Update README.md
Browse files
README.md
CHANGED
@@ -12,31 +12,24 @@ widget:
|
|
12 |
example_title: Airport
|
13 |
---
|
14 |
|
15 |
-
|
16 |
# The Illustrated Image Captioning using transformers
|
17 |
|
18 |
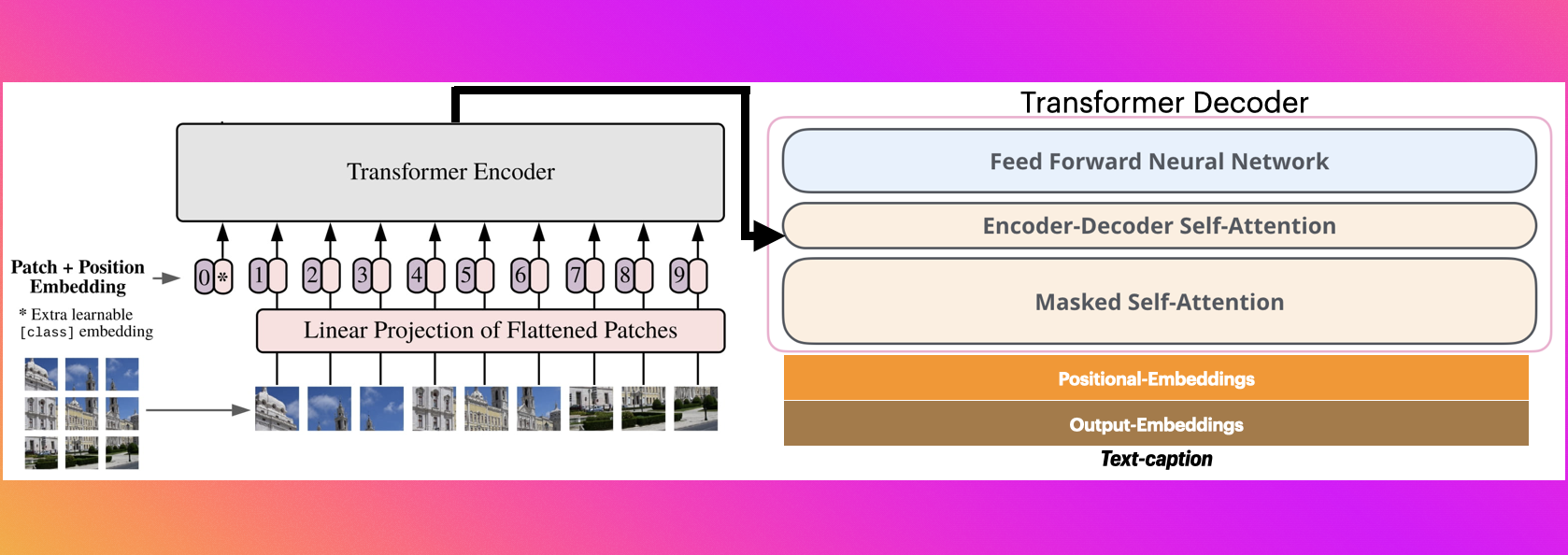
|
19 |
|
20 |
-
|
21 |
|
22 |
|
23 |
# Sample running code
|
24 |
|
25 |
```python
|
26 |
-
|
27 |
from transformers import VisionEncoderDecoderModel, ViTImageProcessor, AutoTokenizer
|
28 |
import torch
|
29 |
from PIL import Image
|
30 |
-
|
31 |
model = VisionEncoderDecoderModel.from_pretrained("nlpconnect/vit-gpt2-image-captioning")
|
32 |
feature_extractor = ViTImageProcessor.from_pretrained("nlpconnect/vit-gpt2-image-captioning")
|
33 |
tokenizer = AutoTokenizer.from_pretrained("nlpconnect/vit-gpt2-image-captioning")
|
34 |
-
|
35 |
device = torch.device("cuda" if torch.cuda.is_available() else "cpu")
|
36 |
model.to(device)
|
37 |
-
|
38 |
-
|
39 |
-
|
40 |
max_length = 16
|
41 |
num_beams = 4
|
42 |
gen_kwargs = {"max_length": max_length, "num_beams": num_beams}
|
@@ -46,36 +39,21 @@ def predict_step(image_paths):
|
|
46 |
i_image = Image.open(image_path)
|
47 |
if i_image.mode != "RGB":
|
48 |
i_image = i_image.convert(mode="RGB")
|
49 |
-
|
50 |
images.append(i_image)
|
51 |
-
|
52 |
pixel_values = feature_extractor(images=images, return_tensors="pt").pixel_values
|
53 |
pixel_values = pixel_values.to(device)
|
54 |
-
|
55 |
output_ids = model.generate(pixel_values, **gen_kwargs)
|
56 |
-
|
57 |
preds = tokenizer.batch_decode(output_ids, skip_special_tokens=True)
|
58 |
preds = [pred.strip() for pred in preds]
|
59 |
return preds
|
60 |
-
|
61 |
-
|
62 |
predict_step(['doctor.e16ba4e4.jpg']) # ['a woman in a hospital bed with a woman in a hospital bed']
|
63 |
-
|
64 |
```
|
65 |
|
66 |
# Sample running code using transformers pipeline
|
67 |
|
68 |
```python
|
69 |
-
|
70 |
from transformers import pipeline
|
71 |
-
|
72 |
image_to_text = pipeline("image-to-text", model="nlpconnect/vit-gpt2-image-captioning")
|
73 |
-
|
74 |
image_to_text("https://ankur3107.github.io/assets/images/image-captioning-example.png")
|
75 |
-
|
76 |
# [{'generated_text': 'a soccer game with a player jumping to catch the ball '}]
|
77 |
-
|
78 |
-
|
79 |
```
|
80 |
-
|
81 |
-
|
|
|
12 |
example_title: Airport
|
13 |
---
|
14 |
|
|
|
15 |
# The Illustrated Image Captioning using transformers
|
16 |
|
17 |
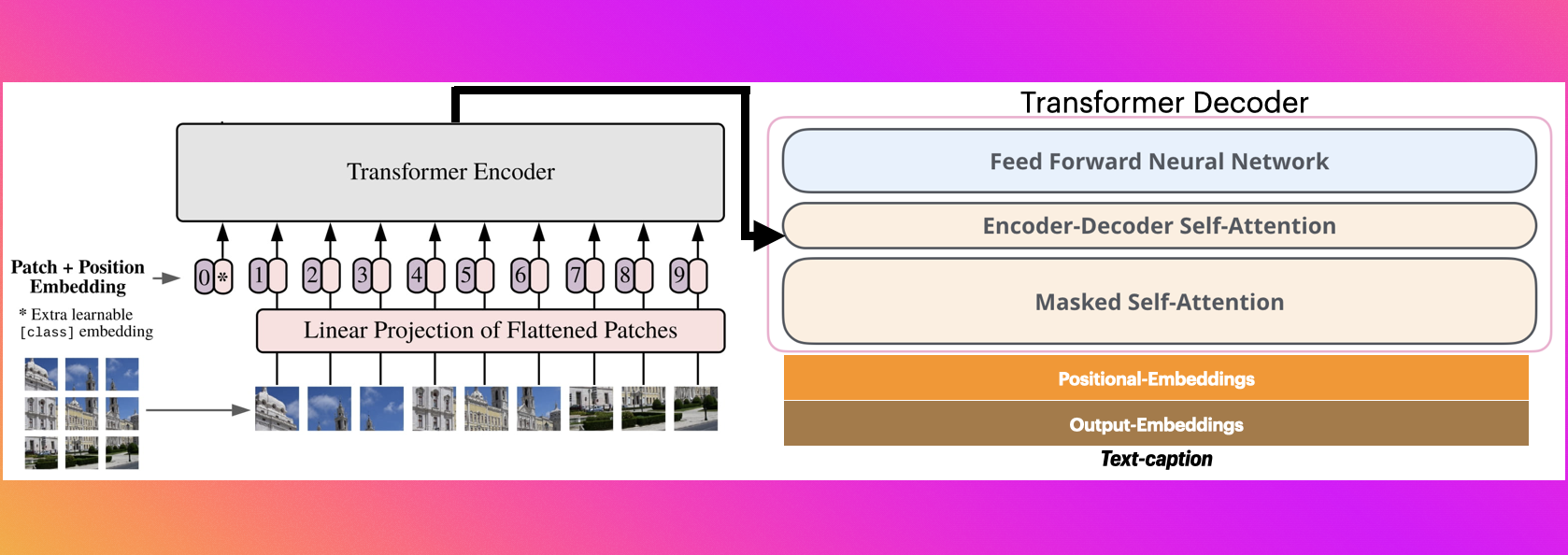
|
18 |
|
19 |
+
* https://ankur3107.github.io/blogs/the-illustrated-image-captioning-using-transformers/
|
20 |
|
21 |
|
22 |
# Sample running code
|
23 |
|
24 |
```python
|
|
|
25 |
from transformers import VisionEncoderDecoderModel, ViTImageProcessor, AutoTokenizer
|
26 |
import torch
|
27 |
from PIL import Image
|
|
|
28 |
model = VisionEncoderDecoderModel.from_pretrained("nlpconnect/vit-gpt2-image-captioning")
|
29 |
feature_extractor = ViTImageProcessor.from_pretrained("nlpconnect/vit-gpt2-image-captioning")
|
30 |
tokenizer = AutoTokenizer.from_pretrained("nlpconnect/vit-gpt2-image-captioning")
|
|
|
31 |
device = torch.device("cuda" if torch.cuda.is_available() else "cpu")
|
32 |
model.to(device)
|
|
|
|
|
|
|
33 |
max_length = 16
|
34 |
num_beams = 4
|
35 |
gen_kwargs = {"max_length": max_length, "num_beams": num_beams}
|
|
|
39 |
i_image = Image.open(image_path)
|
40 |
if i_image.mode != "RGB":
|
41 |
i_image = i_image.convert(mode="RGB")
|
|
|
42 |
images.append(i_image)
|
|
|
43 |
pixel_values = feature_extractor(images=images, return_tensors="pt").pixel_values
|
44 |
pixel_values = pixel_values.to(device)
|
|
|
45 |
output_ids = model.generate(pixel_values, **gen_kwargs)
|
|
|
46 |
preds = tokenizer.batch_decode(output_ids, skip_special_tokens=True)
|
47 |
preds = [pred.strip() for pred in preds]
|
48 |
return preds
|
|
|
|
|
49 |
predict_step(['doctor.e16ba4e4.jpg']) # ['a woman in a hospital bed with a woman in a hospital bed']
|
|
|
50 |
```
|
51 |
|
52 |
# Sample running code using transformers pipeline
|
53 |
|
54 |
```python
|
|
|
55 |
from transformers import pipeline
|
|
|
56 |
image_to_text = pipeline("image-to-text", model="nlpconnect/vit-gpt2-image-captioning")
|
|
|
57 |
image_to_text("https://ankur3107.github.io/assets/images/image-captioning-example.png")
|
|
|
58 |
# [{'generated_text': 'a soccer game with a player jumping to catch the ball '}]
|
|
|
|
|
59 |
```
|
|
|
|