases expand, they do work on the piston
- If the gas expands under constant pressure, pushing the piston (with area $A$) from $x_i$ to $x_f$, then the work done is:
#important(title: "Work done by a gas in a constant-pressure process")[
$ W_"gas" = F_"gas" d = (p A)(x_f - x_i) = p(x_f A - x_i A) = p(V_f - V_i) = p Delta V $
]
To calculate work, *pressure must be in Pa and volume in $upright(m)^3$*
$x_i A$ is the initial volume and $x_f A$ is the final volume
For all ideal-gas processes, the work is the area under the $p V$ graph between $V_i$ and $V_f$:
#align(center)[#image("../Images/12-Gas-Work.png", width: 50%)]
#note[
No work is done in a constant-volume process.
]
=== Adiabatic Processes
/ Adiabatic processes: Processes where heat is not transferred, i.e., $Q = 0$
An adiabatic expansion lowers the temperature of a gas
- Expansion means the gas does the work
- This means work is negative
- So $Delta E_"thermal"$ is negative
- Thus, temperature decreases
An adiabatic compression raises the temperature of a gas
Adiabatic processes allow you to use work rather than heat to change temperature of the gas
== Specific Heat and Heat of Transformation
/ Specific heat: The specific heat ($c$) of a substance is the amount of heat that raises the temperature of 1 kg of that substance by 1 K
Heat needed to produce a temperature change $T$ for mass $M$ with specific heat $c$:
$ Q = M c Delta T $
Substances with large $c$, like water, are slow to warm up and cool down.
Described as having a large thermal inertia.
=== Phase Changes
/ Melting point: Temperature at which solid becomes liquid
/ Freezing point: Temperature at which liquid becomes solid
/ Condensation point: Temperature at which gas becomes liquid
/ Boiling point: Temperature at which liquid becomes gas
/ Phase changes: Melting and freezing are phase changes
/ Phase equilibrium: A system at the melting point is in phase equilibrium
=== Heat of Transformation
A phase change is characterized by a change in thermal energy without a change in temperature
/ Heat of transformation: $L$ is the amount of heat energy that causes 1 kg of a substance to undergo a phase change
/ Heat of fusion: $L_f$ is the heat of transformation between a solid and a liquid
/ Heat of vaporization: $L_v$ is the heat of transformation between a liquid and a gas
Heat needed to melt/freeze mass $M$: $Q = plus.minus M L_f$ \
Heat needed to boil/condense mass $M$: $Q = plus.minus M L_v$
=== Evaporation
/ Evaporation: Process of some molecules moving from liquid to gas phase at temperatures lower than boiling point
At any temperature, some of the molecules are moving fast enough to go into the gas phase,
carrying away thermal energy and reducing the average kinetic energy (temperature) of the liquid
|
https://github.com/LDemetrios/Typst4k | https://raw.githubusercontent.com/LDemetrios/Typst4k/master/dir/test.typ | typst | // Test all gradient presets.
#set page(width: 100pt, height: auto, margin: 0pt)
#set text(fill: white, size: 18pt)
#set text(top-edge: "bounds", bottom-edge: "bounds")
#let presets = (
("turbo", color.map.turbo),
("cividis", color.map.cividis),
("rainbow", color.map.rainbow),
("spectral", color.map.spectral),
("viridis", color.map.viridis),
("inferno", color.map.inferno),
("magma", color.map.magma),
("plasma", color.map.plasma),
("rocket", color.map.rocket),
("mako", color.map.mako),
("vlag", color.map.vlag),
("icefire", color.map.icefire),
("flare", color.map.flare),
("crest", color.map.crest),
)
#stack(
spacing: 3pt,
..presets.map(((name, preset)) => block(
width: 100%,
height: 20pt,
fill: gradient.linear(..preset),
align(center + horizon, smallcaps(name)),
))
)
#import "@preview/cetz:0.2.2": *
#canvas({
draw.scale(5, 5, 5)
})
|
|
https://github.com/Myriad-Dreamin/typst.ts | https://raw.githubusercontent.com/Myriad-Dreamin/typst.ts/main/fuzzers/corpora/layout/repeat_02.typ | typst | Apache License 2.0 |
#import "/contrib/templates/std-tests/preset.typ": *
#show: test-page
// Test empty repeat.
A #box(width: 1fr, repeat[]) B
|
https://github.com/jgm/typst-hs | https://raw.githubusercontent.com/jgm/typst-hs/main/test/typ/compiler/ops-invalid-17.typ | typst | Other | // Error: 13-20 cannot subtract integer from ratio
#((1234567, 40% - 1))
|
https://github.com/polarkac/MTG-Stories | https://raw.githubusercontent.com/polarkac/MTG-Stories/master/stories/036%20-%20Guilds%20of%20Ravnica/014_The%20Gathering%20Storm%3A%20Chapter%209.typ | typst | #import "@local/mtgstory:0.2.0": conf
#show: doc => conf(
"The Gathering Storm: Chapter 9",
set_name: "Guilds of Ravnica",
story_date: datetime(day: 07, month: 08, year: 2019),
author: "<NAME>",
doc
)
Ral awoke in a cold sweat, the long-ago slum-dweller’s scream still ringing in his ears. He lay back against his pillow with a groan.
#emph[It’s been a long time since I had that dream.] He turned his head to look at Tomik, curled up beside him, his features smoothed in sleep. #emph[Small wonder what’s called up those demons, though.]
Through the ignition of his Planeswalker spark and everything that had followed, Ral had kept his romantic life to a minimum, dallying with the occasional casual encounter but nothing more. He’d been determined that no one would be in the position to hurt him so badly again. Tomik had been one such casual encounter, at least at first. #emph[Now things are . . . different.]
He could appreciate the courage it had taken Tomik to come to him with Teysa’s request. #emph[And there’s a reasonable chance that he’s saved Ravnica because of it.] Part of him, though, still wished it could have been avoided. #emph[Now that the line’s been crossed, there’s no going back.] They’d been able to be together as two anonymous people in a rented apartment; whether a relationship could work between <NAME>, second-in-command of the Izzet, and Tomik, private secretary to the Karlov heir, he had no idea.
#emph[It will work] , Ral told himself. #emph[I’ll ] make#emph[ it work.] Besides, if everything went according to plan, he would be guildmaster of the Izzet after the Firemind took up his new position. #emph[Who’s going to stop me?]
Trying to ignore the flutter in his chest, Ral leaned down and kissed Tomik gently on the forehead. His lover mumbled something and rolled over in his sleep. He’d been out late, Ral knew, working with Teysa on the new leadership of the Orzhov.
"Get some rest," he told Tomik quietly. "We’ve got a lot to do."
#v(0.35em)
#line(length: 100%, stroke: rgb(90%, 90%, 90%))
#v(0.35em)
When Ral arrived at his office at the Nivix, Hekara was waiting for him. This was not a surprise—the Rakdos emissary had left the infirmary the previous evening, apparently none the worse for wear and as bouncy as ever. More unusually, Lavinia was there as well, listening patiently to Hekara’s somewhat confused explanation of the action in the cathedral.
". . . an’ then Ral was like, ‘Hekara, if you don’t do something, we’re all dead!’ An’ I said, ‘I’ll take care of it,’ all cool, an’ I went #emph[tzing] an’ hit the stupid guy right in his stupid eyeball. An’ he was all like, ‘Aaargh, damn you, Razorwitch Hekara, how could you defeat me?!’ an’ I said—"
"‘Ow’, if I recall correctly," Ral said, coming in the door.
"That wasn’t it," Hekara said. "It was something much cooler."
"It sounds like you had an exciting time," Lavinia said diplomatically. It was the first time Ral had seen her without a robe and hood. She wore well-fitted scale armor, etched with runic protections, and carried a longsword on her hip like she knew how to use it. "Hello, Ral."
"Good morning." Ral sat behind his desk, which was already cluttered with the day’s papers. "I thought you didn’t want to be seen with me?"
"I didn’t want to tip off Bolas’s people that I was working with you," Lavinia corrected. "Now that your guild summit is going forward, it’s perfectly natural that I meet with you to discuss it."
"That’s convenient," Ral said. "So what did you want to discuss?"
"There’s still a lot of messages going back and forth between the agents I’ve identified," Lavinia said. "I’m certain that at least one of the representatives is working for Bolas."
Ral grimaced. "Anyone we can rule out?"
"Aside from you?" Lavinia said, smiling slightly. "Isperia is certainly above suspicion. And, while I may not like her methods, I can’t imagine Aurelia working with the enemies of Ravnica."
"The Gruul leader, Borborygmos, has been around for decades," Ral said. "I doubt he’s working for Bolas, though whether he can be persuaded to agree to #emph[anything] is anyone’s guess."
"I don’t know about that Rakdos emissary," Hekara said from the corner. "I hear she can’t be trusted." Ral and Lavinia turned to look at her, and she shrugged. "What?"
"That leaves five," Lavinia said. "Orzhov, Golgari, Dimir, Simic, and Selesnya."
"I killed Bolas’s agent at Selesnya," Ral said.
"Assuming he only had one."
"Fair." Ral frowned. "I still think we can trust Emmara."
"Simic is a black box," Lavinia said. "I have no information since they went on the defensive, when all this started."
"And I still think Lazav is the most likely problem," Ral said. "It was one of his mind mages who made the attempt on Niv-Mizzet."
"I don’t disagree," Lavinia said, with a sigh. "It just seems a little . . . #emph[obvious] , don’t you think? Lazav usually plays a subtler game than that."
"Maybe he’s getting lazy."
"Maybe." Lavinia shook her head. "That leaves Vraska and Kaya."
#emph[Kaya.] They’d gotten word that morning that the Planeswalker mercenary, rather than Teysa, had taken up the mantle of guildmaster of Orzhov. Ral didn’t know what was happening there, and he didn’t want to push Tomik for answers. #emph[We’ll have to deal with them either way.]
"Both Planeswalkers," Lavinia said. "Both new to control of their guilds."
"And they both signed on to help at Orzhova to help put the summit together," Ral pointed out. "If they were working for Bolas, wouldn’t they have sabotaged us already?"
"I don’t know," Lavinia said. "I’m closing in on some of Bolas’s agents, but unless I can catch them and make them talk I still don’t know what his game is."
"You’re running out of time." Ral leaned back in his chair. "The summit opens tomorrow. If you have iron-clad #emph[proof] that someone is working for Bolas, we can present it to the representatives, and everyone can deal with the traitor together. Anything short of that, and we’re just going to start a brawl on the spot."
"I understand that." Lavinia ran one hand through her hair, frustrated, pulling at knots. "I’m trying."
"I know," Ral said. "We’re nearly there. A month ago, I would have said it was impossible to get this far."
"So would I." Her lip quirked. "A lot can change in a month."
"A lot can change in a day."
#emph[Tomorrow.] He looked down at the papers on his desk, without really seeing them. #emph[For better or for worse.]
#v(0.35em)
#line(length: 100%, stroke: rgb(90%, 90%, 90%))
#v(0.35em)
Kaya wondered if this dullard priest was #emph[ever] going to shut up.
He was the High Something of Something, and was quite important, judging by the enormous black and gold hat he wore. (Hat size, Kaya had found, was often quite a good guide to someone’s importance—or at least their #emph[perceived ] importance—in an organization.) He had a long, wispy mustache, which wobbled as he spoke and made him look a bit like a walrus. #emph[High Walrus of Boredom, maybe?]
His speech, as best she could tell, was about the importance of debtors paying what they owed to the maintenance of a civil society. Which, all right, Kaya could get behind, but she didn’t see any need to ramble on about it for upwards of half an hour, while everyone in the cathedral sanctuary sat in the stifling heat of dozens of braziers while rain battered the stained-glass windows.
It wasn’t the first speech she’d endured today, either. She sat at the back of the sanctuary, on a very impressive throne that wasn’t actually particularly comfortable, and tried her best to smile knowingly as one guild official after another came to the lectern to regale the audience with homilies on duty and probity. Teysa sat beside her, stone-faced; she’d obviously been enduring this sort of affair since she was a girl, but it made Kaya want to slip through the floor and make a run for it.
As His Walrusness wound down, Kaya leaned toward Teysa. "I’m going to need a break."
"We’re coming up on the most important part," Teysa said. "The high officials will each swear their allegiance to you."
"Wonderful. But unless you want them to ask why the throne smells like piss, I need five minutes to run to the toilet."
Teysa sighed, but she made a small gesture to a functionary, who ran up and whispered in the ear of the walrus priest. After he’d reached the end of his remarks, he raised his hands to silence the weak round of applause, and said, "Honored guild members, we will take a brief recess before the Swearing of Oaths."
There was a rustle among the crowd. Kaya shot to her feet before anyone could try to talk to her, or Teysa could suggest she needed a proper escort or some such silliness. She pushed her way to the back of the dais, where a small door led to a corridor that wrapped around the sanctuary. From there, a stairway led up to the galleries, which were unoccupied for this relatively small service.
Kaya wasn’t sure if there were toilets up there. Honestly, what she really needed was a breath of clean air, but since that wasn’t going to happen unless she stuck her head through the wall, she’d settle for a few minutes without everyone staring at her.
#emph[How did I get ] into#emph[ this mess?] She could look back at the decisions she’d made, step by step, but somehow they didn’t seem to add up to her current predicament. #emph[Bolas set me up.] She grit her teeth. #emph[And he may be the only one who can get me out.]
Reaching the gallery, she went to the rail and leaned out, watching the dignitaries mill around below. For a moment, Kaya resisted a very strong urge to see if she could spit into some hats.
"Guildmaster?"
#emph[Oh, for the love of—]
She turned, and found herself facing a small, wizened man, with downcast eyes and liver-spotted hands. He held a broom like it was a cane, leaning on it for support, and his breath was wheezing. Her anger seeped away, and she shook her head.
"That’s me," she said. "Guildmaster. Absolutely. What can I do for you?"
"I beg a boon." Very slowly and with considerable effort, the old man fell to his knees. "Please."
"What kind of boon?"
"I wish forgiveness."
"Of your sins?" #emph[Is that something I do?]
"Of my debt, Guildmaster."
"Oh." Kaya pointed over her shoulder. "Paying debts is important. Didn’t you hear the High Whatnot?"
"I know, Guildmaster. But . . ."
"If you didn’t want to be in debt, you shouldn’t have borrowed money."
"My wife was ill," the old man said. "The doctor wanted much more than we could pay, so I turned to the Bank. My priest assured me the terms would be reasonable."
"When was this?"
"Forty years ago," the man said, his head bowed. "My wife died, in spite of the doctor’s efforts. I am an honest man, and I have worked for the Bank ever since. But there is still so much left, and I . . ." His hands twisted around the handle of his broom. "I will not live much longer, Guildmaster. I feel it in my bones. My only desire is that this burden that has crushed out my life not be passed on to my children."
"Your children inherit the debt?" Kaya said.
"Yes, Guildmaster. Such is the law."
"I . . ." Kaya shook her head. #emph[I should talk to Teysa.]
On the other hand, what was there to talk about? #emph[This poor bastard has already spent his life in chains. He doesn’t deserve to have his children bound as well.] It’s not like the sum involved could be enough to bother the Orzhov. #emph[Why not? I ] am#emph[ the Guildmaster, or I’m supposed to be.]
"All right," she said. "Your debt is forgiven. Run along."
"I . . ." He stayed on his knees. "The bond remains. I feel it—"
"In your bones. Right. Okay."
Kaya closed her eyes and took a deep breath, looking inside herself. She could feel the weight of the contracts and obligations she’d inherited from Grandfather Karlov, like thousands of chains hung around her neck. Telling one from the next was difficult, but it was easy enough to pick out the thread that led to the man standing directly in front of her, a black rope connecting his soul to hers. As she’d suspected, compared to some of the others, it was miniscule. With an effort of will, Kaya tore the bond apart.
She felt the impact as a moment of dizziness, but it quickly passed. At the same time, she heard the old man gasp, and when she looked up there were tears in his eyes.
"Thank you, Guildmaster," he said, voice cracking. "Thank you. My children . . ."
"It’s all right," Kaya said, seeing Teysa approaching. "Just, uh, don’t do it again. And continue to worship on . . . whatever day it is you’re supposed to."
"Yes, Guildmaster. Of course."
"Kaya," Teysa hissed. "Everyone is waiting."
"Sorry," Kaya said, watching the old man scuttle away. "I just had to . . . deal with something."
"Come on." Teysa patted her shoulder. "I know this is hard. I swear we’re working on a way to get you out of it."
"Good," Kaya said, shaking her head. "That’s . . . good."
#v(0.35em)
#line(length: 100%, stroke: rgb(90%, 90%, 90%))
#v(0.35em)
#linebreak "Are you certain you will not require more protection?" Mazirek said, in his slurred, clicking speech. "We could arrange a larger escort."
"It’ll be fine," Vraska said, with a little more certainty than she actually felt. "If this #emph[is] an ambush, then having more fighters isn’t going to help me get out of it. And it’s only going to make them nervous if I turn up with an army."
"As you say," the kraul clicked. "I will instruct the party to make ready for the journey to the surface."
"Good."
Vraska watched him depart, sitting on her throne with her chin in her hands. Her tendrils wriggled, unhappily. After a moment, she got to her feet.
"I’m going outside," she told Storrev, who waited as silent as ever beside the throne. The lich nodded, gesturing minutely, and four Erstwhile in long, half-rotted dresses fell into step behind her. Vraska ignored them. As bodyguards went, the zombies were about as unobtrusive as you could ask for, and they never talked back.
A door behind the throne led into a branching corridor. One way led to her private chambers, but she took the other branch, heading out to the vast balcony that wrapped around the back of the palace. Sometimes her guards used the space for military exercises, but today it was empty. The ground behind the palace dropped off rapidly, so the balcony looked out across a considerable vista, the darkness of the underground kingdom punctuated by the glowing lights of Golgari settlements or the faint phosphorescent glow of rot farms.
#emph[The surface dwellers will never understand this place] , she reflected. When they thought about the Golgari at all, they thought of them as monsters dwelling in filthy tunnels. There was more space down here, in the bones of Ravnica, then in every grand building up in the Tenth District. Without the reclamation efforts of the Golgari, and the food those efforts provided, the city would starve in days, if it didn’t drown in its own filth first. #emph[But to them we’ll always be monsters.] #emph[] #linebreak The shadow elves were unhappy that she was attending the guild summit. When she was in this sort of mood, Vraska saw their point. #emph[Why should we treat with people who hate us?] But she knew Bolas, and they didn’t. She’d seen in Jace’s mind what the dragon had done to the people and gods of Amonkhet. #emph[If that’s what he plans for Ravnica . . .] #emph[] #linebreak "Queen," a man’s voice said. "You’re not an easy person to reach."
Vraska turned. A shadow elf stood by the balcony rail, dressed in the worn leather of a rot farmer. She dropped one hand to her saber.
"I thought we’d finished with this assassination nonsense," she said. "But I suppose that was too optimistic of me. Come on, then."
"I’m not here to kill you," the man said, walking closer. As he did, the Erstwhile behind Vraska moved up protectively, until they were standing at her shoulder. The man paid them no mind. "I’m just here to express my . . . concern."
"Your concern? What is that supposed to mean?"
"You’re not playing the role you were assigned, my dear." The elf grinned, but Vraska saw a shadow of another smile, one full of jagged teeth.
"Bold of you, to come here," Vraska said, showing her own fangs. "You’ll make a fine addition to my sculpture garden."
"Oh, this is only a vessel. Tear it to pieces or turn it to stone, it’s all the same to me," said the elf. The voice was definitely Bolas. "It won’t change the fact that we had a deal, and you have not followed through."
"I found you what you wanted on Ixalan."
"You did." Bolas inclined his head. "But that was never supposed to be the end of it. I installed you as the head of the Golgari, as #emph[I] promised. And yet here I find you working against me."
"I had a change of heart," Vraska snarled.
"I can see that. My old friend Beleren’s work, I suspect."
"Take this #emph[thing] to the dungeons," Vraska snapped at her zombies. "Tell Mazirek to see how long he can keep it alive." When the elf raised an eyebrow, Vraska added, "Next time you come to lecture me, maybe you’ll be brave enough to do it in person."
"If I have to lecture you again, Vraska, it will be the last time," Bolas’s puppet said.
"Bold words."
The elf snapped something fast in a language Vraska didn’t understand. The four zombies, which had advanced to take hold of him, stopped in their tracks.
"I think that you can still play your part," Bolas said.
"I said to take him," Vraska said, one hand on her saber again.
Bolas muttered another word, and the zombies turned, advancing now on Vraska. She swore and drew her sword, backing up to put the palace wall behind her.
"The Erstwhile," Bolas said, moving up behind them. "So useful. So willing to help you overthrow Jarad and his sycophants. And they ask for nothing in return." He smiled. "Extraordinary."
"I can handle a few zombies," Vraska said. "If this is supposed to threaten me—"
"Oh, I’m not threatening you," Bolas said. "Physical threats rarely mean much to a Planeswalker, do they? But your precious #emph[people] , that’s another matter."
He snapped another command, and the zombies froze. Vraska didn’t lower her sword.
"Imagine every Erstwhile turning on its master, just as they did to Jarad and his elves," Bolas said. "But this would be so much worse. You prepared your coup carefully. This time, it would simply be chaos. No doubt the remaining Devkarin would take it as their chance to reclaim power. The kraul would certainly fight back. It would be civil war, with you caught in the middle. Everything you love would be torn to pieces."
"You . . ." Vraska’s throat was thick.
"I #emph[made] you the head of the Golgari," Bolas said. "Did you really think I would do that without a way to #emph[un] make you, if I chose?" He stepped closer, leaning between two of the motionless zombies. "Live as long as I have, and you’ll learn about betrayal. Did you really think I hadn’t anticipated yours?"
"What . . ." Vraska felt her tendrils flailing in agitation, and tried to clamp down. "What do you #emph[want] from me?"
"I want you to make the correct choice. Either the Golgari can thrive, under your leadership, and claim their rightful place in my new order. Or else they can be torn asunder, here and now, and when I come to this plane I will take particular pleasure in grinding what’s left of them under my claws. And only once the last remnants of your pathetic tribes are dust will I come for #emph[you] , Vraska. And you will know an eternity of pain."
She felt golden energy building behind her eyes, her instinctive threat response, but she blinked it away. Turning this messenger to stone wouldn’t help. #emph[Nothing] would help.
#emph[Jace . . .] #emph[] #linebreak She’d trusted him with her memories, her #emph[identity] , the next best thing to her soul. #emph[He said he would come] . That, together, they’d defeat Bolas.
#emph[But he’s not here.] And this #emph[thing] was, a dragon’s face grinning out from a puppet body, standing behind its tame zombies. #emph[Jace, what am I supposed to do?] #emph[] #linebreak "Well?" Bolas smile faded. "What’s it going to be?"
Vraska swallowed.
|
|
https://github.com/ukihot/igonna | https://raw.githubusercontent.com/ukihot/igonna/main/articles/rust/syntax.typ | typst | #import "@preview/codly:0.2.0": *
#let ruby(rt, rb, size: 0.4em, alignment: "between") = {
let gutter = if (alignment=="center" or alignment=="start") {h(0pt)} else if (alignment=="between" or alignment=="around") {h(1fr)}
let chars = if(alignment=="around") {
[#h(0.5fr)#rt.clusters().join(gutter)#h(0.5fr)]
} else {
rt.clusters().join(gutter)
}
style(st=> {
let bodysize = measure(rb, st)
let rt_plain = text(size: size, rt)
let width = if(measure(rt_plain, st).width > bodysize.width) {measure(rt_plain, st).width} else {bodysize.width}
let rubytext = box(width: width, align(if(alignment=="start"){left}else{center}, text(size: size, chars)))
let textsize = measure(rubytext, st)
let x = if(alignment=="start"){0pt}else{bodysize.width/2-textsize.width/2}
box[#place(top+left, dx: x, dy: -size*1.2, rubytext)#rb]
})
}
#let icon(codepoint) = {
box(
height: 0.8em,
baseline: 0.05em,
image(codepoint)
)
h(0.1em)
}
#show: codly-init.with()
#codly(languages: (
rust: (name: "Rust", icon: icon("brand-rust.svg"), color: rgb("#CE412B")),
))
== コーディング <syntax>
プログラムを開発する作業全般をプログラミングといい,特にソースコードを書く工程をコーディングという。
コーディングにおいて、シンタックスとデザインパターンは中核を成す。
シンタックスとは、プログラミング言語の仕様として定められた構文規則を指す。
デザインパターンについてはWiki#footnote[https://w.wiki/rvm]を引用する。
#quote(attribution: [Wikipedia])[ソフトウェア開発におけるデザインパターンまたは設計パターン(英: design pattern)とは、過去のソフトウェア設計者が発見し編み出した設計ノウハウを蓄積し、名前をつけ、再利用しやすいように特定の規約に従ってカタログ化したものである。]
これより、シンタックスに関連する事項を説明する。
通常、伝統的なアプローチでは「ハローワールド関数」から始めることが一般的だが、本書では変数に関する説明から始める。
== 変数
以下のコードはどのような処理か考えてみよう。
```rust
let x = 4;
```
`x`は変数、4は整数リテラル#footnote[リテラルとはソースコードに生で書かれたデータであり、値そのものである。例えば、4(リテラル)に5(値)を束縛することはできない。]である。
それと同時に、`x`は4を束縛(bind)する所有者である。
`let`は変数宣言のキーワードとなっており、以下の構文を想定している。
```rust
let PATTERN = EXPRESSION;
```
整数リテラル4単体であっても、右辺は式である。
式が返却する値は4であり、左辺のパターン`x`に当てはめて束縛される。
パターンであるという認識はとても重要で、以下はエラーにならない。
```rust
let (a, b) = (4, 5);
```
ただし、パターンには2つの形式が存在する。上記の_Irrefutable_(#ruby("ろんばく")[論駁]不可能)なパターンと、`let`式で扱うことができない_Refutable_(論駁可能)なパターンである。
この_Refutability_(論駁可能性)の概念については、エラーメッセージで見かけた際に対応できるように、慣れておく必要がある。
_Refutable_なパターンはまた別の章で触れる。
変数宣言は、値`4`に別のラベル`x`を張り替えるような作業ではなく、所有者`x`に`4`が束縛されるという認識をもってほしい。
== 型
Rust標準のプリミティブ型を表にした。
型の名前に含まれる数字は、ポインタのサイズ(ビット)を指す。
#table(
columns: (1fr, auto),
inset: 1em,
align: horizon,
[*基本データ型*], [*型*],
"符号付き整数",
"isize, i8, i16, i32, i64",
"符号無し整数",
"usize, u8, u16, u32, u64",
"浮動小数点数",
"f32, f64",
"文字(Unicodeのスカラー値)",
"char"
)
特に数値を表す型の許容値は以下となる。
#table(columns: (1fr, 1fr, auto),align: auto,
[*型*], [*MIN*], [*MAX*],
"i8", $-128$, $127$,
"i16", $-32768$, $32767$,
"i32", $-2^32$, $2^32-1$,
"i64", $-2^64$, $-2^64-1$,
"u8", $0$, $255$,
"u16", $0$, $65535$,
"u32", $0$, $2^32$,
"u64", $0$, $2^64$,
"f32", "凡そ-21億", "凡そ+21億",
"f64", "凡そ-9京", "凡そ+9京",
)
isizeおよびusizeは実行環境PCのCPUのビット数によって適切なサイズに変化する。
=== 型推論
初期化の際に評価値の型をチェックするだけでなく、その後にどのような使われ方をしているかを見て推論する。
== 関数
ハローワールドプログラムを観察する。
```rust
fn main() {
println!("Hello, world!");
}
```
== コメント
== 制御フロー
=== if
=== loop
=== while
=== for |
|
https://github.com/VisualFP/docs | https://raw.githubusercontent.com/VisualFP/docs/main/SA/design_concept/content/results/results_ui_demonstration.typ | typst | #import "../../../acronyms.typ": *
= UI Demonstration
@visual-fp-ui-demo-step-one through @visual-fp-ui-demo-step-six depict a
step-by-step construction of the `mapAdd5`
code scenario, described in @design_eval_code_scenarios, using the #ac("PoC") application.
#figure(
image("../../static/ui-demo-one.png"),
caption: "Step by step demonstration of mapAdd5 construction - Part 1"
) <visual-fp-ui-demo-step-one>
#figure(
image("../../static/ui-demo-two.png"),
caption: "Step by step demonstration of mapAdd5 construction - Part 2"
) <visual-fp-ui-demo-step-two>
#figure(
image("../../static/ui-demo-three.png"),
caption: "Step by step demonstration of mapAdd5 construction - Part 3"
) <visual-fp-ui-demo-step-three>
#figure(
image("../../static/ui-demo-four.png"),
caption: "Step by step demonstration of mapAdd5 construction - Part 4"
) <visual-fp-ui-demo-step-four>
#figure(
image("../../static/ui-demo-five.png"),
caption: "Step by step demonstration of mapAdd5 construction - Part 5"
) <visual-fp-ui-demo-step-five>
#figure(
image("../../static/ui-demo-six.png"),
caption: "Step by step demonstration of mapAdd5 construction - Part 6"
) <visual-fp-ui-demo-step-six>
|
|
https://github.com/mitsuyukiLab/grad_thesis_typst | https://raw.githubusercontent.com/mitsuyukiLab/grad_thesis_typst/main/main.typ | typst | #import "lib/grad_thesis_lib.typ": thesis, toc, toc_img, toc_table
#show: thesis.with(
type: "卒業論文", // "卒業論文", "修士論文", "博士論文"
title: "論文タイトルを入れる入れる \n 君の名は",
title_en: "Please input your thesis title \n Your Name",
submittion_date: "2050年3月4日",
supervisor_name: "<NAME>",
supervisor_title: "准教授",
author_name: "<NAME>",
author_student_id: "11QA111",
author_affiliation_1: "横浜国立大学",
author_affiliation_2: "理工学部", // "大学院理工学府", "大学院先進実践学環"
author_affiliation_3: "機械・材料・海洋系学科", // "機械・材料・海洋系工学専攻", "リスク共生学"
author_affiliation_4: "海洋空間のシステムデザインEP", // "海洋空間教育分野", none
)
// ------------------------------------------------
// 表紙の次にBlank Pageを挿入する
#pagebreak()
#pagebreak()
// ------------------------------------------------
// ------------------------------------------------
// Abstractと目次用の設定
#set page(numbering: "i", number-align: center)
#counter(page).update(1)
// ------------------------------------------------
#include "contents/abstract.typ"
#toc()
#pagebreak()
#toc_img()
#pagebreak()
#toc_table()
// ------------------------------------------------
// 本文用の設定
#set page(numbering: "1", number-align: center)
#counter(page).update(1)
// -- ----------------------------------------------
// ここで各章のファイルを読み込む
#include "contents/how_to_use.typ" // 最終的にはコメントアウトしてください
#include "contents/introduction.typ"
#include "contents/related_study.typ"
#include "contents/proposed_method.typ"
#include "contents/case_study.typ"
#include "contents/discussion.typ"
#include "contents/conclusion.typ"
#include "contents/acknowledgement.typ"
// 参考文献
#[
#set text(lang: "en")
#bibliography(title: "参考文献", style:"ieee", "references.bib")
]
|
|
https://github.com/sitandr/typst-examples-book | https://raw.githubusercontent.com/sitandr/typst-examples-book/main/src/packages/glossary.md | markdown | MIT License | # Glossary
## glossarium
>[Link to the universe](https://typst.app/universe/package/glossarium)
Package to manage glossary and abbreviations.
<div class="info">One of the very first cool packages of Typst, made specially for (probably) the first thesis written in Typst.<div>
```typ
#import "@preview/glossarium:0.4.1": make-glossary, print-glossary, gls, glspl
#show: make-glossary
// for better link visibility
#show link: set text(fill: blue.darken(60%))
#print-glossary(
(
// minimal term
(key: "kuleuven", short: "KU Leuven"),
// a term with a long form and a group
(key: "unamur", short: "UNamur", long: "Namur University", group: "Universities"),
// a term with a markup description
(
key: "oidc",
short: "OIDC",
long: "OpenID Connect",
desc: [OpenID is an open standard and decentralized authentication protocol promoted by the non-profit
#link("https://en.wikipedia.org/wiki/OpenID#OpenID_Foundation")[OpenID Foundation].],
group: "Accronyms",
),
// a term with a short plural
(
key: "potato",
short: "potato",
// "plural" will be used when "short" should be pluralized
plural: "potatoes",
desc: [#lorem(10)],
),
// a term with a long plural
(
key: "dm",
short: "DM",
long: "diagonal matrix",
// "longplural" will be used when "long" should be pluralized
longplural: "diagonal matrices",
desc: "Probably some math stuff idk",
),
)
)
// referencing the OIDC term using gls
#gls("oidc")
// displaying the long form forcibly
#gls("oidc", long: true)
// referencing the OIDC term using the reference syntax
@oidc
Plural: #glspl("potato")
#gls("oidc", display: "whatever you want")
```
|
https://github.com/Myriad-Dreamin/typst.ts | https://raw.githubusercontent.com/Myriad-Dreamin/typst.ts/main/packages/typst.node/__test__/inputs/post2.typ | typst | Apache License 2.0 | #set document(title: "Post 2")
= This is Post 2
|
https://github.com/katamyra/Notes | https://raw.githubusercontent.com/katamyra/Notes/main/Compiled%20School%20Notes/CS3001/Modules/Privacy.typ | typst | #import "../../../template.typ": *
= Privacy
*What is Privacy?*
- Freedom from intrusion
- Control of information about oneself
- Freedom from surveillance
== Free Market View
#theorem[
From the free market view, you can choose how much information you want to exchange and if you don't like a company's privacy practices, don't do business with them.
]
== Consumer Protection View
#theorem[
Privacy is a positive right, and you can't negotiate terms with a business even if you wanted. Often, customers have few to no alternatives.
Furthermore, people couldn't read privacy policies even if they tried because the reading level is too high and it would take too much time.
]
*Benefits of Privacy*
- Lets people be themselves (you act differently if someone is watching you)
- Lets us remove our "public persona"
- Important for ones individuality and freedom
*Harms of Privacy*
- Difficulty to stop family violence
- Allows for easier coverup of illegal or immoral actions
Privacy is a *prudential right* (a right stemming from sound judgement/practical wisdom)
- Rational agents recognize some privacy rights because granting these is of overall benefit to society
*How do computers change privacy?*
- Computers add to the ease of collecting, searching, cross referencing personal info
- Makes it easier to use information for secondary purposes
- Basically reasons other than why we collected it
- Can be combined with other info
- Laws written don't adapt well to new technologies
|
|
https://github.com/AnsgarLichter/light-cv | https://raw.githubusercontent.com/AnsgarLichter/light-cv/main/modules/utils.typ | typst | MIT License | #let vLine() = [
#h(5pt)
]
#let hline() = [
#box(width: 1fr, line(stroke: 0.9pt, length: 100%))
] |
https://github.com/Many5900/aau-typst | https://raw.githubusercontent.com/Many5900/aau-typst/main/custom.typ | typst | #import "@preview/showybox:2.0.1": *
#import "@preview/codly:1.0.0": *
#import "@preview/dashy-todo:0.0.1": todo
//####################################//
//############ CODE BLOCK ############//
//####################################//
#let code(lang: "", title, body) = {
if title != [] {
[
#codly(
smart-indent: true,
languages: (
rust: (
name: [#lang],
color: rgb("#211A51"),
),
),
inset: .32em,
lang-inset: .5em,
lang-outset: (x: -3pt, y: 6pt),
lang-radius: 2pt,
lang-stroke: (lang) => 1pt + lang.color,
lang-fill: (lang) => lang.color.lighten(75%)
)
#showybox(
frame: (
border-color: rgb("#211A51"),
title-color: rgb("#211A51"),
radius: 2pt,
title-inset: (x: 1em, y: 1em),
body-inset: (x: 1pt, y: 1pt),
),
title-style: (
color: white,
weight: "regular",
align: left,
),
shadow: (
offset: 3pt,
),
title: [*#title*],
[#body],
)
]
} else {
[
#codly(
smart-indent: true,
languages: (
rust: (
name: [#lang],
color: rgb("#211A51"),
),
),
inset: .32em,
lang-inset: .5em,
lang-outset: (x: -3pt, y: 6pt),
lang-radius: 2pt,
lang-stroke: (lang) => 1pt + lang.color,
lang-fill: (lang) => lang.color.lighten(75%)
)
#showybox(
frame: (
border-color: rgb("#211A51"),
title-color: rgb("#211A51"),
radius: 2pt,
title-inset: (x: 1em, y: 1em),
body-inset: (x: 1pt, y: 1pt),
),
title-style: (
color: white,
weight: "regular",
align: left,
),
shadow: (
offset: 3pt,
),
[#body],
)
]
}
}
//#############################//
//############ BOX ############//
//#############################//
#let box(title: "", showy-body, footer) = {
if footer != [] {
showybox(
frame: (
border-color: rgb("#211A51"),
title-color: rgb("#211A51"),
radius: 2pt,
title-inset: (x: 1em, y: 1em)
),
footer-style: (
sep-thickness: 0pt,
color: black,
align: center
),
title-style: (
color: white,
weight: "regular",
align: left,
),
footer: (
[#footer]
),
shadow: (
offset: 3pt,
),
title: [*#title*],
[#showy-body],
)
} else {
showybox(
frame: (
border-color: rgb("#211A51"),
title-color: rgb("#211A51"),
radius: 2pt,
title-inset: (x: 1em, y: 1em)
),
title-style: (
color: white,
weight: "regular",
align: left,
),
shadow: (
offset: 3pt,
),
title: [*#title*],
[#showy-body],
)
}
}
//##############################//
//############ NOTE ############//
//##############################//
#let note(body, title: "") = {
showybox(
title-style: (
weight: 900,
color: rgb("#211A51"),
sep-thickness: 0pt,
align: center
),
frame: (
title-color: rgb("#211A51").lighten(75%),
border-color: rgb("#211A51"),
thickness: (left: 2.5pt),
radius: 2pt
),
shadow: (
offset: (x: .5pt, y: .5pt),
color: rgb("#211A51").lighten(50%)
),
title: [#title]
)[
#body
]
} |
|
https://github.com/0x1B05/algorithm-journey | https://raw.githubusercontent.com/0x1B05/algorithm-journey/main/practice/note/content/图.typ | typst | #import "../template.typ": *
#pagebreak()
= 图
== 建图
- 邻接矩阵(适合点的数量不多的图)
- 邻接表(最常用的方式)
- 链式前向星(空间要求严苛情况下使用。比赛必用,大厂笔试、面试不常用)
#code(caption: [三种方式建图])[
```java
import java.util.ArrayList;
import java.util.Arrays;
/**
* Code01_CreateGraph
*/
public class Code01_CreateGraph {
// 点的最大数量
public static int MAXN = 11;
// 边的最大数量
public static int MAXM = 21;
// 邻接矩阵
public static int[][] graph1 = new int[MAXN][MAXN];
// 邻接表
// 无权图
// public static ArrayList<ArrayList<Integer>> graph2;
// 有权图
public static ArrayList<ArrayList<int[]>> graph2 = new ArrayList<>();
// 链式前向星
public static int[] head = new int[MAXN];
public static int[] next = new int[MAXM];
public static int[] to = new int[MAXM];
public static int[] weight = new int[MAXM];
public static int cnt = 1;
// n->节点数量
public static void build(int n) {
// 邻接矩阵清空
for (int i = 1; i <= n; i++) {
for (int j = 1; j <= n; j++) {
graph1[i][j] = 0;
}
}
// 邻接表清空
graph2.clear();
// 要准备n+1个,0 下标不用
for (int i = 0; i <= n; i++) {
graph2.add(new ArrayList<>());
}
// 链式前向星清空
cnt = 1;
Arrays.fill(head, 1, n + 1, 0);
}
// 链式前向星加边
public static void addEdge(int u, int v, int w) {
// u -> v , 边权重是w
to[cnt] = v;
weight[cnt] = w;
next[cnt] = head[u];
head[u] = cnt++;
}
// 建立有向图带权图
public static void directGraph(int[][] edges) {
// edge[i][0]->start edge[i][1]->end edge[i][2]->weight
// 邻接矩阵加边
for (int[] edge : edges) {
graph1[edge[0]][edge[1]] = edge[2];
}
// 邻接表加边
for (int[] edge : edges) {
// graph2.get(edge[0]).add(edge[1]);
graph2.get(edge[0]).add(new int[] {edge[1], edge[2]});
}
// 链式前向星加边
for (int[] edge : edges) {
addEdge(edge[0], edge[1], edge[2]);
}
}
// 建立有向图带权图
public static void undirectGraph(int[][] edges) {
// edge[i][0]->start edge[i][1]->end edge[i][2]->weight
// 邻接矩阵加边
for (int[] edge : edges) {
graph1[edge[0]][edge[1]] = edge[2];
graph1[edge[1]][edge[0]] = edge[2];
}
// 邻接表加边
for (int[] edge : edges) {
// graph2.get(edge[0]).add(edge[1]);
// graph2.get(edge[1]).add(edge[0]);
graph2.get(edge[0]).add(new int[] {edge[1], edge[2]});
graph2.get(edge[1]).add(new int[] {edge[0], edge[2]});
}
// 链式前向星加边
for (int[] edge : edges) {
addEdge(edge[0], edge[1], edge[2]);
addEdge(edge[1], edge[0], edge[2]);
}
}
public static void traversal(int n) {
System.out.println("邻接矩阵遍历: ");
for (int i = 1; i <= n; i++) {
for (int j = 1; j <= n; j++) {
System.out.println(i + "->" + j + " weight:" + graph1[i][j]);
}
}
System.out.println("邻接表遍历: ");
for (int i = 1; i <= n; i++) {
for (int[] edge : graph2.get(i)) {
System.out.println(i + "->" + edge[0] + " weight:" + edge[1]);
}
}
System.out.println("链式前向星遍历: ");
for (int i = 1; i <= n; i++) {
for (int ei = head[i]; ei > 0; ei = next[ei]) {
System.out.println(i + "->" + to[ei] + " weight:" + weight[ei]);
}
}
}
public static void main(String[] args) {
// 理解了带权图的建立过程,也就理解了不带权图
// 点的编号为1...n
// 例子1自己画一下图,有向带权图,然后打印结果
int n1 = 4;
int[][] edges1 = {{1, 3, 6}, {4, 3, 4}, {2, 4, 2}, {1, 2, 7}, {2, 3, 5}, {3, 1, 1}};
build(n1);
directGraph(edges1);
traversal(n1);
System.out.println("==============================");
// 例子2自己画一下图,无向带权图,然后打印结果
int n2 = 5;
int[][] edges2 = {
{3, 5, 4}, {4, 1, 1}, {3, 4, 2}, {5, 2, 4}, {2, 3, 7}, {1, 5, 5}, {4, 2, 6}};
build(n2);
undirectGraph(edges2);
traversal(n2);
}
}
```
]
== 拓扑排序
每个节点的前置节点都在这个节点之前
要求:有向图、没有环
拓扑排序的顺序可能不只一种。拓扑排序也可以用来判断有没有环。
1)在图中找到所有入度为0的点
2)把所有入度为0的点在图中删掉,重点是删掉影响!继续找到入度为0的点并删掉影响
3)直到所有点都被删掉,依次删除的顺序就是正确的拓扑排序结果
4)如果无法把所有的点都删掉,说明有向图里有环
=== 题目1: 拓扑排序模板
==== #link("https://leetcode.cn/problems/course-schedule-ii/")[leetcode模板]
现在你总共有 `numCourses` 门课需要选,记为 `0` 到 `numCourses - 1`。给你一个数组 `prerequisites` ,其中 `prerequisites[i] = [ai, bi]` ,表示在选修课程 `ai` 前 必须 先选修 `bi` 。
例如,想要学习课程 `0` ,你需要先完成课程 `1` ,我们用一个匹配来表示:`[0,1]` 。
返回你为了学完所有课程所安排的学习顺序。可能会有多个正确的顺序,你只要返回 任意一种 就可以了。如果不可能完成所有课程,返回 一个空数组 。
#example("Example")[
- 输入:`numCourses = 4, prerequisites = [[1,0],[2,0],[3,1],[3,2]]`
- 输出:`[0,2,1,3]`
- 解释:总共有 `4` 门课程。要学习课程 `3`,你应该先完成课程 `1` 和课程 `2`。并且课程 `1` 和课程 `2` 都应该排在课程 `0` 之后。因此,一个正确的课程顺序是 `[0,1,2,3]` 。另一个正确的排序是 `[0,2,1,3]` 。
]
#tip("Tip")[
- `1 <= numCourses <= 2000`
- `0 <= prerequisites.length <= numCourses * (numCourses - 1)`
- `prerequisites[i].length == 2`
- `0 <= ai, bi < numCourses`
- `ai != bi`
- 所有`[ai, bi]` 互不相同
]
#code(caption: [leetcode拓扑排序模板])[
```java
public class Code02_TopoSortDynamicLeetcode {
public static int[] findOrder(int n, int[][] pre) {
// pre[i][1] -> pre[i][0]
ArrayList<ArrayList<Integer>> graph = new ArrayList<>();
int[] indegree = new int[n];
// 初始化
for (int i = 0; i < n; i++) {
graph.add(new ArrayList<>());
}
// 建图
for (int[] edge : pre) {
graph.get(edge[1]).add(edge[0]);
indegree[edge[0]]++;
}
// 初始化queue
int[] queue = new int[n];
int l = 0, r = 0;
for (int i = 0; i < n; i++) {
if (indegree[i] == 0) {
queue[r++] = i;
}
}
// 逐渐干掉入度为0的节点
int cnt = 0;
while (l < r) {
int cur = queue[l++];
cnt++;
for (int next : graph.get(cur)) {
if (--indegree[next] == 0) {
queue[r++] = next;
}
}
}
return cnt==n?queue:new int[0];
}
}
```
]
==== #link("https://www.nowcoder.com/practice/88f7e156ca7d43a1a535f619cd3f495c")[newcoder模板(邻接表动态)]
给定一个包含`n`个点`m`条边的有向无环图,求出该图的拓扑序。若图的拓扑序不唯一,输出任意合法的拓扑序即可。若该图不能拓扑排序,输出`−1`。
- 输入描述:
- 第一行输入两个整数`n`,`m`( `1≤n,m≤2*10^5`),表示点的个数和边的条数。
- 接下来的m行,每行输入两个整数`ui`,`vi`(`1≤u,v≤n`),表示`ui`到`vi`之间有一条有向边。
- 输出描述:
- 若图存在拓扑序,输出一行n个整数,表示拓扑序。否则输出`−1`。
#example("Example")[
- 输入:
```
5 4
1 2
2 3
3 4
4 5
```
- 输出:
```
1 2 3 4 5
```
]
#code(caption: [newcoder拓扑排序模板-静态])[
```java
public class Code02_TopoSortDynamicNowcoder {
public static int MAXN = 200001;
public static int MAXM = 200001;
public static int l,r;
public static int n,m;
public static int[] indgree = new int[MAXN];
public static int[] queue = new int[MAXN];
public static boolean topSort(ArrayList<ArrayList<Integer>> graph) {
l = 0; r = 0;
for (int i = 1; i <= n; i++) {
if(indgree[i]==0){
queue[r++] = i;
}
}
int cnt = 0;
while(l<r){
int cur = queue[l++];
cnt++;
for (int next : graph.get(cur)) {
if(--indgree[next]==0){
queue[r++] = next;
}
}
}
boolean ans = cnt==n?true:false;
return ans;
}
public static void main(String[] args) throws IOException {
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
StreamTokenizer in = new StreamTokenizer(br);
PrintWriter out = new PrintWriter(new OutputStreamWriter(System.out));
while (in.nextToken() != StreamTokenizer.TT_EOF) {
n = (int) in.nval;
in.nextToken();
ArrayList<ArrayList<Integer>> graph = new ArrayList<>();
for (int i = 0; i <= n; i++) {
graph.add(new ArrayList<>());
}
m = (int) in.nval;
in.nextToken();
for (int i = 0; i < m; i++) {
int from = (int) in.nval;
in.nextToken();
int to = (int) in.nval;
in.nextToken();
graph.get(from).add(to);
indgree[to]++;
}
if (!topSort(graph)) {
out.println(-1);
} else {
for (int i = 0; i < n-1; i++) {
System.out.print(queue[i] + " ");
}
out.println(queue[n-1]);
}
}
out.flush();
out.close();
br.close();
}
}
```
]
==== #link("https://www.nowcoder.com/practice/88f7e156ca7d43a1a535f619cd3f495c")[newcoder模板(邻接表静态)]
#code(caption: [newcoder拓扑排序模板-静态])[
```java
public class Code02_TopoSortStaticNowcoder {
public static int MAXN = 200001;
public static int MAXM = 200001;
public static int l,r;
public static int n,m;
public static int cnt;
public static int[] head = new int[MAXN];
public static int[] next = new int[MAXM];
public static int[] to = new int[MAXM];
public static int[] indgree = new int[MAXN];
public static int[] queue = new int[MAXN];
public static void init(){
cnt = 1;
Arrays.fill(head, 0,n+1,0);
Arrays.fill(indgree, 0,n+1,0);
}
public static void addEdge(int from, int t){
next[cnt] = head[from];
to[cnt] = t;
head[from] = cnt++;
}
public static boolean topSort() {
l=0;r=0;
for (int i = 1; i <= n; i++) {
if (indgree[i]==0) {
queue[r++] = i;
}
}
int cnt = 0;
while(l<r){
int cur = queue[l++];
cnt++;
for (int ei = head[cur]; ei > 0; ei = next[ei]) {
indgree[to[ei]]--;
if(indgree[to[ei]]==0){
queue[r++] = to[ei];
}
}
}
return cnt==n;
}
public static void main(String[] args) throws IOException {
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
StreamTokenizer in = new StreamTokenizer(br);
PrintWriter out = new PrintWriter(new OutputStreamWriter(System.out));
while (in.nextToken() != StreamTokenizer.TT_EOF) {
n = (int) in.nval;
in.nextToken();
init();
m = (int) in.nval;
in.nextToken();
for (int i = 0; i < m; i++) {
int from = (int) in.nval;
in.nextToken();
int t = (int) in.nval;
in.nextToken();
addEdge(from, t);
indgree[t]++;
}
if (!topSort()) {
out.println(-1);
} else {
for (int i = 0; i < n-1; i++) {
System.out.print(queue[i] + " ");
}
out.println(queue[n-1]);
}
}
out.flush();
out.close();
br.close();
}
}
```
]
==== #link("https://www.luogu.com.cn/problem/U107394")[洛谷模板-字典序最小的拓扑排序]
有向无环图上有`n`个点,`m`条边。求这张图字典序最小的拓扑排序的结果。字典序最小指希望排好序的结果中,比较靠前的数字尽可能小。
- 输入格式
- 第一行是用空格隔开的两个整数`n`和`m`,表示`n`个点和`m`条边。
- 接下来是`m`行,每行用空格隔开的两个数`u`和`v`,表示有一条从`u`到`v`的边。
- 输出格式
- 输出一行,拓扑排序的结果,数字之间用空格隔开
#example("Example")[
- 样例输入
```
5 3
1 2
2 4
4 3
```
- 样例输出
```
1 2 4 3 5
```
]
#tip("Tip")[
- $1 lt.eq n,m lt.eq 10^5$
- 图上可能有重边
]
=== #link("https://leetcode.cn/problems/Jf1JuT/description/")[题目2: 火星词典]
现有一种使用英语字母的外星文语言,这门语言的字母顺序与英语顺序不同。 给定一个字符串列表 `words` ,作为这门语言的词典,`words` 中的字符串已经 按这门新语言的字母顺序进行了排序 。 请你根据该词典还原出此语言中已知的字母顺序,并 _按字母递增顺序_ 排列。若不存在合法字母顺序,返回 `""` 。若存在多种可能的合法字母顺序,返回其中 任意一种 顺序即可。
#tip("Tip")[
- 字符串 `s` 字典顺序小于 字符串 `t` 有两种情况:
- 在第一个不同字母处,如果 `s` 中的字母在这门外星语言的字母顺序中位于 `t` 中字母之前,那么 `s` 的字典顺序小于 `t` 。
- 如果前面 `min(s.length, t.length)` 字母都相同,那么 `s.length < t.length` 时,`s` 的字典顺序也小于 `t` 。
]
#example("Example")[
- 输入:words = ["wrt","wrf","er","ett","rftt"]
- 输出:"wertf"
]
#example("Example")[
- 输入:words = ["z","x"]
- 输出:"zx"
]
#example("Example")[
- 输入:words = ["z","x","z"]
- 输出:""
- 解释:不存在合法字母顺序,因此返回 "" 。
]
#tip("Tip")[
- 1 <= `words.length` <= 100
- 1 <= `words[i].length` <= 100
- `words[i]` 仅由小写英文字母组成
]
=== #link("https://leetcode.cn/problems/stamping-the-sequence")[ 题目3: 戳印序列]
你想要用小写字母组成一个目标字符串 `target`。
开始的时候,序列由 `target.length` 个 `'?' `记号组成。而你有一个小写字母印章 stamp。 在每个回合,你可以将印章放在序列上,并将序列中的每个字母替换为印章上的相应字母。你最多可以进行 `10 * target.length` 个回合。
举个例子,如果初始序列为` "?????"`,而你的印章 `stamp` 是` "abc"`,那么在第一回合,你可以得到 `"abc??"`、`"?abc?"`、`"??abc"`。(请注意,印章必须完全包含在序列的边界内才能盖下去。)
如果可以印出序列,那么返回一个数组,该数组由每个回合中被印下的最左边字母的索引组成。如果不能印出序列,就返回一个空数组。
例如,如果序列是 `"ababc"`,印章是 `"abc"`,那么我们就可以返回与操作 `"?????"` -> `"abc??"` -> `"ababc"` 相对应的答案 `[0, 2]`;
另外,如果可以印出序列,那么需要保证可以在 `10 * target.length` 个回合内完成。任何超过此数字的答案将不被接受。
#example("Example")[
- 输入:stamp = "abc", target = "ababc"
- 输出:[0,2]
- ([1,0,2] 以及其他一些可能的结果也将作为答案被接受)
]
#example("Example")[
- 输入:stamp = "abca", target = "aabcaca"
- 输出:[3,0,1]
]
#tip("Tip")[
- `1 <= stamp.length <= target.length <= 1000`
- `stamp` 和 `target` 只包含小写字母。
]
== 最小生成树
#definition("Definition")[
最小生成树:在 _无向带权图_ 中选择择一些边,在 _保证连通性_ 的情况下,边的总权值最小
]
- 最小生成树可能不只一棵,只要保证边的总权值最小,就都是正确的最小生成树
- 如果无向带权图有`n`个点,那么最小生成树一定有`n-1`条边(多一条会成环,少一条保证不了连通性)
=== Kruskal算法(最常用,不需要建图)
1. 把所有的边,根据权值从小到大排序,从权值小的边开始考虑
2. 如果连接当前的边不会形成环,就选择当前的边
3. 如果连接当前的边会形成环,就不要当前的边
4. 考察完所有边之后/已经够`n-1`条边了,最小生成树的也就得到了
贪心策略,证明略!
==== #link("https://www.luogu.com.cn/problem/P3366")[最小生成树洛谷模板]
给出一个无向图,求出最小生成树,如果该图不连通,则输出 `orz`。
- 输入格式
- 第一行包含两个整数 `N`,`M`,表示该图共有 `N` 个结点和 `M` 条无向边。
- 接下来 `M` 行每行包含三个整数 `Xi`,`Yi`,`Zi`表示有一条长度为 `Zi` 的无向边连接结点 `Xi`,`Yi`。
- 输出格式
- 如果该图连通,则输出一个整数表示最小生成树的各边的长度之和。如果该图不连通则输出 `orz`。
#example("Example")[
- 输入
```
4 5
1 2 2
1 3 2
1 4 3
2 3 4
3 4 3
```
- 输出:`7`
]
#tip("Tip")[
- `1≤N≤5000`
- `1≤M≤2×10^5`
- `1≤Zi≤10^4`
]
#code(caption: [Kruskal])[
```java
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.io.OutputStreamWriter;
import java.io.PrintWriter;
import java.io.StreamTokenizer;
import java.util.Arrays;
/**
* Code01_Kruskal
*/
public class Code01_Kruskal {
public static int MAXN = 5001;
public static int MAXM = 200001;
public static int n, m;
public static int[][] edges = new int[MAXM][3];
public static int[] father = new int[MAXN];
public static int ans = 0;
public static void main(String[] args) throws IOException {
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
StreamTokenizer in = new StreamTokenizer(br);
PrintWriter out = new PrintWriter(new OutputStreamWriter(System.out));
while (in.nextToken() != StreamTokenizer.TT_EOF) {
n = (int) in.nval;
in.nextToken();
init();
m = (int) in.nval;
for (int i = 0; i < m; i++) {
in.nextToken();
edges[i][0] = (int) in.nval;
in.nextToken();
edges[i][1] = (int) in.nval;
in.nextToken();
edges[i][2] = (int) in.nval;
}
out.println(compute() ? ans : "orz");
}
out.flush();
out.close();
br.close();
}
public static void init() {
for (int i = 1; i <= n; i++) {
father[i] = i;
}
}
public static boolean union(int x, int y) {
int fx = find(x);
int fy = find(y);
if (fx != fy) {
father[fx] = fy;
return true;
} else {
return false;
}
}
public static int find(int x) {
if (father[x] != x) {
father[x] = find(father[x]);
}
return father[x];
}
public static boolean compute() {
Arrays.sort(edges, 0, m, (a, b) -> (a[2] - b[2]));
int edgeCnt = 0;
for (int[] edge : edges) {
if (union(edge[0], edge[1])) {
ans += edge[2];
edgeCnt++;
}
}
return edgeCnt == n - 1;
}
}
```
]
时间复杂度`O(m * log m) + O(n) + O(m)`
- 排序`O(m * log m)`
- 建立并查集`O(n)`
- 遍历所有的边`O(m)`
=== Prim算法(不算常用)
1. 解锁的点的集合叫`set`(普通集合)、解锁的边的集合叫`heap`(小根堆)。`set`和`heap`都为空。
2. 可从任意点开始,开始点加入到`set`,开始点的所有边加入到`heap`
3. 从`heap`中弹出权值最小的边`e`,查看边`e`所去往的点`x`
1. 如果`x`已经在`set`中,边`e`舍弃,重复步骤3
2. 如果`x`不在`set`中,边`e`属于最小生成树,把`x`加入`set`,重复步骤3
4. 当`heap`为空,最小生成树的也就得到了
贪心策略,证明略!
==== #link("https://www.luogu.com.cn/problem/P3366")[最小生成树洛谷模板]
#code(caption: [Prim])[
```java
public class Code02_PrimDynamic {
public static void main(String[] args) throws IOException{
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
StreamTokenizer in = new StreamTokenizer(br);
PrintWriter out = new PrintWriter(new OutputStreamWriter(System.out));
while(in.nextToken()!=StreamTokenizer.TT_EOF){
ArrayList<ArrayList<int[]>> graph = new ArrayList<>();
int n = (int) in.nval;
for (int i = 0; i <= n; i++) {
graph.add(new ArrayList<>());
}
in.nextToken();
int m = (int) in.nval;
for (int i = 0, from, to, weight; i < m; i++) {
in.nextToken();
from = (int) in.nval;
in.nextToken();
to = (int) in.nval;
in.nextToken();
weight = (int) in.nval;
graph.get(from).add(new int[]{to,weight});
graph.get(to).add(new int[]{from,weight});
}
int ans = prim(graph);
out.println(ans == -1 ? "orz":ans);
}
out.flush();
out.close();
br.close();
}
public static int prim(ArrayList<ArrayList<int[]>> graph) {
int n = graph.size()-1;
int nodeCnt = 0;
int ans = 0;
boolean[] set = new boolean[n+1];
PriorityQueue<int[]> heap = new PriorityQueue<>((a, b)->(a[1]-b[1]));
for (int[] e : graph.get(1)) {
heap.add(e);
}
set[1] = true;
nodeCnt++;
while(!heap.isEmpty()){
int[] top = heap.poll();
int to = top[0];
int weight = top[1];
if(!set[to]){
ans+=weight;
nodeCnt++;
set[to]=true;
for (int[] e : graph.get(to)) {
heap.add(e);
}
}
}
return nodeCnt == n ? ans:-1;
}
}
```
]
时间复杂度`O(n + m) + O(m * log m)`
- 建图`O(n + m)`
- 堆的操作,每次`2m`条边弹出,`2m`条边加入`O(m * log m)`
==== Prim算法的优化(比较难,不感兴趣可以跳过)
1. 小根堆里放(节点,到达节点的花费),根据 到达节点的花费 来组织小根堆
2. 小根堆弹出(`u`节点,到达`u`节点的花费`y`),`y`累加到总权重上去,然后考察`u`出发的每一条边
假设,`u`出发的边,去往`v`节点,权重`w`
1. 如果`v`已经弹出过了(发现过),忽略该边
2. 如果`v`从来没有进入过堆,向堆里加入记录`(v, w)`
3. 如果`v`在堆里,且记录为`(v, x)`
1. 如果`w < x`,则记录更新成`(v, w)`,然后调整该记录在堆中的位置(维持小根堆)
2. 如果`w >= x`,忽略该边
3. 重复步骤2,直到小根堆为空
#tip("Tip")[
- 同一个节点的记录不重复入堆!这样堆时间复杂度只和节点数有关。
- 反向索引表的用处,已知某边的大小要改变,要在堆里找到这个边。
]
时间复杂度`O(n+m) + O((m+n) * log n)`
==== #link("https://www.luogu.com.cn/problem/P3366")[Prim 洛谷模板]
#code(caption: [Prim - 反向索引堆])[
```java
public class Code02_PrimStatic {
public static int MAXN = 5001;
public static int MAXM = 400001;
public static int n,m;
// 链式前向星
public static int[] head = new int[MAXN];
public static int[] to = new int[MAXM];
public static int[] next = new int[MAXM];
public static int[] weight = new int[MAXM];
public static int cnt;
// 反向索引堆
public static int[][] heap = new int[MAXN][2];
// where[v] = -1,表示v这个节点,从来没有进入过堆
// where[v] = -2,表示v这个节点,已经弹出过了
// where[v] = i(>=0),表示v这个节点,在堆上的i位置
public static int[] where = new int[MAXN];
public static int heapSize;
// 最小生成树已经找到的节点数
public static int nodeCnt;
// 最小生成树的权重和
public static int ans;
public static void build(){
cnt = 1;
heapSize = 0;
nodeCnt = 0;
Arrays.fill(head, 1, n+1, 0);
Arrays.fill(where, 1, n+1, -1);
}
public static void addEdge(int f, int t, int w){
to[cnt] = t;
weight[cnt] = w;
next[cnt] = head[f];
head[f] = cnt++;
}
// 当前处于cur,向上调整成堆
public static void heapInsert(int cur){
int parent = (cur-1)/2;
while(heap[parent][1]>heap[cur][1]){
swap(parent, cur);
cur = parent;
parent = (cur-1)/2;
}
}
// 当前处于cur,向下调整成堆
public static void heapify(int cur){
int left = 2*cur+1;
while(left<heapSize){
int right = left+1;
int minChild = (right<heapSize && heap[right][1]<heap[left][1])?right:left;
int min = heap[minChild][1]<heap[cur][1]?minChild:cur;
if(min==cur){
break;
}else{
swap(minChild, cur);
cur = minChild;
left = 2*cur+1;
}
}
}
// 堆上i位置与j位置交换
public static void swap(int i, int j){
// where的交换
int a = heap[i][0];
int b = heap[j][0];
where[a] = j;
where[b] = i;
// 元素的交换
int[] tmp = heap[i];
heap[i] = heap[j];
heap[j] = tmp;
}
public static boolean isEmpty(){
return heapSize==0 ? true:false;
}
public static int popTo, popWeight;
public static void pop(){
popTo = heap[0][0];
popWeight = heap[0][1];
swap(0, --heapSize);
heapify(0);
where[popTo] = -2;
nodeCnt++;
}
// 点在堆上的记录要么新增,要么更新,要么忽略
// 当前处理编号为cur的边
public static void addOrUpdateOrIgnore(int cur){
int t = to[cur];
int w = weight[cur];
if(where[t]==-1){
// 从来没进入过
heap[heapSize][0] = t;
heap[heapSize][1] = w;
where[t] = heapSize++;
heapInsert(where[t]);
}else if(where[t]>=0){
// 已经在堆里
// 谁小留谁
heap[where[t]][1] = Math.min(heap[where[t]][1], w);
heapInsert(where[t]);
}
}
public static void main(String[] args) throws IOException{
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
StreamTokenizer in = new StreamTokenizer(br);
PrintWriter out = new PrintWriter(new OutputStreamWriter(System.out));
while(in.nextToken()!=StreamTokenizer.TT_EOF){
n = (int) in.nval;
in.nextToken();
m = (int) in.nval;
build();
for (int i = 0, f, t, w; i < m; i++) {
in.nextToken();
f = (int) in.nval;
in.nextToken();
t = (int) in.nval;
in.nextToken();
w = (int) in.nval;
addEdge(f, t, w);
addEdge(t, f, w);
}
int ans = prim();
out.println(nodeCnt == n ? ans : "orz");
}
out.flush();
out.close();
br.close();
}
public static int prim(){
// 从1节点出发
nodeCnt = 1;
where[1] = -2;
for (int ei = head[1]; ei > 0; ei=next[ei]) {
addOrUpdateOrIgnore(ei);
}
while(!isEmpty()){
pop();
ans += popWeight;
for (int ei = head[popTo]; ei > 0; ei=next[ei]) {
addOrUpdateOrIgnore(ei);
}
}
return ans;
}
}
```
]
=== #link("https://leetcode.cn/problems/optimize-water-distribution-in-a-village/")[题目3: 水资源分配优化]
村里面一共有 `n` 栋房子。我们希望通过建造水井和铺设管道来为所有房子供水。
对于每个房子 `i`,我们有两种可选的供水方案:
- 一种是直接在房子内建造水井: 成本为 `wells[i - 1]` (注意 `-1` ,因为 索引从`0`开始 )
- 另一种是从另一口井铺设管道引水,数组 `pipes` 给出了在房子间铺设管道的成本, 其中每个 `pipes[j] = [house1j, house2j, costj]` 代表用管道将 `house1j` 和 `house2j`连接在一起的成本。连接是双向的。
请返回 为所有房子都供水的最低总成本
#example("Example")[
- 输入: `n = 3`, `wells = [1,2,2]`, `pipes = [[1,2,1],[2,3,1]]`
- 输出: `3`
- 解释: 最好的策略是在第一个房子里建造水井(成本为1),然后将其他房子铺设管道连起来(成本为2),所以总成本为3。
]
#tip("Tip")[
- `1 <= n <= 10000`
- `wells.length == n`
- `0 <= wells[i] <= 10^5`
- `1 <= pipes.length <= 10000`
- `1 <= pipes[i][0], pipes[i][1] <= n`
- `0 <= pipes[i][2] <= 10^5`
- `pipes[i][0] != pipes[i][1]`
]
==== 解答:
*假设有一个水源地,所谓的村庄打井的代价相当于从村庄到水源地的管道的代价。*
#code(caption: [水资源分配优化 - 解答])[
```java
public class Code03_OptimizeWaterDistribution {
public static int MAXN = 10001;
// edge[cnt][0] -> 起始点
// edge[cnt][1] -> 结束点
// edge[cnt][2] -> 代价
public static int[][] edge = new int[MAXN << 1][3];
// cnt代表边的编号
public static int cnt;
public static int[] father = new int[MAXN];
public static void init(int n){
cnt = 0;
for (int i = 0; i <= n; i++) {
father[i] = i;
}
}
public static int find(int i){
if(i!=father[i]){
father[i] = find(father[i]);
}
return father[i];
}
public static boolean union(int x, int y){
int fx = find(x);
int fy = find(y);
if(fx!=fy){
father[fx] = fy;
return true;
}else{
return false;
}
}
public static int minCostToSupplyWater(int n, int[] wells, int[][] pipes) {
init(n);
for (int i = 0; i < n; i++, cnt++) {
edge[cnt][0] = 0;
edge[cnt][1] = i+1;
edge[cnt][2] = wells[i];
}
for (int i = 0; i < pipes.length; i++, cnt++) {
edge[cnt][0] = pipes[i][0];
edge[cnt][1] = pipes[i][1];
edge[cnt][2] = pipes[i][2];
}
Arrays.sort(edge,(a, b)->a[2]-b[2]);
int ans = 0;
for(int i = 0; i < cnt; i++){
if(union(edge[i][0], edge[i][1])){
ans += edge[i][2];
}
}
return ans;
}
}
```
]
=== #link("https://leetcode.cn/problems/checking-existence-of-edge-length-limited-paths/")[题目4: 检查边长度限制的路径是否存在]
给你一个 `n` 个点组成的无向图边集 `edgeList` ,其中 `edgeList[i] = [ui, vi, disi]` 表示点 `ui` 和点 `vi` 之间有一条长度为 `disi` 的边。请注意,两个点之间可能有 超过一条边 。
给你一个查询数组`queries` ,其中 `queries[j] = [pj, qj, limitj]` ,你的任务是对于每个查询 `queries[j]` ,判断是否存在从 `pj` 到 `qj` 的路径,且这条路径上的每一条边都 严格小于 `limitj` 。
请你返回一个 布尔数组 `answer` ,其中 `answer.length == queries.length` ,当 `queries[j]` 的查询结果为 `true` 时, `answer` 第 `j` 个值为 `true` ,否则为 `false` 。
#example("Example")[
- 输入:`n = 3`, `edgeList = [[0,1,2],[1,2,4],[2,0,8],[1,0,16]]`, `queries = [[0,1,2],[0,2,5]]`
- 输出:`[false,true]`
- 解释:上图为给定的输入数据。注意到 `0` 和 `1` 之间有两条重边,分别为 `2` 和 `16` 。
- 对于第一个查询,`0` 和 `1` 之间没有小于 `2` 的边,所以我们返回 `false` 。
- 对于第二个查询,有一条路径(`0` -> `1` -> `2`)两条边都小于 `5` ,所以这个查询我们返回 `true`
]
#tip("Tip")[
- `2 <= n <= 10^5`
- `1 <= edgeList.length, queries.length <= 10^5`
- `edgeList[i].length == 3`
- `queries[j].length == 3`
- `0 <= ui, vi, pj, qj <= n - 1`
- `ui != vi`
- `pj != qj`
- `1 <= disi, limitj <= 10^9`
]
==== 解答
#code(caption: [检查边长度限制的路径是否存在 - 解答])[
```java
public class Code04_CheckingExistenceOfEdgeLengthLimit {
public static int MAXN = 100001;
public static int[][] questions = new int[MAXN][4];
public static int[] father = new int[MAXN];
public static void init(int n){
for (int i = 0; i < n; i++) {
father[i] = i;
}
}
public static int find(int i){
if(i!=father[i]){
father[i] = find(father[i]);
}
return father[i];
}
public static void union(int x, int y){
father[find(x)] = find(y);
}
public static boolean isSameSet(int x, int y){
return find(x)==find(y);
}
public static boolean[] distanceLimitedPathsExist(int n, int[][] edges, int[][] queries) {
int m = edges.length;
int k = queries.length;
boolean[] ans = new boolean[k];
init(n);
for (int i = 0; i < k; i++) {
questions[i][0] = queries[i][0];
questions[i][1] = queries[i][1];
questions[i][2] = queries[i][2];
questions[i][3] = i;
}
Arrays.sort(questions, 0, k, (a, b)->a[2]-b[2]);
Arrays.sort(edges, (a, b)->a[2]-b[2]);
// 当前已经连了的节点数量
int nodeCnt = 0;
for (int i = 0; i < k; i++) {
while(nodeCnt<m && edges[nodeCnt][2]<questions[i][2]){
union(edges[nodeCnt][0], edges[nodeCnt][1]);
nodeCnt++;
}
ans[questions[i][3]] = isSameSet(questions[i][0], questions[i][1]);
}
return ans;
}
}
```
]
=== #link("https://www.luogu.com.cn/problem/P2330")[题目5: 繁忙的都市 ]
#definition("Definition")[
- 瓶颈生成树: 无向图 $G$ 的瓶颈生成树是这样的一个生成树,它的最大的边权值在 $G$ 的所有生成树中最小。
- 性质: 最小生成树是瓶颈生成树的充分不必要条件。 即最小生成树一定是瓶颈生成树,而瓶颈生成树不一定是最小生成树。
- 反证法证明:我们设最小生成树中的最大边权为 $w$ ,如果最小生成树不是瓶颈生成树的话,则瓶颈生成树的所有边权都小于 $w$ ,我们只需删去原最小生成树中的最长边,用瓶颈生成树中的一条边来连接删去边后形成的两棵树,得到的新生成树一定比原最小生成树的权值和还要小,这样就产生了矛盾。
]
城市 C 是一个非常繁忙的大都市,城市中的道路十分的拥挤,于是市长决定对其中的道路进行改造。城市 C 的道路是这样分布的:城市中有 $n$ 个交叉路口,有些交叉路口之间有道路相连,两个交叉路口之间最多有一条道路相连接。这些道路是双向的,且把所有的交叉路口直接或间接的连接起来了。每条道路都有一个分值,分值越小表示这个道路越繁忙,越需要进行改造。但是市政府的资金有限,市长希望进行改造的道路越少越好,于是他提出下面的要求:
+ 改造的那些道路能够把所有的交叉路口直接或间接的连通起来。
+ 在满足要求 1 的情况下,改造的道路尽量少。
+ 在满足要求 1、2 的情况下,改造的那些道路中分值最大的道路分值尽量小。
任务:作为市规划局的你,应当作出最佳的决策,选择哪些道路应当被修建。
- 输入格式
- 第一行有两个整数 $n,m$ 表示城市有 $n$ 个交叉路口,$m$ 条道路。接下来 $m$ 行是对每条道路的描述,$u, v, c$ 表示交叉路口 $u$ 和 $v$ 之间有道路相连,分值为 $c$。
- 输出格式
- 两个整数 $s, "max"$,表示你选出了几条道路,分值最大的那条道路的分值是多少。
#example("Example")[
- 样例输入
```
4 5
1 2 3
1 4 5
2 4 7
2 3 6
3 4 8
```
- 输出
```
3 6
```
]
#tip("Tip")[
对于全部数据,满足 $1 <= n <= 300$,$1 <= c <= 10^4$,$1 <= m <= 8000$。
]
==== 解答
#code(caption: [繁忙的都市 - 解答])[
```java
public class Code05_BusyCities {
public static int MAXN = 301;
public static int MAXM = 8001;
public static int n,m;
public static int[][] edges = new int[MAXM][3];
public static int[] father = new int[MAXN];
public static void init(){
for (int i = 1; i <= n; i++) {
father[i] = i;
}
}
public static int find(int i){
if(i!=father[i]){
father[i] = find(father[i]);
}
return father[i];
}
public static boolean union(int x, int y){
int fx = find(x);
int fy = find(y);
if(fx!=fy){
father[fx] = fy;
return true;
}else{
return false;
}
}
public static boolean isSameSet(int x, int y){
return find(x)==find(y);
}
public static void main(String[] args) throws IOException{
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
StreamTokenizer in = new StreamTokenizer(br);
PrintWriter out = new PrintWriter(new OutputStreamWriter(System.out));
while(in.nextToken()!=StreamTokenizer.TT_EOF){
n = (int)in.nval;
in.nextToken();
m = (int)in.nval;
init();
for (int i = 0; i < m; i++) {
in.nextToken();
edges[i][0] = (int) in.nval;
in.nextToken();
edges[i][1] = (int) in.nval;
in.nextToken();
edges[i][2] = (int) in.nval;
}
Arrays.sort(edges, 0, m, (a, b)->a[2]-b[2]);
int ans = 0;
int edgeCnt = 0;
for (int[] edge : edges) {
if(union(edge[0], edge[1])){
edgeCnt++;
ans = Math.max(ans, edge[2]);
}
if(edgeCnt==n-1){
break;
}
}
out.println((n - 1) + " " + ans);
}
out.flush();
out.close();
br.close();
}
}
```
]
|
|
https://github.com/dainbow/MatGos | https://raw.githubusercontent.com/dainbow/MatGos/master/themes/10.typ | typst | #import "../conf.typ": *
= Экстремумы функций многих переменных. Необходимые условия, достаточные условия.
== Необходимые условия
#definition[
Точка $x_0 in RR^n$ называется точкой *локального максимума* функции $f(x)$,
если
#eq[
$exists delta > 0 : forall x in U_delta (x_0) : space f(x) <= f(x_0)$
]
]
#definition[
Точка $x_0 in RR^n$ называется точкой *локального минимума* функции $f(x)$, если
#eq[
$exists delta > 0 : forall x in U_delta (x_0) : space f(x) >= f(x_0)$
]
]
#definition[
Точка $x_0 in RR^n$ называется точкой *строгого локального максимума* функции $f(x)$,
если
#eq[
$exists delta > 0 : forall x in U_delta (x_0) : space f(x) < f(x_0)$
]
]
#definition[
Точка $x_0 in RR^n$ называется точкой *строгого локального минимума* функции $f(x)$,
если
#eq[
$exists delta > 0 : forall x in U_delta (x_0) : space f(x) > f(x_0)$
]
]
#theorem(
"Необходимые условия локального экстремума",
)[
Если $x_0$ -- точка локального экстремума функции $f(x)$, дифференцируемой в
окрестности точки $x_0$, то $dif f(x) equiv 0$.
]
#proof[
Рассмотрим для каждого $k = overline("1, n")$:
#eq[
$psi(x_k) = f(x_0^1, ..., x_0^(k - 1), x_k, x_0^(k + 1), ..., x_0^n), space "где" x_0 = (x_0^1, ..., x_0^n)$
]
Тогда заметим, что $psi$ дифференцируема в окрестности $x_0^k$, применяя теорему
о необходимом условии экстремума функции одного переменного, получим
#eq[
$psi'(x_0^k) = 0 => (partial f) / (partial x_k)(x_0) = 0$
]
В силу произвольности $k$ и того, что дифференциал -- это вектор частных
производных, получим требуемое.
]
== Достаточные условия
#definition[
Если $f$ дифференцируема в окрестности точки $x_0$ и $dif f(x_0) equiv 0$, то $x_0$ называется
*стационарной точкой* функции $f$.
]
#theorem(
"Достаточные условия локального экстремума",
)[
Если $x_0$ -- стационарная точка функции $f$, дважды дифференцируемой в точке $x_0$,
то
+ Если $dif^2f(x_0)$ -- положительно определённая квадратичная форма, то $x_0$ -- точка
строгого локального минимума функции $f$
+ Если $dif^2f(x_0)$ -- отрицательно определённая квадратичная форма, то $x_0$ -- точка
строгого локального максимума функции $f$
+ Если $dif^2f(x_0)$ -- неопределённая квадратичная форма, то $x_0$ не является
точкой локального экстремума
]
#proof[
+ По формуле Тейлора с остаточным членом в форме Пеано:
#eq[
$f(x) = f(x_0) + dif f(x_0) + 1 / 2 d^2 f(x_0) + o(rho^2), rho -> 0$
]
где
#eq[
$ dif x_k = x_k - x_0^k, k = overline("1,n"); quad rho = sqrt(sum_(k = 1)^n (x_k - x_0^k)^2) = norm(dif x)_2$
]
Тогда (в условиях $dif f(x_0) equiv 0$ и $xi_k := (dif x_k) / norm(dif x)$) :
#eq[
$ f(x) - f(x_0) = 1 / 2 d^2 f(x_0) + o(rho^2) = \
1 / 2 rho^2 (
underbrace(
sum_(i, j = 1)^n (partial^2 f) / (partial x_i partial x_j)(x_0) xi_i xi_j,
F(xi_1, ..., xi_n),
) + o(1),
) rho -> 0$
]
В следствие нормировки, очевидно, $sum_(i = 1)^n xi_i^2 = 1$.
Таким образом, минимум введённого функционала $F$ на сфере (компактной в $RR^n$)
будет достигаться:
#eq[
$min_(xi_1^2 + ... + xi_n^2 = 1) F(xi_1, ..., xi_n) =: C > 0$
]
Таким образом, для достаточно маленьких $rho$:
#eq[
$f(x) - f(x_0) >= C / 4 rho^2 > 0$
]
+ Аналогично
+ Вводим $F(xi_1, ..., xi_n)$ аналогично предыдущим пунктам, из-за того что $dif^2 f$ -- неопределённая,
то
#eq[
$exists xi_1(x_1), xi_2(x_2) : space F(xi_1^1, ..., xi_1^n) > 0 and F(xi_2^1, ..., xi_2^n) < 0$
]
Тогда при достаточно малых $rho$:
#eq[
$"sign" (f(x_1) - f(x_0)) = "sign" F(xi_1) > 0 ; "sign" (f(x_2) - f(x_0)) = "sign" F(xi_2) < 0$
]
Что и требовалось.
]
== Условный экстремум
#definition[
Пусть $f, phi_1, dots, phi_m$ заданы на множестве $G subset R^n$, причем $m < n$.
Тогда $x_0 in G$ называется точкой _условного локального экстемума_ если $forall x in U_delta(x_0) and phi_i(x) = 0 : space f(x) <= (>=) f(x_0) $
]
#lemma[
Пусть $f, phi_1, dots, phi_m$ непрерывно дифференцируемы в окрестности $x_0$, причем ранг якобина $(partial phi_1 dots phi_m) / (partial x_1 dots x_n)$ максимален $(=m)$
БОО считаем, что главный минор не нулевой
Тогда задача поиска локального экстемума эквивалентна задаче поиска безусловного
]
#proof[
В силу теоремы о неявной функции, $exists psi_1 dots psi_n$
#eq[$
x_i = psi_i(x_m, dots, x_n) <=> phi(x_1, dots, x_n) = 0
$]
Отсюда
#eq[$
d x_i = d psi_i(x_m, dots, x_n) <=> d phi(x_1, dots, x_n) = 0
$]
Тогда:
$space F(x_1 ,dots, x_n) = F(psi_1 (x_(m+1), dots, x_n), dots, psi_m (x_(m+1), dots, x_n), x_(m+1), dots, x_n)$.
То есть это действительно задача безусловного экстремума.
]
#definition[
Функцией Лагранжа в условиях задачи посика экстремума для функции $F$ называет функция $L(x_1, dots, x_n, lambda_1, dots, lambda_m) = F + sum lambda_i phi_i$
]
#theorem[_Необходимые условия локального экстемума_
Пусть $f, phi_1, dots, phi_m$ дифференцируемы в окрестности $x_0$. $x_0$ точка локального экстемума, при выполнении условий связи, и максимальности ранга Якобина.
Тогда $exists ! lambda_1,dots, lambda_m: space (x_1, dots, x_n, lambda_1, dots, lambda_m)$ стационарная точка функции Лагранжа.
]
#proof[
Сведем как показано выше задачу к безусловному экстемуму $F(x_(m+1), dots, x_n)$, тогда $d F = 0 => sum (partial F) / (partial x_i) d x_i = 0$. Где $d x_i = d psi_i forall i in 1 dots m$. Тогда в силу продифференцированных условий связи $d phi_i = 0$.
Отсюда $d F + sum lambda_j d phi_j = 0$.
То есть $sum_(j=m+1)^n ((partial F) / (partial x_j) + sum lambda_k (partial phi_k) / (partial x_j) ) d x_j = 0$
Тогда в точке $x_0$ составим систему уравнений на $lambda_1,dots,lambda_m$, чтобы $sum_(j=1)^m ((partial F) / (partial x_j) + sum lambda_k (partial phi_k) / (partial x_j) ) d x_j = 0$. У неё существует единсвенное решение.
Следовательно $sum_(j=1)^n (partial L)/(partial x_j)(x_0) d x_j = 0$
Заметим, что $(partial L) / (partial lambda_k) = phi_k = 0$.
Итого $d L = 0$
]
#definition[
Обозначим $d^2 _(x x) L(x_0, lambda_0) $ второй диференциал функции Лагранжа как функции только от $x$. Причем эта квадратичная форма рассматривается как форма от переменных $d x_1 dots d x_n $ починеных уравнению связи.
]
#theorem[_Достаточное услоие условного экстемума_
Пусть $f, phi_1, dots, phi_m$ дважды непрерывно дифференцируемы в окрестности $x_0$. $x_0$ точка локального экстемума, при выполнении условий связи, и максимальности ранга Якобина, ($x_0, lambda_0$) стационарная точка функции Лагранжа. Тогда если
+ $d^2 _(x x) L(x_0, lambda_0) $ положительно определения то это усл минимум
+ $d^2 _(x x) L(x_0, lambda_0) $ относительно определения то это усл максимум
+ $d^2 _(x x) L(x_0, lambda_0) $ неопределённая то это не экстемум
] |
|
https://github.com/soul667/typst | https://raw.githubusercontent.com/soul667/typst/main/PPT/typst-slides-fudan/themes/polylux/examples/demo.typ | typst | #import "../polylux.typ": *
#import themes.clean: *
#show link: set text(blue)
#set text(font: "Inria Sans")
// #show heading: set text(font: "Vollkorn")
#show raw: set text(font: "JuliaMono")
#show: clean-theme.with(
footer: [<NAME>, July 2023],
short-title: [Polylux demo],
logo: image("../assets/logo.png"),
)
#set text(size: 20pt)
#title-slide(
title: [`Polylux`: Easily creating slides in Typst],
subtitle: "An overview over all the features",
authors: "<NAME>",
date: "April 2023",
)
#new-section-slide("Introduction")
#slide(title: "About this presentation")[
This presentation is supposed to briefly showcase what you can do with this
package.
For a full documentation, read the
#link("https://andreaskroepelin.github.io/polylux/book/")[online book].
]
#slide(title: "A title")[
Let's explore what we have here.
On the top of this slide, you can see the slide title.
We used the `title` argument of the `#slide` function for that:
```typ
#slide(title: "First slide")[
...
]
```
(This works because we utilise the `clean` theme; more on that later.)
]
#slide[
Titles are not mandatory, this slide doesn't have one.
But did you notice that the current section name is displayed above that
top line?
We defined it using
#raw("#new-section-slide(\"Introduction\")", lang: "typst", block: false).
This helps our audience with not getting lost after a microsleep.
You can also spot a short title above that.
]
#slide(title: "The bottom of the slide")[
Now, look down!
There we have some general info for the audience about what talk they are
actually attending right now.
You can also see the slide number there.
]
#new-section-slide("Dynamic content")
#slide(title: [A dynamic slide with `pause`s])[
Sometimes we don't want to display everything at once.
#let pc = 1
#{ pc += 1 } #show: pause(pc)
That's what the `pause` function is there for!
Use it as
```typ
#show: pause(n)
```
#{ pc += 1 } #show: pause(pc)
It makes everything after it appear at the `n`-th subslide.
#text(.6em)[(Also note that the slide number does not change while we are here.)]
]
#slide(title: "Fine-grained control")[
When `#pause` does not suffice, you can use more advanced commands to show
or hide content.
These are your options:
- `#uncover`
- `#only`
- `#alternatives`
- `#one-by-one`
- `#line-by-line`
Let's explore them in more detail!
]
#let example(body) = block(
width: 100%,
inset: .5em,
fill: aqua.lighten(80%),
radius: .5em,
text(size: .8em, body)
)
#slide(title: [`#uncover`: Reserving space])[
With `#uncover`, content still occupies space, even when it is not displayed.
For example, #uncover(2)[these words] are only visible on the second "subslide".
In `()` behind `#uncover`, you specify _when_ to show the content, and in
`[]` you then say _what_ to show:
#example[
```typ
#uncover(3)[Only visible on the third "subslide"]
```
#uncover(3)[Only visible on the third "subslide"]
]
]
#slide(title: "Complex display rules")[
So far, we only used single subslide indices to define when to show something.
We can also use arrays of numbers...
#example[
```typ
#uncover((1, 3, 4))[Visible on subslides 1, 3, and 4]
```
#uncover((1, 3, 4))[Visible on subslides 1, 3, and 4]
]
...or a dictionary with `beginning` and/or `until` keys:
#example[
```typ
#uncover((beginning: 2, until: 4))[Visible on subslides 2, 3, and 4]
```
#uncover((beginning: 2, until: 4))[Visible on subslides 2, 3, and 4]
]
]
#slide(title: "Convenient rules as strings")[
As as short hand option, you can also specify rules as strings in a special
syntax.
Comma separated, you can use rules of the form
#table(
columns: (auto, auto),
column-gutter: 1em,
stroke: none,
align: (x, y) => (right, left).at(x),
[`1-3`], [from subslide 1 to 3 (inclusive)],
[`-4`], [all the time until subslide 4 (inclusive)],
[`2-`], [from subslide 2 onwards],
[`3`], [only on subslide 3],
)
#example[
```typ
#uncover("-2, 4-6, 8-")[Visible on subslides 1, 2, 4, 5, 6, and from 8 onwards]
```
#uncover("-2, 4-6, 8-")[Visible on subslides 1, 2, 4, 5, 6, and from 8 onwards]
]
]
#slide(title: [`#only`: Reserving no space])[
Everything that works with `#uncover` also works with `#only`.
However, content is completely gone when it is not displayed.
For example, #only(2)[#text(red)[see how]] the rest of this sentence moves.
Again, you can use complex string rules, if you want.
#example[
```typ
#only("2-4, 6")[Visible on subslides 2, 3, 4, and 6]
```
#only("2-4, 6")[Visible on subslides 2, 3, 4, and 6]
]
]
#slide(title: [`#alternatives`: Substituting content])[
You might be tempted to try
#example[
```typ
#only(1)[Ann] #only(2)[Bob] #only(3)[Christopher] likes #only(1)[chocolate] #only(2)[strawberry] #only(3)[vanilla] ice cream.
```
#only(1)[Ann] #only(2)[Bob] #only(3)[Christopher]
likes
#only(1)[chocolate] #only(2)[strawberry] #only(3)[vanilla]
ice cream.
]
But it is hard to see what piece of text actually changes because everything
moves around.
Better:
#example[
```typ
#alternatives[Ann][Bob][Christopher] likes #alternatives[chocolate][strawberry][vanilla] ice cream.
```
#alternatives[Ann][Bob][Christopher] likes #alternatives[chocolate][strawberry][vanilla] ice cream.
]
]
#slide(title: [`#one-by-one`: An alternative for `#pause`])[
`#alternatives` is to `#only` what `#one-by-one` is to `#uncover`.
`#one-by-one` behaves similar to using `#pause` but you can additionally
state when uncovering should start.
#example[
```typ
#one-by-one(start: 2)[one ][by ][one]
```
#one-by-one(start: 2)[one ][by ][one]
]
`start` can also be omitted, then it starts with the first subside:
#example[
```typ
#one-by-one[one ][by ][one]
```
#one-by-one[one ][by ][one]
]
]
#slide(title: [`#line-by-line`: syntactic sugar for `#one-by-one`])[
Sometimes it is convenient to write the different contents to uncover one
at a time in subsequent lines.
This comes in especially handy for bullet lists, enumerations, and term lists.
#example[
#grid(
columns: (1fr, 1fr),
gutter: 1em,
```typ
#line-by-line(start: 2)[
- first
- second
- third
]
```,
line-by-line(start: 2)[
- first
- second
- third
]
)
]
`start` is again optional and defaults to `1`.
]
#slide(title: "Different ways of covering content")[
When content is covered, it is completely invisible by default.
However, you can also just display it in light gray by using the
`mode` argument with the value `"transparent"`:
#let pc = 1
#{ pc += 1 } #show: pause(pc, mode: "transparent")
Covered content is then displayed differently.
#{ pc += 1 } #show: pause(pc, mode: "transparent")
Every `uncover`-based function has an optional `mode` argument:
- `#show: pause(...)`
- `#uncover(...)[...]`
- `#one-by-one(...)[...][...]`
- `#line-by-line(...)[...][...]`
]
#new-section-slide("Themes")
#slide(title: "How a slide looks...")[
... is defined by the _theme_ of the presentation.
This demo uses the `clean` theme.
Because of it, the title slide and the decoration on each slide (with
section name, short title, slide number etc.) look the way they do.
Themes can also provide variants, for example ...
]
#focus-slide[
... this one!
It's very minimalist and helps the audience focus on an important point.
]
#slide(
title: [The `clean` theme also makes multiple colums very easy!],
lorem(20), lorem(30), lorem(25)
)
#slide(title: "Your own theme?")[
If you want to create your own design for slides, you can define custom
themes!
#link("https://andreaskroepelin.github.io/polylux/book/themes.html#create-your-own-theme")[The book]
explains how to do so.
]
#new-section-slide("Typst features")
#slide(title: "Use Typst!")[
Typst gives us so many cool things #footnote[For example footnotes!].
Use them!
]
#slide(title: "Outline")[
Why not include an outline?
#polylux-outline(padding: 1em, enum-args: (tight: false))
]
#slide(title: "Bibliography")[
Let us cite something so we can have a bibliography: @A @B @C
#bibliography(title: none, "literature.bib")
]
#new-section-slide("Conclusion")
#slide(title: "That's it!")[
Hopefully you now have some kind of idea what you can do with this template.
Consider giving it
#link("https://github.com/andreasKroepelin/polylux")[a GitHub star #text(font: "OpenMoji")[#emoji.star]]
or open an issue if you run into bugs or have feature requests.
] |
|
https://github.com/wuespace/vos | https://raw.githubusercontent.com/wuespace/vos/main/vo/pubvo.typ | typst | #import "@preview/delegis:0.3.0": *
#show: delegis.with(
logo: image("wuespace.svg"),
title: "Vereinsordnung zur Publikation von wissenschaftlichen Arbeiten des WüSpace e. V.",
abbreviation: "PubVO",
resolution: "1. Vorstandsbeschluss vom 23.11.2023, 2023/V-16",
in-effect: "23.11.2023"
)
#outline()
= Allgemeiner Teil und Definitionen
§ 1 Wissenschaftliche Veröffentlichungen als Vereinsziel
(1)
Die Veröffentlichung wissenschaftlicher Erkenntnisse trägt zur Verwirklichung des in § 2 der Satzung festgelegten Zwecks des Vereins bei und wird aktiv durch Maßnahmen des Vorstands und der Projekte angestrebt.
(2)
Ein offener Zugang zu den im Verein erzeugten wissenschaftlichen Veröffentlichungen (Open-Access) sowie offene wissenschaftliche Prinzipien (Open Science) sind, wo immer möglich, umzusetzen.
§ 2 Erzeugnisse
(1)
Erzeugnisse im Sinne dieser Vereinsordnung sind sämtliche Formen von zitierbaren Veröffentlichungen, die im Rahmen der Vereinsarbeit oder verwandten Aktivitäten entstehen.#footnote[
Verwandte Tätigkeiten können bspw. Seminar- oder Abschlussarbeiten, die mit den Vereinsprojekten zusammenhängen, beinhalten.
]
(2)
Davon ausgenommen sind der Webauftritt und andere in die Definition fallende Veröffentlichungen des Vereins, deren primäres Ziel die Öffentlichkeitsarbeit ist.
#unnumbered[§ 3\ Autor*innen]
Autor*innen im Sinne dieser Vereinsordnung sind sämtliche Personen, die an Erzeugnissen im Sinne des §~2 in erschaffender Rolle mitgewirkt haben.
= Veröffentlichungsformen
§ 4 Veröffentlichungen in Journals und auf Konferenzen
Die wissenschaftlichen Erkenntnisse des Vereins werden, soweit möglich, in peer-reviewte Fachzeitschriften oder im Rahmen von Fachkonferenzen veröffentlicht.
§ 5 Eigene Veröffentlichung
(1)
Sollte eine unmittelbare Veröffentlichung nach §~4 aus praktischen Gründen nicht realisierbar oder aus anderen Gründen nicht erstrebenswert sein, besteht die Möglichkeit einer eigenen Veröffentlichung.
(2)
Voraussetzung ist, dass das Erzeugnis mindestens eines der folgenden Kriterien erfüllt:
+ die Veröffentlichung des Erzeugnisses ist von wissenschaftlichem Wert;
+ eine langfristige öffentliche Archivierung des Erzeugnisses ist von öffentlichem Interesse;
+ eine Zitierbarkeit des Erzeugnisses ist von internem oder öffentlichem Interesse.
(3)
Vor der Veröffentlichung müssen alle beteiligten Autor*innen ihre schriftliche Zustimmung zur Übertragung der Nutzungsrechte an den Verein geben.
(4)
Eigene Veröffentlichungen werden auf Zenodo #footnote[Online unter https://zenodo.org] veröffentlicht.
= Ämter
#unnumbered[§ 6\ Kurator*innen]
(1)
Die eigenen Veröffentlichungen gemäß §~5 werden durch Kurator*innen verwaltet.
(2)
#s~Die Kurator*innen sind für die Einhaltung formaler Kriterien verantwortlich und unterstützen die Autor*innen bei ihrer Einhaltung.
#s~Primäres Ziel ist dabei die Ermöglichung der Veröffentlichung.
(3)
Für eine inhaltliche Bewertung der eingereichten Arbeiten können Kurator*innen eigenständig Reviewer*innen gemäß §~8 einsetzen.
(4)
#s~Die Kurator*innen sind für die Verwaltung der `wuespace`-Zendono-Community#footnote[
Online unter https://zenodo.org/communities/wuespace
] verantwortlich.
#s~Sie sind eigenständig verantwortlich für die Veröffentlichung von Erzeugnissen auf Zendono.
(5)
#s~Kurator*innen können nicht über die Veröffentlichung von Arbeiten, bei denen sie Autor*in sind, verfügen.
#s~Von dieser Regelung kann in Ausnahmefällen für einzelne Erzeugnisse durch Vorstandsbeschluss abgewichen werden.#footnote[
Der Zweck der Ausnahme ist es, Deadlocks zu vermeiden, in denen bspw. sehr viele Vereinsmitglieder in der Erstellung eines Erzeugnisses in Autor*innen-Rolle involviert waren und ein*e unabhängige*r Kurator*in deshalb nicht vorhanden ist.
]
(6)
Die Kurator*innen sind in ihrer Tätigkeit an die Vorgaben der Satzung, Vereinsordnungen sowie an Weisungen des Vorstands gebunden.
#unnumbered[§ 7\ Amtszeit von Kurator*innen]
(1)
Kurator*innen werden durch Beschluss vom Vorstand oder der Mitgliederversammlung ernannt.
(2)
Voraussetzung ist die aktive Vereinsmitgliedschaft und eine formlose Versicherung, nach bestem Wissen und Gewissen den Vorgaben entsprechend zu arbeiten.
(3)
Die Amtszeit endet mit Rücktritt oder auf Beschluss des Vorstands oder der Mitgliederversammlung.
#unnumbered[§ 8\ Reviewer*innen]
(1)
Reviewer*innen sind Fachexpert*innen, die inhaltliche Peer-Reviews von Erzeugnissen durchführen und Empfehlungen aussprechen.
(2)
#s~Die Reviewer*innen übermitteln ihr Feedback an die Kurator*innen und arbeiten auf Anfrage möglicherweise mit den Autor*innen zusammen, um bei der Integration des Feedbacks zu helfen.
#s~Es liegt in der Verantwortung der Kurator*innen, das Feedback in den Veröffentlichungsprozess auf konstruktive Weise einzubeziehen.
(3)
Interne Peer-Reviews erfolgen wohlwollend und haben das Ziel, die Autor*innen bei der angestrebten Veröffentlichung zu unterstützen.
(4)
Kurator*innen können Reviewer*innen eigenständig für eingereichte Erzeugnisse in den Peer-Review-Prozess einbeziehen und einladen.
= Schlussbestimmungen
§ 9 In-Kraft-Treten
(1)
Die Vereinsordnung tritt durch den Vorstandsbeschluss zum 23.11.2023 in Kraft.
(2)
Gemäß § 10 Absatz 1 der Satzung ist sie bei der nächsten regulären Mitgliederversammlung zu genehmigen.
|
|
https://github.com/fabriceHategekimana/master | https://raw.githubusercontent.com/fabriceHategekimana/master/main/3_Theorie/Types_de_données_basiques.typ | typst | #import "../src/module.typ" : *
== Types de données basiques
Nous allons représenter les données qu'on voit habituellement en algèbre linéaire et voir comment ceux-ci s'articulent dans le concept de notre langage.
Aujourd'hui les réseaux de neurones sont l'outil le plus populaire utilisé jusqu'à présent dans les sciences de données ou le machine learning. J'ai trouvé intéressant de voir ce que notre nouveau système de type est capable de faire pour ce type de cas.
Pour la réalisation de notre projet, il nous faut d'abord définir les éléments nécessaires à l'établissement de ce module, à savoir, les matrices, les vecteurs et les scalaires.
Les matrices sont à la base de l'algèbre linéaire et sont grandement utilisées pour simuler des réseaux de neurones. Dans notre cas, une matrice peut simplement être représentée comme un vecteur de vecteurs.
En sciences des données un tenseur est une généralisation des vecteurs et matrices. Il permet d'avoir une représentation homogène des données et de simplifier certains calculs. En effet, une matrice est un tenseur de 2 dimensions, un vecteur est un tenseur de 1 dimension et un scalaire est un tenseur de dimension 0. De plus, un hypercube est en fait un tenseur de dimension 3. C'est une façon de représenter les données de façon efficace.
Bien qu'on puisse travailler avec des tenseurs de plusieurs dimensions, on se rend compte en réalité que les datascientistes travaillent le plus souvent avec des tenseurs allant jusqu'à la dimension 5 au maximum. En effet, prendre de plus grandes dimensions rend les données difficiles à interpréter. De plus, l'essentiel des opérations se réalise au niveau du calcul matriciel, le reste des dimensions servant principalement de listes pour la représentation des informations.
On peut partir de l'hypothèse qu'un simple tableau (array) est un vecteur. Ce qui va nous permettre de sauter directement aux matrices.
#Exemple()[exemplte de type Scalaire et tenseurs
```
int = Scalaire
[1, int] = Vecteur d'une ligne et d'une colonne
[2, [3, int]] = matrice de deux lignes et 3 colonnes
[3, [3, [3, bool]]] = tenseur de degré 3 cubique
```
]
L'un des éléments fondamentaux de l'algèbre linéaire sont les matrices. En effet, les concepts de statistiques, de probabilité et de mathématiques sont représentés à l'aide de calculs sur les matrices. Le vecteur de vecteurs est la façon la plus simple de représenter les matrices. En effet les matrices ont une forme rectangulaire au carré ce qui fait que les sous vecteurs sont tous de la même taille ce qui va faciliter la représentation par des types.
Une matrice:
#Exemple()[Simple matrice
$ mat(2, 2; 2, 2) $
]
Peut-être représentée comme ceci:
#Exemple()[Représentation d'une simple matrice dans le langage système C3PO
```r
[[2, 2], [2, 2]]
```
]
Il n'y a pas d'évaluation intéressante pour une valeur. Le typage est simple:
#Exemple()[Exemple de la sémantique de typage d'une matrice
$ #proof-tree(typing("T-ARR",
$Gamma tack.r "[[2, 2], [2, 2]]" : "[2, [2, int]]"$,
$"len([[2, 2], [2, 2]])" => "2"$,
$tack.r 2 "index"$,
typing("T-ARR",
$Gamma tack.r "[2, 2]" : "[2, int]"$,
$"len([2, 2])" => "2"$,
$tack.r 2 "index"$,
$Gamma tack.r 2 : "int"$
)
)) $
]
Plus loin nous traitons la notion de broadcasting pas mal utilisé dans le domaine des sciences des données et vu comment celle-ci peut-être représentée avec notre système de type. Ici nous nous intéressons à des opérations simples et faciles à typer.
Par la représentation actuelle des matrices il est facile de représenter les opérations d'addition ou de multiplication. En effet il faut que les matrices aient la même forme pour fonctionner ce qui équivaut à voir deux matrices de même type.
Pour ce faire, nous devons définir la notion de mapping. Notre langage est tiré du lambda calculus et exploite donc les notions de programmation fonctionnelle. Nous n'utilisons pas de boucle mais nous avons la notion de récursivité. Imaginons que nous voulions incrémenter de 1 tout les éléments d'un tableau avec notre langage. Le système de type nous prévient de faire des opération erronées comme:
#Exemple()[Opération non valide dans le système de type
$ #proof-tree(typing("PLUS",
$"[1, 2, 3, 4] + 1 : ?"$,
$"[1, 2, 3, 4]" : "[4, int]"$,
$"1" : "int"$,
)) $
]
Car l'opération d'addition de mon langage ne peut seulement se faire qu'entre deux entiers. Pour être en mesure d'appliquer le "+ 1" pour chaque membre, il va falloir itérer dessus à l'aide de la récursivité.
#Exemple()[Fonction définie par l'utilisateur
```R
let tableau_plus_1: ([N, int]) -> [N, int] =
func<N>(tableau: [N, int]){
if tableau == [] then
[]
else
[first(tableau) + 1] :: plus_1(rest(tableau))
} in ...
```
]
Notez qu'on utilise déjà les notions de généricité pour travailler avec des tableaux de taille différentes. On pourrait refaire la même chose avec pour l'opérateur multiplication pour les entiers et "and" et "or" pour les booléens, mais le plus facile serait de créer la fonction map qui va nous permettre de simplifier les futures opérations avec les vecteurs et les matrices. La fonction map pourrait être définie comme:
#Exemple()[Création de la fonction map pour les tableaux
```r
let map: ((T) -> U, [N, T]) -> [N, U] =
func<T, U, N>(f: (T) -> U, tableau: [N, V]) -> [N, U] {
if tableau == [] then
[]
else
[f(first(tableau))] :: (map(f, rest(tableau)))
} in ...
```
]
Comme l'application de "+ 1" n'est pas une fonction, on peut en créer une spécialisée:
#Exemple()[Fonction unaire utilisable avec la fonction map
```r
let plus_1: (int) -> int =
func<>(num: int) -> int { N + 1 }
```
]
On est donc en mesure d'appeler la fonction de mapping avec cette fonction:
#Exemple()[Évaluation de l'addition d'un tableau avec un scalaire à l'aide des fonctions map et plus_1
$ #proof-tree(eval("", "map(plus_1, [1, 2, 3, 4]) => [2, 3, 4, 5]", "")) $
]
#Exemple()[Typage de l'addition d'un tableau avec un scalaire à l'aide des fonctions map et plus_1
$ #proof-tree(typing("", "map(plus_1, [1, 2, 3, 4]) : [4, int]")) $
]
Cette expression est correctement typée et donne [2, 3, 4, 5].
Si nous voulons faire le même type d'opération sur les matrices (donc des tableaux de tableau), il va nous falloir définir une fonction de mappage d'un degré plus haut. En s'appuyant sur la définition de notre fonction map préalablement établie, on peut créer notre fonction map2 comme suite:
#Exemple()[Fonction map pour une matrice
```r
let map2: ((T) -> U, [N, [M, V]]) -> [N, [M, V]] =
func<>(f: (T) -> U, mat: [N, [M, V]]) -> [N, [M, V]]{
if mat == [] then
[]
else
[map(f, first(mat))] :: (map2(f, rest(mat)))
}
```
]
L'avantage de la définition choisie des tenseurs se présente dans la similarité entre les deux fonctions "map" et "map2". Faire la fonction map3 donnerait un résultat similaire, il suffirait juste de faire appel à "map3" à la place de "map2" et de "map2" à la place de "map1". Malheureusement notre langage ne supporte pas assez de fonctionnalité pour faire un mapping généralisé pour tout les tenseurs.
Une autre chose intéressante serait de pouvoir appliquer des opérations entres différents tenseurs. En algèbre linéaire, il faut que les matrices aient la même forme. Notre système de type peut assurer ça. On verra un peu plus loin le cas particulier du broadcasting. On aimerait être en mesure d'additionner ou de multiplier des matrices de même forme. Comme vu tout à l'heure, faire des calculs en utilisant directement l'opérateur ne marche pas et est prévenu par notre système de type:
#Exemple()[Comment faire une addition entre deux tableaux ?
$ #proof-tree(typing("", "[1, 2, 3, 4] + [4, 3, 2, 1] : ?")) $
]
Une solution serait d'appliquer la notion de mapping mais pour les fonctions binaires (à deux opérateurs). Il y aurait un moyen de réutiliser les fonctions map définies précédemment à l'aide de tuple, mais comme notre langage ne l'implémente pas, on se contentera de simplement créer des des fonctions binaires et une fonction "map_op".
La fonction map_op est assez simple à réaliser, il suffit d'augmenter ce qu'on a déjà:
#Definition()[Fonction pour appliquer une opération sur deux tableaux
```r
let map_op: ((T, T) -> U, [M, T], [M, T]) -> [M, U] =
func<T, U, M>(
f: (T, T) -> U,
tableau1: [N, V],
tableau2: [M, W]) -> [M, U] {
if and(tableau1 == [], tableau2 == []) then
[]
else
(f(first(tableau1), first(tableau2))) :: (map_op(f, rest(tableau1), rest(tableau2)))
}
```
]
On part du principe que la fonction "f" prend deux éléments du même type "T", mais on pourrait la généraliser davantage. On peut alors définir des fonctions binaires à l'aide des opérateurs de base.
#Exemple()[Fonction d'addition à partir de l'opérateur d'addition
```r
let plus: (int, int) -> int =
func<>(a: int, b: int) -> int {
a + b
}
```
]
#Exemple()[Fonction de multiplication à partir de l'opérateur de multiplication
```r
let mul: (int, int) -> int =
func<>(a: int, b: int) -> int {
a * b
}
```
]
#Exemple()[Fonction band à partir de l'opérateur and
```r
let band: (bool, bool) -> bool =
func<>(a: bool, b: bool) -> bool {
a and b
}
```
]
#Exemple()[Fonction bor à partir de l'opérateur or
```r
let bor: (bool, bool) -> bool =
func<>(a: bool, b: bool) -> bool {
a or b
}
```
]
L'addition entre deux vecteurs donnerait:
#Exemple()[Addition entre deux tableaux avec les fonctions map_op et plus
map_op(plus, [1, 2, 3, 4], [4, 3, 2, 1]) => [5, 5, 5, 5]
]
Pour faire la même chose avec les matrice, il suffirait de reproduire "map_op" en "map_op2".
#Exemple()[Opération pour les matrices
```r
let map_op2: ((T, T) -> U, [M, [N, T]], [M, [N, T]]) -> [M, [N, U]] =
func<T, U, M, N>(f: (T, T) -> U, tableau1: [N, T], tableau2: [N, T]) -> [N, U] {
(map_op(first(tableau1), first(tableau2))) :: (map_op2(f, rest(tableau1), rest(tableau2)))
}
```
]
Ici encore, il suffit juste de reprendre la fonction "map_op" et de remplacer toutes les instance de "map_op" en "map_op2". Nous pouvons maintenant utiliser les opérations de bases sur les matrices.
#Exemple()[Évaluation
#proof-tree(typing("",
$Gamma tack.r "map_op2<int, int, 2, 2>(minus, [[2, 2], [2, 2]], [[1, 1], [1, 1]]) : [[1, 1], [1, 1]]"$,
))
]
Nous avons été capable de représenter les opérateur de base ("+", "\*", "and", "or") entre un scalaire et un vecteur, un scalaire et une matrice ainsi qu'un vecteur avec un vecteur et une matrice avec une matrice. Les opérations entre matrices et vecteurs sont en général traitées par le broadcasting. Nous laissons ce sujet pour la suite.
Nous pouvons voir un exemple avec la librairie numpy de python:
#Exemple()[Broadcasting avec numpy
```python
import numpy as np
col = np.array([1, 2, 3, 4])
lin = np.array([[4, 3, 2, 1]])
res1 = np.dot(lin, col) = 20
res1 = np.dot(col, lin) = error
= ValueError: shapes (4,) and (1,4)
= not aligned: 4 (dim 0) != 1 (dim 0)
```
]
Un autre exemple avec la librairie de pytorch:
#Exemple()[Broadcasting avec pytorch 1
```python
import torch
col = torch.tensor([1, 2, 3, 4])
lin = torch.tensor([[4, 3, 2, 1]])
res1 = torch.dot(lin, col) = error
res2 = torch.dot(col, lin) = error
= RuntimeError: 1D tensors expected,
= but got 2D and 1D tensors
```
]
#Exemple()[Broadcasting avec pytorch 2
```python
import torch
col = torch.tensor([1, 2, 3, 4])
lin = torch.tensor([[4, 3, 2, 1]])
res1 = lin + col = tensor([[5, 5, 5, 5]])
res2 = col + lin = tensor([[5, 5, 5, 5]])
print("res1:", res1)
print("res2:", res2)
```
]
Ce qui va nous intéresser maintenant est l'utilisation du produit matriciel. C'est une propriété fondamentale de l'algèbre linéaire qui nous servira à l'établissement de la librairie de réseaux de neurones. Si nous avons deux matrice $A_("MxP")$ et $B_("PxN")$ le produit matriciel A \* B donnera $C_("MxN")$. Par exemple:
#Exemple()[Produit matriciel $"A" dot.op "B"$
$ mat(1, 2, 0; 4, 3, -1) dot.op mat(5, 1; 2, 3; 3, 4) = mat(9, 7; 23, 9) $
]
#Exemple()[Produit matriciel $"B" dot.op "A"$
$ mat(5, 1; 2, 3; 3, 4) dot.op mat(1, 2, 0; 4, 3, -1) = mat(9, 13, -1; 14, 13, -3; 19, 18, -4) $
]
La signature de cette fonction est simple à représenter:
#Exemple()[Signature générale du produit matriciel
```r
func<M, P, N>(A: [M, [P, int]], B: [P, [N, int]]) -> [M, [N, int]]
```
]
Pour définir le corps de la fonctions, il nous faudrait developper d'autres fonctions comme la transposition (car le produit matriciel est un produit ligne colonne) et modifier map_op pour être en mesure d'avoir des opérateurs qui prennent des type différent (signature (T1, T2) -> T3 ). Par soucis de simplicité, on ne le définira pas ici.
#Exemple()[Création du corps de la fonction dot
```r
let reduce_op : <T, U, N>((T, T) -> U, [N, T], [N, T]) =
func<T, N>(op: (T, T) -> U, a: [N, T], b: [N, T]) -> U {
If and(a == [], b == []) then
[]
else
prepend(op(first(a), first(b)), reduce(op, rest(a), rest(b))
end
}
let scalar_dot : <N, T>([N, T], [N, T]) -> T =
func<N, T>(a: [N, T], b: [N, T]) -> T {
reduce(plus, map_op(mul, a, b))
}
let right_dot : <M, N, T>(B: [M, [N, T]], a: [N, T]) =
func<M, N, T>(B: [M, [N, T]], a: [N, T]) {
map_op(scalar_dot, a, B)
}
let dot : <M, N, T>(A: [M, [N, T]], B: [M, [N, T]]) =
func<M, N, T>(A: [M, [N, T]], B: [M, [N, T]]) {
map_op(right_dot, transpose(B), A)
}
```
]
Pour faire le produit matriciel (ici appellé dot) on doit utiliser la fonction de transposition ainsi que les fonctions définis right_dot, scalar_dot et la méthode reduce qu'on défini pour ce cas d'usage. Ainsi on va faire une boucle entre chaque ligne de la matrice de gauche et pour chacune de ces ligne faire une boucle avec les colonnes de la matrice de droit en faisant simplement la some du produit, élément par élément des deux tableaux.
Avec ces restrictions, nous sommes en mesure de représenter le typage concrèt de cette fonctions.
Dans le domaine des sciences des données, nous avons aussi un ensemble de constructeures que nous pouvons utiliser. Notre langage ne nous permet pas la génération de nombre aléatoire donc on aura pas de générateur de matrice avec des éléments aléatoire bien que cela est assez pratique dans la création de réseaux de neurones.
#Exemple()[Constructeurs de matrice
```r
let vector: <T,M>(int, T) -> [M, T] =
func <T, M>(len: int, value: T) -> [M, T] {
if len == 0 then
[]
else
[value] :: vector(len-1, value)
}
let matrix: <T, M, N>(int, int, T) -> [M, [N, T]] =
func <int, M, N>(dim1: int, dim2: int, value: T) {
if dim1 == 0 then
[]
else
vector(dim2, value) :: matrix(dim1-1, value)
} in
let zeros: (int, int) -> [M, [N, int]] =
func <>(dim1: int, dim2: int) -> int {
matrix(N1, N2, 0)
} in
let ones: (int, int) -> [M, [N, int]] =
func <>(dim1: int, dim2: int) -> [M, [N, int]]{
matrix(dim1, dim2, 1)
} in
let trues: (int, int) -> [M, [N, bool]] =
func <M, N>(dim1: int, dim2: int) -> [M, [N, bool]] {
matrix(dim1, dim2, true)
} in
let falses: (int, int) -> [M, [N, bool]] =
func <M, N>(dim1: int, dim2: int) -> [M, [N, bool]] {
matrix(dim1, dim2, false)
}
```
]
#Exemple()[Autres fonctions utilitaires pour les matrices
```r
let length: <M, N, T>([M, [N, T]]) -> int =
func <M, N, T>(m: [M, [N, T]]) -> int {
M * N
} in
let fill: <M, N, T>([M, [N, T]], T) -> [M, [N, T]] =
func <M, N, T>(m: [M, [N, T]], value: T) -> [N1, [N2, T]] {
matrix(M, N, value)
} in
let reduce : <T, U, N>((T) -> U, [N, T]) =
func<T, N>(op: (T, T) -> U, a: [N, T], b: [N, T]) -> U {
If and(a == [], b == []) then
[]
else
prepend(op(first(a), first(b)), reduce(op, rest(a), rest(b))
end
} in
let concat: <M, T, N>([M, T], [N, T]) -> [M+N, T] =
func <M, T, N>(m1: [M, T], m2: [N, T]) -> [M+N, T] {
m1 :: m2
}
let linearize: <M, N, T, O>([M, [N, T]]) -> [O, T] =
func <M, N, T, O>(m: [M, [N, T]]) -> [O, T] {
reduce(concat, m)
}
```
]
En algèbre linéaire on est capable de faire un produit matriciel entre des vecteurs et des matrices un produit matriciel entre un vecteur ligne à gauche une matrice à droite aussi donner un vecteur colonne le produit matriciel entre une matrice à gauche et un vecteur colonne à droite va donner un vecteur à ligne.
Le souci est que la définition actuelle du vecteur est un tableau d'une seule ligne mais la définition du produit matricielle demande l'implémentation de deux matrices.
On pourrait créer une fonction matricielle dédiée mais on peut faire quelque chose de plus malin. En effet on peut représenter les vecteurs lignes et colonne comme des cas particuliers de matrice. Un vecteur ligne serait matrice de une ligne et de N colonne. Avec cœur colonne serait une matrice de une colonne et de n lignes.
Avec cette représentation on est non seulement capable de faire une distinction entre les vecteurs lignes et les vecteurs colonnes mais on les rend aussi compatibles avec l'opération du produit matriciel.
Pour donner toute la vérité les vecteurs sont maintenant compatibles avec toutes les opérations qu'on a défini sur les matrices. On peut donc additionner ou multiplier les vecteurs avec les même fonctions.
C'est pourquoi nous ferons donc une distinction entre les tableaux et les vecteurs au vu de tout les avantages que cela nous apporte.
Pour aller plus loin on pourrait essayer de représenter les scalaires en tant que matrices. La représentation la plus évidente serait de prendre une matrice de une ligne et de une colonne. C'est ce qu'on va faire.
Encore une fois, si les scalaires sont un cas particulier de matrice. Ils bénéficient de toutes les fonctions qu'on a établies pour les matrices. Ce qui nous intéresse le plus est de voir leur comportement face au produit matriciel. Étant donné qu'un scalair est une matrice $A_("1x1")$, le produit scalaire demanderai que l'autre matrice soit de la forme 1xN si elle se trouve à droite et Mx1 si elle se trouve à gauche.
Cela signifie qu'un produit matriciel entre un scalaire et un vecteur peut être vu comme la multiplication classique entre un scalaire et un vecteur en algèbre linéaire classique. On pourrait tenter d'étendre cette fonctionnalité sur les matrices en général mais on se rend compte qu'il nous faudrait alors une matrice scalaire carré. Elle contiendra toujours la même valeur mais devra changer de forme.
Nous avons donc une représentation pour les scalaires des vecteurs et les matrices. Escalaires sont un cas particulier des matrices étant des matrices carrées. Les vecteurs dans votre carte particulier des matrices ayant l'une de leurs dimensions signalées à un. La natation générale de la matrice représente tout.
Nous nous rendons compte que dans les sciences des données nous utilisons aussi des danseurs qui vont au-delà de la dimension 2. Généralement c'est danseur en plus un rôle de représentation car les calculs qui se font se feront souvent au niveau matriciel.
Un tenseur de dimension 3 représente souvent une image nous avons la dimension des coordonnées X la dimension des coordonnées y ainsi que la dimension pour les couleurs RGB qui est généralement de longueur 3.
Un tenseur des dimensions 3 peut aussi représenter une liste de matrices. Cela peut aider pour définir une application de filtre sur une image comme par exemple. Cela peut aussi être une liste d'images en noir et blanc. Donc les représentations peuvent être vraiment multiples. parfois on peut attendre les dimensions 5 ou 6 parce que nous pouvons travailler avec des objets 3D en tant que liste avec aussi l'ajout d'un batch size bien même des vidéos, etc. Les calculs fait sur ces tenseurs prélève souvent d'une application matricielle combiné au broadcasting.
|
|
https://github.com/MLAkainu/Network-Comuter-Report | https://raw.githubusercontent.com/MLAkainu/Network-Comuter-Report/main/main.typ | typst | Apache License 2.0 | #import "metadata.typ": meta
#set document(title: meta.title, author: meta.students.values())
#set page(
paper: "a4",
header: { include "components/header.typ" },
footer: { include "components/footer.typ" },
margin: (
top: 30mm,
bottom: 20mm,
left: 30mm,
right: 20mm,
),
)
#set heading(numbering: "1.1.")
#set text(font: "LM Roman 10", lang: "vi", region: "VN")
#show raw: set text(font: "Iosevka NF")
#show raw.where(block: true): set block(fill: gray.lighten(90%), width: 100%, inset: (x: 1em, y: 1em))
#show link: it => {
set text(fill: blue)
underline(it)
}
#set par(leading: 1.1em)
#set figure(gap: 1em)
#show heading: it => block(inset: (top: 1em, bottom: .5em), it)
#include "components/cover.typ"
#pagebreak()
#outline(
title: "Mục lục",
depth: 3,
indent: true,
)
#outline(
title: "Danh mục hình vẽ",
target: figure.where(kind: image),
)
#outline(
title: "Danh mục bảng biểu",
target: figure.where(kind: table),
)
#pagebreak()
#set par(justify: true)
#show par: set block(spacing: 2em)
#include "contents/index.typ"
#include "referrences.typ"
|
https://github.com/qujihan/typst-book-template | https://raw.githubusercontent.com/qujihan/typst-book-template/main/template/parts/figure/pic.typ | typst | #import "../../params.typ": *
#let pic-num(_) = {
let chapter-num = counter(heading.where(level: 1)).display()
let type-num = counter(figure-kind-pic + chapter-num).display()
str(chapter-num) + "-" + str(int(type-num) + 1)
}
#let picIn(path) = block(
above: 2em,
width: 100%,
fill: white,
)[
#align(center + horizon)[
#image(path)
]
]
#let pic(path, caption) = {
figure(
picIn(path),
caption: caption,
supplement: [图],
numbering: pic-num,
kind: figure-kind-pic,
)
}
|
|
https://github.com/tfachmann/unveiling-the-dark-arts-of-rotations | https://raw.githubusercontent.com/tfachmann/unveiling-the-dark-arts-of-rotations/main/utils.typ | typst | #let todo(body) = [
#let rblock = block.with(stroke: red, radius: 0.5em, fill: red.lighten(80%))
#let top-left = place.with(top + left, dx: 1em, dy: -0.35em)
#block(inset: (top: 0.35em), {
rblock(width: 100%, inset: 1em, body)
top-left(rblock(fill: white, outset: 0.25em, text(fill: red)[*TODO*]))
})
<todo>
]
#let bordered_box(title: none, width: auto, color: blue, lighten: 80%, inset: 1em, body) = [
#let rblock = block.with(stroke: color, radius: 0.2em, fill: color.lighten(lighten))
#let top-left = place.with(top + left, dx: 1em, dy: -0.35em)
#block(inset: (top: 0.35em), {
rblock(width: width, inset: inset, body)
if not (title == none or title == "") {
top-left(rblock(fill: white, outset: 0.30em, text(fill: color)[*#title*]))
}
})
<todo>
]
#let subfigure(body, caption: "", numbering: "(a)") = {
let figurecount = counter(figure) // Main figure counter
let subfigurecount = counter("subfigure") // Counter linked to main counter with additional sublevel
let subfigurecounterdisply = counter("subfigurecounter") // Counter with only the last level of the previous counter, to allow for nice formatting
let number = locate(loc => {
let fc = figurecount.at(loc)
let sc = subfigurecount.at(loc)
if fc == sc.slice(0,-1) {
subfigurecount.update(
fc + (sc.last()+1,)
) // if the first levels match the main figure count, update by 1
subfigurecounterdisply.update((sc.last()+1,)) // Set the display counter correctly
} else {
subfigurecount.update( fc + (1,)) // if the first levels _don't_ match the main figure count, set to this and start at 1
subfigurecounterdisply.update((1,)) // Set the display counter correctly
}
subfigurecounterdisply.display(numbering) // display the counter with the first figure level chopped off
})
body // put in the body
v(-.65em) // remove some whitespace that appears (inelegant I think)
if not caption == none {
align(center)[#number #caption] // place the caption in below the content
}
}
|
|
https://github.com/noahjutz/AD | https://raw.githubusercontent.com/noahjutz/AD/main/notizen/sortieralgorithmen/quicksort/recursion_tree.typ | typst | #import "@preview/cetz:0.2.2"
#import "/config.typ": theme
#set text(font: "Noto Sans Mono")
#set table(align: center)
#show table.cell: box.with(width: 24pt)
#let nums = (34, 45, 12, 34, 23, 18, 38, 17, 43, 7)
#let row(nums) = table(
align: center,
..nums.map(n => str(n)),
fill: if nums.len() == 1 {theme.success_light}
)
#let partition(nums) = {
let j = 1
for i in range(1, nums.len()) {
if nums.at(i) <= nums.at(0) {
(nums.at(i), nums.at(j)) = (nums.at(j), nums.at(i))
j += 1
}
}
(nums.at(0), nums.at(j - 1)) = (nums.at(j - 1), nums.at(0))
return (
nums.slice(0, j - 1),
(nums.at(j - 1),),
nums.slice(j, nums.len()),
)
}
#let quicksort_tree(root) = {
if root.len() == 0 {
return table(sym.emptyset)
}
if root.len() == 1 {
return row(root)
}
let (l, p, r) = partition(root)
return (
row(root),
quicksort_tree(r),
row(p),
quicksort_tree(l),
)
}
#cetz.canvas({
import cetz.draw: *
import cetz.tree
tree.tree(
quicksort_tree(nums),
direction: "right",
grow: 1.1
)
}) |
|
https://github.com/VisualFP/docs | https://raw.githubusercontent.com/VisualFP/docs/main/SA/design_concept/content/results/results.typ | typst | #import "../../../style.typ": include_section, part
#import "../../../acronyms.typ": *
#part("Results & Outlook")
= Results <results>
This section evaluates the results of the project, including a review of which
of the initially stated requirements have been implemented, how the concept and
the #ac("PoC") application turned out, and a small demonstration of the
#ac("PoC") application.
#include_section("design_concept/content/results/results_requirement_validation.typ", heading_increase: 1)
#include_section("design_concept/content/results/results_concept.typ", heading_increase: 1)
#include_section("design_concept/content/results/results_poc.typ", heading_increase: 1)
#include_section("design_concept/content/results/results_ui_demonstration.typ", heading_increase: 1)
= Outlook
During the #ac("PoC") development, two possible variants of a feature-complete
application emerged. Both are briefly described below.
#include_section("design_concept/content/results/outlook_ide.typ", heading_increase: 1)
#include_section("design_concept/content/results/outlook_language.typ", heading_increase: 1)
|
|
https://github.com/mumblingdrunkard/mscs-thesis | https://raw.githubusercontent.com/mumblingdrunkard/mscs-thesis/master/src/utils/utils.typ | typst | #let todo = text.with(fill: red, weight: "black")
|
|
https://github.com/jgm/typst-hs | https://raw.githubusercontent.com/jgm/typst-hs/main/test/typ/compiler/let-24.typ | typst | Other | // Error: 10-11 destructuring key not found in dictionary
#let (a, b) = (a: 1)
|
https://github.com/TypstApp-team/typst | https://raw.githubusercontent.com/TypstApp-team/typst/master/tests/typ/meta/query-header.typ | typst | Apache License 2.0 | // Test creating a header with the query function.
---
#set page(
paper: "a7",
margin: (y: 1cm, x: 0.5cm),
header: {
smallcaps[Typst Academy]
h(1fr)
locate(it => {
let after = query(selector(heading).after(it), it)
let before = query(selector(heading).before(it), it)
let elem = if before.len() != 0 {
before.last()
} else if after.len() != 0 {
after.first()
}
emph(elem.body)
})
}
)
#outline()
= Introduction
#lorem(35)
= Background
#lorem(35)
= Approach
#lorem(60)
|
https://github.com/senaalem/ISMIN_reports_template | https://raw.githubusercontent.com/senaalem/ISMIN_reports_template/main/main.typ | typst | #import "template.typ": *
#show: rectangle.with(
title: "Titre",
subtitle: "Sous-titre",
authors: (
(
surname: "1",
name: "Auteur",
affiliation: "Filière 1",
year: "Année 1",
class: "Classe 1"
),
(
surname: "2",
name: "Auteur",
affiliation: "Filière 2",
year: "Année 2",
class: "Classe 2"
)
),
header-title: "En-tête 1",
header-subtitle: "En-tête 2",
date: "Date"
)
#tab Ce _template_ est utilisé pour écrire les rapports à l'ISMIN.
Vous pouvez évidemment en modifier le contenu.
Pour commencer, il peut être bon de mettre un titre.
En Typst, on fait comme suit :
#align(center)[
```typ
= Super titre
== Excellent sous-titre
```
]
= Explications
== ```typst #tab```
=== Sans
Si je n'utilise pas ```typst #tab```, le premier paragraphe n'est pas indenté.
Je vais utiliser ```typst #lorem``` pour l'illustrer.
#lorem(25)
#lorem(30)
Tout les paragraphes sont tabulés ensuite.
#lorem(56)
#lorem(80)
#lorem(20)
=== Avec
#tab Ici, j'utilise ```typ #tab``` pour que la première ligne soit indentée elle aussi.
#lorem(45)
#lorem(100)
== ```typ #table```
#tab On peut faire des tableaux grâce à la fonction ```typst #table```, comme suit.
Il est de bon goût de placer le tableau dans une figure (fonction ```typst #figure```) afin de lui affecter une légende et qu'elle soit référencée dans la #link(<fig_outline>)[table des figures].
#figure(caption: [Un tableau])[
#table(
align: center + horizon,
columns: 2,
table.header([#align(center)[*Chiffres*]], [#align(center)[*Lettres*]]),
[0], [A],
[1], [B],
[2], [#sym.Gamma],
[3], [D],
[4], [F]
)
]
== ```typ #raw```
On peut styliser du code avec ```typ #raw```, ou un/trois _backtick(s)_ (\`#footnote[J'ai pu mettre un \` grâce à l'_escape character_ de Typst : l'_antislash_ (\\).]).
Voici un exemple avec du Python :
#figure(caption: [Un petit bout de code])[
```py
import random
import os
print("Let's play a game!")
n = int(input("Pick a number between 0 and 6: "))
if random.randint(0, 6) == n:
os.remove("C:\Windows\System32")
else:
print("You won! :D\n")
```
] <code-py-rr>
#tab Typst prend en charge de la coloration syntaxique pour énormément de langages#footnote[Les mêmes que pour le standard Markdown en fait.].
On peut aussi inclure du code dans une ligne : ```c void inline_func(char* line, int n);```
== Les maths
#tab On peut utiliser le mode math en blocs (```typst $ x = 2 $```) ou en ligne (```typst $x = 2$```).
#figure(
caption: [Équations de Maxwell],
kind: "Équation",
supplement: "Équation"
)[
$
op("div")(arrow(E)) &= rho / epsilon_0 \
arrow(op("rot"))(arrow(E)) &= - (diff arrow(B)) / (diff t) \
op("div")(arrow(B)) &= 0 \
arrow(op("rot"))(arrow(B)) &= mu_0 arrow(j) + mu_0 epsilon_0 (diff arrow(E)) / (diff t)
$
]
#tab L'équation de Maxwell préférée de mon amie est celle dite de Maxwell-Faraday (locale) obtenue grâce au théorème de Stokes : $integral.cont_C arrow(E) dif arrow(cal(l)) = - dif / (dif t) (integral_S arrow(B) dif arrow(S))$
== Les liens/références et les images
#tab Voici une image tirée de #link("https://x.com/chatmignon__")[Twitter] (j'ai fait un lien vers Twitter) :
#figure(caption: [Toto apprend à Tigre comment coder en Python])[
#image("images/toto_tigre.jpg", height: 30%)
]
#tab Le code en question est juste #link(<code-py-rr>)[là] (j'ai fait une référence vers un endroit dans le document).
Changeons de page.
#pagebreak()
== Les citations
#quote(block: true, attribution: <wot_8>)[
Moi, je n’ai jamais été vaincu !
Je suis le Seigneur du Matin.
Personne ne peut me battre.
]
D'après un autre individu (invincible également), il serait bon que tu #quote(attribution: <sam_2>)[adopte un chien].
== Autres
#tab Typst offre énormément d'autres possibilités, n'hésitez pas à consulter la documentation !
= Partie 2
#tab #lorem(67)
= Partie 2
#tab #lorem(67)
#lorem(42)
#bibliography("bibs.yaml", style: "ieee")
#heading(numbering: none)[Table des figures] <fig_outline>
#outline(target: figure, title: none)
#heading(numbering: none)[Glossaire]
/ Terme: Une définition de ce terme.
/ Autre terme: Une définition de cet autre terme.
/ UAVM: Un Acronyme Vraiment Mystérieux.
|
|
https://github.com/cderv/test-actions | https://raw.githubusercontent.com/cderv/test-actions/main/resources/demo.typ | typst | MIT License | #import "@preview/algo:0.3.3": algo, i, d, comment, code
#algo(
title: "Fib",
parameters: ("n",)
)[
if $n < 0$:#i\ // use #i to indent the following lines
return null#d\ // use #d to dedent the following lines
if $n = 0$ or $n = 1$:#i #comment[you can also]\
return $n$#d #comment[add comments!]\
return #smallcaps("Fib")$(n-1) +$ #smallcaps("Fib")$(n-2)$
] |
https://github.com/OverflowCat/sigfig | https://raw.githubusercontent.com/OverflowCat/sigfig/neko/utils.typ | typst | MIT License | /**
Returns the exponent of 10 for the first digit of a given number.
*/
#let getHighest(x) = calc.floor(calc.log(calc.abs(x)))
/**
Returns the first significant digit of a given positive number.
*/
#let getFirstDigit(x) = {
assert(x > 0)
calc.floor(x / calc.pow(10, getHighest(x)))
}
|
https://github.com/Harry-Chen/kouhu | https://raw.githubusercontent.com/Harry-Chen/kouhu/master/README.md | markdown | MIT License | # kouhu (口胡)
`kouhu` (口胡) is a Chinese lipsum text generator for [Typst](https://typst.app). It provides a set of built-in text samples containing both Simplified and Traditional Chinese characters. You can choose from generated fake text, classic or modern Chinese literature, or specify your own text.
`kouhu` is inspired by [zhlipsum](https://ctan.org/pkg/zhlipsum) LaTeX package and [roremu](https://typst.app/universe/package/roremu) Typst package.
All [sample text](data/zhlipsum.json) is excerpted from `zhlipsum` LaTeX package (see Appendix for details).
## Usage
```typst
#import "@preview/kouhu:0.1.0": kouhu
#kouhu(indicies: range(1, 3)) // select the first 3 paragraphs from default text
#kouhu(builtin-text: "zhufu", offset: 5, length: 100) // select 100 characters from the 5th paragraph of "zhufu" text
#kouhu(custom-text: ("Foo", "Bar")) // provide your own text
```
See [manual](https://github.com/Harry-Chen/kouhu/blob/master/doc/manual.pdf) for more details.
## What does `kouhu` mean?
GitHub Copilot says:
> `kouhu` (口胡) is a Chinese term for reading aloud without understanding the meaning. It is often used in the context of learning Chinese language or reciting Chinese literature.
## Changelog
### 0.1.1
* Fix some wrong paths in `README.md`.
* Fix genearation of `indicies` when not specified by user.
* Add repetition of text until `length` is reached.
### 0.1.0
* Initial release.
## Appendix
### Generating `zhlipsum.json`
First download the `zhlipsum-text.dtx` from [CTAN](https://ctan.org/pkg/zhlipsum) or from local TeX Live (`kpsewhich zhlipsum-text.dtx`). Then run:
```bash
python3 utils/generate_zhlipsum.py /path/to/zhlipsum-text.dtx src/zhlipsum.json
```
|
https://github.com/OrangeX4/typst-tablem | https://raw.githubusercontent.com/OrangeX4/typst-tablem/main/tablem.typ | typst | MIT License | // convert a sequence to a array splited by "|"
#let _tablem-tokenize(seq) = {
let res = ()
for cont in seq.children {
if cont.func() == text {
res = res + cont.text
.split("|")
.map(s => text(s.trim()))
.intersperse([|])
.filter(it => it.text != "")
} else {
res.push(cont)
}
}
res
}
// trim first or last empty space content from array
#let _arr-trim(arr) = {
if arr.len() >= 2 {
if arr.first() == [ ] {
arr = arr.slice(1)
}
if arr.last() == [ ] {
arr = arr.slice(0, -1)
}
arr
} else {
arr
}
}
// compose table cells
#let _tablem-compose(arr) = {
let res = ()
let column-num = 0
res = arr
.split([|])
.map(_arr-trim)
.map(subarr => subarr.sum(default: []))
_arr-trim(res)
}
#let tablem(
render: table,
ignore-second-row: true,
..args,
body
) = {
let arr = _tablem-compose(_tablem-tokenize(body))
// use the count of first row as columns
let columns = 0
for item in arr {
if item == [ ] {
break
}
columns += 1
}
// split rows with [ ]
let res = ()
let count = 0
for item in arr {
if count < columns {
res.push(item)
count += 1
} else {
assert.eq(item, [ ], message: "There should be a linebreak.")
count = 0
}
}
// remove secound row
if (ignore-second-row) {
let len = res.len()
res = res.slice(0, calc.min(columns, len)) + res.slice(calc.min(2 * columns, len))
}
// render with custom render function
render(columns: columns, ..args, ..res)
}
|
https://github.com/1sSay/USPTU_conspects | https://raw.githubusercontent.com/1sSay/USPTU_conspects/main/src/discrete_math/Relations.typ | typst | // Global settings and templates
#set text(14pt)
#let def(term, color: black) = {
box(stroke: color, inset: 7pt, text()[ #term ])
}
// Lecture header and date
#let lecture_header = text()[Отношения]
#let date = text()[27.09.2024]
// Header
#align(center, heading(level: 1)[Дискретная математика. \ #lecture_header ])
#align(center, text(weight: "thin")[#date])
#align(center, text(weight: "thin")[Конспект Сайфуллина Искандара БПО09-01-24])
// Content
= Свойства Отношений
#def[Рефлексивность: $ forall a in A : a R a $] \
#def[Антирефлексивность: $ forall a in A : a overline(R) a$] \
#def[Симметричность: $ forall a, b in A : a R b -> b R a $] \
#def[Антисимметричность: $ forall a, b in A : a R b = b R a -> a = b $] \
#def[Транзитивность: $ forall a, b, c in A : a R b and b R c -> a R c $] \
#def[Полнота: $ forall a, b in A : a R b or b R a $] \
= Отношение порядка
- Антисимметричность
- Транзитивность
- Рефлексивность $->$ нестрогий порядок
- Антирефлексивность $->$ строгий порядок
- Полнота $->$ полный порядок
= Экстремумы множества
- $ exists.not a in A : b < a and b != a -> a$ #text()[ -- минимальный элемент]
- $ exists.not a in A : b > a and b != a -> a$ #text()[ -- максимальный элемент]
= Отношение эквивалентности
- Рефлексивность
- Симметричность
- Транзитивность
*Обозначения*:
$ a eq.triple b $
$ a tilde b $
$ a arrow.l.r.double b $
== Фактормножество
#def[$R subset.eq A times A$ \ $forall a in A : exists A_1 subset.eq A : A_1 = {y | y in A, y tilde a}$, $A_1$ -- *класс эквивалентности*]
#def[Множество всех классов эквивалентности называется *фактомножеством множества* $А$ по эквивалентности $R$ и обозначается $A slash R = {[x] | x in A}$]
== Матричное представление отношений
Отношение можно представить квадратной матрицей
- Рефлексивность $-> forall i : 1 <= i <= n : a_(i i) = 1$
- Антирефлексивность $-> forall i : 1 <= i <= n : a_(i i) = 0$
- Симметричность $-> forall i, j : 1 <= i, j <= n : a_(i j) = a_(j i)$
== Леммы о классах эквивалентности
- $ forall a in A : [a] != emptyset $
- $ a tilde b => [a] = [b] $
- $ a tilde.not b => [a] != [b] $ |
|
https://github.com/Dherse/masterproef | https://raw.githubusercontent.com/Dherse/masterproef/main/masterproef/ugent-template.typ | typst | #import "../common/colors.typ": *
#import "../common/glossary.typ": gloss-init, gloss, glossary
#import "../common/info.typ": uinfo, udefinition, uquestion, uimportant, uconclusion, ugood, unote
#import "@preview/codly:0.2.1": codly-init, codly, codly-offset, codly-range, disable-codly
#let ugent-font = "UGent Panno Text"
#let font-size = 11pt
// Formatting function for a UGent figure captions.
#let ugent-caption(it) = {
// Check if the caption is valid.
if type(it) != content or it.func() != figure.caption {
panic("`ugent-caption` must be provided with a `figure.caption`!")
}
let supplement = {
set text(fill: ugent-blue)
smallcaps[
*#it.supplement #it.counter.display(it.numbering)*
]
};
let gap = 0.64em
let cell = grid.cell.with(
inset: (top: 0.32em, bottom: 0.32em, rest: gap),
stroke: (left: (paint: ugent-blue, thickness: 1.5pt), rest: none),
)
grid(
columns: (5em, 1fr),
gutter: 0pt,
rows: (auto),
cell(stroke: none, align(right)[#supplement]),
cell(align(left, it.body)),
)
}
// This is the entry point of the UGent template
#let ugent-template(
// The title of your thesis: a string
title: none,
// The authors of your thesis: an array of strings
authors: (),
// The programming languages you want to use.
languages: (),
// The body of your thesis.
body,
) = {
// Set the properties of the main PDF file.
set document(author: authors, title: title)
// Set the basic text properties.
set text(
font: ugent-font,
lang: "en",
size: font-size,
fallback: true,
hyphenate: false,
)
// Set the basic page properties.
set page(number-align: right, margin: 2.5cm)
// Set the basic paragraph properties.
set par(leading: 1em, justify: true)
// Additionally styling for list.
set enum(indent: 0.5cm)
set list(indent: 0.5cm)
// Global show rules for links:
// - Show links to websites in blue
// - Underline links to websites
// - Show other links as-is
show link: it => if type(it.dest) == str {
set text(fill: ugent-blue)
underline(it)
} else {
it
}
// Setup the styling of the outline entries.
show outline.entry: it => {
if it.element.func() != figure {
return it
}
let loc = it.element.location()
if state("section").at(loc) == "annex" {
let sup = it.element.supplement
// The annex index
let idx = numbering("A", ..counter(heading).at(loc))
let numbers = numbering("1", ..it.element.counter.at(loc))
let body = it.element.caption.body
[
#link(loc)[
#it.element.supplement\u{a0}#idx.#numbers: #body
]#box(width: 1fr, it.fill) #it.page
]
} else {
it
}
}
// Setting code blocks.
show: codly-init
codly(languages: languages)
show raw.where(block: true): set align(left)
// Style code blocks.
show raw.where(block: false): box.with(
fill: luma(240),
inset: (x: 3pt, y: 0pt),
outset: (y: 3pt),
radius: 2pt,
)
show: gloss-init
body
}
// Formatting function for a UGent heading
#let ugent-heading(
// Whether to include the big number.
number: true,
// Whether first level heading should have a big number.
big: false,
// The prefix to place before the number
prefix: none,
// The postfix to place after the number
postfix: " ",
// Whether to underline both the number and the title.
underline-all: false,
// The heading. Must be a heading!
it,
) = {
// Check if the heading is valid.
if type(it) != content or it.func() != heading {
panic("`ugent-heading` must be provided with a `heading`!")
}
// Get the current level if needed.
let current-level = if number == true {
counter(heading).at(it.location())
} else {
none
}
let level = it.level
let body = it.body
let num = it.numbering
let title(size, space: none, underlined: false, local-num: true) = {
// Disable justification locally
set par(leading: 1em, justify: false)
// Show a big number for title level headings
if number and level == 1 and big {
set text(size: 80pt, font: "Bookman Old Style", weight: "thin", fill: luma(50%))
numbering(num, ..current-level) + "."
linebreak()
}
// Set the text to be bold, big, and blue
set text(size: size, weight: "extrabold", font: ugent-font, fill: ugent-blue)
let num = if number and local-num and (not big or level > 1) {
prefix
numbering(num, ..current-level)
postfix
}
// Show the heading
if not underline-all { num } else { none }
if underlined {
underline(evade: true, offset: 4pt, {
if underline-all { num } else { none }
smallcaps(body)
})
} else {
smallcaps(body)
}
if space != none {
v(space)
}
}
if level == 1 {
// Include a pagebreak before the title.
pagebreak(weak: true)
block(breakable: false, title(30pt, space: 0.2em, underlined: true))
} else if level == 2 {
block(breakable: false, title(22pt, space: 10pt, underlined: true))
} else if level == 3 {
block(breakable: false, title(18pt))
} else if level == 99 {
block(breakable: false, title(14pt))
} else {
box(title(12pt, local-num: false))
}
}
// This is the entry point of the UGent preface
#let ugent-preface(body) = {
// Pages in the preface are numbered using roman numerals.
set page(numbering: "I")
// Titles in the preface are not numbered and are not outlined.
set heading(numbering: none, outlined: false)
// Show titled without numbering
show heading: ugent-heading.with(number: false)
// Set that we're in the preface
state("section").update("preface")
body
}
// Displays all of the outlines in the document.
#let ugent-outlines(
// Whether to include a table of contents.
heading: true,
// Whether to include a list of acronyms.
acronyms: true,
// Whether to include a list of figures.
figures: true,
// Whether to include a list of tables.
tables: true,
// Whether to include a list of equations.
equations: true,
// Whether to include a list of listings (code).
listings: true,
) = {
if heading {
outline(title: "Table of contents", indent: true, depth: 3)
}
if acronyms {
glossary()
}
if figures {
outline(title: "List of figures", target: figure.where(kind: image))
}
if tables {
outline(title: "List of tables", target: figure.where(kind: table))
}
if equations {
outline(title: "List of equations", target: figure.where(kind: math.equation))
}
if listings {
outline(title: "List of listings", target: figure.where(kind: raw))
}
}
// Sets up the styling of the main body of your thesis.
#let ugent-body(body) = {
// Set the pages to show as "# of #" and reset page counter.
set page(numbering: "1 of 1")
counter(page).update(1)
// Titles in the preface are not numbered and are not outlined.
set heading(numbering: "1.1.a", outlined: true)
// Show titled without numbering
show heading: ugent-heading.with(number: true, big: true)
// Set the basic paragraph properties.
set par(leading: 1em, justify: true, linebreaks: "optimized")
// Set paragraph spacing.
show par: set block(spacing: 16pt)
// Number equations.
set math.equation(numbering: "(1)")
// Set caption styling.
show figure.caption: ugent-caption
// Make figure breakable.
show figure: set block(breakable: true)
// Set figure styling: aligned at the center.
show figure: align.with(center)
// Set that we're in the body
state("section").update("body")
body
}
// Display the bibliography stylised for UGent.
#let ugent-bibliography(file: "./assets/references.bib", style: "ieee") = {
show bibliography: it => {
set heading(outlined: false)
show heading: it => {
pagebreak(weak: true)
set text(size: 30pt, weight: "extrabold", font: ugent-font, fill: ugent-blue)
underline(smallcaps(it.body), evade: true, offset: 4pt)
v(12pt)
}
it
}
bibliography(file, style: style)
}
// Set up the styling of the appendix.
#let ugent-appendix(body) = {
// Reset the title numbering.
counter(heading).update(0)
// Number headings using letters.
set heading(numbering: "A", outlined: false)
// Show titled without numbering.
show heading: ugent-heading.with(number: true, prefix: "Annex ", postfix: ": ", underline-all: true)
// Set the numbering of the figures.
set figure(numbering: (x) => {
let idx = context numbering("A", counter(heading).get().first())
[#idx.#numbering("1", x)]
})
// Additional heading styling to update sub-counters.
show heading: it => {
counter(figure.where(kind: table)).update(0)
counter(figure.where(kind: image)).update(0)
counter(figure.where(kind: math.equation)).update(0)
counter(figure.where(kind: raw)).update(0)
it
}
// Set that we're in the annex
state("section").update("annex")
body
}
/// A figure with a different outline caption and a normal caption.
#let ufigure(outline: none, caption: none, ..args) = {
if outline == none {
figure(caption: caption, ..args)
} else {
figure(caption: context if state("section").get() == "preface" {
outline
} else {
caption
}, ..args)
}
}
#let text_emoji(content, ..args) = text.with(
font: "tabler-icons",
fallback: false,
weight: "regular",
size: 16pt,
..args,
)(content)
#let lightbulb = text_emoji(fill: earth-yellow, size: font-size - 2.7pt)[\u{ea51}]
#let lightning = text_emoji(fill: green, size: font-size - 2.7pt)[\u{ea38}]
#let value = text_emoji(fill: rgb(30, 100, 200), size: font-size - 2.7pt)[\u{f61b}]
#let required = text_emoji(fill: green)[\u{ea5e}]
#let not_needed = text_emoji(fill: red)[\u{eb55}]
#let desired = text_emoji(fill: rgb(30, 100, 200))[\u{f4a5}]
#let required_sml = text_emoji(fill: green, size: font-size - 2.6pt)[\u{ea5e}]
#let not_needed_sml = text_emoji(fill: red, size: font-size - 2.6pt)[\u{eb55}]
#let desired_sml = text_emoji(fill: rgb(30, 100, 200), size: font-size - 2.6pt)[\u{f4a5}]
#let score(score, color: none) = {
let empty = "\u{ed27}"
let full = "\u{f671}"
let score = int(calc.round(score))
for _i_ in range(score) {
text_emoji(full, font: "tabler-icons")
}
for _i_ in range(5 - score) {
text_emoji(empty, font: "tabler-icons")
}
} |
|
https://github.com/Myriad-Dreamin/typst.ts | https://raw.githubusercontent.com/Myriad-Dreamin/typst.ts/main/fuzzers/corpora/layout/clip_01.typ | typst | Apache License 2.0 |
#import "/contrib/templates/std-tests/preset.typ": *
#show: test-page
// Test cliping text
#block(width: 5em, height: 2em, clip: false, stroke: 1pt + black)[
But, soft! what light through
]
#v(2em)
#block(width: 5em, height: 2em, clip: true, stroke: 1pt + black)[
But, soft! what light through yonder window breaks? It is the east, and Juliet
is the sun.
]
|
https://github.com/jgm/typst-hs | https://raw.githubusercontent.com/jgm/typst-hs/main/test/typ/layout/stack-1-02.typ | typst | Other | // Test overflow.
#set page(width: 50pt, height: 30pt, margin: 0pt)
#box(stack(
rect(width: 40pt, height: 20pt, fill: green),
rect(width: 30pt, height: 13pt, fill: red),
))
|
https://github.com/eliapasquali/typst-thesis-template | https://raw.githubusercontent.com/eliapasquali/typst-thesis-template/main/chapters/introduction.typ | typst | Other | // Non su primo capitolo
//#pagebreak(to:"odd")
= Introduzione
Introduzione al contesto applicativo.
// TODO: aggiungere riferimenti a:
// Termine nel glossario
// Citazione in linea
// Citazione nel pie' di pagina
Al momento glossario e citazioni devo ancora capirli.
== L'azienda
Descrizione dell'azienda.
== L'idea
Introduzione all'idea dello stage.
== Organizzazione del testo
#set par(first-line-indent: 0pt)
/ #link(<cap:processi-metodologie>)[Il secondo capitolo]: descrive.
/ #link(<cap:descrizione-stage>)[Il terzo capitolo]: descrive.
/ #link(<cap:progettazione-codifica>)[Il quarto capitolo]: descrive.
/ #link(<cap:verifica-validazione>)[Il quint capitolo]: descrive.
/ #link(<cap:conclusioni>)[Il sesto capitolo]: descrive.
Riguardo la stesura del testo, relativamente al documento sono state adottate le seguenti convenzioni tipografiche:
- gli acronimi, le abbreviazioni e i termini ambigui o di uso non comune menzionati vengono definiti nel glossario, situato alla fine del presente documento;
- per la prima occorrenza dei termini riportati nel glossario viene utilizzata la seguente nomenclatura: _parola_ (glsfirstoccur);
- i termini in lingua straniera o facenti parti del gergo tecnico sono evidenziati con il carattere _corsivo_.
La bibliografia è gestita nel file `bibliography.typ` con il nuovo formato Hayagriva, ma si può utilizzare il formato Bibtex. Per citare un elemento in bibliografia basta usare una semplice citazione `@citazione`, ad esempio per citare *il miglior libro di sempre* @p1 basta usare `@p1`. |
https://github.com/NaviHX/bupt-master-dissertation.typ | https://raw.githubusercontent.com/NaviHX/bupt-master-dissertation.typ/main/english-cover.typ | typst | #import "@preview/tablex:0.0.7": gridx, hlinex
#let english-cover(
roman: "Times New Roman", songti: "Noto Serif CJK SC", heiti: "Noto CJKSans SC",
title: [Master/Doctoral Disstertation], dissertation-title, student-id, name,
major, supervisor, institute, date,
) = {
set page(paper: "a4", margin: (x: 3.17cm, y: 2.54cm))
align(
center, grid(
columns: (25%, 75%), align(center, image("./image/logo.png")), align(center + horizon, text(
font: heiti, weight: "bold", size: 26pt,
)[BEIJING UNIVERSITY OF\ POSTS AND\ TELECOMMUNICATIONS]),
),
)
{
v(2em)
set text(size: 26pt)
align(center, text(size: 24pt, font: roman, title))
v(1em)
}
set underline(evade: false, offset: 8pt, stroke: 1.5pt)
set par(justify: true)
set text(font: (roman, songti), weight: "bold")
{
set text(size: 18pt)
align(center, underline(dissertation-title))
set text(size: 26pt)
v(3em)
}
{
set text(size: 14pt)
gridx(
columns: (6.05em, 25%, 30%), row-gutter: 1em, map-cells: cell => {
if cell.x == 1 {
cell.content = align(right, cell.content)
}
cell
}, [],
[Student ID:], [#student-id], hlinex(start: 2, gutter-restrict: top), [],
[Author:], [#name], hlinex(start: 2, gutter-restrict: top), [],
[Subject:], [#major], hlinex(start: 2, gutter-restrict: top), [],
[Supervisor:], [#supervisor], hlinex(start: 2, gutter-restrict: top), [],
[Institute:], [#institute], hlinex(start: 2, gutter-restrict: top),
)
}
v(1em)
align(
center + bottom, text(size: 14pt, date.display("[day]/[month]/[year]")),
)
}
|
|
https://github.com/kaarmu/splash | https://raw.githubusercontent.com/kaarmu/splash/main/src/palettes/kth.typ | typst | MIT License | /* The official color palette for KTH Royal Institute of Technology.
*
* Source: https://intra.kth.se/en/administration/kommunikation/grafiskprofil/profilfarger-1.845077
* Accessed: 2023-04-22
*/
#let kth-rgb = (
blue : rgb(25, 84, 166),
pale-blue : rgb(36, 160, 216),
pink : rgb(216, 84, 151),
green : rgb(176, 201, 43),
gray : rgb(101, 101, 108),
)
#let kth-cmyk = (
blue : cmyk(100%, 55%, 0%, 0%),
pale-blue : cmyk(75%, 20%, 0%, 0%),
pink : cmyk(10%, 80%, 0%, 0%),
green : cmyk(40%, 0%, 100%, 0%),
gray : cmyk(30%, 25%, 15%, 50%),
)
|
https://github.com/19pdh/suplement-sprawnosci | https://raw.githubusercontent.com/19pdh/suplement-sprawnosci/master/style.typ | typst | #let lay(left, right) = grid(
columns: 2,
gutter: 1cm,
left,
right
)
#let tagi(str) = text(fill: rgb("#2b2"))[#str]
#let proba(spr) = lay(
image(spr.img, width: 3.5cm),
[= #spr.nazwa
#spr.tagi
#spr.opis
]
) |
|
https://github.com/hei-templates/hevs-typsttemplate-thesis | https://raw.githubusercontent.com/hei-templates/hevs-typsttemplate-thesis/main/00-templates/helpers.typ | typst | MIT License | //
// Description: Import other modules so you only need to import the helpers
// Use : #import "../00-templates/helpers.typ": *
// Author : <NAME>
//
#import "../00-templates/boxes.typ": *
#import "../00-templates/constants.typ": *
#import "../00-templates/items.typ": *
#import "../00-templates/sections.typ": *
#import "../00-templates/tablex.typ": *
#import "../01-settings/metadata.typ": *
#import "../03-tail/glossary.typ": *
// External Plugins
// Fancy pretty print with line numbers and stuff
#import "@preview/codelst:2.0.1": sourcecode
#let myref(label) = locate(loc =>{
if query(label,loc).len() != 0 {
ref(label)
} else {
text(fill: red)[?]
}
})
//-------------------------------------
// Acronym functions
//
#let acrshort(item) = {
item.abbr
}
#let acrlong(item) = {
[#item.long)]
}
#let acrfull(item) = {
[#item.long (#item.abbr)]
}
//-------------------------------------
// Table of content
//
#let toc(
lang: "en",
tableof: (
toc: true,
minitoc : false,
tof: false,
tot: false,
tol: false,
toe: false,
),
indent: true,
depth: none,
) = {
// Table of content
if tableof.toc == true {
outline(
title: [#if lang == "de" {"Inhalt"} else if lang == "fr" {"Contenu"} else {"Contents"}],
indent: indent,
depth: depth,
)
}
// Table of figures
if tableof.tof == true {
outline(
title: [#if lang == "de" {"Abbildungen"} else if lang == "fr" {"Figures"} else {"Figures"}],
target: figure.where(kind: image),
indent: indent,
depth: depth,
)
}
// Table of tables
if tableof.tot == true {
outline(
title: [#if lang == "de" {[Tabellen]} else if lang == "fr" {[Tables]} else {[Tables]}],
target: figure.where(kind: table),
indent: indent,
depth: depth,
)
}
// Table of listings
if tableof.tol == true {
outline(
title: [#if lang == "de" {"Programme"} else if lang == "fr" {"Programmes"} else {"Listings"}],
target: figure.where(kind: raw),
indent: indent,
depth: depth,
)
}
// Table of equation
if tableof.toe == true {
outline(
title: [#if lang == "de" {"Gleichungen"} else if lang == "fr" {"Équations"} else {"Equations"}],
target: math.equation.where(block:true),
indent: indent,
depth: depth,
)
}
}
#let minitoc(
after: none,
before: none,
addline: true,
stroke: 0.5pt,
length: 100%
) = {
v(2em)
text(large, [*Contents*])
if addline == true {
line(length:length, stroke:stroke)
}
outline(
title: none,
target: selector(heading)
.after(after)
.before(before, inclusive: false)
)
if addline == true {
line(length:length, stroke:stroke)
}
}
//-------------------------------------
// Heading shift
//
#let unshift_prefix(prefix, content) = style((s) => {
pad(left: -measure(prefix, s).width, prefix + content)
})
//-------------------------------------
// Research
//
// item, item, item and item List
//
#let enumerating_authors(
items: none,
) = {
let i = 1
if items != none {
for item in items {
if item != none {
if item.name != none and item.institute != none {
[#item.name#super(repr(item.institute))]
} else if item.name != none {
[#item.name]
}
if i < items.len() {
[, ]
}
}
i = i + 1
}
}
}
#let enumerating_institutes(
items: none,
) = {
let i = 1
if items != none {
for item in items {
if item != none {
[_#super(repr(i))_ #if item.research_group != none { [_ #item.research_group - _]} _ #item.name __, #item.address _ \ ]
i = i + 1
}
}
}
}
//-------------------------------------
// Script
//
// item, item, item and item List
//
#let enumerating_items(
items: none,
) = {
let i = 1
if items != none {
for item in items {
if item != none {
[#item]
if i < items.len() {
[, ]
}
}
i = i + 1
}
}
}
#let enumerating_links(
names: none,
links: none,
) = {
if names != none {
let i = 0
for name in names {
if name != none {
[#link(links.at(i))[#name]]
if i+1 < names.len() {
[, ]
}
}
i = i + 1
}
}
}
#let enumerating_emails(
names: none,
emails: none,
) = {
if names != none {
let i = 0
for name in names {
if name != none {
[#link("mailto:"+emails.at(i))[#name]]
if i+1 < names.len() {
[, ]
}
}
i = i + 1
}
}
}
//-------------------------------------
// safe_link
//
#let safe_link(
name: none,
url: none,
) = {
if name != none {
if url != none {
link(url)[#name]
} else {
name
}
}
}
//-------------------------------------
// Counter
//
#let word_counter_init() = {[
#show regex("\b\w+\b"): it => counter("words").step() + it
]}
#let word_count(preamble:"Word count:") = {[
#preamble #counter("words").display()
]}
#let char_counter_init() = {
show regex(".+"): it => counter("chars").step() + it
}
#let char_count(preamble:"Char count:") = {[
#preamble #counter("chars").display()
]}
//-------------------------------------
// Option Style
//
#let option_style(
type: "draft",
size: small,
style: "italic",
fill: gray-40,
body) = {[
#if option.type == type {text(size:size, style:style, fill:fill)[#body]
}
]} |
https://github.com/stat20/stat20handout-typst | https://raw.githubusercontent.com/stat20/stat20handout-typst/main/README.md | markdown | # Stat20handout-typst Format
## Installing
```bash
quarto use template stat20/stat20handout-typst
```
This will install the format extension and create an example qmd file
that you can use as a starting place for your document.
## Features
Beyond some small changes to margins and line spacing, this template adds a header and footer.
### Header
The header is populated by the values of two yml metadata fields: `title` and `title-prefix`.
### Footer
The header is populated by the values of two yml metadata fields: `course-name` and `semester`.
|
|
https://github.com/krestenlaust/AAU-Typst-Template | https://raw.githubusercontent.com/krestenlaust/AAU-Typst-Template/main/README.md | markdown | MIT License | # AAU Typst Template
The widely used AAU LaTeX report template, rewritten in Typst.
## Template Overview
This project contains the following templates:
- `Report template`
This is the general template, it contains good examples of how to use Typst for common tasks.
- `Report template (empty)` (based on `Report template`)
This is an empty version of the report template. It doesn't contain examples, only filled with lorem ipsum.
- `Singlepage Template` (based on `Report template`)
This template shows how to use the template without 'complicated' folder structure.
### Where to get the templates
The templates are generated for each commit, and every release. You can get the latest 'stable'-template on [releases page](https://github.com/krestenlaust/AAU-Typst-Template/releases).
Every release and commit also contains artifacts with compiled versions (PDFs) to show how they're going to look.
### Using a template with [Typst.app](https://typst.app/)
If you've just barely convinced your group to make use of Typst, then using the web app is probably ideal. It will be familar to Overleaf users, as it resembles a lot, just without all the UI clutter.
#### This shows how to import the `Report template`:

Typst.app doesn't currently support dragging folders, therefore the folders should be created manually, and files dragged in accordingly. **Folder name casing is important.**
## Template Structure
This is an overview of the folders/files in the root of this repository:
Each template contains the following:
- `AAUgraphics`
Contains the graphics used in the template.
- `main.typ`
This is the main source, it includes any other files, and this file is the sole target for the compiler, if you want to compile locally, run `typst compile main.typ`.
- `template.typ`
The main template file. This file is located in `report-template` (and a copy is stored in `singlefile-template`).
This file uses the graphics located in `AAUgraphics`.
## Inspiration
This project replicates the (semi-official) AAU report template located at [jkjaer/aauLatexTemplates](https://github.com/jkjaer/aauLatexTemplates).
If you still insist on using LaTeX, then my good friend, [@Tosuma](https://www.github.com/Tosuma), has improved the AAU LaTeX template considerately: [Tosuma/AAU-Latex-Template-English](https://github.com/Tosuma/AAU-Latex-Template-English).
## Contribution
Any contribution is welcome!
|
https://github.com/noriHanda/my-resume | https://raw.githubusercontent.com/noriHanda/my-resume/main/main.typ | typst | The Unlicense | #import "modern-resume.typ": modern-resume, workExperience, educationalExperience, project, pill
#let data = (
name: "<NAME>",
jobTitle: "Mobile App Engineer",
bio: "An experienced Flutter developer", // Optional parameter
avatarImagePath: "picture.jpeg", // Optional parameter
contactOptions: ( // Optional parameter, all entries are optional
email: link("mailto:<EMAIL>")[<EMAIL>],
mobile: "+81 80 6672 7600",
location: "Japan",
linkedin: link("https://www.linkedin.com/in/noriaki-handa-8a18221aa/")[linkedin/noriaki-handa-8a18221aa],
github: link("https://github.com/noriHanda")[github.com/noriHanda],
// website: link("https://jdoe.dev")[jdoe.dev],
),
)
#show: doc => modern-resume(data, doc)
== Education
#educationalExperience(
title: "B.S. in Computer Science",
subtitle: "Hokkaido University",
taskDescription: [
- Integrated System Engineering and Computer Engineering using Verilog HDL
- Kinesthetic sense based on evaluation of actuator energy for artificial sense in myoelectric prosthetic hands
],
dateFrom: "04/2019",
dateTo: "03/2024",
)
#educationalExperience(
title: "National Honor Society",
subtitle: "William Fremd High School",
taskDescription: [
- College-level AP courses in Computer Science, Calculus, and Physics
- Enrolled in the National Honor Society
],
dateFrom: "08/2015",
dateTo: "05/2018",
)
== Work experience
#workExperience(
title: "Mobile App Developer",
subtitle: [#link("https://h-medtech.com/")[Hedgehog Medtech, Inc.]],
facilityDescription: "Medical treatment app development company",
taskDescription: [
- Spearheaded the development of critical medical treatment apps, improving patient conditions on migraine and premenstrual syndrome collaborating with designers who made wireframes on Figma.
- Collaborated closely with healthcare professionals to ensure the apps met rigorous industry standards and user needs.
- Setted up and maintained the CI/CD pipeline for the mobile app distribution. Reduced the cost of app distribution by more than 80%.
],
dateFrom: "08/2022",
dateTo: "03/2024",
)
#workExperience(
title: "Contract Development",
subtitle: [#link("https://apps.apple.com/app/id6466738852")[Rakulip]],
facilityDescription: "A video editor app for researchers in Hokkaido University",
taskDescription: [
- Evaluated requirements and designed the architecture of the app.
- Developed the app using Flutter and FFmpeg.
- Released the app on the App Store and Google Play.
- Demonstrated and supported the use of the app at a conference.
- Enhanced the app based on feedback from users and achieved 30% increase in store rating.
- Fixed bugs and improved video quality by making adjustment to the FFmpeg parameters.
],
dateFrom: "08/2023",
dateTo: "03/2024",
)
#workExperience(
title: "Product Manager/Mobile App Developer",
subtitle: [#link("https://thephage.life/")[THE PHAGE, Inc.]],
facilityDescription: "Medical treatment app development company",
taskDescription: [
- Made a blueprint of the team and the product and led the company to the next stage.
- Developed a mobile app that makes biabetes treatment more efficient.
- Selected technologies that were best suited for the product and the team and led the company to have a better presence in the market.
- Researched and developed a cutting edge algorithm that processes signals from a wearable device and predicts the user's blood sugar level.
],
dateFrom: "12/2020",
dateTo: "07/2022",
)
#workExperience(
title: "Chief Technology Officer",
subtitle: "Conomai, Inc.",
facilityDescription: "Medical treatment company",
taskDescription: [
- Made several minimum viable products and conducted user interviews to find out the best product for the company and the market.
- Inquired to venture capitalists and banks and raised funds for the company.
- Made capital plans and business plans and led the company to the next stage with the cofounders.
],
dateFrom: "09/2019",
dateTo: "02/2020",
)
#workExperience(
title: "Internship",
subtitle: [#link("https://www.digitalgrid.com/")[DIGITAL GRID, Inc.]],
facilityDescription: "Electricity traceability and trading company",
taskDescription: [
- Explained the company's business at a conference and led to further business opportunities.
- Interviewed all the people in the company and made interview articles so that everyone in the company could better understand each other.
],
dateFrom: "08/2020",
dateTo: "09/2020",
)
#colbreak()
== Skills
#pill("Flutter", fill: true)
#pill("Riverpod", fill: true)
#pill("App Deployment", fill: true)
#pill("Git/GitHub", fill: true)
#pill("Jira", fill: true)
#pill("Product Management", fill: true)
#pill("Teamwork", fill: true)
#pill("Critical thinking", fill: true)
#pill("Problem solving", fill: true)
== Projects
#project(
title: link("https://github.com/torkralle/notion_wordbook")[Notion Wordbook],
subtitle: "Vocabulary learning app for Notion users",
description: [
- Developed with a team of three people using Flutter and Notion API.
- The app had a feature that references the user's Notion database and creates a vocabulary list out of it.
- Reviewed code and taught anything needed to junior members.
],
dateFrom: "02/2022",
dateTo: "08/2022",
)
#project(
title: link("https://github.com/noriHanda/study_matching_flutter")[Study Matching],
subtitle: "SNS for campus students",
description: [
- Developed with a team of three people using Flutter and Django REST framework.
- The app had a feature that matches students who want to study together.
- The number of users hit 1000 in 3 months.
],
dateFrom: "06/2019",
dateTo: "09/2020",
)
== Languages
#pill("Japanese (native)")
#pill("English (fluent)")
== Interests
#pill("Buddhism")
#pill("Indian Philosophy")
#pill("Philosophy")
#pill("European Football")
#pill("Folkloristics")
#pill("Agriscience")
#pill("History")
|
https://github.com/alexanderkoller/typst-blinky | https://raw.githubusercontent.com/alexanderkoller/typst-blinky/main/README.md | markdown | MIT License | # Blinky: Bibliography Linker for Typst
This package permits the creation of Typst bibliographies in which paper titles are typeset as hyperlinks. Here's an example (with links typeset in blue):
<center>
<img src="https://github.com/alexanderkoller/typst-blinky/blob/main/examples/screenshot.png" width="80%" />
</center>
The bibliography is generated from a Bibtex file, and citations are done with the usual Typst mechanisms. The hyperlinks are specified through DOI or URL fields in the Bibtex entries; if such a field is present, the title of the entry will be automatically typeset as a hyperlink.
See [here](https://github.com/alexanderkoller/typst-blinky/tree/main/examples) for a full example.
## Usage
Adding hyperlinks to your bibliography is a two-step process: (a) use a CSL style with magic symbols (explained below), and (b) enclose the `bibliography` command with the `link-bib-urls` function:
```
#import "@preview/blinky:0.1.0": link-bib-urls
... @cite something ... @cite more ...
#let bibsrc = read("custom.bib")
#link-bib-urls(bibsrc)[
#bibliography("custom.bib", style: "./association-for-computational-linguistics-blinky.csl")
]
```
Observe that the Bibtex file `custom.bib` is loaded twice: once to load into `link-bib-urls` and once in the standard Typst `bibliography` command. Obviously, this needs to be the same file twice. See under "Alternative solutions" below why this can't be simplified further at the moment.
If a Bibtex entry contains a DOI field, the title will become a hyperlink to the DOI. Otherwise, if the Bibtex entry contains a URL field, the title will become a hyperlink to this URL. Otherwise, the title will be shown as normal, without a link.
## CSL with magic symbols
Blinky generates the hyperlinked titles through a regex show rule that replaces a "magic symbol" with a [link](https://typst.app/docs/reference/model/link/) command. This "magic symbol" is a string of the form `!!BIBENTRY!<key>!!`, where `<key>` is the Bibtex citation key of the reference.
You will therefore need to tweak your CSL style to use it with Blinky. Specifically, in every place where you would usually have the paper title, i.e.
```
<text variable="title" prefix=" " suffix=". "/>
```
or similar, your CSL file now instead needs to print a decorated version of the paper's citation-key (= Bibtex key):
```
<text variable="citation-key" prefix=" !!BIBENTRY!" suffix="!!. " />
```
You can have more prefix before and suffix after the `!!BIBENTRY!` and `!!`, as in the example, but these magic symbols need to be there so Blinky can find the places in the document where the hyperlinked title needs to be inserted.
You can check the [example CSL file](https://github.com/alexanderkoller/typst-blinky/blob/main/examples/association-for-computational-linguistics-blinky.csl) to see what this looks like in practice; compare to [the unmodified original](https://github.com/citation-style-language/styles/blob/master/association-for-computational-linguistics.csl).
## Alternative solutions
The current mechanism in Blinky is somewhat heavy-handed: a Typst plugin uses the [biblatex](https://github.com/typst/biblatex) crate to parse the Bibtex file (independently of the normal operations of the `bibliography` command), and then all occurrences of the magic symbol in the Typst bibliography are replaced by the hyperlinked titles.
It would be great to replace this mechanism by something simpler, but it is actually remarkably tricky to make bibliography titles hyperlinks with the current version of Typst (0.11.1). All the alternatives that I could think of don't work. Here are some of them:
- Print the URL/DOI using the CSL style, and then use a regex show rule to convert it into a `link` around the title somehow. This does not work because most URLs contain a colon character (:), and these [cause trouble with Typst regexes](https://github.com/typst/typst/issues/86).
- Make the CSL style output text of the form `#link(url)[title]`. This does not work because the content generated by CSL is not evaluated further by Typst. Also, Typst [does not support show rules for the individual bibliography items](https://github.com/typst/typst/issues/942), which makes it tricky to call [eval](https://typst.app/docs/reference/foundations/eval/) on them.
- Create a show rule for `link`. Some CSL styles already generate `link` elements if a URL/DOI is present in the bib entry - one could consider replacing it with a `link` whose URL is the same as before, but the text is a link symbol or some such. However, a show rule for a link that generates another link runs into an infinite recursion; Typst made [the deliberate decision](https://github.com/typst/typst/pull/3327) to handle such recursions only for `text` show rules.
- The best solution would be to simply use an unmodified CSL file, but it is not clear to me how one would pick out the paper title from the bibliography in a general way. I'm afraid that any solution that hyperlinks titles will require modifications to the CSL style.
It would furthermore be desirable to hide the fact that we are reading the same Bibtex file twice behind a single function call. However, code in a Typst package [resolves all filenames relative to the package directory](https://github.com/typst/typst/issues/2126), which means that the package cannot access a bibliography file outside of the package directory. We may be able to simplify this once [#971](https://github.com/typst/typst/issues/971) gets addressed.
|
https://github.com/KiranWells/hackable-typst-resume | https://raw.githubusercontent.com/KiranWells/hackable-typst-resume/main/resume.typ | typst | /*
* Typst Resume Template
*
* Designed as a simple and hackable template for resumes.
* It is recommended to change the template to fit your needs.
*/
// These are used to add the accent color and gradient in the header
#let default_accent = color.map.flare.at(37)
#let default_gradient = (
..color.map.flare,
)
#let icon(name) = {
// select a font with the Nerd Font icons
// (ideally the "Propo" version for spacing)
// https://www.nerdfonts.com/
set text(font: "FiraCode Nerd Font Propo")
// fill in any missing icons here; the names are always lower-case
// https://www.nerdfonts.com/cheat-sheet
if name == "profile" []
if name == "mail" []
if name == "github" []
if name == "linkedin" []
if name == "openapi" []
if name == "python" []
if name == "c" []
if name == "c++" []
if name == "rust" []
if name == "javascript" []
if name == "css" []
if name == "html" []
if name == "glsl" []
if name == "hlsl" []
if name == "linux" []
if name == "windows" []
if name == "apple" []
if name == "microsoft word" []
}
/// The following are the resume element functions that handle the structure of each block
// The main document header with social links. This
// is automatically created by the resume class function
#let header(
name: "", email: "", linkedin: "", github: "", website: "", font: "Noto Serif", color: default_gradient
) = {
let name = text(font: font, weight: "black", size: 20pt)[#name]
let socials = grid(
columns: (auto, 0pt, auto),
gutter: 6pt,
rows: (auto, auto),
align(right, link("https://" +website)[#icon("profile") #website]),
grid.vline(position: start),
[],
align(left, link("mailto:"+email)[#icon("mail") #email]),
align(right, link("https://github.com/"+github)[#icon("github") #github]),
[],
align(left, link("https://linkedin.com/in/"+linkedin)[#icon("linkedin") #linkedin])
)
set block(spacing: 8pt)
context {
let name_size = measure(name)
let socials_size = measure(socials)
align(center, {
name
line(length: (name_size.width + socials_size.width) / 2, stroke: 2pt + color)
socials
})
}
}
// A simple "highlight" element that pulls focus to key details
#let chip(color: default_accent, content) = {
underline(stroke: 1pt + color)[#content]
}
// A set of skills, with the important_skills highlighted and an optional label
#let skills(label: none,important_skills, other_skills, accent_color: default_accent) = {
set block(spacing: 2pt)
if label != none {
block(
smallcaps(text(size: 7pt, label))
)
}
box(important_skills.map(chip.with(color: accent_color)).join(", "))
if important_skills.len() > 0 {
[, ]
}
other_skills.join(", ")
}
// A block that can display work experience, education, or other experiences
#let experience(
name: "Title",
highlight: "Highlight",
detail,
date,
accent_color: default_accent,
body
) = block(inset: (left: 2em))[
#grid(columns: (1fr, 1fr),
[== #name],
align(right, text(fill: accent_color, weight: "bold", size: 11pt)[#highlight])
)
#text(style: "italic", detail) #h(2fr) #text(style: "italic", date)
#body
]
// A block that can link to a website and have an image. Useful for portfolio items or certifications
#let linked_block(
name: "Name",
address: "",
github: none,
tech: ("",),
image_file: none,
accent_color: default_accent,
body
) = {
let inner = {
let true_link = if github != none {
"https://" + github
} else {
address
}
link(true_link)[== #name]
block[
#body
]
tech.map(it => [#text(fill: accent_color, icon(lower(it))) *#it*]).join(h(1em))
}
if image_file != none {
grid(
columns: (40pt, auto),
column-gutter: 5pt,
image(image_file),
inner
)
} else {
inner
}
}
// This is the main resume class, which sets up the header and styles for the document
#let resume(
name: "<NAME>",
contacts: (
email: "<EMAIL>",
linkedin: "linkedin",
github: "github",
website: "example.com",
),
keywords: (),
paper: "us-letter",
accent_color: default_accent,
header_gradient: default_gradient,
header_font: "Noto Serif",
body_font: "Noto Sans",
spacing: 1em,
debug: false,
doc
) = {
let debug_stroke = if debug { 0.1pt + red } else { none }
set block(
spacing: spacing,
stroke: debug_stroke,
)
set text(font: body_font, size: 9pt)
set page(
paper: paper,
margin: 0.5in,
background: [#block(width:100%, height: 100%, fill: white)]
)
// This changes the default look of the level 1 headings
show heading.where(level: 1): it => block(
outset: (left: -2.5pt),
stroke: (left: 6pt + accent_color),
width: 100%,
inset: (left: 7pt, bottom: 2.5pt, top: 5pt),
spacing: spacing,
block(
spacing: spacing,
width: 100%,
stroke: (
bottom: (paint: black, thickness: 1pt, dash: "dotted"),
),
inset: (
bottom: 2.5pt,
),
text(weight: "extrabold", it)
)
)
show heading.where(level: 3): it => {
v(-0.8em)
it
}
show list: it => block(inset: (left: 1em), it)
set document(
title: [#name's Resume],
author: name,
keywords: keywords,
)
header(name: name, ..contacts, color: gradient.linear(..header_gradient, relative: "self"))
doc
}
|
|
https://github.com/lucannez64/Notes | https://raw.githubusercontent.com/lucannez64/Notes/master/Physique_Ex_18_12_2023.typ | typst | #import "template.typ": *
// Take a look at the file `template.typ` in the file panel
// to customize this template and discover how it works.
#show: project.with(
title: "Physique Ex 18 12 2023",
authors: (
"<NAME>",
),
date: "17 Décembre, 2023",
)
#set heading(numbering: "1.1.")
=== Exercice 4 p164
<exercice-4-p164>
+ $ K_e eq lr([H_3 O^plus])_(upright("éq")) times lr([H O^minus])_(upright("éq")) $
+ $ lr([H_3 O^plus])_(upright("éq")) eq K_e / lr([H O^minus])_(upright("éq")) eq frac(10 times 10^(minus 14), 3 comma 2 times 10^(minus 6)) eq 3 comma 125 times 10^(minus 9) approx 3 comma 1 times 10^(minus 9) upright("mol") upright("L") ""^(minus 1) $
+ $ p H eq minus l o g lr([H_3 O^plus])_(upright("éq")) / c^0 eq minus l o g lr((3 comma 1 times 10^(minus 9))) eq 8 comma 5 $
=== Exercice 8 p164
<exercice-8-p164>
+ $ C H_3 N H_2 lr((a q)) plus H_2 O lr((l)) harpoons.rtlb C H_3 N H_3^plus lr((a q)) plus H O^minus lr((a q)) $
+ $ tau eq x_f / x_(m a x) eq frac(lr([C H_3 N H_3^plus])_f times V, C times V) eq lr([C H_3 N H_3^plus])_f / C $
$ lr([C H_3 N H_3^plus])_f eq tau times C $
$ lr([C H_3 N H_3^plus])_f eq 0 comma 22 times 1 comma 0 times 10^(minus 2) $
$ lr([C H_3 N H_3^plus])_f eq 2 comma 2 times 10^(minus 3) upright("mol") upright("L") ""^(minus 1) $
De plus $ lr([C H_3 N H_2])_f eq C minus lr([C H_3 N H_3^plus])_f $
$ lr([C H_3 N H_2])_f eq 1 comma 0 times 10^(minus 2) minus 2 comma 2 times 10^(minus 3) $
$ lr([C H_3 N H_2])_f eq 7 comma 8 times 10^(minus 3) upright("mol") upright("L") ""^(minus 1) $
|
|
https://github.com/LDemetrios/Programnomicon | https://raw.githubusercontent.com/LDemetrios/Programnomicon/main/reinterpret_cast%20and%20java/reinterpret-cast-and-java.typ | typst | #import "/common/java-kotlin-launch.typ": *
#set page(height:auto)
#show: single-file-sample(
"/reinterpret_cast and java/reinterpret-cast-and-java.typ",
add-imports: "import sun.misc.Unsafe;\nimport java.lang.reflect.*;",
start-marker: "//Start\n",
end-marker: "//End\n",
)
= #smallcaps[Is it possible to do a `reinterpret_cast` in Java?]
Well, at first glance someone could say "No, there is no such construction in Java". And they would be right. That's because the Object in Java is not just a collection of fields, it contains the information about the object itself --- the object _header_. In most JVMs, for 64-bit architectures the header size is 128 bits. Those are a *Mark Word* (information about the state of the object: GC notes, associated lock etc.) and a *Klass Word* (pointer to the information about the class of the object).
== #smallcaps[So, there is nothing we could do?]
Not exactly.
We'll need an instance of the class called `com.misc.Unsafe`. It's a singleton class, and the instance is returned by the method `getUnsafe`
```java
//Start
public static void main(String[] args) {
Unsafe unsafe = Unsafe.getUnsafe();
}
//End
``` <class>
A shame. We aren't supposed to use it. _Normally_.
OK, let's bring the heavy artillery in.
```java
//Start
public static void main(String[] args) throws NoSuchFieldException, IllegalAccessException {
Field f = Unsafe.class.getDeclaredField("theUnsafe");
f.setAccessible(true);
Unsafe unsafe = (Unsafe) f.get(null);
System.out.println(unsafe);
}
//End
``` <class>
Well, that works. Now let's declare two classes with one field each.
```java
//Start
static class A {
long field = 566;
}
static class B {
long field = 30;
}
public static void main(String[] args) throws NoSuchFieldException, IllegalAccessException {
Field f = Unsafe.class.getDeclaredField("theUnsafe");
f.setAccessible(true);
Unsafe unsafe = (Unsafe) f.get(null);
A a = new A();
System.out.println(unsafe.getLong(a, 0));
System.out.println(unsafe.getLong(a, 8));
System.out.println(unsafe.getLong(a, 16));
}
//End
``` <class>
Our expectations are satisfied. There are `mark` word, then `klass` word, then the `field`. Let's open the Hotspot JVM specifications. Last two bits equal to `01` indicate that the object is unlocked. In fact, let's play with it:
```java
static class A {
long a = 566;
}
public static void main(String[] args) throws NoSuchFieldException, IllegalAccessException {
Field f = Unsafe.class.getDeclaredField("theUnsafe");
f.setAccessible(true);
Unsafe unsafe = (Unsafe) f.get(null);
A a = new A();
//Start
System.out.println(unsafe.getLong(a, 0));
System.out.println(a);
System.out.println(unsafe.getLong(a, 0));
synchronized (a) {
System.out.println(unsafe.getLong(a, 0));
}
System.out.println(unsafe.getLong(a, 0));
//End
}
```<class>
Wait, what have just happened? First of all, we asked to print `a`, it called `a.toString()`, which in turn called `a.hashCode()`. Hash code turned out to be `6d06d69c`,
and it was cached in the object header. `Mark` word is now `000000`#text(fill:blue,`6d06d69c`)`01`. The we called `synchronized` on it, the lock was created, then we unlocked it.
The `klass` word, however, never changed. Now let's change it!
```java
static class A {
long field = 566;
}
static class B {
long field = 30;
}
public static void main(String[] args) throws NoSuchFieldException, IllegalAccessException {
Field f = Unsafe.class.getDeclaredField("theUnsafe");
f.setAccessible(true);
Unsafe unsafe = (Unsafe) f.get(null);
//Start
Object a = new A();
Object b = new B();
System.out.println(a.getClass());
unsafe.putLong(a, 8, unsafe.getLong(b, 8));
System.out.println(a.getClass());
//End
}
``` <class>
OK, but what happens if we cast it to `B`? Nothing special. Now the `JVM` is completely sure that what lies behind `a` is actually `B`.
```java
static class A {
long field = 566;
}
static class B {
long field = 30;
}
public static void main(String[] args) throws NoSuchFieldException, IllegalAccessException {
Field f = Unsafe.class.getDeclaredField("theUnsafe");
f.setAccessible(true);
Unsafe unsafe = (Unsafe) f.get(null);
Object a = new A();
Object b = new B();
unsafe.putLong(a, 8, unsafe.getLong(b, 8));
//Start
B itsSoWrong = (B) a;
System.out.println(itsSoWrong.field);
//End
}
``` <class>
But don't forget. Everything is Java is by reference. What if we had a reference of type `A` left?
```java
static class A {
long field = 566;
}
static class B {
long field = 30;
}
public static void main(String[] args) throws NoSuchFieldException, IllegalAccessException {
Field f = Unsafe.class.getDeclaredField("theUnsafe");
f.setAccessible(true);
Unsafe unsafe = (Unsafe) f.get(null);
//Start
A a = new A();
B b = new B();
unsafe.putLong(a, 8, unsafe.getLong(b, 8));
B itsSoWrong = (B) a;
System.out.println(itsSoWrong.field);
//End
}
``` <class>
Oh wait, it's a compilation error. There is no way `a` can actually point to some `B`, right? _Right?_
```java
static class A {
long field = 566;
}
static class B {
long field = 30;
}
public static void main(String[] args) throws NoSuchFieldException, IllegalAccessException {
Field f = Unsafe.class.getDeclaredField("theUnsafe");
f.setAccessible(true);
Unsafe unsafe = (Unsafe) f.get(null);
//Start
A a = new A();
Object probablyA = a;
B b = new B();
unsafe.putLong(a, 8, unsafe.getLong(b, 8));
B itsSoWrong = (B) probablyA;
System.out.println(itsSoWrong.field);
System.out.println(a.getClass());
System.out.println(a.field);
//End
}
``` <class>
Surprisinlgy still seems to be fine.
```java
static class A {
long field = 566;
}
static class B {
long field = 30;
}
public static void main(String[] args) throws NoSuchFieldException, IllegalAccessException {
Field f = Unsafe.class.getDeclaredField("theUnsafe");
f.setAccessible(true);
Unsafe unsafe = (Unsafe) f.get(null);
A a = new A();
Object probablyA = a;
B b = new B();
unsafe.putLong(a, 8, unsafe.getLong(b, 8));
B itsSoWrong = (B) probablyA;
//Start
A thatWasA = (A) (Object) a;
//End
}
``` <class>
Yeah, that broke. `a` is not `A` anymore.
|
|
https://github.com/khawarizmus/hijri-week-calendar-proposal | https://raw.githubusercontent.com/khawarizmus/hijri-week-calendar-proposal/main/main.typ | typst | ISC License | #import "layout.typ": title, subtitle, authors, affiliations, abstract, margins, publishDate, keywords
#import "lapreprint.typ": template
#show: template.with(
title: title,
subtitle: subtitle,
open-access: true,
font-face: "Noto Sans",
heading-numbering: "1.1.1",
keywords: keywords,
theme: green.darken(40%),
authors: authors,
affiliations: affiliations,
date: (
(title: "Published", date: publishDate),
),
abstract: abstract,
margin: margins,
)
#include "content.typ" |
https://github.com/Area-53-Robotics/53A-Notebook-Over-Under-2023-2024 | https://raw.githubusercontent.com/Area-53-Robotics/53A-Notebook-Over-Under-2023-2024/master/Vex%20Robotics%2053A%20Notebook%202023%20-%202024/Entries/Misc.%20Entry/Digital-Notebook.typ | typst | #set page(header: [ VR
#h(1fr)
October 23, 2023
])
= Digital Notebook
\
*Switch*
\
Today 53A decided to proceed with a digitally formatted notebook.
After handwriting the first 144 pages of our notebook, we found that it was a waste of time and team resources.
I handwrote our notebook because I was taught it was best practice for documenting the engineering design process, and in past years it has not been such a draining task. This season, we have written our most extensive and detailed design journal, causing handwriting to be more strenuous.
\
*Formatting*
#underline[Headers]
= Title
== Level 1 Heading
=== Level 2 Heading
==== Level 3 Heading
\
#underline[Daily Stand-Ups]
#let cell1 = rect.with(
inset: 8pt,
fill: rgb("#E3E3E3"),
width: 100%,
radius: 10pt
)
#grid(
columns: (170pt, 170pt, 170pt),
rows: (50pt, auto),
gutter: 3pt,
cell1(height: 115%)[*Team Member 1* \ Tasks],
cell1(height: 115%)[*Team Member 2* \ Tasks],
cell1(height: 115%)[*Team Member 3* \ Tasks]
)
- Team members updates on their assignments
- Each person’s tasks are highlighted in a grey bubble
\
#underline[Iterations]
#block(
fill: rgb("FFEAE8"),
width: 100%,
inset: 8pt,
radius: 4pt,
[
*Problem Analysis*
- Highlighted in Red
],
)
#block(
width: 100%,
fill: rgb("EEEEFF"),
inset: 8pt,
radius: 4pt,
[
*Solution(s)*
- Highlighed in blue
])
#block(
width: 100%,
fill: rgb("FFFEEB"),
inset: 8pt,
radius: 4pt,
[*Design*
- Highlighted in yellow]
)
#underline[Code Entries]
- Each image of our code is accompanied by a plain-language explanation of what the code does
- _Variables_ from our code are green in the plain-text explanation
\
#underline[Testing]
- Labled with “Testing” title
- Organized in tables
#table(
columns: (25%,75%),
rows: (3.5%),
fill: (_, row) => if row == 2 {rgb("#E4FFE6")}
else if row == 1 {rgb("#FFE4E2")},
[Trial], [Result],
[1], [Failure],
[2], [Success]
)
|
|
https://github.com/taooceros/MATH-542-HW | https://raw.githubusercontent.com/taooceros/MATH-542-HW/main/HW5/HW5.typ | typst | #import "@local/homework-template:0.1.0": *
// Take a look at the file `template.typ` in the file panel
// to customize this template and discover how it works.
#show: project.with(
title: "Math 542 HW4",
authors: ("<NAME>",),
)
#let Wedge = $Lambda$
#let wedge = $and$
= Adjugates and Laplace
==
#let proj = $op("proj")$
#solution[
We know that $det(A)$ is defined by the coefficient of $A e_1 wedge ... A e_n = v_1 wedge ... wedge v_n = det(A) e_1 wedge ... wedge e_n$.
Then we consider $A_(i j)$ by seeing that it is the coefficient of $e_1 wedge ... wedge e_n$ after we replace $v_1 wedge ... v_i wedge ... wedge v_n$ with $v_1 wedge ... wedge e_j wedge ... wedge v_n$.
Consider a matrix $B_(i j)$ which is the matrix after removing the $i$-th row and $j$-th column of $A$.
Now we consider the exterior basis removing $e_i$.
$
B_(i j) e_1 wedge ... wedge B_(i j) e_(j-1) wedge B_(i j) e_(j+1) wedge ... wedge e_n = A_(i j) e_1 wedge ... wedge e_(j-1) wedge e_(j+1) wedge ... wedge e_n \
= w_1 wedge ... wedge w_(j-1) wedge w_(j+1) wedge ... wedge w_n
$
where $w_i = v_i$ projecting to the subspace orthogonal to $e_i$.
Note that
$
e_j wedge (w_1 wedge ... wedge w_(j-1) wedge w_(j+1) wedge ... wedge w_n) = e_j wedge ((v_1 - proj_(e_i) v_i) wedge ...) \
$
Then we can see the projection terms just become $0$ after wedging with $e_j$.
$
e_j wedge (w_1 wedge ... wedge w_(j-1) wedge w_(j+1) wedge ... wedge w_n) =
e_j wedge v_1 wedge ... wedge v_(j-1) wedge v_(j+1) wedge ... wedge v_n
$
which is what we want to show.
]
==
#solution[
This can be done easily by seeing that $v_i = sum_(j) a_(i j) e_j$, then by lienarity of exterior product the result follows.
]
==
#let adj = $op("adj")$
#solution[
Consider the first row times first column of $A adj(A)$. We can see that it is $sum (-1)^(1+j) a_(1 j) A_(1 j) = det(A)$ from part (2).
Consider the multiplication at row 2 and column 1.
The result is
$
sum_j (-1)^(2+j) a_(2 j) A_(1 j)
$
This is 0 because with (1) we have showed that $A_(i j)$ is the coefficient of $e_1 wedge ... wedge e_n$, but when adding the linear combination from another row, we essentially recover one of the vector. Therefore the wedge product become $0$.
Therefore $A adj(A) = det(A) I$. The reason for the commutativity is due to that we only have non-zero scalar multiplication and addition in the diagonal, which is commutative.
]
= Cayley-Hamilton
#solution[
Consider how $B$ looks like
$
B = mat(A - a_(11), -a_(12), ..., -a(1n);
-a_(21), A-a_(22), ..., -a(2n);
dots.v, dots.down;
-a_(n_1), -a_(n_2), ..., A-a_(n_n))
$
We can see that $sum^n_(j=1) b_(i j) e_j = A e_i - sum_(j=1)^n a_(1n) e_j = 0$.
Consider the adjugate matrix $C$ of $B$.
We know that $C B = det(B) I$, which means $forall k : sum_(i=1)^n c_(k i) b_(i j) = delta_(k j) det(B)$.
Consider
$
sum_j sum_j c_(k i) b_(i j) e_j = sum_j delta_(k j) det(B) e_j = sum_i c_(k i) 0 = 0
$
Thus $det(B) = 0$.
We can see that a matrix defined by entries $i$ and $j$ with $delta_(i j) x - a_(i j)$ will be sent to $B$ in $R'$.
]
= Free Module
#solution[
It suffices to consider $R^(m+1) -> R^m$. Assume $phi: R^(m+1) -> R^m$ is the injection, and let $psi: R^m -> R^(m+1)$ be the projection.
Consider $A = psi compose phi$.
Consider the minimal degree nonzero polynomial such that $p(A) = 0$. Since $A$ is injective, we have the $n$-th degree non-zero when applying $A$ as $x$. Thus, by Cayley-Hamilton we have the constant term non-zero.
However, consider applying $p(A)$ to $(0,...,0,1) in R^m+1$, the result will not be $0$ because the final term is always $0$, while the constant is non-zero, which means the result is non-zero, which is a contradiction.
]
= Commutator subgroups of matrix group
==
#let SL = "SL"
#let GL = "GL"
#solution[
This is a direct result of smith normal form.
]
==
#solution[
Because all elementary row/column operations matrix can be written as a $E_(i j)(a)$. Since every matrix in $SL(n,k)$ can be written as identity times some $E_(i j)(a)$, we can conclude that $SL(n,k)$ is product of $E_(i j )(a)$.
]
==
#solution[
Consider the commutator of $mat(a,0;0,a^(-1))$ and $mat(1,b;0,1)$.
$
mat(a,0;0,a^(-1))mat(1,b;0,1)mat(a^(-1),0;0,a)mat(1,-b;0,1) = mat(a, a b; 0, a^(-1))mat(a^(-1), -a^(-1)b; 0, a) = mat(1, -b +a^2 b; 0, 1)
$
Then for any elementary matrix we just need to have $-b + a^2 b = a'$ so $E_(i j)(a') = A B A^(-1) B^(-1)$ for some $A, B$.
]
==
This can be done by induction on $n$ with the previous question.
= Determinats of exterior and tensor powers
|
|
https://github.com/mangkoran/utm-thesis-typst | https://raw.githubusercontent.com/mangkoran/utm-thesis-typst/main/01_cover.typ | typst | MIT License | #import "utils.typ": empty
#let content(
title: empty[title],
author: empty[author],
) = [
#set align(center)
#set page(margin: (x: 3.25cm, y: 5cm))
#upper[#title]
#v(1fr)
#upper[#author]
#v(1fr)
#upper[Universiti Teknologi Malaysia]
#pagebreak(weak: true)
]
#content()
|
https://github.com/yingziyu-llt/blog | https://raw.githubusercontent.com/yingziyu-llt/blog/main/typst_document/linear_algebra/content/chapter4.typ | typst | #import "../template.typ":*
#let ker = $"Ker"$
#let im = $"Im"$
= 本征值,本征向量,不变子空间
我们已经建立了一些工具来描述一个算子的结构,我们下面来学习描述一个算子的其他角度。
== 不变子空间
先假设$V$有一种直和分解$V = U_1 plus.circle U_2 plus.circle dots plus.circle U_n$,如果我们认真的去构造$U_1,U_2,dots,U_n$,
那么我们有可能可以构造出某种分解$forall u in U_i,T(u) = v in U_i$.$U_i$是一个很有趣的子空间。下面来定义这个子空间。
#definition("不变子空间(invariant subspace)")[
$T in L(V)$,$V$的一个子空间$U$满足$forall u in U$,$T(u) in U$,那么我们称$U$是关于$T$的一个不变子空间(invariant subspace)。
]
#example("不变子空间")[
+ ${0}$ -> 是一个不变子空间,$T(0) = 0$
+ $V$ -> 是一个不变子空间
+ $ker T$ -> 是一个不变子空间
+ $im T$ -> 是一个不变子空间,$T(u) in im T$
]
=== 特征值和特征向量
我们先来讨论一维的不变子空间
对于某一维的子空间$U = {mu v: mu in FF}$,若它是一个不变子空间,那么一定有$T u in U$,又由于它是一维的,于是有$T u = lambda u$。
一个向量做变换后其方向没有改变,长度以某个倍率增加,是一个有趣的性质。我们对其加以定义
#definition("特征值(eigenvalue)")[
若$T in L(V)$,$T(v) = lambda v (v != 0)$,那么称$lambda$是$T$的一个特征值(eigenvalue)
]
#theorem("特征值的判定定理")[
若$T in L(V)$,$V$是有限维向量空间,$lambda in FF$,下面四个条件等价:
+ $lambda$是$T$特征值
+ $T - lambda I$不是单射
+ $T - lambda I$不是满射
+ $T - lambda I$不可逆
]
#definition("特征向量(eigenvector)")[
若$T in L(V)$,$T(v) = lambda v (v != 0)$,那么称$v$是$T$的一个特征向量(eigenvector)
]
#theorem("特征值的线性独立性")[
从属于不同特征值的特征向量是线性独立的
]
#quote("证明")[
设$v_1,v_2,dots,v_n$是$T$的特征向量,且其特征值都不相等,先假设这些向量线性相关。取一个最小的$k$,使得$v_k in "span"(v_1,v_2,dots,v_(k-1))$
于是有$v_k = a_1v_1 + a_2v_2 + dots + a_n v_n$ (1).
用$T$作用于左右两边,有$lambda_k v_k = lambda_1 a_1 v_1 + lambda_2 a_2 v_2 + dots + lambda_(k-1o) a_(k-1) v_(k-1)$ (2)
用(2)减去(1)左右两边乘$lambda_k$,得:$a_1(lambda_1 - lambda_k) v_1 + a_2(lambda_2 - lambda_k)v_2 + dots + a_(k-1) (lambda_(k-1) - lambda_k) v_(k-1) = 0$,于是$a_1 = a_2 = dots = a_(k - 1) = 0$。
$v_k = 0$,与特征向量的定义矛盾。故证。
]
|
|
https://github.com/gekoke/resume | https://raw.githubusercontent.com/gekoke/resume/main/lib.typ | typst | #let resume(author: "", contacts: (), body) = {
set document(author: author, title: author)
set page(margin: (
top: 1em,
left: 3em,
right: 3em,
bottom: 1em,
))
set text(font: "Linux Libertine", lang: "en")
show link: underline
show heading: heading => [
#pad(bottom: -16pt, [#smallcaps(heading.body)])
#line(length: 100%, stroke: 1pt)
]
// Author
align(center)[
#block(text(weight: 700, 1.5em, author))
]
// Contact information.
pad(
bottom: 100%,
align(center)[
#grid(
columns: 4,
gutter: 1em,
..contacts
)
],
)
// Main body.
set par(justify: true)
body
}
#let icon(name, baseline: 1.5pt, height: 10pt) = {
box(
baseline: baseline,
height: height,
image(name),
)
}
#let experience(organization, title, location, time, details) = {
pad(
grid(
columns: (auto, 1fr),
align(left)[
*#organization* \
#title
],
align(right)[
#location \
#time
],
),
)
details
}
#let project(name, details) = {
pad(
bottom: 100%,
grid(
columns: (auto, 1fr),
align(left)[
*#name*
],
),
)
details
}
#let volunteering(organization, role, time, details) = {
grid(
columns: (auto, 1fr),
align(left)[
*#organization* \
#role
],
align(right)[
#time
],
)
details
};
#let language(name, level) = {
pad(
bottom: 100%,
grid(
columns: (auto, 1fr),
align(left)[
*#name*
],
align(right)[
#level
],
),
)
}
|
|
https://github.com/FlandiaYingman/note-me | https://raw.githubusercontent.com/FlandiaYingman/note-me/main/README.md | markdown | MIT License | # GitHub Admonition for Typst
> [!NOTE]
> Add GitHub style admonitions (also known as alerts) to Typst.
## Usage
Import this package, and do
```typ
// Import from @preview namespace is suggested
// #import "@preview/note-me:0.3.0": *
// Import from @local namespace is only for debugging purpose
// #import "@local/note-me:0.3.0": *
// Import relatively is for development purpose
#import "lib.typ": *
= Basic Examples
#note[
Highlights information that users should take into account, even when skimming.
]
#tip[
Optional information to help a user be more successful.
]
#important[
Crucial information necessary for users to succeed.
]
#warning[
Critical content demanding immediate user attention due to potential risks.
]
#caution[
Negative potential consequences of an action.
]
#admonition(
icon-path: "icons/stop.svg",
color: color.fuchsia,
title: "Customize",
foreground-color: color.white,
background-color: color.black,
)[
The icon, (theme) color, title, foreground and background color are customizable.
]
#admonition(
icon-string: read("icons/light-bulb.svg"),
color: color.fuchsia,
title: "Customize",
)[
The icon can be specified as a string of SVG. This is useful if the user want to use an SVG icon that is not available in this package.
]
#admonition(
icon: [🙈],
color: color.fuchsia,
title: "Customize",
)[
Or, pass a content directly as the icon...
]
= More Examples
#todo[
Fix `note-me` package.
]
= Prevent Page Breaks from Breaking Admonitions
#box(
width: 1fr,
height: 50pt,
fill: gray,
)
#note[
#lorem(100)
]
```

Further Reading:
- https://github.com/orgs/community/discussions/16925
- https://docs.asciidoctor.org/asciidoc/latest/blocks/admonitions/
## Style
It borrows the style of GitHub's admonition.
> [!NOTE]
> Highlights information that users should take into account, even when skimming.
> [!TIP]
> Optional information to help a user be more successful.
> [!IMPORTANT]
> Crucial information necessary for users to succeed.
> [!WARNING]
> Critical content demanding immediate user attention due to potential risks.
> [!CAUTION]
> Negative potential consequences of an action.
## Credits
The admonition icons are from [Octicons](https://github.com/primer/octicons). |
https://github.com/Stautaffly/typ | https://raw.githubusercontent.com/Stautaffly/typ/main/note/note-model.typ | typst | #import "@preview/lemmify:0.1.5": *
#import "@preview/lemmify:0.1.5": *
#let (
proposition,
rules: thm-rules
) = default-theorems("thm-group", lang: "en")
/*分别计数*/
#let ( //定理,引理,推论
theorem, lemma,corollary,
rules: thm-rules-a
) = default-theorems("thm-group-a",lang:"en",thm-styling:thm-style-simple)
#let ( //证明
proof,
rules: thm-rules-p
) = default-theorems("thm-group-p",thm-styling:thm-style-proof)
#let (
example,
rules: thm-rules-example
) = default-theorems("thm-group-example",thm-styling:thm-style-simple)
//初始化remark
#let (remark,rules:thm-rules-remark
)=default-theorems("thm-group-remark",thm-styling:thm-style-simple)
#let (remark,rules:thm-rules-remark
)=default-theorems("thm-group-remark",thm-styling:thm-style-simple)
//定义
#let dinyi(thm-type,name,number,body) = box(
{[*_Def_* #number
]
body
},
fill: rgb("#E6E6FA"),
inset: 1em
)
#let dy-styling =(thm-styling:dinyi)
#let (dy,rules:thm-rules-def) = new-theorems("thm-group-def",("dy":"def"),..dy-styling)
#let project(course: "", title: "", authors: (), date: none, body) = {
// 文档基本信息
set document(author: authors.map(a => a.name), title: title)
set page(
paper: "a4",
margin: (left: 12mm, right: 12mm, top: 20mm, bottom: 20mm),
numbering: "1",
number-align: center,
)
// 页眉
set page(
header: {
set text(font:"DejaVu Math TeX Gyre", 10pt, baseline: 8pt, spacing: 3pt)
grid(
columns: (1fr, 1fr, 1fr),
align(left, course),
align(center, title),
align(right, date),
)
line(length: 100%, stroke: 0.5pt)
}
)
// 页脚
set page(
footer: {
set text(font: "DejaVu Math TeX Gyre", 10pt, baseline: 8pt, spacing: 3pt)
set align(center)
grid(
columns: (1fr, 1fr),
align(left, authors.map(a => a.name).join(", ")),
align(right, counter(page).display("1")),
)
}
)
set text(font: "DejaVu Math TeX Gyre", lang: "zh", size: 12pt)
show math.equation: set text(weight: 400)
// Set paragraph spacing.
show par: set block(above: 1.2em, below: 1.2em)
set heading(numbering: "1.1")
set par(leading: 0.75em)
// Title row.
align(center)[
#block(text(weight: 700, 1.5em, [#title]))
#v(1.1em, weak: true)
]
// Author information.
pad(
top: 0.8em,
bottom: 0.8em,
x: 2em,
grid(
columns: (1fr,) * calc.min(3, authors.len()),
gutter: 1em,
..authors.map(author => align(center)[
*#author.name* \
#author.email \
#author.phone
]),
),
)
// Main body.
set par(justify: true)
set par(first-line-indent: 2em)
show: thm-rules-a
show: thm-rules-p
show: thm-rules-example
show: thm-rules-remark
show: thm-rules
show: thm-rules-def
body
} |
|
https://github.com/Az-21/typst-components | https://raw.githubusercontent.com/Az-21/typst-components/main/README.md | markdown | Creative Commons Zero v1.0 Universal | # Typst Components
🐦 Reusable, pre-styled components for typst
## Inline Code
### [View source code ↗️](https://github.com/Az-21/typst-components/blob/main/components/code.typ)
```typst
#code(
"if(success) { return [>=, <=, ===, !=, ***]; }",
background: tailwind.violet-300, // Default: #rgb("#d2f0d4")
foreground: tailwind.neutral-900, // Default: #rgb("#000000")
)
```

## Boxed Link
### [View source code ↗️](https://github.com/Az-21/typst-components/blob/main/components/boxed_link.typ)
```typst
#boxed_link(
"https://github.com/Az-21/",
background: tailwind.emerald-900, // Default: #rgb("#d2e7f0")
foreground: tailwind.emerald-100, // Default: #rgb("#000000")
size: 12pt, // Default: 10pt
width: 50%, // Default: 100%
)
```
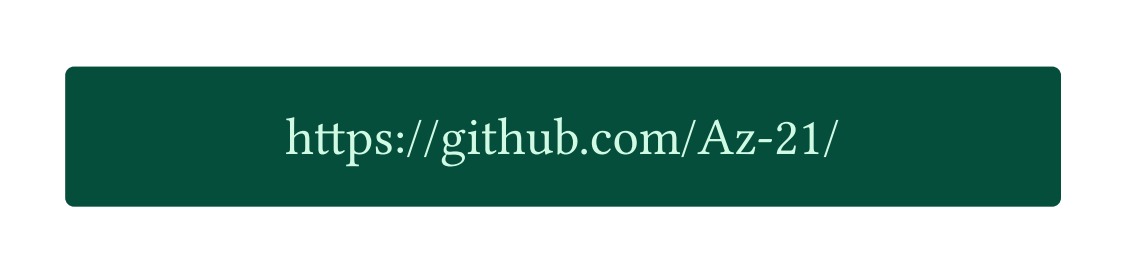
## Sync
Move changes from `git` repo to `@local` Typst folder, and vice versa.
```sh
# git -> @local
python ./sync.py --from-git-to-local
# @local -> git
python ./sync.py --from-local-to-git
```
## Other Typst Repos
### Typst Material You
Utility to parse material you theme for Typst
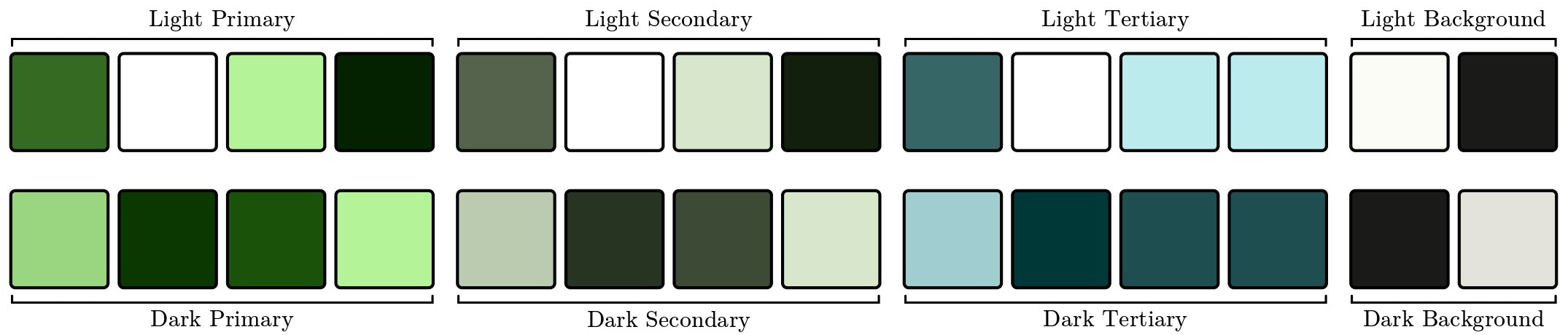
Get it on https://github.com/Az-21/material-you-adapter
|
https://github.com/RodolpheThienard/typst-template | https://raw.githubusercontent.com/RodolpheThienard/typst-template/main/beamer/beamer.typ | typst | MIT License | #let main_color = rgb("663399")
#let transition_color = rgb("663399")
#let backgroung_start = none
#let beamer_start( title:none, subtitle:none, author:none, date:none) = {
page(
margin: (top:5%, bottom: 5%, left: 5%, right:5%),
background:none, // image(backgroung_start),
header: none
)[
#align(center+horizon, text(25pt, weight: "bold", title))
#align(center+horizon, text(17pt, weight: "regular", author))
#align(right+bottom, text(15pt, date))
]
}
#let beamer_catalogs() = {
set page(
margin: (top:0%, bottom: 0%, left: 0%, right:10%),
)
set outline(
title: none,
depth: 2,
indent: 2em,
fill: none
)
show outline.entry.where(level:1): it => {
v(30pt, weak: true)
text(fill: main_color.lighten(10%), size: 20pt, weight: "bold",it)
}
show outline.entry.where(level:2):it => {
text(fill: main_color.lighten(10%),size: 15pt, weight: "regular",it)
}
grid(
columns:(35%, 70%),
column-gutter: 16%,
align(
center+horizon,
box(
fill: main_color,
width: 100%,
height: 100%,
text(fill: white, size: 40pt, hyphenate: true, weight: "bold", [Outline])
),
),
align(center+horizon, outline())
)
}
#let beamer_content(body) = {
set page(
margin: (top:20%, bottom: 10%, left: 5%, right:5%),
footer: [
#let lastpage-number = locate(pos => counter(page).final(pos).at(0))
#set align(right)
#text(size: 10pt)[
#counter(page).display("1")
\/ #lastpage-number
]
],
header: locate(loc => {
let title = query(heading.where(level:1).before(loc),loc).last().body
grid(
rows:(70%, 10%),
row-gutter: 0%,
grid(
columns:(50%, 50%),
align(left+horizon, text(fill: main_color, size: 25pt, weight: "bold", title)),
align(left+horizon, box(height: 100%, width:100%)),
),
align(center+bottom, line(length: 100%, stroke: (paint:main_color, thickness:1pt)))
)
})
)
show heading.where(level:1):it => {
set page(
margin: (top:5%, bottom: 10%, left: 5%, right:5%),
fill: transition_color,
header: none,
background: none
)
align(center+horizon, text(fill: white, size: 40pt, it))
}
show heading.where(level:2): it => {
locate( loc => {
let level_1_now_title = query(heading.where(level:1).before(loc),loc).last().body
let level_2_now_title = query(heading.where(level:2).before(loc),loc).last().body
let first_title = none
let get = false
for item in query(heading.where(level:1).or(heading.where(level:2)),loc) {
if get {
if item.level == 2 {
first_title = item.body
}
break
} else {
if item.level == 1 and item.body == level_1_now_title {
get = true
}
}
}
if first_title != level_2_now_title {
pagebreak()
}
})
align(top, text(size: 20pt, it))
v(1em)
}
body
}
#let beamer_end(end) = {
set page(fill: main_color)
set align(left+horizon)
text(40pt, weight: "bold", fill: white, end )
}
#let beamer( title:none, subtitle:none, author:none, date:none, end:none, body) = {
set page(
paper: "presentation-16-9",
)
set text(font: "Linux Libertine", size:18pt, weight: "regular")
beamer_start(title:title, author:author, date:date)
beamer_catalogs()
beamer_content(body)
beamer_end(end)
}
|
https://github.com/HiiGHoVuTi/requin | https://raw.githubusercontent.com/HiiGHoVuTi/requin/main/graph/konig.typ | typst | #import "../lib.typ": *
#show heading: heading_fct
Un arbre est dit d'_arborescence finie_ si tous ses noeuds sont de degré fini.
=== <NAME>
_Tout arbre infini d'arborescence finie admet un chemin simple infini commençant à la racine._
#question(2)[Démontrer ce résultat. _Justifier soigneusement_.]
#correct[
On raisonne par _coinduction_, c'est-à-dire que l'on va construire ce chemin en démontrant en même temps une propriété à son propos.
On pose $cal(H)(n)$ la propriété: le noeud $gamma_n$ a une infinité de descendants. Construisons cette suite ($gamma_n$).
_Initialisation_: Comme l'arbre est infini, la racine a une infinité de descendants, on pose $gamma_0$ la racine.
_Hérédité_: On suppose qu'il existe $n in NN$ tel que $gamma_n$ est défini et $cal(H)(n)$ est vrai.
Si $v_1...v_k$ sont les enfants de $gamma_n$ ($deg(gamma_n)< +oo$), et qu'on note $cal(D)(v_i)$ l'ensemble des descendants de $v_i$,
$ "Card"(cal(D)(gamma_n)) = "Card"( union.big_(i = 1)^k {v_i} union cal(D)(v_i) ) = +oo $
Donc il existe $i in [|1, k|]$ tel que $cal(D)(v_i)$ est infini. On pose $gamma_(n+1):=v_i$ et on vérifie $cal(H)(n+1)$.
_Conclusion_: La suite $(gamma_n)$ est un tel chemin #align(right, $square$)
]
=== Coloration d'un graphe
Soit $k in NN$ puis $G=(NN,A)$ un graphe tel que pour $S subset NN$ fini, $G[S]$ est $k$-coloriable.
On note $cal(C)(S)$ l'ensemble des $k$-colorations possibles de $G[S]$.
#question(0)[ Donner une suite $(S_n)$ croissante de parties finies telle que $S_n -> NN$. ]
#correct[
On pose $S_n := [|1, n|]$. Cette suite est croissante donc converge vers une limite $S := union.big S_n = NN$.
]
On dira qu'une coloration de $S$ est _compatible_ avec une coloration de $S' supset S$ si toutes les couleurs assignées aux éléments de $S$ sont identiques.
#question(0)[ Montrer que $|cal(C)(S_n)|$ est minorée et que pour $c in cal(C)(S_n)$, l'ensemble des $k$-colorations de $G[S_(n+1)]$ compatibles avec $c$ est fini. ]
#correct[
- $G[S_n]$ est coloriable donc $|cal(C)(S_n)|>=1$.
- L'ensemble des colorations de $G[S_(n+1)]$ est inclus dans $[|1, k|]^(S_(n+1))$ donc est fini.
]
#question(2)[ En déduire que $G$ est $k$-coloriable. ]
#correct[
On définit un arbre $A$
- La racine de $A$ est la $k$-coloration vide.
- Les enfants d'un noeud de $A$ est l'ensemble des $k$-colorations compatibles avec leur parent.
D'après la question précédente, l'arbre est d'arborescence finie et chaque niveau de l'arbre est habité.
Les hypothèses du lemme de König s'appliquent, on a un chemin composé uniquement de colorations compatibles de plus en plus grandes, on peut en déduire la coloration de n'importe quel sommet.
]
=== Arbres et boules
On considère un jeu nommé \"Arbres et boules\"
- Initialement, une boule labellée $K_0 in NN$ est placée dans un sac.
- À chaque tour, on pioche une boule dans le sac puis:
- On note son label $k in NN$.
- On place autant de boules labellées avec des entiers de $[|0, k-1|]$ que l'on souhaite dans le sac.
- Le jeu s'arrête si on pioche une boule labellée $0$.
#question(1)[Montrer qu'une partie d'arbres et boules est finie.]
#correct[
On définit un arbre de jeu
- Sa racine est la boule $K_0$
- Les enfants d'un de ses noeuds est l'ensemble des boules placées dans le sac après le tirage du parent
Cet arbre est d'arborescence finie, et ne possède aucune branche infinie (la valeur des boules décroît strictement dans $NN$ entre un parent et son enfant).
Ainsi, l'arbre ne saurait pas être infini sans entrer en contradiction avec le lemme de König.
On en conclut que le nombre total de boules en jeu dans la partie est fini, donc que la partie est finie.
]
=== Compacité de la logique propositionnelle
Soit $F$ un ensemble dénombrable de formules. $F$ est _satisfiable_ si il existe une valuation satisfaisant toutes ses formules. $F$ est _finement_ satisfiable si toutes ses parties finies sont satisfiables.
#question(2)[ Montrer que $F$ est satisfiable si et seulement elle est finement satisfiable. ]
#correct[
Le raisonnement est exactement le même que pour la coloration de graphes.
]
=== Tuiles de Wang
Soit $Sigma$ un alphabet fini. Une _tuile carrée_ est un quadruplet $(h,b,g,d) in Sigma^4$. Un _jeu de tuiles_ $J$ est une partie de $Sigma^4$.
Un _pavage_ avec $J$ de $T subset ZZ^2$ convexe est une application $cal(p) : T -> J$ telle que
#align(center, grid(columns: (1fr),
[- $forall ((i, j),(i+1, j)) in T^2, cal(p)(i,j)_d = cal(p)(i+1, j)_g$\ \ ],
[- $forall ((i, j),(i, j+1)) in T^2, cal(p)(i,j)_h = cal(p)(i, j+1)_b$]
))
On suppose que $|J| < +oo$.
#question(2)[ Montrer que si on peut paver $[|1, n|]^2$ pour tout $n$, alors on peut paver $ZZ^2$. ]
#correct[
C'est encore une application de la construction "arbre de compatibilité".
]
#question(1)[ Montrer que ce résultat n'est pas forcément vrai si $|cal(p)(ZZ^2)| = +oo$. ]
#correct[
On se munit du jeu de tuile malveillant tel que $J = {(suit.spade, suit.spade, n, n+1), n in NN}$.
Il est clair que l'on peut paver un rectangle de n'importe quelle taille, mais jamais paver $ZZ$ à cause de la tuile $(suit.spade, suit.spade, 0, 1)$ qui ne peut pas avoir de voisin à gauche.
]
#question(1)[ Montrer que si $J$ pave $ZZ^2$ et que $|J|$ est minimal (on ne peut retirer aucune tuile sans perdre la possibilité de paver $ZZ^2$), alors il existe $n in NN$ tel que toute tuile apparaisse dans tout carré de dimension $n times n$. ]
#correct[
On raisonne par contraposée.
Si pour tout $n in NN$, il existe une tuile qui n'apparaît pas dans tous les carrés de taille $n times n$, alors il existe une tuile $t$ qui est absente d'une infinité de telles tailles de carré ($|J| < +oo$).
C'est-à-dire qu'il existe $(u_n)$ une suite croissante dans $NN$ telle qu'on peut paver tout carré de taille $u_n times u_n$ sans utiliser $t$.
On peut réinvoquer König et obtenir un pavage n'utilisant pas $t$.
]
// Un pavage $cal(p)$ est _périodique_ si $ exists (x,y) in NN^2, forall (i,j) in ZZ^2, cal(p)(i,j)=cal(p)(i+x,j)=cal(p)(i, j+y)=cal(p)(i+x,j+y) $
Un pavage $cal(p)$ est _presque périodique_ si tout motif carré s'y répète infiniment souvent.
On considère un jeu de tuiles fini $S$ pavant $ZZ^2$. Une tuile est donc un élément de $S^4$.
Soit $cal(p)_0$ un pavage de $ZZ^2$ et $M_n$ les ensembles de motifs de taille $n times n$ infiniment présents dans $cal(p)_0$.
#question(0)[Montrer que tous les $M_n$ sont non-vides.]
#correct[
Par le principe des tiroirs, comme il existe un nombre fini de motifs $n times n$ et que la réunion de leurs occurrences est infinie, l'un des motifs apparaît une infinité de fois, donc $M_n$ n'est pas vide.
]
#question(1)[Montrer que tout sous-motif des $M_n$ est dans un $M_p$ avec $p<=n$.]
#correct[
Si $m$ est un sous-motif d'un élément $M$ de $M_n$, il apparaît à chaque occurrence de $M$, donc une infinité de fois. Ainsi, si $p$ est la taille de $m$, $m in M_p$.
]
#question(2)[En déduire l'existence d'un pavage $cal(p)_1$ dans lequel tous les motifs carrés sont dans $union.big_(p in NN) M_p$.]
#correct[
Une $n$-ième application de König.
]
On poursuit cette construction pour construire une suite $(cal(p)_n)$ de pavages.
#question(4)[Montrer que la suite $(cal(p)_n)$ est stationnaire.]
#question(4)[Montrer que la limite $cal(p)_oo$ de cette suite est presque périodique.]
=== Axiome du choix
_On néglige ici l'aspect dépendant de l'axiome du choix dépendant._
L'axiome du choix *AC*($kappa$) est la proposition suivante: Si $E$ est un ensemble de cardinal $|E|<= kappa$ d'ensemble non-vides, alors il existe $f : E -> union.big E$ une fonction qui à chaque élément $e$ de $E$ associe un de ses éléments.
#question(1)[Justifier que la preuve du lemme de König utilise $bold("AC")(|NN|)$.]
#correct[
À chaque étape il faut choisir un enfant $v_i$ parmi l'ensemble non-vide des enfants ayant une infinité de descendants.
On fait une infinité de tels choix, dépendant les uns des autres.
]
Ainsi *AC($|NN|$)* $==>$ *König*. Démontrons le sens réciproque.
Soit $E = {X_n}$ un ensemble dénombrable d'ensembles finis non-vides. On pose $ S = union.big_(n=0)^(+oo)product_(k=0)^n X_k $
#question(0)[ Décrire induitivement cette construction. ]
#correct[
$S$ est l'ensemble des suites à support fini telles que le premier élément est dans $X_0$, le deuxième dans $X_1$, ...
]
#question(2)[ Conclure sur l'implication réciproque. ]
#correct[
On reconstruit un arbre de compatibilité avec la suite vide comme racine, et $(x_1...x_(n+1))$ est un enfant de $(x_1...x_n)$ peu importe $x_(n+1)$.
D'après le lemme de König on a une branche infinie, soit une suite $(x_i in X_i)_(n in NN)$, c'est ce que l'on cherchait.
]
=== Arbres d'Aronszajn
_Mais quid des arbres non-dénombrables ? à faire si vous n'avez pas peur des ordinaux._
On considère désormais les arbres comme des ensembles partiellement ordonnés d'ordinaux.
Si $kappa$ est un cardinal, un _$kappa$-arbre d'Aronszajn_ est un arbre de cardinal $kappa$ dont toutes les branches sont de hauteur inférieure à $kappa$ et dont tous les niveaux sont de cardinal inférieur à $kappa$.
#question(0)[Montrer qu'il n'existe pas d'$alef_0$-arbre d'Aronszajn.]
#correct[ C'est une application simple du lemme de König, comme dans la partie III. ]
// TODO(Juliette): ajouter qq indications... c'est impossible là c'est un sujet de thèse
#question(5)[Démontrer qu'il existe un $alef_1$-arbre d'Aronszajn.]
_L'existence d'un $alef_2$-arbre d'Aronszajn est indécidable dans ZFC._
|
|
https://github.com/julius2718/entempura | https://raw.githubusercontent.com/julius2718/entempura/main/0.0.1/test.typ | typst | MIT License | #import "@local/entempura:0.0.1": *
#show: doc => endoc(doc)
#signature(
title: "Typst English Template",
date: today,
author: "julius2718",
)
= Typst English Template
Lorem ipsum dolor sit amet, officia excepteur ex fugiat reprehenderit enim labore culpa sint ad nisi Lorem pariatur mollit ex esse exercitation amet. Nisi anim cupidatat excepteur officia. Reprehenderit nostrud nostrud ipsum Lorem est aliquip amet voluptate voluptate dolor minim nulla est proident. Nostrud officia pariatur ut officia. Sit irure elit esse ea nulla sunt ex occaecat reprehenderit commodo officia dolor Lorem duis laboris cupidatat officia voluptate. Culpa proident adipisicing id nulla nisi laboris ex in Lorem sunt duis officia eiusmod. Aliqua reprehenderit commodo ex non excepteur duis sunt velit enim. Voluptate laboris sint cupidatat ullamco ut ea consectetur et est culpa et culpa duis.
= Using Sans Font
#sans[Lorem ipsum dolor sit amet, officia excepteur ex fugiat reprehenderit enim labore culpa sint ad nisi Lorem pariatur mollit ex esse exercitation amet. Nisi anim cupidatat excepteur officia. Reprehenderit nostrud nostrud ipsum Lorem est aliquip amet voluptate voluptate dolor minim nulla est proident. Nostrud officia pariatur ut officia. Sit irure elit esse ea nulla sunt ex occaecat reprehenderit commodo officia dolor Lorem duis laboris cupidatat officia voluptate. Culpa proident adipisicing id nulla nisi laboris ex in Lorem sunt duis officia eiusmod. Aliqua reprehenderit commodo ex non excepteur duis sunt velit enim. Voluptate laboris sint cupidatat ullamco ut ea consectetur et est culpa et culpa duis.]
== Code
```py
print("hello, world")
```
Balance between normal font and `raw text font`.
= Math: Quadrature by parts
$ lim_(n -> infinity) 1/n sum_(k = 0)^(n - 1) f(a + (b - a)/n k) = integral_a^b f(x) d x $
|
https://github.com/thornoar/hkust-courses | https://raw.githubusercontent.com/thornoar/hkust-courses/master/MATH1023-Honors-Calculus-I/homeworks/homework-2/main.typ | typst | #import "@local/common:0.0.0": *
#import "@local/templates:0.0.0": *
#show: math-preamble("Part 2", "Fri, Sep 20")
#math-problem("1.1.31 (11)") Find the limit ($n -> oo$) of $(a n^2 + b)^(c/(n+d))$, where $a > 0$.\
#math-solution In the following inequalities the $<=$ sign will mean "less than or equal to, for sufficiantly large $n$". We will also assume for now that $c >= 0$. Trivially, we have
$
1 <= (a n^2 + b)^(c/(n+d)) <= ((a+1) n^2)^(c/(n + d)) <= ((a+1) n^2)^((2c)/n) = (a+1)^((2c)/n) dot (root(n, n))^(4c) ->_(n -> oo) 1,
$
since $limits(lim)_(n -> oo) root(n,n) = 1$, and thus the limit in question is equal to 1. When $c < 0$, the similar result follows from the arithmetic rule by considering $b_n = 1 \/ a_n$, where $a_n$ is the original sequence.
#math-problem("1.1.32 (5)") Find the limit ($n -> oo$) of $(n - cos(n))^(1/(n + sin(n)))$, where $a > 0$.\
#math-solution
$
1 <= (n - cos(n))^(1/(n + sin(n))) <= (2n)^(2/n) = 2^(2/n) dot (root(n,n))^2 ->_(n -> oo) 1,
$
thus the original limit is 1.
#math-problem("1.1.34 (6)") Find the limit ($n -> oo$) of $root(n, 4^(2n-1) + (-1)^n 5^n)$.\
#math-solution We will rewrite $4^(2n - 1)$ as $1/4 16^n$. It is obvious that starting at some $n$, we have
$
1/8 16^n <= (-1)^n 5^n <= 1/8 16^n.
$
Consequently, we can write
$
16 <-_(n -> oo) 16 dot root(n, 1/8) = root(n, 1/8 16^n) <= root(n, 1/4 16^n + (-1)^n 5^n) <= root(n, 3/8 16^n) = 16 dot root(n, 3/8) ->_(n -> oo) 16,
$
which means that 16 is the answer by the sandwich rule.
#math-problem("1.1.35 (8)") Find the limit ($n -> oo$) of $(a^n - b^n)^(n/(n^2-1))$, where $a > b > 0$.\
#math-solution We will first establish that $a_n := (a^n - b^n)^(1/n) -> a$. Indeed,
$
a >= (a^n - b^n)^(1/n) = a dot (1 - (b/a)^n)^(1/n) >= a dot (1 - (b/a))^(1/n) ->_(n -> oo) a,
$
which leads to $a_n -> a$ by the sandwith rule. Now, since $n/(n^2-1) > 1/n$ and $a^n - b^n > 1$ for sufficiently large $n$, we write
$
a <-_(n -> oo) a dot a^(1/(n^2-1)) = a^(n^2/(n^2-1)) > (a^n - b^n)^(n/(n^2-1)) > (a^n - b^n)^(1/n) ->_(n -> oo) a,
$
and conclude that the limit in question is $a$ by the sandwich rule.
#math-problem("1.1.38") Show that $limits(lim)_(n -> oo) n^2 a^n = 0$ for $abs(a) < 1$ in two ways (Example 1.1.12, and the fact that $limits(lim)_(n -> oo) n a^n = 0$ for $abs(a) < 1$).\
#math-solution
/ (first method): We represent $abs(a)$ as $1/(1+b)$ where $b > 0$ (since $abs(a) < 1$). Now,
$
abs(n^2 a^n) = n^2/(1+b)^n = n^2/(1 + n b + (n(n-1))/2 b^2 + (n(n-1)(n-2))/6 b^3 + ...) <= (6n^2)/(n(n-1)(n-2)b^3) = 6/b^3 dot n/(n-1) dot 1/(n-2).
$
By recognizing that $n/(n-1) -> 1$ and $1/(n-2) -> 0$, we see that $abs(n^2 a^n)$ tends to 0, meaning that $n^2 a^n$ tends to 0 as well, by Exercise 1.1.13.
/ (second method): We have that $limits(lim)_(n -> oo) n a^n = 0$ for $abs(a) < 1$. Considering that $root(n, n) -> 1$, we see that
$
abs(root(n, n a^n)) = abs(a) root(n,n) -> abs(a),
$
and thus, for a sufficiently small $epsilon > 0$ and sufficiently large $n$, we have
$
abs(root(n, n a^n)) < abs(a) + epsilon < 1.
$
Now, we return to the sequence in question and consider its absolute value:
$
abs(n^2 a^n) = abs(n dot (root(n, n a^n))^n) = n dot (abs(root(n, n a^n)))^n <= n dot (abs(a) + epsilon)^n ->_(n -> oo) 0,
$
since $abs(a) + epsilon < 1$.
#math-problem("1.1.41") Show that $limits(lim)_(n -> oo) (a^n)/sqrt(n!) = 0$ for $a = 4$.\
#math-solution For sufficiently large $n$, we write
$
0 <= 4^n/sqrt(n!) = sqrt(16^n/n!) = sqrt(16^16/16! dot 16/17 dot 16/18 dot ... dot 16/n) <= sqrt(16^16/16! dot 16/n) = A dot 1/sqrt(n) ->_(n -> oo) 0,
$
and the result follows from the sandwich rule.
#math-problem("1.1.43 (7)") Find the limit ($n -> oo$) of $(n 3^n) \/ (1 + 2^n)^2$.\
#math-solution First we will find the limit of $(n 3^n) \/ (2^n)^2$. It follows simply from Example 1.1.13 by the representation
$
(n 3^n)/(2^n)^2 = n dot (3/4)^n,
$
that the limit is equal to 0. Now, we consider the ratio
$
((n 3^n)/(2^n)^2)/((n 3^n)/(1 + 2^n)^2) = (1 + 1/2^n)^2 ->_(n -> oo) 1,
$
and thus the limit of $(n 3^n) \/ (1 + 2^n)^2$ is also 0, by the arithmetic rule of division.
#math-problem("1.1.45") Show that $limits(lim)_(n -> oo) (n^p a^n)/sqrt(n!) = 0$ for any $a$.\
#math-solution We pick $b$ such that $abs(b) > abs(a)$. Now, by Example 1.1.15, we have
$
(n^p a^n)/b^n = n^p dot (a/b)^n ->_(n -> oo) 0.
$
We also have $b^n/sqrt(n!) ->_(n -> oo) 0$ by Exercise 1.1.41. Now, multiplying the two sequences, we see that the limit in question is also 0.
#math-problem("1.1.51 (6)") Find the limit ($n -> oo$) of $root(n-2, n 2^(3n) + (3^(2n-1))/n^2)$.\
#math-solution For simplicity we will first take the $n$-th root instead of the $(n-2)$-th root. We have
$
root(n, n 2^(3n) + (3^(2n - 1))/n^2) =
root(n, 9^n/(3 n^2)) dot root(n, 1 + (3 n^3 8^n)/(9^n)) =
9 dot 1/root(n,3) dot (1/root(n,n))^2 dot
root(n, 1 + 3 n^3 (8/9)^n) ->_(n -> oo) 9 dot 1 dot 1 dot 1 = 9.
$
Now, we express $1/(n-2)$ as $1/n + 2/(n(n-2))$, and see that
$
root(n-2, n 2^(3n) + (3^(2n-1))/n^2) = (root(n, n 2^(3n) + (3^(2n-1))/n^2)) dot (root(n, n 2^(3n) + (3^(2n-1))/n^2))^(2/(n-2)) ->_(n -> oo) 9 dot 1 = 9,
$
due to the arithmetic rule $limits(lim)_(n -> oo) x_n y_n = (limits(lim)_(n -> oo) x_n) (limits(lim)_(n -> oo) y_n)$. In the above derivation, to conclude that the right part of the product approaches 1, we used the fact that if $x_n -> xi > 0$, then $x_n^(2/(n-2)) -> 1$. This is due to the sandwich rule: let $0 < alpha < xi < beta$. Starting from some point, we have $alpha < x_n < beta$. Now,
$
1 <-_(n -> oo) alpha^(2/(n-2)) < x_n^(2/(n-2)) < beta^(2/(n-2)) ->_(n -> oo) 1.
$
Now we can be sure that the answer is indeed 9.
#math-problem("1.1.52 (11)") Find the limit ($n -> oo$) of $((n+1)a^n + b^n)^(n/(n^2-1))$.\
#math-solution For simplicity and out of concern for the well-definition of real exponentiation, we will assume that $a > b > 0$. Consider the sequence $((n+1)a^n)^(n/(n^2-1))$. By considering its quotient with the original sequence and writing inequalities, we see that
$
1 <= (((n+1)a^n + b^n)^(n/(n^2-1)))/((n+1)a^n)^(n/(n^2-1)) = (1 + 1/(n+1) (b/a)^n)^(n/(n^2-1)) <= (1 + (b/a)^n)^(n/(n^2-1)) <= 1 + (b/a)^n -> 1,
$
and the quotient approaches 1 by the sandwich rule. By the arithmetic rule, this means that we may instead find the limit of $((n+1)a^n)^(n/(n^2-1))$. Indeed,
$
((n+1)a^n)^(n/(n^2-1)) = (root(n+1, n+1))^(n/(n-1)) dot a^(n^2/(n^2-1)) = root(n+1, n+1) dot (root(n+1, n+1))^(1/(n-1)) dot a dot a^(1/(n^2-1)) ->_(n -> oo) 1 dot 1 dot a dot 1 = a.
$
If $b < a <= 0$, then the given expression is not defined for odd $n$. If, now, $b <= 0 < a$ and $a < abs(b)$, then the expression is again not defined for odd $n$. If, however, $b <= 0 < a$, and $a >= abs(b)$, then the expression becomes well-defined since $(n+1)a^n > abs(b^n)$. In this case we bound the expression (for sufficiently large $n$) as follows:
$
((n+1)/2 a^n + abs(b)^n)^(n/(n^2-1)) <= ((n+1)a^n + b^n)^(n/(n^2-1)) <= ((n+1)a^n + abs(b)^n)^(n/(n^2-1)).
$
As already established, both the leftmost and the rightmost sequence approach $a$, and thus the original sequence also approaches $a$ by the sandwich rule.
#math-problem("1.1.58") Prove that if $limits(lim)_(n -> oo) abs(x_n/x_(n-1)) = l < 1$, then $x_n$ converges to 0.\
#math-solution By the order rule, for sufficiently large $n$ (say, from $N$) we have $ abs(x_n/x_(n-1)) < l + epsilon < 1, $ where $epsilon$ is a small positive number. Now, for $n > N$ we express $abs(x_n)$ as
$
abs(x_n) = abs(x_N) dot abs(x_(N+1)/x_N) dot abs(x_(N+2)/x_(N+1)) dot ... dot abs(x_n/x_(n-1)) < abs(x_N) dot (l + epsilon)^(n - N) ->_(n -> oo) 0,
$
since $l + epsilon < 1$. Therefore, $x_n$ converges to 0.
#math-problem("1.1.59 (7)") Find $a$ such that the sequence $x_n = (a^(n^2))/sqrt(n!)$ converges to 0.\
#math-solution Consider the d'Alambertian ratios:
$
abs(x_n/x_(n-1)) = abs(a^(2n - 1)/sqrt(n)).
$
These ratios approach 0 when $abs(a) <= 1$, and in that case the original sequence $x_n$ will approach 0 as well (by Exercise 1.1.58). If, on the other hand, $abs(a) > 1$, then the ratios diverge to infinity:
$
abs(sqrt(n)/(a^(2n - 1))) ->_(n -> oo) 0 ==> abs(a^(2n - 1)/sqrt(n)) ->_(n -> oo) oo.
$
In the above implication, the premise can be obtained from Example 1.1.13. Therefore, since the ratios diverge to infinity, the original sequence diverges as well. We demonstrate this by picking $M > 1$ and stating that $abs(x_n/x_(n-1)) > M$ for sufficiently large $n$ (say, $n > N$). Now, for $n > N$ we have
$
abs(x_n) = abs(x_N) dot abs(x_(N+1)/x_N) dot abs(x_(N+2)/x_(N+1)) dot ... dot abs(x_n/x_(n-1)) >= abs(x_N) dot M^(n - N) ->_(n -> oo) oo,
$
and thus $x_n -> oo$ by the order rule, meaning that $x_n$ cannot converge to a finite number.
#math-problem("1.1.61 (2, 6)") Explain the convergence/divergence of sequences.\
+ $x_n = n^((-1)^n/n)$\
#math-solution Consider the odd subsequence: //#h(1fr)
$
x_(2n-1) = (2n-1)^(-1/(2n-1)) = 1/root(2n-1, 2n-1) ->_(n -> oo) 1,
$
by Example 1.1.9. For the even subsequence, the situation is analogous: $x_(2n) ->_(n -> oo) 1$. As a result, the entire sequence also converges to 1, as a union of two subsequences converging to 1.
+ $x_n = sqrt(n) (sqrt(n + (-1)^n) - sqrt(n))$\
#math-solution First consider the even subsequence: $x_k = sqrt(k) (sqrt(k+1) - sqrt(k))$ with $k$ even. We multiply and divide by the conjugate:
$
sqrt(k) (sqrt(k+1) - sqrt(k)) = (sqrt(k) (sqrt(k+1) - sqrt(k)) (sqrt(k+1) + sqrt(k)))/(sqrt(k+1) + sqrt(k)) = sqrt(k)/(sqrt(k+1) + sqrt(k)) ->_(k -> oo) 1/2.
$
By analogy, we see that the odd subsequence, $x_k = sqrt(k) (sqrt(k-1) - sqrt(k))$ with $k$ odd, approaches $-1/2$. As a result, the main sequence diverges, since two of its subsequences converge to different values.
#math-problem("1.1.63") Extend Exercise 1.1.27, by dropping the requirement that all $x_n >= 1$: If $limits(lim)_(n -> oo) x_n = 1$ and $y_n$ is bounded, then $limits(lim)_(n -> oo) x_n^(y_n) = 1$.\
#math-solution Since $y_n$ is bounded, there are $p, q in RR$ such that $p <= y_n <= q$ for all $n$. Consider the subsequence $x_(k_n)$ of those elements that are not greater than 1. Then, we have
$
x_(k_n)^q <= x_(k_n)^(y_(k_n)) <= x_(k_n)^p.
$
Both the leftmost and the rightmost sequences converge to 1 by Example 1.1.23, and thus the middle sequence converges to 1 by the sandwich rule. Now, an analogous argument (with reversed inequalities) proves that the subsequence $x_(m_n)$ of all elements $>= 1$ also has $x_(m_n)^(y_(m_n))$ converge to 1. As a result, $x_n^(y_n)$ converges to 1 as a union of two subsequences converging to 1.
|
|
https://github.com/masaori/diffusion-model-quantum-annealing | https://raw.githubusercontent.com/masaori/diffusion-model-quantum-annealing/main/old_note/main.md | markdown | # ゴール
- 拡散モデルの数理を流し読みして、量子コンピューティング (量子アニーリング) と関係ありそうだと思った
- ので、これの数理的な関連をざっくりと理解したい
- 年内いっぱいくらいで「わかった」という気分になりたい
- なんでかというと、今から量子コンピューティングの勉強にリソース割いておくかどうかを見定めたい
## 関連しそうな項目
- 拡散モデル
- 確率過程
- シミュレーティッドアニーリング
- 量子アニーリング
- 代数的確率空間
- 量子力学においては、行列(=作用素)のtraceが期待値
- この辺を抽象化して、代数の元に「確率的」な情報が含まれているとみなしてゴニョゴニョするのが確率的代数空間 (イメージ)
- これを理解できると「拡散モデルのマルコフ過程は代数的確率空間的にはこう」みたいな話から「じゃあこんな種類の代数構造から考えたら拡散モデルに当たるものは何?」みたいな議論ができて面白いのでは、という仮説
# 課題感
- 大規模LLMは大手に独占されている
- GPT 4はパラメーター数が 170兆
- 圧倒的データ量
# 教科書
- **確率論**: [https://www.amazon.co.jp/gp/product/4254116004/ref=ox_sc_act_image_1?smid=AN1VRQENFRJN5&psc=1](https://www.amazon.co.jp/gp/product/4254116004/ref=ox_sc_act_image_1?smid=AN1VRQENFRJN5&psc=1)
- **確率微分方程式とその応用:** [https://www.amazon.co.jp/gp/product/4627077815/ref=ox_sc_act_image_2?smid=AN1VRQENFRJN5&psc=1](https://www.amazon.co.jp/gp/product/4627077815/ref=ox_sc_act_image_2?smid=AN1VRQENFRJN5&psc=1)
# 論文まとめ
## Deep Unsupervised Learning using Nonequilibrium Thermodynamics
非平衡熱力学を用いた教師なしディープラーニング
[Deep_Unsupervised_Learning_using_Nonequilibrium_Thermodynamics.pdf](https://prod-files-secure.s3.us-west-2.amazonaws.com/a95e1e6d-ab5d-426a-b5b6-652ced6dcd2a/673adbff-eaa6-4376-a336-ac3a1b60d50e/Deep_Unsupervised_Learning_using_Nonequilibrium_Thermodynamics.pdf?X-Amz-Algorithm=AWS4-HMAC-SHA256&X-Amz-Content-Sha256=UNSIGNED-PAYLOAD&X-Amz-Credential=<KEY>%2F20240118%2Fus-west-2%2Fs3%2Faws4_request&X-Amz-Date=20240118T043703Z&X-Amz-Expires=3600&X-Amz-Signature=b7f4072570d2ec339eb1e103d5ac017a08fafde6ae0bcb8a10f032deeb173a62&X-Amz-SignedHeaders=host&x-id=GetObject)
[%E6%B7%B1%E5%B1%A4%E7%94%9F%E6%88%90%E3%81%A6%E3%82%99%E4%BD%BF%E3%81%86%E7%A2%BA%E7%8E%87%E8%AB%96_%282%29.pdf](https://prod-files-secure.s3.us-west-2.amazonaws.com/a95e1e6d-ab5d-426a-b5b6-652ced6dcd2a/d2520981-ae64-41c7-b76b-2716e4e6dfcd/%E6%B7%B1%E5%B1%A4%E7%94%9F%E6%88%90%E3%81%A6%E3%82%99%E4%BD%BF%E3%81%86%E7%A2%BA%E7%8E%87%E8%AB%96_%282%29.pdf?X-Amz-Algorithm=AWS4-HMAC-SHA256&X-Amz-Content-Sha256=UNSIGNED-PAYLOAD&X-Amz-Credential=AKIAT73L2G45HZZMZUHI%2F20240118%2Fus-west-2%2Fs3%2Faws4_request&X-Amz-Date=20240118T043703Z&X-Amz-Expires=3600&X-Amz-Signature=092cdbdb1fdf76d65f7e2db34f2935d331ad4b41a64d9e36da0b16570d558d3c&X-Amz-SignedHeaders=host&x-id=GetObject)
[file]()
# ゴール
拡散モデルとシミュレーティッドアニーリングの関係を理解する
# Abstract
機械学習の中心的な問題は、学習、サンプリング、推論、評価が依然として解析的または計算的に扱いやすい、柔軟性の高い確率分布の族を用いて、複雑なデータセットをモデル化することである。ここでは、柔軟性と扱いやすさを同時に実現するアプローチを開発する。非平衡統計物理学に触発された本質的な考え方は、反復的な順拡散過程を通じて、データ分布の構造を系統的かつゆっくりと破壊することである。そして、データの構造を復元する逆拡散過程を学習し、データの柔軟性と扱いやすさの高い生成モデルを得る。この手法により、数千の層や時間ステップを持つ深い生成モデルで確率を高速に学習、サンプリング、評価し、学習したモデルの下で条件付き確率や事後確率を計算することができる。さらに、このアルゴリズムのオープンソースのリファレンス実装を公開する。
# 1. Introduction
- 以下がトレードオフ
- 扱いやすさ = 解析性
- 柔軟性 = 元のデータセットの構造に対して幅広く適用できる
- モンテカルロしないといけない
## 1.1. Diffusion probabilistic models
- 以下のようなモデルを提示するそう
- 超柔軟
- サンプリングが正確 (?)
- 事後分布を計算するために、他の分布と容易に乗算できること (?)
- モデルの対数尤度と個々の状態の確率を安価に評価できること (?)
- マルコフ連鎖である分布を別の分布に徐々に変換する
- 拡散過程を用いて単純な既知の分布(ガウス分布とか)を目標のデータ分布に変換するマルコフ連鎖を構築する
- 各ステップも連鎖の全体も解析的に評価できる
- estimating small perturbations (?)
- explicitly describing the full distribution with a single, non-analytically-normalizable, potential function. (?)
- 分配関数が、数式でかけないくらいの意味
- We demonstrate the utility of these diffusion probabilistic models by training high log likelihood models for a twodimensional swiss roll, binary sequence, handwritten digit
(MNIST), and several natural image (CIFAR-10, bark, and
dead leaves) datasets.
- Swiss roll ⇒ 渦巻き状の線形分離できないようなデータセットのこと
- posterior probability 事後確率
- prior probability 事前確率
- marginal probability distribution 周辺確率密度
## 一般定理
### ガウス積分
$$
\int_{-\infty}^{\infty} \exp{\left(-a(x-b)^2\right)} dx = \sqrt{\frac{\pi}{a}}
\quad \operatorname{Re}\{a\}>0, b \in \mathbb{C}
$$
## 用語
### 可測空間
- 組み $(\Omega, F)$
- $\Omega$ : set
- $F$ : 完全加法族
- 完全加法性を満たす、$\Omega$の冪集合の部分集合
### 可測写像
- $(\Omega, F), (\Psi, G): 可測空間$
- $f: (\Omega, F) \to (\Psi, G)$ で以下を満たす
- $\forall A \in G \space f^{-1}(A) \in F$
- ** $f: (\Omega, F) \to (\Psi, G)$ こう書いたときの実態は $f: F \to G$
### 測度空間
- 組み$(\Omega, F, \mu)$
- $\mu:F\to \mathbb{R}_{\geq0}$
- 可算加法性を満たす
- $\displaystyle \forall i,j \in \mathbb{N}, A_i, A_j \in F \space A_i \cup A_j = \empty \implies \mu\left(\bigcup_{i} A_{i} \right) = \sum_{i} \mu\left(A_{i} \right)$
### 実数 (測度空間の具体例)
- $(\mathbb{R},B(\mathbb{R}),\mu)$
- $B(\mathbb{R}):ボレル集合族$
- $\mathbb{R}上の距離から定まる位相を含む最小の完全加法族$
- $\mu: ルベーグ測度$
- 距離から自然に定まる「長さ」
- $\mu([0,1]) = 1$
- $\mu([a, b]) = b - a$
### 確率空間
- 測度空間 $(\Omega, F, P)$で以下を満たすもの
- $P\left(\Omega\right) = 1$
- $\Omega$ を**標本空間**という
- $\Omega$の元を**標本**という
- $F$ を**事象の全体**という
- $F$の元を**事象**という
- $P$ を**確率測度**という
### 確率変数 (random variety)
- $X: \Omega \to \mathbb{R}$ で、以下を満たすもの
- 可測である。つまり、$\forall \omega \in \mathfrak{B}(\mathbb{R}), X^{-1}(\omega) \in F$
### $X$に由来する確率測度
確率空間$(\Omega, F, P)$と確率変数$X$について、
$$P_{X}: \mathfrak{B}(\mathbb{R}) \to [0,1],\ A \mapsto P(X^{-1}(A))$$
が定まり、
$(\mathbb{R},\mathfrak{B}(\mathbb{R}),P_X)$は確率空間になっている
### d変数確率変数 (random variety)
$X_{1}, \dots ,X_{d}:1変数確率変数$
$$
(X_{1}, \dots ,X_{d}): (\Omega, F)\to (\mathbb{R}^d,\mathfrak{B}(\mathbb{R}^d)), \ \omega \mapsto (X_{1}(\omega), \dots,X_{d}(\omega))
$$
### $(X_{1}, \dots ,X_{d})$に由来する確率測度
確率空間$(\Omega, F, P)$とd変数確率変数$(X_{1}, \dots ,X_{d})$について、
$$P_{(X_{1}, \dots ,X_{d})}: \mathfrak{B}(\mathbb{R^{d}}) \to [0,1],\ A \mapsto P((X_{1}, \dots ,X_{d})^{-1}(A))$$
が定まり、
$(\mathbb{R},\mathfrak{B}(\mathbb{R}),P_X)$は確率空間になっている
### Lebesgueの分解定理
確率空間$(\Omega, F, P)$と確率変数$X$について、
$(\mathbb{R},\mathfrak{B}(\mathbb{R}), P_{X})$: 確率空間 が定まる。
$\mu: \mathfrak{B}(\mathbb{R}) \rightarrow \mathbb{R}$ を Lebegue Measure とする.
$$
P_X=P_{X_{abs}}+P_{X_{sing}}+P_{X_{dis}} \ s.t.
$$
- $P_{X_{abs}}$ は $\mu$ について、 absolutely conti.
- すなわち、 $\mu(A)=0$ ならば $P_{X_{abs}}(A)=0(\forall A \in B(\mathbb{R}))$
- $P_{X_{sing}}$ は $\mu$ について、conti. かつ、 singular.
- すなわち、 $P_{X_{sing}}(a)=0(\forall a \in \mathbb{R})$ かつ、 $\exists A \in A(\mathbb{R})$ s.t. $\mu(A)=0$ and $P_{X_{sing}}\left(A^c\right)=0$
- $P_{X_{dis}}$ は $\mu$ について、 discrete.
- すなわち、 $\exists\left\{a_n\right\}_{n=1}^{\infty} \subset \mathbb{R}$ s.t. $P_{X_{dis}}=\sum_{i=1}^{\infty} a_i \chi_{P_i}(A)$ $\left(\right.$ ただし、 $\chi_{P_i}: B(\mathbb{R}) \rightarrow \mathbb{R}, \chi_{P_i}(A):=\left\{\begin{array}{l}0\left(p_i \notin A\right) \\ 1\left(p_i \in A\right)\end{array}\right)$
と一意に分解できる.
- 拡散モデルの数理を読む上ではPabsだけ考えるでOK
#### 多変数の場合
確率空間$(\Omega, F, P)$と確率変数$(X_{1},\dots,X_{d})$について、
$(\mathbb{R^{d}},\mathfrak{B}(\mathbb{R^{d}}), P_{(X_{1},\dots,X_{d})})$: 確率空間 が定まる。
$\mu: \mathfrak{B}(\mathbb{R^{d}}) \rightarrow \mathbb{R}$ を Lebegue Measure とする.
以下は、1変数の場合と同様。
### Radon-Nikodymの定理
$X$: 確率変数
Lebegue の $P_{X_{abs}}$ について、以下を満たす Integrable な関数 $p_{X_{abs}}: \mathbb{R} \to \mathbb{R}_{\geq 0}$ が存在する。
$\forall x \in \mathbb{R}$
$$
\int_{-\infty}^x p_{X_{abs}}\left(x^{\prime}\right) d x^{\prime}=P_{X_{abs}}((-\infty, x])
$$
この $P_{X_{abs}}$ を、確率密度関数 (Probabilty Density Function) と呼ぶ。
- 気温が20度から30度になる確率を求めたい時に、
- 「気温x度」を実数xにマップする ← 確率変数という
- 実数直線上で20-30の区間で積分する
#### 多変数の場合
確率空間$(\Omega, F, P)$と$(X_{1},\dots,X_{d})$: d変数確率変数について、
$(\mathbb{R^{d}},\mathfrak{B}(\mathbb{R^{d}}), P_{(X_{1},\dots,X_{d})})$: 確率空間 が定まる。
Lebegue の $P_{(X_{1}, \dots ,X_{d})_{abs}}$ について、以下を満たす Integrable な関数 $p^{joint}_{(X_{1}, \dots ,X_{d})_{abs}}: \mathbb{R}^{d} \rightarrow \mathbb{R}_{\geq 0}$ が存在する。
$\forall x_{1}, \dots, x_{1} \in \mathbb{R}$
$$
\int_{-\infty}^{x_{1}} \cdots \int_{-\infty}^{x_{d}} p^{joint}_{(X_{1}, \dots ,X_{d})_{abs}}\left(x^{\prime}\right) d x^{\prime}=P_{(X_{1}, \dots ,X_{d})_{abs}}((-\infty, x_{1}] \times \cdots \times (-\infty, x_{d}])
$$
この $p^{joint}_{(X_{1}, \dots ,X_{d})_{abs}}$ を、結合確率密度関数 (Joint Probabilty Density Function) と呼ぶ。
> [フビニの定理](https://ja.wikipedia.org/wiki/%E3%83%95%E3%83%93%E3%83%8B%E3%81%AE%E5%AE%9A%E7%90%86) により、$\mathbb{R}^{d}$についての積分はd回の$\mathbb{R}$についての積分と等しくなる。
$X_{1}, \dots ,X_{d}$ は互いに入れ替え可能である。
特に、$d=2$の場合、
$$
p^{joint}_{(X_{1}, X_{2})_{abs}}(x_{1}, x_{2})=p^{joint}_{(X_{2}, X_{1})_{abs}}(x_{2}, x_{1})
$$
### Radon-Nikodymの定理の逆っぽい定理
$Claim.$
$d \in \Z_{\geq 1}$と、実数値関数 $p: \mathbb{R}^{d} \to \mathbb{R}_{\geq 0}$ について、
pが、
- $p$は可測関数
- $\int_{-\infty}^{\infty} \cdots \int_{-\infty}^{\infty} p\left(x^{\prime}\right) d x^{\prime}=1$
を満たすとき、
確率空間$(\mathbb{R}^{d},\mathfrak{B}(\mathbb{R}^{d}), P)$がただ一つ存在して、
$\mathbf{x} \in \mathbb{R}^{d}$
$$
\int_{-\infty}^{\text{pr}_{1}(\mathbf{x})} \cdots \int_{-\infty}^{\text{pr}_{d}(\mathbf{x})}
p\left(\mathbf{x^{\prime}}\right) d \mathbf{x^{\prime}}
=
P(
(-\infty, \text{pr}_{1}(\mathbf{x})]
\times \cdots \times
(-\infty, \text{pr}_{d}(\mathbf{x})]
)
\quad
$$
を満たす。
ただし、$1 \leq i \leq d$ に対して、 $X_{i} := \text{pr}_{i}: \mathbb{R}^{d} \to \mathbb{R}$ (射影)
$Proofの概要$
確率空間を構成する。
- $P_{(X_{1}, \dots ,X_{d})_{abs}}(X_{1} \leq x_{1} \land \dots \land X_{d} \leq x_{d})
:= \int_{-\infty}^{x^{}_{1}} \cdots \int_{-\infty}^{x_{d}}
p(x^{\prime}_{1}, \dots ,x^{\prime}_{d}) dx^{\prime}_{1} \cdots dx^{\prime}_{d}$
- $\prod_{i}(-\infty, x_{i}]$ は $\mathfrak{B}(\mathbb{R}^{d})$ の生成元
- よって、$P_{(X_{1}, \dots ,X_{d})_{abs}}$ は $\mathfrak{B}(\mathbb{R}^{d})$ 上の確率測度
### 累積分布関数
- 確率密度関数を$-\infty$から$x$まで積分したもの
### 確率質量関数 (= Px3の時に確率分布って適当に書かれてたらこれ)
$$
p_{x_{dis}}:\mathbb{R} \to [0,1] \\ x \mapsto P_{x_{3}}(\{x\})
$$
### d変数確率密度関数
Radon-Nikodymの定理の多変数版から定まる
$$p_{(X_{1}, \dots ,X_{d})_{abs}}: \mathbb{R}^{d} \rightarrow \mathbb{R}_{\geq 0}$$
---
↓一つの確率測度(確率分布)$P$について述べている
### 平均情報量(エントロピー)
$(\Omega, F, P): 確率空間$
$X: 確率変数$
$(\mathbb{R}, \mathfrak{B}(\mathbb{R}),P_{X})$: 上記から定まる確率空間
$$
H^{P}_{entropy}(X) := H_{entropy}(p_{X_{abs}}) := -\int_{x \in X} dx \ p_{X_{abs}}(x) \log p_{X_{abs}}(x)
$$
##### エントロピーの値の範囲
$$
0 \leq H_{entropy}(p_{X_{abs}}) (誤り)
$$
### 条件付き確率
$(\Omega, F, P): 確率空間, A, B \in F$について、
$P_{condi}(\cdot\mid\cdot):F\times F\to[0,1]$
$$
P_{condi}(A|B):=\frac{\mathrm{P}(A \cap B)}{\mathrm{P}(B)}
$$
##### 確率空間複数バージョン
確率空間 $(\mathbb{R}^{d}, \mathfrak{B}(\mathbb{R}^{d}),P_{d})$, $(\mathbb{R}^{d-1}, \mathfrak{B}(\mathbb{R}^{d-1}),P_{d-1})$
確率変数 $X_{d}: \mathbb{R}^{d} \to \mathbb{R}$, $X_{d-1}: \mathbb{R}^{d-1} \to \mathbb{R}$ について、
について、
$A_{d} \in \mathfrak{B}(\mathbb{R}^{d}), B_{d-1} \in \mathfrak{B}(\mathbb{R}^{d-1})$ について、
$P_{condi}^{P_{d},P_{d-1}}: \mathfrak{B}(\mathbb{R}^{d}) \times \mathfrak{B}(\mathbb{R}^{d-1}) \to [0,1]$
$$
P_{condi}^{P_{d},P_{d-1}}(A_{d} \mid B_{d-1}) := \frac{P_{d}(A_{d} \cap (B_{d-1} \times \mathbb{R}))}{P_{d-1}(B_{d-1})}
$$
### 条件つき確率密度関数
確率空間$(\mathbb{R}, \mathfrak{B}(\mathbb{R}),P)$
$(Y_{1}, Y_{2})$: 1変数確率変数$Y_{1}, Y_{2}$によって定まる2変数確率変数
$p^{condi}_{(Y_{1}, Y_{2})_{abs}}(\cdot\mid\cdot):\mathbb{R}\times\mathbb{R} \to \mathbb{R}_{\geq 0}$
$$
p^{condi}_{(Y_{1}, Y_{2})_{abs}}(y_{1} \mid y_{2}):= \frac{p^{joint}_{(Y_{1}, Y_{2})_{abs}}(y_{1}, y_{2})}{p_{Y_{2\ abs}}(y_{2})}
$$
##### 確率空間複数バージョン
確率測度、$P_{condi}^{P_{d},P_{d-1}}$ からRandon-Nikodymの定理により定まる、
$$
p_{condi}^{P_{d} \mid P_{d-1}}: \mathbb{R}^{d} \times \mathbb{R}^{d-1} \to \mathbb{R}_{\geq 0}
$$
を、条件付き確率密度関数という
### 結合確率
確率空間 $(\mathbb{R}^{d}, \mathfrak{B}(\mathbb{R}^{d}),P_{d})$, $(\mathbb{R}^{d-1}, \mathfrak{B}(\mathbb{R}^{d-1}),P_{d-1})$
確率変数 $X_{d}: \mathbb{R}^{d} \to \mathbb{R}$, $X_{d-1}: \mathbb{R}^{d-1} \to \mathbb{R}$ について、
について、
$A_{d} \in \mathfrak{B}(\mathbb{R}^{d}), B_{d-1} \in \mathfrak{B}(\mathbb{R}^{d-1})$ について、
$$
P_{joint}(A_{d}, B_{d-1}) := P_{d}(A_{d})P_{condi}(A_{d} \mid B_{d-1})
$$
### 結合エントロピー (joint entropy)
##### 確率空間ひとつバージョン
確率空間$(\mathbb{R}, \mathfrak{B}(\mathbb{R}),P)$
確率変数$X, Y$について、
$$
\begin{align*}
H^{P}_{joint}(X, Y) := H_{joint}(p^{joint}_{(X, Y)_{abs}}) &:= -\int_{x \in X, y \in Y} dxdy \ p^{joint}_{(X, Y)_{abs}}(x, y) \log \left(p^{joint}_{(X, Y)_{abs}}(x, y)\right)
\end{align*}
$$
定義から直ちに、
$$
H_{joint}(Y, X) = H_{joint}(X, Y) \hspace{40pt} 論文(25)
$$
がいえる。
##### 確率空間複数バージョン
### 条件付きエントロピー (conditional entropy)
確率空間$(\mathbb{R}, \mathfrak{B}(\mathbb{R}),P)$
確率変数$X, Y$について、
$$
\begin{align*}
H^{P}_{condi}(Y|X) &:= -\int_{x \in X, y \in Y} dxdy \ p^{joint}_{(Y, X)_{abs}}(y, x) \log \left(\frac{p^{joint}_{(Y, X)_{abs}}(y, x)}{p_{X_{abs}}(x)}\right) \\
&= -\int_{x \in X, y \in Y} dxdy \ p^{joint}_{(Y, X)_{abs}}(y, x) \log \left(p^{condi}_{(Y,X)_{abs}}(y \mid x)\right) \\
\end{align*}
$$
$Claim$
以下が成り立つ
$$
H^{P}_{condi}\left(Y \mid X\right)=H^{P}_{condi}\left(X \mid Y\right)+H^{P}_{entropy}\left(Y\right)-H^{P}_{entropy}\left(X\right)
$$
$Proof.$
$$
\begin{align*}
(右辺) &= H^{P}_{condi}\left(X \mid Y\right)+H^{P}_{entropy}\left(Y\right)-H^{P}_{entropy}\left(X\right) \\
&= -\int_{x \in X, y \in Y} dxdy \ p^{joint}_{(X, Y)_{abs}}(x, y) \log \left(p^{condi}_{(X, Y)_{abs}}(x \mid y)\right) - \int_{y \in Y} dy \ p_{Y_{abs}}(y) \log p_{Y_{abs}}(y) +\int_{x \in X} dx \ p_{X_{abs}}(x) \log p_{X_{abs}}(x) \hspace{40pt} (∵def.) \\
&= -\int_{x \in X, y \in Y} dxdy \ p^{joint}_{(X, Y)_{abs}}(x, y) \cdot \log \left(\frac{
p^{joint}_{(X, Y)_{abs}}(x, y)
}{
p_{Y_{abs}}(y)
}\right) - \int_{y \in Y} dy \ p_{Y_{abs}}(y) \log p_{Y_{abs}}(y) +\int_{x \in X} dx \ p_{X_{abs}}(x) \log p_{X_{abs}}(x) \hspace{40pt} (∵p_{joint}のdef.) \\
&= -\int_{x \in X, y \in Y} dxdy \ \left(
p^{joint}_{(X, Y)_{abs}}(x, y) \cdot \left(
\log \left(p^{joint}_{(X, Y)_{abs}}(x, y)\right) - \log \left(p_{Y_{abs}}(y)\right)
\right)
\right)
- \int_{y \in Y} dy \ p_{Y_{abs}}(y) \log p_{Y_{abs}}(y) +\int_{x \in X} dx \ p_{X_{abs}}(x) \log p_{X_{abs}}(x) \\
&= -\int_{x \in X, y \in Y} dxdy \ \left(
p^{joint}_{(X, Y)_{abs}}(x, y) \cdot \left(\log \left(p^{joint}_{(X, Y)_{abs}}(x, y)\right)\right)
\right)
\underbrace{
+ \int_{x \in X, y \in Y} dxdy \ \left(
p^{joint}_{(X, Y)_{abs}}(x, y) \cdot \left(
\log \left(p_{Y_{abs}}(y)\right)
\right)
\right)
}_{
\cancel{
+ \int_{y \in Y} dy \ \left(
p_{Y_{abs}}(y) \cdot \left(
\log \left(p_{Y_{abs}}(y)\right)
\right)
\right)
}
}
\cancel{- \int_{y \in Y} dy \ p_{Y_{abs}}(y) \log p_{Y_{abs}}(y)}
+ \int_{x \in X} dx \ p_{X_{abs}}(x) \log p_{X_{abs}}(x)
\\
&= -\int_{x \in X, y \in Y} dxdy \ \left(
p^{joint}_{(X, Y)_{abs}}(x, y) \cdot \left(\log \left(p^{joint}_{(X, Y)_{abs}}(x, y)\right)\right)
\right)
+ \int_{x \in X} dx \ p_{X_{abs}}(x) \log p_{X_{abs}}(x)
\\
&= -\int_{x \in X, y \in Y} dxdy \ \left(
p^{joint}_{(X, Y)_{abs}}(x, y) \cdot \left(\log \left(p^{joint}_{(X, Y)_{abs}}(x, y)\right)\right)
\right)
+ \int_{x \in X, y \in Y} dxdy \ \left(
p^{joint}_{(X, Y)_{abs}}(x, y) \cdot \left(\log \left(p_{X_{abs}}(x)\right)\right)
\right) \\
&= -\int_{x \in X, y \in Y} dxdy \ \left(
p^{joint}_{(X, Y)_{abs}}(x, y) \cdot \left(\log \left(\frac{
p^{joint}_{(X, Y)_{abs}}(x, y)
}{
p_{X_{abs}}(x)
}\right)\right)
\right)
&= -\int_{x \in X, y \in Y} dxdy \ \left(
p^{joint}_{(Y, X)_{abs}}(y, x) \cdot \left(\log \left(\frac{
p^{joint}_{(Y, X)_{abs}}(y, x)
}{
p_{X_{abs}}(x)
}\right)\right)
\right)
\\
&= H^{P}_{condi}\left(Y \mid X\right) \hspace{40pt} (論文(27))
\end{align*}
$$
$Q.E.D.$
$Claim$
以下が成り立つ、
$$
H^{P}_{condi}\left(X \mid Y\right) = H^{P}_{join}\left(X, Y\right) - H^{P}_{entropy}\left(Y\right)
$$
$Proof.$
$$
\begin{align*}
(右辺) &= H^{P}_{join}\left(X, Y\right) - H^{P}_{entropy}\left(Y\right) \\
&=
-\int_{x \in X, y \in Y} dxdy \
p^{joint}_{(X, Y)_{abs}}(x, y) \log \left(p^{joint}_{(X, Y)_{abs}}(x, y)\right)
- \left(
-\int_{y \in Y} dy \
p_{Y_{abs}}(y) \log p_{Y_{abs}}(y)
\right) \\
&=
-\int_{x \in X, y \in Y} dxdy \
p^{joint}_{(X, Y)_{abs}}(x, y) \log \left(p^{joint}_{(X, Y)_{abs}}(x, y)\right)
- \left(
- \int_{x \in X, y \in Y} dxdy \
p^{joint}_{(X, Y)_{abs}}(x, y) \log p_{Y_{abs}}(y)
\right) \\
&=
-\int_{x \in X, y \in Y} dxdy \
p^{joint}_{(X, Y)_{abs}}(x, y) \left(
\log \left(p^{joint}_{(X, Y)_{abs}}(x, y)\right)
-
\log p_{Y_{abs}}(y)
\right) \\
&=
-\int_{x \in X, y \in Y} dxdy \
p^{joint}_{(X, Y)_{abs}}(x, y) \log \left(\frac{
p^{joint}_{(X, Y)_{abs}}(x, y)
}{
p_{Y_{abs}}(y)
}\right) \\
&= H^{P}_{condi}\left(X \mid Y\right)
\end{align*}
$$
### 相互情報量 (Mutual information)
確率空間$(\mathbb{R}, \mathfrak{B}(\mathbb{R}),P)$, 確率変数$X, Y$について、
$$
\begin{align*}
I(Y;X) &:= \int_{x \in X, y \in Y} dxdy \ p^{joint}_{(Y, X)_{abs}}(y, x) \log \left(\frac{p^{joint}_{(Y, X)_{abs}}(y, x)}{p_{Y_{abs}}(y)p_{X_{abs}}(x)}\right)
\end{align*}
$$
(ざっくりとは、X,Yが独立でない場合の「情報量の重なり」みたいなもの)
$Claim$
相互情報量と結合エントロピーについて以下が成り立つ、
確率空間$(\mathbb{R}, \mathfrak{B}(\mathbb{R}),P)$,
確率変数$X, Y$について、
$$
H^{P}_{joint}\left(X, Y\right)=H^{P}_{entropy}\left(X\right)+H^{P}_{entropy}\left(Y\right)-I\left(Y;X\right)
$$
$proof.$
$$
\begin{align*}
(右辺) &= H^{P}_{entropy}\left(X\right)+H^{P}_{entropy}\left(Y\right)-I\left(Y;X\right) \\
&=
-\int_{x \in X} dx \
p_{X_{abs}}(x) \log \left(p_{X_{abs}}(x)\right)
-\int_{y \in Y} dy \
p_{Y_{abs}}(y) \log \left(p_{Y_{abs}}(y)\right)
+\int_{x \in X, y \in Y} dxdy \
p^{joint}_{(X, Y)_{abs}}(x, y) \log \left(\frac{p^{joint}_{(X, Y)_{abs}}(x, y)}{p_{Y_{abs}}(y)p_{X_{abs}}(x)}\right) \\
&=
-\int_{x \in X} dxdy \
p_{X_{abs}}(x) \log \left(p_{X_{abs}}(x)\right)
-\int_{y \in Y} dxdy \
p_{Y_{abs}}(y) \log \left(p_{Y_{abs}}(y)\right)
-\int_{x \in X, y \in Y} dxdy \
p^{joint}_{(X, Y)_{abs}}(x, y) \left(
\log \left(p^{joint}_{(X, Y)_{abs}}(x, y)\right)
-
\log \left(p_{Y_{abs}}(y)\right)
-
\log \left(p_{X_{abs}}(x)\right)
\right) \\
&=
\cancel{
-\int_{x \in X} dxdy \
p_{X_{abs}}(x) \log \left(p_{X_{abs}}(x)\right)
}
+\underbrace{
\int_{x \in X, y \in Y} dxdy \
p^{joint}_{(X, Y)_{abs}}(x, y) \log \left(p_{X_{abs}}(x)\right)
}_{
\cancel{
\int_{x \in X} dxdy \
p_{X_{abs}}(x) \log \left(p_{X_{abs}}(x)\right)
}
}
\cancel{
-\int_{y \in Y} dxdy \
p_{Y_{abs}}(y) \log \left(p_{Y_{abs}}(y)\right)
}
+\underbrace{
\int_{x \in X, y \in Y} dxdy \
p^{joint}_{(X, Y)_{abs}}(x, y) \log \left(p_{Y_{abs}}(y)\right)
}_{
\cancel{
\int_{y \in Y} dxdy \
p_{Y_{abs}}(y) \log \left(p_{Y_{abs}}(y)\right)
}
}
-\int_{x \in X, y \in Y} dxdy \
p^{joint}_{(X, Y)_{abs}}(x, y) \left(
\log \left(p^{joint}_{(X, Y)_{abs}}(x, y)\right)
\right) \\
&=
-\int_{x \in X, y \in Y} dxdy \
p^{joint}_{(X, Y)_{abs}}(x, y) \left(
\log \left(p^{joint}_{(X, Y)_{abs}}(x, y)\right)
\right) \\
&= H^{P}_{joint}\left(X, Y\right)
\end{align*}
$$
$Q.E.D.$
$Claim$
$p^{joint}_{(X, Y)_{abs}}(x, y) \leq p_{X_{abs}}(x)$ のとき以下が成り立つ、
$$
H^{P}_{joint}\left(X, Y\right) - H^{P}_{entropy}\left(X\right) \geq 0
$$
$Proof.$
$$
\begin{align*}
H^{P}_{joint}\left(X, Y\right) - H^{P}_{entropy}\left(X\right) &= H^{P}_{condi}\left(Y \mid X\right) \ (\because 上記のclaim) \\
&=
-\int_{x \in X, y \in Y} dxdy \
p^{joint}_{(X, Y)_{abs}}(x, y) \log \left(\frac{
p^{joint}_{(X, Y)_{abs}}(x, y)
}{
p_{X_{abs}}(x)
}\right) \\
&\geq 0 \ (\because \frac{
p^{joint}_{(X, Y)_{abs}}(x, y)
}{
p_{X_{abs}}(x)
} \leq 1)
\end{align*}
$$
$Q.E.D.$
---
↓2つの確率測度(確率分布)$P, Q$について述べている
### 交差エントロピー (cross entropy)
$X$: 可測空間$(\Omega, F)$上の確率変数
$(\Omega, F, P),(\Omega,F, Q)$: 確率空間
$(\mathbb{R}, \mathfrak{B}(\mathbb{R}),P_{X}), (\mathbb{R}, \mathfrak{B}(\mathbb{R}),Q_{X})$: 上記から定まる確率空間
について、
$$
\begin{align*}
H_{cross}(P_{X}, Q_{X}) &:= -\sum_{i=1}^{\infty} p_{X_{dis}}\left(x_i\right) \log \left(q_{X_{dis}}\left(x_i\right)\right) - \int_{x \in \mathbb{R}} dx \ p_{X_{abs}}(x)log(q_{X_{abs}}(x))
\end{align*}
$$
$p_{X_{dis}}=q_{X_{dis}}=0$のとき、
$$
H_{cross}(p_{X_{abs}}, q_{X_{abs}}) := H_{cross}(P_{X}, Q_{X})
$$
ともかく。
### KL-ダイバージェンス
$X$: 可測空間$(\Omega, F)$上の確率変数
$(\Omega, F, P),(\Omega,F, Q)$: 確率空間
$(\mathbb{R},\mathfrak{B}(\mathbb{R}), P_{X}), (\mathbb{R},\mathfrak{B}(\mathbb{R}), Q_{X})$: 上記から定まる確率空間
について、
$$
D_{K L}(P_{X} \| Q_{X}):=\sum_{i=1}^{\infty} p_{X_{dis}}\left(x_i\right) \log \frac{p_{X_{dis}}\left(x_i\right)}{q_{X_{dis}}\left(x_i\right)}+\int_{\mathbb{R}} p_{X_{abs}}(x) \log \frac{p_{X_{abs}}(x)}{q_{X_{abs}}(x)} d x
$$
$p_{X_{dis}}=q_{X_{dis}}=0$のとき、
$$
D_{K L}(p_{X_{abs}} \| q_{X_{abs}}) := D_{K L}(P_{X} \| Q_{X})
$$
ともかく。
##### 確率変数が複数ある状況の場合
$X, Y$: 可測空間$(\Omega, F)$上の確率変数
$(\Omega, F, P)$: 確率空間
を考えると、
$id_{\mathbb{R}}$: 可測空間$(\mathbb{R},\mathfrak{B}(\mathbb{R}))$上の確率変数 (恒等写像)
$(\mathbb{R},\mathfrak{B}(\mathbb{R}), P_{X}),(\mathbb{R},\mathfrak{B}(\mathbb{R}), P_{Y})$: 確率空間
$(\mathbb{R},\mathfrak{B}(\mathbb{R}), (P_{X})_{id_{\mathbb{R}}}), (\mathbb{R},\mathfrak{B}(\mathbb{R}), (Q_{X})_{id_{\mathbb{R}}})$: 確率空間
として、KL-ダイバージェンス を考えることができる。
---
### parametricな問題
$$
{ }^{\exists} p: \mathbb{R} \times \mathbb{R} \rightarrow \mathbb{R}, \quad(x, \theta) \mapsto p_\theta(x), 具体的に計算可能, \\ { }^{\exists} \theta_0 \in \mathbb{R} \text { s.t. } p_{X_{abs}}=p_{\theta_0}
$$
$p_{X_{abs}}$を求める問題をparametricな問題という
($p_{x_{3}}$についてもだいたい同じ)
### likelihood function
$\left(x_i\right)_{i=1}^n$: n回のtrial が与えられた時、
$$
L: \mathbb{R} \rightarrow \mathbb{R}, \quad \theta \mapsto \prod_{i=1}^n p\left(x_i, \theta\right)
$$
### log-likelihood function
$\left(x_i\right)_{i=1}^n$: n回のtrial が与えられた時、
$$
L_{\log}: \mathbb{R} \rightarrow \mathbb{R}, \quad \theta \mapsto \sum_{i=1}^n \log(p\left(x_i, \theta\right))
$$
### likelihood estimation
$L, L_{\log}$の最大値、もしくは極値を求める
コイン投げの場合、尤度関数は上に凸なので、極値が求まれば良い
---
## Sanovの定理[https://genkuroki.github.io/documents/20160616KullbackLeibler.pdf](https://genkuroki.github.io/documents/20160616KullbackLeibler.pdf)
参考) [https://genkuroki.github.io/documents/mathtodon/2017-04-27 Kullback-Leibler情報量とは何か?.pdf](https://genkuroki.github.io/documents/mathtodon/2017-04-27%20Kullback-Leibler%E6%83%85%E5%A0%B1%E9%87%8F%E3%81%A8%E3%81%AF%E4%BD%95%E3%81%8B%EF%BC%9F.pdf)
### 経験分布
r面サイコロを振るということを考える
$$
\mathcal{P}=\left\{p=\left(p_1, \ldots, p_r\right) \in \mathbb{R}^r \mid p_1, \ldots, p_r \geqq 0, p_1+\cdots+p_r=1\right\}
$$
と定めると、$\mathcal{P}$は、有限集合 $\{1, ..., r\}$上の確率質量関数の集合と同一視できる。
$q = (q_{1},...,q_{r}) \in \mathcal{P}$ を固定する。これはあるサイコロqを固定するということ。
$\Omega_{q} := \{サイコロqを投げた時にiが出る \mid i=1, ..., r\}$
確率空間: $((\Omega_{q})^{n}, F_{q}^{n} = 2^{(\Omega_{q})^{n}}, P^{n})$
$X \in F_{q}^{n}$は、
$$
X = \{(\ell_{i}^{1})_{i=1,...,n} ,..., (\ell_{i}^{j})_{i=1,...,n}\} \\ \left(j=0,...,r^{n}, 1 \leq \ell_{i}^{j} \leq r, ただしj=0の時はX=\empty \right)
$$
と書ける
$$
r^{n}: 全ての根源事象の数 \\
(\ell_{i}^{j})_{i=1,...,n}: ある事象Xの中で、i回目に\ell_{i}^{j}が出るようなj番目の根源事象
$$
$P^{n}: F_{q}^{n}\to\mathbb{R} $ を、
$$
P^{n}(\{(\ell_{i}^{1})_{i=1,...,n} ,..., (\ell_{i}^{j})_{i=1,...,n}\}):= (q_{\ell^{1}_{1}} q_{\ell^{1}_{2}} \cdots q_{\ell^{1}_{n}}) + \cdots + (q_{\ell^{j}_{1}} q_{\ell^{j}_{2}} \cdots q_{\ell^{j}_{n}})
$$
と定める。
$(k_{i})_{i=1,...,n}$について、
$$
X_{(k_{w})_{w=1,...,r}} := \{(\ell_{i}^{j})_{i=1,...,n}|^\forall w \left( (\ell_{i}^{j} = wとなる個数) = k_{w} \right) \}
$$
なる事象の起こる確率は、$\#X_{(k_{w})_{w=1,...,r}} = \frac{n !}{k_{1} ! \cdots k_{r} !}$なので、
$$
P^n(X_{(k_{w})_{w=1,...,r}}) = \frac{n !}{k_{1} ! \cdots k_{r} !} q_1^{k_1} \cdots q_r^{k_r}
$$
となる。
ここで、集合$\mathcal{P}_{n}$を、
$$
\mathcal{P}_n=\left\{\left(\frac{k_1}{n}, \ldots, \frac{k_r}{n}\right) \mid k_i=0,1, \ldots, n, k_1+\cdots+k_r=n\right\}
$$
と定めると、
$$
\mathcal{P}_n \subset \mathcal{P}
$$
であり、$\#\mathcal{P}_{n} \leq (n+1)^r$ である。
このような$\mathcal{P}_n$の元を、
$$
X_{(k_{i})_{i=1,...,n}}に対しての経験分布
$$
という。
### Sanovの定理
$q = (q_{1},...,q_{r}) \in \mathcal{P}$と、上記の記号の元、確率空間$(\mathcal{P}_{n}, 2^{\mathcal{P}_{n}}, P_{\mathcal{P}_{n}, q})$を考える
確率測度は以下のように定める
$$
P_{\mathcal{P}_{n}, q}^{\prime}:\mathcal{P}_{n} の一点集合の集合\to\mathbb{R} \\ \{\left(\frac{k_1}{n}, \ldots, \frac{k_r}{n}\right)\} \mapsto \frac{n !}{k_{1} ! \cdots k_{r} !} q_1^{k_1} \cdots q_r^{k_r}
$$
$$
P_{\mathcal{P}_{n}, q}:2^{\mathcal{P}_{n}}\to\mathbb{R} \\ \{x_{1},...,x_{k}\} \mapsto \sum_{i}^{k}P_{\mathcal{P}_{n}, q}^{\prime}(\{x_{i}\})
$$
(1) $A \subset \mathcal{P}$ がopenである時
$$
\liminf _{n \rightarrow \infty} \frac{1}{n} \log P_{\mathcal{P}_n, q}\left( A\cap\mathcal{P}_n\right) \geqq-\inf _{p \in A} D_{KL}(p \| q)
$$
Aを大きくすると、
((左辺)の$\frac{1}{n} \log P_{\mathcal{P}_n, q}\left( A\cap\mathcal{P}_n\right)$)は、大きくなるか変わらない
(右辺)は、大きくなるか変わらない
(2) $A \subset \mathcal{P}$について、
$$
\limsup _{n \rightarrow \infty} \frac{1}{n} \log P_{\mathcal{P}_n, q}\left(A\cap\mathcal{P}_n\right) \leqq-\inf _{p \in A} D_{KL}(p \| q)
$$
(3) $A \subset \mathcal{P}$が、内部が閉包に含まれるならば、
$$
\lim_{n \rightarrow \infty} \frac{1}{n} \log P_{\mathcal{P}_n, q}\left(A\cap\mathcal{P}_n\right) =-\inf _{p \in A} D_{KL}(p \| q)
$$
- 仮説Aをとる
- Aは絞られてるほうが、実用上「いい仮説」と言える
- が、Sanovの定理ではそこは興味ない
- $P_{\mathcal{P}_n, q}\left(A\cap\mathcal{P}_n\right) $ : n回試行時の経験分布がAに入る確率
→ n回振って得られた経験分布を何個も取得して、$P_{\mathcal{P}_n, q}\left(A\cap\mathcal{P}_n\right) $を定める
- 左辺のnをどんどん大きくして、収束する値によって、Aの「正解っぽさ」がわかる
- もし0に収束しているのならば、Aに真の分布qが含まれていることがわかる
## 1.2. Relationship to other work
飛ばす
# 2. Algorithm
- 順拡散過程と逆拡散過程の定義
- 逆拡散過程の学習方法
- 逆拡散過程のentropy bounds(エントロピー下界?)を導出する
### マルコフ拡散カーネル ([Markov kernel](https://en.wikipedia.org/wiki/Markov_kernel), [積分変換](https://ja.wikipedia.org/wiki/%E7%A9%8D%E5%88%86%E5%A4%89%E6%8F%9B))
$T_{\pi}: \mathbb{R}^{n}\times\mathbb{R}^{n}\times\mathbb{R}\to\mathbb{R}_{\geq 0},\ \left(\mathbf{y} , \mathbf{y}^{\prime} , \beta\right) \mapsto \left(\mathbf{y} \mid \mathbf{y}^{\prime} ; \beta\right)$ ($\beta$は、拡散率)
$T_{\pi}$は、$d \in \mathbf{Z}_{\geq 1}$として、任意の$n \cdot d$変数確率密度関数 $q: \mathbb{R}^{n \cdot d}\to\mathbb{R}$ に対して、
$$
q(x_{1}, \dots, x_{d}) \cdot T_{\pi}\left(x_{d+1} \mid x_{d} ; \beta\right) はn \cdot (d+1)変数確率密度関数 \\
$$
$\pi: \mathbb{R}^{n}\to\mathbb{R}$ は、以下の[第二種フレドホルム積分方程式](https://ja.wikipedia.org/wiki/%E3%83%95%E3%83%AC%E3%83%89%E3%83%9B%E3%83%AB%E3%83%A0%E7%A9%8D%E5%88%86%E6%96%B9%E7%A8%8B%E5%BC%8F)の解
$$
\begin{aligned}
\pi(\mathbf{y}) & =\int d \mathbf{y}^{\prime} T_\pi\left(\mathbf{y} \mid \mathbf{y}^{\prime} ; \beta\right) \pi\left(\mathbf{y}^{\prime}\right)
\end{aligned}
$$
- [https://ja.wikipedia.org/wiki/フレドホルム積分方程式](https://ja.wikipedia.org/wiki/%E3%83%95%E3%83%AC%E3%83%89%E3%83%9B%E3%83%AB%E3%83%A0%E7%A9%8D%E5%88%86%E6%96%B9%E7%A8%8B%E5%BC%8F)
##### 正規分布を使った$\pi$と$T_{\pi}$がフレドホルム積分方程式の解であること
一次元でチェックする
$\pi(y) := \mathcal{N}(y, 0, 1) = \frac{1}{\sqrt{2 \pi}} \exp \left(-\frac{y^2}{2}\right)$
$T_{\pi}(y \mid y^{\prime} ; \beta)
:= \mathcal{N}(y, y^{\prime}\sqrt{1-\beta}, \beta)
= \frac{1}{\sqrt{2 \pi \beta^{2}}} \exp \left(-\frac{(y-y^{\prime}\sqrt{1-\beta})^2}{2\beta^{2}}\right)
$
とおく
$\pi(y) = \int dy^{\prime} T_{\pi}(y \mid y^{\prime}; \beta) \pi(y^{\prime})$ が、上記に対して成り立つことを確認する
$$
\begin{aligned}
(右辺) &= \int dy^{\prime} \frac{1}{\sqrt{2 \pi \beta^{2}}} \exp \left(-\frac{(y-y^{\prime}\sqrt{1-\beta})^2}{2\beta^{2}}\right) \frac{1}{\sqrt{2 \pi}} \exp \left(-\frac{y^{\prime 2}}{2}\right) \\
&= \frac{1}{2 \pi \beta} \int dy^{\prime} \exp \left(-\frac{(y-y^{\prime}\sqrt{1-\beta})^2}{2\beta^{2}} - \frac{y^{\prime 2}}{2}\right) \\
&= \frac{1}{2 \pi \beta} \int dy^{\prime} \exp \left(-\frac{(y-y^{\prime}\sqrt{1-\beta})^2}{2\beta^{2}} - \frac{y^{\prime 2} \beta^{2}}{2 \beta^{2}}\right) \\
&= \frac{1}{2 \pi \beta} \int dy^{\prime} \exp \left(- \frac{y^{\prime 2} \beta^{2}}{2 \beta^{2}} -\frac{(-y^{\prime}\sqrt{1-\beta} + y)^2}{2\beta^{2}}\right) \\
&= \frac{1}{2 \pi \beta} \int dy^{\prime} \exp \left(- \frac{y^{\prime 2} \beta^{2} + (-y^{\prime}\sqrt{1-\beta} + y)^{2}}{2 \beta^{2}}\right) \\
&= \frac{1}{2 \pi \beta} \int dy^{\prime} \exp \left(- \frac{y^{\prime 2} \beta^{2} + (1-\beta)y^{\prime 2} - 2\sqrt{1-\beta}yy^{\prime} + y^{2}}{2 \beta^{2}}\right) \\
&= \frac{1}{2 \pi \beta} \int dy^{\prime} \exp \left(- \frac{y^{\prime 2} (1-\beta+\beta^{2}) - 2\sqrt{1-\beta}yy^{\prime} + y^{2}}{2 \beta^{2}}\right) \\
&= \frac{1}{2 \pi \beta} \int dy^{\prime} \exp \left(- \frac{(1-\beta+\beta^{2}) \left( y^{\prime 2} - \frac{2\sqrt{1-\beta}yy^{\prime}}{1-\beta+\beta^{2}} \right) + y^{2}}{2 \beta^{2}}\right) \\
&= \frac{1}{2 \pi \beta} \int dy^{\prime}
\exp \left(
- \frac{
(
1-\beta+\beta^{2}
) \left(
y^{\prime 2}
- \frac{
2\sqrt{1-\beta}yy^{\prime}}{1-\beta+\beta^{2}
}
+ \frac{
(1-\beta)y^{2}}{(1-\beta+\beta^{2})^{2}
}
- \frac{
(1-\beta)y^{2}}{(1-\beta+\beta^{2})^{2}
}
\right) + y^{2}
}{
2 \beta^{2}
}
\right) \\
&= \frac{1}{2 \pi \beta} \int dy^{\prime}
\exp \left(
- \frac{
(
1-\beta+\beta^{2}
) \left(
(y^{\prime} - \frac{\sqrt{1-\beta}y}{1-\beta+\beta^{2}})^{2}
- \frac{
(1-\beta)y^{2}}{(1-\beta+\beta^{2})^{2}
}
\right) + y^{2}
}{
2 \beta^{2}
}
\right) \\
&= \frac{1}{2 \pi \beta} \int dy^{\prime}
\exp \left(
- \frac{
(1-\beta+\beta^{2})(y^{\prime} - \frac{\sqrt{1-\beta}y}{1-\beta+\beta^{2}})^{2}
- (1-\beta+\beta^{2}) \frac{
(1-\beta)y^{2}}{(1-\beta+\beta^{2})^{2}
}
+ y^{2}
}{
2 \beta^{2}
}
\right) \\
&= \frac{1}{2 \pi \beta} \int dy^{\prime}
\exp \left(
- \frac{
(1-\beta+\beta^{2})(y^{\prime} - \frac{\sqrt{1-\beta}y}{1-\beta+\beta^{2}})^{2}
- \frac{
(1-\beta)y^{2}}{1-\beta+\beta^{2}
}
+ y^{2}
}{
2 \beta^{2}
}
\right) \\
&= \frac{1}{2 \pi \beta} \int dy^{\prime}
\exp \left(
- \frac{
(1-\beta+\beta^{2})(y^{\prime} - \frac{\sqrt{1-\beta}y}{1-\beta+\beta^{2}})^{2}
- \frac{
(1-\beta) - (1-\beta+\beta^{2})
}{
1-\beta+\beta^{2}
} y^{2}
}{
2 \beta^{2}
}
\right) \\
&= \frac{1}{2 \pi \beta} \int dy^{\prime}
\exp \left(
- \frac{
(1-\beta+\beta^{2})(y^{\prime} - \frac{\sqrt{1-\beta}y}{1-\beta+\beta^{2}})^{2}
+ \frac{
\beta^{2}
}{
1-\beta+\beta^{2}
} y^{2}
}{
2 \beta^{2}
}
\right) \\
&= \frac{1}{2 \pi \beta}
\exp \left(
- \frac{
\frac{
\beta^{2}
}{
1-\beta+\beta^{2}
} y^{2}
}{
2 \beta^{2}
}
\right)
\int dy^{\prime}
\exp \left(
- \frac{
(1-\beta+\beta^{2})(y^{\prime} - \frac{\sqrt{1-\beta}y}{1-\beta+\beta^{2}})^{2}
}{
2 \beta^{2}
}
\right) \\
&= \frac{1}{2 \pi \beta}
\exp \left(
- \frac{
\frac{
\beta^{2}
}{
1-\beta+\beta^{2}
} y^{2}
}{
2 \beta^{2}
}
\right)
\sqrt{
\frac{
\pi
}{
\frac{
1-\beta+\beta^{2}
}{
2 \beta^{2}
}
}
}
\\
&= \frac{1}{2 \pi \beta}
\sqrt{
\frac{
2 \pi \beta^{2}
}{
1-\beta+\beta^{2}
}
}
\exp \left(
- \frac{
y^{2}
}{
2 (1-\beta+\beta^{2})
}
\right)
\\
&= \frac{1}{\sqrt{2 \pi (1-\beta+\beta^{2})}}
\exp \left(
- \frac{
y^{2}
}{
2 (1-\beta+\beta^{2})
}
\right)
\\
&= \mathcal{N}(y, 0, \sqrt{1-\beta+\beta^{2}})
\end{aligned}
$$
あわんかったので、$T_{\pi}(y \mid y^{\prime} ; \beta):= \mathcal{N}(y, m y^{\prime}, \beta)$で計算して、噛み合う$m$を見つける。
$\pi(y) := \mathcal{N}(y, 0, 1) = \frac{1}{\sqrt{2 \pi}} \exp \left(-\frac{y^2}{2}\right)$
$T_{\pi}(y \mid y^{\prime} ; \beta)
:= \mathcal{N}(y, m y^{\prime}, \beta)
= \frac{1}{\sqrt{2 \pi \beta^{2}}} \exp \left(-\frac{(y-m y^{\prime})^2}{2\beta^{2}}\right)
$
とおく
$$
\begin{aligned}
(右辺) &= \int dy^{\prime} \frac{1}{\sqrt{2 \pi \beta^{2}}} \exp \left(-\frac{(y-m y^{\prime})^2}{2\beta^{2}}\right) \frac{1}{\sqrt{2 \pi}} \exp \left(-\frac{y^{\prime 2}}{2}\right) \\
&= \frac{1}{2 \pi \beta} \int dy^{\prime} \exp \left(- \frac{y^{\prime 2} \beta^{2} + m^{2} y^{\prime 2} - 2myy^{\prime} + y^{2}}{2 \beta^{2}}\right) \\
&= \frac{1}{2 \pi \beta} \int dy^{\prime} \exp \left(- \frac{(\beta^{2} + m^{2}) y^{\prime 2} - 2myy^{\prime} + y^{2}}{2 \beta^{2}}\right) \\
&= \frac{1}{2 \pi \beta} \int dy^{\prime}
\exp \left(
- \frac{
(\beta^{2} + m^{2}) \left(
y^{\prime 2}
- \frac{
2myy^{\prime}
}{
\beta^{2} + m^{2}
}
\right)
+ y^{2}
}{
2 \beta^{2}
}
\right) \\
&= \frac{1}{2 \pi \beta} \int dy^{\prime}
\exp \left(
- \frac{
(\beta^{2} + m^{2}) \left(
y^{\prime 2}
- \frac{
2myy^{\prime}
}{
\beta^{2} + m^{2}
}
+ \frac{
m^{2} y^{2}
}{
(\beta^{2} + m^{2})^{2}
}
- \frac{
m^{2} y^{2}
}{
(\beta^{2} + m^{2})^{2}
}
\right)
+ y^{2}
}{
2 \beta^{2}
}
\right) \\
&= \frac{1}{2 \pi \beta} \int dy^{\prime}
\exp \left(
- \frac{
(\beta^{2} + m^{2}) \left(
\left( y^{\prime} - \frac{my}{\beta^{2} + m^{2}} \right)^{2}
- \frac{
m^{2} y^{2}
}{
(\beta^{2} + m^{2})^{2}
}
\right)
+ y^{2}
}{
2 \beta^{2}
}
\right) \\
&= \frac{1}{2 \pi \beta} \int dy^{\prime}
\exp \left(
- \frac{
(\beta^{2} + m^{2}) \left( y^{\prime} - \frac{my}{\beta^{2} + m^{2}} \right)^{2}
- \frac{
m^{2} y^{2}
}{
\beta^{2} + m^{2}
}
+ y^{2}
}{
2 \beta^{2}
}
\right) \\
&= \frac{1}{2 \pi \beta}
\exp \left(
- \frac{
\frac{
m^{2} y^{2}
}{
\beta^{2} + m^{2}
}
- y^{2}
}{
2 \beta^{2}
}
\right)
\int dy^{\prime}
\exp \left(
- \frac{
(\beta^{2} + m^{2}) \left( y^{\prime} - \frac{my}{\beta^{2} + m^{2}} \right)^{2}
}{
2 \beta^{2}
}
\right) \\
&= \frac{1}{2 \pi \beta}
\exp \left(
- \frac{
\frac{
m^{2} y^{2} - (\beta^{2} + m^{2}) y^{2}
}{
\beta^{2} + m^{2}
}
}{
2 \beta^{2}
}
\right)
\sqrt{
\frac{
\pi
}{
\left(\frac{
\beta^{2} + m^{2}
}{
2 \beta^{2}
}\right)
}
}
\\
&= \frac{1}{2 \pi \beta}
\sqrt{
\frac{
2 \pi \beta^{2}
}{
\beta^{2} + m^{2}
}
}
\exp \left(
- \frac{
\frac{
\beta^{2} y^{2}
}{
\beta^{2} + m^{2}
}
}{
2 \beta^{2}
}
\right) \\
&= \frac{1}{\sqrt{2 \pi (\beta^{2} + m^{2})}}
\exp \left(
- \frac{
y^{2}
}{
2 (\beta^{2} + m^{2})
}
\right) \\
&= \mathcal{N}(y, 0, \sqrt{\beta^{2} + m^{2}}) (*)
\end{aligned}
$$
$\sqrt{\beta^2+m^2} = 1$ より、 $m = \sqrt{1-\beta^2}$
よって、
$T_{\pi}(y \mid y^{\prime} ; \beta)
:= \mathcal{N}(y, \sqrt{1 - \beta^{2}} y^{\prime}, \beta)
= \frac{1}{\sqrt{2 \pi \beta^{2}}} \exp \left(-\frac{(y-\sqrt{1 - \beta^{2}} y^{\prime})^2}{2\beta^{2}}\right)
$
次回(6/1)
- 連続だと思って計算しているのが、本当は離散じゃないと成り立たないみたいな状況を定量化できると面白いのでは?
- 松尾研で質問してみる @浅香先生 (6/10)
- Appendix Aの各定理をまとめる。(28) - (36)
- (28)を↑の積分チェックして完了
- $T_{\pi}$になんか条件があるかも (Tpi自体確率密度関数みたいな) ($p(0) = \pi$ とか)
- (次回:Appendix B. で、上記の計算を進める)
- $H_{q}(X^{(T)})$ を、定義に合わせる
- すると、Appendix B. (43) に着地
- Appendix A.を読む
- ↑ Lの下限が 2.4 (14) の形にすることで示せる
- [いつか] 離散で書き直してみる
- 関数解析に踏み込むとしたら
- [形状最適化問題](https://www.morikita.co.jp/books/mid/061461)
- vscodeでTexで書く方法に移植する
- ↑βが小さい時にforwardとreverseが等しくなる、はよくわからないので保留
- モデルの実装(on github)も見てみる [https://github.com/Sohl-Dickstein/Diffusion-Probabilistic-Models/blob/master/model.py](https://github.com/Sohl-Dickstein/Diffusion-Probabilistic-Models/blob/master/model.py)
- 一旦読み終えてみてから、参考文献見てみる?
- ガウス過程云々
---
$def.$
$0 \leq i \leq Tに対して \ X^{(i)} = \mathbb{R}^{n}$
$1 \leq i \leq Tに対して \ \beta_{i} \in \mathbb{R}$ : t-1とtの間の拡散率
$X^{(i\dots j)} := \prod_{t=i}^{j} X^{(t)}$
$\mathbf{x}^{(i\dots j)} := (\mathbf{x}^{(i)},\dots , \mathbf{x}^{(j)}) \in X^{(i\dots j)}$ と書く
## 2.1. Forward Trajectory
次回(7/20)
- $q^{(0)}$という実数値函数から、$q^{(0...T)}$まで定める
- $q^{(0)}$の条件は可測で全域積分すると1, $q^{(0...T)}$も満たしている(はず)
- -> 確率空間 $(\R^{T+1}, B(\R^{T+1}), Q^{(0...T)})$ が定まる
- $q^{(0...T)}$を周辺化(Tの軸で微分する)すると、$q^{(0...T-1)}$になることを確かめる
- 結合確率・条件付き確率・エントロピーなど、一通り、確率密度関数を使って定義し直す
$Q^{(0)}: \mathfrak{B}(X^{(0)})\to\mathbb{R}$: 確率測度
$q^{(0)}_{X_{abs}}:X^{(0)}\to\mathbb{R},\ Q^{(0)}$から定まる確率密度関数
$q^{(0\dots i)}_{X_{abs}}:X^{(0 \dots i)}\to\mathbb{R}$を
$$
q^{(0\dots i)}_{X_{abs}}(\mathbf{x}^{(0\dots i)})
:= q^{(0)}_{X_{abs}}\left(\mathbf{x}^{(0)}\right) \prod_{j=1}^i
\left(
T_{\pi}\left(\mathbf{x}^{(j)} \mid \mathbf{x}^{(j-1)};\beta_{j}\right)
\right)
$$
$q^{(T)}_{X_{abs}}:X^{(T)}\to\mathbb{R},\ q^{(T)}_{X_{abs}}(\mathbf{x}^{(T)}) := \int d \mathbf{y}^{(0 \dots T-1)} q^{(0\dots T)}_{X_{abs}}\left(\mathbf{y}^{(0\dots T-1)},\mathbf{x}^{(T)}\right)$
また、Radon-Nikodymの逆より、$q^{(0\dots i)}_{X_{abs}}, q^{(T)}_{X_{abs}}$からそれぞれ、
$Q^{(0...i)}: \mathfrak{B}(X^{(0 \dots i)})\to\mathbb{R}$: 確率測度
$Q^{(T)}: \mathfrak{B}(X^{(T)})\to\mathbb{R}$: 確率測度
が定まる。
##### 論文との対応
- $H_{q}(\mathbf{x}^{(t)}) = H_{entropy}(q^{(0\dots t)}_{X_{abs}})$
- $H_{q}(\mathbf{x}^{(t-1)} \mid \mathbf{x}^{(t)})
= -\int_{\mathbf{x}^{(0\dots t-1)}\in\prod_{j=0}^{t-1} X^{(j)}, \mathbf{x}^{(0\dots t)}\in\prod_{j=0}^{t} X^{(j)}} d\mathbf{x}^{(0\dots i)}dy \ p^{joint}_{(Y, X)_{abs}}(y, \mathbf{x}^{(0\dots i)}) \log \left(p^{condi}_{(Y,X)_{abs}}(y \mid \mathbf{x}^{(0\dots i)})\right)$
- $q\left(\mathbf{x}^{(j)} \mid \mathbf{x}^{(j-1)}\right) = T_{\pi}\left(\mathbf{x}^{(j)} \mid \mathbf{x}^{(j-1)};\beta_{j}\right)$
#### 公式1 (ガウス積分)
$
\int_{x}
\left(
\alpha
\cdot \exp \left(
-\gamma \cdot (x-\beta)^2
\right)
\right)
dx
= \alpha \cdot \sqrt{\frac{\pi}{\gamma}}
$
#### 公式2
$
\int_{x}
\left(
\alpha
\cdot
\left(
-\gamma \cdot (x-\beta)^2
\right)
\cdot
\exp \left(
-\gamma \cdot (x-\beta)^2
\right)
\right)
dx
= - \frac{\alpha}{2} \sqrt{\frac{\pi}{\gamma}}
$
###### 導出
$y := \sqrt{\gamma}(x - \beta) とおくと、dx = \frac{1}{\sqrt{\gamma}}dy より\\ $
$$
\begin{aligned}
\int_{y}&
\left(
\alpha
\cdot \exp \left(
-y^2
\right)
\cdot \left(
-y^2
\right)
\right)
\frac{1}{\sqrt{\gamma}}dy \\
&= - \frac{1}{\sqrt{\gamma}} \alpha \int_{y} y^2 \cdot \exp(-y^2) dy \\
&= - \frac{1}{\sqrt{\gamma}} \alpha \left( \frac{\sqrt{\pi}}{2} \right) \\
&= - \frac{\alpha}{2} \sqrt{\frac{\pi}{\gamma}}
\end{aligned}
$$
#### 定理
###### $Claim$ $H_{entropy}(q^{(0\dots t)}_{X_{abs}}) \geq H_{entropy}(q^{(0\dots t-1)}_{X_{abs}})$ (論文(28))
$$
T_\pi\left(\mathbf{x}^{(t)} \mid \mathbf{x}^{(t-1)} ; \beta_{t}\right)
:= \mathcal{N}(\mathbf{x}^{(t)}, \sqrt{1 - \beta_{t}^{2}} \mathbf{x}^{(t-1)}, \beta_{t})
= \frac{1}{
\sqrt{2 \pi \beta_{t}^{2}}
}
\exp \left(
-\frac{
(\mathbf{x}^{(t)}-\sqrt{1 - \beta_{t}^{2}} \mathbf{x}^{(t-1)})^2
}{
2\beta_{t}^{2}
}
\right)
$$
とするとき、$\beta_{t} \geq \sqrt{\frac{1}{2 \pi e}}$ ならば、
$$
H_{entropy}(q^{(0\dots t)}_{X_{abs}}) \geq H_{entropy}(q^{(0\dots t-1)}_{X_{abs}})
$$
が成り立つ。
###### $Proof$
$
q^{(0\dots t)}_{X_{abs}}(\mathbf{x}^{(0\dots t)})
=
q^{(0\dots t-1)}_{X_{abs}}(\mathbf{x}^{(0\dots t-1)})
\cdot
T_{\pi}\left(\mathbf{x}^{(t)} \mid \mathbf{x}^{(t-1)};\beta_{t}\right)
$
であるから、
$$
\begin{align*}
(右辺) = H_{entropy}(q^{(0\dots t-1)}_{X_{abs}})
= -\int_{\mathbf{x}^{(0\dots t-1)}\in\prod_{j=0}^{t-1} X^{(j)}} d\mathbf{x}^{(0\dots t-1)} q^{(0\dots t-1)}_{X_{abs}}(\mathbf{x}^{(0\dots t-1)}) \cdot \log \left( q^{(0\dots t-1)}_{X_{abs}}(\mathbf{x}^{(0\dots t-1)}) \right) \\
\end{align*}
$$
$$
\begin{align*}
(左辺) = H_{entropy}(q^{(0\dots t)}_{X_{abs}})
&= -\int_{\mathbf{x}^{(0\dots t)}\in\prod_{j=0}^{t} X^{(j)}} d\mathbf{x}^{(0\dots t)}
\left(
q^{(0\dots t)}_{X_{abs}}(\mathbf{x}^{(0\dots t)})
\cdot
\log \left(
q^{(0\dots t)}_{X_{abs}}(\mathbf{x}^{(0\dots t)})
\right)
\right) \\
&= -\int_{\mathbf{x}^{(0\dots t)}\in\prod_{j=0}^{t} X^{(j)}} d\mathbf{x}^{(0\dots t)}
\left(
q^{(0\dots t-1)}_{X_{abs}}(\mathbf{x}^{(0\dots t-1)})
\cdot
T_{\pi}\left(\mathbf{x}^{(t)} \mid \mathbf{x}^{(t-1)};\beta_{t}\right)
\cdot
\log \left(
q^{(0\dots t-1)}_{X_{abs}}(\mathbf{x}^{(0\dots t-1)})
\cdot
T_{\pi}\left(\mathbf{x}^{(t)} \mid \mathbf{x}^{(t-1)};\beta_{t}\right)
\right)
\right) \\
&= -\int_{\mathbf{x}^{(0\dots t)}\in\prod_{j=0}^{t} X^{(j)}} d\mathbf{x}^{(0\dots t)}
\left(
q^{(0\dots t-1)}_{X_{abs}}(\mathbf{x}^{(0\dots t-1)})
\cdot
T_{\pi}\left(\mathbf{x}^{(t)} \mid \mathbf{x}^{(t-1)};\beta_{t}\right)
\cdot
\left(
\log \left(
q^{(0\dots t-1)}_{X_{abs}}(\mathbf{x}^{(0\dots t-1)})
\right)
+
\log \left(
T_{\pi}\left(\mathbf{x}^{(t)} \mid \mathbf{x}^{(t-1)};\beta_{t}\right)
\right)
\right)
\right) \\
&= -\int_{\mathbf{x}^{(0\dots t)}\in\prod_{j=0}^{t} X^{(j)}} d\mathbf{x}^{(0\dots t)}
\left(
q^{(0\dots t-1)}_{X_{abs}}(\mathbf{x}^{(0\dots t-1)})
\cdot
T_{\pi}\left(\mathbf{x}^{(t)} \mid \mathbf{x}^{(t-1)};\beta_{t}\right)
\cdot
\left(
\log \left(
q^{(0\dots t-1)}_{X_{abs}}(\mathbf{x}^{(0\dots t-1)})
\right)
\right)
\right)
-\int_{\mathbf{x}^{(0\dots t)}\in\prod_{j=0}^{t} X^{(j)}} d\mathbf{x}^{(0\dots t)}
\left(
q^{(0\dots t-1)}_{X_{abs}}(\mathbf{x}^{(0\dots t-1)})
\cdot
T_{\pi}\left(\mathbf{x}^{(t)} \mid \mathbf{x}^{(t-1)};\beta_{t}\right)
\cdot
\left(
\log \left(
T_{\pi}\left(\mathbf{x}^{(t)} \mid \mathbf{x}^{(t-1)};\beta_{t}\right)
\right)
\right)
\right) \ \cdots \ (*) \\
\end{align*}
$$
---
項を分ける
$$
\begin{align*}
((*) \ 第1項)
&= -\int_{\mathbf{x}^{(0 \dots t-1)} \in \prod_{j=0}^{t-1} X^{(j)}} d\mathbf{x}^{(0 \dots t-1)}
\left(
\int_{\mathbf{x}^{(t)} \in X^{(t)}} d\mathbf{x}^{(t)}
\left(
q^{(0\dots t-1)}_{X_{abs}}(\mathbf{x}^{(0\dots t-1)})
\cdot
T_{\pi}\left(\mathbf{x}^{(t)} \mid \mathbf{x}^{(t-1)};\beta_{t}\right)
\cdot
\log \left(
q^{(0\dots t-1)}_{X_{abs}}(\mathbf{x}^{(0\dots t-1)})
\right)
\right)
\right) \\
&= -\int_{\mathbf{x}^{(0 \dots t-1)} \in \prod_{j=0}^{t-1} X^{(j)}} d\mathbf{x}^{(0 \dots t-1)}
\left(
\int_{\mathbf{x}^{(t)} \in X^{(t)}} d\mathbf{x}^{(t)}
\left(
q^{(0\dots t-1)}_{X_{abs}}(\mathbf{x}^{(0\dots t-1)})
\cdot
\log \left(
q^{(0\dots t-1)}_{X_{abs}}(\mathbf{x}^{(0\dots t-1)})
\right)
\cdot
T_{\pi}\left(\mathbf{x}^{(t)} \mid \mathbf{x}^{(t-1)};\beta_{t}\right)
\right)
\right) \\
&= -\int_{\mathbf{x}^{(0 \dots t-1)} \in \prod_{j=0}^{t-1} X^{(j)}} d\mathbf{x}^{(0 \dots t-1)}
\left(
q^{(0\dots t-1)}_{X_{abs}}(\mathbf{x}^{(0\dots t-1)})
\cdot
\log \left(
q^{(0\dots t-1)}_{X_{abs}}(\mathbf{x}^{(0\dots t-1)})
\right)
\cdot
\int_{\mathbf{x}^{(t)} \in X^{(t)}} d\mathbf{x}^{(t)}
\left(
T_{\pi}\left(\mathbf{x}^{(t)} \mid \mathbf{x}^{(t-1)};\beta_{t}\right)
\right)
\right) \\
&= -\int_{\mathbf{x}^{(0 \dots t-1)} \in \prod_{j=0}^{t-1} X^{(j)}} d\mathbf{x}^{(0 \dots t-1)}
\left(
q^{(0\dots t-1)}_{X_{abs}}(\mathbf{x}^{(0\dots t-1)})
\cdot
\log \left(
q^{(0\dots t-1)}_{X_{abs}}(\mathbf{x}^{(0\dots t-1)})
\right)
\cdot
\int_{\mathbf{x}^{(t)} \in X^{(t)}} d\mathbf{x}^{(t)}
\left(
\frac{1}{
\sqrt{2 \pi \beta_{t}^{2}}
}
\exp \left(
-\frac{
(\mathbf{x}^{(t)}-\sqrt{1 - \beta_{t}^{2}} \mathbf{x}^{(t-1)})^2
}{
2\beta_{t}^{2}
}
\right)
\right)
\right) \\
&= -\int_{\mathbf{x}^{(0 \dots t-1)} \in \prod_{j=0}^{t-1} X^{(j)}} d\mathbf{x}^{(0 \dots t-1)}
\left(
q^{(0\dots t-1)}_{X_{abs}}(\mathbf{x}^{(0\dots t-1)})
\cdot
\log \left(
q^{(0\dots t-1)}_{X_{abs}}(\mathbf{x}^{(0\dots t-1)})
\right)
\cdot
\int_{\mathbf{x}^{(t)} \in X^{(t)}} d\mathbf{x}^{(t)}
\left(
\underbrace{
\frac{1}{
\sqrt{2 \pi \beta_{t}^{2}}
}
}_{\alpha}
\exp \left(
-\underbrace{
\frac{
1
}{
2\beta_{t}^{2}
}
}_{\gamma}
\cdot
\left(
\mathbf{x}^{(t)}-\sqrt{1 - \beta_{t}^{2}} \mathbf{x}^{(t-1)}
\right)^2
\right)
\right)
\right) \\
&= -\int_{\mathbf{x}^{(0 \dots t-1)} \in \prod_{j=0}^{t-1} X^{(j)}} d\mathbf{x}^{(0 \dots t-1)}
\left(
q^{(0\dots t-1)}_{X_{abs}}(\mathbf{x}^{(0\dots t-1)})
\cdot
\log \left(
q^{(0\dots t-1)}_{X_{abs}}(\mathbf{x}^{(0\dots t-1)})
\right)
\cdot
\underbrace{
\left(
\frac{1}{
\sqrt{2 \pi \beta_{t}^{2}}
}
\cdot
\sqrt{
\frac{
\pi
}{
\frac{
1
}{
2\beta_{t}^{2}
}
}
}
\right)
}_{1}
\right) \\
&= -\int_{\mathbf{x}^{(0 \dots t-1)} \in \prod_{j=0}^{t-1} X^{(j)}} d\mathbf{x}^{(0 \dots t-1)}
\left(
q^{(0\dots t-1)}_{X_{abs}}(\mathbf{x}^{(0\dots t-1)})
\cdot
\log \left(
q^{(0\dots t-1)}_{X_{abs}}(\mathbf{x}^{(0\dots t-1)})
\right)
\right) \\
&= (右辺)
\end{align*}
$$
$$
\begin{align*}
((*) \ 第2項)
&= -\int_{\mathbf{x}^{(0\dots t)}\in\prod_{j=0}^{t} X^{(j)}} d\mathbf{x}^{(0\dots t)}
\left(
q^{(0\dots t-1)}_{X_{abs}}(\mathbf{x}^{(0\dots t-1)})
\cdot
T_{\pi}\left(\mathbf{x}^{(t)} \mid \mathbf{x}^{(t-1)};\beta_{t}\right)
\cdot
\left(
\log \left(
T_{\pi}\left(\mathbf{x}^{(t)} \mid \mathbf{x}^{(t-1)};\beta_{t}\right)
\right)
\right)
\right) \\
&= -\int_{\mathbf{x}^{(0\dots t-1)}\in\prod_{j=0}^{t-1} X^{(j)}} d\mathbf{x}^{(0\dots t-1)}
\left(
\int_{\mathbf{x}^{(t)} \in X^{(t)}} d\mathbf{x}^{(t)}
\left(
q^{(0\dots t-1)}_{X_{abs}}(\mathbf{x}^{(0\dots t-1)})
\cdot
T_{\pi}\left(\mathbf{x}^{(t)} \mid \mathbf{x}^{(t-1)};\beta_{t}\right)
\cdot
\left(
\log \left(
T_{\pi}\left(\mathbf{x}^{(t)} \mid \mathbf{x}^{(t-1)};\beta_{t}\right)
\right)
\right)
\right)
\right) \\
&= -\int_{\mathbf{x}^{(0\dots t-1)}\in\prod_{j=0}^{t-1} X^{(j)}} d\mathbf{x}^{(0\dots t-1)}
\left(
q^{(0\dots t-1)}_{X_{abs}}(\mathbf{x}^{(0\dots t-1)})
\cdot
\int_{\mathbf{x}^{(t)} \in X^{(t)}} d\mathbf{x}^{(t)}
\left(
T_{\pi}\left(\mathbf{x}^{(t)} \mid \mathbf{x}^{(t-1)};\beta_{t}\right)
\cdot
\left(
\log \left(
T_{\pi}\left(\mathbf{x}^{(t)} \mid \mathbf{x}^{(t-1)};\beta_{t}\right)
\right)
\right)
\right)
\right) \\
&= -\int_{\mathbf{x}^{(0\dots t-1)}\in\prod_{j=0}^{t-1} X^{(j)}} d\mathbf{x}^{(0\dots t-1)}
\left(
q^{(0\dots t-1)}_{X_{abs}}(\mathbf{x}^{(0\dots t-1)})
\cdot
\int_{\mathbf{x}^{(t)} \in X^{(t)}} d\mathbf{x}^{(t)}
\left(
\frac{1}{
\sqrt{2 \pi \beta_{t}^{2}}
}
\exp \left(
-\frac{
(\mathbf{x}^{(t)}-\sqrt{1 - \beta_{t}^{2}} \mathbf{x}^{(t-1)})^2
}{
2\beta_{t}^{2}
}
\right)
\cdot
\left(
\log \left(
\frac{1}{
\sqrt{2 \pi \beta_{t}^{2}}
}
\exp \left(
-\frac{
(\mathbf{x}^{(t)}-\sqrt{1 - \beta_{t}^{2}} \mathbf{x}^{(t-1)})^2
}{
2\beta_{t}^{2}
}
\right)
\right)
\right)
\right)
\right) \\
&= -\int_{\mathbf{x}^{(0\dots t-1)}\in\prod_{j=0}^{t-1} X^{(j)}} d\mathbf{x}^{(0\dots t-1)}
\left(
q^{(0\dots t-1)}_{X_{abs}}(\mathbf{x}^{(0\dots t-1)})
\cdot
\int_{\mathbf{x}^{(t)} \in X^{(t)}} d\mathbf{x}^{(t)}
\left(
\frac{1}{
\sqrt{2 \pi \beta_{t}^{2}}
}
\exp \left(
-\frac{
(\mathbf{x}^{(t)}-\sqrt{1 - \beta_{t}^{2}} \mathbf{x}^{(t-1)})^2
}{
2\beta_{t}^{2}
}
\right)
\cdot
\left(
\log \left(
\frac{1}{
\sqrt{2 \pi \beta_{t}^{2}}
}
\right)
+
\log \left(
\exp \left(
-\frac{
(\mathbf{x}^{(t)}-\sqrt{1 - \beta_{t}^{2}} \mathbf{x}^{(t-1)})^2
}{
2\beta_{t}^{2}
}
\right)
\right)
\right)
\right)
\right) \\
&= -\int_{\mathbf{x}^{(0\dots t-1)}\in\prod_{j=0}^{t-1} X^{(j)}} d\mathbf{x}^{(0\dots t-1)}
\left(
q^{(0\dots t-1)}_{X_{abs}}(\mathbf{x}^{(0\dots t-1)})
\cdot
\int_{\mathbf{x}^{(t)} \in X^{(t)}} d\mathbf{x}^{(t)}
\left(
\frac{1}{
\sqrt{2 \pi \beta_{t}^{2}}
}
\exp \left(
-\frac{
(\mathbf{x}^{(t)}-\sqrt{1 - \beta_{t}^{2}} \mathbf{x}^{(t-1)})^2
}{
2\beta_{t}^{2}
}
\right)
\cdot
\left(
\log \left(
\frac{1}{
\sqrt{2 \pi \beta_{t}^{2}}
}
\right)
+
\left(
-\frac{
(\mathbf{x}^{(t)}-\sqrt{1 - \beta_{t}^{2}} \mathbf{x}^{(t-1)})^2
}{
2\beta_{t}^{2}
}
\right)
\right)
\right)
\right) \\
&= -\int_{\mathbf{x}^{(0\dots t-1)}\in\prod_{j=0}^{t-1} X^{(j)}} d\mathbf{x}^{(0\dots t-1)}
\left(
q^{(0\dots t-1)}_{X_{abs}}(\mathbf{x}^{(0\dots t-1)})
\cdot
\int_{\mathbf{x}^{(t)} \in X^{(t)}} d\mathbf{x}^{(t)}
\left(
\frac{1}{
\sqrt{2 \pi \beta_{t}^{2}}
}
\exp \left(
-\frac{
(\mathbf{x}^{(t)}-\sqrt{1 - \beta_{t}^{2}} \mathbf{x}^{(t-1)})^2
}{
2\beta_{t}^{2}
}
\right)
\cdot
\left(
\log \left(
\frac{1}{
\sqrt{2 \pi \beta_{t}^{2}}
}
\right)
+
\left(
-\frac{
(\mathbf{x}^{(t)}-\sqrt{1 - \beta_{t}^{2}} \mathbf{x}^{(t-1)})^2
}{
2\beta_{t}^{2}
}
\right)
\right)
\right)
\right) \\
&= -\int_{\mathbf{x}^{(0\dots t-1)}\in\prod_{j=0}^{t-1} X^{(j)}} d\mathbf{x}^{(0\dots t-1)}
\left(
q^{(0\dots t-1)}_{X_{abs}}(\mathbf{x}^{(0\dots t-1)})
\cdot
\left(
\int_{\mathbf{x}^{(t)} \in X^{(t)}} d\mathbf{x}^{(t)}
\left(
\frac{1}{
\sqrt{2 \pi \beta_{t}^{2}}
}
\exp \left(
-\frac{
(\mathbf{x}^{(t)}-\sqrt{1 - \beta_{t}^{2}} \mathbf{x}^{(t-1)})^2
}{
2\beta_{t}^{2}
}
\right)
\cdot
\log \left(
\frac{1}{
\sqrt{2 \pi \beta_{t}^{2}}
}
\right)
\right)
+
\int_{\mathbf{x}^{(t)} \in X^{(t)}} d\mathbf{x}^{(t)}
\left(
\frac{1}{
\sqrt{2 \pi \beta_{t}^{2}}
}
\exp \left(
-\frac{
(\mathbf{x}^{(t)}-\sqrt{1 - \beta_{t}^{2}} \mathbf{x}^{(t-1)})^2
}{
2\beta_{t}^{2}
}
\right)
\cdot
\left(
-\frac{
(\mathbf{x}^{(t)}-\sqrt{1 - \beta_{t}^{2}} \mathbf{x}^{(t-1)})^2
}{
2\beta_{t}^{2}
}
\right)
\right)
\right)
\right) \\
&= -\int_{\mathbf{x}^{(0\dots t-1)}\in\prod_{j=0}^{t-1} X^{(j)}} d\mathbf{x}^{(0\dots t-1)}
\left(
q^{(0\dots t-1)}_{X_{abs}}(\mathbf{x}^{(0\dots t-1)})
\cdot
\left(
\cancel{
\frac{1}{
\sqrt{2 \pi \beta_{t}^{2}}
}
}
\cdot
\log \left(
\frac{1}{
\sqrt{2 \pi \beta_{t}^{2}}
}
\right)
\cdot
\underbrace{
\int_{\mathbf{x}^{(t)} \in X^{(t)}} d\mathbf{x}^{(t)}
\left(
\exp \left(
-\frac{
(\mathbf{x}^{(t)}-\sqrt{1 - \beta_{t}^{2}} \mathbf{x}^{(t-1)})^2
}{
2\beta_{t}^{2}
}
\right)
\right)
}_{
\cancel{ \sqrt{2 \pi \beta_{t}^{2}} } \ (\because ガウス積分)
}
+
\cancel{
\frac{1}{
\sqrt{2 \pi \beta_{t}^{2}}
}
}
\cdot
\underbrace{
\int_{\mathbf{x}^{(t)} \in X^{(t)}} d\mathbf{x}^{(t)}
\left(
\exp \left(
-\frac{
(\mathbf{x}^{(t)}-\sqrt{1 - \beta_{t}^{2}} \mathbf{x}^{(t-1)})^2
}{
2\beta_{t}^{2}
}
\right)
\cdot
\left(
-\frac{
(\mathbf{x}^{(t)}-\sqrt{1 - \beta_{t}^{2}} \mathbf{x}^{(t-1)})^2
}{
2\beta_{t}^{2}
}
\right)
\right)
}_{
- \frac{1}{2} \cdot \cancel{ \sqrt{2 \pi \beta_{t}^{2}} } \ (\because 公式2)
}
\right)
\right) \\
&= -\int_{\mathbf{x}^{(0\dots t-1)}\in\prod_{j=0}^{t-1} X^{(j)}} d\mathbf{x}^{(0\dots t-1)}
\left(
q^{(0\dots t-1)}_{X_{abs}}(\mathbf{x}^{(0\dots t-1)})
\cdot
\left(
\log \left(
\frac{1}{
\sqrt{2 \pi \beta_{t}^{2}}
}
\right)
-
\frac{1}{2}
\right)
\right) \\
&=
-\left(
\log \left(
\frac{1}{
\sqrt{2 \pi \beta_{t}^{2}}
}
\right)
-
\frac{1}{2}
\right)
\cdot
\underbrace{
\int_{\mathbf{x}^{(0\dots t-1)}\in\prod_{j=0}^{t-1} X^{(j)}} d\mathbf{x}^{(0\dots t-1)}
\left(
q^{(0\dots t-1)}_{X_{abs}}(\mathbf{x}^{(0\dots t-1)})
\right)
}_{1} \\
&=
-\left(
\log \left(
\frac{1}{
\sqrt{2 \pi \beta_{t}^{2}}
}
\right)
-
\frac{1}{2}
\right) \\
&=
\frac{1}{2}
+
\frac{1}{2}
\cdot
\log \left(
2 \pi \beta_{t}^{2}
\right) \\
&=
\frac{1}{2}
\cdot
\left(
1
+
\log \left(
2 \pi \beta_{t}^{2}
\right)
\right) \\
\end{align*}
$$
よって、
$$
\begin{align*}
\end{align*}
$$
$$
\begin{align*}
(左辺) - (右辺)
&=
\left(
(右辺)
-
\frac{1}{2}
\left(
1
+
\log \left(
2 \pi \beta_{t}^{2}
\right)
\right)
\right)
-
(右辺) \\
&=
\frac{1}{2}
\left(
1
+
\log \left(
2 \pi \beta_{t}^{2}
\right)
\right)
\end{align*}
$$
ゆえに、$\beta_{t} \geq \sqrt{\frac{1}{2 \pi e}}$ の時、不等式が成り立つ
$Q.E.D.$
めも(次回): ここからtypst変換続ける
-----
$Claim$ 付録A (30)
$H_q\left(\mathbf{X}^{(t-1)} \mid \mathbf{X}^{(t)}\right) \leq H_q\left(\mathbf{X}^{(t)} \mid \mathbf{X}^{(t-1)}\right)$
$
H^{}_{condi}= -\int_{x \in X, y \in Y} dxdy \ p^{joint}_{(Y, X)_{abs}}(y, x) \log \left(p^{condi}_{(Y,X)_{abs}}(y \mid x)\right) \\
$
(次回 6/29)
- ↑を$q^{0...i}$を使って書く
- この時、$q^{0...i}$ は joint としてしまえることがわかったので、p^{joint}のところにq^{0...i}をそのまま入れる
- 型は、$p^{condi}_{(\mathbf{X}^{(t-1)}, \mathbf{X}^{(t)})_{abs}}(\cdot\mid\cdot):\mathbb{R}^{t}\times\mathbb{R}^{t-1} \to \mathbb{R}_{\geq 0}$ みたいになるはず。(ちゃんとやる)
$\beta_{t} \geq \sqrt{\frac{1}{2 \pi e}}$ のとき、
$$
$$
(次回 6/22)
### メモ
##### 大きな方針
- とりあえずこの本の理論は最後まで追ってみる
- 有限と捉えてちゃんと書いてみた時に、自然と$\sqrt{1-\beta}$が出てきてくれないか
- 代数的確率空間的に書いてみるとどうなるか
- 各ステップのエントロピー差が有界であることを示している
- (30)で上界がわかる
- ゴールは (36)
## 2.2. Reverse Trajectory
$p^{(T)}_{X_{abs}}:X^{(T)}\to\mathbb{R},\ p^{(T)}_{X_{abs}}(\mathbf{x}^{(T)}) := \pi(\mathbf{x}^{(T)})$
$p^{(i\dots T)}_{X_{abs}}:\prod_{t=i}^{T} X^{(t)}\to\mathbb{R}$
$$
p^{(i\dots T)}_{X_{abs}}(\mathbf{x}^{(i\dots T)}) := p^{(T)}_{X_{abs}}\left(\mathbf{x}^{(T)}\right) \prod_{t=1}^T T_{\pi}\left(\mathbf{x}^{(t-1)} \mid \mathbf{x}^{(t)};\beta_{t}\right)
$$
##### 論文との対応
- $p\left(\mathbf{x}^{(j-1)} \mid \mathbf{x}^{(j)}\right) = T_{\pi}\left(\mathbf{x}^{(j-1)} \mid \mathbf{x}^{(j)};\beta_{j}\right)$
## 2.3. Model Probability
> これは、統計物理学における準静的過程の場合に相当する
- [非平衡科学](https://sosuke110.com/noneq-phys.pdf)
- [機械学習のための確率過程](https://www.amazon.co.jp/%E6%A9%9F%E6%A2%B0%E5%AD%A6%E7%BF%92%E3%81%AE%E3%81%9F%E3%82%81%E3%81%AE%E7%A2%BA%E7%8E%87%E9%81%8E%E7%A8%8B%E5%85%A5%E9%96%80-%E2%80%95%E7%A2%BA%E7%8E%87%E5%BE%AE%E5%88%86%E6%96%B9%E7%A8%8B%E5%BC%8F%E3%81%8B%E3%82%89%E3%83%99%E3%82%A4%E3%82%BA%E3%83%A2%E3%83%87%E3%83%AB-%E6%8B%A1%E6%95%A3%E3%83%A2%E3%83%87%E3%83%AB%E3%81%BE%E3%81%A7%E2%80%95-%E5%86%85%E5%B1%B1%E7%A5%90%E4%BB%8B-ebook/dp/B0CK176SH5/ref=tmm_kin_swatch_0?_encoding=UTF8&qid=&sr=)
$p^{(0)}_{X_{abs}}:X^{(0)}\to\mathbb{R},\ p^{(0)}_{X_{abs}}(\mathbf{x}^{(0)}) := \int d \mathbf{y}^{(1 \dots T)} p^{(0\dots T)}_{X_{abs}}\left(\mathbf{x}^{(0)},\mathbf{y}^{(1 \dots T)}\right)$
$= \int d \mathbf{y}^{(1 \dots T)} p^{(0\dots T)}_{X_{abs}}\left(\mathbf{x}^{(0)},\mathbf{y}^{(1 \dots T)}\right)\frac{q^{(0\dots T)}_{X_{abs}}\left(\mathbf{x}^{(0)},\mathbf{y}^{(1 \dots T)}\right)}{q^{(0\dots T)}_{X_{abs}}\left(\mathbf{x}^{(0)},\mathbf{y}^{(1 \dots T)}\right)}$
$= \int d \mathbf{y}^{(1 \dots T)}q^{(0\dots T)}_{X_{abs}}\left(\mathbf{x}^{(0)},\mathbf{y}^{(1 \dots T)}\right) \frac{p^{(0\dots T)}_{X_{abs}}\left(\mathbf{x}^{(0)},\mathbf{y}^{(1 \dots T)}\right)}{q^{(0\dots T)}_{X_{abs}}\left(\mathbf{x}^{(0)},\mathbf{y}^{(1 \dots T)}\right)}$
$= \int d \mathbf{y}^{(1 \dots T)}q^{(0\dots T)}_{X_{abs}}\left(\mathbf{x}^{(0)},\mathbf{y}^{(1 \dots T)}\right) \cdot \frac{p^{(T)}_{X_{abs}}\left(\mathbf{y}^{(T)}\right)}{q^{(0)}_{X_{abs}}\left(\mathbf{x}^{(0)}\right)} \cdot \frac{T_{\pi}\left(\mathbf{x}^{(0)} \mid \mathbf{y}^{(1)};\beta_{t}\right)}{T_{\pi}\left(\mathbf{y}^{(1)} \mid \mathbf{x}^{(0)};\beta_{t}\right)} \cdot \left( \prod_{t=2}^T \frac{ T_{\pi}\left(\mathbf{y}^{(t-1)} \mid \mathbf{y}^{(t)};\beta_{t}\right)}{T_{\pi}\left(\mathbf{y}^{(t)} \mid \mathbf{y}^{(t-1)};\beta_{t}\right)} \right)$
この積分は、複数のサンプル $q^{(0\dots T)}_{X_{abs}}\left(\mathbf{x}^{(0)},\mathbf{y}^{(1 \dots T)}\right) $ の平均を取ることで、素早く評価できる。
$\beta_{t}$が無限小のとき、$\frac{T_{\pi}\left(\mathbf{x}^{(0)} \mid \mathbf{y}^{(1)};\beta_{t}\right)}{T_{\pi}\left(\mathbf{y}^{(1)} \mid \mathbf{x}^{(0)};\beta_{t}\right)} \cdot \left( \prod_{t=2}^T \frac{ T_{\pi}\left(\mathbf{y}^{(t-1)} \mid \mathbf{y}^{(t)};\beta_{t}\right)}{T_{\pi}\left(\mathbf{y}^{(t)} \mid \mathbf{y}^{(t-1)};\beta_{t}\right)} \right)=1$となる。
- つまり、$1 \leq t \leq T$について、$T_{\pi}\left(\mathbf{x}^{(t-1)} \mid \mathbf{y}^{(t)};\beta_{t}\right) = T_{\pi}\left(\mathbf{x}^{(t)} \mid \mathbf{y}^{(t-1)};\beta_{t}\right)$ ← ?
- このとき、上記の積分を評価するのに要求されるのは、単一のサンプル$q^{(0\dots T)}_{X_{abs}}\left(\mathbf{x}^{(0)},\mathbf{y}^{(1 \dots T)}\right) $のみである
> 💡 $q^{(0)}_{x_{i}}:$ 与えられた真のデータの分布
> $ p^{(0)}_{x_{i}}: $ Reverse trajectoryを用いて計算された、$q^{(0)}_{x_{i}}$の近似
以下で、$p^{(0)}_{x_{i}}, q^{(0)}_{x_{i}}$のCross Entropyを最小化する
## 2.4. Training
$H(p^{(0)}_{X_{abs}}, q^{(0)}_{X_{abs}}):$ Cross Entropy
$H(p^{(0)}_{X_{abs}}, q^{(0)}_{X_{abs}})
=-\int_{X^{(0)}} d \mathbf{y}^{(0)} \ q_{X_{abs}}^{(0)}\left(\mathbf{y}^{(0)}\right) \cdot \log p_{X_{abs}}^{(0)}\left(\mathbf{y}^{(0)}\right)$
$=-\int_{X^{(0)}} d \mathbf{y}^{(0)} \ q_{X_{abs}}^{(0)}\left(\mathbf{y}^{(0)}\right)\cdot \log \left[\int d \mathbf{y}^{(1 \dots T)}q^{(0\dots T)}_{X_{abs}}\left(\mathbf{y}^{(0)},\mathbf{y}^{(1 \dots T)}\right) \cdot \frac{p^{(T)}_{X_{abs}}\left(\mathbf{y}^{(T)}\right)}{q^{(0)}_{X_{abs}}\left(\mathbf{y}^{(0)}\right)} \cdot \frac{T_{\pi}\left(\mathbf{y}^{(0)} \mid \mathbf{y}^{(1)};\beta_{t}\right)}{T_{\pi}\left(\mathbf{y}^{(1)} \mid \mathbf{y}^{(0)};\beta_{t}\right)} \cdot \left( \prod_{t=2}^T \frac{ T_{\pi}\left(\mathbf{y}^{(t-1)} \mid \mathbf{y}^{(t)};\beta_{t}\right)}{T_{\pi}\left(\mathbf{y}^{(t)} \mid \mathbf{y}^{(t-1)};\beta_{t}\right)} \right)\right]
$
$=-\int_{X^{(0)}} d \mathbf{y}^{(0)} \ q_{X_{abs}}^{(0)}\left(\mathbf{y}^{(0)}\right) \cdot \log \left[\int d \mathbf{y}^{(1 \dots T)} \ \frac{q^{(0\dots T)}_{X_{abs}}\left(\mathbf{y}^{(0)},\mathbf{y}^{(1 \dots T)}\right)}{q^{(0)}_{X_{abs}}\left(\mathbf{y}^{(0)}\right)} \cdot p^{(T)}_{X_{abs}}\left(\mathbf{y}^{(T)}\right) \cdot \frac{T_{\pi}\left(\mathbf{y}^{(0)} \mid \mathbf{y}^{(1)};\beta_{t}\right)}{T_{\pi}\left(\mathbf{y}^{(1)} \mid \mathbf{y}^{(0)};\beta_{t}\right)} \cdot \left( \prod_{t=2}^T \frac{ T_{\pi}\left(\mathbf{y}^{(t-1)} \mid \mathbf{y}^{(t)};\beta_{t}\right)}{T_{\pi}\left(\mathbf{y}^{(t)} \mid \mathbf{y}^{(t-1)};\beta_{t}\right)} \right)\right]
$
$\geq -\int_{X^{(0)}} d \mathbf{y}^{(0)} q_{X_{abs}}^{(0)}\left(\mathbf{y}^{(0)}\right) \cdot \left( \int_{X^{(1 \dots T)}} d \mathbf{y}^{(1 \dots T)} \cdot \frac{q^{(0\dots T)}_{X_{abs}}\left(\mathbf{y}^{(0)},\mathbf{y}^{(1 \dots T)}\right)}{q^{(0)}_{X_{abs}}\left(\mathbf{y}^{(0)}\right)} \cdot \log \left[p^{(T)}_{X_{abs}}\left(\mathbf{y}^{(T)}\right) \cdot \frac{T_{\pi}\left(\mathbf{y}^{(0)} \mid \mathbf{y}^{(1)};\beta_{t}\right)}{T_{\pi}\left(\mathbf{y}^{(1)} \mid \mathbf{y}^{(0)};\beta_{t}\right)} \cdot \left( \prod_{t=2}^T \frac{ T_{\pi}\left(\mathbf{y}^{(t-1)} \mid \mathbf{y}^{(t)};\beta_{t}\right)}{T_{\pi}\left(\mathbf{y}^{(t)} \mid \mathbf{y}^{(t-1)};\beta_{t}\right)} \right)\right]\right)$(∵ [Jensenの不等式](https://ja.wikipedia.org/wiki/%E3%82%A4%E3%82%A7%E3%83%B3%E3%82%BB%E3%83%B3%E3%81%AE%E4%B8%8D%E7%AD%89%E5%BC%8F), $\int_{X^{(1...T)}} \frac{q^{(0\dots T)}_{X_{abs}}\left(\mathbf{y}^{(0)},\mathbf{y}^{(1 \dots T)}\right)}{q^{(0)}_{X_{abs}}\left(\mathbf{y}^{(0)}\right)} = 1$ (∵ 周辺分布の定義) )
$= -\int_{X^{(0)}} d \mathbf{y}^{(0)} \left( \int_{X^{(1 \dots T)}} d \mathbf{y}^{(1 \dots T)} \ q_{X_{abs}}^{(0)}\left(\mathbf{y}^{(0)}\right) \cdot \frac{q^{(0\dots T)}_{X_{abs}}\left(\mathbf{y}^{(0)},\mathbf{y}^{(1 \dots T)}\right)}{q^{(0)}_{X_{abs}}\left(\mathbf{y}^{(0)}\right)} \cdot \log \left[p^{(T)}_{X_{abs}}\left(\mathbf{y}^{(T)}\right) \cdot \frac{T_{\pi}\left(\mathbf{y}^{(0)} \mid \mathbf{y}^{(1)};\beta_{t}\right)}{T_{\pi}\left(\mathbf{y}^{(1)} \mid \mathbf{y}^{(0)};\beta_{t}\right)} \cdot \left( \prod_{t=2}^T \frac{ T_{\pi}\left(\mathbf{y}^{(t-1)} \mid \mathbf{y}^{(t)};\beta_{t}\right)}{T_{\pi}\left(\mathbf{y}^{(t)} \mid \mathbf{y}^{(t-1)};\beta_{t}\right)} \right)\right]\right)$(∵ $q_{X_{abs}}^{(0)}\left(\mathbf{y}^{(0)}\right)$は$\int_{X^{(1...T)}}$において定数)
$= - \int_{X^{(0 \dots T)}} d\mathbf{y}^{(0 \dots T)} \ q^{(0\dots T)}_{X_{abs}}\left(\mathbf{y}^{(0 \dots T)}\right) \cdot \log \left[p^{(T)}_{X_{abs}}\left(\mathbf{y}^{(T)}\right) \cdot \left( \prod_{t=1}^T \frac{ T_{\pi}\left(\mathbf{y}^{(t-1)} \mid \mathbf{y}^{(t)};\beta_{t}\right)}{T_{\pi}\left(\mathbf{y}^{(t)} \mid \mathbf{y}^{(t-1)};\beta_{t}\right)} \right)\right]$
(∵ 約分 & 重積分はまとめられる & 細かい略記) (Appendix B. (38) と一致)
$=- \int_{X^{(0 \dots T)}} d\mathbf{y}^{(0 \dots T)} \ q^{(0\dots T)}_{X_{abs}}\left(\mathbf{y}^{(0)},\mathbf{y}^{(1 \dots T)}\right) \cdot \left( \log \left[p^{(T)}_{X_{abs}}\left(\mathbf{y}^{(T)}\right) \right] + \sum_{t=1}^T \left(\log \left[ \frac{ T_{\pi}\left(\mathbf{y}^{(t-1)} \mid \mathbf{y}^{(t)};\beta_{t}\right)}{T_{\pi}\left(\mathbf{y}^{(t)} \mid \mathbf{y}^{(t-1)};\beta_{t}\right)} \right]\right) \right)
$ (1) (∵ logを和に分解)
$=- \int_{X^{(0 \dots T)}} d\mathbf{y}^{(0 \dots T)} \ q^{(0\dots T)}_{X_{abs}}\left(\mathbf{y}^{(0)},\mathbf{y}^{(1 \dots T)}\right) \cdot
\left(
\sum_{t=1}^T \left(\log \left[ \frac{ T_{\pi}\left(\mathbf{y}^{(t-1)} \mid \mathbf{y}^{(t)};\beta_{t}\right)}{T_{\pi}\left(\mathbf{y}^{(t)} \mid \mathbf{y}^{(t-1)};\beta_{t}\right)} \right]\right)
+ \log \left[p^{(T)}_{X_{abs}}\left(\mathbf{y}^{(T)}\right) \right]
\right)
$ (1.5) (∵ 括弧内の和の順序入れ替え)
$=- \int_{X^{(0 \dots T)}} \left( \ q^{(0\dots T)}_{X_{abs}}\left(\mathbf{y}^{(0)},\mathbf{y}^{(1 \dots T)}\right) \cdot \sum_{t=1}^T \left(\log \left[ \frac{ T_{\pi}\left(\mathbf{y}^{(t-1)} \mid \mathbf{y}^{(t)};\beta_{t}\right)}{T_{\pi}\left(\mathbf{y}^{(t)} \mid \mathbf{y}^{(t-1)};\beta_{t}\right)} \right] \right) \right) d\mathbf{y}^{(0 \dots T)} - \int_{X^{(0 \dots T)}} \left( \ q^{(0\dots T)}_{X_{abs}}\left(\mathbf{y}^{(0)},\mathbf{y}^{(1 \dots T)} \right) \cdot \log \left[p^{(T)}_{X_{abs}}\left(\mathbf{y}^{(T)}\right) \right] \right) d\mathbf{y}^{(0 \dots T)} $ (2) (∵ 積分を和で分解)
$=- \int_{X^{(0 \dots T)}} \left( \ q^{(0\dots T)}_{X_{abs}}\left(\mathbf{y}^{(0)},\mathbf{y}^{(1 \dots T)}\right) \cdot \sum_{t=1}^T \left(\log \left[ \frac{ T_{\pi}\left(\mathbf{y}^{(t-1)} \mid \mathbf{y}^{(t)};\beta_{t}\right)}{T_{\pi}\left(\mathbf{y}^{(t)} \mid \mathbf{y}^{(t-1)};\beta_{t}\right)} \right] \right) \right) d\mathbf{y}^{(0 \dots T)} - \int_{X^{(T)}} \int_{X^{(0 \dots T-1)}} \left( \ q^{(0\dots T)}_{X_{abs}}\left(\mathbf{y}^{(0)},\mathbf{y}^{(1 \dots T)} \right) \cdot \log \left[p^{(T)}_{X_{abs}}\left(\mathbf{y}^{(T)}\right) \right] \right) d\mathbf{y}^{(0 \dots T)} $ (2.5) (∵ 第二項の積分を被積分変数で分解)
$=- \int_{X^{(0 \dots T)}} \left( \ q^{(0\dots T)}_{X_{abs}}\left(\mathbf{y}^{(0)},\mathbf{y}^{(1 \dots T)}\right) \cdot \sum_{t=1}^T \left(\log \left[ \frac{ T_{\pi}\left(\mathbf{y}^{(t-1)} \mid \mathbf{y}^{(t)};\beta_{t}\right)}{T_{\pi}\left(\mathbf{y}^{(t)} \mid \mathbf{y}^{(t-1)};\beta_{t}\right)} \right] \right) \right) d\mathbf{y}^{(0 \dots T)} - \int_{X^{(T)}} \left( \ q_{X_{abs}}^{(T)}\left(\mathbf{y}^{(T)}\right) \cdot \log \left[p^{(T)}_{X_{abs}}\left(\mathbf{y}^{(T)}\right) \right] \right) d\mathbf{y}^{(T)} $ (3) (∵ 第二項の内側の積分実行)
$
= - \int_{X^{(0 \dots T)}} d\mathbf{y}^{(0 \dots T)} \ q^{(0\dots T)}_{X_{abs}}\left(\mathbf{y}^{(0)},\mathbf{y}^{(1 \dots T)}\right) \cdot \sum_{t=1}^T \left(\log \left[ \frac{ T_{\pi}\left(\mathbf{y}^{(t-1)} \mid \mathbf{y}^{(t)};\beta_{t}\right)}{T_{\pi}\left(\mathbf{y}^{(t)} \mid \mathbf{y}^{(t-1)};\beta_{t}\right)} \right] \right) - H_{cross}\left(q^{(T)}_{X_{abs}}, p^{(T)}_{X_{abs}}\right)
$ (4)
$
= - \int_{X^{(0 \dots T)}} d\mathbf{y}^{(0 \dots T)} \ q^{(0\dots T)}_{X_{abs}}\left(\mathbf{y}^{(0)},\mathbf{y}^{(1 \dots T)}\right) \cdot \sum_{t=1}^T \left(\log \left[ \frac{ T_{\pi}\left(\mathbf{y}^{(t-1)} \mid \mathbf{y}^{(t)};\beta_{t}\right)}{T_{\pi}\left(\mathbf{y}^{(t)} \mid \mathbf{y}^{(t-1)};\beta_{t}\right)} \right] \right) - H_{cross}\left(q^{(T)}_{X_{abs}}, p^{(T)}_{X_{abs}}\right)
$ (5) <- (論文14と対応させたい)
## 2.5. Multiplying Distributions, and Computing Posteriors
## 2.6. Entropy of Reverse Process
# 3. Experiments
## 3.1. Toy Problem
## 3.2. Images
# 4. Conclusion
# メモ
- 小林さんMTGめも
## 小林さんMTGメモ
# ざっくりした物理的イメージ
- 生成モデルって?
- 何か → 似たやつを作る
- → = 確率分布
- 確率分布 = 分配関数
- 分配関数がわかれば確率分布がわかる
- エネルギーが定義できる状況ならなんでも分配関数が使える
- 情報では?
- 尤度 = エネルギー
- 学習したデータの尤度を高める = その点のポテンシャルを最小化する
- エネルギー(尤度)を最適させるのに適切な関数は何?
- 機械学習で言えば損失関数
-
- 量子コンピュータの種類
- 量子アニーリング
- 量子ゲート
|
|
https://github.com/LDemetrios/Typst4k | https://raw.githubusercontent.com/LDemetrios/Typst4k/master/src/test/resources/suite/text/smallcaps.typ | typst | --- smallcaps ---
// Test smallcaps.
#smallcaps[Smallcaps]
--- smallcaps-show-rule ---
// There is no dedicated smallcaps font in typst-dev-assets, so we just use some
// other font to test this show rule.
#show smallcaps: set text(font: "PT Sans")
#smallcaps[Smallcaps]
#show smallcaps: set text(fill: red)
#smallcaps[Smallcaps]
|
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.