text
stringlengths 17
1.47k
| positive
stringlengths 673
4.43k
| negative
stringlengths 677
2.81k
|
---|---|---|
Make output data cleaner on the eyes :) | '''
Execute overstate functions
'''
# Import salt libs
import salt.overstate
import salt.output
def over(env='base', os_fn=None):
'''
Execute an overstate sequence to orchestrate the executing of states
over a group of systems
'''
stage_num = 0
overstate = salt.overstate.OverState(__opts__, env, os_fn)
for stage in overstate.stages_iter():
if isinstance(stage, dict):
# This is highstate data
print('Stage execution results:')
for key, val in stage.items():
salt.output.display_output(
{key: val},
'highstate',
opts=__opts__)
elif isinstance(stage, list):
# This is a stage
if stage_num == 0:
print('Executing the following Over State:')
else:
print('Executed Stage:')
salt.output.display_output(stage, 'overstatestage', opts=__opts__)
stage_num += 1
return overstate.over_run
def show_stages(env='base', os_fn=None):
'''
Display the stage data to be executed
'''
overstate = salt.overstate.OverState(__opts__, env, os_fn)
salt.output.display_output(
overstate.over,
'overstatestage',
opts=__opts__)
return overstate.over
| '''
Execute overstate functions
'''
# Import salt libs
import salt.overstate
import salt.output
def over(env='base', os_fn=None):
'''
Execute an overstate sequence to orchestrate the executing of states
over a group of systems
'''
overstate = salt.overstate.OverState(__opts__, env, os_fn)
for stage in overstate.stages_iter():
if isinstance(stage, dict):
# This is highstate data
for key, val in stage.items():
salt.output.display_output(
{key: val},
'highstate',
opts=__opts__)
elif isinstance(stage, list):
# This is a stage
salt.output.display_output(stage, 'overstatestage', opts=__opts__)
return overstate.over_run
def show_stages(env='base', os_fn=None):
'''
Display the stage data to be executed
'''
overstate = salt.overstate.OverState(__opts__, env, os_fn)
salt.output.display_output(
overstate.over,
'overstatestage',
opts=__opts__)
return overstate.over
|
Fix the list tag style | module.exports = {
inputStyle: {
"backgroundColor": "white",
"border": `1px solid transparent`,
"borderRadius": `1px`,
"boxShadow": `0 2px 6px rgba(0, 0, 0, 0.3)`,
"boxSizing": `border-box`,
"MozBoxSizing": `border-box`,
"fontSize": `14px`,
"height": `32px`,
"marginTop": `27px`,
"outline": `none`,
"padding": `0 12px`,
"textOverflow": `ellipses`,
"width": `400px`,
},
divStatusStyle: {
position: 'absolute',
top: '15px',
right: '15px',
},
divAppbaseStyle: {
position: 'absolute',
width: '200px',
bottom: '15px',
left: '0px',
right: '0px',
marginLeft: 'auto',
marginRight: 'auto',
textAlign: 'center',
},
divContainer: {
padding: "1em"
},
divListTag: {
backgroundColor: "#ddd",
margin: "5px",
padding: "5px",
lineHeight: "20px",
display: "inline-block",
},
divListItem: {
margin: "5px",
padding: "3px"
},
divScroll: {
overflow: "auto",
height: "400px",
width: "100%",
margin: "5px",
},
}; | module.exports = {
inputStyle: {
"backgroundColor": "white",
"border": `1px solid transparent`,
"borderRadius": `1px`,
"boxShadow": `0 2px 6px rgba(0, 0, 0, 0.3)`,
"boxSizing": `border-box`,
"MozBoxSizing": `border-box`,
"fontSize": `14px`,
"height": `32px`,
"marginTop": `27px`,
"outline": `none`,
"padding": `0 12px`,
"textOverflow": `ellipses`,
"width": `400px`,
},
divStatusStyle: {
position: 'absolute',
top: '15px',
right: '15px',
},
divAppbaseStyle: {
position: 'absolute',
width: '200px',
bottom: '15px',
left: '0px',
right: '0px',
marginLeft: 'auto',
marginRight: 'auto',
textAlign: 'center',
},
divContainer: {
padding: "1em"
},
divListTag: {
backgroundColor: "#ddd",
margin: "5px",
padding: "5px"
},
divListItem: {
margin: "5px",
padding: "3px"
},
divScroll: {
overflow: "auto",
height: "400px",
width: "100%",
margin: "5px",
},
}; |
Add getter and setters for unit field | package com.kduda.battleships.models.board;
import com.kduda.battleships.models.units.Unit;
import javafx.scene.paint.Color;
import javafx.scene.shape.Rectangle;
public class Cell extends Rectangle {
public final Position POSITION;
public final CellType TYPE;
private final Board BOARD;
public boolean wasShot = false;
private Unit unit = null;
public Cell(int x, int y, Board board) {
super(40, 40);
this.POSITION = new Position(x, y);
this.BOARD = board;
if (y > 11) {
this.TYPE = CellType.Land;
setFill(Color.SANDYBROWN);
} else {
this.TYPE = CellType.Sea;
setFill(Color.BLUE);
}
setStroke(Color.BLACK);
}
public Unit getUnit() {
return unit;
}
public void setUnit(Unit unit) {
this.unit = unit;
}
public boolean shootCell() {
wasShot = true;
setFill(Color.GRAY);
if (unit != null) {
unit.hit();
setFill(Color.RED);
if (!unit.isAlive()) {
BOARD.units--;
}
return true;
}
return false;
}
}
| package com.kduda.battleships.models.board;
import com.kduda.battleships.models.units.Unit;
import javafx.scene.paint.Color;
import javafx.scene.shape.Rectangle;
public class Cell extends Rectangle {
public final Position POSITION;
public final CellType TYPE;
private final Board BOARD;
public Unit unit = null;
public boolean wasShot = false;
public Cell(int x, int y, Board board) {
super(40, 40);
this.POSITION = new Position(x, y);
this.BOARD = board;
if (y > 11) {
this.TYPE = CellType.Land;
setFill(Color.SANDYBROWN);
} else {
this.TYPE = CellType.Sea;
setFill(Color.BLUE);
}
setStroke(Color.BLACK);
}
public boolean shootCell() {
wasShot = true;
setFill(Color.GRAY);
if (unit != null) {
unit.hit();
setFill(Color.RED);
if (!unit.isAlive()) {
BOARD.units--;
}
return true;
}
return false;
}
}
|
Fix showing UUIDs instead of player names in the grave inventory | package com.forgeessentials.afterlife;
import com.forgeessentials.util.UserIdent;
import net.minecraft.inventory.InventoryBasic;
import net.minecraft.item.ItemStack;
import java.util.ArrayList;
import java.util.List;
public class InventoryGrave extends InventoryBasic {
private Grave grave;
public InventoryGrave(Grave grave)
{
super(new UserIdent(grave.owner).getUsername() + "'s grave.", false, grave.getSize());
this.grave = grave;
}
@Override
public void openInventory()
{
for (int i = 0; i < getSizeInventory(); i++)
{
setInventorySlotContents(i, (ItemStack) null);
}
for (int i = 0; i < grave.inv.length; i++)
{
if (grave.inv[i] != null)
{
setInventorySlotContents(i, grave.inv[i].copy());
}
}
super.openInventory();
}
@Override
public void closeInventory()
{
List<ItemStack> list = new ArrayList<ItemStack>();
for (int i = 0; i < getSizeInventory(); i++)
{
ItemStack is = getStackInSlot(i);
if (is != null)
{
list.add(is);
}
}
grave.inv = list.toArray(new ItemStack[list.size()]);
grave.checkGrave();
grave.setOpen(false);
super.closeInventory();
}
}
| package com.forgeessentials.afterlife;
import java.util.ArrayList;
import java.util.List;
import net.minecraft.inventory.InventoryBasic;
import net.minecraft.item.ItemStack;
public class InventoryGrave extends InventoryBasic {
private Grave grave;
public InventoryGrave(Grave grave)
{
super(grave.owner + "'s grave.", false, grave.getSize());
this.grave = grave;
}
@Override
public void openInventory()
{
for (int i = 0; i < getSizeInventory(); i++)
{
setInventorySlotContents(i, (ItemStack) null);
}
for (int i = 0; i < grave.inv.length; i++)
{
if (grave.inv[i] != null)
{
setInventorySlotContents(i, grave.inv[i].copy());
}
}
super.openInventory();
}
@Override
public void closeInventory()
{
List<ItemStack> list = new ArrayList<ItemStack>();
for (int i = 0; i < getSizeInventory(); i++)
{
ItemStack is = getStackInSlot(i);
if (is != null)
{
list.add(is);
}
}
grave.inv = list.toArray(new ItemStack[list.size()]);
grave.checkGrave();
grave.setOpen(false);
super.closeInventory();
}
}
|
Add long description content type | # -*- coding: utf-8 -*-
"""Packaging implementation"""
from os.path import dirname, join
from setuptools import setup
def read_file(filename):
with open(join(dirname(__file__), filename)) as file:
return file.read()
setup(
name='dj-email-url',
version='1.0.4',
url='https://github.com/migonzalvar/dj-email-url',
license='BSD',
author='Miguel Gonzalez',
author_email='[email protected]',
description='Use an URL to configure email backend settings in your '
'Django Application.',
long_description=read_file('README.rst') + '\n'
+ read_file('CHANGELOG.rst'),
long_description_content_type='text/x-rst',
py_modules=['dj_email_url'],
zip_safe=False,
include_package_data=True,
platforms='any',
classifiers=[
'Environment :: Web Environment',
'Intended Audience :: Developers',
'License :: OSI Approved :: BSD License',
'Operating System :: OS Independent',
'Programming Language :: Python',
'Programming Language :: Python :: 3',
'Programming Language :: Python :: 3.5',
'Programming Language :: Python :: 3.6',
'Programming Language :: Python :: 3.7',
'Programming Language :: Python :: 3.8',
'Programming Language :: Python :: 3.9',
'Programming Language :: Python :: 3.10',
'Topic :: Internet :: WWW/HTTP :: Dynamic Content',
'Topic :: Software Development :: Libraries :: Python Modules',
]
)
| # -*- coding: utf-8 -*-
"""Packaging implementation"""
from os.path import dirname, join
from setuptools import setup
def read_file(filename):
with open(join(dirname(__file__), filename)) as file:
return file.read()
setup(
name='dj-email-url',
version='1.0.4',
url='https://github.com/migonzalvar/dj-email-url',
license='BSD',
author='Miguel Gonzalez',
author_email='[email protected]',
description='Use an URL to configure email backend settings in your '
'Django Application.',
long_description=read_file('README.rst') + '\n'
+ read_file('CHANGELOG.rst'),
py_modules=['dj_email_url'],
zip_safe=False,
include_package_data=True,
platforms='any',
classifiers=[
'Environment :: Web Environment',
'Intended Audience :: Developers',
'License :: OSI Approved :: BSD License',
'Operating System :: OS Independent',
'Programming Language :: Python',
'Programming Language :: Python :: 3',
'Programming Language :: Python :: 3.5',
'Programming Language :: Python :: 3.6',
'Programming Language :: Python :: 3.7',
'Programming Language :: Python :: 3.8',
'Programming Language :: Python :: 3.9',
'Programming Language :: Python :: 3.10',
'Topic :: Internet :: WWW/HTTP :: Dynamic Content',
'Topic :: Software Development :: Libraries :: Python Modules',
]
)
|
Use double instead of single quotes for doc string | import warnings
import functools
__all__ = ['deprecated']
class deprecated(object):
"""Decorator to mark deprecated functions with warning.
Adapted from <http://wiki.python.org/moin/PythonDecoratorLibrary>.
Parameters
----------
alt_func : str
If given, tell user what function to use instead.
behavior : {'warn', 'raise'}
Behavior during call to deprecated function: 'warn' = warn user that
function is deprecated; 'raise' = raise error.
"""
def __init__(self, alt_func=None, behavior='warn'):
self.alt_func = alt_func
self.behavior = behavior
def __call__(self, func):
alt_msg = ''
if self.alt_func is not None:
alt_msg = ' Use `%s` instead.' % self.alt_func
msg = 'Call to deprecated function `%s`.' % func.__name__
msg += alt_msg
@functools.wraps(func)
def wrapped(*args, **kwargs):
if self.behavior == 'warn':
warnings.warn_explicit(msg,
category=DeprecationWarning,
filename=func.func_code.co_filename,
lineno=func.func_code.co_firstlineno + 1)
elif self.behavior == 'raise':
raise DeprecationWarning(msg)
return func(*args, **kwargs)
# modify doc string to display deprecation warning
doc = 'Deprecated function.' + alt_msg
if wrapped.__doc__ is None:
wrapped.__doc__ = doc
else:
wrapped.__doc__ = doc + '\n\n' + wrapped.__doc__
return wrapped
| import warnings
import functools
__all__ = ['deprecated']
class deprecated(object):
'''Decorator to mark deprecated functions with warning.
Adapted from <http://wiki.python.org/moin/PythonDecoratorLibrary>.
Parameters
----------
alt_func : str
If given, tell user what function to use instead.
behavior : {'warn', 'raise'}
Behavior during call to deprecated function: 'warn' = warn user that
function is deprecated; 'raise' = raise error.
'''
def __init__(self, alt_func=None, behavior='warn'):
self.alt_func = alt_func
self.behavior = behavior
def __call__(self, func):
alt_msg = ''
if self.alt_func is not None:
alt_msg = ' Use `%s` instead.' % self.alt_func
msg = 'Call to deprecated function `%s`.' % func.__name__
msg += alt_msg
@functools.wraps(func)
def wrapped(*args, **kwargs):
if self.behavior == 'warn':
warnings.warn_explicit(msg,
category=DeprecationWarning,
filename=func.func_code.co_filename,
lineno=func.func_code.co_firstlineno + 1)
elif self.behavior == 'raise':
raise DeprecationWarning(msg)
return func(*args, **kwargs)
# modify doc string to display deprecation warning
doc = 'Deprecated function.' + alt_msg
if wrapped.__doc__ is None:
wrapped.__doc__ = doc
else:
wrapped.__doc__ = doc + '\n\n' + wrapped.__doc__
return wrapped
|
Remove dependency links for pybloom. | from setuptools import setup
setup(
name = 'fastqp',
provides = 'fastqp',
version = "0.1.6",
author = 'Matthew Shirley',
author_email = '[email protected]',
url = 'http://mattshirley.com',
description = 'Simple NGS read quality assessment using Python',
license = 'MIT',
packages = ['fastqp', 'fastqp.backports'],
install_requires = ['six', 'matplotlib', 'numpy', 'simplesam'],
entry_points = { 'console_scripts': [ 'fastqp = fastqp.cli:main' ] },
classifiers = [
"Development Status :: 4 - Beta",
"License :: OSI Approved :: MIT License",
"Environment :: Console",
"Intended Audience :: Science/Research",
"Natural Language :: English",
"Operating System :: Unix",
"Programming Language :: Python :: 2.6",
"Programming Language :: Python :: 2.7",
"Programming Language :: Python :: 3.3",
"Programming Language :: Python :: 3.4",
"Topic :: Scientific/Engineering :: Bio-Informatics"
]
)
| from setuptools import setup
setup(
name = 'fastqp',
provides = 'fastqp',
version = "0.1.5",
author = 'Matthew Shirley',
author_email = '[email protected]',
url = 'http://mattshirley.com',
description = 'Simple NGS read quality assessment using Python',
license = 'MIT',
packages = ['fastqp', 'fastqp.backports'],
install_requires=['six', 'matplotlib', 'numpy', 'pyfaidx'],
entry_points = { 'console_scripts': [ 'fastqp = fastqp.cli:main' ] },
classifiers = [
"Development Status :: 4 - Beta",
"License :: OSI Approved :: MIT License",
"Environment :: Console",
"Intended Audience :: Science/Research",
"Natural Language :: English",
"Operating System :: Unix",
"Programming Language :: Python :: 2.6",
"Programming Language :: Python :: 2.7",
"Programming Language :: Python :: 3.3",
"Programming Language :: Python :: 3.4",
"Topic :: Scientific/Engineering :: Bio-Informatics"
]
)
|
Disable days before today for selection | import React from "react";
import DayPicker, {DateUtils} from "react-day-picker";
import moment from 'moment';
import "./MeetingDaysField.scss";
class MeetingDaysField extends React.Component {
static propTypes = {
days: React.PropTypes.array.isRequired,
month: React.PropTypes.object.isRequired,
onDayChange: React.PropTypes.func.isRequired,
onMonthChange: React.PropTypes.func.isRequired
};
handleDayClick(e, day, {selected, disabled}){
// We need to pass it upwards
if(disabled){
return;
}
this.props.onDayChange(selected, day);
}
isDaySelected(day){
return this.props.days.findIndex((d) => DateUtils.isSameDay(d, day)) > -1;
}
isDayBeforeToday(day) {
return moment(day).add(1, 'day').isBefore();
}
render(){
let { month, onMonthChange } = this.props;
return (
<div className="MeetingDaysField form-group clearfix">
<label htmlFor="meeting-name" className="col-form-label col-xs-3">Wybierz dni</label>
<div className="col-xs-4">
<DayPicker initialMonth={month} firstDayOfWeek={1} selectedDays={this.isDaySelected.bind(this)}
disabledDays={this.isDayBeforeToday.bind(this)} fromMonth={new Date()}
onDayClick={this.handleDayClick.bind(this)} onMonthChange={onMonthChange} />
</div>
<div className="col-xs-5">
{this.props.children}
</div>
</div>
);
}
}
export default MeetingDaysField;
| import React from "react";
import DayPicker, {DateUtils} from "react-day-picker";
import "./MeetingDaysField.scss";
class MeetingDaysField extends React.Component {
static propTypes = {
days: React.PropTypes.array.isRequired,
month: React.PropTypes.object.isRequired,
onDayChange: React.PropTypes.func.isRequired,
onMonthChange: React.PropTypes.func.isRequired
};
handleDayClick(e, day, {selected, disabled}){
// We need to pass it upwards
if(disabled){
return;
}
this.props.onDayChange(selected, day);
}
isDaySelected(day){
return this.props.days.findIndex((d) => DateUtils.isSameDay(d, day)) > -1;
}
render(){
let { month, onMonthChange } = this.props;
return (
<div className="MeetingDaysField form-group clearfix">
<label htmlFor="meeting-name" className="col-form-label col-xs-3">Wybierz dni</label>
<div className="col-xs-4">
<DayPicker initialMonth={month} firstDayOfWeek={1} selectedDays={this.isDaySelected.bind(this)}
onDayClick={this.handleDayClick.bind(this)} onMonthChange={onMonthChange} />
</div>
<div className="col-xs-5">
{this.props.children}
</div>
</div>
);
}
}
export default MeetingDaysField;
|
Move from ps2ascii to ps2txt, for better results | # -*- coding: utf-8 -*-:
import urllib2
import hashlib
import os
def construct_body(link):
if link.content == u'˘':
# this signals that content generation previously failed
return
try:
opener = urllib2.build_opener()
opener.addheaders = [('Accept-Charset', 'utf-8'), ('User-agent', 'Mozilla/5.0')]
f = opener.open(link.url)
data = f.read()
f.close()
opener.close()
subtype = f.info().subtype
if subtype == 'pdf':
filename = hashlib.md5(link.url).hexdigest()
thefile = open('/tmp/%s.pdf' % filename, "wb")
thefile.write(data)
thefile.close()
os.system(("ps2txt /tmp/%s.pdf /tmp/%s.txt") %(filename , filename))
link.content = open("/tmp/%s.txt" % filename).read()
link.save()
elif subtype == 'html':
from readability.readability import Document
readable_article = Document(data).summary()
link.content = readable_article
link.save()
else:
link.content = u'˘'
link.save()
except:
link.content = u'˘'
link.save()
pass
| # -*- coding: utf-8 -*-:
import urllib2
import hashlib
import os
def construct_body(link):
if link.content == u'˘':
# this signals that content generation previously failed
return
try:
opener = urllib2.build_opener()
opener.addheaders = [('Accept-Charset', 'utf-8'), ('User-agent', 'Mozilla/5.0')]
f = opener.open(link.url)
data = f.read()
f.close()
opener.close()
subtype = f.info().subtype
if subtype == 'pdf':
filename = hashlib.md5(link.url).hexdigest()
thefile = open('/tmp/%s.pdf' % filename, "wb")
thefile.write(data)
thefile.close()
os.system(("ps2ascii /tmp/%s.pdf /tmp/%s.txt") %(filename , filename))
link.content = open("/tmp/%s.txt" % filename).read()
link.save()
elif subtype == 'html':
from readability.readability import Document
readable_article = Document(data).summary()
link.content = readable_article
link.save()
else:
link.content = u'˘'
link.save()
except:
link.content = u'˘'
link.save()
pass
|
Add safety check for `console.log` on app startup. | (function () {
'use strict';
// Initialise RequireJS module loader
require.config({
urlArgs: 'm=' + (new Date()).getTime(),
baseUrl: '/app/',
paths: {
// RequireJS extensions
text: '../lib/text/text',
// Vendor libraries
knockout: '../lib/knockout/dist/knockout.debug',
lodash: '../lib/lodash/lodash',
jquery: '../lib/jquery/dist/jquery',
bootstrap: '../lib/bootstrap/dist/js/bootstrap',
typeahead: '../lib/typeahead.js/dist/typeahead.jquery',
bloodhound: '../lib/typeahead.js/dist/bloodhound',
page: '../lib/page/page',
// Application modules
config: 'config/',
models: 'models/',
services: 'services/',
ui: 'ui/',
util: 'util/'
},
shim: {
bloodhound: {
exports: 'Bloodhound'
},
typeahead: {
deps: [ 'jquery' ]
}
}
});
// Boot the application
require([ 'jquery' ], function () {
require([ 'bootstrap' ], function () {
var environment = 'development';
if (environment !== 'production' && console && typeof console.log === 'function') {
console.log('Running in "' + environment + '" environment');
}
require([ 'config/env/' + environment, 'util/router' ], function (configure, router) {
configure();
router();
});
});
});
}());
| (function () {
'use strict';
// Initialise RequireJS module loader
require.config({
urlArgs: 'm=' + (new Date()).getTime(),
baseUrl: '/app/',
paths: {
// RequireJS extensions
text: '../lib/text/text',
// Vendor libraries
knockout: '../lib/knockout/dist/knockout.debug',
lodash: '../lib/lodash/lodash',
jquery: '../lib/jquery/dist/jquery',
bootstrap: '../lib/bootstrap/dist/js/bootstrap',
typeahead: '../lib/typeahead.js/dist/typeahead.jquery',
bloodhound: '../lib/typeahead.js/dist/bloodhound',
page: '../lib/page/page',
// Application modules
config: 'config/',
models: 'models/',
services: 'services/',
ui: 'ui/',
util: 'util/'
},
shim: {
bloodhound: {
exports: 'Bloodhound'
},
typeahead: {
deps: [ 'jquery' ]
}
}
});
// Boot the application
require([ 'jquery' ], function () {
require([ 'bootstrap' ], function () {
var environment = 'development';
if (environment !== 'production') {
console.log('Running in "' + environment + '" environment');
}
require([ 'config/env/' + environment, 'util/router' ], function (configure, router) {
configure();
router();
});
});
});
}());
|
Error: Call to undefined function apc_clear_cache() | <?php
namespace tagadvance\gilligan\session;
use PHPUnit\Framework\TestCase;
use tagadvance\gilligan\cache\APC;
/**
*
* @author Tag <[email protected]>
*/
class APCSessionHandlerTest extends TestCase {
const SESSION_ID = 'CAFEBABE';
/**
*
* @var APCSessionHandler
*/
private $handler;
function setUp() {
apcu_clear_cache();
$timeToLive = get_cfg_var('session.gc_maxlifetime');
$apc = new APC($timeToLive);
$this->handler = new APCSessionHandler($apc);
}
function testReadAndWrite() {
$writeData = 'foo';
$this->handler->write(self::SESSION_ID, $writeData);
$readData = $this->handler->read(self::SESSION_ID);
$this->assertEquals($expected = $writeData, $actual = $readData);
}
function testDestroy() {
$this->handler->write(self::SESSION_ID, 'foo');
$this->handler->destroy(self::SESSION_ID);
$actual = $this->handler->read(self::SESSION_ID);
$this->assertEquals($expected = '', $actual);
}
function testGarbageCollection() {
$this->handler->write(self::SESSION_ID, 'foo');
$result = $this->handler->gc($maxLifetime = 0);
$this->assertTrue($result);
}
function tearDown() {
apcu_clear_cache();
}
} | <?php
namespace tagadvance\gilligan\session;
use PHPUnit\Framework\TestCase;
use tagadvance\gilligan\cache\APC;
/**
*
* @author Tag <[email protected]>
*/
class APCSessionHandlerTest extends TestCase {
const SESSION_ID = 'CAFEBABE';
/**
*
* @var APCSessionHandler
*/
private $handler;
function setUp() {
apc_clear_cache();
$timeToLive = get_cfg_var('session.gc_maxlifetime');
$apc = new APC($timeToLive);
$this->handler = new APCSessionHandler($apc);
}
function testReadAndWrite() {
$writeData = 'foo';
$this->handler->write(self::SESSION_ID, $writeData);
$readData = $this->handler->read(self::SESSION_ID);
$this->assertEquals($expected = $writeData, $actual = $readData);
}
function testDestroy() {
$this->handler->write(self::SESSION_ID, 'foo');
$this->handler->destroy(self::SESSION_ID);
$actual = $this->handler->read(self::SESSION_ID);
$this->assertEquals($expected = '', $actual);
}
function testGarbageCollection() {
$this->handler->write(self::SESSION_ID, 'foo');
$result = $this->handler->gc($maxLifetime = 0);
$this->assertTrue($result);
}
function tearDown() {
apc_clear_cache();
}
} |
Fix bug in checkConfirmationKey, call the model directly instead of
eval(arg) | from django.contrib.sites.models import Site
from django.core.mail import send_mail
from okupy.libraries.encryption import random_string
from okupy.libraries.exception import OkupyException, log_extra_data
import logging
logger = logging.getLogger('okupy')
def sendConfirmationEmail(request, form, model):
'''
Create a random URL, add it to the appropriate table along with
the user data, and send an email to the user to confirm his email address
'''
random_url = random_string(30)
inactive_email = model(email = form.cleaned_data['email'],
user = form.cleaned_data['username'],
url = random_url)
try:
inactive_email.save()
except Exception as error:
logger.error(error, extra = log_extra_data(request, form))
raise OkupyException('Could not save to DB')
send_mail('[%s]: Please confirm your email address' % Site.objects.get_current().name or None,
'To confirm your email address, please click <a href="/%s">here</a>' % random_url,
'[email protected]',
[form.cleaned_data['email']])
def checkConfirmationKey(key, model):
'''
Check if the URL matches any of the confirmation keys in the database
'''
try:
result = model.objects.get(url = key)
except model.DoesNotExist:
raise OkupyException('URL not found')
return result
| from django.contrib.sites.models import Site
from django.core.mail import send_mail
from okupy.libraries.encryption import random_string
from okupy.libraries.exception import OkupyException, log_extra_data
import logging
logger = logging.getLogger('okupy')
def sendConfirmationEmail(request, form, model):
'''
Create a random URL, add it to the appropriate table along with
the user data, and send an email to the user to confirm his email address
'''
random_url = random_string(30)
inactive_email = model(email = form.cleaned_data['email'],
user = form.cleaned_data['username'],
url = random_url)
try:
inactive_email.save()
except Exception as error:
logger.error(error, extra = log_extra_data(request, form))
raise OkupyException('Could not save to DB')
send_mail('[%s]: Please confirm your email address' % Site.objects.get_current().name or None,
'To confirm your email address, please click <a href="/%s">here</a>' % random_url,
'[email protected]',
[form.cleaned_data['email']])
def checkConfirmationKey(key, model):
'''
Check if the URL matches any of the confirmation keys in the database
'''
try:
result = eval(model).objects.get(url = key)
except eval(model).DoesNotExist as error:
raise OkupyException('URL not found')
return result
|
Add the null check/initialization for the characteristics delegation to active as well. | package com.oath.cyclops.internal.stream.spliterators;
import java.util.Iterator;
import java.util.Spliterator;
import java.util.Spliterators;
import java.util.function.Consumer;
/**
* Created by johnmcclean on 22/12/2016.
*/
public class IteratableSpliterator<T> implements Spliterator<T>, CopyableSpliterator<T>{
private final Iterable<T> source;
Spliterator<T> active;
public IteratableSpliterator(final Iterable<T> source) {
this.source = source;
}
@Override
public void forEachRemaining(Consumer<? super T> action) {
if(active==null)
active = source.spliterator();
active.forEachRemaining(action);
}
@Override
public Spliterator<T> trySplit() {
if(active==null)
active=source.spliterator();
return active.trySplit();
}
@Override
public long estimateSize() {
if(active==null)
active=source.spliterator();
return active.estimateSize();
}
@Override
public int characteristics() {
if(active==null)
active=source.spliterator();
return active.characteristics();
}
@Override
public boolean tryAdvance(Consumer<? super T> action) {
if(active==null)
active=source.spliterator();
return active.tryAdvance(action);
}
@Override
public Spliterator<T> copy() {
return new IteratableSpliterator<>(source);
}
}
| package com.oath.cyclops.internal.stream.spliterators;
import java.util.Iterator;
import java.util.Spliterator;
import java.util.Spliterators;
import java.util.function.Consumer;
/**
* Created by johnmcclean on 22/12/2016.
*/
public class IteratableSpliterator<T> implements Spliterator<T>, CopyableSpliterator<T>{
private final Iterable<T> source;
Spliterator<T> active;
public IteratableSpliterator(final Iterable<T> source) {
this.source = source;
}
@Override
public void forEachRemaining(Consumer<? super T> action) {
if(active==null)
active = source.spliterator();
active.forEachRemaining(action);
}
@Override
public Spliterator<T> trySplit() {
if(active==null)
active=source.spliterator();
return active.trySplit();
}
@Override
public long estimateSize() {
if(active==null)
active=source.spliterator();
return active.estimateSize();
}
@Override
public int characteristics() {
return active.characteristics();
}
@Override
public boolean tryAdvance(Consumer<? super T> action) {
if(active==null)
active=source.spliterator();
return active.tryAdvance(action);
}
@Override
public Spliterator<T> copy() {
return new IteratableSpliterator<>(source);
}
}
|
Fix deoplete source compete position sent to language servers. | from .base import Base
import re
CompleteOutputs = "g:LanguageClient_omniCompleteResults"
class Source(Base):
def __init__(self, vim):
super().__init__(vim)
self.name = "LanguageClient"
self.mark = "[LC]"
self.rank = 1000
self.min_pattern_length = 0
self.filetypes = vim.eval(
"get(g:, 'LanguageClient_serverCommands', {})").keys()
self.input_pattern += r'(\.|::|->)\w*$'
self.complete_pos = re.compile(r"\w*$")
def get_complete_position(self, context):
m = self.complete_pos.search(context['input'])
return m.start() if m else -1
def gather_candidates(self, context):
if context["is_async"]:
outputs = self.vim.eval(CompleteOutputs)
if len(outputs) != 0:
context["is_async"] = False
# TODO: error handling.
candidates = outputs[0].get("result", [])
# log(str(candidates))
return candidates
else:
context["is_async"] = True
self.vim.command("let {} = []".format(CompleteOutputs))
self.vim.funcs.LanguageClient_omniComplete({
"character": context["complete_position"] + len(context["complete_str"]),
})
return []
# f = open("/tmp/deoplete.log", "w")
# def log(message):
# f.writelines([message])
# f.flush()
| from .base import Base
import re
CompleteOutputs = "g:LanguageClient_omniCompleteResults"
class Source(Base):
def __init__(self, vim):
super().__init__(vim)
self.name = "LanguageClient"
self.mark = "[LC]"
self.rank = 1000
self.min_pattern_length = 1
self.filetypes = vim.eval(
"get(g:, 'LanguageClient_serverCommands', {})").keys()
self.input_pattern += r'(\.|::|->)\w*$'
self.complete_pos = re.compile(r"\w*$")
def get_complete_position(self, context):
m = self.complete_pos.search(context['input'])
return m.start() if m else -1
def gather_candidates(self, context):
if context["is_async"]:
outputs = self.vim.eval(CompleteOutputs)
if len(outputs) != 0:
context["is_async"] = False
# TODO: error handling.
candidates = outputs[0].get("result", [])
# log(str(candidates))
return candidates
else:
context["is_async"] = True
self.vim.command("let {} = []".format(CompleteOutputs))
self.vim.funcs.LanguageClient_omniComplete({
"character": context["complete_position"],
})
return []
# f = open("/tmp/deoplete.log", "w")
# def log(message):
# f.writelines([message])
# f.flush()
|
Correct location of bracket so that grouping by beta loops is done correctly. | '''Utility procedures for manipulating HANDE data.'''
import numpy as np
def groupby_beta_loops(data, name='iterations'):
'''Group a HANDE DMQMC data table by beta loop.
Parameters
----------
data : :class:`pandas.DataFrame`
DMQMC data table (e.g. obtained by :func:`pyhande.extract.extract_data`.
Returns
-------
grouped : :class:`pandas.DataFrameGroupBy`
GroupBy object with data table grouped by beta loop.
'''
# Exploit the fact that (except for possibly the last beta loop due to wall
# time) each beta loop contains the same set of iterations.
indx = np.arange(len(data)) // len(data[name].unique())
return data.groupby(indx)
def groupby_iterations(data):
'''Group a HANDE QMC data table by blocks of iterations.
Parameters
----------
data : :class:`pandas.DataFrame`
QMC data table (e.g. obtained by :func:`pyhande.extract.extract_data`.
Returns
-------
grouped : :class:`pandas.DataFrameGroupBy`
GroupBy object with data table grouped into blocks within which the
iteration count increases monotonically.
'''
indx = np.zeros(len(data))
prev_iteration = -1
curr_indx = 0
for i in range(len(data)):
if data['iterations'].iloc[i] < prev_iteration:
# new block of iterations
curr_indx += 1
indx[i] = curr_indx
prev_iteration = data['iterations'].iloc[i]
return data.groupby(indx)
| '''Utility procedures for manipulating HANDE data.'''
import numpy as np
def groupby_beta_loops(data):
'''Group a HANDE DMQMC data table by beta loop.
Parameters
----------
data : :class:`pandas.DataFrame`
DMQMC data table (e.g. obtained by :func:`pyhande.extract.extract_data`.
Returns
-------
grouped : :class:`pandas.DataFrameGroupBy`
GroupBy object with data table grouped by beta loop.
'''
# Exploit the fact that (except for possibly the last beta loop due to wall
# time) each beta loop contains the same set of iterations.
indx = np.arange(len(data)) // len(data['iterations']).unique()
return data.groupby(indx)
def groupby_iterations(data):
'''Group a HANDE QMC data table by blocks of iterations.
Parameters
----------
data : :class:`pandas.DataFrame`
QMC data table (e.g. obtained by :func:`pyhande.extract.extract_data`.
Returns
-------
grouped : :class:`pandas.DataFrameGroupBy`
GroupBy object with data table grouped into blocks within which the
iteration count increases monotonically.
'''
indx = np.zeros(len(data))
prev_iteration = -1
curr_indx = 0
for i in range(len(data)):
if data['iterations'].iloc[i] < prev_iteration:
# new block of iterations
curr_indx += 1
indx[i] = curr_indx
prev_iteration = data['iterations'].iloc[i]
return data.groupby(indx)
|
Load class is return by reference rather than returning copy | <?php
if (!function_exists('loadClass'))
{
function &loadClass($className, $package='library', $vendors=[APP_PATH, SYSTEM_PATH])
{
static $instance = array();
if (!is_array($vendors))
{
$vendors = array($vendors);
}
$className = strtolower($className);
$class = false;
if (isset($instance[$package]) && isset($instance[$package][$className]))
{
return $instance[$package][$className];
}
foreach ($vendors as $vendor)
{
$vendor = rtrim(strtolower($vendor), '/') . '/';
if (file_exists($vendor . $package . '/' . $className . '.php'))
{
include ($vendor . $package . '/' . $className . '.php');
$class = $className;
break;
}
}
if ($class === false)
{
exit('Class "'.$className.'" is not found on "'.$package.'" package.');
}
$class = new $class();
$instance[$package][$className] = $class;
return $class;
}
}
if (!function_exists('isLoaded'))
{
function isLoaded($package='', $name='')
{
static $loaded = array();
if ($package === '' && $name === '')
{
return $loaded;
}
$loaded[$package][$name] = strtolower($name);
}
}
?>
| <?php
if (!function_exists('loadClass'))
{
function loadClass($className, $package='library', $vendors=[APP_PATH, SYSTEM_PATH])
{
static $instance = array();
if (!is_array($vendors))
{
$vendors = array($vendors);
}
$className = strtolower($className);
$class = false;
if (isset($instance[$package]) && isset($instance[$package][$className]))
{
return $instance[$package][$className];
}
foreach ($vendors as $vendor)
{
$vendor = rtrim(strtolower($vendor), '/') . '/';
if (file_exists($vendor . $package . '/' . $className . '.php'))
{
include ($vendor . $package . '/' . $className . '.php');
$class = $className;
break;
}
}
if ($class === false)
{
exit('Class "'.$className.'" is not found on "'.$package.'" package.');
}
$class = new $class();
$instance[$package][$className] = $class;
return $class;
}
}
if (!function_exists('isLoaded'))
{
function isLoaded($package='', $name='')
{
static $loaded = array();
if ($package === '' && $name === '')
{
return $loaded;
}
$loaded[$package][$name] = strtolower($name);
}
}
?>
|
Rename SLURM invoices application for better Django dashoard menu item. | from django.apps import AppConfig
from django.db.models import signals
class SlurmInvoicesConfig(AppConfig):
name = 'nodeconductor_assembly_waldur.slurm_invoices'
verbose_name = 'Batch packages'
def ready(self):
from nodeconductor_assembly_waldur.invoices import registrators
from waldur_slurm import models as slurm_models
from . import handlers, registrators as slurm_registrators
registrators.RegistrationManager.add_registrator(
slurm_models.Allocation,
slurm_registrators.AllocationRegistrator
)
signals.post_save.connect(
handlers.add_new_allocation_to_invoice,
sender=slurm_models.Allocation,
dispatch_uid='waldur_slurm.handlers.add_new_allocation_to_invoice',
)
signals.post_save.connect(
handlers.terminate_invoice_when_allocation_cancelled,
sender=slurm_models.Allocation,
dispatch_uid='waldur_slurm.handlers.terminate_invoice_when_allocation_cancelled',
)
signals.pre_delete.connect(
handlers.terminate_invoice_when_allocation_deleted,
sender=slurm_models.Allocation,
dispatch_uid='waldur_slurm.handlers.terminate_invoice_when_allocation_deleted',
)
signals.post_save.connect(
handlers.update_invoice_item_on_allocation_usage_update,
sender=slurm_models.Allocation,
dispatch_uid='waldur_slurm.handlers.update_invoice_item_on_allocation_usage_update',
)
| from django.apps import AppConfig
from django.db.models import signals
class SlurmInvoicesConfig(AppConfig):
name = 'nodeconductor_assembly_waldur.slurm_invoices'
verbose_name = 'SLURM invoices'
def ready(self):
from nodeconductor_assembly_waldur.invoices import registrators
from waldur_slurm import models as slurm_models
from . import handlers, registrators as slurm_registrators
registrators.RegistrationManager.add_registrator(
slurm_models.Allocation,
slurm_registrators.AllocationRegistrator
)
signals.post_save.connect(
handlers.add_new_allocation_to_invoice,
sender=slurm_models.Allocation,
dispatch_uid='waldur_slurm.handlers.add_new_allocation_to_invoice',
)
signals.post_save.connect(
handlers.terminate_invoice_when_allocation_cancelled,
sender=slurm_models.Allocation,
dispatch_uid='waldur_slurm.handlers.terminate_invoice_when_allocation_cancelled',
)
signals.pre_delete.connect(
handlers.terminate_invoice_when_allocation_deleted,
sender=slurm_models.Allocation,
dispatch_uid='waldur_slurm.handlers.terminate_invoice_when_allocation_deleted',
)
signals.post_save.connect(
handlers.update_invoice_item_on_allocation_usage_update,
sender=slurm_models.Allocation,
dispatch_uid='waldur_slurm.handlers.update_invoice_item_on_allocation_usage_update',
)
|
Add FIXME to address removing debug information from generated shaders. | # Copyright 2013 The Flutter Authors. All rights reserved.
# Use of this source code is governed by a BSD-style license that can be
# found in the LICENSE file.
import sys
import argparse
import errno
import os
import subprocess
def MakeDirectories(path):
try:
os.makedirs(path)
except OSError as exc:
if exc.errno == errno.EEXIST and os.path.isdir(path):
pass
else:
raise
def Main():
parser = argparse.ArgumentParser()
parser.add_argument("--output",
type=str, required=True,
help="The location to generate the Metal library to.")
parser.add_argument("--depfile",
type=str, required=True,
help="The location of the depfile.")
parser.add_argument("--source",
type=str, action="append", required=True,
help="The source file to compile. Can be specified multiple times.")
args = parser.parse_args()
MakeDirectories(os.path.dirname(args.depfile))
command = [
"xcrun",
"metal",
# TODO: Embeds both sources and driver options in the output. This aids in
# debugging but should be removed from release builds.
"-MO",
"-gline-tables-only",
# Both user and system header will be tracked.
"-MMD",
"-MF",
args.depfile,
"-o",
args.output
]
command += args.source
subprocess.check_call(command)
if __name__ == '__main__':
if sys.platform != 'darwin':
raise Exception("This script only runs on Mac")
Main()
| # Copyright 2013 The Flutter Authors. All rights reserved.
# Use of this source code is governed by a BSD-style license that can be
# found in the LICENSE file.
import sys
import argparse
import errno
import os
import subprocess
def MakeDirectories(path):
try:
os.makedirs(path)
except OSError as exc:
if exc.errno == errno.EEXIST and os.path.isdir(path):
pass
else:
raise
def Main():
parser = argparse.ArgumentParser()
parser.add_argument("--output",
type=str, required=True,
help="The location to generate the Metal library to.")
parser.add_argument("--depfile",
type=str, required=True,
help="The location of the depfile.")
parser.add_argument("--source",
type=str, action="append", required=True,
help="The source file to compile. Can be specified multiple times.")
args = parser.parse_args()
MakeDirectories(os.path.dirname(args.depfile))
command = [
"xcrun",
"metal",
"-MO",
"-gline-tables-only",
# Both user and system header will be tracked.
"-MMD",
"-MF",
args.depfile,
"-o",
args.output
]
command += args.source
subprocess.check_call(command)
if __name__ == '__main__':
if sys.platform != 'darwin':
raise Exception("This script only runs on Mac")
Main()
|
Update UserSuForm to enhance compatibility with custom user models.
In custom user models, we cannot rely on there being a 'username'
field. Instead, we should use whichever field has been specified as
the username field. | # -*- coding: utf-8 -*-
from django import forms
from django.conf import settings
from django.utils.translation import ugettext_lazy as _
from . import get_user_model
class UserSuForm(forms.Form):
username_field = get_user_model().USERNAME_FIELD
user = forms.ModelChoiceField(
label=_('Users'), queryset=get_user_model()._default_manager.order_by(
username_field), required=True) # pylint: disable=W0212
use_ajax_select = False
def __init__(self, *args, **kwargs):
super(UserSuForm, self).__init__(*args, **kwargs)
if 'ajax_select' in settings.INSTALLED_APPS and getattr(
settings, 'AJAX_LOOKUP_CHANNELS', None):
from ajax_select.fields import AutoCompleteSelectField
lookup = settings.AJAX_LOOKUP_CHANNELS.get('django_su', None)
if lookup is not None:
old_field = self.fields['user']
self.fields['user'] = AutoCompleteSelectField(
'django_su',
required=old_field.required,
label=old_field.label,
)
self.use_ajax_select = True
def get_user(self):
return self.cleaned_data.get('user', None)
def __str__(self):
if 'formadmin' in settings.INSTALLED_APPS:
try:
from formadmin.forms import as_django_admin
return as_django_admin(self)
except ImportError:
pass
return super(UserSuForm, self).__str__()
| # -*- coding: utf-8 -*-
from django import forms
from django.conf import settings
from django.utils.translation import ugettext_lazy as _
from . import get_user_model
class UserSuForm(forms.Form):
user = forms.ModelChoiceField(
label=_('Users'), queryset=get_user_model()._default_manager.order_by(
'username'), required=True) # pylint: disable=W0212
use_ajax_select = False
def __init__(self, *args, **kwargs):
super(UserSuForm, self).__init__(*args, **kwargs)
if 'ajax_select' in settings.INSTALLED_APPS and getattr(
settings, 'AJAX_LOOKUP_CHANNELS', None):
from ajax_select.fields import AutoCompleteSelectField
lookup = settings.AJAX_LOOKUP_CHANNELS.get('django_su', None)
if lookup is not None:
old_field = self.fields['user']
self.fields['user'] = AutoCompleteSelectField(
'django_su',
required=old_field.required,
label=old_field.label,
)
self.use_ajax_select = True
def get_user(self):
return self.cleaned_data.get('user', None)
def __str__(self):
if 'formadmin' in settings.INSTALLED_APPS:
try:
from formadmin.forms import as_django_admin
return as_django_admin(self)
except ImportError:
pass
return super(UserSuForm, self).__str__()
|
Fix tool description in argparse help | #!/usr/bin/env python
import os
import argparse
import json
from pathlib import Path
from jinja2 import Template
import pyyaks.logger
def get_opt():
parser = argparse.ArgumentParser(description='Make aimpoint monitor web page')
parser.add_argument("--data-root",
default=".",
help="Root directory for asol and index files")
return parser.parse_args()
# Options
opt = get_opt()
# Set up logging
loglevel = pyyaks.logger.INFO
logger = pyyaks.logger.get_logger(name='make_web_page', level=loglevel,
format="%(asctime)s %(message)s")
def main():
# Files
index_template_file = Path(__file__).parent / 'data' / 'index_template.html'
index_file = os.path.join(opt.data_root, 'index.html')
info_file = os.path.join(opt.data_root, 'info.json')
# Jinja template context
logger.info('Loading info file {}'.format(info_file))
context = json.load(open(info_file, 'r'))
template = Template(open(index_template_file).read())
context['static'] = True
html = template.render(**context)
logger.info('Writing index file {}'.format(index_file))
with open(index_file, 'w') as fh:
fh.write(html)
if __name__ == '__main__':
main()
| #!/usr/bin/env python
import os
import argparse
import json
from pathlib import Path
from jinja2 import Template
import pyyaks.logger
def get_opt():
parser = argparse.ArgumentParser(description='Get aimpoint drift data '
'from aspect solution files')
parser.add_argument("--data-root",
default=".",
help="Root directory for asol and index files")
return parser.parse_args()
# Options
opt = get_opt()
# Set up logging
loglevel = pyyaks.logger.INFO
logger = pyyaks.logger.get_logger(name='make_web_page', level=loglevel,
format="%(asctime)s %(message)s")
def main():
# Files
index_template_file = Path(__file__).parent / 'data' / 'index_template.html'
index_file = os.path.join(opt.data_root, 'index.html')
info_file = os.path.join(opt.data_root, 'info.json')
# Jinja template context
logger.info('Loading info file {}'.format(info_file))
context = json.load(open(info_file, 'r'))
template = Template(open(index_template_file).read())
context['static'] = True
html = template.render(**context)
logger.info('Writing index file {}'.format(index_file))
with open(index_file, 'w') as fh:
fh.write(html)
if __name__ == '__main__':
main()
|
Update method name to explain what the method does | <?php
namespace Lily\Example\Application;
use Lily\Application\MiddlewareApplication;
use Lily\Application\RoutedApplication;
use Lily\Middleware as MW;
use Lily\Util\Response;
use Lily\Example\Controller\AdminController;
class AdminApplication extends MiddlewareApplication
{
protected function middleware()
{
return array(
$this->routedApplication(),
$this->ensureAuthenticated(),
new MW\Cookie(array('salt' => 'random')),
);
}
private function routedApplication()
{
return new RoutedApplication(array(
array('GET', '/admin', $this->action('index')),
array('GET', '/admin/login', $this->action('login')),
array('POST', '/admin/login', $this->action('login_process')),
array('GET', '/admin/logout', $this->action('logout')),
));
}
private function action($action)
{
return function ($request) use ($action) {
$controller = new AdminController;
return $controller->{$action}($request);
};
}
private function ensureAuthenticated()
{
return function ($handler) {
return function ($request) use ($handler) {
$isLogin = $request['uri'] === '/admin/login';
$isAuthed = isset($request['cookies']['authed']);
if ($isLogin AND $isAuthed) {
return Response::redirect('/admin');
} else if ( ! $isLogin AND ! $isAuthed) {
return Response::redirect('/admin/login');
}
return $handler($request);
};
};
}
}
| <?php
namespace Lily\Example\Application;
use Lily\Application\MiddlewareApplication;
use Lily\Application\RoutedApplication;
use Lily\Middleware as MW;
use Lily\Util\Response;
use Lily\Example\Controller\AdminController;
class AdminApplication extends MiddlewareApplication
{
protected function middleware()
{
return array(
$this->routedApplication(),
$this->adminAuthMiddleware(),
new MW\Cookie(array('salt' => 'random')),
);
}
private function routedApplication()
{
return new RoutedApplication(array(
array('GET', '/admin', $this->action('index')),
array('GET', '/admin/login', $this->action('login')),
array('POST', '/admin/login', $this->action('login_process')),
array('GET', '/admin/logout', $this->action('logout')),
));
}
private function action($action)
{
return function ($request) use ($action) {
$controller = new AdminController;
return $controller->{$action}($request);
};
}
private function adminAuthMiddleware()
{
return function ($handler) {
return function ($request) use ($handler) {
$isLogin = $request['uri'] === '/admin/login';
$isAuthed = isset($request['cookies']['authed']);
if ($isLogin AND $isAuthed) {
return Response::redirect('/admin');
} else if ( ! $isLogin AND ! $isAuthed) {
return Response::redirect('/admin/login');
}
return $handler($request);
};
};
}
}
|
Add a note acknowledging the source of this class | """
Registry class and global node registry.
This class was adapted from the Celery Project's task registry.
"""
import inspect
class NotRegistered(KeyError):
pass
class NodeRegistry(dict):
NotRegistered = NotRegistered
def register(self, node):
"""Register a node class in the node registry."""
self[node.name] = inspect.isclass(node) and node or node.__class__
def unregister(self, name):
"""Unregister node by name."""
try:
# Might be a node class
name = name.name
except AttributeError:
pass
self.pop(name)
def get_by_attr(self, attr, value=None):
"""Return all nodes of a specific type that have a matching attr.
If `value` is given, only return nodes where the attr value matches."""
ret = {}
for name, node in self.iteritems():
if hasattr(node, attr) and value is None\
or hasattr(node, name) and getattr(node, name) == value:
ret[name] = node
return ret
def __getitem__(self, key):
try:
return dict.__getitem__(self, key)
except KeyError:
raise self.NotRegistered(key)
def pop(self, key, *args):
try:
return dict.pop(self, key, *args)
except KeyError:
raise self.NotRegistered(key)
nodes = NodeRegistry()
| """
Registry class and global node registry.
"""
import inspect
class NotRegistered(KeyError):
pass
class NodeRegistry(dict):
NotRegistered = NotRegistered
def register(self, node):
"""Register a node class in the node registry."""
self[node.name] = inspect.isclass(node) and node or node.__class__
def unregister(self, name):
"""Unregister node by name."""
try:
# Might be a node class
name = name.name
except AttributeError:
pass
self.pop(name)
def get_by_attr(self, attr, value=None):
"""Return all nodes of a specific type that have a matching attr.
If `value` is given, only return nodes where the attr value matches."""
ret = {}
for name, node in self.iteritems():
if hasattr(node, attr) and value is None\
or hasattr(node, name) and getattr(node, name) == value:
ret[name] = node
return ret
def __getitem__(self, key):
try:
return dict.__getitem__(self, key)
except KeyError:
raise self.NotRegistered(key)
def pop(self, key, *args):
try:
return dict.pop(self, key, *args)
except KeyError:
raise self.NotRegistered(key)
nodes = NodeRegistry()
|
Remove colors from REPL prompt
They weren't playing nice with Readline.
There's still an optional dependency on Blessings, but that is only
used to strip away the trailing ps2. | from . import *
import readline
ps1 = '\n% '
ps2 = '| '
try:
from blessings import Terminal
term = Terminal()
def fancy_movement():
print(term.move_up() + term.clear_eol() + term.move_up())
except ImportError:
def fancy_movement():
pass
def getfilefunc(mod, droplast=True):
return Func(tuple(fixtags(flattenbody(mod, droplast=droplast))))
def runfile(fname):
invoke(getfilefunc(parseFile(fname)), stdlib())
def readProgram():
try:
yield input(ps1)
while True:
line = input(ps2)
if not line:
fancy_movement()
return
yield line
except EOFError:
print()
raise SystemExit
def interactive():
env = stdlib()
while True:
try:
retval, = invoke(getfilefunc(parseString('\n'.join(readProgram())), droplast=False), env)
if retval is not None:
print(arepr(retval))
except KeyboardInterrupt:
print()
except Exception as e:
print(e)
import sys
if len(sys.argv) > 1:
runfile(sys.argv[1])
else:
interactive()
| from . import *
import readline
ps1 = '\n% '
ps2 = '| '
try:
from blessings import Terminal
term = Terminal()
ps1 = term.bold_blue(ps1)
ps2 = term.bold_blue(ps2)
def fancy_movement():
print(term.move_up() + term.clear_eol() + term.move_up())
except ImportError:
def fancy_movement():
pass
def getfilefunc(mod, droplast=True):
return Func(tuple(fixtags(flattenbody(mod, droplast=droplast))))
def runfile(fname):
invoke(getfilefunc(parseFile(fname)), stdlib())
def readProgram():
try:
yield input(ps1)
while True:
line = input(ps2)
if not line:
fancy_movement()
return
yield line
except EOFError:
print()
raise SystemExit
def interactive():
env = stdlib()
while True:
try:
retval, = invoke(getfilefunc(parseString('\n'.join(readProgram())), droplast=False), env)
if retval is not None:
print(arepr(retval))
except KeyboardInterrupt:
print()
except Exception as e:
print(e)
import sys
if len(sys.argv) > 1:
runfile(sys.argv[1])
else:
interactive()
|
Make jumping between changes loop back around | import sublime
import sublime_plugin
try:
from GitGutter.view_collection import ViewCollection
except ImportError:
from view_collection import ViewCollection
class GitGutterBaseChangeCommand(sublime_plugin.WindowCommand):
def lines_to_blocks(self, lines):
blocks = []
last_line = -2
for line in lines:
if line > last_line+1:
blocks.append(line)
last_line = line
return blocks
def run(self):
view = self.window.active_view()
inserted, modified, deleted = ViewCollection.diff(view)
inserted = self.lines_to_blocks(inserted)
modified = self.lines_to_blocks(modified)
all_changes = sorted(inserted + modified + deleted)
row, col = view.rowcol(view.sel()[0].begin())
current_row = row + 1
line = self.jump(all_changes, current_row)
self.window.active_view().run_command("goto_line", {"line": line})
class GitGutterNextChangeCommand(GitGutterBaseChangeCommand):
def jump(self, all_changes, current_row):
return next((change for change in all_changes
if change > current_row), all_changes[0])
class GitGutterPrevChangeCommand(GitGutterBaseChangeCommand):
def jump(self, all_changes, current_row):
return next((change for change in reversed(all_changes)
if change < current_row), all_changes[-1]) | import sublime
import sublime_plugin
try:
from GitGutter.view_collection import ViewCollection
except ImportError:
from view_collection import ViewCollection
class GitGutterBaseChangeCommand(sublime_plugin.WindowCommand):
def lines_to_blocks(self, lines):
blocks = []
last_line = -2
for line in lines:
if line > last_line+1:
blocks.append(line)
last_line = line
return blocks
def run(self):
view = self.window.active_view()
inserted, modified, deleted = ViewCollection.diff(view)
inserted = self.lines_to_blocks(inserted)
modified = self.lines_to_blocks(modified)
all_changes = sorted(inserted + modified + deleted)
row, col = view.rowcol(view.sel()[0].begin())
current_row = row + 1
line = self.jump(all_changes, current_row)
self.window.active_view().run_command("goto_line", {"line": line})
class GitGutterNextChangeCommand(GitGutterBaseChangeCommand):
def jump(self, all_changes, current_row):
return next((change for change in all_changes
if change > current_row), current_row)
class GitGutterPrevChangeCommand(GitGutterBaseChangeCommand):
def jump(self, all_changes, current_row):
return next((change for change in reversed(all_changes)
if change < current_row), current_row) |
Add subdomain and context to mock | # -*- coding: utf-8 -*-
try:
from unittest import mock
except ImportError:
import mock
class MockFulfil(object):
"""
A Mock object that helps mock away the Fulfil API
for testing.
"""
responses = []
models = {}
context = {}
subdomain = 'mock-test'
def __init__(self, target, responses=None):
self.target = target
self.reset_mocks()
if responses:
self.responses.extend(responses)
def __enter__(self):
self.start()
return self
def __exit__(self, type, value, traceback):
self.stop()
self.reset_mocks()
return type is None
def model(self, model_name):
return self.models.setdefault(
model_name, mock.MagicMock(name=model_name)
)
def start(self):
"""
Start the patch
"""
self._patcher = mock.patch(target=self.target)
MockClient = self._patcher.start()
instance = MockClient.return_value
instance.model.side_effect = mock.Mock(
side_effect=self.model
)
def stop(self):
"""
End the patch
"""
self._patcher.stop()
def reset_mocks(self):
"""
Reset all the mocks
"""
self.models = {}
self.context = {}
| # -*- coding: utf-8 -*-
try:
from unittest import mock
except ImportError:
import mock
class MockFulfil(object):
"""
A Mock object that helps mock away the Fulfil API
for testing.
"""
responses = []
models = {}
def __init__(self, target, responses=None):
self.target = target
self.reset_mocks()
if responses:
self.responses.extend(responses)
def __enter__(self):
self.start()
return self
def __exit__(self, type, value, traceback):
self.stop()
self.reset_mocks()
return type is None
def model(self, model_name):
return self.models.setdefault(
model_name, mock.MagicMock(name=model_name)
)
def start(self):
"""
Start the patch
"""
self._patcher = mock.patch(target=self.target)
MockClient = self._patcher.start()
instance = MockClient.return_value
instance.model.side_effect = mock.Mock(
side_effect=self.model
)
def stop(self):
"""
End the patch
"""
self._patcher.stop()
def reset_mocks(self):
"""
Reset all the mocks
"""
self.models = {}
|
Add filter.rank to __all__ of filter package | from .lpi_filter import inverse, wiener, LPIFilter2D
from .ctmf import median_filter
from ._canny import canny
from .edges import (sobel, hsobel, vsobel, scharr, hscharr, vscharr, prewitt,
hprewitt, vprewitt, roberts , roberts_positive_diagonal,
roberts_negative_diagonal)
from ._denoise import denoise_tv_chambolle, tv_denoise
from ._denoise_cy import denoise_bilateral, denoise_tv_bregman
from ._rank_order import rank_order
from ._gabor import gabor_kernel, gabor_filter
from .thresholding import threshold_otsu, threshold_adaptive
from . import rank
__all__ = ['inverse',
'wiener',
'LPIFilter2D',
'median_filter',
'canny',
'sobel',
'hsobel',
'vsobel',
'scharr',
'hscharr',
'vscharr',
'prewitt',
'hprewitt',
'vprewitt',
'roberts',
'roberts_positive_diagonal',
'roberts_negative_diagonal',
'denoise_tv_chambolle',
'tv_denoise',
'denoise_bilateral',
'denoise_tv_bregman',
'rank_order',
'gabor_kernel',
'gabor_filter',
'threshold_otsu',
'threshold_adaptive',
'rank']
| from .lpi_filter import inverse, wiener, LPIFilter2D
from .ctmf import median_filter
from ._canny import canny
from .edges import (sobel, hsobel, vsobel, scharr, hscharr, vscharr, prewitt,
hprewitt, vprewitt, roberts , roberts_positive_diagonal,
roberts_negative_diagonal)
from ._denoise import denoise_tv_chambolle, tv_denoise
from ._denoise_cy import denoise_bilateral, denoise_tv_bregman
from ._rank_order import rank_order
from ._gabor import gabor_kernel, gabor_filter
from .thresholding import threshold_otsu, threshold_adaptive
__all__ = ['inverse',
'wiener',
'LPIFilter2D',
'median_filter',
'canny',
'sobel',
'hsobel',
'vsobel',
'scharr',
'hscharr',
'vscharr',
'prewitt',
'hprewitt',
'vprewitt',
'roberts',
'roberts_positive_diagonal',
'roberts_negative_diagonal',
'denoise_tv_chambolle',
'tv_denoise',
'denoise_bilateral',
'denoise_tv_bregman',
'rank_order',
'gabor_kernel',
'gabor_filter',
'threshold_otsu',
'threshold_adaptive']
|
Add login functionality with test | process.env.NODE_ENV = 'test';
const supertest = require('supertest');
const chai = require('chai');
const chaiHttp = require('chai-http');
const app = require('../app.js');
const request = supertest(app);
const expect = chai.expect;
const User = require('../models').User;
// const should = chai.should();
chai.use(chaiHttp);
describe('Routes: signin', () => {
describe('POST /api/user/signin', () => {
// This function will run before every test to clear database
before((done) => {
User.sync({ force: true }) // drops table and re-creates it
.then(() => {
User.create({
email: '[email protected]',
username: 'blessing',
password: '1234'
});
done();
})
.catch((error) => {
done(error);
});
});
describe('status 200', () => {
it('returns authenticated user token', (done) => {
// Test's logic...
const user = {
username: 'ayeni',
password: '1234'
};
request.post('/api/user/signin')
.send(user)
.expect(200)
.end((err, res) => {
expect(res.body).to.be.an('object');
done(err);
});
});
});
});
});
| process.env.NODE_ENV = 'test';
const supertest = require('supertest');
const chai = require('chai');
const chaiHttp = require('chai-http');
const app = require('../app.js');
const request = supertest(app);
const expect = chai.expect;
const User = require('../models').User;
// const should = chai.should();
chai.use(chaiHttp);
describe('Routes: signin', () => {
describe('POST /api/user/signin', () => {
// This function will run before every test to clear database
before((done) => {
User.sync({ force: true }) // drops table and re-creates it
.then(() => {
User.create({
email: '[email protected]',
username: 'blessing',
password: '1234'
});
done();
})
.catch((error) => {
done(error);
});
});
describe('status 200', () => {
it('returns authenticated user token', (done) => {
// Test's logic...
const user = {
username: 'tobi',
password: '1234'
};
request.post('/api/user/signup')
.send(user)
.expect(200)
.end((err, res) => {
expect(res.body).to.include.keys('Token');
done(err);
});
});
});
});
});
|
Use local variable instead of member variable | package fi.nls.oskari.routing;
import com.ibatis.common.resources.Resources;
import com.ibatis.sqlmap.client.SqlMapClient;
import com.ibatis.sqlmap.client.SqlMapClientBuilder;
import java.io.Reader;
public class RoutingService {
private SqlMapClient client = null;
private static String SQL_MAP_LOCATION = "META-INF/SqlMapConfig.xml";
private SqlMapClient getSqlMapClient() {
if (client != null) { return client; }
Reader reader = null;
try {
reader = Resources.getResourceAsReader(SQL_MAP_LOCATION);
client = SqlMapClientBuilder.buildSqlMapClient(reader);
return client;
} catch (Exception e) {
throw new RuntimeException("Failed to retrieve SQL client", e);
} finally {
if (reader != null) {
try {
reader.close();
} catch (Exception e) {
throw new RuntimeException(e);
}
}
}
}
public Long hevonen() {
try {
SqlMapClient client = getSqlMapClient();
Long results = (Long)client.queryForObject("Routing.foo");
return results;
} catch (Exception e) {
System.out.println(e.getMessage());
throw new RuntimeException("Failed to query", e);
}
}
}
| package fi.nls.oskari.routing;
import com.ibatis.common.resources.Resources;
import com.ibatis.sqlmap.client.SqlMapClient;
import com.ibatis.sqlmap.client.SqlMapClientBuilder;
import java.io.Reader;
public class RoutingService {
private SqlMapClient client = null;
private static String SQL_MAP_LOCATION = "META-INF/SqlMapConfig.xml";
protected SqlMapClient getSqlMapClient() {
if (client != null) {
return client;
}
Reader reader = null;
try {
reader = Resources.getResourceAsReader(SQL_MAP_LOCATION);
client = SqlMapClientBuilder.buildSqlMapClient(reader);
return client;
} catch (Exception e) {
throw new RuntimeException("Failed to retrieve SQL client", e);
} finally {
if (reader != null) {
try {
reader.close();
} catch (Exception e) {
throw new RuntimeException(e);
}
}
}
}
public Long hevonen() {
try {
client = getSqlMapClient();
Long results = (Long)client.queryForObject("Routing.foo");
return results;
} catch (Exception e) {
System.out.println(e.getMessage());
throw new RuntimeException("Failed to query", e);
}
}
}
|
Throw the execution error of not a 404 | package com.scoopit.example;
import com.scoopit.client.ScoopApiExecutionException;
import com.scoopit.client.ScoopClient;
import com.scoopit.model.Post;
import com.scoopit.model.Topic;
import com.scoopit.model.User;
public class GetTopicAndUser {
public static void main(String[] args) throws ScoopApiExecutionException {
// get yours on https://www.scoop.it/dev/apps
ScoopClient scoopit = new ScoopClient("<youapikey>", "<youapisecret>");
System.out.println("============******===========");
Topic t = scoopit.getTopic(9014);
System.out.println(t);
for (Post p : t.curatedPosts) {
System.out.println(p);
}
System.out.println("============******===========");
User u = scoopit.getUser(0l);
System.out.println(u);
System.out.println("============******===========");
System.out.println(scoopit.resolveId("fixies", Type.Topic));
try {
System.out.println(scoopit.resolveId("some-topic-that-should-,not-exists", Type.Topic));
} catch (ScoopApiExecutionException e) {
if (e.response.getCode() == 404) {
System.out.println("=> not found!");
} else {
throw e;
}
}
}
}
| package com.scoopit.example;
import com.scoopit.client.ScoopApiExecutionException;
import com.scoopit.client.ScoopClient;
import com.scoopit.model.Post;
import com.scoopit.model.Topic;
import com.scoopit.model.User;
public class GetTopicAndUser {
public static void main(String[] args) throws ScoopApiExecutionException {
// get yours on https://www.scoop.it/dev/apps
ScoopClient scoopit = new ScoopClient("<youapikey>", "<youapisecret>");
System.out.println("============******===========");
Topic t = scoopit.getTopic(9014);
System.out.println(t);
for (Post p : t.curatedPosts) {
System.out.println(p);
}
System.out.println("============******===========");
User u = scoopit.getUser(0l);
System.out.println(u);
System.out.println("============******===========");
System.out.println(scoopit.resolveId("fixies", Type.Topic));
try {
System.out.println(scoopit.resolveId("some-topic-that-should-,not-exists", Type.Topic));
} catch (ScoopApiExecutionException e) {
if (e.response.getCode() == 404) {
System.out.println("=> not found!");
}
}
}
}
|
Add repoze.{squeeze,profile} and stompservice to our dependency list | from setuptools import setup, find_packages
setup(
name='moksha',
version='0.1',
description='',
author='',
author_email='',
#url='',
install_requires=[
"TurboGears2",
"ToscaWidgets >= 0.9.1",
"zope.sqlalchemy",
"Shove",
"feedcache",
"feedparser",
"tw.jquery",
"repoze.squeeze",
"repoze.profile",
"stompservice"
],
packages=find_packages(exclude=['ez_setup']),
include_package_data=True,
test_suite='nose.collector',
tests_require=['WebTest', 'BeautifulSoup'],
package_data={'moksha': ['i18n/*/LC_MESSAGES/*.mo',
'templates/*/*',
'public/*/*']},
#message_extractors = {'moksha': [
# ('**.py', 'python', None),
# ('templates/**.mako', 'mako', None),
# ('templates/**.html', 'genshi', None),
# ('public/**', 'ignore', None)]},
entry_points="""
[paste.app_factory]
main = moksha.config.middleware:make_app
[paste.app_install]
main = pylons.util:PylonsInstaller
[moksha.widget]
orbited = moksha.hub:OrbitedWidget
""",
)
| from setuptools import setup, find_packages
setup(
name='moksha',
version='0.1',
description='',
author='',
author_email='',
#url='',
install_requires=[
"TurboGears2",
"ToscaWidgets >= 0.9.1",
"zope.sqlalchemy",
"Shove",
"feedcache",
"feedparser",
"tw.jquery",
],
packages=find_packages(exclude=['ez_setup']),
include_package_data=True,
test_suite='nose.collector',
tests_require=['WebTest', 'BeautifulSoup'],
package_data={'moksha': ['i18n/*/LC_MESSAGES/*.mo',
'templates/*/*',
'public/*/*']},
#message_extractors = {'moksha': [
# ('**.py', 'python', None),
# ('templates/**.mako', 'mako', None),
# ('templates/**.html', 'genshi', None),
# ('public/**', 'ignore', None)]},
entry_points="""
[paste.app_factory]
main = moksha.config.middleware:make_app
[paste.app_install]
main = pylons.util:PylonsInstaller
[moksha.widget]
orbited = moksha.hub:OrbitedWidget
""",
)
|
Make name of pdf the name of the file with .pdf appended | class MCProjectDatasetsViewContainerComponentController {
/*@ngInject*/
constructor(mcdsstore, $stateParams, datasetsAPI, mcStateStore, projectDatasetsViewService) {
this.mcdsstore = mcdsstore;
this.$stateParams = $stateParams;
this.datasetsAPI = datasetsAPI;
this.mcStateStore = mcStateStore;
this.projectDatasetsViewService = projectDatasetsViewService;
this.state = {
datasets: []
};
}
$onInit() {
this.loadDatasets();
}
loadDatasets() {
this.datasetsAPI.getDatasetsForProject(this.$stateParams.project_id).then(
(datasets) => {
let p = this.mcStateStore.getState('project');
let transformed = [];
datasets.forEach(ds => {
transformed.push(this.mcdsstore.transformDataset(ds, p));
});
this.mcdsstore.reloadDatasets(transformed);
this.state.datasets = this.mcdsstore.getDatasets();
}
);
}
handleNewDataset() {
this.loadDatasets();
}
}
angular.module('materialscommons').component('mcProjectDatasetsViewContainer', {
template: `
<mc-project-datasets-view datasets="$ctrl.state.datasets" on-new-dataset="$ctrl.handleNewDataset(dataset)">
</mc-project-datasets-view>`,
controller: MCProjectDatasetsViewContainerComponentController
}); | class MCProjectDatasetsViewContainerComponentController {
/*@ngInject*/
constructor(mcdsstore, $stateParams, datasetsAPI, mcprojectstore2, projectDatasetsViewService) {
this.mcdsstore = mcdsstore;
this.$stateParams = $stateParams;
this.datasetsAPI = datasetsAPI;
this.mcprojectstore = mcprojectstore2;
this.projectDatasetsViewService = projectDatasetsViewService;
this.state = {
datasets: []
};
}
$onInit() {
this.loadDatasets();
}
loadDatasets() {
this.datasetsAPI.getDatasetsForProject(this.$stateParams.project_id).then(
(datasets) => {
let p = this.mcprojectstore.getCurrentProject();
let transformed = [];
datasets.forEach(ds => {
transformed.push(this.mcdsstore.transformDataset(ds, p));
});
this.mcdsstore.reloadDatasets(transformed);
this.state.datasets = this.mcdsstore.getDatasets();
}
);
}
handleNewDataset() {
this.loadDatasets();
}
}
angular.module('materialscommons').component('mcProjectDatasetsViewContainer', {
template: `
<mc-project-datasets-view datasets="$ctrl.state.datasets" on-new-dataset="$ctrl.handleNewDataset(dataset)">
</mc-project-datasets-view>`,
controller: MCProjectDatasetsViewContainerComponentController
}); |
Fix NPE when no adding parameters or exceptions | package asteroid.nodes;
import asteroid.A;
import java.util.List;
import java.util.ArrayList;
import org.codehaus.groovy.ast.Parameter;
import org.codehaus.groovy.ast.ClassNode;
import org.codehaus.groovy.ast.MethodNode;
import org.codehaus.groovy.ast.stmt.Statement;
/**
* Builder to create instance of type {@link MethodNode}
*
* @since 0.1.0
*/
public class MethodNodeBuilder {
private final String name;
private int modifiers;
private Statement code;
private ClassNode returnType;
private Parameter[] parameters = new Parameter[0];
private ClassNode[] exceptions = new ClassNode[0];
private MethodNodeBuilder(String name) {
this.name = name;
}
public static MethodNodeBuilder method(String name) {
return new MethodNodeBuilder(name);
}
public MethodNodeBuilder returnType(Class returnType) {
this.returnType = A.NODES.clazz(returnType).build();
return this;
}
public MethodNodeBuilder modifiers(int modifiers) {
this.modifiers = modifiers;
return this;
}
public MethodNodeBuilder parameters(Parameter... parameters) {
this.parameters = parameters;
return this;
}
public MethodNodeBuilder exceptions(ClassNode... exceptions) {
this.exceptions = exceptions;
return this;
}
public MethodNodeBuilder code(Statement code) {
this.code = code;
return this;
}
public MethodNode build() {
MethodNode methodNode =
new MethodNode(name,
modifiers,
returnType,
parameters,
exceptions,
code);
return methodNode;
}
}
| package asteroid.nodes;
import asteroid.A;
import java.util.List;
import java.util.ArrayList;
import org.codehaus.groovy.ast.Parameter;
import org.codehaus.groovy.ast.ClassNode;
import org.codehaus.groovy.ast.MethodNode;
import org.codehaus.groovy.ast.stmt.Statement;
/**
* Builder to create instance of type {@link MethodNode}
*
* @since 0.1.0
*/
public class MethodNodeBuilder {
private final String name;
private int modifiers;
private Statement code;
private ClassNode returnType;
private Parameter[] parameters;
private ClassNode[] exceptions;
private MethodNodeBuilder(String name) {
this.name = name;
}
public static MethodNodeBuilder method(String name) {
return new MethodNodeBuilder(name);
}
public MethodNodeBuilder returnType(Class returnType) {
this.returnType = A.NODES.clazz(returnType).build();
return this;
}
public MethodNodeBuilder modifiers(int modifiers) {
this.modifiers = modifiers;
return this;
}
public MethodNodeBuilder parameters(Parameter... parameters) {
this.parameters = parameters;
return this;
}
public MethodNodeBuilder exceptions(ClassNode... exceptions) {
this.exceptions = exceptions;
return this;
}
public MethodNodeBuilder code(Statement code) {
this.code = code;
return this;
}
public MethodNode build() {
MethodNode methodNode =
new MethodNode(name,
modifiers,
returnType,
parameters,
exceptions,
code);
return methodNode;
}
}
|
Add a check to ensure apiKey is included in config when using GeoCarrot’s GeoIP lookup. | export default class UserLocation {
constructor({
apiKey = null,
cacheTtl = 604800, // 7 days
fallback = 'exact', // If IP-based geolocation fails
specificity = 'general',
}) {
let coordsLoaded = false;
const coords = {
latitude: null,
longitude: null,
accuracy: null,
};
if (apiKey === null && (specificity === 'general' || fallback === 'general')) {
throw new Error('An API key must be included when using GeoCarrot\'s GeoIP lookup.');
}
const promise = new Promise((resolve, reject) => {
if (coordsLoaded) {
resolve(coords);
} else if (specificity === 'exact') {
navigator.geolocation.getCurrentPosition(
(pos) => {
coordsLoaded = true;
coords.latitude = pos.coords.latitude;
coords.longitude = pos.coords.longitude;
coords.accuracy = pos.coords.accuracy;
resolve(coords);
},
(err) => {
reject(`${err.message} (error code: ${err.code})`);
}
);
} else if (specificity === 'general') {
// Use GeoIP lookup to get general area
} else {
throw new Error('Invalid configuration value for location specificity.');
}
});
console.log(apiKey, cacheTtl, fallback);
return promise;
}
}
| export default class UserLocation {
constructor({
apiKey,
cacheTtl = 604800, // 7 days
fallback = 'exact', // If IP-based geolocation fails
specificity = 'general',
}) {
let coordsLoaded = false;
const coords = {
latitude: null,
longitude: null,
accuracy: null,
};
const promise = new Promise((resolve, reject) => {
if (coordsLoaded) {
resolve(coords);
} else if (specificity === 'exact') {
navigator.geolocation.getCurrentPosition(
(pos) => {
coordsLoaded = true;
coords.latitude = pos.coords.latitude;
coords.longitude = pos.coords.longitude;
coords.accuracy = pos.coords.accuracy;
resolve(coords);
},
(err) => {
reject(`${err.message} (error code: ${err.code})`);
}
);
} else if (specificity === 'general') {
// Use GeoIP lookup to get general area
} else {
throw new Error('Invalid configuration value for location specificity.');
}
});
console.log(apiKey, cacheTtl, fallback);
return promise;
}
}
|
Expand default test years for PUDL to 2011-2015
Tests successfully passed for all EIA923 tables ingesting from 2011
through 2015 (2016 still needs some id_mapping or exhaustie ID love). | """Tests excercising the pudl module for use with PyTest."""
import pytest
import pudl.pudl
import pudl.ferc1
import pudl.constants as pc
def test_init_db():
"""Create a fresh PUDL DB and pull in some FERC1 & EIA923 data."""
pudl.ferc1.init_db(refyear=2015,
years=range(2007, 2016),
def_db=True,
verbose=True,
testing=True)
pudl.pudl.init_db(ferc1_tables=pc.ferc1_pudl_tables,
ferc1_years=range(2007, 2016),
eia923_tables=pc.eia923_pudl_tables,
eia923_years=range(2011, 2016),
verbose=True,
debug=False,
testing=True)
ferc1_engine = pudl.ferc1.db_connect_ferc1(testing=True)
pudl.ferc1.drop_tables_ferc1(ferc1_engine)
pudl_engine = pudl.pudl.db_connect_pudl(testing=True)
pudl.pudl.drop_tables_pudl(pudl_engine)
| """Tests excercising the pudl module for use with PyTest."""
import pytest
import pudl.pudl
import pudl.ferc1
import pudl.constants as pc
def test_init_db():
"""Create a fresh PUDL DB and pull in some FERC1 & EIA923 data."""
pudl.ferc1.init_db(refyear=2015,
years=range(2007, 2016),
def_db=True,
verbose=True,
testing=True)
pudl.pudl.init_db(ferc1_tables=pc.ferc1_pudl_tables,
ferc1_years=range(2007, 2016),
eia923_tables=pc.eia923_pudl_tables,
eia923_years=range(2014, 2016),
verbose=True,
debug=False,
testing=True)
ferc1_engine = pudl.ferc1.db_connect_ferc1(testing=True)
pudl.ferc1.drop_tables_ferc1(ferc1_engine)
pudl_engine = pudl.pudl.db_connect_pudl(testing=True)
pudl.pudl.drop_tables_pudl(pudl_engine)
|
Fix integration with premium version of PvPManager | package com.elmakers.mine.bukkit.protection;
import me.NoChance.PvPManager.PvPManager;
import me.NoChance.PvPManager.PvPlayer;
import org.bukkit.Location;
import org.bukkit.entity.Player;
import org.bukkit.plugin.Plugin;
/**
* This a manager specifically for the PvPManager plugin.
*
* This is why it has kind of a funky name.
*/
public class PvPManagerManager implements PVPManager {
private boolean enabled = false;
private PvPManager manager = null;
public void setEnabled(boolean enabled) {
this.enabled = enabled;
}
public boolean isEnabled() {
return enabled && manager != null;
}
public void initialize(Plugin plugin) {
if (enabled) {
try {
Plugin pvpPlugin = plugin.getServer().getPluginManager().getPlugin("PvPManager");
if (pvpPlugin instanceof PvPManager) {
manager = (PvPManager)pvpPlugin;
}
} catch (Throwable ex) {
}
if (manager != null) {
plugin.getLogger().info("PvPManager found, will respect PVP settings");
}
} else {
manager = null;
}
}
@Override
public boolean isPVPAllowed(Player player, Location location) {
if (!enabled || manager == null || player == null) return true;
PvPlayer pvpPlayer = PvPlayer.get(player);
return pvpPlayer != null && pvpPlayer.hasPvPEnabled();
}
}
| package com.elmakers.mine.bukkit.protection;
import me.NoChance.PvPManager.PvPManager;
import me.NoChance.PvPManager.PvPlayer;
import org.bukkit.Location;
import org.bukkit.entity.Player;
import org.bukkit.plugin.Plugin;
/**
* This a manager specifically for the PvPManager plugin.
*
* This is why it has kind of a funky name.
*/
public class PvPManagerManager implements PVPManager {
private boolean enabled = false;
private PvPManager manager = null;
public void setEnabled(boolean enabled) {
this.enabled = enabled;
}
public boolean isEnabled() {
return enabled && manager != null;
}
public void initialize(Plugin plugin) {
if (enabled) {
try {
Plugin pvpPlugin = plugin.getServer().getPluginManager().getPlugin("PvPManager");
if (pvpPlugin instanceof PvPManager) {
manager = (PvPManager)pvpPlugin;
}
} catch (Throwable ex) {
}
if (manager != null) {
plugin.getLogger().info("PvPManager found, will respect PVP settings");
}
} else {
manager = null;
}
}
@Override
public boolean isPVPAllowed(Player player, Location location) {
if (!enabled || manager == null || player == null) return true;
PvPlayer pvpPlayer = manager.getPlayerHandler().get(player);
return pvpPlayer != null && pvpPlayer.hasPvPEnabled();
}
}
|
Configure gh-pages commit user name and email | module.exports = function (grunt) {
'use strict';
grunt.loadNpmTasks('grunt-contrib-connect');
grunt.loadNpmTasks('grunt-contrib-uglify');
grunt.loadNpmTasks('grunt-gh-pages');
grunt.initConfig({
'connect': {
demo: {
options: {
open: {
target: 'http://localhost:8000/'
},
keepalive: true
}
}
},
pkg: grunt.file.readJSON('bower.json'),
uglify: {
rating: {
files: {
'bootstrap-rating.min.js': ['bootstrap-rating.js']
}
},
options: {
banner:
'// <%= pkg.name %> - v<%= pkg.version %> - (c) <%= grunt.template.today("yyyy") %> <%= pkg.author.name %> \n' +
'// <%= pkg.homepage %> <%= pkg.license %>\n'
}
},
'gh-pages': {
options: {
user: {
name: '<%= pkg.author.name %>',
email: '<%= pkg.author.email %>'
}
},
src: [
'index.html',
'bootstrap-rating.js',
'bootstrap-rating.css',
'bower_components/**/*'
]
}
});
grunt.registerTask('serve', ['connect']);
grunt.registerTask('deploy', ['gh-pages']);
};
| module.exports = function (grunt) {
'use strict';
grunt.loadNpmTasks('grunt-contrib-connect');
grunt.loadNpmTasks('grunt-contrib-uglify');
grunt.loadNpmTasks('grunt-gh-pages');
grunt.initConfig({
'connect': {
demo: {
options: {
open: {
target: 'http://localhost:8000/'
},
keepalive: true
}
}
},
pkg: grunt.file.readJSON('bower.json'),
uglify: {
rating: {
files: {
'bootstrap-rating.min.js': ['bootstrap-rating.js']
}
},
options: {
banner:
'// <%= pkg.name %> - v<%= pkg.version %> - (c) <%= grunt.template.today("yyyy") %> <%= pkg.author.name %> \n' +
'// <%= pkg.homepage %> <%= pkg.license %>\n'
}
},
'gh-pages': {
src: [
'index.html',
'bootstrap-rating.js',
'bootstrap-rating.css',
'bower_components/**/*'
]
}
});
grunt.registerTask('serve', ['connect']);
grunt.registerTask('deploy', ['gh-pages']);
};
|
Add support for null values in property mapping | from types import UnicodeType, StringType
class PropertyMappingFailedException(Exception):
pass
def get_transformed_properties(source_properties, prop_map):
results = {}
for key, value in prop_map.iteritems():
if type(value) in (StringType, UnicodeType):
if value in source_properties:
results[key] = source_properties[value]
else:
raise PropertyMappingFailedException("property %s not found in source feature" %
(value))
elif type(value) == dict:
if "static" in value:
results[key] = value["static"]
elif "mapping" in value:
if not "key" in value:
raise PropertyMappingFailedException(
"Found mapping, but no key specified to map")
source_value = source_properties[value['key']]
if source_value is None:
source_value = "null"
if source_value in value['mapping']:
results[key] = value['mapping'][source_value]
else:
raise PropertyMappingFailedException(
"value:%s not found in mapping for key:%s" %
(source_value, key))
else:
raise PropertyMappingFailedException(
"Failed to find key for mapping in dict for field:%s" %
(key,))
else:
raise PropertyMappingFailedException("Unhandled mapping for key:%s value type:%s" %
(key, type(value)))
return results
| from types import UnicodeType, StringType
class PropertyMappingFailedException(Exception):
pass
def get_transformed_properties(source_properties, prop_map):
results = {}
for key, value in prop_map.iteritems():
if type(value) in (StringType, UnicodeType):
if value in source_properties:
results[key] = source_properties[value]
else:
raise PropertyMappingFailedException("property %s not found in source feature" %
(value))
elif type(value) == dict:
if "static" in value:
results[key] = value["static"]
elif "mapping" in value:
if not "key" in value:
raise PropertyMappingFailedException(
"Found mapping, but no key specified to map")
source_value = source_properties[value['key']]
if source_value in value['mapping']:
results[key] = value['mapping'][source_value]
else:
raise PropertyMappingFailedException(
"value:%s not found in mapping for key:%s" %
(source_value, key))
else:
raise PropertyMappingFailedException(
"Failed to find key for mapping in dict for field:%s" %
(key,))
else:
raise PropertyMappingFailedException("Unhandled mapping for key:%s value type:%s" %
(key, type(value)))
return results
|
Speed up icons module import
## What was the problem?
I observed that doing a simple import of bootstrap-vue:
```js
import BootstrapVue from 'bootstrap-vue';
```
takes hundreds of milliseconds (give or take, depending on user's CPU).
I tried to do some profiling and found out that most of the time is
spent in `icons` module, specifically in `makeIcon`:
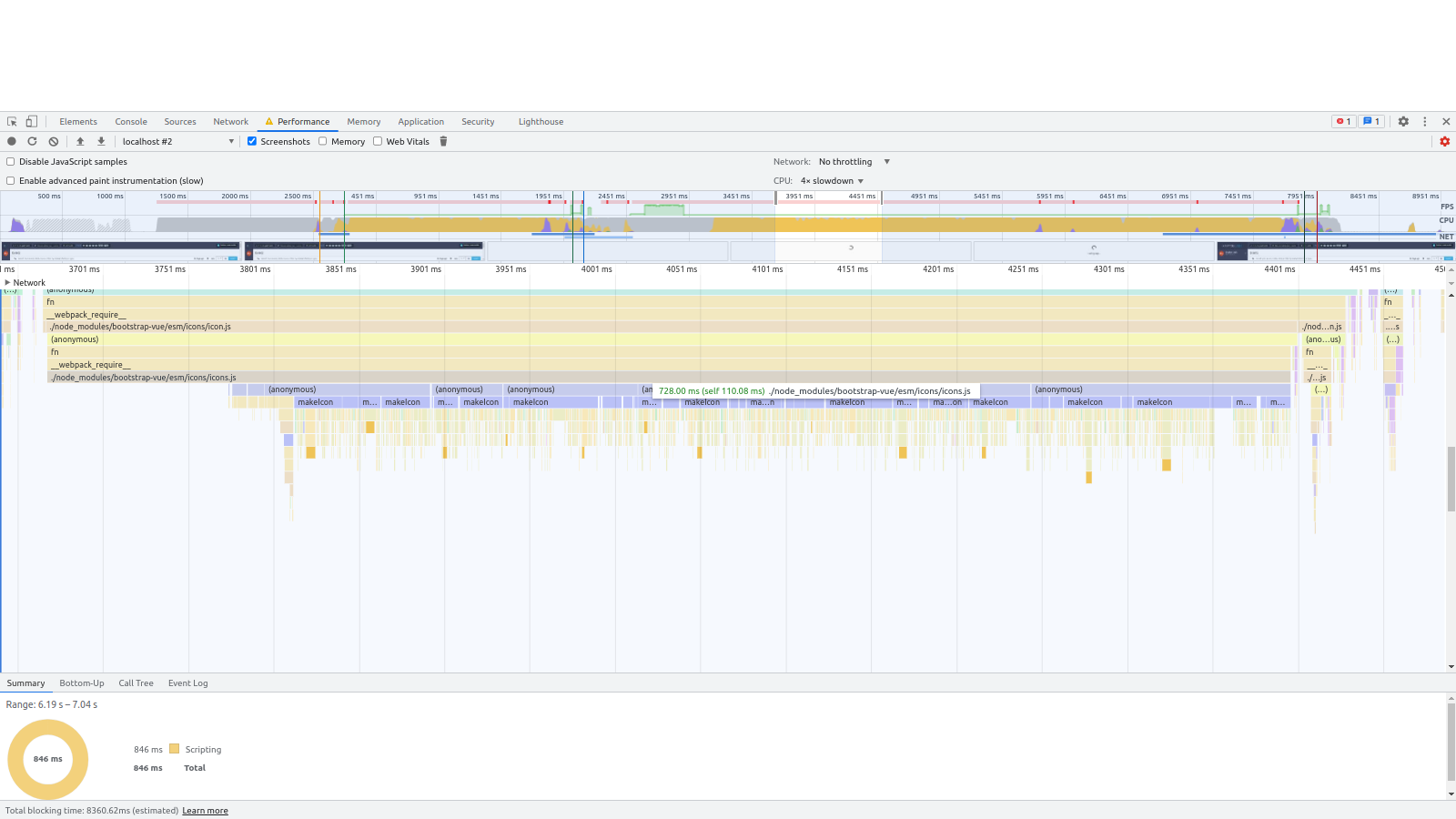
When zoomed it, you can see that most of the time is spent in functions `omit` and `Vue.extend`:
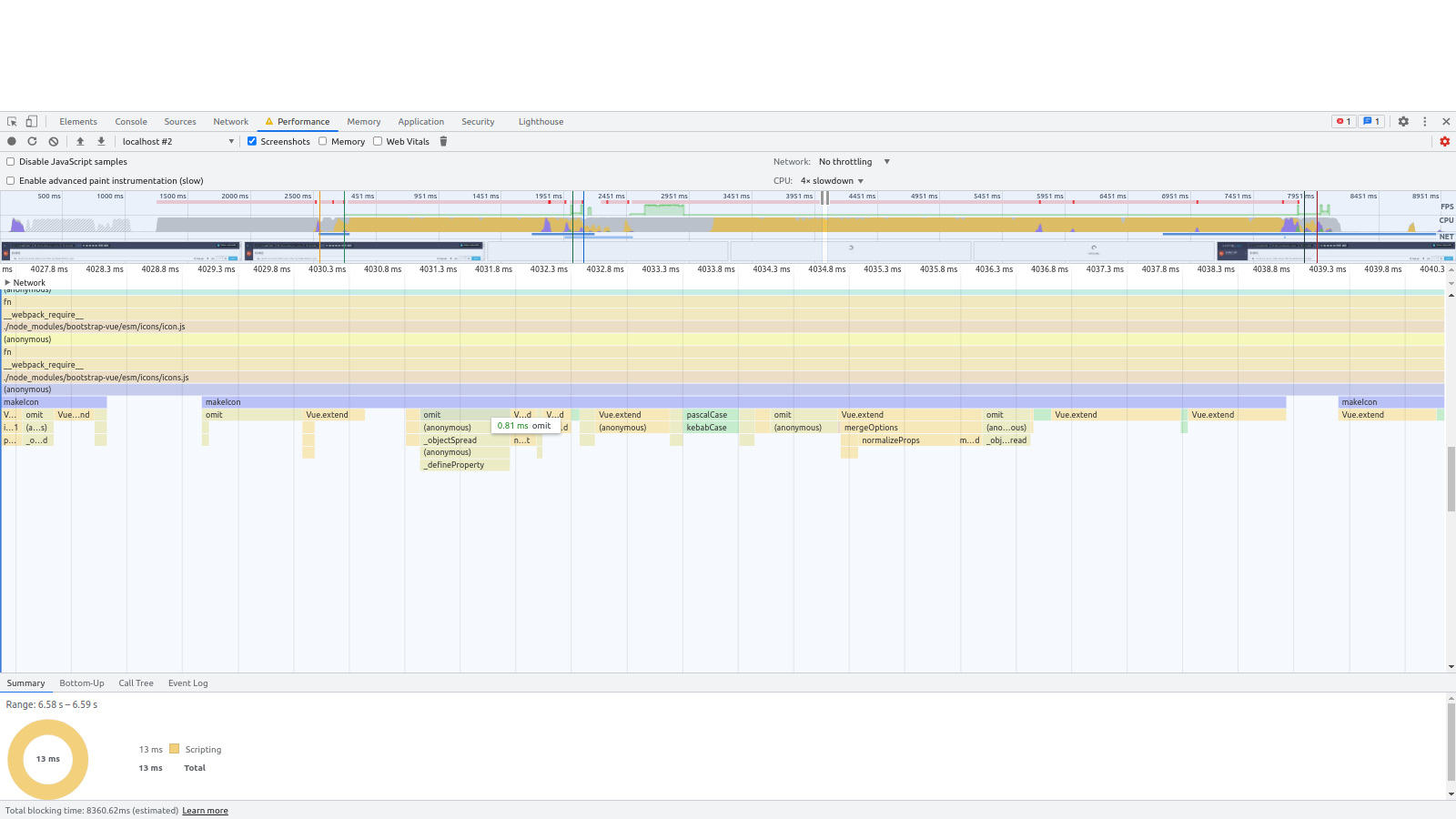
Each call is just a few milliseconds, but there are hundreds of icons,
so it takes hundreds of milliseconds.
## What is the fix?
Don't call `omit` in each `makeIcon` call, but only once.
This speeds things up roughly by half. The other half would be to
somehow avoid repetitive cal of `Vue.extend`, but I don't think that's
possible. At least not as easily as with `omit`. | import { Vue, mergeData } from '../../vue'
import { omit } from '../../utils/object'
import { kebabCase, pascalCase, trim } from '../../utils/string'
import { BVIconBase, props as BVIconBaseProps } from './icon-base'
const iconProps = omit(BVIconBaseProps, ['content'])
/**
* Icon component generator function
*
* @param {string} icon name (minus the leading `BIcon`)
* @param {string} raw `innerHTML` for SVG
* @return {VueComponent}
*/
export const makeIcon = (name, content) => {
// For performance reason we pre-compute some values, so that
// they are not computed on each render of the icon component
const kebabName = kebabCase(name)
const iconName = `BIcon${pascalCase(name)}`
const iconNameClass = `bi-${kebabName}`
const iconTitle = kebabName.replace(/-/g, ' ')
const svgContent = trim(content || '')
return /*#__PURE__*/ Vue.extend({
name: iconName,
functional: true,
props: iconProps,
render(h, { data, props }) {
return h(
BVIconBase,
mergeData(
// Defaults
{
props: { title: iconTitle },
attrs: { 'aria-label': iconTitle }
},
// User data
data,
// Required data
{
staticClass: iconNameClass,
props: { ...props, content: svgContent }
}
)
)
}
})
}
| import { Vue, mergeData } from '../../vue'
import { omit } from '../../utils/object'
import { kebabCase, pascalCase, trim } from '../../utils/string'
import { BVIconBase, props as BVIconBaseProps } from './icon-base'
/**
* Icon component generator function
*
* @param {string} icon name (minus the leading `BIcon`)
* @param {string} raw `innerHTML` for SVG
* @return {VueComponent}
*/
export const makeIcon = (name, content) => {
// For performance reason we pre-compute some values, so that
// they are not computed on each render of the icon component
const kebabName = kebabCase(name)
const iconName = `BIcon${pascalCase(name)}`
const iconNameClass = `bi-${kebabName}`
const iconTitle = kebabName.replace(/-/g, ' ')
const svgContent = trim(content || '')
return /*#__PURE__*/ Vue.extend({
name: iconName,
functional: true,
props: omit(BVIconBaseProps, ['content']),
render(h, { data, props }) {
return h(
BVIconBase,
mergeData(
// Defaults
{
props: { title: iconTitle },
attrs: { 'aria-label': iconTitle }
},
// User data
data,
// Required data
{
staticClass: iconNameClass,
props: { ...props, content: svgContent }
}
)
)
}
})
}
|
Change the default no of users from 9 | # created by Chirath R, [email protected]
from django.conf.urls import url
from django.contrib.auth.decorators import login_required
from django.views.generic import TemplateView
from registration.views import UserSignUpView, login, UserUpdateView, ProfileDetailView, ProfileListView
urlpatterns = [
url(r'^login/$', login, name="login"),
url(r'^signup/$', UserSignUpView.as_view(), name="signup"),
url(r'^$', ProfileListView.as_view(), name="profile_list"),
url(r'^(?P<pk>[0-9]+)/$', ProfileDetailView.as_view(), name="profile"),
url(r'^(?P<pk>[0-9]+)/update/$', login_required(UserUpdateView.as_view()), name="update_profile"),
url(
r'^signup/success/$',
TemplateView.as_view(template_name='registration/signup_success.html'),
name="signup_success"
),
url(
r'^signup/already-logged-in/$',
TemplateView.as_view(template_name='registration/already_logged_in.html'),
name="already_logged_in"
),
url(
r'^permission-denied/$',
TemplateView.as_view(template_name='registration/permission_denied.html'),
name="permission_denied"
),
url(
r'^error/$',
TemplateView.as_view(template_name='registration/error.html'),
name="error"
),
]
| # created by Chirath R, [email protected]
from django.conf.urls import url
from django.contrib.auth.decorators import login_required
from django.views.generic import TemplateView
from registration.views import UserSignUpView, login, UserUpdateView, ProfileDetailView, ProfileListView
urlpatterns = [
url(r'^login/$', login, name="login"),
url(r'^signup/$', UserSignUpView.as_view(), name="signup"),
url(r'^$', ProfileListView.as_view(), name="profile_list"),
url(r'^(?P<pk>[0-9])/$', ProfileDetailView.as_view(), name="profile"),
url(r'^(?P<pk>[0-9])/update/$', login_required(UserUpdateView.as_view()), name="update_profile"),
url(
r'^signup/success/$',
TemplateView.as_view(template_name='registration/signup_success.html'),
name="signup_success"
),
url(
r'^signup/already-logged-in/$',
TemplateView.as_view(template_name='registration/already_logged_in.html'),
name="already_logged_in"
),
url(
r'^permission-denied/$',
TemplateView.as_view(template_name='registration/permission_denied.html'),
name="permission_denied"
),
url(
r'^error/$',
TemplateView.as_view(template_name='registration/error.html'),
name="error"
),
]
|
:bug: Fix the stupid route's bug | // Routes
import Layout from '@/components/Layout'
import Welcome from '@/components/Welcome'
import Login from '@/components/auth/Login'
import UpdateProfile from '@/components/auth/UpdateProfile'
import App from '@/components/App'
import NotFound from '@/components/errors/404'
import Firestore from '@/components/Firestore'
import Vue from 'vue'
const routes = [{
path: '/',
component: Layout,
children: [{
path: '/',
component: Welcome,
name: 'Welcome'
},
{
path: '/login',
component: Login,
name: 'login'
},
{
path: '/update-profile',
component: UpdateProfile,
name: 'Profile Update',
meta: {
auth: true
}
},
{
path: '/app',
component: App,
name: 'Realtime Database',
meta: {
auth: true
}
},
{
path: '/firestore',
component: Firestore,
name: 'Cloud Firestore',
meta: {
auth: true
}
},
{
path: '*',
component: NotFound
}
]
}]
export default routes;
| // Routes
import Layout from '@/components/Layout'
import Welcome from '@/components/Welcome'
import Login from '@/components/auth/Login'
import UpdateProfile from '@/components/auth/UpdateProfile'
import App from '@/components/App'
import NotFound from '@/components/errors/404'
import Firestore from '@/components/Firestore'
import Vue from 'vue'
const routes = [{
path: '/',
component: Layout,
children: [{
path: '/',
component: Welcome,
name: 'Welcome'
},
{
path: '/login',
component: Login,
name: 'login'
},
{
path: '/update-profile',
component: UpdateProfile,
name: 'Profile Update',
meta: {
auth: true
}
},
{
path: '/app',
component: App,
name: 'Realtime Database',
meta: {
auth: true
}
},
{
path: '/firestore',
component: Firestore,
name: 'Cloud Firestore',
meta: {
auth: true
}
},
{
path: '*',
components: NotFound
}
]
}]
export default routes; |
Fix for MPLy-10287
Can now click on markers to open POIs.
Buddy: Jean |
// MarkerController is reponsible for event handling such as moving to and opening the POI when selected.
class MarkerController {
constructor(searchbar, markerController, mapController) {
this._onPoiCardClickedCallback = null;
this._eegeoPoiSourceIdMap = {};
this._markerController = markerController;
this._mapController = mapController;
searchbar.on("searchresultselect", (event) => { this.goToResult(event); });
searchbar.on("searchresultsupdate", (event)=> { this.updateMarkers(event) });
}
goToResult(event) {
this._markerController.selectMarker(event.result.sourceId);
this._mapController.getMap().setView(event.result.location.latLng, 15);
this._openPoiView(event.result.resultId, event.result);
}
updateMarkers(event) {
let markerIDs = this._markerController.getAllMarkerIds();
for (var i = 0; i < markerIDs.length; ++i)
{
const marker = this._markerController.getMarker(markerIDs[i]);
marker.on("click", () => {
var id = marker.id;
if(event.results[id])
{
this._openPoiView(id, event.results[id]);
}
});
}
}
_openPoiView(markerId, poi) {
// Here we create our POI view from the poi data. You may want to add your own POI view here instead.
const element = PoiViewContainer(poi);
let location = poi.location;
const marker = this._markerController.getMarker(markerId);
if (marker) {
marker.closePopup();
}
this._mapController.addPopupWithOffsetForPois(location, element);
const options = {
durationSeconds: 0.0,
allowInterruption: true
}
}
}
|
// MarkerController is reponsible for event handling such as moving to and opening the POI when selected.
class MarkerController {
constructor(searchbar, markerController, mapController) {
this._onPoiCardClickedCallback = null;
this._eegeoPoiSourceIdMap = {};
this._markerController = markerController;
this._mapController = mapController;
searchbar.on("searchresultselect", (event) => { this.goToResult(event); });
}
goToResult(event) {
this._markerController.selectMarker(event.result.sourceId);
this._mapController.getMap().setView(event.result.location.latLng, 15);
this._openPoiView(event.result.resultId, event.result);
}
_openPoiView(markerId, poi) {
// Here we create our POI view from the poi data. You may want to add your own POI view here instead.
const element = PoiViewContainer(poi);
let location = poi.location;
const marker = this._markerController.getMarker(markerId);
if (marker) {
marker.closePopup();
}
this._mapController.addPopupWithOffsetForPois(location, element);
const options = {
durationSeconds: 0.0,
allowInterruption: true
}
}
}
|
Set atwho.js minLen param to 1 default was 0. | jQuery(function( $ ) {
var mOptions = $.extend({}, mOpt);
console.log(mOptions);
$('#cmessage').atwho({
at: "@",
displayTpl: "<li>${username}<small> ${name}</small></li>",
insertTpl: "${atwho-at}${username}",
callbacks: {
remoteFilter: function(query, callback) {
console.log('Query: ' + query);
//
/*
if(query === null || query.length < 1){
return callback(null);
}*/
$.ajax({
url: mOptions.path + "index.php?mq=" + query,
type: 'GET',
dataType: 'json',
success: function(data) {
callback(data);
console.log('Data: ' + data);
},
error: function() {
console.warn('Didn\'t Work');
},
beforeSend: function(xhr) {
//xhr.setRequestHeader('Authorization', localStorageService.get('authToken'));
}
});
}
},
searchKey: "username",
limit: 5,
maxLen: 15,
minLen: 1,
displayTimeout: 300,
highlightFirst: true,
});
});
| jQuery(function( $ ) {
var mOptions = $.extend({}, mOpt);
console.log(mOptions);
$('#cmessage').atwho({
at: "@",
displayTpl: "<li>${username}<small> ${name}</small></li>",
insertTpl: "${atwho-at}${username}",
callbacks: {
remoteFilter: function(query, callback) {
console.log('Query: ' + query);
//
if(query === null || query.length < 1){
return callback(null);
}
$.ajax({
url: mOptions.path + "index.php?mq=" + query,
type: 'GET',
dataType: 'json',
success: function(data) {
callback(data);
console.log('Data: ' + data);
},
error: function() {
console.warn('Didn\'t Work');
},
beforeSend: function(xhr) {
//xhr.setRequestHeader('Authorization', localStorageService.get('authToken'));
}
});
}
},
searchKey: "username",
limit: 5,
maxLen: 15,
displayTimeout: 300,
highlightFirst: true,
delay: 50,
});
});
|
:bug: Fix mixin before declaration issues
Add check for declaration node content type
Fixes #227
Fixes #230 | 'use strict';
var helpers = require('../helpers');
module.exports = {
'name': 'mixins-before-declarations',
'defaults': {
'exclude': [
'breakpoint',
'mq'
]
},
'detect': function (ast, parser) {
var result = [],
error;
ast.traverseByType('include', function (node, i, parent) {
var depth = 0,
declarationCount = [depth];
parent.forEach( function (item) {
if (item.type === 'ruleset') {
depth++;
declarationCount[depth] = 0;
}
else if (item.type === 'declaration') {
if (item.first().is('property')) {
var prop = item.first();
if(prop.first().is('ident')) {
declarationCount[depth]++;
}
}
}
else if (item.type === 'include') {
item.forEach('simpleSelector', function (name) {
if (parser.options.exclude.indexOf(name.content[0].content) === -1 && declarationCount[depth] > 0) {
error = {
'ruleId': parser.rule.name,
'line': item.start.line,
'column': item.start.column,
'message': 'Mixins should come before declarations',
'severity': parser.severity
};
result = helpers.addUnique(result, error);
}
});
}
});
});
return result;
}
};
| 'use strict';
var helpers = require('../helpers');
module.exports = {
'name': 'mixins-before-declarations',
'defaults': {
'exclude': [
'breakpoint',
'mq'
]
},
'detect': function (ast, parser) {
var result = [],
error;
ast.traverseByType('include', function (node, i, parent) {
var depth = 0,
declarationCount = [depth];
parent.traverse( function (item) {
if (item.type === 'ruleset') {
depth++;
declarationCount[depth] = 0;
}
else if (item.type === 'declaration') {
declarationCount[depth]++;
}
else if (item.type === 'include') {
item.forEach('simpleSelector', function (name) {
if (parser.options.exclude.indexOf(name.content[0].content) === -1 && declarationCount[depth] > 0) {
error = {
'ruleId': parser.rule.name,
'line': item.start.line,
'column': item.start.column,
'message': 'Mixins should come before declarations',
'severity': parser.severity
};
result = helpers.addUnique(result, error);
}
});
}
});
});
return result;
}
};
|
Use Form::checkbox() helper instead of raw HTML in login form. | @extends('layouts.application.guest')
@section('content')
<form action="{{ route('dashboard.user.login') }}" id="loginForm" method="post">
{{ csrf_field() }}
<div>
<img src="{{ asset('images/login-dummy-avatar.png') }}" class="dummy-avatar"/>
</div>
@php($isLoginSet = !empty(old('login')))
{{-- Login --}}
{!!
Form::textInput()
->setIdAndName('login')
->setPlaceholder(__('Dashboard::views/user/login.login.placeholder'))
->setLeftAddonIcon('fa fa-user')
->setAutoValue(true)
->setAutofocus(!$isLoginSet)
!!}
{{-- Password --}}
{!!
Form::passwordInput()
->setIdAndName('password')
->setPlaceholder(__('Dashboard::views/user/login.password.placeholder'))
->setLeftAddonIcon('fa fa-key')
->setAutofocus($isLoginSet)
!!}
{{-- Remember me --}}
{!!
Form::checkbox()
->setIdAndName('remember-me')
->setLabel(__('Dashboard::views/user/login.remember-me.label'))
->setValue(1)
!!}
<div>
<button type="submit" class="btn btn-info">
{{ __('Dashboard::views/user/login.submit') }}
</button>
</div>
</form>
@endsection | @extends('layouts.application.guest')
@section('content')
<form action="{{ route('dashboard.user.login') }}" id="loginForm" method="post">
{{ csrf_field() }}
<div>
<img src="{{ asset('images/login-dummy-avatar.png') }}" class="dummy-avatar"/>
</div>
@php($isLoginSet = !empty(old('login')))
{{-- Login --}}
{!!
Form::textInput()
->setIdAndName('login')
->setPlaceholder(__('Dashboard::views/user/login.login.placeholder'))
->setLeftAddonIcon('fa fa-user')
->setAutoValue(true)
->setAutofocus(!$isLoginSet)
!!}
{{-- Password --}}
{!!
Form::passwordInput()
->setIdAndName('password')
->setPlaceholder(__('Dashboard::views/user/login.password.placeholder'))
->setLeftAddonIcon('fa fa-key')
->setAutofocus($isLoginSet)
!!}
<div class="checkbox">
<label for="remember-me">
<input
type="checkbox"
id="remember-me"
name="remember-me"
value="1"
placeholder=""/>
{{ __('Dashboard::views/user/login.remember-me.label') }}
</label>
</div>
<div>
<button type="submit" class="btn btn-info">
{{ __('Dashboard::views/user/login.submit') }}
</button>
</div>
</form>
@endsection |
Fix incorrect attributes in ARGparams class | #!/usr/bin/env python
# -*- coding: utf-8 -*-
"""ARG parameters class
"""
from __future__ import print_function, division
class ARGparams(object):
"""Class for ARG model parameters.
Attributes
----------
scale : float
rho : float
delta : float
beta : float
theta : list
Raises
------
AssertionError
"""
def __init__(self, scale=.001, rho=.9, delta=1.1, theta=None):
"""Initialize the class instance.
"""
if theta:
assert len(theta) == 3, "Wrong number of parameters in theta!"
[scale, rho, delta] = theta
self.scale = scale
self.rho = rho
self.delta = delta
assert scale > 0, "Scale must be greater than zero!"
self.beta = self.rho / self.scale
self.theta = [scale, rho, delta]
def __repr__(self):
"""This is what is shown when you interactively explore the instance.
"""
params = (self.scale, self.rho, self.delta)
string = "scale = %.2f, rho = %.2f, delta = %.2f" % params
return string
def __str__(self):
"""This is what is shown when you print() the instance.
"""
return self.__repr__()
| #!/usr/bin/env python
# -*- coding: utf-8 -*-
"""ARG parameters class
"""
from __future__ import print_function, division
class ARGparams(object):
"""Class for ARG model parameters.
Attributes
----------
scale : float
rho : float
delta : float
Methods
-------
convert_to_theta
Convert parameters to the vector
"""
def __init__(self, scale=.001, rho=.9, delta=1.1, theta=None):
"""Initialize the class instance.
"""
if theta:
assert len(theta) == 3, "Wrong number of parameters in theta!"
[scale, rho, delta] = theta
self.scale = scale
self.rho = rho
self.delta = delta
assert scale > 0, "Scale must be greater than zero!"
self.beta = self.rho / self.scale
self.theta = [scale, rho, delta]
def __repr__(self):
"""This is what is shown when you interactively explore the instance.
"""
params = (self.scale, self.rho, self.delta)
string = "scale = %.2f, rho = %.2f, delta = %.2f" % params
return string
def __str__(self):
"""This is what is shown when you print() the instance.
"""
return self.__repr__()
|
Switch state immediately to DEAD state/do not schedule it | package org.zalando.nakadi.service.subscription.state;
import org.zalando.nakadi.service.subscription.StreamingContext;
import javax.annotation.Nullable;
import java.io.IOException;
public class CleanupState extends State {
private final Exception exception;
public CleanupState(@Nullable final Exception e) {
this.exception = e;
}
CleanupState() {
this(null);
}
@Override
public void onEnter() {
try {
getContext().unregisterAuthorizationUpdates();
} catch (final RuntimeException ex) {
getLog().error("Unexpected fail during removing callback for registration updates", ex);
}
try {
if (null != exception) {
getOut().onException(exception);
}
} finally {
try {
getContext().unregisterSession();
} finally {
if (null != exception) {
getContext().switchStateImmediately(StreamingContext.DEAD_STATE);
} else{
switchState(StreamingContext.DEAD_STATE);
}
}
try {
getContext().getZkClient().close();
} catch (final IOException e) {
getLog().error("Unexpected fail to release zk connection", e);
}
getContext().getCurrentSpan().finish();
}
}
}
| package org.zalando.nakadi.service.subscription.state;
import org.zalando.nakadi.service.subscription.StreamingContext;
import javax.annotation.Nullable;
import java.io.IOException;
public class CleanupState extends State {
private final Exception exception;
public CleanupState(@Nullable final Exception e) {
this.exception = e;
}
CleanupState() {
this(null);
}
@Override
public void onEnter() {
try {
getContext().unregisterAuthorizationUpdates();
} catch (final RuntimeException ex) {
getLog().error("Unexpected fail during removing callback for registration updates", ex);
}
try {
if (null != exception) {
getOut().onException(exception);
}
} finally {
try {
getContext().unregisterSession();
} finally {
switchState(StreamingContext.DEAD_STATE);
}
try {
getContext().getZkClient().close();
} catch (final IOException e) {
getLog().error("Unexpected fail to release zk connection", e);
}
getContext().getCurrentSpan().finish();
}
}
}
|
Add a backward-compatibility shim to lessen the burden upgrading from 0.4 to 0.5 |
class TypeRegistered(type):
"""
As classes of this metaclass are created, they keep a registry in the
base class of all handler referenced by the keys in cls._handles.
"""
def __init__(cls, name, bases, namespace):
super(TypeRegistered, cls).__init__(name, bases, namespace)
if not hasattr(cls, '_registry'):
cls._registry = {}
types_handled = getattr(cls, '_handles', [])
cls._registry.update((type_, cls) for type_ in types_handled)
class BaseHandler(object):
"""
Abstract base class for handlers.
"""
__metaclass__ = TypeRegistered
def __init__(self, base):
"""
Initialize a new handler to handle `type`.
:Parameters:
- `base`: reference to pickler/unpickler
"""
self._base = base
def flatten(self, obj, data):
"""
Flatten `obj` into a json-friendly form.
:Parameters:
- `obj`: object of `type`
"""
raise NotImplementedError("Abstract method.")
def restore(self, obj):
"""
Restores the `obj` to `type`
:Parameters:
- `object`: json-friendly object
"""
raise NotImplementedError("Abstract method.")
# for backward compatibility, provide 'registry'
# jsonpickle 0.4 clients will call it with something like:
# registry.register(handled_type, handler_class)
class registry:
@staticmethod
def register(handled_type, handler_class):
pass
|
class TypeRegistered(type):
"""
As classes of this metaclass are created, they keep a registry in the
base class of all handler referenced by the keys in cls._handles.
"""
def __init__(cls, name, bases, namespace):
super(TypeRegistered, cls).__init__(name, bases, namespace)
if not hasattr(cls, '_registry'):
cls._registry = {}
types_handled = getattr(cls, '_handles', [])
cls._registry.update((type_, cls) for type_ in types_handled)
class BaseHandler(object):
"""
Abstract base class for handlers.
"""
__metaclass__ = TypeRegistered
def __init__(self, base):
"""
Initialize a new handler to handle `type`.
:Parameters:
- `base`: reference to pickler/unpickler
"""
self._base = base
def flatten(self, obj, data):
"""
Flatten `obj` into a json-friendly form.
:Parameters:
- `obj`: object of `type`
"""
raise NotImplementedError("Abstract method.")
def restore(self, obj):
"""
Restores the `obj` to `type`
:Parameters:
- `object`: json-friendly object
"""
raise NotImplementedError("Abstract method.")
|
[DDW-212] Fix missing application menu on Windows build | export default (app, window, openAbout) => (
[{
label: 'Daedalus',
submenu: [{
label: 'About',
click() {
openAbout();
}
}, {
label: 'Close',
accelerator: 'Ctrl+W',
click() {
app.quit();
}
}]
}, {
label: 'Edit',
submenu: [{
label: 'Undo',
accelerator: 'Ctrl+Z',
role: 'undo'
}, {
label: 'Redo',
accelerator: 'Shift+Ctrl+Z',
role: 'redo'
}, {
type: 'separator'
}, {
label: 'Cut',
accelerator: 'Ctrl+X',
role: 'cut'
}, {
label: 'Copy',
accelerator: 'Ctrl+C',
role: 'copy'
}, {
label: 'Paste',
accelerator: 'Ctrl+V',
role: 'paste'
}, {
label: 'Select All',
accelerator: 'Ctrl+A',
role: 'selectall'
}]
}, {
label: 'View',
submenu: [
{
label: 'Reload',
accelerator: 'Ctrl+R',
click() { window.webContents.reload(); }
},
{
label: 'Toggle Full Screen',
accelerator: 'F11',
click() { window.setFullScreen(!window.isFullScreen()); }
},
{
label: 'Toggle Developer Tools',
accelerator: 'Alt+Ctrl+I',
click() { window.toggleDevTools(); }
}
]
}]
);
| export const winLinuxMenu = (app, window, openAbout) => (
[{
label: 'Daedalus',
submenu: [{
label: 'About',
click() {
openAbout();
}
}, {
label: 'Close',
accelerator: 'Ctrl+W',
click() {
app.quit();
}
}]
}, {
label: 'Edit',
submenu: [{
label: 'Undo',
accelerator: 'Ctrl+Z',
role: 'undo'
}, {
label: 'Redo',
accelerator: 'Shift+Ctrl+Z',
role: 'redo'
}, {
type: 'separator'
}, {
label: 'Cut',
accelerator: 'Ctrl+X',
role: 'cut'
}, {
label: 'Copy',
accelerator: 'Ctrl+C',
role: 'copy'
}, {
label: 'Paste',
accelerator: 'Ctrl+V',
role: 'paste'
}, {
label: 'Select All',
accelerator: 'Ctrl+A',
role: 'selectall'
}]
}, {
label: 'View',
submenu: [
{
label: 'Reload',
accelerator: 'Ctrl+R',
click() { window.webContents.reload(); }
},
{
label: 'Toggle Full Screen',
accelerator: 'F11',
click() { window.setFullScreen(!window.isFullScreen()); }
},
{
label: 'Toggle Developer Tools',
accelerator: 'Alt+Ctrl+I',
click() { window.toggleDevTools(); }
}
]
}]
);
|
Add missing import to FTP module | """
Implementation of FTP-related features.
"""
import Pyro4
from .core import BaseAgent
from .common import address_to_host_port
class FTPAgent(BaseAgent):
"""
An agent that provides basic FTP functionality.
"""
def ftp_configure(self, addr, user, passwd, path, perm='elr'):
from pyftpdlib.authorizers import DummyAuthorizer
from pyftpdlib.handlers import FTPHandler
from pyftpdlib.servers import FTPServer
# Create authorizer
authorizer = DummyAuthorizer()
authorizer.add_user(user, passwd, path, perm=perm)
# Create handler
handler = FTPHandler
handler.authorizer = authorizer
# Create server
host, port = address_to_host_port(addr)
# TODO: is this necessary? Or would `None` be sufficient?
if port is None:
port = 0
self.ftp_server = FTPServer((host, port), handler)
return self.ftp_server.socket.getsockname()
@Pyro4.oneway
def ftp_run(self):
# Serve forever
self.ftp_server.serve_forever()
def ftp_addr(self):
return self.ftp_server.socket.getsockname()
def ftp_retrieve(self, addr, origin, destiny, user, passwd):
import ftplib
host, port = addr
ftp = ftplib.FTP()
ftp.connect(host, port)
ftp.login(user, passwd)
ftp.retrbinary('RETR %s' % origin, open(destiny, 'wb').write)
ftp.close()
return destiny
| """
Implementation of FTP-related features.
"""
from .core import BaseAgent
from .common import address_to_host_port
class FTPAgent(BaseAgent):
"""
An agent that provides basic FTP functionality.
"""
def ftp_configure(self, addr, user, passwd, path, perm='elr'):
from pyftpdlib.authorizers import DummyAuthorizer
from pyftpdlib.handlers import FTPHandler
from pyftpdlib.servers import FTPServer
# Create authorizer
authorizer = DummyAuthorizer()
authorizer.add_user(user, passwd, path, perm=perm)
# Create handler
handler = FTPHandler
handler.authorizer = authorizer
# Create server
host, port = address_to_host_port(addr)
# TODO: is this necessary? Or would `None` be sufficient?
if port is None:
port = 0
self.ftp_server = FTPServer((host, port), handler)
return self.ftp_server.socket.getsockname()
@Pyro4.oneway
def ftp_run(self):
# Serve forever
self.ftp_server.serve_forever()
def ftp_addr(self):
return self.ftp_server.socket.getsockname()
def ftp_retrieve(self, addr, origin, destiny, user, passwd):
import ftplib
host, port = addr
ftp = ftplib.FTP()
ftp.connect(host, port)
ftp.login(user, passwd)
ftp.retrbinary('RETR %s' % origin, open(destiny, 'wb').write)
ftp.close()
return destiny
|
Fix styles for centering button in app | import React from 'react';
const styles = {
container: {
backgroundColor: '#EEE',
display: 'flex',
alignItems: 'center',
flexDirection: 'column',
flexWrap: 'wrap',
height: '100%',
width: '100%'
},
header: {
fontSize: '65px',
color: '#333',
textAlign: 'center',
width: '100%',
fontWeight: 100
},
counter: {
textAlign: 'center',
fontSize: '120px',
width: '200px',
margin: '0 auto'
},
button: {
margin: '10px'
},
buttonWrapper: {
textAlign: 'center'
}
};
function Button(props){
return (
<button type='button'
style={styles.button}
className={props.className}
onClick={props.handleClick}>
{props.children}
</button>
);
}
export default function Page(props){
return (
<div style={styles.container}>
<div className='col-sm-12'>
<h1 style={styles.header}>Counter:</h1>
<div style={styles.counter}>{props.counter}</div>
<div className='col-sm-offset-3 col-sm-3' style={styles.buttonWrapper}>
<Button className='btn btn-lg btn-success' handleClick={props.onIncrement}>Increment</Button>
</div>
<div className='col-sm-3' style={styles.buttonWrapper}>
<Button className='btn btn-lg btn-danger' handleClick={props.onDecrement}>Decrement</Button>
</div>
</div>
</div>
);
}
| import React from 'react';
const styles = {
container: {
backgroundColor: '#EEE',
display: 'flex',
alignItems: 'center',
flexDirection: 'column',
flexWrap: 'wrap',
height: '100%',
width: '100%'
},
header: {
fontSize: '65px',
color: '#333',
textAlign: 'center',
fontWeight: 100
},
counter: {
fontSize: '120px'
},
button: {
margin: 10
}
};
function Button(props){
return (
<button type='button'
style={styles.button}
className={props.className}
onClick={props.handleClick}>
{props.children}
</button>
);
}
export default function Page(props){
return (
<div style={styles.container} className='text-center'>
<h1 style={styles.header}>Counter:</h1>
<div style={styles.counter}>{props.counter}</div>
<div className='col-sm-12'>
<div className='col-sm-offset-4 col-sm-2'>
<Button className='btn btn-lg btn-success' handleClick={props.onIncrement}>Increment</Button>
</div>
<div className='col-sm-2'>
<Button className='btn btn-lg btn-danger' handleClick={props.onDecrement}>Decrement</Button>
</div>
</div>
</div>
);
}
|
Add parameter restriction from tempKey | import { applyMiddleware, compose, createStore } from 'redux'
import thunk from 'redux-thunk'
import { browserHistory } from 'react-router'
import makeRootReducer from './reducers'
import { updateLocation } from './location'
import { fetchApiKey } from './auth'
export default (initialState = {}) => {
// ======================================================
// Middleware Configuration
// ======================================================
const middleware = [thunk]
// ======================================================
// Store Enhancers
// ======================================================
const enhancers = []
let composeEnhancers = compose
if (__DEV__) {
const composeWithDevToolsExtension = window.__REDUX_DEVTOOLS_EXTENSION_COMPOSE__
if (typeof composeWithDevToolsExtension === 'function') {
composeEnhancers = composeWithDevToolsExtension
}
}
// ======================================================
// Store Instantiation
// ======================================================
const store = createStore(
makeRootReducer(),
initialState,
composeEnhancers(
applyMiddleware(...middleware),
...enhancers
)
)
store.asyncReducers = {}
// To unsubscribe, invoke `store.unsubscribeHistory()` anytime
store.unsubscribeHistory = browserHistory.listen(updateLocation(store))
// ======================================================
// Authentication Instantiation
// ======================================================
const tempKey = window.location.search.replace(/^\?/, '').split('=')
const param = tempKey[0] === 'einmalCode' ? tempKey[1] : null
store.dispatch(fetchApiKey(param))
// ======================================================
// HMR Setup
// ======================================================
if (module.hot) {
module.hot.accept('./reducers', () => {
const reducers = require('./reducers').default
store.replaceReducer(reducers(store.asyncReducers))
})
}
return store
}
| import { applyMiddleware, compose, createStore } from 'redux'
import thunk from 'redux-thunk'
import { browserHistory } from 'react-router'
import makeRootReducer from './reducers'
import { updateLocation } from './location'
import { fetchApiKey } from './auth'
export default (initialState = {}) => {
// ======================================================
// Middleware Configuration
// ======================================================
const middleware = [thunk]
// ======================================================
// Store Enhancers
// ======================================================
const enhancers = []
let composeEnhancers = compose
if (__DEV__) {
const composeWithDevToolsExtension = window.__REDUX_DEVTOOLS_EXTENSION_COMPOSE__
if (typeof composeWithDevToolsExtension === 'function') {
composeEnhancers = composeWithDevToolsExtension
}
}
// ======================================================
// Store Instantiation
// ======================================================
const store = createStore(
makeRootReducer(),
initialState,
composeEnhancers(
applyMiddleware(...middleware),
...enhancers
)
)
store.asyncReducers = {}
// To unsubscribe, invoke `store.unsubscribeHistory()` anytime
store.unsubscribeHistory = browserHistory.listen(updateLocation(store))
// ======================================================
// Authentication Instantiation
// ======================================================
const tempKey = window.location.search.replace(/^\?/, '').split('=')[1]
store.dispatch(fetchApiKey(tempKey))
// ======================================================
// HMR Setup
// ======================================================
if (module.hot) {
module.hot.accept('./reducers', () => {
const reducers = require('./reducers').default
store.replaceReducer(reducers(store.asyncReducers))
})
}
return store
}
|
Update product listing test to use product ids rather than index | from django.test import TestCase
from django.core.urlresolvers import reverse
from whats_fresh_api.models import *
from django.contrib.gis.db import models
import json
class ListProductTestCase(TestCase):
fixtures = ['test_fixtures']
def test_url_endpoint(self):
url = reverse('entry-list-products')
self.assertEqual(url, '/entry/products')
def test_list_items(self):
"""
Tests to see if the list of products contains the proper products and
proper product data
"""
response = self.client.get(reverse('entry-list-products'))
items = response.context['item_list']
product_dict = {}
for product in items:
product_id = product['link'].split('/')[-1]
product_dict[str(product_id)] = product
for product in Product.objects.all():
self.assertEqual(
product_dict[str(product.id)]['description'],
product.description)
self.assertEqual(
product_dict[str(product.id)]['name'], product.name)
self.assertEqual(
product_dict[str(product.id)]['link'],
reverse('edit-product', kwargs={'id': product.id}))
self.assertEqual(
product_dict[str(product.id)]['modified'],
product.modified.strftime("%I:%M %P, %d %b %Y"))
self.assertEqual(
sort(product_dict[str(product.id)]['preparations']),
sort([prep.name for prep in product.preparations.all()]))
| from django.test import TestCase
from django.core.urlresolvers import reverse
from whats_fresh_api.models import *
from django.contrib.gis.db import models
import json
class ListProductTestCase(TestCase):
fixtures = ['test_fixtures']
def test_url_endpoint(self):
url = reverse('entry-list-products')
self.assertEqual(url, '/entry/products')
def test_list_items(self):
"""
Tests to see if the list of products contains the proper productss and
proper product data
"""
response = self.client.get(reverse('entry-list-products'))
items = response.context['item_list']
for product in Product.objects.all():
self.assertEqual(
items[product.id-1]['description'], product.description)
self.assertEqual(
items[product.id-1]['name'], product.name)
self.assertEqual(
items[product.id-1]['link'],
reverse('edit-product', kwargs={'id': product.id}))
self.assertEqual(
items[product.id-1]['modified'],
product.modified.strftime("%I:%M %P, %d %b %Y"))
self.assertEqual(
sort(items[product.id-1]['preparations']),
sort([prep.name for prep in product.preparations.all()]))
|
Add filter for tags autocomplete for creating a new bookmark | (function(){
'use strict'
const New = {
templateUrl: 'pages/new/new.html',
controllerAs: 'model',
controller: function(BookmarkFactory, TagFactory, $state, $q){
const model = this;
model.saveBookmark = saveBookmark;
model.loadTags = loadTags;
model.validationClass = validationClass;
model.resource_types = ["video", "conversation thread", "book/publication", "blog/post", "other"];
// NOTE: add & remove class based on form validation
function validationClass(value){
if (value.$dirty){
return value.$invalid ? 'remove icon red' : 'checkmark icon green';
}
}
// TODO: look into add cache for search
function loadTags($query) {
return TagFactory.getTags()
.then(function(response) {
return response.filter(function(tag) {
return tag.name.toLowerCase().indexOf($query.toLowerCase()) != -1;
});
});
};
// NOTE: save new bookmarks
function saveBookmark(){
return BookmarkFactory.saveBookmark(model.newBookmark)
.then(saveBookmarkResponse)
}//end saveBookmark
function saveBookmarkResponse(response){
if(response.status == "success"){
return $state.go("show", { id: response.success.id});
} else {
return model.error = response.errors;
}
}//end saveBookmarkResponse
}//end controller
}//end New
angular
.module("shareMark")
.component("new", New);
}());
| (function(){
'use strict'
const New = {
templateUrl: 'pages/new/new.html',
controllerAs: 'model',
controller: function(BookmarkFactory, TagFactory, $state){
const model = this;
model.saveBookmark = saveBookmark;
model.loadTags = loadTags;
model.validationClass = validationClass;
model.resource_types = ["video", "conversation thread", "book/publication", "blog/post", "other"];
// NOTE: add & remove class based on form validation
function validationClass(value){
if (value.$dirty){
return value.$invalid ? 'remove icon red' : 'checkmark icon green';
}
}
// NOTE: ngTagsInput module
function loadTags(query) {
model.testing = "clicked";
return TagFactory.getTags()
.then(function(response){
return response;
});
}//end loadTags
// NOTE: save new bookmarks
function saveBookmark(){
return BookmarkFactory.saveBookmark(model.newBookmark)
.then(saveBookmarkResponse)
}//end saveBookmark
function saveBookmarkResponse(response){
if(response.status == "success"){
return $state.go("show", { id: response.success.id});
} else {
return model.error = response.errors;
}
}//end saveBookmarkResponse
}//end controller
}//end New
angular
.module("shareMark")
.component("new", New);
}());
|
Add phpstorm meta for packages
Former-commit-id: 2d54f8adf92c1a82f7c3d5a02fd0fd18af88ca26 | <?php
namespace Concrete\Core\Support\Symbol;
use Core;
class MetadataGenerator
{
public function render()
{
$file = '<?php namespace PHPSTORM_META { $STATIC_METHOD_TYPES = array(\\Core::make(\'\') => array(' . PHP_EOL;
$bindings = Core::getBindings();
foreach ($bindings as $name => $binding) {
/** @var \Closure $binding */
$reflection = new \ReflectionFunction($binding['concrete']);
$static = $reflection->getStaticVariables();
$className = null;
if (!isset($static['concrete'])) {
try {
$class = Core::make($name);
$className = get_class($class);
} catch (\Exception $e) {}
} else {
$className = $static['concrete'];
}
if ($className !== null && $className !== get_class($this)) {
if ($className[0] !== '\\') {
$className = '\\' . $className;
}
$file .= '\'' . $name . '\' instanceof ' . $className . ',' . PHP_EOL;
}
}
$file .= '), \Package::getByHandle(\'\') => array(';
$packages = \Package::getAvailablePackages(false);
foreach ($packages as $package) {
/** @var \Package $package */
$file .= '\'' . $package->getPackageHandle() . '\' instanceof \\' . get_class($package) . ',' . PHP_EOL;
}
$file .= '));}';
return $file;
}
} | <?php
namespace Concrete\Core\Support\Symbol;
use Core;
class MetadataGenerator
{
public function render()
{
$file = '<?php namespace PHPSTORM_META { $STATIC_METHOD_TYPES = array(\\Core::make(\'\') => array(' . PHP_EOL;
$bindings = Core::getBindings();
foreach ($bindings as $name => $binding) {
/** @var \Closure $binding */
$reflection = new \ReflectionFunction($binding['concrete']);
$static = $reflection->getStaticVariables();
$className = null;
if (!isset($static['concrete'])) {
try {
$class = Core::make($name);
$className = get_class($class);
} catch (\Exception $e) {}
} else {
$className = $static['concrete'];
}
if ($className !== null && $className !== get_class($this)) {
if ($className[0] !== '\\') {
$className = '\\' . $className;
}
$file .= '\'' . $name . '\' instanceof ' . $className . ',' . PHP_EOL;
}
}
$file .= '));}';
return $file;
}
} |
CRM-2628: Optimize Static segments
- fix migration for mySQL | <?php
namespace Oro\Bundle\SegmentBundle\Migrations\Schema\v1_3;
use Psr\Log\LoggerInterface;
use Oro\Bundle\EntityBundle\ORM\DatabaseDriverInterface;
use Oro\Bundle\MigrationBundle\Migration\ArrayLogger;
use Oro\Bundle\MigrationBundle\Migration\ParametrizedMigrationQuery;
class UpdateSegmentSnapshotDataQuery extends ParametrizedMigrationQuery
{
/**
* {@inheritdoc}
*/
public function getDescription()
{
$logger = new ArrayLogger();
$this->updateSnapshotData($logger, true);
return $logger->getMessages();
}
/**
* {@inheritdoc}
*/
public function execute(LoggerInterface $logger)
{
$this->updateSnapshotData($logger);
}
/**
* Collect integer_entity_id field in oro_segment_snapshot table
*
* @param LoggerInterface $logger
* @param bool $dryRun
*/
protected function updateSnapshotData(LoggerInterface $logger, $dryRun = false)
{
$query = <<<SQL
UPDATE oro_segment_snapshot set integer_entity_id = CAST(entity_id as %s) WHERE entity_id %s '^[0-9]+$';
SQL;
$type = 'UNSIGNED';
$function = 'REGEXP';
if ($this->connection->getDriver()->getName() === DatabaseDriverInterface::DRIVER_POSTGRESQL) {
$function = '~';
$type = 'int';
}
$query = sprintf($query, $type, $function);
$this->logQuery($logger, $query);
if (!$dryRun) {
$this->connection->prepare($query)->execute();
}
}
}
| <?php
namespace Oro\Bundle\SegmentBundle\Migrations\Schema\v1_3;
use Psr\Log\LoggerInterface;
use Oro\Bundle\EntityBundle\ORM\DatabaseDriverInterface;
use Oro\Bundle\MigrationBundle\Migration\ArrayLogger;
use Oro\Bundle\MigrationBundle\Migration\ParametrizedMigrationQuery;
class UpdateSegmentSnapshotDataQuery extends ParametrizedMigrationQuery
{
/**
* {@inheritdoc}
*/
public function getDescription()
{
$logger = new ArrayLogger();
$this->updateSnapshotData($logger, true);
return $logger->getMessages();
}
/**
* {@inheritdoc}
*/
public function execute(LoggerInterface $logger)
{
$this->updateSnapshotData($logger);
}
/**
* Collect integer_entity_id field in oro_segment_snapshot table
*
* @param LoggerInterface $logger
* @param bool $dryRun
*/
protected function updateSnapshotData(LoggerInterface $logger, $dryRun = false)
{
$query = <<<SQL
UPDATE oro_segment_snapshot set integer_entity_id = CAST(entity_id as int) WHERE entity_id %s '^[0-9]+$';
SQL;
$function = 'REGEXP';
if ($this->connection->getDriver()->getName() === DatabaseDriverInterface::DRIVER_POSTGRESQL) {
$function = '~';
}
$query = sprintf($query, $function);
$this->logQuery($logger, $query);
if (!$dryRun) {
$this->connection->prepare($query)->execute();
}
}
}
|
Remove post method from CommandInterceptor binding | <?php
declare(strict_types=1);
namespace BEAR\QueryRepository;
use BEAR\RepositoryModule\Annotation\AbstractCacheControl;
use BEAR\RepositoryModule\Annotation\Cacheable;
use BEAR\RepositoryModule\Annotation\Purge;
use BEAR\RepositoryModule\Annotation\Refresh;
use Ray\Di\AbstractModule;
class QueryRepositoryAopModule extends AbstractModule
{
/**
* {@inheritdoc}
*/
protected function configure()
{
$this->bindPriorityInterceptor(
$this->matcher->annotatedWith(Cacheable::class),
$this->matcher->startsWith('onGet'),
[CacheInterceptor::class]
);
$this->bindInterceptor(
$this->matcher->annotatedWith(Cacheable::class),
$this->matcher->logicalOr(
$this->matcher->startsWith('onPut'),
$this->matcher->logicalOr(
$this->matcher->startsWith('onPatch'),
$this->matcher->startsWith('onDelete')
)
),
[CommandInterceptor::class]
);
$this->bindInterceptor(
$this->matcher->logicalNot(
$this->matcher->annotatedWith(Cacheable::class)
),
$this->matcher->logicalOr(
$this->matcher->annotatedWith(Purge::class),
$this->matcher->annotatedWith(Refresh::class)
),
[RefreshInterceptor::class]
);
$this->bindInterceptor(
$this->matcher->annotatedWith(AbstractCacheControl::class),
$this->matcher->startsWith('onGet'),
[HttpCacheInterceptor::class]
);
}
}
| <?php
declare(strict_types=1);
namespace BEAR\QueryRepository;
use BEAR\RepositoryModule\Annotation\AbstractCacheControl;
use BEAR\RepositoryModule\Annotation\Cacheable;
use BEAR\RepositoryModule\Annotation\Purge;
use BEAR\RepositoryModule\Annotation\Refresh;
use Ray\Di\AbstractModule;
class QueryRepositoryAopModule extends AbstractModule
{
/**
* {@inheritdoc}
*/
protected function configure()
{
$this->bindPriorityInterceptor(
$this->matcher->annotatedWith(Cacheable::class),
$this->matcher->startsWith('onGet'),
[CacheInterceptor::class]
);
$this->bindInterceptor(
$this->matcher->annotatedWith(Cacheable::class),
$this->matcher->logicalOr(
$this->matcher->startsWith('onPost'),
$this->matcher->logicalOr(
$this->matcher->startsWith('onPut'),
$this->matcher->logicalOr(
$this->matcher->startsWith('onPatch'),
$this->matcher->startsWith('onDelete')
)
)
),
[CommandInterceptor::class]
);
$this->bindInterceptor(
$this->matcher->logicalNot(
$this->matcher->annotatedWith(Cacheable::class)
),
$this->matcher->logicalOr(
$this->matcher->annotatedWith(Purge::class),
$this->matcher->annotatedWith(Refresh::class)
),
[RefreshInterceptor::class]
);
$this->bindInterceptor(
$this->matcher->annotatedWith(AbstractCacheControl::class),
$this->matcher->startsWith('onGet'),
[HttpCacheInterceptor::class]
);
}
}
|
Fix access denied page styling | import React from 'react';
import AuthStore from '../stores/AuthStore';
import AlertPanel from './AlertPanel';
import Config from '../config/Config';
import MetadataStore from '../stores/MetadataStore';
const METHODS_TO_BIND = [
'handleUserLogout'
];
module.exports = class AccessDeniedPage extends React.Component {
constructor() {
super(...arguments);
METHODS_TO_BIND.forEach((method) => {
this[method] = this[method].bind(this);
});
}
handleUserLogout() {
AuthStore.logout();
}
getFooter() {
return (
<div className="button-collection flush-bottom">
<button className="button button-primary"
onClick={this.handleUserLogout}>
Log out
</button>
</div>
);
}
render() {
return (
<div className="application-wrapper">
<div className="page">
<div className="page-body-content vertical-center">
<AlertPanel title="Access denied">
<p className="tall">
You do not have access to this service. Please contact your {Config.productName} administrator or see <a href={MetadataStore.buildDocsURI('/administration/id-and-access-mgt/')} target="_blank">security documentation</a> for more information.
</p>
{this.getFooter()}
</AlertPanel>
</div>
</div>
</div>
);
}
};
| import React from 'react';
import AuthStore from '../stores/AuthStore';
import AlertPanel from './AlertPanel';
import Config from '../config/Config';
import MetadataStore from '../stores/MetadataStore';
const METHODS_TO_BIND = [
'handleUserLogout'
];
module.exports = class AccessDeniedPage extends React.Component {
constructor() {
super(...arguments);
METHODS_TO_BIND.forEach((method) => {
this[method] = this[method].bind(this);
});
}
handleUserLogout() {
AuthStore.logout();
}
getFooter() {
return (
<div className="button-collection flush-bottom">
<button className="button button-primary"
onClick={this.handleUserLogout}>
Log out
</button>
</div>
);
}
render() {
return (
<div className="page flex flex-direction-top-to-bottom flex-item-grow-1">
<div className="page-body-content flex flex-direction-top-to-bottom
flex-item-grow-1 horizontal-center vertical-center">
<AlertPanel
footer={this.getFooter()}
title="Access denied">
<p className="tall">
You do not have access to this service. Please contact your {Config.productName} administrator or see <a href={MetadataStore.buildDocsURI('/administration/id-and-access-mgt/')} target="_blank">security documentation</a> for more information.
</p>
</AlertPanel>
</div>
</div>
);
}
};
|
Add getCartByUser in shared cart. | "use strict";
angular.module("piadinamia").factory("sharedCartService",
["cartService", "$firebase", "Firebase", "FBURL",
function (cartService, $firebase, Firebase, FBURL) {
var myCustomers = [],
myCatalogName,
fburl;
return {
init: function (customers, catalogName, userId) {
var customersRef;
myCustomers = customers;
myCatalogName = catalogName;
fburl = FBURL + "/users/" + userId +
"/catalogs/" + catalogName + "/customers";
customersRef = new Firebase(fburl);
$firebase(customersRef).$on("change", function () {
$firebase(customersRef).$on("loaded", function (customers) {
myCustomers = [];
angular.forEach(customers, function (item) {
myCustomers.push(item);
});
});
});
},
getCartByItem: function () {},
getCartByUser: function () {
var cartByUser = {};
myCustomers.forEach(function (userId) {
var cartRef,
url = FBURL + "/users/" + userId +
"/catalogs/" + myCatalogName + "/cart",
myCart;
cartRef = new Firebase(url);
$firebase(cartRef).$on("loaded", function (cart) {
myCart = [];
angular.forEach(cart, function (item) {
myCart.push(item);
});
cartByUser[userId] = myCart;
});
});
}
};
}]);
| "use strict";
angular.module("piadinamia").factory("sharedCartService",
["$firebase", "Firebase", "FBURL",
function ($firebase, Firebase, FBURL) {
var myCustomers = [],
fburl;
return {
init: function (customers, catalogName, userId) {
var customersRef;
myCustomers = customers;
fburl = FBURL + "/users/" + userId +
"/catalogs/" + catalogName + "/customers";
customersRef = new Firebase(fburl);
$firebase(customersRef).$on("change", function() {
$firebase(customersRef).$on("loaded", function (customers) {
myCustomers = [];
angular.forEach(customers, function(item) {
myCustomers.push(item);
});
});
});
},
getCartByItem: function () {},
getCartByUser: function () {}
};
}]);
|
Add relationship to user show view | <?php
namespace App\Http\Controllers;
use App\Models\User;
use Illuminate\Database\Eloquent\ModelNotFoundException;
use Illuminate\Http\Request;
use Ramsey\Uuid\Uuid;
class UserController extends Controller
{
public function icon($user)
{
if (!Uuid::isValid($user)) {
abort(422);
}
try {
$user = User::findOrFail($user);
$file = $user->icon ? $user->icon : '/images/user.png';
return response()->file(public_path($file));
} catch (ModelNotFoundException $e) {
abort(404);
}
}
public function index(Request $request)
{
$offset = intval($request->input('start', 0));
$users = User::select(['user_id', 'display_name'])
->offset(is_int($offset) ? $offset : 0)
->take(10)
->get();
return view('user/index', ['users' => $users, 'title' => 'View User']);
}
public function create()
{
return view('user/create', ['title' => 'Create User']);
}
public function show($user)
{
try {
if (!Uuid::isValid($user)) {
abort(422);
}
$user = User::with('roles')->with('permissions')->findOrFail($user);
return view('user/show', ['theUser' => $user]);
} catch (ModelNotFoundException $e) {
abort(404);
}
}
} | <?php
namespace App\Http\Controllers;
use App\Models\User;
use Illuminate\Database\Eloquent\ModelNotFoundException;
use Illuminate\Http\Request;
use Ramsey\Uuid\Uuid;
class UserController extends Controller
{
public function icon($user)
{
if (!Uuid::isValid($user)) {
abort(422);
}
try {
$user = User::findOrFail($user);
$file = $user->icon ? $user->icon : '/images/user.png';
return response()->file(public_path($file));
} catch (ModelNotFoundException $e) {
abort(404);
}
}
public function index(Request $request)
{
$offset = intval($request->input('start', 0));
$users = User::select(['user_id', 'display_name'])
->offset(is_int($offset) ? $offset : 0)
->take(10)
->get();
return view('user/index', ['users' => $users, 'title' => 'View User']);
}
public function create()
{
return view('user/create', ['title' => 'Create User']);
}
public function show($user)
{
try {
$user = User::findOrFail($user);
return view('user/show', ['theUser' => $user]);
} catch (ModelNotFoundException $e) {
abort(404);
}
}
} |
Use current user for tests | from .settings import * # noqa
DEBUG = True
TEMPLATE_DEBUG = True
DATABASES = {
'default': {
'ENGINE': 'django.db.backends.sqlite3',
'NAME': 'db.sqlite',
},
'volatile': {
'ENGINE': 'django.db.backends.sqlite3',
'NAME': ':memory:',
}
}
# Non root user, ROOT for current one
RUN_AS_USER = None
LOGGING = {
'version': 1,
'disable_existing_loggers': False,
'formatters': {
'default': {
'format': '%(asctime)s [%(levelname)s] %(module)s - %(message)s'
},
'simple': {
'format': '%(levelname)s %(message)s'
},
},
'handlers': {
'null': {
'level': 'DEBUG',
'class': 'logging.NullHandler',
},
'console': {
'level': 'DEBUG',
'formatter': 'default',
'class': 'logging.StreamHandler',
},
},
'loggers': {
'': {
'handlers': ['null'],
'level': 'DEBUG',
'propagate': True,
},
'django.db.backends': {
'handlers': ['null'],
'propagate': False,
'level': 'DEBUG',
},
}
}
| from .settings import * # noqa
DEBUG = True
TEMPLATE_DEBUG = True
DATABASES = {
'default': {
'ENGINE': 'django.db.backends.sqlite3',
'NAME': 'db.sqlite',
},
'volatile': {
'ENGINE': 'django.db.backends.sqlite3',
'NAME': ':memory:',
}
}
# Non root user (root under development)
RUN_AS_USER = 'nobody'
LOGGING = {
'version': 1,
'disable_existing_loggers': False,
'formatters': {
'default': {
'format': '%(asctime)s [%(levelname)s] %(module)s - %(message)s'
},
'simple': {
'format': '%(levelname)s %(message)s'
},
},
'handlers': {
'null': {
'level': 'DEBUG',
'class': 'logging.NullHandler',
},
'console': {
'level': 'DEBUG',
'formatter': 'default',
'class': 'logging.StreamHandler',
},
},
'loggers': {
'': {
'handlers': ['null'],
'level': 'DEBUG',
'propagate': True,
},
'django.db.backends': {
'handlers': ['null'],
'propagate': False,
'level': 'DEBUG',
},
}
}
|
Fix area order. Algorithm is direction sensitive | iD.svg.Areas = function(projection) {
return function drawAreas(surface, graph, entities, filter) {
var areas = [];
for (var i = 0; i < entities.length; i++) {
var entity = entities[i];
if (entity.geometry(graph) === 'area') {
var points = graph.childNodes(entity).map(function(n) {
return projection(n.loc);
});
areas.push({
entity: entity,
area: entity.isDegenerate() ? 0 : Math.abs(d3.geom.polygon(points).area())
});
}
}
areas.sort(function(a, b) { return b.area - a.area; });
var lineString = iD.svg.LineString(projection, graph);
function drawPaths(group, areas, filter, classes) {
var paths = group.selectAll('path.area')
.filter(filter)
.data(areas, iD.Entity.key);
paths.enter()
.append('path')
.attr('class', classes);
paths
.order()
.attr('d', lineString)
.call(iD.svg.TagClasses())
.call(iD.svg.MemberClasses(graph));
paths.exit()
.remove();
return paths;
}
areas = _.pluck(areas, 'entity');
var fill = surface.select('.layer-fill'),
stroke = surface.select('.layer-stroke'),
fills = drawPaths(fill, areas, filter, 'way area fill'),
strokes = drawPaths(stroke, areas, filter, 'way area stroke');
};
};
| iD.svg.Areas = function(projection) {
return function drawAreas(surface, graph, entities, filter) {
var areas = [];
for (var i = 0; i < entities.length; i++) {
var entity = entities[i];
if (entity.geometry(graph) === 'area') {
var points = graph.childNodes(entity).map(function(n) {
return projection(n.loc);
});
areas.push({
entity: entity,
area: entity.isDegenerate() ? 0 : d3.geom.polygon(points).area()
});
}
}
areas.sort(function(a, b) { return a.area - b.area; });
var lineString = iD.svg.LineString(projection, graph);
function drawPaths(group, areas, filter, classes) {
var paths = group.selectAll('path.area')
.filter(filter)
.data(areas, iD.Entity.key);
paths.enter()
.append('path')
.attr('class', classes);
paths
.order()
.attr('d', lineString)
.call(iD.svg.TagClasses())
.call(iD.svg.MemberClasses(graph));
paths.exit()
.remove();
return paths;
}
areas = _.pluck(areas, 'entity');
var fill = surface.select('.layer-fill'),
stroke = surface.select('.layer-stroke'),
fills = drawPaths(fill, areas, filter, 'way area fill'),
strokes = drawPaths(stroke, areas, filter, 'way area stroke');
};
};
|
Fix supertest to not overwrite request | var assert = require('chai').assert;
var http = require('http');
var mathServer = require('../lib/mathServer.js');
var request = require('supertest');
process.env.NODE_ENV = 'test';
describe.only('Acceptance test', function () {
var port = 8006;
var server;
before(function () {
server = mathServer.MathServer({
port: port,
CONTAINERS: './dummy_containers.js'
});
server.listen();
request = request('http://localhost:' + port);
});
after(function (done) {
server.close();
done();
});
describe('As basic behavior we', function () {
it('should be able to create server and get title from html body', function (done) {
http.get("http://127.0.0.1:" + port, function (res) {
res.on('data', function (body) {
var str = body.toString('utf-8');
var n = str.match(/<title>\s*([^\s]*)\s*<\/title>/);
assert.equal(n[1], 'Macaulay2');
done();
});
});
});
it('should show title', function (done) {
request.get('/').expect(200).end(function(error, result) {
assert.match(result.text, /<title>\s*Macaulay2\s*<\/title>/);
done();
});
});
});
});
| var assert = require('chai').assert;
var http = require('http');
var mathServer = require('../lib/mathServer.js');
var request = require('supertest');
process.env.NODE_ENV = 'test';
describe('Acceptance test', function () {
var port = 8006;
var server;
before(function () {
process.on('stdout', function(data) {
console.log('**' + data);
});
process.on('stderr', function(data) {
console.log('**' + data);
});
server = mathServer.MathServer({
port: port,
CONTAINERS: './dummy_containers.js'
});
server.listen();
});
after(function (done) {
server.close();
done();
});
describe('As basic behavior we', function () {
it('should be able to create server and get title from html body', function (done) {
http.get("http://127.0.0.1:" + port, function (res) {
res.on('data', function (body) {
var str = body.toString('utf-8');
var n = str.match(/<title>\s*([^\s]*)\s*<\/title>/);
assert.equal(n[1], 'Macaulay2');
done();
});
});
});
it('should show title', function (done) {
request = request('http://localhost:' + port);
request.get('/').expect(200).end(function(error, result) {
assert.match(result.text, /<title>\s*Macaulay2\s*<\/title>/);
done();
});
});
});
});
|
Use ES5 syntax for getter | var get_system_locale_name = require('../build/Release/get_system_locale_name')
module.exports = {
get name() {
var systemLocaleName = get_system_locale_name()
return parseLocaleName(systemLocaleName)
}
}
//
// PRIVATE
//
/**
* POSIX specifies the following format:
* [language[_territory][.codeset][@modifier]]
*/
var LOCALE_NAME_REGEXP = /^([a-z]+)(_[A-Z]+)?(\.[a-zA-Z0-9_-]+)?(@[a-zA-Z0-9]+)?$/
/**
* Parses `localeName` as a POSIX locale.
*/
function parseLocaleName(localeName) {
if (null == localeName) {
return
}
var res = {
locale: localeName
}
var m = localeName.match(LOCALE_NAME_REGEXP)
if (null != m) {
m.shift()
res.language = m.shift()
while (m.length > 0) {
var val = m.shift()
if (null == val) {
continue
}
switch (val[0]) {
case '_':
res.territory = val.substr(1)
break
case '.':
res.codeset = val.substr(1)
break
case '@':
res.modifier = val.substr(1)
break
default:
// Ignore...
break
}
}
}
return res
}
| var get_system_locale_name = require('../build/Release/get_system_locale_name')
module.exports.__defineGetter__('name', function getSystemLocaleName() {
var systemLocaleName = get_system_locale_name()
return parseLocaleName(systemLocaleName)
})
//
// PRIVATE
//
/**
* POSIX specifies the following format:
* [language[_territory][.codeset][@modifier]]
*/
var LOCALE_NAME_REGEXP = /^([a-z]+)(_[A-Z]+)?(\.[a-zA-Z0-9_-]+)?(@[a-zA-Z0-9]+)?$/
/**
* Parses `localeName` as a POSIX locale.
*/
function parseLocaleName(localeName) {
if (null == localeName) {
return
}
var res = {
locale: localeName
}
var m = localeName.match(LOCALE_NAME_REGEXP)
if (null != m) {
m.shift()
res.language = m.shift()
while (m.length > 0) {
var val = m.shift()
if (null == val) {
continue
}
switch (val[0]) {
case '_':
res.territory = val.substr(1)
break
case '.':
res.codeset = val.substr(1)
break
case '@':
res.modifier = val.substr(1)
break
default:
// Ignore...
break
}
}
}
return res
}
|
Fix to improperly disabled docs | #!/usr/bin/env python
from ez_setup import use_setuptools
use_setuptools()
from setuptools import setup
import re
main_py = open('flatcat/__init__.py').read()
metadata = dict(re.findall("__([a-z]+)__ = '([^']+)'", main_py))
requires = [
'morfessor',
]
setup(name='Morfessor FlatCat',
version=metadata['version'],
author=metadata['author'],
author_email='[email protected]',
url='http://www.cis.hut.fi/projects/morpho/',
description='Morfessor FlatCat',
packages=['flatcat', 'flatcat.tests'],
classifiers=[
'Development Status :: 4 - Beta',
'Intended Audience :: Science/Research',
'License :: OSI Approved :: BSD License',
'Operating System :: OS Independent',
'Programming Language :: Python',
'Topic :: Scientific/Engineering',
],
license="BSD",
scripts=['scripts/flatcat',
'scripts/flatcat-train',
'scripts/flatcat-segment',
'scripts/flatcat-diagnostics',
'scripts/flatcat-reformat'
],
install_requires=requires,
#extras_require={
# 'docs': [l.strip() for l in open('docs/build_requirements.txt')]
#}
)
| #!/usr/bin/env python
from ez_setup import use_setuptools
use_setuptools()
from setuptools import setup
import re
main_py = open('flatcat/__init__.py').read()
metadata = dict(re.findall("__([a-z]+)__ = '([^']+)'", main_py))
requires = [
'morfessor',
]
setup(name='Morfessor FlatCat',
version=metadata['version'],
author=metadata['author'],
author_email='[email protected]',
url='http://www.cis.hut.fi/projects/morpho/',
description='Morfessor FlatCat',
packages=['flatcat', 'flatcat.tests'],
classifiers=[
'Development Status :: 4 - Beta',
'Intended Audience :: Science/Research',
'License :: OSI Approved :: BSD License',
'Operating System :: OS Independent',
'Programming Language :: Python',
'Topic :: Scientific/Engineering',
],
license="BSD",
scripts=['scripts/flatcat',
'scripts/flatcat-train',
'scripts/flatcat-segment',
'scripts/flatcat-diagnostics',
'scripts/flatcat-reformat'
],
install_requires=requires,
extras_require={
'docs': [l.strip() for l in open('docs/build_requirements.txt')]
}
)
|
CRM-1659: Implement DoctrineExtensions for Oro - fixed php 5.3 incompatible test | <?php
namespace Oro\Tests\DBAL\Types;
use Doctrine\DBAL\Platforms\MySqlPlatform;
use Doctrine\DBAL\Types\Type;
use Oro\DBAL\Types\PercentType;
use Oro\Tests\Connection\TestUtil;
class PercentTypeTest extends \PHPUnit_Framework_TestCase
{
/**
* @var PercentType
*/
protected $percentType;
protected function setUp()
{
if (!Type::hasType(PercentType::TYPE)) {
Type::addType(PercentType::TYPE, 'Oro\DBAL\Types\PercentType');
}
$this->percentType = Type::getType(PercentType::TYPE);
}
public function testGetName()
{
$this->assertEquals('percent', $this->percentType->getName());
}
public function testGetSQLDeclaration()
{
$platform = TestUtil::getEntityManager()->getConnection()->getDatabasePlatform();
$output = $this->percentType->getSQLDeclaration(array(), $platform);
$this->assertEquals("DOUBLE PRECISION", $output);
}
public function testConvertToPHPValue()
{
$platform = TestUtil::getEntityManager()->getConnection()->getDatabasePlatform();
$this->assertNull($this->percentType->convertToPHPValue(null, $platform));
$this->assertEquals(12.4, $this->percentType->convertToPHPValue(12.4, $platform));
}
public function testRequiresSQLCommentHint()
{
$platform = TestUtil::getEntityManager()->getConnection()->getDatabasePlatform();
$this->assertTrue($this->percentType->requiresSQLCommentHint($platform));
}
}
| <?php
namespace Oro\Tests\DBAL\Types;
use Doctrine\DBAL\Platforms\MySqlPlatform;
use Doctrine\DBAL\Types\Type;
use Oro\DBAL\Types\PercentType;
use Oro\Tests\Connection\TestUtil;
class PercentTypeTest extends \PHPUnit_Framework_TestCase
{
/**
* @var PercentType
*/
protected $percentType;
protected function setUp()
{
if (!Type::hasType(PercentType::TYPE)) {
Type::addType(PercentType::TYPE, 'Oro\DBAL\Types\PercentType');
}
$this->percentType = Type::getType(PercentType::TYPE);
}
public function testGetName()
{
$this->assertEquals('percent', $this->percentType->getName());
}
public function testGetSQLDeclaration()
{
$platform = TestUtil::getEntityManager()->getConnection()->getDatabasePlatform();
$output = $this->percentType->getSQLDeclaration([], $platform);
$this->assertEquals("DOUBLE PRECISION", $output);
}
public function testConvertToPHPValue()
{
$platform = TestUtil::getEntityManager()->getConnection()->getDatabasePlatform();
$this->assertNull($this->percentType->convertToPHPValue(null, $platform));
$this->assertEquals(12.4, $this->percentType->convertToPHPValue(12.4, $platform));
}
public function testRequiresSQLCommentHint()
{
$platform = TestUtil::getEntityManager()->getConnection()->getDatabasePlatform();
$this->assertTrue($this->percentType->requiresSQLCommentHint($platform));
}
}
|
Add link to Google Scholar page. | <html>
<head>
<title>Papers</title>
<meta http-equiv="Content-Type" content="text/html; charset=ISO-8859-1">
<link href="css/style.css" rel="stylesheet" type="text/css">
</head>
<body>
<div id="dvmaincontainer">
<?php include 'menu.php'; ?>
<div id="dvbodycontainer">
<div id="dvbannerbgcontainer">
<div class="lftcontainer">
<div class="dvbannerleft">
<p>PUBLICATIONS</p>
</div>
</div>
<div id="dvbanner">
</div>
</div>
<div id="dvmainpanel"> <p>
You can find my Google Scholar page
<a href="http://scholar.google.com/citations?user=eY2crRsAAAAJ">here</a>
to see more statistics and anything I might have missed.
<?php include 'papers.html'; ?>
</p> </div>
</div>
</div>
</body>
</html>
| <html>
<head>
<title>Papers</title>
<meta http-equiv="Content-Type" content="text/html; charset=ISO-8859-1">
<link href="css/style.css" rel="stylesheet" type="text/css">
</head>
<body>
<div id="dvmaincontainer">
<?php include 'menu.php'; ?>
<div id="dvbodycontainer">
<div id="dvbannerbgcontainer">
<div class="lftcontainer">
<div class="dvbannerleft">
<p>PUBLICATIONS</p>
</div>
</div>
<div id="dvbanner">
</div>
</div>
<div id="dvmainpanel">
<?php include 'papers.html'; ?>
</div>
</div>
</div>
</body>
</html>
|
Add name and id to success action | import _ from 'lodash'
import { take, put, spawn, call, select } from 'redux-saga/effects'
import {
TASK__START,
TASK__PROGRESS,
TASK__ERROR,
TASK__CLEAR,
} from '../../actions'
function* taskSaga(task, action) {
yield put({ ...action, type: TASK__PROGRESS })
try {
const result = yield call(task, action.payload)
const { id, name, payload, onSuccessDispatch } = action
if (onSuccessDispatch) {
yield put({
type: onSuccessDispatch,
name,
id,
payload,
result,
})
}
yield put({ type: TASK__CLEAR, id, name })
} catch (error) {
const { id, name } = action
yield put({
type: TASK__ERROR,
id,
name,
errorMessage: error.message,
})
}
}
export default (registry) =>
function* tasksSaga() {
while (true) {
const action = yield take(TASK__START)
const { name, id } = action
const task = registry[action.name]
if (!task) {
throw Error(`Task "${name}" is not registered!`)
}
const status = yield select((state) =>
_.get(state, ['tasks', name, id, 'status'])
)
if (status === 'progress') {
throw Error(
`Cannot start task "${name}.${id}" because it is already in progress!`
)
}
yield spawn(taskSaga, task, action)
}
}
| import _ from 'lodash'
import { take, put, spawn, call, select } from 'redux-saga/effects'
import {
TASK__START,
TASK__PROGRESS,
TASK__SUCCESS,
TASK__ERROR,
TASK__CLEAR,
} from '../../actions'
function* taskSaga(task, action) {
yield put({ ...action, type: TASK__PROGRESS })
try {
const result = yield call(task, action.payload)
const { id, name, payload, onSuccessDispatch } = action
if (onSuccessDispatch) {
yield put({
type: onSuccessDispatch,
payload,
result,
})
}
yield put({ type: TASK__CLEAR, id, name })
} catch (error) {
const { id, name } = action
yield put({
type: TASK__ERROR,
id,
name,
errorMessage: error.message,
})
}
}
export default (registry) =>
function* tasksSaga() {
while (true) {
const action = yield take(TASK__START)
const { name, id } = action
const task = registry[action.name]
if (!task) {
throw Error(`Task "${name}" is not registered!`)
}
const status = yield select((state) =>
_.get(state, ['tasks', name, id, 'status'])
)
if (status === 'progress') {
throw Error(
`Cannot start task "${name}.${id}" because it is already in progress!`
)
}
yield spawn(taskSaga, task, action)
}
}
|
Fix problems in displaying common style of some article components
Fix problems in displaying common style of some article components | /*eslint no-unused-vars:0*/
'use strict'
import _ from 'lodash'
import React, { Component } from 'react'
import classNames from 'classnames'
import commonStyles from './Common.scss'
import getArticleComponent from './getArticleComponent'
import styles from './Body.scss'
export class Body extends Component {
constructor(props) {
super(props)
}
render() {
const { data, customStyles } = this.props
if (Array.isArray(data)) {
let Blocks = data.map((ele) => {
let type = ele.type
let Component = getArticleComponent(type)
if (!Component) {
return null
}
return (
<div
key={ele.id}
className={classNames(commonStyles['component'], commonStyles[type])}
style={_.get(customStyles, 'component', {})}
>
<Component
alignment={ele.alignment}
content={ele.content}
customStyles={ele.styles}
id={ele.id}
/>
</div>
)
})
return (
<div
className={commonStyles['components']}
style={_.get(customStyles, 'components', {})}
>
{Blocks}
</div>
)
}
}
}
| /*eslint no-unused-vars:0*/
'use strict'
import _ from 'lodash'
import React, { Component } from 'react'
import classNames from 'classnames'
import commonStyles from './Common.scss'
import getArticleComponent from './getArticleComponent'
import styles from './Body.scss'
export class Body extends Component {
constructor(props) {
super(props)
}
render() {
const { data, customStyles } = this.props
if (Array.isArray(data)) {
let Blocks = data.map((ele) => {
let type = ele.type
let Component = getArticleComponent(type)
if (!Component) {
return null
}
return (
<div
key={ele.id}
className={classNames(commonStyles['component'], styles[type])}
style={_.get(customStyles, 'component', {})}
>
<Component
alignment={ele.alignment}
content={ele.content}
customStyles={ele.styles}
id={ele.id}
/>
</div>
)
})
return (
<div
className={commonStyles['components']}
style={_.get(customStyles, 'components', {})}
>
{Blocks}
</div>
)
}
}
}
|
Save a bit of space when saving logs | define("game-manager", ["game"], function(Game) {
function GameManager() {
this.games = this.load() || [];
this.next_id = this.getNextId();
}
GameManager.prototype = {
addGame: function() {
var game = new Game(this.next_id++);
this.games.push(game);
this.save();
return game;
},
deleteGame: function(game) {
var index = this.games.indexOf(game);
if (index == -1)
throw new Error("Bad game");
this.games.splice(index, 1);
},
findGame: function(id) {
for (var i = 0; i < this.games.length; i++)
if (this.games[i].id == id)
return this.games[i];
},
listGames: function() {
return this.games;
},
getNextId: function() {
var next = 1;
this.games.forEach(function(g) {
next = Math.max(next, g.id + 1);
});
return next;
},
load: function() {
return (JSON.parse(localStorage.getItem('games')) || []).map(function(game) {
return new Game(game.id, game);
});
},
save: function() {
// remove "deleted": false fields from all logs (unnecessary)
this.games.map(function(game) {
game.log.map(function(e) {
if (!e.deleted)
e.deleted = undefined;
});
});
localStorage.setItem('games', JSON.stringify(this.games));
},
};
return GameManager;
});
| define("game-manager", ["game"], function(Game) {
function GameManager() {
this.games = this.load() || [];
this.next_id = this.getNextId();
}
GameManager.prototype = {
addGame: function() {
var game = new Game(this.next_id++);
this.games.push(game);
this.save();
return game;
},
deleteGame: function(game) {
var index = this.games.indexOf(game);
if (index == -1)
throw new Error("Bad game");
this.games.splice(index, 1);
},
findGame: function(id) {
for (var i = 0; i < this.games.length; i++)
if (this.games[i].id == id)
return this.games[i];
},
listGames: function() {
return this.games;
},
getNextId: function() {
var next = 1;
this.games.forEach(function(g) {
next = Math.max(next, g.id + 1);
});
return next;
},
load: function() {
return (JSON.parse(localStorage.getItem('games')) || []).map(function(game) {
return new Game(game.id, game);
});
},
save: function() {
localStorage.setItem('games', JSON.stringify(this.games));
},
};
return GameManager;
});
|
Remove unused if statement for error handling
Producer was trying to catch an error that could not been tested so this
line was removed to avoid dead code. | <?php
namespace MicroQueue;
class Producer
{
private $queue = null;
const ERROR_PRODUCER_QUEUE_IS_FULL = 'Queue reached max number of messages';
const ERROR_PRODUCER_SEND_UNKNOWN_ERROR = 'Unknown error';
const PRODUCER_DEFAULT_MESSAGE_TYPE = 1;
const ERROR_PRODUCER_MESSAGE_BUFFER_OVERFLOW = 'Message size is larger than the allowed value';
public function __construct(Queue $queue)
{
$this->queue = $queue;
}
public function publish($message)
{
if (empty($message)) throw new \RuntimeException('Message cannot be empty');
$queueResource = $this->queue->getResource();
$serializeMessage = true;
$isBlocking = true;
$errorCode = 0;
$result = @msg_send(
$queueResource,
self::PRODUCER_DEFAULT_MESSAGE_TYPE,
$message,
$serializeMessage,
$isBlocking,
$errorCode
);
if (false === $result) throw new \RuntimeException($this->parseErrorCode($errorCode));
return true;
}
private function parseErrorCode($errorCode)
{
if (PosixErrorCode::EINVAL == $errorCode) return self::ERROR_PRODUCER_MESSAGE_BUFFER_OVERFLOW;
return self::ERROR_PRODUCER_SEND_UNKNOWN_ERROR;
}
}
| <?php
namespace MicroQueue;
class Producer
{
private $queue = null;
const ERROR_PRODUCER_QUEUE_IS_FULL = 'Queue reached max number of messages';
const ERROR_PRODUCER_SEND_UNKNOWN_ERROR = 'Unknown error';
const PRODUCER_DEFAULT_MESSAGE_TYPE = 1;
const ERROR_PRODUCER_MESSAGE_BUFFER_OVERFLOW = 'Message size is larger than the allowed value';
public function __construct(Queue $queue)
{
$this->queue = $queue;
}
public function publish($message)
{
if (empty($message)) throw new \RuntimeException('Message cannot be empty');
$queueResource = $this->queue->getResource();
$serializeMessage = true;
$isBlocking = true;
$errorCode = 0;
$result = @msg_send(
$queueResource,
self::PRODUCER_DEFAULT_MESSAGE_TYPE,
$message,
$serializeMessage,
$isBlocking,
$errorCode
);
if (false === $result) throw new \RuntimeException($this->parseErrorCode($errorCode));
return true;
}
private function parseErrorCode($errorCode)
{
if (MSG_EAGAIN == $errorCode) {
return self::ERROR_PRODUCER_QUEUE_IS_FULL;
} elseif (PosixErrorCode::EINVAL == $errorCode) {
return self::ERROR_PRODUCER_MESSAGE_BUFFER_OVERFLOW;
}
}
}
|
Include mask size on docker CIDR. | from pyinfra import inventory, state
from pyinfra_docker import deploy_docker
from pyinfra_etcd import deploy_etcd
from pyinfra_kubernetes import deploy_kubernetes_master, deploy_kubernetes_node
SUDO = True
FAIL_PERCENT = 0
def get_etcd_nodes():
return [
'http://{0}:2379'.format(
etcd_node.fact.network_devices[etcd_node.data.etcd_interface]
['ipv4']['address'],
)
for etcd_node in inventory.get_group('etcd_nodes')
]
# Install/configure etcd cluster
with state.limit('etcd_nodes'):
deploy_etcd()
# Install/configure the masters (apiserver, controller, scheduler)
with state.limit('kubernetes_masters'):
deploy_kubernetes_master(etcd_nodes=get_etcd_nodes())
# Install/configure the nodes
with state.limit('kubernetes_nodes'):
# Install Docker
deploy_docker(config={
# Make Docker use the Vagrant provided interface which has it's own /24
'bip': '{{ host.fact.network_devices[host.data.network_interface].ipv4.address }}/24',
})
# Install Kubernetes node components (kubelet, kube-proxy)
first_master = inventory.get_group('kubernetes_masters')[0]
deploy_kubernetes_node(
master_address='http://{0}'.format((
first_master
.fact.network_devices[first_master.data.network_interface]
['ipv4']['address']
)),
)
| from pyinfra import inventory, state
from pyinfra_docker import deploy_docker
from pyinfra_etcd import deploy_etcd
from pyinfra_kubernetes import deploy_kubernetes_master, deploy_kubernetes_node
SUDO = True
FAIL_PERCENT = 0
def get_etcd_nodes():
return [
'http://{0}:2379'.format(
etcd_node.fact.network_devices[etcd_node.data.etcd_interface]
['ipv4']['address'],
)
for etcd_node in inventory.get_group('etcd_nodes')
]
# Install/configure etcd cluster
with state.limit('etcd_nodes'):
deploy_etcd()
# Install/configure the masters (apiserver, controller, scheduler)
with state.limit('kubernetes_masters'):
deploy_kubernetes_master(etcd_nodes=get_etcd_nodes())
# Install/configure the nodes
with state.limit('kubernetes_nodes'):
# Install Docker
deploy_docker(config={
# Make Docker use the Vagrant provided interface which has it's own /24
'bip': '{{ host.fact.network_devices[host.data.network_interface].ipv4.address }}',
})
# Install Kubernetes node components (kubelet, kube-proxy)
first_master = inventory.get_group('kubernetes_masters')[0]
deploy_kubernetes_node(
master_address='http://{0}'.format((
first_master
.fact.network_devices[first_master.data.network_interface]
['ipv4']['address']
)),
)
|
Fix layer model serialize method to use new organization | cinema.models.LayerModel = Backbone.Model.extend({
constructor: function (defaults, options) {
Backbone.Model.call(this, {}, options);
if (typeof defaults === 'string') {
this.setFromString(defaults);
} else if (defaults) {
this.set('state', defaults);
}
},
/**
* Convert an object that maps layer identifiers to color-by values into
* a single string that is consumable by other parts of the application.
*/
serialize: function () {
var query = '';
_.each(this.get('state'), function (v, k) {
query += k + v;
});
return query;
},
/**
* Convert a query string to an object that maps layer identifiers to
* their color-by value. The query string is a sequence of two-character
* pairs, where the first character identifies the layer ID and the second
* character identifies which field it should be colored by.
*
* The query string is then saved to the model.
*/
unserialize: function (query) {
var obj = {};
if (query.length % 2) {
return console.error('Query string "' + query + '" has odd length.');
}
for (var i = 0; i < query.length; i += 2) {
obj[query[i]] = query[i + 1];
}
return obj;
},
/**
* Set the layers by a "query string".
*/
setFromString: function (query) {
var obj = this.unserialize(query);
this.set('state', obj);
}
});
| cinema.models.LayerModel = Backbone.Model.extend({
constructor: function (defaults, options) {
Backbone.Model.call(this, {}, options);
if (typeof defaults === 'string') {
this.setFromString(defaults);
} else if (defaults) {
this.set('state', defaults);
}
},
/**
* Convert an object that maps layer identifiers to color-by values into
* a single string that is consumable by other parts of the application.
*/
serialize: function () {
var query = '';
_.each(this.attributes, function (v, k) {
query += k + v;
});
return query;
},
/**
* Convert a query string to an object that maps layer identifiers to
* their color-by value. The query string is a sequence of two-character
* pairs, where the first character identifies the layer ID and the second
* character identifies which field it should be colored by.
*
* The query string is then saved to the model.
*/
unserialize: function (query) {
var obj = {};
if (query.length % 2) {
return console.error('Query string "' + query + '" has odd length.');
}
for (var i = 0; i < query.length; i += 2) {
obj[query[i]] = query[i + 1];
}
return obj;
},
/**
* Set the layers by a "query string".
*/
setFromString: function (query) {
var obj = this.unserialize(query);
this.set('state', obj);
}
});
|
Add actions to start vs computer
Change onClick action handlers for buttons | import React from "react";
import Container from "./Container.js";
import Button from "./../Shared/Button.js";
import setCurrentScreenAction from "../../actions/ScreenActions.js";
import { GAME_SCREEN } from "../../actions/ScreenActions.js";
import {setGameModeAction} from "../../actions/GameActions.js";
import {VS_HUMAN, EASY, MEDIUM, HARD} from "../../reducers/Game.js";
const screenTitle = "Tic Tac Toe React";
const MenuScreen = React.createClass({
PropTypes: {
dispatch: React.PropTypes.func.isRequired
},
headerStyle: {
textAlign: "center",
color: "white"
},
render () {
return (
<Container>
<h1 style={this.headerStyle}>
{screenTitle}
</h1>
<Button onClick={() => {
this.props.dispatch(setCurrentScreenAction(GAME_SCREEN))
this.props.dispatch(setGameModeAction(VS_HUMAN));
}
}
text="Play with Human"
useWrapper
/>
<h2 style={this.headerStyle}>
{"Play with computer"}
</h2>
<Button onClick={() => {
this.props.dispatch(setCurrentScreenAction(GAME_SCREEN))
this.props.dispatch(setGameModeAction(EASY));
}
}
text="EASY"
useWrapper
/>
<Button onClick={() => {
this.props.dispatch(setCurrentScreenAction(GAME_SCREEN))
this.props.dispatch(setGameModeAction(MEDIUM));
}
}
text="MEDIUM"
useWrapper
/>
<Button onClick={() => {
this.props.dispatch(setCurrentScreenAction(GAME_SCREEN))
this.props.dispatch(setGameModeAction(HARD));
}
}
text="HARD"
useWrapper
/>
</Container>
);
}
});
export default MenuScreen;
| import React from "react";
import Container from "./Container.js";
import Button from "./../Shared/Button.js";
import setCurrentScreenAction from "../../actions/ScreenActions.js";
import { GAME_SCREEN } from "../../actions/ScreenActions.js";
const screenTitle = "Tic Tac Toe React";
const MenuScreen = React.createClass({
PropTypes: {
dispatch: React.PropTypes.func.isRequired
},
headerStyle: {
textAlign: "center",
color: "white"
},
render () {
return (
<Container>
<h1 style={this.headerStyle}>
{screenTitle}
</h1>
<Button onClick={() => this.props.dispatch(
setCurrentScreenAction(GAME_SCREEN)
)}
text="Play with Human"
useWrapper
/>
<h2 style={this.headerStyle}>
{"Play with computer"}
</h2>
<Button buttonClickHandler={()=>1}
text="EASY"
useWrapper
/>
<Button buttonClickHandler={()=>1}
text="MEDIUM"
useWrapper
/>
<Button buttonClickHandler={()=>1}
text="HARD"
useWrapper
/>
</Container>
);
}
});
export default MenuScreen;
|
Fix Pipeline Args Bug on dict length | #!/usr/bin/python
# -*- coding: utf-8 -*-
import random
from EmeraldAI.Entities.BaseObject import BaseObject
class PipelineArgs(BaseObject):
def __init__(self, input):
# Original Input
self.Input = input
self.Normalized = input
# Input Language | List of EmeraldAI.Entities.BaseObject.Word objects | List of parameters
self.Language = None
self.WordList = None
self.SentenceList = None
self.ParameterList = None
# Input with parameterized data
self.ParameterizedInput = None
# TODO Conversation History
self.History = None
# Response with patameters | Raw response string | Response ID | Response found
self.Response = None
self.ResponseRaw = None
self.ResponseID = None
self.ResponseFound = False
self.ResponseAudio = None
# TODO
self.SessionID = 0
# TODO - List of Errors
self.Error = None
def GetSentencesWithHighestValue(self, margin=0):
if self.SentenceList != None and self.SentenceList:
highestRanking = max(node.Rating for node in self.SentenceList.values())
if margin > 0:
result = [node for node in self.SentenceList.values() if node.Rating>=(highestRanking-margin)]
else:
result = [node for node in self.SentenceList.values() if node.Rating==highestRanking]
return result
return None
def GetRandomSentenceWithHighestValue(self, margin=0):
result = self.GetSentencesWithHighestValue(margin)
if(result != None):
return random.choice(result)
return None
| #!/usr/bin/python
# -*- coding: utf-8 -*-
import random
from EmeraldAI.Entities.BaseObject import BaseObject
class PipelineArgs(BaseObject):
def __init__(self, input):
# Original Input
self.Input = input
self.Normalized = input
# Input Language | List of EmeraldAI.Entities.BaseObject.Word objects | List of parameters
self.Language = None
self.WordList = None
self.SentenceList = None
self.ParameterList = None
# Input with parameterized data
self.ParameterizedInput = None
# TODO Conversation History
self.History = None
# Response with patameters | Raw response string | Response ID | Response found
self.Response = None
self.ResponseRaw = None
self.ResponseID = None
self.ResponseFound = False
self.ResponseAudio = None
# TODO
self.SessionID = 0
# TODO - List of Errors
self.Error = None
def GetSentencesWithHighestValue(self, margin=0):
if self.SentenceList != None and len(self.SentenceList) > 0:
highestRanking = max(node.Rating for node in self.SentenceList.values())
if margin > 0:
result = [node for node in self.SentenceList.values() if node.Rating>=(highestRanking-margin)]
else:
result = [node for node in self.SentenceList.values() if node.Rating==highestRanking]
return result
return None
def GetRandomSentenceWithHighestValue(self, margin=0):
return random.choice(self.GetSentencesWithHighestValue(margin))
|
Fix for the jobqueue task. | <?php
namespace BasilFX\JobQueue\Contrib;
use Phalcon\CLI\Task;
use BasilFX\JobQueue\Job;
use BasilFX\JobQueue\Exception;
/**
* Phalcon JobQueue related tasks.
*/
class PhalconTask extends Task
{
/**
* Start the job broker loop.
*/
public function mainAction()
{
$running = true;
$success = 0;
$failed = 0;
echo "Starting worker task. To interrupt, press CTRL + C.\n";
// This is the main loop that will poll for tasks and process them.
while ($running) {
// Fetch the next job from the queue.
$job = $this->jobQueue->getNext();
if (!$job) {
usleep(500000);
continue;
}
// Process a job.
try {
echo "Processing job with ID {$job->getId()}.\n";
$start = microtime(true);
$this->jobQueue->process($job);
$time = (microtime(true) - $start) * 1000;
echo "Job with ID {$job->getId()} finished in $time ms.\n";
$success++;
} catch (\Exception $e) {
echo "Job with ID {$job->getId()} failed: {$e->getMessage()}.\n";
$failed++;
}
}
echo "Worker task stopped, $success successful and $failed failed tasks processed.\n";
}
}
| <?php
namespace BasilFX\JobQueue\Contrib;
use Phalcon\CLI\Task;
use BasilFX\JobQueue\Job;
use BasilFX\JobQueue\Exception;
/**
* @description("Job queue tasks runner")
*/
class JobQueueTask extends Task
{
/**
* Start the job broker loop.
*
* @description("Spawn a new worker process to handle queued jobs")
*/
public function mainAction()
{
$running = true;
echo "Starting worker task until. To interrupt, press CTRL + C.\n";
// This is the main loop that will poll for tasks and process them.
while ($running) {
// Fetch the next job from the queue.
$job = $this->jobQueue->getNext();
if (!$job) {
usleep(500000);
continue;
}
// Process a job.
try {
echo "Processing job with ID {$job->getId()}.\n";
$start = microtime(true);
$this->jobQueue->process($job);
$time = (microtime(true) - $start) * 1000;
echo "Job with ID {$job->getId()} finished in $time ms.\n";
} catch (\Exception $e) {
echo "Job with ID {$job->getId()} failed: {$e->getMessage()}.\n";
}
}
echo "Worker task stopped.\n";
}
}
|
Add tension support to the Line series component | (function(d3, fc) {
'use strict';
fc.series.line = function() {
// convenience functions that return the x & y screen coords for a given point
var x = function(d) { return line.xScale.value(line.xValue.value(d)); };
var y = function(d) { return line.yScale.value(line.yValue.value(d)); };
var lineData = d3.svg.line()
.defined(function(d) {
return !isNaN(y(d));
})
.x(x)
.y(y);
var line = function(selection) {
selection.each(function(data) {
var path = d3.select(this)
.selectAll('path.line')
.data([data]);
path.enter()
.append('path')
.attr('class', 'line');
path.attr('d', lineData);
line.decorate.value(path);
});
};
line.decorate = fc.utilities.property(fc.utilities.fn.noop);
line.xScale = fc.utilities.property(d3.time.scale());
line.yScale = fc.utilities.property(d3.scale.linear());
line.yValue = fc.utilities.property(function(d) { return d.close; });
line.xValue = fc.utilities.property(function(d) { return d.date; });
return d3.rebind(line, lineData, 'interpolate', 'tension');
};
}(d3, fc)); | (function(d3, fc) {
'use strict';
fc.series.line = function() {
// convenience functions that return the x & y screen coords for a given point
var x = function(d) { return line.xScale.value(line.xValue.value(d)); };
var y = function(d) { return line.yScale.value(line.yValue.value(d)); };
var lineData = d3.svg.line()
.defined(function(d) {
return !isNaN(y(d));
})
.x(x)
.y(y);
var line = function(selection) {
selection.each(function(data) {
var path = d3.select(this)
.selectAll('path.line')
.data([data]);
path.enter()
.append('path')
.attr('class', 'line');
path.attr('d', lineData);
line.decorate.value(path);
});
};
line.decorate = fc.utilities.property(fc.utilities.fn.noop);
line.xScale = fc.utilities.property(d3.time.scale());
line.yScale = fc.utilities.property(d3.scale.linear());
line.yValue = fc.utilities.property(function(d) { return d.close; });
line.xValue = fc.utilities.property(function(d) { return d.date; });
return d3.rebind(line, lineData, 'interpolate');
};
}(d3, fc)); |
Index forks only after their description has been set | <?php
namespace phorkie;
class Forker
{
public function forkLocal($repo)
{
$new = $this->fork($repo->gitDir);
\copy($repo->gitDir . '/description', $new->gitDir . '/description');
$this->index($new);
return $new;
}
public function forkRemote($cloneUrl, $originalUrl)
{
$new = $this->fork($cloneUrl);
file_put_contents(
$new->gitDir . '/description',
'Fork of ' . $originalUrl
);
$this->index($new);
return $new;
}
protected function fork($pathOrUrl)
{
$rs = new Repositories();
$new = $rs->createNew();
$vc = $new->getVc();
\rmdir($new->gitDir);//VersionControl_Git wants an existing dir, git clone not
$vc->getCommand('clone')
//this should be setOption, but it fails with a = between name and value
->addArgument('--separate-git-dir')
->addArgument($GLOBALS['phorkie']['cfg']['gitdir'] . '/' . $new->id . '.git')
->addArgument($pathOrUrl)
->addArgument($new->workDir)
->execute();
foreach (\glob($new->gitDir . '/hooks/*') as $hookfile) {
\unlink($hookfile);
}
return $new;
}
protected function index($repo)
{
$db = new Database();
$db->getIndexer()->addRepo($repo);
}
}
?>
| <?php
namespace phorkie;
class Forker
{
public function forkLocal($repo)
{
$new = $this->fork($repo->gitDir);
\copy($repo->gitDir . '/description', $new->gitDir . '/description');
return $new;
}
public function forkRemote($cloneUrl, $originalUrl)
{
$new = $this->fork($cloneUrl);
file_put_contents(
$new->gitDir . '/description',
'Fork of ' . $originalUrl
);
return $new;
}
protected function fork($pathOrUrl)
{
$rs = new Repositories();
$new = $rs->createNew();
$vc = $new->getVc();
\rmdir($new->gitDir);//VersionControl_Git wants an existing dir, git clone not
$vc->getCommand('clone')
//this should be setOption, but it fails with a = between name and value
->addArgument('--separate-git-dir')
->addArgument($GLOBALS['phorkie']['cfg']['gitdir'] . '/' . $new->id . '.git')
->addArgument($pathOrUrl)
->addArgument($new->workDir)
->execute();
foreach (\glob($new->gitDir . '/hooks/*') as $hookfile) {
\unlink($hookfile);
}
$db = new Database();
$db->getIndexer()->addRepo($new);
return $new;
}
}
?>
|
Trim whitespace from podcast titles | if (!cbus.hasOwnProperty("server")) { cbus.server = {} }
(function() {
const request = require("request");
const x2j = require("xml2js");
const path = require("path");
cbus.server.getPodcastInfo = function(podcastUrl, callback) {
var podcastData = {};
request({
url: podcastUrl,
headers: REQUEST_HEADERS
}, function(err, result, body) {
x2j.parseString(body, function(err, result) {
if (err) {
console.log("error parsing xml", err);
callback(null)
} else {
var channel = result.rss.channel[0];
// console.log(channel);
// title
if (existsRecursive(channel, ["title", 0])) {
podcastData.title = channel.title[0].trim();
}
// publisher
if (existsRecursive(channel, ["itunes:author", 0])) {
podcastData.publisher = channel["itunes:author"][0];
}
// description
if (channel.description && channel.description[0]) {
podcastData.description = channel.description[0];
}
// image
if (existsRecursive(channel, ["image", 0, "url", 0])) {
podcastData.image = channel["image"][0]["url"][0];
} else if (existsRecursive(channel, ["itunes:image", 0])) {
podcastData.image = channel["itunes:image"][0].$.href;
}
callback(podcastData);
}
});
});
}
}());
| if (!cbus.hasOwnProperty("server")) { cbus.server = {} }
(function() {
const request = require("request");
const x2j = require("xml2js");
const path = require("path");
cbus.server.getPodcastInfo = function(podcastUrl, callback) {
var podcastData = {};
request({
url: podcastUrl,
headers: REQUEST_HEADERS
}, function(err, result, body) {
x2j.parseString(body, function(err, result) {
if (err) {
console.log("error parsing xml", err);
callback(null)
} else {
var channel = result.rss.channel[0];
// console.log(channel);
// title
if (existsRecursive(channel, ["title", 0])) {
podcastData.title = channel.title[0];
}
// publisher
if (existsRecursive(channel, ["itunes:author", 0])) {
podcastData.publisher = channel["itunes:author"][0];
}
// description
if (channel.description && channel.description[0]) {
podcastData.description = channel.description[0];
}
// image
if (existsRecursive(channel, ["image", 0, "url", 0])) {
podcastData.image = channel["image"][0]["url"][0];
} else if (existsRecursive(channel, ["itunes:image", 0])) {
podcastData.image = channel["itunes:image"][0].$.href;
}
callback(podcastData);
}
});
});
}
}());
|
Update user roles in store after owner transfer
Closes #3466
- Transferring the owner role is now done via a separate
endpoint and not through Ember-Data. As a result the
user role data needs to be updated manually.
- Updated the owner endpoint to return a response body
containing the updated user objects.
- Updated tests. | var TransferOwnerController = Ember.Controller.extend({
actions: {
confirmAccept: function () {
var user = this.get('model'),
url = this.get('ghostPaths.url').api('users', 'owner'),
self = this;
self.get('popover').closePopovers();
ic.ajax.request(url, {
type: 'PUT',
data: {
owner: [{
'id': user.get('id')
}]
}
}).then(function (response) {
// manually update the roles for the users that just changed roles
// because store.pushPayload is not working with embedded relations
if (response && Ember.isArray(response.users)) {
response.users.forEach(function (userJSON) {
var user = self.store.getById('user', userJSON.id),
role = self.store.getById('role', userJSON.roles[0].id);
user.set('role', role);
});
}
self.notifications.closePassive();
self.notifications.showSuccess('Ownership successfully transferred to ' + user.get('name'));
}).catch(function (error) {
self.notifications.closePassive();
self.notifications.showAPIError(error);
});
},
confirmReject: function () {
return false;
}
},
confirm: {
accept: {
text: 'YEP - I\'M SURE',
buttonClass: 'button-delete'
},
reject: {
text: 'CANCEL',
buttonClass: 'button'
}
}
});
export default TransferOwnerController; | var TransferOwnerController = Ember.Controller.extend({
actions: {
confirmAccept: function () {
var user = this.get('model'),
url = this.get('ghostPaths.url').api('users', 'owner'),
self = this;
self.get('popover').closePopovers();
ic.ajax.request(url, {
type: 'PUT',
data: {
owner: [{
'id': user.get('id')
}]
}
}).then(function () {
self.notifications.closePassive();
self.notifications.showSuccess('Ownership successfully transferred to ' + user.get('name'));
}).catch(function (error) {
self.notifications.closePassive();
self.notifications.showAPIError(error);
});
},
confirmReject: function () {
return false;
}
},
confirm: {
accept: {
text: 'YEP - I\'M SURE',
buttonClass: 'button-delete'
},
reject: {
text: 'CANCEL',
buttonClass: 'button'
}
}
});
export default TransferOwnerController; |
Use __destruct to validate implicitly. | <?php namespace Essence;
use Closure;
class Essence
{
/**
* @var array
*/
protected $configuration = [
"exception" => "Essence\Exceptions\AssertionException",
];
/**
* @var array
*/
protected $defaultConfiguration;
/**
* @var AssertionBuilder
*/
protected $builder;
/**
* @param mixed $value
* @return Essence
*/
public function __construct($value = null)
{
$this->defaultConfiguration = $this->configuration;
$this->builder = new AssertionBuilder($value);
}
/**
* Throws an exception (specified in the configuration) with the given error message.
*
* @param string|null $message
* @throws Exceptions\AssertionException|object
* @return void
*/
public function throwOnFailure($message = null)
{
$class = $this->configuration["exception"];
throw new $class($message);
}
/**
* Returns the configuration array.
*
* @return array
*/
public function getConfiguration()
{
return $this->configuration;
}
/**
* Allows you to configure Essence via a Closure.
*
* @param Closure $callback
* @return void
*/
public function configure(Closure $callback)
{
if ( ! is_array($configuration = $callback($this->configuration))) {
throw new Exceptions\InvalidConfigurationException($configuration);
}
$this->configuration = array_merge($this->defaultConfiguration, $configuration);
}
/**
* This trick allows us to implicitly perform the validation.
*
* @return void
*/
public function __destruct()
{
if ( ! $this->builder->validate()) {
$this->throwOnFailure($this->builder->getMessage());
}
}
}
| <?php namespace Essence;
use Closure;
class Essence
{
/**
* @var array
*/
protected $configuration = [
"exception" => "Essence\Exceptions\AssertionException",
];
/**
* @var array
*/
protected $defaultConfiguration;
/**
* @return Essence
*/
public function __construct()
{
$this->defaultConfiguration = $this->configuration;
}
/**
* Throws an exception (specified in the configuration) with the given error message.
*
* @param string|null $message
* @throws Exceptions\AssertionException|object
* @return void
*/
public function throwOnFailure($message = null)
{
$class = $this->configuration["exception"];
throw new $class($message);
}
/**
* Returns the configuration array.
*
* @return array
*/
public function getConfiguration()
{
return $this->configuration;
}
/**
* Allows you to configure Essence via a Closure.
*
* @param Closure $callback
* @return void
*/
public function configure(Closure $callback)
{
if ( ! is_array($configuration = $callback($this->configuration))) {
throw new Exceptions\InvalidConfigurationException($configuration);
}
$this->configuration = array_merge($this->defaultConfiguration, $configuration);
}
}
|
Increase version so that my tests can run... :-] | from setuptools import setup, find_packages
setup(
name='tw2.wysihtml5',
version='0.3',
description='WYSIHTML5 widget for ToscaWidgets2',
author='Moritz Schlarb',
author_email='[email protected]',
url='https://github.com/toscawidgets/tw2.wysihtml5',
install_requires=[
"tw2.core",
"tw2.forms",
'tw2.bootstrap.forms',
"Mako",
## Add other requirements here
# "Genshi",
],
packages=find_packages(exclude=['ez_setup', 'tests']),
namespace_packages=['tw2', 'tw2.bootstrap'],
zip_safe=False,
include_package_data=True,
test_suite='nose.collector',
entry_points="""
[tw2.widgets]
# Register your widgets so they can be listed in the WidgetBrowser
widgets = tw2.wysihtml5
bootstrap = tw2.bootstrap.wysihtml5
""",
keywords=[
'toscawidgets.widgets',
],
classifiers=[
'Development Status :: 3 - Alpha',
'Environment :: Web Environment',
'Environment :: Web Environment :: ToscaWidgets',
'Topic :: Software Development :: Libraries :: Python Modules',
'Topic :: Software Development :: Widget Sets',
'Intended Audience :: Developers',
'Operating System :: OS Independent',
'Programming Language :: Python',
],
)
| from setuptools import setup, find_packages
setup(
name='tw2.wysihtml5',
version='0.2',
description='WYSIHTML5 widget for ToscaWidgets2',
author='Moritz Schlarb',
author_email='[email protected]',
url='https://github.com/toscawidgets/tw2.wysihtml5',
install_requires=[
"tw2.core",
"tw2.forms",
'tw2.bootstrap.forms',
"Mako",
## Add other requirements here
# "Genshi",
],
packages=find_packages(exclude=['ez_setup', 'tests']),
namespace_packages=['tw2', 'tw2.bootstrap'],
zip_safe=False,
include_package_data=True,
test_suite='nose.collector',
entry_points="""
[tw2.widgets]
# Register your widgets so they can be listed in the WidgetBrowser
widgets = tw2.wysihtml5
bootstrap = tw2.bootstrap.wysihtml5
""",
keywords=[
'toscawidgets.widgets',
],
classifiers=[
'Development Status :: 3 - Alpha',
'Environment :: Web Environment',
'Environment :: Web Environment :: ToscaWidgets',
'Topic :: Software Development :: Libraries :: Python Modules',
'Topic :: Software Development :: Widget Sets',
'Intended Audience :: Developers',
'Operating System :: OS Independent',
'Programming Language :: Python',
],
)
|
Send methods need to return the result
Signed-off-by: Stefan Marr <[email protected]> | class AbstractObject(object):
def __init__(self):
pass
def send(self, frame, selector_string, arguments, universe):
# Turn the selector string into a selector
selector = universe.symbol_for(selector_string)
invokable = self.get_class(universe).lookup_invokable(selector)
return invokable.invoke(frame, self, arguments)
def send_does_not_understand(self, frame, selector, arguments, universe):
# Compute the number of arguments
number_of_arguments = selector.get_number_of_signature_arguments()
arguments_array = universe.new_array_with_length(number_of_arguments)
for i in range(0, number_of_arguments):
arguments_array.set_indexable_field(i, arguments[i])
args = [selector, arguments_array]
return self.send(frame, "doesNotUnderstand:arguments:", args, universe)
def send_unknown_global(self, frame, global_name, universe):
arguments = [global_name]
return self.send(frame, "unknownGlobal:", arguments, universe)
def send_escaped_block(self, frame, block, universe):
arguments = [block]
return self.send(frame, "escapedBlock:", arguments, universe)
def get_class(self, universe):
raise NotImplementedError("Subclasses need to implement get_class(universe).")
def is_invokable(self):
return False
def __str__(self):
from som.vm.universe import get_current
return "a " + self.get_class(get_current()).get_name().get_string()
| class AbstractObject(object):
def __init__(self):
pass
def send(self, frame, selector_string, arguments, universe):
# Turn the selector string into a selector
selector = universe.symbol_for(selector_string)
invokable = self.get_class(universe).lookup_invokable(selector)
return invokable.invoke(frame, self, arguments)
def send_does_not_understand(self, frame, selector, arguments, universe):
# Compute the number of arguments
number_of_arguments = selector.get_number_of_signature_arguments()
arguments_array = universe.new_array_with_length(number_of_arguments)
for i in range(0, number_of_arguments):
arguments_array.set_indexable_field(i, arguments[i])
args = [selector, arguments_array]
self.send(frame, "doesNotUnderstand:arguments:", args, universe)
def send_unknown_global(self, frame, global_name, universe):
arguments = [global_name]
self.send(frame, "unknownGlobal:", arguments, universe)
def send_escaped_block(self, frame, block, universe):
arguments = [block]
self.send(frame, "escapedBlock:", arguments, universe)
def get_class(self, universe):
raise NotImplementedError("Subclasses need to implement get_class(universe).")
def is_invokable(self):
return False
def __str__(self):
from som.vm.universe import get_current
return "a " + self.get_class(get_current()).get_name().get_string()
|
Add verbose name for SLURM package [WAL-1141] | from decimal import Decimal
from django.db import models
from django.core.validators import MinValueValidator
from django.utils.translation import ugettext_lazy as _
from nodeconductor.structure import models as structure_models
from nodeconductor_assembly_waldur.common import mixins as common_mixins
class SlurmPackage(common_mixins.ProductCodeMixin, models.Model):
class Meta(object):
verbose_name = _('SLURM package')
verbose_name_plural = _('SLURM packages')
PRICE_MAX_DIGITS = 14
PRICE_DECIMAL_PLACES = 10
service_settings = models.OneToOneField(structure_models.ServiceSettings,
related_name='+',
limit_choices_to={'type': 'SLURM'})
cpu_price = models.DecimalField(default=0,
verbose_name=_('Price for CPU hour'),
max_digits=PRICE_MAX_DIGITS,
decimal_places=PRICE_DECIMAL_PLACES,
validators=[MinValueValidator(Decimal('0'))])
gpu_price = models.DecimalField(default=0,
verbose_name=_('Price for GPU hour'),
max_digits=PRICE_MAX_DIGITS,
decimal_places=PRICE_DECIMAL_PLACES,
validators=[MinValueValidator(Decimal('0'))])
ram_price = models.DecimalField(default=0,
verbose_name=_('Price for GB RAM'),
max_digits=PRICE_MAX_DIGITS,
decimal_places=PRICE_DECIMAL_PLACES,
validators=[MinValueValidator(Decimal('0'))])
| from decimal import Decimal
from django.db import models
from django.core.validators import MinValueValidator
from django.utils.translation import ugettext_lazy as _
from nodeconductor.structure import models as structure_models
from nodeconductor_assembly_waldur.common import mixins as common_mixins
class SlurmPackage(common_mixins.ProductCodeMixin, models.Model):
PRICE_MAX_DIGITS = 14
PRICE_DECIMAL_PLACES = 10
service_settings = models.OneToOneField(structure_models.ServiceSettings,
related_name='+',
limit_choices_to={'type': 'SLURM'})
cpu_price = models.DecimalField(default=0,
verbose_name=_('Price for CPU hour'),
max_digits=PRICE_MAX_DIGITS,
decimal_places=PRICE_DECIMAL_PLACES,
validators=[MinValueValidator(Decimal('0'))])
gpu_price = models.DecimalField(default=0,
verbose_name=_('Price for GPU hour'),
max_digits=PRICE_MAX_DIGITS,
decimal_places=PRICE_DECIMAL_PLACES,
validators=[MinValueValidator(Decimal('0'))])
ram_price = models.DecimalField(default=0,
verbose_name=_('Price for GB RAM'),
max_digits=PRICE_MAX_DIGITS,
decimal_places=PRICE_DECIMAL_PLACES,
validators=[MinValueValidator(Decimal('0'))])
|
Handle single param definition (following Yahoo! changes) | # pipeurlbuilder.py
#
import urllib
from pipe2py import util
def pipe_urlbuilder(context, _INPUT, conf, **kwargs):
"""This source builds a url and yields it forever.
Keyword arguments:
context -- pipeline context
_INPUT -- not used
conf:
BASE -- base
PATH -- path elements
PARAM -- query parameters
Yields (_OUTPUT):
url
"""
for item in _INPUT:
#note: we could cache get_value results if item==True
url = util.get_value(conf['BASE'], item, **kwargs)
if not url.endswith('/'):
url += '/'
if 'PATH' in conf:
path = conf['PATH']
if not isinstance(path, list):
path = [path]
path = [util.get_value(p, item, **kwargs) for p in path if p]
url += "/".join(p for p in path if p)
url = url.rstrip("/")
#Ensure url is valid
url = util.url_quote(url)
param_defs = conf['PARAM']
if not isinstance(param_defs, list):
param_defs = [param_defs]
params = dict([(util.get_value(p['key'], item, **kwargs), util.get_value(p['value'], item, **kwargs)) for p in param_defs if p])
if params and params.keys() != [u'']:
url += "?" + urllib.urlencode(params)
yield url
| # pipeurlbuilder.py
#
import urllib
from pipe2py import util
def pipe_urlbuilder(context, _INPUT, conf, **kwargs):
"""This source builds a url and yields it forever.
Keyword arguments:
context -- pipeline context
_INPUT -- not used
conf:
BASE -- base
PATH -- path elements
PARAM -- query parameters
Yields (_OUTPUT):
url
"""
for item in _INPUT:
#note: we could cache get_value results if item==True
url = util.get_value(conf['BASE'], item, **kwargs)
if not url.endswith('/'):
url += '/'
if 'PATH' in conf:
path = conf['PATH']
if not isinstance(path, list):
path = [path]
path = [util.get_value(p, item, **kwargs) for p in path if p]
url += "/".join(p for p in path if p)
url = url.rstrip("/")
#Ensure url is valid
url = util.url_quote(url)
params = dict([(util.get_value(p['key'], item, **kwargs), util.get_value(p['value'], item, **kwargs)) for p in conf['PARAM'] if p])
if params and params.keys() != [u'']:
url += "?" + urllib.urlencode(params)
yield url
|
Add index and rename foreign key. | <?php
declare(strict_types=1);
return [
[
'table' => 'schema_generator_comment',
'columns' => [
'id' => ['type' => 'integer'],
'title' => ['type' => 'string', 'null' => true],
],
],
[
'table' => 'schema_generator',
'columns' => [
'id' => ['type' => 'integer'],
'relation_id' => ['type' => 'integer'],
'title' => ['type' => 'string', 'null' => true],
'body' => 'text',
],
'constraints' => [
'primary' => ['type' => 'primary', 'columns' => ['id']],
'relation_fk' => [
'type' => 'foreign',
'columns' => ['relation_id'],
'references' => ['schema_generator_comment', 'id'],
],
],
'indexes' => [
'relation_idx' => [
'type' => 'index',
'columns' => ['relation_id'],
],
'title_idx' => [
'type' => 'index',
'columns' => ['title'],
],
],
],
];
| <?php
declare(strict_types=1);
return [
[
'table' => 'schema_generator_comment',
'columns' => [
'id' => ['type' => 'integer'],
'title' => ['type' => 'string', 'null' => true],
],
],
[
'table' => 'schema_generator',
'columns' => [
'id' => ['type' => 'integer'],
'relation_id' => ['type' => 'integer', 'null' => true],
'title' => ['type' => 'string', 'null' => true],
'body' => 'text',
],
'constraints' => [
'primary' => ['type' => 'primary', 'columns' => ['id']],
'relation_idx' => [
'type' => 'foreign',
'columns' => ['relation_id'],
'references' => ['schema_generator_comment', 'id'],
],
],
'indexes' => [
'title_idx' => [
'type' => 'index',
'columns' => ['title'],
],
],
],
];
|
Implement hashCode() corresponding to the equals() implementation | package org.bouncycastle.crypto.params;
public class GOST3410ValidationParameters
{
private int x0;
private int c;
private long x0L;
private long cL;
public GOST3410ValidationParameters(
int x0,
int c)
{
this.x0 = x0;
this.c = c;
}
public GOST3410ValidationParameters(
long x0L,
long cL)
{
this.x0L = x0L;
this.cL = cL;
}
public int getC()
{
return c;
}
public int getX0()
{
return x0;
}
public long getCL()
{
return cL;
}
public long getX0L()
{
return x0L;
}
public boolean equals(
Object o)
{
if (!(o instanceof GOST3410ValidationParameters))
{
return false;
}
GOST3410ValidationParameters other = (GOST3410ValidationParameters)o;
if (other.c != this.c)
{
return false;
}
if (other.x0 != this.x0)
{
return false;
}
if (other.cL != this.cL)
{
return false;
}
if (other.x0L != this.x0L)
{
return false;
}
return true;
}
public int hashCode()
{
return x0 ^ c ^ (int) x0L ^ (int)(x0L >> 32) ^ (int) cL ^ (int)(cL >> 32);
}
}
| package org.bouncycastle.crypto.params;
public class GOST3410ValidationParameters
{
private int x0;
private int c;
private long x0L;
private long cL;
public GOST3410ValidationParameters(
int x0,
int c)
{
this.x0 = x0;
this.c = c;
}
public GOST3410ValidationParameters(
long x0L,
long cL)
{
this.x0L = x0L;
this.cL = cL;
}
public int getC()
{
return c;
}
public int getX0()
{
return x0;
}
public long getCL()
{
return cL;
}
public long getX0L()
{
return x0L;
}
public boolean equals(
Object o)
{
if (!(o instanceof GOST3410ValidationParameters))
{
return false;
}
GOST3410ValidationParameters other = (GOST3410ValidationParameters)o;
if (other.c != this.c)
{
return false;
}
if (other.x0 != this.x0)
{
return false;
}
if (other.cL != this.cL)
{
return false;
}
if (other.x0L != this.x0L)
{
return false;
}
return true;
}
}
|
Revert "cleaned up code a little"
This reverts commit 7ddff91c9736bcedb7c03bb036ade86776885079. | package com.lagopusempire.lagopuscommandsystem.parsing;
import java.util.ArrayList;
import java.util.List;
/**
*
* @author MrZoraman
*/
public abstract class SyntaxParserBase
{
private final String script;
private final StringBuilder builder = new StringBuilder();
private final List<String> elements = new ArrayList<>();
public SyntaxParserBase(String script)
{
this.script = script;
}
protected abstract void iterate(int index, char c);
protected void finished() { }
protected void flush()
{
if(builder.length() > 0)
{
elements.add(builder.toString());
builder.setLength(0);
}
}
protected void push(char c)
{
builder.append(c);
}
public String[] parse()
{
char[] chars = script.toCharArray();
int ii = 0;
for(ii = 0; ii < chars.length; ii++)
{
iterate(ii, chars[ii]);
}
finished();
flush();
return elementsToArray();
}
private String[] elementsToArray()
{
return elements.toArray(new String[elements.size()]);
}
}
| package com.lagopusempire.lagopuscommandsystem.parsing;
import java.util.ArrayList;
import java.util.List;
/**
*
* @author MrZoraman
*/
public abstract class SyntaxParserBase
{
private final String script;
private final StringBuilder builder = new StringBuilder();
private final List<String> elements = new ArrayList<>();
public SyntaxParserBase(String script)
{
this.script = script;
}
protected abstract void iterate(int index, char c);
protected void finished() { }
protected void flush()
{
if(builder.length() > 0)
{
elements.add(builder.toString());
builder.setLength(0);
}
}
protected void push(char c)
{
builder.append(c);
}
public String[] parse()
{
char[] chars = script.toCharArray();
for(int ii = 0; ii < chars.length; ii++)
{
iterate(ii, chars[ii]);
}
finished();
flush();
return elementsToArray();
}
private String[] elementsToArray()
{
return elements.toArray(new String[elements.size()]);
}
}
|
Fix scoper code not working on PHP < 8 | <?php
declare( strict_types=1 );
use Isolated\Symfony\Component\Finder\Finder;
$symfonyPolyfills = ( static function (): array {
$files = [];
foreach (
Finder::create()
->files()
->in( __DIR__ . '/vendor/symfony/polyfill-*' )
->name( 'bootstrap.php' )
->name( 'bootstrap80.php' ) as $bootstrap
) {
$files[] = $bootstrap->getPathName();
}
return $files;
} )();
return [
'prefix' => 'WPSentry\\ScopedVendor',
'finders' => [
Finder::create()
->files()
->ignoreVCS( true )
->notName( '/LICENSE|.*\\.md|.*\\.dist|Makefile|composer\\.json|composer\\.lock/' )
->exclude( [
'doc',
'test',
'test_old',
'tests',
'Tests',
'vendor-bin',
] )
->in( 'vendor' ),
Finder::create()->append( [
'composer.json',
] ),
],
'whitelist' => [
'Sentry\\*',
'Monolog\\*',
'Symfony\\Polyfill\\*',
],
'files-whitelist' => array_merge( [
'vendor/ralouphie/getallheaders/src/getallheaders.php',
], $symfonyPolyfills ),
'whitelist-global-classes' => true,
'whitelist-global-constants' => true,
'whitelist-global-functions' => true,
];
| <?php
declare( strict_types=1 );
use Isolated\Symfony\Component\Finder\Finder;
$polyfillsBootstrap = Finder::create()
->files()
->in( __DIR__ . '/vendor/symfony/polyfill-*' )
->name( 'bootstrap.php' )
->name( 'bootstrap80.php' );
return [
'prefix' => 'WPSentry\\ScopedVendor',
'finders' => [
Finder::create()
->files()
->ignoreVCS( true )
->notName( '/LICENSE|.*\\.md|.*\\.dist|Makefile|composer\\.json|composer\\.lock/' )
->exclude( [
'doc',
'test',
'test_old',
'tests',
'Tests',
'vendor-bin',
] )
->in( 'vendor' ),
Finder::create()->append( [
'composer.json',
] ),
],
'whitelist' => [
'Sentry\\*',
'Monolog\\*',
'Symfony\\Polyfill\\*',
],
'files-whitelist' => array_merge( [
'vendor/ralouphie/getallheaders/src/getallheaders.php',
], array_map(
static function ( $file ) {
return $file->getPathName();
},
iterator_to_array( $polyfillsBootstrap )
) ),
'whitelist-global-classes' => true,
'whitelist-global-constants' => true,
'whitelist-global-functions' => true,
];
|
Fix header comparison debug output | <?php
/**
* CakePHP(tm) : Rapid Development Framework (http://cakephp.org)
* Copyright (c) Cake Software Foundation, Inc. (http://cakefoundation.org)
*
* Licensed under The MIT License
* For full copyright and license information, please see the LICENSE.txt
* Redistributions of files must retain the above copyright notice
*
* @copyright Copyright (c) Cake Software Foundation, Inc. (http://cakefoundation.org)
* @since 3.7.0
* @license http://www.opensource.org/licenses/mit-license.php MIT License
*/
namespace Cake\TestSuite\Constraint\Response;
use Cake\Http\Response;
/**
* HeaderEquals
*
* @internal
*/
class HeaderEquals extends ResponseBase
{
/**
* @var string
*/
protected $headerName;
/**
* @var string|null
*/
protected $responseHeader;
/**
* Constructor.
*
* @param Response $response Response
* @param string $headerName Header name
*/
public function __construct($response, $headerName)
{
parent::__construct($response);
$this->headerName = $headerName;
$this->responseHeader = $this->response->getHeaderLine($this->headerName);
}
/**
* Checks assertion
*
* @param mixed $other Expected content
* @return bool
*/
public function matches($other)
{
return $this->responseHeader === $other;
}
/**
* Assertion message
*
* @return string
*/
public function toString()
{
return sprintf('equals content in header \'%s\': `%s`', $this->headerName, $this->responseHeader);
}
}
| <?php
/**
* CakePHP(tm) : Rapid Development Framework (http://cakephp.org)
* Copyright (c) Cake Software Foundation, Inc. (http://cakefoundation.org)
*
* Licensed under The MIT License
* For full copyright and license information, please see the LICENSE.txt
* Redistributions of files must retain the above copyright notice
*
* @copyright Copyright (c) Cake Software Foundation, Inc. (http://cakefoundation.org)
* @since 3.7.0
* @license http://www.opensource.org/licenses/mit-license.php MIT License
*/
namespace Cake\TestSuite\Constraint\Response;
use Cake\Http\Response;
/**
* HeaderEquals
*
* @internal
*/
class HeaderEquals extends ResponseBase
{
/**
* @var string
*/
protected $headerName;
/**
* Constructor.
*
* @param Response $response Response
* @param string $headerName Header name
*/
public function __construct($response, $headerName)
{
parent::__construct($response);
$this->headerName = $headerName;
}
/**
* Checks assertion
*
* @param mixed $other Expected content
* @return bool
*/
public function matches($other)
{
return $this->response->getHeaderLine($this->headerName) === $other;
}
/**
* Assertion message
*
* @return string
*/
public function toString()
{
return sprintf('equals content in header \'%s\'', $this->headerName);
}
}
|
Remove indentation level for easier review | """
Python introspection helpers.
"""
from types import CodeType as code, FunctionType as function
def copycode(template, changes):
if hasattr(code, "replace"):
return template.replace(**{"co_" + k : v for k, v in changes.items()})
names = [
"argcount", "nlocals", "stacksize", "flags", "code", "consts",
"names", "varnames", "filename", "name", "firstlineno", "lnotab",
"freevars", "cellvars"
]
if hasattr(code, "co_kwonlyargcount"):
names.insert(1, "kwonlyargcount")
if hasattr(code, "co_posonlyargcount"):
# PEP 570 added "positional only arguments"
names.insert(1, "posonlyargcount")
values = [
changes.get(name, getattr(template, "co_" + name))
for name in names
]
return code(*values)
def copyfunction(template, funcchanges, codechanges):
names = [
"globals", "name", "defaults", "closure",
]
values = [
funcchanges.get(name, getattr(template, "__" + name + "__"))
for name in names
]
return function(copycode(template.__code__, codechanges), *values)
def preserveName(f):
"""
Preserve the name of the given function on the decorated function.
"""
def decorator(decorated):
return copyfunction(decorated,
dict(name=f.__name__), dict(name=f.__name__))
return decorator
| """
Python introspection helpers.
"""
from types import CodeType as code, FunctionType as function
def copycode(template, changes):
if hasattr(code, "replace"):
return template.replace(**{"co_" + k : v for k, v in changes.items()})
else:
names = [
"argcount", "nlocals", "stacksize", "flags", "code", "consts",
"names", "varnames", "filename", "name", "firstlineno", "lnotab",
"freevars", "cellvars"
]
if hasattr(code, "co_kwonlyargcount"):
names.insert(1, "kwonlyargcount")
if hasattr(code, "co_posonlyargcount"):
# PEP 570 added "positional only arguments"
names.insert(1, "posonlyargcount")
values = [
changes.get(name, getattr(template, "co_" + name))
for name in names
]
return code(*values)
def copyfunction(template, funcchanges, codechanges):
names = [
"globals", "name", "defaults", "closure",
]
values = [
funcchanges.get(name, getattr(template, "__" + name + "__"))
for name in names
]
return function(copycode(template.__code__, codechanges), *values)
def preserveName(f):
"""
Preserve the name of the given function on the decorated function.
"""
def decorator(decorated):
return copyfunction(decorated,
dict(name=f.__name__), dict(name=f.__name__))
return decorator
|
Lib: Move things, clean up stuff... | /*
* tuxedo
* https://github.com/goliatone/tuxedo
* Created with gbase.
* Copyright (c) 2014 goliatone
* Licensed under the MIT license.
*/
/* jshint strict: false, plusplus: true */
/*global define: false, require: false, module: false, exports: false */
var Handlebars = require('handlebars'),
helpers = require('./helpers'),
momentHelper = require('handlebars-helper-moment')(),
faker = require('faker');
// Merge the built-in helpers with any that are passed in the options
var CoreHelpers = {};
Handlebars.Utils.extend(CoreHelpers, helpers);
Handlebars.Utils.extend(CoreHelpers, faker.Date);
Handlebars.Utils.extend(CoreHelpers, faker.Name);
Handlebars.Utils.extend(CoreHelpers, faker.Image);
Handlebars.Utils.extend(CoreHelpers, faker.Lorem);
Handlebars.Utils.extend(CoreHelpers, faker.Address);
Handlebars.Utils.extend(CoreHelpers, faker.Company);
Handlebars.Utils.extend(CoreHelpers, faker.Internet);
Handlebars.Utils.extend(CoreHelpers, faker.PhoneNumber);
Handlebars.Utils.extend(CoreHelpers, momentHelper);
module.exports = {
parse: function(string, options) {
options = options || {};
var mergeHelpers = {};
Handlebars.Utils.extend(mergeHelpers, CoreHelpers);
Handlebars.Utils.extend(mergeHelpers, options.helpers);
// Reset indexes on each parse
return Handlebars.compile(string)(options.data, {
helpers: mergeHelpers
});
}
}; | /*
* tuxedo
* https://github.com/goliatone/tuxedo
* Created with gbase.
* Copyright (c) 2014 goliatone
* Licensed under the MIT license.
*/
/* jshint strict: false, plusplus: true */
/*global define: false, require: false, module: false, exports: false */
var Handlebars = require('handlebars'),
helpers = require('./helpers'),
faker = require('faker');
module.exports = {
parse: function(string, options) {
options = options || {};
// Merge the built-in helpers with any that are passed in the options
var combinedHelpers = {};
Handlebars.Utils.extend(combinedHelpers, helpers);
Handlebars.Utils.extend(combinedHelpers, faker.Date);
Handlebars.Utils.extend(combinedHelpers, faker.Name);
Handlebars.Utils.extend(combinedHelpers, faker.Image);
Handlebars.Utils.extend(combinedHelpers, faker.Lorem);
Handlebars.Utils.extend(combinedHelpers, faker.Address);
Handlebars.Utils.extend(combinedHelpers, faker.Company);
Handlebars.Utils.extend(combinedHelpers, faker.Internet);
Handlebars.Utils.extend(combinedHelpers, faker.PhoneNumber);
Handlebars.Utils.extend(combinedHelpers, require('handlebars-helper-moment')());
Handlebars.Utils.extend(combinedHelpers, options.helpers);
// Reset indexes on each parse
return Handlebars.compile(string)(options.data, {
helpers: combinedHelpers
});
}
}; |
Change if statement to method call instead compare | <?php
namespace Furniture\UserBundle\Killer\Subscriber;
use Furniture\UserBundle\Killer\Killer;
use Symfony\Component\EventDispatcher\EventSubscriberInterface;
use Symfony\Component\HttpFoundation\RedirectResponse;
use Symfony\Component\HttpKernel\Event\GetResponseEvent;
use Symfony\Component\HttpKernel\HttpKernelInterface;
use Symfony\Component\HttpKernel\KernelEvents;
use Symfony\Component\Routing\Generator\UrlGeneratorInterface;
class CollectSessionSubscriber implements EventSubscriberInterface
{
/**
* @var Killer
*/
private $killer;
/**
* Construct
*
* @param Killer $killer
*/
public function __construct(Killer $killer)
{
$this->killer = $killer;
}
/**
* User add session to collection.
*
* @param GetResponseEvent $event
*/
public function collectSession(GetResponseEvent $event)
{
if (!$event->isMasterRequest()) {
return;
}
$request = $event->getRequest();
$session = $request->getSession();
$id = $session->getId();
$this->killer->collectionSessionId($id);
}
/**
* {@inheritDoc}
*/
public static function getSubscribedEvents()
{
return [
KernelEvents::REQUEST => [
['collectSession', 0],
],
];
}
}
| <?php
namespace Furniture\UserBundle\Killer\Subscriber;
use Furniture\UserBundle\Killer\Killer;
use Symfony\Component\EventDispatcher\EventSubscriberInterface;
use Symfony\Component\HttpFoundation\RedirectResponse;
use Symfony\Component\HttpKernel\Event\GetResponseEvent;
use Symfony\Component\HttpKernel\HttpKernelInterface;
use Symfony\Component\HttpKernel\KernelEvents;
use Symfony\Component\Routing\Generator\UrlGeneratorInterface;
class CollectSessionSubscriber implements EventSubscriberInterface
{
/**
* @var Killer
*/
private $killer;
/**
* Construct
*
* @param Killer $killer
*/
public function __construct(Killer $killer)
{
$this->killer = $killer;
}
/**
* Kill user if necessary
*
* @param GetResponseEvent $event
*/
public function collectSession(GetResponseEvent $event)
{
if ($event->getRequestType() !== HttpKernelInterface::MASTER_REQUEST) {
return;
}
$request = $event->getRequest();
$session = $request->getSession();
$id = $session->getId();
$this->killer->collectionSessionId($id);
}
/**
* {@inheritDoc}
*/
public static function getSubscribedEvents()
{
return [
KernelEvents::REQUEST => [
['collectSession', 0],
],
];
}
}
|
Update cancel old job message | from __future__ import unicode_literals, absolute_import
from django.core.management.base import BaseCommand
from ci import models, views, TimeUtils
from datetime import timedelta
class Command(BaseCommand):
help = 'Cancel old Civet jobs. When a specific civet client is no longer running, it can leave jobs lying around that other clients have to ignore.'
def add_arguments(self, parser):
parser.add_argument('--dryrun', default=False, action='store_true', help="Don't make any changes, just report what would have happened")
parser.add_argument('--days', required=True, type=int, help="Cancel jobs older than this many days")
def handle(self, *args, **options):
dryrun = options["dryrun"]
days = options["days"]
d = TimeUtils.get_local_time() - timedelta(days=days)
jobs = models.Job.objects.filter(active=True, ready=True, status=models.JobStatus.NOT_STARTED, created__lt=d)
count = jobs.count()
prefix = ""
if dryrun:
prefix = "DRY RUN: "
for job in jobs.all():
self.stdout.write("%sCancel job %s: %s: %s" % (prefix, job.pk, job, job.created))
if not dryrun:
views.set_job_canceled(job, "Canceled due to civet client not running this job in too long a time")
job.event.set_complete_if_done()
if count == 0:
self.stdout.write("No jobs to cancel")
| from __future__ import unicode_literals, absolute_import
from django.core.management.base import BaseCommand
from ci import models, views, TimeUtils
from datetime import timedelta
class Command(BaseCommand):
help = 'Cancel old Civet jobs. When a specific civet client is no longer running, it can leave jobs lying around that other clients have to ignore.'
def add_arguments(self, parser):
parser.add_argument('--dryrun', default=False, action='store_true', help="Don't make any changes, just report what would have happened")
parser.add_argument('--days', required=True, type=int, help="Cancel jobs older than this many days")
def handle(self, *args, **options):
dryrun = options["dryrun"]
days = options["days"]
d = TimeUtils.get_local_time() - timedelta(days=days)
jobs = models.Job.objects.filter(active=True, ready=True, status=models.JobStatus.NOT_STARTED, created__lt=d)
count = jobs.count()
prefix = ""
if dryrun:
prefix = "DRY RUN: "
for job in jobs.all():
self.stdout.write("%sCancel job %s: %s: %s" % (prefix, job.pk, job, job.created))
if not dryrun:
views.set_job_canceled(job, "Civet client hasn't run this job in too long a time")
job.event.set_complete_if_done()
if count == 0:
self.stdout.write("No jobs to cancel")
|
Make comment about time accuracy more universal | import time
from influxdb import InfluxDBClient
from influxdb import SeriesHelper
from . import config
# expects Cozify devices type json data
def getMultisensorData(data):
out = []
for device in data:
state=data[device]['state']
devtype = state['type']
if devtype == 'STATE_MULTI_SENSOR':
name=data[device]['name']
if 'lastSeen' in state:
timestamp=state['lastSeen']
else:
# if no time of measurement is known we must make a reasonable assumption
# Stored here in milliseconds to match accuracy of what the hub will give you
timestamp = time.time() * 1000
if 'temperature' in state:
temperature=state['temperature']
else:
temperature=None
if 'humidity' in state:
humidity=state['humidity']
else:
humidity=None
out.append({
'name': name,
'time': timestamp,
'temperature': temperature,
'humidity': humidity
})
return out
| import time
from influxdb import InfluxDBClient
from influxdb import SeriesHelper
from . import config
# expects Cozify devices type json data
def getMultisensorData(data):
out = []
for device in data:
state=data[device]['state']
devtype = state['type']
if devtype == 'STATE_MULTI_SENSOR':
name=data[device]['name']
if 'lastSeen' in state:
timestamp=state['lastSeen']
else:
# if no time of measurement is known we must make a reasonable assumption
# Stored here in milliseconds, influxDB internally stores as nanoseconds
timestamp = time.time() * 1000
if 'temperature' in state:
temperature=state['temperature']
else:
temperature=None
if 'humidity' in state:
humidity=state['humidity']
else:
humidity=None
out.append({
'name': name,
'time': timestamp,
'temperature': temperature,
'humidity': humidity
})
return out
|
Add annotations about expected outcomes | package sfBugsNew;
import edu.umd.cs.findbugs.annotations.Confidence;
import edu.umd.cs.findbugs.annotations.DesireNoWarning;
import edu.umd.cs.findbugs.annotations.NoWarning;
public class Bug1242 {
public static class Child extends Parent<Something> {
@Override
@DesireNoWarning("BC_UNCONFIRMED_CAST_OF_RETURN_VALUE")
@NoWarning(value="BC_UNCONFIRMED_CAST_OF_RETURN_VALUE", confidence=Confidence.MEDIUM)
public Something getDuplicateValue() {
Something value = super.getDuplicateValue();
System.err.println("getValue() called.");
return value;
}
}
public static interface Duplicatable<T extends Duplicatable<T>> extends Cloneable {
T getDuplicate();
}
public static class Interim<T extends Interim<T>> implements Duplicatable<T> {
@Override
public T getDuplicate() {
try {
return (T) clone();
} catch (CloneNotSupportedException cnse) {
return null;
}
}
}
// Alternative that produces no bug warnings:
// public class Parent<T extends Duplicatable<T>>
public static class Parent<T extends Interim<T>> {
private T value;
public void setValue(T newValue) {
value = newValue;
}
public T getDuplicateValue() {
if (value == null) {
return null;
}
T duplicate = value.getDuplicate();
return duplicate;
}
}
public static class Something extends Interim<Something> {
public void doStuff() {
System.err.println("Hello");
}
}
}
| package sfBugsNew;
import java.io.Serializable;
public class Bug1242 {
public static class Child extends Parent<Something>
{
@Override
public Something getDuplicateValue()
{
Something value = super.getDuplicateValue();
System.err.println("getValue() called.");
return value;
}
}
public static interface Duplicatable<T extends Duplicatable<T>> extends Cloneable
{
T getDuplicate();
}
public static class Interim<T extends Interim<T>> implements Duplicatable<T>
{
@Override
public T getDuplicate()
{
try
{
return (T) clone();
}
catch (CloneNotSupportedException cnse)
{
return null;
}
}
}
// Alternative that produces no bug warnings:
//public class Parent<T extends Duplicatable<T>>
public static class Parent<T extends Interim<T>>
{
private T value;
public void setValue(T newValue)
{
value = newValue;
}
public T getDuplicateValue()
{
if (value == null)
{
return null;
}
T duplicate = value.getDuplicate();
return duplicate;
}
}
public static class Something extends Interim<Something>
{
public void doStuff()
{
System.err.println("Hello");
}
}
}
|
Prepare for Yii2 2.0 RC | <?php
namespace gilek\gtreetable;
use yii\web\AssetBundle;
class GTreeTableAsset extends AssetBundle {
/**
* @inheritdoc
*/
public $sourcePath = '@gilek/gtreetable/gtreetable';
/**
* @inheritdoc
*/
public $jsOptions = [
'position' => \yii\web\View::POS_HEAD
];
/**
* @inheritdoc
*/
public $depends = [
'yii\web\JqueryAsset',
'yii\jui\JuiAsset',
];
public $publishOptions = [
'forceCopy' => YII_ENV_DEV
];
public $language;
public $draggable = false;
public $minSuffix = '.min';
public function registerAssetFiles($view)
{
if ($this->draggable === true) {
$this->js[] = 'jquery.browser.js';
}
$this->js[] = 'bootstrap-gtreetable'.(YII_ENV_DEV ? '' : $this->minSuffix).'.js';
$this->css[] = 'gtreetable'.(YII_ENV_DEV ? '' : $this->minSuffix).'.css';
if ($this->language !== null) {
$this->js[] = 'languages/bootstrap-gtreetable.'.$this->language. (YII_ENV_DEV ? '' : '.'.$this->minSuffix). '.js';
}
parent::registerAssetFiles($view);
}
}
| <?php
namespace gilek\gtreetable;
use yii\web\AssetBundle;
class GTreeTableAsset extends AssetBundle {
/**
* @inheritdoc
*/
public $sourcePath = '@gilek/gtreetable/gtreetable';
/**
* @inheritdoc
*/
public $jsOptions = [
'position' => \yii\web\View::POS_HEAD
];
/**
* @inheritdoc
*/
public $depends = [
'yii\web\JqueryAsset',
'yii\jui\JuiAsset.php',
];
public $publishOptions = [
'forceCopy' => YII_ENV_DEV
];
public $language;
public $draggable = false;
public $minSuffix = '.min';
public function registerAssetFiles($view)
{
if ($this->draggable === true) {
$this->js[] = 'jquery.browser.js';
}
$this->js[] = 'bootstrap-gtreetable'.(YII_ENV_DEV ? '' : $this->minSuffix).'.js';
$this->css[] = 'gtreetable'.(YII_ENV_DEV ? '' : $this->minSuffix).'.css';
if ($this->language !== null) {
$this->js[] = 'languages/bootstrap-gtreetable.'.$this->language. (YII_ENV_DEV ? '' : '.'.$this->minSuffix). '.js';
}
parent::registerAssetFiles($view);
}
}
|
Access the shiro username (aka "principal") from the vaadin UI. | package no.priv.bang.ukelonn.impl;
import org.apache.shiro.SecurityUtils;
import org.apache.shiro.subject.Subject;
import com.vaadin.server.VaadinRequest;
import com.vaadin.ui.Button;
import com.vaadin.ui.Button.ClickEvent;
import com.vaadin.ui.Label;
import com.vaadin.ui.Notification;
import com.vaadin.ui.UI;
import com.vaadin.ui.VerticalLayout;
public class UkelonnUI extends UI {
private static final long serialVersionUID = 1388525490129647161L;
@Override
protected void init(VaadinRequest request) {
Subject currentUser = SecurityUtils.getSubject();
// Create the content root layout for the UI
VerticalLayout content = new VerticalLayout();
setContent(content);
// Display the greeting
content.addComponent(new Label("Hello " + currentUser.getPrincipal()));
// Have a clickable button
content.addComponent(new Button("Push Me!",
new Button.ClickListener() {
private static final long serialVersionUID = 2723190031041985566L;
@Override
public void buttonClick(ClickEvent e) {
Notification.show("Pushed!");
}
}));
}
}
| package no.priv.bang.ukelonn.impl;
import com.vaadin.server.VaadinRequest;
import com.vaadin.ui.Button;
import com.vaadin.ui.Button.ClickEvent;
import com.vaadin.ui.Label;
import com.vaadin.ui.Notification;
import com.vaadin.ui.UI;
import com.vaadin.ui.VerticalLayout;
public class UkelonnUI extends UI {
private static final long serialVersionUID = 1388525490129647161L;
@Override
protected void init(VaadinRequest request) {
// Create the content root layout for the UI
VerticalLayout content = new VerticalLayout();
setContent(content);
// Display the greeting
content.addComponent(new Label("Hello World!"));
// Have a clickable button
content.addComponent(new Button("Push Me!",
new Button.ClickListener() {
private static final long serialVersionUID = 2723190031041985566L;
@Override
public void buttonClick(ClickEvent e) {
Notification.show("Pushed!");
}
}));
}
}
|
Use production mode for stockflux components build | const path = require('path');
module.exports = {
mode: 'production',
entry: path.resolve('src', 'index.js'),
output: {
filename: 'index.js',
path: path.resolve(__dirname, 'dist'),
library: 'stockflux-components',
libraryTarget: 'umd'
},
module: {
rules: [
{
test: /\.jsx?$/,
include: [
path.resolve(__dirname, 'src')
],
enforce: 'pre',
use: [
{ loader: 'eslint-loader' }
],
},
{
test: /\.jsx?$/,
include: [
path.resolve(__dirname, 'src')
],
use: [
{ loader: 'babel-loader' }
]
},
{
test: /\.css$/,
include: [
path.resolve(__dirname, 'src')
],
use: [
{ loader: 'style-loader' },
{ loader: 'css-loader' }
]
},
{
test: /\.png$/,
include: [
path.resolve(__dirname, 'src')
],
use: [
{ loader: 'url-loader' }
]
}
],
},
externals: {
react: 'react',
'openfin-react-hooks': 'openfin-react-hooks',
'openfin-layouts': 'openfin-layouts',
}
};
| const path = require('path');
module.exports = {
mode: 'development', // TODO: production mode
entry: path.resolve('src', 'index.js'),
output: {
filename: 'index.js',
path: path.resolve(__dirname, 'dist'),
library: 'stockflux-components',
libraryTarget: 'umd'
},
module: {
rules: [
{
test: /\.jsx?$/,
include: [
path.resolve(__dirname, 'src')
],
enforce: 'pre',
use: [
{ loader: 'eslint-loader' }
],
},
{
test: /\.jsx?$/,
include: [
path.resolve(__dirname, 'src')
],
use: [
{ loader: 'babel-loader' }
]
},
{
test: /\.css$/,
include: [
path.resolve(__dirname, 'src')
],
use: [
{ loader: 'style-loader' },
{ loader: 'css-loader' }
]
},
{
test: /\.png$/,
include: [
path.resolve(__dirname, 'src')
],
use: [
{ loader: 'url-loader' }
]
}
],
},
externals: {
react: 'react',
'openfin-react-hooks': 'openfin-react-hooks',
'openfin-layouts': 'openfin-layouts',
}
};
|
[api] Allow for `construct` to be a function. | var assert = require('assert');
module.exports = function (options) {
options = options || {};
var Transport = options.Transport;
var name = Transport.name || options.name;
var construct = options.construct;
var instance;
beforeEach(function () {
var create = typeof construct === 'function'
? construct()
: construct;
instance = new Transport(create);
});
// TODO How to assert that error event is thrown? How to make a transport fail?
describe('.log()', function () {
it('should be present', function () {
assert.ok(instance.log);
assert.equal('function', typeof instance.log);
});
it('(with no callback) should return true', function () {
var info = {
level: 'debug',
message: 'foo'
};
info.raw = JSON.stringify(info);
var result = instance.log(info);
assert(true, result);
});
it('(with callback) should return true', function (done) {
var info = {
level: 'debug',
message: 'foo'
};
info.raw = JSON.stringify(info);
var result = instance.log(info, function () {
assert(true, result);
done();
});
});
});
describe('events', function () {
it('should emit the "logged" event', function (done) {
instance.once('logged', done);
var info = {
level: 'debug',
message: 'foo'
};
info.raw = JSON.stringify(info);
instance.log(info);
});
});
};
| var assert = require('assert');
module.exports = function (options) {
options = options || {};
var Transport = options.Transport;
var name = Transport.name || options.name;
var construct = options.construct;
var instance;
beforeEach(function () {
instance = new Transport(construct);
});
// TODO How to assert that error event is thrown? How to make a transport fail?
describe('.log()', function () {
it('should be present', function () {
assert.ok(instance.log);
assert.equal('function', typeof instance.log);
});
it('(with no callback) should return true', function () {
var info = {
level: 'debug',
message: 'foo'
};
info.raw = JSON.stringify(info);
var result = instance.log(info);
assert(true, result);
});
it('(with callback) should return true', function (done) {
var info = {
level: 'debug',
message: 'foo'
};
info.raw = JSON.stringify(info);
var result = instance.log(info, function () {
assert(true, result);
done();
});
});
});
describe('events', function () {
it('should emit the "logged" event', function (done) {
instance.once('logged', done);
var info = {
level: 'debug',
message: 'foo'
};
info.raw = JSON.stringify(info);
instance.log(info);
});
});
};
|
Remove call to method MemberId::any. | <?php
/**
* PHP version 5.6
*
* This source file is subject to the license that is bundled with this package in the file LICENSE.
*/
namespace Hexagonal\DomainEvents;
use Ewallet\DataBuilders\A;
use DateTime;
use Ewallet\Accounts\MemberId;
use Hexagonal\Fakes\DomainEvents\InstantaneousEvent;
use Hexagonal\JmsSerializer\JsonSerializer;
use Mockery;
use Money\Money;
use PHPUnit_Framework_TestCase as TestCase;
class PersistEventsSubscriberTest extends TestCase
{
/** @test */
function it_should_subscribe_to_all_event_types()
{
$subscriber = new PersistEventsSubscriber(
Mockery::mock(EventStore::class),
Mockery::mock(StoredEventFactory::class)
);
$this->assertTrue($subscriber->isSubscribedTo(new InstantaneousEvent(
MemberId::with('any'), Money::MXN(100000), new DateTime('now')
)));
$this->assertTrue($subscriber->isSubscribedTo(
A::transferWasMadeEvent()->build())
);
}
/** @test */
function it_should_persist_an_event()
{
$store = Mockery::mock(EventStore::class);
$subscriber = new PersistEventsSubscriber(
$store,
new StoredEventFactory(new JsonSerializer())
);
$store
->shouldReceive('append')
->once()
->with(Mockery::type(StoredEvent::class))
;
$subscriber->handle(A::transferWasMadeEvent()->build());
}
}
| <?php
/**
* PHP version 5.6
*
* This source file is subject to the license that is bundled with this package in the file LICENSE.
*/
namespace Hexagonal\DomainEvents;
use Ewallet\DataBuilders\A;
use DateTime;
use Ewallet\Accounts\MemberId;
use Hexagonal\Fakes\DomainEvents\InstantaneousEvent;
use Hexagonal\JmsSerializer\JsonSerializer;
use Mockery;
use Money\Money;
use PHPUnit_Framework_TestCase as TestCase;
class PersistEventsSubscriberTest extends TestCase
{
/** @test */
function it_should_subscribe_to_all_event_types()
{
$subscriber = new PersistEventsSubscriber(
Mockery::mock(EventStore::class),
Mockery::mock(StoredEventFactory::class)
);
$this->assertTrue($subscriber->isSubscribedTo(new InstantaneousEvent(
MemberId::any(), Money::MXN(100000), new DateTime('now')
)));
$this->assertTrue($subscriber->isSubscribedTo(
A::transferWasMadeEvent()->build())
);
}
/** @test */
function it_should_persist_an_event()
{
$store = Mockery::mock(EventStore::class);
$subscriber = new PersistEventsSubscriber(
$store,
new StoredEventFactory(new JsonSerializer())
);
$store
->shouldReceive('append')
->once()
->with(Mockery::type(StoredEvent::class))
;
$subscriber->handle(A::transferWasMadeEvent()->build());
}
}
|
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.