Spaces:
Sleeping
Sleeping
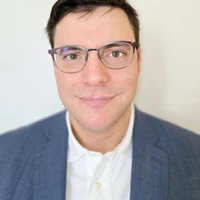
code refactoring, added logic to allow users to save their details in the browser local storage
54af9e3
import abc | |
import json | |
from streamlit_local_storage import LocalStorage | |
ls: LocalStorage = LocalStorage() | |
class SavedDetails(abc.ABC): | |
def __init__(self, type_: str): | |
self.type_ = type_ | |
def save_to_local_storage(self, key: str): | |
ls.setItem(key, json.dumps(self.__dict__)) | |
def load(cls, json_data: str): | |
data = json.loads(json_data) | |
type_ = data.get("type_") | |
if not type_ or type_ != cls.type_: | |
raise ValueError(f"the expected type is {cls.type_} but is actually {type_}") | |
return cls.__init__(**{k: v for k, v in data if k != "type"}) | |
class PersonalDetails(SavedDetails): | |
type_ = "personal_details" | |
def __init__(self, full_name, email, contact_number): | |
super().__init__(self.type_) | |
self.full_name = full_name | |
self.email = email | |
self.contact_number = contact_number | |
class LocationDetails(SavedDetails): | |
type_ = "location_details" | |
def __init__(self, owner_or_tenant: str, community: str, building: str, unit_number: str): | |
super().__init__(self.type_) | |
self.owner_or_tenant = owner_or_tenant | |
self.community = community | |
self.building = building | |
self.unit_number = unit_number | |
class ContractorDetails(SavedDetails): | |
type_ = "contractor_details" | |
def __init__(self, contractor_name:str, contractor_contact_number:str, contractor_email:str): | |
super().__init__(self.type_) | |
self.contractor_name = contractor_name | |
self.contractor_contact_number = contractor_contact_number | |
self.contractor_email = contractor_email | |