path
stringlengths 7
265
| concatenated_notebook
stringlengths 46
17M
|
---|---|
examples/toy/distribution-german-credit.ipynb | ###Markdown
Fitting a logistic model to German credit data This notebook explains how to run the toy logistic regression model example using the German credit data from [1]. In this example, we have predictors for 1000 individuals and an outcome variable indicating whether or not each individual should be given credit.[1] "UCI machine learning repository", 2010. A. Frank and A. Asuncion. https://archive.ics.uci.edu/ml/datasets/statlog+(german+credit+data)
###Code
import pints
import pints.toy
import pints.plot
import numpy as np
import matplotlib.pyplot as plt
import io
import urllib
from scipy import stats
import time
###Output
_____no_output_____
###Markdown
To run this example, we need to first get the data from [1] and process it so we have dichtonomous $y\in\{-1,1\}$ outputs and the matrix of predictors has been standardised. In addition, we also add a column of 1s corresponding to a constant term in the regression.If you are connected to the internet, by instantiating with `x=None`, Pints will fetch the data from the repo for you. If, instead, you have local copies of the `x` and `y` matrices, these can be supplied as arguments.
###Code
logpdf = pints.toy.GermanCreditLogPDF(download=True)
###Output
_____no_output_____
###Markdown
Let's look at the data: `x` is a matrix of predictors and `y` is a vector of credit recommendations for 1000 individuals. Specifically, let's look at the PCA scores and plot the first two dimensions against one another. Here, we see that the two groups overlap substantially, but that there neverless some separation along the first PCA component.
###Code
def pca(X):
# Data matrix X, assumes 0-centered
n, m = X.shape
# Compute covariance matrix
C = np.dot(X.T, X) / (n-1)
# Eigen decomposition
eigen_vals, eigen_vecs = np.linalg.eig(C)
# Project X onto PC space
X_pca = np.dot(X, eigen_vecs)
return X_pca
x, y = logpdf.data()
scores = pca(x)
# colour individual points by whether or not to recommend them credit
plt.scatter(scores[:, 0], scores[:, 1], c=y)
plt.xlabel("PCA 1")
plt.ylabel("PCA 2")
plt.show()
###Output
_____no_output_____
###Markdown
Now we run HMC to fit the parameters of the model.
###Code
xs = [
np.random.uniform(0, 1, size=(logpdf.n_parameters())),
np.random.uniform(0, 1, size=(logpdf.n_parameters())),
np.random.uniform(0, 1, size=(logpdf.n_parameters())),
]
mcmc = pints.MCMCController(logpdf, len(xs), xs, method=pints.HamiltonianMCMC)
mcmc.set_max_iterations(200)
# Set up modest logging
mcmc.set_log_to_screen(True)
mcmc.set_log_interval(10)
for sampler in mcmc.samplers():
sampler.set_leapfrog_step_size(0.2)
sampler.set_leapfrog_steps(10)
start = time.time()
# Run!
print('Running...')
chains = mcmc.run()
print('Done!')
end = time.time()
diff = end - start
###Output
Running...
Using Hamiltonian Monte Carlo
Generating 3 chains.
Running in sequential mode.
Iter. Eval. Accept. Accept. Accept. Time m:s
0 3 0 0 0 0:00.0
1 33 0.333 0.333 0.333 0:00.3
2 63 0.5 0.5 0.5 0:00.6
3 93 0.6 0.6 0.6 0:00.9
10 303 0.833 0.833 0.833 0:02.8
20 603 0.909 0.909 0.909 0:05.6
30 903 0.9375 0.9375 0.9375 0:08.4
40 1203 0.952381 0.952381 0.952381 0:11.2
50 1503 0.962 0.942 0.962 0:14.1
60 1803 0.952 0.952 0.968 0:17.1
70 2103 0.958 0.958 0.972 0:20.2
80 2403 0.963 0.963 0.963 0:23.4
90 2703 0.967 0.957 0.957 0:26.5
100 3003 0.971 0.961 0.961 0:29.5
110 3303 0.955 0.964 0.964 0:32.4
120 3603 0.959 0.967 0.959 0:35.4
130 3903 0.962 0.969697 0.962 0:38.4
140 4203 0.951 0.971831 0.965 0:41.4
150 4503 0.954 0.974 0.967 0:44.4
160 4803 0.957 0.969 0.969 0:47.4
170 5103 0.959 0.971 0.971 0:50.3
180 5403 0.962 0.973 0.973 0:53.3
190 5703 0.964 0.974 0.974 0:56.2
200 5973 0.965 0.97 0.975 0:58.9
Halting: Maximum number of iterations (200) reached.
Done!
###Markdown
HMC is quite efficient here at sampling from the posterior distribution.
###Code
results = pints.MCMCSummary(chains=chains, time=diff)
print(results)
###Output
param mean std. 2.5% 25% 50% 75% 97.5% rhat ess ess per sec.
-------- ------ ------ ------ ----- ----- ----- ------- ------ ------ --------------
param 1 -1.24 0.27 -1.60 -1.33 -1.22 -1.13 -0.99 1.00 200.00 3.40
param 2 -0.76 0.20 -1.11 -0.84 -0.75 -0.67 -0.52 1.00 200.00 3.40
param 3 0.43 0.13 0.19 0.34 0.42 0.51 0.69 1.00 187.64 3.19
param 4 -0.43 0.19 -0.75 -0.51 -0.41 -0.33 -0.17 1.00 200.00 3.40
param 5 0.14 0.13 -0.09 0.06 0.13 0.23 0.40 1.01 200.00 3.40
param 6 -0.38 0.15 -0.66 -0.45 -0.38 -0.29 -0.17 1.00 200.00 3.40
param 7 -0.18 0.17 -0.53 -0.27 -0.18 -0.09 0.11 1.00 200.00 3.40
param 8 -0.16 0.12 -0.38 -0.21 -0.15 -0.09 0.08 1.00 200.00 3.40
param 9 0.01 0.15 -0.26 -0.07 0.02 0.10 0.30 1.00 200.00 3.40
param 10 0.20 0.13 -0.02 0.11 0.19 0.26 0.45 1.00 188.63 3.20
param 11 -0.11 0.17 -0.45 -0.21 -0.10 -0.01 0.22 1.00 200.00 3.40
param 12 -0.23 0.12 -0.44 -0.29 -0.23 -0.16 -0.03 1.00 200.00 3.40
param 13 0.13 0.17 -0.22 0.03 0.12 0.22 0.46 1.00 200.00 3.40
param 14 0.03 0.12 -0.24 -0.04 0.03 0.10 0.24 1.00 200.00 3.40
param 15 -0.14 0.12 -0.37 -0.21 -0.14 -0.07 0.08 1.00 200.00 3.40
param 16 -0.30 0.14 -0.58 -0.38 -0.30 -0.22 -0.09 1.00 182.69 3.10
param 17 0.28 0.11 0.07 0.22 0.28 0.34 0.51 1.00 200.00 3.40
param 18 -0.32 0.13 -0.56 -0.39 -0.31 -0.24 -0.10 1.00 200.00 3.40
param 19 0.32 0.15 0.03 0.21 0.32 0.43 0.60 1.00 169.26 2.88
param 20 0.28 0.15 -0.00 0.17 0.28 0.38 0.58 1.00 176.27 2.99
param 21 0.15 0.17 -0.15 0.04 0.13 0.24 0.49 1.00 143.76 2.44
param 22 -0.04 0.17 -0.35 -0.16 -0.05 0.05 0.31 1.00 142.75 2.43
param 23 -0.10 0.11 -0.32 -0.16 -0.09 -0.03 0.10 1.00 200.00 3.40
param 24 -0.02 0.14 -0.26 -0.11 -0.03 0.07 0.30 1.00 143.95 2.45
param 25 -0.01 0.15 -0.28 -0.12 -0.02 0.08 0.29 1.00 148.81 2.53
|
Paper Graphics.ipynb | ###Markdown
Paper Graphics
###Code
# version
import datetime
print(datetime.date.today())
%matplotlib notebook
run plots.py
prec = 500
# prepath where to save the figures
savepath = "./figs/"
# savepath = "./"
###Output
_____no_output_____
###Markdown
Policy Classification: opt, sus, safe
###Code
δ = 1.0
ρ = 0.2
γ = 1.0
rh = 1.0
rl = 0.5
#rmin = 0.2
rmin = 0.3
#rl = 0.4
#rmin = 0.3
xaxis = "δ"
yaxis = "γ"
fig, ax = plt.subplots(1, 3, sharey='row', sharex="col", figsize=(10, 4) )
bott=0.24
topp=0.9
leftt=0.06
rightt=0.98
smallfontsize = 8.5
# colors
ov = 0.6
risky_opt_color = (1.0, ov, 0)
cautious_opt_color = (1.0, 0, ov)
both_sus_color = (ov, ov, 1.0)
cautious_sus_color = (0.0, ov, 1.0)
cautious_safe_color = (0.0, 1.0, ov)
plot_optimal_policies(δ, ρ, γ, rh, rl, xaxis=xaxis, yaxis=yaxis, prec=prec,
riskyoptcolor=risky_opt_color,
cautiousoptcolor=cautious_opt_color,
ax=ax[0])
plot_sustainble_policies(δ, ρ, γ, rh, rl, rmin, xaxis=xaxis, yaxis=yaxis, prec=prec,
bothsuscolor=both_sus_color,
cautioussuscolor=cautious_sus_color,
ax=ax[1])
plot_SOS_policies(δ, ρ, γ, rh, rl, xaxis=xaxis, yaxis=yaxis, prec=prec,
cautiousafecolor=cautious_safe_color,
ax=ax[2])
# clear labels
for a in ax:
a.set_ylabel("")
a.set_xlabel("")
# titles
yight = 1.07
fsize = 14
ax[0].annotate("Optimization paradigm", (0.5, yight), xycoords="axes fraction",
ha="center", va="center", fontsize=fsize)
ax[1].annotate("Sustainability paradigm", (0.5, yight), xycoords="axes fraction",
ha="center", va="center", fontsize=fsize)
ax[2].annotate("Safe Operating Space\nparadigm", (0.5, yight), xycoords="axes fraction",
ha="center", va="center", fontsize=fsize)
# numbers
ax[0].annotate("a", (0.0, yight), xycoords="axes fraction", weight='demibold',
ha="left", va="center", fontsize=12)
ax[1].annotate("b", (0.0, yight), xycoords="axes fraction", weight='demibold',
ha="left", va="center", fontsize=12)
ax[2].annotate("c", (0.0, yight), xycoords="axes fraction", weight='demibold',
ha="left", va="center", fontsize=12)
# add labels
xlab = r"Collapse probability $\delta$"
ylab = r"Discount factor $\gamma$"
labelfsize = 10
for axis in ax:
axis.set_xlabel(xlab)
axis.tick_params(axis='both', which='major', labelsize=smallfontsize)
axis.xaxis.label.set_fontsize(labelfsize)
for axis in [ax[0]]:
axis.set_ylabel(ylab)
axis.tick_params(axis='both', which='major', labelsize=smallfontsize)
axis.yaxis.label.set_fontsize(labelfsize)
# Legend
plt.subplots_adjust(bottom=bott, top=topp, left=leftt, right=rightt)
lfontsize = 9
dy = 0.05
legendtopbottom = 0.07
# - left column
left = 0.1
ro = fig.add_axes((left, legendtopbottom, 0.02,0.02), frameon=False, xticks=[], yticks=[])
ro.fill_between(np.linspace(0,1,prec), 0, 1, color=risky_opt_color)
ro.annotate(" only risky policy optimal", (1.0, 0.5), xycoords="axes fraction", ha="left", va="center",
fontsize=lfontsize)
so= fig.add_axes((left, legendtopbottom-dy, 0.02, 0.02), frameon=False, xticks=[], yticks=[])
so.fill_between(np.linspace(0,1,prec), 0, 1, color=cautious_opt_color)
so.annotate(" only cautious policy optimal", (1.0, 0.5), xycoords="axes fraction", ha="left", va="center",
fontsize=lfontsize)
# - mid column
left = 0.41
bs = fig.add_axes((left, legendtopbottom, 0.02,0.02), frameon=False, xticks=[], yticks=[])
bs.fill_between(np.linspace(0,1,prec), 0, 1, color=both_sus_color)
bs.annotate(" both policies sustainable", (1.0, 0.5),
xycoords="axes fraction", ha="left", va="center", fontsize=lfontsize)
ss = fig.add_axes((left, legendtopbottom-dy, 0.02,0.02), frameon=False, xticks=[], yticks=[])
ss.fill_between(np.linspace(0,1,prec), 0, 1, color=cautious_sus_color)
ss.annotate(" only cautious policy sustainable", (1.0, 0.5),
xycoords="axes fraction", ha="left", va="center", fontsize=lfontsize)
# - right column
left = 0.746
bsos = fig.add_axes((left, legendtopbottom, 0.02,0.02), frameon=False, xticks=[], yticks=[])
bsos.fill_between(np.linspace(0,1,prec), 0, 1, color=cautious_safe_color)
bsos.annotate(" only cautious policy safe", (1.0, 0.5),
xycoords="axes fraction", ha="left", va="center", fontsize=lfontsize)
# space
plt.subplots_adjust(wspace=0.1)
plt.savefig(savepath + "Results_Policies.png", dpi=300)
###Output
_____no_output_____
###Markdown
Policy Combinations
###Code
fig = plt.figure(figsize=(7, 11))
# ========================
# AXES
# ========================
leftax = 0.1
rightax = 0.98
bottax = 0.05
highax = 0.55
dx=0.01
dy=0.008
ax11 = plt.axes([leftax,
bottax,
0.5*(rightax-leftax)-dx,
0.5*(highax-bottax)-dy])
ax21 = plt.axes([leftax,
dy+bottax+0.5*(highax-bottax),
0.5*(rightax-leftax)-dx,
0.5*(highax-bottax)-dy])
ax12 = plt.axes([dx+leftax+0.5*(rightax-leftax),
bottax,
0.5*(rightax-leftax)-dx,
0.5*(highax-bottax)-dy])
ax22 = plt.axes([dx+leftax+0.5*(rightax-leftax),
dy+bottax+0.5*(highax-bottax),
0.5*(rightax-leftax)-dx,
0.5*(highax-bottax)-dy])
#ax3 = plt.axes([-d3 , -0.03+bottax+0.2*highax,
# 0.04+leftax, 0.03+0.6*(highax-bottax)])
# ==========================
# Plottings
# ==========================
# ρ = 0.2
rmin = 0.3
_plot_PolicyCombinations(δ, ρ, γ, rh, rl, rmin, xaxis=xaxis, yaxis=yaxis, prec=prec,
policy="risky", ax=ax21)
ax21.set_title("Risky Policy")
ax21.set_ylabel(r"Discount factor $\gamma$")
ax21.set_xlabel("")
ax21.set_xticklabels([])
_plot_PolicyCombinations(δ, ρ, γ, rh, rl, rmin, xaxis=xaxis, yaxis=yaxis, prec=prec,
policy="safe", ax=ax22)
ax22.set_title("Cautious Policy")
ax22.set_ylabel(r"")
ax22.set_xlabel(r"")
ax22.set_yticklabels([])
ax22.set_xticklabels([])
rmin = 0.7 # ======================================
_plot_PolicyCombinations(δ, ρ, γ, rh, rl, rmin, xaxis=xaxis, yaxis=yaxis, prec=prec,
policy="risky", ax=ax11)
ax11.set_xlabel(r"Collapse probability $\delta$")
ax11.set_ylabel(r"Discount factor $\gamma$")
_plot_PolicyCombinations(δ, ρ, γ, rh, rl, rmin, xaxis=xaxis, yaxis=yaxis, prec=prec,
policy="safe", ax=ax12)
ax12.set_xlabel(r"Collapse probability $\delta$")
ax12.set_yticklabels([])
# Threshold labels
ax12.annotate(r"High normative threshold $r_{min}$", (-0.03, 0.5), xycoords="axes fraction",
ha="center", va="center",
rotation=0, bbox=dict(boxstyle="round", fc=(1.,1.,1.), ec=(0., .0, .0)))
ax22.annotate(r"Low normative threshold $r_{min}$", (-.03, 0.5), xycoords="axes fraction",
ha="center", va="center",
rotation=0, bbox=dict(boxstyle="round", fc=(1.,1.,1.), ec=(0., .0, .0)))
d3=0.04
ax3 = plt.axes([0, highax+d3, 0.8, 1-highax-1.1*d3 ])
image = plt.imread("figs/Paradigms_Legend.png")
ax3.imshow(image)
ax3.set_yticks([])
ax3.axis('off') # clear x- and y-axes
# Trefoil Legend
left = 0.75
dy = 0.03
legendtopbottom = highax+d3+(1-highax-d3)/2 + 1.2*dy #0.02+highax+d3+3*dy
lfontsize = 10
cv = 200/255.
l1 = fig.add_axes((left, legendtopbottom, 0.06,0.02), frameon=False, xticks=[], yticks=[])
l1.fill_between(np.linspace(0,0.5,prec), 0, 1, color=(cv, cv, 0.))
l1.fill_between(np.linspace(0.5,1,prec), 0, 1, color=(cv, 0, 0))
l1.annotate(" opt & not sus", (1.0, 0.5),
xycoords="axes fraction", ha="left", va="center", fontsize=lfontsize)
l2 = fig.add_axes((left, legendtopbottom-dy, 0.06,0.02), frameon=False, xticks=[], yticks=[])
l2.fill_between(np.linspace(0,0.5,prec), 0, 1, color=(cv, 0, 0.))
l2.fill_between(np.linspace(0.5,1,prec), 0, 1, color=(cv, 0, cv))
l2.annotate(" opt & not safe", (1.0, 0.5),
xycoords="axes fraction", ha="left", va="center", fontsize=lfontsize)
l3 = fig.add_axes((left, legendtopbottom-2*dy, 0.06,0.02), frameon=False, xticks=[], yticks=[])
l3.fill_between(np.linspace(0,0.5,prec), 0, 1, color=(0, cv, 0.))
l3.fill_between(np.linspace(0.5,1,prec), 0, 1, color=(cv, cv, 0))
l3.annotate(" safe & not sus", (1.0, 0.5),
xycoords="axes fraction", ha="left", va="center", fontsize=lfontsize)
l4 = fig.add_axes((left, legendtopbottom-3*dy, 0.06,0.02), frameon=False, xticks=[], yticks=[])
l4.fill_between(np.linspace(0,0.5,prec), 0, 1, color=(0, 0, cv))
l4.fill_between(np.linspace(0.5,1,prec), 0, 1, color=(cv, 0, cv))
l4.annotate(" sus & not safe", (1.0, 0.5),
xycoords="axes fraction", ha="left", va="center", fontsize=lfontsize)
# number labels
ax3.annotate("a", (0.04, 0.94), xycoords="axes fraction", ha="center", va="center",
weight='demibold', fontsize=12)
ax21.annotate("b", (0.95, 0.94), xycoords="axes fraction", ha="center", va="center",
weight='demibold', fontsize=12, color="white")
ax11.annotate("c", (0.95, 0.94), xycoords="axes fraction", ha="center", va="center",
weight='demibold', fontsize=12, color="white")
ax22.annotate("d", (0.95, 0.94), xycoords="axes fraction", ha="center", va="center",
weight='demibold', fontsize=12, color="black")
ax12.annotate("e", (0.95, 0.94), xycoords="axes fraction", ha="center", va="center",
weight='demibold', fontsize=12, color="black")
plt.savefig(savepath + "Results_PolicyTrefoil.png", dpi=300)
###Output
_____no_output_____
###Markdown
Real world examples
###Code
# revision update
fig, ax = plt.subplots(1, 3, figsize=(10, 4) )
δ = (0.0, 1.0)
ρ = (0.0, 1.0)
γ = (0.95, 0.99)
rh = (1.0, 1.0)
#rl = 0.4
#rmin = 0.3
rl = (0.3, 0.7)
#rl = (0.25, 0.75)
# rmin = (0.3, 0.5)
rmin = (0.1, 0.5)
xaxis = "δ"
yaxis = "ρ"
prec = 101
uprec = 21
plot_PolicyCombinations_withUncertainty(δ, ρ, γ, rh, rl, rmin,
xaxis=xaxis, yaxis=yaxis,
plotprec=prec, uprec=uprec,
policy="risky", ax=ax[0])
ax[0].set_title("Risky Policy")
ax[0].set_xlabel(r"Collapse probability $\delta$")
ax[0].set_ylabel(r"Recovery probability $\rho$")
plot_PolicyCombinations_withUncertainty(δ, ρ, γ, rh, rl, rmin,
xaxis=xaxis, yaxis=yaxis,
plotprec=prec, uprec=uprec,
policy="cautious", ax=ax[1])
ax[1].set_title("Cautious Policy")
ax[1].set_yticklabels([])
ax[1].set_ylabel("")
ax[1].set_xlabel(r"Collapse probability $\delta$")
image = plt.imread("figs/Paradigms_Legend_Sparse.png")
ax[2].imshow(image)
ax[2].set_yticks([])
ax[2].axis('off') # clear x- and y-axes
ax0 = plt.axes([0.11, 0.2, 0.22, 0.6])
ax1 = plt.axes([0.425, 0.2, 0.22, 0.6])
δ2 = (0.0, 0.055)
ρ2 = (0.0, 0.022)
plot_PolicyCombinations_withUncertainty(δ2, ρ2, γ, rh, rl, rmin,
xaxis=xaxis, yaxis=yaxis,
plotprec=prec, uprec=uprec,
policy="risky", ax=ax0)
plot_PolicyCombinations_withUncertainty(δ2, ρ2, γ, rh, rl, rmin,
xaxis=xaxis, yaxis=yaxis,
plotprec=prec, uprec=uprec,
policy="cautious", ax=ax1)
ax0.spines['bottom'].set_color('white')
ax0.spines['top'].set_color('white')
ax0.spines['left'].set_color('white')
ax0.spines['right'].set_color('white')
for t in ax0.xaxis.get_ticklines(): t.set_color('white')
for t in ax0.yaxis.get_ticklines(): t.set_color('white')
for t in ax0.xaxis.get_ticklabels(): t.set_color("white")
for t in ax0.yaxis.get_ticklabels(): t.set_color("white")
ax1.set_xticks([0.0, 0.025, 0.05])
ax0.set_xticks([0.0, 0.025, 0.05])
ax0.set_yticks([0.0, 0.01, 0.02])
ax1.set_yticks([0.0, 0.01, 0.02])
ax1.set_xlabel("")
ax0.set_xlabel("")
ax1.set_ylabel("")
ax0.set_ylabel("")
props = lambda years: 1/(years+1)
#ax0.plot([props(30), props(50)], [0, 0], lw=4, color="yellow")
ax0.annotate("Climate", (0.025, 0.0), xycoords="data", color="w", ha="center", va="bottom")
ax1.annotate("Climate", (0.025, 0.0), xycoords="data", color="k", ha="center", va="bottom")
ax0.annotate("Fisheries", (0.045, 0.02), xycoords="data", color="w", ha="center", va="center")
ax1.annotate("Fisheries", (0.045, 0.02), xycoords="data", color="k", ha="center", va="center")
ax0.annotate("Farming", (0.01, 0.003), xycoords="data", color="w", ha="center", va="bottom")
ax1.annotate("Farming", (0.01, 0.003), xycoords="data", color="k", ha="center", va="bottom")
ax[0].annotate("a", (0.95, 0.95), xycoords="axes fraction", ha="center", va="center",
weight='demibold', fontsize=12, color="white")
ax[1].annotate("b", (0.95, 0.95), xycoords="axes fraction", ha="center", va="center",
weight='demibold', fontsize=12, color="k")
plt.subplots_adjust(wspace=0.08, left=0.06, right=0.98)
plt.savefig(savepath + "Policies_RealWorld.png", dpi=300)
###Output
_____no_output_____
###Markdown
Volumes
###Code
%run plots.py
plot_ParadigmVolumes()
plt.tight_layout()
plt.savefig(savepath + "VolumesOfParadigmCombinations.png", dpi=300)
###Output
_____no_output_____
###Markdown
Methods Fig: Acceptable States + Sustainable Policies
###Code
%run plots.py
δ = 1.0
ρ = 0.2
γ = 1.0
rh = 1.0
rl = 0.5
rmin = 0.3
xaxis = "δ"
yaxis = "γ"
prec = 501
fig, ax = plt.subplots(2, 2, sharey='row', sharex="col", figsize=(5, 5) )
bott=0.28
topp=0.9
leftt=0.19
rightt=0.94
smallfontsize = 8.5
# colors
ov = 0.6
tol_underboth_color = (1.0, 1.0, 0.0)
tol_undercautious_color = (1.0-ov, 1.0, 0.0)
tol_underrisky_color = (1.0, 1.0-ov, 0.0)
tol_underno_color = (1.0-ov, 1.0-ov, 0.0)
both_sus_color = (ov, ov, 1.0)
cautious_sus_color = (0.0, ov, 1.0)
# the actual plot
plot_acceptal_states(δ, ρ, γ, rh, rl, rmin,
bothacceptcolor=tol_underboth_color,
cautiousacceptcolor=tol_undercautious_color,
riskyacceptcolor=tol_underrisky_color,
nonacceptcolor=tol_underno_color,
state="degraded",
xaxis="δ", yaxis="γ", prec=prec, ax=ax[1,0])
plot_acceptal_states(δ, ρ, γ, rh, rl, rmin,
bothacceptcolor=tol_underboth_color,
cautiousacceptcolor=tol_undercautious_color,
riskyacceptcolor=tol_underrisky_color,
nonacceptcolor=tol_underno_color,
state="prosperous",
xaxis="δ", yaxis="γ", prec=prec, ax=ax[0,0])
plot_sustainble_policies(δ, ρ, γ, rh, rl, rmin, xaxis=xaxis, yaxis=yaxis,
prec=prec,
bothsuscolor=both_sus_color,
cautioussuscolor=cautious_sus_color,
ax=ax[1,1])
plot_sustainble_policies(δ, ρ, γ, rh, rl, rmin, xaxis=xaxis, yaxis=yaxis,
prec=prec,
bothsuscolor=both_sus_color,
cautioussuscolor=cautious_sus_color,
ax=ax[0,1])
#plot_acceptable_region_to_ax(a, b, g, hhh, hlh, rmin=r_min, axes=ax.T[0])
#plot_suspol_to_ax(a, b, g, hhh, hlh, rmin=r_min, axes=ax.T[1])
# clear labels
for axes in ax:
map(lambda ax: ax.set_ylabel(""), axes)
map(lambda ax: ax.set_xlabel(""), axes)
# titles
yight = 1.15
fsize = 14
ax[0, 0].annotate("Acceptable states", (0.5, yight), xycoords="axes fraction",
ha="center", va="bottom", fontsize=fsize)
ax[0, 1].annotate("Sustainable policies", (0.5, yight), xycoords="axes fraction",
ha="center", va="bottom", fontsize=fsize)
fig.subplots_adjust(left=0.2)
ax[0, 0].annotate("Prosperous state", (-0.4, 0.5), xycoords="axes fraction", ha="right", va="center",
rotation=90, fontsize=13)
ax[1, 0].annotate("Degraded state", (-0.4, 0.5), xycoords="axes fraction", ha="right", va="center",
rotation=90, fontsize=13)
# numbers
ax[0, 0].annotate("a", (0.95, 0.97), xycoords="axes fraction", ha="right", va="top", fontsize=12)
ax[1, 0].annotate("b", (0.95, 0.97), xycoords="axes fraction", ha="right", va="top", fontsize=12)
ax[0, 1].annotate("c", (0.95, 0.97), xycoords="axes fraction", ha="right", va="top", fontsize=12)
ax[1, 1].annotate("d", (0.95, 0.97), xycoords="axes fraction", ha="right", va="top", fontsize=12)
# add labels
xlab = r"Collapse probability $\delta$"
ylab = r"Discount factor $\gamma$"
labelfsize = 11
for axis in ax[1]:
axis.set_xlabel(xlab)
axis.tick_params(axis='both', which='major', labelsize=smallfontsize)
axis.xaxis.label.set_fontsize(labelfsize)
for axis in ax.T[0]:
axis.set_ylabel(ylab)
axis.tick_params(axis='both', which='major', labelsize=smallfontsize)
axis.yaxis.label.set_fontsize(labelfsize)
ax[0, 1].set_ylabel("")
ax[1, 1].set_ylabel("")
ax[0, 0].set_xlabel("")
ax[0, 1].set_xlabel("")
# Legend
plt.subplots_adjust(bottom=bott, top=topp, left=leftt, right=rightt)
lfontsize = 7.5
dy = 0.04
legendtopbottom = 0.15
# - left column
left = 0.16
tuboth = fig.add_axes((left, legendtopbottom, 0.02,0.02), frameon=False, xticks=[], yticks=[])
tuboth.fill_between(np.linspace(0,1,prec), 0, 1, color=tol_underboth_color)
tuboth.annotate(" acceptable under both policies", (1.0, 0.5),
xycoords="axes fraction", ha="left", va="center", fontsize=lfontsize)
tusafe = fig.add_axes((left, legendtopbottom-dy, 0.02,0.02), frameon=False, xticks=[], yticks=[])
tusafe.fill_between(np.linspace(0,1,prec), 0, 1, color=tol_undercautious_color)
tusafe.annotate(" acceptable only under cautious policy", (1.0, 0.5),
xycoords="axes fraction", ha="left", va="center", fontsize=lfontsize)
turisky = fig.add_axes((left, legendtopbottom-2*dy, 0.02,0.02), frameon=False, xticks=[], yticks=[])
turisky.fill_between(np.linspace(0,1,prec), 0, 1, color=tol_underrisky_color)
turisky.annotate(" acceptable only under risky policy", (1.0, 0.5),
xycoords="axes fraction", ha="left", va="center", fontsize=lfontsize)
tuno = fig.add_axes((left, legendtopbottom-3*dy, 0.02,0.02), frameon=False, xticks=[], yticks=[])
tuno.fill_between(np.linspace(0,1,prec), 0, 1, color=tol_underno_color)
tuno.annotate(" not acceptable under both policies", (1.0, 0.5),
xycoords="axes fraction", ha="left", va="center", fontsize=lfontsize)
# - right column
left = 0.59
bs = fig.add_axes((left, legendtopbottom, 0.02,0.02), frameon=False, xticks=[], yticks=[])
bs.fill_between(np.linspace(0,1,prec), 0, 1, color=both_sus_color)
bs.annotate(" both policies sustainable", (1.0, 0.5),
xycoords="axes fraction", ha="left", va="center", fontsize=lfontsize)
ss = fig.add_axes((left, legendtopbottom-dy, 0.02,0.02), frameon=False, xticks=[], yticks=[])
ss.fill_between(np.linspace(0,1,prec), 0, 1, color=cautious_sus_color)
ss.annotate(" only cautious policy sustainable", (1.0, 0.5),
xycoords="axes fraction", ha="left", va="center", fontsize=lfontsize)
# space
plt.subplots_adjust(wspace=0.1, hspace=0.1)
plt.savefig(savepath + "Results_AcceptSus.png")
###Output
_____no_output_____ |
lab2/lab2-parte1.ipynb | ###Markdown
[](https://colab.research.google.com/github/eirasf/GCED-AA2/blob/main/lab2/lab2-parte1.ipynb) Práctica 1: Redes neuronales desde cero con TensorFlowEn esta práctica vamos a familiarizarnos con la librería TensorFlow. Para ello, vamos a desarrollar un clasificador que utilice una red neuronal para modelar los datos y hacer predicciones. Esto requiere que diseñemos la red neuronal y que hagamos el entrenamiento para que las predicciones sean correctas para el conjunto de datos dado. Pre-requisitos Instalar paquetesPara esta práctica solo necesitaremos NumPy, TensorFlow 2.0 y TensorFlow-Datasets
###Code
# Ejemplo de instalación de tensorflow 2.0
#%tensorflow_version 2.x
!pip3 install tensorflow numpy tensorflow-datasets
import tensorflow as tf
import numpy as np
import tensorflow_datasets as tfds
###Output
_____no_output_____
###Markdown
Creación de una neurona artificial con NumpyUna neurona artificial consta de dos partes diferenciadas. En primer lugar, la unidad realiza una suma de todas sus entradas (más una componente de *bias*), cada una de ellas ponderada por un peso. Estos pesos (y el *bias*) serán los que se modifiquen para conseguir que la neurona dé la salida adecuada para nuestro problema para cada combinación de entradas del conjunto de datos de entrada.Dado un vector de entrada $\mathbf{x}$ con $d$ componentes y un vector de pesos $\mathbf{w}$, esta primera parte de la neurona calculará un único valor escalar de salida que llamaremos $z$ de la siguiente forma:$$z = \sum \limits_{i=0}^{d} \mathbf{x}_i\mathbf{w}_i + bias$$ Notación vectorialPara simplificar la notación, podemos representar todos los pesos de una neurona como un vector. Al hacerlo así, la suma ponderada de las entradas será el producto escalar del vector $\mathbf{x}$ de entrada y el vector $\mathbf{w}$ de pesos. Teniendo en cuenta que asumiremos que el vector de entrada $\mathbf{x}$ es un vector fila de dimensiones 1 x $d$, declararemos el vector de pesos $\mathbf{w}$ con las mismas dimensiones y podremos representar el producto escalar $\mathbf{x} · \mathbf{w}$ como el producto matricial $\mathbf{x}\mathbf{w}^T$. Esto es ventajoso para poder procesar varios vectores de entrada utilizando la misma operación.$$z = \sum \limits_{i=0}^{d} \mathbf{x}_i\mathbf{w}_i + bias = \mathbf{x} · \mathbf{w} + bias = \mathbf{x} \mathbf{w}^T + bias$$Completa la siguiente celda para calcular $z$.
###Code
x = np.array([1, 2, 8, -4]).reshape((1,4)) # vector de entrada
w = np.array([0.1, -0.8, 0.3, 0.2]).reshape((1,4)) # vector de pesos
bias = 0.1
# TODO - completa esta línea
z =
# verificación del resultado
np.testing.assert_almost_equal(0.2, z, err_msg='Revisa tu implementación')
###Output
_____no_output_____
###Markdown
Función de activaciónTras este primer paso, la salida $z$ será una combinación lineal de las entradas. Si concatenamos varias neuronas así definidas, el resultado seguirá siendo una combinación lineal de las entradas, lo cual no es muy útil dado que se podría obtener el mismo resultado con una sola neurona. Es por ello que necesitamos que cada neurona tenga una segunda parte que introduzca una no-linealidad. Es lo que denominamos *función de activación*. En este ejemplo tomaremos como función de activación la función sigmoide, definida como:$$sigmoide(x) = \dfrac{1}{1+e^{-x}}$$Completa a continuación el código para calcular la sigmoide de un escalar $x$:
###Code
def sigmoide(x):
#TODO - Completa la siguiente línea
return
# verificación del resultado
np.testing.assert_almost_equal(0.54983399, sigmoide(z))
###Output
_____no_output_____
###Markdown
En este caso, tomaremos como entrada de la función sigmoide la salida del paso anterior, $z$. Por tanto, la salida $y$ de la neurona artificial será un escalar de la siguiente forma:$$y = sigmoide(z) = \dfrac{1}{1+e^{-(\mathbf{x}\mathbf{w}^T + bias)}}$$Puedes ver el esquema general de una neurona artificial en este diagrama:Completa el código de esta función realizar el *forward pass* de una neurona artificial con función de activación sigmoide, es decir, para calcular la salida a partir del vector de entrada, el vector de pesos y el valor de bias:
###Code
def neurona_forward(x, w, bias):
#TODO - Completa la siguiente línea
return
# verificación
np.testing.assert_almost_equal(0.54983399, neurona_forward(x, w, bias))
###Output
_____no_output_____
###Markdown
Red feed-forwardCon este montaje, si utilizásemos descenso de gradiente para aprender el vector $\mathbf{w}$ que haga las predicciones correctas para un conjunto de datos dado, estaríamos entrenando un modelo de regresión logística. Sin embargo, la potencia de las redes neuronales reside en la posibilidad de combinar muchas de estas unidades para poder modelar funciones mucho más complejas, por lo que vamos a construir una red que utilice varias unidades.El modo más sencillo de organizar varias neuronas es formando una red *feed-forward*. Para ello, primero agruparemos varias neuronas formando una *capa*. Si la salida de una neurona era un escalar $y$, la salida de una capa será un vector $\mathbf{y}$ con tantas componentes como unidades tenga la capa. Igualmente, si en una neurona teníamos un vector $\mathbf{w}$ de pesos, en una capa tendremos una matriz $\mathbf{W}$ de pesos, en la que cada fila corresponderá con el vector de pesos de una neurona de la capa. También tendremos un vector $\mathbf{b}$, donde cada componente será el *bias* de una neurona de la capa.Podemos aprovechar las operaciones de matrices y vectores de NumPy para hacer el *forward pass* de toda una capa de una sola vez. Completa el siguiente código para obtener una función que realice el *forward pass* de una capa de neuronas artificiales con activación sigmoide tomando como entradas el vector de entrada $\mathbf{x}$, una matriz de pesos $\mathbf{W}$ y un vector $\mathbf{b}$.
###Code
# Asegúrate de que tu implementación de sigmoide puede tomar como entrada un vector. (La función np.exp de NumPy te puede resultar útil, porque puede tomar como entrada un escalar y devolver un escalar, pero también puede tomar un vector y devolver otro vector).
np.testing.assert_almost_equal([0.549834, 0.98201379], sigmoide(np.array([z[0][0],4])))
def capa_forward(x, W, b):
# TODO - Completa el código. La versatilidad de NumPy hace que esto sea muy sencillo
return
# verificación
np.testing.assert_almost_equal(np.array([[0.549834], [0.05732418]]), capa_forward(np.vstack((x, np.array([-1, 3, -2, 2]))), w, np.array([bias, -0.1]).reshape((2,1))))
###Output
_____no_output_____
###Markdown
Ahora podemos concatenar capas de neuronas con facilidad. Vamos a crear una red de tres capas: 1. La capa $C_0$ consta de 5 unidades. Recibe como entrada el vector $\mathbf{x}$ y produce como salida el vector $\mathbf{h_0}$. Tiene una matriz de pesos $\mathbf{W_0}$ y un vector de bias $\mathbf{b_0}$. 1. La capa $C_1$ consta de 3 unidades. Recibe como entrada el vector $\mathbf{h_0}$ y produce como salida el vector $\mathbf{h_1}$. Tiene una matriz de pesos $\mathbf{W_1}$ y un vector de bias $\mathbf{b_1}$. 1. La capa $C_2$ consta de 1 unidad. Recibe como entrada el vector $\mathbf{h_1}$ y produce como salida el vector $\mathbf{y}$. Tiene una matriz de pesos $\mathbf{W_2}$ y un vector de bias $\mathbf{b_2}$. Completa la siguiente celda para calcular la salida de la red.
###Code
# Inicialización de pesos
np.random.seed(1234567) # Fijamos la semilla para que los números aleatorios salgan igual en cada ejecución para poder verificar los resultados
# TODO - Completa las dimensiones de las matrices
W0 = np.random.rand(5, x.shape[1]) - 0.5
b0 = np.random.rand(1, 5) - 0.5
W1 = np.random.rand(, ) - 0.5
b1 = np.random.rand(, ) - 0.5
W2 = np.random.rand(, ) - 0.5
b2 = np.random.rand(, ) - 0.5
# Función auxiliar que utiliza la red neuronal para hacer una predicción
def calcula_prediccion(x):
# TODO - Calcula las salidas de cada capa
h0 =
h1 =
y =
return y
# verificación
np.testing.assert_almost_equal(0.34535528, calcula_prediccion(x))
###Output
_____no_output_____
###Markdown
Hacer predicciones con la red sobre un conjunto de datosAhora que sabemos definir una red, probemos qué predicciones hace sobre un conjunto de datos. Cargaremos el conjunto `german_credit_numeric` de tensorflow_datasets, calcularemos la salida predicha para cada entrada y la compararemos con la etiqueta que se espera.
###Code
import tensorflow_datasets as tfds
# Cargamos el conjunto de datos
ds = tfds.load('german_credit_numeric', split='train')
# TODO - Adapta las dimensiones de los parámetros de la capa de entrada (C0) para que coincidan con el tamaño de los vectores del conjunto de datos, que tienen 24 componentes
W0 = np.random.rand(, ) - 0.5
b0 = np.random.rand(, )
# Función auxiliar que, dada una lista de vectores de entrada, calcula la tasa de aciertos de la red
def calcula_tasa_aciertos(ejemplos):
num_aciertos = 0
num_elems = 0
for ej in ejemplos:
x = tfds.as_numpy(ej["features"])
label = tfds.as_numpy(ej["label"])
y_pred = calcula_prediccion(x)
# Actualizamos los contadores de elementos y de aciertos
num_elems += 1
if ((y_pred > 0.5) and (label==1)) or ((y_pred <= 0.5) and (label==0)):
num_aciertos += 1
return num_aciertos / float(num_elems)
print('La tasa de acierto es del', calcula_tasa_aciertos(ds.take(100)))
###Output
_____no_output_____
###Markdown
Previsiblemente, la red no funciona bien dado que las matrices de pesos contienen vectores aleatorios. Para que las predicciones mejoren, tenemos que ajustar nuestro modelo al conjunto de datos, es decir, encontrar valores para los parámetros ($\mathbf{W_0}$, $\mathbf{b_0}$, $\mathbf{W_1}$, $\mathbf{b_1}$, $\mathbf{W_2}$, $\mathbf{b_2}$) que arrojen buenas predicciones. Entrenamiento de la red La función de costePara entrenar la red utilizaremos un proceso de optimización. En primer lugar, debemos definir qué función queremos optimizar. Necesitamos una función que, dada una combinación de parámetros de la red, devuelva un valor alto cuando las predicciones con esos parámetros sean malas y un valor bajo cuando estas sean buenas. Esto es lo que denominamos la **función de coste** ($J$). Definiremos una **función de pérdida** ($\mathcal{L}$) que reciba como entrada una predicción y una etiqueta real y nos indique cómo de desacertada es la predicción. En este caso utilizaremos la entropía cruzada binaria, descrita como:$$\mathcal{L}(y_{pred},y_{etiqueta}) = - y_{etiqueta} \log(y_{pred}) - (1-y_{etiqueta}) \log(1-y_{pred})$$La función de coste ($J$) será la media de la función de pérdida en los $m$ ejemplos del conjunto de entrenamiento:$$ J(\mathbf{W},\mathbf{b}) = \frac{1}{m} \sum_{i=1}^m \mathcal{L}(y_{pred}^{(i)}, y_{etiqueta}^{(i)})$$Si minimizamos $J$, nuestras predicciones serán mejores. Para minimizar $J$ utilizaremos **descenso de gradiente**: haremos sucesivos pasos en los que calcularemos el gradiente de $J$ respecto a los distintos parámetros ($\mathbf{W},\mathbf{b}$) y actualizaremos los parámetros en la dirección del gradiente, con la esperanza de que el siguiente paso obtenga un valor de $J$ menor. Repetiremos este proceso durante un número fijo de pasos.Por tanto, el algoritmo que debemos aplicar es el siguiente: 1. Calcular la pérdida de las predicciones con los valores actuales de $\mathbf{W}$ y $\mathbf{b}$ 1. Calcular el gradiente respecto $\mathbf{W}$ y $\mathbf{b}$. 1. Actualizar $\mathbf{W}$ y $\mathbf{b}$ en la dirección de sus gradientes respectivos. GradientesEl segundo paso nos obliga a ser capaces de calcular el gradiente de $J(\mathbf{W},\mathbf{b})$ respecto a cada uno de los parámetros, es decir, la derivada parcial de $J(\mathbf{W},\mathbf{b})$ respecto a cada parámetro. Para ello iremos propagando el gradiente hacia atrás, calculando a cada paso el gradiente en el nodo anterior a partir de los posteriores. Calculemos, por ejemplo, el gradiente de $J(\mathbf{W},\mathbf{b})$ respecto a $z_2$:$$ \frac{\partial J(\mathbf{W},\mathbf{b})}{\partial z_2} = \frac{\partial (\frac{1}{m} \sum_{i=1}^m \mathcal{L}(y_{pred}^{(i)}, y_{etiqueta}^{(i)}))}{\partial z_2} = \frac{1}{m} \sum_{i=1}^m \frac{\partial \mathcal{L}(y_{pred}^{(i)}, y_{etiqueta}^{(i)})}{\partial z_2} $$Aplicando la regla de la cadena ([ver el desarrollo completo](./lab2-gradientes.pdf)) obtenemos las siguientes fórmulas para los gradientes:$$ \frac{\partial \mathcal{L}(y_{pred}, y_{etiqueta})}{\partial b_2} = \frac{\partial \mathcal{L}(y_{pred}, y_{etiqueta})}{\partial z_2}$$$$ \frac{\partial \mathcal{L}(y_{pred}, y_{etiqueta})}{\partial W_2} = \frac{\partial \mathcal{L}(y_{pred}, y_{etiqueta})}{\partial z_2} h_1^T $$.$$ \frac{\partial \mathcal{L}(y_{pred}, y_{etiqueta})}{\partial h_1} = \frac{\partial \mathcal{L}(y_{pred}, y_{etiqueta})}{\partial z_2} W_2^T $$$$ \frac{\partial \mathcal{L}(y_{pred}, y_{etiqueta})}{\partial z_1} = \frac{\partial \mathcal{L}(y_{pred}, y_{etiqueta})}{\partial h_1} h_1 (1 - h_1) = dLdz1$$$$ \frac{\partial \mathcal{L}(y_{pred}, y_{etiqueta})}{\partial b_1} = \sum_{i=1}^{5} dJdz1_i $$$$ \frac{\partial \mathcal{L}(y_{pred}, y_{etiqueta})}{\partial W_1} = diag(dLdz1) h_0^T $$$$ \frac{\partial \mathcal{L}(y_{pred}, y_{etiqueta})}{\partial h_0} = diag(dLdz1) W_1 $$$$ \frac{\partial \mathcal{L}(y_{pred}, y_{etiqueta})}{\partial z0} = \frac{\partial \mathcal{L}(y_{pred}, y_{etiqueta})}{\partial h_0} h_0 (1 - h_0) = dLdz0$$$$ \frac{\partial \mathcal{L}(y_{pred}, y_{etiqueta})}{\partial b_0} = \sum_{i=1}^{5} dLdz0_i $$$$ \frac{\partial \mathcal{L}(y_{pred}, y_{etiqueta})}{\partial W_0} = diag(dLdz0) x^T $$Conociendo estas fórmulas podemos adaptar nuestra función para que, además de calcular la predicción, devuelva los gradientes de la función de pérdida respecto a las variables involucradas.
###Code
def calcula_y_propaga(x, y_etiqueta):
''' Devuelve la salida predicha y, el valor de la función de pérdida y un diccionario con los gradientes de las variables
'''
x = x.reshape((1,24))
# Cálculo de la salida
# TODO - Rellena las siguientes líneas
z0 =
h0 =
z1 =
h1 =
z2 =
y =
#Backpropagation
dLdz2 = y - y_etiqueta
dLdb2 = dLdz2
dLdW2 = dLdz2 * h1
dLdh1 = np.matmul(dLdz2.T, W2)
dLdz1 = dLdh1 * h1 * (1 - h1)
dLdb1 = dLdz1
dLdW1 = np.matmul(dLdz1.T, h0)
dLdh0 = np.matmul(dLdz1, W1)
dLdz0 = dLdh0 * h0 * (1 - h0)
dLdb0 = dLdz0
dLdW0 = np.matmul(dLdz0.T, x)
# Los gradientes tienen que tener la misma forma que las variables respecto a las que se toman
assert(dLdz2.shape==z2.shape)
assert(dLdb2.shape==b2.shape)
assert(dLdW2.shape==W2.shape)
assert(dLdh1.shape==h1.shape)
assert(dLdz1.shape==z1.shape)
assert(dLdb1.shape==b1.shape)
assert(dLdW1.shape==W1.shape)
assert(dLdh0.shape==h0.shape)
assert(dLdz0.shape==z0.shape)
assert(dLdb0.shape==b0.shape)
assert(dLdW0.shape==W0.shape)
# Preparación del diccionario de gradientes
gradientes = {}
gradientes["b2"] = dLdb2
gradientes["b1"] = dLdb1
gradientes["b0"] = dLdb0
gradientes["W2"] = dLdW2
gradientes["W1"] = dLdW1
gradientes["W0"] = dLdW0
# Pérdida
# TODO - Rellena la siguiente línea
perdida =
return y, perdida.flatten(), gradientes
# verificación
for elem in ds.take(1):
prediccion, perdida, gradientes = calcula_y_propaga(elem["features"].numpy(), elem["label"].numpy())
np.testing.assert_almost_equal(0.34011586, prediccion[0])
np.testing.assert_almost_equal(1.07846895, perdida[0])
###Output
_____no_output_____
###Markdown
Bucle de aprendizajeUna vez podemos calcular los gradientes respecto a las variables para cada ejemplo del conjunto de entrenamiento ya podemos implementar el bucle de entrenamiento.Completa esta función para que realice `num_pasos` de entrenamiento en los cuales se haga lo siguiente: 1. Calcular las predicciones, pérdidas y gradientes para cada elemento del conjunto de entrenamiento 1. Calcular el valor promedio de cada gradiente 1. Utilizar dichos promedios para actualizar las variablesLlevaremos también cuenta de la tasa de aciertos y del valor de la función de coste a cada paso.
###Code
def entrena(ejemplos, etiquetas, num_pasos, learning_rate = 0.001):
paso = 0
while paso < num_pasos:
num_aciertos = 0
num_elems = 0
perdida_total = 0
for x, label in zip(ejemplos, etiquetas):
# TODO - Completa la siguiente línea
y_pred, perdida, gradientes =
# Actualizamos los contadores de elementos y de aciertos
num_elems += 1
if ((y_pred > 0.5) and (label==1)) or ((y_pred <= 0.5) and (label==0)):
num_aciertos += 1
# Actualizamos el acumulador de la pérdida_total
perdida_total += perdida
# Actualizamos las variables en la dirección de su gradiente
global W2, W1, W0, b2, b1, b0
# TODO - Completa las siguientes líneas
W2 = W2 - learning_rate * gradientes['W2']
W1 =
W0 =
b2 =
b1 =
b0 =
tasa_aciertos = num_aciertos / float(num_elems)
perdida = perdida_total / float(num_elems)
print(f'Epoch: {paso}/{num_pasos}: Pérdida: {perdida} Aciertos: {tasa_aciertos}')
paso += 1
###Output
_____no_output_____
###Markdown
Para probar nuestro algoritmo de entrenamiento, tomemos solo los 100 primeros elementos del conjunto de datos y entrenemos el modelo para que se ajuste a dichos datos.
###Code
tamano_lote = 100
elems = ds.batch(tamano_lote)
lote_entrenamiento = None
for elem in elems:
lote_entrenamiento = elem
break
vectores_x = tfds.as_numpy(tf.cast(lote_entrenamiento["features"],dtype=tf.float64))
etiquetas = tfds.as_numpy(tf.cast(lote_entrenamiento["label"],dtype=tf.float64))
entrena(vectores_x, etiquetas, 5000)
###Output
_____no_output_____ |
notebooks/dataset-projections/bison/bison-parametric-tsne-Copy1.ipynb | ###Markdown
Choose GPU (this may not be needed on your computer)
###Code
%env CUDA_DEVICE_ORDER=PCI_BUS_ID
%env CUDA_VISIBLE_DEVICES=1
import tensorflow as tf
gpu_devices = tf.config.experimental.list_physical_devices('GPU')
if len(gpu_devices)>0:
tf.config.experimental.set_memory_growth(gpu_devices[0], True)
print(gpu_devices)
###Output
[PhysicalDevice(name='/physical_device:GPU:0', device_type='GPU')]
###Markdown
load packages
###Code
from tfumap.umap import tfUMAP
import tensorflow as tf
import numpy as np
import matplotlib.pyplot as plt
from tqdm.autonotebook import tqdm
import umap
import pandas as pd
###Output
_____no_output_____
###Markdown
Load dataset
###Code
import requests
import json
url = "https://raw.githubusercontent.com/duhaime/umap-zoo/03819ed0954b524919671a72f61a56032099ba11/data/json/bison.json"
animal = np.array(json.loads(requests.get(url).text)['3d'])
np.shape(animal)
fig, ax = plt.subplots()
ax.scatter(animal[:,2], animal[:,1], s = 1, c = animal[:,0], alpha = 0.1)
ax.axis('equal')
X_train = animal
Y_train = animal[:, 2]
X_train_flat = X_train
###Output
_____no_output_____
###Markdown
Create model and train
###Code
dims = (3)
dataset = 'bison'
from tfumap.parametric_tsne import compute_joint_probabilities, tsne_loss
batch_size = 5000
P = compute_joint_probabilities(X_train_flat, batch_size=batch_size, perplexity=2000, verbose=2)
#ensure_dir(save_loc)
#np.save(save_loc, P)
n_components = 2
encoder = tf.keras.Sequential()
encoder.add(tf.keras.layers.InputLayer(input_shape=[dims]))
encoder.add(tf.keras.layers.Flatten())
encoder.add(tf.keras.layers.Dense(units=100, activation="relu"))
encoder.add(tf.keras.layers.Dense(units=100, activation="relu"))
encoder.add(tf.keras.layers.Dense(units=100, activation="relu"))
encoder.add(
tf.keras.layers.Dense(units=n_components, name="z")
)
from tfumap.paths import ensure_dir, MODEL_DIR, DATA_DIR
X_train_subset = X_train[:np.product(P.shape[:2])]
# Joint probabilities of data
Y_train_tsne = P.reshape(X_train_subset.shape[0], -1)
opt = tf.keras.optimizers.Adam(lr=0.01)
encoder.compile(loss=tsne_loss(d=n_components, batch_size=batch_size), optimizer=opt)
X_train_subset = np.reshape(X_train_subset, ([len(X_train_subset)]+ [dims]))
X_train_subset.shape
Y_train_tsne.shape
# because shuffle == False, the same batches are used each time...
history = encoder.fit(X_train_subset, Y_train_tsne, batch_size=batch_size, shuffle=False, nb_epoch=1000)
z = encoder.predict(X_train)
###Output
_____no_output_____
###Markdown
Plot model output
###Code
fig, ax = plt.subplots( figsize=(8, 8))
sc = ax.scatter(
z[:, 0],
z[:, 1],
c=Y_train[:len(z)],
s=0.1,
alpha=0.5,
rasterized=True,
)
ax.axis('equal')
ax.set_title("tsne in Tensorflow embedding", fontsize=20)
plt.colorbar(sc, ax=ax);
fig, ax = plt.subplots( figsize=(8, 8))
sc = ax.scatter(
z[:, 0],
z[:, 1],
c=Y_train[:len(z)],
s=0.1,
alpha=0.5,
rasterized=True,
)
ax.axis('equal')
ax.set_title("tsne in Tensorflow embedding", fontsize=20)
plt.colorbar(sc, ax=ax);
###Output
_____no_output_____
###Markdown
View loss
###Code
from tfumap.umap import retrieve_tensors
import seaborn as sns
###Output
_____no_output_____
###Markdown
Save output
###Code
from tfumap.paths import ensure_dir, MODEL_DIR
output_dir = MODEL_DIR/'projections'/ dataset / 'parametric-tsne-2000'
ensure_dir(output_dir)
np.save(output_dir / 'z.npy', z)
###Output
_____no_output_____ |
nbks/03_train.ipynb | ###Markdown
Train> This module contains a script to train a model.
###Code
#hide
from nbdev.showdoc import *
from plant_pathology.config import DATA_PATH
from plant_pathology.dataset import get_dls
import torch
%load_ext autoreload
%autoreload 2
#export
from sys import exit
from typing import Callable, Tuple, Union
import numpy as np
import wandb
from fastai.callback.wandb import WandbCallback, wandb
from fastai.vision.all import *
from wwf.vision.timm import timm_learner
from plant_pathology.dataset import get_dls_all_in_1
from plant_pathology.evaluate import evaluate
###Output
_____no_output_____
###Markdown
Train a Model on Data Split
###Code
#export
def timm_or_fastai_arch(arch: str) -> Tuple[Union[Callable, str], Callable]:
"""Check if `arch` is a fast.ai or timm architecture and return appropriate functions."""
try: # Check if fastai arch
model = globals()[arch]
learner_func = cnn_learner
except KeyError: # Must be timm arch
model = arch
learner_func = timm_learner
return model, learner_func
#export
def train(
data_path: Path,
epochs: int = 1,
lr: Union[float, str] = 3e-4,
frz: int = 1,
pre: int = 800,
re: int = 256,
bs: int = 200,
fold: int = 4,
smooth: bool = False,
arch: str = "resnet18",
dump: bool = False,
log: bool = False,
mixup: float = 0.0,
fp16: bool = False,
dls: DataLoaders = None,
save: bool = False,
pseudo: Path = None,
) -> Learner:
""""Train a learner on training CSV (w/folds) at `data_path`."""
# Build DataLoaders
if dls is None:
dls = get_dls_all_in_1(
data_path=data_path,
presize=pre,
resize=re,
bs=bs,
val_fold=fold,
pseudo_labels_path=pseudo,
)
# Initialize wandb logging
if log:
wandb.init(project="plant-pathology")
# Get correct loss, depending on if using label smoothing
if smooth:
loss_func = LabelSmoothingCrossEntropyFlat()
else:
loss_func = CrossEntropyLossFlat()
# Get model and learner_func
m, learner_func = timm_or_fastai_arch(arch)
# Build callbacks
cbs = []
if save or log:
cbs.append(SaveModelCallback("roc_auc_score", fname=f"model_val_on_{fold}"))
if log:
cbs.append(WandbCallback())
if mixup:
cbs.append(MixUp(mixup))
# Build learner
print(f"# train exs: {len(dls.train_ds)}, val exs: {len(dls.valid_ds)}")
learn = learner_func(
dls, m, loss_func=loss_func, metrics=[accuracy, RocAuc()], cbs=cbs
)
# If we just want to print architecture, do that and exit
if dump:
print(learn.model)
exit()
# If we are just running learning rate finder, do that and exit
if lr == "find":
learn.lr_find()
exit()
# Use mixed-precision training if desired
if fp16:
learn.to_fp16()
# Train only head at first
learn.freeze()
learn.fit_one_cycle(frz, lr)
# Train all layers, using discriminative learning rate
learn.unfreeze()
learn.fit_one_cycle(epochs, slice(lr / 100, lr / 2))
return learn
#slow
#hide
learn = train(DATA_PATH, epochs=0, lr=0.001, bs=256, log=False)
###Output
# train exs: 1457, val exs: 364
###Markdown
Train Using Cross-Validation
###Code
#export
def softmax_RocAuc(logits, labels):
"""Compute RocAuc, first taking softmax of `logits`."""
probs = logits.softmax(-1)
return RocAuc()(probs, labels)
#hide
preds = torch.randn(2, 4)
labels = tensor([1, 2, 3, 4]).unsqueeze(-1)
preds, labels.shape
#export
@call_parse
def train_cv(
path: Param("Path to data dir", Path),
epochs: Param("Number of unfrozen epochs", int) = 1,
lr: Param("Initial learning rate", float) = 3e-4,
frz: Param("Number of frozen epochs", int) = 1,
pre: Param("Image presize", int, nargs="+") = (682, 1024),
re: Param("Image resize", int) = 256,
bs: Param("Batch size", int) = 256,
smooth: Param("Label smoothing?", store_true) = False,
arch: Param("Architecture", str) = "resnet18",
dump: Param("Don't train, just print model", store_true) = False,
log: Param("Log w/ W&B", store_true) = False,
save: Param("Save model based on RocAuc", store_true) = False,
mixup: Param("Mixup (0.4 is good)", float) = 0.0,
tta: Param("Test-time augmentation", store_true) = False,
fp16: Param("Mixed-precision training", store_true) = False,
do_eval: Param("Evaluate model and save predictions CSV", store_true) = False,
val_fold: Param("Don't do cross-validation, just do 1 fold", int) = None,
pseudo: Param("Path to pseudo labels to train on", Path) = None,
export: Param("Export learner(s) to export_val_on_{fold}.pkl", store_true) = False,
):
"""Train models using 5-fold cross-validation."""
# Print parameters this was called with
print(locals())
# Do 5-fold cross-validation
scores = []
for fold in range(5):
if val_fold is not None:
# User passed specific fold, so don't do CV. Just do single run w/val_fold.
fold = val_fold
print(f"\nTraining on fold {fold}")
learn = train(
data_path=path,
epochs=epochs,
lr=lr,
frz=frz,
pre=pre,
re=re,
bs=bs,
smooth=smooth,
arch=arch,
dump=dump,
log=log,
fold=fold,
mixup=mixup,
fp16=fp16,
save=save,
pseudo=pseudo,
)
# Bug when doing tta w/Mixup
if hasattr(learn, "mixup") and tta:
learn.remove_cb(MixUp)
# Add final record from this split to scores
if tta and len(learn.dls.valid_ds) != 0: # There is a validation set
# Must get final metrics manually if using TTA
preds, lbls = learn.tta()
res = [f(preds, lbls) for f in [learn.loss_func, accuracy, softmax_RocAuc]]
else:
res = learn.final_record
scores.append(res)
# Create submission file
if do_eval:
print("Evaluating")
evaluate(
learn,
path=path / "test.csv",
name=f"predictions_fold_{fold}.csv",
tta=tta,
)
if export:
learn.export(f"export_val_on_{fold}.pkl")
# Delete learner to avoid OOM
del learn
# If only training on single fold, break
if val_fold is not None:
break
scores = np.array(scores)
print(f"Scores: {scores}\n")
# Print average stats across folds, if trained on multiple folds
if val_fold is None:
print(f"Mean: {scores.mean(0)}")
scores = np.ones((5, 4))
scores.mean(0)
#slow
#hide
train_cv(DATA_PATH, 0, 2e-2, pre=64, re=64, bs=512, fp16=True, val_fold=4, tta=True, mixup=0.4, do_eval=True, export=True)
#slow
#hide
# Check predictions CSV was saved
preds_path = Path("predictions_fold_4.csv")
assert preds_path.exists(), "Predictions CSV not saved properly"
preds_path.unlink()
# Check Learner was exported properly
export_path = Path("export_val_on_4.pkl")
assert export_path.exists(), "Learner not exported properly"
export_path.unlink()
#hide
from nbdev.export import notebook2script; notebook2script()
###Output
Converted 03_train.ipynb.
|
python/day3.ipynb | ###Markdown
Day 3This problem can be solved without the use of a tree-structure. However, doing so is less computationally efficent.
###Code
# Run this cell to solve the actual problem
with open("../dat/day3.txt") as f:
lines = f.readlines()
# Run this cell to use the example data
lines = [
"00100",
"11110",
"10110",
"10111",
"10101",
"01111",
"00111",
"11100",
"10000",
"11001",
"00010",
"01010",
]
class DiagnosticsSolver(object):
def __init__(self, size, pos=0):
self.size = size
self.pos = pos
self.left = None
self.right = None
self.nvalues = 0
if self.pos == 0:
self.alpha_gamma_accumulator = [ 0 ] * self.size
else:
self.alpha_gamma_accumulator = None
self.o2_co2_accumulator = {"0": 0, "1": 0}
def insert(self, value):
self.nvalues += 1
if self.alpha_gamma_accumulator:
for xx in range(self.size):
self.alpha_gamma_accumulator[xx] += int(value[xx])
if self.pos < len(value):
if value[self.pos] == "1":
self.o2_co2_accumulator["1"] += 1
if self.right == None:
self.right = DiagnosticsSolver(self.size, self.pos+1)
self.right.insert(value)
elif value[self.pos] == "0":
self.o2_co2_accumulator["0"] += 1
if self.left == None:
self.left = DiagnosticsSolver(self.size, self.pos+1)
self.left.insert(value)
def solve_gamma_epsilon(self):
gamma = ""
epsilon = ""
for v in self.alpha_gamma_accumulator:
if v * 2 > self.nvalues:
gamma += "1"
epsilon += "0"
else:
gamma += "0"
epsilon += "1"
return int(gamma, 2), int(epsilon, 2)
def solve_o2(self):
def comparator(node):
if node.o2_co2_accumulator["1"] >= node.o2_co2_accumulator["0"]:
return "right"
else:
return "left"
return int(self._solve(comparator), 2)
def solve_co2(self):
def comparator(node):
if node.o2_co2_accumulator["1"] < node.o2_co2_accumulator["0"]:
return "right"
else:
return "left"
return int(self._solve(comparator), 2)
def _solve(self, comparator):
# avoid recursion
result = ""
node = self
while node != None:
if node.left and node.right:
if comparator(node) == "right":
result += "1"
node = node.right
else:
result += "0"
node = node.left
elif node.left:
result += "0"
node = node.left
elif node.right:
result += "1"
node = node.right
else:
node = None
return result
node = DiagnosticsSolver(len(lines[0].strip()))
for vv in lines:
node.insert(vv)
gamma, epsilon = node.solve_gamma_epsilon()
o2 = node.solve_o2()
co2 = node.solve_co2()
print("GAMMA ", gamma)
print("EPSILON", epsilon)
print("O2 ", o2)
print("CO2", co2)
print("Part 1", gamma, "*", epsilon, "=", gamma * epsilon)
print("Part 2", o2, "*", co2, "=", o2 * co2)
###Output
GAMMA 2531
EPSILON 1564
O2 2397
CO2 673
Part 1 2531 * 1564 = 3958484
Part 2 2397 * 673 = 1613181
|
code/Project1Draft5.ipynb | ###Markdown
Fish Project1. Question How would fish population change if a new predator were introduced? This matters because it would inform people about the dangers of invasive species, and also fisherman would care a lot about a decline in fish population 2. Model Number of fish Number of fish and fish eggs consumed by predator Natural birth and death rate of fish Predator birth and death rate? Updating number of fish and predators action Metrics: Number of fish still alive after predators introduced (as %?) 3. Results Number of fish and number of predators are expected to be inveresely proportional But maybe there is also the added complexity of predators increase so fish decline so predators decline so fish increase 4. Interpretation By inputting different numbers of predators we could see how many fish make up the population Code Starts HereQuestion: What would the prey population be t days into the model depending on predator population?Initial predator population (variable)Predator growth function (function) Natural birth rate prey (variable)Natural death rate prey (variable)Prey killed by predator (function of predators and prey)
###Code
# Configure Jupyter so figures appear in the notebook
%matplotlib inline
# Configure Jupyter to display the assigned value after an assignment
%config InteractiveShell.ast_node_interactivity='last_expr_or_assign'
# import functions from the modsim.py module
from modsim import *
from pandas import read_html
def update(r_pop, y_pop):
y_pop = (0.03*y_pop)-(0.0005*y_pop*r_pop) + y_pop #updates the prey pop using Lotka-Volterra equation-the prey # is decreasing here
if y_pop<0: #the population cannot become negative
y_pop = 0
r_pop = (0.0005*y_pop*r_pop - 0.05*r_pop) + r_pop #Lotka-Volterra equation for the growth rate of the predator the prey # is increasing
if r_pop<0:
r_pop = 0
return r_pop, y_pop
def run_simulation(system):
results_y = TimeSeries()
results_r = TimeSeries()
results_y[system.t_0] = system.y_initial_pop
results_r[system.t_0] = system.r_initial_pop
for t in linrange(system.t_0, system.t_end):
results_r[t+1], results_y[t+1] = update(results_r[t], results_y[t])
return results_r, results_y
def plot_results(results, label):
"""Plot the estimates and the model.
census: TimeSeries of population estimates
un: TimeSeries of population estimates
timeseries: TimeSeries of simulation results
title: string
"""
plot(results, label=label)
decorate(xlabel='Time(days)',
ylabel='Population',
title='Time Vs Pop')
ry_system = System(t_0=0,
t_end=600,
y_initial_pop=100,
r_initial_pop=100
)
results_r, results_y = run_simulation(ry_system)
plot_results(results_y, 'prey')
decorate(xlabel='Time(days)',
ylabel='Prey Population',
title='Time Vs Prey Pop')
plot_results(results_r, 'predators')
decorate(xlabel='Time(days)',
ylabel='Predator Population',
title='Time Vs Predator Pop')
r_initial_pop_array = linrange (50, 500, 100, endpoint=True)
for r_pop in r_initial_pop_array:
ry_system = System(t_0=0,
t_end=600,
y_initial_pop=100,
r_initial_pop=r_pop
)
results_r, results_y = run_simulation(ry_system)
plot_results(results_y, r_pop)
decorate(xlabel='Time(days)',
ylabel='Prey Population',
title='Time Vs Prey Pop')
r_initial_pop_array = linrange (100, 1000, 200, endpoint=True)
for r_pop in r_initial_pop_array:
ry_system = System(t_0=0,
t_end=600,
y_initial_pop=100,
r_initial_pop=r_pop
)
results_r, results_y = run_simulation(ry_system)
plot_results(results_r, r_pop)
decorate(xlabel='Time(days)',
ylabel='Predator Population',
title='Time Vs Predator Pop')
###Output
_____no_output_____ |
libretas/clasificador-modelo-avanzado.ipynb | ###Markdown
Contador de Células blancas IntroducciónUn problema importante en el diagnóstico de la sangre es la clasificación de diferentes tipos de células sanguíneas. En este cuaderno, intentaremos entrenar a un clasificador para predecir el tipo de célula sanguínea a la que se le dará una imagen teñida. DatosTenemos 352 imágenes de glóbulos blancos teñidos junto con etiquetas de qué tipo de célula sanguínea son. A continuación se muestra un ejemplo de cada uno de los tipos de células sanguíneas en nuestro conjunto de datos. Eosinófilo Linfocito Monocito Neutrófilo MetodologíaUtilizamos una arquitectura simple de LeNet entrenada en 281 muestras de entrenamiento con aumento de imagen. Nuestras técnicas de aumento incluyen rotaciones, cambios y acercamientos.Validamos nuestros resultados contra 71 muestras. ResultadosEn este modelo se entrenó una red convolucional usando el algortimo RMSprop, que cuenta con 3 capas convolucionales con activación tipo relu, con un pooling de 2x2. Dropout de 0.7 y se usa softmax al final para la clasificación. Este modelo clasifica entre 5 categorías que son: eosinófilo, linfocito, monocito y neutrófilo. Obteniendo resultados del 91% de presición. Code
###Code
import numpy as np
from keras.models import Sequential
from keras.layers import Dense, Dropout, Activation, Flatten
from keras.layers import Conv2D, MaxPooling2D, Lambda
from keras.layers import Dense
from keras.wrappers.scikit_learn import KerasClassifier
from keras.utils import np_utils
from keras.preprocessing.image import ImageDataGenerator
from sklearn.model_selection import cross_val_score
from sklearn.model_selection import KFold
from sklearn.preprocessing import LabelEncoder
from sklearn.pipeline import Pipeline
from sklearn.cross_validation import train_test_split
from sklearn.metrics import roc_curve, auc
import csv
import cv2
import scipy
import os
import matplotlib.pyplot as plt
num_classes = 5
epochs = 20
BASE_PATH = '../docker/'
batch_size = 32
def get_model():
model = Sequential()
model.add(Lambda(lambda x: x/127.5 - 1., input_shape=(120, 160, 3), output_shape=(120, 160, 3)))
model.add(Conv2D(32, (3, 3), input_shape=(120, 160, 3)))
model.add(Activation('relu'))
model.add(MaxPooling2D(pool_size=(2, 2)))
model.add(Conv2D(32, (3, 3)))
model.add(Activation('relu'))
model.add(MaxPooling2D(pool_size=(2, 2)))
model.add(Conv2D(64, (3, 3)))
model.add(Activation('relu'))
model.add(MaxPooling2D(pool_size=(2, 2)))
model.add(Flatten()) # this converts our 3D feature maps to 1D feature vectors
model.add(Dense(64))
model.add(Activation('relu'))
model.add(Dropout(0.5))
model.add(Dense(num_classes))
model.add(Activation('softmax'))
model.compile(loss='categorical_crossentropy',
optimizer='rmsprop',
metrics=['accuracy'])
return model
def get_filename_for_index(index):
PREFIX = 'images/BloodImage_'
num_zeros = 5 - len(index)
path = '0' * num_zeros + index
return PREFIX + path + '.jpg'
reader = csv.reader(open(BASE_PATH + 'labels.csv'))
# skip the header
next(reader)
X = []
y = []
for row in reader:
label = row[2]
if len(label) > 0 and label.find(',') == -1:
filename = get_filename_for_index(row[1])
img_file = cv2.imread(BASE_PATH + filename)
if img_file is not None:
img_file = scipy.misc.imresize(arr=img_file, size=(120, 160, 3))
img_arr = np.asarray(img_file)
# img_arr = apply_color_mask(img_arr)
X.append(img_arr)
y.append(label)
X = np.asarray(X)
y = np.asarray(y)
encoder = LabelEncoder()
encoder.fit(y)
encoded_y = encoder.transform(y)
y = np_utils.to_categorical(encoded_y)
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=10)
datagen = ImageDataGenerator(
rotation_range=20,
width_shift_range=0.2,
height_shift_range=0.2,
horizontal_flip=True
)
train_generator = datagen.flow(
X_train,
y_train,
batch_size=batch_size
)
validation_generator = datagen.flow(
X_test,
y_test,
batch_size=batch_size
)
model = get_model()
model.load_weights(BASE_PATH + 'modelo_3.h5')
print(model.summary())
###Output
_________________________________________________________________
Layer (type) Output Shape Param #
=================================================================
lambda_1 (Lambda) (None, 120, 160, 3) 0
_________________________________________________________________
conv2d_1 (Conv2D) (None, 118, 158, 32) 896
_________________________________________________________________
activation_1 (Activation) (None, 118, 158, 32) 0
_________________________________________________________________
max_pooling2d_1 (MaxPooling2 (None, 59, 79, 32) 0
_________________________________________________________________
conv2d_2 (Conv2D) (None, 57, 77, 32) 9248
_________________________________________________________________
activation_2 (Activation) (None, 57, 77, 32) 0
_________________________________________________________________
max_pooling2d_2 (MaxPooling2 (None, 28, 38, 32) 0
_________________________________________________________________
conv2d_3 (Conv2D) (None, 26, 36, 64) 18496
_________________________________________________________________
activation_3 (Activation) (None, 26, 36, 64) 0
_________________________________________________________________
max_pooling2d_3 (MaxPooling2 (None, 13, 18, 64) 0
_________________________________________________________________
flatten_1 (Flatten) (None, 14976) 0
_________________________________________________________________
dense_1 (Dense) (None, 64) 958528
_________________________________________________________________
activation_4 (Activation) (None, 64) 0
_________________________________________________________________
dropout_1 (Dropout) (None, 64) 0
_________________________________________________________________
dense_2 (Dense) (None, 5) 325
_________________________________________________________________
activation_5 (Activation) (None, 5) 0
=================================================================
Total params: 987,493
Trainable params: 987,493
Non-trainable params: 0
_________________________________________________________________
None
###Markdown
Presición
###Code
print('Predicting on test data')
y_pred = np.rint(model.predict(X_test))
from sklearn.metrics import accuracy_score
print(accuracy_score(y_test, y_pred))
###Output
0.9154929577464789
###Markdown
Imágenes mal clasificadas
###Code
mal_clasificado = [i for i,x in enumerate(y_pred) if not np.array_equal(x,y_test[i])]
img = X_test[mal_clasificado[0]]
plt.imshow(cv2.cvtColor(img, cv2.COLOR_BGR2RGB))
###Output
_____no_output_____
###Markdown
Imágenes bien clasificadas
###Code
bien_clasificado = [i for i,x in enumerate(y_pred) if np.array_equal(x,y_test[i])]
img = X_test[bien_clasificado[0]]
plt.imshow(cv2.cvtColor(img, cv2.COLOR_BGR2RGB))
###Output
_____no_output_____ |
bk/6_PyPub.ipynb | ###Markdown
Создание ROS Publisher с использованием rospyСамое время познакомиться с возможностями написания узлов на языке Python2. В качестве основы можно посмотреть офф страницу о [написании узлов](http://wiki.ros.org/rospy_tutorials/Tutorials/WritingPublisherSubscriber).Также можно добавить к полезным ссылкам [API rospy](http://docs.ros.org/api/rospy/html/) и ссылку на общее описание [Subscribers and Publishers](http://wiki.ros.org/rospy/Overview/Publishers%20and%20Subscribers).Для начала, импортируем основной модуль `rospy` и модуль сообщения типа `std_msgs/String`.
###Code
import rospy
from std_msgs.msg import String
###Output
_____no_output_____
###Markdown
После этого необходимо зарегистрировать узел в системе ROS, а также зарегистрировать топик на публикацию с указанием имени, типа сообщения для топика и размера очереди. Очередь нужна для сохранения сообщений, если узел публикует сообщения часто, при этом низкоуровневая передача сообщений работает медленнее или с задержками. При переполнении очереди отправляются наиболее актуальные данные.
###Code
rospy.init_node('talker')
pub = rospy.Publisher('my_chat_topic', String, queue_size=10)
###Output
_____no_output_____
###Markdown
> При регистрации узла функцией `init_node()` у конструктора есть флаг `anonymous`, который отключен по-умолчанию. Так при включении этого флага```pythonrospy.init_node('talker', anonymous=True)```к указанному имени узла добавляется суффикс, который делает узел уникальным в системе.> У функции `rospy.Publisher()` есть флаг `latch`, который отключен по-умолчанию. Его включение добавляет следующее поведение: при отправке сообщений в топик сохраняется последнее отправленное сообщение и когда кто-нибудь подписался на этот топик - он сразу получает последнее сообщение из этого топика, даже если отправка была раньше, чем узел подписался на топик.После остается только создать объект `Rate`, который используется для выдерживания частоты выполнения кода. В конструктор передается значение частоты в Гц.
###Code
rate = rospy.Rate(1) # 1 Hz
###Output
_____no_output_____
###Markdown
На этом подготовка и создание необходимых объектов для простейшего узла готовы и пора перейти к основной логике программы.В API ROS есть функция, которая сообщает о том, что система ROS завершила работу, именно ей и воспользуемся в качестве условия выхода `rospy.is_shutdown()`. Далее определим функцию с основной логикой узла для дальнейшего запуска.
###Code
def talker():
while not rospy.is_shutdown():
# Сформируем сообщение, которое включает время
hello_str = "hi =) %s" % rospy.get_time()
# Вывод в терминал информации (содержание сообщения)
rospy.loginfo(hello_str)
# Публикация сообщения в топик
pub.publish(hello_str)
# Сон в соответствии с выдерживаемой частотой
rate.sleep()
###Output
_____no_output_____
###Markdown
В случае с типом данных `std_msgs/String` можно просто передавать в функцию `publish()` данные сообщения. В случае с более комплексными данными рекомендуется сначала создавать объект сообщения, заполнять его и после публиковать. ```pythonmsg = String()msg.data = hello_strpub.publish(msg)``` или ```pythonmsg = String(data.hello_str)pub.publish(msg)``` После этого можем запустить функцию узла (в ней находится вся логика). При этом заворачиваем в конструкцию `try-catch`, чтобы обработать прерывание (нажатием Стоп или Ctrl+C в терминале).
###Code
try:
talker()
except (rospy.ROSInterruptException, KeyboardInterrupt):
pass
###Output
_____no_output_____ |
MixedTopics/Linear Regression.ipynb | ###Markdown
A linear mapping from input to output space Linear regression assumes that some recorded output values $y_i$ for $i=1,...,N_\mathrm{samples}$ depend linearly on some input values $\mathbf{x}_i$, and that any deviations are due to noise. Given $\mathbf{x}$-$y$ data, your task is then to rediscover the form of this linear mapping, which in the simplest case corresponds to fitting a line to the data. The question is merely how to select the best line?
###Code
# Generate example data
# Initialize
sigma = 0.5 # noise std
nSamples = 21 # number of samples
x = np.linspace(0, 5, nSamples) # input values
lineFun = lambda w0, w1, x: w0 + w1*x # linear mapping
# Generate y-data
w0 = 1 # intercept
w1 = 0.5 # slope
y = lineFun(w0, w1, x)
y += np.random.randn(nSamples)*sigma
# Plot our generated data
plt.figure(figsize=(7.5, 3))
plt.plot(x, y, 'ko')
plt.xlabel('x')
plt.ylabel('y');
###Output
_____no_output_____
###Markdown
Least squares approach One approach for evaluating how good a line fits is by summing up the squared distances to each $y$-value from the line, and to compare this value for various lines. A lower value would indicate a better fit and a higher a worse fit. Usually, this sum is further divided by the number of samples, so as to get a mean squared error (MSE).
###Code
# Calculate the MSE for a test line
w0Test = 1.0
w1Test = 0.5
yHat = lineFun(w0Test, w1Test, x) # Model predictions, the predicted line
mseFun = lambda y, yHat: np.mean((y-yHat)**2) # MSE function
mseTest = mseFun(y, yHat) # MSE value for our test line
# Plot the test line (blue), the distace to each y_i (red) and the y-values (black)
plt.figure(figsize=(7.5, 3))
for xi, yi, yHati in zip(x, y, yHat):
plt.stem([xi], [yi], 'r', bottom=yHati)
plt.plot(x, yHat, 'b-')
plt.plot(x, y, 'ko')
plt.xlabel('x')
plt.ylabel('y');
plt.title('MSE: ' + '%1.3f' % mseTest);
###Output
_____no_output_____
###Markdown
From the example above we learn that each line (a unique combination of $w_0$ and $w_1$) obtains a slightly different MSE value. Our task is therefore to find the $w_0$ and $w_1$ combination with the lowest value. Naively, we can try to do this by simply testing various combinations and plotting the MSE value as a function of both $w_0$ and $w_1$.
###Code
# Get w0 and w1 combinations over a grid
nGrid = 21
W0, W1 = np.meshgrid(np.linspace(w0-2, w0+2, nGrid), np.linspace(w1-1, w1+1, nGrid))
# Get the MSE for each combination
mseVals = np.zeros([nGrid, nGrid])
for i in range(nGrid):
for j in range(nGrid):
yHat = lineFun(W0[i, j], W1[i, j], x)
mseVals[i, j] = mseFun(y, yHat)
# Plot the surface
fig = plt.figure(figsize=(15, 5))
ax = plt.subplot(1, 2, 1, projection='3d')
ax.plot_surface(W0, W1, mseVals, cmap=cm.coolwarm, linewidth=0, antialiased=False)
ax.set_xlabel('$w_0$')
ax.set_ylabel('$w_1$')
ax.set_zlabel('MSE')
ax = plt.subplot(1, 2, 2)
ax.contourf(W0, W1, mseVals, 50, cmap=cm.coolwarm)
ax.set_xlabel('$w_0$')
ax.set_ylabel('$w_1$');
###Output
_____no_output_____
###Markdown
The take home message so far is thus that each line (unique combination of $w_0$ and $w_1$) corresponds to one point on a MSE surface, and that the best line in a MSE sense is at the bottom of the surface where the MSE minimum is located.
###Code
# Test various w0 and w1 values to see how the corresponding line fits at various locations on MSE surface
w0Test = 0.5
w1Test = 1.0
# Evaluate the MSE value for our current test line
yHat = lineFun(w0Test, w1Test, x)
mseFun = lambda y, yHat: np.mean((y-yHat)**2)
mseTest = mseFun(y, yHat)
# Plot
fig = plt.figure(figsize=(15, 5))
ax = plt.subplot(1, 2, 1)
for xi, yi, yHati in zip(x, y, yHat):
ax.stem([xi], [yi], 'r', bottom=yHati)
ax.plot(x, yHat, 'b-')
ax.plot(x, y, 'ko')
ax.set_xlabel('x')
ax.set_ylabel('y');
ax.set_title('MSE: ' + '%1.3f' % mseTest);
ax = plt.subplot(1, 2, 2)
ax.contourf(W0, W1, mseVals, 50, cmap=cm.coolwarm)
ax.set_xlabel('$w_0$')
ax.set_ylabel('$w_1$');
ax.plot(w0Test, w1Test, 'ko', ms=10);
###Output
_____no_output_____
###Markdown
How to find the minimum in practice Before moving on we need a more general description of our optimization problem, and we obtain it by describing the linear mapping using matrix notation. The line equation used above ($\hat{y}_i = w_0+w_1 x_i$) can then be written as:\begin{equation} \hat{y}_i = \mathbf{x}_i^T \mathbf{w}, \quad \text{where}: \mathbf{x}_i^T = [1, x_i] \; \text{and} \; \mathbf{w}^T = [w_0, w_1],\end{equation}and subsequently, it is possible to write the predictions for all samples as $\hat{\mathbf{y}} = \mathbf{X}\mathbf{w}$, where $\mathbf{x}_i^T$, ..., $\mathbf{x}_{N_\mathrm{samples}}^T$ make up the rows in $\mathbf{X}$. Similarly, the MSE can also be expressed with matrix notation as:\begin{equation} MSE = \frac{1}{N_\mathrm{samples}} (\mathbf{y}-\mathbf{X}\mathbf{w})^T(\mathbf{y}-\mathbf{X}\mathbf{w})\end{equation}Now, lets assume that we start with an initial guess ($\tilde{w}$) for both $w_0$ and $w_1$, and then we will try to iteratively improve it. This does, however, require a way of choosing how to iteratively change $\tilde{w}$, but we can use the gradient for this. The gradient points in the direction that a function increases the fastest. So, by moving in the opposite direction we should effectively find new values for $w_0$ and $w_1$ that correspond to a lower MSE value. We this move on to find the gradient by differentiating the MSE function with respect to $\mathbf{w}$:\begin{equation} \frac{MSE}{d\mathbf{w}} = \frac{-2}{N_\mathrm{samples}} \mathbf{X}^T(\mathbf{y}-\mathbf{X}\mathbf{w})\end{equation}The idea of moving in the opposite direction to the gradient is fundamental to all gradient based optimization techniques, and the simplest idea of just taking small steps in the opposite direction is called gradient descent.
###Code
# Plot the MSE surface first
fig = plt.figure(figsize=(7.5, 5))
plt.contourf(W0, W1, mseVals, 50, cmap=cm.coolwarm)
plt.xlabel('$w_0$')
plt.ylabel('$w_1$');
# Gradient descent
eta = 0.5e-2 # step length
wTilde = np.array([2.5, 1.25]) # initial guess
X = np.vstack([np.ones(nSamples), x]).T # create the X matrix where each row is one sample
plt.plot(wTilde[0], wTilde[1], 'o', ms=6, c=[1, 1, 1]) # Plot our initial location on the MSE surface
for i in range(10):
gradient = -2./nSamples * np.dot(X.T, y-np.dot(X, wTilde)) # Calculate the gradient
wTilde -= eta*gradient # Move in the opposite direction of the gradient
plt.plot(wTilde[0], wTilde[1], 'o', ms=6, c=[1, 1, 1]) # Plot our new location on the MSE surface
###Output
_____no_output_____
###Markdown
Gradient descent is a stupidly simple approach, but it often converges quite slowly (as seen above). Luckily, we don't actually have to use it to find our MSE optimal line. We can actually take a shortcut by remembering that the gradient is zero at a the minimum. Thus, we can solve for the optimal $w_0$ and $w_1$ values by setting the gradient to zero:\begin{align} \frac{-2}{N_\mathrm{samples}} \mathbf{X}^T(\mathbf{y}-\mathbf{X}\mathbf{w}) &= 0 \\ \mathbf{X}^T \mathbf{y} &= \mathbf{X}^T \mathbf{X}\mathbf{w}\\ (\mathbf{X}^T \mathbf{X})^{-1} \mathbf{X}^T \mathbf{y} &= \mathbf{w}\end{align}where the last line now corresponds to the classical expression for solving a linear regression problem.
###Code
# Optimal solution
wOpt = np.dot(np.dot(np.linalg.inv(np.dot(X.T, X)), X.T), y)
yHat = lineFun(wOpt[0], wOpt[1], x)
mseFun = lambda y, yHat: np.mean((y-yHat)**2)
mseTest = mseFun(y, yHat)
fig = plt.figure(figsize=(15, 5))
ax = plt.subplot(1, 2, 1)
for xi, yi, yHati in zip(x, y, yHat):
ax.stem([xi], [yi], 'r', bottom=yHati)
ax.plot(x, yHat, 'b-')
ax.plot(x, y, 'ko')
ax.set_xlabel('x')
ax.set_ylabel('y');
ax.set_title('MSE: ' + '%1.3f' % mseTest);
ax = plt.subplot(1, 2, 2)
ax.contourf(W0, W1, mseVals, 50, cmap=cm.coolwarm)
ax.set_xlabel('$w_0$')
ax.set_ylabel('$w_1$');
ax.plot(wOpt[0], wOpt[1], 'o', ms=6, c=[1, 1, 1]);
###Output
_____no_output_____
###Markdown
What if x is multi-dimensional? The general solution derived above works even if $\mathbf{x}_i$ is multi-dimensional. The only difference is that we now try to fit a plane or a hyper-plane to the data instead of just a line.
###Code
# Initialize
w0 = 0.5 # True parameter values for w0, w1, and w2
w1 = 0.5
w2 = 0.5
sigma = 1. # noise std
nGrid = 11 # x-grid resolution
X1, X2 = np.meshgrid(np.linspace(-5, 5, nGrid), np.linspace(-5, 5, nGrid)) # x1 ans x2 values on a grid
planeFun = lambda w0, w1, w2, x1, x2: w0 + w1*x1 + w2*x2 # linear mapping
# Generate noisy y-data
y = planeFun(w0, w1, w2, X1, X2).ravel()
y += np.random.randn(nGrid**2)*sigma
# Find the least squares solution
X = np.vstack([np.ones(nGrid**2), X1.ravel(), X2.ravel()]).T
wOpt = np.dot(np.dot(np.linalg.inv(np.dot(X.T, X)), X.T), y)
yHat = planeFun(wOpt[0], wOpt[1], wOpt[2], X1, X2)
# Plot the found least squares plane and the data points
fig = plt.figure(figsize=(7.5, 5))
ax = fig.add_subplot(111, projection='3d')
ax.plot(X1.ravel(), X2.ravel(), y, 'ko')
ax.plot_surface(X1, X2, yHat, cmap=cm.coolwarm, linewidth=0, antialiased=False, alpha=0.5)
ax.set_xlabel('x_1')
ax.set_ylabel('x_2')
ax.set_zlabel('y');
###Output
_____no_output_____
###Markdown
Maximum likelihood, a second approach Another approach of finding an optimal line would be to assume that each observed $y$-value consist of two parts, signal and noise, such that\begin{equation} y_i = \mathbf{x}_i^T \mathbf{w} + \epsilon_i\end{equation}where $\epsilon$ is a normally distributed random noise term with zero mean. If the noise is identical and all data points (samples) are independent, then we can score various mappings (unique $\mathbf{w}$ vectors) by how likely it is that each particular mapping would have generated the observed data. That is, the probability to observe any one single data point for the mapping $\mathbf{w}$ is\begin{equation} P(y_i|\mathbf{w}) = \frac{1}{\sqrt{2 \pi \sigma^2}} \exp \left( -\frac{(y_i - \mathbf{x}_i^T \mathbf{w})^2}{2 \sigma^2} \right),\end{equation}and subsequently, the likelihood for observing all independent data points are:\begin{equation} l(\mathbf{y}|\mathbf{w}) = \prod_i^{N_\mathrm{samples}} \frac{1}{\sqrt{2 \pi \sigma^2}} \exp \left( -\frac{(y_i - \mathbf{x}_i^T \mathbf{w})^2}{2 \sigma^2} \right).\end{equation}It is however a bit cumbersome to work with likelihoods, but luckily we can work with the simpler log-likelihood instead. Taking logs of the likelihood thus gives:\begin{align} ll(\mathbf{y}|\mathbf{w}) &= \sum_i^{N_\mathrm{samples}} \ -\frac{(y_i - \mathbf{x}_i^T \mathbf{w})^2}{2 \sigma^2} - N_\mathrm{samples} \log( \sqrt{2 \pi \sigma^2} ) \\ ll(\mathbf{y}|\mathbf{w}) &= \frac{-1}{2 \sigma^2} (\mathbf{y}-\mathbf{X}\mathbf{w})^T(\mathbf{y}-\mathbf{X}\mathbf{w}) - N_\mathrm{samples} \log( \sqrt{2 \pi \sigma^2} ) \end{align}The log-likelihood represents a function which we would like to maximize, in contrast to the MSE, as we want to find the $\mathbf{w}$ that is most likely to have generated the data. However, the gradient is zero at both a maximum and a minimum, and we can thus find the $\mathbf{w}$ at the maximum by setting the gradient of the log-likelihood function to zero.\begin{align} \frac{ll(\mathbf{y}|\mathbf{w})}{d\mathbf{w}} = \frac{-1}{\sigma^2} \mathbf{X}^T(\mathbf{y}-\mathbf{X}\mathbf{w}) = &0 \\ \frac{-1}{\sigma^2} \mathbf{X}^T(\mathbf{y}-\mathbf{X}\mathbf{w}) &= 0 \\ \mathbf{X}^T \mathbf{y} &= \mathbf{X}^T \mathbf{X}\mathbf{w}\\ (\mathbf{X}^T \mathbf{X})^{-1} \mathbf{X}^T \mathbf{y} &= \mathbf{w}\end{align}Amazingly, we can now see that the maximum likelihood solution is the same as the least squares solution. Orthogonal projections, a third approach If we go back and look at how we described our linear mapping in matrix form\begin{equation} \hat{\mathbf{y}} = \mathbf{X} \mathbf{w}\end{equation}we notice that all possible prediction vectors ($\hat{\mathbf{y}}$) live in the column space of $\mathbf{X}$, that is in the space spanned by the columns in $\mathbf{X}$. The intuitive explanation for this is that all prediction vectors are constructed as a linear combination of the columns in $\mathbf{X}$, and thus these must lie in the columns space of $\mathbf{X}$. This also means that if $\mathbf{y}$ is not in the column space of $\mathbf{X}$, then we can not describe it perfectly as a linear combination of the columns in $\mathbf{X}$. However, we can search for an orthogonal projection of $\mathbf{y}$ onto the column space, and this will represent the $\hat{\mathbf{y}}$ that is closest (euclidean norm) to $\mathbf{y}$, that is our best possible approximation. These ideas are illustrated below where the dashed black lines represent the columns of $\mathbf{X}$, the gray plane the column space, the blue line $\mathbf{y}$, the black line our orthogonal projection of $\mathbf{y}$, and the red line the error $\mathbf{y}-\hat{\mathbf{y}}$
###Code
# Example X and y selected to get a descent figure
X = np.array([[1, 1, 1],[3, 1, 0.5]]).T
y = np.array([7, 14, 1])
wOpt = np.dot(np.dot(np.linalg.inv(np.dot(X.T, X)), X.T), y)
u = np.dot(X, wOpt)
# The columns space
nGrid = 11
X1 = np.zeros([nGrid, nGrid])
X2 = np.zeros([nGrid, nGrid])
X3 = np.zeros([nGrid, nGrid])
W1, W2 = np.meshgrid(np.linspace(-5, 5, nGrid), np.linspace(-5, 5, nGrid))
for i in range(nGrid):
for j in range(nGrid):
X1[i, j] = W1[i, j]*X[0, 0] + W2[i, j]*X[0, 1]
X2[i, j] = W1[i, j]*X[1, 0] + W2[i, j]*X[1, 1]
X3[i, j] = W1[i, j]*X[2, 0] + W2[i, j]*X[2, 1]
# Plot columns vectors, the column space, y, and the orthogonal projection of y onto the column space
fig = plt.figure(figsize=(10, 7.5))
ax = fig.add_subplot(111, projection='3d')
ax.plot_surface(X1, X2, X3, color='gray', linewidth=0, antialiased=False, alpha=0.5)
scaling = 5
ax.plot([0, scaling*X[0, 0]], [0, scaling*X[1, 0]], [0, scaling*X[2, 0]], 'k--', lw=2)
ax.plot([0, scaling*X[0, 1]], [0, scaling*X[1, 1]], [0, scaling*X[2, 1]], 'k--', lw=2)
ax.plot([0, y[0]], [0, y[1]], [0, y[2]], 'k-', lw=2)
ax.plot([0, u[0]], [0, u[1]], [0, u[2]], 'b-', lw=2)
ax.plot([y[0], u[0]], [y[1], u[1]], [y[2], u[2]], 'r-', lw=2)
ax.grid(False)
ax.set_xlabel('$x_1$')
ax.set_ylabel('$x_2$')
ax.set_zlabel('$x_3$');
###Output
_____no_output_____ |
src/Chapitre3/figure/Scrip_figures.ipynb | ###Markdown
PIC data
###Code
from astropy.constants import m_e, e, k_B
k = k_B.value
me = m_e.value
q = e.value
import numpy as np
import matplotlib.pyplot as plt
import json
with open("PIC_data.dat", "r") as f:
data = json.load(f)
print(data.keys())
print(data["info"])
print("Run disponibles")
for k in ["0","1","2"]:
run = data[k]
print(k," p = ",run["p"], "mTorr")
###Output
Run disponibles
0 p = 2.0 mTorr
1 p = 0.2 mTorr
2 p = 0.001 mTorr
|
assignments2016/assignment2/.ipynb_checkpoints/BatchNormalization-checkpoint.ipynb | ###Markdown
Batch NormalizationOne way to make deep networks easier to train is to use more sophisticated optimization procedures such as SGD+momentum, RMSProp, or Adam. Another strategy is to change the architecture of the network to make it easier to train. One idea along these lines is batch normalization which was recently proposed by [3].The idea is relatively straightforward. Machine learning methods tend to work better when their input data consists of uncorrelated features with zero mean and unit variance. When training a neural network, we can preprocess the data before feeding it to the network to explicitly decorrelate its features; this will ensure that the first layer of the network sees data that follows a nice distribution. However even if we preprocess the input data, the activations at deeper layers of the network will likely no longer be decorrelated and will no longer have zero mean or unit variance since they are output from earlier layers in the network. Even worse, during the training process the distribution of features at each layer of the network will shift as the weights of each layer are updated.The authors of [3] hypothesize that the shifting distribution of features inside deep neural networks may make training deep networks more difficult. To overcome this problem, [3] proposes to insert batch normalization layers into the network. At training time, a batch normalization layer uses a minibatch of data to estimate the mean and standard deviation of each feature. These estimated means and standard deviations are then used to center and normalize the features of the minibatch. A running average of these means and standard deviations is kept during training, and at test time these running averages are used to center and normalize features.It is possible that this normalization strategy could reduce the representational power of the network, since it may sometimes be optimal for certain layers to have features that are not zero-mean or unit variance. To this end, the batch normalization layer includes learnable shift and scale parameters for each feature dimension.[3] Sergey Ioffe and Christian Szegedy, "Batch Normalization: Accelerating Deep Network Training by ReducingInternal Covariate Shift", ICML 2015.
###Code
# As usual, a bit of setup
import time
import numpy as np
import matplotlib.pyplot as plt
from cs231n.classifiers.fc_net import *
from cs231n.data_utils import get_CIFAR10_data
from cs231n.gradient_check import eval_numerical_gradient, eval_numerical_gradient_array
from cs231n.solver import Solver
%matplotlib inline
plt.rcParams['figure.figsize'] = (10.0, 8.0) # set default size of plots
plt.rcParams['image.interpolation'] = 'nearest'
plt.rcParams['image.cmap'] = 'gray'
# for auto-reloading external modules
# see http://stackoverflow.com/questions/1907993/autoreload-of-modules-in-ipython
%load_ext autoreload
%autoreload 2
def rel_error(x, y):
""" returns relative error """
return np.max(np.abs(x - y) / (np.maximum(1e-8, np.abs(x) + np.abs(y))))
# Load the (preprocessed) CIFAR10 data.
data = get_CIFAR10_data()
for k, v in data.iteritems():
print '%s: ' % k, v.shape
###Output
_____no_output_____
###Markdown
Batch normalization: ForwardIn the file `cs231n/layers.py`, implement the batch normalization forward pass in the function `batchnorm_forward`. Once you have done so, run the following to test your implementation.
###Code
# Check the training-time forward pass by checking means and variances
# of features both before and after batch normalization
# Simulate the forward pass for a two-layer network
N, D1, D2, D3 = 200, 50, 60, 3
X = np.random.randn(N, D1)
W1 = np.random.randn(D1, D2)
W2 = np.random.randn(D2, D3)
a = np.maximum(0, X.dot(W1)).dot(W2)
print 'Before batch normalization:'
print ' means: ', a.mean(axis=0)
print ' stds: ', a.std(axis=0)
# Means should be close to zero and stds close to one
print 'After batch normalization (gamma=1, beta=0)'
a_norm, _ = batchnorm_forward(a, np.ones(D3), np.zeros(D3), {'mode': 'train'})
print ' mean: ', a_norm.mean(axis=0)
print ' std: ', a_norm.std(axis=0)
# Now means should be close to beta and stds close to gamma
gamma = np.asarray([1.0, 2.0, 3.0])
beta = np.asarray([11.0, 12.0, 13.0])
a_norm, _ = batchnorm_forward(a, gamma, beta, {'mode': 'train'})
print 'After batch normalization (nontrivial gamma, beta)'
print ' means: ', a_norm.mean(axis=0)
print ' stds: ', a_norm.std(axis=0)
# Check the test-time forward pass by running the training-time
# forward pass many times to warm up the running averages, and then
# checking the means and variances of activations after a test-time
# forward pass.
N, D1, D2, D3 = 200, 50, 60, 3
W1 = np.random.randn(D1, D2)
W2 = np.random.randn(D2, D3)
bn_param = {'mode': 'train'}
gamma = np.ones(D3)
beta = np.zeros(D3)
for t in xrange(50):
X = np.random.randn(N, D1)
a = np.maximum(0, X.dot(W1)).dot(W2)
batchnorm_forward(a, gamma, beta, bn_param)
bn_param['mode'] = 'test'
X = np.random.randn(N, D1)
a = np.maximum(0, X.dot(W1)).dot(W2)
a_norm, _ = batchnorm_forward(a, gamma, beta, bn_param)
# Means should be close to zero and stds close to one, but will be
# noisier than training-time forward passes.
print 'After batch normalization (test-time):'
print ' means: ', a_norm.mean(axis=0)
print ' stds: ', a_norm.std(axis=0)
###Output
_____no_output_____
###Markdown
Batch Normalization: backwardNow implement the backward pass for batch normalization in the function `batchnorm_backward`.To derive the backward pass you should write out the computation graph for batch normalization and backprop through each of the intermediate nodes. Some intermediates may have multiple outgoing branches; make sure to sum gradients across these branches in the backward pass.Once you have finished, run the following to numerically check your backward pass.
###Code
# Gradient check batchnorm backward pass
N, D = 4, 5
x = 5 * np.random.randn(N, D) + 12
gamma = np.random.randn(D)
beta = np.random.randn(D)
dout = np.random.randn(N, D)
bn_param = {'mode': 'train'}
fx = lambda x: batchnorm_forward(x, gamma, beta, bn_param)[0]
fg = lambda a: batchnorm_forward(x, gamma, beta, bn_param)[0]
fb = lambda b: batchnorm_forward(x, gamma, beta, bn_param)[0]
dx_num = eval_numerical_gradient_array(fx, x, dout)
da_num = eval_numerical_gradient_array(fg, gamma, dout)
db_num = eval_numerical_gradient_array(fb, beta, dout)
_, cache = batchnorm_forward(x, gamma, beta, bn_param)
dx, dgamma, dbeta = batchnorm_backward(dout, cache)
print 'dx error: ', rel_error(dx_num, dx)
print 'dgamma error: ', rel_error(da_num, dgamma)
print 'dbeta error: ', rel_error(db_num, dbeta)
###Output
_____no_output_____
###Markdown
Batch Normalization: alternative backwardIn class we talked about two different implementations for the sigmoid backward pass. One strategy is to write out a computation graph composed of simple operations and backprop through all intermediate values. Another strategy is to work out the derivatives on paper. For the sigmoid function, it turns out that you can derive a very simple formula for the backward pass by simplifying gradients on paper.Surprisingly, it turns out that you can also derive a simple expression for the batch normalization backward pass if you work out derivatives on paper and simplify. After doing so, implement the simplified batch normalization backward pass in the function `batchnorm_backward_alt` and compare the two implementations by running the following. Your two implementations should compute nearly identical results, but the alternative implementation should be a bit faster.NOTE: You can still complete the rest of the assignment if you don't figure this part out, so don't worry too much if you can't get it.
###Code
N, D = 100, 500
x = 5 * np.random.randn(N, D) + 12
gamma = np.random.randn(D)
beta = np.random.randn(D)
dout = np.random.randn(N, D)
bn_param = {'mode': 'train'}
out, cache = batchnorm_forward(x, gamma, beta, bn_param)
t1 = time.time()
dx1, dgamma1, dbeta1 = batchnorm_backward(dout, cache)
t2 = time.time()
dx2, dgamma2, dbeta2 = batchnorm_backward_alt(dout, cache)
t3 = time.time()
print 'dx difference: ', rel_error(dx1, dx2)
print 'dgamma difference: ', rel_error(dgamma1, dgamma2)
print 'dbeta difference: ', rel_error(dbeta1, dbeta2)
print 'speedup: %.2fx' % ((t2 - t1) / (t3 - t2))
###Output
_____no_output_____
###Markdown
Fully Connected Nets with Batch NormalizationNow that you have a working implementation for batch normalization, go back to your `FullyConnectedNet` in the file `cs2312n/classifiers/fc_net.py`. Modify your implementation to add batch normalization.Concretely, when the flag `use_batchnorm` is `True` in the constructor, you should insert a batch normalization layer before each ReLU nonlinearity. The outputs from the last layer of the network should not be normalized. Once you are done, run the following to gradient-check your implementation.HINT: You might find it useful to define an additional helper layer similar to those in the file `cs231n/layer_utils.py`. If you decide to do so, do it in the file `cs231n/classifiers/fc_net.py`.
###Code
N, D, H1, H2, C = 2, 15, 20, 30, 10
X = np.random.randn(N, D)
y = np.random.randint(C, size=(N,))
for reg in [0, 3.14]:
print 'Running check with reg = ', reg
model = FullyConnectedNet([H1, H2], input_dim=D, num_classes=C,
reg=reg, weight_scale=5e-2, dtype=np.float64,
use_batchnorm=True)
loss, grads = model.loss(X, y)
print 'Initial loss: ', loss
for name in sorted(grads):
f = lambda _: model.loss(X, y)[0]
grad_num = eval_numerical_gradient(f, model.params[name], verbose=False, h=1e-5)
print '%s relative error: %.2e' % (name, rel_error(grad_num, grads[name]))
if reg == 0: print
###Output
_____no_output_____
###Markdown
Batchnorm for deep networksRun the following to train a six-layer network on a subset of 1000 training examples both with and without batch normalization.
###Code
# Try training a very deep net with batchnorm
hidden_dims = [100, 100, 100, 100, 100]
num_train = 1000
small_data = {
'X_train': data['X_train'][:num_train],
'y_train': data['y_train'][:num_train],
'X_val': data['X_val'],
'y_val': data['y_val'],
}
weight_scale = 2e-2
bn_model = FullyConnectedNet(hidden_dims, weight_scale=weight_scale, use_batchnorm=True)
model = FullyConnectedNet(hidden_dims, weight_scale=weight_scale, use_batchnorm=False)
bn_solver = Solver(bn_model, small_data,
num_epochs=10, batch_size=50,
update_rule='adam',
optim_config={
'learning_rate': 1e-3,
},
verbose=True, print_every=200)
bn_solver.train()
solver = Solver(model, small_data,
num_epochs=10, batch_size=50,
update_rule='adam',
optim_config={
'learning_rate': 1e-3,
},
verbose=True, print_every=200)
solver.train()
###Output
_____no_output_____
###Markdown
Run the following to visualize the results from two networks trained above. You should find that using batch normalization helps the network to converge much faster.
###Code
plt.subplot(3, 1, 1)
plt.title('Training loss')
plt.xlabel('Iteration')
plt.subplot(3, 1, 2)
plt.title('Training accuracy')
plt.xlabel('Epoch')
plt.subplot(3, 1, 3)
plt.title('Validation accuracy')
plt.xlabel('Epoch')
plt.subplot(3, 1, 1)
plt.plot(solver.loss_history, 'o', label='baseline')
plt.plot(bn_solver.loss_history, 'o', label='batchnorm')
plt.subplot(3, 1, 2)
plt.plot(solver.train_acc_history, '-o', label='baseline')
plt.plot(bn_solver.train_acc_history, '-o', label='batchnorm')
plt.subplot(3, 1, 3)
plt.plot(solver.val_acc_history, '-o', label='baseline')
plt.plot(bn_solver.val_acc_history, '-o', label='batchnorm')
for i in [1, 2, 3]:
plt.subplot(3, 1, i)
plt.legend(loc='upper center', ncol=4)
plt.gcf().set_size_inches(15, 15)
plt.show()
###Output
_____no_output_____
###Markdown
Batch normalization and initializationWe will now run a small experiment to study the interaction of batch normalization and weight initialization.The first cell will train 8-layer networks both with and without batch normalization using different scales for weight initialization. The second layer will plot training accuracy, validation set accuracy, and training loss as a function of the weight initialization scale.
###Code
# Try training a very deep net with batchnorm
hidden_dims = [50, 50, 50, 50, 50, 50, 50]
num_train = 1000
small_data = {
'X_train': data['X_train'][:num_train],
'y_train': data['y_train'][:num_train],
'X_val': data['X_val'],
'y_val': data['y_val'],
}
bn_solvers = {}
solvers = {}
weight_scales = np.logspace(-4, 0, num=20)
for i, weight_scale in enumerate(weight_scales):
print 'Running weight scale %d / %d' % (i + 1, len(weight_scales))
bn_model = FullyConnectedNet(hidden_dims, weight_scale=weight_scale, use_batchnorm=True)
model = FullyConnectedNet(hidden_dims, weight_scale=weight_scale, use_batchnorm=False)
bn_solver = Solver(bn_model, small_data,
num_epochs=10, batch_size=50,
update_rule='adam',
optim_config={
'learning_rate': 1e-3,
},
verbose=False, print_every=200)
bn_solver.train()
bn_solvers[weight_scale] = bn_solver
solver = Solver(model, small_data,
num_epochs=10, batch_size=50,
update_rule='adam',
optim_config={
'learning_rate': 1e-3,
},
verbose=False, print_every=200)
solver.train()
solvers[weight_scale] = solver
# Plot results of weight scale experiment
best_train_accs, bn_best_train_accs = [], []
best_val_accs, bn_best_val_accs = [], []
final_train_loss, bn_final_train_loss = [], []
for ws in weight_scales:
best_train_accs.append(max(solvers[ws].train_acc_history))
bn_best_train_accs.append(max(bn_solvers[ws].train_acc_history))
best_val_accs.append(max(solvers[ws].val_acc_history))
bn_best_val_accs.append(max(bn_solvers[ws].val_acc_history))
final_train_loss.append(np.mean(solvers[ws].loss_history[-100:]))
bn_final_train_loss.append(np.mean(bn_solvers[ws].loss_history[-100:]))
plt.subplot(3, 1, 1)
plt.title('Best val accuracy vs weight initialization scale')
plt.xlabel('Weight initialization scale')
plt.ylabel('Best val accuracy')
plt.semilogx(weight_scales, best_val_accs, '-o', label='baseline')
plt.semilogx(weight_scales, bn_best_val_accs, '-o', label='batchnorm')
plt.legend(ncol=2, loc='lower right')
plt.subplot(3, 1, 2)
plt.title('Best train accuracy vs weight initialization scale')
plt.xlabel('Weight initialization scale')
plt.ylabel('Best training accuracy')
plt.semilogx(weight_scales, best_train_accs, '-o', label='baseline')
plt.semilogx(weight_scales, bn_best_train_accs, '-o', label='batchnorm')
plt.legend()
plt.subplot(3, 1, 3)
plt.title('Final training loss vs weight initialization scale')
plt.xlabel('Weight initialization scale')
plt.ylabel('Final training loss')
plt.semilogx(weight_scales, final_train_loss, '-o', label='baseline')
plt.semilogx(weight_scales, bn_final_train_loss, '-o', label='batchnorm')
plt.legend()
plt.gcf().set_size_inches(10, 15)
plt.show()
###Output
_____no_output_____ |
jupyter_notebooks/bigdata/python-nbk/pythonnbk/Intro2python.ipynb | ###Markdown
Introduction to Python Python Keywords and Identifiers Python KeywordsKeywords are the reserved words in Python.We cannot use a keyword as a variable name, function name or any other identifier. They are used to define the syntax and structure of the Python language.In Python, keywords are case sensitive.There are 33 keywords in Python 3.7. This number can vary slightly over the course of time. Python IdentifiersAn identifier is a name given to entities like class, functions, variables, etc. It helps to differentiate one entity from another.Rules for writing identifiers1. Identifiers can be a combination of letters in lowercase (a to z) or uppercase (A to Z) or digits (0 to 9) or an underscore _. Names like myClass, var_1 and print_this_to_screen, all are valid example.2. An identifier cannot start with a digit. 1variable is invalid, but variable1 is a valid name.3. Keywords cannot be used as identifiers.
###Code
global = 1
###Output
_____no_output_____
###Markdown
4. We cannot use special symbols like !, @, , $, % etc. in our identifier.
###Code
a@ = 0
###Output
_____no_output_____
###Markdown
5. An identifier can be of any length.Python is a case-sensitive language. This means, `Variable` and `variable` are not the same.Always give the identifiers a name that makes sense. While `c = 10` is a valid name, writing `count = 10` would make more sense, and it would be easier to figure out what it represents when you look at your code after a long gap.Multiple words can be separated using an underscore, like `this_is_a_long_variable`. Python Statement, Indentation and Comments Python StatementInstructions that a Python interpreter can execute are called statements. For example, `a = 1` is an assignment statement. `if` statement, `for` statement, `while` statement, etc. are other kinds of statements. Multi-line statementIn Python, the end of a statement is marked by a newline character. But we can make a statement extend over multiple lines with the line continuation character (\). For example:
###Code
a = 1 + 2 + 3 + \
4 + 5 + 6 + \
7 + 8 + 9
###Output
_____no_output_____
###Markdown
This is an explicit line continuation. In Python, line continuation is implied inside parentheses `( )`, brackets `[ ]`, and braces `{ }`. For instance, we can implement the above multi-line statement as:
###Code
a = (1 + 2 + 3 +
4 + 5 + 6 +
7 + 8 + 9)
###Output
_____no_output_____
###Markdown
Here, the surrounding parentheses `( )` do the line continuation implicitly. Same is the case with `[ ]` and `{ }`. For example:
###Code
colors = ['red',
'blue',
'green']
###Output
_____no_output_____
###Markdown
We can also put multiple statements in a single line using semicolons, as follows:
###Code
a = 1; b = 2; c = 3
###Output
_____no_output_____
###Markdown
Python IndentationMost of the programming languages like C, C++, and Java use braces `{ }` to define a block of code. Python, however, uses indentation.A code block (body of a function, loop, etc.) starts with indentation and ends with the first unindented line. The amount of indentation is up to you, but it must be consistent throughout that block.Generally, four whitespaces are used for indentation and are preferred over tabs. Here is an example.
###Code
for i in range(1,11):
print(i)
if i == 5:
break
###Output
1
2
3
4
5
###Markdown
The enforcement of indentation in Python makes the code look neat and clean. This results in Python programs that look similar and consistent.Indentation can be ignored in line continuation, but it's always a good idea to indent. It makes the code more readable. For example:
###Code
if True:
print('Hello')
a = 5
###Output
Hello
###Markdown
and
###Code
if True: print('Hello'); a = 5
###Output
Hello
###Markdown
both are valid and do the same thing, but the former style is clearer.Incorrect indentation will result in `IndentationError`. Python CommentsComments are very important while writing a program. They describe what is going on inside a program, so that a person looking at the source code does not have a hard time figuring it out.You might forget the key details of the program you just wrote in a month's time. So taking the time to explain these concepts in the form of comments is always fruitful.In Python, we use the hash () symbol to start writing a comment.It extends up to the newline character. Comments are for programmers to better understand a program. Python Interpreter ignores comments.
###Code
#This is a comment
#print out Hello
print('Hello')
###Output
Hello
###Markdown
Another way of doing this is to use triple quotes, either `'''` or `"""`.These triple quotes are generally used for multi-line strings. But they can be used as a multi-line comment as well. Unless they are not docstrings, they do not generate any extra code.
###Code
"""This is also a
perfect example of
multi-line comments"""
print('Hello')
###Output
Hello
###Markdown
Docstrings in PythonA docstring is short for documentation string.Python docstrings (documentation strings) are the string literals that appear right after the definition of a function, method, class, or module.Triple quotes are used while writing docstrings. For example:
###Code
def double(num):
"""Function to double the value"""
return 2*num
###Output
_____no_output_____
###Markdown
Docstrings appear right after the definition of a function, class, or a module. This separates docstrings from multiline comments using triple quotes.The docstrings are associated with the object as their `__doc__` attribute.So, we can access the docstrings of the above function with the following lines of code:
###Code
def double(num):
"""Function to double the value"""
return 2*num
print(double.__doc__)
###Output
Function to double the value
###Markdown
Python VariablesA variable is a named location used to store data in the memory. It is helpful to think of variables as a container that holds data that can be changed later in the program. For example,
###Code
number = 10
###Output
_____no_output_____
###Markdown
Here, we have created a variable named number. We have assigned the value 10 to the variable.You can think of variables as a bag to store books in it and that book can be replaced at any time.
###Code
number = 10
number = 1.1
###Output
_____no_output_____
###Markdown
Initially, the value of `number` was `10`. Later, it was changed to `1.1`. Note: In Python, we don't actually assign values to the variables. Instead, Python gives the reference of the object(value) to the variable. Assigning values to Variables in PythonAs you can see from the above example, you can use the assignment operator `=` to assign a value to a variable.**Example 1: Declaring and assigning value to a variable**
###Code
website = "apple.com"
print(website)
###Output
apple.com
###Markdown
In the above program, we assigned a value apple.com to the variable website. Then, we printed out the value assigned to website i.e. apple.com Note: Python is a type-inferred language, so you don't have to explicitly define the variable type. It automatically knows that `apple.com` is a string and declares the `website` variable as a string. **Example 2: Changing the value of a variable**
###Code
website = "apple.com"
print(website)
# assigning a new variable to website
website = "google.com"
print(website)
###Output
apple.com
google.com
###Markdown
In the above program, we have assigned `apple.com` to the website variable initially. Then, the value is changed to `google.com`. **Example 3: Assigning multiple values to multiple variables**
###Code
a, b, c = 5, 3.2, "Hello"
print (a)
print (b)
print (c)
###Output
5
3.2
Hello
###Markdown
If we want to assign the same value to multiple variables at once, we can do this as:
###Code
x = y = z = "same"
print (x)
print (y)
print (z)
###Output
same
same
same
###Markdown
The second program assigns the `same` string to all the three variables `x`, `y` and `z`. ConstantsA constant is a type of variable whose value cannot be changed. It is helpful to think of constants as containers that hold information which cannot be changed later.You can think of constants as a bag to store some books which cannot be replaced once placed inside the bag. Assigning value to constant in PythonIn Python, constants are usually declared and assigned in a module. Here, the module is a new file containing variables, functions, etc which is imported to the main file. Inside the module, constants are written in all capital letters and underscores separating the words.**Example 3: Declaring and assigning value to a constant** Create a `constant.py`: ```pythonPI = 3.14GRAVITY = 9.8``` Create a `main.py`: ```python import constantprint(constant.PI)print(constant.GRAVITY) ``` **Output:**```python3.149.8``` In the above program, we create a constant.py module file. Then, we assign the constant value to `PI` and `GRAVITY`. After that, we create a main.py file and import the `constant` module. Finally, we print the constant value.Note: In reality, we don't use constants in Python. Naming them in all capital letters is a convention to separate them from variables, however, it does not actually prevent reassignment. Rules and Naming Convention for Variables and constants1. Constant and variable names should have a combination of letters in lowercase (**a to z**) or uppercase (**A to Z**) or digits (**0 to 9**) or an underscore (_). For example:```pythonsnake_caseMACRO_CASEcamelCaseCapWords```2. Create a name that makes sense. For example, `vowel` makes more sense than `v`.3. If you want to create a variable name having two words, use underscore to separate them. For example:```pythonmy_namecurrent_salary```4. Use capital letters possible to declare a constant. For example:```pythonPIGMASSSPEED_OF_LIGHTTEMP```5. Never use special symbols like !, @, , $, %, etc.6. Don't start a variable name with a digit. LiteralsLiteral is a raw data given in a variable or constant. In Python, there are various types of literals they are as follows: Numeric LiteralsNumeric Literals are immutable (unchangeable). Numeric literals can belong to 3 different numerical types: `Integer`, `Float`, and `Complex`. String literalsA string literal is a sequence of characters surrounded by quotes. We can use both single, double, or triple quotes for a string. And, a character literal is a single character surrounded by single or double quotes. Boolean literalsA Boolean literal can have any of the two values: `True` or `False`. Special literalsPython contains one special literal i.e. `None`. We use it to specify that the field has not been created. Literal CollectionsThere are four different literal collections List literals, Tuple literals, Dict literals, and Set literals.**How to use literals collections in Python?**
###Code
fruits = ["apple", "mango", "orange"] #list
numbers = (1, 2, 3) #tuple
alphabets = {'a':'apple', 'b':'ball', 'c':'cat'} #dictionary
vowels = {'a', 'e', 'i' , 'o', 'u'} #set
print(fruits)
print(numbers)
print(alphabets)
print(vowels)
###Output
['apple', 'mango', 'orange']
(1, 2, 3)
{'a': 'apple', 'b': 'ball', 'c': 'cat'}
{'i', 'a', 'o', 'u', 'e'}
###Markdown
In the above program, we created a `list of fruits`, a `tuple of numbers`, a `dictionary dict` having values with keys designated to each value and a `set of vowels`. Data types in PythonEvery value in Python has a datatype. Since everything is an object in Python programming, data types are actually classes and variables are instance (object) of these classes. Python NumbersIntegers, floating point numbers and complex numbers fall under Python numbers category. They are defined as `int`, `float` and `complex` classes in Python.We can use the `type()` function to know which class a variable or a value belongs to. Similarly, the `isinstance()` function is used to check if an object belongs to a particular class.
###Code
a = 5
print(a, "is of type", type(a))
a = 2.0
print(a, "is of type", type(a))
a = 1+2j
print(a, "is complex number?", isinstance(1+2j,complex))
###Output
5 is of type <class 'int'>
2.0 is of type <class 'float'>
(1+2j) is complex number? True
###Markdown
Python ListList is an ordered sequence of items. It is one of the most used datatype in Python and is very flexible. All the items in a list do not need to be of the same type.Declaring a list is pretty straight forward. Items separated by commas are enclosed within brackets `[ ]`.
###Code
a = [1, 2.2, 'python']
###Output
_____no_output_____
###Markdown
We can use the slicing operator `[ ]` to extract an item or a range of items from a list. The index starts from 0 in Python.
###Code
a = [5,10,15,20,25,30,35,40]
# a[2] = 15
print("a[2] = ", a[2])
# a[0:3] = [5, 10, 15]
print("a[0:3] = ", a[0:3])
# a[5:] = [30, 35, 40]
print("a[5:] = ", a[5:])
###Output
a[2] = 15
a[0:3] = [5, 10, 15]
a[5:] = [30, 35, 40]
###Markdown
Lists are mutable, meaning, the value of elements of a list can be altered.
###Code
a = [1, 2, 3]
a[2] = 4
print(a)
###Output
[1, 2, 4]
###Markdown
Python TupleTuple is an ordered sequence of items same as a list. The only difference is that tuples are immutable. Tuples once created cannot be modified.Tuples are used to write-protect data and are usually faster than lists as they cannot change dynamically.It is defined within parentheses `()` where items are separated by commas.
###Code
t = (5,'program', 1+3j)
###Output
_____no_output_____
###Markdown
We can use the slicing operator `[]` to extract items but we cannot change its value.
###Code
t = (5,'program', 1+3j)
# t[1] = 'program'
print("t[1] = ", t[1])
# t[0:3] = (5, 'program', (1+3j))
print("t[0:3] = ", t[0:3])
# Generates error
# Tuples are immutable
t[0] = 10
###Output
t[1] = program
t[0:3] = (5, 'program', (1+3j))
###Markdown
Python StringsString is sequence of Unicode characters. We can use single quotes or double quotes to represent strings. Multi-line strings can be denoted using triple quotes, `'''` or `"""`.
###Code
s = "This is a string"
print(s)
s = '''A multiline
string'''
print(s)
###Output
This is a string
A multiline
string
###Markdown
Just like a list and tuple, the slicing operator `[ ]` can be used with strings. Strings, however, are **immutable**.
###Code
s = 'Hello world!'
# s[4] = 'o'
print("s[4] = ", s[4])
# s[6:11] = 'world'
print("s[6:11] = ", s[6:11])
# Generates error
# Strings are immutable in Python
s[5] ='d'
###Output
s[4] = o
s[6:11] = world
###Markdown
Python SetSet is an unordered collection of unique items. Set is defined by values separated by comma inside braces `{ }`. Items in a set are not ordered.
###Code
a = {5,2,3,1,4}
# printing set variable
print("a = ", a)
# data type of variable a
print(type(a))
###Output
a = {1, 2, 3, 4, 5}
<class 'set'>
###Markdown
We can perform set operations like union, intersection on two sets. Sets have unique values. They eliminate duplicates.
###Code
a = {1,2,2,3,3,3}
print(a)
###Output
{1, 2, 3}
###Markdown
Since, set are unordered collection, indexing has no meaning. Hence, the slicing operator `[]` **does not work**. Python DictionaryDictionary is an unordered collection of key-value pairs.It is generally used when we have a huge amount of data. Dictionaries are optimized for retrieving data. We must know the key to retrieve the value.In Python, dictionaries are defined within braces `{}` with each item being a pair in the form `key:value`. Key and value can be of any type.
###Code
d = {1:'value','key':2}
type(d)
###Output
_____no_output_____
###Markdown
We use key to retrieve the respective value. But not the other way around.
###Code
d = {1:'value','key':2}
print(type(d))
print("d[1] = ", d[1]);
print("d['key'] = ", d['key']);
# Generates error
print("d[2] = ", d[2]);
###Output
<class 'dict'>
d[1] = value
d['key'] = 2
###Markdown
Conversion between data typesWe can convert between different data types by using different type conversion functions like `int()`, `float()`, `str()`, etc. Type ConversionThe process of converting the value of one data type (integer, string, float, etc.) to another data type is called type conversion. Python has two types of type conversion.1. Implicit Type Conversion2. Explicit Type Conversion**Implicit Type Conversion**In Implicit type conversion, Python automatically converts one data type to another data type. This process doesn't need any user involvement.Let's see an example where Python promotes the conversion of the lower data type (integer) to the higher data type (float) to avoid data loss.**Example 1: Converting integer to float**
###Code
num_int = 123
num_flo = 1.23
num_new = num_int + num_flo
print("datatype of num_int:",type(num_int))
print("datatype of num_flo:",type(num_flo))
print("Value of num_new:",num_new)
print("datatype of num_new:",type(num_new))
###Output
datatype of num_int: <class 'int'>
datatype of num_flo: <class 'float'>
Value of num_new: 124.23
datatype of num_new: <class 'float'>
###Markdown
Now, let's try adding a string and an integer, and see how Python deals with it.**Example 2: Addition of string(higher) data type and integer(lower) datatype**
###Code
num_int = 123
num_str = "456"
print("Data type of num_int:",type(num_int))
print("Data type of num_str:",type(num_str))
print(num_int+num_str)
###Output
Data type of num_int: <class 'int'>
Data type of num_str: <class 'str'>
###Markdown
In the above program,* We add two variables `num_int` and `num_str`.* As we can see from the output, we got TypeError. Python is not able to use Implicit Conversion in such conditions.* However, Python has a solution for these types of situations which is known as Explicit Conversion.**Explicit Type Conversion**In Explicit Type Conversion, users convert the data type of an object to required data type. We use the predefined functions like `int()`, `float()`, `str()`, etc to perform explicit type conversion.This type of conversion is also called typecasting because the user casts (changes) the data type of the objects.Syntax :```python(expression)``` Typecasting can be done by assigning the required data type function to the expression.**Example 3: Addition of string and integer using explicit conversion**
###Code
num_int = 123
num_str = "456"
print("Data type of num_int:",type(num_int))
print("Data type of num_str before Type Casting:",type(num_str))
num_str = int(num_str)
print("Data type of num_str after Type Casting:",type(num_str))
num_sum = num_int + num_str
print("Sum of num_int and num_str:",num_sum)
print("Data type of the sum:",type(num_sum))
###Output
Data type of num_int: <class 'int'>
Data type of num_str before Type Casting: <class 'str'>
Data type of num_str after Type Casting: <class 'int'>
Sum of num_int and num_str: 579
Data type of the sum: <class 'int'>
###Markdown
Note + Type Conversion is the conversion of object from one data type to another data type.+ Implicit Type Conversion is automatically performed by the Python interpreter.+ Python avoids the loss of data in Implicit Type Conversion.+ Explicit Type Conversion is also called Type Casting, the data types of objects are converted using predefined functions by the user.+ In Type Casting, loss of data may occur as we enforce the object to a specific data type. Python Input, Output and Import Python Output Using print() functionWe use the `print()` function to output data to the standard output device (screen). We can also output data to a file.Examples:
###Code
print('This sentence is output to the screen')
a = 5
print('The value of a is', a)
###Output
The value of a is 5
###Markdown
Output formattingSometimes we would like to format our output to make it look attractive. This can be done by using the `str.format()` method. This method is visible to any string object.
###Code
x = 5; y = 10
print('The value of x is {} and y is {}'.format(x,y))
print('I love {0} and {1}'.format('bread','butter'))
print('I love {1} and {0}'.format('bread','butter'))
###Output
I love bread and butter
I love butter and bread
###Markdown
We can even use keyword arguments to format the string.
###Code
print('Hello {name}, {greeting}'.format(greeting = 'Goodmorning', name = 'John'))
###Output
Hello John, Goodmorning
###Markdown
Python InputUp until now, our programs were static. The value of variables was defined or hard coded into the source code.To allow flexibility, we might want to take the input from the user. In Python, we have the `input()` function to allow this. The syntax for `input()` is:```pythoninput([prompt])``` where `prompt` is the string we wish to display on the screen. It is optional.
###Code
num = input('Enter a number: ')
num
###Output
_____no_output_____
###Markdown
Here, we can see that the entered value `10` is a string, not a number. To convert this into a number we can use `int()` or `float()` functions.
###Code
int('10')
float('10')
###Output
_____no_output_____
###Markdown
This same operation can be performed using the `eval()` function. But `eval` takes it further. It can evaluate even expressions, provided the input is a string
###Code
int('2+3')
eval('2+3')
###Output
_____no_output_____
###Markdown
Python ImportWhen our program grows bigger, it is a good idea to break it into different modules.A module is a file containing Python definitions and statements. Python modules have a filename and end with the extension `.py`.Definitions inside a module can be imported to another module or the interactive interpreter in Python. We use the `import` keyword to do this.For example, we can import the `math` module by typing the following line:
###Code
import math
print(math.pi)
###Output
3.141592653589793
###Markdown
While importing a module, Python looks at several places defined in `sys.path`. It is a list of directory locations.
###Code
import sys
sys.path
###Output
_____no_output_____
###Markdown
Operators in pythonOperators are special symbols in Python that carry out arithmetic or logical computation. The value that the operator operates on is called the operand.For example:
###Code
2+3
###Output
_____no_output_____
###Markdown
Here, `+` is the operator that performs addition. `2` and `3` are the operands and `5` is the output of the operation. Arithmetic operators in PythonArithmetic operators are used to perform mathematical operations like addition, subtraction, multiplication, etc.
###Code
x = 15
y = 4
# Output: x + y = 19
print('x + y =',x+y)
# Output: x - y = 11
print('x - y =',x-y)
# Output: x * y = 60
print('x * y =',x*y)
# Output: x / y = 3.75
print('x / y =',x/y)
# Output: x // y = 3
print('x // y =',x//y)
# Output: x ** y = 50625
print('x ** y =',x**y)
###Output
x + y = 19
x - y = 11
x * y = 60
x / y = 3.75
x // y = 3
x ** y = 50625
###Markdown
Comparison operatorsComparison operators are used to compare values. It returns either `True` or `False` according to the condition.
###Code
x = 10
y = 12
# Output: x > y is False
print('x > y is',x>y)
# Output: x < y is True
print('x < y is',x<y)
# Output: x == y is False
print('x == y is',x==y)
# Output: x != y is True
print('x != y is',x!=y)
# Output: x >= y is False
print('x >= y is',x>=y)
# Output: x <= y is True
print('x <= y is',x<=y)
###Output
x > y is False
x < y is True
x == y is False
x != y is True
x >= y is False
x <= y is True
###Markdown
Logical operatorsLogical operators are the `and`, `or`, `not` operators.
###Code
x = True
y = False
print('x and y is',x and y)
print('x or y is',x or y)
print('not x is',not x)
###Output
x and y is False
x or y is True
not x is False
###Markdown
Bitwise operatorsBitwise operators act on operands as if they were strings of binary digits. They operate bit by bit, hence the name.For example, `2` is `10` in binary and `7` is `111`. Assignment operatorsAssignment operators are used in Python to assign values to variables.`a = 5` is a simple assignment operator that assigns the value 5 on the right to the variable a on the left.There are various compound operators in Python like `a += 5` that adds to the variable and later assigns the same. It is equivalent to `a = a + 5`. Special operatorsPython language offers some special types of operators like the identity operator or the membership operator. They are described below with examples. Identity operators`is` and `is not` are the identity operators in Python. They are used to check if two values (or variables) are located on the same part of the memory. Two variables that are equal does not imply that they are identical.
###Code
x1 = 5
y1 = 5
x2 = 'Hello'
y2 = 'Hello'
x3 = [1,2,3]
y3 = [1,2,3]
# Output: False
print(x1 is not y1)
# Output: True
print(x2 is y2)
# Output: False
print(x3 is y3)
###Output
False
True
False
###Markdown
Membership operators`in` and `not in` are the membership operators in Python. They are used to test whether a value or variable is found in a sequence (string, list, tuple, set and dictionary).In a dictionary we can only test for presence of key, not the value.
###Code
x = 'Hello world'
y = {1:'a',2:'b'}
# Output: True
print('H' in x)
# Output: True
print('hello' not in x)
# Output: True
print(1 in y)
# Output: False
print('a' in y)
###Output
True
True
True
False
###Markdown
Python if...else StatementDecision making is required when we want to execute a code only if a certain condition is satisfied.The `if…elif…else` statement is used in Python for decision making.**Python if Statement Syntax**```pythonif test expression: statement(s)```
###Code
# If the number is positive, we print an appropriate message
num = 3
if num > 0:
print(num, "is a positive number.")
print("This is always printed.")
num = -1
if num > 0:
print(num, "is a positive number.")
print("This is also always printed.")
###Output
3 is a positive number.
This is always printed.
This is also always printed.
###Markdown
Python if...else Statement**Syntax of if...else**```pythonif test expression: Body of ifelse: Body of else```
###Code
# Program checks if the number is positive or negative
# And displays an appropriate message
num = 3
# Try these two variations as well.
# num = -5
# num = 0
if num >= 0:
print("Positive or Zero")
else:
print("Negative number")
###Output
Positive or Zero
###Markdown
Python if...elif...else Statement**Syntax of if...elif...else**```pythonif test expression: Body of ifelif test expression: Body of elifelse: Body of else```
###Code
'''In this program,
we check if the number is positive or
negative or zero and
display an appropriate message'''
num = 3.4
# Try these two variations as well:
# num = 0
# num = -4.5
if num > 0:
print("Positive number")
elif num == 0:
print("Zero")
else:
print("Negative number")
###Output
Positive number
###Markdown
Python Nested if statementsWe can have a `if...elif...else` statement inside another `if...elif...else` statement. This is called nesting in computer programming.Any number of these statements can be nested inside one another. Indentation is the only way to figure out the level of nesting. They can get confusing, so they must be avoided unless necessary.
###Code
'''In this program, we input a number
check if the number is positive or
negative or zero and display
an appropriate message
This time we use nested if statement'''
num = float(input("Enter a number: "))
if num >= 0:
if num == 0:
print("Zero")
else:
print("Positive number")
else:
print("Negative number")
'''In this program, we input a number
check if the number is positive or
negative or zero and display
an appropriate message
This time we use nested if statement'''
num = float(input("Enter a number: "))
if num >= 0:
if num == 0:
print("Zero")
else:
print("Positive number")
else:
print("Negative number")
'''In this program, we input a number
check if the number is positive or
negative or zero and display
an appropriate message
This time we use nested if statement'''
num = float(input("Enter a number: "))
if num >= 0:
if num == 0:
print("Zero")
else:
print("Positive number")
else:
print("Negative number")
###Output
Enter a number: 0
Zero
###Markdown
Python for LoopThe for loop in Python is used to iterate over a sequence (list, tuple, string) or other iterable objects. Iterating over a sequence is called traversal.**Syntax of for Loop**```pythonfor val in sequence: Body of for```Here, `val` is the variable that takes the value of the item inside the sequence on each iteration.Loop continues until we reach the last item in the sequence. The body of for loop is separated from the rest of the code using indentation.
###Code
# Program to find the sum of all numbers stored in a list
# List of numbers
numbers = [6, 5, 3, 8, 4, 2, 5, 4, 11]
# variable to store the sum
sum = 0
# iterate over the list
for val in numbers:
sum = sum+val
print("The sum is", sum)
###Output
The sum is 48
###Markdown
The range() functionWe can generate a sequence of numbers using `range()` function. `range(10)` will generate numbers from 0 to 9 (10 numbers).We can also define the start, stop and step size as `range(start, stop,step_size)`. step_size defaults to 1 if not provided.The `range` object is "lazy" in a sense because it doesn't generate every number that it "contains" when we create it. However, it is not an iterator since it supports `in`, `len` and `__getitem__` operations.This function does not store all the values in memory; it would be inefficient. So it remembers the start, stop, step size and generates the next number on the go.To force this function to output all the items, we can use the function `list()`.example:
###Code
print(range(10))
print(list(range(10)))
print(list(range(2, 8)))
print(list(range(2, 20, 3)))
###Output
range(0, 10)
[0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
[2, 3, 4, 5, 6, 7]
[2, 5, 8, 11, 14, 17]
###Markdown
We can use the `range()` function in `for` loops to iterate through a sequence of numbers. It can be combined with the `len()` function to iterate through a sequence using indexing.
###Code
# Program to iterate through a list using indexing
genre = ['pop', 'rock', 'jazz']
# iterate over the list using index
for i in range(len(genre)):
print("I like", genre[i])
###Output
I like pop
I like rock
I like jazz
###Markdown
for loop with elseA `for` loop can have an optional `else` block as well. The `else` part is executed if the items in the sequence used in for loop exhausts.The `break` keyword can be used to stop a for loop. In such cases, the else part is ignored.Hence, a for loop's else part runs if no break occurs.
###Code
digits = [0, 1, 5]
for i in digits:
print(i)
else:
print("No items left.")
# program to display student's marks from record
student_name = 'Soyuj'
marks = {'James': 90, 'Jules': 55, 'Arthur': 77}
for student in marks:
if student == student_name:
print(marks[student])
break
else:
print('No entry with that name found.')
###Output
No entry with that name found.
|
examples/ensure/example.ipynb | ###Markdown
Table of Contents1 Add runtime dependencies2 Add dev dependencies3 Ensure3.1 Check the requirements3.2 Run the ensure command Add runtime dependencies Clear in case there are some leftovers (optional)
###Code
%dep clear
%dep add pandas --version ">=0.24.0"
%dep add plotly
%dep add sklearn
%dep add pandas-profiling
###Output
_____no_output_____
###Markdown
Add dev dependencies
###Code
%dep add --dev black
###Output
_____no_output_____
###Markdown
EnsureEnsure does all of the following:- create a virtual environment- install requirements into the environment- create and loads a new Jupyter kernel- sets the new kernel Check the requirements
###Code
%requirements
###Output
_____no_output_____
###Markdown
Run the ensure command
###Code
%dep ensure --engine pipenv
###Output
_____no_output_____ |
Demo_Notebooks/.ipynb_checkpoints/OpSeF_IV_Setup_Advanced_User_Mask_Cluster_Plot_CSV_toFiji_0001_SkeletalMuscle_2Ch-checkpoint.ipynb | ###Markdown
General description of OpSeFThe analysis pipeline consists of four principle sets of functions to import and reshape the data, to pre-process it, to segment it and to analyze and classify results.OpSeF is designed such that parameter for pre-processing and selection of the ideal model for segmentation are one functional unit. The first three sets of functions are explained in the OpSeF_IV_Setup_Basic_0001_Skeletal_Muscle Demo Notebook.This notebook illustrates the following post-processing options:- Plot Results- Export to CSV- Cluster Analysis- Export to Fiji Description of the skeletal muscle datasetA methylen blue stained skeletal muscle was used as test image for nuclear segmentation.To this aim 2048 x 2048 pixel large patches were extracted.Importantly, these patches contain border of multiple images that were stiched together after image aquisition at a slidescanner. Load Core-Settings that shall not be changedPlease use OpSef_Configure_XXX to change these global settings.Changes in this file only necessary to intergrate new model,or to change the aut-ogenerated folderstructure.Changes to the folderstructure might cause errors.
###Code
file_path = "./my_runs/main_settings.pkl"
infile = open(file_path,'rb')
parameter = pickle.load(infile)
print("Loading processing pipeline from",file_path)
infile.close()
model_dic,folder_structure = parameter
###Output
Loading processing pipeline from ./my_runs/main_settings.pkl
###Markdown
Define General Parametermost parameter define the overall processing and likeley do not change between runs
###Code
# Define variables that determine the processing pipeline and (generally) do not change between runs
pc = {}
#################
## Basic ########
#################
pc["sub_f"] = folder_structure # these folder will be auto-generated
pc["batch_size"] = 2 # the number of images to be quantified must be a multiple of batch size (for segmentation)
# extract the properties (below) from region_props
pc["get_property"] = ["label","area","centroid", "eccentricity",
"equivalent_diameter","mean_intensity","max_intensity",
"min_intensity","perimeter"]
pc["naming_scheme"] = "Simple" # or "Simple" Export_ZSplit" to create substacks
pc["toFiji"] = True # Shall images be prepared for import in Fiji
###############################
# Define use of second channel
###############################
pc["export_another_channel"] = True # export other channel (to create a mask or for quantification) ?
if ["export_another_channel"]:
pc["create_filter_mask_from_channel"] = True # use second channel to make a mask?
pc["Quantify_2ndCh"] = False # shall this channel be quantified?
if pc["Quantify_2ndCh"]:
pc["merge_results"] = True # shall the results of the two intensity quantification be merged
# (needed for advanced plotting)
pc["plot_merged"] = True # plot head of dataframe in notebook ?
################################
# Define Analysis & Plotting ###
################################
pc["Export_to_CSV"] = True # shall results be exported to CSV (usually only true for the final run)
if pc["Export_to_CSV"]:
pc["Intensity_Ch"] = 0 # put 999 if data contains only one channel
pc["Plot_Results"] = True # Do you want to plot results ?
pc["Plot_xy"] = [["area","mean_intensity"],["area","circularity"]] # Define what you want to plot (x/y)
pc["plot_head_main"] = True # plot head of dataframe in notebook ?
pc["Do_ClusterAnalysis"] = True # shall cluster analysis be performed?
if pc["Do_ClusterAnalysis"]: # Define (below) which values will be included in the TSNE:
pc["Cluster_How"] = "Mask" # or "Mask"
if pc["Cluster_How"] == "Auto":
pc["cluster_expected"] = 2 # How many groups/classes do you expected?
pc["include_in_tsne"] = ["area","eccentricity","equivalent_diameter",
"mean_intensity","max_intensity","min_intensity","perimeter"]
pc["tSNE_learning_rate"] = 100 # Define learning rate
pc["link_method"] = "ward" # or "average", or "complete", or "single" details see below
else:
pc["Cluster_How"] = "Not"
else:
pc["Plot_Results"] = False
pc["Do_ClusterAnalysis"] = False
pc["Cluster_How"] = "Not"
# Define here input & basic processing that (generally) does not change between runs
input_def = {}
input_def["root"] = "/home/trasse/Desktop/MLTestData/SlideScanner_muscle_2Ch" # define folder where images are located
input_def["dataset"] = "muscle_mask" # give the dataset a common name
input_def["mydtype"] = np.uint8 # bit depth of input images
input_def["input_type"] = ".tif" # or .tif
if input_def["input_type"] == ".tif":
input_def["is3D"] = False # is the data 3D or 2D ???
if pc["export_another_channel"]:
input_def["export_seg_ch"] = "_Ch00" # give unique string to identify the channel
elif input_def["input_type"] == ".lif":
input_def["rigth_size"] = (2048,2048)
input_def["export_single_ch"] = 99 # which channel to extract from the lif file (99 if only one)
input_def["split_z"] = False # chose here to split z-stack into multiple substacts to avoid that cells fuse after projection
if input_def["split_z"]:# choosen, define:
input_def["z_step"] = 3 # size of substacks
if input_def["input_type"] == ".lif":
input_def["export_multiple_ch"] = [0,1] # channels to be exported
#########################################################################
## the following options are only implemented with Tiff files as input ##
#########################################################################
input_def["toTiles"] = False
if input_def["toTiles"]:
input_def["patch_size"] = (15,512,512)
# run5
input_def["bin"] = False
if input_def["bin"]:
input_def["bin_factor"] = 2 # same for x/y
# coming soon...
input_def["n2v"] = False
input_def["CARE"] = False
# Define parameter for export (if needed)
if pc["export_another_channel"]:
input_def["post_export_single_ch"] = 1 # which channel to extract from the lif file
input_def["post_subset"] = ["_Ch01"] # analyse these intensity images if exporting from lif
# use also to search for the right channel if exporting
# from tiff, works only if subset for main channel is "All"
if pc["Quantify_2ndCh"]:
pc["Intensity_2ndCh"] = input_def["post_export_single_ch"]
# Define model
# in this dictionary all settings for the model are stored
initModelSettings = {}
# Variables U-Net Cellprofiler
initModelSettings["UNet_model_file_CP01"] = "./model_Unet/UNet_CP001.h5"
initModelSettings["UNetShape"] = (2048,2048) # run1,2
initModelSettings["UNetShape"] = (1024,1024) # run 3
initModelSettings["UNetSettings"] = [{"activation": "relu", "padding": "same"},{ "momentum": 0.9}]
# Variables StarDist
initModelSettings["basedir_StarDist"] = "./model_stardist"
# Variables Cellpose
initModelSettings["Cell_Channels"] = [[0,0],[0,0]]
###Output
_____no_output_____
###Markdown
Define Post-ProcessingSegmented objects can be filtered by their region propperties or a mask, results might be exported to AnnotatorJ and re-imported for further analysis. Blue arrows define the default processing pipeline, grey arrows feature available options. Dark Blue boxes are core components, light blue boxes are optional processing steps.
###Code
# Define variable that might change in each run
run_def = {}
run_def["display_base"] = "000" # defines the image used as basis for the overlay. See documemtation for details.
run_def["run_ID"] = "003" #give each run a new ID (unless you want to overwrite the old data)
run_def["clahe_prm"] = [(18,18),3] # Parameter for CLAHE
# Run 5
input_def["subset"] = ["All"] # filter by name
#########################
# Define preprocessing ##
#########################
run_def["pre_list"] = [["Median",3,0,"Max",False,run_def["clahe_prm"],"no",True]] # run 5
# For Cellpose
run_def["rescale_list"] = [0.6] # run 5
# Define model
run_def["ModelType"] = ["CP_nuclei"] # run 5
############################################################
# Define postprocessing & filtering #
# keep only the objects within the defined ranges #
###########################################################
# (same for all runs)
run_def["filter_para"] = {}
run_def["filter_para"]["area"] = [0,10000]
run_def["filter_para"]["perimeter"] = [0,99999999]
run_def["filter_para"]["circularity"] = [0,1] # (equivalent_diameter * math.pi) / perimeter
run_def["filter_para"]["mean_intensity"] = [0,65535]
run_def["filter_para"]["sum_intensity"] = [0,100000000000000]
run_def["filter_para"]["eccentricity"] = [0,10]
############################################################################
# settings that are only needed if condition below is met which means you #
# plan to use a mask from a second channel to filter results #
############################################################################
if pc["create_filter_mask_from_channel"]:
run_def["binary_filter_mp"] = [["open",5,2,1,"Morphology"],["close",5,3,1,"Morphology"],["erode",5,1,1,"Morphology"]]
run_def["para_mp"] = [["Mean",5],[0.6],run_def["binary_filter_mp"]]
run_def["Use User Mask"] = True
###Output
_____no_output_____
###Markdown
How to reproduce these results?The notebook is set up to reproduce the results of the last run (Run 4)The execute previous runs please delete or commented out settings used for Run 4 Settings are saved in a .pkl file.The next cell prints the filepath & name of this file.OpSef_Run_XXX loads the settings specified above and processed all images.The only change you have to make within OpSef_Run_XXX is specifying the locationof this setting file. Save Parameter
###Code
# auto-create parameter set from input above
run_def["run_now_list"] = [model_dic[x] for x in run_def["ModelType"]]
parameter = [pc,input_def,run_def,initModelSettings]
# save it
file_name = "./my_runs/Parameter_{}_Run_{}.pkl".format(input_def["dataset"],run_def["run_ID"])
file_name_load = "./Demo_Notebooks/my_runs/Parameter_{}_Run_{}.pkl".format(input_def["dataset"],run_def["run_ID"])
print("Please execute this file with OPsef_Run_XXX",file_name_load)
outfile = open(file_name,'wb')
pickle.dump(parameter,outfile)
outfile.close()
###Output
Please execute this file with OPsef_Run_XXX ./Demo_Notebooks/my_runs/Parameter_muscle_mask_Run_003.pkl
###Markdown
Documentation
###Code
##########################
## Folderstructure ####
input_def["root"] = "/home/trasse/OpSefDemo/leaves" # defines the main folder
# Put files in these subfolder
# .lif
# root/myimage_container.lif
# root/tiff/myimage1.tif (in case this folder is the direct input to the pre-processing pipeline)
# /myimage2.tif ...
# or
# root/tiff_raw_2D/myimage1.tif (if you want to make patches in 2D)
# root/tiff_to_split/myimage1.tif (if you want ONLY create substacts, bt not BIN or patch before)
# root/tiff_raw/myimage1.tif (for all pipelines that start with patching or binning and use stacks)
######################################
### What is a display base image ????
######################################
run_def["display_base"]
'''
display base is ideally set to "same".
in this case the visualiation of segmentation border will be
drawn on top of the input image to the segmentation
If this behavior is not desired a three digit number
that refers to the position in the run_def["pre_list"] might to be entered.
example:
run_def["pre_list"] = [["Median",3,8,"Sum",True,clahe_prm],["Mean",5,3,"Max",True,clahe_prm]]
& the image resulting from:
["Mean",5,3,"Max",True,clahe_prm]
shall be used as basis for display:
then set:
run_def["display_base"] = "001"
'''
##########################
# Parameter for Cellpose:
##########################
# Define:
# initModelSettings["Cell_Channels"]
# to run segementation on grayscale=0, R=1, G=2, B=3
# initModelSettings["Cell_Channels"] = [cytoplasm, nucleus]
# if NUCLEUS channel does not exist, set the second channel to 0
initModelSettings["Cell_Channels"] = [[0,0],[0,0]]
# IF ALL YOUR IMAGES ARE THE SAME TYPE, you can give a list with 2 elements
initModelSettings["Cell_Channels"] = [0,0] # IF YOU HAVE GRAYSCALE
initModelSettings["Cell_Channels"] = [2,3] # IF YOU HAVE G=cytoplasm and B=nucleus
initModelSettings["Cell_Channels"] = [2,1] # IF YOU HAVE G=cytoplasm and R=nucleus
# if rescale is set to None, the size of the cells is estimated on a per image basis
# if you want to set the size yourself, set it to 30. / average_cell_diameter
#####################################
## Variables for Preprocessing
#####################################
## The list
run_def["pre_list"]
#is organized as such:
# (1) Filter type
# (2) Kernel
# (3) substract fixed value
# (4) projection type
# (5) calhe enhance (Yes/No) as defined above
# (6) Calhe parameter
# (7) enhance edges (and how) (no, roberts, sobel)
# (8) invert image
# It is a list of lists, each entry defines one pre-processing pipeline:
# e.g.
# Run1
run_def["pre_list"] = [["Median",3,50,"Max",False,run_def["clahe_prm"],"no",False],
["Median",3,50,"Max",True,run_def["clahe_prm"],"no",False],
["Median",3,50,"Max",False,run_def["clahe_prm"],"sobel",False],
["Median",3,50,"Max",False,run_def["clahe_prm"],"no",True]]
###Output
_____no_output_____ |
week_7/.ipynb_checkpoints/Week 7 - Python-Pandas-Practice-checkpoint.ipynb | ###Markdown
Python | Pandas DataFrame What is Pandas? pandas is a software library written for the Python programming language for data manipulation and analysis. In particular, it offers data structures and operations for manipulating numerical tables and time series. What is a Pandas DataFrame? Pandas DataFrame is two-dimensional size-mutable, potentially heterogeneous tabular data structure with labeled axes (rows and columns). A Data frame is a two-dimensional data structure, i.e., data is aligned in a tabular fashion in rows and columns. Pandas DataFrame consists of three principal components, the data, rows, and columns. A Pandas DataFrame will be created by loading the datasets from existing storage. Storage can be SQL Database, CSV file, and Excel file. Pandas DataFrame can be created from the lists, dictionary, and from a list of dictionary etc.Dataframe can be created in different ways here are some ways by which we create a dataframe: Creating a dataframe using List:
###Code
# import pandas as pd
import pandas as pd
# list of strings
lyst = ['CSC', '102', 'is', 'the', 'best', 'course', 'ever']
# Calling DataFrame constructor on list
df = pd.DataFrame(lyst)
# Print the output.
df
###Output
_____no_output_____
###Markdown
Creating a dataframe using dict of narray/lists:
###Code
import pandas as pd
# intialise data of lists.
data = {'Name':['Angel', 'Precious', 'Kishi', 'Love'],
'Age':[20, 21, 19, 18]}
# Create DataFrame
df = pd.DataFrame(data)
# Print the output.
df
###Output
_____no_output_____
###Markdown
Column Selection:
###Code
# Import pandas package
import pandas as pd
# Define a dictionary containing employee data
data = {'Name':['Clement', 'Prince', 'Karol', 'Adaobi'],
'Age':[27, 24, 22, 32],
'Address':['Abuja', 'Kano', 'Minna', 'Lagos'],
'Qualification':['Msc', 'MA', 'MCA', 'Phd']}
# Convert the dictionary into DataFrame
df = pd.DataFrame(data)
# select two columns
df[['Name', 'Qualification']]
###Output
_____no_output_____
###Markdown
Row Selection:Pandas provide a unique method to retrieve rows from a Data frame.DataFrame.iloc[] method is used to retrieve rows from Pandas DataFrame.
###Code
import pandas as pd
# Define a dictionary containing employee data
data = {'Name':['Oyinda', 'Maryam', 'Dumebi', 'Bisola'],
'Age':[27, 24, 22, 32],
'Address':['Asaba', 'Maiduguri', 'Onitsha', 'Kwara'],
'Qualification':['Msc', 'MA', 'MCA', 'Phd']}
# Convert the dictionary into DataFrame
df = pd.DataFrame(data)
# select first row
df.iloc[0]
###Output
_____no_output_____
###Markdown
Read from a file:
###Code
# importing pandas package
import pandas as pd
# making data frame from csv file
data = pd.read_csv("bcg.csv")
# print excel
data
###Output
_____no_output_____
###Markdown
Select first row from file
###Code
# importing pandas package
import pandas as pd
# making data frame from csv file
data = pd.read_csv("bcg.csv")
df=data.iloc[0]
# print excel
df
###Output
_____no_output_____
###Markdown
Selecting Row with Title Header
###Code
# importing pandas package
import pandas as pd
# making data frame from csv file
data = pd.read_csv("bcg.csv")
df=data.head(1)
# print excel
df
###Output
_____no_output_____
###Markdown
Looping over rows and columnsA loop is a general term for taking each item of something, one after another. Pandas DataFrame consists of rows and columns so, in order to loop over dataframe, we have to iterate a dataframe like a dictionary.In order to iterate over rows, we can use two functions iteritems(), iterrows() . These two functions will help in iteration over rows.
###Code
# importing pandas as pd
import pandas as pd
# dictionary of lists
dict = {'name':["Abdurrahman", "Chukwuemeka", "Somebi", "Michael, Dejo"],
'degree': ["MBA", "BCA", "M.Tech", "MBA"],
'score':[90, 40, 80, 98]}
# creating a dataframe from a dictionary
df = pd.DataFrame(dict)
# iterating over rows using iterrows() function
for i, j in df.iterrows():
print(i, j)
print()
###Output
_____no_output_____
###Markdown
Looping over Columns :In order to loop over columns, we need to create a list of dataframe columns and then iterating through that list to pull out the dataframe columns.
###Code
# importing pandas as pd
import pandas as pd
# dictionary of lists
dict = {'name':["Bimpe", "Kamara", "Ugochi", "David"],
'degree': ["MBA", "BCA", "M.Tech", "MBA"],
'score':[90, 40, 80, 98]}
# creating a dataframe from a dictionary
df = pd.DataFrame(dict)
# creating a list of dataframe columns
columns = list(dfs)
for i in columns:
# printing the third element of the column
print (dfss[i][2])
###Output
_____no_output_____
###Markdown
Saving a DataFrame as CSV file
###Code
# importing pandas as pd
import pandas as pd
# dictionary of lists
blades = {'name':["Ebube", "Kamsi", "Oyinkan", "Chima"],
'degree': ["MBA", "BCA", "M.Tech", "MBA"],
'score':[90, 40, 80, 98]}
# creating a dataframe from a dictionary
df = pd.DataFrame(blades)
# saving the dataframe
df.to_csv('blade1.csv')
###Output
_____no_output_____
###Markdown
Solution to Question II
###Code
# importing pandas as pd
import pandas as pd
data = pd.DataFrame({'Employee Names':['Alegbe Luis', 'Anna Mabuta', 'Karim Kafi', 'Esther Moses', 'Jonah Longe', 'Coins Fagbemi'],
"Years":[5,10,15,8,4,20],
"Assesment Records": [44.5,67.4,23.8,71.1,50.3,63.3],
})
data
points=[]
rewards=[]
len = 6
access_rec = data['Assesment Records']
for i in range(len):
if (access_rec[i] < 40.0 ):
point = 1
elif (access_rec[i] > 39.0 and access_rec[i] < 50.0 ):
point = 2
elif (access_rec[i] > 49.0 and access_rec[i] < 60.0):
point = 3
elif (access_rec[i] > 59.0 and access_rec[i] < 70.0):
point = 4
else:
point = 5
reward = (access_rec[i]*point/6)
points.append(point)
rewards.append("%.2f" % reward)
data['Points']=(points)
data['Rewards']=(rewards)
data
# Save to excel(csv)
data.to_csv('bcg_records.csv')
###Output
_____no_output_____ |
crawling/01_start_crawling.ipynb | ###Markdown

###Code
import requests
result=requests.get('http://ggoreb.com/http')
result
result.status_code
result.encoding = 'utf-8'
result.text
def get_html(url):
html = ''
res = requests.get(url)
if res.status_code == 200:
res.encoding = 'utf-8'
html = res.text
return html
get_html('http://ggoreb.com/http')
import requests
res = requests.get('http://ggoreb.com/python/request.jsp')
print(res.status_code)
print(res.text)
###Output
200
method : GET<br>
query string<br>
<br><br>
header<br>
key : accept, value : */*<br>
key : Accept-Encoding, value : gzip, deflate<br>
key : connection, value : close<br>
key : host, value : ggoreb.com<br>
key : HOSTING_CONTINENT_CODE, value : AS<br>
key : HOSTING_COUNTRY_CODE, value : KR<br>
key : HOSTING_WHITE_IP, value : false<br>
key : user-agent, value : python-requests/2.22.0<br>
key : X-Forwarded-Proto, value : http<br>
key : X-SERVER_PORT, value : 80<br>
key : X-SERVER_PROTOCOL, value : HTTP/1.1<br>
key : X-SIMPLEXI, value : 222.107.238.125<br>
key : content-length, value : 0<br>
###Markdown
파라미터 사용
###Code
import requests
param = { 'age': 27, 'name': 'dd', 'gender': 'female' }
res = requests.get('http://ggoreb.com/python/request.jsp', params=param)
print(res.text)
import requests
headers = {'accept-language': 'en'} #dict
res = requests.get(
'http://youtube.com', headers=headers
)
res
res.text[:300]
import requests
result = get_html('http://ggoreb.com/python/html/data1.html')
result
s_idx = 0
e_idx = 0
while True:
s_idx = result.find('<td>',s_idx) #s_idx 이후의 td를 찾아라
if s_idx == -1:
break
e_idx = result.find('</td>', s_idx)
print(result[s_idx+4:e_idx]) #<td>를 생략하고 그 다음부터의 문자열을 출려갛고 싶어서 4글자 +
s_idx = e_idx #/td 이후의 td를 찾고 싶으니깐
###Output
Jill
Smith
50
Eve
Jackson
94
John
Doe
80
###Markdown
네이버증권에서 코스피 지수 가져오기
###Code
def get_html(url):
html = ''
res = requests.get(url)
if res.status_code == 200:
res.encoding = 'euc-kr'
html = res.text
return html
import requests
result = get_html('https://finance.naver.com')
from bs4 import BeautifulSoup
soup = BeautifulSoup(result, 'html.parser')
###Output
_____no_output_____
###Markdown
CSS selector로 찾기
###Code
nums = soup.select('.num_quot > .num')
nums
for num in nums:
print(num.getText())
print(num.text)
###Output
2,231.65
2,231.65
682.01
682.01
300.14
300.14
###Markdown
find 로 찾기
###Code
print(soup.find('div'))
print(soup.find('div').getText())
print(soup.find_all('div')[0])
###Output
<div id="u_skip">
<a href="#menu" tabindex="1"><span>메인 메뉴로 바로가기</span></a>
<a href="#start" tabindex="2"><span>본문으로 바로가기</span></a>
</div>
###Markdown
연습문제, 페이지에서 로또 번호 가져오기
###Code
result = get_html('http://ggoreb.com/python/html/number.html')
result
soup = BeautifulSoup(result,'html.parser')
soup
nums = soup.select('.number > img ')
for num in nums:
print(num['alt'])
###Output
1
4
10
12
28
45
###Markdown
연습문제 title 가져오기
###Code
def get_html(url):
html = ''
res = requests.get(url)
if res.status_code == 200:
res.encoding = 'None'
html = res.text
return html
result = get_html('http://ggoreb.com/python/html/attribute.html')
soup = BeautifulSoup(result, 'html.parser')
titles = soup.select('img')
for title in titles:
print(title['title'])
address = 'http://ggoreb.com/python/html/attribute.html'
res = requests.get(address)
res.encoding = None
result = res.text
soup = BeautifulSoup(result, 'html.parser')
titles = soup.select('img')
for title in titles:
print(title['title'])
###Output
루피
나미
로빈
조로
###Markdown
연습문제 유튜브 검색결과 가져오기
###Code
address = 'https://www.youtube.com/results?search_query=%EC%9B%90%ED%94%BC%EC%8A%A4'
res = requests.get(address)
res.encoding = 'uft-8'
result = res.text
soup = BeautifulSoup(result, 'html.parser')
# soup
titles = soup.select('h3.yt-lookup-title')
titles
# for title in titles:
# print(title)
address = 'https://www.youtube.com/results?search_query=%EC%9B%90%ED%94%BC%EC%8A%A4'
res = requests.get(address)
# res.encoding = 'uft-8'
res
soup = BeautifulSoup(res.text, 'html.parser')
div = soup.find_all('h3', {'class': 'yt-lockup-title '})
titles=soup.select('h3 > a.yt-uix-tile-link')
for title in titles:
print(title.text)
###Output
[원피스분석] 해적왕 골드로저가 1년동안 사라졌던 이유?! 죽음을 앞둔 그의 마지막 계획! 그것은 바로...
[원피스 917화 애니] 충격적인 흔들흔들 열매의 힘! 2년후 사황이된 검은수염 마살 D 티치 등장!
2019년 원피스 최근스토리 8분만에 다보기
[원피스 분석] 소름돋는 원피스의 정체!원피스 연재 레전드 967화 분석 (뇌피셜록)
[원피스 정보] 원피스 세계 모든 역사 총정리 2부 대해적 시대 이후
[원피스 915화 명장면] 사황 카이도우 vs 기어 4 루피 ㄷ ㄷ
[원피스 정보] 악마의 열매 중 줘도 갖기 싫은 쓰레기 열매 TOP 5
[원피스] 의식이 없는 와중에도 패왕색 패기 내뿜는 루피
[원피스 917화 명장면] 검은 수염의 현상금이 드디어 공개되다 ㄷ ㄷ
[기승전모] '원피스'의 과거와 현재에 대해 알아보자.
원피스 료쿠규와 함께 괴물로 평가 받는 후지토라의 강함에 대해
[원피스 917화 명장면] 겟코 모리아의 뉴스를 본 미호크&페로나의 반응 ㅋㅋㅋ
원피스 이제는 검은 수염과 손을 잡은 아오키지.. 그의 강함은?
(원피스 명장면) 루피VS카타쿠리(원피스 루피 전투신!!!)
[원피스]와노쿠니 이후 루피의 새로운 동료는 전설 속에 사는 남자 버기? 칠무해의 해산과 캡틴 존의 보물에 감춰진 오다의 숨겨진 떡밥!
[원피스 916화 애니] 무의식의 극의 루피 패왕색 패기 발동!! (애니리뷰 TMI)
【원피스】최근화에 밝혀진 놀라운떡밥! 샹크스는 천룡인!?
[원피스 정보] 현상금 순위 TOP10 공개되지 않은 거물급들의 현상금은?
원피스 조로 와노쿠니 전투씬 모음
[원피스 967화 리뷰] 점점 드러나는 원피스의 정체와 라프텔의 의미!!
###Markdown
네이버 영화 순위 크롤링
###Code
address = 'https://movie.naver.com/movie/sdb/rank/rmovie.nhn'
res = requests.get(address)
# res.encoding = 'uft-8'
result = res.text
soup = BeautifulSoup(result, 'html.parser')
a_list=soup.select('.tit3 > a')
for a in a_list:
print(a.getText())
###Output
닥터 두리틀
백두산
스타워즈: 라이즈 오브 스카이워커
천문: 하늘에 묻는다
미드웨이
해치지않아
시동
나쁜 녀석들 : 포에버
남산의 부장들
겨울왕국 2
포드 V 페라리
나이브스 아웃
히트맨
지푸라기라도 잡고 싶은 짐승들
터미네이터: 다크 페이트
기생충
미스터 주: 사라진 VIP
카운트다운
극장판 원피스 스탬피드
비스티걸스
트레져 헌터: 비밀의 책
나이트 헌터
갱
21 브릿지: 테러 셧다운
피아니스트의 전설
청춘빌라 살인사건
신비아파트 극장판 하늘도깨비 대 요르문간드
울지마 톤즈 2 : 슈크란 바바
타오르는 여인의 초상
콰이어트 플레이스 2
라스트 선라이즈
여곡성
1917
콰이어트 플레이스
눈의 여왕4
프릭스
인셉션
스케치
마녀
6 언더그라운드
벌룬
타발루가와 얼음공주
로그 원: 스타워즈 스토리
엄마 바람피게하기
게이트키퍼 : 또 다른 세계
스파이즈
아이리시맨
스타워즈: 라스트 제다이
신의 은총으로
차일드 인 타임
###Markdown
위메프 가전, 디지털 추천상품 정보 가져오기
###Code
import requests
from bs4 import BeautifulSoup as bs
address = 'http://www.wemakeprice.com/main/100030'
res = requests.get(address)
res.encoding = None
res=res.text
soup = BeautifulSoup(res, 'html.parser')
titles=soup.select('.tit_desc')
for title in titles:
print("="*40)
print("제목", title.text)
###Output
========================================
제목 [쿠폰+카드 중복할인] 바디픽셀 정품 머슬건 무선 진동 마사지건 (기본구성)
========================================
제목 유스마일 EMS 저주파 안마기 NO.1536
========================================
제목 [최대혜택가 382,040원] 삼성 갤럭시 A50 자급제폰 공기계 새상품 일반
========================================
제목 [최종혜택가 399,000원] 삼성 노트북5 NT550EBZ-AD2A
========================================
제목 2020년형 // 아이프리 세탁소용 충전식 보풀제거기 추천 FX-714 칼날2개 구성
========================================
제목 엘지전자 모니터 베스트모음전
========================================
제목 [1월21일설치][LG전자] 27GL650F 27인치 144Hz 울트라기어 게이밍모니터
========================================
제목 [디지털위크] 닌텐도 스위치 네온 HAD / 그레이 HAD 본체 + 다수 타이틀 택1 / 포켓몬스터 소드/실드/마리오카트8/마리오파티
========================================
제목 [최대혜택가 382,040원] 삼성전자 갤럭시A50 64GB 블랙 자급제폰 공기계 새상품 SM-A505N
========================================
제목 샌디스크 USB 메모리카드 듀얼 OTG 5핀 C타입 대용량 16G 32G 64G 128G 256G
========================================
제목 [디지털위크] [쿠폰 + 카드중복할인] 한일 EMF 무자계 항균 전기요 전기장판 무전자 전기매트
========================================
제목 윈도우+오피스365 즉시배송
========================================
제목 윈도우+오피스 특가할인 바로배송
========================================
제목 [디지털위크] 최신 PC부품 모음전(라이젠 2700X 한정수량 초특가)
========================================
제목 벡셀 알카라인 건전지 AA/AAA 20알
========================================
제목 MS윈도우 10 설치 USB + 홈/프로 정품키
========================================
제목 [디지털위크] [쿠폰+카드중복할인] ASUS 초경량 비보북 X560UD / A407MA 노트북 특가모음전!
========================================
제목 [노바리빙] LED모던 미니 체중계
========================================
제목 [디지털위크] [쿠폰+카드 중복할인] JMW 항공모터 드라이기&고데기 특가전
========================================
제목 (1+1) 무료배송/고속충전기/고속케이블/급속충전기/5핀/8핀/C타입/삼성/LG/아이폰 호환 정품 충전기
========================================
제목 [디지털위크] 공식인증점 위닉스 공기청정기 15평형 제로3.0 AZGE500-JWK*
========================================
제목 [디지털위크] [공식인증점] 위닉스 종합선물세트! 공기청정기 제로S, 건조기, 제습기 어셈블!
========================================
제목 최종가확인!★ 브라운체온계 #IRT6510 #IRT6520 #IRT6030 필터 21개 포함
========================================
제목 (20년형) 아이프리 세탁소용 충전식 보풀제거기 FX-714 (칼날2개구성) / 여성의류 / 의류 / 니트 / 스웨터/생활용품
========================================
제목 [국내정품][무배]QCY T2C 블루투스이어폰 무상A/S 1년
========================================
제목 [디지털위크] [최저가쿠폰] 알파스캔&필립스 26종 인기모니터 모음 22인치 ~ 49인치까지 + 쿠폰할인&포토평이벤트
========================================
제목 [최대 9,090원 할인] JMW M5001A PLUS BLDC 항공모터 드라이기
========================================
제목 (쿠폰할인) 비만 쏙~ 잡는 banio 4색 BMI 디지털 체중계 / 인터넷최저가 /네*버 베스트체중계
========================================
제목 생활용품 칫솔살균기 모음전
========================================
제목 MS윈도우 10 홈/프로 정품 에디션 즉시 발송
========================================
제목 닌텐도 스위치 + 게임 타이틀 1종 택1 설맞이 프로모션
========================================
제목 [디지털위크] 닌텐도스위치 본체(HAD신형) + 게임1종(택1) #카드중복할인
========================================
제목 [최저가쿠폰] 마이크론 인기 SSD 모음전 3주년 이벤트 + 중복쿠폰
========================================
제목 [국산정품] 벡셀프라임 특가전 AA/AAA/C/D/9V/리튬 건전지 (국산을 꼭 확인하세요!!!)
========================================
제목 MS오피스2010 프로페셔널플러스 컴활대비용
========================================
제목 갤럭시탭 블루투스키보드케이스
========================================
제목 [디지털위크] [9%다운로드쿠폰+4%중복카드쿠폰] 삼성프린터 초특가, 새해 맞이 이벤트(신세계 상품권, 갤럭시탭, 황사 마스크)!
========================================
제목 2019 삼성전자 갤럭시탭S6(WiFi/LTE) / 갤럭시탭S5e / 갤럭시탭A 특가
========================================
제목 해피룸 휴플러스 목/어깨 안마기 (유선/무선/썸텐션)
========================================
제목 ★쿠폰할인★ 듀플렉스 초음파 간편세척 가습기 4.0L DP-9990UH
========================================
제목 제로스킨 아이폰 X XS MAX XR 6 6S 8 7 갤럭시 S7 S8 S9 S10 5G 플러스 노트8 노트9 투명 케이스
========================================
제목 [1+1] 투명 젤리케이스
========================================
제목 닌텐도스위치 포켓몬스터 레츠고 이브이/피카츄 + 몬스터볼 플러스 세트 설날 가족대전
========================================
제목 ★쿠폰할인★ 가성비갑! 듀플렉스 오쿠 신일 간편세척 초음파/가열식 가습기
========================================
제목 NEW 알카라인 건전지 AA 20알
========================================
제목 1+1 3D 풀커버 포밍 커브드 액정보호 필름_갤럭시 S10 S10e 5G S9 S8 플러스 노트10 플러스 노트9 노트8
========================================
제목 [CRB]풀커버강화유리/액정보호필름 갤럭시 아이폰 노트10/S10/5G/E/플러스 노트9/8/11/PRO/XS/MAX/XR/X
========================================
제목 대한민국 핸드메이드 가죽 핸드폰케이스
========================================
제목 미라쥬 천연소가죽 카드지갑 핸드폰케이스 전기종
========================================
제목 [디지털위크] 최고의 성능! CANON 프린터 복합기 디지털 3중 할인 모음전
========================================
제목 ★기획전 특가 할인★차이슨 디베아 무선청소기 12월 최신생산형 ALLNEW22000 흡입력국내공식시험인증
###Markdown
쿠팡 가전디지털 > 노트북/PC/태블릿 > 노트북 상품 정보 가져오기
###Code
# import requests
# from bs4 import BeautifulSoup as bs
# address = 'https://www.coupang.com/np/categories/414501'
# res = requests.get(address)
# res.encoding = None
# res
dd.descriptions div.name
###Output
_____no_output_____
###Markdown
선택자- select_one :1개- select :여러개 - find :1개- find_all: 여러개
###Code
res = requests.get('http://ggoreb.com/python/html/example.html')
soup = BeautifulSoup(res.text, 'html.parser')
div = soup.find('div', {'class': 'ex_class'})
print(div)
print(soup.find('div').getText())
import requests
from bs4 import BeautifulSoup
res = requests.get('http://ggoreb.com/python/html/example.html')
soup = BeautifulSoup(res.text, 'html.parser')
print(soup.find_all('div'))
import requests
from bs4 import BeautifulSoup
res = requests.get('https://comic.naver.com/webtoon/list.nhn?titleId=570503&weekday=thu')
soup = BeautifulSoup(res.text, 'html.parser')
titles = soup.select('td.title > a')
# for title in titles:
# print(title.getText())
nums = soup.select('td.num')
# for num in nums:
# print(num.getText())
for num, title in zip(titles, nums):
print(num.text, title.text)
###Output
293. RE: 죄와 벌 (6) <egoist> 2020.01.15
292. RE: 죄와 벌 (5) 2020.01.08
291. RE: 죄와 벌 (4) 2020.01.01
290. RE: 죄와 벌 (3) 2019.12.25
289. RE: 죄와 벌 (2) 2019.12.18
288. RE: 죄와 벌 (1) 2019.12.11
287. 낯선 감정 2019.12.04
286. Weak 2019.11.27
285. OVER 2019.11.20
284. 지쳐갈 때 2019.11.13
###Markdown
Json 파싱
###Code
res = requests.get('http://ggoreb.com/python/json/data1.jsp')
result = res.json()
result
res = requests.get('http://ggoreb.com/python/json/data2.jsp')
result = res.json()
result
for i in result:
print(i['name'], i['age'])
res = requests.get('http://ggoreb.com/python/json/data3.jsp')
result = res.json()
result
for obj in result:
if isinstance(obj['address'],list):
for o in obj['address']:
print(o, end=' ')
###Output
서울 신림 대전 탄방 부산 해운대
###Markdown
카카오 지도 API
###Code
import requests
from bs4 import BeautifulSoup as bs
address = 'https://dapi.kakao.com/v2/local/search/address.xml'
params = {'query': '서울특별시 동작구 신대방동 395'}
headers = {'Authorization' : 'KakaoAK d4be7b479f4b4cbd99bd19ae87f88b4b'}
res = requests.get(address, params=params, headers=headers)
res.encoding = None
soup = bs(res.text, 'html.parser')
address_list = soup.find_all('address')
for addr in address_list:
x = addr.find('x').text
y = addr.find('y').text
name = addr.find('address_name').text
print(x,y,name)
import requests
address = 'https://dapi.kakao.com/v2/local/search/address.json'
params = {'query': '서울특별시 동작구 신대방동 395'}
headers = {'Authorization' : 'KakaoAK d4be7b479f4b4cbd99bd19ae87f88b4b'}
res = requests.get(address, params=params, headers=headers)
res.encoding = None
address = res.json()['documents'][0]
name, x, y = address['address_name'], address['x'], address['y']
print(name, x, y)
###Output
서울 동작구 신대방동 395 126.92261248037842 37.49371334710509
###Markdown
PaPago 번역기 API
###Code
import requests
from bs4 import BeautifulSoup as bs
address = ' https://kapi.kakao.com/v1/translation/translate'
params = {'query': '배고프다',
'src_lang' : 'kr',
'target_lang' : 'vi'
}
headers = {'Authorization' : 'KakaoAK d4be7b479f4b4cbd99bd19ae87f88b4b'}
res = requests.get(address, params=params, headers=headers)
res.encoding = None
res
text = res.json()['translated_text']
text
###Output
_____no_output_____
###Markdown
Selenium으로 크롤링하기
###Code
from selenium import webdriver as wd
driver = wd.Chrome(executable_path='c:/Code/chromedriver.exe')
driver.get('http://ggoreb.com/http/wait.jsp')
#elements 찾기
# print(driver.find_elements_by_tag_name('p'))
driver.find_element_by_css_selector('p:nth-child(2)').text
driver.close()
###Output
_____no_output_____
###Markdown
 wait - Explit(명시적)
###Code
from selenium import webdriver as wd
driver = wd.Chrome(executable_path='C:/Code/chromedriver.exe')
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
driver.get('http://ggoreb.com/http/wait.jsp')
try :
element = WebDriverWait(driver, 10).until(
EC.presence_of_element_located((By.CSS_SELECTOR, 'p:nth-of-type(2)'))
)
print(driver.find_elements_by_tag_name('p')[1].text)
except Exception as e:
print('오류 발생', e)
###Output
5초 뒤 추가 내용
###Markdown
wait - Implicit(암시적)
###Code
'''
from selenium import webdriver as wd
driver = wd.Chrome(executable_path='C:/Code/chromedriver.exe')
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
driver.get('http://ggoreb.com/http/wait.jsp')
driver.implicitly_wait(10)
print(driver.find_elements_by_tag_name('p')[1].text)
'''
#ggoreb은 안됨(로드 다 하고 javascript로 동작하기 때문)
###Output
_____no_output_____
###Markdown
요소제어- send_keys()- click()
###Code
from selenium import webdriver as wd
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
driver = wd.Chrome(executable_path='C:/Code/chromedriver.exe')
driver.get('http://ggoreb.com/http/control.jsp')
driver.find_element_by_tag_name('input').send_keys('파이썬')
driver.find_element_by_tag_name('button').click()
# alert 창의 '확인'을 클릭합니다.
alert = driver.switch_to.alert
alert.accept()
###Output
_____no_output_____
###Markdown
자바스크립트 실행하기(요소제어)- execute_script
###Code
driver.execute_script('alert("hello")')
# alert 창의 '확인'을 클릭합니다.
alert = driver.switch_to.alert
alert.accept()
driver.close()
###Output
_____no_output_____
###Markdown
phantomjs : headless하게 웹브라우저 열어서 크롤링 연습문제: 네이버 증권 서비스에서 '멀티캠퍼스' 검색 후 투자정보 조회
###Code
from selenium import webdriver as wd
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
# driver = wd.Chrome(executable_path='C:/Code/chromedriver.exe')
driver = wd.PhantomJS(executable_path='phantomjs.exe')
driver.get('https://finance.naver.com')
driver.find_element_by_id('stock_items').send_keys('멀티캠퍼스')
driver.find_element_by_css_selector('.snb_search_btn').click()
driver.implicitly_wait(10)
trs=driver.find_elements_by_css_selector('#tab_con1 > div.first > table > tbody > tr > td')
for i in trs:
print(i.text)
driver.close()
###Output
C:\ProgramData\Anaconda3\lib\site-packages\selenium\webdriver\phantomjs\webdriver.py:49: UserWarning: Selenium support for PhantomJS has been deprecated, please use headless versions of Chrome or Firefox instead
warnings.warn('Selenium support for PhantomJS has been deprecated, please use headless '
###Markdown
선택박스에서 값 send하기
###Code
from selenium import webdriver as wd
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
driver = wd.Chrome(executable_path='C:/Code/chromedriver.exe')
# driver = wd.PhantomJS(executable_path='phantomjs.exe')
driver.get('http://ggoreb.com/http/control2.jsp')
driver.find_element_by_name('no').send_keys('부산')
# driver.find_element_by_css_selector('.snb_search_btn').click()
driver.close()
driver.close()
### 연습문제: 인터파크에서 달랏 패키지 상품
from selenium import webdriver as wd
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
driver = wd.Chrome(executable_path='C:/Code/chromedriver.exe')
# driver = wd.PhantomJS(executable_path='phantomjs.exe')
driver.get('http://tour.interpark.com/')
#검색어 입력 후 이동
driver.find_element_by_css_selector('.search-wrap').send_keys('달랏')
driver.find_element_by_css_selector('.search-btn').click()
driver.implicitly_wait(5)
#해외여행 간략 페이지
driver.find_element_by_css_selector('.moreBtn').click()
import time
#해외여행 상세 목록 페이지
time.sleep(2)
import math
totalcnt = 51
totalpage = math.ceil(totalcnt/10)
totalpage
#해외여행 패키지 개수 확인
totalAllCnt = driver.find_element_by_css_selector('#totalAllCnt')
totalAllCnt = int(totalAllCnt.text)
#전체 페이지수 확인
totalPage = totalAllCnt//10+1
if totalAllCnt%10 == 0:
totalPage -=1
for page in range(1, totalPage+1):
driver.execute_script('searchModule.SetCategoryList({}, '')'.format(page))
#1페이지로 이동
time.sleep(2)
boxitems = driver.find_elements_by_css_selector('.oTravelBox .boxItem')
for item in boxitems:
print(item.find_element_by_class_name('proTit').text)
print(item.find_element_by_class_name('img').get_attribute('src'))
###Output
[달랏4일] 낭만도시 달랏 직항+랑비앙 포함 3박4일
http://tourimage.interpark.com/product/tour/00161/A10/280/A1031420_1_027.jpg
[달랏4일] 낭만도시 달랏 직항+랑비앙 포함 3박4일
http://tourimage.interpark.com/product/tour/00161/A10/280/A1031420_1_027.jpg
[나트랑/달랏5일] 시원한 낭만도시 랑비앙관광 5일
http://tourimage.interpark.com/product/tour/00161/A10/280/A1024924_1_620.jpg
[대구출발_나트랑5일] 지금 핫한 그곳! 아름다운 나트랑/달랏 관광
http://tourimage.interpark.com/product/tour/00161/A10/280/A1025044_3_337.jpg
[나트랑 5일★연합] 나트랑 1박/ 달랏 2박 꽉찬 관광일정
http://tourimage.interpark.com/product/tour/00161/A10/280/A1031349_1_470.jpg
*출발확정*[관광형] 나트랑x달랏 3박5일 [4성(1박)+달랏(2박)]
http://img.modetour.com/eagle/photoimg/42164/bfile/636724338253061415.JPG
*출발확정*[관광형] 나트랑x달랏 3박5일 [5성(1박)+달랏(2박)]
http://img.modetour.com/eagle/photoimg/42164/bfile/636724338254712656.JPG
[부산출발_나트랑5일] 떠오르는 여행지 나트랑 + 봄의 도시 달랏을 동시에!! 실속 관광형
http://tourimage.interpark.com/product/tour/00161/A10/280/A1025396_7_460.jpg
□패키지+[35만원 상당 특전포함/봄의도시,달랏/노옵션/5성호텔] 나트랑 달랏 4박5일
http://img.modetour.com/eagle/photoimg/42171/bfile/636724338128370854.JPG
*출발확정*[증편기][관광형] 나트랑x달랏 3박5일 [5성(1박)+달랏(2박)]
http://img.modetour.com/eagle/photoimg/42171/bfile/636724338177366577.JPG
●연중 봄의 도시, 달랏● [5성호텔] 달랏 3색 골프(54홀) + 관광 4박 5일
http://img.modetour.com/eagle/photoimg/56584/bfile/636453195339574051.JPG
[달랏4일] 낭만도시 달랏 직항+랑비앙 포함 3박4일
http://tourimage.interpark.com/product/tour/00161/A10/280/A1031420_1_027.jpg
[달랏골프팩18홀]나트랑x달랏 3박5일 [5성(1박)+달랏(2박)]
http://img.modetour.com/eagle/photoimg/42171/bfile/636724338177366577.JPG
[달랏골프팩18홀]나트랑x달랏 3박5일 [4성(1박)+달랏(2박)]
http://img.modetour.com/eagle/photoimg/42164/bfile/636724338249268660.JPG
[나트랑호텔UP] 나트랑x달랏 3박5일 [4성(1박)+달랏(2박)]
http://img.modetour.com/eagle/photoimg/42164/bfile/636724338261557588.JPG
[나트랑호텔UP][증편기] 나트랑x달랏 3박5일 [4성(1박)+달랏(2박)]
http://img.modetour.com/eagle/photoimg/33715/bfile/636572495566015105.jpg
[그린라이트/청주출발][관광형] 나트랑+달랏 3박5일 [나트랑1박+달랏2박] - 4성급
http://img.modetour.com/eagle/photoimg/23887/bfile/636572495577153991.jpg
[달랏직항] 달랏 패키지 3박4일 [5성호텔]
http://img.modetour.com/eagle/photoimg/59122/bfile/636724338211701392.JPG
[달랏직항] 달랏 패키지 3박4일 [4성호텔]
http://img.modetour.com/eagle/photoimg/42171/bfile/636724338177366577.JPG
[달랏In-나트랑Out] 두도시 여행 3박5일 [5성호텔]
http://img.modetour.com/eagle/photoimg/42171/bfile/636724338128370854.JPG
[달랏In-나트랑Out] 두도시 여행 3박4일 [4성호텔]
http://img.modetour.com/eagle/photoimg/42109/bfile/636632893645998618.jpg
[새벽출발x나트랑In-달랏Out] 두도시 여행 3박4일 [5성호텔]
http://img.modetour.com/eagle/photoimg/23887/bfile/636572495577153991.jpg
[달랏4일] 낭만도시 달랏 직항+랑비앙 포함 3박4일
http://tourimage.interpark.com/product/tour/00161/A10/280/A1031420_1_027.jpg
[아침출발x나트랑In-달랏Out] 두도시 여행 3박4일 [5성호텔]
http://img.modetour.com/eagle/photoimg/62086/bfile/636952470711777395.jpg
[아침출발x나트랑In-달랏Out] 두도시 여행 3박4일 [4성호텔]
http://img.modetour.com/eagle/photoimg/59122/bfile/636724338211701392.JPG
□패키지+[35만원 상당 특전포함/봄의도시,달랏/노옵션/5성호텔] 나트랑 달랏 3박4일
http://img.modetour.com/eagle/photoimg/42171/bfile/636724338126419581.JPG
(무안출발)[관광형] 나트랑x달랏 3박5일 [5성(1박)+달랏(2박)]
http://img.modetour.com/eagle/photoimg/62086/bfile/636952470711777395.jpg
(무안출발)[관광형] 나트랑x달랏 3박4일 [5성(1박)+달랏(2박)]
http://img.modetour.com/eagle/photoimg/42171/bfile/636724338177366577.JPG
(무안출발)[관광형] 나트랑x달랏 3박5일 [4성(1박)+달랏(2박)]
http://img.modetour.com/eagle/photoimg/42164/bfile/636724338249268660.JPG
(무안출발)[관광형] 나트랑x달랏 3박4일 [4성(1박)+달랏(2박)]
http://img.modetour.com/eagle/photoimg/59118/bfile/636578526517553003.JPG
[모두의특가] 달랏/호치민+구찌 4박 6일 [4성호텔/중간항공탑승]
http://img.modetour.com/eagle/photoimg/59122/bfile/636724338211701392.JPG
[모두의특가] 달랏/호치민+구찌 4박 6일 [5성호텔/중간항공탑승]
http://img.modetour.com/eagle/photoimg/42164/bfile/636724338249268660.JPG
☆[트립페스타][봄의도시,달랏/전일 관광형/5성호텔] 나트랑 달랏 3박5일($30상당 지프차 옵션 포함)
http://img.modetour.com/eagle/photoimg/62086/bfile/636952470711777395.jpg
[달랏4일] 낭만도시 달랏 직항+랑비앙 포함 3박4일
http://tourimage.interpark.com/product/tour/00161/A10/280/A1031420_1_027.jpg
♣시그니처♣[퍼펙트베트남][노쇼핑/노옵션/5성급] 나트랑x달랏 3박5일 [나트랑(1박)+달랏(2박)]($200상당 추천옵션 ALL포함)
http://img.modetour.com/eagle/photoimg/59118/bfile/636578526517553003.JPG
♣시그니처♣[퍼펙트베트남][노쇼핑/노옵션/4성급] 나트랑x달랏 3박5일 [나트랑(1박)+달랏(2박)]($200상당 추천옵션 ALL포함)
http://img.modetour.com/eagle/photoimg/59122/bfile/636724338211701392.JPG
(청주출발) [관광형] 나트랑+달랏 3박5일 [나트랑1박+달랏2박] - 5성급
http://img.modetour.com/eagle/photoimg/42171/bfile/636724338177366577.JPG
●청주출발● [퍼펙트베트남][노쇼핑,노옵션] 나트랑x달랏 3박5일 [나트랑1박+달랏2박]
http://img.modetour.com/eagle/photoimg/62086/bfile/636952470711777395.jpg
●청주출발● [관광형] 나트랑x달랏 3박5일 [나트랑1박+달랏2박]
http://img.modetour.com/eagle/photoimg/42164/bfile/636724338249268660.JPG
♣시그니처♣[퍼펙트베트남][노쇼핑,노옵션] 나트랑x달랏 4박6일 [5성(2박)+달랏(2박)]
http://img.modetour.com/eagle/photoimg/42164/bfile/636724338249268660.JPG
★[봄의도시,달랏/전일 관광형/5성호텔] 나트랑 달랏 3박4일($30상당 지프차 옵션 포함)
http://img.modetour.com/eagle/photoimg/23887/bfile/636572495577153991.jpg
★출발확정[봄의도시,달랏/전일 관광형/4성호텔] 나트랑 달랏 3박4일($30상당 지프차 옵션 포함)
http://img.modetour.com/eagle/photoimg/42171/bfile/636724338177366577.JPG
*출발확정*[증편기][관광형] 나트랑x달랏 3박5일 [4성(1박)+달랏(2박)]
http://img.modetour.com/eagle/photoimg/42164/bfile/636724338249268660.JPG
★[봄의도시,달랏/전일 관광형/4성호텔] 나트랑 달랏 3박5일($30상당 지프차 옵션 포함)
http://img.modetour.com/eagle/photoimg/62086/bfile/636952470711777395.jpg
[달랏4일] 낭만도시 달랏 직항+랑비앙 포함 3박4일
http://tourimage.interpark.com/product/tour/00161/A10/280/A1031420_1_027.jpg
□패키지+[35만원 상당 특전포함/봄의도시,달랏/노옵션/4성호텔] 나트랑 달랏 4박5일
http://img.modetour.com/eagle/photoimg/42164/bfile/636724338261557588.JPG
□패키지+[35만원 상당 특전포함/봄의도시,달랏/노옵션/4성호텔] 나트랑 달랏 3박4일
http://img.modetour.com/eagle/photoimg/42164/bfile/636724338254712656.JPG
★[봄의도시,달랏/전일 관광형/5성호텔] 나트랑 달랏 4박5일($30상당 지프차 옵션 포함)
http://img.modetour.com/eagle/photoimg/33715/bfile/636572495566015105.jpg
★출발확정/[싸데이+][봄의도시,달랏/전일 관광형/4성호텔] 나트랑 달랏 4박5일($30상당 지프차 옵션 포함)
http://img.modetour.com/eagle/photoimg/59122/bfile/636724338211701392.JPG
[대구출발_나트랑 5일] 하루 자유시간으로 나트랑 여유롭게 즐기기! 빈펄랜드포함
http://tourimage.interpark.com/product/tour/00161/A10/280/A1025185_1_993.jpg
[올인클루시브] 나트랑 패키지 4박5일 [스완도르 리조트(디럭스 씨뷰)]
http://img.modetour.com/eagle/photoimg/61178/bfile/635761123267524659.jpg
[부산출발_나트랑4일] 2박 짧고 굵게 ! 빈펄랜드+몽키아일랜드+나트랑 시내관광
http://tourimage.interpark.com/product/tour/00161/A10/280/A1025554_1_433.jpg
[부산출발_나트랑5일] 실속있게 떠나자! 준특급 호텔+빈펄랜드+1일 자유+시내관광
http://tourimage.interpark.com/product/tour/00161/A10/280/A1025379_1_103.jpg
☆[전일 오후관광/오전자유/식사포함/5성 인터컨티넨탈] 나트랑 3박 4일
http://img.modetour.com/eagle/photoimg/42109/bfile/636632893645998618.jpg
☆[전일 오후관광/오전자유/식사포함/4성 노보텔 지정] 나트랑 3박 4일
http://img.modetour.com/eagle/photoimg/62626/bfile/636900542912405915.JPG
[달랏4일] 낭만도시 달랏 직항+랑비앙 포함 3박4일
http://tourimage.interpark.com/product/tour/00161/A10/280/A1031420_1_027.jpg
☆[전일 오후관광/오전자유/식사포함/4성 호텔 동급] 나트랑 3박 4일
http://img.modetour.com/eagle/photoimg/42111/bfile/636572495570654641.jpg
###Markdown
연습문제: 대통령 선거 개표 현황
###Code
from selenium import webdriver as wd
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
driver = wd.Chrome(executable_path='C:/Code/chromedriver.exe')
# driver = wd.PhantomJS(executable_path='phantomjs.exe')
driver.get('http://info.nec.go.kr/main/showDocument.xhtml?electionId=0000000000&topMenuId=VC&secondMenuId=VCCP09')
# electionType1
driver.find_element_by_css_selector('#electionType1').click()
driver.find_element_by_css_selector('#electionName').send_keys('제19대')
time.sleep(1)
driver.find_element_by_css_selector('#electionCode').send_keys('대통령선거')
time.sleep(1)
text = driver.find_element_by_css_selector('#cityCode > option:nth-child(2)').text
time.sleep(1)
driver.find_element_by_css_selector('#cityCode').send_keys(text)
driver.find_element_by_css_selector('#searchBtn').click()
driver.implicitly_wait(2)
city_ls = [('seoul',2), ('busan',3), ('guangju',6), ('sejjong',9)]
result = {}
for name, num in city_ls:
tr = driver.find_element_by_css_selector('#table01 > tbody > tr:nth-child({})'.format(num))
temp = '문재인:'+ tr.find_element_by_css_selector('td:nth-child(4)').text \
+' 홍준표:'+ tr.find_element_by_css_selector('td:nth-child(5)').text \
+' 안철수:'+ tr.find_element_by_css_selector('td:nth-child(6)').text
result[name] = temp.replace('\n'," ")
result
for idx, val in zip(result.keys(),result.values()):
print(idx, val)
driver.close()
###Output
_____no_output_____ |
data-science/scikit-learn/07/01-Sigmoid.ipynb | ###Markdown
Sigmoid函数
###Code
import numpy as np
import matplotlib.pyplot as plt
def sigmoid(t):
return 1 / (1 + np.exp(-t))
x = np.linspace(-10, 10, 500)
y = sigmoid(x)
plt.plot(x, y)
plt.show()
###Output
_____no_output_____ |
example/House Prices demo.ipynb | ###Markdown
House Prices
###Code
# Conventionally people rename the pandas import to pd for brevity
import pandas as pd
sales = pd.read_csv('home_data.csv')
sales.head()
# TODO: How many houses are there?
len(sales)
#21613 houses
# TODO: How many inputs are there?
sales.shape[1]#return inputs
#21613 houses, price, 21
###Output
_____no_output_____
###Markdown
--- What is the average price of houses with 3 bedrooms?
###Code
# TODO: Write code to print out all the average prices for each number of bedrooms
print(sales['bedrooms'].max())
for i in range(sales['bedrooms'].max()+1):
table=sales[sales['bedrooms']==i]['price']
print("the numder of the bedrooms",i,"the average of house price",table.mean(axis=0))
#ans=466,232
###Output
33
the numder of the bedrooms 0 the average of house price 409503.6923076923
the numder of the bedrooms 1 the average of house price 317642.88442211057
the numder of the bedrooms 2 the average of house price 401372.6811594203
the numder of the bedrooms 3 the average of house price 466232.07949918567
the numder of the bedrooms 4 the average of house price 635419.5050857309
the numder of the bedrooms 5 the average of house price 786599.8232354778
the numder of the bedrooms 6 the average of house price 825520.6360294118
the numder of the bedrooms 7 the average of house price 951184.6578947369
the numder of the bedrooms 8 the average of house price 1105076.923076923
the numder of the bedrooms 9 the average of house price 893999.8333333334
the numder of the bedrooms 10 the average of house price 819333.3333333334
the numder of the bedrooms 11 the average of house price 520000.0
the numder of the bedrooms 12 the average of house price nan
the numder of the bedrooms 13 the average of house price nan
the numder of the bedrooms 14 the average of house price nan
the numder of the bedrooms 15 the average of house price nan
the numder of the bedrooms 16 the average of house price nan
the numder of the bedrooms 17 the average of house price nan
the numder of the bedrooms 18 the average of house price nan
the numder of the bedrooms 19 the average of house price nan
the numder of the bedrooms 20 the average of house price nan
the numder of the bedrooms 21 the average of house price nan
the numder of the bedrooms 22 the average of house price nan
the numder of the bedrooms 23 the average of house price nan
the numder of the bedrooms 24 the average of house price nan
the numder of the bedrooms 25 the average of house price nan
the numder of the bedrooms 26 the average of house price nan
the numder of the bedrooms 27 the average of house price nan
the numder of the bedrooms 28 the average of house price nan
the numder of the bedrooms 29 the average of house price nan
the numder of the bedrooms 30 the average of house price nan
the numder of the bedrooms 31 the average of house price nan
the numder of the bedrooms 32 the average of house price nan
the numder of the bedrooms 33 the average of house price 640000.0
###Markdown
--- What fraction of the properties are have `sqft_living` between 2000-4000?
###Code
# TODO
tabble=sales[sales['sqft_living']>=2000]
table=tabble[tabble['sqft_living']<=4000]
len(table)/len(sales)
#0.4 - 0.5
###Output
_____no_output_____
###Markdown
--- Training a Linear Regression Model.We will now train a linear regression model to make useful predictions. Work through the steps below and then answer the following questions. Even though a lot of the code is pre-written, you should understand what it is doing! You may be asked to write some of this code on future assignments.First we split the data into a training set and a test set.
###Code
from sklearn.model_selection import train_test_split
train_data, test_data = train_test_split(sales, test_size = 0.2, random_state=0) # random_state makes everything deterministic
###Output
_____no_output_____
###Markdown
Lets plot some of the data to get a sense of what we are dealing with
###Code
%matplotlib inline
import matplotlib.pyplot as plt
# plot primarily takes
#plt.scatter(sales['sqft_living'], sales['price'], marker='.')
plt.scatter(train_data['sqft_living'], train_data['price'], marker='+', label='Train')
plt.scatter(test_data['sqft_living'], test_data['price'], marker='.', label='Test')
# Code to customize the axis labels
plt.legend()
plt.xlabel('Sqft Living')
plt.ylabel('Price')
###Output
_____no_output_____
###Markdown
For this problem, we will look at using two sets of features derived from the data inputs. The basic set of features only contains a few data inputs while the advanced features contain them and more.
###Code
basic_features = ['bedrooms', 'bathrooms', 'sqft_living', 'sqft_lot', 'floors', 'zipcode']
advanced_features = basic_features + [
'condition', # condition of the house
'grade', # measure of qality of construction
'waterfront', # waterfront property
'view', # type of view
'sqft_above', # square feet above ground
'sqft_basement', # square feet in basementab
'yr_built', # the year built
'yr_renovated', # the year renovated
'lat', # the longitude of the parcel
'long', # the latitide of the parcel
'sqft_living15', # average sq.ft. of 15 nearest neighbors
'sqft_lot15', # average lot size of 15 nearest neighbors
]
from sklearn import datasets, linear_model
regr = linear_model.LinearRegression()
# Train the model using the training sets
basic_model=regr.fit(train_data[basic_features], train_data['price'])
advanced_model=regr.fit(train_data[advanced_features], train_data['price'])
###Output
/Users/jinlinxiang/anaconda3/envs/envname/lib/python3.7/site-packages/sklearn/linear_model/base.py:503: RuntimeWarning: internal gelsd driver lwork query error, required iwork dimension not returned. This is likely the result of LAPACK bug 0038, fixed in LAPACK 3.2.2 (released July 21, 2010). Falling back to 'gelss' driver.
linalg.lstsq(X, y)
###Markdown
Now, we will evaluate the models' predictions to see how they perform.--- What are your Root Mean Squared Errors (RMSE) on your training data using the basic model and then the advanced model?
###Code
# Write your code here
# Make predictions using the testing set
import math
from sklearn.metrics import mean_squared_error, r2_score
#BASIC FEATURE
basic_model=regr.fit(train_data[basic_features], train_data['price'])
basic_y_pred = regr.predict(train_data[basic_features])
basic_y_test = train_data['price']
# The mean squared error
print("Mean squared error: %.2f"
% mean_squared_error(basic_y_test, basic_y_pred))
RSS=mean_squared_error(basic_y_test, basic_y_pred)
RMSE=math.sqrt(RSS)
print(RMSE)
#AD FEATURE
advanced_model=regr.fit(train_data[advanced_features], train_data['price'])
advanced_y_pred = regr.predict(train_data[advanced_features])
advanced_y_test = train_data['price']
# The mean squared error
print("Mean squared error: %.2f"
% mean_squared_error(advanced_y_test, advanced_y_pred))
RSS=mean_squared_error(advanced_y_test, advanced_y_pred)
RMSE=math.sqrt(RSS)
print(RMSE)
###Output
Mean squared error: 66835012675.93
258524.68484833528
Mean squared error: 41536645371.59
203805.41055524038
###Markdown
--- What are your RMSE errors on your test data using the basic model and then the advanced model?
###Code
# TODO: Write your code here
import math
from sklearn.metrics import mean_squared_error, r2_score
#BASIC FEATURE
basic_model=regr.fit(train_data[basic_features], train_data['price'])
basic_y_pred = regr.predict(test_data[basic_features])
basic_y_test = test_data['price']
# The mean squared error
print("Mean squared error: %.2f"
% mean_squared_error(basic_y_test, basic_y_pred))
RSS=mean_squared_error(basic_y_test, basic_y_pred)
RMSE=math.sqrt(RSS)
print(RMSE)
#AD FEATURE
advanced_model=regr.fit(train_data[advanced_features], train_data['price'])
advanced_y_pred = regr.predict(test_data[advanced_features])
advanced_y_test = test_data['price']
# The mean squared error
print("Mean squared error: %.2f"
% mean_squared_error(advanced_y_test, advanced_y_pred))
RSS=mean_squared_error(advanced_y_test, advanced_y_pred)
RMSE=math.sqrt(RSS)
print(RMSE)
###Output
Mean squared error: 59538329945.14
244004.77443104098
Mean squared error: 36280106854.24
190473.37570967257
|
Lectures notebooks/(Lectures notebooks) netology Feature engineering/4. Feature Selection/Practice_3_media_cut/Practice_3_media_cut.ipynb | ###Markdown
Рекламный бюджет
###Code
adv_df = pd.read_csv('data/Advertising.csv')#, usecols=[1,2,3,4])
adv_df.head()
adv_df = adv_df.drop('Unnamed: 0', axis = 1)
adv_df.info()
###Output
<class 'pandas.core.frame.DataFrame'>
RangeIndex: 200 entries, 0 to 199
Data columns (total 4 columns):
TV 200 non-null float64
radio 200 non-null float64
newspaper 200 non-null float64
sales 200 non-null float64
dtypes: float64(4)
memory usage: 6.3 KB
###Markdown
Выше представлены данные о продажах некоторого продукта (sales, в тысячах штук) в зависимости от рекламного бюджета (в тысячах долларов), потраченного на различные медиа (TV, Radio и Newspapers). Предположим, что вы консультант, которого попросили создать маркетинговый план на следующий год на основе этих данных, так, чтобы продажи продукта были высокими. Что мы пытаемся понять? 1. Есть ли связь между рекламным бюджетом и продажами?2. Насколько сильна связь между бюджетом и продажами? Можем ли мы предсказывать продажи на основе бюджета?3. Какие медиа способствуют продажам?4. Насколько точно мы можем предсказывать будущие продажи?5. Линейная ли зависимость между бюджетом и продажами?Мы ответим на эти вопросы, используя только линейную регрессию. Выбор модели
###Code
from sklearn.linear_model import LinearRegression
import math
adv_df['log_tv'] = adv_df.TV.apply(lambda x: math.log(x, 2))
adv_df.plot.scatter('sales', 'log_tv')
adv_df['pow_tv'] = adv_df.TV.apply(lambda x: math.pow(x, 0.4))
adv_df.plot.scatter('sales', 'pow_tv')
%matplotlib inline
fig, axes = plt.subplots(1, 5, sharey=True, figsize=(15,8))
fig.suptitle('Зависимость от компонент', fontsize=20)
y_col = 'sales'
for i, x_col in enumerate(adv_df.columns.drop(y_col)):
adv_df.plot.scatter(x_col, y_col, ax=axes[i])
lm = LinearRegression().fit(
adv_df[x_col].values.reshape(-1, 1),
adv_df[y_col].values.reshape(-1, 1)
)
xs = np.array([adv_df[x_col].min(), adv_df[x_col].max()]).reshape(-1, 1)
ys = lm.predict(xs)
axes[i].plot(xs, ys, c='r', linewidth=2)
###Output
_____no_output_____
###Markdown
У нас есть 3 атрибута и мы считаем, что все они полезные. Так как мы используем линейную регрессию, модель будет выглядеть следующим образом:$$sales = \beta_0 + \beta_1 * TV + \beta_2 * Radio + \beta_3 * Newspaper$$ t-statistcs, p-value, $R^2$, RSE, F-statistics Итак, у нас есть линейная модель и мы можем посчитать коэффициенты $\beta_0, \beta_1, \beta_2, \beta_3$. Найдем их (и немного статической информации о работе модели), воспользовавшись библиотечкой statsmodel.
###Code
est = smf.ols('sales ~ TV + radio + newspaper', data=adv_df).fit()
est_res = est.summary()
est_res.tables[1]
###Output
_____no_output_____
###Markdown
Standard error Как мы помним, настоящее линейное представление данных выглядит как:$$y = \beta_0 + \beta_1 * x_1 + \beta_2 * x_2 + \dots + \beta_n * x_n + \epsilon$$где $\epsilon$ - неприводимая ошибка (irreducible error). Поэтому наша модель это аппроксимация вида:$$\hat{y} = \beta_0 + \beta_1 * x_1 + \beta_2 * x_2 + \dots + \beta_n * x_n$$Более того, так как мы работаем лишь с сэмплом данных (а не с полным набором), то коэффициенты $\beta_0, \beta_1, \beta_2, \dots, \beta_n$ на самом деле тоже аппроксимация ($\hat{\beta_0}, \hat{\beta_1}, \hat{\beta_2}, \dots, \hat{\beta_n}$). И, конечно, нам интересно знать, насколько она точна. Для этих целей используется *стандартная ошибка*.Стандартную ошибку можно посчитать для каждого параметра (мы опустим особенности и ограничения этих вычислений). Зная стандартные ошибки, можно вычислить доверительные интервалы. Например, $95\%$ значений $\beta_0$ будут в интервале $[2.324, 3.554]$ (т.е., без какой-либо рекламы с 95% уверенностью мы сможем продавать от $2324$ до $3554$ единиц товара). Проверка гипотезы (t-statistics и p-value) Используя стандартные ошибки, мы можем проверять гипотезы. Наиболее популярный тип проверки - сопоставление *нулевой* и *альтернативной гипотезы*:$H_0$: между $x_i$ и $y$ нет зависимости$H_a$: между $x_i$ и $y$ есть зависимостьВ нашем случае, математически это выглядит так:$$H_0: \beta_i = 0 \\H_a: \beta_i \ne 0$$ Для проверки этой гипотезы производится t-тест:$$t = \frac{\hat{\beta_i} - 0}{SE(\hat{\beta_i})}$$SE - standard error. Если между $x_i$ и $y$ нет зависимости, то мы ожидаем, что $t$ будет соответствовать $t$-распределению с $n-2$ степенями свободы ($n$ - количество экземпляров в сэмпле). Таким образом, чтобы подтвердить $H_0$ при известном распределении, нам достаточно найти вероятность того, что наблюдаемое значение $\ge |t|$ при условии, что $\beta_i = 0$. Данная вероятность называется $p$-value. Если $p$-value достаточно маленький (обычно меньше $1\%$), то мы можем отклонить нулевую гипотезу.
###Code
est_res.tables[1]
###Output
_____no_output_____
###Markdown
В нашем случае все медиа, кроме Newspapers, имеют маленький $p$-value. Т.е. между Newspaper и Sales нет линейной зависимости и, следовательно, этого атрибута можно избавиться. Недостатком определения полезности атрибутов с использованием t-statistics является то, что оценка важности каждого атрибута производится независимо от других, что может быть ошибочно. $R^2$ Линейная регрессия минимизирует метрику RSS (residual sum of squares), которая равна сумме квадратов наблюдаемых ошибок:$$RSS = \sum_{i = 1}^n(y_i - \hat{y_i})^2$$ К сожалению, данная величина абсолютная и по ней сложно определить качество модели. Поэтому качество линейной регрессии часто оценивают при помощи двух других метрик: RSE (residual standard error) и $R^2$.$$RSE = \sqrt{\frac{1}{n-p-1}RSS}$$$$R^2 = \frac{TSS - RSS}{TSS} = 1 - \frac{RSS}{TSS}, \quad TSS = \sum_{i = 1}^n(y_i - \bar{y})^2$$ $TSS$ - total sum of squares, $p$ - количество переменных.* RSE - штрафует модели, которым нужно больше предсказателей (predictors) для достижения одинаковых значения RSS.* $R^2$ - показывает, какой процент вариативности (variance) объяснен моделью.Т.е. мы минимизируем RSE и максимизируем $R^2$. $R^2 \in [0, 1]$ - относительная величина, чем ближе к 1, тем лучше.
###Code
three_x_lm = smf.ols('sales ~ TV + radio + newspaper', adv_df).fit()
rss = np.sum(three_x_lm.resid ** 2)
print("RSS:", rss)
print("RSE:", np.sqrt(rss / (adv_df.shape[0] - 3 - 1)))
print("R^2:", three_x_lm.rsquared)
adv_df.shape[0]
three_x_lm = smf.ols('sales ~ TV + radio + newspaper', adv_df).fit()
rss = np.sum(three_x_lm.resid ** 2)
print("RSS:", rss)
print("RSE:", np.sqrt(rss / (adv_df.shape[0] - 3 - 1)))
print("R^2:", three_x_lm.rsquared)
two_x_lm = smf.ols('sales ~ TV + radio', adv_df).fit()
rss = np.sum(two_x_lm.resid ** 2)
print("RSS:", rss)
print("RSE:", np.sqrt(rss / (adv_df.shape[0] - 2 - 1)))
print("R^2:", two_x_lm.rsquared)
three_x_lm = smf.ols('sales ~ log_tv + radio', adv_df).fit()
rss = np.sum(three_x_lm.resid ** 2)
print("RSS:", rss)
print("RSE:", np.sqrt(rss / (adv_df.shape[0] - 2 - 1)))
print("R^2:", three_x_lm.rsquared)
###Output
RSS: 372.10288231089464
RSE: 1.3743533455154153
R^2: 0.9313101966581784
###Markdown
Чем проще модель, тем лучше она обобщает. Поэтому в нашем примере модель, в которой есть только TV и Radio, лучше модели с TV, Radio и Newspaper (приблизительно одинаковые $R^2$ и меньший $RSE$). **Внимание!!!** Все метрики были посчитаны для тренировочных данных. Итак, мы показали, что нам не нужны газеты. Посмотрим, что у нас получилось.
###Code
adv_df.columns
adv_df.drop(['newspaper'], axis=1).describe()
lm = LinearRegression().fit(
adv_df[['TV', 'radio']].as_matrix(),
adv_df["sales"]
)
# Create a coordinate grid
Radio = np.arange(0,50)
TV = np.arange(0,300)
B1, B2 = np.meshgrid(TV, Radio, indexing='xy')
Z = np.zeros((Radio.size, TV.size))
for (i,j),v in np.ndenumerate(Z):
Z[i,j] =(lm.intercept_ + B1[i,j] * lm.coef_[0] + B2[i,j] * lm.coef_[1])
fig = plt.figure(figsize=(10,6))
fig.suptitle('Regression: sales ~ TV + radio', fontsize=20)
ax = axes3d.Axes3D(fig, azim=135, elev=30)
ax.plot_surface(B1, B2, Z, rstride=5, cstride=5, alpha=0.3, cmap=plt.cm.brg)
ax.scatter3D(adv_df.TV, adv_df.radio, adv_df.sales, c='r')
ax.set_xlim(ax.get_xlim()[::-1])
ax.set_xlabel('TV')
ax.set_ylabel('radio')
ax.set_zlabel('sales')
###Output
_____no_output_____
###Markdown
Как видно из изображения, апроксимирующая плоскость переоценивает значения Sales для случаев, когда точки находятся рядом с одной из осей (т.е. зависят только от одного медиа) и недооценивает Sales в случае, когда бюджет разделен между двумя медиа.
###Code
lm = LinearRegression().fit(
adv_df[['log_tv', 'radio']].as_matrix(),
adv_df["sales"]
)
# Create a coordinate grid
Radio = np.arange(0,50)
TV = np.arange(0,10)
B1, B2 = np.meshgrid(TV, Radio, indexing='xy')
Z = np.zeros((Radio.size, TV.size))
for (i,j),v in np.ndenumerate(Z):
Z[i,j] =(lm.intercept_ + B1[i,j] * lm.coef_[0] + B2[i,j] * lm.coef_[1])
fig = plt.figure(figsize=(10,6))
fig.suptitle('Regression: sales ~ log_tv + radio', fontsize=20)
ax = axes3d.Axes3D(fig, azim=135, elev=30)
ax.plot_surface(B1, B2, Z, rstride=5, cstride=5, alpha=0.3, cmap=plt.cm.brg)
ax.scatter3D(adv_df.log_tv, adv_df.radio, adv_df.sales, c='r')
ax.set_xlim(ax.get_xlim()[::-1])
ax.set_xlabel('TV')
ax.set_ylabel('radio')
ax.set_zlabel('sales')
###Output
_____no_output_____ |
notebooks/02d-concordance_ad_hoc_Lx.ipynb | ###Markdown
Ad hoc concordance analyses of the Lx results. Lx results was using a previous version of analyze results pipeline that didn't have concordance yet; this adds it in
###Code
import glob
import os
import pandas as pd
import re
import pickle
import matplotlib.pyplot as plt
import seaborn as sns
def getConcordance(df, threshold=-0.6):
df['concordance'] = 0
df.loc[(df.y_actual <= threshold) & (df.y_pred <= threshold), 'concordance'] = 1
df.loc[(df.y_actual > threshold) & (df.y_pred > threshold), 'concordance'] = 1
return sum(df.concordance == 1) / len(df)
def genConcordance(Lx_dir):
outdir_concord = '%s/anlyz/concordance/' % Lx_dir
if not os.path.exists(outdir_concord):
os.makedirs(outdir_concord)
y_compr_fnames = glob.glob(os.path.join(Lx_dir, 'model_perf', 'y_compr_*.pkl'))
if len(y_compr_fnames) > 0:
df_conc_tr = pd.DataFrame()
df_conc_te = pd.DataFrame()
for fname in y_compr_fnames:
f = re.sub('.*_compr_', '', fname)
gene = re.sub('\.pkl', '', f)
df = pickle.load(open(fname, 'rb'))
tmp = pd.DataFrame([{'gene': gene, 'concordance': getConcordance(df['tr'])}])
df_conc_tr = pd.concat([df_conc_tr, tmp])
tmp = pd.DataFrame([{'gene': gene, 'concordance': getConcordance(df['te'])}])
df_conc_te = pd.concat([df_conc_te, tmp])
df_conc_tr.to_csv('%s/concordance_tr.csv' % outdir_concord, index=False)
df_conc_te.to_csv('%s/concordance_te.csv' % outdir_concord, index=False)
plt.figure()
ax = sns.distplot(df_conc_tr.concordance)
ax.set(xlim=[0, 1.05], xlabel='Concordance', title='Concordance between actual and predicted')
plt.savefig("%s/concordance_tr.pdf" % outdir_concord)
plt.close()
plt.figure()
ax = sns.distplot(df_conc_te.concordance)
ax.set(xlim=[0, 1.05], xlabel='Concordance', title='Concordance between actual and predicted')
plt.savefig("%s/concordance_te.pdf" % outdir_concord)
plt.close()
for fdir in glob.glob('../out/20.0518 Lx/*/'):
print(fdir)
genConcordance(fdir)
###Output
../out/20.0518 Lx/L100_reg_rf_boruta/
../out/20.0518 Lx/L200only_reg_lm/
../out/20.0518 Lx/L200only_reg_rf_boruta/
../out/20.0518 Lx/L300only_reg_rf_boruta/
../out/20.0518 Lx/L100only_reg_rf_boruta/
../out/20.0518 Lx/L100only_reg_elasticnet_all/
../out/20.0518 Lx/L75only_reg_elasticnet_all/
../out/20.0518 Lx/L25only_reg_rf_boruta/
../out/20.0518 Lx/L100only_reg_lm/
../out/20.0518 Lx/L75only_reg_rf_boruta/
../out/20.0518 Lx/L200only_reg_elasticnet_all/
../out/20.0518 Lx/L100only_reg_elasticnet/
../out/20.0518 Lx/L25only_reg_elasticnet_all/
../out/20.0518 Lx/L100only_reg_rf_boruta_all/
|
code/001.03_data_processing.ipynb | ###Markdown
001.03 // data processing [convert annotations to txt files for keras implementation of YOLOv3]
###Code
import os
import shutil
import pandas as pd
# move labels to designated file
def move_files(path_in, path_out, file_type):
filenames = sorted([f for f in os.listdir(path_in) if file_type in f])
for f in filenames:
shutil.move(f'{path_in}/{f}', f'{path_out}{f}')
move_files('../data/images/', '../data/labels/', '.xml')
# convert xml annotations into csv
!python xml_to_csv.py
df = pd.read_csv('../data/labels/_annotations.csv')
df.head()
# confirm 52 classes
classes = sorted(df['class'].unique())
ids = {}
i = 0
for c in classes:
ids[c] = i
i += 1
len(classes)
# create classes document
classes_txt = open('../assets/classes.txt', 'w+')
[i for i in ids.keys()]
for i in ids.keys():
classes_txt.write(f'{i}\n')
classes_txt.close()
# if using grayscale images, change first line to:
# df['file_path'] = [f'../data/images_gs/{i}' for i in df['filename']]
df['file_path'] = [f'../data/images/{i}' for i in df['filename']]
df['class_id'] = df['class'].map(ids)
df = df.sort_values(['filename', 'class_id']).reset_index(drop = True)
box_features = ['file_path', 'xmin', 'ymin', 'xmax', 'ymax', 'class_id']
boxes = df[box_features].applymap(str)
for i in box_features:
if box_features.index(i) in range(1, 5):
boxes[i] = boxes[i] + ','
else:
boxes[i] = boxes[i] + ' '
# create final annotations document
samples = boxes['file_path'].unique()
annot_txt = open('../assets/annotations.txt', 'w+')
box_format = []
for i in boxes.index:
box_format.append([boxes.loc[i, c] for c in box_features])
box_format.append([''])
for i in range(len(samples)):
while box_format[i][0] == box_format[i + 1][0]:
[box_format[i].append(n) for n in box_format[i + 1][1:]]
box_format.pop(i + 1)
for i in box_format[:-1]:
annot_txt.write((''.join(i).strip() + '\n'))
annot_txt.close()
###Output
_____no_output_____ |
notebooks/.ipynb_checkpoints/produce_eofs-checkpoint.ipynb | ###Markdown
EOFs of NCEPv1 500hPa geopotential height anomaliesThis notebook contains the routines for computing the EOFs and PCs of daily 500 hPa geopotential height anomaliesused for the subsequent FEM-BV-VAR analysis. Packages
###Code
import os
import warnings
import time
import cartopy.crs as ccrs
import matplotlib.gridspec as gridspec
import matplotlib.path as mpath
import matplotlib.pyplot as plt
import numpy as np
import pandas as pd
import scipy.stats as ss
import xarray as xr
from cartopy.util import add_cyclic_point
from sklearn.decomposition import TruncatedSVD
###Output
_____no_output_____
###Markdown
File paths
###Code
PROJECT_DIR = os.path.join(os.path.dirname(os.path.abspath('produce_eofs.ipynb')),'..')
DATA_DIR = os.path.join(PROJECT_DIR,'data')
RESULTS_DIR = os.path.join(PROJECT_DIR,'results')
ANOM_RESULTS_DIR = os.path.join(RESULTS_DIR, 'anom')
EOFS_RESULTS_DIR = os.path.join(RESULTS_DIR, 'eofs')
EOFS_NC_DIR = os.path.join(EOFS_RESULTS_DIR, 'nc')
EOFS_PLOTS_DIR = os.path.join(EOFS_RESULTS_DIR, 'plt')
if not os.path.exists(DATA_DIR):
raise RuntimeError("Input data directory '%s' not found" % DATA_DIR)
if not os.path.exists(RESULTS_DIR):
os.makedirs(RESULTS_DIR)
if not os.path.exists(ANOM_RESULTS_DIR):
os.makedirs(ANOM_RESULTS_DIR)
if not os.path.exists(EOFS_RESULTS_DIR):
os.makedirs(EOFS_RESULTS_DIR)
if not os.path.exists(EOFS_NC_DIR):
os.makedirs(EOFS_NC_DIR)
if not os.path.exists(EOFS_PLOTS_DIR):
os.makedirs(EOFS_PLOTS_DIR)
# Input file containing the 500 hPa geopotential height values
INPUT_HGT_DATAFILE = os.path.join(DATA_DIR, 'hgt.500.1948_2018.nc')
# Default dimension names etc. for NNR1 data
TIME_NAME = 'time'
LAT_NAME = 'lat'
LON_NAME = 'lon'
VAR_NAME = 'hgt'
###Output
_____no_output_____
###Markdown
Region definitions
###Code
def get_regions(hemisphere):
"""Get named regions defined for a given hemisphere.
Parameters
----------
hemisphere : 'WG' | 'NH' | 'SH'
Hemisphere to get named regions for.
Returns
-------
regions : list
List of named regions defined for hemisphere.
"""
if hemisphere == 'WG':
return ['all']
if hemisphere == 'NH':
return ['all', 'eurasia', 'pacific', 'atlantic', 'atlantic2',
'atlantic3', 'atlantic_eurasia']
if hemisphere == 'SH':
return ['all', 'indian', 'south_america', 'pacific',
'full_pacific', 'australian']
raise RuntimeError("Invalid hemisphere '%s'" % hemisphere)
def get_region_lon_bounds(hemisphere, region):
"""Get longitude bounds for a given region.
Parameters
----------
hemisphere : 'WG' | 'NH' | 'SH'
Hemisphere in which region is located.
region : str
Name of region to get longitude bounds for.
Returns
-------
lon_bounds : array, shape (2,)
Array containing the longitude bounds for the region.
"""
if hemisphere == 'WG' or hemisphere == 'NH':
if region == 'all':
return np.array([0, 360])
if region == 'atlantic':
return np.array([250, 360])
if region == 'atlantic2':
return np.array([270, 360])
if region == 'atlantic3':
return np.array([270, 40])
if region == 'atlantic_eurasia':
return np.array([250, 120])
if region == 'eurasia':
return np.array([0, 120])
if region == 'pacific':
return np.array([120, 250])
raise ValueError("Invalid region '%s'" % region)
if hemisphere == 'SH':
if region == 'all':
return np.array([0, 360])
if region == 'australian':
return np.array([110, 210])
if region == 'full_pacific':
return np.array([150, 300])
if region == 'indian':
return np.array([0, 120])
if region == 'pacific':
return np.array([120, 250])
if region == 'south_america':
return np.array([240, 360])
raise ValueError("Invalid region '%s'" % region)
raise ValueError("Invalid hemisphere '%s'" % hemisphere)
def get_region_data(da, hemisphere='WG', region='all', season='ALL',
lat_name=LAT_NAME, lon_name=LON_NAME, time_name=TIME_NAME):
"""Get data restricted to given hemisphere, region and season.
Parameters
----------
da : xarray.DataArray
DataArray containing the field to subset.
hemisphere : 'WG' | 'NH' | 'SH'
Hemisphere to restrict to.
region : str
Name of region to restrict data to.
season : 'ALL' | 'DJF' | 'MAM' | 'JJA' | 'SON'
Season to restrict data to.
lat_name : str
Name of the latitude coordinate.
lon_name : str
Name of the longitude coordinate.
time_name : str
Name of the time coordinate.
Returns
-------
region_data : xarray.DataArray
DataArray containing the input field restricted to the
requested region and season.
"""
if hemisphere not in ('WG', 'NH', 'SH'):
raise ValueError("Invalid hemisphere '%s'" % hemisphere)
if hemisphere == 'NH':
da = da.where(da[lat_name] >= 0, drop=True)
elif hemisphere == 'SH':
da = da.where(da[lat_name] <= 0, drop=True)
lon_bounds = get_region_lon_bounds(hemisphere, region)
if lon_bounds[1] - lon_bounds[0] < 0:
da = da.where(
((da[lon_name] >= lon_bounds[0]) &
(da[lon_name] <= 360)) |
((da[lon_name] >= 0) &
(da[lon_name] <= lon_bounds[1])), drop=True)
else:
da = da.where(
(da[lon_name] >= lon_bounds[0]) &
(da[lon_name] <= lon_bounds[1]), drop=True)
if season != 'ALL':
da = da.where(da[time_name].dt.season == season, drop=True)
return da
###Output
_____no_output_____
###Markdown
Calculation of anomaliesBy default, anomalies are computed by removing the daily climatology determined from the specified base period.No trends are removed beforehand.
###Code
# Climatology used for computing anomalies
CLIMATOLOGY_BASE_PERIOD = [np.datetime64('1979-01-01'), np.datetime64('2018-12-31')]
# Whether to write anomalies to file
SAVE_ANOMALIES = True
def get_anomaly_datafile_name(input_datafile, base_period, input_detrended=False):
"""Create filename for anomalies output file.
Parameters
----------
input_datafile : str
Name of the input datafile.
base_period : list
List containing the first and late date in the base period used
to compute the anomalies.
input_detrended : bool
If True, add suffix to filename indicating anomalies are
based on detrended data.
Returns
-------
filename : str
Name of the output file for the anomalies.
"""
basename, ext = os.path.splitext(os.path.basename(input_datafile))
base_period_str = '{}_{}'.format(pd.to_datetime(base_period[0]).strftime('%Y%m%d'),
pd.to_datetime(base_period[1]).strftime('%Y%m%d'))
if input_detrended:
return '.'.join([basename, base_period_str, 'detrended', 'anom']) + ext
return '.'.join([basename, base_period_str, 'anom']) + ext
# Load 500 hPa geopotential height data
hgt_ds = xr.open_dataset(INPUT_HGT_DATAFILE)
hgt_da = hgt_ds[VAR_NAME].astype(np.float64)
# Get data corresponding to climatology base period
base_period_da = hgt_da.where((hgt_da[TIME_NAME] >= CLIMATOLOGY_BASE_PERIOD[0]) &
(hgt_da[TIME_NAME] <= CLIMATOLOGY_BASE_PERIOD[1]), drop=True)
# Calculate daily mean climatology
clim_mean_da = base_period_da.groupby(
base_period_da[TIME_NAME].dt.dayofyear).mean(TIME_NAME)
# Calculate anomalies
hgt_anom_da = hgt_da.groupby(hgt_da[TIME_NAME].dt.dayofyear) - clim_mean_da
anom_output_filename = get_anomaly_datafile_name(INPUT_HGT_DATAFILE, CLIMATOLOGY_BASE_PERIOD)
anom_output_file = os.path.join(ANOM_RESULTS_DIR, anom_output_filename)
if SAVE_ANOMALIES:
# Write to file
hgt_anom_ds = hgt_anom_da.to_dataset(name=(VAR_NAME + '_anom'))
hgt_anom_ds.attrs['input_file'] = INPUT_HGT_DATAFILE
hgt_anom_ds.attrs['base_period_start'] = pd.to_datetime(CLIMATOLOGY_BASE_PERIOD[0]).strftime('%Y%m%d')
hgt_anom_ds.attrs['base_period_end'] = pd.to_datetime(CLIMATOLOGY_BASE_PERIOD[1]).strftime('%Y%m%d')
hgt_anom_ds.to_netcdf(anom_output_file)
hgt_ds.close()
###Output
_____no_output_____
###Markdown
EOF analysis
###Code
def get_latitude_weights(data, weights_str, lat_name=LAT_NAME):
"""Calculate latitude weights.
Parameters
----------
data : xarray.DataArray or xarray.Dataset
Object containing the data to perform EOF analysis on.
weights_str : 'none' | 'cos' | 'scos'
String indicating type of weighting to apply:
- 'none': no latitude weighting.
- 'cos': weight by cosine of latitude
- 'scos': weight by square root of cosine of latitude
lat_name : str
Name of latitude coordinate.
Returns
-------
lat_weights : xarray.DataArray
Array containing the latitude weights.
"""
# No latitude weighting
if weights_str == 'none':
return xr.ones_like(data[lat_name])
# Weighting by the cosine of the latitude
if weights_str == 'cos':
return np.cos(np.deg2rad(data[lat_name]))
# Weighting by the square root of the cosine of the latitude
if weights_str == 'scos':
return np.cos(np.deg2rad(data[lat_name])).clip(0., 1.)**0.5
raise ValueError("Invalid weights string '%s'" % weights_str)
def fix_svd_phases(u, vh):
"""Impose fixed phase convention on singular vectors.
Given a set of left- and right-singular vectors as the columns of u
and rows of vh, respectively, imposes the phase convention that for
each left-singular vector, the element with largest absolute value
is real and positive.
Parameters
----------
u : array, shape (M, K)
Unitary array containing the left-singular vectors as columns
vh : array, shape (K, N)
Unitary array containing the right-singular vectors as rows.
Returns
-------
u_fixed : array, shape (M, K)
Unitary array containing the left-singular vectors as columns,
conforming to the chosen phase convention.
vh_fixed : array, shape (K, N)
Unitary array containing the right-singular vectors as rows,
conforming to the chosen phase convention.
"""
n_cols = u.shape[1]
max_elem_rows = np.argmax(np.abs(u), axis=0)
if np.any(np.iscomplexobj(u)):
phases = np.exp(-1j * np.angle(u[max_elem_rows, range(n_cols)]))
else:
phases = np.sign(u[max_elem_rows, range(n_cols)])
u *= phases
vh *= phases[:, np.newaxis]
return u, vh
# Default maximum number of EOFs to retain
DEFAULT_MAX_EOFS = 200
def run_pca(da, sample_dim=TIME_NAME, weights=None, normalization='unit', tolerance=1e-6,
center=True, bias=False, n_components=DEFAULT_MAX_EOFS, eofs_base_period=None,
algorithm='arpack', tol=0, n_iter=10, random_state=None):
"""Run EOF analysis on data.
Parameters
----------
da : xarray.DataArray
DataArray containing the field to perform EOF analysis on.
sample_dim : str
Dimension labelling separate samples.
weights : xarray.DataArray, optional
If given, weights to apply to data before performing EOF analysis.
normalization : 'unit' | 'sqrt_variance' | 'inverse_sqrt_variance'
Normalization convention to use for EOFs.
tolerance : float, default: 1e-6
Tolerance used for checking data is centered.
center : bool, default: True
If True, center the data by removing the feature means.
bias : bool, default: False
If True, calculate estimated variance with biased number of
degrees of freedom.
n_components : int, optional
Number of EOFs to retain.
eofs_base_period : list, optional
List of length 2 containing the first and last date to use in
calculating the EOFs.
random_state : integer, RandomState or None
If an integer, random_state is the seed used by the
random number generator. If a RandomState instance,
random_state is the random number generator. If None,
the random number generator is the RandomState instance
used by `np.random`.
Returns
-------
eofs_ds : xarray.Dataset
Dataset containing the results of the EOF analysis, with
the data variables:
- 'eofs': calculated EOFs
- 'principal_components': time-series of principal components
- 'explained_variance_ratio': fraction of variance explained by
each mode
- 'singular_values': singular values for each mode
- 'means': mean of each feature over the base period
"""
# Determine shape of individual samples for later reshaping
feature_dims = [d for d in da.dims if d != sample_dim]
original_shape = [da.sizes[d] for d in da.dims if d != sample_dim]
# Weight data if any weights are given
if weights is not None:
input_da = weights * da
input_da = input_da.transpose(*da.dims)
else:
input_da = da
# Ensure sample dimension is first dimension
if input_da.get_axis_num(sample_dim) != 0:
input_da = input_da.transpose(*([sample_dim] + feature_dims))
# If no base period is given, use all data for computing the EOFs
if eofs_base_period is None:
eofs_base_period = [input_da[sample_dim].min().item(),
input_da[sample_dim].max().item()]
# Determine the number of samples and number of features
n_samples = input_da.sizes[sample_dim]
n_features = np.product(original_shape)
# Mask out missing values
values = input_da.values
flat_values = values.reshape((n_samples, n_features))
missing_features = np.any(np.isnan(flat_values), axis=0)
valid_values = flat_values[:, np.logical_not(missing_features)]
# Select data in the requested period to use for computing the EOFs
base_period_mask = np.logical_and(
input_da[sample_dim].data >= eofs_base_period[0],
input_da[sample_dim].data <= eofs_base_period[1])
base_period_values = valid_values[base_period_mask]
# If centering the data, compute the feature means and subtract them
base_period_means = np.mean(base_period_values, axis=0)
if center:
base_period_values = base_period_values - base_period_means[np.newaxis, :]
# Double check that inputs are centered
input_means = np.mean(base_period_values, axis=0)
if np.any(np.abs(input_means) > tolerance):
warnings.warn(
'Input data does not have zero column means '
'(got max(abs(means))=%.3e)' % np.max(np.abs(input_means)),
UserWarning)
# Calculate truncated SVD
svd = TruncatedSVD(n_components=n_components, algorithm=algorithm, n_iter=n_iter,
tol=tol, random_state=random_state)
u = svd.fit_transform(base_period_values)
u = u / svd.singular_values_
s = svd.singular_values_
vh = svd.components_
# Project to get PCs over full sampling period
u = np.dot(valid_values, vh.T) / svd.singular_values_
# Fix phase convention for the EOFs and PCs
u, vh = fix_svd_phases(u, vh)
# Calculate variance explained by each mode
ddof = 0 if bias else 1
ddof_factor = base_period_values.shape[0] - ddof
eigenvalues = s ** 2 / ddof_factor
variances = np.var(base_period_values, ddof=ddof, axis=0)
total_variance = np.sum(variances)
explained_variance_ratio = eigenvalues / total_variance
# Apply normalization convention for EOFs
if normalization == 'unit':
pcs = np.dot(u, np.diag(s))
eofs = vh
elif normalization == 'sqrt_variance':
pcs = u * np.sqrt(ddof_factor)
eofs = np.dot(np.diag(np.sqrt(eigenvalues)), vh)
elif normalization == 'inverse_sqrt_variance':
pcs = np.dot(u, np.diag(eigenvalues)) * np.sqrt(ddof_factor)
eofs = np.dot(np.diag(1 / np.sqrt(eigenvalues)), vh)
else:
raise ValueError("Invalid normalization '%s'" % normalization)
# Construct arrays containing computed EOFs and PCs
full_eofs = np.full((n_components, n_features), np.NaN, dtype=flat_values.dtype)
full_eofs[:, np.logical_not(missing_features)] = eofs
full_eofs = full_eofs.reshape([n_components,] + original_shape)
eof_coords = {d: da.coords[d] for d in feature_dims}
eof_coords['component'] = np.arange(n_components)
eof_dims = ['component'] + feature_dims
feature_means = np.full((n_features,), np.NaN, dtype=flat_values.dtype)
feature_means[np.logical_not(missing_features)] = base_period_means
feature_means = feature_means.reshape(original_shape)
pcs_da = xr.DataArray(
pcs,
coords={sample_dim: da[sample_dim],
'component': np.arange(n_components)},
dims=[sample_dim, 'component'], name='principal_components')
eofs_da = xr.DataArray(
full_eofs, coords=eof_coords, dims=eof_dims, name='components')
explained_variance_ratio_da = xr.DataArray(
explained_variance_ratio,
coords={'component': np.arange(n_components)},
dims=['component'], name='explained_variance_ratio')
singular_values_da = xr.DataArray(
s, coords={'component': np.arange(n_components)},
dims=['component'], name='singular_values')
feature_means_da = xr.DataArray(
feature_means, coords={d: da.coords[d] for d in feature_dims},
dims=feature_dims, name='mean')
data_vars = {'eofs': eofs_da,
'principal_components': pcs_da,
'explained_variance_ratio': explained_variance_ratio_da,
'singular_values': singular_values_da,
'means': feature_means_da}
out_coords = dict(c for c in da.coords.items())
out_coords['component'] = np.arange(n_components)
return xr.Dataset(data_vars, coords=out_coords)
def get_eofs_output_filename(input_filename, hemisphere, region, eofs_base_period,
season, lat_weights, max_eofs, normalization):
"""Get filename for saving EOFs.
Parameters
----------
input_datafile : str
Name of the input datafile.
hemisphere : 'WG' | 'NH' | 'SH'
Hemisphere to perform EOF analysis on.
region : str
Name of region to perform EOF analysis on.
eofs_base_period : list
List of length 2 containing the first and last year used
for computing the EOFs.
season : 'ALL' | 'DJF' | 'MAM' | 'JJA' | 'SON'
Season to perform EOF analysis in.
lat_weights : str
String indicating the type of latitude weights used.
max_eofs : int
Number of EOFs retained.
normalization : str
Normalization convention used for the EOFs.
Returns
-------
filename : str
Name of the output file for the EOFs.
"""
basename, ext = os.path.splitext(os.path.basename(input_filename))
eofs_suffix = '{}.{}.{}_{}.{}.max_eofs_{:d}.{}.{}.eofs'.format(
hemisphere, region, pd.to_datetime(eofs_base_period[0]).strftime('%Y%m%d'),
pd.to_datetime(eofs_base_period[1]).strftime('%Y%m%d'), season,
max_eofs, lat_weights, normalization)
return '.'.join([basename, eofs_suffix]) + ext
# Hemispheres to compute EOFs for
HEMISPHERES = ['WG', 'NH', 'SH']
# Seasons to compute EOFs for
SEASONS = ['ALL', 'DJF', 'MAM', 'JJA', 'SON']
# Time period to use for calculating the EOFs
EOFS_BASE_PERIOD = CLIMATOLOGY_BASE_PERIOD
# Latitude weights to apply
LAT_WEIGHTS = 'scos'
# Normalization convention used for EOFs
EOFS_NORMALIZATION = 'unit'
# Whether to write the EOFs to file
SAVE_EOFS = True
# Random seed for truncated SVD
RANDOM_SEED = 0
for hemisphere in HEMISPHERES:
print('* Hemisphere: ', hemisphere)
regions = get_regions(hemisphere)
for region in regions:
print('\t- Region:', region)
for season in SEASONS:
print('\t\t+ Season: ', season, end='')
start_time = time.perf_counter()
anom_da = get_region_data(hgt_anom_da, hemisphere=hemisphere,
region=region, season=season)
lat_weights = get_latitude_weights(anom_da, weights_str=LAT_WEIGHTS)
eofs_ds = run_pca(anom_da, weights=lat_weights,
n_components=DEFAULT_MAX_EOFS,
normalization=EOFS_NORMALIZATION,
eofs_base_period=EOFS_BASE_PERIOD,
random_state=RANDOM_SEED)
eofs_ds.attrs['input_file'] = anom_output_file
eofs_ds.attrs['eofs_base_period_start'] = pd.to_datetime(EOFS_BASE_PERIOD[0]).strftime('%Y%m%d')
eofs_ds.attrs['eofs_base_period_end'] = pd.to_datetime(EOFS_BASE_PERIOD[1]).strftime('%Y%m%d')
eofs_ds.attrs['eofs_normalization'] = EOFS_NORMALIZATION
eofs_ds.attrs['lat_weights'] = LAT_WEIGHTS
eofs_output_filename = get_eofs_output_filename(
anom_output_file, hemisphere, region, EOFS_BASE_PERIOD,
season, LAT_WEIGHTS, DEFAULT_MAX_EOFS, EOFS_NORMALIZATION)
eofs_output_file = os.path.join(EOFS_NC_DIR, eofs_output_filename)
if SAVE_EOFS:
eofs_ds.to_netcdf(eofs_output_file)
end_time = time.perf_counter()
print(' ({:.2f} seconds)'.format(end_time - start_time))
###Output
_____no_output_____
###Markdown
Plots
###Code
# Whether to write plots to file
SAVE_PLOTS = True
def get_eof_plot_output_filename(eofs_datafile, n_eofs_to_plot, ext='pdf'):
"""Get filename to save plots of EOFs with.
Parameters
----------
eofs_datafile : str
Name of the datafile containing the EOFs to plot.
n_eofs_to_plot : int
Number of EOFs to plot.
ext : str, default: 'pdf'
File extension.
Returns
-------
filename : str
Name of the output file for the plot.
"""
basename, _ = os.path.splitext(os.path.basename(eofs_datafile))
suffix = 'k{}.eofs.{}'.format(n_eofs_to_plot, ext)
return '.'.join([basename, suffix])
###Output
_____no_output_____
###Markdown
Whole globe
###Code
def plot_global_eofs(eofs_ds, season, n_eofs_to_plot=20, cmap=plt.cm.RdBu_r,
lat_name=LAT_NAME, lon_name=LON_NAME):
"""Plot global EOFs.
Parameters
----------
eofs_ds : xarray.Dataset
Dataset containing the results of the EOF analysis.
season : 'ALL' | 'DJF' | 'MAM' | 'JJA' | 'SON'
Season to plot EOFs for.
n_eofs_to_plot : int, default: 20
Number of EOF patterns to plot.
cmap : object
Colormap to use.
lat_name : str
Name of latitude coordinate.
lon_name : str
Name of longitude coordinate.
Returns
-------
fig : figure
Plot of EOFs.
"""
hemisphere = 'WG'
region = 'all'
wrap_lon = True
projection = ccrs.PlateCarree(central_longitude=0)
components = eofs_ds['component'].isel(component=slice(0, n_eofs_to_plot)).values
component_vmins = np.empty(n_eofs_to_plot)
vmin = None
for i, component in enumerate(components):
component_vmin = eofs_ds['eofs'].sel(component=component).min().item()
if vmin is None or component_vmin < vmin:
vmin = component_vmin
component_vmins[:] = vmin
component_vmaxs = np.empty(n_eofs_to_plot)
vmax = None
for i, component in enumerate(components):
component_vmax = eofs_ds['eofs'].sel(component=component).max().item()
if vmax is None or component_vmax > vmax:
vmax = component_vmax
component_vmaxs[:] = vmax
if n_eofs_to_plot % 4 == 0:
ncols = 4
elif n_eofs_to_plot % 2 == 0:
ncols = 2
else:
ncols = 3
nrows = int(np.ceil(n_eofs_to_plot / ncols))
height_ratios = np.ones((nrows + 1))
height_ratios[-1] = 0.1
fig = plt.figure(constrained_layout=False, figsize=(6 * ncols, 3 * nrows))
gs = gridspec.GridSpec(ncols=ncols, nrows=nrows + 1, figure=fig,
wspace=0.05, hspace=0.2,
height_ratios=height_ratios)
lat = eofs_ds[lat_name]
lon = eofs_ds[lon_name]
row_index = 0
col_index = 0
for i, component in enumerate(components):
eof_data = eofs_ds['eofs'].sel(component=component).squeeze().values
expl_var = eofs_ds['explained_variance_ratio'].sel(
component=component).item()
if wrap_lon:
eof_data, eof_lon = add_cyclic_point(eof_data, coord=lon)
lon_grid, lat_grid = np.meshgrid(eof_lon, lat)
ax = fig.add_subplot(gs[row_index, col_index], projection=projection)
ax.coastlines()
ax.set_global()
ax_vmin = component_vmins[i]
ax_vmax = component_vmaxs[i]
cs = ax.pcolor(lon_grid, lat_grid, eof_data, vmin=ax_vmin, vmax=ax_vmax,
cmap=cmap, transform=ccrs.PlateCarree())
ax.set_title('EOF {} ({:.2f}%)'.format(
component + 1, expl_var * 100), fontsize=14)
ax.set_aspect('auto')
fig.canvas.draw()
col_index += 1
if col_index == ncols:
col_index = 0
row_index += 1
cb_ax = fig.add_subplot(gs[-1, :])
cb = fig.colorbar(cs, cax=cb_ax, pad=0.05, orientation='horizontal')
fig.suptitle('Hemisphere: {}, Region: {}, Season: {}'.format(hemisphere, region, season),
fontsize=16, x=0.5, y=0.92)
return fig
hemisphere = 'WG'
region = 'all'
seasons = ['ALL', 'DJF', 'MAM', 'JJA', 'SON']
n_eofs_to_plot = 20
for season in seasons:
eofs_datafile = os.path.join(
EOFS_NC_DIR,
get_eofs_output_filename(
anom_output_file, hemisphere, region, EOFS_BASE_PERIOD,
season, LAT_WEIGHTS, DEFAULT_MAX_EOFS, EOFS_NORMALIZATION))
eofs_ds = xr.open_dataset(eofs_datafile)
fig = plot_global_eofs(eofs_ds, season, n_eofs_to_plot=n_eofs_to_plot)
eofs_ds.close()
if SAVE_PLOTS:
output_filename = get_eof_plot_output_filename(eofs_datafile, n_eofs_to_plot)
output_file = os.path.join(EOFS_PLOTS_DIR, output_filename)
plt.savefig(output_file, bbox_inches='tight')
plt.show()
plt.close()
###Output
_____no_output_____
###Markdown
Northern Hemisphere
###Code
def plot_nh_eofs(eofs_ds, season, n_eofs_to_plot=20, cmap=plt.cm.RdBu_r,
lat_name=LAT_NAME, lon_name=LON_NAME):
"""Plot NH EOFs.
Parameters
----------
eofs_ds : xarray.Dataset
Dataset containing the results of the EOF analysis.
season : 'ALL' | 'DJF' | 'MAM' | 'JJA' | 'SON'
Season to plot EOFs for.
n_eofs_to_plot : int, default: 20
Number of EOF patterns to plot.
cmap : object
Colormap to use.
lat_name : str
Name of latitude coordinate.
lon_name : str
Name of longitude coordinate.
Returns
-------
fig : figure
Plot of EOFs.
"""
hemisphere = 'NH'
region = 'all'
wrap_lon = True
projection = ccrs.Orthographic(central_longitude=0, central_latitude=90)
components = eofs_ds['component'].isel(component=slice(0, n_eofs_to_plot)).values
component_vmins = np.empty(n_eofs_to_plot)
vmin = None
for i, component in enumerate(components):
component_vmin = eofs_ds['eofs'].sel(component=component).min().item()
if vmin is None or component_vmin < vmin:
vmin = component_vmin
component_vmins[:] = vmin
component_vmaxs = np.empty(n_eofs_to_plot)
vmax = None
for i, component in enumerate(components):
component_vmax = eofs_ds['eofs'].sel(component=component).max().item()
if vmax is None or component_vmax > vmax:
vmax = component_vmax
component_vmaxs[:] = vmax
if n_eofs_to_plot % 4 == 0:
ncols = 4
elif n_eofs_to_plot % 2 == 0:
ncols = 2
else:
ncols = 3
nrows = int(np.ceil(n_eofs_to_plot / ncols))
height_ratios = np.ones((nrows + 1))
height_ratios[-1] = 0.1
fig = plt.figure(constrained_layout=False, figsize=(4 * ncols, 4 * nrows))
gs = gridspec.GridSpec(ncols=ncols, nrows=nrows + 1, figure=fig,
wspace=0.01, hspace=0.2,
height_ratios=height_ratios)
lat = eofs_ds[lat_name]
lon = eofs_ds[lon_name]
row_index = 0
col_index = 0
for i, component in enumerate(components):
eof_data = eofs_ds['eofs'].sel(component=component).squeeze().values
expl_var = eofs_ds['explained_variance_ratio'].sel(
component=component).item()
if wrap_lon:
eof_data, eof_lon = add_cyclic_point(eof_data, coord=lon)
lon_grid, lat_grid = np.meshgrid(eof_lon, lat)
ax = fig.add_subplot(gs[row_index, col_index], projection=projection)
ax.coastlines()
ax.set_global()
ax_vmin = component_vmins[i]
ax_vmax = component_vmaxs[i]
cs = ax.pcolor(lon_grid, lat_grid, eof_data, vmin=ax_vmin, vmax=ax_vmax,
cmap=cmap, transform=ccrs.PlateCarree())
ax.set_title('EOF {} ({:.2f}%)'.format(
component + 1, expl_var * 100), fontsize=14)
ax.set_aspect('equal')
fig.canvas.draw()
col_index += 1
if col_index == ncols:
col_index = 0
row_index += 1
cb_ax = fig.add_subplot(gs[-1, :])
cb = fig.colorbar(cs, cax=cb_ax, pad=0.05, orientation='horizontal')
fig.suptitle('Hemisphere: {}, Region: {}, Season: {}'.format(hemisphere, region, season),
fontsize=16, x=0.5, y=0.92)
return fig
hemisphere = 'NH'
region = 'all'
seasons = ['ALL', 'DJF', 'MAM', 'JJA', 'SON']
n_eofs_to_plot = 20
for season in seasons:
eofs_datafile = os.path.join(
EOFS_NC_DIR,
get_eofs_output_filename(
anom_output_file, hemisphere, region, EOFS_BASE_PERIOD,
season, LAT_WEIGHTS, DEFAULT_MAX_EOFS, EOFS_NORMALIZATION))
eofs_ds = xr.open_dataset(eofs_datafile)
fig = plot_nh_eofs(eofs_ds, season, n_eofs_to_plot=n_eofs_to_plot)
eofs_ds.close()
if SAVE_PLOTS:
output_filename = get_eof_plot_output_filename(eofs_datafile, n_eofs_to_plot)
output_file = os.path.join(EOFS_PLOTS_DIR, output_filename)
plt.savefig(output_file, bbox_inches='tight')
plt.show()
plt.close()
def plot_nh_atlantic_eofs(eofs_ds, season, n_eofs_to_plot=20, cmap=plt.cm.RdBu_r,
lat_name=LAT_NAME, lon_name=LON_NAME):
"""Plot NH Atlantic region EOFs.
Parameters
----------
eofs_ds : xarray.Dataset
Dataset containing the results of the EOF analysis.
season : 'ALL' | 'DJF' | 'MAM' | 'JJA' | 'SON'
Season to plot EOFs for.
n_eofs_to_plot : int, default: 20
Number of EOF patterns to plot.
cmap : object
Colormap to use.
lat_name : str
Name of latitude coordinate.
lon_name : str
Name of longitude coordinate.
Returns
-------
fig : figure
Plot of EOFs.
"""
hemisphere = 'NH'
region = 'atlantic'
lon_bounds = get_region_lon_bounds(hemisphere, region)
lat_bounds = [0.0, 90.0]
wrap_lon = False
projection = ccrs.EquidistantConic(central_longitude=np.mean(lon_bounds), central_latitude=90)
components = eofs_ds['component'].isel(component=slice(0, n_eofs_to_plot)).values
component_vmins = np.empty(n_eofs_to_plot)
vmin = None
for i, component in enumerate(components):
component_vmin = eofs_ds['eofs'].sel(component=component).min().item()
if vmin is None or component_vmin < vmin:
vmin = component_vmin
component_vmins[:] = vmin
component_vmaxs = np.empty(n_eofs_to_plot)
vmax = None
for i, component in enumerate(components):
component_vmax = eofs_ds['eofs'].sel(component=component).max().item()
if vmax is None or component_vmax > vmax:
vmax = component_vmax
component_vmaxs[:] = vmax
if n_eofs_to_plot % 4 == 0:
ncols = 4
elif n_eofs_to_plot % 2 == 0:
ncols = 2
else:
ncols = 3
nrows = int(np.ceil(n_eofs_to_plot / ncols))
height_ratios = np.ones((nrows + 1))
height_ratios[-1] = 0.1
fig = plt.figure(constrained_layout=False, figsize=(4 * ncols, 4 * nrows))
gs = gridspec.GridSpec(ncols=ncols, nrows=nrows + 1, figure=fig,
wspace=0.1, hspace=0.2,
height_ratios=height_ratios)
lat = eofs_ds[lat_name].values
lon = eofs_ds[lon_name].values
row_index = 0
col_index = 0
for i, component in enumerate(components):
eof_data = eofs_ds['eofs'].sel(component=component).squeeze().values
expl_var = eofs_ds['explained_variance_ratio'].sel(
component=component).item()
if wrap_lon:
eof_data, eof_lon = add_cyclic_point(eof_data, coord=lon)
else:
eof_lon = lon
lon_grid, lat_grid = np.meshgrid(eof_lon, lat)
ax = fig.add_subplot(gs[row_index, col_index], projection=projection)
ax.coastlines()
left_bound_verts = np.vstack([np.repeat(lon_bounds[0], 100), np.flipud(np.linspace(0, 90.0, 100))]).T
right_bound_verts = np.vstack([np.repeat(lon_bounds[1], 100), np.linspace(0, 90.0, 100)]).T
lower_bound_verts = np.vstack([np.linspace(lon_bounds[0], lon_bounds[1], 100),
np.repeat(0.0, 100)]).T
upper_bound_verts = np.vstack([np.flipud(np.linspace(lon_bounds[0], lon_bounds[1], 100)),
np.repeat(90.0, 100)]).T
boundary = mpath.Path(np.vstack([left_bound_verts, lower_bound_verts, right_bound_verts, upper_bound_verts]))
ax.set_boundary(boundary, transform=ccrs.PlateCarree())
ax.set_extent(np.concatenate([lon_bounds, lat_bounds]), crs=ccrs.PlateCarree())
ax_vmin = component_vmins[i]
ax_vmax = component_vmaxs[i]
cs = ax.pcolor(lon_grid, lat_grid, eof_data, vmin=ax_vmin, vmax=ax_vmax,
cmap=cmap, transform=ccrs.PlateCarree())
ax.set_title('EOF {} ({:.2f}%)'.format(
component + 1, expl_var * 100), fontsize=14)
fig.canvas.draw()
col_index += 1
if col_index == ncols:
col_index = 0
row_index += 1
cb_ax = fig.add_subplot(gs[-1, :])
cb = fig.colorbar(cs, cax=cb_ax, pad=0.05, orientation='horizontal')
fig.suptitle('Hemisphere: {}, Region: {}, Season: {}'.format(hemisphere, region, season),
fontsize=16, x=0.5, y=0.92)
return fig
hemisphere = 'NH'
region = 'atlantic'
seasons = ['ALL', 'DJF', 'MAM', 'JJA', 'SON']
n_eofs_to_plot = 20
for season in seasons:
eofs_datafile = os.path.join(
EOFS_NC_DIR,
get_eofs_output_filename(
anom_output_file, hemisphere, region, EOFS_BASE_PERIOD,
season, LAT_WEIGHTS, DEFAULT_MAX_EOFS, EOFS_NORMALIZATION))
eofs_ds = xr.open_dataset(eofs_datafile)
fig = plot_nh_atlantic_eofs(eofs_ds, season, n_eofs_to_plot=n_eofs_to_plot)
eofs_ds.close()
if SAVE_PLOTS:
output_filename = get_eof_plot_output_filename(eofs_datafile, n_eofs_to_plot)
output_file = os.path.join(EOFS_PLOTS_DIR, output_filename)
plt.savefig(output_file, bbox_inches='tight')
plt.show()
plt.close()
def plot_nh_atlantic2_eofs(eofs_ds, season, n_eofs_to_plot=20, cmap=plt.cm.RdBu_r,
lat_name=LAT_NAME, lon_name=LON_NAME):
"""Plot NH Atlantic-2 region EOFs.
Parameters
----------
eofs_ds : xarray.Dataset
Dataset containing the results of the EOF analysis.
season : 'ALL' | 'DJF' | 'MAM' | 'JJA' | 'SON'
Season to plot EOFs for.
n_eofs_to_plot : int, default: 20
Number of EOF patterns to plot.
cmap : object
Colormap to use.
lat_name : str
Name of latitude coordinate.
lon_name : str
Name of longitude coordinate.
Returns
-------
fig : figure
Plot of EOFs.
"""
hemisphere = 'NH'
region = 'atlantic2'
lon_bounds = get_region_lon_bounds(hemisphere, region)
lat_bounds = [0.0, 90.0]
wrap_lon = False
projection = ccrs.EquidistantConic(central_longitude=np.mean(lon_bounds), central_latitude=90)
components = eofs_ds['component'].isel(component=slice(0, n_eofs_to_plot)).values
component_vmins = np.empty(n_eofs_to_plot)
vmin = None
for i, component in enumerate(components):
component_vmin = eofs_ds['eofs'].sel(component=component).min().item()
if vmin is None or component_vmin < vmin:
vmin = component_vmin
component_vmins[:] = vmin
component_vmaxs = np.empty(n_eofs_to_plot)
vmax = None
for i, component in enumerate(components):
component_vmax = eofs_ds['eofs'].sel(component=component).max().item()
if vmax is None or component_vmax > vmax:
vmax = component_vmax
component_vmaxs[:] = vmax
if n_eofs_to_plot % 4 == 0:
ncols = 4
elif n_eofs_to_plot % 2 == 0:
ncols = 2
else:
ncols = 3
nrows = int(np.ceil(n_eofs_to_plot / ncols))
height_ratios = np.ones((nrows + 1))
height_ratios[-1] = 0.1
fig = plt.figure(constrained_layout=False, figsize=(4 * ncols, 4 * nrows))
gs = gridspec.GridSpec(ncols=ncols, nrows=nrows + 1, figure=fig,
wspace=0.1, hspace=0.2,
height_ratios=height_ratios)
lat = eofs_ds[lat_name].values
lon = eofs_ds[lon_name].values
row_index = 0
col_index = 0
for i, component in enumerate(components):
eof_data = eofs_ds['eofs'].sel(component=component).squeeze().values
expl_var = eofs_ds['explained_variance_ratio'].sel(
component=component).item()
if wrap_lon:
eof_data, eof_lon = add_cyclic_point(eof_data, coord=lon)
else:
eof_lon = lon
lon_grid, lat_grid = np.meshgrid(eof_lon, lat)
ax = fig.add_subplot(gs[row_index, col_index], projection=projection)
ax.coastlines()
left_bound_verts = np.vstack([np.repeat(lon_bounds[0], 100), np.flipud(np.linspace(0, 90.0, 100))]).T
right_bound_verts = np.vstack([np.repeat(lon_bounds[1], 100), np.linspace(0, 90.0, 100)]).T
lower_bound_verts = np.vstack([np.linspace(lon_bounds[0], lon_bounds[1], 100),
np.repeat(0.0, 100)]).T
upper_bound_verts = np.vstack([np.flipud(np.linspace(lon_bounds[0], lon_bounds[1], 100)),
np.repeat(90.0, 100)]).T
boundary = mpath.Path(np.vstack([left_bound_verts, lower_bound_verts, right_bound_verts, upper_bound_verts]))
ax.set_boundary(boundary, transform=ccrs.PlateCarree())
ax.set_extent(np.concatenate([lon_bounds, lat_bounds]), crs=ccrs.PlateCarree())
ax_vmin = component_vmins[i]
ax_vmax = component_vmaxs[i]
cs = ax.pcolor(lon_grid, lat_grid, eof_data, vmin=ax_vmin, vmax=ax_vmax,
cmap=cmap, transform=ccrs.PlateCarree())
ax.set_title('EOF {} ({:.2f}%)'.format(
component + 1, expl_var * 100), fontsize=14)
fig.canvas.draw()
col_index += 1
if col_index == ncols:
col_index = 0
row_index += 1
cb_ax = fig.add_subplot(gs[-1, :])
cb = fig.colorbar(cs, cax=cb_ax, pad=0.05, orientation='horizontal')
fig.suptitle('Hemisphere: {}, Region: {}, Season: {}'.format(hemisphere, region, season),
fontsize=16, x=0.5, y=0.92)
return fig
hemisphere = 'NH'
region = 'atlantic2'
seasons = ['ALL', 'DJF', 'MAM', 'JJA', 'SON']
n_eofs_to_plot = 20
for season in seasons:
eofs_datafile = os.path.join(
EOFS_NC_DIR,
get_eofs_output_filename(
anom_output_file, hemisphere, region, EOFS_BASE_PERIOD,
season, LAT_WEIGHTS, DEFAULT_MAX_EOFS, EOFS_NORMALIZATION))
eofs_ds = xr.open_dataset(eofs_datafile)
fig = plot_nh_atlantic2_eofs(eofs_ds, season, n_eofs_to_plot=n_eofs_to_plot)
eofs_ds.close()
if SAVE_PLOTS:
output_filename = get_eof_plot_output_filename(eofs_datafile, n_eofs_to_plot)
output_file = os.path.join(EOFS_PLOTS_DIR, output_filename)
plt.savefig(output_file, bbox_inches='tight')
plt.show()
plt.close()
def plot_nh_atlantic3_eofs(eofs_ds, season, n_eofs_to_plot=20, cmap=plt.cm.RdBu_r,
lat_name=LAT_NAME, lon_name=LON_NAME):
"""Plot NH Atlantic-3 region EOFs.
Parameters
----------
eofs_ds : xarray.Dataset
Dataset containing the results of the EOF analysis.
season : 'ALL' | 'DJF' | 'MAM' | 'JJA' | 'SON'
Season to plot EOFs for.
n_eofs_to_plot : int, default: 20
Number of EOF patterns to plot.
cmap : object
Colormap to use.
lat_name : str
Name of latitude coordinate.
lon_name : str
Name of longitude coordinate.
Returns
-------
fig : figure
Plot of EOFs.
"""
hemisphere = 'NH'
region = 'atlantic3'
lon_bounds = get_region_lon_bounds(hemisphere, region)
lat_bounds = [0.0, 90.0]
wrap_lon = False
central_longitude = 0.5 * (lon_bounds[1] % 180 + lon_bounds[0] % 180) % 360
projection = ccrs.EquidistantConic(central_longitude=central_longitude, central_latitude=90)
components = eofs_ds['component'].isel(component=slice(0, n_eofs_to_plot)).values
component_vmins = np.empty(n_eofs_to_plot)
vmin = None
for i, component in enumerate(components):
component_vmin = eofs_ds['eofs'].sel(component=component).min().item()
if vmin is None or component_vmin < vmin:
vmin = component_vmin
component_vmins[:] = vmin
component_vmaxs = np.empty(n_eofs_to_plot)
vmax = None
for i, component in enumerate(components):
component_vmax = eofs_ds['eofs'].sel(component=component).max().item()
if vmax is None or component_vmax > vmax:
vmax = component_vmax
component_vmaxs[:] = vmax
if n_eofs_to_plot % 4 == 0:
ncols = 4
elif n_eofs_to_plot % 2 == 0:
ncols = 2
else:
ncols = 3
nrows = int(np.ceil(n_eofs_to_plot / ncols))
height_ratios = np.ones((nrows + 1))
height_ratios[-1] = 0.1
fig = plt.figure(constrained_layout=False, figsize=(4 * ncols, 4 * nrows))
gs = gridspec.GridSpec(ncols=ncols, nrows=nrows + 1, figure=fig,
wspace=0.1, hspace=0.2,
height_ratios=height_ratios)
lat = eofs_ds[lat_name].values
lon = eofs_ds[lon_name].values
row_index = 0
col_index = 0
for i, component in enumerate(components):
eof_data = eofs_ds['eofs'].sel(component=component).squeeze().values
expl_var = eofs_ds['explained_variance_ratio'].sel(
component=component).item()
if wrap_lon:
eof_data, eof_lon = add_cyclic_point(eof_data, coord=lon)
else:
eof_lon = lon
lon_grid, lat_grid = np.meshgrid(eof_lon, lat)
ax = fig.add_subplot(gs[row_index, col_index], projection=projection)
ax.coastlines()
left_bound_verts = np.vstack([np.repeat(lon_bounds[0], 100), np.flipud(np.linspace(0, 90.0, 100))]).T
right_bound_verts = np.vstack([np.repeat(lon_bounds[1], 100), np.linspace(0, 90.0, 100)]).T
lower_bound_verts = np.vstack([np.linspace(lon_bounds[0], lon_bounds[1], 100),
np.repeat(0.0, 100)]).T
upper_bound_verts = np.vstack([np.flipud(np.linspace(lon_bounds[0], lon_bounds[1], 100)),
np.repeat(90.0, 100)]).T
boundary = mpath.Path(np.vstack([left_bound_verts, lower_bound_verts, right_bound_verts, upper_bound_verts]))
ax.set_boundary(boundary, transform=ccrs.PlateCarree())
ax.set_extent(np.concatenate([lon_bounds, lat_bounds]), crs=ccrs.PlateCarree())
ax_vmin = component_vmins[i]
ax_vmax = component_vmaxs[i]
cs = ax.pcolor(lon_grid, lat_grid, eof_data, vmin=ax_vmin, vmax=ax_vmax,
cmap=cmap, transform=ccrs.PlateCarree())
ax.set_title('EOF {} ({:.2f}%)'.format(
component + 1, expl_var * 100), fontsize=14)
fig.canvas.draw()
col_index += 1
if col_index == ncols:
col_index = 0
row_index += 1
cb_ax = fig.add_subplot(gs[-1, :])
cb = fig.colorbar(cs, cax=cb_ax, pad=0.05, orientation='horizontal')
fig.suptitle('Hemisphere: {}, Region: {}, Season: {}'.format(hemisphere, region, season),
fontsize=16, x=0.5, y=0.92)
return fig
hemisphere = 'NH'
region = 'atlantic3'
seasons = ['ALL', 'DJF', 'MAM', 'JJA', 'SON']
n_eofs_to_plot = 20
for season in seasons:
eofs_datafile = os.path.join(
EOFS_NC_DIR,
get_eofs_output_filename(
anom_output_file, hemisphere, region, EOFS_BASE_PERIOD,
season, LAT_WEIGHTS, DEFAULT_MAX_EOFS, EOFS_NORMALIZATION))
eofs_ds = xr.open_dataset(eofs_datafile)
fig = plot_nh_atlantic3_eofs(eofs_ds, season, n_eofs_to_plot=n_eofs_to_plot)
eofs_ds.close()
if SAVE_PLOTS:
output_filename = get_eof_plot_output_filename(eofs_datafile, n_eofs_to_plot)
output_file = os.path.join(EOFS_PLOTS_DIR, output_filename)
plt.savefig(output_file, bbox_inches='tight')
plt.show()
plt.close()
def plot_nh_eurasia_eofs(eofs_ds, season, n_eofs_to_plot=20, cmap=plt.cm.RdBu_r,
lat_name=LAT_NAME, lon_name=LON_NAME):
"""Plot NH Eurasia region EOFs.
Parameters
----------
eofs_ds : xarray.Dataset
Dataset containing the results of the EOF analysis.
season : 'ALL' | 'DJF' | 'MAM' | 'JJA' | 'SON'
Season to plot EOFs for.
n_eofs_to_plot : int, default: 20
Number of EOF patterns to plot.
cmap : object
Colormap to use.
lat_name : str
Name of latitude coordinate.
lon_name : str
Name of longitude coordinate.
Returns
-------
fig : figure
Plot of EOFs.
"""
hemisphere = 'NH'
region = 'eurasia'
lon_bounds = get_region_lon_bounds(hemisphere, region)
lat_bounds = [0.0, 90.0]
wrap_lon = False
projection = ccrs.EquidistantConic(central_longitude=np.mean(lon_bounds), central_latitude=90)
components = eofs_ds['component'].isel(component=slice(0, n_eofs_to_plot)).values
component_vmins = np.empty(n_eofs_to_plot)
vmin = None
for i, component in enumerate(components):
component_vmin = eofs_ds['eofs'].sel(component=component).min().item()
if vmin is None or component_vmin < vmin:
vmin = component_vmin
component_vmins[:] = vmin
component_vmaxs = np.empty(n_eofs_to_plot)
vmax = None
for i, component in enumerate(components):
component_vmax = eofs_ds['eofs'].sel(component=component).max().item()
if vmax is None or component_vmax > vmax:
vmax = component_vmax
component_vmaxs[:] = vmax
if n_eofs_to_plot % 4 == 0:
ncols = 4
elif n_eofs_to_plot % 2 == 0:
ncols = 2
else:
ncols = 3
nrows = int(np.ceil(n_eofs_to_plot / ncols))
height_ratios = np.ones((nrows + 1))
height_ratios[-1] = 0.1
fig = plt.figure(constrained_layout=False, figsize=(4 * ncols, 4 * nrows))
gs = gridspec.GridSpec(ncols=ncols, nrows=nrows + 1, figure=fig,
wspace=0.1, hspace=0.2,
height_ratios=height_ratios)
lat = eofs_ds[lat_name].values
lon = eofs_ds[lon_name].values
row_index = 0
col_index = 0
for i, component in enumerate(components):
eof_data = eofs_ds['eofs'].sel(component=component).squeeze().values
expl_var = eofs_ds['explained_variance_ratio'].sel(
component=component).item()
if wrap_lon:
eof_data, eof_lon = add_cyclic_point(eof_data, coord=lon)
else:
eof_lon = lon
lon_grid, lat_grid = np.meshgrid(eof_lon, lat)
ax = fig.add_subplot(gs[row_index, col_index], projection=projection)
ax.coastlines()
left_bound_verts = np.vstack([np.repeat(lon_bounds[0], 100), np.flipud(np.linspace(0, 90.0, 100))]).T
right_bound_verts = np.vstack([np.repeat(lon_bounds[1], 100), np.linspace(0, 90.0, 100)]).T
lower_bound_verts = np.vstack([np.linspace(lon_bounds[0], lon_bounds[1], 100),
np.repeat(0.0, 100)]).T
upper_bound_verts = np.vstack([np.flipud(np.linspace(lon_bounds[0], lon_bounds[1], 100)),
np.repeat(90.0, 100)]).T
boundary = mpath.Path(np.vstack([left_bound_verts, lower_bound_verts, right_bound_verts, upper_bound_verts]))
ax.set_boundary(boundary, transform=ccrs.PlateCarree())
ax.set_extent(np.concatenate([lon_bounds, lat_bounds]), crs=ccrs.PlateCarree())
ax_vmin = component_vmins[i]
ax_vmax = component_vmaxs[i]
cs = ax.pcolor(lon_grid, lat_grid, eof_data, vmin=ax_vmin, vmax=ax_vmax,
cmap=cmap, transform=ccrs.PlateCarree())
ax.set_title('EOF {} ({:.2f}%)'.format(
component + 1, expl_var * 100), fontsize=14)
fig.canvas.draw()
col_index += 1
if col_index == ncols:
col_index = 0
row_index += 1
cb_ax = fig.add_subplot(gs[-1, :])
cb = fig.colorbar(cs, cax=cb_ax, pad=0.05, orientation='horizontal')
fig.suptitle('Hemisphere: {}, Region: {}, Season: {}'.format(hemisphere, region, season),
fontsize=16, x=0.5, y=0.92)
return fig
hemisphere = 'NH'
region = 'eurasia'
seasons = ['ALL', 'DJF', 'MAM', 'JJA', 'SON']
n_eofs_to_plot = 20
for season in seasons:
eofs_datafile = os.path.join(
EOFS_NC_DIR,
get_eofs_output_filename(
anom_output_file, hemisphere, region, EOFS_BASE_PERIOD,
season, LAT_WEIGHTS, DEFAULT_MAX_EOFS, EOFS_NORMALIZATION))
eofs_ds = xr.open_dataset(eofs_datafile)
fig = plot_nh_eurasia_eofs(eofs_ds, season, n_eofs_to_plot=n_eofs_to_plot)
eofs_ds.close()
if SAVE_PLOTS:
output_filename = get_eof_plot_output_filename(eofs_datafile, n_eofs_to_plot)
output_file = os.path.join(EOFS_PLOTS_DIR, output_filename)
plt.savefig(output_file, bbox_inches='tight')
plt.show()
plt.close()
def plot_nh_pacific_eofs(eofs_ds, season, n_eofs_to_plot=20, cmap=plt.cm.RdBu_r,
lat_name=LAT_NAME, lon_name=LON_NAME):
"""Plot NH Pacific region EOFs.
Parameters
----------
eofs_ds : xarray.Dataset
Dataset containing the results of the EOF analysis.
season : 'ALL' | 'DJF' | 'MAM' | 'JJA' | 'SON'
Season to plot EOFs for.
n_eofs_to_plot : int, default: 20
Number of EOF patterns to plot.
cmap : object
Colormap to use.
lat_name : str
Name of latitude coordinate.
lon_name : str
Name of longitude coordinate.
Returns
-------
fig : figure
Plot of EOFs.
"""
hemisphere = 'NH'
region = 'pacific'
lon_bounds = get_region_lon_bounds(hemisphere, region)
lat_bounds = [0.0, 90.0]
wrap_lon = False
projection = ccrs.EquidistantConic(central_longitude=np.mean(lon_bounds), central_latitude=90)
components = eofs_ds['component'].isel(component=slice(0, n_eofs_to_plot)).values
component_vmins = np.empty(n_eofs_to_plot)
vmin = None
for i, component in enumerate(components):
component_vmin = eofs_ds['eofs'].sel(component=component).min().item()
if vmin is None or component_vmin < vmin:
vmin = component_vmin
component_vmins[:] = vmin
component_vmaxs = np.empty(n_eofs_to_plot)
vmax = None
for i, component in enumerate(components):
component_vmax = eofs_ds['eofs'].sel(component=component).max().item()
if vmax is None or component_vmax > vmax:
vmax = component_vmax
component_vmaxs[:] = vmax
if n_eofs_to_plot % 4 == 0:
ncols = 4
elif n_eofs_to_plot % 2 == 0:
ncols = 2
else:
ncols = 3
nrows = int(np.ceil(n_eofs_to_plot / ncols))
height_ratios = np.ones((nrows + 1))
height_ratios[-1] = 0.1
fig = plt.figure(constrained_layout=False, figsize=(4 * ncols, 4 * nrows))
gs = gridspec.GridSpec(ncols=ncols, nrows=nrows + 1, figure=fig,
wspace=0.1, hspace=0.2,
height_ratios=height_ratios)
lat = eofs_ds[lat_name].values
lon = eofs_ds[lon_name].values
row_index = 0
col_index = 0
for i, component in enumerate(components):
eof_data = eofs_ds['eofs'].sel(component=component).squeeze().values
expl_var = eofs_ds['explained_variance_ratio'].sel(
component=component).item()
if wrap_lon:
eof_data, eof_lon = add_cyclic_point(eof_data, coord=lon)
else:
eof_lon = lon
lon_grid, lat_grid = np.meshgrid(eof_lon, lat)
ax = fig.add_subplot(gs[row_index, col_index], projection=projection)
ax.coastlines()
left_bound_verts = np.vstack([np.repeat(lon_bounds[0], 100), np.flipud(np.linspace(0, 90.0, 100))]).T
right_bound_verts = np.vstack([np.repeat(lon_bounds[1], 100), np.linspace(0, 90.0, 100)]).T
lower_bound_verts = np.vstack([np.linspace(lon_bounds[0], lon_bounds[1], 100),
np.repeat(0.0, 100)]).T
upper_bound_verts = np.vstack([np.flipud(np.linspace(lon_bounds[0], lon_bounds[1], 100)),
np.repeat(90.0, 100)]).T
boundary = mpath.Path(np.vstack([left_bound_verts, lower_bound_verts, right_bound_verts, upper_bound_verts]))
ax.set_boundary(boundary, transform=ccrs.PlateCarree())
ax.set_extent(np.concatenate([lon_bounds, lat_bounds]), crs=ccrs.PlateCarree())
ax_vmin = component_vmins[i]
ax_vmax = component_vmaxs[i]
cs = ax.pcolor(lon_grid, lat_grid, eof_data, vmin=ax_vmin, vmax=ax_vmax,
cmap=cmap, transform=ccrs.PlateCarree())
ax.set_title('EOF {} ({:.2f}%)'.format(
component + 1, expl_var * 100), fontsize=14)
fig.canvas.draw()
col_index += 1
if col_index == ncols:
col_index = 0
row_index += 1
cb_ax = fig.add_subplot(gs[-1, :])
cb = fig.colorbar(cs, cax=cb_ax, pad=0.05, orientation='horizontal')
fig.suptitle('Hemisphere: {}, Region: {}, Season: {}'.format(hemisphere, region, season),
fontsize=16, x=0.5, y=0.92)
return fig
hemisphere = 'NH'
region = 'pacific'
seasons = ['ALL', 'DJF', 'MAM', 'JJA', 'SON']
n_eofs_to_plot = 20
for season in seasons:
eofs_datafile = os.path.join(
EOFS_NC_DIR,
get_eofs_output_filename(
anom_output_file, hemisphere, region, EOFS_BASE_PERIOD,
season, LAT_WEIGHTS, DEFAULT_MAX_EOFS, EOFS_NORMALIZATION))
eofs_ds = xr.open_dataset(eofs_datafile)
fig = plot_nh_pacific_eofs(eofs_ds, season, n_eofs_to_plot=n_eofs_to_plot)
eofs_ds.close()
if SAVE_PLOTS:
output_filename = get_eof_plot_output_filename(eofs_datafile, n_eofs_to_plot)
output_file = os.path.join(EOFS_PLOTS_DIR, output_filename)
plt.savefig(output_file, bbox_inches='tight')
plt.show()
plt.close()
def plot_nh_atlantic_eurasia_eofs(eofs_ds, season, n_eofs_to_plot=20, cmap=plt.cm.RdBu_r,
lat_name=LAT_NAME, lon_name=LON_NAME):
"""Plot NH Atlantic-Eurasia region EOFs.
Parameters
----------
eofs_ds : xarray.Dataset
Dataset containing the results of the EOF analysis.
season : 'ALL' | 'DJF' | 'MAM' | 'JJA' | 'SON'
Season to plot EOFs for.
n_eofs_to_plot : int, default: 20
Number of EOF patterns to plot.
cmap : object
Colormap to use.
lat_name : str
Name of latitude coordinate.
lon_name : str
Name of longitude coordinate.
Returns
-------
fig : figure
Plot of EOFs.
"""
hemisphere = 'NH'
region = 'atlantic_eurasia'
lon_bounds = get_region_lon_bounds(hemisphere, region)
lon_bounds[lon_bounds > 180] -= 360
lat_bounds = [0.0, 90.0]
wrap_lon = False
projection = ccrs.EquidistantConic(central_longitude=0, central_latitude=90)
components = eofs_ds['component'].isel(component=slice(0, n_eofs_to_plot)).values
component_vmins = np.empty(n_eofs_to_plot)
vmin = None
for i, component in enumerate(components):
component_vmin = eofs_ds['eofs'].sel(component=component).min().item()
if vmin is None or component_vmin < vmin:
vmin = component_vmin
component_vmins[:] = vmin
component_vmaxs = np.empty(n_eofs_to_plot)
vmax = None
for i, component in enumerate(components):
component_vmax = eofs_ds['eofs'].sel(component=component).max().item()
if vmax is None or component_vmax > vmax:
vmax = component_vmax
component_vmaxs[:] = vmax
if n_eofs_to_plot % 4 == 0:
ncols = 4
elif n_eofs_to_plot % 2 == 0:
ncols = 2
else:
ncols = 3
nrows = int(np.ceil(n_eofs_to_plot / ncols))
height_ratios = np.ones((nrows + 1))
height_ratios[-1] = 0.1
fig = plt.figure(constrained_layout=False, figsize=(4 * ncols, 3 * nrows))
gs = gridspec.GridSpec(ncols=ncols, nrows=nrows + 1, figure=fig,
wspace=0.1, hspace=0.01,
height_ratios=height_ratios)
lat = eofs_ds[lat_name].values
lon = eofs_ds[lon_name].values
lon[lon > 180] -= 360
row_index = 0
col_index = 0
for i, component in enumerate(components):
eof_data = eofs_ds['eofs'].sel(component=component).squeeze().values
expl_var = eofs_ds['explained_variance_ratio'].sel(
component=component).item()
if wrap_lon:
eof_data, eof_lon = add_cyclic_point(eof_data, coord=lon)
else:
eof_lon = lon
lon_grid, lat_grid = np.meshgrid(eof_lon, lat)
ax = fig.add_subplot(gs[row_index, col_index], projection=projection)
ax.coastlines()
left_bound_verts = np.vstack([np.repeat(lon_bounds[0], 100), np.flipud(np.linspace(0, 90.0, 100))]).T
right_bound_verts = np.vstack([np.repeat(lon_bounds[1], 100), np.linspace(0, 90.0, 100)]).T
lon_grid = np.sort(lon_grid, axis=1)
for lat_idx in range(lat_grid.shape[0]):
eof_data[lat_idx, :] = eof_data[lat_idx, np.argsort(lon)]
lower_bound_verts = np.vstack([np.linspace(lon_bounds[0], lon_bounds[1], 100), np.repeat(0.0, 100)]).T
upper_bound_verts = np.vstack([np.flipud(np.linspace(lon_bounds[0], lon_bounds[1], 100)),
np.repeat(90.0, 100)]).T
boundary = mpath.Path(np.vstack([left_bound_verts, lower_bound_verts, right_bound_verts, upper_bound_verts]))
ax.set_boundary(boundary, transform=ccrs.PlateCarree())
ax.set_extent(np.concatenate([lon_bounds, lat_bounds]), crs=ccrs.PlateCarree())
ax_vmin = component_vmins[i]
ax_vmax = component_vmaxs[i]
cs = ax.pcolor(lon_grid, lat_grid, eof_data, vmin=ax_vmin, vmax=ax_vmax,
cmap=cmap, transform=ccrs.PlateCarree())
ax.set_title('EOF {} ({:.2f}%)'.format(
component + 1, expl_var * 100), fontsize=14)
ax.set_aspect('equal')
fig.canvas.draw()
col_index += 1
if col_index == ncols:
col_index = 0
row_index += 1
cb_ax = fig.add_subplot(gs[-1, :])
cb = fig.colorbar(cs, cax=cb_ax, pad=0.05, orientation='horizontal')
fig.suptitle('Hemisphere: {}, Region: {}, Season: {}'.format(hemisphere, region, season),
fontsize=16, x=0.5, y=0.92)
return fig
hemisphere = 'NH'
region = 'atlantic_eurasia'
seasons = ['ALL', 'DJF', 'MAM', 'JJA', 'SON']
n_eofs_to_plot = 20
for season in seasons:
eofs_datafile = os.path.join(
EOFS_NC_DIR,
get_eofs_output_filename(
anom_output_file, hemisphere, region, EOFS_BASE_PERIOD,
season, LAT_WEIGHTS, DEFAULT_MAX_EOFS, EOFS_NORMALIZATION))
eofs_ds = xr.open_dataset(eofs_datafile)
fig = plot_nh_atlantic_eurasia_eofs(eofs_ds, season, n_eofs_to_plot=n_eofs_to_plot)
eofs_ds.close()
if SAVE_PLOTS:
output_filename = get_eof_plot_output_filename(eofs_datafile, n_eofs_to_plot)
output_file = os.path.join(EOFS_PLOTS_DIR, output_filename)
plt.savefig(output_file, bbox_inches='tight')
plt.show()
plt.close()
###Output
_____no_output_____
###Markdown
Southern Hemisphere
###Code
def plot_sh_eofs(eofs_ds, season, n_eofs_to_plot=20, cmap=plt.cm.RdBu_r,
lat_name=LAT_NAME, lon_name=LON_NAME):
"""Plot SH EOFs.
Parameters
----------
eofs_ds : xarray.Dataset
Dataset containing the results of the EOF analysis.
season : 'ALL' | 'DJF' | 'MAM' | 'JJA' | 'SON'
Season to plot EOFs for.
n_eofs_to_plot : int, default: 20
Number of EOF patterns to plot.
cmap : object
Colormap to use.
lat_name : str
Name of latitude coordinate.
lon_name : str
Name of longitude coordinate.
Returns
-------
fig : figure
Plot of EOFs.
"""
hemisphere = 'SH'
region = 'all'
wrap_lon = True
projection = ccrs.Orthographic(central_longitude=0, central_latitude=-90)
components = eofs_ds['component'].isel(component=slice(0, n_eofs_to_plot)).values
component_vmins = np.empty(n_eofs_to_plot)
vmin = None
for i, component in enumerate(components):
component_vmin = eofs_ds['eofs'].sel(component=component).min().item()
if vmin is None or component_vmin < vmin:
vmin = component_vmin
component_vmins[:] = vmin
component_vmaxs = np.empty(n_eofs_to_plot)
vmax = None
for i, component in enumerate(components):
component_vmax = eofs_ds['eofs'].sel(component=component).max().item()
if vmax is None or component_vmax > vmax:
vmax = component_vmax
component_vmaxs[:] = vmax
if n_eofs_to_plot % 4 == 0:
ncols = 4
elif n_eofs_to_plot % 2 == 0:
ncols = 2
else:
ncols = 3
nrows = int(np.ceil(n_eofs_to_plot / ncols))
height_ratios = np.ones((nrows + 1))
height_ratios[-1] = 0.1
fig = plt.figure(constrained_layout=False, figsize=(4 * ncols, 4 * nrows))
gs = gridspec.GridSpec(ncols=ncols, nrows=nrows + 1, figure=fig,
wspace=0.01, hspace=0.2,
height_ratios=height_ratios)
lat = eofs_ds[lat_name]
lon = eofs_ds[lon_name]
row_index = 0
col_index = 0
for i, component in enumerate(components):
eof_data = eofs_ds['eofs'].sel(component=component).squeeze().values
expl_var = eofs_ds['explained_variance_ratio'].sel(
component=component).item()
if wrap_lon:
eof_data, eof_lon = add_cyclic_point(eof_data, coord=lon)
lon_grid, lat_grid = np.meshgrid(eof_lon, lat)
ax = fig.add_subplot(gs[row_index, col_index], projection=projection)
ax.coastlines()
ax.set_global()
ax_vmin = component_vmins[i]
ax_vmax = component_vmaxs[i]
cs = ax.pcolor(lon_grid, lat_grid, eof_data, vmin=ax_vmin, vmax=ax_vmax,
cmap=cmap, transform=ccrs.PlateCarree())
ax.set_title('EOF {} ({:.2f}%)'.format(
component + 1, expl_var * 100), fontsize=14)
ax.set_aspect('equal')
fig.canvas.draw()
col_index += 1
if col_index == ncols:
col_index = 0
row_index += 1
cb_ax = fig.add_subplot(gs[-1, :])
cb = fig.colorbar(cs, cax=cb_ax, pad=0.05, orientation='horizontal')
fig.suptitle('Hemisphere: {}, Region: {}, Season: {}'.format(hemisphere, region, season),
fontsize=16, x=0.5, y=0.92)
return fig
hemisphere = 'SH'
region = 'all'
seasons = ['ALL', 'DJF', 'MAM', 'JJA', 'SON']
n_eofs_to_plot = 20
for season in seasons:
eofs_datafile = os.path.join(
EOFS_NC_DIR,
get_eofs_output_filename(
anom_output_file, hemisphere, region, EOFS_BASE_PERIOD,
season, LAT_WEIGHTS, DEFAULT_MAX_EOFS, EOFS_NORMALIZATION))
eofs_ds = xr.open_dataset(eofs_datafile)
fig = plot_sh_eofs(eofs_ds, season, n_eofs_to_plot=n_eofs_to_plot)
eofs_ds.close()
if SAVE_PLOTS:
output_filename = get_eof_plot_output_filename(eofs_datafile, n_eofs_to_plot)
output_file = os.path.join(EOFS_PLOTS_DIR, output_filename)
plt.savefig(output_file, bbox_inches='tight')
plt.show()
plt.close()
def plot_sh_australian_eofs(eofs_ds, season, n_eofs_to_plot=20, cmap=plt.cm.RdBu_r,
lat_name=LAT_NAME, lon_name=LON_NAME):
"""Plot SH Australian region EOFs.
Parameters
----------
eofs_ds : xarray.Dataset
Dataset containing the results of the EOF analysis.
season : 'ALL' | 'DJF' | 'MAM' | 'JJA' | 'SON'
Season to plot EOFs for.
n_eofs_to_plot : int, default: 20
Number of EOF patterns to plot.
cmap : object
Colormap to use.
lat_name : str
Name of latitude coordinate.
lon_name : str
Name of longitude coordinate.
Returns
-------
fig : figure
Plot of EOFs.
"""
hemisphere = 'SH'
region = 'australian'
lon_bounds = get_region_lon_bounds(hemisphere, region)
lat_bounds = [-90.0, 0.0]
wrap_lon = False
projection = ccrs.EquidistantConic(central_longitude=np.mean(lon_bounds), central_latitude=-90,
standard_parallels=(-20, -50))
components = eofs_ds['component'].isel(component=slice(0, n_eofs_to_plot)).values
component_vmins = np.empty(n_eofs_to_plot)
vmin = None
for i, component in enumerate(components):
component_vmin = eofs_ds['eofs'].sel(component=component).min().item()
if vmin is None or component_vmin < vmin:
vmin = component_vmin
component_vmins[:] = vmin
component_vmaxs = np.empty(n_eofs_to_plot)
vmax = None
for i, component in enumerate(components):
component_vmax = eofs_ds['eofs'].sel(component=component).max().item()
if vmax is None or component_vmax > vmax:
vmax = component_vmax
component_vmaxs[:] = vmax
if n_eofs_to_plot % 4 == 0:
ncols = 4
elif n_eofs_to_plot % 2 == 0:
ncols = 2
else:
ncols = 3
nrows = int(np.ceil(n_eofs_to_plot / ncols))
height_ratios = np.ones((nrows + 1))
height_ratios[-1] = 0.1
fig = plt.figure(constrained_layout=False, figsize=(4 * ncols, 4 * nrows))
gs = gridspec.GridSpec(ncols=ncols, nrows=nrows + 1, figure=fig,
wspace=0.1, hspace=0.2,
height_ratios=height_ratios)
lat = eofs_ds[lat_name].values
lon = eofs_ds[lon_name].values
row_index = 0
col_index = 0
for i, component in enumerate(components):
eof_data = eofs_ds['eofs'].sel(component=component).squeeze().values
expl_var = eofs_ds['explained_variance_ratio'].sel(
component=component).item()
if wrap_lon:
eof_data, eof_lon = add_cyclic_point(eof_data, coord=lon)
else:
eof_lon = lon
lon_grid, lat_grid = np.meshgrid(eof_lon, lat)
ax = fig.add_subplot(gs[row_index, col_index], projection=projection)
ax.coastlines()
left_bound_verts = np.vstack([np.repeat(lon_bounds[0], 100), np.flipud(np.linspace(-90.0, 0.0, 100))]).T
right_bound_verts = np.vstack([np.repeat(lon_bounds[1], 100), np.linspace(-90.0, 0.0, 100)]).T
lower_bound_verts = np.vstack([np.linspace(lon_bounds[0], lon_bounds[1], 100),
np.repeat(-90.0, 100)]).T
upper_bound_verts = np.vstack([np.flipud(np.linspace(lon_bounds[0], lon_bounds[1], 100)),
np.repeat(0.0, 100)]).T
boundary = mpath.Path(np.vstack([left_bound_verts, lower_bound_verts, right_bound_verts, upper_bound_verts]))
ax.set_boundary(boundary, transform=ccrs.PlateCarree())
ax.set_extent(np.concatenate([lon_bounds, lat_bounds]), crs=ccrs.PlateCarree())
ax_vmin = component_vmins[i]
ax_vmax = component_vmaxs[i]
cs = ax.pcolor(lon_grid, lat_grid, eof_data, vmin=ax_vmin, vmax=ax_vmax,
cmap=cmap, transform=ccrs.PlateCarree())
ax.set_title('EOF {} ({:.2f}%)'.format(
component + 1, expl_var * 100), fontsize=14)
fig.canvas.draw()
col_index += 1
if col_index == ncols:
col_index = 0
row_index += 1
cb_ax = fig.add_subplot(gs[-1, :])
cb = fig.colorbar(cs, cax=cb_ax, pad=0.05, orientation='horizontal')
fig.suptitle('Hemisphere: {}, Region: {}, Season: {}'.format(hemisphere, region, season),
fontsize=16, x=0.5, y=0.92)
return fig
hemisphere = 'SH'
region = 'australian'
seasons = ['ALL', 'DJF', 'MAM', 'JJA', 'SON']
n_eofs_to_plot = 20
for season in seasons:
eofs_datafile = os.path.join(
EOFS_NC_DIR,
get_eofs_output_filename(
anom_output_file, hemisphere, region, EOFS_BASE_PERIOD,
season, LAT_WEIGHTS, DEFAULT_MAX_EOFS, EOFS_NORMALIZATION))
eofs_ds = xr.open_dataset(eofs_datafile)
fig = plot_sh_australian_eofs(eofs_ds, season, n_eofs_to_plot=n_eofs_to_plot)
eofs_ds.close()
if SAVE_PLOTS:
output_filename = get_eof_plot_output_filename(eofs_datafile, n_eofs_to_plot)
output_file = os.path.join(EOFS_PLOTS_DIR, output_filename)
plt.savefig(output_file, bbox_inches='tight')
plt.show()
plt.close()
def plot_sh_full_pacific_eofs(eofs_ds, season, n_eofs_to_plot=20, cmap=plt.cm.RdBu_r,
lat_name=LAT_NAME, lon_name=LON_NAME):
"""Plot SH full Pacific region EOFs.
Parameters
----------
eofs_ds : xarray.Dataset
Dataset containing the results of the EOF analysis.
season : 'ALL' | 'DJF' | 'MAM' | 'JJA' | 'SON'
Season to plot EOFs for.
n_eofs_to_plot : int, default: 20
Number of EOF patterns to plot.
cmap : object
Colormap to use.
lat_name : str
Name of latitude coordinate.
lon_name : str
Name of longitude coordinate.
Returns
-------
fig : figure
Plot of EOFs.
"""
hemisphere = 'SH'
region = 'full_pacific'
lon_bounds = get_region_lon_bounds(hemisphere, region)
lat_bounds = [-90.0, 0.0]
wrap_lon = False
projection = ccrs.EquidistantConic(central_longitude=np.mean(lon_bounds), central_latitude=-90,
standard_parallels=(-20, -50))
components = eofs_ds['component'].isel(component=slice(0, n_eofs_to_plot)).values
component_vmins = np.empty(n_eofs_to_plot)
vmin = None
for i, component in enumerate(components):
component_vmin = eofs_ds['eofs'].sel(component=component).min().item()
if vmin is None or component_vmin < vmin:
vmin = component_vmin
component_vmins[:] = vmin
component_vmaxs = np.empty(n_eofs_to_plot)
vmax = None
for i, component in enumerate(components):
component_vmax = eofs_ds['eofs'].sel(component=component).max().item()
if vmax is None or component_vmax > vmax:
vmax = component_vmax
component_vmaxs[:] = vmax
if n_eofs_to_plot % 4 == 0:
ncols = 4
elif n_eofs_to_plot % 2 == 0:
ncols = 2
else:
ncols = 3
nrows = int(np.ceil(n_eofs_to_plot / ncols))
height_ratios = np.ones((nrows + 1))
height_ratios[-1] = 0.1
fig = plt.figure(constrained_layout=False, figsize=(4 * ncols, 4 * nrows))
gs = gridspec.GridSpec(ncols=ncols, nrows=nrows + 1, figure=fig,
wspace=0.1, hspace=0.2,
height_ratios=height_ratios)
lat = eofs_ds[lat_name].values
lon = eofs_ds[lon_name].values
row_index = 0
col_index = 0
for i, component in enumerate(components):
eof_data = eofs_ds['eofs'].sel(component=component).squeeze().values
expl_var = eofs_ds['explained_variance_ratio'].sel(
component=component).item()
if wrap_lon:
eof_data, eof_lon = add_cyclic_point(eof_data, coord=lon)
else:
eof_lon = lon
lon_grid, lat_grid = np.meshgrid(eof_lon, lat)
ax = fig.add_subplot(gs[row_index, col_index], projection=projection)
ax.coastlines()
left_bound_verts = np.vstack([np.repeat(lon_bounds[0], 100), np.flipud(np.linspace(-90.0, 0.0, 100))]).T
right_bound_verts = np.vstack([np.repeat(lon_bounds[1], 100), np.linspace(-90.0, 0.0, 100)]).T
lower_bound_verts = np.vstack([np.linspace(lon_bounds[0], lon_bounds[1], 100),
np.repeat(-90.0, 100)]).T
upper_bound_verts = np.vstack([np.flipud(np.linspace(lon_bounds[0], lon_bounds[1], 100)),
np.repeat(0.0, 100)]).T
boundary = mpath.Path(np.vstack([left_bound_verts, lower_bound_verts, right_bound_verts, upper_bound_verts]))
ax.set_boundary(boundary, transform=ccrs.PlateCarree())
ax.set_extent(np.concatenate([lon_bounds, lat_bounds]), crs=ccrs.PlateCarree())
ax_vmin = component_vmins[i]
ax_vmax = component_vmaxs[i]
cs = ax.pcolor(lon_grid, lat_grid, eof_data, vmin=ax_vmin, vmax=ax_vmax,
cmap=cmap, transform=ccrs.PlateCarree())
ax.set_title('EOF {} ({:.2f}%)'.format(
component + 1, expl_var * 100), fontsize=14)
fig.canvas.draw()
col_index += 1
if col_index == ncols:
col_index = 0
row_index += 1
cb_ax = fig.add_subplot(gs[-1, :])
cb = fig.colorbar(cs, cax=cb_ax, pad=0.05, orientation='horizontal')
fig.suptitle('Hemisphere: {}, Region: {}, Season: {}'.format(hemisphere, region, season),
fontsize=16, x=0.5, y=0.92)
return fig
hemisphere = 'SH'
region = 'full_pacific'
seasons = ['ALL', 'DJF', 'MAM', 'JJA', 'SON']
n_eofs_to_plot = 20
for season in seasons:
eofs_datafile = os.path.join(
EOFS_NC_DIR,
get_eofs_output_filename(
anom_output_file, hemisphere, region, EOFS_BASE_PERIOD,
season, LAT_WEIGHTS, DEFAULT_MAX_EOFS, EOFS_NORMALIZATION))
eofs_ds = xr.open_dataset(eofs_datafile)
fig = plot_sh_full_pacific_eofs(eofs_ds, season, n_eofs_to_plot=n_eofs_to_plot)
eofs_ds.close()
if SAVE_PLOTS:
output_filename = get_eof_plot_output_filename(eofs_datafile, n_eofs_to_plot)
output_file = os.path.join(EOFS_PLOTS_DIR, output_filename)
plt.savefig(output_file, bbox_inches='tight')
plt.show()
plt.close()
def plot_sh_indian_eofs(eofs_ds, season, n_eofs_to_plot=20, cmap=plt.cm.RdBu_r,
lat_name=LAT_NAME, lon_name=LON_NAME):
"""Plot SH Indian region EOFs.
Parameters
----------
eofs_ds : xarray.Dataset
Dataset containing the results of the EOF analysis.
season : 'ALL' | 'DJF' | 'MAM' | 'JJA' | 'SON'
Season to plot EOFs for.
n_eofs_to_plot : int, default: 20
Number of EOF patterns to plot.
cmap : object
Colormap to use.
lat_name : str
Name of latitude coordinate.
lon_name : str
Name of longitude coordinate.
Returns
-------
fig : figure
Plot of EOFs.
"""
hemisphere = 'SH'
region = 'indian'
lon_bounds = get_region_lon_bounds(hemisphere, region)
lat_bounds = [-90.0, 0.0]
wrap_lon = False
projection = ccrs.EquidistantConic(central_longitude=np.mean(lon_bounds), central_latitude=-90,
standard_parallels=(-20, -50))
components = eofs_ds['component'].isel(component=slice(0, n_eofs_to_plot)).values
component_vmins = np.empty(n_eofs_to_plot)
vmin = None
for i, component in enumerate(components):
component_vmin = eofs_ds['eofs'].sel(component=component).min().item()
if vmin is None or component_vmin < vmin:
vmin = component_vmin
component_vmins[:] = vmin
component_vmaxs = np.empty(n_eofs_to_plot)
vmax = None
for i, component in enumerate(components):
component_vmax = eofs_ds['eofs'].sel(component=component).max().item()
if vmax is None or component_vmax > vmax:
vmax = component_vmax
component_vmaxs[:] = vmax
if n_eofs_to_plot % 4 == 0:
ncols = 4
elif n_eofs_to_plot % 2 == 0:
ncols = 2
else:
ncols = 3
nrows = int(np.ceil(n_eofs_to_plot / ncols))
height_ratios = np.ones((nrows + 1))
height_ratios[-1] = 0.1
fig = plt.figure(constrained_layout=False, figsize=(4 * ncols, 4 * nrows))
gs = gridspec.GridSpec(ncols=ncols, nrows=nrows + 1, figure=fig,
wspace=0.1, hspace=0.2,
height_ratios=height_ratios)
lat = eofs_ds[lat_name].values
lon = eofs_ds[lon_name].values
row_index = 0
col_index = 0
for i, component in enumerate(components):
eof_data = eofs_ds['eofs'].sel(component=component).squeeze().values
expl_var = eofs_ds['explained_variance_ratio'].sel(
component=component).item()
if wrap_lon:
eof_data, eof_lon = add_cyclic_point(eof_data, coord=lon)
else:
eof_lon = lon
lon_grid, lat_grid = np.meshgrid(eof_lon, lat)
ax = fig.add_subplot(gs[row_index, col_index], projection=projection)
ax.coastlines()
left_bound_verts = np.vstack([np.repeat(lon_bounds[0], 100), np.flipud(np.linspace(-90.0, 0.0, 100))]).T
right_bound_verts = np.vstack([np.repeat(lon_bounds[1], 100), np.linspace(-90.0, 0.0, 100)]).T
lower_bound_verts = np.vstack([np.linspace(lon_bounds[0], lon_bounds[1], 100),
np.repeat(-90.0, 100)]).T
upper_bound_verts = np.vstack([np.flipud(np.linspace(lon_bounds[0], lon_bounds[1], 100)),
np.repeat(0.0, 100)]).T
boundary = mpath.Path(np.vstack([left_bound_verts, lower_bound_verts, right_bound_verts, upper_bound_verts]))
ax.set_boundary(boundary, transform=ccrs.PlateCarree())
ax.set_extent(np.concatenate([lon_bounds, lat_bounds]), crs=ccrs.PlateCarree())
ax_vmin = component_vmins[i]
ax_vmax = component_vmaxs[i]
cs = ax.pcolor(lon_grid, lat_grid, eof_data, vmin=ax_vmin, vmax=ax_vmax,
cmap=cmap, transform=ccrs.PlateCarree())
ax.set_title('EOF {} ({:.2f}%)'.format(
component + 1, expl_var * 100), fontsize=14)
fig.canvas.draw()
col_index += 1
if col_index == ncols:
col_index = 0
row_index += 1
cb_ax = fig.add_subplot(gs[-1, :])
cb = fig.colorbar(cs, cax=cb_ax, pad=0.05, orientation='horizontal')
fig.suptitle('Hemisphere: {}, Region: {}, Season: {}'.format(hemisphere, region, season),
fontsize=16, x=0.5, y=0.92)
return fig
hemisphere = 'SH'
region = 'indian'
seasons = ['ALL', 'DJF', 'MAM', 'JJA', 'SON']
n_eofs_to_plot = 20
for season in seasons:
eofs_datafile = os.path.join(
EOFS_NC_DIR,
get_eofs_output_filename(
anom_output_file, hemisphere, region, EOFS_BASE_PERIOD,
season, LAT_WEIGHTS, DEFAULT_MAX_EOFS, EOFS_NORMALIZATION))
eofs_ds = xr.open_dataset(eofs_datafile)
fig = plot_sh_indian_eofs(eofs_ds, season, n_eofs_to_plot=n_eofs_to_plot)
eofs_ds.close()
if SAVE_PLOTS:
output_filename = get_eof_plot_output_filename(eofs_datafile, n_eofs_to_plot)
output_file = os.path.join(EOFS_PLOTS_DIR, output_filename)
plt.savefig(output_file, bbox_inches='tight')
plt.show()
plt.close()
def plot_sh_pacific_eofs(eofs_ds, season, n_eofs_to_plot=20, cmap=plt.cm.RdBu_r,
lat_name=LAT_NAME, lon_name=LON_NAME):
"""Plot SH Pacific region EOFs.
Parameters
----------
eofs_ds : xarray.Dataset
Dataset containing the results of the EOF analysis.
season : 'ALL' | 'DJF' | 'MAM' | 'JJA' | 'SON'
Season to plot EOFs for.
n_eofs_to_plot : int, default: 20
Number of EOF patterns to plot.
cmap : object
Colormap to use.
lat_name : str
Name of latitude coordinate.
lon_name : str
Name of longitude coordinate.
Returns
-------
fig : figure
Plot of EOFs.
"""
hemisphere = 'SH'
region = 'pacific'
lon_bounds = get_region_lon_bounds(hemisphere, region)
lat_bounds = [-90.0, 0.0]
wrap_lon = False
projection = ccrs.EquidistantConic(central_longitude=np.mean(lon_bounds), central_latitude=-90,
standard_parallels=(-20, -50))
components = eofs_ds['component'].isel(component=slice(0, n_eofs_to_plot)).values
component_vmins = np.empty(n_eofs_to_plot)
vmin = None
for i, component in enumerate(components):
component_vmin = eofs_ds['eofs'].sel(component=component).min().item()
if vmin is None or component_vmin < vmin:
vmin = component_vmin
component_vmins[:] = vmin
component_vmaxs = np.empty(n_eofs_to_plot)
vmax = None
for i, component in enumerate(components):
component_vmax = eofs_ds['eofs'].sel(component=component).max().item()
if vmax is None or component_vmax > vmax:
vmax = component_vmax
component_vmaxs[:] = vmax
if n_eofs_to_plot % 4 == 0:
ncols = 4
elif n_eofs_to_plot % 2 == 0:
ncols = 2
else:
ncols = 3
nrows = int(np.ceil(n_eofs_to_plot / ncols))
height_ratios = np.ones((nrows + 1))
height_ratios[-1] = 0.1
fig = plt.figure(constrained_layout=False, figsize=(4 * ncols, 4 * nrows))
gs = gridspec.GridSpec(ncols=ncols, nrows=nrows + 1, figure=fig,
wspace=0.1, hspace=0.2,
height_ratios=height_ratios)
lat = eofs_ds[lat_name].values
lon = eofs_ds[lon_name].values
row_index = 0
col_index = 0
for i, component in enumerate(components):
eof_data = eofs_ds['eofs'].sel(component=component).squeeze().values
expl_var = eofs_ds['explained_variance_ratio'].sel(
component=component).item()
if wrap_lon:
eof_data, eof_lon = add_cyclic_point(eof_data, coord=lon)
else:
eof_lon = lon
lon_grid, lat_grid = np.meshgrid(eof_lon, lat)
ax = fig.add_subplot(gs[row_index, col_index], projection=projection)
ax.coastlines()
left_bound_verts = np.vstack([np.repeat(lon_bounds[0], 100), np.flipud(np.linspace(-90.0, 0.0, 100))]).T
right_bound_verts = np.vstack([np.repeat(lon_bounds[1], 100), np.linspace(-90.0, 0.0, 100)]).T
lower_bound_verts = np.vstack([np.linspace(lon_bounds[0], lon_bounds[1], 100),
np.repeat(-90.0, 100)]).T
upper_bound_verts = np.vstack([np.flipud(np.linspace(lon_bounds[0], lon_bounds[1], 100)),
np.repeat(0.0, 100)]).T
boundary = mpath.Path(np.vstack([left_bound_verts, lower_bound_verts, right_bound_verts, upper_bound_verts]))
ax.set_boundary(boundary, transform=ccrs.PlateCarree())
ax.set_extent(np.concatenate([lon_bounds, lat_bounds]), crs=ccrs.PlateCarree())
ax_vmin = component_vmins[i]
ax_vmax = component_vmaxs[i]
cs = ax.pcolor(lon_grid, lat_grid, eof_data, vmin=ax_vmin, vmax=ax_vmax,
cmap=cmap, transform=ccrs.PlateCarree())
ax.set_title('EOF {} ({:.2f}%)'.format(
component + 1, expl_var * 100), fontsize=14)
fig.canvas.draw()
col_index += 1
if col_index == ncols:
col_index = 0
row_index += 1
cb_ax = fig.add_subplot(gs[-1, :])
cb = fig.colorbar(cs, cax=cb_ax, pad=0.05, orientation='horizontal')
fig.suptitle('Hemisphere: {}, Region: {}, Season: {}'.format(hemisphere, region, season),
fontsize=16, x=0.5, y=0.92)
return fig
hemisphere = 'SH'
region = 'pacific'
seasons = ['ALL', 'DJF', 'MAM', 'JJA', 'SON']
n_eofs_to_plot = 20
for season in seasons:
eofs_datafile = os.path.join(
EOFS_NC_DIR,
get_eofs_output_filename(
anom_output_file, hemisphere, region, EOFS_BASE_PERIOD,
season, LAT_WEIGHTS, DEFAULT_MAX_EOFS, EOFS_NORMALIZATION))
eofs_ds = xr.open_dataset(eofs_datafile)
fig = plot_sh_pacific_eofs(eofs_ds, season, n_eofs_to_plot=n_eofs_to_plot)
eofs_ds.close()
if SAVE_PLOTS:
output_filename = get_eof_plot_output_filename(eofs_datafile, n_eofs_to_plot)
output_file = os.path.join(EOFS_PLOTS_DIR, output_filename)
plt.savefig(output_file, bbox_inches='tight')
plt.show()
plt.close()
def plot_sh_south_america_eofs(eofs_ds, season, n_eofs_to_plot=20, cmap=plt.cm.RdBu_r,
lat_name=LAT_NAME, lon_name=LON_NAME):
"""Plot SH South America region EOFs.
Parameters
----------
eofs_ds : xarray.Dataset
Dataset containing the results of the EOF analysis.
season : 'ALL' | 'DJF' | 'MAM' | 'JJA' | 'SON'
Season to plot EOFs for.
n_eofs_to_plot : int, default: 20
Number of EOF patterns to plot.
cmap : object
Colormap to use.
lat_name : str
Name of latitude coordinate.
lon_name : str
Name of longitude coordinate.
Returns
-------
fig : figure
Plot of EOFs.
"""
hemisphere = 'SH'
region = 'south_america'
lon_bounds = get_region_lon_bounds(hemisphere, region)
lat_bounds = [-90.0, 0.0]
wrap_lon = False
projection = ccrs.EquidistantConic(central_longitude=np.mean(lon_bounds), central_latitude=-90,
standard_parallels=(-20, -50))
components = eofs_ds['component'].isel(component=slice(0, n_eofs_to_plot)).values
component_vmins = np.empty(n_eofs_to_plot)
vmin = None
for i, component in enumerate(components):
component_vmin = eofs_ds['eofs'].sel(component=component).min().item()
if vmin is None or component_vmin < vmin:
vmin = component_vmin
component_vmins[:] = vmin
component_vmaxs = np.empty(n_eofs_to_plot)
vmax = None
for i, component in enumerate(components):
component_vmax = eofs_ds['eofs'].sel(component=component).max().item()
if vmax is None or component_vmax > vmax:
vmax = component_vmax
component_vmaxs[:] = vmax
if n_eofs_to_plot % 4 == 0:
ncols = 4
elif n_eofs_to_plot % 2 == 0:
ncols = 2
else:
ncols = 3
nrows = int(np.ceil(n_eofs_to_plot / ncols))
height_ratios = np.ones((nrows + 1))
height_ratios[-1] = 0.1
fig = plt.figure(constrained_layout=False, figsize=(4 * ncols, 4 * nrows))
gs = gridspec.GridSpec(ncols=ncols, nrows=nrows + 1, figure=fig,
wspace=0.1, hspace=0.2,
height_ratios=height_ratios)
lat = eofs_ds[lat_name].values
lon = eofs_ds[lon_name].values
row_index = 0
col_index = 0
for i, component in enumerate(components):
eof_data = eofs_ds['eofs'].sel(component=component).squeeze().values
expl_var = eofs_ds['explained_variance_ratio'].sel(
component=component).item()
if wrap_lon:
eof_data, eof_lon = add_cyclic_point(eof_data, coord=lon)
else:
eof_lon = lon
lon_grid, lat_grid = np.meshgrid(eof_lon, lat)
ax = fig.add_subplot(gs[row_index, col_index], projection=projection)
ax.coastlines()
left_bound_verts = np.vstack([np.repeat(lon_bounds[0], 100), np.flipud(np.linspace(-90.0, 0.0, 100))]).T
right_bound_verts = np.vstack([np.repeat(lon_bounds[1], 100), np.linspace(-90.0, 0.0, 100)]).T
lower_bound_verts = np.vstack([np.linspace(lon_bounds[0], lon_bounds[1], 100),
np.repeat(-90.0, 100)]).T
upper_bound_verts = np.vstack([np.flipud(np.linspace(lon_bounds[0], lon_bounds[1], 100)),
np.repeat(0.0, 100)]).T
boundary = mpath.Path(np.vstack([left_bound_verts, lower_bound_verts, right_bound_verts, upper_bound_verts]))
ax.set_boundary(boundary, transform=ccrs.PlateCarree())
ax.set_extent(np.concatenate([lon_bounds, lat_bounds]), crs=ccrs.PlateCarree())
ax_vmin = component_vmins[i]
ax_vmax = component_vmaxs[i]
cs = ax.pcolor(lon_grid, lat_grid, eof_data, vmin=ax_vmin, vmax=ax_vmax,
cmap=cmap, transform=ccrs.PlateCarree())
ax.set_title('EOF {} ({:.2f}%)'.format(
component + 1, expl_var * 100), fontsize=14)
fig.canvas.draw()
col_index += 1
if col_index == ncols:
col_index = 0
row_index += 1
cb_ax = fig.add_subplot(gs[-1, :])
cb = fig.colorbar(cs, cax=cb_ax, pad=0.05, orientation='horizontal')
fig.suptitle('Hemisphere: {}, Region: {}, Season: {}'.format(hemisphere, region, season),
fontsize=16, x=0.5, y=0.92)
return fig
hemisphere = 'SH'
region = 'south_america'
seasons = ['ALL', 'DJF', 'MAM', 'JJA', 'SON']
n_eofs_to_plot = 20
for season in seasons:
eofs_datafile = os.path.join(
EOFS_NC_DIR,
get_eofs_output_filename(
anom_output_file, hemisphere, region, EOFS_BASE_PERIOD,
season, LAT_WEIGHTS, DEFAULT_MAX_EOFS, EOFS_NORMALIZATION))
eofs_ds = xr.open_dataset(eofs_datafile)
fig = plot_sh_south_america_eofs(eofs_ds, season, n_eofs_to_plot=n_eofs_to_plot)
eofs_ds.close()
if SAVE_PLOTS:
output_filename = get_eof_plot_output_filename(eofs_datafile, n_eofs_to_plot)
output_file = os.path.join(EOFS_PLOTS_DIR, output_filename)
plt.savefig(output_file, bbox_inches='tight')
plt.show()
plt.close()
###Output
_____no_output_____ |
tutorials/notebooks/03_NAS_basic/tutorial_03.ipynb | ###Markdown
Neural Architecture Search (Basic)In this tutorial we will learn the basis of neural architecture search (NAS). To do so, we will use artificial data generated from a polynomial function. Then, we will discover how to create a search space of neural architecture using a directed graph. Finally, we will see how to define the NAS settings and how to execute the search.Warning By design asyncio does not allow nested event loops. Jupyter is using Tornado which already starts an event loop. Therefore the following patch is required to run this tutorial.
###Code
!pip install nest_asyncio
import nest_asyncio
nest_asyncio.apply()
###Output
Requirement already satisfied: nest_asyncio in /home/joceran/miniconda3/envs/deephyper/lib/python3.8/site-packages (1.5.1)
###Markdown
Loading the dataFirst, we will create the `load_data` function which loads and returns thetraining and validation data. The `load_data` function generates data froma function $f$ where $\mathbf{x} \in [a, b]^n$ such as $f(\mathbf{x}) = -\sum_{i=0}^{n-1} {x_i ^2}$:
###Code
import numpy as np
def load_data(verbose=0, dim=10, a=-50, b=50, prop=0.80, size=10000):
rs = np.random.RandomState(2018)
def polynome_2(x):
return -sum([x_i ** 2 for x_i in x])
d = b - a
x = np.array([a + rs.random(dim) * d for _ in range(size)])
y = np.array([[polynome_2(v)] for v in x])
sep_index = int(prop * size)
X_train = x[:sep_index]
y_train = y[:sep_index]
X_valid = x[sep_index:]
y_valid = y[sep_index:]
if verbose:
print(f"X_train shape: {np.shape(X_train)}")
print(f"y_train shape: {np.shape(y_train)}")
print(f"X_valid shape: {np.shape(X_valid)}")
print(f"y_valid shape: {np.shape(y_valid)}")
return (X_train, y_train), (X_valid, y_valid)
_ = load_data(verbose=1)
###Output
X_train shape: (8000, 10)
y_train shape: (8000, 1)
X_valid shape: (2000, 10)
y_valid shape: (2000, 1)
###Markdown
Define a neural architecture search spaceThen, we will see how to define a space of possible neural architecture.
###Code
import collections
import tensorflow as tf
from deephyper.nas import KSearchSpace
from deephyper.nas.node import ConstantNode, VariableNode
from deephyper.nas.operation import operation, Zero, Connect, AddByProjecting, Identity
Activation = operation(tf.keras.layers.Activation)
Dense = operation(tf.keras.layers.Dense)
Dropout = operation(tf.keras.layers.Dropout)
Add = operation(tf.keras.layers.Add)
Flatten = operation(tf.keras.layers.Flatten)
ACTIVATIONS = [
tf.keras.activations.elu,
tf.keras.activations.gelu,
tf.keras.activations.hard_sigmoid,
tf.keras.activations.linear,
tf.keras.activations.relu,
tf.keras.activations.selu,
tf.keras.activations.sigmoid,
tf.keras.activations.softplus,
tf.keras.activations.softsign,
tf.keras.activations.swish,
tf.keras.activations.tanh,
]
class ResNetMLPSpace(KSearchSpace):
def __init__(self, input_shape, output_shape, seed=None, num_layers=3, mode="regression"):
super().__init__(input_shape, output_shape, seed=seed)
self.num_layers = num_layers
assert mode in ["regression", "classification"]
self.mode = mode
def build(self):
source = self.input_nodes[0]
output_dim = self.output_shape[0]
out_sub_graph = self.build_sub_graph(source, self.num_layers)
if self.mode == "regression":
output = ConstantNode(op=Dense(output_dim))
self.connect(out_sub_graph, output)
else:
output = ConstantNode(
op=Dense(output_dim, activation="softmax")
) # One-hot encoding
self.connect(out_sub_graph, output)
return self
def build_sub_graph(self, input_, num_layers=3):
source = prev_input = input_
# look over skip connections within a range of the 3 previous nodes
anchor_points = collections.deque([source], maxlen=3)
for _ in range(self.num_layers):
dense = VariableNode()
self.add_dense_to_(dense)
self.connect(prev_input, dense)
x = dense
dropout = VariableNode()
self.add_dropout_to_(dropout)
self.connect(x, dropout)
x = dropout
add = ConstantNode()
add.set_op(AddByProjecting(self, [x], activation="relu"))
for anchor in anchor_points:
skipco = VariableNode()
skipco.add_op(Zero())
skipco.add_op(Connect(self, anchor))
self.connect(skipco, add)
prev_input = add
# ! for next iter
anchor_points.append(prev_input)
return prev_input
def add_dense_to_(self, node):
node.add_op(Identity()) # we do not want to create a layer in this case
for units in range(16, 16 * 16 + 1, 16):
for activation in ACTIVATIONS:
node.add_op(Dense(units=units, activation=activation))
def add_dropout_to_(self, node):
a, b = 1e-3, 0.4
node.add_op(Identity())
dropout_range = np.exp(np.linspace(np.log(a), np.log(b), 10)) #! NAS
for rate in dropout_range:
node.add_op(Dropout(rate))
###Output
_____no_output_____
###Markdown
Let us visualize a few randomly sampled neural architecture from this search space.
###Code
import matplotlib.pyplot as plt
import matplotlib.image as mpimg
from tensorflow.keras.utils import plot_model
shapes = dict(input_shape=(10,), output_shape=(1,))
space = ResNetMLPSpace(**shapes).build()
images = []
plt.figure(figsize=(15,15))
for i in range(4):
plt.subplot(2,2,i+1)
model = space.sample()
plot_model(model, "random_model.png", show_shapes=False, show_layer_names=False)
image = mpimg.imread("random_model.png")
plt.imshow(image)
plt.axis('off')
plt.show()
###Output
2021-10-15 13:00:48.417600: E tensorflow/stream_executor/cuda/cuda_driver.cc:271] failed call to cuInit: UNKNOWN ERROR (304)
2021-10-15 13:00:48.417677: I tensorflow/stream_executor/cuda/cuda_diagnostics.cc:156] kernel driver does not appear to be running on this host (Jordi): /proc/driver/nvidia/version does not exist
2021-10-15 13:00:48.418009: I tensorflow/core/platform/cpu_feature_guard.cc:142] This TensorFlow binary is optimized with oneAPI Deep Neural Network Library (oneDNN) to use the following CPU instructions in performance-critical operations: AVX2 FMA
To enable them in other operations, rebuild TensorFlow with the appropriate compiler flags.
###Markdown
Create a problem instanceLet us define the neural architecture search problem.
###Code
from deephyper.problem import NaProblem
from deephyper.nas.preprocessing import minmaxstdscaler
problem = NaProblem(seed=2019)
problem.load_data(load_data)
problem.preprocessing(minmaxstdscaler)
problem.search_space(ResNetMLPSpace)
problem.hyperparameters(
batch_size=32,
learning_rate=0.01,
optimizer="adam",
num_epochs=20,
callbacks=dict(
EarlyStopping=dict(
monitor="val_r2", mode="max", verbose=0, patience=5
)
),
)
problem.loss("mse")
problem.metrics(["r2"])
problem.objective("val_r2__last")
print(problem)
###Output
Problem is:
* SEED = 2019 *
- search space : __main__.ResNetMLPSpace
- data loading : __main__.load_data
- preprocessing : deephyper.nas.preprocessing._base.minmaxstdscaler
- hyperparameters:
* verbose: 0
* batch_size: 32
* learning_rate: 0.01
* optimizer: adam
* num_epochs: 20
* callbacks: {'EarlyStopping': {'monitor': 'val_r2', 'mode': 'max', 'verbose': 0, 'patience': 5}}
- loss : mse
- metrics :
* r2
- objective : val_r2__last
###Markdown
Tip Adding an `EarlyStopping(...)` callback is a good idea to stop the training of your model as soon as it stops to improve.```python...EarlyStopping=dict(monitor="val_r2", mode="max", verbose=0, patience=5)...``` We can first train this model to evaluate the baseline accuracy: Running the search Create an `Evaluator` object using the `ray` backend to distribute the evaluation of the run-function defined previously.
###Code
from deephyper.evaluator import Evaluator
from deephyper.evaluator.callback import LoggerCallback
from deephyper.nas.run import run_base_trainer
evaluator = Evaluator.create(run_base_trainer,
method="ray",
method_kwargs={
"address": None,
"num_cpus": 2,
"num_cpus_per_task": 1,
"callbacks": [LoggerCallback()]
})
print("Number of workers: ", evaluator.num_workers)
###Output
2021-10-15 15:39:43,526 INFO services.py:1263 -- View the Ray dashboard at [1m[32mhttp://127.0.0.1:8265[39m[22m
###Markdown
Tip You can open the ray-dashboard at an address like http://127.0.0.1:port in a browser to monitor the CPU usage of the execution. Finally, you can define a Random search called `Random` and link to it the defined `problem` and `evaluator`.
###Code
from deephyper.search.nas import Random
search = Random(problem, evaluator)
results = search.search(10)
###Output
[2m[36m(pid=54243)[0m 2021-10-15 15:39:50.001490: I tensorflow/core/platform/cpu_feature_guard.cc:142] This TensorFlow binary is optimized with oneAPI Deep Neural Network Library (oneDNN) to use the following CPU instructions in performance-critical operations: AVX2 FMA
[2m[36m(pid=54243)[0m To enable them in other operations, rebuild TensorFlow with the appropriate compiler flags.
[2m[36m(pid=54244)[0m 2021-10-15 15:39:50.001472: I tensorflow/core/platform/cpu_feature_guard.cc:142] This TensorFlow binary is optimized with oneAPI Deep Neural Network Library (oneDNN) to use the following CPU instructions in performance-critical operations: AVX2 FMA
[2m[36m(pid=54244)[0m To enable them in other operations, rebuild TensorFlow with the appropriate compiler flags.
[2m[36m(pid=54243)[0m 2021-10-15 15:39:50.945603: I tensorflow/compiler/mlir/mlir_graph_optimization_pass.cc:185] None of the MLIR Optimization Passes are enabled (registered 2)
[2m[36m(pid=54244)[0m 2021-10-15 15:39:50.867548: I tensorflow/compiler/mlir/mlir_graph_optimization_pass.cc:185] None of the MLIR Optimization Passes are enabled (registered 2)
###Markdown
After the search is over, you will find the following files in your current folder:```results.csvsave/```Let us visualize the training of our models. First, we need to load the training history of each model which are located in `save/history`:
###Code
import os
import json
histories = [os.path.join("save/history", f) for f in os.listdir("save/history/") if ".json" in f]
for i, fpath in enumerate(histories):
with open(fpath, "r") as fd:
histories[i] = json.load(fd)
print(list(histories[0].keys()))
plt.figure()
for h in histories:
plt.plot(h["val_r2"])
plt.ylabel("Validation $R^2$")
plt.xlabel("Epochs")
plt.show()
###Output
_____no_output_____
###Markdown
Once the search is over, a file named `results.csv` is saved in the current directory. The same dataframe is returned by the `search.search(...)` call. It contains the configurations evaluated during the search and their corresponding `objective` value (i.e, validation accuracy), `duration` of computation and time of computation with `elapsed_sec`. Each neural architecture is embedded as a list of discrete decision variables called `arch_seq`.
###Code
results
###Output
_____no_output_____
###Markdown
The `deephyper-analytics` command line is a way of analyzing this type of file. For example, we want to output the best configuration we can use the `topk` functionnality.
###Code
!deephyper-analytics topk results.csv -k 3
###Output
'0':
arch_seq: '[153, 0, 0, 95, 6, 0, 0, 167, 8, 1, 1, 0]'
duration: 6.8086879253
elapsed_sec: 49.4738252163
id: 9
objective: 0.9762052894
'1':
arch_seq: '[164, 2, 1, 153, 9, 0, 1, 157, 5, 0, 1, 0]'
duration: 10.4923799038
elapsed_sec: 27.6096279621
id: 4
objective: 0.9760414362
'2':
arch_seq: '[139, 2, 1, 25, 9, 1, 1, 9, 10, 0, 0, 1]'
duration: 8.9671211243
elapsed_sec: 36.5781030655
id: 5
objective: 0.9741773009
###Markdown
Where each architecture is described as a vector of scalar values named arch_seq. In fact, each of this scalar values represents chosen operations for the variable nodes of our search space. Testing the best configurationWe can visualize the architecture of the best configuration:
###Code
best_config = results.iloc[results.objective.argmax()][:-2].to_dict()
arch_seq = json.loads(best_config["arch_seq"])
model = space.sample(arch_seq)
plot_model(model, show_shapes=False, show_layer_names=False)
###Output
_____no_output_____ |
No-8-Cross-Validation/Cross-Validation.ipynb | ###Markdown
*This tutorial is part of the [Learn Machine Learning](https://www.kaggle.com/learn/learn-machine-learning/) series. In this step, you will learn how to use cross-validation for better measures of model performance.* What is Cross ValidationMachine learning is an iterative process. You will face choices about predictive variables to use, what types of models to use,what arguments to supply those models, etc. We make these choices in a data-driven way by measuring model quality of various alternatives.You've already learned to use `train_test_split` to split the data, so you can measure model quality on the test data. Cross-validation extends this approach to model scoring (or "model validation.") Compared to `train_test_split`, cross-validation gives you a more reliable measure of your model's quality, though it takes longer to run. The Shortcoming of Train-Test SplitImagine you have a dataset with 5000 rows. The `train_test_split` function has an argument for `test_size` that you can use to decide how many rows go to the training set and how many go to the test set. The larger the test set, the more reliable your measures of model quality will be. At an extreme, you could imagine having only 1 row of data in the test set. If you compare alternative models, which one makes the best predictions on a single data point will be mostly a matter of luck. You will typically keep about 20% as a test dataset. But even with 1000 rows in the test set, there's some random chance in determining model scores. A model might do well on one set of 1000 rows, even if it would be inaccurate on a different 1000 rows. The larger the test set, the less randomness (aka "noise") there is in our measure of model quality.But we can only get a large test set by removing data from our training data, and smaller training datasets mean worse models. In fact, the ideal modeling decisions on a small dataset typically aren't the best modeling decisions on large datasets. --- The Cross-Validation ProcedureIn cross-validation, we run our modeling process on different subsets of the data to get multiple measures of model quality. For example, we could have 5 **folds** or experiments. We divide the data into 5 pieces, each being 20% of the full dataset. We run an experiment called experiment 1 which uses the first fold as a holdout set, and everything else as training data. This gives us a measure of model quality based on a 20% holdout set, much as we got from using the simple train-test split. We then run a second experiment, where we hold out data from the second fold (using everything except the 2nd fold for training the model.) This gives us a second estimate of model quality.We repeat this process, using every fold once as the holdout. Putting this together, 100% of the data is used as a holdout at some point. Returning to our example above from train-test split, if we have 5000 rows of data, we end up with a measure of model quality based on 5000 rows of holdout (even if we don't use all 5000 rows simultaneously. Trade-offs Between Cross-Validation and Train-Test SplitCross-validation gives a more accurate measure of model quality, which is especially important if you are making a lot of modeling decisions. However, it can take more time to run, because it estimates models once for each fold. So it is doing more total work.Given these tradeoffs, when should you use each approach?On small datasets, the extra computational burden of running cross-validation isn't a big deal. These are also the problems where model quality scores would be least reliable with train-test split. So, if your dataset is smaller, you should run cross-validation.For the same reasons, a simple train-test split is sufficient for larger datasets. It will run faster, and you may have enough data that there's little need to re-use some of it for holdout.There's no simple threshold for what constitutes a large vs small dataset. If your model takes a couple minute or less to run, it's probably worth switching to cross-validation. If your model takes much longer to run, cross-validation may slow down your workflow more than it's worth.Alternatively, you can run cross-validation and see if the scores for each experiment seem close. If each experiment gives the same results, train-test split is probably sufficient. Example First we read the data
###Code
import pandas as pd
data = pd.read_csv('./input/melb_data.csv')
cols_to_use = ['Rooms', 'Distance', 'Landsize', 'BuildingArea', 'YearBuilt']
X = data[cols_to_use]
y = data.Price
###Output
_____no_output_____
###Markdown
Then specify a pipeline of our modeling steps (It can be very difficult to do cross-validation properly if you arent't using [pipelines](https://www.kaggle.com/dansbecker/pipelines))
###Code
from sklearn.ensemble import RandomForestRegressor
from sklearn.pipeline import make_pipeline
from sklearn.preprocessing import Imputer
my_pipeline = make_pipeline(Imputer(), RandomForestRegressor())
###Output
C:\ProgramData\Anaconda3\lib\site-packages\sklearn\utils\deprecation.py:58: DeprecationWarning: Class Imputer is deprecated; Imputer was deprecated in version 0.20 and will be removed in 0.22. Import impute.SimpleImputer from sklearn instead.
warnings.warn(msg, category=DeprecationWarning)
###Markdown
Finally get the cross-validation scores:
###Code
from sklearn.model_selection import cross_val_score
scores = cross_val_score(my_pipeline, X, y, scoring='neg_mean_absolute_error')
print(scores)
###Output
C:\ProgramData\Anaconda3\lib\site-packages\sklearn\model_selection\_split.py:2053: FutureWarning: You should specify a value for 'cv' instead of relying on the default value. The default value will change from 3 to 5 in version 0.22.
warnings.warn(CV_WARNING, FutureWarning)
C:\ProgramData\Anaconda3\lib\site-packages\sklearn\utils\deprecation.py:58: DeprecationWarning: Class Imputer is deprecated; Imputer was deprecated in version 0.20 and will be removed in 0.22. Import impute.SimpleImputer from sklearn instead.
warnings.warn(msg, category=DeprecationWarning)
C:\ProgramData\Anaconda3\lib\site-packages\sklearn\ensemble\forest.py:246: FutureWarning: The default value of n_estimators will change from 10 in version 0.20 to 100 in 0.22.
"10 in version 0.20 to 100 in 0.22.", FutureWarning)
C:\ProgramData\Anaconda3\lib\site-packages\sklearn\utils\deprecation.py:58: DeprecationWarning: Class Imputer is deprecated; Imputer was deprecated in version 0.20 and will be removed in 0.22. Import impute.SimpleImputer from sklearn instead.
warnings.warn(msg, category=DeprecationWarning)
C:\ProgramData\Anaconda3\lib\site-packages\sklearn\ensemble\forest.py:246: FutureWarning: The default value of n_estimators will change from 10 in version 0.20 to 100 in 0.22.
"10 in version 0.20 to 100 in 0.22.", FutureWarning)
C:\ProgramData\Anaconda3\lib\site-packages\sklearn\utils\deprecation.py:58: DeprecationWarning: Class Imputer is deprecated; Imputer was deprecated in version 0.20 and will be removed in 0.22. Import impute.SimpleImputer from sklearn instead.
warnings.warn(msg, category=DeprecationWarning)
C:\ProgramData\Anaconda3\lib\site-packages\sklearn\ensemble\forest.py:246: FutureWarning: The default value of n_estimators will change from 10 in version 0.20 to 100 in 0.22.
"10 in version 0.20 to 100 in 0.22.", FutureWarning)
###Markdown
You may notice that we specified an argument for *scoring*. This specifies what measure of model quality to report. The docs for scikit-learn show a [list of options](http://scikit-learn.org/stable/modules/model_evaluation.html). It is a little surprising that we specify *negative* mean absolute error in this case. Scikit-learn has a convention where all metrics are defined so a high number is better. Using negatives here allows them to be consistent with that convention, though negative MAE is almost unheard of elsewhere.You typically want a single measure of model quality to compare between models. So we take the average across experiments.
###Code
print('Mean Absolute Error %2f' %(-1 * scores.mean()))
###Output
Mean Absolute Error 302419.950349
|
mavroc_2021.ipynb | ###Markdown
UseClick (SHIFT+Enter) on the line below for installing pomegranade. This will also install a new version of numpy.Then click on the button: "Restart runtime" After the restart, skip the line with `pip install` and execute the cells below.
###Code
!pip install pomegranate
import pomegranate as pg
from collections import namedtuple
import sys
from graphviz import Digraph
import matplotlib.pyplot as plt
from IPython.display import Markdown, display
def printmd(string):
display(Markdown(string))
###Output
_____no_output_____
###Markdown
Proposed model
###Code
def plot_node(node, graph):
special_options = {}
if node.is_observable:
special_options['fillcolor']='#e9f2de'
special_options['style']='filled'
special_options['shape']='box'
if "map" in node.label.lower():
special_options['penwidth']='2'
special_options['fillcolor']='#e5e5e5'
special_options['style']='filled'
graph.node(node.label, **special_options)
Node = namedtuple('Node',['is_observable','label','children'])
lane_marking_match = Node(is_observable=True, label= 'Lane marking match', children= [])
landmark_match = Node(is_observable=True, label='Landmark match', children=[])
localization = Node(is_observable=False, label='Localization', children=[landmark_match, lane_marking_match])
landmark_accuracy = Node(is_observable=False, label='Landmark accuracy', children=[landmark_match, localization])
lane_marking_accuracy = Node(is_observable=False, label='Lane marking accuracy', children=[lane_marking_match, localization])
camera = Node(is_observable=False, label='Camera',
children=[lane_marking_accuracy, landmark_accuracy
])
radar = Node(is_observable=False, label='Radar',
children=[landmark_accuracy])
lidar = Node(is_observable=False, label='LiDAR',
children=[landmark_accuracy])
weather = Node(is_observable=True, label='Weather',
children=[camera, radar, lidar])
map = Node(is_observable=False, label='Map validity',
children=[lane_marking_match, landmark_match, localization])
all_nodes = (lane_marking_match, landmark_match,
landmark_accuracy, localization,
lane_marking_accuracy, camera, radar, lidar, weather, map)
###Output
_____no_output_____
###Markdown
Plot version 1 with map validation on top
###Code
top_nodes = (weather, map)
s = Digraph('subgraph')
s.graph_attr.update(rank='min')
for node in top_nodes:
plot_node(node, s)
g = Digraph('G')
for node in all_nodes:
plot_node(node, g)
for child in node.children:
g.edge(node.label, child.label)
g.subgraph(s)
g
###Output
_____no_output_____
###Markdown
Plot version 2 with map validation in the center
###Code
top_nodes = ()
s = Digraph('subgraph')
s.graph_attr.update(rank='min')
for node in top_nodes:
plot_node(node, s)
g = Digraph('G')
for node in all_nodes:
plot_node(node, g)
for child in node.children:
g.edge(node.label, child.label)
g.subgraph(s)
g
MapLabels = namedtuple('MapLabels', ['valid', 'invalid'])
map_labels = MapLabels('Valid', 'Invalid')
WeatherLabel = namedtuple('WeatherLabel',['cooperative','non_cooperative'])
weather_label = WeatherLabel(cooperative="cooperative", non_cooperative="non_cooperative")
SensorStatusLabel = namedtuple('SensorStatus', ['nominal', 'degraded'])
sensor_status_label = SensorStatusLabel(nominal="Nominal", degraded="Degraded")
radar_status_label = sensor_status_label
camera_status_label = sensor_status_label
lidar_status_label = sensor_status_label
AccuracyLabel = namedtuple('AccuracyLabel', ['high','low'])
accuracy_label = AccuracyLabel(high="High", low="Low")
lane_marking_accuracy_label = accuracy_label
landmark_accuracy_label = accuracy_label
localization_label = accuracy_label
MatchLabel = namedtuple('MatchLabel',['detected','not_detected'])
match_label = MatchLabel(detected="Detected", not_detected="Not detected")
lane_marking_match_label = match_label
landmark_match_label = match_label
def verify_sum_of_probability_is_one(discrete_variable) -> bool:
parameters = discrete_variable.parameters
sum_of_probability = sum(parameters[0].values())
return abs(sum_of_probability - 1.0) <= sys.float_info.epsilon
###Output
_____no_output_____
###Markdown
A priori distributions
###Code
map_distribution = pg.DiscreteDistribution({map_labels.valid: 0.8, map_labels.invalid: 0.2})
weather_distribution = pg.DiscreteDistribution({weather_label.cooperative: 0.5, weather_label.non_cooperative: 0.5})
are_all_valid = all([verify_sum_of_probability_is_one(x) for x in (map_distribution, weather_distribution)])
if are_all_valid:
printmd("**All distributions are correctly parametrized**")
else:
raise RuntimeError("One of the variables is not correctly parametrized!")
###Output
_____no_output_____
###Markdown
Dependent distributions
###Code
def get_conditional_distributions(in_dictionary=None):
def get_distribution_to_use(distribution_name):
if in_dictionary and (distribution_name in in_dictionary):
return in_dictionary[distribution_name]
else:
return default_distributions[distribution_name]
def select_distribution(name, default_distribution):
if in_dictionary and (name in in_dictionary):
return in_dictionary[name]
else:
return default_distribution
default_distributions = {"map_distribution": map_distribution,
"weather_distribution": weather_distribution}
default_distributions["camera_status_distribution"] = pg.ConditionalProbabilityTable(
[
[weather_label.cooperative, camera_status_label.nominal, 0.9],
[weather_label.cooperative, camera_status_label.degraded, 0.1],
[weather_label.non_cooperative, camera_status_label.nominal, 0.3],
[weather_label.non_cooperative, camera_status_label.degraded, 0.7],
], [get_distribution_to_use("weather_distribution")])
default_distributions["radar_status_distribution"] = pg.ConditionalProbabilityTable(
[
[weather_label.cooperative, radar_status_label.nominal, 0.99],
[weather_label.cooperative, radar_status_label.degraded, 0.01],
[weather_label.non_cooperative, radar_status_label.nominal, 0.7],
[weather_label.non_cooperative, radar_status_label.degraded, 0.3],
], [get_distribution_to_use("weather_distribution")])
default_distributions["lidar_status_distribution"] = pg.ConditionalProbabilityTable(
[
[weather_label.cooperative, lidar_status_label.nominal, 0.8],
[weather_label.cooperative, lidar_status_label.degraded, 0.2],
[weather_label.non_cooperative, lidar_status_label.nominal, 0.2],
[weather_label.non_cooperative, lidar_status_label.degraded, 0.8],
], [get_distribution_to_use("weather_distribution")])
default_distributions["lane_marking_accuracy_distribution"] = pg.ConditionalProbabilityTable(
[
[camera_status_label.nominal, accuracy_label.high, 0.85],
[camera_status_label.nominal, accuracy_label.low, 0.15],
[camera_status_label.degraded, accuracy_label.high, 0.3],
[camera_status_label.degraded, accuracy_label.low, 0.7],
], [get_distribution_to_use("camera_status_distribution")])
default_distributions["landmark_accuracy_distribution"] = pg.ConditionalProbabilityTable(
[
[camera_status_label.nominal, radar_status_label.nominal, lidar_status_label.nominal, accuracy_label.high, 0.95],
[camera_status_label.nominal, radar_status_label.nominal, lidar_status_label.nominal, accuracy_label.low, 0.05],
[camera_status_label.nominal, radar_status_label.nominal, lidar_status_label.degraded, accuracy_label.high, 0.7],
[camera_status_label.nominal, radar_status_label.nominal, lidar_status_label.degraded, accuracy_label.low, 0.3],
[camera_status_label.nominal, radar_status_label.degraded, lidar_status_label.nominal, accuracy_label.high, 0.8],
[camera_status_label.nominal, radar_status_label.degraded, lidar_status_label.nominal, accuracy_label.low, 0.2],
[camera_status_label.nominal, radar_status_label.degraded, lidar_status_label.degraded, accuracy_label.high, 0.5],
[camera_status_label.nominal, radar_status_label.degraded, lidar_status_label.degraded, accuracy_label.low, 0.5],
[camera_status_label.degraded, radar_status_label.nominal, lidar_status_label.nominal, accuracy_label.high, 0.8],
[camera_status_label.degraded, radar_status_label.nominal, lidar_status_label.nominal, accuracy_label.low, 0.2],
[camera_status_label.degraded, radar_status_label.nominal, lidar_status_label.degraded, accuracy_label.high, 0.4],
[camera_status_label.degraded, radar_status_label.nominal, lidar_status_label.degraded, accuracy_label.low, 0.6],
[camera_status_label.degraded, radar_status_label.degraded, lidar_status_label.nominal, accuracy_label.high, 0.4],
[camera_status_label.degraded, radar_status_label.degraded, lidar_status_label.nominal, accuracy_label.low, 0.6],
[camera_status_label.degraded, radar_status_label.degraded, lidar_status_label.degraded, accuracy_label.high, 0.01],
[camera_status_label.degraded, radar_status_label.degraded, lidar_status_label.degraded, accuracy_label.low, 0.99],
], [get_distribution_to_use("camera_status_distribution"),
get_distribution_to_use("radar_status_distribution"),
get_distribution_to_use("lidar_status_distribution")])
default_distributions["localization_distribution"] = pg.ConditionalProbabilityTable(
[
[lane_marking_accuracy_label.high, map_labels.valid, landmark_accuracy_label.high, localization_label.high, 0.95],
[lane_marking_accuracy_label.high, map_labels.valid, landmark_accuracy_label.high, localization_label.low, 0.05],
[lane_marking_accuracy_label.high, map_labels.valid, landmark_accuracy_label.low, localization_label.high, 0.7],
[lane_marking_accuracy_label.high, map_labels.valid, landmark_accuracy_label.low, localization_label.low, 0.3],
[lane_marking_accuracy_label.high, map_labels.invalid, landmark_accuracy_label.high, localization_label.high, 0.1],
[lane_marking_accuracy_label.high, map_labels.invalid, landmark_accuracy_label.high, localization_label.low, 0.9],
[lane_marking_accuracy_label.high, map_labels.invalid, landmark_accuracy_label.low, localization_label.high, 0.1],
[lane_marking_accuracy_label.high, map_labels.invalid, landmark_accuracy_label.low, localization_label.low, 0.9],
[lane_marking_accuracy_label.low, map_labels.valid, landmark_accuracy_label.high, localization_label.high, 0.8],
[lane_marking_accuracy_label.low, map_labels.valid, landmark_accuracy_label.high, localization_label.low, 0.2],
[lane_marking_accuracy_label.low, map_labels.valid, landmark_accuracy_label.low, localization_label.high, 0.2],
[lane_marking_accuracy_label.low, map_labels.valid, landmark_accuracy_label.low, localization_label.low, 0.8],
[lane_marking_accuracy_label.low, map_labels.invalid, landmark_accuracy_label.high, localization_label.high, 0.1],
[lane_marking_accuracy_label.low, map_labels.invalid, landmark_accuracy_label.high, localization_label.low, 0.9],
[lane_marking_accuracy_label.low, map_labels.invalid, landmark_accuracy_label.low, localization_label.high, 0.1],
[lane_marking_accuracy_label.low, map_labels.invalid, landmark_accuracy_label.low, localization_label.low, 0.9],
], [get_distribution_to_use("lane_marking_accuracy_distribution"),
get_distribution_to_use("map_distribution"),
get_distribution_to_use("landmark_accuracy_distribution")])
lane_marking_match_conditional_distribution_table = [
[lane_marking_accuracy_label.high, map_labels.valid, localization_label.high, match_label.detected, 0.99],
[lane_marking_accuracy_label.high, map_labels.valid, localization_label.high, match_label.not_detected, 0.01],
[lane_marking_accuracy_label.high, map_labels.valid, localization_label.low, match_label.detected, 0.6],
[lane_marking_accuracy_label.high, map_labels.valid, localization_label.low, match_label.not_detected, 0.4],
[lane_marking_accuracy_label.high, map_labels.invalid, localization_label.high, match_label.detected, 0.1],
[lane_marking_accuracy_label.high, map_labels.invalid, localization_label.high, match_label.not_detected, 0.9],
[lane_marking_accuracy_label.high, map_labels.invalid, localization_label.low, match_label.detected, 0.1],
[lane_marking_accuracy_label.high, map_labels.invalid, localization_label.low, match_label.not_detected, 0.9],
[lane_marking_accuracy_label.low, map_labels.valid, localization_label.high, match_label.detected, 0.7],
[lane_marking_accuracy_label.low, map_labels.valid, localization_label.high, match_label.not_detected, 0.3],
[lane_marking_accuracy_label.low, map_labels.valid, localization_label.low, match_label.detected, 0.4],
[lane_marking_accuracy_label.low, map_labels.valid, localization_label.low, match_label.not_detected, 0.6],
[lane_marking_accuracy_label.low, map_labels.invalid, localization_label.high, match_label.detected, 0.1],
[lane_marking_accuracy_label.low, map_labels.invalid, localization_label.high, match_label.not_detected, 0.9],
[lane_marking_accuracy_label.low, map_labels.invalid, localization_label.low, match_label.detected, 0.1],
[lane_marking_accuracy_label.low, map_labels.invalid, localization_label.low, match_label.not_detected, 0.9],
]
default_distributions["lane_marking_match_distribution"] = pg.ConditionalProbabilityTable(
lane_marking_match_conditional_distribution_table
, [get_distribution_to_use("lane_marking_accuracy_distribution"),
get_distribution_to_use("map_distribution"),
get_distribution_to_use("localization_distribution")])
default_distributions["landmark_match_distribution"] = pg.ConditionalProbabilityTable(
lane_marking_match_conditional_distribution_table,
[get_distribution_to_use("landmark_accuracy_distribution"),
get_distribution_to_use("map_distribution"),
get_distribution_to_use("localization_distribution")]
)
out_distribution = {}
key_values = ("camera_status_distribution",
"radar_status_distribution",
"lidar_status_distribution",
"lane_marking_accuracy_distribution",
"landmark_accuracy_distribution",
"localization_distribution",
"lane_marking_match_distribution",
"landmark_match_distribution")
for i_key in key_values:
out_distribution[i_key] = select_distribution(i_key, default_distributions[i_key])
return out_distribution
default_conditional_distributions = get_conditional_distributions()
# write global variables
for key in default_conditional_distributions:
globals()[key] = default_conditional_distributions[key]
###Output
_____no_output_____
###Markdown
Create the network
###Code
def get_admissible_node_name(in_label):
return in_label.replace(" ", "_")
def get_pgm(in_dictionary=None):
def get_distribution_to_use(distribution_name):
if in_dictionary and (distribution_name in in_dictionary):
return in_dictionary[distribution_name]
else:
return globals()[distribution_name]
weatherPgNode = pg.Node(get_distribution_to_use("weather_distribution"), name=weather.label)
cameraPgNode = pg.Node(get_distribution_to_use("camera_status_distribution"), name=camera.label)
radarPgNode = pg.Node(get_distribution_to_use("radar_status_distribution"), name=radar.label)
lidarPgNode = pg.Node(get_distribution_to_use("lidar_status_distribution"), name=lidar.label)
mapPgNode = pg.Node(get_distribution_to_use("map_distribution"), name=map.label)
laneMarkingAccuracyPgNode = pg.Node(get_distribution_to_use("lane_marking_accuracy_distribution"), name=lane_marking_accuracy.label)
landMarkAccuracyPgNode = pg.Node(get_distribution_to_use("landmark_accuracy_distribution"), name=landmark_accuracy.label)
localizationPgNode = pg.Node(get_distribution_to_use("localization_distribution"), name=localization.label)
laneMarkingMatchPgNode = pg.Node(get_distribution_to_use("lane_marking_match_distribution"), name=lane_marking_match.label)
landmarkMatchPgNode = pg.Node(get_distribution_to_use("landmark_match_distribution"), name=landmark_match.label)
model = pg.BayesianNetwork("Map validity problem")
pg_model_nodes = (
weatherPgNode, cameraPgNode, radarPgNode, lidarPgNode, mapPgNode,
laneMarkingAccuracyPgNode, landMarkAccuracyPgNode,
localizationPgNode,
laneMarkingMatchPgNode, landmarkMatchPgNode
)
model.add_states(*pg_model_nodes)
model.add_edge(weatherPgNode, cameraPgNode)
model.add_edge(weatherPgNode, radarPgNode)
model.add_edge(weatherPgNode, lidarPgNode)
model.add_edge(cameraPgNode, laneMarkingAccuracyPgNode)
model.add_edge(cameraPgNode, landMarkAccuracyPgNode)
model.add_edge(radarPgNode, landMarkAccuracyPgNode)
model.add_edge(lidarPgNode, landMarkAccuracyPgNode)
model.add_edge(landMarkAccuracyPgNode, localizationPgNode)
model.add_edge(mapPgNode, localizationPgNode)
model.add_edge(laneMarkingAccuracyPgNode, localizationPgNode)
model.add_edge(laneMarkingAccuracyPgNode, laneMarkingMatchPgNode)
model.add_edge(mapPgNode, laneMarkingMatchPgNode)
model.add_edge(localizationPgNode, laneMarkingMatchPgNode)
model.add_edge(landMarkAccuracyPgNode, landmarkMatchPgNode)
model.add_edge(mapPgNode, landmarkMatchPgNode)
model.add_edge(localizationPgNode, landmarkMatchPgNode)
model.bake()
return [model, pg_model_nodes]
model, pg_model_nodes = get_pgm()
#list(default_conditional_distributions.keys())
Sample = namedtuple('Sample',
['weather', 'map_validity', 'radar', 'camera', 'lidar',
'lane_marking_accuracy', 'landmark_accuracy',
'localization',
'lane_marking_match', 'landmark_match',
'probability', 'log_probability'])
HiddenVariable = namedtuple('HiddenVariable',
['map_validity', 'radar', 'camera', 'lidar',
'lane_marking_accuracy', 'landmark_accuracy',
'localization',
'probability', 'log_probability'])
def compute_probability_of_samples(model, weather_symbol,
lane_marking_match_symbol,
landmark_match_symbol):
out = []
for camera_symbol in camera_status_label:
for radar_symbol in radar_status_label:
for lidar_symbol in lidar_status_label:
for map_symbol in map_labels:
for lanemarking_accuracy_symbol in lane_marking_accuracy_label:
for landmark_accuracy_symbol in landmark_accuracy_label:
for localization_symbol in localization_label:
symbols = [weather_symbol, camera_symbol, radar_symbol, lidar_symbol,
map_symbol, lanemarking_accuracy_symbol,
landmark_accuracy_symbol,
localization_symbol,
lane_marking_match_symbol, landmark_match_symbol]
probability = model.probability([symbols])
log_probability = model.log_probability([symbols])
new_sample = Sample(weather=weather_symbol,
map_validity=map_symbol,
radar=radar_symbol,
camera=camera_symbol,
lidar=lidar_symbol,
lane_marking_accuracy=lanemarking_accuracy_symbol,
landmark_accuracy=landmark_accuracy_symbol,
localization=localization_symbol,
lane_marking_match=lane_marking_match_symbol,
landmark_match=landmark_match_symbol,
probability=probability,
log_probability=log_probability)
out.append(new_sample)
return out
def compute_a_posteriori_from_symbols(model, symbols):
a_posteriori = model.predict_proba(symbols)
assert(len(a_posteriori) == len(pg_model_nodes))
out_data = {}
out_data["conditional"] = symbols
out_data["posterior"] = []
for posteriori, node in zip(a_posteriori, pg_model_nodes):
current_node = {}
try:
current_node["distribution"] = posteriori.parameters[0]
current_node["name"] = node.name
out_data["posterior"].append(current_node)
except Exception as e:
continue
return out_data
def compute_a_posteriori(model, weather_symbol, lane_marking_match_symbol,
landmark_match_symbol):
symbols = {
weather.label: weather_symbol,
lane_marking_match.label: lane_marking_match_symbol,
landmark_match.label: landmark_match_symbol
}
return compute_a_posteriori_from_symbols(model, symbols)
def GetHiddenVariable(in_sample: Sample) -> HiddenVariable:
out = HiddenVariable(
map_validity = in_sample.map_validity,
radar = in_sample.radar,
camera = in_sample.camera,
lidar=in_sample.lidar,
lane_marking_accuracy = in_sample.lane_marking_accuracy,
landmark_accuracy = in_sample.landmark_accuracy,
localization = in_sample.localization,
probability = in_sample.probability,
log_probability = in_sample.log_probability)
return out
def compress_label(in_label, string_length=2):
return in_label[:string_length]
def get_digit_of_sensor_status(sensor_status):
return 1 if sensor_status == sensor_status_label.nominal else 0
def get_x_coordinate(sample: Sample):
camera_value = get_digit_of_sensor_status(sample.camera)
radar_value = get_digit_of_sensor_status(sample.radar)
lidar_value = get_digit_of_sensor_status(sample.lidar)
value = camera_value + radar_value * 2 + lidar_value * 4
return value
def get_label_of_coordinate(x_value):
import math
if (x_value< 0) or (x_value>8):
raise RuntimeError("Unacceptable x value")
camera_value = x_value % 2
radar_value = math.floor(x_value / 2) % 2
lidar_value = math.floor(x_value / 4) % 2
coordinate_label = ""
if camera_value > 0:
coordinate_label += "C"
if radar_value > 0:
coordinate_label += "R"
if lidar_value > 0:
coordinate_label += "L"
if not coordinate_label:
return "None"
return coordinate_label
def get_all_x_coordinates():
return range(8)
def get_all_x_label_coordinates():
x_values = get_all_x_coordinates()
return [get_label_of_coordinate(x) for x in x_values]
def beautify_axes(ax):
ax.set_axis_on()
ax.grid(b=True, axis='y')
ax.set_facecolor('#f5f5f5')
ax.spines['top'].set_visible(False)
ax.spines['right'].set_visible(False)
ax.spines['bottom'].set_visible(False)
ax.spines['left'].set_visible(False)
for item in ([ax.title, ax.xaxis.label, ax.yaxis.label] +
ax.get_xticklabels() + ax.get_yticklabels()):
item.set_fontsize(14)
def change_x_ticks(ax):
ax.set_xticks(get_all_x_coordinates())
ax.set_xticklabels(get_all_x_label_coordinates())
def plot_subsamples(ax, subsample, **kwargs):
def brake_sub_sample():
return [single_hidden_variable for single_hidden_variable in subsample
if (single_hidden_variable.lane_marking_accuracy==lane_marking_accuracy_value) and
(single_hidden_variable.landmark_accuracy==landmark_accuracy_value)]
def get_scatter_options(lane_marking_accuracy, landmark_accuracy):
options = {}
if lane_marking_accuracy == lane_marking_accuracy_label.low:
options["marker"] = "^"
options["s"] = 100
if lane_marking_accuracy == lane_marking_accuracy_label.high:
options["marker"] = "s"
options["s"] = 130
if landmark_accuracy == landmark_accuracy_label.low:
options["c"] = "#1b9e77"
if landmark_accuracy == landmark_accuracy_label.high:
options["c"] = "#d95f02"
return options
legend_text=[]
for lane_marking_accuracy_value in lane_marking_accuracy_label:
for landmark_accuracy_value in landmark_accuracy_label:
x = [get_x_coordinate(single_hidden_variable)for single_hidden_variable in brake_sub_sample()]
y = [single_hidden_variable.log_probability for single_hidden_variable in brake_sub_sample()]
scatter_options = get_scatter_options(lane_marking_accuracy_value, landmark_accuracy_value)
ax.scatter(x, y, **scatter_options,**kwargs)
legend_text.append("LA: {}, LM: {}".format(compress_label(lane_marking_accuracy_value),
compress_label(landmark_accuracy_value)))
title = "Map: {}, Localization: {}".format(subsample[0].map_validity, subsample[0].localization)
ax.set_title(title)
ax.legend(legend_text, fontsize=16)
beautify_axes(ax)
change_x_ticks(ax)
def get_subsamples(in_sample):
out_data=[]
for map_status in map_labels:
for localization_status in localization_label:
new_subsample = [sample_data for sample_data in in_sample if (sample_data.map_validity==map_status) and (sample_data.localization==localization_status)]
out_data.append(new_subsample)
return out_data
def make_plot(in_sample: Sample) -> None:
fig, axs = plt.subplots(2, 2, sharex=True, sharey=True, frameon=False, figsize=(20,15))
subsample = get_subsamples(in_sample)
counter = 0
for row_idx in range(len(axs)):
for col_idx in range(len(axs[0])):
plot_subsamples(axs[row_idx][col_idx], subsample[counter], alpha=0.7)
counter += 1
def get_y_values(node_names, posterior_distributions, symbol_label):
y_values = [None for node in node_names]
for i_posterior in posterior_distributions:
if i_posterior["name"] in node_names:
index = node_names.index(i_posterior["name"])
y_values[index] = i_posterior["distribution"][symbol_label]
return y_values
def make_low_level_plot(ax, x, posterior_distribution, labels):
for i_label in labels:
y = get_y_values(x, posterior_distribution, i_label)
ax.scatter(x, y, label=i_label, s=100)
def plot_sensor_status(ax, posterior_distribution):
x = [camera.label, radar.label, lidar.label]
make_low_level_plot(ax, x, posterior_distribution, sensor_status_label)
def plot_accuracy_status(ax, posterior_distribution):
x = [localization.label, landmark_accuracy.label, lane_marking_accuracy.label]
make_low_level_plot(ax, x, posterior_distribution, accuracy_label)
def plot_posterior(posterior_distributions):
fig, axs = plt.subplots(1, 2, frameon=False, figsize=(15,4))
plot_sensor_status(axs[0], posterior_distributions)
plot_accuracy_status(axs[1], posterior_distributions)
for ax in axs:
ax.legend(fontsize=16)
ax.set_ylim([0, 1])
beautify_axes(ax)
def print_probability_of_map_validity(posterior_distributions):
map_node_index = 3
printmd("**Map posteriori distribution: {}**".format(posterior_distributions[map_node_index]["distribution"]))
def make_analysis(model, weather_symbol, lane_marking_match_symbol, landmark_match_symbol):
title = weather.label + ": " + weather_symbol + ", " + lane_marking_match.label + ": " + lane_marking_match_symbol + ", " + landmark_match.label + ": " + landmark_match_symbol
print("")
printmd("### {}".format(title))
temp_data = compute_a_posteriori(model, weather_symbol,
lane_marking_match_symbol=lane_marking_match_symbol,
landmark_match_symbol=landmark_match_symbol)
plot_posterior(temp_data["posterior"])
plt.show()
print_probability_of_map_validity(temp_data["posterior"])
###Output
_____no_output_____
###Markdown
Simulation results - posterior distributionsThe plot below show the probability distribution of the unknown nodes given the meaurements we have. In the paper here we also add the formal definition of what the posteriors below are, e.g., P(A|....)
###Code
for weather_symbol in weather_label:
for lane_marking_symbol in lane_marking_match_label:
for landmark_match_symbol in landmark_match_label:
make_analysis(model, weather_symbol, lane_marking_symbol, landmark_match_symbol)
temp_data = compute_a_posteriori(model, weather_label.cooperative,
match_label.detected,
match_label.detected)
temp_data['posterior'][0]["distribution"]
def get_digit_of_measurement(measurement_label):
if (measurement_label == weather_label.cooperative) or (measurement_label == match_label.detected):
return 1
else:
return 0
def get_x_coordinate(weather_label, lane_marking_match_label, landmark_match_label):
weather_value = get_digit_of_measurement(weather_label)
lane_marking_value = get_digit_of_measurement(lane_marking_match_label)
landmark_value = get_digit_of_measurement(landmark_match_label)
value = weather_value + lane_marking_value * 2 + landmark_value * 4
return value
def get_label_of_coordinate(x_value):
import math
if (x_value< 0) or (x_value>8):
raise RuntimeError("Unacceptable x value")
weather_value = x_value % 2
lane_marking_value = math.floor(x_value / 2) % 2
landmark_value = math.floor(x_value / 4) % 2
coordinate_label = ""
if weather_value > 0:
coordinate_label += "W"
if lane_marking_value > 0:
coordinate_label += "La"
if landmark_value > 0:
coordinate_label += "Lm"
if not coordinate_label:
return "None"
return coordinate_label
def get_all_x_label_coordinates_for_posterior_plots():
x_values = list(range(8))
return [get_label_of_coordinate(x) for x in x_values]
def change_x_ticks_posterior_plots(ax):
ax.set_xticks(list(range(8)))
ax.set_xticklabels(get_all_x_label_coordinates())
x = []
y_dictionary = {lane_marking_accuracy.label: [],
localization.label: [],
landmark_accuracy.label: []}
for weather_symbol in weather_label:
for lane_marking_symbol in lane_marking_match_label:
for landmark_match_symbol in landmark_match_label:
out_data = compute_a_posteriori(model, weather_symbol, lane_marking_symbol, landmark_match_symbol)
x.append(get_x_coordinate(weather_symbol, lane_marking_symbol, landmark_match_symbol))
for i_posterior in out_data["posterior"]:
if i_posterior["name"] in y_dictionary:
y_dictionary[i_posterior["name"]].append(i_posterior["distribution"][accuracy_label.high])
fig, ax = plt.subplots(1, 1, frameon=False, figsize=(14,6))
for i_data in y_dictionary:
compressed_label = i_data
#if i_data == lane_marking_accuracy.label:
#compress_label = "Lm"
#elif i_data == localization.label:
#compress_label = "Loc"
#else:
#compress_label = "Lm"
label = compress_label# + "_Hi"
ax.scatter(x, y_dictionary[i_data], label=i_data, s=100)
ax.legend(fontsize=16)
ax.set_ylim([0, 1])
ax.set_ylabel("Conditional probability of high accuracy")
beautify_axes(ax)
change_x_ticks_posterior_plots(ax)
x = []
y_dictionary = {camera.label: [],
radar.label: [],
lidar.label: []}
for weather_symbol in weather_label:
for lane_marking_symbol in lane_marking_match_label:
for landmark_match_symbol in landmark_match_label:
out_data = compute_a_posteriori(model, weather_symbol, lane_marking_symbol, landmark_match_symbol)
x.append(get_x_coordinate(weather_symbol, lane_marking_symbol, landmark_match_symbol))
for i_posterior in out_data["posterior"]:
if i_posterior["name"] in y_dictionary:
y_dictionary[i_posterior["name"]].append(i_posterior["distribution"][sensor_status_label.nominal])
fig, ax = plt.subplots(1, 1, frameon=False, figsize=(14,6))
for i_data in y_dictionary:
compressed_label = i_data
#if i_data == lane_marking_accuracy.label:
#compress_label = "Lm"
#elif i_data == localization.label:
#compress_label = "Loc"
#else:
#compress_label = "Lm"
label = compress_label# + "_Hi"
ax.scatter(x, y_dictionary[i_data], label=i_data, s=100)
ax.legend(fontsize=16)
ax.set_ylim([0, 1.1])
ax.set_ylabel("Conditional probability of nominal status")
beautify_axes(ax)
change_x_ticks_posterior_plots(ax)
###Output
_____no_output_____
###Markdown
Not relevant anymore - discard sesssion Simulation results -- probability of each sample The plots below show the *log-probability* of the different combination of node status under the case of map valid and localization accuracy high, map invalid localization accuracy low, etc..**The plots below show the probability of each samples, not the a posteriori of each variable given some measurements.**The x axis shows the combination of sensor status of camera, lidar, and radar. For example: RL means that radar and lidar are in the nominal status and camera is in the degraded status.If all sensors are in the degraded state, then we write "None". The label of the x-axis are visible on the lower plots only.For every x value we have 4 probability values: high lane marking accuracy and high landmark, high lane marking accuracy and low landmakr accuracy, etc...In the legend - LA: Lane marking- LM: landmark Nominal case
###Code
make_plot(compute_probability_of_samples(model,
weather_label.cooperative,
lane_marking_match_symbol=match_label.detected,
landmark_match_symbol=match_label.detected)
)
###Output
_____no_output_____
###Markdown
In this case the highest probability is that map is valid, localization is valid, and all sensors are in the nominal state. **Please note that the probability scale is logaritmic** Cooperative weather, but no matches
###Code
make_plot(compute_probability_of_samples(model,
weather_label.cooperative,
lane_marking_match_symbol=match_label.not_detected,
landmark_match_symbol=match_label.not_detected)
)
###Output
_____no_output_____
###Markdown
In this case, we see that the most likely case is that map is invalid and localization is also with low accuracy. This is interesting, since localization should be high due to the good status of the sensors. At the same time, if the map is invalid, localization cannot be high accuracy, or even valid. Hence the most likely event is that the map is invlaid **and** the localization is low accuracy**NOTE** We may want to change the localization label from high and low, to valid and invalid. Cooperative weather and one match only Lane marking has a match, landmark no
###Code
make_plot(compute_probability_of_samples(model,
weather_label.cooperative,
lane_marking_match_symbol=match_label.detected,
landmark_match_symbol=match_label.not_detected)
)
###Output
_____no_output_____
###Markdown
I am not completely sure of the correctness of this result. There is nothing clearly wrong with it, but it is complex and I would like to make sure that the outcome is reasonable. Lane marking has no match, landmark yes
###Code
make_plot(compute_probability_of_samples(model,
weather_label.cooperative,
lane_marking_match_symbol=match_label.not_detected,
landmark_match_symbol=match_label.detected)
)
###Output
_____no_output_____
###Markdown
Receving operating curveThe idea of this section is to perform an analysis of sensitivity of the model w.r.t. some parameters. It is currently unclear to me, what is the sensitivity we are looking for, but I guess that the ROC could be a good one.The ROC is a curve on two dimensions, see https://de.wikipedia.org/wiki/ROC-KurveFor computing the ROC curve we need to compute the probability of estimating a valid map, given that the map is valid (true positive rate) and the probability to compute a valid map, given that the map is invalid (false negative rate).We can then change some parameters in the model and see what happens to the curve... so idea. Maybe it will even work ;)
###Code
def find_probability_value_in_posterior(posteriors, variable_name, label):
for i_posterior in posteriors:
if i_posterior["name"] == variable_name:
return i_posterior["distribution"][label]
raise RuntimeError("Unable to find given variable_name. Name={}".format(variable_name))
# Returns the first key, value pair of a dictionary
def get_head_of_dictionary(in_dict):
first_pair = list(in_dict.items())[0]
out={}
out[first_pair[0]] = first_pair[1]
return out
# Returns the first key, value pair of a dictionary
def get_tail_of_dictionary(in_dict):
key_value_pairs=list(in_dict.items())[1:]
out = {}
for i_pair in key_value_pairs:
out[i_pair[0]] = i_pair[1]
return out
def compute_conditional_of_symbols(model, symbols_to_compute_a_posteriori, symbols_to_which_to_condition_over):
# we compute here the following probability
# P(a_1, a_2, a_3| b_1,b_2,b_3) = P(a_1|a_2,a_3,b_1,b_2,b_3)*P(a_2|a_3,b_1,b_2,b_3)*P(a_3|a_3,b_1,b_2,b_3)
# This is necessary, since pomegranate can compute the posterior of 1 variable, but not of a group of variables
if len(symbols_to_compute_a_posteriori) == 1:
posterior = compute_a_posteriori_from_symbols(model, symbols_to_which_to_condition_over)
variable_name = list(symbols_to_compute_a_posteriori.keys())[0]
variable_value = list(symbols_to_compute_a_posteriori.values())[0]
probability_value = find_probability_value_in_posterior(posterior["posterior"], variable_name, variable_value)
else:
head_of_symbols_to_compute_a_posteriori = get_head_of_dictionary(symbols_to_compute_a_posteriori)
tail_of_symbols_to_compute_a_posteriori = get_tail_of_dictionary(symbols_to_compute_a_posteriori)
new_conditional = symbols_to_which_to_condition_over.copy()
new_conditional.update(tail_of_symbols_to_compute_a_posteriori)
probability_value = compute_conditional_of_symbols(model, head_of_symbols_to_compute_a_posteriori, new_conditional) * compute_conditional_of_symbols(model, tail_of_symbols_to_compute_a_posteriori, symbols_to_which_to_condition_over)
return probability_value
def get_all_evidence_values_that_lead_to_an_estimated_valid_map(model, probability_valid_map_threshold):
evidence_values_that_lead_to_an_estimated_valid_map = []
for weather_measurement in weather_label:
for lane_marking_match_measurement in lane_marking_match_label:
for landmark_match_measurement in landmark_match_label:
conditioning_symbols = {weather.label: weather_measurement, landmark_match.label: landmark_match_measurement, lane_marking_match.label: lane_marking_match_measurement}
probability_to_find = {map.label: map_labels.valid}
probability_valid_map = compute_conditional_of_symbols(model, probability_to_find, conditioning_symbols)
if probability_valid_map >= probability_valid_map_threshold:
# this conditioning_symbols needs to be in output
evidence_values_that_lead_to_an_estimated_valid_map.append(conditioning_symbols)
return evidence_values_that_lead_to_an_estimated_valid_map
def compute_true_positive(model, probability_valid_map_threshold):
symbols_for_which_probability_need_to_be_computed = get_all_evidence_values_that_lead_to_an_estimated_valid_map(model, probability_valid_map_threshold)
true_positive = 0.0
for i_symbol in symbols_for_which_probability_need_to_be_computed:
probability_of_i_symbol = compute_conditional_of_symbols(model, i_symbol, {map.label: map_labels.valid})
true_positive += probability_of_i_symbol
return true_positive
def compute_false_positive(model, probability_valid_map_threshold):
symbols_for_which_probability_need_to_be_computed = get_all_evidence_values_that_lead_to_an_estimated_valid_map(model, probability_valid_map_threshold)
false_positive = 0.0
for i_symbol in symbols_for_which_probability_need_to_be_computed:
probability_of_i_symbol = compute_conditional_of_symbols(model, i_symbol, {map.label: map_labels.invalid})
false_positive += probability_of_i_symbol
return false_positive
def compute_pair_true_positive_false_positive(model, probability_valid_map_threshold):
true_positive = compute_true_positive(model, probability_valid_map_threshold)
false_positive = compute_false_positive(model, probability_valid_map_threshold)
return (true_positive, false_positive)
compute_pair_true_positive_false_positive(model, 0.8)
###Output
_____no_output_____
###Markdown
ROC curve as function of the map validity threshold probability
###Code
n_threshold_values = 30
threshold = [value / (n_threshold_values) for value in range(n_threshold_values + 1)]
x = [0 for id in threshold]
y = [0 for id in threshold]
for idx in range(len(threshold)):
current_pair = compute_pair_true_positive_false_positive(model, threshold[idx])
x[idx] = current_pair[1]
y[idx] = current_pair[0]
fig, axs = plt.subplots(1, 1, frameon=False, figsize=(6,6))
axs.scatter(x, y, s = 100)
#axs.plot((0, 1), (0, 1), color="k", linewidth=0.5)
axs.set_title("ROC curve")
axs.set_xlabel("False positive")
axs.set_ylabel("True positive")
beautify_axes(axs)
###Output
_____no_output_____
###Markdown
X axes is the probability of false positive, i.e. we declare a map valid when it is not. The y axes is the probability of true positive, i.e. we declare a map valid when it is. This kind of plot must always lie over the 45 degree straight line connecting (0,0) to (1,1), otherwise the estimator makes no sense.**This plot shows the estimator makes sense.**We only have a few points, since the input variables takes discrete values and this set of values leads to a finite number of pairs of true positive, false positive values. TPR and FPR as function of thresholdThe plot below shows the TPR and FPR separately on two plots as function of the threshold used for claiming that a map is valid. Interesting, 0,4 looks like a better value than 0.5**A note on better value**Better value only means *a different compromise* between false positive and true positive. We are not improving the classifier here. We are only selecting the threshold value which leads to a point of the ROC curve which we claim as best compromise.In order to improve the classifier, we need to tune the other parameters of the model. coming soon :)
###Code
fig, axs = plt.subplots(2, 1, frameon=False, figsize=(15,10))
axs[0].scatter(threshold, y, s=80)
axs[0].set_ylabel("True positive")
axs[1].scatter(threshold, x, s=80)
axs[1].set_ylabel("False positive")
axs[1].set_xlabel("Threshold")
beautify_axes(axs[0])
beautify_axes(axs[1])
###Output
_____no_output_____ |
sensepy/tutorial/.ipynb_checkpoints/custom-kernel-cox-process-checkpoint.ipynb | ###Markdown
Tutorial: Sensing Cox Process IIDefining kernels, and plotting maps Fitting the Cox Process
###Code
from stpy.kernels import KernelFunction
from stpy.borel_set import BorelSet,HierarchicalBorelSets
from stpy.point_processes.poisson_rate_estimator import PoissonRateEstimator
import torch
import pandas as pd
import geopandas
import numpy as np
import matplotlib.pyplot as plt
import contextily as ctx
from scipy.interpolate import griddata
from stpy.point_processes.poisson.poisson import PoissonPointProcess
from sensepy.capture_thompson import CaptureThompson
## Demonstration of fitting a Cox Process
# lenghtscale of squared exponential kernel
gamma = 0.12
# borel set a square with boundaries [-1,1]^2
D = BorelSet(2, bounds=torch.Tensor([[-1., 1.], [-1, 1]]).double())
# quadtree with [-1,1], levels signifies number of splits
hs2d = HierarchicalBorelSets(d=2, interval=[(-1, 1), (-1, 1)], levels=5)
# kernel function
k = KernelFunction(kernel_name = "squared_exponential", gamma=gamma, d=2)
# creating estimator - the first argument is Poisson process object if existing
m = 20 # number of basis functions
b = 0.1 # minimal value of the rate function
B = 10e10 # maximal value of the rate function
steps = 200 # number of Langevin steps for sampling
estimator = PoissonRateEstimator(None, hs2d, d=2,
kernel_object=k, B=B, b=b,
m=m, jitter=10e-3,
steps = steps)
# loading data
N = 2000
name = '../benchmarks/data/taxi_data.csv'
df = pd.read_csv(name)
df = df.head(N)
df = df[df['Longitude'] < -8.580]
df = df[df['Longitude'] > -8.64]
df = df[df['Latitude'] > 41.136]
df = df[df['Latitude'] < 41.17]
gdf = geopandas.GeoDataFrame(df, geometry=geopandas.points_from_xy(df.Longitude,df.Latitude))
gdf.crs = "EPSG:4326"
# cleaning nans
obs = df.values[:, [1,2]].astype(float)
obs = obs[~np.isnan(obs)[:, 0], :]
x_max = np.max(obs[:, 0]) # longitude
x_min = np.min(obs[:, 0])
y_max = np.max(obs[:, 1]) # lattitude
y_min = np.min(obs[:, 1])
lat = df['Latitude']
long = df['Longitude']
left, right = long.min(),long.max()
down, up = lat.min(), lat.max()
# transform from map to [-1,1]
transform_x = lambda x: (2 / (x_max - x_min)) * x + (1 - (2 * x_max / (x_max - x_min)))
transform_y = lambda y: (2 / (y_max - y_min)) * y + (1 - (2 * y_max / (y_max - y_min)))
# transform from [-1,1] to map
inv_transform_x = lambda x: (x_max - x_min) / 2 * x + (x_min + x_max) / 2
inv_transform_y = lambda x: (y_max - y_min) / 2 * x + (y_min + y_max) / 2
# transform to [-1,1]
obs[:, 0] = np.apply_along_axis(transform_x, 0, obs[:, 0])
obs[:, 1] = np.apply_along_axis(transform_y, 0, obs[:, 1])
# extract temporal information of the dataset
df['Date']= pd.to_datetime(df['Date'])
# time section of the dataset in minutes
dt = (df['Date'].max() - df['Date'].min()).seconds//60
data = [(D, torch.from_numpy(obs), dt)]
# load data in the above format
estimator.load_data(data)
# fit the Cox process by calculating MAP
estimator.fit_gp()
# Plotting the fitted Map
n = 30 # discretization
xtest = D.return_discretization(n)
Map = BorelSet(d=2, bounds=torch.Tensor([[left, right], [down, up]]).double())
xtest_orig = Map.return_discretization(n).numpy()
f = estimator.rate_value(xtest)
xx = xtest_orig[:, 0]
yy = xtest_orig[:, 1]
grid_x, grid_y = np.mgrid[min(xx):max(xx):100j, min(yy):max(yy):100j]
grid_z_f = griddata((xx, yy), f[:, 0].detach().numpy(), (grid_x, grid_y), method='linear')
fig, ax = plt.subplots(figsize=(10, 10))
gdf.plot(ax=ax, color='red', figsize=(10, 10))
cs = ax.contourf(grid_x, grid_y, grid_z_f, levels=20, alpha=0.5)
ax.contour(cs, colors='k', alpha=0.5)
ctx.add_basemap(ax, crs=gdf.crs.to_string())
plt.show()
###Output
_____no_output_____ |
ex01/Antonio_Angulo/Ex01_Antonio.ipynb | ###Markdown
Esté um notebook Colab contendo exercícios de programação em python, numpy e pytorch. Coloque seu nome
###Code
print('Meu nome é: Antonio Lincoln Angulo Salas')
###Output
_____no_output_____
###Markdown
Parte 1:Exercícios de Processamento de DadosNesta parte pode-se usar as bibliotecas nativas do python como a `collections`, `re` e `random`. Também pode-se usar o NumPy. Exercício 1.1Crie um dicionário com os `k` itens mais frequentes de uma lista.Por exemplo, dada a lista de itens `L=['a', 'a', 'd', 'b', 'd', 'c', 'e', 'a', 'b', 'e', 'e', 'a']` e `k=2`, o resultado deve ser um dicionário cuja chave é o item e o valor é a sua frequência: {'a': 4, 'e': 3}
###Code
def top_k(L, k):
i_count = {}
for i in L:
if i in i_count:
i_count[i] += 1
else:
i_count[i] = 1
resultado ={}
for j in range(k):
if len(str(max(i_count.values())))>0:
val = max(i_count.values())
for key, value in i_count.items():
if val == value:
resultado[key] = val
del i_count[key]
break
else:
continue
return resultado
###Output
_____no_output_____
###Markdown
Mostre que sua implementação está correta usando uma entrada com poucos itens:
###Code
L = ['f', 'a', 'a', 'd', 'b', 'd', 'c', 'e', 'a', 'b', 'e', 'e', 'a', 'd']
k = 3
resultado = top_k(L=L, k=k)
print(f'resultado: {resultado}')
###Output
resultado: {'a': 4, 'd': 3, 'e': 3}
###Markdown
Mostre que sua implementação é eficiente usando uma entrada com 10M de itens:
###Code
import random
L = random.choices('abcdefghijklmnopqrstuvwxyz', k=10_000_000)
k = 10000
%%timeit
resultado = top_k(L=L, k=k)
###Output
100000 loops, best of 5: 6.48 µs per loop
###Markdown
Exercício 1.2Em processamento de linguagem natural, é comum convertemos as palavras de um texto para uma lista de identificadores dessas palavras. Dado o dicionário `V` abaixo onde as chaves são palavras e os valores são seus respectivos identificadores, converta o texto `D` para uma lista de identificadores.Palavras que não existem no dicionário deverão ser convertidas para o identificador do token `unknown`.O código deve ser insensível a maiúsculas (case-insensitive).Se atente que pontuações (vírgulas, ponto final, etc) também são consideradas palavras.
###Code
def tokens_to_ids(text, vocabulary):
id = {}
text = text.lower()
for k,v in vocabulary.items():
text = text.replace(k,' ' + str(v))
id = text.split()
for g in range(len(id)):
if not id[g].isdigit():
id[g]= '-1'
return id
###Output
_____no_output_____
###Markdown
Mostre que sua implementação esta correta com um exemplo pequeno:---
###Code
V = {'eu': 1, 'de': 2, 'gosto': 3, 'comer': 4, '.': 5, 'unknown': -1}
D = 'Eu gosto de comer pizza.'
print(tokens_to_ids(D, V))
###Output
['1', '3', '2', '4', '-1', '5']
###Markdown
Mostre que sua implementação é eficiente com um exemplo grande:
###Code
V = {'eu': 1, 'de': 2, 'gosto': 3, 'comer': 4, '.': 5, 'unknown': -1}
D = ' '.join(1_000_000 * ['Eu gosto de comer pizza.'])
%%timeit
resultado = tokens_to_ids(D, V)
###Output
1 loop, best of 5: 1.16 s per loop
###Markdown
Exercício 1.3Em aprendizado profundo é comum termos que lidar com arquivos muito grandes.Dado um arquivo de texto onde cada item é separado por `\n`, escreva um programa que amostre `k` itens desse arquivo aleatoriamente.Nota 1: Assuma amostragem de uma distribuição uniforme, ou seja, todos os itens tem a mesma probablidade de amostragem.Nota 2: Assuma que o arquivo não cabe em memória.Nota 3: Utilize apenas bibliotecas nativas do python.
###Code
def sample(path: str, k: int):
import random
rndSamples = sorted(random.sample(range(0,total_size-1),total_size - n_samples))
with open(filename, 'r') as file:
samples = file.read().splitlines()
for ele in sorted(rndSamples, reverse = True):
del samples[ele]
return samples
###Output
_____no_output_____
###Markdown
Mostre que sua implementação está correta com um exemplo pequeno:
###Code
filename = 'small.txt'
total_size = 100
n_samples = 10
with open(filename, 'w') as fout:
fout.write('\n'.join(f'line {i}' for i in range(total_size)))
samples = sample(path=filename, k=n_samples)
print(samples)
print(len(samples) == n_samples)
###Output
['line 12', 'line 20', 'line 21', 'line 29', 'line 37', 'line 48', 'line 59', 'line 83', 'line 85', 'line 99']
True
###Markdown
Mostre que sua implementação é eficiente com um exemplo grande:
###Code
filename = 'large.txt'
total_size = 1_000_000
n_samples = 10000
with open(filename, 'w') as fout:
fout.write('\n'.join(f'line {i}' for i in range(total_size)))
%%timeit
samples = sample(path=filename, k=n_samples)
assert len(samples) == n_samples
###Output
1 loop, best of 5: 2.76 s per loop
###Markdown
Parte 2:Exercícios de NumpyNesta parte deve-se usar apenas a biblioteca NumPy. Aqui não se pode usar o PyTorch. Exercício 2.1Quantos operações de ponto flutuante (flops) de soma e de multiplicação tem a multiplicação matricial $AB$, sendo que a matriz $A$ tem tamanho $m \times n$ e a matriz $B$ tem tamanho $n \times p$? Resposta:- número de somas: $m \times p$- número de multiplicações: $m \times n \times p$ Exercício 2.2Em programação matricial, não se faz o loop em cada elemento da matriz,mas sim, utiliza-se operações matriciais.Dada a matriz `A` abaixo, calcule a média dos valores de cada linha sem utilizar laços explícitos.Utilize apenas a biblioteca numpy.
###Code
import numpy as np
A = np.arange(24).reshape(4, 6)
print(A)
print(A.mean())
###Output
11.5
###Markdown
Exercício 2.3Seja a matriz $C$ que é a normalização da matriz $A$:$$ C(i,j) = \frac{A(i,j) - A_{min}}{A_{max} - A_{min}} $$Normalizar a matriz `A` do exercício acima de forma que seus valores fiquem entre 0 e 1.
###Code
Amin = A.min()
Amax = A.max()
C = (A-Amin)/(Amax-Amin)
print(C)
###Output
[[0. 0.04347826 0.08695652 0.13043478 0.17391304 0.2173913 ]
[0.26086957 0.30434783 0.34782609 0.39130435 0.43478261 0.47826087]
[0.52173913 0.56521739 0.60869565 0.65217391 0.69565217 0.73913043]
[0.7826087 0.82608696 0.86956522 0.91304348 0.95652174 1. ]]
###Markdown
Exercício 2.4Modificar o exercício anterior de forma que os valores de cada *coluna* da matriz `A` sejam normalizados entre 0 e 1 independentemente dos valores das outras colunas.
###Code
Amin = A.min(0)
Amax = A.max(0)
C = (A-Amin)/(Amax-Amin)
print(C)
###Output
[[0. 0. 0. 0. 0. 0. ]
[0.33333333 0.33333333 0.33333333 0.33333333 0.33333333 0.33333333]
[0.66666667 0.66666667 0.66666667 0.66666667 0.66666667 0.66666667]
[1. 1. 1. 1. 1. 1. ]]
###Markdown
Exercício 2.5Modificar o exercício anterior de forma que os valores de cada *linha* da matriz `A` sejam normalizados entre 0 e 1 independentemente dos valores das outras linhas.
###Code
Amin = A.min(1)
Amax = A.max(1)
C = (A.transpose()-Amin)/(Amax-Amin)
print(C.transpose())
###Output
[[0. 0.2 0.4 0.6 0.8 1. ]
[0. 0.2 0.4 0.6 0.8 1. ]
[0. 0.2 0.4 0.6 0.8 1. ]
[0. 0.2 0.4 0.6 0.8 1. ]]
###Markdown
Exercício 2.6A [função softmax](https://en.wikipedia.org/wiki/Softmax_function) é bastante usada em apredizado de máquina para converter uma lista de números para uma distribuição de probabilidade, isto é, os números ficarão normalizados entre zero e um e sua soma será igual à um.Implemente a função softmax com suporte para batches, ou seja, o softmax deve ser aplicado a cada linha da matriz. Deve-se usar apenas a biblioteca numpy. Se atente que a exponenciação gera estouro de representação quando os números da entrada são muito grandes. Tente corrigir isto.
###Code
import numpy as np
def softmax(A):
'''
Aplica a função de softmax à matriz `A`.
Entrada:
`A` é uma matriz M x N, onde M é o número de exemplos a serem processados
independentemente e N é o tamanho de cada exemplo.
Saída:
Uma matriz M x N, onde a soma de cada linha é igual a um.
'''
Amax = A.max(1,keepdims=True)
exp_A = np.exp(A - Amax)
Asum = np.sum(exp_A,axis=1,keepdims=True)
s_max = exp_A / Asum
return s_max
###Output
_____no_output_____
###Markdown
Mostre que sua implementação está correta usando uma matriz pequena como entrada:
###Code
A = np.array([[0.5, -1, 1000],
[-2, 0, 0.5]])
softmax(A)
###Output
_____no_output_____
###Markdown
O código a seguir verifica se sua implementação do softmax está correta. - A soma de cada linha de A deve ser 1;- Os valores devem estar entre 0 e 1
###Code
np.allclose(softmax(A).sum(axis=1), 1) and softmax(A).min() >= 0 and softmax(A).max() <= 1
###Output
_____no_output_____
###Markdown
Mostre que sua implementação é eficiente usando uma matriz grande como entrada:
###Code
A = np.random.uniform(low=-10, high=10, size=(128, 100_000))
%%timeit
softmax(A)
SM = softmax(A)
np.allclose(SM.sum(axis=1), 1) and SM.min() >= 0 and SM.max() <= 1
###Output
_____no_output_____
###Markdown
Exercício 2.7A codificação one-hot é usada para codificar entradas categóricas. É uma codificação onde apenas um bit é 1 e os demais são zero, conforme a tabela a seguir.| Decimal | Binary | One-hot| ------- | ------ | -------| 0 | 000 | 1 0 0 0 0 0 0 0| 1 | 001 | 0 1 0 0 0 0 0 0| 2 | 010 | 0 0 1 0 0 0 0 0| 3 | 011 | 0 0 0 1 0 0 0 0| 4 | 100 | 0 0 0 0 1 0 0 0| 5 | 101 | 0 0 0 0 0 1 0 0| 6 | 110 | 0 0 0 0 0 0 1 0| 7 | 111 | 0 0 0 0 0 0 0 1 Implemente a função one_hot(y, n_classes) que codifique o vetor de inteiros y que possuem valores entre 0 e n_classes-1.
###Code
def one_hot(y, n_classes):
oneHotM = np.zeros((y.size, y.max()+1))
oneHotM[np.arange(y.size),y] = 1
return oneHotM
N_CLASSES = 9
N_SAMPLES = 10
y = (np.random.rand((N_SAMPLES)) * N_CLASSES).astype(np.int)
print(y)
print(one_hot(y, N_CLASSES))
###Output
[3 7 6 2 7 5 3 8 1 5]
[[0. 0. 0. 1. 0. 0. 0. 0. 0.]
[0. 0. 0. 0. 0. 0. 0. 1. 0.]
[0. 0. 0. 0. 0. 0. 1. 0. 0.]
[0. 0. 1. 0. 0. 0. 0. 0. 0.]
[0. 0. 0. 0. 0. 0. 0. 1. 0.]
[0. 0. 0. 0. 0. 1. 0. 0. 0.]
[0. 0. 0. 1. 0. 0. 0. 0. 0.]
[0. 0. 0. 0. 0. 0. 0. 0. 1.]
[0. 1. 0. 0. 0. 0. 0. 0. 0.]
[0. 0. 0. 0. 0. 1. 0. 0. 0.]]
###Markdown
Mostre que sua implementação é eficiente usando uma matriz grande como entrada:
###Code
N_SAMPLES = 100_000
N_CLASSES = 1_000
y = (np.random.rand((N_SAMPLES)) * N_CLASSES).astype(np.int)
%%timeit
one_hot(y, N_CLASSES)
###Output
1 loop, best of 5: 184 ms per loop
###Markdown
Exercício 2.8Implemente uma classe que normalize um array de pontos flutuantes `array_a` para a mesma média e desvio padrão de um outro array `array_b`, conforme exemplo abaixo:```array_a = np.array([-1, 1.5, 0])array_b = np.array([1.4, 0.8, 0.3, 2.5])normalize = Normalizer(array_b)normalized_array = normalize(array_a)print(normalized_array) Deve imprimir [0.3187798 2.31425165 1.11696854]```
###Code
class Normalizer(object):
def __init__(self, b_arr):
b = np.array(b_arr)
self.bm = b.mean()
self.bstd = b.std()
def __call__(self, a_arr):
a = np.array(a_arr)
self.am = a.mean()
self.astd = a.std()
self.n = (a - self.am)/self.astd
return self.n * self.bstd + self.bm
###Output
_____no_output_____
###Markdown
Mostre que seu código está correto com o exemplo abaixo:
###Code
import numpy as np
array_a = np.array([-1, 1.5, 0])
array_b = np.array([1.4, 0.8, 0.3, 2.5])
normalize = Normalizer(array_b)
normalized_array = normalize(array_a)
print(normalized_array)
###Output
_____no_output_____
###Markdown
Parte 3:Exercícios Pytorch: Grafo Computacional e GradientesNesta parte pode-se usar quaisquer bibliotecas. Um dos principais fundamentos para que o PyTorch seja adequado para deep learning é a sua habilidade de calcular o gradiente automaticamente a partir da expressões definidas. Essa facilidade é implementada através do cálculo automático do gradiente e construção dinâmica do grafo computacional. Grafo computacionalSeja um exemplo simples de uma função de perda J dada pela Soma dos Erros ao Quadrado (SEQ - Sum of Squared Errors): $$ J = \sum_i (x_i w - y_i)^2 $$que pode ser reescrita como:$$ \hat{y_i} = x_i w $$$$ e_i = \hat{y_i} - y_i $$$$ e2_i = e_i^2 $$$$ J = \sum_i e2_i $$As redes neurais são treinadas através da minimização de uma função de perda usando o método do gradiente descendente. Para ajustar o parâmetro $w$ precisamos calcular o gradiente $ \frac{ \partial J}{\partial w} $. Usando aregra da cadeia podemos escrever:$$ \frac{ \partial J}{\partial w} = \frac{ \partial J}{\partial e2_i} \frac{ \partial e2_i}{\partial e_i} \frac{ \partial e_i}{\partial \hat{y_i} } \frac{ \partial \hat{y_i}}{\partial w}$$ ``` y_pred = x * w e = y_pred - y e2 = e**2 J = e2.sum()``` As quatro expressões acima, para o cálculo do J podem ser representadas pelo grafo computacional visualizado a seguir: os círculos são as variáveis (tensores), os quadrados são as operações, os números em preto são os cálculos durante a execução das quatro expressões para calcular o J (forward, predict). O cálculo do gradiente, mostrado em vermelho, é calculado pela regra da cadeia, de trás para frente (backward). Para entender melhor o funcionamento do grafo computacional com os tensores, recomenda-se leitura em:https://pytorch.org/docs/stable/notes/autograd.html
###Code
import torch
torch.__version__
###Output
_____no_output_____
###Markdown
**Tensor com atributo .requires_grad=True**Quando um tensor possui o atributo `requires_grad` como verdadeiro, qualquer expressão que utilizar esse tensor irá construir um grafo computacional para permitir posteriormente, após calcular a função a ser derivada, poder usar a regra da cadeia e calcular o gradiente da função em termos dos tensores que possuem o atributo `requires_grad`.
###Code
y = torch.arange(0, 8, 2).float()
y
x = torch.arange(0, 4).float()
x
w = torch.ones(1, requires_grad=True)
w
###Output
_____no_output_____
###Markdown
Cálculo automático do gradiente da função perda J Seja a expressão: $$ J = \sum_i ((x_i w) - y_i)^2 $$Queremos calcular a derivada de $J$ em relação a $w$. Forward passDurante a execução da expressão, o grafo computacional é criado. Compare os valores de cada parcela calculada com os valores em preto da figura ilustrativa do grafo computacional.
###Code
# predict (forward)
y_pred = x * w; print('y_pred =', y_pred)
# cálculo da perda J: loss
e = y_pred - y; print('e =',e)
e2 = e.pow(2) ; print('e2 =', e2)
J = e2.sum() ; print('J =', J)
###Output
y_pred = tensor([0., 1., 2., 3.], grad_fn=<MulBackward0>)
e = tensor([ 0., -1., -2., -3.], grad_fn=<SubBackward0>)
e2 = tensor([0., 1., 4., 9.], grad_fn=<PowBackward0>)
J = tensor(14., grad_fn=<SumBackward0>)
###Markdown
Backward pass O `backward()` varre o grafo computacional a partir da variável a ele associada (raiz) e calcula o gradiente para todos os tensores que possuem o atributo `requires_grad` como verdadeiro.Observe que os tensores que tiverem o atributo `requires_grad` serão sempre folhas no grafo computacional.O `backward()` destroi o grafo após sua execução. Esse comportamento é padrão no PyTorch. A título ilustrativo, se quisermos depurar os gradientes dos nós que não são folhas no grafo computacional, precisamos primeiro invocar `retain_grad()` em cada um desses nós, como a seguir. Entretanto nos exemplos reais não há necessidade de verificar o gradiente desses nós.
###Code
e2.retain_grad()
e.retain_grad()
y_pred.retain_grad()
###Output
_____no_output_____
###Markdown
E agora calculamos os gradientes com o `backward()`.w.grad é o gradiente de J em relação a w.
###Code
if w.grad: w.grad.zero_()
J.backward()
print(w.grad)
###Output
tensor([-28.])
###Markdown
Mostramos agora os gradientes que estão grafados em vermelho no grafo computacional:
###Code
print(e2.grad)
print(e.grad)
print(y_pred.grad)
###Output
tensor([1., 1., 1., 1.])
tensor([ 0., -2., -4., -6.])
tensor([ 0., -2., -4., -6.])
###Markdown
Exercício 3.1Calcule o mesmo gradiente ilustrado no exemplo anterior usando a regra das diferenças finitas, de acordo com a equação a seguir, utilizando um valor de $\Delta w$ bem pequeno.$$ \frac{\partial J}{\partial w} = \frac{J(w + \Delta w) - J(w - \Delta w)}{2 \Delta w} $$
###Code
def J_func(w, x, y):
return (x * w - y).pow(2).sum()
# Calcule o gradiente usando a regra diferenças finitas
# Confira com o valor já calculado anteriormente
x = torch.arange(0, 4).float()
y = torch.arange(0, 8, 2).float()
w = torch.ones(1)
dw = 0.1
grad = grad = (J_func(w + dw, x, y) - J_func(w - dw, x, y)) / (2 * dw)
print('grad=', grad)
###Output
grad= tensor(-28.0000)
###Markdown
Exercício 3.2Minimizando $J$ pelo gradiente descendente$$ w_{k+1} = w_k - \lambda \frac {\partial J}{\partial w} $$Supondo que valor inicial ($k=0$) $w_0 = 1$, use learning rate $\lambda = 0.01$ para calcular o valor do novo $w_{20}$, ou seja, fazendo 20 atualizações de gradientes. Deve-se usar a função `J_func` criada no exercício anterior.Confira se o valor do primeiro gradiente está de acordo com os valores já calculado acima
###Code
import matplotlib.pyplot as plt
learning_rate = 0.01
iteracoes = 20
x = torch.arange(0, 4).float()
y = torch.arange(0, 8, 2).float()
w = torch.ones(1)
X = []
Y = []
for i in range(iteracoes):
J = J_func(w, x, y)
X.append(i)
Y.append(J)
grad = (J_func(w + dw, x, y) - J_func(w - dw, x, y)) / 2 / dw
w -= learning_rate * grad
print('i =', i, '\tJ=', J, '\tgrad =',grad, '\tw =', w)
# Plote o gráfico da loss J pela iteração i
plt.plot(X, Y)
plt.xlabel('Iteração')
plt.ylabel('Loss')
plt.show()
###Output
i = 0 J= tensor(14.) grad = tensor(-28.0000) w = tensor([1.2800])
i = 1 J= tensor(7.2576) grad = tensor(-20.1600) w = tensor([1.4816])
i = 2 J= tensor(3.7623) grad = tensor(-14.5152) w = tensor([1.6268])
i = 3 J= tensor(1.9504) grad = tensor(-10.4509) w = tensor([1.7313])
i = 4 J= tensor(1.0111) grad = tensor(-7.5247) w = tensor([1.8065])
i = 5 J= tensor(0.5241) grad = tensor(-5.4178) w = tensor([1.8607])
i = 6 J= tensor(0.2717) grad = tensor(-3.9008) w = tensor([1.8997])
i = 7 J= tensor(0.1409) grad = tensor(-2.8086) w = tensor([1.9278])
i = 8 J= tensor(0.0730) grad = tensor(-2.0222) w = tensor([1.9480])
i = 9 J= tensor(0.0379) grad = tensor(-1.4560) w = tensor([1.9626])
i = 10 J= tensor(0.0196) grad = tensor(-1.0483) w = tensor([1.9730])
i = 11 J= tensor(0.0102) grad = tensor(-0.7548) w = tensor([1.9806])
i = 12 J= tensor(0.0053) grad = tensor(-0.5434) w = tensor([1.9860])
i = 13 J= tensor(0.0027) grad = tensor(-0.3913) w = tensor([1.9899])
i = 14 J= tensor(0.0014) grad = tensor(-0.2817) w = tensor([1.9928])
i = 15 J= tensor(0.0007) grad = tensor(-0.2028) w = tensor([1.9948])
i = 16 J= tensor(0.0004) grad = tensor(-0.1460) w = tensor([1.9962])
i = 17 J= tensor(0.0002) grad = tensor(-0.1052) w = tensor([1.9973])
i = 18 J= tensor(0.0001) grad = tensor(-0.0757) w = tensor([1.9981])
i = 19 J= tensor(5.3059e-05) grad = tensor(-0.0545) w = tensor([1.9986])
###Markdown
Exercício 3.3Repita o exercício 2 mas usando agora o calculando o gradiente usando o método backward() do pytorch. Confira se o primeiro valor do gradiente está de acordo com os valores anteriores. Execute essa próxima célula duas vezes. Os valores devem ser iguais.
###Code
import matplotlib.pyplot as plt
learning_rate = 0.01
iteracoes = 20
x = torch.arange(0, 4).float()
y = torch.arange(0, 8, 2).float()
w = torch.ones(1, requires_grad=True)
X = []
Y = []
for i in range(iteracoes):
J = J_func(w, x, y)
X.append(i)
Y.append(J.detach())
J.backward()
grad = w.grad
with torch.no_grad():
w -= learning_rate * grad
print('i =', i, '\tJ=', J, '\tgrad =',grad, '\tw =', w)
w.grad.zero_()
# Plote aqui a loss pela iteração
plt.plot(X, Y)
plt.xlabel('Iteração')
plt.ylabel('Loss')
plt.show()
###Output
i = 0 J= tensor(14., grad_fn=<SumBackward0>) grad = tensor([-28.]) w = tensor([1.2800], requires_grad=True)
i = 1 J= tensor(7.2576, grad_fn=<SumBackward0>) grad = tensor([-20.1600]) w = tensor([1.4816], requires_grad=True)
i = 2 J= tensor(3.7623, grad_fn=<SumBackward0>) grad = tensor([-14.5152]) w = tensor([1.6268], requires_grad=True)
i = 3 J= tensor(1.9504, grad_fn=<SumBackward0>) grad = tensor([-10.4509]) w = tensor([1.7313], requires_grad=True)
i = 4 J= tensor(1.0111, grad_fn=<SumBackward0>) grad = tensor([-7.5247]) w = tensor([1.8065], requires_grad=True)
i = 5 J= tensor(0.5241, grad_fn=<SumBackward0>) grad = tensor([-5.4178]) w = tensor([1.8607], requires_grad=True)
i = 6 J= tensor(0.2717, grad_fn=<SumBackward0>) grad = tensor([-3.9008]) w = tensor([1.8997], requires_grad=True)
i = 7 J= tensor(0.1409, grad_fn=<SumBackward0>) grad = tensor([-2.8086]) w = tensor([1.9278], requires_grad=True)
i = 8 J= tensor(0.0730, grad_fn=<SumBackward0>) grad = tensor([-2.0222]) w = tensor([1.9480], requires_grad=True)
i = 9 J= tensor(0.0379, grad_fn=<SumBackward0>) grad = tensor([-1.4560]) w = tensor([1.9626], requires_grad=True)
i = 10 J= tensor(0.0196, grad_fn=<SumBackward0>) grad = tensor([-1.0483]) w = tensor([1.9730], requires_grad=True)
i = 11 J= tensor(0.0102, grad_fn=<SumBackward0>) grad = tensor([-0.7548]) w = tensor([1.9806], requires_grad=True)
i = 12 J= tensor(0.0053, grad_fn=<SumBackward0>) grad = tensor([-0.5434]) w = tensor([1.9860], requires_grad=True)
i = 13 J= tensor(0.0027, grad_fn=<SumBackward0>) grad = tensor([-0.3913]) w = tensor([1.9899], requires_grad=True)
i = 14 J= tensor(0.0014, grad_fn=<SumBackward0>) grad = tensor([-0.2817]) w = tensor([1.9928], requires_grad=True)
i = 15 J= tensor(0.0007, grad_fn=<SumBackward0>) grad = tensor([-0.2028]) w = tensor([1.9948], requires_grad=True)
i = 16 J= tensor(0.0004, grad_fn=<SumBackward0>) grad = tensor([-0.1460]) w = tensor([1.9962], requires_grad=True)
i = 17 J= tensor(0.0002, grad_fn=<SumBackward0>) grad = tensor([-0.1052]) w = tensor([1.9973], requires_grad=True)
i = 18 J= tensor(0.0001, grad_fn=<SumBackward0>) grad = tensor([-0.0757]) w = tensor([1.9981], requires_grad=True)
i = 19 J= tensor(5.3059e-05, grad_fn=<SumBackward0>) grad = tensor([-0.0545]) w = tensor([1.9986], requires_grad=True)
|
notebooks/1.0-MS-GlassdoorScraper.ipynb | ###Markdown
Table of Contents1 Importing libraries2 Function definitions3 Scraping data science jobs from Glassdoor Importing libraries
###Code
from selenium.webdriver.common.action_chains import ActionChains
from selenium.common.exceptions import NoSuchElementException, ElementClickInterceptedException
from selenium import webdriver
import time
import pandas as pd
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
from selenium.common.exceptions import TimeoutException, NoSuchElementException
from selenium.webdriver.common.keys import Keys
from selenium.webdriver.common.action_chains import ActionChains
from fake_useragent import UserAgent
from urllib.request import urlopen, Request
from urllib.parse import urlparse
from bs4 import BeautifulSoup as soup
from IPython.core.display import display, HTML
display(HTML("<style>.container { width:100% !important; }</style>"))
display(HTML("<style>.output_result { max-width:100% !important; }</style>"))
#display(HTML('<style>.prompt{width: 0px; min-width: 0px; visibility: collapse}</style>'))
import warnings
warnings.filterwarnings('ignore')
warnings.filterwarnings("ignore", category=UserWarning, module='bs4')
pd.options.display.max_rows = 999
###Output
_____no_output_____
###Markdown
Function definitions
###Code
def requestAndParse(url):
try:
# define headers to be provided for request authentication
headers = {'User-Agent': 'Mozilla/5.0 (X11; Linux x86_64) '
'AppleWebKit/537.11 (KHTML, like Gecko) '
'Chrome/23.0.1271.64 Safari/537.11',
'Accept': 'text/html,application/xhtml+xml,application/xml;q=0.9,*/*;q=0.8',
'Accept-Charset': 'ISO-8859-1,utf-8;q=0.7,*;q=0.3',
'Accept-Encoding': 'none',
'Accept-Language': 'en-US,en;q=0.8',
'Connection': 'keep-alive'}
request_obj = Request(url = url, headers = headers)
opened_url = urlopen(request_obj)
page_html = opened_url.read()
opened_url.close()
page_soup = soup(page_html, "html.parser")
return page_soup, requested_url
except Exception as e:
print(e)
#Initializes driver
def initialize_driver(path):
#Initializing the webdriver
options = webdriver.ChromeOptions()
ua = UserAgent()
userAgent = ua.random
options.add_argument(f'user-agent={userAgent}')
#makes the driver more 'undetectale'
options.add_argument("--disable-blink-features"), options.add_argument("--disable-blink-features=AutomationControlled")
options.add_experimental_option("excludeSwitches", ["enable-automation"])
options.add_experimental_option('useAutomationExtension', False)
#Change the path to where chromedriver is in your home folder.
driver = webdriver.Chrome(executable_path=path, options=options)
driver.maximize_window()
return driver
#Brings job ids from job position and location search
def job_ids(driver, keyword, location,pagelimit=33):
eids = []
jids = []
pagenum_limit = 33
pagenum = 0
try:
# fill in search bar keyword field
position_field = driver.find_element_by_xpath("//*[@id='sc.keyword']")
position_field.send_keys(Keys.COMMAND+'a')
position_field.send_keys(Keys.DELETE)
position_field.send_keys(keyword)
# fill in search bar location field
location_field = driver.find_element_by_xpath("//*[@id='sc.location']")
location_field.send_keys(Keys.COMMAND+'a')
location_field.send_keys(Keys.DELETE)
location_field.send_keys(location)
# click search button and then view all jobs
time.sleep(2)
driver.find_element_by_xpath("//*[@type='submit']").click()
time.sleep(3)
driver.find_element_by_xpath("//a[@data-test='jobs-location-see-all-link']").click()
while int(pagenum) <= pagelimit:
# close a random popup if it shows up
try:
driver.find_element_by_xpath("//*[@id='JAModal']/div/div[2]/span").click()
except NoSuchElementException:
pass
try:
driver.find_element_by_css_selector('[alt="Close"]').click() #clicking to the X.
except Exception:
pass
time.sleep(1)
eids += [x.get_attribute('data-brandviews').split(':')[2][4:] for x in driver.find_elements_by_css_selector("li.react-job-listing")]
jids += [x.get_attribute('data-id') for x in driver.find_elements_by_css_selector("li.react-job-listing")]
# close a random popup if it shows up
try:
driver.find_element_by_xpath("//*[@id='JAModal']/div/div[2]/span").click()
except NoSuchElementException:
pass
pages = driver.find_element_by_xpath("//div[@data-test='ResultsFooter']").text.split()
pagenum = pages[1]
if(pagenum == pages[3]):
break
# go to next page
try:
driver.find_element_by_xpath('.//a[@data-test="pagination-next"]').click()
except NoSuchElementException:
pass
return eids, jids
except NoSuchElementException:
pass
def parse_url(url):
try:
# define headers to be provided for request authentication
headers = {'User-Agent': 'Mozilla/5.0 (X11; Linux x86_64) '
'AppleWebKit/537.11 (KHTML, like Gecko) '
'Chrome/23.0.1271.64 Safari/537.11',
'Accept': 'text/html,application/xhtml+xml,application/xml;q=0.9,*/*;q=0.8',
'Accept-Charset': 'ISO-8859-1,utf-8;q=0.7,*;q=0.3',
'Accept-Encoding': 'none',
'Accept-Language': 'en-US,en;q=0.8',
'Connection': 'keep-alive'}
request_obj = Request(url = url, headers = headers)
opened_url = urlopen(request_obj)
page_html = opened_url.read()
opened_url.close()
page_soup = soup(page_html, "html.parser")
if type(page_soup.find("div", class_="css-ur1szg e11nt52q0").find("span", class_="css-1pmc6te e11nt52q4")) == type(None):
rating=''
company=page_soup.find("div", class_="css-16nw49e e11nt52q1").getText()
else:
rating = page_soup.find("div", class_="css-ur1szg e11nt52q0").find("span", class_="css-1pmc6te e11nt52q4").getText()
company = page_soup.find("div", class_="css-16nw49e e11nt52q1").getText().replace(rating,'')
rating = rating[:-1]
position = page_soup.find("div", class_="css-17x2pwl e11nt52q6").getText()
jobloc = page_soup.find("div", class_="css-1v5elnn e11nt52q2").getText()
if type(page_soup.find("span", class_="small css-10zcshf e1v3ed7e1")) == type(None):
salary=''
else:
salary = page_soup.find("span", class_="small css-10zcshf e1v3ed7e1").getText()#.split(':')[1].strip()
desc = ' '.join([para.get_text() for para in page_soup.find("div", {'id':"JobDescriptionContainer"}).find_all(['li','p'])])
except Exception as e:
print(e)
rating=''
company=''
position=''
jobloc=''
salary=''
desc=''
return position, desc, salary, jobloc, company, rating
###Output
_____no_output_____
###Markdown
Scraping data science jobs from Glassdoor
###Code
driver = initialize_driver("/usr/local/bin/chromedriver")
url = "https://www.glassdoor.com/blog/tag/job-search/"
driver.get(url)
locations = ['remote', 'boulder, co', 'detroit, mi', 'saint louis, mo', 'new orleans, la', 'las vegas, nv', 'new mexico',
'salt lake city, ut', 'cincinnati, oh', 'bentonville, ar', 'minneapolis, mn', 'madison, wi', 'sacramento, ca',
'new jersey', 'houston, tx', 'san antonio, tx', 'san diego, ca', 'fort worth, tx', 'jacksonville, fl',
'columbus, oh', 'north carolina', 'indianapolis, in', 'washington, dc']
for location in locations:
print('Getting Job IDs for ', location)
eids, jids = job_ids(driver,'data science jobs', location)
time.sleep(1)
pd.DataFrame({'eids':eids, 'jids':jids}).to_csv("_".join(keyword.split()+location.split(', '))+'.csv')
keyword='data scientist'
jobdict = {'position':[], 'desc':[], 'salary':[], 'jobloc':[], 'company':[], 'rating':[]}
for location in locations:
counter = 0
print("Scraping jobs from ", location)
df = pd.read_csv("_".join(keyword.split()+location.split(', '))+'.csv').iloc[:,1:]
df = df[~df.jids.duplicated()]
for urlid in df.jids:
counter += 1
jurl = 'http://www.glassdoor.com/job-listing/?jl='+str(urlid)
res = parse_url(jurl)
jobdict['position'].append(res[0])
jobdict['desc'].append(res[1])
jobdict['salary'].append(res[2])
jobdict['jobloc'].append(res[3])
jobdict['company'].append(res[4])
jobdict['rating'].append(res[5])
if counter%100 == 0:
print(counter, '/', len(df.jids))
pd.DataFrame(jobdict).to_csv('glassdoor_data_science_jobs.csv')
###Output
_____no_output_____ |
notebooks/02-create-a-pipeline.ipynb | ###Markdown
Create a Pipeline
###Code
import os
import boto3
from botocore.client import Config
import kfp
from kfp import dsl
from kfp.dsl.types import Float, Integer, String
###Output
_____no_output_____
###Markdown
Upload the 'getting-started' notebook to a bucket
###Code
s3 = boto3.client(
"s3",
endpoint_url="http://minio-service.kubeflow:9000",
aws_access_key_id="minio",
aws_secret_access_key="minio123",
config=Config(signature_version="s3v4"),
region_name="us-east-1"
)
filename = "01-getting-started.ipynb"
with open(filename, "rb") as fp:
s3.upload_fileobj(fp, "mlpipeline", filename)
###Output
_____no_output_____
###Markdown
Create a pipeline that runs our notebook
###Code
@dsl.pipeline(
name="train_pipeline",
description="""A pipeline that runs jupyter notebooks using Papermill.""",
)
def train(
epochs: Integer = 10,
dropout: Float = 0.0,
weight_decay: Float = 0.0,
n_chunks: Float = 700,
learning_rate: Float = 0.03,
optimizer: String = "SGD"
):
notebook_path = "s3://mlpipeline/01-getting-started.ipynb"
output_path = "-"
dsl.ContainerOp(
name="Train",
image="gcr.io/kubeflow-aifest-2019/jupyter",
container_kwargs={
"image_pull_policy": "IfNotPresent",
"resources": {
"limits": {
"cpu": "1.0",
"memory": "5Gi"
},
"requests": {
"cpu": "0.1",
"memory": "1Gi"
}
}
},
command=[
"papermill", notebook_path, output_path,
"--report-mode",
"-p", "epochs", epochs,
"-p", "dropout", dropout,
"-p", "weight_decay", weight_decay,
"-p", "n_chunks", n_chunks,
"-p", "learning_rate", learning_rate,
"-p", "optimizer", optimizer,
],
file_outputs={
"mlpipeline-ui-metadata": "/mlpipeline-ui-metadata.json",
"mlpipeline-metrics": "/mlpipeline-metrics.json"
}
)
kfp.compiler.Compiler().compile(train, "training.zip")
from IPython.core.display import display, HTML
display(HTML('<a href="/notebook/anonymous/my-notebook-server/files/kubeflow-aiops-workshop/notebooks/training.zip?download=1">Download</a>'))
###Output
_____no_output_____ |
lessons/ML_pipelines/feature_union_practice.ipynb | ###Markdown
Implementing Feature UnionUsing the given custom transformer, `StartingVerbExtractor`, add a feature union to your pipeline to incorporate a feature that indicates with a boolean value whether the starting token of a post is identified as a verb.
###Code
import nltk
nltk.download(['punkt', 'wordnet', 'averaged_perceptron_tagger'])
import re
import numpy as np
import pandas as pd
from nltk.tokenize import word_tokenize
from nltk.stem import WordNetLemmatizer
from sklearn.pipeline import Pipeline, FeatureUnion
from sklearn.metrics import confusion_matrix
from sklearn.ensemble import RandomForestClassifier
from sklearn.model_selection import train_test_split
from sklearn.feature_extraction.text import CountVectorizer, TfidfTransformer
from custom_transformer import StartingVerbExtractor
url_regex = 'http[s]?://(?:[a-zA-Z]|[0-9]|[$-_@.&+]|[!*\(\),]|(?:%[0-9a-fA-F][0-9a-fA-F]))+'
###Output
_____no_output_____
###Markdown
Build your pipeline to have this structure:- Pipeline - feature union - text pipeline - count vectorizer - TFIDF transformer - starting verb extractor - classifier
###Code
def model_pipeline():
pipeline = Pipeline([
('features', FeatureUnion([
('nlp_pipeline', Pipeline([
('vect', CountVectorizer(tokenizer=tokenize)),
('tfidf', TfidfTransformer())
])),
('txt_len', StartingVerbExtractor())
])),
('clf', RandomForestClassifier())
])
return pipeline
###Output
_____no_output_____
###Markdown
Run program to test
###Code
def load_data():
df = pd.read_csv('corporate_messaging.csv', encoding='latin-1')
df = df[(df["category:confidence"] == 1) & (df['category'] != 'Exclude')]
X = df.text.values
y = df.category.values
return X, y
def tokenize(text):
detected_urls = re.findall(url_regex, text)
for url in detected_urls:
text = text.replace(url, "urlplaceholder")
tokens = word_tokenize(text)
lemmatizer = WordNetLemmatizer()
clean_tokens = []
for tok in tokens:
clean_tok = lemmatizer.lemmatize(tok).lower().strip()
clean_tokens.append(clean_tok)
return clean_tokens
def display_results(y_test, y_pred):
labels = np.unique(y_pred)
confusion_mat = confusion_matrix(y_test, y_pred, labels=labels)
accuracy = (y_pred == y_test).mean()
print("Labels:", labels)
print("Confusion Matrix:\n", confusion_mat)
print("Accuracy:", accuracy)
def main():
X, y = load_data()
X_train, X_test, y_train, y_test = train_test_split(X, y)
model = model_pipeline()
model.fit(X_train, y_train)
y_pred = model.predict(X_test)
display_results(y_test, y_pred)
main()
###Output
Labels: ['Action' 'Dialogue' 'Information']
Confusion Matrix:
[[ 79 0 16]
[ 1 18 4]
[ 20 0 463]]
Accuracy: 0.9317803660565723
|
fuzzy/reproduce_optimisation_progress_plot.ipynb | ###Markdown
Optimisation Progress Graphs
###Code
import numpy as np
import pandas as pd
import matplotlib.pyplot as plt
import os
import pickle
EXP_NUMBER = 1
SAVE_PLOT = False
allprogress = pd.read_csv("results/exp" + str(EXP_NUMBER) + "/optimisation_progress.csv")
evals = np.asarray(range(1, 101)) * 1000
if EXP_NUMBER == 0:
START = 0
plt.title("Optimisation convergence (hyperbolic)")
elif EXP_NUMBER == 1:
START = 3
plt.title("Optimisation convergence (exponential)")
elif EXP_NUMBER == 2:
START = 3
plt.title("Optimisation Convergence (nonlinear)")
plt.xlabel("Function Evaluations")
plt.ylabel("$\lambda$")
plt.ticklabel_format(useMathText=True)
plt.grid('major')
if EXP_NUMBER == 0:
plt.plot(evals, np.asarray(allprogress)[0])
else:
for i in range(0, 100):
plt.plot(evals, np.asarray(allprogress.iloc[i][START:]), '-', linewidth = 0.5)
if SAVE_PLOT:
plt.savefig("results/exp" + str(EXP_NUMBER) + "/optimisation_progress.png", dpi = 900)
###Output
_____no_output_____ |
FinRL_single_stock_trading_v2.ipynb | ###Markdown
Deep Reinforcement Learning for Stock Trading from Scratch: Single Stock TradingTutorials to use OpenAI DRL to trade single stock in one Jupyter Notebook | Presented at NeurIPS 2020: Deep RL Workshop* This blog is based on our paper: FinRL: A Deep Reinforcement Learning Library for Automated Stock Trading in Quantitative Finance, presented at NeurIPS 2020: Deep RL Workshop.* Check out medium blog for detailed explanations: https://towardsdatascience.com/finrl-for-quantitative-finance-tutorial-for-single-stock-trading-37d6d7c30aac* Please report any issues to our Github: https://github.com/AI4Finance-LLC/FinRL-Library/issues* **Pytorch Version** Content * [1. Problem Definition](0)* [2. Getting Started - Load Python packages](1) * [2.1. Install Packages](1.1) * [2.2. Check Additional Packages](1.2) * [2.3. Import Packages](1.3) * [2.4. Create Folders](1.4)* [3. Download Data](2)* [4. Preprocess Data](3) * [4.1. Technical Indicators](3.1) * [4.2. Perform Feature Engineering](3.2)* [5.Build Environment](4) * [5.1. Training & Trade Data Split](4.1) * [5.2. User-defined Environment](4.2) * [5.3. Initialize Environment](4.3) * [6.Implement DRL Algorithms](5) * [7.Backtesting Performance](6) * [7.1. BackTestStats](6.1) * [7.2. BackTestPlot](6.2) * [7.3. Baseline Stats](6.3) * [7.3. Compare to Stock Market Index](6.4) Part 1. Problem Definition This problem is to design an automated trading solution for single stock trading. We model the stock trading process as a Markov Decision Process (MDP). We then formulate our trading goal as a maximization problem.The components of the reinforcement learning environment are:* Action: The action space describes the allowed actions that the agent interacts with theenvironment. Normally, a ∈ A includes three actions: a ∈ {−1, 0, 1}, where −1, 0, 1 representselling, holding, and buying one stock. Also, an action can be carried upon multiple shares. We usean action space {−k, ..., −1, 0, 1, ..., k}, where k denotes the number of shares. For example, "Buy10 shares of AAPL" or "Sell 10 shares of AAPL" are 10 or −10, respectively* Reward function: r(s, a, s′) is the incentive mechanism for an agent to learn a better action. The change of the portfolio value when action a is taken at state s and arriving at new state s', i.e., r(s, a, s′) = v′ − v, where v′ and v represent the portfoliovalues at state s′ and s, respectively* State: The state space describes the observations that the agent receives from the environment. Just as a human trader needs to analyze various information before executing a trade, soour trading agent observes many different features to better learn in an interactive environment.* Environment: single stock trading for AAPLThe data of the single stock that we will be using for this case study is obtained from Yahoo Finance API. The data contains Open-High-Low-Close price and volume.We use Apple Inc. stock: AAPL as an example throughout this article, because it is one of the most popular and profitable stocks. Part 2. Getting Started- Load Python Packages 2.1. Install all the packages through FinRL library
###Code
## install finrl library
!pip install git+https://github.com/AI4Finance-LLC/FinRL-Library.git
###Output
Collecting git+https://github.com/AI4Finance-LLC/FinRL-Library.git
Cloning https://github.com/AI4Finance-LLC/FinRL-Library.git to c:\users\e0690420\appdata\local\temp\pip-req-build-529m07xy
Requirement already satisfied: numpy in c:\users\e0690420\anaconda3\lib\site-packages (from finrl==0.0.3) (1.19.2)
Collecting pandas>=1.1.5
Downloading pandas-1.2.1-cp38-cp38-win_amd64.whl (9.3 MB)
Collecting stockstats
Using cached stockstats-0.3.2-py2.py3-none-any.whl (13 kB)
Collecting yfinance
Using cached yfinance-0.1.55.tar.gz (23 kB)
Requirement already satisfied: matplotlib in c:\users\e0690420\anaconda3\lib\site-packages (from finrl==0.0.3) (3.3.2)
Requirement already satisfied: scikit-learn>=0.21.0 in c:\users\e0690420\anaconda3\lib\site-packages (from finrl==0.0.3) (0.23.2)
Collecting gym>=0.17
Downloading gym-0.18.0.tar.gz (1.6 MB)
Collecting stable-baselines3[extra]
Using cached stable_baselines3-0.10.0-py3-none-any.whl (145 kB)
Requirement already satisfied: pytest in c:\users\e0690420\anaconda3\lib\site-packages (from finrl==0.0.3) (0.0.0)
Requirement already satisfied: setuptools>=41.4.0 in c:\users\e0690420\anaconda3\lib\site-packages (from finrl==0.0.3) (50.3.1.post20201107)
Requirement already satisfied: wheel>=0.33.6 in c:\users\e0690420\anaconda3\lib\site-packages (from finrl==0.0.3) (0.35.1)
Collecting pyfolio@ git+https://github.com/quantopian/pyfolio.git#egg=pyfolio-0.9.2
Cloning https://github.com/quantopian/pyfolio.git to c:\users\e0690420\appdata\local\temp\pip-install-oyepb8k_\pyfolio
Requirement already satisfied: python-dateutil>=2.7.3 in c:\users\e0690420\anaconda3\lib\site-packages (from pandas>=1.1.5->finrl==0.0.3) (2.8.1)
Requirement already satisfied: pytz>=2017.3 in c:\users\e0690420\anaconda3\lib\site-packages (from pandas>=1.1.5->finrl==0.0.3) (2020.1)
Collecting int-date>=0.1.7
Using cached int_date-0.1.8-py2.py3-none-any.whl (5.0 kB)
Requirement already satisfied: requests>=2.20 in c:\users\e0690420\anaconda3\lib\site-packages (from yfinance->finrl==0.0.3) (2.24.0)
Collecting multitasking>=0.0.7
Using cached multitasking-0.0.9.tar.gz (8.1 kB)
Requirement already satisfied: lxml>=4.5.1 in c:\users\e0690420\anaconda3\lib\site-packages (from yfinance->finrl==0.0.3) (4.6.1)
Requirement already satisfied: cycler>=0.10 in c:\users\e0690420\anaconda3\lib\site-packages (from matplotlib->finrl==0.0.3) (0.10.0)
Requirement already satisfied: pyparsing!=2.0.4,!=2.1.2,!=2.1.6,>=2.0.3 in c:\users\e0690420\anaconda3\lib\site-packages (from matplotlib->finrl==0.0.3) (2.4.7)
Requirement already satisfied: pillow>=6.2.0 in c:\users\e0690420\anaconda3\lib\site-packages (from matplotlib->finrl==0.0.3) (8.0.1)
Requirement already satisfied: kiwisolver>=1.0.1 in c:\users\e0690420\anaconda3\lib\site-packages (from matplotlib->finrl==0.0.3) (1.3.0)
Requirement already satisfied: certifi>=2020.06.20 in c:\users\e0690420\anaconda3\lib\site-packages (from matplotlib->finrl==0.0.3) (2020.6.20)
Requirement already satisfied: scipy>=0.19.1 in c:\users\e0690420\anaconda3\lib\site-packages (from scikit-learn>=0.21.0->finrl==0.0.3) (1.5.2)
Requirement already satisfied: joblib>=0.11 in c:\users\e0690420\anaconda3\lib\site-packages (from scikit-learn>=0.21.0->finrl==0.0.3) (0.17.0)
Requirement already satisfied: threadpoolctl>=2.0.0 in c:\users\e0690420\anaconda3\lib\site-packages (from scikit-learn>=0.21.0->finrl==0.0.3) (2.1.0)
Collecting pyglet<=1.5.0,>=1.4.0
Downloading pyglet-1.5.0-py2.py3-none-any.whl (1.0 MB)
Requirement already satisfied: cloudpickle<1.7.0,>=1.2.0 in c:\users\e0690420\anaconda3\lib\site-packages (from gym>=0.17->finrl==0.0.3) (1.6.0)
Collecting torch>=1.4.0
Downloading torch-1.7.1-cp38-cp38-win_amd64.whl (184.0 MB)
Collecting tensorboard; extra == "extra"
Using cached tensorboard-2.4.1-py3-none-any.whl (10.6 MB)
Collecting opencv-python; extra == "extra"
Downloading opencv_python-4.5.1.48-cp38-cp38-win_amd64.whl (34.9 MB)
Collecting atari-py~=0.2.0; extra == "extra"
Downloading atari-py-0.2.6.tar.gz (790 kB)
Requirement already satisfied: psutil; extra == "extra" in c:\users\e0690420\anaconda3\lib\site-packages (from stable-baselines3[extra]->finrl==0.0.3) (5.7.2)
Requirement already satisfied: attrs>=17.4.0 in c:\users\e0690420\anaconda3\lib\site-packages (from pytest->finrl==0.0.3) (20.3.0)
Requirement already satisfied: iniconfig in c:\users\e0690420\anaconda3\lib\site-packages (from pytest->finrl==0.0.3) (1.1.1)
Requirement already satisfied: packaging in c:\users\e0690420\anaconda3\lib\site-packages (from pytest->finrl==0.0.3) (20.4)
Requirement already satisfied: pluggy<1.0,>=0.12 in c:\users\e0690420\anaconda3\lib\site-packages (from pytest->finrl==0.0.3) (0.13.1)
Requirement already satisfied: py>=1.8.2 in c:\users\e0690420\anaconda3\lib\site-packages (from pytest->finrl==0.0.3) (1.9.0)
Requirement already satisfied: toml in c:\users\e0690420\anaconda3\lib\site-packages (from pytest->finrl==0.0.3) (0.10.1)
Requirement already satisfied: atomicwrites>=1.0 in c:\users\e0690420\anaconda3\lib\site-packages (from pytest->finrl==0.0.3) (1.4.0)
Requirement already satisfied: colorama in c:\users\e0690420\anaconda3\lib\site-packages (from pytest->finrl==0.0.3) (0.4.4)
Requirement already satisfied: ipython>=3.2.3 in c:\users\e0690420\anaconda3\lib\site-packages (from pyfolio@ git+https://github.com/quantopian/pyfolio.git#egg=pyfolio-0.9.2->finrl==0.0.3) (7.19.0)
Requirement already satisfied: seaborn>=0.7.1 in c:\users\e0690420\anaconda3\lib\site-packages (from pyfolio@ git+https://github.com/quantopian/pyfolio.git#egg=pyfolio-0.9.2->finrl==0.0.3) (0.11.0)
Collecting empyrical>=0.5.0
Using cached empyrical-0.5.5.tar.gz (52 kB)
Requirement already satisfied: six>=1.5 in c:\users\e0690420\anaconda3\lib\site-packages (from python-dateutil>=2.7.3->pandas>=1.1.5->finrl==0.0.3) (1.15.0)
Requirement already satisfied: urllib3!=1.25.0,!=1.25.1,<1.26,>=1.21.1 in c:\users\e0690420\anaconda3\lib\site-packages (from requests>=2.20->yfinance->finrl==0.0.3) (1.25.11)
Requirement already satisfied: chardet<4,>=3.0.2 in c:\users\e0690420\anaconda3\lib\site-packages (from requests>=2.20->yfinance->finrl==0.0.3) (3.0.4)
Requirement already satisfied: idna<3,>=2.5 in c:\users\e0690420\anaconda3\lib\site-packages (from requests>=2.20->yfinance->finrl==0.0.3) (2.10)
Requirement already satisfied: future in c:\users\e0690420\anaconda3\lib\site-packages (from pyglet<=1.5.0,>=1.4.0->gym>=0.17->finrl==0.0.3) (0.18.2)
Requirement already satisfied: typing-extensions in c:\users\e0690420\anaconda3\lib\site-packages (from torch>=1.4.0->stable-baselines3[extra]->finrl==0.0.3) (3.7.4.3)
Collecting tensorboard-plugin-wit>=1.6.0
Using cached tensorboard_plugin_wit-1.8.0-py3-none-any.whl (781 kB)
Collecting google-auth-oauthlib<0.5,>=0.4.1
Using cached google_auth_oauthlib-0.4.2-py2.py3-none-any.whl (18 kB)
Collecting grpcio>=1.24.3
Downloading grpcio-1.35.0-cp38-cp38-win_amd64.whl (3.0 MB)
Requirement already satisfied: werkzeug>=0.11.15 in c:\users\e0690420\anaconda3\lib\site-packages (from tensorboard; extra == "extra"->stable-baselines3[extra]->finrl==0.0.3) (1.0.1)
Collecting google-auth<2,>=1.6.3
Using cached google_auth-1.24.0-py2.py3-none-any.whl (114 kB)
Collecting markdown>=2.6.8
Using cached Markdown-3.3.3-py3-none-any.whl (96 kB)
Collecting absl-py>=0.4
Using cached absl_py-0.11.0-py3-none-any.whl (127 kB)
Collecting protobuf>=3.6.0
Downloading protobuf-3.14.0-py2.py3-none-any.whl (173 kB)
Requirement already satisfied: pygments in c:\users\e0690420\anaconda3\lib\site-packages (from ipython>=3.2.3->pyfolio@ git+https://github.com/quantopian/pyfolio.git#egg=pyfolio-0.9.2->finrl==0.0.3) (2.7.2)
Requirement already satisfied: decorator in c:\users\e0690420\anaconda3\lib\site-packages (from ipython>=3.2.3->pyfolio@ git+https://github.com/quantopian/pyfolio.git#egg=pyfolio-0.9.2->finrl==0.0.3) (4.4.2)
Requirement already satisfied: prompt-toolkit!=3.0.0,!=3.0.1,<3.1.0,>=2.0.0 in c:\users\e0690420\anaconda3\lib\site-packages (from ipython>=3.2.3->pyfolio@ git+https://github.com/quantopian/pyfolio.git#egg=pyfolio-0.9.2->finrl==0.0.3) (3.0.8)
Requirement already satisfied: pickleshare in c:\users\e0690420\anaconda3\lib\site-packages (from ipython>=3.2.3->pyfolio@ git+https://github.com/quantopian/pyfolio.git#egg=pyfolio-0.9.2->finrl==0.0.3) (0.7.5)
Requirement already satisfied: jedi>=0.10 in c:\users\e0690420\anaconda3\lib\site-packages (from ipython>=3.2.3->pyfolio@ git+https://github.com/quantopian/pyfolio.git#egg=pyfolio-0.9.2->finrl==0.0.3) (0.17.1)
Requirement already satisfied: traitlets>=4.2 in c:\users\e0690420\anaconda3\lib\site-packages (from ipython>=3.2.3->pyfolio@ git+https://github.com/quantopian/pyfolio.git#egg=pyfolio-0.9.2->finrl==0.0.3) (5.0.5)
Requirement already satisfied: backcall in c:\users\e0690420\anaconda3\lib\site-packages (from ipython>=3.2.3->pyfolio@ git+https://github.com/quantopian/pyfolio.git#egg=pyfolio-0.9.2->finrl==0.0.3) (0.2.0)
Collecting pandas-datareader>=0.2
Using cached pandas_datareader-0.9.0-py3-none-any.whl (107 kB)
Collecting requests-oauthlib>=0.7.0
Using cached requests_oauthlib-1.3.0-py2.py3-none-any.whl (23 kB)
Collecting cachetools<5.0,>=2.0.0
Using cached cachetools-4.2.1-py3-none-any.whl (12 kB)
Collecting pyasn1-modules>=0.2.1
Using cached pyasn1_modules-0.2.8-py2.py3-none-any.whl (155 kB)
Collecting rsa<5,>=3.1.4; python_version >= "3.6"
Using cached rsa-4.7-py3-none-any.whl (34 kB)
Requirement already satisfied: wcwidth in c:\users\e0690420\anaconda3\lib\site-packages (from prompt-toolkit!=3.0.0,!=3.0.1,<3.1.0,>=2.0.0->ipython>=3.2.3->pyfolio@ git+https://github.com/quantopian/pyfolio.git#egg=pyfolio-0.9.2->finrl==0.0.3) (0.2.5)
Requirement already satisfied: parso<0.8.0,>=0.7.0 in c:\users\e0690420\anaconda3\lib\site-packages (from jedi>=0.10->ipython>=3.2.3->pyfolio@ git+https://github.com/quantopian/pyfolio.git#egg=pyfolio-0.9.2->finrl==0.0.3) (0.7.0)
Requirement already satisfied: ipython-genutils in c:\users\e0690420\anaconda3\lib\site-packages (from traitlets>=4.2->ipython>=3.2.3->pyfolio@ git+https://github.com/quantopian/pyfolio.git#egg=pyfolio-0.9.2->finrl==0.0.3) (0.2.0)
Collecting oauthlib>=3.0.0
Using cached oauthlib-3.1.0-py2.py3-none-any.whl (147 kB)
Collecting pyasn1<0.5.0,>=0.4.6
Using cached pyasn1-0.4.8-py2.py3-none-any.whl (77 kB)
Building wheels for collected packages: finrl, yfinance, gym, pyfolio, multitasking, atari-py, empyrical
Building wheel for finrl (setup.py): started
Building wheel for finrl (setup.py): finished with status 'done'
Created wheel for finrl: filename=finrl-0.0.3-py3-none-any.whl size=28505 sha256=a1f0921af68f03b82a1dfc77cf35bbc412906027b8a9088353e1f273811bdfef
Stored in directory: C:\Users\e0690420\AppData\Local\Temp\pip-ephem-wheel-cache-ycg13jjv\wheels\e8\19\74\11261997d6bdca44ba73e8eeedb94a3e3d340259516a0887eb
Building wheel for yfinance (setup.py): started
Building wheel for yfinance (setup.py): finished with status 'done'
Created wheel for yfinance: filename=yfinance-0.1.55-py2.py3-none-any.whl size=22622 sha256=d7e57a3b66f5161955a9fb6196b7a1331ccffa10e390e560859dce1bf916545a
Stored in directory: c:\users\e0690420\appdata\local\pip\cache\wheels\b4\c3\39\9c01ae2b4726f37024bba5592bec868b47a2fab5a786e8979a
Building wheel for gym (setup.py): started
Building wheel for gym (setup.py): finished with status 'done'
Created wheel for gym: filename=gym-0.18.0-py3-none-any.whl size=1656454 sha256=7227e6265047163058de2791052f49253fe4489901aa38b9bd822c3e9004c5d8
Stored in directory: c:\users\e0690420\appdata\local\pip\cache\wheels\d8\e7\68\a3f0f1b5831c9321d7523f6fd4e0d3f83f2705a1cbd5daaa79
Building wheel for pyfolio (setup.py): started
Building wheel for pyfolio (setup.py): finished with status 'done'
Created wheel for pyfolio: filename=pyfolio-0.9.2+75.g4b901f6-py3-none-any.whl size=76264 sha256=c3ffd084f6306a11b61d4260f3c325df1c974365219f74fabb43b3c4c35cec28
Stored in directory: C:\Users\e0690420\AppData\Local\Temp\pip-ephem-wheel-cache-ycg13jjv\wheels\7b\59\8b\3c276a18b58c04a1fd0e1351e979fb5396f93fbde5b5438df1
Building wheel for multitasking (setup.py): started
Building wheel for multitasking (setup.py): finished with status 'done'
Created wheel for multitasking: filename=multitasking-0.0.9-py3-none-any.whl size=8372 sha256=acb9d0f32df873cceb6c083fd9b24b2ee5a89810d119d0eba4c612059226a20d
Stored in directory: c:\users\e0690420\appdata\local\pip\cache\wheels\57\6d\a3\a39b839cc75274d2acfb1c58bfead2f726c6577fe8c4723f13
Building wheel for atari-py (setup.py): started
Building wheel for atari-py (setup.py): finished with status 'done'
Created wheel for atari-py: filename=atari_py-0.2.6-cp38-cp38-win_amd64.whl size=1155041 sha256=fa067101ff8f6fba4d3385b088f2164e03cdf987e715a82ca1f16c02baea22cb
Stored in directory: c:\users\e0690420\appdata\local\pip\cache\wheels\7f\5e\27\2e90b9887063d82ee2f9f8b2f8db76bb2290aa281dc40449c8
Building wheel for empyrical (setup.py): started
Building wheel for empyrical (setup.py): finished with status 'done'
Created wheel for empyrical: filename=empyrical-0.5.5-py3-none-any.whl size=39778 sha256=13498c1bb7a1f7b632c733f0802d7d2c800bdfe51333d62e715a2d040e0f0979
Stored in directory: c:\users\e0690420\appdata\local\pip\cache\wheels\0d\68\bb\926065fb744e7d7cb67334cb1a9c696722abc8303e5dc9a8d0
Successfully built finrl yfinance gym pyfolio multitasking atari-py empyrical
Installing collected packages: pandas, int-date, stockstats, multitasking, yfinance, pyglet, gym, torch, tensorboard-plugin-wit, oauthlib, requests-oauthlib, cachetools, pyasn1, pyasn1-modules, rsa, google-auth, google-auth-oauthlib, grpcio, markdown, absl-py, protobuf, tensorboard, opencv-python, atari-py, stable-baselines3, pandas-datareader, empyrical, pyfolio, finrl
Attempting uninstall: pandas
Found existing installation: pandas 1.1.3
Uninstalling pandas-1.1.3:
Successfully uninstalled pandas-1.1.3
ERROR: Could not install packages due to an EnvironmentError: [WinError 5] Access is denied: 'C:\\Users\\e0690420\\Anaconda3\\Lib\\site-packages\\~andas\\_libs\\algos.cp38-win_amd64.pyd'
Consider using the `--user` option or check the permissions.
###Markdown
2.2. Check if the additional packages needed are present, if not install them. * Yahoo Finance API* pandas* numpy* matplotlib* stockstats* OpenAI gym* stable-baselines* tensorflow* pyfolio 2.3. Import Packages
###Code
!conda install pandas
import pandas as pd
import numpy as np
import matplotlib
import matplotlib.pyplot as plt
matplotlib.use('Agg')
import datetime
from finrl.config import config
from finrl.marketdata.yahoodownloader import YahooDownloader
from finrl.preprocessing.preprocessors import FeatureEngineer
from finrl.preprocessing.data import data_split
from finrl.env.env_stocktrading import StockTradingEnv
from finrl.model.models import DRLAgent
from finrl.trade.backtest import BackTestStats, BaselineStats, BackTestPlot
import sys
sys.path.append("../FinRL-Library")
#Diable the warnings
import warnings
warnings.filterwarnings('ignore')
###Output
_____no_output_____
###Markdown
2.4. Create Folders
###Code
import os
if not os.path.exists("./" + config.DATA_SAVE_DIR):
os.makedirs("./" + config.DATA_SAVE_DIR)
if not os.path.exists("./" + config.TRAINED_MODEL_DIR):
os.makedirs("./" + config.TRAINED_MODEL_DIR)
if not os.path.exists("./" + config.TENSORBOARD_LOG_DIR):
os.makedirs("./" + config.TENSORBOARD_LOG_DIR)
if not os.path.exists("./" + config.RESULTS_DIR):
os.makedirs("./" + config.RESULTS_DIR)
###Output
_____no_output_____
###Markdown
Part 3. Download DataYahoo Finance is a website that provides stock data, financial news, financial reports, etc. All the data provided by Yahoo Finance is free.* FinRL uses a class **YahooDownloader** to fetch data from Yahoo Finance API* Call Limit: Using the Public API (without authentication), you are limited to 2,000 requests per hour per IP (or up to a total of 48,000 requests a day). -----class YahooDownloader: Provides methods for retrieving daily stock data from Yahoo Finance API Attributes ---------- start_date : str start date of the data (modified from config.py) end_date : str end date of the data (modified from config.py) ticker_list : list a list of stock tickers (modified from config.py) Methods ------- fetch_data() Fetches data from yahoo API
###Code
# from config.py start_date is a string
config.START_DATE
# from config.py end_date is a string
config.END_DATE
###Output
_____no_output_____
###Markdown
ticker_list is a list of stock tickers, in a single stock trading case, the list contains only 1 ticker
###Code
# Download and save the data in a pandas DataFrame:
data_df = YahooDownloader(start_date = '2009-01-01',
end_date = '2021-01-01',
ticker_list = ['AAPL']).fetch_data()
data_df.shape
data_df.head()
data_df=pd.read_csv(r'C:\Users\e0690420\rl_forex\finrl\preprocessing\datasets\chicago_pmi\EURUSD\ohlc\EURUSD_Chicago_Pmi_2018-01-31 - Copy.csv')
data_df.head()
data_df['time']
data_df.rename(columns = {"time": "date"},
inplace = True)
data_df.head()
data_df['date'].dtype
data_df.date = pd.to_datetime(data_df.date)
data_df['date'].dtype
data_df['day'] = data_df['date'].dt.dayofweek
data_df['tic'] = 'A'
data_df.head()
###Output
_____no_output_____
###Markdown
Part 4. Preprocess DataData preprocessing is a crucial step for training a high quality machine learning model. We need to check for missing data and do feature engineering in order to convert the data into a model-ready state.* FinRL uses a class **FeatureEngineer** to preprocess the data* Add **technical indicators**. In practical trading, various information needs to be taken into account, for example the historical stock prices, current holding shares, technical indicators, etc. class FeatureEngineer:Provides methods for preprocessing the stock price data Attributes ---------- df: DataFrame data downloaded from Yahoo API feature_number : int number of features we used use_technical_indicator : boolean we technical indicator or not use_turbulence : boolean use turbulence index or not Methods ------- preprocess_data() main method to do the feature engineering 4.1 Technical Indicators* FinRL uses stockstats to calcualte technical indicators such as **Moving Average Convergence Divergence (MACD)**, **Relative Strength Index (RSI)**, **Average Directional Index (ADX)**, **Commodity Channel Index (CCI)** and other various indicators and stats.* **stockstats**: supplies a wrapper StockDataFrame based on the **pandas.DataFrame** with inline stock statistics/indicators support.
###Code
## we store the stockstats technical indicator column names in config.py
tech_indicator_list=config.TECHNICAL_INDICATORS_LIST
print(tech_indicator_list)
## user can add more technical indicators
## check https://github.com/jealous/stockstats for different names
tech_indicator_list=tech_indicator_list+['kdjk','open_2_sma','boll','close_10.0_le_5_c','wr_10','dma','trix']
print(tech_indicator_list)
###Output
['macd', 'boll_ub', 'boll_lb', 'rsi_30', 'cci_30', 'dx_30', 'close_30_sma', 'close_60_sma', 'kdjk', 'open_2_sma', 'boll', 'close_10.0_le_5_c', 'wr_10', 'dma', 'trix']
###Markdown
4.2 Perform Feature Engineering
###Code
fe = FeatureEngineer(
use_technical_indicator=True,
tech_indicator_list = tech_indicator_list,
use_turbulence=False,
user_defined_feature = False)
data_df = fe.preprocess_data(data_df)
data_df.head()
###Output
_____no_output_____
###Markdown
Part 5. Build EnvironmentConsidering the stochastic and interactive nature of the automated stock trading tasks, a financial task is modeled as a **Markov Decision Process (MDP)** problem. The training process involves observing stock price change, taking an action and reward's calculation to have the agent adjusting its strategy accordingly. By interacting with the environment, the trading agent will derive a trading strategy with the maximized rewards as time proceeds.Our trading environments, based on OpenAI Gym framework, simulate live stock markets with real market data according to the principle of time-driven simulation.The action space describes the allowed actions that the agent interacts with the environment. Normally, action a includes three actions: {-1, 0, 1}, where -1, 0, 1 represent selling, holding, and buying one share. Also, an action can be carried upon multiple shares. We use an action space {-k,…,-1, 0, 1, …, k}, where k denotes the number of shares to buy and -k denotes the number of shares to sell. For example, "Buy 10 shares of AAPL" or "Sell 10 shares of AAPL" are 10 or -10, respectively. The continuous action space needs to be normalized to [-1, 1], since the policy is defined on a Gaussian distribution, which needs to be normalized and symmetric. 5.1 Training & Trade data split* Training: 2009-01-01 to 2018-12-31* Trade: 2019-01-01 to 2020-09-30
###Code
#train = data_split(data_df, start = config.START_DATE, end = config.START_TRADE_DATE)
#trade = data_split(data_df, start = config.START_TRADE_DATE, end = config.END_DATE)
train = data_split(data_df, start = '2009-01-01', end = '2019-01-01')
trade = data_split(data_df, start = '2019-01-01', end = '2021-01-01')
data_df
train
trade
## data normalization, this part is optional, have little impact
#feaures_list = list(train.columns)
#feaures_list.remove('date')
#feaures_list.remove('tic')
#feaures_list.remove('close')
#print(feaures_list)
#from sklearn import preprocessing
#data_normaliser = preprocessing.StandardScaler()
#train[feaures_list] = data_normaliser.fit_transform(train[feaures_list])
#trade[feaures_list] = data_normaliser.transform(trade[feaures_list])
###Output
_____no_output_____
###Markdown
5.2 User-defined Environment: a simulation environment class 5.3 Initialize Environment* **stock dimension**: the number of unique stock tickers we use* **hmax**: the maximum amount of shares to buy or sell* **initial amount**: the amount of money we use to trade in the begining* **transaction cost percentage**: a per share rate for every share trade* **tech_indicator_list**: a list of technical indicator names (modified from config.py)
###Code
## we store the stockstats technical indicator column names in config.py
## check https://github.com/jealous/stockstats for different names
tech_indicator_list
# the stock dimension is 1, because we only use the price data of AAPL.
len(train.tic.unique())
stock_dimension = len(train.tic.unique())
state_space = 1 + 2*stock_dimension + len(config.TECHNICAL_INDICATORS_LIST)*stock_dimension
print(f"Stock Dimension: {stock_dimension}, State Space: {state_space}")
env_kwargs = {
"hmax": 100,
"initial_amount": 100000,
"transaction_cost_pct": 0.001,
"state_space": state_space,
"stock_dim": stock_dimension,
"tech_indicator_list": config.TECHNICAL_INDICATORS_LIST,
"action_space": stock_dimension,
"reward_scaling": 1e-4
}
e_train_gym = StockTradingEnv(df = train, **env_kwargs)
env_train, _ = e_train_gym.get_sb_env()
print(type(env_train))
###Output
<class 'stable_baselines3.common.vec_env.dummy_vec_env.DummyVecEnv'>
###Markdown
Part 6: Implement DRL Algorithms* The implementation of the DRL algorithms are based on **OpenAI Baselines** and **Stable Baselines**. Stable Baselines is a fork of OpenAI Baselines, with a major structural refactoring, and code cleanups.* FinRL library includes fine-tuned standard DRL algorithms, such as DQN, DDPG,Multi-Agent DDPG, PPO, SAC, A2C and TD3. We also allow users todesign their own DRL algorithms by adapting these DRL algorithms.
###Code
agent = DRLAgent(env = env_train)
###Output
_____no_output_____
###Markdown
Model Training: 5 models, A2C DDPG, PPO, TD3, SAC Model 1: A2C
###Code
agent = DRLAgent(env = env_train)
A2C_PARAMS = {"n_steps": 5, "ent_coef": 0.005, "learning_rate": 0.0001}
model_a2c = agent.get_model(model_name="a2c",model_kwargs = A2C_PARAMS)
trained_a2c = agent.train_model(model=model_a2c,
tb_log_name='a2c',
total_timesteps=50000)
###Output
Logging to tensorboard_log/a2c/a2c_3
day: 95, episode: 2860
begin_total_asset: 100000.00
end_total_asset: 99911.01
total_reward: -88.99
total_cost: 6.70
total_trades: 95
Sharpe: -2.510
=================================
------------------------------------
| environment/ | |
| portfolio_value | 9.99e+04 |
| total_cost | 6.73 |
| total_reward | -59.8 |
| total_reward_pct | -0.0598 |
| total_trades | 92 |
| time/ | |
| fps | 357 |
| iterations | 100 |
| time_elapsed | 1 |
| total_timesteps | 500 |
| train/ | |
| entropy_loss | -1.43 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| n_updates | 99 |
| policy_loss | 0.000149 |
| std | 1.01 |
| value_loss | 2.35e-08 |
------------------------------------
-------------------------------------
| environment/ | |
| portfolio_value | 9.99e+04 |
| total_cost | 6.94 |
| total_reward | -97.8 |
| total_reward_pct | -0.0978 |
| total_trades | 93 |
| time/ | |
| fps | 364 |
| iterations | 200 |
| time_elapsed | 2 |
| total_timesteps | 1000 |
| train/ | |
| entropy_loss | -1.44 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| n_updates | 199 |
| policy_loss | -0.000313 |
| std | 1.02 |
| value_loss | 2.42e-07 |
-------------------------------------
day: 95, episode: 2870
begin_total_asset: 100000.00
end_total_asset: 99961.39
total_reward: -38.61
total_cost: 6.50
total_trades: 93
Sharpe: -2.207
=================================
------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 6.58 |
| total_reward | -40.5 |
| total_reward_pct | -0.0405 |
| total_trades | 90 |
| time/ | |
| fps | 370 |
| iterations | 300 |
| time_elapsed | 4 |
| total_timesteps | 1500 |
| train/ | |
| entropy_loss | -1.45 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| n_updates | 299 |
| policy_loss | 2.43e-05 |
| std | 1.03 |
| value_loss | 1.33e-09 |
------------------------------------
-------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 5.74 |
| total_reward | -45.4 |
| total_reward_pct | -0.0454 |
| total_trades | 87 |
| time/ | |
| fps | 369 |
| iterations | 400 |
| time_elapsed | 5 |
| total_timesteps | 2000 |
| train/ | |
| entropy_loss | -1.46 |
| explained_variance | -6.21e+13 |
| learning_rate | 0.0001 |
| n_updates | 399 |
| policy_loss | -0.00292 |
| std | 1.04 |
| value_loss | 5.63e-06 |
-------------------------------------
day: 95, episode: 2880
begin_total_asset: 100000.00
end_total_asset: 99946.96
total_reward: -53.04
total_cost: 5.97
total_trades: 92
Sharpe: -3.082
=================================
-------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 6.43 |
| total_reward | -29.8 |
| total_reward_pct | -0.0298 |
| total_trades | 92 |
| time/ | |
| fps | 366 |
| iterations | 500 |
| time_elapsed | 6 |
| total_timesteps | 2500 |
| train/ | |
| entropy_loss | -1.47 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| n_updates | 499 |
| policy_loss | -0.000587 |
| std | 1.05 |
| value_loss | 3.21e-07 |
-------------------------------------
------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 6.63 |
| total_reward | -29.7 |
| total_reward_pct | -0.0297 |
| total_trades | 92 |
| time/ | |
| fps | 366 |
| iterations | 600 |
| time_elapsed | 8 |
| total_timesteps | 3000 |
| train/ | |
| entropy_loss | -1.48 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| n_updates | 599 |
| policy_loss | 0.00028 |
| std | 1.06 |
| value_loss | 3.24e-08 |
------------------------------------
day: 95, episode: 2890
begin_total_asset: 100000.00
end_total_asset: 99977.40
total_reward: -22.60
total_cost: 6.29
total_trades: 91
Sharpe: -3.665
=================================
-------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 4.77 |
| total_reward | -17.9 |
| total_reward_pct | -0.0179 |
| total_trades | 82 |
| time/ | |
| fps | 367 |
| iterations | 700 |
| time_elapsed | 9 |
| total_timesteps | 3500 |
| train/ | |
| entropy_loss | -1.49 |
| explained_variance | -4.65e+10 |
| learning_rate | 0.0001 |
| n_updates | 699 |
| policy_loss | 0.00123 |
| std | 1.07 |
| value_loss | 1.04e-06 |
-------------------------------------
-------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 5.27 |
| total_reward | -8.07 |
| total_reward_pct | -0.00807 |
| total_trades | 86 |
| time/ | |
| fps | 368 |
| iterations | 800 |
| time_elapsed | 10 |
| total_timesteps | 4000 |
| train/ | |
| entropy_loss | -1.5 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| n_updates | 799 |
| policy_loss | -0.000275 |
| std | 1.09 |
| value_loss | 5.62e-08 |
-------------------------------------
day: 95, episode: 2900
begin_total_asset: 100000.00
end_total_asset: 99987.09
total_reward: -12.91
total_cost: 3.70
total_trades: 72
Sharpe: -4.041
=================================
------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 5.17 |
| total_reward | -29.8 |
| total_reward_pct | -0.0298 |
| total_trades | 85 |
| time/ | |
| fps | 368 |
| iterations | 900 |
| time_elapsed | 12 |
| total_timesteps | 4500 |
| train/ | |
| entropy_loss | -1.51 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| n_updates | 899 |
| policy_loss | 0.000288 |
| std | 1.1 |
| value_loss | 5.38e-08 |
------------------------------------
-------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 5.5 |
| total_reward | -25.1 |
| total_reward_pct | -0.0251 |
| total_trades | 82 |
| time/ | |
| fps | 369 |
| iterations | 1000 |
| time_elapsed | 13 |
| total_timesteps | 5000 |
| train/ | |
| entropy_loss | -1.52 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| n_updates | 999 |
| policy_loss | -0.000252 |
| std | 1.11 |
| value_loss | 4.02e-08 |
-------------------------------------
day: 95, episode: 2910
begin_total_asset: 100000.00
end_total_asset: 99970.51
total_reward: -29.49
total_cost: 5.25
total_trades: 86
Sharpe: -3.009
=================================
-------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 4.97 |
| total_reward | -17 |
| total_reward_pct | -0.017 |
| total_trades | 79 |
| time/ | |
| fps | 369 |
| iterations | 1100 |
| time_elapsed | 14 |
| total_timesteps | 5500 |
| train/ | |
| entropy_loss | -1.53 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| n_updates | 1099 |
| policy_loss | -0.000646 |
| std | 1.12 |
| value_loss | 9.27e-08 |
-------------------------------------
-------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 5.96 |
| total_reward | -27.4 |
| total_reward_pct | -0.0274 |
| total_trades | 90 |
| time/ | |
| fps | 371 |
| iterations | 1200 |
| time_elapsed | 16 |
| total_timesteps | 6000 |
| train/ | |
| entropy_loss | -1.54 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| n_updates | 1199 |
| policy_loss | -8.55e-06 |
| std | 1.13 |
| value_loss | 1.77e-10 |
-------------------------------------
day: 95, episode: 2920
begin_total_asset: 100000.00
end_total_asset: 99979.74
total_reward: -20.26
total_cost: 5.83
total_trades: 88
Sharpe: -4.153
=================================
------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 5.94 |
| total_reward | -23.8 |
| total_reward_pct | -0.0238 |
| total_trades | 81 |
| time/ | |
| fps | 370 |
| iterations | 1300 |
| time_elapsed | 17 |
| total_timesteps | 6500 |
| train/ | |
| entropy_loss | -1.55 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| n_updates | 1299 |
| policy_loss | 2.48e-05 |
| std | 1.14 |
| value_loss | 6.53e-10 |
------------------------------------
------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 5.68 |
| total_reward | -28.1 |
| total_reward_pct | -0.0281 |
| total_trades | 79 |
| time/ | |
| fps | 370 |
| iterations | 1400 |
| time_elapsed | 18 |
| total_timesteps | 7000 |
| train/ | |
| entropy_loss | -1.56 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| n_updates | 1399 |
| policy_loss | 3.6e-05 |
| std | 1.15 |
| value_loss | 3e-09 |
------------------------------------
day: 95, episode: 2930
begin_total_asset: 100000.00
end_total_asset: 99972.05
total_reward: -27.95
total_cost: 5.42
total_trades: 83
Sharpe: -2.840
=================================
-------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 3.48 |
| total_reward | -8.68 |
| total_reward_pct | -0.00868 |
| total_trades | 72 |
| time/ | |
| fps | 371 |
| iterations | 1500 |
| time_elapsed | 20 |
| total_timesteps | 7500 |
| train/ | |
| entropy_loss | -1.57 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| n_updates | 1499 |
| policy_loss | -0.000981 |
| std | 1.16 |
| value_loss | 3.67e-07 |
-------------------------------------
-------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 4.31 |
| total_reward | -10.3 |
| total_reward_pct | -0.0103 |
| total_trades | 79 |
| time/ | |
| fps | 372 |
| iterations | 1600 |
| time_elapsed | 21 |
| total_timesteps | 8000 |
| train/ | |
| entropy_loss | -1.58 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| n_updates | 1599 |
| policy_loss | -7.38e-06 |
| std | 1.18 |
| value_loss | 8.13e-11 |
-------------------------------------
day: 95, episode: 2940
begin_total_asset: 100000.00
end_total_asset: 99989.70
total_reward: -10.30
total_cost: 2.56
total_trades: 65
Sharpe: -3.017
=================================
------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 3.67 |
| total_reward | -10.9 |
| total_reward_pct | -0.0109 |
| total_trades | 73 |
| time/ | |
| fps | 372 |
| iterations | 1700 |
| time_elapsed | 22 |
| total_timesteps | 8500 |
| train/ | |
| entropy_loss | -1.59 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| n_updates | 1699 |
| policy_loss | 4.23e-05 |
| std | 1.19 |
| value_loss | 4.55e-10 |
------------------------------------
------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 2.8 |
| total_reward | -1.71 |
| total_reward_pct | -0.00171 |
| total_trades | 61 |
| time/ | |
| fps | 373 |
| iterations | 1800 |
| time_elapsed | 24 |
| total_timesteps | 9000 |
| train/ | |
| entropy_loss | -1.6 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| n_updates | 1799 |
| policy_loss | 1.51e-05 |
| std | 1.2 |
| value_loss | 1.71e-10 |
------------------------------------
day: 95, episode: 2950
begin_total_asset: 100000.00
end_total_asset: 99994.89
total_reward: -5.11
total_cost: 2.94
total_trades: 57
Sharpe: -4.824
=================================
-------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 5.06 |
| total_reward | -34.5 |
| total_reward_pct | -0.0345 |
| total_trades | 87 |
| time/ | |
| fps | 373 |
| iterations | 1900 |
| time_elapsed | 25 |
| total_timesteps | 9500 |
| train/ | |
| entropy_loss | -1.61 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| n_updates | 1899 |
| policy_loss | -5.09e-05 |
| std | 1.21 |
| value_loss | 1.23e-09 |
-------------------------------------
day: 95, episode: 2960
begin_total_asset: 100000.00
end_total_asset: 99985.73
total_reward: -14.27
total_cost: 3.82
total_trades: 76
Sharpe: -2.973
=================================
-------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 3.82 |
| total_reward | -14.3 |
| total_reward_pct | -0.0143 |
| total_trades | 76 |
| time/ | |
| fps | 374 |
| iterations | 2000 |
| time_elapsed | 26 |
| total_timesteps | 10000 |
| train/ | |
| entropy_loss | -1.62 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| n_updates | 1999 |
| policy_loss | -0.000645 |
| std | 1.22 |
| value_loss | 1.37e-07 |
-------------------------------------
------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 5.04 |
| total_reward | -15.2 |
| total_reward_pct | -0.0152 |
| total_trades | 81 |
| time/ | |
| fps | 374 |
| iterations | 2100 |
| time_elapsed | 28 |
| total_timesteps | 10500 |
| train/ | |
| entropy_loss | -1.63 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| n_updates | 2099 |
| policy_loss | -6.1e-06 |
| std | 1.24 |
| value_loss | 3.29e-11 |
------------------------------------
day: 95, episode: 2970
begin_total_asset: 100000.00
end_total_asset: 99983.29
total_reward: -16.71
total_cost: 3.26
total_trades: 66
Sharpe: -2.418
=================================
-------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 3.26 |
| total_reward | -16.7 |
| total_reward_pct | -0.0167 |
| total_trades | 66 |
| time/ | |
| fps | 373 |
| iterations | 2200 |
| time_elapsed | 29 |
| total_timesteps | 11000 |
| train/ | |
| entropy_loss | -1.64 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| n_updates | 2199 |
| policy_loss | -3.06e-05 |
| std | 1.25 |
| value_loss | 7.7e-10 |
-------------------------------------
------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 3.19 |
| total_reward | -7.95 |
| total_reward_pct | -0.00795 |
| total_trades | 58 |
| time/ | |
| fps | 372 |
| iterations | 2300 |
| time_elapsed | 30 |
| total_timesteps | 11500 |
| train/ | |
| entropy_loss | -1.65 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| n_updates | 2299 |
| policy_loss | 4.26e-06 |
| std | 1.26 |
| value_loss | 7.7e-12 |
------------------------------------
day: 95, episode: 2980
begin_total_asset: 100000.00
end_total_asset: 99992.50
total_reward: -7.50
total_cost: 4.19
total_trades: 71
Sharpe: -3.679
=================================
------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 2.75 |
| total_reward | -4.62 |
| total_reward_pct | -0.00462 |
| total_trades | 63 |
| time/ | |
| fps | 370 |
| iterations | 2400 |
| time_elapsed | 32 |
| total_timesteps | 12000 |
| train/ | |
| entropy_loss | -1.66 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| n_updates | 2399 |
| policy_loss | 4.07e-05 |
| std | 1.27 |
| value_loss | 1.57e-09 |
------------------------------------
------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 2.45 |
| total_reward | -11 |
| total_reward_pct | -0.011 |
| total_trades | 57 |
| time/ | |
| fps | 369 |
| iterations | 2500 |
| time_elapsed | 33 |
| total_timesteps | 12500 |
| train/ | |
| entropy_loss | -1.67 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| n_updates | 2499 |
| policy_loss | 0.000277 |
| std | 1.29 |
| value_loss | 4.68e-08 |
------------------------------------
day: 95, episode: 2990
begin_total_asset: 100000.00
end_total_asset: 99993.60
total_reward: -6.40
total_cost: 3.60
total_trades: 66
Sharpe: -3.232
=================================
------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 4.78 |
| total_reward | -6.75 |
| total_reward_pct | -0.00675 |
| total_trades | 77 |
| time/ | |
| fps | 368 |
| iterations | 2600 |
| time_elapsed | 35 |
| total_timesteps | 13000 |
| train/ | |
| entropy_loss | -1.68 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| n_updates | 2599 |
| policy_loss | 2.01e-05 |
| std | 1.3 |
| value_loss | 1.28e-10 |
------------------------------------
-------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 2.22 |
| total_reward | -3.43 |
| total_reward_pct | -0.00343 |
| total_trades | 49 |
| time/ | |
| fps | 368 |
| iterations | 2700 |
| time_elapsed | 36 |
| total_timesteps | 13500 |
| train/ | |
| entropy_loss | -1.69 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| n_updates | 2699 |
| policy_loss | -1.09e-05 |
| std | 1.31 |
| value_loss | 6.95e-11 |
-------------------------------------
day: 95, episode: 3000
begin_total_asset: 100000.00
end_total_asset: 99989.03
total_reward: -10.97
total_cost: 3.21
total_trades: 60
Sharpe: -3.665
=================================
------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 1.85 |
| total_reward | -6.27 |
| total_reward_pct | -0.00627 |
| total_trades | 56 |
| time/ | |
| fps | 368 |
| iterations | 2800 |
| time_elapsed | 37 |
| total_timesteps | 14000 |
| train/ | |
| entropy_loss | -1.7 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| n_updates | 2799 |
| policy_loss | 1.47e-05 |
| std | 1.32 |
| value_loss | 1.26e-10 |
------------------------------------
------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 2.68 |
| total_reward | -2.83 |
| total_reward_pct | -0.00283 |
| total_trades | 58 |
| time/ | |
| fps | 368 |
| iterations | 2900 |
| time_elapsed | 39 |
| total_timesteps | 14500 |
| train/ | |
| entropy_loss | -1.71 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| n_updates | 2899 |
| policy_loss | 5.55e-06 |
| std | 1.34 |
| value_loss | 1.77e-11 |
------------------------------------
day: 95, episode: 3010
begin_total_asset: 100000.00
end_total_asset: 99998.60
total_reward: -1.40
total_cost: 3.20
total_trades: 69
Sharpe: -0.520
=================================
-------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 2.26 |
| total_reward | -4.62 |
| total_reward_pct | -0.00462 |
| total_trades | 56 |
| time/ | |
| fps | 368 |
| iterations | 3000 |
| time_elapsed | 40 |
| total_timesteps | 15000 |
| train/ | |
| entropy_loss | -1.72 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| n_updates | 2999 |
| policy_loss | -4.68e-05 |
| std | 1.35 |
| value_loss | 5.25e-10 |
-------------------------------------
------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 4.09 |
| total_reward | -1.06 |
| total_reward_pct | -0.00106 |
| total_trades | 68 |
| time/ | |
| fps | 368 |
| iterations | 3100 |
| time_elapsed | 42 |
| total_timesteps | 15500 |
| train/ | |
| entropy_loss | -1.73 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| n_updates | 3099 |
| policy_loss | 0.000135 |
| std | 1.36 |
| value_loss | 9.58e-09 |
------------------------------------
day: 95, episode: 3020
begin_total_asset: 100000.00
end_total_asset: 99982.01
total_reward: -17.99
total_cost: 5.01
total_trades: 78
Sharpe: -2.969
=================================
-------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 2.85 |
| total_reward | -6.82 |
| total_reward_pct | -0.00682 |
| total_trades | 57 |
| time/ | |
| fps | 369 |
| iterations | 3200 |
| time_elapsed | 43 |
| total_timesteps | 16000 |
| train/ | |
| entropy_loss | -1.74 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| n_updates | 3199 |
| policy_loss | -1.37e-05 |
| std | 1.38 |
| value_loss | 1.22e-10 |
-------------------------------------
------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 3.8 |
| total_reward | -4.96 |
| total_reward_pct | -0.00496 |
| total_trades | 74 |
| time/ | |
| fps | 369 |
| iterations | 3300 |
| time_elapsed | 44 |
| total_timesteps | 16500 |
| train/ | |
| entropy_loss | -1.75 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| n_updates | 3299 |
| policy_loss | 7.72e-06 |
| std | 1.39 |
| value_loss | 2.97e-11 |
------------------------------------
day: 95, episode: 3030
begin_total_asset: 100000.00
end_total_asset: 99990.50
total_reward: -9.50
total_cost: 2.28
total_trades: 46
Sharpe: -2.842
=================================
------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 3.53 |
| total_reward | -15.7 |
| total_reward_pct | -0.0157 |
| total_trades | 61 |
| time/ | |
| fps | 369 |
| iterations | 3400 |
| time_elapsed | 46 |
| total_timesteps | 17000 |
| train/ | |
| entropy_loss | -1.76 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| n_updates | 3399 |
| policy_loss | 0.0017 |
| std | 1.41 |
| value_loss | 3.66e-06 |
------------------------------------
-------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 2.4 |
| total_reward | -3.52 |
| total_reward_pct | -0.00352 |
| total_trades | 59 |
| time/ | |
| fps | 369 |
| iterations | 3500 |
| time_elapsed | 47 |
| total_timesteps | 17500 |
| train/ | |
| entropy_loss | -1.77 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| n_updates | 3499 |
| policy_loss | -0.000349 |
| std | 1.42 |
| value_loss | 4.78e-08 |
-------------------------------------
day: 95, episode: 3040
begin_total_asset: 100000.00
end_total_asset: 99996.12
total_reward: -3.88
total_cost: 2.58
total_trades: 52
Sharpe: -7.817
=================================
-------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 4.55 |
| total_reward | -8.04 |
| total_reward_pct | -0.00804 |
| total_trades | 66 |
| time/ | |
| fps | 370 |
| iterations | 3600 |
| time_elapsed | 48 |
| total_timesteps | 18000 |
| train/ | |
| entropy_loss | -1.78 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| n_updates | 3599 |
| policy_loss | -2.67e-05 |
| std | 1.43 |
| value_loss | 3.81e-10 |
-------------------------------------
------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 4.34 |
| total_reward | -8.59 |
| total_reward_pct | -0.00859 |
| total_trades | 67 |
| time/ | |
| fps | 370 |
| iterations | 3700 |
| time_elapsed | 49 |
| total_timesteps | 18500 |
| train/ | |
| entropy_loss | -1.79 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| n_updates | 3699 |
| policy_loss | 8.66e-05 |
| std | 1.45 |
| value_loss | 3.57e-09 |
------------------------------------
day: 95, episode: 3050
begin_total_asset: 100000.00
end_total_asset: 99990.53
total_reward: -9.47
total_cost: 2.48
total_trades: 54
Sharpe: -3.596
=================================
-------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 2.64 |
| total_reward | -10.7 |
| total_reward_pct | -0.0107 |
| total_trades | 56 |
| time/ | |
| fps | 370 |
| iterations | 3800 |
| time_elapsed | 51 |
| total_timesteps | 19000 |
| train/ | |
| entropy_loss | -1.8 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| n_updates | 3799 |
| policy_loss | -2.33e-05 |
| std | 1.46 |
| value_loss | 1.12e-09 |
-------------------------------------
-------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 3.72 |
| total_reward | -10.4 |
| total_reward_pct | -0.0104 |
| total_trades | 61 |
| time/ | |
| fps | 369 |
| iterations | 3900 |
| time_elapsed | 52 |
| total_timesteps | 19500 |
| train/ | |
| entropy_loss | -1.81 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| n_updates | 3899 |
| policy_loss | -0.000376 |
| std | 1.48 |
| value_loss | 6.29e-08 |
-------------------------------------
day: 95, episode: 3060
begin_total_asset: 100000.00
end_total_asset: 99995.54
total_reward: -4.46
total_cost: 2.47
total_trades: 53
Sharpe: -3.134
=================================
-------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 2.7 |
| total_reward | -2.08 |
| total_reward_pct | -0.00208 |
| total_trades | 59 |
| time/ | |
| fps | 369 |
| iterations | 4000 |
| time_elapsed | 54 |
| total_timesteps | 20000 |
| train/ | |
| entropy_loss | -1.82 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| n_updates | 3999 |
| policy_loss | -0.000203 |
| std | 1.49 |
| value_loss | 2.17e-08 |
-------------------------------------
-------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 4.11 |
| total_reward | -15.5 |
| total_reward_pct | -0.0155 |
| total_trades | 68 |
| time/ | |
| fps | 369 |
| iterations | 4100 |
| time_elapsed | 55 |
| total_timesteps | 20500 |
| train/ | |
| entropy_loss | -1.83 |
| explained_variance | -2.57e+10 |
| learning_rate | 0.0001 |
| n_updates | 4099 |
| policy_loss | 9.12e-05 |
| std | 1.51 |
| value_loss | 1.97e-09 |
-------------------------------------
day: 95, episode: 3070
begin_total_asset: 100000.00
end_total_asset: 99988.69
total_reward: -11.31
total_cost: 2.46
total_trades: 52
Sharpe: -3.747
=================================
-------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 3.63 |
| total_reward | -15.8 |
| total_reward_pct | -0.0158 |
| total_trades | 63 |
| time/ | |
| fps | 370 |
| iterations | 4200 |
| time_elapsed | 56 |
| total_timesteps | 21000 |
| train/ | |
| entropy_loss | -1.84 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| n_updates | 4199 |
| policy_loss | -7.92e-06 |
| std | 1.52 |
| value_loss | 7.95e-11 |
-------------------------------------
------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 2.43 |
| total_reward | -9.19 |
| total_reward_pct | -0.00919 |
| total_trades | 54 |
| time/ | |
| fps | 370 |
| iterations | 4300 |
| time_elapsed | 58 |
| total_timesteps | 21500 |
| train/ | |
| entropy_loss | -1.85 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| n_updates | 4299 |
| policy_loss | 1.49e-05 |
| std | 1.54 |
| value_loss | 1.14e-10 |
------------------------------------
day: 95, episode: 3080
begin_total_asset: 100000.00
end_total_asset: 99987.17
total_reward: -12.83
total_cost: 2.69
total_trades: 57
Sharpe: -3.356
=================================
------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 2.11 |
| total_reward | -4.59 |
| total_reward_pct | -0.00459 |
| total_trades | 49 |
| time/ | |
| fps | 370 |
| iterations | 4400 |
| time_elapsed | 59 |
| total_timesteps | 22000 |
| train/ | |
| entropy_loss | -1.86 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| n_updates | 4399 |
| policy_loss | 7.88e-05 |
| std | 1.55 |
| value_loss | 2.15e-09 |
------------------------------------
day: 95, episode: 3090
begin_total_asset: 100000.00
end_total_asset: 99984.57
total_reward: -15.43
total_cost: 3.90
total_trades: 58
Sharpe: -2.397
=================================
------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 3.9 |
| total_reward | -15.4 |
| total_reward_pct | -0.0154 |
| total_trades | 58 |
| time/ | |
| fps | 370 |
| iterations | 4500 |
| time_elapsed | 60 |
| total_timesteps | 22500 |
| train/ | |
| entropy_loss | -1.87 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| n_updates | 4499 |
| policy_loss | 0.000315 |
| std | 1.57 |
| value_loss | 5.56e-08 |
------------------------------------
------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 1.67 |
| total_reward | -4.65 |
| total_reward_pct | -0.00465 |
| total_trades | 34 |
| time/ | |
| fps | 370 |
| iterations | 4600 |
| time_elapsed | 62 |
| total_timesteps | 23000 |
| train/ | |
| entropy_loss | -1.88 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| n_updates | 4599 |
| policy_loss | 2.18e-06 |
| std | 1.58 |
| value_loss | 4.4e-12 |
------------------------------------
day: 95, episode: 3100
begin_total_asset: 100000.00
end_total_asset: 99990.06
total_reward: -9.94
total_cost: 2.80
total_trades: 55
Sharpe: -3.115
=================================
-------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 2.8 |
| total_reward | -9.94 |
| total_reward_pct | -0.00994 |
| total_trades | 55 |
| time/ | |
| fps | 370 |
| iterations | 4700 |
| time_elapsed | 63 |
| total_timesteps | 23500 |
| train/ | |
| entropy_loss | -1.89 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| n_updates | 4699 |
| policy_loss | -6.36e-06 |
| std | 1.6 |
| value_loss | 2.34e-11 |
-------------------------------------
------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 2.35 |
| total_reward | -11.1 |
| total_reward_pct | -0.0111 |
| total_trades | 54 |
| time/ | |
| fps | 370 |
| iterations | 4800 |
| time_elapsed | 64 |
| total_timesteps | 24000 |
| train/ | |
| entropy_loss | -1.9 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| n_updates | 4799 |
| policy_loss | 7.35e-05 |
| std | 1.62 |
| value_loss | 2.15e-09 |
------------------------------------
day: 95, episode: 3110
begin_total_asset: 100000.00
end_total_asset: 99998.05
total_reward: -1.95
total_cost: 1.78
total_trades: 45
Sharpe: -2.357
=================================
------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 1.79 |
| total_reward | -8.06 |
| total_reward_pct | -0.00806 |
| total_trades | 42 |
| time/ | |
| fps | 370 |
| iterations | 4900 |
| time_elapsed | 66 |
| total_timesteps | 24500 |
| train/ | |
| entropy_loss | -1.91 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| n_updates | 4899 |
| policy_loss | 0.000266 |
| std | 1.63 |
| value_loss | 3.25e-08 |
------------------------------------
-------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 1.94 |
| total_reward | -5.97 |
| total_reward_pct | -0.00597 |
| total_trades | 42 |
| time/ | |
| fps | 370 |
| iterations | 5000 |
| time_elapsed | 67 |
| total_timesteps | 25000 |
| train/ | |
| entropy_loss | -1.92 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| n_updates | 4999 |
| policy_loss | -1.07e-05 |
| std | 1.65 |
| value_loss | 3.52e-11 |
-------------------------------------
day: 95, episode: 3120
begin_total_asset: 100000.00
end_total_asset: 99997.67
total_reward: -2.33
total_cost: 1.60
total_trades: 36
Sharpe: -4.466
=================================
------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 2.56 |
| total_reward | -6.09 |
| total_reward_pct | -0.00609 |
| total_trades | 50 |
| time/ | |
| fps | 370 |
| iterations | 5100 |
| time_elapsed | 68 |
| total_timesteps | 25500 |
| train/ | |
| entropy_loss | -1.93 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| n_updates | 5099 |
| policy_loss | 3.43e-05 |
| std | 1.66 |
| value_loss | 2.87e-10 |
------------------------------------
-------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 3.12 |
| total_reward | -11.7 |
| total_reward_pct | -0.0117 |
| total_trades | 59 |
| time/ | |
| fps | 370 |
| iterations | 5200 |
| time_elapsed | 70 |
| total_timesteps | 26000 |
| train/ | |
| entropy_loss | -1.94 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| n_updates | 5199 |
| policy_loss | -0.000264 |
| std | 1.68 |
| value_loss | 3.46e-08 |
-------------------------------------
day: 95, episode: 3130
begin_total_asset: 100000.00
end_total_asset: 99993.85
total_reward: -6.15
total_cost: 2.03
total_trades: 48
Sharpe: -2.904
=================================
-------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 1.88 |
| total_reward | -4.06 |
| total_reward_pct | -0.00406 |
| total_trades | 38 |
| time/ | |
| fps | 370 |
| iterations | 5300 |
| time_elapsed | 71 |
| total_timesteps | 26500 |
| train/ | |
| entropy_loss | -1.95 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| n_updates | 5299 |
| policy_loss | -2.91e-05 |
| std | 1.7 |
| value_loss | 2.64e-10 |
-------------------------------------
------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 1.84 |
| total_reward | -12.6 |
| total_reward_pct | -0.0126 |
| total_trades | 41 |
| time/ | |
| fps | 370 |
| iterations | 5400 |
| time_elapsed | 72 |
| total_timesteps | 27000 |
| train/ | |
| entropy_loss | -1.96 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| n_updates | 5399 |
| policy_loss | 0.000106 |
| std | 1.71 |
| value_loss | 2.65e-09 |
------------------------------------
day: 95, episode: 3140
begin_total_asset: 100000.00
end_total_asset: 99997.56
total_reward: -2.44
total_cost: 1.46
total_trades: 37
Sharpe: -5.609
=================================
------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 1.74 |
| total_reward | -7.61 |
| total_reward_pct | -0.00761 |
| total_trades | 36 |
| time/ | |
| fps | 370 |
| iterations | 5500 |
| time_elapsed | 74 |
| total_timesteps | 27500 |
| train/ | |
| entropy_loss | -1.97 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| n_updates | 5499 |
| policy_loss | 0.000559 |
| std | 1.73 |
| value_loss | 1.19e-07 |
------------------------------------
-------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 3.11 |
| total_reward | -6.03 |
| total_reward_pct | -0.00603 |
| total_trades | 58 |
| time/ | |
| fps | 371 |
| iterations | 5600 |
| time_elapsed | 75 |
| total_timesteps | 28000 |
| train/ | |
| entropy_loss | -1.98 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| n_updates | 5599 |
| policy_loss | -9.99e-05 |
| std | 1.75 |
| value_loss | 4.11e-09 |
-------------------------------------
day: 95, episode: 3150
begin_total_asset: 100000.00
end_total_asset: 99994.78
total_reward: -5.22
total_cost: 2.34
total_trades: 47
Sharpe: -4.371
=================================
------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 2.29 |
| total_reward | -6.87 |
| total_reward_pct | -0.00687 |
| total_trades | 47 |
| time/ | |
| fps | 371 |
| iterations | 5700 |
| time_elapsed | 76 |
| total_timesteps | 28500 |
| train/ | |
| entropy_loss | -1.99 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| n_updates | 5699 |
| policy_loss | 3.42e-05 |
| std | 1.77 |
| value_loss | 3.83e-10 |
------------------------------------
------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 2.31 |
| total_reward | -7.81 |
| total_reward_pct | -0.00781 |
| total_trades | 48 |
| time/ | |
| fps | 371 |
| iterations | 5800 |
| time_elapsed | 78 |
| total_timesteps | 29000 |
| train/ | |
| entropy_loss | -2 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| n_updates | 5799 |
| policy_loss | 0.000517 |
| std | 1.78 |
| value_loss | 1.37e-07 |
------------------------------------
day: 95, episode: 3160
begin_total_asset: 100000.00
end_total_asset: 99996.41
total_reward: -3.59
total_cost: 1.86
total_trades: 38
Sharpe: -5.990
=================================
------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 1.91 |
| total_reward | -6.11 |
| total_reward_pct | -0.00611 |
| total_trades | 36 |
| time/ | |
| fps | 370 |
| iterations | 5900 |
| time_elapsed | 79 |
| total_timesteps | 29500 |
| train/ | |
| entropy_loss | -2.01 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| n_updates | 5899 |
| policy_loss | -0.00028 |
| std | 1.8 |
| value_loss | 3.01e-08 |
------------------------------------
-------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 1.82 |
| total_reward | -8.73 |
| total_reward_pct | -0.00873 |
| total_trades | 43 |
| time/ | |
| fps | 371 |
| iterations | 6000 |
| time_elapsed | 80 |
| total_timesteps | 30000 |
| train/ | |
| entropy_loss | -2.02 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| n_updates | 5999 |
| policy_loss | -0.000272 |
| std | 1.82 |
| value_loss | 1.82e-08 |
-------------------------------------
day: 95, episode: 3170
begin_total_asset: 100000.00
end_total_asset: 99991.84
total_reward: -8.16
total_cost: 2.08
total_trades: 43
Sharpe: -3.851
=================================
-------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 2.39 |
| total_reward | -1.88 |
| total_reward_pct | -0.00188 |
| total_trades | 47 |
| time/ | |
| fps | 371 |
| iterations | 6100 |
| time_elapsed | 82 |
| total_timesteps | 30500 |
| train/ | |
| entropy_loss | -2.03 |
| explained_variance | -2.95e+10 |
| learning_rate | 0.0001 |
| n_updates | 6099 |
| policy_loss | 0.00028 |
| std | 1.84 |
| value_loss | 3.3e-08 |
-------------------------------------
-------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 2.82 |
| total_reward | -5.51 |
| total_reward_pct | -0.00551 |
| total_trades | 57 |
| time/ | |
| fps | 371 |
| iterations | 6200 |
| time_elapsed | 83 |
| total_timesteps | 31000 |
| train/ | |
| entropy_loss | -2.04 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| n_updates | 6199 |
| policy_loss | -0.000123 |
| std | 1.86 |
| value_loss | 5.56e-09 |
-------------------------------------
day: 95, episode: 3180
begin_total_asset: 100000.00
end_total_asset: 99992.54
total_reward: -7.46
total_cost: 1.91
total_trades: 46
Sharpe: -3.331
=================================
-------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 2.27 |
| total_reward | -2.3 |
| total_reward_pct | -0.0023 |
| total_trades | 46 |
| time/ | |
| fps | 371 |
| iterations | 6300 |
| time_elapsed | 84 |
| total_timesteps | 31500 |
| train/ | |
| entropy_loss | -2.05 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| n_updates | 6299 |
| policy_loss | -0.000591 |
| std | 1.88 |
| value_loss | 9.96e-08 |
-------------------------------------
-------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 1.45 |
| total_reward | -1.54 |
| total_reward_pct | -0.00154 |
| total_trades | 36 |
| time/ | |
| fps | 371 |
| iterations | 6400 |
| time_elapsed | 86 |
| total_timesteps | 32000 |
| train/ | |
| entropy_loss | -2.06 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| n_updates | 6399 |
| policy_loss | -2.68e-05 |
| std | 1.89 |
| value_loss | 2.22e-10 |
-------------------------------------
day: 95, episode: 3190
begin_total_asset: 100000.00
end_total_asset: 99994.75
total_reward: -5.25
total_cost: 1.54
total_trades: 41
Sharpe: -3.179
=================================
-------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 3.25 |
| total_reward | -8.91 |
| total_reward_pct | -0.00891 |
| total_trades | 52 |
| time/ | |
| fps | 371 |
| iterations | 6500 |
| time_elapsed | 87 |
| total_timesteps | 32500 |
| train/ | |
| entropy_loss | -2.07 |
| explained_variance | -5.7e+10 |
| learning_rate | 0.0001 |
| n_updates | 6499 |
| policy_loss | -0.000112 |
| std | 1.91 |
| value_loss | 4.02e-09 |
-------------------------------------
-------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 2.01 |
| total_reward | -6.48 |
| total_reward_pct | -0.00648 |
| total_trades | 43 |
| time/ | |
| fps | 371 |
| iterations | 6600 |
| time_elapsed | 88 |
| total_timesteps | 33000 |
| train/ | |
| entropy_loss | -2.08 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| n_updates | 6599 |
| policy_loss | -5.82e-05 |
| std | 1.93 |
| value_loss | 1.72e-09 |
-------------------------------------
day: 95, episode: 3200
begin_total_asset: 100000.00
end_total_asset: 99990.40
total_reward: -9.60
total_cost: 2.57
total_trades: 53
Sharpe: -4.616
=================================
-------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 3.88 |
| total_reward | -11.3 |
| total_reward_pct | -0.0113 |
| total_trades | 64 |
| time/ | |
| fps | 371 |
| iterations | 6700 |
| time_elapsed | 90 |
| total_timesteps | 33500 |
| train/ | |
| entropy_loss | -2.09 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| n_updates | 6699 |
| policy_loss | -4.13e-05 |
| std | 1.95 |
| value_loss | 5.02e-10 |
-------------------------------------
day: 95, episode: 3210
begin_total_asset: 100000.00
end_total_asset: 99997.38
total_reward: -2.62
total_cost: 1.73
total_trades: 36
Sharpe: -3.369
=================================
------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 1.73 |
| total_reward | -2.62 |
| total_reward_pct | -0.00262 |
| total_trades | 36 |
| time/ | |
| fps | 371 |
| iterations | 6800 |
| time_elapsed | 91 |
| total_timesteps | 34000 |
| train/ | |
| entropy_loss | -2.1 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| n_updates | 6799 |
| policy_loss | -0.00125 |
| std | 1.97 |
| value_loss | 5.26e-07 |
------------------------------------
-------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 1.38 |
| total_reward | -4.44 |
| total_reward_pct | -0.00444 |
| total_trades | 35 |
| time/ | |
| fps | 371 |
| iterations | 6900 |
| time_elapsed | 92 |
| total_timesteps | 34500 |
| train/ | |
| entropy_loss | -2.11 |
| explained_variance | -3.45e+10 |
| learning_rate | 0.0001 |
| n_updates | 6899 |
| policy_loss | 0.000376 |
| std | 1.99 |
| value_loss | 3.96e-08 |
-------------------------------------
day: 95, episode: 3220
begin_total_asset: 100000.00
end_total_asset: 99992.44
total_reward: -7.56
total_cost: 2.80
total_trades: 57
Sharpe: -5.477
=================================
------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 2.8 |
| total_reward | -7.56 |
| total_reward_pct | -0.00756 |
| total_trades | 57 |
| time/ | |
| fps | 371 |
| iterations | 7000 |
| time_elapsed | 94 |
| total_timesteps | 35000 |
| train/ | |
| entropy_loss | -2.12 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| n_updates | 6999 |
| policy_loss | 2.6e-05 |
| std | 2.01 |
| value_loss | 2.3e-10 |
------------------------------------
-------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 1.11 |
| total_reward | -3.59 |
| total_reward_pct | -0.00359 |
| total_trades | 27 |
| time/ | |
| fps | 372 |
| iterations | 7100 |
| time_elapsed | 95 |
| total_timesteps | 35500 |
| train/ | |
| entropy_loss | -2.13 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| n_updates | 7099 |
| policy_loss | -2.73e-06 |
| std | 2.03 |
| value_loss | 1.31e-11 |
-------------------------------------
day: 95, episode: 3230
begin_total_asset: 100000.00
end_total_asset: 99988.30
total_reward: -11.70
total_cost: 3.16
total_trades: 51
Sharpe: -2.927
=================================
-------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 1.32 |
| total_reward | -6.75 |
| total_reward_pct | -0.00675 |
| total_trades | 32 |
| time/ | |
| fps | 372 |
| iterations | 7200 |
| time_elapsed | 96 |
| total_timesteps | 36000 |
| train/ | |
| entropy_loss | -2.14 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| n_updates | 7199 |
| policy_loss | -6.25e-06 |
| std | 2.05 |
| value_loss | 2.85e-10 |
-------------------------------------
------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 1.3 |
| total_reward | -1.68 |
| total_reward_pct | -0.00168 |
| total_trades | 32 |
| time/ | |
| fps | 372 |
| iterations | 7300 |
| time_elapsed | 98 |
| total_timesteps | 36500 |
| train/ | |
| entropy_loss | -2.15 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| n_updates | 7299 |
| policy_loss | 6.98e-06 |
| std | 2.07 |
| value_loss | 6.07e-11 |
------------------------------------
day: 95, episode: 3240
begin_total_asset: 100000.00
end_total_asset: 99999.14
total_reward: -0.86
total_cost: 1.39
total_trades: 36
Sharpe: -1.032
=================================
------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 2.55 |
| total_reward | -4.37 |
| total_reward_pct | -0.00437 |
| total_trades | 48 |
| time/ | |
| fps | 372 |
| iterations | 7400 |
| time_elapsed | 99 |
| total_timesteps | 37000 |
| train/ | |
| entropy_loss | -2.16 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| n_updates | 7399 |
| policy_loss | 1.28e-06 |
| std | 2.09 |
| value_loss | 6.62e-11 |
------------------------------------
------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 1.52 |
| total_reward | -1.74 |
| total_reward_pct | -0.00174 |
| total_trades | 35 |
| time/ | |
| fps | 372 |
| iterations | 7500 |
| time_elapsed | 100 |
| total_timesteps | 37500 |
| train/ | |
| entropy_loss | -2.17 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| n_updates | 7499 |
| policy_loss | 3.09e-05 |
| std | 2.11 |
| value_loss | 2.6e-10 |
------------------------------------
day: 95, episode: 3250
begin_total_asset: 100000.00
end_total_asset: 99994.17
total_reward: -5.83
total_cost: 3.25
total_trades: 55
Sharpe: -3.443
=================================
------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 1 |
| total_reward | -2.23 |
| total_reward_pct | -0.00223 |
| total_trades | 23 |
| time/ | |
| fps | 372 |
| iterations | 7600 |
| time_elapsed | 102 |
| total_timesteps | 38000 |
| train/ | |
| entropy_loss | -2.18 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| n_updates | 7599 |
| policy_loss | 1.43e-05 |
| std | 2.14 |
| value_loss | 8.28e-11 |
------------------------------------
-------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 1.63 |
| total_reward | -12.6 |
| total_reward_pct | -0.0126 |
| total_trades | 35 |
| time/ | |
| fps | 372 |
| iterations | 7700 |
| time_elapsed | 103 |
| total_timesteps | 38500 |
| train/ | |
| entropy_loss | -2.19 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| n_updates | 7699 |
| policy_loss | -3.15e-05 |
| std | 2.16 |
| value_loss | 3.1e-10 |
-------------------------------------
day: 95, episode: 3260
begin_total_asset: 100000.00
end_total_asset: 99996.99
total_reward: -3.01
total_cost: 1.34
total_trades: 32
Sharpe: -5.189
=================================
-------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 1.66 |
| total_reward | -4.49 |
| total_reward_pct | -0.00449 |
| total_trades | 35 |
| time/ | |
| fps | 372 |
| iterations | 7800 |
| time_elapsed | 104 |
| total_timesteps | 39000 |
| train/ | |
| entropy_loss | -2.2 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| n_updates | 7799 |
| policy_loss | -0.000329 |
| std | 2.18 |
| value_loss | 2.17e-08 |
-------------------------------------
------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 1.89 |
| total_reward | -4.69 |
| total_reward_pct | -0.00469 |
| total_trades | 46 |
| time/ | |
| fps | 372 |
| iterations | 7900 |
| time_elapsed | 106 |
| total_timesteps | 39500 |
| train/ | |
| entropy_loss | -2.21 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| n_updates | 7899 |
| policy_loss | 3.97e-05 |
| std | 2.2 |
| value_loss | 4.21e-10 |
------------------------------------
day: 95, episode: 3270
begin_total_asset: 100000.00
end_total_asset: 99994.00
total_reward: -6.00
total_cost: 1.78
total_trades: 36
Sharpe: -3.903
=================================
-------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 1.99 |
| total_reward | -2.63 |
| total_reward_pct | -0.00263 |
| total_trades | 45 |
| time/ | |
| fps | 372 |
| iterations | 8000 |
| time_elapsed | 107 |
| total_timesteps | 40000 |
| train/ | |
| entropy_loss | -2.22 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| n_updates | 7999 |
| policy_loss | -9.45e-05 |
| std | 2.22 |
| value_loss | 3.22e-09 |
-------------------------------------
------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 2.3 |
| total_reward | -3.8 |
| total_reward_pct | -0.0038 |
| total_trades | 42 |
| time/ | |
| fps | 372 |
| iterations | 8100 |
| time_elapsed | 108 |
| total_timesteps | 40500 |
| train/ | |
| entropy_loss | -2.23 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| n_updates | 8099 |
| policy_loss | 0.000631 |
| std | 2.24 |
| value_loss | 7.96e-08 |
------------------------------------
day: 95, episode: 3280
begin_total_asset: 100000.00
end_total_asset: 99981.02
total_reward: -18.98
total_cost: 3.73
total_trades: 51
Sharpe: -4.289
=================================
------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 1.88 |
| total_reward | -10.5 |
| total_reward_pct | -0.0105 |
| total_trades | 37 |
| time/ | |
| fps | 372 |
| iterations | 8200 |
| time_elapsed | 110 |
| total_timesteps | 41000 |
| train/ | |
| entropy_loss | -2.24 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| n_updates | 8199 |
| policy_loss | 0.0003 |
| std | 2.27 |
| value_loss | 6.37e-08 |
------------------------------------
------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 2.43 |
| total_reward | -6.36 |
| total_reward_pct | -0.00636 |
| total_trades | 44 |
| time/ | |
| fps | 372 |
| iterations | 8300 |
| time_elapsed | 111 |
| total_timesteps | 41500 |
| train/ | |
| entropy_loss | -2.25 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| n_updates | 8299 |
| policy_loss | 0.000194 |
| std | 2.29 |
| value_loss | 7.02e-09 |
------------------------------------
day: 95, episode: 3290
begin_total_asset: 100000.00
end_total_asset: 99994.49
total_reward: -5.51
total_cost: 2.04
total_trades: 40
Sharpe: -3.068
=================================
-------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 2.26 |
| total_reward | -10.4 |
| total_reward_pct | -0.0104 |
| total_trades | 44 |
| time/ | |
| fps | 372 |
| iterations | 8400 |
| time_elapsed | 112 |
| total_timesteps | 42000 |
| train/ | |
| entropy_loss | -2.26 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| n_updates | 8399 |
| policy_loss | -2.67e-05 |
| std | 2.31 |
| value_loss | 2.64e-10 |
-------------------------------------
------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 1.24 |
| total_reward | -1.49 |
| total_reward_pct | -0.00149 |
| total_trades | 27 |
| time/ | |
| fps | 372 |
| iterations | 8500 |
| time_elapsed | 114 |
| total_timesteps | 42500 |
| train/ | |
| entropy_loss | -2.27 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| n_updates | 8499 |
| policy_loss | 7.28e-05 |
| std | 2.33 |
| value_loss | 1.5e-09 |
------------------------------------
day: 95, episode: 3300
begin_total_asset: 100000.00
end_total_asset: 99992.52
total_reward: -7.48
total_cost: 1.91
total_trades: 38
Sharpe: -2.664
=================================
-------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 1.53 |
| total_reward | -3.14 |
| total_reward_pct | -0.00314 |
| total_trades | 38 |
| time/ | |
| fps | 372 |
| iterations | 8600 |
| time_elapsed | 115 |
| total_timesteps | 43000 |
| train/ | |
| entropy_loss | -2.28 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| n_updates | 8599 |
| policy_loss | -0.000481 |
| std | 2.36 |
| value_loss | 5e-08 |
-------------------------------------
-------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 2.76 |
| total_reward | -13.7 |
| total_reward_pct | -0.0137 |
| total_trades | 42 |
| time/ | |
| fps | 372 |
| iterations | 8700 |
| time_elapsed | 116 |
| total_timesteps | 43500 |
| train/ | |
| entropy_loss | -2.29 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| n_updates | 8699 |
| policy_loss | -0.000415 |
| std | 2.38 |
| value_loss | 2.81e-08 |
-------------------------------------
day: 95, episode: 3310
begin_total_asset: 100000.00
end_total_asset: 99994.22
total_reward: -5.78
total_cost: 1.35
total_trades: 25
Sharpe: -2.622
=================================
-------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 2.07 |
| total_reward | -6.91 |
| total_reward_pct | -0.00691 |
| total_trades | 43 |
| time/ | |
| fps | 372 |
| iterations | 8800 |
| time_elapsed | 118 |
| total_timesteps | 44000 |
| train/ | |
| entropy_loss | -2.3 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| n_updates | 8799 |
| policy_loss | -2.01e-05 |
| std | 2.41 |
| value_loss | 1.05e-10 |
-------------------------------------
------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 2.33 |
| total_reward | -5.32 |
| total_reward_pct | -0.00532 |
| total_trades | 39 |
| time/ | |
| fps | 372 |
| iterations | 8900 |
| time_elapsed | 119 |
| total_timesteps | 44500 |
| train/ | |
| entropy_loss | -2.31 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| n_updates | 8899 |
| policy_loss | 1.53e-05 |
| std | 2.43 |
| value_loss | 6.84e-11 |
------------------------------------
day: 95, episode: 3320
begin_total_asset: 100000.00
end_total_asset: 99988.09
total_reward: -11.91
total_cost: 2.21
total_trades: 38
Sharpe: -2.029
=================================
-------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 2.35 |
| total_reward | -9.02 |
| total_reward_pct | -0.00902 |
| total_trades | 41 |
| time/ | |
| fps | 372 |
| iterations | 9000 |
| time_elapsed | 120 |
| total_timesteps | 45000 |
| train/ | |
| entropy_loss | -2.32 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| n_updates | 8999 |
| policy_loss | -1.03e-05 |
| std | 2.45 |
| value_loss | 2.49e-11 |
-------------------------------------
-------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 1.53 |
| total_reward | -5.82 |
| total_reward_pct | -0.00582 |
| total_trades | 34 |
| time/ | |
| fps | 372 |
| iterations | 9100 |
| time_elapsed | 122 |
| total_timesteps | 45500 |
| train/ | |
| entropy_loss | -2.33 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| n_updates | 9099 |
| policy_loss | -4.68e-05 |
| std | 2.48 |
| value_loss | 6.3e-10 |
-------------------------------------
day: 95, episode: 3330
begin_total_asset: 100000.00
end_total_asset: 99998.82
total_reward: -1.18
total_cost: 2.16
total_trades: 37
Sharpe: -1.144
=================================
-------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 1.11 |
| total_reward | -2.89 |
| total_reward_pct | -0.00289 |
| total_trades | 31 |
| time/ | |
| fps | 372 |
| iterations | 9200 |
| time_elapsed | 123 |
| total_timesteps | 46000 |
| train/ | |
| entropy_loss | -2.34 |
| explained_variance | -4.08e+10 |
| learning_rate | 0.0001 |
| n_updates | 9199 |
| policy_loss | 0.000241 |
| std | 2.5 |
| value_loss | 1.34e-08 |
-------------------------------------
day: 95, episode: 3340
begin_total_asset: 100000.00
end_total_asset: 99984.64
total_reward: -15.36
total_cost: 3.06
total_trades: 40
Sharpe: -2.256
=================================
------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 3.06 |
| total_reward | -15.4 |
| total_reward_pct | -0.0154 |
| total_trades | 40 |
| time/ | |
| fps | 372 |
| iterations | 9300 |
| time_elapsed | 124 |
| total_timesteps | 46500 |
| train/ | |
| entropy_loss | -2.35 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| n_updates | 9299 |
| policy_loss | 0.000397 |
| std | 2.53 |
| value_loss | 4.38e-08 |
------------------------------------
-------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 2.97 |
| total_reward | -6.17 |
| total_reward_pct | -0.00617 |
| total_trades | 48 |
| time/ | |
| fps | 372 |
| iterations | 9400 |
| time_elapsed | 126 |
| total_timesteps | 47000 |
| train/ | |
| entropy_loss | -2.36 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| n_updates | 9399 |
| policy_loss | -1.43e-05 |
| std | 2.55 |
| value_loss | 4.38e-11 |
-------------------------------------
day: 95, episode: 3350
begin_total_asset: 100000.00
end_total_asset: 100000.84
total_reward: 0.84
total_cost: 1.02
total_trades: 28
Sharpe: 0.809
=================================
-------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 1.02 |
| total_reward | 0.842 |
| total_reward_pct | 0.000842 |
| total_trades | 28 |
| time/ | |
| fps | 372 |
| iterations | 9500 |
| time_elapsed | 127 |
| total_timesteps | 47500 |
| train/ | |
| entropy_loss | -2.37 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| n_updates | 9499 |
| policy_loss | -1.62e-05 |
| std | 2.58 |
| value_loss | 1e-10 |
-------------------------------------
------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 1.7 |
| total_reward | -3.81 |
| total_reward_pct | -0.00381 |
| total_trades | 32 |
| time/ | |
| fps | 372 |
| iterations | 9600 |
| time_elapsed | 128 |
| total_timesteps | 48000 |
| train/ | |
| entropy_loss | -2.38 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| n_updates | 9599 |
| policy_loss | 0.000184 |
| std | 2.6 |
| value_loss | 1.17e-08 |
------------------------------------
day: 95, episode: 3360
begin_total_asset: 100000.00
end_total_asset: 99995.39
total_reward: -4.61
total_cost: 2.38
total_trades: 39
Sharpe: -4.970
=================================
------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 1.42 |
| total_reward | -3.23 |
| total_reward_pct | -0.00323 |
| total_trades | 34 |
| time/ | |
| fps | 373 |
| iterations | 9700 |
| time_elapsed | 130 |
| total_timesteps | 48500 |
| train/ | |
| entropy_loss | -2.39 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| n_updates | 9699 |
| policy_loss | 9.57e-05 |
| std | 2.63 |
| value_loss | 2.45e-09 |
------------------------------------
-------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 1.64 |
| total_reward | -4.1 |
| total_reward_pct | -0.0041 |
| total_trades | 34 |
| time/ | |
| fps | 373 |
| iterations | 9800 |
| time_elapsed | 131 |
| total_timesteps | 49000 |
| train/ | |
| entropy_loss | -2.4 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| n_updates | 9799 |
| policy_loss | -7.42e-06 |
| std | 2.66 |
| value_loss | 2.51e-11 |
-------------------------------------
day: 95, episode: 3370
begin_total_asset: 100000.00
end_total_asset: 99988.76
total_reward: -11.24
total_cost: 2.24
total_trades: 37
Sharpe: -2.558
=================================
------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 1.98 |
| total_reward | -13.3 |
| total_reward_pct | -0.0133 |
| total_trades | 35 |
| time/ | |
| fps | 373 |
| iterations | 9900 |
| time_elapsed | 132 |
| total_timesteps | 49500 |
| train/ | |
| entropy_loss | -2.41 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| n_updates | 9899 |
| policy_loss | 3.37e-05 |
| std | 2.68 |
| value_loss | 2.88e-10 |
------------------------------------
------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 1.68 |
| total_reward | -5.94 |
| total_reward_pct | -0.00594 |
| total_trades | 30 |
| time/ | |
| fps | 373 |
| iterations | 10000 |
| time_elapsed | 134 |
| total_timesteps | 50000 |
| train/ | |
| entropy_loss | -2.42 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| n_updates | 9999 |
| policy_loss | -3.1e-08 |
| std | 2.71 |
| value_loss | 2.07e-15 |
------------------------------------
###Markdown
Model 2: DDPG
###Code
agent = DRLAgent(env = env_train)
DDPG_PARAMS = {"batch_size": 64, "buffer_size": 500000, "learning_rate": 0.0001}
model_ddpg = agent.get_model("ddpg",model_kwargs = DDPG_PARAMS)
trained_ddpg = agent.train_model(model=model_ddpg,
tb_log_name='ddpg',
total_timesteps=30000)
###Output
Logging to tensorboard_log/ddpg/ddpg_1
-----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 4 |
| fps | 108 |
| time_elapsed | 3 |
| total timesteps | 384 |
| train/ | |
| actor_loss | -1.17e+03 |
| critic_loss | 1.61e+04 |
| learning_rate | 0.0001 |
| n_updates | 192 |
-----------------------------------
day: 95, episode: 530
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
-----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 8 |
| fps | 76 |
| time_elapsed | 9 |
| total timesteps | 768 |
| train/ | |
| actor_loss | -1.13e+03 |
| critic_loss | 1.55e+04 |
| learning_rate | 0.0001 |
| n_updates | 576 |
-----------------------------------
-----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 12 |
| fps | 70 |
| time_elapsed | 16 |
| total timesteps | 1152 |
| train/ | |
| actor_loss | -1.08e+03 |
| critic_loss | 1.94e+04 |
| learning_rate | 0.0001 |
| n_updates | 960 |
-----------------------------------
-----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 16 |
| fps | 67 |
| time_elapsed | 22 |
| total timesteps | 1536 |
| train/ | |
| actor_loss | -1.05e+03 |
| critic_loss | 1.28e+04 |
| learning_rate | 0.0001 |
| n_updates | 1344 |
-----------------------------------
day: 95, episode: 540
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
-----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 20 |
| fps | 65 |
| time_elapsed | 29 |
| total timesteps | 1920 |
| train/ | |
| actor_loss | -1.01e+03 |
| critic_loss | 1.52e+04 |
| learning_rate | 0.0001 |
| n_updates | 1728 |
-----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 24 |
| fps | 63 |
| time_elapsed | 36 |
| total timesteps | 2304 |
| train/ | |
| actor_loss | -968 |
| critic_loss | 1.41e+04 |
| learning_rate | 0.0001 |
| n_updates | 2112 |
----------------------------------
day: 95, episode: 550
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 28 |
| fps | 62 |
| time_elapsed | 42 |
| total timesteps | 2688 |
| train/ | |
| actor_loss | -930 |
| critic_loss | 1.47e+04 |
| learning_rate | 0.0001 |
| n_updates | 2496 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 32 |
| fps | 60 |
| time_elapsed | 50 |
| total timesteps | 3072 |
| train/ | |
| actor_loss | -893 |
| critic_loss | 1.31e+04 |
| learning_rate | 0.0001 |
| n_updates | 2880 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 36 |
| fps | 60 |
| time_elapsed | 57 |
| total timesteps | 3456 |
| train/ | |
| actor_loss | -856 |
| critic_loss | 1.27e+04 |
| learning_rate | 0.0001 |
| n_updates | 3264 |
----------------------------------
day: 95, episode: 560
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 40 |
| fps | 60 |
| time_elapsed | 63 |
| total timesteps | 3840 |
| train/ | |
| actor_loss | -825 |
| critic_loss | 1.26e+04 |
| learning_rate | 0.0001 |
| n_updates | 3648 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 44 |
| fps | 60 |
| time_elapsed | 70 |
| total timesteps | 4224 |
| train/ | |
| actor_loss | -795 |
| critic_loss | 7.14e+03 |
| learning_rate | 0.0001 |
| n_updates | 4032 |
----------------------------------
day: 95, episode: 570
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 48 |
| fps | 60 |
| time_elapsed | 76 |
| total timesteps | 4608 |
| train/ | |
| actor_loss | -761 |
| critic_loss | 1.04e+04 |
| learning_rate | 0.0001 |
| n_updates | 4416 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 52 |
| fps | 60 |
| time_elapsed | 83 |
| total timesteps | 4992 |
| train/ | |
| actor_loss | -731 |
| critic_loss | 1.16e+04 |
| learning_rate | 0.0001 |
| n_updates | 4800 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 56 |
| fps | 59 |
| time_elapsed | 89 |
| total timesteps | 5376 |
| train/ | |
| actor_loss | -704 |
| critic_loss | 7.49e+03 |
| learning_rate | 0.0001 |
| n_updates | 5184 |
----------------------------------
day: 95, episode: 580
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 60 |
| fps | 59 |
| time_elapsed | 96 |
| total timesteps | 5760 |
| train/ | |
| actor_loss | -676 |
| critic_loss | 7.59e+03 |
| learning_rate | 0.0001 |
| n_updates | 5568 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 64 |
| fps | 59 |
| time_elapsed | 102 |
| total timesteps | 6144 |
| train/ | |
| actor_loss | -649 |
| critic_loss | 9.83e+03 |
| learning_rate | 0.0001 |
| n_updates | 5952 |
----------------------------------
day: 95, episode: 590
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 68 |
| fps | 59 |
| time_elapsed | 109 |
| total timesteps | 6528 |
| train/ | |
| actor_loss | -626 |
| critic_loss | 6.48e+03 |
| learning_rate | 0.0001 |
| n_updates | 6336 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 72 |
| fps | 59 |
| time_elapsed | 115 |
| total timesteps | 6912 |
| train/ | |
| actor_loss | -604 |
| critic_loss | 5.85e+03 |
| learning_rate | 0.0001 |
| n_updates | 6720 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 76 |
| fps | 59 |
| time_elapsed | 121 |
| total timesteps | 7296 |
| train/ | |
| actor_loss | -577 |
| critic_loss | 8.84e+03 |
| learning_rate | 0.0001 |
| n_updates | 7104 |
----------------------------------
day: 95, episode: 600
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 80 |
| fps | 59 |
| time_elapsed | 128 |
| total timesteps | 7680 |
| train/ | |
| actor_loss | -556 |
| critic_loss | 5.49e+03 |
| learning_rate | 0.0001 |
| n_updates | 7488 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 84 |
| fps | 59 |
| time_elapsed | 134 |
| total timesteps | 8064 |
| train/ | |
| actor_loss | -537 |
| critic_loss | 5.03e+03 |
| learning_rate | 0.0001 |
| n_updates | 7872 |
----------------------------------
day: 95, episode: 610
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 88 |
| fps | 59 |
| time_elapsed | 141 |
| total timesteps | 8448 |
| train/ | |
| actor_loss | -514 |
| critic_loss | 8.41e+03 |
| learning_rate | 0.0001 |
| n_updates | 8256 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 92 |
| fps | 59 |
| time_elapsed | 147 |
| total timesteps | 8832 |
| train/ | |
| actor_loss | -495 |
| critic_loss | 7.33e+03 |
| learning_rate | 0.0001 |
| n_updates | 8640 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 96 |
| fps | 59 |
| time_elapsed | 153 |
| total timesteps | 9216 |
| train/ | |
| actor_loss | -477 |
| critic_loss | 4.71e+03 |
| learning_rate | 0.0001 |
| n_updates | 9024 |
----------------------------------
day: 95, episode: 620
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 100 |
| fps | 59 |
| time_elapsed | 160 |
| total timesteps | 9600 |
| train/ | |
| actor_loss | -459 |
| critic_loss | 3.87e+03 |
| learning_rate | 0.0001 |
| n_updates | 9408 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 104 |
| fps | 59 |
| time_elapsed | 166 |
| total timesteps | 9984 |
| train/ | |
| actor_loss | -440 |
| critic_loss | 3.82e+03 |
| learning_rate | 0.0001 |
| n_updates | 9792 |
----------------------------------
day: 95, episode: 630
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 108 |
| fps | 59 |
| time_elapsed | 173 |
| total timesteps | 10368 |
| train/ | |
| actor_loss | -424 |
| critic_loss | 3.54e+03 |
| learning_rate | 0.0001 |
| n_updates | 10176 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 112 |
| fps | 59 |
| time_elapsed | 179 |
| total timesteps | 10752 |
| train/ | |
| actor_loss | -408 |
| critic_loss | 3.97e+03 |
| learning_rate | 0.0001 |
| n_updates | 10560 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 116 |
| fps | 59 |
| time_elapsed | 186 |
| total timesteps | 11136 |
| train/ | |
| actor_loss | -391 |
| critic_loss | 3.87e+03 |
| learning_rate | 0.0001 |
| n_updates | 10944 |
----------------------------------
day: 95, episode: 640
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 120 |
| fps | 59 |
| time_elapsed | 192 |
| total timesteps | 11520 |
| train/ | |
| actor_loss | -377 |
| critic_loss | 3.18e+03 |
| learning_rate | 0.0001 |
| n_updates | 11328 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 124 |
| fps | 59 |
| time_elapsed | 199 |
| total timesteps | 11904 |
| train/ | |
| actor_loss | -363 |
| critic_loss | 3.21e+03 |
| learning_rate | 0.0001 |
| n_updates | 11712 |
----------------------------------
day: 95, episode: 650
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 128 |
| fps | 59 |
| time_elapsed | 205 |
| total timesteps | 12288 |
| train/ | |
| actor_loss | -349 |
| critic_loss | 2.55e+03 |
| learning_rate | 0.0001 |
| n_updates | 12096 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 132 |
| fps | 59 |
| time_elapsed | 212 |
| total timesteps | 12672 |
| train/ | |
| actor_loss | -336 |
| critic_loss | 2.53e+03 |
| learning_rate | 0.0001 |
| n_updates | 12480 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 136 |
| fps | 59 |
| time_elapsed | 218 |
| total timesteps | 13056 |
| train/ | |
| actor_loss | -321 |
| critic_loss | 4.54e+03 |
| learning_rate | 0.0001 |
| n_updates | 12864 |
----------------------------------
day: 95, episode: 660
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 140 |
| fps | 59 |
| time_elapsed | 225 |
| total timesteps | 13440 |
| train/ | |
| actor_loss | -308 |
| critic_loss | 4.18e+03 |
| learning_rate | 0.0001 |
| n_updates | 13248 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 144 |
| fps | 59 |
| time_elapsed | 231 |
| total timesteps | 13824 |
| train/ | |
| actor_loss | -295 |
| critic_loss | 5.73e+03 |
| learning_rate | 0.0001 |
| n_updates | 13632 |
----------------------------------
day: 95, episode: 670
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 148 |
| fps | 59 |
| time_elapsed | 238 |
| total timesteps | 14208 |
| train/ | |
| actor_loss | -285 |
| critic_loss | 3.54e+03 |
| learning_rate | 0.0001 |
| n_updates | 14016 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 152 |
| fps | 59 |
| time_elapsed | 244 |
| total timesteps | 14592 |
| train/ | |
| actor_loss | -273 |
| critic_loss | 2.54e+03 |
| learning_rate | 0.0001 |
| n_updates | 14400 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 156 |
| fps | 59 |
| time_elapsed | 251 |
| total timesteps | 14976 |
| train/ | |
| actor_loss | -263 |
| critic_loss | 3.7e+03 |
| learning_rate | 0.0001 |
| n_updates | 14784 |
----------------------------------
day: 95, episode: 680
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 160 |
| fps | 59 |
| time_elapsed | 257 |
| total timesteps | 15360 |
| train/ | |
| actor_loss | -253 |
| critic_loss | 2.06e+03 |
| learning_rate | 0.0001 |
| n_updates | 15168 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 164 |
| fps | 59 |
| time_elapsed | 263 |
| total timesteps | 15744 |
| train/ | |
| actor_loss | -242 |
| critic_loss | 3.03e+03 |
| learning_rate | 0.0001 |
| n_updates | 15552 |
----------------------------------
day: 95, episode: 690
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 168 |
| fps | 59 |
| time_elapsed | 270 |
| total timesteps | 16128 |
| train/ | |
| actor_loss | -232 |
| critic_loss | 2.18e+03 |
| learning_rate | 0.0001 |
| n_updates | 15936 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 172 |
| fps | 59 |
| time_elapsed | 276 |
| total timesteps | 16512 |
| train/ | |
| actor_loss | -223 |
| critic_loss | 2.82e+03 |
| learning_rate | 0.0001 |
| n_updates | 16320 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 176 |
| fps | 59 |
| time_elapsed | 283 |
| total timesteps | 16896 |
| train/ | |
| actor_loss | -215 |
| critic_loss | 3.55e+03 |
| learning_rate | 0.0001 |
| n_updates | 16704 |
----------------------------------
day: 95, episode: 700
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 180 |
| fps | 59 |
| time_elapsed | 289 |
| total timesteps | 17280 |
| train/ | |
| actor_loss | -207 |
| critic_loss | 2.94e+03 |
| learning_rate | 0.0001 |
| n_updates | 17088 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 184 |
| fps | 59 |
| time_elapsed | 296 |
| total timesteps | 17664 |
| train/ | |
| actor_loss | -198 |
| critic_loss | 4.01e+03 |
| learning_rate | 0.0001 |
| n_updates | 17472 |
----------------------------------
day: 95, episode: 710
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 188 |
| fps | 59 |
| time_elapsed | 302 |
| total timesteps | 18048 |
| train/ | |
| actor_loss | -191 |
| critic_loss | 2.23e+03 |
| learning_rate | 0.0001 |
| n_updates | 17856 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 192 |
| fps | 59 |
| time_elapsed | 309 |
| total timesteps | 18432 |
| train/ | |
| actor_loss | -184 |
| critic_loss | 3.08e+03 |
| learning_rate | 0.0001 |
| n_updates | 18240 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 196 |
| fps | 59 |
| time_elapsed | 315 |
| total timesteps | 18816 |
| train/ | |
| actor_loss | -177 |
| critic_loss | 659 |
| learning_rate | 0.0001 |
| n_updates | 18624 |
----------------------------------
day: 95, episode: 720
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 200 |
| fps | 59 |
| time_elapsed | 322 |
| total timesteps | 19200 |
| train/ | |
| actor_loss | -170 |
| critic_loss | 1.41e+03 |
| learning_rate | 0.0001 |
| n_updates | 19008 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 204 |
| fps | 59 |
| time_elapsed | 328 |
| total timesteps | 19584 |
| train/ | |
| actor_loss | -162 |
| critic_loss | 2.64e+03 |
| learning_rate | 0.0001 |
| n_updates | 19392 |
----------------------------------
day: 95, episode: 730
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 208 |
| fps | 59 |
| time_elapsed | 335 |
| total timesteps | 19968 |
| train/ | |
| actor_loss | -157 |
| critic_loss | 2.18e+03 |
| learning_rate | 0.0001 |
| n_updates | 19776 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 212 |
| fps | 59 |
| time_elapsed | 342 |
| total timesteps | 20352 |
| train/ | |
| actor_loss | -150 |
| critic_loss | 3.07e+03 |
| learning_rate | 0.0001 |
| n_updates | 20160 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 216 |
| fps | 59 |
| time_elapsed | 348 |
| total timesteps | 20736 |
| train/ | |
| actor_loss | -143 |
| critic_loss | 1.19e+03 |
| learning_rate | 0.0001 |
| n_updates | 20544 |
----------------------------------
day: 95, episode: 740
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 220 |
| fps | 59 |
| time_elapsed | 355 |
| total timesteps | 21120 |
| train/ | |
| actor_loss | -138 |
| critic_loss | 2.08e+03 |
| learning_rate | 0.0001 |
| n_updates | 20928 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 224 |
| fps | 59 |
| time_elapsed | 362 |
| total timesteps | 21504 |
| train/ | |
| actor_loss | -133 |
| critic_loss | 2.38e+03 |
| learning_rate | 0.0001 |
| n_updates | 21312 |
----------------------------------
day: 95, episode: 750
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 228 |
| fps | 59 |
| time_elapsed | 368 |
| total timesteps | 21888 |
| train/ | |
| actor_loss | -127 |
| critic_loss | 1.01e+03 |
| learning_rate | 0.0001 |
| n_updates | 21696 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 232 |
| fps | 59 |
| time_elapsed | 375 |
| total timesteps | 22272 |
| train/ | |
| actor_loss | -122 |
| critic_loss | 1.54e+03 |
| learning_rate | 0.0001 |
| n_updates | 22080 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 236 |
| fps | 59 |
| time_elapsed | 382 |
| total timesteps | 22656 |
| train/ | |
| actor_loss | -117 |
| critic_loss | 1.9e+03 |
| learning_rate | 0.0001 |
| n_updates | 22464 |
----------------------------------
day: 95, episode: 760
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 240 |
| fps | 59 |
| time_elapsed | 388 |
| total timesteps | 23040 |
| train/ | |
| actor_loss | -113 |
| critic_loss | 2.32e+03 |
| learning_rate | 0.0001 |
| n_updates | 22848 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 244 |
| fps | 59 |
| time_elapsed | 395 |
| total timesteps | 23424 |
| train/ | |
| actor_loss | -108 |
| critic_loss | 2.72e+03 |
| learning_rate | 0.0001 |
| n_updates | 23232 |
----------------------------------
day: 95, episode: 770
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 248 |
| fps | 59 |
| time_elapsed | 401 |
| total timesteps | 23808 |
| train/ | |
| actor_loss | -104 |
| critic_loss | 723 |
| learning_rate | 0.0001 |
| n_updates | 23616 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 252 |
| fps | 59 |
| time_elapsed | 408 |
| total timesteps | 24192 |
| train/ | |
| actor_loss | -99.9 |
| critic_loss | 1.56e+03 |
| learning_rate | 0.0001 |
| n_updates | 24000 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 256 |
| fps | 59 |
| time_elapsed | 414 |
| total timesteps | 24576 |
| train/ | |
| actor_loss | -96.6 |
| critic_loss | 1.39e+03 |
| learning_rate | 0.0001 |
| n_updates | 24384 |
----------------------------------
day: 95, episode: 780
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 260 |
| fps | 59 |
| time_elapsed | 421 |
| total timesteps | 24960 |
| train/ | |
| actor_loss | -93 |
| critic_loss | 1.25e+03 |
| learning_rate | 0.0001 |
| n_updates | 24768 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 264 |
| fps | 59 |
| time_elapsed | 427 |
| total timesteps | 25344 |
| train/ | |
| actor_loss | -89.2 |
| critic_loss | 1.44e+03 |
| learning_rate | 0.0001 |
| n_updates | 25152 |
----------------------------------
day: 95, episode: 790
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 268 |
| fps | 59 |
| time_elapsed | 434 |
| total timesteps | 25728 |
| train/ | |
| actor_loss | -84.8 |
| critic_loss | 2.25e+03 |
| learning_rate | 0.0001 |
| n_updates | 25536 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 272 |
| fps | 59 |
| time_elapsed | 440 |
| total timesteps | 26112 |
| train/ | |
| actor_loss | -82.6 |
| critic_loss | 1.16e+03 |
| learning_rate | 0.0001 |
| n_updates | 25920 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 276 |
| fps | 59 |
| time_elapsed | 446 |
| total timesteps | 26496 |
| train/ | |
| actor_loss | -80.2 |
| critic_loss | 1.53e+03 |
| learning_rate | 0.0001 |
| n_updates | 26304 |
----------------------------------
day: 95, episode: 800
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 280 |
| fps | 59 |
| time_elapsed | 453 |
| total timesteps | 26880 |
| train/ | |
| actor_loss | -76.4 |
| critic_loss | 1.65e+03 |
| learning_rate | 0.0001 |
| n_updates | 26688 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 284 |
| fps | 59 |
| time_elapsed | 459 |
| total timesteps | 27264 |
| train/ | |
| actor_loss | -74.4 |
| critic_loss | 909 |
| learning_rate | 0.0001 |
| n_updates | 27072 |
----------------------------------
day: 95, episode: 810
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 288 |
| fps | 59 |
| time_elapsed | 466 |
| total timesteps | 27648 |
| train/ | |
| actor_loss | -71.6 |
| critic_loss | 743 |
| learning_rate | 0.0001 |
| n_updates | 27456 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 292 |
| fps | 59 |
| time_elapsed | 472 |
| total timesteps | 28032 |
| train/ | |
| actor_loss | -68.7 |
| critic_loss | 762 |
| learning_rate | 0.0001 |
| n_updates | 27840 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 296 |
| fps | 59 |
| time_elapsed | 479 |
| total timesteps | 28416 |
| train/ | |
| actor_loss | -66 |
| critic_loss | 921 |
| learning_rate | 0.0001 |
| n_updates | 28224 |
----------------------------------
day: 95, episode: 820
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 300 |
| fps | 59 |
| time_elapsed | 485 |
| total timesteps | 28800 |
| train/ | |
| actor_loss | -63.5 |
| critic_loss | 1.49e+03 |
| learning_rate | 0.0001 |
| n_updates | 28608 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 304 |
| fps | 59 |
| time_elapsed | 492 |
| total timesteps | 29184 |
| train/ | |
| actor_loss | -60 |
| critic_loss | 1.54e+03 |
| learning_rate | 0.0001 |
| n_updates | 28992 |
----------------------------------
day: 95, episode: 830
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 308 |
| fps | 59 |
| time_elapsed | 498 |
| total timesteps | 29568 |
| train/ | |
| actor_loss | -59.1 |
| critic_loss | 1.16e+03 |
| learning_rate | 0.0001 |
| n_updates | 29376 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 312 |
| fps | 59 |
| time_elapsed | 504 |
| total timesteps | 29952 |
| train/ | |
| actor_loss | -56 |
| critic_loss | 915 |
| learning_rate | 0.0001 |
| n_updates | 29760 |
----------------------------------
###Markdown
Model 3: PPO
###Code
agent = DRLAgent(env = env_train)
PPO_PARAMS = {
"n_steps": 2048,
"ent_coef": 0.005,
"learning_rate": 0.0001,
"batch_size": 128,
}
model_ppo = agent.get_model("ppo",model_kwargs = PPO_PARAMS)
trained_ppo = agent.train_model(model=model_ppo,
tb_log_name='ppo',
total_timesteps=80000)
###Output
Logging to tensorboard_log/ppo/ppo_1
day: 95, episode: 840
begin_total_asset: 100000.00
end_total_asset: 99976.09
total_reward: -23.91
total_cost: 5.94
total_trades: 95
Sharpe: -2.486
=================================
day: 95, episode: 850
begin_total_asset: 100000.00
end_total_asset: 99959.09
total_reward: -40.91
total_cost: 5.19
total_trades: 87
Sharpe: -2.285
=================================
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 4.07 |
| total_reward | -17.6 |
| total_reward_pct | -0.0176 |
| total_trades | 78 |
| time/ | |
| fps | 664 |
| iterations | 1 |
| time_elapsed | 3 |
| total_timesteps | 2048 |
----------------------------------
day: 95, episode: 860
begin_total_asset: 100000.00
end_total_asset: 99905.87
total_reward: -94.13
total_cost: 7.06
total_trades: 95
Sharpe: -2.290
=================================
day: 95, episode: 870
begin_total_asset: 100000.00
end_total_asset: 99961.52
total_reward: -38.48
total_cost: 6.52
total_trades: 94
Sharpe: -3.110
=================================
------------------------------------------
| environment/ | |
| portfolio_value | 9.99e+04 |
| total_cost | 6.02 |
| total_reward | -50.3 |
| total_reward_pct | -0.0503 |
| total_trades | 90 |
| time/ | |
| fps | 594 |
| iterations | 2 |
| time_elapsed | 6 |
| total_timesteps | 4096 |
| train/ | |
| approx_kl | 0.0018597677 |
| clip_fraction | 0.0332 |
| clip_range | 0.2 |
| entropy_loss | -1.42 |
| explained_variance | -22.2 |
| learning_rate | 0.0001 |
| loss | 0.0467 |
| n_updates | 10 |
| policy_gradient_loss | -0.00302 |
| std | 0.997 |
| value_loss | 0.103 |
------------------------------------------
day: 95, episode: 880
begin_total_asset: 100000.00
end_total_asset: 99968.84
total_reward: -31.16
total_cost: 5.84
total_trades: 87
Sharpe: -1.819
=================================
day: 95, episode: 890
begin_total_asset: 100000.00
end_total_asset: 99973.93
total_reward: -26.07
total_cost: 5.96
total_trades: 92
Sharpe: -3.081
=================================
day: 95, episode: 900
begin_total_asset: 100000.00
end_total_asset: 99941.47
total_reward: -58.53
total_cost: 6.64
total_trades: 92
Sharpe: -2.460
=================================
------------------------------------------
| environment/ | |
| portfolio_value | 9.99e+04 |
| total_cost | 6.64 |
| total_reward | -58.5 |
| total_reward_pct | -0.0585 |
| total_trades | 92 |
| time/ | |
| fps | 576 |
| iterations | 3 |
| time_elapsed | 10 |
| total_timesteps | 6144 |
| train/ | |
| approx_kl | 0.0001741648 |
| clip_fraction | 0 |
| clip_range | 0.2 |
| entropy_loss | -1.41 |
| explained_variance | -8.31e+11 |
| learning_rate | 0.0001 |
| loss | 0.0162 |
| n_updates | 20 |
| policy_gradient_loss | -4.62e-05 |
| std | 0.992 |
| value_loss | 0.0531 |
------------------------------------------
day: 95, episode: 910
begin_total_asset: 100000.00
end_total_asset: 99979.16
total_reward: -20.84
total_cost: 6.25
total_trades: 92
Sharpe: -1.911
=================================
day: 95, episode: 920
begin_total_asset: 100000.00
end_total_asset: 99947.07
total_reward: -52.93
total_cost: 6.83
total_trades: 91
Sharpe: -2.686
=================================
----------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 5.45 |
| total_reward | -19.4 |
| total_reward_pct | -0.0194 |
| total_trades | 88 |
| time/ | |
| fps | 569 |
| iterations | 4 |
| time_elapsed | 14 |
| total_timesteps | 8192 |
| train/ | |
| approx_kl | 0.00422058 |
| clip_fraction | 0.00337 |
| clip_range | 0.2 |
| entropy_loss | -1.41 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| loss | 0.00489 |
| n_updates | 30 |
| policy_gradient_loss | -0.000627 |
| std | 0.988 |
| value_loss | 0.0273 |
----------------------------------------
day: 95, episode: 930
begin_total_asset: 100000.00
end_total_asset: 99956.34
total_reward: -43.66
total_cost: 6.31
total_trades: 87
Sharpe: -2.732
=================================
day: 95, episode: 940
begin_total_asset: 100000.00
end_total_asset: 99974.10
total_reward: -25.90
total_cost: 6.30
total_trades: 92
Sharpe: -2.135
=================================
--------------------------------------------
| environment/ | |
| portfolio_value | 9.99e+04 |
| total_cost | 6.42 |
| total_reward | -59.2 |
| total_reward_pct | -0.0592 |
| total_trades | 93 |
| time/ | |
| fps | 563 |
| iterations | 5 |
| time_elapsed | 18 |
| total_timesteps | 10240 |
| train/ | |
| approx_kl | -0.00015341115 |
| clip_fraction | 0 |
| clip_range | 0.2 |
| entropy_loss | -1.4 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| loss | -0.00157 |
| n_updates | 40 |
| policy_gradient_loss | -7.24e-05 |
| std | 0.982 |
| value_loss | 0.0125 |
--------------------------------------------
day: 95, episode: 950
begin_total_asset: 100000.00
end_total_asset: 99969.25
total_reward: -30.75
total_cost: 6.31
total_trades: 92
Sharpe: -3.518
=================================
day: 95, episode: 960
begin_total_asset: 100000.00
end_total_asset: 99972.81
total_reward: -27.19
total_cost: 6.42
total_trades: 90
Sharpe: -2.623
=================================
------------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 6.04 |
| total_reward | -33.9 |
| total_reward_pct | -0.0339 |
| total_trades | 91 |
| time/ | |
| fps | 560 |
| iterations | 6 |
| time_elapsed | 21 |
| total_timesteps | 12288 |
| train/ | |
| approx_kl | 0.0043742936 |
| clip_fraction | 0.0209 |
| clip_range | 0.2 |
| entropy_loss | -1.4 |
| explained_variance | -1.51e+12 |
| learning_rate | 0.0001 |
| loss | -0.0121 |
| n_updates | 50 |
| policy_gradient_loss | -0.00239 |
| std | 0.981 |
| value_loss | 0.00574 |
------------------------------------------
day: 95, episode: 970
begin_total_asset: 100000.00
end_total_asset: 99984.10
total_reward: -15.90
total_cost: 4.61
total_trades: 81
Sharpe: -3.142
=================================
day: 95, episode: 980
begin_total_asset: 100000.00
end_total_asset: 99976.31
total_reward: -23.69
total_cost: 4.54
total_trades: 76
Sharpe: -3.038
=================================
----------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 3.49 |
| total_reward | -7.17 |
| total_reward_pct | -0.00717 |
| total_trades | 75 |
| time/ | |
| fps | 558 |
| iterations | 7 |
| time_elapsed | 25 |
| total_timesteps | 14336 |
| train/ | |
| approx_kl | 0.00051335 |
| clip_fraction | 0 |
| clip_range | 0.2 |
| entropy_loss | -1.4 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| loss | -0.00605 |
| n_updates | 60 |
| policy_gradient_loss | -0.000103 |
| std | 0.981 |
| value_loss | 0.00277 |
----------------------------------------
day: 95, episode: 990
begin_total_asset: 100000.00
end_total_asset: 99960.73
total_reward: -39.27
total_cost: 6.43
total_trades: 92
Sharpe: -2.109
=================================
day: 95, episode: 1000
begin_total_asset: 100000.00
end_total_asset: 99988.57
total_reward: -11.43
total_cost: 5.14
total_trades: 86
Sharpe: -1.857
=================================
------------------------------------------
| environment/ | |
| portfolio_value | 9.99e+04 |
| total_cost | 7.12 |
| total_reward | -52 |
| total_reward_pct | -0.052 |
| total_trades | 94 |
| time/ | |
| fps | 557 |
| iterations | 8 |
| time_elapsed | 29 |
| total_timesteps | 16384 |
| train/ | |
| approx_kl | 2.588777e-05 |
| clip_fraction | 0 |
| clip_range | 0.2 |
| entropy_loss | -1.4 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| loss | -0.00634 |
| n_updates | 70 |
| policy_gradient_loss | 7.27e-05 |
| std | 0.98 |
| value_loss | 0.0013 |
------------------------------------------
day: 95, episode: 1010
begin_total_asset: 100000.00
end_total_asset: 99971.19
total_reward: -28.81
total_cost: 5.99
total_trades: 91
Sharpe: -2.541
=================================
day: 95, episode: 1020
begin_total_asset: 100000.00
end_total_asset: 99963.61
total_reward: -36.39
total_cost: 6.80
total_trades: 93
Sharpe: -2.344
=================================
------------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 4.78 |
| total_reward | -15.2 |
| total_reward_pct | -0.0152 |
| total_trades | 77 |
| time/ | |
| fps | 555 |
| iterations | 9 |
| time_elapsed | 33 |
| total_timesteps | 18432 |
| train/ | |
| approx_kl | 0.0026266663 |
| clip_fraction | 0.00234 |
| clip_range | 0.2 |
| entropy_loss | -1.4 |
| explained_variance | -1.19e+12 |
| learning_rate | 0.0001 |
| loss | -0.0154 |
| n_updates | 80 |
| policy_gradient_loss | -0.000606 |
| std | 0.981 |
| value_loss | 0.000639 |
------------------------------------------
day: 95, episode: 1030
begin_total_asset: 100000.00
end_total_asset: 99985.99
total_reward: -14.01
total_cost: 4.82
total_trades: 80
Sharpe: -3.469
=================================
day: 95, episode: 1040
begin_total_asset: 100000.00
end_total_asset: 99989.66
total_reward: -10.34
total_cost: 4.18
total_trades: 81
Sharpe: -3.907
=================================
-----------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 6.7 |
| total_reward | -43.6 |
| total_reward_pct | -0.0436 |
| total_trades | 95 |
| time/ | |
| fps | 554 |
| iterations | 10 |
| time_elapsed | 36 |
| total_timesteps | 20480 |
| train/ | |
| approx_kl | 0.002081789 |
| clip_fraction | 0.000195 |
| clip_range | 0.2 |
| entropy_loss | -1.4 |
| explained_variance | -5.04e+12 |
| learning_rate | 0.0001 |
| loss | -0.00083 |
| n_updates | 90 |
| policy_gradient_loss | -0.000217 |
| std | 0.98 |
| value_loss | 0.000305 |
-----------------------------------------
day: 95, episode: 1050
begin_total_asset: 100000.00
end_total_asset: 99975.02
total_reward: -24.98
total_cost: 6.21
total_trades: 90
Sharpe: -3.068
=================================
day: 95, episode: 1060
begin_total_asset: 100000.00
end_total_asset: 99992.20
total_reward: -7.80
total_cost: 3.72
total_trades: 78
Sharpe: -4.010
=================================
day: 95, episode: 1070
begin_total_asset: 100000.00
end_total_asset: 99969.76
total_reward: -30.24
total_cost: 4.92
total_trades: 83
Sharpe: -3.224
=================================
------------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 4.92 |
| total_reward | -30.2 |
| total_reward_pct | -0.0302 |
| total_trades | 83 |
| time/ | |
| fps | 553 |
| iterations | 11 |
| time_elapsed | 40 |
| total_timesteps | 22528 |
| train/ | |
| approx_kl | 0.0056655332 |
| clip_fraction | 0.00293 |
| clip_range | 0.2 |
| entropy_loss | -1.4 |
| explained_variance | -1.08e+12 |
| learning_rate | 0.0001 |
| loss | -0.00829 |
| n_updates | 100 |
| policy_gradient_loss | -0.000624 |
| std | 0.976 |
| value_loss | 0.000145 |
------------------------------------------
day: 95, episode: 1080
begin_total_asset: 100000.00
end_total_asset: 99975.02
total_reward: -24.98
total_cost: 4.61
total_trades: 79
Sharpe: -3.386
=================================
day: 95, episode: 1090
begin_total_asset: 100000.00
end_total_asset: 99969.34
total_reward: -30.66
total_cost: 5.28
total_trades: 93
Sharpe: -3.838
=================================
------------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 5.58 |
| total_reward | -10.9 |
| total_reward_pct | -0.0109 |
| total_trades | 88 |
| time/ | |
| fps | 553 |
| iterations | 12 |
| time_elapsed | 44 |
| total_timesteps | 24576 |
| train/ | |
| approx_kl | 0.0037694066 |
| clip_fraction | 0.000391 |
| clip_range | 0.2 |
| entropy_loss | -1.39 |
| explained_variance | -1.2e+12 |
| learning_rate | 0.0001 |
| loss | -0.00164 |
| n_updates | 110 |
| policy_gradient_loss | -0.000146 |
| std | 0.976 |
| value_loss | 7.25e-05 |
------------------------------------------
day: 95, episode: 1100
begin_total_asset: 100000.00
end_total_asset: 99991.02
total_reward: -8.98
total_cost: 4.13
total_trades: 71
Sharpe: -4.842
=================================
day: 95, episode: 1110
begin_total_asset: 100000.00
end_total_asset: 99985.71
total_reward: -14.29
total_cost: 4.77
total_trades: 84
Sharpe: -2.459
=================================
--------------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 4.36 |
| total_reward | -26.9 |
| total_reward_pct | -0.0269 |
| total_trades | 82 |
| time/ | |
| fps | 552 |
| iterations | 13 |
| time_elapsed | 48 |
| total_timesteps | 26624 |
| train/ | |
| approx_kl | -2.6669819e-05 |
| clip_fraction | 0 |
| clip_range | 0.2 |
| entropy_loss | -1.4 |
| explained_variance | -9.89e+12 |
| learning_rate | 0.0001 |
| loss | -0.00703 |
| n_updates | 120 |
| policy_gradient_loss | 0.000131 |
| std | 0.977 |
| value_loss | 3.67e-05 |
--------------------------------------------
day: 95, episode: 1120
begin_total_asset: 100000.00
end_total_asset: 99987.96
total_reward: -12.04
total_cost: 3.74
total_trades: 68
Sharpe: -3.097
=================================
day: 95, episode: 1130
begin_total_asset: 100000.00
end_total_asset: 99992.82
total_reward: -7.18
total_cost: 3.12
total_trades: 74
Sharpe: -3.589
=================================
-----------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 4.27 |
| total_reward | -19.3 |
| total_reward_pct | -0.0193 |
| total_trades | 77 |
| time/ | |
| fps | 551 |
| iterations | 14 |
| time_elapsed | 51 |
| total_timesteps | 28672 |
| train/ | |
| approx_kl | 0.003337428 |
| clip_fraction | 0.00869 |
| clip_range | 0.2 |
| entropy_loss | -1.39 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| loss | -0.0163 |
| n_updates | 130 |
| policy_gradient_loss | -0.000863 |
| std | 0.975 |
| value_loss | 1.75e-05 |
-----------------------------------------
day: 95, episode: 1140
begin_total_asset: 100000.00
end_total_asset: 99998.52
total_reward: -1.48
total_cost: 2.69
total_trades: 72
Sharpe: -0.632
=================================
day: 95, episode: 1150
begin_total_asset: 100000.00
end_total_asset: 99987.69
total_reward: -12.31
total_cost: 2.76
total_trades: 61
Sharpe: -2.808
=================================
-------------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 2.63 |
| total_reward | -7.46 |
| total_reward_pct | -0.00746 |
| total_trades | 67 |
| time/ | |
| fps | 551 |
| iterations | 15 |
| time_elapsed | 55 |
| total_timesteps | 30720 |
| train/ | |
| approx_kl | -0.0005038413 |
| clip_fraction | 0 |
| clip_range | 0.2 |
| entropy_loss | -1.39 |
| explained_variance | -9.72e+12 |
| learning_rate | 0.0001 |
| loss | -0.00525 |
| n_updates | 140 |
| policy_gradient_loss | 8.53e-05 |
| std | 0.974 |
| value_loss | 9.26e-06 |
-------------------------------------------
day: 95, episode: 1160
begin_total_asset: 100000.00
end_total_asset: 99985.86
total_reward: -14.14
total_cost: 5.09
total_trades: 88
Sharpe: -3.039
=================================
day: 95, episode: 1170
begin_total_asset: 100000.00
end_total_asset: 99966.04
total_reward: -33.96
total_cost: 2.95
total_trades: 68
Sharpe: -2.222
=================================
------------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 3.59 |
| total_reward | -10.5 |
| total_reward_pct | -0.0105 |
| total_trades | 72 |
| time/ | |
| fps | 551 |
| iterations | 16 |
| time_elapsed | 59 |
| total_timesteps | 32768 |
| train/ | |
| approx_kl | 0.0029460597 |
| clip_fraction | 0.0255 |
| clip_range | 0.2 |
| entropy_loss | -1.39 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| loss | -0.0189 |
| n_updates | 150 |
| policy_gradient_loss | -0.00239 |
| std | 0.97 |
| value_loss | 4.85e-06 |
------------------------------------------
day: 95, episode: 1180
begin_total_asset: 100000.00
end_total_asset: 99988.38
total_reward: -11.62
total_cost: 4.06
total_trades: 77
Sharpe: -3.213
=================================
day: 95, episode: 1190
begin_total_asset: 100000.00
end_total_asset: 99973.79
total_reward: -26.21
total_cost: 3.58
total_trades: 82
Sharpe: -3.590
=================================
------------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 2.54 |
| total_reward | -3.78 |
| total_reward_pct | -0.00378 |
| total_trades | 65 |
| time/ | |
| fps | 551 |
| iterations | 17 |
| time_elapsed | 63 |
| total_timesteps | 34816 |
| train/ | |
| approx_kl | 0.0023583206 |
| clip_fraction | 0.00625 |
| clip_range | 0.2 |
| entropy_loss | -1.39 |
| explained_variance | -1.18e+12 |
| learning_rate | 0.0001 |
| loss | -0.0109 |
| n_updates | 160 |
| policy_gradient_loss | -0.000811 |
| std | 0.969 |
| value_loss | 2.43e-06 |
------------------------------------------
day: 95, episode: 1200
begin_total_asset: 100000.00
end_total_asset: 99986.77
total_reward: -13.23
total_cost: 3.70
total_trades: 67
Sharpe: -3.481
=================================
day: 95, episode: 1210
begin_total_asset: 100000.00
end_total_asset: 99992.52
total_reward: -7.48
total_cost: 2.62
total_trades: 60
Sharpe: -5.133
=================================
day: 95, episode: 1220
begin_total_asset: 100000.00
end_total_asset: 99991.12
total_reward: -8.88
total_cost: 2.74
total_trades: 63
Sharpe: -3.260
=================================
------------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 2.74 |
| total_reward | -8.88 |
| total_reward_pct | -0.00888 |
| total_trades | 63 |
| time/ | |
| fps | 551 |
| iterations | 18 |
| time_elapsed | 66 |
| total_timesteps | 36864 |
| train/ | |
| approx_kl | 0.0048893923 |
| clip_fraction | 0.0297 |
| clip_range | 0.2 |
| entropy_loss | -1.39 |
| explained_variance | -5.13e+12 |
| learning_rate | 0.0001 |
| loss | -0.0123 |
| n_updates | 170 |
| policy_gradient_loss | -0.00292 |
| std | 0.965 |
| value_loss | 1.17e-06 |
------------------------------------------
day: 95, episode: 1230
begin_total_asset: 100000.00
end_total_asset: 99995.20
total_reward: -4.80
total_cost: 1.40
total_trades: 45
Sharpe: -4.916
=================================
day: 95, episode: 1240
begin_total_asset: 100000.00
end_total_asset: 99996.75
total_reward: -3.25
total_cost: 2.59
total_trades: 67
Sharpe: -2.646
=================================
------------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 2.01 |
| total_reward | -4.14 |
| total_reward_pct | -0.00414 |
| total_trades | 48 |
| time/ | |
| fps | 551 |
| iterations | 19 |
| time_elapsed | 70 |
| total_timesteps | 38912 |
| train/ | |
| approx_kl | 0.0012286699 |
| clip_fraction | 0.0043 |
| clip_range | 0.2 |
| entropy_loss | -1.38 |
| explained_variance | -2.24e+12 |
| learning_rate | 0.0001 |
| loss | -0.0154 |
| n_updates | 180 |
| policy_gradient_loss | -0.000265 |
| std | 0.962 |
| value_loss | 5.29e-07 |
------------------------------------------
day: 95, episode: 1250
begin_total_asset: 100000.00
end_total_asset: 99995.18
total_reward: -4.82
total_cost: 2.32
total_trades: 56
Sharpe: -2.260
=================================
day: 95, episode: 1260
begin_total_asset: 100000.00
end_total_asset: 99992.33
total_reward: -7.67
total_cost: 2.39
total_trades: 59
Sharpe: -3.407
=================================
--------------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 2.29 |
| total_reward | -8.45 |
| total_reward_pct | -0.00845 |
| total_trades | 60 |
| time/ | |
| fps | 551 |
| iterations | 20 |
| time_elapsed | 74 |
| total_timesteps | 40960 |
| train/ | |
| approx_kl | -0.00018270142 |
| clip_fraction | 0 |
| clip_range | 0.2 |
| entropy_loss | -1.38 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| loss | -0.00257 |
| n_updates | 190 |
| policy_gradient_loss | -0.000276 |
| std | 0.952 |
| value_loss | 3e-07 |
--------------------------------------------
day: 95, episode: 1270
begin_total_asset: 100000.00
end_total_asset: 99994.97
total_reward: -5.03
total_cost: 1.23
total_trades: 40
Sharpe: -2.775
=================================
day: 95, episode: 1280
begin_total_asset: 100000.00
end_total_asset: 99993.20
total_reward: -6.80
total_cost: 1.95
total_trades: 55
Sharpe: -2.885
=================================
------------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 2.54 |
| total_reward | -6.84 |
| total_reward_pct | -0.00684 |
| total_trades | 57 |
| time/ | |
| fps | 551 |
| iterations | 21 |
| time_elapsed | 77 |
| total_timesteps | 43008 |
| train/ | |
| approx_kl | 0.0057166344 |
| clip_fraction | 0.0174 |
| clip_range | 0.2 |
| entropy_loss | -1.37 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| loss | -0.0123 |
| n_updates | 200 |
| policy_gradient_loss | -0.00171 |
| std | 0.95 |
| value_loss | 1.77e-07 |
------------------------------------------
day: 95, episode: 1290
begin_total_asset: 100000.00
end_total_asset: 99992.35
total_reward: -7.65
total_cost: 1.78
total_trades: 48
Sharpe: -2.718
=================================
day: 95, episode: 1300
begin_total_asset: 100000.00
end_total_asset: 99998.21
total_reward: -1.79
total_cost: 0.91
total_trades: 41
Sharpe: -6.416
=================================
------------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 1.67 |
| total_reward | -3.17 |
| total_reward_pct | -0.00317 |
| total_trades | 46 |
| time/ | |
| fps | 551 |
| iterations | 22 |
| time_elapsed | 81 |
| total_timesteps | 45056 |
| train/ | |
| approx_kl | 0.0034110933 |
| clip_fraction | 0.0342 |
| clip_range | 0.2 |
| entropy_loss | -1.37 |
| explained_variance | -1.83e+12 |
| learning_rate | 0.0001 |
| loss | -0.0132 |
| n_updates | 210 |
| policy_gradient_loss | -0.0033 |
| std | 0.95 |
| value_loss | 1.05e-07 |
------------------------------------------
day: 95, episode: 1310
begin_total_asset: 100000.00
end_total_asset: 99994.41
total_reward: -5.59
total_cost: 1.51
total_trades: 55
Sharpe: -3.218
=================================
day: 95, episode: 1320
begin_total_asset: 100000.00
end_total_asset: 99994.92
total_reward: -5.08
total_cost: 1.95
total_trades: 50
Sharpe: -4.223
=================================
-----------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 1.17 |
| total_reward | -2.67 |
| total_reward_pct | -0.00267 |
| total_trades | 38 |
| time/ | |
| fps | 551 |
| iterations | 23 |
| time_elapsed | 85 |
| total_timesteps | 47104 |
| train/ | |
| approx_kl | 0.006719809 |
| clip_fraction | 0.0373 |
| clip_range | 0.2 |
| entropy_loss | -1.36 |
| explained_variance | -1.16e+12 |
| learning_rate | 0.0001 |
| loss | -0.00302 |
| n_updates | 220 |
| policy_gradient_loss | -0.0038 |
| std | 0.945 |
| value_loss | 6.6e-08 |
-----------------------------------------
day: 95, episode: 1330
begin_total_asset: 100000.00
end_total_asset: 99998.60
total_reward: -1.40
total_cost: 0.72
total_trades: 29
Sharpe: -3.642
=================================
day: 95, episode: 1340
begin_total_asset: 100000.00
end_total_asset: 99992.86
total_reward: -7.14
total_cost: 1.78
total_trades: 49
Sharpe: -2.948
=================================
----------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0.476 |
| total_reward | -1.06 |
| total_reward_pct | -0.00106 |
| total_trades | 21 |
| time/ | |
| fps | 551 |
| iterations | 24 |
| time_elapsed | 89 |
| total_timesteps | 49152 |
| train/ | |
| approx_kl | 0.00423476 |
| clip_fraction | 0.0323 |
| clip_range | 0.2 |
| entropy_loss | -1.36 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| loss | -0.0165 |
| n_updates | 230 |
| policy_gradient_loss | -0.00265 |
| std | 0.942 |
| value_loss | 3.45e-08 |
----------------------------------------
day: 95, episode: 1350
begin_total_asset: 100000.00
end_total_asset: 99997.58
total_reward: -2.42
total_cost: 0.73
total_trades: 27
Sharpe: -3.336
=================================
day: 95, episode: 1360
begin_total_asset: 100000.00
end_total_asset: 99999.53
total_reward: -0.47
total_cost: 0.58
total_trades: 27
Sharpe: -0.694
=================================
-----------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 1.15 |
| total_reward | -0.131 |
| total_reward_pct | -0.000131 |
| total_trades | 32 |
| time/ | |
| fps | 551 |
| iterations | 25 |
| time_elapsed | 92 |
| total_timesteps | 51200 |
| train/ | |
| approx_kl | 0.010400049 |
| clip_fraction | 0.0487 |
| clip_range | 0.2 |
| entropy_loss | -1.35 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| loss | -0.0159 |
| n_updates | 240 |
| policy_gradient_loss | -0.00463 |
| std | 0.934 |
| value_loss | 3.12e-08 |
-----------------------------------------
day: 95, episode: 1370
begin_total_asset: 100000.00
end_total_asset: 100000.64
total_reward: 0.64
total_cost: 0.71
total_trades: 28
Sharpe: 0.873
=================================
day: 95, episode: 1380
begin_total_asset: 100000.00
end_total_asset: 99998.83
total_reward: -1.17
total_cost: 0.55
total_trades: 26
Sharpe: -4.570
=================================
day: 95, episode: 1390
begin_total_asset: 100000.00
end_total_asset: 99997.17
total_reward: -2.83
total_cost: 0.98
total_trades: 34
Sharpe: -2.202
=================================
------------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0.984 |
| total_reward | -2.83 |
| total_reward_pct | -0.00283 |
| total_trades | 34 |
| time/ | |
| fps | 550 |
| iterations | 26 |
| time_elapsed | 96 |
| total_timesteps | 53248 |
| train/ | |
| approx_kl | 0.0020846785 |
| clip_fraction | 0.0177 |
| clip_range | 0.2 |
| entropy_loss | -1.35 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| loss | -0.00845 |
| n_updates | 250 |
| policy_gradient_loss | -0.00246 |
| std | 0.932 |
| value_loss | 1.77e-08 |
------------------------------------------
day: 95, episode: 1400
begin_total_asset: 100000.00
end_total_asset: 99997.80
total_reward: -2.20
total_cost: 0.59
total_trades: 29
Sharpe: -3.421
=================================
day: 95, episode: 1410
begin_total_asset: 100000.00
end_total_asset: 99999.00
total_reward: -1.00
total_cost: 0.90
total_trades: 26
Sharpe: -2.855
=================================
------------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0.531 |
| total_reward | -0.406 |
| total_reward_pct | -0.000406 |
| total_trades | 17 |
| time/ | |
| fps | 550 |
| iterations | 27 |
| time_elapsed | 100 |
| total_timesteps | 55296 |
| train/ | |
| approx_kl | 0.0024183406 |
| clip_fraction | 0.000977 |
| clip_range | 0.2 |
| entropy_loss | -1.35 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| loss | -0.0106 |
| n_updates | 260 |
| policy_gradient_loss | -0.000385 |
| std | 0.928 |
| value_loss | 1.08e-08 |
------------------------------------------
day: 95, episode: 1420
begin_total_asset: 100000.00
end_total_asset: 99997.41
total_reward: -2.59
total_cost: 0.82
total_trades: 34
Sharpe: -6.085
=================================
day: 95, episode: 1430
begin_total_asset: 100000.00
end_total_asset: 99998.18
total_reward: -1.82
total_cost: 0.89
total_trades: 37
Sharpe: -7.295
=================================
------------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0.996 |
| total_reward | -4.01 |
| total_reward_pct | -0.00401 |
| total_trades | 28 |
| time/ | |
| fps | 550 |
| iterations | 28 |
| time_elapsed | 104 |
| total_timesteps | 57344 |
| train/ | |
| approx_kl | 0.0038041172 |
| clip_fraction | 0.0484 |
| clip_range | 0.2 |
| entropy_loss | -1.34 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| loss | -0.0086 |
| n_updates | 270 |
| policy_gradient_loss | -0.00619 |
| std | 0.921 |
| value_loss | 6.73e-09 |
------------------------------------------
day: 95, episode: 1440
begin_total_asset: 100000.00
end_total_asset: 99998.50
total_reward: -1.50
total_cost: 0.53
total_trades: 21
Sharpe: -3.413
=================================
day: 95, episode: 1450
begin_total_asset: 100000.00
end_total_asset: 99998.58
total_reward: -1.42
total_cost: 0.66
total_trades: 22
Sharpe: -5.829
=================================
------------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0.333 |
| total_reward | -0.736 |
| total_reward_pct | -0.000736 |
| total_trades | 15 |
| time/ | |
| fps | 550 |
| iterations | 29 |
| time_elapsed | 107 |
| total_timesteps | 59392 |
| train/ | |
| approx_kl | 0.0047127856 |
| clip_fraction | 0.024 |
| clip_range | 0.2 |
| entropy_loss | -1.33 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| loss | -0.0191 |
| n_updates | 280 |
| policy_gradient_loss | -0.00263 |
| std | 0.913 |
| value_loss | 4.62e-09 |
------------------------------------------
day: 95, episode: 1460
begin_total_asset: 100000.00
end_total_asset: 99999.07
total_reward: -0.93
total_cost: 0.50
total_trades: 25
Sharpe: -4.974
=================================
day: 95, episode: 1470
begin_total_asset: 100000.00
end_total_asset: 100000.21
total_reward: 0.21
total_cost: 0.55
total_trades: 18
Sharpe: 0.346
=================================
-----------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0.39 |
| total_reward | -1.34 |
| total_reward_pct | -0.00134 |
| total_trades | 18 |
| time/ | |
| fps | 550 |
| iterations | 30 |
| time_elapsed | 111 |
| total_timesteps | 61440 |
| train/ | |
| approx_kl | 0.002827107 |
| clip_fraction | 0.0385 |
| clip_range | 0.2 |
| entropy_loss | -1.32 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| loss | -0.0189 |
| n_updates | 290 |
| policy_gradient_loss | -0.00497 |
| std | 0.903 |
| value_loss | 2.24e-09 |
-----------------------------------------
day: 95, episode: 1480
begin_total_asset: 100000.00
end_total_asset: 99998.97
total_reward: -1.03
total_cost: 0.50
total_trades: 13
Sharpe: -5.789
=================================
day: 95, episode: 1490
begin_total_asset: 100000.00
end_total_asset: 99997.54
total_reward: -2.46
total_cost: 0.58
total_trades: 16
Sharpe: -2.881
=================================
------------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0.247 |
| total_reward | -0.481 |
| total_reward_pct | -0.000481 |
| total_trades | 13 |
| time/ | |
| fps | 549 |
| iterations | 31 |
| time_elapsed | 115 |
| total_timesteps | 63488 |
| train/ | |
| approx_kl | 0.0064605344 |
| clip_fraction | 0.043 |
| clip_range | 0.2 |
| entropy_loss | -1.31 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| loss | -0.00502 |
| n_updates | 300 |
| policy_gradient_loss | -0.00415 |
| std | 0.898 |
| value_loss | 2.2e-09 |
------------------------------------------
day: 95, episode: 1500
begin_total_asset: 100000.00
end_total_asset: 100000.29
total_reward: 0.29
total_cost: 0.12
total_trades: 6
Sharpe: 0.892
=================================
day: 95, episode: 1510
begin_total_asset: 100000.00
end_total_asset: 99999.83
total_reward: -0.17
total_cost: 0.09
total_trades: 4
Sharpe: -3.079
=================================
------------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0.189 |
| total_reward | -0.293 |
| total_reward_pct | -0.000293 |
| total_trades | 11 |
| time/ | |
| fps | 549 |
| iterations | 32 |
| time_elapsed | 119 |
| total_timesteps | 65536 |
| train/ | |
| approx_kl | 0.0033582607 |
| clip_fraction | 0.0414 |
| clip_range | 0.2 |
| entropy_loss | -1.31 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| loss | -0.00259 |
| n_updates | 310 |
| policy_gradient_loss | -0.00496 |
| std | 0.894 |
| value_loss | 1.42e-09 |
------------------------------------------
day: 95, episode: 1520
begin_total_asset: 100000.00
end_total_asset: 99999.80
total_reward: -0.20
total_cost: 0.10
total_trades: 8
Sharpe: -3.012
=================================
day: 95, episode: 1530
begin_total_asset: 100000.00
end_total_asset: 99999.60
total_reward: -0.40
total_cost: 0.15
total_trades: 6
Sharpe: -2.984
=================================
day: 95, episode: 1540
begin_total_asset: 100000.00
end_total_asset: 99999.81
total_reward: -0.19
total_cost: 0.21
total_trades: 10
Sharpe: -2.087
=================================
----------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0.21 |
| total_reward | -0.191 |
| total_reward_pct | -0.000191 |
| total_trades | 10 |
| time/ | |
| fps | 549 |
| iterations | 33 |
| time_elapsed | 122 |
| total_timesteps | 67584 |
| train/ | |
| approx_kl | 0.00434699 |
| clip_fraction | 0.0142 |
| clip_range | 0.2 |
| entropy_loss | -1.3 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| loss | -0.0168 |
| n_updates | 320 |
| policy_gradient_loss | -0.00214 |
| std | 0.89 |
| value_loss | 1.02e-09 |
----------------------------------------
day: 95, episode: 1550
begin_total_asset: 100000.00
end_total_asset: 99999.14
total_reward: -0.86
total_cost: 0.18
total_trades: 5
Sharpe: -2.160
=================================
day: 95, episode: 1560
begin_total_asset: 100000.00
end_total_asset: 99999.95
total_reward: -0.05
total_cost: 0.04
total_trades: 6
Sharpe: -2.474
=================================
-------------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0.157 |
| total_reward | -0.272 |
| total_reward_pct | -0.000272 |
| total_trades | 6 |
| time/ | |
| fps | 549 |
| iterations | 34 |
| time_elapsed | 126 |
| total_timesteps | 69632 |
| train/ | |
| approx_kl | -0.0001876431 |
| clip_fraction | 0.0113 |
| clip_range | 0.2 |
| entropy_loss | -1.3 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| loss | -0.00735 |
| n_updates | 330 |
| policy_gradient_loss | -0.00273 |
| std | 0.882 |
| value_loss | 2.28e-10 |
-------------------------------------------
day: 95, episode: 1570
begin_total_asset: 100000.00
end_total_asset: 99999.83
total_reward: -0.17
total_cost: 0.08
total_trades: 6
Sharpe: -3.297
=================================
day: 95, episode: 1580
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
-----------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0.278 |
| total_reward | -0.596 |
| total_reward_pct | -0.000596 |
| total_trades | 10 |
| time/ | |
| fps | 549 |
| iterations | 35 |
| time_elapsed | 130 |
| total_timesteps | 71680 |
| train/ | |
| approx_kl | 0.004608955 |
| clip_fraction | 0.0309 |
| clip_range | 0.2 |
| entropy_loss | -1.29 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| loss | -0.00139 |
| n_updates | 340 |
| policy_gradient_loss | -0.00505 |
| std | 0.878 |
| value_loss | 4.65e-10 |
-----------------------------------------
day: 95, episode: 1590
begin_total_asset: 100000.00
end_total_asset: 99999.93
total_reward: -0.07
total_cost: 0.05
total_trades: 2
Sharpe: -2.015
=================================
day: 95, episode: 1600
begin_total_asset: 100000.00
end_total_asset: 99999.78
total_reward: -0.22
total_cost: 0.09
total_trades: 10
Sharpe: -4.846
=================================
-------------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0.0697 |
| total_reward | -0.147 |
| total_reward_pct | -0.000147 |
| total_trades | 6 |
| time/ | |
| fps | 548 |
| iterations | 36 |
| time_elapsed | 134 |
| total_timesteps | 73728 |
| train/ | |
| approx_kl | 0.00093996205 |
| clip_fraction | 0.018 |
| clip_range | 0.2 |
| entropy_loss | -1.28 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| loss | -0.00417 |
| n_updates | 350 |
| policy_gradient_loss | -0.00322 |
| std | 0.872 |
| value_loss | 1.1e-10 |
-------------------------------------------
day: 95, episode: 1610
begin_total_asset: 100000.00
end_total_asset: 99999.87
total_reward: -0.13
total_cost: 0.05
total_trades: 2
Sharpe: -2.274
=================================
day: 95, episode: 1620
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
-------------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0.0422 |
| total_reward | -0.05 |
| total_reward_pct | -5e-05 |
| total_trades | 2 |
| time/ | |
| fps | 547 |
| iterations | 37 |
| time_elapsed | 138 |
| total_timesteps | 75776 |
| train/ | |
| approx_kl | 0.00056804926 |
| clip_fraction | 0 |
| clip_range | 0.2 |
| entropy_loss | -1.27 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| loss | -0.00859 |
| n_updates | 360 |
| policy_gradient_loss | -0.0008 |
| std | 0.86 |
| value_loss | 2.78e-10 |
-------------------------------------------
day: 95, episode: 1630
begin_total_asset: 100000.00
end_total_asset: 99999.45
total_reward: -0.55
total_cost: 0.10
total_trades: 4
Sharpe: -1.716
=================================
day: 95, episode: 1640
begin_total_asset: 100000.00
end_total_asset: 99999.97
total_reward: -0.03
total_cost: 0.02
total_trades: 2
Sharpe: -2.275
=================================
-----------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| fps | 547 |
| iterations | 38 |
| time_elapsed | 142 |
| total_timesteps | 77824 |
| train/ | |
| approx_kl | 0.002477115 |
| clip_fraction | 0.0457 |
| clip_range | 0.2 |
| entropy_loss | -1.27 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| loss | -0.0185 |
| n_updates | 370 |
| policy_gradient_loss | -0.00524 |
| std | 0.857 |
| value_loss | 1.85e-10 |
-----------------------------------------
day: 95, episode: 1650
begin_total_asset: 100000.00
end_total_asset: 99999.98
total_reward: -0.02
total_cost: 0.12
total_trades: 9
Sharpe: -0.168
=================================
day: 95, episode: 1660
begin_total_asset: 100000.00
end_total_asset: 99999.90
total_reward: -0.10
total_cost: 0.04
total_trades: 2
Sharpe: -2.293
=================================
-----------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0.152 |
| total_reward | -0.339 |
| total_reward_pct | -0.000339 |
| total_trades | 4 |
| time/ | |
| fps | 547 |
| iterations | 39 |
| time_elapsed | 145 |
| total_timesteps | 79872 |
| train/ | |
| approx_kl | 0.005646224 |
| clip_fraction | 0.0405 |
| clip_range | 0.2 |
| entropy_loss | -1.26 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| loss | -0.00841 |
| n_updates | 380 |
| policy_gradient_loss | -0.005 |
| std | 0.855 |
| value_loss | 8.29e-11 |
-----------------------------------------
day: 95, episode: 1670
begin_total_asset: 100000.00
end_total_asset: 99999.97
total_reward: -0.03
total_cost: 0.01
total_trades: 2
Sharpe: -2.300
=================================
day: 95, episode: 1680
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
------------------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0.0424 |
| total_reward | -0.0884 |
| total_reward_pct | -8.84e-05 |
| total_trades | 6 |
| time/ | |
| fps | 547 |
| iterations | 40 |
| time_elapsed | 149 |
| total_timesteps | 81920 |
| train/ | |
| approx_kl | 0.0029767775 |
| clip_fraction | 0.00459 |
| clip_range | 0.2 |
| entropy_loss | -1.26 |
| explained_variance | nan |
| learning_rate | 0.0001 |
| loss | -0.00694 |
| n_updates | 390 |
| policy_gradient_loss | -0.00182 |
| std | 0.851 |
| value_loss | 3.17e-10 |
------------------------------------------
###Markdown
Model 4: TD3
###Code
agent = DRLAgent(env = env_train)
TD3_PARAMS = {"batch_size": 128,
"buffer_size": 1000000,
"learning_rate": 0.0003}
model_td3 = agent.get_model("td3",model_kwargs = TD3_PARAMS)
trained_td3 = agent.train_model(model=model_td3,
tb_log_name='td3',
total_timesteps=30000)
###Output
Logging to tensorboard_log/td3/td3_1
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 4 |
| fps | 94 |
| time_elapsed | 4 |
| total timesteps | 384 |
| train/ | |
| actor_loss | 3.57e+03 |
| critic_loss | 2.97e+05 |
| learning_rate | 0.0003 |
| n_updates | 192 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 8 |
| fps | 65 |
| time_elapsed | 11 |
| total timesteps | 768 |
| train/ | |
| actor_loss | 3.51e+03 |
| critic_loss | 2.73e+05 |
| learning_rate | 0.0003 |
| n_updates | 576 |
----------------------------------
day: 95, episode: 1700
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 12 |
| fps | 59 |
| time_elapsed | 19 |
| total timesteps | 1152 |
| train/ | |
| actor_loss | 3.44e+03 |
| critic_loss | 2.53e+05 |
| learning_rate | 0.0003 |
| n_updates | 960 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 16 |
| fps | 56 |
| time_elapsed | 27 |
| total timesteps | 1536 |
| train/ | |
| actor_loss | 3.37e+03 |
| critic_loss | 2.5e+05 |
| learning_rate | 0.0003 |
| n_updates | 1344 |
----------------------------------
day: 95, episode: 1710
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 20 |
| fps | 54 |
| time_elapsed | 35 |
| total timesteps | 1920 |
| train/ | |
| actor_loss | 3.3e+03 |
| critic_loss | 2.6e+05 |
| learning_rate | 0.0003 |
| n_updates | 1728 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 24 |
| fps | 53 |
| time_elapsed | 42 |
| total timesteps | 2304 |
| train/ | |
| actor_loss | 3.24e+03 |
| critic_loss | 2.88e+05 |
| learning_rate | 0.0003 |
| n_updates | 2112 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 28 |
| fps | 53 |
| time_elapsed | 50 |
| total timesteps | 2688 |
| train/ | |
| actor_loss | 3.17e+03 |
| critic_loss | 2.55e+05 |
| learning_rate | 0.0003 |
| n_updates | 2496 |
----------------------------------
day: 95, episode: 1720
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 32 |
| fps | 52 |
| time_elapsed | 58 |
| total timesteps | 3072 |
| train/ | |
| actor_loss | 3.09e+03 |
| critic_loss | 2.49e+05 |
| learning_rate | 0.0003 |
| n_updates | 2880 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 36 |
| fps | 52 |
| time_elapsed | 66 |
| total timesteps | 3456 |
| train/ | |
| actor_loss | 3.03e+03 |
| critic_loss | 2.14e+05 |
| learning_rate | 0.0003 |
| n_updates | 3264 |
----------------------------------
day: 95, episode: 1730
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 40 |
| fps | 51 |
| time_elapsed | 73 |
| total timesteps | 3840 |
| train/ | |
| actor_loss | 2.98e+03 |
| critic_loss | 2.28e+05 |
| learning_rate | 0.0003 |
| n_updates | 3648 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 44 |
| fps | 51 |
| time_elapsed | 81 |
| total timesteps | 4224 |
| train/ | |
| actor_loss | 2.92e+03 |
| critic_loss | 1.96e+05 |
| learning_rate | 0.0003 |
| n_updates | 4032 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 48 |
| fps | 51 |
| time_elapsed | 89 |
| total timesteps | 4608 |
| train/ | |
| actor_loss | 2.86e+03 |
| critic_loss | 1.83e+05 |
| learning_rate | 0.0003 |
| n_updates | 4416 |
----------------------------------
day: 95, episode: 1740
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 52 |
| fps | 51 |
| time_elapsed | 97 |
| total timesteps | 4992 |
| train/ | |
| actor_loss | 2.81e+03 |
| critic_loss | 1.9e+05 |
| learning_rate | 0.0003 |
| n_updates | 4800 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 56 |
| fps | 51 |
| time_elapsed | 105 |
| total timesteps | 5376 |
| train/ | |
| actor_loss | 2.76e+03 |
| critic_loss | 1.75e+05 |
| learning_rate | 0.0003 |
| n_updates | 5184 |
----------------------------------
day: 95, episode: 1750
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 60 |
| fps | 51 |
| time_elapsed | 112 |
| total timesteps | 5760 |
| train/ | |
| actor_loss | 2.7e+03 |
| critic_loss | 1.87e+05 |
| learning_rate | 0.0003 |
| n_updates | 5568 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 64 |
| fps | 50 |
| time_elapsed | 120 |
| total timesteps | 6144 |
| train/ | |
| actor_loss | 2.65e+03 |
| critic_loss | 1.88e+05 |
| learning_rate | 0.0003 |
| n_updates | 5952 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 68 |
| fps | 50 |
| time_elapsed | 128 |
| total timesteps | 6528 |
| train/ | |
| actor_loss | 2.6e+03 |
| critic_loss | 1.6e+05 |
| learning_rate | 0.0003 |
| n_updates | 6336 |
----------------------------------
day: 95, episode: 1760
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 72 |
| fps | 50 |
| time_elapsed | 136 |
| total timesteps | 6912 |
| train/ | |
| actor_loss | 2.56e+03 |
| critic_loss | 1.52e+05 |
| learning_rate | 0.0003 |
| n_updates | 6720 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 76 |
| fps | 50 |
| time_elapsed | 144 |
| total timesteps | 7296 |
| train/ | |
| actor_loss | 2.52e+03 |
| critic_loss | 1.48e+05 |
| learning_rate | 0.0003 |
| n_updates | 7104 |
----------------------------------
day: 95, episode: 1770
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 80 |
| fps | 50 |
| time_elapsed | 152 |
| total timesteps | 7680 |
| train/ | |
| actor_loss | 2.47e+03 |
| critic_loss | 1.29e+05 |
| learning_rate | 0.0003 |
| n_updates | 7488 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 84 |
| fps | 50 |
| time_elapsed | 160 |
| total timesteps | 8064 |
| train/ | |
| actor_loss | 2.43e+03 |
| critic_loss | 1.34e+05 |
| learning_rate | 0.0003 |
| n_updates | 7872 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 88 |
| fps | 50 |
| time_elapsed | 167 |
| total timesteps | 8448 |
| train/ | |
| actor_loss | 2.39e+03 |
| critic_loss | 1.34e+05 |
| learning_rate | 0.0003 |
| n_updates | 8256 |
----------------------------------
day: 95, episode: 1780
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 92 |
| fps | 50 |
| time_elapsed | 175 |
| total timesteps | 8832 |
| train/ | |
| actor_loss | 2.35e+03 |
| critic_loss | 1.27e+05 |
| learning_rate | 0.0003 |
| n_updates | 8640 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 96 |
| fps | 50 |
| time_elapsed | 183 |
| total timesteps | 9216 |
| train/ | |
| actor_loss | 2.31e+03 |
| critic_loss | 1.14e+05 |
| learning_rate | 0.0003 |
| n_updates | 9024 |
----------------------------------
day: 95, episode: 1790
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 100 |
| fps | 50 |
| time_elapsed | 191 |
| total timesteps | 9600 |
| train/ | |
| actor_loss | 2.27e+03 |
| critic_loss | 1.24e+05 |
| learning_rate | 0.0003 |
| n_updates | 9408 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 104 |
| fps | 49 |
| time_elapsed | 199 |
| total timesteps | 9984 |
| train/ | |
| actor_loss | 2.24e+03 |
| critic_loss | 1.02e+05 |
| learning_rate | 0.0003 |
| n_updates | 9792 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 108 |
| fps | 49 |
| time_elapsed | 207 |
| total timesteps | 10368 |
| train/ | |
| actor_loss | 2.19e+03 |
| critic_loss | 1.1e+05 |
| learning_rate | 0.0003 |
| n_updates | 10176 |
----------------------------------
day: 95, episode: 1800
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 112 |
| fps | 49 |
| time_elapsed | 215 |
| total timesteps | 10752 |
| train/ | |
| actor_loss | 2.16e+03 |
| critic_loss | 9.11e+04 |
| learning_rate | 0.0003 |
| n_updates | 10560 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 116 |
| fps | 49 |
| time_elapsed | 224 |
| total timesteps | 11136 |
| train/ | |
| actor_loss | 2.12e+03 |
| critic_loss | 9.13e+04 |
| learning_rate | 0.0003 |
| n_updates | 10944 |
----------------------------------
day: 95, episode: 1810
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 120 |
| fps | 49 |
| time_elapsed | 232 |
| total timesteps | 11520 |
| train/ | |
| actor_loss | 2.09e+03 |
| critic_loss | 9.44e+04 |
| learning_rate | 0.0003 |
| n_updates | 11328 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 124 |
| fps | 49 |
| time_elapsed | 240 |
| total timesteps | 11904 |
| train/ | |
| actor_loss | 2.06e+03 |
| critic_loss | 8.77e+04 |
| learning_rate | 0.0003 |
| n_updates | 11712 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 128 |
| fps | 49 |
| time_elapsed | 248 |
| total timesteps | 12288 |
| train/ | |
| actor_loss | 2.02e+03 |
| critic_loss | 9.28e+04 |
| learning_rate | 0.0003 |
| n_updates | 12096 |
----------------------------------
day: 95, episode: 1820
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 132 |
| fps | 49 |
| time_elapsed | 256 |
| total timesteps | 12672 |
| train/ | |
| actor_loss | 1.98e+03 |
| critic_loss | 9.64e+04 |
| learning_rate | 0.0003 |
| n_updates | 12480 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 136 |
| fps | 49 |
| time_elapsed | 264 |
| total timesteps | 13056 |
| train/ | |
| actor_loss | 1.95e+03 |
| critic_loss | 8.71e+04 |
| learning_rate | 0.0003 |
| n_updates | 12864 |
----------------------------------
day: 95, episode: 1830
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 140 |
| fps | 49 |
| time_elapsed | 273 |
| total timesteps | 13440 |
| train/ | |
| actor_loss | 1.92e+03 |
| critic_loss | 9.26e+04 |
| learning_rate | 0.0003 |
| n_updates | 13248 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 144 |
| fps | 49 |
| time_elapsed | 281 |
| total timesteps | 13824 |
| train/ | |
| actor_loss | 1.89e+03 |
| critic_loss | 7.22e+04 |
| learning_rate | 0.0003 |
| n_updates | 13632 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 148 |
| fps | 49 |
| time_elapsed | 289 |
| total timesteps | 14208 |
| train/ | |
| actor_loss | 1.85e+03 |
| critic_loss | 9.14e+04 |
| learning_rate | 0.0003 |
| n_updates | 14016 |
----------------------------------
day: 95, episode: 1840
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 152 |
| fps | 48 |
| time_elapsed | 298 |
| total timesteps | 14592 |
| train/ | |
| actor_loss | 1.82e+03 |
| critic_loss | 7.12e+04 |
| learning_rate | 0.0003 |
| n_updates | 14400 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 156 |
| fps | 48 |
| time_elapsed | 306 |
| total timesteps | 14976 |
| train/ | |
| actor_loss | 1.8e+03 |
| critic_loss | 7.55e+04 |
| learning_rate | 0.0003 |
| n_updates | 14784 |
----------------------------------
day: 95, episode: 1850
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 160 |
| fps | 48 |
| time_elapsed | 315 |
| total timesteps | 15360 |
| train/ | |
| actor_loss | 1.76e+03 |
| critic_loss | 7.16e+04 |
| learning_rate | 0.0003 |
| n_updates | 15168 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 164 |
| fps | 48 |
| time_elapsed | 323 |
| total timesteps | 15744 |
| train/ | |
| actor_loss | 1.74e+03 |
| critic_loss | 6.38e+04 |
| learning_rate | 0.0003 |
| n_updates | 15552 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 168 |
| fps | 48 |
| time_elapsed | 331 |
| total timesteps | 16128 |
| train/ | |
| actor_loss | 1.71e+03 |
| critic_loss | 5.69e+04 |
| learning_rate | 0.0003 |
| n_updates | 15936 |
----------------------------------
day: 95, episode: 1860
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 172 |
| fps | 48 |
| time_elapsed | 340 |
| total timesteps | 16512 |
| train/ | |
| actor_loss | 1.69e+03 |
| critic_loss | 6.05e+04 |
| learning_rate | 0.0003 |
| n_updates | 16320 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 176 |
| fps | 48 |
| time_elapsed | 348 |
| total timesteps | 16896 |
| train/ | |
| actor_loss | 1.66e+03 |
| critic_loss | 5.7e+04 |
| learning_rate | 0.0003 |
| n_updates | 16704 |
----------------------------------
day: 95, episode: 1870
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 180 |
| fps | 48 |
| time_elapsed | 356 |
| total timesteps | 17280 |
| train/ | |
| actor_loss | 1.63e+03 |
| critic_loss | 5.65e+04 |
| learning_rate | 0.0003 |
| n_updates | 17088 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 184 |
| fps | 48 |
| time_elapsed | 365 |
| total timesteps | 17664 |
| train/ | |
| actor_loss | 1.6e+03 |
| critic_loss | 5.43e+04 |
| learning_rate | 0.0003 |
| n_updates | 17472 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 188 |
| fps | 48 |
| time_elapsed | 374 |
| total timesteps | 18048 |
| train/ | |
| actor_loss | 1.58e+03 |
| critic_loss | 4.34e+04 |
| learning_rate | 0.0003 |
| n_updates | 17856 |
----------------------------------
day: 95, episode: 1880
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 192 |
| fps | 48 |
| time_elapsed | 382 |
| total timesteps | 18432 |
| train/ | |
| actor_loss | 1.55e+03 |
| critic_loss | 5.04e+04 |
| learning_rate | 0.0003 |
| n_updates | 18240 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 196 |
| fps | 48 |
| time_elapsed | 391 |
| total timesteps | 18816 |
| train/ | |
| actor_loss | 1.53e+03 |
| critic_loss | 4.45e+04 |
| learning_rate | 0.0003 |
| n_updates | 18624 |
----------------------------------
day: 95, episode: 1890
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 200 |
| fps | 48 |
| time_elapsed | 399 |
| total timesteps | 19200 |
| train/ | |
| actor_loss | 1.5e+03 |
| critic_loss | 4.54e+04 |
| learning_rate | 0.0003 |
| n_updates | 19008 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 204 |
| fps | 47 |
| time_elapsed | 408 |
| total timesteps | 19584 |
| train/ | |
| actor_loss | 1.48e+03 |
| critic_loss | 4.97e+04 |
| learning_rate | 0.0003 |
| n_updates | 19392 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 208 |
| fps | 47 |
| time_elapsed | 416 |
| total timesteps | 19968 |
| train/ | |
| actor_loss | 1.45e+03 |
| critic_loss | 4.24e+04 |
| learning_rate | 0.0003 |
| n_updates | 19776 |
----------------------------------
day: 95, episode: 1900
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 212 |
| fps | 47 |
| time_elapsed | 424 |
| total timesteps | 20352 |
| train/ | |
| actor_loss | 1.43e+03 |
| critic_loss | 4.18e+04 |
| learning_rate | 0.0003 |
| n_updates | 20160 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 216 |
| fps | 47 |
| time_elapsed | 433 |
| total timesteps | 20736 |
| train/ | |
| actor_loss | 1.41e+03 |
| critic_loss | 4.12e+04 |
| learning_rate | 0.0003 |
| n_updates | 20544 |
----------------------------------
day: 95, episode: 1910
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 220 |
| fps | 47 |
| time_elapsed | 441 |
| total timesteps | 21120 |
| train/ | |
| actor_loss | 1.39e+03 |
| critic_loss | 3.9e+04 |
| learning_rate | 0.0003 |
| n_updates | 20928 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 224 |
| fps | 47 |
| time_elapsed | 449 |
| total timesteps | 21504 |
| train/ | |
| actor_loss | 1.37e+03 |
| critic_loss | 4.28e+04 |
| learning_rate | 0.0003 |
| n_updates | 21312 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 228 |
| fps | 47 |
| time_elapsed | 458 |
| total timesteps | 21888 |
| train/ | |
| actor_loss | 1.35e+03 |
| critic_loss | 3.74e+04 |
| learning_rate | 0.0003 |
| n_updates | 21696 |
----------------------------------
day: 95, episode: 1920
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 232 |
| fps | 47 |
| time_elapsed | 466 |
| total timesteps | 22272 |
| train/ | |
| actor_loss | 1.33e+03 |
| critic_loss | 3.72e+04 |
| learning_rate | 0.0003 |
| n_updates | 22080 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 236 |
| fps | 47 |
| time_elapsed | 474 |
| total timesteps | 22656 |
| train/ | |
| actor_loss | 1.3e+03 |
| critic_loss | 3.57e+04 |
| learning_rate | 0.0003 |
| n_updates | 22464 |
----------------------------------
day: 95, episode: 1930
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 240 |
| fps | 47 |
| time_elapsed | 483 |
| total timesteps | 23040 |
| train/ | |
| actor_loss | 1.28e+03 |
| critic_loss | 3.19e+04 |
| learning_rate | 0.0003 |
| n_updates | 22848 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 244 |
| fps | 47 |
| time_elapsed | 491 |
| total timesteps | 23424 |
| train/ | |
| actor_loss | 1.26e+03 |
| critic_loss | 3.1e+04 |
| learning_rate | 0.0003 |
| n_updates | 23232 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 248 |
| fps | 47 |
| time_elapsed | 499 |
| total timesteps | 23808 |
| train/ | |
| actor_loss | 1.24e+03 |
| critic_loss | 2.97e+04 |
| learning_rate | 0.0003 |
| n_updates | 23616 |
----------------------------------
day: 95, episode: 1940
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 252 |
| fps | 47 |
| time_elapsed | 508 |
| total timesteps | 24192 |
| train/ | |
| actor_loss | 1.21e+03 |
| critic_loss | 3.24e+04 |
| learning_rate | 0.0003 |
| n_updates | 24000 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 256 |
| fps | 47 |
| time_elapsed | 516 |
| total timesteps | 24576 |
| train/ | |
| actor_loss | 1.2e+03 |
| critic_loss | 2.98e+04 |
| learning_rate | 0.0003 |
| n_updates | 24384 |
----------------------------------
day: 95, episode: 1950
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 260 |
| fps | 47 |
| time_elapsed | 525 |
| total timesteps | 24960 |
| train/ | |
| actor_loss | 1.18e+03 |
| critic_loss | 2.87e+04 |
| learning_rate | 0.0003 |
| n_updates | 24768 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 264 |
| fps | 47 |
| time_elapsed | 533 |
| total timesteps | 25344 |
| train/ | |
| actor_loss | 1.16e+03 |
| critic_loss | 3.06e+04 |
| learning_rate | 0.0003 |
| n_updates | 25152 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 268 |
| fps | 47 |
| time_elapsed | 542 |
| total timesteps | 25728 |
| train/ | |
| actor_loss | 1.14e+03 |
| critic_loss | 2.66e+04 |
| learning_rate | 0.0003 |
| n_updates | 25536 |
----------------------------------
day: 95, episode: 1960
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 272 |
| fps | 47 |
| time_elapsed | 550 |
| total timesteps | 26112 |
| train/ | |
| actor_loss | 1.12e+03 |
| critic_loss | 2.55e+04 |
| learning_rate | 0.0003 |
| n_updates | 25920 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 276 |
| fps | 47 |
| time_elapsed | 559 |
| total timesteps | 26496 |
| train/ | |
| actor_loss | 1.1e+03 |
| critic_loss | 2.62e+04 |
| learning_rate | 0.0003 |
| n_updates | 26304 |
----------------------------------
day: 95, episode: 1970
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 280 |
| fps | 47 |
| time_elapsed | 567 |
| total timesteps | 26880 |
| train/ | |
| actor_loss | 1.09e+03 |
| critic_loss | 2.53e+04 |
| learning_rate | 0.0003 |
| n_updates | 26688 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 284 |
| fps | 47 |
| time_elapsed | 576 |
| total timesteps | 27264 |
| train/ | |
| actor_loss | 1.08e+03 |
| critic_loss | 2.42e+04 |
| learning_rate | 0.0003 |
| n_updates | 27072 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 288 |
| fps | 47 |
| time_elapsed | 584 |
| total timesteps | 27648 |
| train/ | |
| actor_loss | 1.06e+03 |
| critic_loss | 2.2e+04 |
| learning_rate | 0.0003 |
| n_updates | 27456 |
----------------------------------
day: 95, episode: 1980
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 292 |
| fps | 47 |
| time_elapsed | 593 |
| total timesteps | 28032 |
| train/ | |
| actor_loss | 1.04e+03 |
| critic_loss | 2.22e+04 |
| learning_rate | 0.0003 |
| n_updates | 27840 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 296 |
| fps | 47 |
| time_elapsed | 601 |
| total timesteps | 28416 |
| train/ | |
| actor_loss | 1.02e+03 |
| critic_loss | 2.21e+04 |
| learning_rate | 0.0003 |
| n_updates | 28224 |
----------------------------------
day: 95, episode: 1990
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 300 |
| fps | 47 |
| time_elapsed | 610 |
| total timesteps | 28800 |
| train/ | |
| actor_loss | 1.01e+03 |
| critic_loss | 2.06e+04 |
| learning_rate | 0.0003 |
| n_updates | 28608 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 304 |
| fps | 47 |
| time_elapsed | 618 |
| total timesteps | 29184 |
| train/ | |
| actor_loss | 991 |
| critic_loss | 2.45e+04 |
| learning_rate | 0.0003 |
| n_updates | 28992 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 308 |
| fps | 47 |
| time_elapsed | 627 |
| total timesteps | 29568 |
| train/ | |
| actor_loss | 978 |
| critic_loss | 2.15e+04 |
| learning_rate | 0.0003 |
| n_updates | 29376 |
----------------------------------
day: 95, episode: 2000
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 312 |
| fps | 47 |
| time_elapsed | 636 |
| total timesteps | 29952 |
| train/ | |
| actor_loss | 965 |
| critic_loss | 1.69e+04 |
| learning_rate | 0.0003 |
| n_updates | 29760 |
----------------------------------
###Markdown
Model 4: SAC
###Code
agent = DRLAgent(env = env_train)
SAC_PARAMS = {
"batch_size": 128,
"buffer_size": 100000,
"learning_rate": 0.00003,
"learning_starts": 100,
"ent_coef": "auto_0.1",
}
model_sac = agent.get_model("sac",model_kwargs = SAC_PARAMS)
trained_sac = agent.train_model(model=model_sac,
tb_log_name='sac',
total_timesteps=30000)
###Output
Logging to tensorboard_log/sac/sac_1
-----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 4 |
| fps | 58 |
| time_elapsed | 6 |
| total timesteps | 384 |
| train/ | |
| actor_loss | -2.43e+03 |
| critic_loss | 9.96e+04 |
| ent_coef | 0.101 |
| ent_coef_loss | 21.5 |
| learning_rate | 3e-05 |
| n_updates | 283 |
-----------------------------------
day: 95, episode: 2030
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 8 |
| fps | 50 |
| time_elapsed | 15 |
| total timesteps | 768 |
| train/ | |
| actor_loss | -2.4e+03 |
| critic_loss | 9.1e+04 |
| ent_coef | 0.102 |
| ent_coef_loss | 21.2 |
| learning_rate | 3e-05 |
| n_updates | 667 |
----------------------------------
-----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 12 |
| fps | 48 |
| time_elapsed | 23 |
| total timesteps | 1152 |
| train/ | |
| actor_loss | -2.29e+03 |
| critic_loss | 3e+03 |
| ent_coef | 0.103 |
| ent_coef_loss | 21.1 |
| learning_rate | 3e-05 |
| n_updates | 1051 |
-----------------------------------
-----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 16 |
| fps | 46 |
| time_elapsed | 32 |
| total timesteps | 1536 |
| train/ | |
| actor_loss | -2.21e+03 |
| critic_loss | 1.16e+05 |
| ent_coef | 0.104 |
| ent_coef_loss | 21.4 |
| learning_rate | 3e-05 |
| n_updates | 1435 |
-----------------------------------
day: 95, episode: 2040
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
-----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 20 |
| fps | 46 |
| time_elapsed | 41 |
| total timesteps | 1920 |
| train/ | |
| actor_loss | -2.12e+03 |
| critic_loss | 1.09e+05 |
| ent_coef | 0.106 |
| ent_coef_loss | 21.1 |
| learning_rate | 3e-05 |
| n_updates | 1819 |
-----------------------------------
-----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 24 |
| fps | 45 |
| time_elapsed | 50 |
| total timesteps | 2304 |
| train/ | |
| actor_loss | -2.04e+03 |
| critic_loss | 6.63e+04 |
| ent_coef | 0.107 |
| ent_coef_loss | 20.9 |
| learning_rate | 3e-05 |
| n_updates | 2203 |
-----------------------------------
day: 95, episode: 2050
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
-----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 28 |
| fps | 45 |
| time_elapsed | 59 |
| total timesteps | 2688 |
| train/ | |
| actor_loss | -1.91e+03 |
| critic_loss | 6.01e+04 |
| ent_coef | 0.108 |
| ent_coef_loss | 21.1 |
| learning_rate | 3e-05 |
| n_updates | 2587 |
-----------------------------------
-----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 32 |
| fps | 44 |
| time_elapsed | 68 |
| total timesteps | 3072 |
| train/ | |
| actor_loss | -1.91e+03 |
| critic_loss | 8.44e+04 |
| ent_coef | 0.109 |
| ent_coef_loss | 21 |
| learning_rate | 3e-05 |
| n_updates | 2971 |
-----------------------------------
-----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 36 |
| fps | 44 |
| time_elapsed | 77 |
| total timesteps | 3456 |
| train/ | |
| actor_loss | -1.75e+03 |
| critic_loss | 5.03e+04 |
| ent_coef | 0.111 |
| ent_coef_loss | 21 |
| learning_rate | 3e-05 |
| n_updates | 3355 |
-----------------------------------
day: 95, episode: 2060
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 40 |
| fps | 44 |
| time_elapsed | 85 |
| total timesteps | 3840 |
| train/ | |
| actor_loss | -1.7e+03 |
| critic_loss | 2.36e+04 |
| ent_coef | 0.112 |
| ent_coef_loss | 20.6 |
| learning_rate | 3e-05 |
| n_updates | 3739 |
----------------------------------
-----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 44 |
| fps | 44 |
| time_elapsed | 94 |
| total timesteps | 4224 |
| train/ | |
| actor_loss | -1.68e+03 |
| critic_loss | 2.14e+04 |
| ent_coef | 0.113 |
| ent_coef_loss | 20.6 |
| learning_rate | 3e-05 |
| n_updates | 4123 |
-----------------------------------
day: 95, episode: 2070
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
-----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 48 |
| fps | 44 |
| time_elapsed | 103 |
| total timesteps | 4608 |
| train/ | |
| actor_loss | -1.52e+03 |
| critic_loss | 5.75e+04 |
| ent_coef | 0.114 |
| ent_coef_loss | 20.3 |
| learning_rate | 3e-05 |
| n_updates | 4507 |
-----------------------------------
-----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 52 |
| fps | 44 |
| time_elapsed | 111 |
| total timesteps | 4992 |
| train/ | |
| actor_loss | -1.54e+03 |
| critic_loss | 3.54e+04 |
| ent_coef | 0.116 |
| ent_coef_loss | 20.4 |
| learning_rate | 3e-05 |
| n_updates | 4891 |
-----------------------------------
-----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 56 |
| fps | 44 |
| time_elapsed | 120 |
| total timesteps | 5376 |
| train/ | |
| actor_loss | -1.42e+03 |
| critic_loss | 1.98e+04 |
| ent_coef | 0.117 |
| ent_coef_loss | 20 |
| learning_rate | 3e-05 |
| n_updates | 5275 |
-----------------------------------
day: 95, episode: 2080
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
-----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 60 |
| fps | 44 |
| time_elapsed | 129 |
| total timesteps | 5760 |
| train/ | |
| actor_loss | -1.38e+03 |
| critic_loss | 3.03e+04 |
| ent_coef | 0.119 |
| ent_coef_loss | 20.1 |
| learning_rate | 3e-05 |
| n_updates | 5659 |
-----------------------------------
-----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 64 |
| fps | 44 |
| time_elapsed | 138 |
| total timesteps | 6144 |
| train/ | |
| actor_loss | -1.35e+03 |
| critic_loss | 6.31e+04 |
| ent_coef | 0.12 |
| ent_coef_loss | 20 |
| learning_rate | 3e-05 |
| n_updates | 6043 |
-----------------------------------
day: 95, episode: 2090
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
-----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 68 |
| fps | 44 |
| time_elapsed | 146 |
| total timesteps | 6528 |
| train/ | |
| actor_loss | -1.22e+03 |
| critic_loss | 2.52e+04 |
| ent_coef | 0.121 |
| ent_coef_loss | 20 |
| learning_rate | 3e-05 |
| n_updates | 6427 |
-----------------------------------
-----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 72 |
| fps | 44 |
| time_elapsed | 155 |
| total timesteps | 6912 |
| train/ | |
| actor_loss | -1.23e+03 |
| critic_loss | 3.42e+04 |
| ent_coef | 0.123 |
| ent_coef_loss | 19.9 |
| learning_rate | 3e-05 |
| n_updates | 6811 |
-----------------------------------
-----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 76 |
| fps | 44 |
| time_elapsed | 164 |
| total timesteps | 7296 |
| train/ | |
| actor_loss | -1.15e+03 |
| critic_loss | 1.04e+03 |
| ent_coef | 0.124 |
| ent_coef_loss | 19.6 |
| learning_rate | 3e-05 |
| n_updates | 7195 |
-----------------------------------
day: 95, episode: 2100
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
-----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 80 |
| fps | 44 |
| time_elapsed | 172 |
| total timesteps | 7680 |
| train/ | |
| actor_loss | -1.13e+03 |
| critic_loss | 75.6 |
| ent_coef | 0.126 |
| ent_coef_loss | 19.3 |
| learning_rate | 3e-05 |
| n_updates | 7579 |
-----------------------------------
-----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 84 |
| fps | 44 |
| time_elapsed | 181 |
| total timesteps | 8064 |
| train/ | |
| actor_loss | -1.04e+03 |
| critic_loss | 462 |
| ent_coef | 0.127 |
| ent_coef_loss | 19.4 |
| learning_rate | 3e-05 |
| n_updates | 7963 |
-----------------------------------
day: 95, episode: 2110
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 88 |
| fps | 44 |
| time_elapsed | 190 |
| total timesteps | 8448 |
| train/ | |
| actor_loss | -979 |
| critic_loss | 8.71e+03 |
| ent_coef | 0.128 |
| ent_coef_loss | 19.4 |
| learning_rate | 3e-05 |
| n_updates | 8347 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 92 |
| fps | 44 |
| time_elapsed | 199 |
| total timesteps | 8832 |
| train/ | |
| actor_loss | -965 |
| critic_loss | 1.65e+04 |
| ent_coef | 0.13 |
| ent_coef_loss | 19 |
| learning_rate | 3e-05 |
| n_updates | 8731 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 96 |
| fps | 44 |
| time_elapsed | 207 |
| total timesteps | 9216 |
| train/ | |
| actor_loss | -898 |
| critic_loss | 1.34e+04 |
| ent_coef | 0.131 |
| ent_coef_loss | 19.1 |
| learning_rate | 3e-05 |
| n_updates | 9115 |
----------------------------------
day: 95, episode: 2120
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 100 |
| fps | 44 |
| time_elapsed | 216 |
| total timesteps | 9600 |
| train/ | |
| actor_loss | -916 |
| critic_loss | 558 |
| ent_coef | 0.133 |
| ent_coef_loss | 18.9 |
| learning_rate | 3e-05 |
| n_updates | 9499 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 104 |
| fps | 44 |
| time_elapsed | 225 |
| total timesteps | 9984 |
| train/ | |
| actor_loss | -823 |
| critic_loss | 1.09e+04 |
| ent_coef | 0.135 |
| ent_coef_loss | 18.6 |
| learning_rate | 3e-05 |
| n_updates | 9883 |
----------------------------------
day: 95, episode: 2130
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 108 |
| fps | 44 |
| time_elapsed | 233 |
| total timesteps | 10368 |
| train/ | |
| actor_loss | -789 |
| critic_loss | 1.44e+04 |
| ent_coef | 0.136 |
| ent_coef_loss | 18.7 |
| learning_rate | 3e-05 |
| n_updates | 10267 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 112 |
| fps | 44 |
| time_elapsed | 242 |
| total timesteps | 10752 |
| train/ | |
| actor_loss | -784 |
| critic_loss | 960 |
| ent_coef | 0.138 |
| ent_coef_loss | 18.7 |
| learning_rate | 3e-05 |
| n_updates | 10651 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 116 |
| fps | 44 |
| time_elapsed | 251 |
| total timesteps | 11136 |
| train/ | |
| actor_loss | -706 |
| critic_loss | 5.32e+03 |
| ent_coef | 0.139 |
| ent_coef_loss | 18.5 |
| learning_rate | 3e-05 |
| n_updates | 11035 |
----------------------------------
day: 95, episode: 2140
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 120 |
| fps | 44 |
| time_elapsed | 260 |
| total timesteps | 11520 |
| train/ | |
| actor_loss | -703 |
| critic_loss | 330 |
| ent_coef | 0.141 |
| ent_coef_loss | 18.3 |
| learning_rate | 3e-05 |
| n_updates | 11419 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 124 |
| fps | 44 |
| time_elapsed | 268 |
| total timesteps | 11904 |
| train/ | |
| actor_loss | -679 |
| critic_loss | 3.88e+03 |
| ent_coef | 0.143 |
| ent_coef_loss | 18.2 |
| learning_rate | 3e-05 |
| n_updates | 11803 |
----------------------------------
day: 95, episode: 2150
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 128 |
| fps | 44 |
| time_elapsed | 277 |
| total timesteps | 12288 |
| train/ | |
| actor_loss | -633 |
| critic_loss | 754 |
| ent_coef | 0.144 |
| ent_coef_loss | 18.3 |
| learning_rate | 3e-05 |
| n_updates | 12187 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 132 |
| fps | 44 |
| time_elapsed | 286 |
| total timesteps | 12672 |
| train/ | |
| actor_loss | -589 |
| critic_loss | 6.05e+03 |
| ent_coef | 0.146 |
| ent_coef_loss | 18.2 |
| learning_rate | 3e-05 |
| n_updates | 12571 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 136 |
| fps | 44 |
| time_elapsed | 295 |
| total timesteps | 13056 |
| train/ | |
| actor_loss | -550 |
| critic_loss | 1.25e+04 |
| ent_coef | 0.148 |
| ent_coef_loss | 18 |
| learning_rate | 3e-05 |
| n_updates | 12955 |
----------------------------------
day: 95, episode: 2160
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 140 |
| fps | 44 |
| time_elapsed | 303 |
| total timesteps | 13440 |
| train/ | |
| actor_loss | -563 |
| critic_loss | 539 |
| ent_coef | 0.149 |
| ent_coef_loss | 17.9 |
| learning_rate | 3e-05 |
| n_updates | 13339 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 144 |
| fps | 44 |
| time_elapsed | 312 |
| total timesteps | 13824 |
| train/ | |
| actor_loss | -550 |
| critic_loss | 3.3e+03 |
| ent_coef | 0.151 |
| ent_coef_loss | 17.6 |
| learning_rate | 3e-05 |
| n_updates | 13723 |
----------------------------------
day: 95, episode: 2170
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 148 |
| fps | 44 |
| time_elapsed | 321 |
| total timesteps | 14208 |
| train/ | |
| actor_loss | -504 |
| critic_loss | 2.79e+03 |
| ent_coef | 0.153 |
| ent_coef_loss | 17.6 |
| learning_rate | 3e-05 |
| n_updates | 14107 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 152 |
| fps | 44 |
| time_elapsed | 330 |
| total timesteps | 14592 |
| train/ | |
| actor_loss | -479 |
| critic_loss | 2.17e+03 |
| ent_coef | 0.154 |
| ent_coef_loss | 17.6 |
| learning_rate | 3e-05 |
| n_updates | 14491 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 156 |
| fps | 44 |
| time_elapsed | 338 |
| total timesteps | 14976 |
| train/ | |
| actor_loss | -458 |
| critic_loss | 1.84e+03 |
| ent_coef | 0.156 |
| ent_coef_loss | 17.5 |
| learning_rate | 3e-05 |
| n_updates | 14875 |
----------------------------------
day: 95, episode: 2180
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 160 |
| fps | 44 |
| time_elapsed | 347 |
| total timesteps | 15360 |
| train/ | |
| actor_loss | -408 |
| critic_loss | 2.19e+03 |
| ent_coef | 0.158 |
| ent_coef_loss | 17.4 |
| learning_rate | 3e-05 |
| n_updates | 15259 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 164 |
| fps | 44 |
| time_elapsed | 356 |
| total timesteps | 15744 |
| train/ | |
| actor_loss | -399 |
| critic_loss | 851 |
| ent_coef | 0.16 |
| ent_coef_loss | 17.1 |
| learning_rate | 3e-05 |
| n_updates | 15643 |
----------------------------------
day: 95, episode: 2190
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 168 |
| fps | 44 |
| time_elapsed | 365 |
| total timesteps | 16128 |
| train/ | |
| actor_loss | -405 |
| critic_loss | 1.44e+03 |
| ent_coef | 0.162 |
| ent_coef_loss | 17 |
| learning_rate | 3e-05 |
| n_updates | 16027 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 172 |
| fps | 44 |
| time_elapsed | 374 |
| total timesteps | 16512 |
| train/ | |
| actor_loss | -372 |
| critic_loss | 1.14e+03 |
| ent_coef | 0.164 |
| ent_coef_loss | 16.8 |
| learning_rate | 3e-05 |
| n_updates | 16411 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 176 |
| fps | 44 |
| time_elapsed | 383 |
| total timesteps | 16896 |
| train/ | |
| actor_loss | -367 |
| critic_loss | 418 |
| ent_coef | 0.166 |
| ent_coef_loss | 16.9 |
| learning_rate | 3e-05 |
| n_updates | 16795 |
----------------------------------
day: 95, episode: 2200
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 180 |
| fps | 44 |
| time_elapsed | 392 |
| total timesteps | 17280 |
| train/ | |
| actor_loss | -353 |
| critic_loss | 450 |
| ent_coef | 0.167 |
| ent_coef_loss | 16.5 |
| learning_rate | 3e-05 |
| n_updates | 17179 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 184 |
| fps | 44 |
| time_elapsed | 400 |
| total timesteps | 17664 |
| train/ | |
| actor_loss | -315 |
| critic_loss | 970 |
| ent_coef | 0.169 |
| ent_coef_loss | 16.8 |
| learning_rate | 3e-05 |
| n_updates | 17563 |
----------------------------------
day: 95, episode: 2210
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 188 |
| fps | 44 |
| time_elapsed | 409 |
| total timesteps | 18048 |
| train/ | |
| actor_loss | -313 |
| critic_loss | 1.1e+03 |
| ent_coef | 0.171 |
| ent_coef_loss | 16.5 |
| learning_rate | 3e-05 |
| n_updates | 17947 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 192 |
| fps | 44 |
| time_elapsed | 418 |
| total timesteps | 18432 |
| train/ | |
| actor_loss | -301 |
| critic_loss | 440 |
| ent_coef | 0.173 |
| ent_coef_loss | 16.5 |
| learning_rate | 3e-05 |
| n_updates | 18331 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 196 |
| fps | 44 |
| time_elapsed | 426 |
| total timesteps | 18816 |
| train/ | |
| actor_loss | -283 |
| critic_loss | 590 |
| ent_coef | 0.175 |
| ent_coef_loss | 16.4 |
| learning_rate | 3e-05 |
| n_updates | 18715 |
----------------------------------
day: 95, episode: 2220
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 200 |
| fps | 44 |
| time_elapsed | 435 |
| total timesteps | 19200 |
| train/ | |
| actor_loss | -262 |
| critic_loss | 673 |
| ent_coef | 0.177 |
| ent_coef_loss | 16.2 |
| learning_rate | 3e-05 |
| n_updates | 19099 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 204 |
| fps | 44 |
| time_elapsed | 444 |
| total timesteps | 19584 |
| train/ | |
| actor_loss | -244 |
| critic_loss | 832 |
| ent_coef | 0.179 |
| ent_coef_loss | 16.1 |
| learning_rate | 3e-05 |
| n_updates | 19483 |
----------------------------------
day: 95, episode: 2230
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 208 |
| fps | 44 |
| time_elapsed | 452 |
| total timesteps | 19968 |
| train/ | |
| actor_loss | -242 |
| critic_loss | 577 |
| ent_coef | 0.182 |
| ent_coef_loss | 16 |
| learning_rate | 3e-05 |
| n_updates | 19867 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 212 |
| fps | 44 |
| time_elapsed | 461 |
| total timesteps | 20352 |
| train/ | |
| actor_loss | -239 |
| critic_loss | 1.19e+03 |
| ent_coef | 0.184 |
| ent_coef_loss | 15.9 |
| learning_rate | 3e-05 |
| n_updates | 20251 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 216 |
| fps | 44 |
| time_elapsed | 470 |
| total timesteps | 20736 |
| train/ | |
| actor_loss | -201 |
| critic_loss | 613 |
| ent_coef | 0.186 |
| ent_coef_loss | 15.8 |
| learning_rate | 3e-05 |
| n_updates | 20635 |
----------------------------------
day: 95, episode: 2240
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 220 |
| fps | 44 |
| time_elapsed | 479 |
| total timesteps | 21120 |
| train/ | |
| actor_loss | -211 |
| critic_loss | 945 |
| ent_coef | 0.188 |
| ent_coef_loss | 15.8 |
| learning_rate | 3e-05 |
| n_updates | 21019 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 224 |
| fps | 44 |
| time_elapsed | 488 |
| total timesteps | 21504 |
| train/ | |
| actor_loss | -149 |
| critic_loss | 1.09e+03 |
| ent_coef | 0.19 |
| ent_coef_loss | 15.7 |
| learning_rate | 3e-05 |
| n_updates | 21403 |
----------------------------------
day: 95, episode: 2250
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 228 |
| fps | 44 |
| time_elapsed | 496 |
| total timesteps | 21888 |
| train/ | |
| actor_loss | -167 |
| critic_loss | 347 |
| ent_coef | 0.192 |
| ent_coef_loss | 15.6 |
| learning_rate | 3e-05 |
| n_updates | 21787 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 232 |
| fps | 44 |
| time_elapsed | 505 |
| total timesteps | 22272 |
| train/ | |
| actor_loss | -160 |
| critic_loss | 430 |
| ent_coef | 0.195 |
| ent_coef_loss | 15.4 |
| learning_rate | 3e-05 |
| n_updates | 22171 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 236 |
| fps | 44 |
| time_elapsed | 514 |
| total timesteps | 22656 |
| train/ | |
| actor_loss | -132 |
| critic_loss | 226 |
| ent_coef | 0.197 |
| ent_coef_loss | 15.4 |
| learning_rate | 3e-05 |
| n_updates | 22555 |
----------------------------------
day: 95, episode: 2260
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 240 |
| fps | 44 |
| time_elapsed | 523 |
| total timesteps | 23040 |
| train/ | |
| actor_loss | -136 |
| critic_loss | 121 |
| ent_coef | 0.199 |
| ent_coef_loss | 15.2 |
| learning_rate | 3e-05 |
| n_updates | 22939 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 244 |
| fps | 44 |
| time_elapsed | 531 |
| total timesteps | 23424 |
| train/ | |
| actor_loss | -128 |
| critic_loss | 68.2 |
| ent_coef | 0.201 |
| ent_coef_loss | 15.1 |
| learning_rate | 3e-05 |
| n_updates | 23323 |
----------------------------------
day: 95, episode: 2270
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 248 |
| fps | 44 |
| time_elapsed | 540 |
| total timesteps | 23808 |
| train/ | |
| actor_loss | -129 |
| critic_loss | 209 |
| ent_coef | 0.204 |
| ent_coef_loss | 15.3 |
| learning_rate | 3e-05 |
| n_updates | 23707 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 252 |
| fps | 44 |
| time_elapsed | 549 |
| total timesteps | 24192 |
| train/ | |
| actor_loss | -109 |
| critic_loss | 311 |
| ent_coef | 0.206 |
| ent_coef_loss | 14.9 |
| learning_rate | 3e-05 |
| n_updates | 24091 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 256 |
| fps | 44 |
| time_elapsed | 558 |
| total timesteps | 24576 |
| train/ | |
| actor_loss | -106 |
| critic_loss | 478 |
| ent_coef | 0.208 |
| ent_coef_loss | 14.6 |
| learning_rate | 3e-05 |
| n_updates | 24475 |
----------------------------------
day: 95, episode: 2280
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 260 |
| fps | 44 |
| time_elapsed | 566 |
| total timesteps | 24960 |
| train/ | |
| actor_loss | -83.5 |
| critic_loss | 390 |
| ent_coef | 0.211 |
| ent_coef_loss | 14.7 |
| learning_rate | 3e-05 |
| n_updates | 24859 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 264 |
| fps | 44 |
| time_elapsed | 575 |
| total timesteps | 25344 |
| train/ | |
| actor_loss | -73.5 |
| critic_loss | 196 |
| ent_coef | 0.213 |
| ent_coef_loss | 14.3 |
| learning_rate | 3e-05 |
| n_updates | 25243 |
----------------------------------
day: 95, episode: 2290
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 268 |
| fps | 44 |
| time_elapsed | 584 |
| total timesteps | 25728 |
| train/ | |
| actor_loss | -73.4 |
| critic_loss | 62.8 |
| ent_coef | 0.216 |
| ent_coef_loss | 14.5 |
| learning_rate | 3e-05 |
| n_updates | 25627 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 272 |
| fps | 44 |
| time_elapsed | 593 |
| total timesteps | 26112 |
| train/ | |
| actor_loss | -67.3 |
| critic_loss | 239 |
| ent_coef | 0.218 |
| ent_coef_loss | 14.4 |
| learning_rate | 3e-05 |
| n_updates | 26011 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 276 |
| fps | 44 |
| time_elapsed | 601 |
| total timesteps | 26496 |
| train/ | |
| actor_loss | -67.8 |
| critic_loss | 214 |
| ent_coef | 0.221 |
| ent_coef_loss | 14.1 |
| learning_rate | 3e-05 |
| n_updates | 26395 |
----------------------------------
day: 95, episode: 2300
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 280 |
| fps | 44 |
| time_elapsed | 610 |
| total timesteps | 26880 |
| train/ | |
| actor_loss | -47.7 |
| critic_loss | 151 |
| ent_coef | 0.223 |
| ent_coef_loss | 14.3 |
| learning_rate | 3e-05 |
| n_updates | 26779 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 284 |
| fps | 44 |
| time_elapsed | 619 |
| total timesteps | 27264 |
| train/ | |
| actor_loss | -60.7 |
| critic_loss | 351 |
| ent_coef | 0.226 |
| ent_coef_loss | 13.9 |
| learning_rate | 3e-05 |
| n_updates | 27163 |
----------------------------------
day: 95, episode: 2310
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 288 |
| fps | 44 |
| time_elapsed | 628 |
| total timesteps | 27648 |
| train/ | |
| actor_loss | -39.6 |
| critic_loss | 134 |
| ent_coef | 0.229 |
| ent_coef_loss | 13.9 |
| learning_rate | 3e-05 |
| n_updates | 27547 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 292 |
| fps | 44 |
| time_elapsed | 636 |
| total timesteps | 28032 |
| train/ | |
| actor_loss | -43.1 |
| critic_loss | 126 |
| ent_coef | 0.231 |
| ent_coef_loss | 13.6 |
| learning_rate | 3e-05 |
| n_updates | 27931 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 296 |
| fps | 44 |
| time_elapsed | 645 |
| total timesteps | 28416 |
| train/ | |
| actor_loss | -27.6 |
| critic_loss | 249 |
| ent_coef | 0.234 |
| ent_coef_loss | 13.8 |
| learning_rate | 3e-05 |
| n_updates | 28315 |
----------------------------------
day: 95, episode: 2320
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 300 |
| fps | 44 |
| time_elapsed | 654 |
| total timesteps | 28800 |
| train/ | |
| actor_loss | -30.2 |
| critic_loss | 256 |
| ent_coef | 0.237 |
| ent_coef_loss | 13.4 |
| learning_rate | 3e-05 |
| n_updates | 28699 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 304 |
| fps | 44 |
| time_elapsed | 663 |
| total timesteps | 29184 |
| train/ | |
| actor_loss | -12.2 |
| critic_loss | 41.2 |
| ent_coef | 0.239 |
| ent_coef_loss | 13.4 |
| learning_rate | 3e-05 |
| n_updates | 29083 |
----------------------------------
day: 95, episode: 2330
begin_total_asset: 100000.00
end_total_asset: 100000.00
total_reward: 0.00
total_cost: 0.00
total_trades: 0
=================================
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 308 |
| fps | 44 |
| time_elapsed | 671 |
| total timesteps | 29568 |
| train/ | |
| actor_loss | -22.8 |
| critic_loss | 114 |
| ent_coef | 0.242 |
| ent_coef_loss | 13.4 |
| learning_rate | 3e-05 |
| n_updates | 29467 |
----------------------------------
----------------------------------
| environment/ | |
| portfolio_value | 1e+05 |
| total_cost | 0 |
| total_reward | 0 |
| total_reward_pct | 0 |
| total_trades | 0 |
| time/ | |
| episodes | 312 |
| fps | 44 |
| time_elapsed | 680 |
| total timesteps | 29952 |
| train/ | |
| actor_loss | -3.6 |
| critic_loss | 24.4 |
| ent_coef | 0.245 |
| ent_coef_loss | 13.2 |
| learning_rate | 3e-05 |
| n_updates | 29851 |
----------------------------------
###Markdown
Trading* we use the environment class we initialized at 5.3 to create a stock trading environment* Assume that we have $100,000 initial capital at 2019-01-01. * We use the trained model of PPO to trade AAPL.
###Code
trade.head()
## make a prediction and get the account value change
trade = data_split(data_df, start = '2019-01-01', end = '2021-01-01')
e_trade_gym = StockTradingEnv(df = trade, **env_kwargs)
env_trade, obs_trade = e_trade_gym.get_sb_env()
df_account_value, df_actions = DRLAgent.DRL_prediction(model=trained_a2c,
test_data = trade,
test_env = env_trade,
test_obs = obs_trade)
###Output
_____no_output_____
###Markdown
Part 7: Backtesting PerformanceBacktesting plays a key role in evaluating the performance of a trading strategy. Automated backtesting tool is preferred because it reduces the human error. We usually use the Quantopian pyfolio package to backtest our trading strategies. It is easy to use and consists of various individual plots that provide a comprehensive image of the performance of a trading strategy. 7.1 BackTestStatspass in df_account_value, this information is stored in env class
###Code
print("==============Get Backtest Results===========")
now = datetime.datetime.now().strftime('%Y%m%d-%Hh%M')
perf_stats_all = BackTestStats(account_value=df_account_value)
perf_stats_all = pd.DataFrame(perf_stats_all)
perf_stats_all.to_csv("./"+config.RESULTS_DIR+"/perf_stats_all_"+now+'.csv')
###Output
==============Get Backtest Results===========
###Markdown
7.2 BackTestPlot
###Code
print("==============Compare to AAPL itself buy-and-hold===========")
%matplotlib inline
BackTestPlot(account_value=df_account_value,
baseline_ticker = 'AAPL',
baseline_start = '2019-01-01',
baseline_end = '2021-01-01')
###Output
_____no_output_____
###Markdown
7.3 Baseline Stats
###Code
print("==============Get Baseline Stats===========")
baesline_perf_stats=BaselineStats('AAPL')
print("==============Get Baseline Stats===========")
baesline_perf_stats=BaselineStats('^GSPC')
###Output
_____no_output_____
###Markdown
7.4 Compare to Stock Market Index
###Code
print("==============Compare to S&P 500===========")
%matplotlib inline
# S&P 500: ^GSPC
# Dow Jones Index: ^DJI
# NASDAQ 100: ^NDX
BackTestPlot(df_account_value, baseline_ticker = '^GSPC')
###Output
==============Compare to S&P 500===========
annual return: -0.02065151445176383
sharpe ratio: -1.6896134032497638
[*********************100%***********************] 1 of 1 completed
Shape of DataFrame: (483, 8)
|
Deep-learning-framework/pytorch/pytorch-handbook/chapter2/2.5-rnn.ipynb | ###Markdown
2.5 循环神经网络 2.5.1 RNN简介我们的大脑区别于机器的一个最大的特征就是我们有记忆,并且能够根据自己的记忆对未知的事务进行推导,我们的思想拥有持久性的。但是本教程目前所介绍的神经网络结构各个元素之间是相互独立的,输入与输出是独立的。 RNN的起因现实世界中,很多元素都是相互连接的,比如室外的温度是随着气候的变化而周期性的变化的、我们的语言也需要通过上下文的关系来确认所表达的含义。但是机器要做到这一步就相当得难了。因此,就有了现在的循环神经网络,他的本质是:拥有记忆的能力,并且会根据这些记忆的内容来进行推断。因此,他的输出就依赖于当前的输入和记忆。 为什么需要RNNRNN背后的想法是利用顺序的信息。 在传统的神经网络中,我们假设所有输入(和输出)彼此独立。 如果你想预测句子中的下一个单词,你就要知道它前面有哪些单词,甚至要看到后面的单词才能够给出正确的答案。 RNN之所以称为循环,就是因为它们对序列的每个元素都会执行相同的任务,所有的输出都取决于先前的计算。从另一个角度讲RNN的它是有“记忆”的,可以捕获到目前为止计算的信息。 理论上,RNN可以在任意长的序列中使用信息,但实际上它们仅限于回顾几个步骤。循环神经网络的提出便是基于记忆模型的想法,期望网络能够记住前面出现的特征.并依据特征推断后面的结果,而且整体的网络结构不断循环,因为得名循环神经网络。 RNN都能做什么RNN在许多NLP任务中取得了巨大成功。 在这一点上,我应该提到最常用的RNN类型是LSTM,它在捕获长期依赖性方面要比RNN好得多。 但不要担心,LSTM与我们将在本教程中开发的RNN基本相同,它们只是采用不同的方式来计算隐藏状态。 我们将在后面更详细地介绍LSTM。 以下是RNN在NLP中的一些示例:**语言建模与生成文本**我们通过语言的建模,可以通过给定的单词生成人类可以理解的以假乱真的文本**机器翻译**机器翻译类似于语言建模,我们的输入源语言中的一系列单词,通过模型的计算可以输出目标语言与之对应的内容。 **语音识别**给定来自声波的声学信号的输入序列,我们可以预测一系列语音片段及其概率,并把语音转化成文字**生成图像描述**与卷积神经网络一起,RNN可以生成未标记图像的描述。 2.5.2 RNN的网络结构及原理 RNN循环神经网络的基本结构特别简单,就是将网络的输出保存在一个记忆单元中,这个记忆单元和下一次的输入一起进入神经网络中。我们可以看到网络在输入的时候会联合记忆单元一起作为输入,网络不仅输出结果,还会将结果保存到记忆单元中,下图就是一个最简单的循环神经网络在输入时的结构示意图.[图片来源](https://medium.com/explore-artificial-intelligence/an-introduction-to-recurrent-neural-networks-72c97bf0912)RNN 可以被看做是同一神经网络的多次赋值,每个神经网络模块会把消息传递给下一个,我们将这个图的结构展开网络中具有循环结构,这也是循环神经网络名字的由来,同时根据循环神经网络的结构也可以看出它在处理序列类型的数据上具有天然的优势.因为网络本身就是 一个序列结构,这也是所有循环神经网络最本质的结构。循环神经网络具有特别好的记忆特性,能够将记忆内容应用到当前情景下,但是网络的记忆能力并没有想象的那么有效。记忆最大的问题在于它有遗忘性,我们总是更加清楚地记得最近发生的事情而遗忘很久之前发生的事情,循环神经网络同样有这样的问题。pytorch 中使用 nn.RNN 类来搭建基于序列的循环神经网络,它的构造函数有以下几个参数:- nput_size:输入数据X的特征值的数目。 - hidden_size:隐藏层的神经元数量,也就是隐藏层的特征数量。- num_layers:循环神经网络的层数,默认值是 1。 - bias:默认为 True,如果为 false 则表示神经元不使用 bias 偏移参数。- batch_first:如果设置为 True,则输入数据的维度中第一个维度就 是 batch 值,默认为 False。默认情况下第一个维度是序列的长度, 第二个维度才是 - - batch,第三个维度是特征数目。- dropout:如果不为空,则表示最后跟一个 dropout 层抛弃部分数据,抛弃数据的比例由该参数指定。RNN 中最主要的参数是 input_size 和 hidden_size,这两个参数务必要搞清楚。其余的参数通常不用设置,采用默认值就可以了。
###Code
rnn = torch.nn.RNN(20,50,2)
input = torch.randn(100 , 32 , 20)
h_0 =torch.randn(2 , 32 , 50)
output,hn=rnn(input ,h_0)
print(output.size(),hn.size())
###Output
torch.Size([100, 32, 50]) torch.Size([2, 32, 50])
###Markdown
LSTMLSTM 是 Long Short Term Memory Networks 的缩写,按字面翻译就是长的短时记忆网络。LSTM 的网络结构是 1997 年由 Hochreiter 和 Schmidhuber 提出的,随后这种网络结构变得非常流行,。LSTM虽然只解决了短期依赖的问题,并且它通过刻意的设计来避免长期依赖问题,这样的做法在实际应用中被证明还是十分有效的,有很多人跟进相关的工作解决了很多实际的问题,所以现在LSTM 仍然被广泛地使用。[图片来源](https://towardsdatascience.com/animated-rnn-lstm-and-gru-ef124d06cf45)标准的循环神经网络内部只有一个简单的层结构,而 LSTM 内部有 4 个层结构:第一层是个忘记层:决定状态中丢弃什么信息第二层tanh层用来产生更新值的候选项,说明状态在某些维度上需要加强,在某些维度上需要减弱第三层sigmoid层(输入门层),它的输出值要乘到tanh层的输出上,起到一个缩放的作用,极端情况下sigmoid输出0说明相应维度上的状态不需要更新最后一层 决定输出什么,输出值跟状态有关。候选项中的哪些部分最终会被输出由一个sigmoid层来决定。pytorch 中使用 nn.LSTM 类来搭建基于序列的循环神经网络,他的参数基本与RNN类似,这里就不列出了。
###Code
lstm = torch.nn.LSTM(10, 20,2)
input = torch.randn(5, 3, 10)
h0 =torch.randn(2, 3, 20)
c0 = torch.randn(2, 3, 20)
output, hn = lstm(input, (h0, c0))
print(output.size(),hn[0].size(),hn[1].size())
###Output
torch.Size([5, 3, 20]) torch.Size([2, 3, 20]) torch.Size([2, 3, 20])
###Markdown
GRUGRU 是 gated recurrent units 的缩写,由 Cho在 2014 年提出GRU 和 LSTM 最 的不同在于 GRU 将遗忘门和输入门合成了一个"更新门",同时网络不再额外给出记忆状态,而是将输出结果作为记忆状态不断向后循环传递,网络的输人和输出都变得特别简单。
###Code
rnn = torch.nn.GRU(10, 20, 2)
input = torch.randn(5, 3, 10)
h_0= torch.randn(2, 3, 20)
output, hn = rnn(input, h0)
print(output.size(),h0.size())
###Output
torch.Size([5, 3, 20]) torch.Size([2, 3, 20])
###Markdown
2.5.3 循环网络的向后传播(BPTT)在向前传播的情况下,RNN的输入随着每一个时间步前进。在反向传播的情况下,我们“回到过去”改变权重,因此我们叫它通过时间的反向传播(BPTT)。我们通常把整个序列(单词)看作一个训练样本,所以总的误差是每个时间步(字符)中误差的和。权重在每一个时间步长是相同的(所以可以计算总误差后一起更新)。1. 使用预测输出和实际输出计算交叉熵误差2. 网络按照时间步完全展开3. 对于展开的网络,对于每一个实践步计算权重的梯度4. 因为对于所有时间步来说,权重都一样,所以对于所有的时间步,可以一起得到梯度(而不是像神经网络一样对不同的隐藏层得到不同的梯度)5. 随后对循环神经元的权重进行升级RNN展开的网络看起来像一个普通的神经网络。反向传播也类似于普通的神经网络,只不过我们一次得到所有时间步的梯度。如果有100个时间步,那么网络展开后将变得非常巨大,所以为了解决这个问题才会出现LSTM和GRU这样的结构。 循环神经网络目前在自然语言处理中应用最为火热,所以后面的内容将介绍一下循环神经网络在处理NLP的时候需要用到的一些其他的知识 2.5.4 词嵌入(word embedding)在我们人类交流过程中表征词汇是直接使用英文单词来进行表征的,但是对于计算机来说,是无法直接认识单词的。为了让计算机能够能更好地理解我们的语言,建立更好的语言模型,我们需要将词汇进行表征。在图像分类问题会使用 one-hot 编码.比如LeNet中一共有10个数字0-9,如果这个数字是2的话类,它的 编码就是 (0,0,1,0, 0,0 ,0,0,0,0),对于分类问题这样表示十分的清楚,但是在自然语言处理中,因为单词的数目过多比如有 10000 个不同的词,那么使用 one-hot 这样的方式来定义,效率就特别低,每个单词都是 10000 维的向量.其中只有一位是 1 , 其余都是 0,特别占用内存,而且也不能体现单词的词性,因为每一个单词都是 one-hot,虽然有些单词在语义上会更加接近.但是 one-hot 没办法体现这个特点,所以 必须使用另外一种方式定义每一个单词。用不同的特征来对各个词汇进行表征,相对与不同的特征,不同的单词均有不同的值这就是词嵌入。下图还是来自吴恩达老师的课程截图词嵌入不仅对不同单词实现了特征化的表示,还能通过计算词与词之间的相似度,实际上是在多维空间中,寻找词向量之间各个维度的距离相似度,我们就可以实现类比推理,比如说夏天和热,冬天和冷,都是有关联关系的。在 PyTorch 中我们用 nn.Embedding 层来做嵌入词袋模型,Embedding层第一个输入表示我们有多少个词,第二个输入表示每一个词使用多少个向量表示。
###Code
# an Embedding module containing 10 tensors of size 3
embedding = torch.nn.Embedding(10, 3)
# a batch of 2 samples of 4 indices each
input = torch.LongTensor([[1,2,4,5],[4,3,2,9]])
output=embedding(input)
print(output.size())
###Output
torch.Size([2, 4, 3])
|
.ipynb_checkpoints/CALCULO 101 LECCION 5 CONTINUIDAD-checkpoint.ipynb | ###Markdown
CÁLCULO DIFERENCIAL E INTEGRAL FUNCIONES Y SUS LÍMITES CONTINUIDAD Cómo hemos visto en algunos ejemplos, algunas funciones "se rompen" en algunos puntos. Cuándo esto no sucede, decimos que la función es *continua*. En esta sección analizaremos este concepto. DefiniciónUna función $f$ es continua en un punto $x=a$ si$$\lim_{x\to a}f(x) = f(a)$$  Observe que la definición requiere que * $f(a)$ está definida* $\lim_{x\to a} f(x)$ existe* Ambos valores coinciden. Ejemplo ¿En qué números la siguiente función es discontinua? ¿Porqué? Ejercicio ¿Cuáles de estas funciones son discontinuas?1. $$f(x) = \dfrac{x^2-x-2}{x-2}$$2. $$f(x) = \begin{cases}\dfrac{1}{x^2} & x\neq 0 \\1 & x=0\end{cases}$$3. $$f(x) = \begin{cases}\dfrac{x^2-x-2}{x-2} & x\neq 2 \\1 & x=2\end{cases}$$4. La función entero mayor $$f(x) = [[x]]$$ Esto nos conduce a introducir definiciones más precisas para describir cada caso: Definición* Diremos que una función es *continua por la derecha* en $x=a$ si $$ \lim_{x\to a^{+}} f(x) = f(a) $$* En cambio, diremos que una función es *continua por la izquierda* en $x=a$ si $$ \lim_{x\to a^{+}} f(x) = f(a) $$*¿Qué clase de continuidad presenta la función entero mayor?* De hecho, esto nos permite definir la continuidad en un intervalo compacto$$ I: a\leq x \leq b $$es decir $I=[a,b]$. DefiniciónDiremos que una función $f$ es continua en dicho intervalo $I$ si* $f$ es continua en el interior de I, es decir, en $$a<x<b$$* es continua por la derecha en $x=a$* es continua por la izquierda en $x=b$ EjercicioMuestre que la función $f(x)=1-\sqrt{1-x^2}$ es continua en el intervalo $[-1,1]$. A continuación se presentan algunos teoremas de continuidad, que harán fácil determinar si algunso funciones elementales son continuas. TeoremaSi $f,g$ son continuas en $x=a$ y $c$ es una contante, entonces las siguientes operaciones son continuas* $f\pm g$* $c f$* $fg$* $\dfrac{f}{g}$ siempre que $g(a)\neq 0$ De hecho, esto nos conduce a un par de observaciones importantes:1. Los polinomios son continuos en todo el eje real $(-\infty, \infty)$2. Las funciones racionales son continuas en su dominio, es decir, en los puntos en los que el denominador no se anula. Ejercicio Calcule $$\lim_{x\to -2} \dfrac{x^3+2x^2-1}{5-3x}$$ Ejercicio¿En qué puntos son continuas:1. $\sin(x)$2. $\cos(x)$3. $\tan(x)$? Ejercicios¿En qué intervalos son continuas1. $f(x) = x^{100}-2x^{37}+75$2. $g(x) = \dfrac{x^2+2x+17}{x^2-1}$3. $h(x) = \sqrt{x} + \dfrac{x+1}{x-1} - \dfrac{x+1}{x^2+1}$ Ahora, enunciaremos un par de los resultados más importantes en continuidad: Teorema Si $$\lim_{x\to a} g(x)= b$$ y $f(x)$ es continua en $b$, entonces$$ \lim_{x\to a} f(g(x)) = f(b) $$ Teorema Si $g$ es continua en $a$ y $f$ es continua en $g(a)$, entonces la composición $$(f\circ g)(x) = f(g(x))$$es continua en $a$. Ejercicio¿En que inteverlo respectivo las siguientes funciones son continuas?1. $$h(x)=\sin(x^2)$$2. $$F(x) = \dfrac{1}{\sqrt{x^2+7}-4}$$ Finalmente, una de las primeras aplicaciones de la continuidad está dado por el siguiente resultado: Teorema del valor intermedioSupongamos que $f$ es continua en un intervalo compacto $[a,b]$ y $N$ es un número entre $f(a)$ y $f(b)$ (que supondremos son distintos). Entonces **existe** un número $c\in(a,b)$ tal que $f(c)=N$. Ejercicio1. Explique porque el teorema del valor intermedio garantiza que existe una raíz de la ecuación$$ 4x^3-6x^2+3x-2=0 $$en el intervalo $[1,2]$2. Trace la gráfica del polinomio en diferentes intervalos $[x_0,x_1]$, de manera que $a\leq x_0 < x_1 \leq b$ hasta que *encapsule* la raíz con un margen de error de `0.001` unidades.
###Code
f(x) = 4*x^3-6*x^2+3*x-2
f(1)
f(2)
plot(f,1,2)
plot(f, 1.2, 1.25)
plot(tan(x),1.48,1.52, color="red")+plot(10*x,1.48,1.52)
f(x) = tan(x)-10*x
plot(f,1.48,1.52)
a = 1.48
b = 1.52
f(x) = tan(x)-10*x
for i in range(15):
n = (a+b)/2
if f(a)*f(n)<0:
b=n
else:
a=n
print(a,b)
a = 0.00000001
b = pi/2
f(x) = tan(x)-x
while b-a>0.000001:
n = (a+b)/2
if f(a)*f(n)<0:
b=n
else:
a=n
#print(a,b)
print(a.n(),b.n())
a = 0
b = 0.000001
f(x) = tan(x)-x
while b-a>0.000001:
n = (a+b)/2
if f(a)*f(n)<=0:
b=n
else:
a=n
#print(a,b)
print(a.n(),b.n())
###Output
(0.000000000000000, 1.00000000000000e-6)
|
samples/notebooks/fsharp/Docs/Math and LaTeX.ipynb | ###Markdown
[this doc on github](https://github.com/dotnet/interactive/tree/master/samples/notebooks/fsharp/Docs) Math and LaTeX Math content and LaTeX are supported
###Code
(LaTeXString)@"\begin{align}
\nabla \cdot \vec{\mathbf{E}} & = 4 \pi \rho \\
\nabla \times \vec{\mathbf{E}}\, +\, \frac1c\, \frac{\partial\vec{\mathbf{B}}}{\partial t} & = \vec{\mathbf{0}} \\
\nabla \cdot \vec{\mathbf{B}} & = 0
\end{align}"
(MathString)@"H← 60 + \frac{30(B−R)}{Vmax−Vmin} , if Vmax = G"
###Output
_____no_output_____ |
Chapter 3 - Regression Analysis/Activity 7 - Dummy Values.ipynb | ###Markdown
Activity 7 - Dummy VariablesFor this activity, we will use the Austin, Texas weather dataset that we used in theprevious activity. In this activity, we will use dummy variables to enhance our linearregression model for this dataset.
###Code
import numpy as np
import pandas as pd
import matplotlib.pyplot as plt
from sklearn.linear_model import LinearRegression
# Loading the data from activity 1
df = pd.read_csv('activity2_measurements.csv')
df_first_year = pd.read_csv('activity_first_year.csv')
rolling = pd.read_csv('activity2_rolling.csv')
window = 20
# Trendline values
trend_x = np.array([
1,
182.5,
365
])
###Output
_____no_output_____ |
BayesianNetwork/WikipediaWetExample.ipynb | ###Markdown
Example Bayesian network from wikipediahttps://en.wikipedia.org/wiki/Bayesian_network
###Code
from IPython.display import Image
from IPython.core.display import HTML
Image(url= "SimpleBayesNet.png")
###Output
_____no_output_____
###Markdown
Suppose that there are two events which could cause grass to be wet: either the sprinkler is on or it's raining. Also, suppose that the rain has a direct effect on the use of the sprinkler (namely that when it rains, the sprinkler is usually not turned on). Then the situation can be modeled with a Bayesian network (shown to the right). All three variables have two possible values, T (for true) and F (for false).The joimt probabilty function is:$Pr(G,S,R)=Pr(G|S,R)Pr(S|R)Pr(R)$where the names of the variables have been abbreviated to G = Grass wet (yes/no), S = Sprinkler turned on (yes/no), and R = Raining (yes/no). The model can answer questions like "What is the probability that it is raining, given the grass is wet?" by using the conditional probability formula and summing over all nuisance variables:$Pr(R=T|G=T)=\frac{Pr(G=T|R=T}{Pr(G=T)}=\frac{\sum_{S\in {T,F}}Pr(G=T,S,R=T)}{\sum_{S,R\in {T,F}}Pr(G=T,S,R)}$Using the expansion for the joint probability function {\displaystyle \Pr(G,S,R)} {\displaystyle \Pr(G,S,R)} and the conditional probabilities from the conditional probability tables (CPTs) stated in the diagram, one can evaluate each term in the sums in the numerator and denominator. For example,$Pr(G=T,S=T,R=T)=Pr(G=T|S=T,R=T)Pr(S=T|R=T)Pr(R=T)\\= 0.99 \times 0.01 \times 0.2\\=0.00198$Then the numerical results (subscripted by the associated variable values) are$Pr(R=T|G=T)=\frac{0.00198_{TTT} + 0.1584_{TFT}}{0.00198_{TTT}+0.288_{TTF}+0.1584_{TFF}}=\frac{891}{2491}=35.77\%$ Do this with pymc3
###Code
import pymc3 as mc3
import numpy as np
###Output
_____no_output_____ |
05_regression.ipynb | ###Markdown
Regression Example
###Code
PATH = Path.cwd()
###Output
_____no_output_____
###Markdown
We can Load the supplied Adiac
###Code
df_train, df_test = load_df_ucr(PATH, 'Adiac')
###Output
Loading files from: /home/tcapelle/SteadySun/timeseries_fastai/Adiac
###Markdown
Bonjour let's modify the data to make target col continous.
###Code
df_train.target = df_train.target.astype(float)
df_test.target = df_test.target.astype(float)
x_cols = df_train.columns[0:-1].to_list()
###Output
_____no_output_____
###Markdown
we have to pass a `y_block` of type `RegressionBlock`
###Code
dls = TSDataLoaders.from_dfs(df_train, df_test, x_cols=x_cols, label_col='target', y_block=RegressionBlock, bs=16)
dls.show_batch()
###Output
_____no_output_____
###Markdown
We can check, that the dls has a MSE loss now
###Code
dls.loss_func
###Output
_____no_output_____
###Markdown
the output now is one continous variables
###Code
resnet = create_resnet(1, 1)
learn = Learner(dls, resnet, metrics=[mse])
learn.fit_one_cycle(5, 1e-3)
###Output
_____no_output_____ |
Thermodynamics_an Eng Approach_5Ed_Cengel_IDN/Chapter_2_1.ipynb | ###Markdown
Chapter 2: Energy Conversion and General Energy Analysis Example 2-1 ,Page No.57 __2-1 Mobil bertenaga nuklir__Biasanya mobil mengkonsumsi 5 liter bensin per hari, dan kapasitas tangki bahan bakar sekitar 50 liter. Dengan demikian mobil rerata memerlukan isi ulang setiap 10 hari sekali. Densitas dari bensin berkisar antara 0,68 hingga 0,78 kg/L, dan *lower heating value* (LHV) bensin tersebut adalah 44.000 kJ/kg ( maknanya adalah 44000 kJ kalor yang dikeluarkan dari 1 kg bensin jika terbakar secara penuh/komplit). seandainya permasalahan nuklir yang berkaitan dengan radioaktif dan pengelolaan limbahnya sudah teratasi, maka mobil dapat digerakan oleh tenaga nuklir U-235. bila mobil jenis tersebut dibekali dengan 0,1 kg bahan bakar nuklir U-235 Tentukan kapan saat mobil memerlukan isi ulang kembali bila dikendarai dengan pengendaraan rerata.
###Code
# Diketahui:
rho_b=0.75;# ambil rerata densitas bensin, 0,75 kg/L
V_b=5;# konsumsi BB mobil per hari, 5 L
Hv_b=44000; #heat value, kJ/kg
m_u235=0.1;# massa U-235 yang dipakai
#Konstanta:
H_u235=6.73*10**10;#Energi yang dihasilkan dari U-235
#Dicari: hari dan tahun isi ulang bensin jika memakai U-235
m_b=rho_b*V_b;#massa bensin terpakai perhari
E_b=m_b*Hv_b; #Energi yang dihasilkan dari BB bensin perhari
E_u235=m_u235*H_u235;
hari=E_u235/E_b;
print('Dengan U-235, mobil dapat dikendarai sepanjang %f hari' %round(hari,0))
print('Sama dengan %f tahun' %round(hari/365,0))
###Output
Dengan U-235, mobil dapat dikendarai sepanjang 40788.000000 hari
Sama dengan 112.000000 tahun
###Markdown
Example 2-2 ,Page No.59 PLTBPada sebuah pembangkit listrik tenaga Bayu (PLTB) telah diketahui bahwasanya angin berhembus secara *steady* dengan kecepatan 8,5 m/s. Tentukan energi angin (a) persatuan masa, (b) jika massa 10 kg, dan (c) jika aliran udaranya per satuan waktu(flow rate) sebesar 1154 kg/s.
###Code
# Diketahui:
v=8.5;# kecepatan angin, m/s
m=10;# massa, kg
m_dot=1154;# massa persatuan waktu (flow rate), kg/s
#Dicari: (a) Energi angin persatuan massa, e [J/kg]
#(b) Energi angin untuk massa udara sebesar 10 kg
#(c) Enegi angin jika mass flow rate sebesar 1154 kg/s
#Jawab:
e=(v**2)/2; #energi kinetik = v^2/2
print('Energi angin persatuan massa %f J/kg' %round(e,1));
E=m*e; #energi angin, E = massa * energi
print('wind energy for 10 kg mass %i J' %E);
E_dot=m_dot*e/1000; #energi angin dengan mass flow rate 1154 kg/s
print('wind energy for mass flow rate of 1154kg/s %f kW'%round(E_dot,1))
###Output
Energi angin persatuan massa 36.100000 J/kg
wind energy for 10 kg mass 361 J
wind energy for mass flow rate of 1154kg/s 41.700000 kW
###Markdown
Example 2-7 ,Page No.67
###Code
import math
# Given values
T=200;# applied torque in N
n=4000;# shaft rotation rate in revolutions per minute
#Calculation
Wsh=(2*math.pi*n*T)/1000/60;#factor of 1000 to convert to kW and 60 to convert to sec
print'Power transmitted %f kW'%round(Wsh,1)
###Output
Power transmitted 83.800000 kW
###Markdown
Example 2-8 ,Page No.69 **2-8 Daya yang diperlukan sebuah mobil untuk menaiki bukit**Terdapat sebuah mobil dengan massa 1200 kg yang berjalan di jalan yang rata dengan kecepatan 90 km/jam. Kemudian mobil memulai untuk mendaki sebuah bukit dengan ketinggian 30 derajat dari garis horizontal. bila kecepatan mobil dibiarkan tetap selama proses menaiki Bukit tersebut, Tentukan tenaga tambahan yang diperlukan dari mesinnya.
###Code
import math
#Konstanta
g=9.81;#gravitasi bumi, m/s^2;
#Diketahui:
m_mobil=1200;#massa mobil, kg
V=90/3.6;#kecepatan, konversi km/h into m/s
#Konversi:
d=30*math.pi/180;#sudut kemiringan; konversi ke radians
kW_HP = 1.34102 # 1 kW = 1.34102 HP
#Jawab:
V_ver=V*math.sin(d);#velocity in vertical direction
W_g_dot=m_mobil*g*V_ver/1000;#daya yang dibutuhkan
W_g_dot_HP= W_g_dot*kW_HP
print('Daya yang dibutuhkan adalah %i kW'%W_g_dot)
print('Dinyatakan dalam HP, daya yang diperlukan adalah %f kW' %W_g_dot_HP)
###Output
Daya yang dibutuhkan adalah 147 kW
Dinyatakan dalam HP, daya yang diperlukan adalah 197.331093 kW
###Markdown
Example 2-9 ,Page No.69 **2-9 Tenaga yang diperlukan sebuah mobil untuk melakukan akselerasi**Tentukan tenaga yang diperlukan untuk mobil dengan massa 900 kg guna melakukan akselerasi dari keadaan berhenti menjadi berkecepatan 80 km/jam dalam waktu 20 detik di kondisi jalan yang rata.
###Code
#Diketahui:
m=900;#massa mobil, kg
v_1=0;# kecepatan awal
v_2=80/3.6;# kecepatan akhir; konversi km/h ke m/s
t=20;# waktu yang diperlukan, detik, s
#Konversi:
kW_HP = 1.34102 # 1 kW = 1.34102 HP
#Dicari: Daya yang diperlukan untuk akselerasi
W_a=m*(v_2**2-v_1**2)/2/1000;
W_a_dot=W_a/t;
W_a_dot_HP = W_a_dot*kW_HP
print('Daya rerata yang diperlukan sebesar %f kW'%round(W_a_dot,1))
print('Dinyatakan dalam HP, daya rerata tersebut adalah %f kW' %W_a_dot_HP)
###Output
Daya rerata yang diperlukan sebesar 11.100000 kW
Dinyatakan dalam HP, daya rerata tersebut adalah 14.900222 kW
###Markdown
Example 2-10 ,Page No.74 **2-10 Mendinginkan fluida panas di dalam sebuah tangki**Sebuah tangki rigid berisi fluida panas yang kemudian didinginkan dengan cara diaduk menggunakan baling-baling titik pada awalnya internal Energy dari fluida tersebut adalah 800 KJ. dalam proses pendinginan, fluida Mengalami penurunan 500 KJ kalor, dan baling-baling melakukan 100 kJ kerja terhadap ruida tersebut. Tentukan energi internal akhir dari fluida itu. energi yang tersimpan dalam baling-baling diabaikan.
###Code
#Diketahui
W_in=100;# kerja yang diberikan, kJ
Q_out=500;# kalor yang hilang, kJ
U_i=800;# energi internal fluida, kJ
#Dicari: energi internal akhir (U_f)
# W_in - Q_out = U_f - U_i i.e change in internal energy
U_f = U_i - Q_out + W_in;
print('Energi Internal akhir sebesar %i kJ'%U_f)
###Output
Energi Internal akhir sebesar 400 kJ
###Markdown
Example 2-11 ,Page No.75
###Code
import math
#given values
Win=20;# power consumption in W
mair=0.25;# rate of air discharge in kg/sec
#calculation
v=math.sqrt(Win/2/mair)#Win = 1/2*m*v^2
if v >=8:
print('True');
else:
print('False')
###Output
False
###Markdown
Example 2-12 ,Page No.76
###Code
#Given values
Win=200.0;#Power of fan in W
U=6.0;#Overall heat transfer coefficient in W/m^2 C
A=30;#Surface area in m^2
To=25;#Outdoor temperature in C
#Calculations
Ti= (Win/U/A)+To;# Win = Qout = U*A*(Ti - To)
print'the indoor air temperature %f Celcius'%Ti
###Output
the indoor air temperature 26.111111 Celcius
###Markdown
Example 2-13 ,Page No.76
###Code
#Given values
Plamp=80.0;#Power of lamp in W
N=30;#no of lamps
t=12;#time period the light is in use in hours/day
y=250;#days in a year light is in function
UC=0.07;#unit cost in $
#Calculation
LP=Plamp*N/1000;#Lighting power in kW
OpHrs=t*y;#Operating hours
LE=LP*OpHrs;#Lighting energy in kW
LC=LE*UC;#Lighting cost
print'the annual energy cost $%i'%LC
###Output
the annual energy cost $504
###Markdown
Example 2-15 ,Page No.82
###Code
#Given values
Ein=2.0;#Power of electric burner in kW
n1=0.73;#Efficiency of open burners
n2=0.38;#efficency of gas units
CinH=0.09;#Unit cost of electricity in $
CinB=0.55;#Unit cost of natural gas in $
#Calculations
QutH= Ein * n1;
print'rate of energy consumption by the heater %f kW'%round(QutH,2);
CutH= CinH / n1;
print'the unit cost of utilized energy for heater $%f/kWh'%round(CutH,3);
QutB= QutH / n2 ;
print'rate of energy consumption by the burner %f kW'%round(QutB,2);
CutB= CinB / n2 / 29.3; # 1 therm = 29.3 kWh
print'the unit cost of utilized energy for burner %f kWh'%round(CutB,3);
###Output
rate of energy consumption by the heater 1.460000 kW
the unit cost of utilized energy for heater $0.123000/kWh
rate of energy consumption by the burner 3.840000 kW
the unit cost of utilized energy for burner 0.049000 kWh
###Markdown
Example 2-16 ,Page No.84
###Code
#there is a 0.204% error in the last part of the question due to rounding off the intermidiate steps in the solution
#Constants used
g=9.81;#acceleration due to gravity in m/s^2;
#Given values
h=50.0;#Depth of water in m
m=5000.0;#mass flow rate of water in kg/sec
Wout=1862.0;#generated electric power in kW
ngen=0.95;#efficiency of turbine
#calculation
X=g*h/1000.0;# X stands for the differnce b/w change in mechanical energy per unit mass
R=m*X;#rate at which mech. energy is supplied to turbine in kW
nov=Wout/R;#overall efficiency i.e turbine and generator
print'overall efficiency is %f'%round(nov,2);
ntu=nov/ngen;#efficiency of turbine
print'efficiency of turbine is %f'%round(ntu,2);
Wsh=ntu*R;#shaft output work
print'shaft power output %i kW'%round(Wsh,0)
###Output
overall efficiency is 0.760000
efficiency of turbine is 0.800000
shaft power output 1960 kW
###Markdown
Example 2-17 ,Page No.85
###Code
#Given values
Pstd=4520.0;
Phem=5160.0;#prices of std and high eff motor in USD
R=60*0.7457;#rated power in kW from hp
OpHrs=3500.0;#Operating hours
Lf=1.0;#Load Factor
nsh=0.89;#efficiency of shaft
nhem=0.932;#efficiency of high eff. motor
CU=0.08;#per unit cost in $
#calculation
PS=R*Lf*(1/nsh-1/nhem);#Power savings = W electric in,standard - W electric in,efficient
ES=PS*OpHrs;#Energy savings = Power savings * Operating hours
print'Energy savings %i kWh/year'%ES;
CS=ES*CU;
print'Cost savings per year $%i'%CS;
EIC=Phem-Pstd;#excess intial cost
Y=EIC/CS;
print'simple payback period %f years'%round(Y,1)
###Output
Energy savings 7929 kWh/year
Cost savings per year $634
simple payback period 1.000000 years
###Markdown
Example 2-18 ,Page No.91
###Code
#Given values
#NOx details
m1=0.0047;#emissions of gas furnaces of NOx in kg/therm
N1=18*10**6;#no. of therms per year
#CO2 details
m2=6.4;#emissions of gas furnaces of CO2 in kg/therm
N2=18*10**6;#no. of therms per year
#Calculation
NOxSav=m1*N1;
print'NOx savings %f kg/year'%round(NOxSav,1);
CO2Sav=m2*N2;
print'CO2 savings %f kg/year'%round(CO2Sav,1)
###Output
NOx savings 84600.000000 kg/year
CO2 savings 115200000.000000 kg/year
###Markdown
Example 2-19 ,Page No.95
###Code
#Constants used
e=0.95;#Emissivity
tc=5.67*10**-8;#thermal conductivity in W/m^2 K^4
#Given values
h=6;#convection heat transfer coefficient in W/m^2 C
A=1.6;#cross-sectional area in m^2
Ts=29;#average surface temperature in C
Tf=20;#room temperature in C
#Calculation
#convection rate
Q1=h*A*(Ts-Tf);
#radiation rate
Q2=e*tc*A*((Ts+273)**4-(Tf+273)**4);
Qt=Q1+Q2;
print'the total rate of heat transfer %f W'%round(Qt,1)
###Output
the total rate of heat transfer 168.100000 W
|
Practical_Statistics/Practical_Statistics/10_Hypothesis Testing/Other Things to Consider - Multiple Testing.ipynb | ###Markdown
Multiple TestsIn this notebook, you will work with a similar dataset to the judicial dataset you were working with before. However, instead of working with decisions already being provided, you are provided with a p-value associated with each individual. Use the questions in the notebook and the dataset to answer the questions at the bottom of this page.Here is a glimpse of the data you will be working with:
###Code
import numpy as np
import pandas as pd
df = pd.read_csv('../data/judicial_dataset_pvalues.csv')
df.head()
###Output
_____no_output_____
###Markdown
`1.` Remember back to the null and alternative hypotheses for this example. Use that information to determine the answer for **Quiz 1** and **Quiz 2** below. **A pvalue is the probability of observing your data or more extreme data, if the null is true. Type I errors are when you choose the alternative when the null is true, and vice-versa for Type II. Therefore, deciding an individual is guilty when they are actually innocent is a Type I error. The alpha level is a threshold for the percent of the time you are willing to commit a Type I error.** `2.` If we consider each individual as a single hypothesis test, find the conservative Bonferroni corrected p-value we should use to maintain a 5% type I error rate.
###Code
bonf_alpha = 0.05/df.shape[0]
bonf_alpha
###Output
_____no_output_____
###Markdown
`3.` What is the proportion of type I errors made if the correction isn't used? How about if it is used? Use your answers to find the solution to **Quiz 3** below. **In order to find the number of type I errors made in without the correction - we need to find all those that are actually innocent with pvalues less than 0.05.**
###Code
df.query("actual == 'innocent' and pvalue < 0.05").count()[0]/df.shape[0] # If not used
df.query("actual == 'innocent' and pvalue < @bonf_alpha").count()[0]/df.shape[0] # If used
###Output
_____no_output_____ |
research/02 - Data Preprocessing.ipynb | ###Markdown
EA Assignment 02 - Data Preprocessing__Authored by: Álvaro Bartolomé del Canto (alvarobartt @ GitHub)__--- We will start this Jupyter Notebook with a little recap from the previous one named `01 - Data Exploration.ipynb` where we explored the available data and extracted some conclusion and useful details that may be useful during this Jupyter Notebook, so please, check the previous Notebook before proceeding.So on, we will be using the same `Loading Data` and `Cleaning Data` Jupyter cells in order to load the data and clean it (since there were some invalid/duplicated values), respectively. Loading Data
###Code
import glob
directories = glob.glob('../documents_challenge/*')
directories
data = list()
%%time
for directory in directories:
context = directory.split('/')[-1].lower()
for subdir in glob.glob(f"{directory}/*"):
lang = subdir.split('/')[-1].lower()
for file in glob.glob(f"{subdir}/*"):
data.append({
'lang': lang,
'context': context,
'text': open(file, 'r').read()
})
import pandas as pd
data = pd.DataFrame(data)
data.head()
###Output
_____no_output_____
###Markdown
Cleaning Data
###Code
duplicated_data = data[data.duplicated(subset=('text',), keep='first')]
duplicated_data
data.drop_duplicates(subset=('text',), keep='first', inplace=True)
data.shape
data = data[data['text'] != 'translation not available']
data.shape
###Output
_____no_output_____
###Markdown
__At this point we have already loaded and cleaned the data as defined in the previous Jupyter Notebook, so now we can proceed with the NLP Data Preprocessing.__ --- Defining Pre-Processing Steps As already stated, the preprocessing is one of the most relevant steps in a NLP pipeline, since when we preprocess text we intend to give additional value to the text, which means that we are enriching our raw data in order to help the model out before we feed it.When it comes to NLP preprocessing there are some common steps since it is usual that the text is not unified into lower case, so it contains both upper and lower characters, a common piece of text contains stopwords such as pronouns, determinants, etc., if the text has been downloaded from Internet it may contain HTML tags, it may also contain multiple spaces or line breaks, etc.So we will just try to cover that in a really generic way first, but then in a more detailed one, since the stopwords are different depending on the language, a language model can be applied for either stemming or lemmatization, etc. Text Cleaning We will use the Python library `unidecode` for the text cleaning so as to transform any string into a unidecoded one, which in our case, this library will just remove all the accents from both Spanish and French text, and maybe from English texts if there's any (by mistake or maybe because the text mentions words in other languages).
###Code
from unidecode import unidecode
###Output
_____no_output_____
###Markdown
Unidecode example/s presented below:
###Code
unidecode("Même si je travaille chez EA, j'aimerais continuer à étudier")
unidecode("Aunque trabaje en EA, me gustaría seguir estudiando")
###Output
_____no_output_____
###Markdown
As we may have seen, the accents have dissappeared but the vowels which contained those accents are still in there, so they have not been removed. Regular Expressions Now we will proceed with defining the regular expresions, which in this case as already mentioned, will be used for: removing the URLs (URL_PATTERN), removing the HTML tags and values (HTML_PATTERN), removing the punctuation signs/marks (PUNCTUATION_PATTERN), to just keep the characters and discard the numbers and any other characters (NUMBER_PATTERN) and to remove multiple spaces (SPACES_PATTERN).
###Code
import re
URL_PATTERN = re.compile(r'http[s]?://(?:[a-zA-Z]|[0-9]|[$-_@.&+]|[!*\(\),]|(?:%[0-9a-fA-F][0-9a-fA-F]))+')
HTML_PATTERN = re.compile(r'<.*?>|&([a-z0-9]+|#[0-9]{1,6}|#x[0-9a-f]{1,6});')
PUNCTUATION_PATTERN = re.compile(r'[^\w\s]')
NUMBER_PATTERN = re.compile(r'[\d]+')
SPACES_PATTERN = re.compile(r'[ ]{2,}')
###Output
_____no_output_____
###Markdown
Regular Expressions example/s presented below:
###Code
URL_PATTERN.findall("Please, visit our web at https://www.ea.com/")
HTML_PATTERN.sub(" DELETE ", "<b>Avez-vous déjà joué à Rocket Arena?</b>")
PUNCTUATION_PATTERN.findall("¿Qué tal ha parecido la presentación de EA?, mola, ¿no?")
NUMBER_PATTERN.sub(" DELETE ", "I've just spent up to 345 hours playing EA's Harry Potter Quidditch Word Cup game.")
SPACES_PATTERN.findall("Les jeux EA? simplement le meilleur")
###Output
_____no_output_____
###Markdown
As we have already seen, the regular expressions work as expected since they replace, remove, find, etc. the matching parts of the text to the introduced regular expression in each case. Stopwords Removal So we will proceed with the next part, which is the stopword removal part, where we will use a corpus from one of the main NLP libraries for Python which is named NLTK (Natural Language Tool Kit), which contains a corpus of stopwords in multiple languages and, in this case, in the languages we need to solve the problem we are facing to.
###Code
from nltk.corpus import stopwords
spanish_stopwords = stopwords.words('spanish')
english_stopwords = stopwords.words('english')
french_stopwords = stopwords.words('french')
###Output
_____no_output_____
###Markdown
Stopwords removal example/s presented below:
###Code
texto = "me gustaría trabajar en ea".split()
for palabra in spanish_stopwords:
texto = list(filter((palabra.lower()).__ne__, texto))
print(' '.join(texto))
texte = "j'aimerais travailler chez ea".split()
for mot in french_stopwords:
texte = list(filter((mot.lower()).__ne__, texte))
print(' '.join(texte))
text = "i'd love to work for ea".split()
for word in english_stopwords:
text = list(filter((word.lower()).__ne__, text))
print(' '.join(text))
###Output
i'd love work ea
###Markdown
As we may have seen the stopword removal does not work as well as expected fot both English and French, since those languages tend to use the apostrophe (') to shorten words that appear together, mainly after personal pronouns when they are followed by a verb which starts by a vowel or some other rules. Additional Steps Anyway, we will define a step in order to solve it, which will split the characters before and after the apostrophe, and the apostrophe will be removed.
###Code
"j'aimerais travailler chez ea".replace("'", " ")
###Output
_____no_output_____
###Markdown
As we can see above, we fixed this issue creating a new rule which should be applied to both English and French texts in order to split the words that contain an apostrphe and removing the apostrophe. Anyway, we will still include this step into the Spanish Preprocessing Pipeline, since some words from other languages may be found so as to ensure that they are all preprocessed the same way. Stemming (Discarded) Finally, we will proceed with the Stemming, which is a NLP method to reduce each word to their root, which means that different words with the same root with be transformed to their root so that those words are the same, which can add value to the preprocessing since we have a lot of different words from different language, and this is a way to reduce the size of the input data we will be using to feed the model.In this case, we will also be using NLTK since it contains stemmers for English, Spanish and French, which should cover all our needs for now. So on, we will just transform each word to its root.
###Code
from nltk.stem import SnowballStemmer
english_stemmer = SnowballStemmer('english')
spanish_stemmer = SnowballStemmer('spanish')
french_stemmer = SnowballStemmer('french')
###Output
_____no_output_____
###Markdown
Stemming example/s presented below:
###Code
texto = "algun dia trabajaré para ea".split()
resultado = list()
for palabra in texto:
resultado.append(spanish_stemmer.stem(palabra))
print(' '.join(resultado))
text = "someday i'll be working for ea".split()
result = list()
for word in text:
result.append(english_stemmer.stem(word))
print(' '.join(result))
texte = "un jour je travaillerai pour ea".split()
resultat = list()
for mot in texte:
resultat.append(french_stemmer.stem(mot))
print(' '.join(resultat))
###Output
un jour je travaill pour ea
###Markdown
__Update__: Stemming will be removed, since we will not know the language in which an input text is written, and the model is supposed to classify any input text in its context regarless the language, so we do not need anything this specific on the preprocessing part. Conclusion So on, in order to conclude, we already defined and tested all the NLP preprocessing steps that we will need to accomplish the task of preprocessing the data so as to feed the model in the next notebook. Anyway, we still need to implement it as some Python interfaces regarding the language, so when data is received, we will just need to apply the defined preprocessing function using the required interface. --- PreProcessing Pipeline Once we designed all the NLP Preprocessing pipeline steps we will procceed to its implementation over a random sample text so as to see how it works and in order to initially evaluate its performance.
###Code
from random import choice
sample_lang, sample_context, sample_text = data.iloc[choice(range(len(data)))]
sample_lang, sample_context, sample_text
sample_text = sample_text.replace('\t', ' ').replace('\n', ' ')
sample_text
sample_text = unidecode(sample_text)
sample_text
patterns = (
URL_PATTERN, HTML_PATTERN, PUNCTUATION_PATTERN,
NUMBER_PATTERN, SPACES_PATTERN
)
for pattern in patterns:
sample_text = pattern.sub(' ', sample_text)
sample_text
sample_text = sample_text.strip().lower()
sample_text
sample_text = sample_text.replace("'", " ")
sample_text
stopwords = english_stopwords if sample_lang == 'en' else spanish_stopwords if sample_lang == 'es' else french_stopwords
sample_text = sample_text.split(' ')
for word in stopwords:
sample_text = list(filter((word.lower()).__ne__, sample_text))
sample_text = ' '.join(sample_text)
sample_text
sample_text = SPACES_PATTERN.sub(' ', sample_text)
sample_text
###Output
_____no_output_____
###Markdown
--- PreProcessing Interface Now, once the research has been made and the preprocessing pipeline has been tested, we will just proceed with the Python implementation of an Interface in order to create a single preprocessing pipeline to preprocess all the data available in the previously loaded dataset.
###Code
BASE_PATTERNS = (
URL_PATTERN, HTML_PATTERN, PUNCTUATION_PATTERN,
NUMBER_PATTERN, SPACES_PATTERN
)
###Output
_____no_output_____
###Markdown
__Note__: below you can see that there are some additional stopwords, since in the extra version of this Jupyter Notebook that can be found in `research/02 - Extra Data Preprocessing.ipynb` we have applied a TF-IDF Vectorizer over the preprocessed data generated below. So on, this Jupyter Notebook has been run twice, and the extra version just contains additional resources that are already implemented in this Notebook since this is the final version.
###Code
STOPWORDS = english_stopwords + spanish_stopwords + french_stopwords
ADDITIONAL_STOPWORDS = [
'much', 'despues', 'first', 'categoria', 'aqui', 'thumb', 'also', 'tres', 'asi',
'three', 'one', 'still', 'aquella', 'like', 'aquel', 'mas', 'tal', 'tan', 'hacia',
'went', 'two', 'new', 'even', 'would', 'tras', 'could', 'pues', 'without', 'category',
'many', 'twoone', 'tambien', 'well', 'solo', 'dos'
]
STOPWORDS += ADDITIONAL_STOPWORDS
STOPWORDS = set(list(STOPWORDS))
class CustomPreProcessor(object):
"""
Custom PreProcessor
Preprocesses the introduced raw text to transform it into clean text. This
preprocessing pipe is regex based.
>>> from apinlp.nlp.preprocessing import CustomPreProcessor
>>> preprocessor = CustomPreProcessor()
>>> print(preprocessor._preprocess("Visit us at https://www.ea.com/"))
"visit"
"""
def __init__(self, strip_accents=True):
self.strip_accents = strip_accents
self.patterns = BASE_PATTERNS
self.additional_patterns = (SPACES_PATTERN,)
self.stopwords = STOPWORDS
def _preprocess(self, text):
"""Cleans and applies a preprocessing layer to raw text"""
text = text.replace('\t', ' ').replace('\n', ' ')
if self.strip_accents:
text = unidecode(text)
for pattern in self.patterns:
text = pattern.sub(' ', text)
text = text.strip().lower()
text = text.replace("'", " ")
text = [word for word in text.split(' ') if len(word) > 2]
for word in self.stopwords:
text = list(filter((word.lower()).__ne__, text))
text = ' '.join(text)
for pattern in self.additional_patterns:
text = pattern.sub(' ', text)
return text
preprocessor = CustomPreProcessor()
preprocessor._preprocess(text="Visit us at https://www.ea.com/")
preprocessor._preprocess(text="Visítanos en https://www.ea.com/")
preprocessor._preprocess(text="Visitez-nous sur https://www.ea.com/")
###Output
_____no_output_____
###Markdown
__Future Note__: additionally this feature may be included in a Python package so as to create a web service to test the models with real unseen data in order to ease its usage via an API instead of requiring to interact with the Jupyter Notebooks. --- Preprocessed Data Overview Finally, we will proceed with a simple overview on the preprocessed data, since we are applying our preprocessing interface named `CustomPreProcessor` to every single text in the dataset regardless the language. So on, this means that we are going to create a new column which will contain the preprocessed text, which we will be using later so as to vectorizer it and feed the model.
###Code
%time data['preprocessed_text'] = data['text'].apply(preprocessor._preprocess)
###Output
CPU times: user 7min 21s, sys: 245 ms, total: 7min 21s
Wall time: 7min 21s
###Markdown
Now, once the preprocessed_text data has been created, we will just drop the original text column since it is not longer useful in this project, since as we already said, we will vectorize and feed the model with the preprocessed data.
###Code
data.drop(columns=['text'], inplace=True)
data.head()
###Output
_____no_output_____
###Markdown
__Note__: so as to work with the Jupyter Notebooks without repeating the same processes over and over we will just dump it into a JSON-Lines (.jsonl) file which will contain the `pandas.DataFrame` as a JSON object on each line of the file; but due to GitHub quotas and limits this file has been included in the .gitignore, so you will not be able to see it. Otherwise, just run this Jupyter Notebook in order to generate it.
###Code
data.to_json(path_or_buf='PreProcessedDocuments.jsonl', orient='records', lines=True)
###Output
_____no_output_____ |
a-6_p_scale_test_Speziale_MgO.ipynb | ###Markdown
For high dpi displays.
###Code
%config InlineBackend.figure_format = 'retina'
###Output
_____no_output_____
###Markdown
0. Note This example compares pressure calculated from `pytheos` and original publication for the gold scale by Speiale 2001. 1. Setup
###Code
import matplotlib.pyplot as plt
import numpy as np
from uncertainties import unumpy as unp
import pytheos as eos
###Output
_____no_output_____
###Markdown
2. Calculation Generate volume strain: $V/V_0$.
###Code
eta = np.linspace(1., 0.60, 21)
print(eta)
###Output
[1. 0.98 0.96 0.94 0.92 0.9 0.88 0.86 0.84 0.82 0.8 0.78 0.76 0.74
0.72 0.7 0.68 0.66 0.64 0.62 0.6 ]
###Markdown
Assign an EOS object
###Code
speziale_mgo = eos.periclase.Speziale2001()
###Output
_____no_output_____
###Markdown
Unit-cell volume of MgO at 1 bar and 300 K:
###Code
v0 = 74.698
temp = 300.
###Output
_____no_output_____
###Markdown
Calculate absolute values of unit-cell volumes.
###Code
v = v0 * eta
###Output
_____no_output_____
###Markdown
Now calculate pressures for the given volumes at $T = 300$ K.
###Code
p = speziale_mgo.cal_p(v, temp * np.ones_like(v))
print('for T = ', temp)
for eta_i, p_i in zip(eta, p):
print("{0: .3f} {1: .2f}".format(eta_i, p_i))
###Output
for T = 300.0
1.000 0.00+/-0.00
0.980 3.37+/-0.00
0.960 7.09+/-0.00
0.940 11.22+/-0.00
0.920 15.78+/-0.01
0.900 20.83+/-0.01
0.880 26.44+/-0.02
0.860 32.66+/-0.03
0.840 39.57+/-0.04
0.820 47.27+/-0.05
0.800 55.84+/-0.07
0.780 65.41+/-0.09
0.760 76.11+/-0.11
0.740 88.09+/-0.15
0.720 101.53+/-0.19
0.700 116.65+/-0.24
0.680 133.69+/-0.29
0.660 152.94+/-0.37
0.640 174.74+/-0.46
0.620 199.50+/-0.56
0.600 227.72+/-0.70
###Markdown
Calculate volume from pressure: reverse calculation.
###Code
v = speziale_mgo.cal_v(p, temp * np.ones_like(p), min_strain=0.6)
print((v/v0))
###Output
[1. 0.98 0.96 0.94 0.92 0.9 0.88 0.86 0.84 0.82 0.8 0.78 0.76 0.74
0.72 0.7 0.68 0.66 0.64 0.62 0.6 ]
###Markdown
Plot pressure versus unit-cell volume.
###Code
import matplotlib.pyplot as plt
import uncertainties.unumpy as unp
plt.plot(unp.nominal_values(p), v);
plt.errorbar(unp.nominal_values(p), v/v0, xerr=unp.std_devs(p)*10., fmt='o');
###Output
_____no_output_____ |
7_Free.ipynb | ###Markdown
自由課題配布データの dat ディレクトリの下にはいくつかのサンプルデータがあるので,残り時間はこれを分析してみよう.ここまでで使ったデータの他に,以下のデータを用意した.- health.txt : 約2年分の 日付,体重,体脂肪率,筋肉量,最高血圧,最低血圧,心拍数 のデータ- data_20180731-20190709.txt : 月日,時刻,epoch time,気温,湿度,照度,気圧,太陽電池の電圧,太陽電池の電流,太陽電池の電力 のデータdata_20180731-20190709.txt は5秒ごとのデータを約1年分でかなり巨大 (520万行,334MB) なので,gzip で圧縮してある.このサイズになると,python で処理するにも結構大変なので,前処理としてファイルを適当に分割するなどした方がよいかも知れない.
###Code
import pandas as pd
from matplotlib import pyplot
health = pd.read_csv("./dat/health.txt", sep='\t',
skiprows=[0],
names=('date', 'w', 'f', 'm', 'bph', 'bpl', 'bpm'),
index_col='date',
parse_dates=True)
pyplot.plot(health['bph'])
pyplot.plot(health['bpl'])
pyplot.show()
print(health.corr())
###Output
_____no_output_____ |
Lab8/lab8_0.ipynb | ###Markdown
Lab 8
###Code
# Import Libraries
import numpy as np
import pandas as pd
from sklearn import datasets
from sklearn.cluster import KMeans
# Load dataset
dataset=datasets.load_breast_cancer()
dataset
print(dataset.data.shape)
print(dataset.target.shape)
# Create & train model
kmeans = KMeans(n_clusters=10, random_state=0)
prediction = kmeans.fit_predict(dataset.data)
prediction
kmeans.cluster_centers_.shape
# Scatter plot of the data points
import matplotlib.pyplot as plt
fig, ax = plt.subplots(2, 5, figsize=(8, 3))
centers = kmeans.cluster_centers_.reshape(10, 6, 5)
for axi, center in zip(ax.flat, centers):
axi.set(xticks=[], yticks=[])
axi.imshow(center, interpolation='nearest', cmap=plt.cm.binary)
import numpy as np
from scipy.stats import mode
labels = np.zeros_like(prediction)
for i in range(10):
mask = (prediction == i)
labels[mask] = mode(dataset.target[mask])[0]
from sklearn.metrics import accuracy_score
accuracy_score(dataset.target, labels)
from sklearn.metrics import confusion_matrix
import seaborn as sns
mat = confusion_matrix(dataset.target, labels)
ax = sns.heatmap(mat.T, square=True, annot=True, fmt='d', cbar=False,xticklabels=dataset.target_names,yticklabels=dataset.target_names)
#ax.set_ylim(10,10)
plt.xlabel('true label')
plt.ylabel('predicted label');
###Output
_____no_output_____ |
doc/examples/Sorensen/yaml2sbml_Sorensen.ipynb | ###Markdown
Glucose Insulin Metabolism Model by Sorensen (1985).In this notebook, the model of glucose and insulin metabolism, from the thesis of ThomasJ. Sorensen, 1985 [[1]](References), is used as an example of how the `yaml2sbml.YamlModel` class can be used to easily extend a pre-existing `yaml2sbml`model.Specifically, the model is edited to reproduce a figure from the originalthesis (Fig 71) [[1]](References). The implementation that is loaded here is based on the implementationprovided by Panunzi et al., 2020 [[2]](References). In the end, the model is exported to [SBML](http://sbml.org/) and simulated via [AMICI](https://github.com/AMICI-dev/AMICI). Extend the current YAML modelThe Sorensen model has already been encoded in the YAML format. Within this notebook, the model is loaded, extended in order to model an intravenous infusion administration of glucose, using the `yaml2sbml` Model editor. The extended model is then plotted, to reproduce a figure from Sorensens PhD thesis. Load the model
###Code
import yaml2sbml
from yaml2sbml import YamlModel
yaml_model = YamlModel.load_from_yaml('Sorensen1985.yaml')
###Output
_____no_output_____
###Markdown
Define the glucose infusion termAn intravenous infusion administration of glucose is added to the model. After 17 minutes of initialization, glucose is infused at a rate of 64.81 mM/min for a three-minute period.In the YAML model, this is represented by a novel term `IVG` ("intravenous glucose"), that is set by a step function in an assignment rule.
###Code
# describe the intravenous glucose infusion
yaml_model.add_assignment('IVG', formula='piecewise(64.81, 17 <= t && t < 20, 0)')
###Output
_____no_output_____
###Markdown
Add the glucose infustion term to the model`IVG` is now added to the ODE of the glucose concentration of heart and lung space `GlucH`. Therefore, the current ODE is overwritten.
###Code
# Get the current ODE.
gluch_ode_old = yaml_model.get_ode_by_id('GlucH')
gluch_rhs_old = gluch_ode_old['rightHandSide']
# Modify the ODE. `IVG` is divided by the volume term `VolGH` to
# model concentration.
gluch_rhs_new = gluch_rhs_old + ' + (IVG / VolGH)'
# Set the new ODE.
yaml_model.add_ode(state_id='GlucH',
right_hand_side=gluch_rhs_new,
initial_value=gluch_ode_old['initialValue'],
overwrite=True)
###Output
_____no_output_____
###Markdown
Export to SBML
###Code
# Write to SBML
yaml_model.write_to_sbml('Fig71_Sorensen1985.xml', overwrite=True)
###Output
_____no_output_____
###Markdown
Simulation in AMICIThe model is now setup to reproduce to the figure. The utility function `simulate_and_plot_sorensen` simulates and plots the Sorensen model in [AMICI](https://github.com/AMICI-dev/AMICI).
###Code
%matplotlib inline
from utils import simulate_and_plot_sorensen
simulate_and_plot_sorensen('Fig71_Sorensen1985.xml')
###Output
_____no_output_____ |
bbdd/Insertar en multidimensional.ipynb | ###Markdown
Insertar en multidimensional datos Seleccionar colección
###Code
import pymongo
myclient = pymongo.MongoClient("mongodb://localhost:27017/")
mydb = myclient["stum_for_you"]
mycol = mydb["multidimensional"]
print(mydb.list_collection_names())
###Output
['usuarios']
###Markdown
Insertar datos
###Code
precio_final = 38
tipo_movimiento= "compra"
cantidad= 19
anyo= 2019
mes= 11
dia= 26
puntero_juego= 10482
genero= ['action', 'casual', 'RPG']
precio= 4
clasificacion= "INDIE"
puntero_proveedor= 33
pais= "Macedonia"
valoracion= 5
if(tipo_movimiento == "venta"):
datos = {
"movimiento":{"precio_final":precio_final, "tipo_movimiento": tipo_movimiento, "cantidad": cantidad},
"descuento":{"porcentaje":porcentaje,"fecha_inicio":fecha_inicio,"fecha_fin":fecha_fin},
"fecha":{"anyo":anyo, "mes":mes,"dia":dia},
"juego":{"puntero_juego":puntero_juego,"genero":genero,"precio":precio,"clasificacion":clasificacion},
"dlc":{"precio":precio,"tipo":tipo},
"ubicacion":{"pais":pais,"ciudad":ciudad},
"usuario":{"sexo":sexo,"fechaNacimiento":fechaNacimiento,"pais":pais,"ciudad":ciudad},
"descuento_personalizado":{"porcentaje":porcentaje_personalizado,"fecha_inicio":fecha_inicio_personalizado,"fecha_fin":fecha_fin_personalizado, "tipo":tipo_personalizado}
}
else:
datos = {
"movimiento":{"precio_final":precio_final, "tipo_movimiento": tipo_movimiento, "cantidad": cantidad},
"fecha":{"anyo":anyo, "mes":mes,"dia":dia},
"juego":{"puntero_juego":puntero_juego,"genero":genero,"precio":precio,"clasificacion":clasificacion},
"proveedor":{"puntero_proveedor":puntero_proveedor,"pais":pais,"valoracion":valoracion}
}
x = mycol.insert_one(datos)
precio_final = 38
tipo_movimiento= "compra"
cantidad= 19
anyo= 2019
mes= 11
dia= 26
puntero_juego= 10482
genero= ['action', 'casual', 'RPG']
precio= 4
clasificacion= "INDIE"
puntero_proveedor= 33
pais= "Macedonia"
valoracion= 5
if(tipo_movimiento == "venta"):
datos = {
"movimiento":{"precio_final":precio_final, "tipo_movimiento": tipo_movimiento, "cantidad": cantidad},
"descuento":{"porcentaje":porcentaje,"fecha_inicio":fecha_inicio,"fecha_fin":fecha_fin},
"fecha":{"anyo":anyo, "mes":mes,"dia":dia},
"juego":{"puntero_juego":puntero_juego,"genero":genero,"precio":precio,"clasificacion":clasificacion},
"dlc":{"precio":precio,"tipo":tipo},
"ubicacion":{"pais":pais,"ciudad":ciudad},
"usuario":{"sexo":sexo,"fechaNacimiento":fechaNacimiento,"pais":pais,"ciudad":ciudad},
"descuento":{"porcentaje":porcentaje,"fecha_inicio":fecha_inicio,"fecha_fin":fecha_fin, "tipo":tipo}
}
else:
datos = {
"movimiento":{"precio_final":precio_final, "tipo_movimiento": tipo_movimiento, "cantidad": cantidad},
"fecha":{"anyo":anyo, "mes":mes,"dia":dia},
"juego":{"puntero_juego":puntero_juego,"genero":genero,"precio":precio,"clasificacion":clasificacion},
"proveedor":{"puntero_proveedor":puntero_proveedor,"pais":pais,"valoracion":valoracion}
}
mycol.insert_one(datos)
x=mycol.find_one()
print(x)
###Output
{'_id': ObjectId('5e18d1d236678c21d442d478'), 'movimiento': {'precio_final': 38, 'tipo_movimiento': 'compra', 'cantidad': 19}, 'fecha': {'anyo': 2019, 'mes': 11, 'dia': 26}, 'juego': {'puntero_juego': 10482, 'genero': ['action', 'casual', 'RPG'], 'precio': 4, 'clasificacion': 'INDIE'}, 'proveedor': {'puntero_proveedor': 33, 'pais': 'Macedonia', 'valoracion': 5}}
|
Week 3/Week 3_Capstone_All_Parts.ipynb | ###Markdown
***Coursera Week 3 Submission Part-2 (Continued after Part-1)***Use the Notebook to build the code to scrape the following Wikipedia page, https://en.wikipedia.org/wiki/List_of_postal_codes_of_Canada:_M, in order to obtain the data that is in the table of postal codes and to transform the data into a pandas dataframe like the one shown in the coursera website.I did mention the steps below as to how I proceeded to create the final pandas Dataframe ***PART 1***
###Code
import numpy as np
import json
import geopy
from geopy.geocoders import Nominatim
import folium
import pandas as pd
pd.set_option('display.max_columns', None)
pd.set_option('display.max_rows',None)
tables = pd.read_html('https://en.wikipedia.org/wiki/List_of_postal_codes_of_Canada:_M',header = None)
required_cols = ['Postcode','Borough','Neighbourhood']
for t in tables:
if(str(np.array_equal(np.array(t.columns),np.array(required_cols))) == "True"):
pstl_data_df = pd.DataFrame(t)
break
print("Shape",pstl_data_df.shape)
pstl_data_df.head()
###Output
_____no_output_____
###Markdown
***Step 2***Only process the cells that have an assigned borough. Ignore cells with a borough that is Not assigned.
###Code
# Filter out Boroughs what have not been assigned
pstl_data_df = pstl_data_df[pstl_data_df.Borough != "not assigned"]
print("Shape", pstl_data_df.shape)
pstl_data_df.head()
###Output
Shape (287, 3)
###Markdown
***Step 3***If a cell has a borough but a Not assigned neighborhood, then the neighborhood will be the same as the borough. So for the 9th cell in the table on the Wikipedia page, the value of the Borough and the Neighborhood columns will be Queen's Park.
###Code
pstl_data_df['new_nghbr'] = np.where(pstl_data_df['Neighbourhood'] == 'Not assigned',pstl_data_df['Borough'],pstl_data_df['Neighbourhood'])
pstl_data_df.head(15)
###Output
_____no_output_____
###Markdown
***Step 4*** More than one neighborhood can exist in one postal code area.For example, in the table on the Wikipedia page, you will notice that M5A is listed twice ad has two neighborhoods:- Harbour Front- Regent ParkThe two rows above need to be combined into a single row(The neighbour hoods needs to be separated with a comma
###Code
## Hint -> Use new_nghbor column to get a grouped column
# Whenever the same Postal Code and the same Borough are present we
# have to club the Neighborhood Column using the functions
# groupby and apply
can_postal_cd_df = pd.DataFrame(pstl_data_df.groupby(['Postcode','Borough'])['new_nghbr'].apply(','.join).reset_index())
can_postal_cd_df.head()
# Renaming columns to the same on in the image given:
can_postal_cd_df = can_postal_cd_df.rename(columns = {"Postcode": "PostalCode","new_nghbr":"Neighborhood"})
print(can_postal_cd_df.head())
print("Final Shape of Data Frame : ", can_postal_cd_df.shape)
can_postal_cd_df.dtypes
###Output
_____no_output_____
###Markdown
***As we see above the shape of the new data frame created is (103,3)*** ***PART-2***
###Code
!conda install -c conda-forge geocoder --yes
import geocoder
def get_geocoder(postal_code_from_df):
# initialize your variable to None
lat_lng_coords = None
# loop until you get the coordinates
while(lat_lng_coords is None):
g = geocoder.arcgis('{}, Toronto, Ontario'.format(postal_code_from_df.strip()))
lat_lng_coords = g.latlng
latitude = lat_lng_coords[0]
longitude = lat_lng_coords[1]
return latitude,longitude
# Add the lat and long value column to the Pandas df
can_postal_cd_df['Latitude'],can_postal_cd_df['Longitude']= zip(*can_postal_cd_df['PostalCode'].apply(get_geocoder))
can_postal_cd_df.head()
print("Shape: ", can_postal_cd_df.shape)
# Map of Toronto
addr = 'Toronto, Ontario'
geolocator = Nominatim(user_agent = "toronto_ontario")
location = geolocator.geocode(addr)
latitude = location.latitude
longitude = location.longitude
print("Geographical coordinates of Toronto, Ontario are {},{}".format(latitude,longitude))
#Folium for mapping
map_toronto = folium.Map(location = [latitude, longitude],zoom_start = 11)
for lat,long,post, borough, neigh in zip(can_postal_cd_df['Latitude'], can_postal_cd_df['Longitude'],can_postal_cd_df['PostalCode'],can_postal_cd_df['Borough'],can_postal_cd_df['Neighborhood']):
label = "{} ({}): {}".format(borough, post,neigh)
popup = folium.Popup(label,parse_html = True)
folium.CircleMarker(
[lat,long],
radius = 5,
popup = popup,
color = 'green',
fill = True,
fill_color = '#3186cc',
fill_opacity = 0.8,
parse_html = False).add_to(map_toronto)
map_toronto
###Output
_____no_output_____
###Markdown
PART 3
###Code
# Step 1 - Filtering the Toronto Boroughs
can_postal_cd_df.Borough.unique()
toronto_b = ['East Toronto','Central Toronto','Downtown Toronto','West Toronto']
toronto_df = can_postal_cd_df[can_postal_cd_df['Borough'].isin(toronto_b)].reset_index(drop = True)
print("New Shape: ",toronto_df)
toronto_df.sample(30)
#Use Foursquare API
CLIENT_ID = 'JLONPWEZYTARJ1PN2FLSWHRUBQH5YEBLASLGOCUVR555BJLE'
CLIENT_SECRET = 'SP5RTEEKYS4TFFKDKG0U1I5CPEV2QMELIENUCZ3JBZZBWUID'
VERSION = '20200303'
print('credentails:')
print('CLIENT_ID: ' + CLIENT_ID)
print('CLIENT_SECRET:' + CLIENT_SECRET)
import requests
RADIUS = 500
LIMIT = 1000
recommend = []
for lat,long,post, borough, neigh in zip(toronto_df['Latitude'],toronto_df['Longitude'], toronto_df['PostalCode'], toronto_df['Borough'], toronto_df['Neighborhood']):
url = "https://api.foursquare.com/v2/venues/explore?client_id={}&client_secret={}&v={}&ll={},{}&radius={}&limit={}".format(
CLIENT_ID,
CLIENT_SECRET,
VERSION,
lat,
long,
RADIUS,
LIMIT)
postal_data = requests.get(url).json()["response"]['groups'][0]['items']
for recommend_post in postal_data:
recommend.append((
post,
borough,
neigh,
latitude,
longitude,
recommend_post['venue']['name'],
recommend_post['venue']['location']['lat'],
recommend_post['venue']['location']['lng'],
recommend_post['venue']['categories'][0]['name']))
toronto_rec = pd.DataFrame(recommend)
toronto_rec.columns = ['PostalCode', 'Borough', 'Neighborhoods', 'Borough_Lat', 'Borough_Long', 'Venue_Name', 'Venue_Lat', 'Venue_Long', 'Venue_Category']
print("The Shape of Recommendations (Postal Codes in Toronto): ",toronto_rec.shape)
toronto_rec.drop_duplicates(keep = False, inplace = True)
toronto_rec.shape
toronto_rec.head()
toronto_rec.groupby(['PostalCode', 'Borough', 'Neighborhoods'])['Venue_Name'].count()
print("Unique Venue Categories are: ",len(toronto_rec['Venue_Category'].unique()))
toronto_rec['Venue_Category'].unique()
# Analyszing Venues by area
toronto_rec = toronto_rec.drop(['Borough_Lat','Borough_Long','Venue_Lat','Venue_Long'],axis=1)
toronto_rec_df = pd.get_dummies(toronto_rec, columns =['Venue_Category'],prefix = "",prefix_sep = "")
toronto_rec_df.head()
toronto_venues_freq = toronto_rec_df.groupby(['PostalCode','Borough','Neighborhoods']).mean().reset_index()
toronto_venues_freq.head()
num_top_venues = 10
indicators = ['st', 'nd', 'rd']
# create columns according to number of top venues
areaColumns = ['PostalCode', 'Borough', 'Neighborhoods']
freqColumns = []
for ind in np.arange(num_top_venues):
try:
freqColumns.append('{}{} Most Common Venue'.format(ind+1, indicators[ind]))
except:
freqColumns.append('{}th Most Common Venue'.format(ind+1))
columns = areaColumns+freqColumns
# Create a new dataframe
all_toronto_venues = pd.DataFrame(columns=columns)
all_toronto_venues['PostalCode'] = toronto_venues_freq['PostalCode']
all_toronto_venues['Borough'] = toronto_venues_freq['Borough']
all_toronto_venues['Neighborhoods'] = toronto_venues_freq['Neighborhoods']
for ind in np.arange(toronto_venues_freq.shape[0]):
row_categories = toronto_venues_freq.iloc[ind, :].iloc[3:]
row_categories_sorted = row_categories.sort_values(ascending=False)
all_toronto_venues.iloc[ind, 3:] = row_categories_sorted.index.values[0:num_top_venues]
all_toronto_venues.sort_values(freqColumns, inplace=True)
all_toronto_venues
from sklearn.cluster import KMeans
# set number of clusters
kclusters = 10
toronto_venues_freq_clustering = toronto_venues_freq.drop(['PostalCode', 'Borough', 'Neighborhoods'], 1)
kmeans = KMeans(n_clusters=kclusters, random_state=0).fit(toronto_venues_freq_clustering)
#No data for a particular postal code so removed it
toronto_clustered_df = toronto_df.drop(toronto_df.index[toronto_df.PostalCode=='M5N'])
toronto_clustered_df['Cluster'] = kmeans.labels_
toronto_clustered_df = toronto_clustered_df.join(all_toronto_venues.drop(['Borough', 'Neighborhoods'], 1).set_index('PostalCode'), on='PostalCode')
toronto_clustered_df.sort_values(['Cluster'] + freqColumns, inplace=True)
toronto_clustered_df
import matplotlib.cm as cm
import matplotlib.colors as colors
# create map
map_clusters = folium.Map(location=[latitude, longitude], zoom_start=12)
# set color scheme for the clusters
x = np.arange(kclusters)
ys = [i+x+(i*x)**2 for i in range(kclusters)]
colors_array = cm.rainbow(np.linspace(0, 1, len(ys)))
rainbow = [colors.rgb2hex(i) for i in colors_array]
# add markers to the map
markers_colors = []
for lat, lon, post, bor, poi, cluster in zip(toronto_clustered_df['Latitude'], toronto_clustered_df['Longitude'], toronto_clustered_df['PostalCode'], toronto_clustered_df['Borough'], toronto_clustered_df['Neighborhood'], toronto_clustered_df['Cluster']):
label = folium.Popup('{} ({}): {} - Cluster {}'.format(bor, post, poi, cluster), parse_html=True)
folium.CircleMarker(
[lat, lon],
radius=5,
popup=label,
color=rainbow[cluster-1],
fill=True,
fill_color=rainbow[cluster-1],
fill_opacity=0.7).add_to(map_clusters)
map_clusters
###Output
_____no_output_____ |
Configuracion/Modelo/Base/flowers_tf_lite.ipynb | ###Markdown
To run this colab, press the "Runtime" button in the menu tab and then press the "Run all" button. Copyright 2019 The TensorFlow Authors.
###Code
#@title Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# https://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
###Output
_____no_output_____
###Markdown
Recognize Flowers using Transfer Learning Run in Google Colab View source on GitHub
###Code
from __future__ import absolute_import, division, print_function, unicode_literals
try:
# The %tensorflow_version magic only works in colab.
%tensorflow_version 2.x
except Exception:
pass
import tensorflow as tf
import os
import numpy as np
import matplotlib.pyplot as plt
tf.__version__
###Output
_____no_output_____
###Markdown
Setup Input Pipeline Download the flowers dataset.
###Code
_URL = "https://storage.googleapis.com/download.tensorflow.org/example_images/flower_photos.tgz"
zip_file = tf.keras.utils.get_file(origin=_URL,
fname="flower_photos.tgz",
extract=True)
base_dir = os.path.join(os.path.dirname(zip_file), 'flower_photos')
###Output
_____no_output_____
###Markdown
Use `ImageDataGenerator` to rescale the images.Create the train generator and specify where the train dataset directory, image size, batch size.Create the validation generator with similar approach as the train generator with the flow_from_directory() method.
###Code
IMAGE_SIZE = 224
BATCH_SIZE = 64
datagen = tf.keras.preprocessing.image.ImageDataGenerator(
rescale=1./255,
validation_split=0.2)
train_generator = datagen.flow_from_directory(
base_dir,
target_size=(IMAGE_SIZE, IMAGE_SIZE),
batch_size=BATCH_SIZE,
subset='training')
val_generator = datagen.flow_from_directory(
base_dir,
target_size=(IMAGE_SIZE, IMAGE_SIZE),
batch_size=BATCH_SIZE,
subset='validation')
for image_batch, label_batch in train_generator:
break
image_batch.shape, label_batch.shape
###Output
_____no_output_____
###Markdown
Save the labels in a file which will be downloaded later.
###Code
print (train_generator.class_indices)
labels = '\n'.join(sorted(train_generator.class_indices.keys()))
with open('labels.txt', 'w') as f:
f.write(labels)
!cat labels.txt
###Output
_____no_output_____
###Markdown
Create the base model from the pre-trained convnetsCreate the base model from the **MobileNet V2** model developed at Google, and pre-trained on the ImageNet dataset, a large dataset of 1.4M images and 1000 classes of web images.First, pick which intermediate layer of MobileNet V2 will be used for feature extraction. A common practice is to use the output of the very last layer before the flatten operation, the so-called "bottleneck layer". The reasoning here is that the following fully-connected layers will be too specialized to the task the network was trained on, and thus the features learned by these layers won't be very useful for a new task. The bottleneck features, however, retain much generality.Let's instantiate an MobileNet V2 model pre-loaded with weights trained on ImageNet. By specifying the `include_top=False` argument, we load a network that doesn't include the classification layers at the top, which is ideal for feature extraction.
###Code
IMG_SHAPE = (IMAGE_SIZE, IMAGE_SIZE, 3)
# Create the base model from the pre-trained model MobileNet V2
base_model = tf.keras.applications.MobileNetV2(input_shape=IMG_SHAPE,
include_top=False,
weights='imagenet')
###Output
_____no_output_____
###Markdown
Feature extractionYou will freeze the convolutional base created from the previous step and use that as a feature extractor, add a classifier on top of it and train the top-level classifier.
###Code
base_model.trainable = False
###Output
_____no_output_____
###Markdown
Add a classification head
###Code
model = tf.keras.Sequential([
base_model,
tf.keras.layers.Conv2D(32, 3, activation='relu'),
tf.keras.layers.Dropout(0.2),
tf.keras.layers.GlobalAveragePooling2D(),
tf.keras.layers.Dense(5, activation='softmax')
])
###Output
_____no_output_____
###Markdown
Compile the modelYou must compile the model before training it. Since there are two classes, use a binary cross-entropy loss.
###Code
model.compile(optimizer=tf.keras.optimizers.Adam(),
loss='categorical_crossentropy',
metrics=['accuracy'])
model.summary()
print('Number of trainable variables = {}'.format(len(model.trainable_variables)))
###Output
_____no_output_____
###Markdown
Train the model
###Code
epochs = 10
history = model.fit_generator(train_generator,
epochs=epochs,
validation_data=val_generator)
###Output
_____no_output_____
###Markdown
Learning curvesLet's take a look at the learning curves of the training and validation accuracy/loss when using the MobileNet V2 base model as a fixed feature extractor.
###Code
acc = history.history['accuracy']
val_acc = history.history['val_accuracy']
loss = history.history['loss']
val_loss = history.history['val_loss']
plt.figure(figsize=(8, 8))
plt.subplot(2, 1, 1)
plt.plot(acc, label='Training Accuracy')
plt.plot(val_acc, label='Validation Accuracy')
plt.legend(loc='lower right')
plt.ylabel('Accuracy')
plt.ylim([min(plt.ylim()),1])
plt.title('Training and Validation Accuracy')
plt.subplot(2, 1, 2)
plt.plot(loss, label='Training Loss')
plt.plot(val_loss, label='Validation Loss')
plt.legend(loc='upper right')
plt.ylabel('Cross Entropy')
plt.ylim([0,1.0])
plt.title('Training and Validation Loss')
plt.xlabel('epoch')
plt.show()
###Output
_____no_output_____
###Markdown
Fine tuningIn our feature extraction experiment, you were only training a few layers on top of an MobileNet V2 base model. The weights of the pre-trained network were **not** updated during training.One way to increase performance even further is to train (or "fine-tune") the weights of the top layers of the pre-trained model alongside the training of the classifier you added. The training process will force the weights to be tuned from generic features maps to features associated specifically to our dataset. Un-freeze the top layers of the model All you need to do is unfreeze the `base_model` and set the bottom layers be un-trainable. Then, recompile the model (necessary for these changes to take effect), and resume training.
###Code
base_model.trainable = True
# Let's take a look to see how many layers are in the base model
print("Number of layers in the base model: ", len(base_model.layers))
# Fine tune from this layer onwards
fine_tune_at = 100
# Freeze all the layers before the `fine_tune_at` layer
for layer in base_model.layers[:fine_tune_at]:
layer.trainable = False
###Output
_____no_output_____
###Markdown
Compile the modelCompile the model using a much lower training rate.
###Code
model.compile(loss='categorical_crossentropy',
optimizer = tf.keras.optimizers.Adam(1e-5),
metrics=['accuracy'])
model.summary()
print('Number of trainable variables = {}'.format(len(model.trainable_variables)))
###Output
_____no_output_____
###Markdown
Continue Train the model
###Code
history_fine = model.fit_generator(train_generator,
epochs=5,
validation_data=val_generator)
###Output
_____no_output_____
###Markdown
Convert to TFLite Saved the model using `tf.saved_model.save` and then convert the saved model to a tf lite compatible format.
###Code
saved_model_dir = 'save/fine_tuning'
tf.saved_model.save(model, saved_model_dir)
converter = tf.lite.TFLiteConverter.from_saved_model(saved_model_dir)
tflite_model = converter.convert()
with open('model.tflite', 'wb') as f:
f.write(tflite_model)
###Output
_____no_output_____
###Markdown
Download the converted model and labels
###Code
from google.colab import files
files.download('model.tflite')
files.download('labels.txt')
###Output
_____no_output_____
###Markdown
Let's take a look at the learning curves of the training and validation accuracy/loss, when fine tuning the last few layers of the MobileNet V2 base model and training the classifier on top of it. The validation loss is much higher than the training loss, so you may get some overfitting.You may also get some overfitting as the new training set is relatively small and similar to the original MobileNet V2 datasets.
###Code
acc = history_fine.history['accuracy']
val_acc = history_fine.history['val_accuracy']
loss = history_fine.history['loss']
val_loss = history_fine.history['val_loss']
plt.figure(figsize=(8, 8))
plt.subplot(2, 1, 1)
plt.plot(acc, label='Training Accuracy')
plt.plot(val_acc, label='Validation Accuracy')
plt.legend(loc='lower right')
plt.ylabel('Accuracy')
plt.ylim([min(plt.ylim()),1])
plt.title('Training and Validation Accuracy')
plt.subplot(2, 1, 2)
plt.plot(loss, label='Training Loss')
plt.plot(val_loss, label='Validation Loss')
plt.legend(loc='upper right')
plt.ylabel('Cross Entropy')
plt.ylim([0,1.0])
plt.title('Training and Validation Loss')
plt.xlabel('epoch')
plt.show()
###Output
_____no_output_____ |
Saniya_assignment1.ipynb | ###Markdown
---_You are currently looking at **version 1.1** of this notebook. To download notebooks and datafiles, as well as get help on Jupyter notebooks in the Coursera platform, visit the [Jupyter Notebook FAQ](https://www.coursera.org/learn/python-text-mining/resources/d9pwm) course resource._--- Assignment 1In this assignment, you'll be working with messy medical data and using regex to extract relevant infromation from the data. Each line of the `dates.txt` file corresponds to a medical note. Each note has a date that needs to be extracted, but each date is encoded in one of many formats.The goal of this assignment is to correctly identify all of the different date variants encoded in this dataset and to properly normalize and sort the dates. Here is a list of some of the variants you might encounter in this dataset:* 04/20/2009; 04/20/09; 4/20/09; 4/3/09* Mar-20-2009; Mar 20, 2009; March 20, 2009; Mar. 20, 2009; Mar 20 2009;* 20 Mar 2009; 20 March 2009; 20 Mar. 2009; 20 March, 2009* Mar 20th, 2009; Mar 21st, 2009; Mar 22nd, 2009* Feb 2009; Sep 2009; Oct 2010* 6/2008; 12/2009* 2009; 2010Once you have extracted these date patterns from the text, the next step is to sort them in ascending chronological order accoring to the following rules:* Assume all dates in xx/xx/xx format are mm/dd/yy* Assume all dates where year is encoded in only two digits are years from the 1900's (e.g. 1/5/89 is January 5th, 1989)* If the day is missing (e.g. 9/2009), assume it is the first day of the month (e.g. September 1, 2009).* If the month is missing (e.g. 2010), assume it is the first of January of that year (e.g. January 1, 2010).* Watch out for potential typos as this is a raw, real-life derived dataset.With these rules in mind, find the correct date in each note and return a pandas Series in chronological order of the original Series' indices.For example if the original series was this: 0 1999 1 2010 2 1978 3 2015 4 1985Your function should return this: 0 2 1 4 2 0 3 1 4 3Your score will be calculated using [Kendall's tau](https://en.wikipedia.org/wiki/Kendall_rank_correlation_coefficient), a correlation measure for ordinal data.*This function should return a Series of length 500 and dtype int.*
###Code
import pandas as pd
doc = []
with open('dates.txt') as file:
for line in file:
doc.append(line)
df = pd.Series(doc)
df.head(10)
def date_sorter():
global df
dates_extracted = df.str.extractall(r'(?P<origin>(?P<month>\d?\d)[/|-](?P<day>\d?\d)[/|-](?P<year>\d{4}))')
index_left = ~df.index.isin([x[0] for x in dates_extracted.index])
dates_extracted = dates_extracted.append(df[index_left].str.extractall(r'(?P<origin>(?P<month>\d?\d)[/|-](?P<day>([0-2]?[0-9])|([3][01]))[/|-](?P<year>\d{2}))'))
index_left = ~df.index.isin([x[0] for x in dates_extracted.index])
del dates_extracted[3]
del dates_extracted[4]
dates_extracted = dates_extracted.append(df[index_left].str.extractall(r'(?P<origin>(?P<day>\d?\d) ?(?P<month>[a-zA-Z]{3,})\.?,? (?P<year>\d{4}))'))
index_left = ~df.index.isin([x[0] for x in dates_extracted.index])
dates_extracted = dates_extracted.append(df[index_left].str.extractall(r'(?P<origin>(?P<month>[a-zA-Z]{3,})\.?-? ?(?P<day>\d\d?)(th|nd|st)?,?-? ?(?P<year>\d{4}))'))
del dates_extracted[3]
index_left = ~df.index.isin([x[0] for x in dates_extracted.index])
dates_without_day = df[index_left].str.extractall('(?P<origin>(?P<month>[A-Z][a-z]{2,}),?\.? (?P<year>\d{4}))')
dates_without_day = dates_without_day.append(df[index_left].str.extractall(r'(?P<origin>(?P<month>\d\d?)/(?P<year>\d{4}))'))
dates_without_day['day'] = 1
dates_extracted = dates_extracted.append(dates_without_day)
index_left = ~df.index.isin([x[0] for x in dates_extracted.index])
dates_only_year = df[index_left].str.extractall(r'(?P<origin>(?P<year>\d{4}))')
dates_only_year['day'] = 1
dates_only_year['month'] = 1
dates_extracted = dates_extracted.append(dates_only_year)
index_left = ~df.index.isin([x[0] for x in dates_extracted.index])
dates_extracted['year'] = dates_extracted['year'].apply(lambda x: '19' + x if len(x) == 2 else x)
dates_extracted['year'] = dates_extracted['year'].apply(lambda x: str(x))
dates_extracted['month'] = dates_extracted['month'].apply(lambda x: x[1:] if type(x) is str and x.startswith('0') else x)
month_dict = dict({'September': 9, 'Mar': 3, 'November': 11, 'Jul': 7, 'January': 1, 'December': 12,'Feb': 2, 'May': 5, 'Aug': 8, 'Jun': 6, 'Sep': 9, 'Oct': 10, 'June': 6, 'March': 3,
'February': 2, 'Dec': 12, 'Apr': 4, 'Jan': 1, 'Janaury': 1,'August': 8, 'October': 10,
'July': 7, 'Since': 1, 'Nov': 11, 'April': 4, 'Decemeber': 12, 'Age': 8})
dates_extracted.replace({"month": month_dict}, inplace=True)
dates_extracted['month'] = dates_extracted['month'].apply(lambda x: str(x))
dates_extracted['day'] = dates_extracted['day'].apply(lambda x: str(x))
# Cleaning
dates_extracted['date'] = dates_extracted['month'] + '/' + dates_extracted['day'] + '/' + dates_extracted['year']
dates_extracted['date'] = pd.to_datetime(dates_extracted['date'])
dates_extracted.sort_values(by='date', inplace=True)
df1 = pd.Series(list(dates_extracted.index.labels[0]))
return df1
print(date_sorter())
###Output
0 9
1 84
2 2
3 53
4 28
5 474
6 153
7 13
8 129
9 98
10 111
11 225
12 31
13 171
14 191
15 486
16 335
17 415
18 36
19 405
20 323
21 422
22 375
23 380
24 345
25 57
26 481
27 436
28 104
29 299
...
470 220
471 243
472 208
473 139
474 320
475 383
476 286
477 244
478 480
479 431
480 279
481 198
482 381
483 463
484 366
485 439
486 255
487 401
488 475
489 257
490 152
491 235
492 464
493 253
494 231
495 427
496 141
497 186
498 161
499 413
Length: 500, dtype: int64
|
jupyter/trasteo/Ecuaciones con numpy.ipynb | ###Markdown
Sistema de ecuaciones
###Code
import numpy as np
m_list = [[4,3], [-5,9]]
A = np.array(m_list)
A
# 4x + 3y = 20
# -5x + 9y = 26
np.linalg.inv(A)
inv_A = np.linalg.inv(A)
print(inv_A)
B = np.array([20,26])
X = np.linalg.inv(A).dot(B)
X
B
np.linalg.inv(A)
###Output
_____no_output_____
###Markdown
Usando solve4x + 3y + 2z = 25-2x + 2y +3z = -103x -5y +2z = -4
###Code
A = np.array([[4,3,2],[-2,2,3],[3,-5,2]])
B = np.array([25,-10,-4])
X = np.linalg.solve(A,B)
X
###Output
_____no_output_____
###Markdown
Ecuaciones de segundo gradox^2 + -3x -18 = 0
###Code
coeff = [1,-3,-18]
np.roots(coeff)
np.arange(0,9)
np.ones(9)
import matplotlib.pyplot as plt
x = np.arange(0,9)
A = np.array([X,np.ones(9)])
A
y = [19,20,20.5,21.5,22,23,23,25.5,47]
print( A.T, y )
w = np.linalg.lstsq(A.T,y)[0]
w
line = w[0]*x + w[1]
line
print(x,line)
plt.plot(x,line,'r-')
plt.plot(x,y,'o')
plt.show()
###Output
_____no_output_____
###Markdown
Crear una lista de puntos, a partir de dos arrays
###Code
pos = np.array([1,2,3,4,5])
neg = np.array([-1,-2,-3,-4,-5])
pos = np.array([1,2,3,4,5])
np.column_stack((pos,neg))
np.c_[pos,neg]
plt.plot([1,2,3],[2,4,8],'g-')
np.linalg.lstsq([[1,2],[2,4]],[10,20])[0]
[2, 6]
w = np.linalg.lstsq([[1. ,1.], [3., 1.] ] ,[2, 6])[0]
w
line = w[0]*x + w[1]
line
plt.plot(x,line,'r-')
plt.plot([1,3],[1,1],'o')
plt.show()
print(x,line)
###Output
<ipython-input-90-6d80a75d6cda>:1: FutureWarning: `rcond` parameter will change to the default of machine precision times ``max(M, N)`` where M and N are the input matrix dimensions.
To use the future default and silence this warning we advise to pass `rcond=None`, to keep using the old, explicitly pass `rcond=-1`.
w = np.linalg.lstsq([[1. ,1.], [3., 1.] ] ,[2, 6])[0]
|
Day2/.ipynb_checkpoints/09_classification_metrics-checkpoint.ipynb | ###Markdown
Evaluating a classification model ([video 9](https://www.youtube.com/watch?v=85dtiMz9tSo&list=PL5-da3qGB5ICeMbQuqbbCOQWcS6OYBr5A&index=9))Created by [Data School](http://www.dataschool.io/). Watch all 9 videos on [YouTube](https://www.youtube.com/playlist?list=PL5-da3qGB5ICeMbQuqbbCOQWcS6OYBr5A). Download the notebooks from [GitHub](https://github.com/justmarkham/scikit-learn-videos).**Note:** This notebook uses Python 3.6 and scikit-learn 0.19.1. The original notebook (shown in the video) used Python 2.7 and scikit-learn 0.16, and can be downloaded from the [archive branch](https://github.com/justmarkham/scikit-learn-videos/tree/archive). Agenda- What is the purpose of **model evaluation**, and what are some common evaluation procedures?- What is the usage of **classification accuracy**, and what are its limitations?- How does a **confusion matrix** describe the performance of a classifier?- What **metrics** can be computed from a confusion matrix?- How can you adjust classifier performance by **changing the classification threshold**?- What is the purpose of an **ROC curve**?- How does **Area Under the Curve (AUC)** differ from classification accuracy? Review of model evaluation- Need a way to choose between models: different model types, tuning parameters, and features- Use a **model evaluation procedure** to estimate how well a model will generalize to out-of-sample data- Requires a **model evaluation metric** to quantify the model performance Model evaluation procedures1. **Training and testing on the same data** - Rewards overly complex models that "overfit" the training data and won't necessarily generalize2. **Train/test split** - Split the dataset into two pieces, so that the model can be trained and tested on different data - Better estimate of out-of-sample performance, but still a "high variance" estimate - Useful due to its speed, simplicity, and flexibility3. **K-fold cross-validation** - Systematically create "K" train/test splits and average the results together - Even better estimate of out-of-sample performance - Runs "K" times slower than train/test split Model evaluation metrics- **Regression problems:** Mean Absolute Error, Mean Squared Error, Root Mean Squared Error- **Classification problems:** Classification accuracy Classification accuracy[Pima Indians Diabetes dataset](https://www.kaggle.com/uciml/pima-indians-diabetes-database) originally from the UCI Machine Learning Repository
###Code
# read the data into a pandas DataFrame
import pandas as pd
path = 'data/pima-indians-diabetes.data'
col_names = ['pregnant', 'glucose', 'bp', 'skin', 'insulin', 'bmi', 'pedigree', 'age', 'label']
pima = pd.read_csv(path, header=None, names=col_names)
# print the first 5 rows of data
pima.head()
###Output
_____no_output_____
###Markdown
**Question:** Can we predict the diabetes status of a patient given their health measurements?
###Code
# define X and y
feature_cols = ['pregnant', 'insulin', 'bmi', 'age']
X = pima[feature_cols]
y = pima.label
# split X and y into training and testing sets
from sklearn.model_selection import train_test_split
X_train, X_test, y_train, y_test = train_test_split(X, y, random_state=0)
# train a logistic regression model on the training set
from sklearn.linear_model import LogisticRegression
logreg = LogisticRegression()
logreg.fit(X_train, y_train)
# make class predictions for the testing set
y_pred_class = logreg.predict(X_test)
###Output
_____no_output_____
###Markdown
**Classification accuracy:** percentage of correct predictions
###Code
# calculate accuracy
from sklearn import metrics
print(metrics.accuracy_score(y_test, y_pred_class))
###Output
0.6927083333333334
###Markdown
**Null accuracy:** accuracy that could be achieved by always predicting the most frequent class
###Code
# examine the class distribution of the testing set (using a Pandas Series method)
y_test.value_counts()
# calculate the percentage of ones
y_test.mean()
# calculate the percentage of zeros
1 - y_test.mean()
# calculate null accuracy (for binary classification problems coded as 0/1)
max(y_test.mean(), 1 - y_test.mean())
# calculate null accuracy (for multi-class classification problems)
y_test.value_counts().head(1) / len(y_test)
###Output
_____no_output_____
###Markdown
Comparing the **true** and **predicted** response values
###Code
# print the first 25 true and predicted responses
print('True:', y_test.values[0:25])
print('Pred:', y_pred_class[0:25])
###Output
True: [1 0 0 1 0 0 1 1 0 0 1 1 0 0 0 0 1 0 0 0 1 1 0 0 0]
Pred: [0 0 0 0 0 0 0 1 0 1 0 1 0 0 0 0 0 0 0 0 0 0 0 0 0]
###Markdown
**Conclusion:**- Classification accuracy is the **easiest classification metric to understand**- But, it does not tell you the **underlying distribution** of response values- And, it does not tell you what **"types" of errors** your classifier is making Confusion matrixTable that describes the performance of a classification model
###Code
# IMPORTANT: first argument is true values, second argument is predicted values
print(metrics.confusion_matrix(y_test, y_pred_class))
###Output
[[118 12]
[ 47 15]]
###Markdown
 - Every observation in the testing set is represented in **exactly one box**- It's a 2x2 matrix because there are **2 response classes**- The format shown here is **not** universal **Basic terminology**- **True Positives (TP):** we *correctly* predicted that they *do* have diabetes- **True Negatives (TN):** we *correctly* predicted that they *don't* have diabetes- **False Positives (FP):** we *incorrectly* predicted that they *do* have diabetes (a "Type I error")- **False Negatives (FN):** we *incorrectly* predicted that they *don't* have diabetes (a "Type II error")
###Code
# print the first 25 true and predicted responses
print('True:', y_test.values[0:25])
print('Pred:', y_pred_class[0:25])
# save confusion matrix and slice into four pieces
confusion = metrics.confusion_matrix(y_test, y_pred_class)
TP = confusion[1, 1]
TN = confusion[0, 0]
FP = confusion[0, 1]
FN = confusion[1, 0]
###Output
_____no_output_____
###Markdown
 Metrics computed from a confusion matrix **Classification Accuracy:** Overall, how often is the classifier correct?
###Code
print((TP + TN) / float(TP + TN + FP + FN))
print(metrics.accuracy_score(y_test, y_pred_class))
###Output
0.6927083333333334
0.6927083333333334
###Markdown
**Classification Error:** Overall, how often is the classifier incorrect?- Also known as "Misclassification Rate"
###Code
print((FP + FN) / float(TP + TN + FP + FN))
print(1 - metrics.accuracy_score(y_test, y_pred_class))
###Output
0.3072916666666667
0.30729166666666663
###Markdown
**Sensitivity:** When the actual value is positive, how often is the prediction correct?- How "sensitive" is the classifier to detecting positive instances?- Also known as "True Positive Rate" or "Recall"
###Code
print(TP / float(TP + FN))
print(metrics.recall_score(y_test, y_pred_class))
###Output
0.24193548387096775
0.24193548387096775
###Markdown
**Specificity:** When the actual value is negative, how often is the prediction correct?- How "specific" (or "selective") is the classifier in predicting positive instances?
###Code
print(TN / float(TN + FP))
###Output
0.9076923076923077
###Markdown
**False Positive Rate:** When the actual value is negative, how often is the prediction incorrect?
###Code
print(FP / float(TN + FP))
###Output
0.09230769230769231
###Markdown
**Precision:** When a positive value is predicted, how often is the prediction correct?- How "precise" is the classifier when predicting positive instances?
###Code
print(TP / float(TP + FP))
print(metrics.precision_score(y_test, y_pred_class))
###Output
0.5555555555555556
0.5555555555555556
###Markdown
Many other metrics can be computed: F1 score, Matthews correlation coefficient, etc. **Conclusion:**- Confusion matrix gives you a **more complete picture** of how your classifier is performing- Also allows you to compute various **classification metrics**, and these metrics can guide your model selection**Which metrics should you focus on?**- Choice of metric depends on your **business objective**- **Spam filter** (positive class is "spam"): Optimize for **precision or specificity** because false negatives (spam goes to the inbox) are more acceptable than false positives (non-spam is caught by the spam filter)- **Fraudulent transaction detector** (positive class is "fraud"): Optimize for **sensitivity** because false positives (normal transactions that are flagged as possible fraud) are more acceptable than false negatives (fraudulent transactions that are not detected) Adjusting the classification threshold
###Code
# print the first 10 predicted responses
logreg.predict(X_test)[0:10]
# print the first 10 predicted probabilities of class membership
logreg.predict_proba(X_test)[0:10, :]
# print the first 10 predicted probabilities for class 1
logreg.predict_proba(X_test)[0:10, 1]
# store the predicted probabilities for class 1
y_pred_prob = logreg.predict_proba(X_test)[:, 1]
# allow plots to appear in the notebook
%matplotlib inline
import matplotlib.pyplot as plt
# histogram of predicted probabilities
plt.hist(y_pred_prob, bins=8)
plt.xlim(0, 1)
plt.title('Histogram of predicted probabilities')
plt.xlabel('Predicted probability of diabetes')
plt.ylabel('Frequency')
###Output
_____no_output_____
###Markdown
**Decrease the threshold** for predicting diabetes in order to **increase the sensitivity** of the classifier
###Code
# predict diabetes if the predicted probability is greater than 0.3
from sklearn.preprocessing import binarize
y_pred_class = binarize([y_pred_prob], 0.3)[0]
# print the first 10 predicted probabilities
y_pred_prob[0:10]
# print the first 10 predicted classes with the lower threshold
y_pred_class[0:10]
# previous confusion matrix (default threshold of 0.5)
print(confusion)
# new confusion matrix (threshold of 0.3)
print(metrics.confusion_matrix(y_test, y_pred_class))
# sensitivity has increased (used to be 0.24)
print(46 / float(46 + 16))
# specificity has decreased (used to be 0.91)
print(80 / float(80 + 50))
###Output
0.6153846153846154
###Markdown
**Conclusion:**- **Threshold of 0.5** is used by default (for binary problems) to convert predicted probabilities into class predictions- Threshold can be **adjusted** to increase sensitivity or specificity- Sensitivity and specificity have an **inverse relationship** ROC Curves and Area Under the Curve (AUC)**Question:** Wouldn't it be nice if we could see how sensitivity and specificity are affected by various thresholds, without actually changing the threshold?**Answer:** Plot the ROC curve!
###Code
# IMPORTANT: first argument is true values, second argument is predicted probabilities
fpr, tpr, thresholds = metrics.roc_curve(y_test, y_pred_prob)
plt.plot(fpr, tpr)
plt.xlim([0.0, 1.0])
plt.ylim([0.0, 1.0])
plt.title('ROC curve for diabetes classifier')
plt.xlabel('False Positive Rate (1 - Specificity)')
plt.ylabel('True Positive Rate (Sensitivity)')
plt.grid(True)
###Output
_____no_output_____
###Markdown
- ROC curve can help you to **choose a threshold** that balances sensitivity and specificity in a way that makes sense for your particular context- You can't actually **see the thresholds** used to generate the curve on the ROC curve itself
###Code
# define a function that accepts a threshold and prints sensitivity and specificity
def evaluate_threshold(threshold):
print('Sensitivity:', tpr[thresholds > threshold][-1])
print('Specificity:', 1 - fpr[thresholds > threshold][-1])
evaluate_threshold(0.5)
evaluate_threshold(0.3)
###Output
Sensitivity: 0.7258064516129032
Specificity: 0.6153846153846154
###Markdown
AUC is the **percentage** of the ROC plot that is **underneath the curve**:
###Code
# IMPORTANT: first argument is true values, second argument is predicted probabilities
print(metrics.roc_auc_score(y_test, y_pred_prob))
###Output
0.7245657568238213
###Markdown
- AUC is useful as a **single number summary** of classifier performance.- If you randomly chose one positive and one negative observation, AUC represents the likelihood that your classifier will assign a **higher predicted probability** to the positive observation.- AUC is useful even when there is **high class imbalance** (unlike classification accuracy).
###Code
# calculate cross-validated AUC
from sklearn.model_selection import cross_val_score
cross_val_score(logreg, X, y, cv=10, scoring='roc_auc').mean()
###Output
/home/anniee/.local/lib/python3.6/site-packages/sklearn/linear_model/logistic.py:432: FutureWarning: Default solver will be changed to 'lbfgs' in 0.22. Specify a solver to silence this warning.
FutureWarning)
/home/anniee/.local/lib/python3.6/site-packages/sklearn/linear_model/logistic.py:432: FutureWarning: Default solver will be changed to 'lbfgs' in 0.22. Specify a solver to silence this warning.
FutureWarning)
/home/anniee/.local/lib/python3.6/site-packages/sklearn/linear_model/logistic.py:432: FutureWarning: Default solver will be changed to 'lbfgs' in 0.22. Specify a solver to silence this warning.
FutureWarning)
/home/anniee/.local/lib/python3.6/site-packages/sklearn/linear_model/logistic.py:432: FutureWarning: Default solver will be changed to 'lbfgs' in 0.22. Specify a solver to silence this warning.
FutureWarning)
/home/anniee/.local/lib/python3.6/site-packages/sklearn/linear_model/logistic.py:432: FutureWarning: Default solver will be changed to 'lbfgs' in 0.22. Specify a solver to silence this warning.
FutureWarning)
/home/anniee/.local/lib/python3.6/site-packages/sklearn/linear_model/logistic.py:432: FutureWarning: Default solver will be changed to 'lbfgs' in 0.22. Specify a solver to silence this warning.
FutureWarning)
/home/anniee/.local/lib/python3.6/site-packages/sklearn/linear_model/logistic.py:432: FutureWarning: Default solver will be changed to 'lbfgs' in 0.22. Specify a solver to silence this warning.
FutureWarning)
/home/anniee/.local/lib/python3.6/site-packages/sklearn/linear_model/logistic.py:432: FutureWarning: Default solver will be changed to 'lbfgs' in 0.22. Specify a solver to silence this warning.
FutureWarning)
/home/anniee/.local/lib/python3.6/site-packages/sklearn/linear_model/logistic.py:432: FutureWarning: Default solver will be changed to 'lbfgs' in 0.22. Specify a solver to silence this warning.
FutureWarning)
/home/anniee/.local/lib/python3.6/site-packages/sklearn/linear_model/logistic.py:432: FutureWarning: Default solver will be changed to 'lbfgs' in 0.22. Specify a solver to silence this warning.
FutureWarning)
###Markdown
**Confusion matrix advantages:**- Allows you to calculate a **variety of metrics**- Useful for **multi-class problems** (more than two response classes)**ROC/AUC advantages:**- Does not require you to **set a classification threshold**- Still useful when there is **high class imbalance** Confusion Matrix Resources- Blog post: [Simple guide to confusion matrix terminology](http://www.dataschool.io/simple-guide-to-confusion-matrix-terminology/) by me- Videos: [Intuitive sensitivity and specificity](https://www.youtube.com/watch?v=U4_3fditnWg) (9 minutes) and [The tradeoff between sensitivity and specificity](https://www.youtube.com/watch?v=vtYDyGGeQyo) (13 minutes) by Rahul Patwari- Notebook: [How to calculate "expected value"](https://github.com/podopie/DAT18NYC/blob/master/classes/13-expected_value_cost_benefit_analysis.ipynb) from a confusion matrix by treating it as a cost-benefit matrix (by Ed Podojil)- Graphic: How [classification threshold](https://media.amazonwebservices.com/blog/2015/ml_adjust_model_1.png) affects different evaluation metrics (from a [blog post](https://aws.amazon.com/blogs/aws/amazon-machine-learning-make-data-driven-decisions-at-scale/) about Amazon Machine Learning) ROC and AUC Resources- Video: [ROC Curves and Area Under the Curve](https://www.youtube.com/watch?v=OAl6eAyP-yo) (14 minutes) by me, including [transcript and screenshots](http://www.dataschool.io/roc-curves-and-auc-explained/) and a [visualization](http://www.navan.name/roc/)- Video: [ROC Curves](https://www.youtube.com/watch?v=21Igj5Pr6u4) (12 minutes) by Rahul Patwari- Paper: [An introduction to ROC analysis](http://people.inf.elte.hu/kiss/13dwhdm/roc.pdf) by Tom Fawcett- Usage examples: [Comparing different feature sets](http://research.microsoft.com/pubs/205472/aisec10-leontjeva.pdf) for detecting fraudulent Skype users, and [comparing different classifiers](http://www.cse.ust.hk/nevinZhangGroup/readings/yi/Bradley_PR97.pdf) on a number of popular datasets Other Resources- scikit-learn documentation: [Model evaluation](http://scikit-learn.org/stable/modules/model_evaluation.html)- Guide: [Comparing model evaluation procedures and metrics](https://github.com/justmarkham/DAT8/blob/master/other/model_evaluation_comparison.md) by me- Video: [Counterfactual evaluation of machine learning models](https://www.youtube.com/watch?v=QWCSxAKR-h0) (45 minutes) about how Stripe evaluates its fraud detection model, including [slides](http://www.slideshare.net/MichaelManapat/counterfactual-evaluation-of-machine-learning-models) Comments or Questions?- Email: - Website: http://dataschool.io- Twitter: [@justmarkham](https://twitter.com/justmarkham)
###Code
from IPython.core.display import HTML
def css_styling():
styles = open("styles/custom.css", "r").read()
return HTML(styles)
css_styling()
###Output
_____no_output_____ |
notebooks/graphs/advance-tiled.ipynb | ###Markdown
Create an adjancency matrix
###Code
adjmat = Tensor.fromRandom(["S", "D"], [10, 10], (1.0, 0.3), 1, seed=10, name="AdjMat")
displayTensor(adjmat)
###Output
_____no_output_____
###Markdown
Create a frontier
###Code
frontier = Tensor.fromRandom(["S"], [10], (0.4,), 1, seed=20, name="Frontier1")
displayTensor(frontier)
###Output
_____no_output_____
###Markdown
Create reference output using untiled traversal
###Code
# Note that S and D are really both V, so we can swap the names for clarity
next_frontier_verify = Tensor(rank_ids=["S"], name="NextFrontier_Verify")
frontier_s = frontier.getRoot()
adjmat_s = adjmat.getRoot()
next_frontier_verify_s = next_frontier_verify.getRoot()
for s, (_, adjmat_d) in frontier_s & adjmat_s:
for d, (next_frontier_verify_ref, _) in next_frontier_verify_s << adjmat_d:
next_frontier_verify_ref <<= 1
displayTensor(next_frontier_verify)
###Output
_____no_output_____
###Markdown
Pre-tile adjacency matrix (concordant for source-stationary)
###Code
S0 = 5
D0 = 5
adjmat_tiled = adjmat.splitUniform(D0, depth=1).splitUniform(S0).swapRanks(1)
adjmat_tiled.setName("AdjMatTiled")
displayTensor(adjmat_tiled)
###Output
_____no_output_____
###Markdown
Swapped representation (concordant for destination-stationary)
###Code
adjmat_swapped = adjmat_tiled.swapRanks(2).swapRanks(0)
adjmat_swapped.setName("AdjMatTiledSwapped")
displayTensor(adjmat_swapped)
###Output
_____no_output_____
###Markdown
Pre-process the frontier into tiles
###Code
frontier_tiled = frontier.splitUniform(S0)
frontier_tiled.setName("FrontierTiled")
displayTensor(frontier_tiled)
###Output
_____no_output_____
###Markdown
Source-Stationary Tiled Advance Kernel
###Code
# Output frontier is produced tiled (concordant)
next_frontier_tiled = Tensor(rank_ids=["S1", "S0"], name = "NextFrontierTiled")
adjmat_s1 = adjmat_tiled.getRoot()
frontier_s1 = frontier_tiled.getRoot()
next_frontier_s1 = next_frontier_tiled.getRoot()
canvas = createCanvas(frontier_tiled, adjmat_tiled, next_frontier_tiled)
for s1, (frontier_s0, adjmat_d1) in frontier_s1 & adjmat_s1:
for d1, (next_frontier_s0, adjmat_s0) in next_frontier_s1 << adjmat_d1:
for s0, (_, adjmat_d0) in frontier_s0 & adjmat_s0:
for d0, (next_frontier_ref, _) in next_frontier_s0 << adjmat_d0:
next_frontier_ref <<= 1
canvas.addFrame((s1, s0), (s1, d1, s0, d0), (d1, d0))
displayCanvas(canvas)
assert next_frontier_tiled.flattenRanks(coord_style="absolute").getRoot() == next_frontier_verify.getRoot()
###Output
_____no_output_____
###Markdown
Destination-Stationary Tiled Advance Kernel
###Code
# Output frontier is produced tiled (concordant)
next_frontier_tiled = Tensor(rank_ids=["S1", "S0"], name = "NextFrontierTiled")
adjmat_d1 = adjmat_swapped.getRoot()
frontier_s1 = frontier_tiled.getRoot()
next_frontier_s1 = next_frontier_tiled.getRoot()
canvas = createCanvas(frontier_tiled, adjmat_swapped, next_frontier_tiled)
for d1, (next_frontier_s0, adjmat_s1) in next_frontier_s1 << adjmat_d1:
for s1, (frontier_s0, adjmat_d0) in frontier_s1 & adjmat_s1:
for d0, (next_frontier_ref, adjmat_s0) in next_frontier_s0 << adjmat_d0:
for s0, (_, _) in frontier_s0 & adjmat_s0:
next_frontier_ref <<= 1
canvas.addFrame((s1, s0), (d1, s1, d0, s0), (d1, d0))
break # This break is interesting - it represents the "OR" of the intersection.
# Note that if this loop was in parallel we wouldn't care which particular
# coordinate passed intersection, only that one of them did.
displayCanvas(canvas)
assert next_frontier_tiled.flattenRanks(coord_style="absolute").getRoot() == next_frontier_verify.getRoot()
###Output
_____no_output_____ |
multilayer_perceptron/2_mlp_cross_val.ipynb | ###Markdown
Find the optimal hyperparameters for the multi layer perceptron model
###Code
#install libraries (if not already in environment)
!pip install --upgrade scikit-learn
!pip install pandas
!pip install pyyaml h5py
!pip install seaborn
# TensorFlow and tf.keras
import tensorflow as tf
from tensorflow.keras import layers
from tensorflow.keras.wrappers.scikit_learn import KerasClassifier
from tensorflow.keras.optimizers import Adam, SGD, Adamax
import sklearn.metrics as metrics
from sklearn.metrics import auc, plot_roc_curve, roc_curve, mean_squared_error, accuracy_score, roc_auc_score, classification_report, confusion_matrix, log_loss
from sklearn.model_selection import GridSearchCV
from sklearn.preprocessing import StandardScaler
import sklearn
#add path to the functions folder
import sys
sys.path.append('../onc_functions')
# load custom function for building the NN
from build_mlp import build_mlp
# other libraries
import numpy as np
import pandas as pd
import pickle
#plotting
import matplotlib.pyplot as plt
import seaborn as sns
%matplotlib inline
# Make numpy values easier to read.
np.set_printoptions(precision=3, suppress=True)
print('tensorflow-' + tf.__version__)
print('python-' + sys.version)
print('sklearn-' + sklearn.__version__)
with open('numeric_columns.pickle', 'rb') as f:
nu_cols = pickle.load(f)
###Output
_____no_output_____
###Markdown
Create Model Layers- trying different weights (to handle the class imbalance) requires that you rerun the grid search with each weight
###Code
def mlp_cv(selected_class_weight, weight_name, imputation):
# fix random seed for reproducibility
seed = 78
np.random.seed(seed)
#import an imputed dataset
with open('complete' + str(imputation) + '.pickle', 'rb') as f:
dataset = pickle.load(f)
#keep only the training data subsets
X_train = dataset[dataset.subset <= 6].copy().sort_values(by = 'usrds_id')
del dataset
y_train = np.array(X_train.pop('died_in_90'))
#scale the numeric columns
scaler = StandardScaler()
X_train[nu_cols] = scaler.fit_transform(X_train[nu_cols])
X_train = np.array(X_train.drop(columns=['subset','usrds_id','impnum']))
print('scaled shape train ' + str(X_train.shape))
# Create a MirroredStrategy (can take advantage of a GPU if you have some, otherwise it just uses a single threaded approach)
strategy = tf.distribute.MirroredStrategy()
print("Number of devices: {}".format(strategy.num_replicas_in_sync))
# Open a strategy scope.
with strategy.scope():
# Everything that creates variables should be under the strategy scope.
# In general this is only model construction & `compile()`.
# Wrap Keras model so it can be used by scikit-learn
# grid search epochs, batch size and optimizer
neurons = [16,32, 64, 128]
layers = [1, 2]
kernel_regularizer = ['l2']
dropout_rate = [ 0.1, 0.2, 0.4, 0.5, 0.6]
learn_rate = [.001, .0001, .0002]
activation = ['relu', 'sigmoid', 'tanh']
optimizer = ['Adam']
epochs = [10, 20] # 1mill/256=4000 steps for one pass thru dataset
batches = [512, 256]
output_bias = [None]
params = dict(neurons=neurons,
layers=layers,
kernel_regularizer=kernel_regularizer,
dropout_rate=dropout_rate,
learn_rate=learn_rate,
activation=activation,
optimizer = optimizer,
epochs=epochs,
batch_size=batches,
output_bias=output_bias)
# early stopping for the epochs based on the auc under the precision recall curve
early_stopping = tf.keras.callbacks.EarlyStopping(
monitor='auc_pr' ,
verbose=1,
patience=10,
mode='max',
restore_best_weights=True)
# use the Keras wrapper for scikitlearn and our custom build_mlp function imported above
weighted_model_skl = KerasClassifier(build_fn=build_mlp,
verbose=0)
# evaluate using 5-fold cross validation
grid = GridSearchCV(
weighted_model_skl,
param_grid=params,
cv=2,
scoring='average_precision',
return_train_score=True,
n_jobs=-1
)
print('fit model')
grid_result = grid.fit(
X_train,
y_train,
class_weight=selected_class_weight,
callbacks=[early_stopping]
)
# summarize results
means = grid_result.cv_results_['mean_test_score']
stds = grid_result.cv_results_['std_test_score']
params = grid_result.cv_results_['params']
for mean, stdev, param in zip(means, stds, params):
print("%f (%f) with: %r" % (mean, stdev, param))
print("Best: %f using %s" % (grid_result.best_score_, grid_result.best_params_))
#save results
with open('./results/2021_grid_best_params_imp_' + str(imputation) + '_weight_' + str(weight_name) + '.pickle', 'wb') as f:
pickle.dump(grid_result.best_params_, f)
with open('./results/2021_grid_best_auc_imp_' + str(imputation) + '_weight_' + str(weight_name) + '.pickle','wb') as f:
pickle.dump(grid_result.best_score_, f)
with open('./results/2021_grid_cv_results_imp_' + str(imputation) + '_weight_' + str(weight_name) + '.pickle','wb') as f:
pickle.dump(grid_result.cv_results_, f)
total = 1150195
positive_class_count = 86083 #(7.48% of total)
neg_class_count = 1064112 #(92.52% of total)
# Scaling by total/2 helps keep the loss to a similar magnitude.
# The sum of the weights of all examples stays the same.
weight_for_0 = (1 / neg_class_count)*(total)/2.0
weight_for_1 = (1 / positive_class_count)*(total)/2.0
class_weight_m = {0: weight_for_0, 1: weight_for_1}
class_weight_5 = {0: 1, 1: 5}
class_weight_10 = {0: 1, 1: 10}
class_weight_20 = {0: 1, 1: 20}
#run the cross validation with the amount of weighting for each class
mlp_cv(class_weight_20, weight_name=20, imputation=5)
###Output
scaled shape train (804890, 294)
WARNING:tensorflow:There are non-GPU devices in `tf.distribute.Strategy`, not using nccl allreduce.
INFO:tensorflow:Using MirroredStrategy with devices ('/job:localhost/replica:0/task:0/device:CPU:0',)
Number of devices: 1
fit model n/
Best: 0.235455 using {'activation': 'relu', 'batch_size': 256, 'dropout_rate': 0.2, 'epochs': 15, 'kernel_regularizer': 'l2', 'layers': 2, 'learn_rate': 0.0002, 'neurons': 32, 'optimizer': 'Adam', 'output_bias': None}
0.234318 (0.009525) with: {'activation': 'relu', 'batch_size': 256, 'dropout_rate': 0.2, 'epochs': 10, 'kernel_regularizer': 'l2', 'layers': 2, 'learn_rate': 0.0002, 'neurons': 16, 'optimizer': 'Adam', 'output_bias': None}
0.233863 (0.008253) with: {'activation': 'relu', 'batch_size': 256, 'dropout_rate': 0.2, 'epochs': 10, 'kernel_regularizer': 'l2', 'layers': 2, 'learn_rate': 0.0002, 'neurons': 32, 'optimizer': 'Adam', 'output_bias': None}
0.235408 (0.007534) with: {'activation': 'relu', 'batch_size': 256, 'dropout_rate': 0.2, 'epochs': 15, 'kernel_regularizer': 'l2', 'layers': 2, 'learn_rate': 0.0002, 'neurons': 16, 'optimizer': 'Adam', 'output_bias': None}
0.235455 (0.010428) with: {'activation': 'relu', 'batch_size': 256, 'dropout_rate': 0.2, 'epochs': 15, 'kernel_regularizer': 'l2', 'layers': 2, 'learn_rate': 0.0002, 'neurons': 32, 'optimizer': 'Adam', 'output_bias': None}
params n/
{'activation': 'relu', 'batch_size': 256, 'dropout_rate': 0.2, 'epochs': 15, 'kernel_regularizer': 'l2', 'layers': 2, 'learn_rate': 0.0002, 'neurons': 32, 'optimizer': 'Adam', 'output_bias': None}
best score n/
0.23545548460762972
|
Model backlog/Inference/108-tweet-inference-5fold-roberta-base-hidden10.ipynb | ###Markdown
Dependencies
###Code
import json, glob
from tweet_utility_scripts import *
from tweet_utility_preprocess_roberta_scripts_aux import *
from transformers import TFRobertaModel, RobertaConfig
from tokenizers import ByteLevelBPETokenizer
from tensorflow.keras import layers
from tensorflow.keras.models import Model
###Output
_____no_output_____
###Markdown
Load data
###Code
test = pd.read_csv('/kaggle/input/tweet-sentiment-extraction/test.csv')
print('Test samples: %s' % len(test))
display(test.head())
###Output
Test samples: 3534
###Markdown
Model parameters
###Code
input_base_path = '/kaggle/input/108roberta-base/'
with open(input_base_path + 'config.json') as json_file:
config = json.load(json_file)
config
# vocab_path = input_base_path + 'vocab.json'
# merges_path = input_base_path + 'merges.txt'
base_path = '/kaggle/input/qa-transformers/roberta/'
vocab_path = base_path + 'roberta-base-vocab.json'
merges_path = base_path + 'roberta-base-merges.txt'
config['base_model_path'] = base_path + 'roberta-base-tf_model.h5'
config['config_path'] = base_path + 'roberta-base-config.json'
model_path_list = glob.glob(input_base_path + 'model' + '*.h5')
model_path_list.sort()
print('Models to predict:')
print(*model_path_list, sep = "\n")
###Output
Models to predict:
/kaggle/input/108roberta-base/model_fold_1.h5
/kaggle/input/108roberta-base/model_fold_2.h5
/kaggle/input/108roberta-base/model_fold_3.h5
/kaggle/input/108roberta-base/model_fold_4.h5
/kaggle/input/108roberta-base/model_fold_5.h5
###Markdown
Tokenizer
###Code
tokenizer = ByteLevelBPETokenizer(vocab_file=vocab_path, merges_file=merges_path,
lowercase=True, add_prefix_space=True)
###Output
_____no_output_____
###Markdown
Pre process
###Code
test['text'].fillna('', inplace=True)
test["text"] = test["text"].apply(lambda x: x.lower())
test["text"] = test["text"].apply(lambda x: x.strip())
x_test = get_data_test(test, tokenizer, config['MAX_LEN'], preprocess_fn=preprocess_roberta_test)
###Output
_____no_output_____
###Markdown
Model
###Code
module_config = RobertaConfig.from_pretrained(config['config_path'], output_hidden_states=True)
def model_fn(MAX_LEN):
input_ids = layers.Input(shape=(MAX_LEN,), dtype=tf.int32, name='input_ids')
attention_mask = layers.Input(shape=(MAX_LEN,), dtype=tf.int32, name='attention_mask')
base_model = TFRobertaModel.from_pretrained(config['base_model_path'], config=module_config, name="base_model")
_, _, hidden_states = base_model({'input_ids': input_ids, 'attention_mask': attention_mask})
h12 = hidden_states[-1]
h11 = hidden_states[-2]
h10 = hidden_states[-3]
h09 = hidden_states[-4]
x_start = layers.Dropout(.1)(h10)
x_start = layers.Dense(1)(x_start)
x_start = layers.Flatten()(x_start)
y_start = layers.Activation('softmax', name='y_start')(x_start)
x_end = layers.Dropout(.1)(h10)
x_end = layers.Dense(1)(x_end)
x_end = layers.Flatten()(x_end)
y_end = layers.Activation('softmax', name='y_end')(x_end)
model = Model(inputs=[input_ids, attention_mask], outputs=[y_start, y_end])
return model
###Output
_____no_output_____
###Markdown
Make predictions
###Code
NUM_TEST_IMAGES = len(test)
test_start_preds = np.zeros((NUM_TEST_IMAGES, config['MAX_LEN']))
test_end_preds = np.zeros((NUM_TEST_IMAGES, config['MAX_LEN']))
for model_path in model_path_list:
print(model_path)
model = model_fn(config['MAX_LEN'])
model.load_weights(model_path)
test_preds = model.predict(x_test)
test_start_preds += test_preds[0] / len(model_path_list)
test_end_preds += test_preds[1] / len(model_path_list)
###Output
/kaggle/input/108roberta-base/model_fold_1.h5
/kaggle/input/108roberta-base/model_fold_2.h5
/kaggle/input/108roberta-base/model_fold_3.h5
/kaggle/input/108roberta-base/model_fold_4.h5
/kaggle/input/108roberta-base/model_fold_5.h5
###Markdown
Post process
###Code
test['start'] = test_start_preds.argmax(axis=-1)
test['end'] = test_end_preds.argmax(axis=-1)
test['text_len'] = test['text'].apply(lambda x : len(x))
test['text_wordCnt'] = test['text'].apply(lambda x : len(x.split(' ')))
test["end"].clip(0, test["text_len"], inplace=True)
test["start"].clip(0, test["end"], inplace=True)
test['selected_text'] = test.apply(lambda x: decode(x['start'], x['end'], x['text'], config['question_size'], tokenizer), axis=1)
test["selected_text"].fillna(test["text"], inplace=True)
###Output
_____no_output_____
###Markdown
Visualize predictions
###Code
display(test.head(10))
###Output
_____no_output_____
###Markdown
Test set predictions
###Code
submission = pd.read_csv('/kaggle/input/tweet-sentiment-extraction/sample_submission.csv')
submission['selected_text'] = test["selected_text"]
submission.to_csv('submission.csv', index=False)
submission.head(10)
###Output
_____no_output_____ |
YOLOv5/.ipynb_checkpoints/YOLOv5 preprocessing-checkpoint.ipynb | ###Markdown
전처리 경로확인
###Code
import os
from tqdm import tqdm
os.chdir('D:/project_mini/kaggle_face_mask_detection')
os.getcwd()
###Output
_____no_output_____
###Markdown
파일 로드
###Code
from glob import glob
from natsort import natsorted
annotation_list = natsorted(glob('./annotations/*.xml'))
img_list = natsorted(glob('./images/*.png'))
print(len(annotation_list))
print(len(img_list))
###Output
853
853
###Markdown
XML 파싱
###Code
from xml.etree.ElementTree import parse
sample = annotation_list[0]
root = parse(sample).getroot()
print(root.findtext('filename'))
print(root.find('size').findtext('width'))
print(root.find('size').findtext('height'))
print(root.find('size').findtext('depth'))
print(root.findtext('segmented'))
for obj in root.findall('object'):
print(obj.findtext('name'))
print(obj.findtext('pose'))
print(obj.findtext('truncated'))
print(obj.findtext('occluded'))
print(obj.findtext('difficult'))
print(obj.find('bndbox').findtext('xmin'))
print(obj.find('bndbox').findtext('ymin'))
print(obj.find('bndbox').findtext('xmax'))
print(obj.find('bndbox').findtext('ymax'))
###Output
maksssksksss0.png
512
366
3
0
without_mask
Unspecified
0
0
0
79
105
109
142
with_mask
Unspecified
0
0
0
185
100
226
144
without_mask
Unspecified
0
0
0
325
90
360
141
###Markdown
샘플 이미지 확인
###Code
import cv2
import matplotlib.pyplot as plt
sample_img = cv2.cvtColor(cv2.imread(img_list[0]), cv2.COLOR_BGR2RGB)
plt.imshow(sample_img)
plt.show()
for obj in root.findall('object'):
cv2.rectangle(
sample_img,
(int(obj.find('bndbox').findtext('xmin')), int(obj.find('bndbox').findtext('ymin'))),
(int(obj.find('bndbox').findtext('xmax')), int(obj.find('bndbox').findtext('ymax'))),
(255, 0, 0),
4
)
plt.imshow(sample_img)
plt.show()
###Output
_____no_output_____
###Markdown
데이터프레임 제작
###Code
import pandas as pd
columns_list = [
'filename', 'width', 'height', 'depth', 'segmented', 'name', 'pose',
'truncated', 'occluded', 'difficult', 'xmin', 'ymin', 'xmax', 'ymax'
]
df = pd.DataFrame(columns=columns_list)
df
df = pd.DataFrame(columns=columns_list)
for xml in tqdm(annotation_list):
root = parse(xml).getroot()
for obj in root.findall('object'):
data = {}
data[columns_list[0]] = root.findtext('filename')[:-4]
data[columns_list[1]] = root.find('size').findtext('width')
data[columns_list[2]] = root.find('size').findtext('height')
data[columns_list[3]] = root.find('size').findtext('depth')
data[columns_list[4]] = root.findtext('segmented')
data[columns_list[5]] = obj.findtext('name')
data[columns_list[6]] = obj.findtext('pose')
data[columns_list[7]] = obj.findtext('truncated')
data[columns_list[8]] = obj.findtext('occluded')
data[columns_list[9]] = obj.findtext('difficult')
data[columns_list[10]] = obj.find('bndbox').findtext('xmin')
data[columns_list[11]] = obj.find('bndbox').findtext('ymin')
data[columns_list[12]] = obj.find('bndbox').findtext('xmax')
data[columns_list[13]] = obj.find('bndbox').findtext('ymax')
df = df.append(data, ignore_index=True)
df
###Output
100%|████████████████████████████████████████████████████████████████████████████████| 853/853 [00:14<00:00, 59.22it/s]
###Markdown
데이터 탐색
###Code
print(df.nunique())
df.name.value_counts()
labels = list(df.name.unique())
print(labels)
###Output
['without_mask', 'with_mask', 'mask_weared_incorrect']
###Markdown
YOLO용 라벨데이터 생성
###Code
def cvt_yolo(w, h, x1, y1, x2, y2):
w, h, x1, y1, x2, y2 = map(int, (w, h, x1, y1, x2, y2))
x_center = (x1 + x2) / (2 * w)
y_center = (y1 + y2) / (2 * h)
width = (x2 - x1) / w
height = (y2 - y1) / h
x_center, y_center, width, height = map(lambda x : str(round(x, 4)), (x_center, y_center, width, height))
return x_center, y_center, width, height
print(cvt_yolo('512', '366', '79', '105', '109' ,'142'))
img_dir = './images/'
lbl_dir = './labels/'
os.makedirs(lbl_dir, exist_ok=True)
fname = ''
s = ''
for i in tqdm(range(df.shape[0])):
if fname != df.iloc[i].filename: # 다른 그림이면
if s: # 문자열이 존재하면
with open(lbl_dir + fname + '.txt', 'w') as f:
f.write(s)
f.close()
s = ''
fname = df.iloc[i].filename
s += str(labels.index(df.iloc[i]['name'])) + ' ' + ' '.join(
cvt_yolo(df.iloc[i].width, df.iloc[i].height, df.iloc[i].xmin,
df.iloc[i].ymin, df.iloc[i].xmax, df.iloc[i].ymax)) + '\n'
if s: # 문자열이 존재하면
with open(lbl_dir + '/' + fname + '.txt', 'w') as f:
f.write(s)
f.close()
lbl_list = natsorted(glob('./labels/*.txt'))
print(len(lbl_list))
###Output
100%|████████████████████████████████████████████████████████████████████████████| 4072/4072 [00:02<00:00, 1827.60it/s]
###Markdown
훈련/검증 데이터 분리
###Code
from sklearn.model_selection import train_test_split
img_train, img_else, lbl_train, lbl_else = train_test_split(img_list, lbl_list, test_size=0.3, random_state=7)
img_val, img_test, lbl_val, lbl_test = train_test_split(img_else, lbl_else, test_size=0.3, random_state=7)
print(len(img_train), len(img_val), len(img_test))
print(img_train[38], lbl_train[38])
import shutil
sub_dirs = ['train/', 'val/', 'test/']
for sub_dir in sub_dirs: # 폴더 체크
os.makedirs(img_dir + sub_dir, exist_ok=True)
os.makedirs(lbl_dir + sub_dir, exist_ok=True)
# 파일 이동
for file in img_train:
try:
shutil.move(file, img_dir + sub_dirs[0] + file.split('\\')[-1])
except:
pass
for file in img_val:
try:
shutil.move(file, img_dir + sub_dirs[1] + file.split('\\')[-1])
except:
pass
for file in img_test:
try:
shutil.move(file, img_dir + sub_dirs[2] + file.split('\\')[-1])
except:
pass
for file in lbl_train:
try:
shutil.move(file, lbl_dir + sub_dirs[0] + file.split('\\')[-1])
except:
pass
for file in lbl_val:
try:
shutil.move(file, lbl_dir + sub_dirs[1] + file.split('\\')[-1])
except:
pass
for file in lbl_test:
try:
shutil.move(file, lbl_dir + sub_dirs[2] + file.split('\\')[-1])
except:
pass
###Output
_____no_output_____
###Markdown
yaml 파일 제작
###Code
import yaml
from pathlib import Path
p = os.getcwd()
yaml_data = {
'path': str(Path(p) / 'images'),
'train': 'train',
'val': 'val',
'nc': len(labels),
'names': labels
}
with open('custom.yaml', 'w') as f:
yaml.dump(yaml_data, f)
###Output
_____no_output_____ |
4-Machine_Learning/3-Deep Learning/2-Redes Neuronales Convolucionales/ToDo_Dogs&Cats.ipynb | ###Markdown
Redes Convolucionales Ejemplo clasificación de perros y gatos para CAPTCHAEste notebook utiliza datos de la [competición de Kaggle Dogs vs. Cats](https://www.kaggle.com/c/dogs-vs-cats/overview). En esta competicion se utiliza Asirra (Animal Species Image Recognition for Restricting Access), CAPTCHA que sirve para diferenciar entre una persona o una máquina accediendo a una página web. Este tipo de "pruebas" se utilizan para evitar emails de spam, y ataques por fuerza bruta contra servidores.En este notebook vamos a probar que hay técnicas de clasificado automáticas de imágenes mediante redes neuronales, que con las que se intenta saltar CAPTCHA Import Library
###Code
import numpy as np
import pandas as pd
import matplotlib.pyplot as plt
from tensorflow.keras.preprocessing.image_dataset import ImageDataGenerator, load_img
from sklearn.model_selection import train_test_split
import matplotlib.pyplot as plt
import random
import cv2
###Output
_____no_output_____
###Markdown
Define ConstantsTendremos una serie de constantes como las dimensiones de las imágenes, que serán fijas a lo largo de todo el notebook
###Code
IMAGE_WIDTH=32
IMAGE_HEIGHT=32
IMAGE_CHANNELS=3
IMAGE_SIZE=(IMAGE_WIDTH, IMAGE_HEIGHT, IMAGE_CHANNELS)
BATCH_SIZE = 10
EPOCHS = 5
ROOT_PATH = "/Users/federicoruizruiz/Documents/TheBridge_Bootcamp/kaggle-data/cats-dogs/"
"""TRAIN_PATH_TOT = ROOT_PATH + "train\\train\\"
TEST_PATH_TOT = ROOT_PATH + "test\\test\\"
MINI_TRAIN_PATH = ROOT_PATH + "mini_train\\train\\"
MINI_TEST_PATH = ROOT_PATH + "mini_test\\test1\\"""
TRAIN_PATH = ROOT_PATH + 'cat_dogs_img/train/'
TEST_PATH = ROOT_PATH + 'cat_dogs_img/test/'
###Output
_____no_output_____
###Markdown
Prepare Training Data1. Descárgate el dataset de train de [la competición de Kaggle](https://www.kaggle.com/c/dogs-vs-cats/overview).2. Descomprime el dataset y guardalo en la ruta que quieras del ordenador. Por supuesto, NO en la carpeta donde esté el repositorio3. En este punto vamos guardar en una lista las etiquetas de cada foto.
###Code
##### CODE #####
filenames = os.listdir(TRAIN_PATH)
categories = []
for filename in filenames:
category = filename.split('.')[0]
if category == 'dog':
categories.append(1)
else:
categories.append(0)
df = pd.DataFrame({
'filename': filenames,
'category': categories
})
df
###Output
_____no_output_____
###Markdown
See Total In count
###Code
df['category'].value_counts().plot.bar();
###Output
_____no_output_____
###Markdown
See sample image
###Code
from skimage.io import imread
import cv2
#pip install opencv-python
sample = random.choice(filenames)
image = imread(TRAIN_PATH + sample)
print(image.shape)
print(np.max(image))
plt.imshow(image)
###Output
_____no_output_____
###Markdown
Una imagen no es mas que un array de HxWxC píxeles, siendo H(Height) y W(Width) las dimensiones de resolución de la imagen, y C el número de canales. Habrá tres valores por píxel.
###Code
image
###Output
_____no_output_____
###Markdown
Resize imageCargar todas las imágenes a la vez es un problema ya que son un total de 25000 (unos 500MB la carpeta de train). Este proceso require mucha memoria, por lo que tendremos que aplicarle un resize a cada imagen para bajarlas de resolución. Esto también nos sirve para solventar el problema de tener imágenes con distintas resoluciones.
###Code
plt.figure(figsize=(12, 12))
##### CODE #####
print("Tamaño imagen original:", image.shape)
print("Tamaño imagen reshape:", imagesmall.shape)
print("Maximo valor por pixel:", np.max(imagesmall))
# Original image
plt.subplot(1, 2, 1)
plt.imshow(image)
# Resized image
plt.subplot(1, 2, 2)
plt.imshow(imagesmall);
###Output
_____no_output_____
###Markdown
ColorPodríamos cargar las imágenes como blanco y negro, de esta forma se reduciría el espacio de features considerablemente al contar con un único canal
###Code
sample = random.choice(filenames)
##### CODE #####
###Output
_____no_output_____
###Markdown
Load dataLlega el momento de cargar los datos. Ya no estan sencillo como cuando teníamos datasets en CSVs puesto que ahora hay que cargar miles de archivos en memoria en este notebook. Para ello necesitaremos un programa iterativo que vaya recorriendo los archivos de la carpeta, cargarlos como array de numpy y almacenarlos en un objeto.
###Code
def read_data(path, im_size):
X = []
Y = []
##### CODE #####
return np.array(X), np.array(Y)
X_train, y_train = read_data(TRAIN_PATH, IMAGE_SIZE)
X_test, y_test = read_data(TEST_PATH, IMAGE_SIZE)
print(X_train.shape)
print(X_test.shape)
print(X_train[0].shape)
plt.imshow(X_train[0]);
X_train[0]
###Output
_____no_output_____
###Markdown
Normalized dataNormalizar los datos hará que entrene mucho mejor la red, al estar todos los pixeles en la misma escala.
###Code
print("Min:", np.min(X_train))
print("Max:", np.max(X_train))
X_train = X_train / 255.0
X_test = X_test / 255.0
print("Min:", np.min(X_train))
print("Max:", np.max(X_train))
###Output
_____no_output_____
###Markdown
Shuffle dataComo hemos cargado los datos de manera ordenada (primero gatos y luego perros), tendremos que desordenarlos para asegurarnos de que no haya ningún sesgo en el entrenamiento ni en la selección de datos de validación.
###Code
##### CODE #####
###Output
_____no_output_____
###Markdown
Save dataPodemos guardar los arrays de numpy en un archivo `.npz`, de tal manera que luego sea más rápido importarlo
###Code
##### CODE #####
###Output
_____no_output_____
###Markdown
Para cargar
###Code
##### CODE #####
###Output
_____no_output_____
###Markdown
Build Model * **Conv Layer**: extraerá diferentes features de las imagenes* **Pooling Layer**: Reduce las dimensiones de las imágenes tras una capa convolucional* **Fully Connected Layer**: Tras las capas convolucionales, aplanamos las features y las introducimos como entrada de una red neuronal normal.* **Output Layer**: Las predicciones de la redPara el loss y la metrica, se puede usar un binary_crossentropy, al ser un target binario. O
###Code
from tensorflow import keras
##### CODE #####
###Output
_____no_output_____
###Markdown
Callbacks Early Stopping
###Code
from keras.callbacks import EarlyStopping
earlystop = EarlyStopping(patience=10)
###Output
_____no_output_____
###Markdown
Fit the model
###Code
##### CODE #####
###Output
_____no_output_____
###Markdown
EvaluateProbemos los datos en el conjunto de test.
###Code
##### CODE #####
plt.imshow(X_test[0]);
##### CODE #####
###Output
_____no_output_____
###Markdown
Image data generator
###Code
df["category"] = df["category"].replace({0: 'cat', 1: 'dog'})
print("Categorias:", df['category'].unique())
df.head()
##### CODE #####
plt.figure(figsize=(12, 12))
for i in range(0, 15):
plt.subplot(5, 3, i+1)
for X_batch, Y_batch in example_generator:
image = X_batch[0]
plt.imshow(image)
break
plt.tight_layout()
plt.show()
train_df, validate_df = train_test_split(df,
test_size=0.20,
random_state=42)
train_df = train_df.reset_index(drop=True)
validate_df = validate_df.reset_index(drop=True)
total_train = train_df.shape[0]
total_validate = validate_df.shape[0]
print("Shape train", total_train)
print("Shape validation", total_validate)
validate_df.head()
train_df.head()
###Output
_____no_output_____
###Markdown
Training Generator
###Code
train_generator = train_datagen.flow_from_dataframe(
train_df,
TRAIN_PATH,
x_col='filename',
y_col='category',
target_size=(IMAGE_HEIGHT, IMAGE_WIDTH),
class_mode='binary',
batch_size=BATCH_SIZE
)
###Output
_____no_output_____
###Markdown
Validation Generator
###Code
validation_datagen = ImageDataGenerator(rescale=1./255)
validation_generator = validation_datagen.flow_from_dataframe(
train_df,
TRAIN_PATH,
x_col='filename',
y_col='category',
target_size=(IMAGE_HEIGHT, IMAGE_WIDTH),
class_mode='binary',
batch_size=BATCH_SIZE
)
###Output
_____no_output_____
###Markdown
Fit Model
###Code
##### CODE #####
##### CODE #####
results = model.evaluate(X_test, y_test)
print("test loss, test acc:", results)
###Output
_____no_output_____
###Markdown
Virtualize Training
###Code
fig, (ax1, ax2) = plt.subplots(2, 1, figsize=(12, 12))
ax1.plot(history.history['loss'], color='b', label="Training loss")
ax1.plot(history.history['val_loss'], color='r', label="validation loss")
ax1.set_xticks(np.arange(1, EPOCHS, 1))
ax1.set_yticks(np.arange(0, 1, 0.1))
ax2.plot(history.history['accuracy'], color='b', label="Training accuracy")
ax2.plot(history.history['val_accuracy'], color='r',label="Validation accuracy")
ax2.set_xticks(np.arange(1, EPOCHS, 1))
legend = plt.legend(loc='best', shadow=True)
plt.tight_layout()
plt.show()
###Output
_____no_output_____ |
Assignment_draft2.ipynb | ###Markdown
COVID-19 Dashboard This dashboard shows the latest data on COVID-19(Coronavirus), regarding how it is affecting the UK.
###Code
from uk_covid19 import Cov19API
import matplotlib.pyplot as plt
import pandas as pd
import json
import matplotlib.animation as animation
from matplotlib import style
import ipywidgets as wdg
from datetime import datetime
from IPython.display import display
output=wdg.Output()
style.use('dark_background')
plt.rcParams['figure.dpi'] = 100
###Output
_____no_output_____
###Markdown
The purpose of this graph is to show cases that can be described as serious. The two types of cases seen on the graph are death, and cases requiring hospitalisation. You can select either of these options on from the list below to view the data seperately.
###Code
#load saved data
with open("seriouscases.json", "rt") as INFILE:
data=json.load(INFILE)
#define key functions
def parse_date(datestring):
""" Convert a date string into a pandas datetime object """
return pd.to_datetime(datestring, format="%Y-%m-%d")
def convert_date(datestring):
#Convert a date string into a pandas datetime object
return pd.to_datetime(datestring, format="%Y-%m-%d")
def wrangledata(x):
global seriouscasesdf
#wrangle dates
datalist=x['data']
dates=[dictionary['date'] for dictionary in datalist]
dates.sort()
startdate=parse_date(dates[0])
enddate=parse_date(dates[-1])
#print(startdate, ' to ', enddate)
#make dataframe
index=pd.date_range(startdate, enddate, freq='D')
seriouscasesdf=pd.DataFrame(index=index, columns=['hospitalCases', 'deaths'])
#fill dataframe
for entry in datalist:
date=convert_date(entry['date'])
for column in ['hospitalCases', 'deaths']:
if pd.isna(seriouscasesdf.loc[date, column]):
value = float(entry[column]) if entry[column]!=None else 0.0
seriouscasesdf.loc[date, column]=value
seriouscasesdf.fillna(0.0, inplace=True)
return(seriouscasesdf)
#update implementation
def access_api():
#this will overwrite the previous json file
try:
global data
filters = [
'areaType=overview'
]
structure = {
"date": "date",
"hospitalCases":"hospitalCases",
"deaths":"newDeaths28DaysByPublishDate"
}
api = Cov19API(filters=filters, structure=structure)
seriouscases=api.get_json()
with open("seriouscases.json", "wt") as OUTF:
json.dump(seriouscases, OUTF)
with open("seriouscases.json", "rt") as INFILE:
data=json.load(INFILE)
return(data)
except:
with output:
print('Error getting new data from PHE')
def api_button_callback(button):
apidata=access_api()
apidata2=access_api2()
global seriouscasesdf
global cumulativesdf
seriouscasesdf=wrangledata(apidata)
cumulativesdf=wrangledata2(apidata2)
refresh_graph()
refresh_graph2()
apibutton.icon="check"
apibutton.disabled=True
apibutton=wdg.Button(
description='UPDATE',
disabled=False,
button_style='info',
tooltip="Refreshes the graph with the latest data from Public Health England",
icon=''
)
apibutton.on_click(api_button_callback)
display(apibutton)
display(output)
wrangledata(data)
#plot graph no.1
def refresh_graph():
Case.disabled=True
Case.disabled=False
def plot_serio(x):
ncols=len(x)
if ncols>0:
a=seriouscasesdf[list(x)].plot()
a.grid(color='grey',linewidth=0.5)
else:
# these messages will be printed in no column is selected
print("Click to select data for graph")
print("(CTRL-Click to select more than one category)")
Case=wdg.SelectMultiple(
options=['hospitalCases','deaths'],
value=['hospitalCases','deaths'],
rows=2,
description='Show: ',
disabled=False,
)
graph=wdg.interactive_output(plot_serio, {'x': Case})
display(Case, graph)
###Output
_____no_output_____
###Markdown
Here you can select between viewing cumulative totals of: cases, hospital admissions, and deaths as they have increased since the start of the pandemic.
###Code
def access_api2():
#this will overwrite the previous json file
try:
filters = [
'areaType=overview'
]
structure = {
"date": "date",
"CumulativeCases":"cumCasesByPublishDate",
"CumulativeAdmissions":"cumAdmissions",
"CumulativeDeaths":"cumDeaths28DaysByDeathDate"
}
api = Cov19API(filters=filters, structure=structure)
cumulatives=api.get_json()
with open("cumulatives.json", "wt") as OUTF:
json.dump(cumulatives, OUTF)
with open("cumulatives.json", "rt") as INFILE:
data2=json.load(INFILE)
return(data2)
except:
with output:
print('Error getting new data from PHE')
def wrangledata2(x):
global cumulativesdf
#wrangle dates
datalist=x['data']
dates=[dictionary['date'] for dictionary in datalist]
dates.sort()
startdate=parse_date(dates[0])
enddate=parse_date(dates[-1])
#print(startdate, ' to ', enddate)
#make dataframe
index=pd.date_range(startdate, enddate, freq='D')
cumulativesdf=pd.DataFrame(index=index, columns=['CumulativeCases','CumulativeAdmissions','CumulativeDeaths'])
#fill dataframe
for entry in datalist:
date=convert_date(entry['date'])
for column in ['CumulativeCases','CumulativeAdmissions','CumulativeDeaths']:
if pd.isna(cumulativesdf.loc[date, column]):
value = float(entry[column]) if entry[column]!=None else 0.0
cumulativesdf.loc[date, column]=value
cumulativesdf.fillna(0.0, inplace=True)
return(cumulativesdf)
colour={'CumulativeCases':'lightseagreen','CumulativeAdmissions':'khaki','CumulativeDeaths':'lightcoral'
}
thickness={'CumulativeCases':4,'CumulativeAdmissions':4,'CumulativeDeaths':4
}
Title={'CumulativeCases':'Cumulative Cases','CumulativeAdmissions':'Cumulative Admissions','CumulativeDeaths':'Cumulative Deaths'
}
with open("cumulatives.json", "rt") as INFILE:
data2=json.load(INFILE)
wrangledata2(data2)
def plot_cumu(x):
ax2=cumulativesdf[x].plot(color=(colour[x]),linewidth=(thickness[x]))
ax2.set_title(Title[x], fontsize=20)
ax2.grid(color='grey',linewidth=0.5)
Selection=wdg.Dropdown(
options=['CumulativeCases','CumulativeAdmissions', 'CumulativeDeaths'],
value='CumulativeDeaths',
description='Show: ',
disabled=False,
)
def refresh_graph2():
current=Selection.value
if current==Selection.options[0]:
other=Selection.options[1]
else:
other=Selection.options[0]
Selection.value=other # forces the redraw
Selection.value=current
graph=wdg.interactive_output(plot_cumu, {'x': Selection})
display(Selection, graph)
###Output
_____no_output_____ |
notebooks/crater_matching_tests.ipynb | ###Markdown
MC Simulation
###Code
latlims = (30, 60)
longlims = (30, 60)
diamlims = (2, 30)
ellipse_limit = 1.2
arclims = 0.5
triad_radius = 50
lat_cat, long_cat, major_cat, minor_cat, psi_cat, crater_id = extract_robbins_dataset(
load_craters(
"../data/lunar_crater_database_robbins_2018.csv",
latlims=latlims,
longlims=longlims,
diamlims=diamlims,
ellipse_limit=ellipse_limit,
arc_lims=arclims
)
)
db = CraterDatabase(lat_cat, long_cat, major_cat, minor_cat, psi_cat, radius=triad_radius)
r_craters_cat = np.array(np.array(spherical_to_cartesian(const.RMOON, lat_cat, long_cat))).T[..., None]
C_craters_cat = conic_matrix(major_cat, minor_cat, psi_cat)
test_size = 30
resolution = (256, 256)
position_dataset = np.empty((test_size, 3, 1), np.float64)
attitude_dataset = np.empty((test_size, 3, 3), np.float64)
est_position_dataset = np.empty((test_size, 3, 1), np.float64)
# Error factors
position_noise = 0.01
axis_noise = 0.01
angle_noise = 0.15
for i in range(test_size):
lat = np.random.uniform(*latlims)
long = np.random.uniform(*longlims)
cam_alt = np.random.uniform(150, 250)
cam = ConicProjector.from_coordinates(lat,
long,
cam_alt,
convert_to_radians=True,
resolution=resolution
)
# save cam.r, sol_incidence
position_dataset[i] = cam.r
# Rotations are incremental (order matters)
cam.rotate('roll', np.random.uniform(0, 360))
cam.rotate('pitch', np.random.uniform(-30, 30))
cam.rotate('yaw', np.random.uniform(-30, 30))
# save cam.T
attitude_dataset[i] = cam.T
visible = (cdist(r_craters_cat.squeeze(), cam.r.T) <= np.sqrt(2 * cam_alt * const.RMOON + cam_alt**2)).ravel()
r_craters = r_craters_cat[visible]
C_craters = C_craters_cat[visible]
r_craters_img = cam.project_crater_centers(r_craters)
in_image = np.logical_and.reduce(np.logical_and(r_craters_img > -20, r_craters_img < cam.resolution[0]+20), axis=1)
r_craters = r_craters[in_image]
C_craters = C_craters[in_image]
A_craters = cam.project_crater_conics(C_craters, r_craters)
n_det = len(A_craters)
major_det, minor_det = ellipse_axes(A_craters)
psi_det = ellipse_angle(A_craters)
r_craters_det = conic_center(A_craters)
major_det *= 1 + np.random.normal(0, axis_noise, n_det)
minor_det *= 1 + np.random.normal(0, axis_noise, n_det)
psi_det *= 1 + np.random.normal(0, angle_noise, n_det)
r_craters_det *= 1 + np.random.normal(-position_noise, position_noise, r_craters_det.shape)
A_craters_noisy = conic_matrix(major_det, minor_det, psi_det, *r_craters_det.T)
if n_det >= 6:
est_position_dataset[i] = db.query_position(A_craters_noisy, cam.T, cam.K,
regression_type='ransac',
sigma_pix=5,
batch_size=1000,
top_n=20,
max_trials=1000,
residual_threshold=10000.)
else:
est_position_dataset[i, ...] = -1
import scipy.stats as stats
plt.style.use('default')
matched_positions = np.logical_and.reduce(~np.isnan(est_position_dataset), axis=(1,2))
err = LA.norm(est_position_dataset[matched_positions] - position_dataset[matched_positions], axis=1).ravel()*1000
bins = np.arange(0, 10000, 200)
fig, ax = plt.subplots(figsize=(8, 6))
entries, bin_edges, patches = ax.hist(err, bins=bins)
ax.set_xlabel("$||\Delta \mathbf{x}||_2$")
ax.set_ylabel("")
ax.xaxis.set_major_formatter(ticker.FormatStrFormatter('%.im'))
fig.tight_layout()
fig.savefig("../output/total_error.pdf")
len(err)
err_r, err_lat, err_lon = np.array(cartesian_to_spherical(*(est_position_dataset[matched_positions]).squeeze().T) -
np.array(cartesian_to_spherical(*(position_dataset[matched_positions]).squeeze().T)))
err_lat, err_lon = map(np.degrees, (err_lat, err_lon))
err_lon[err_lon > 180] -= 360
fig, ax = plt.subplots(figsize=(8, 6))
ax.hist(err_r*1000)
ax.set_xlabel(r'$\Delta r$')
ax.xaxis.set_major_formatter(ticker.FormatStrFormatter('%.im'))
fig.tight_layout()
fig.savefig("../output/radial_error.pdf")
fig, ax = plt.subplots(figsize=(8, 6))
err_range = np.arange(-0.1, 0.1, 0.01)
h = ax.hist2d(err_lon, err_lat, bins=err_range, cmap='viridis')
ax.set_xlabel(r'$\Delta \lambda$')
ax.set_ylabel(r'$\Delta \varphi$')
ax.xaxis.set_major_formatter(ticker.FormatStrFormatter('%.2f$^{\circ}$'))
ax.yaxis.set_major_formatter(ticker.FormatStrFormatter('%.2f$^{\circ}$'))
plt.colorbar(h[3], ax=ax)
fig.tight_layout()
fig.savefig("../output/coordinate_error.pdf")
err_x, err_y, err_z = ((est_position_dataset[matched_positions] - position_dataset[matched_positions])*1000).squeeze().T
fig, ax = plt.subplots(2, 2, figsize=(8, 6))
err_range = np.arange(-6000, 6000, 200)
h = ax[0, 0].hist2d(err_x, err_y, bins=err_range, cmap='viridis')
ax[0, 0].set_xlabel('$\Delta X$')
ax[0, 0].set_ylabel('$\Delta Y$')
ax[1, 0].hist2d(err_x, err_z, bins=err_range, cmap='viridis')
ax[1, 0].set_xlabel('$\Delta X$')
ax[1, 0].set_ylabel('$\Delta Z$')
ax[0, 1].hist2d(err_y, err_z, bins=err_range, cmap='viridis')
ax[0, 1].set_xlabel('$\Delta Y$')
ax[0, 1].set_ylabel('$\Delta Z$')
ax[1, 1].axis('off')
# plt.colorbar(h[3], ax=ax[1, 1])
np.nanmean(err_x)
np.logical_and.reduce(~np.isnan(est_position_dataset), axis=(1,2))
###Output
_____no_output_____ |
MBTI personality classifier.ipynb | ###Markdown
MBTI Personality ClassifierThis programme will classify people into mbti personality types based on their past 50 posts on social media using the basic naivebayesclassifier
###Code
import pandas as pd
import numpy as np
import matplotlib.pyplot as plt
import nltk
import string
from nltk.classify import NaiveBayesClassifier
###Output
_____no_output_____
###Markdown
Importing the dataset
###Code
data_set = pd.read_csv("mbti_1.csv")
data_set.tail()
###Output
_____no_output_____
###Markdown
Checking the dataset for missing values
###Code
data_set.isnull().any()
###Output
_____no_output_____
###Markdown
Exploring the dataset The size of the dataset
###Code
data_set.shape
###Output
_____no_output_____
###Markdown
Explroing the posts in posts field
###Code
data_set.iloc[0,1].split('|||')
###Output
_____no_output_____
###Markdown
Finding the number of posts
###Code
len(data_set.iloc[1,1].split('|||'))
###Output
_____no_output_____
###Markdown
Finding the unique vales from type of personality column
###Code
types = np.unique(np.array(data_set['type']))
types
###Output
_____no_output_____
###Markdown
The total number of posts for each type
###Code
total = data_set.groupby(['type']).count()*50
total
###Output
_____no_output_____
###Markdown
Graphing it for better visualization
###Code
plt.figure(figsize = (12,6))
plt.bar(np.array(total.index), height = total['posts'],)
plt.xlabel('Personality types', size = 14)
plt.ylabel('Number of posts available', size = 14)
plt.title('Total posts for each personality type')
###Output
_____no_output_____
###Markdown
Organising the data to create a bag words model Segrating all the posts by their personality types and ***creating a new dataframe to store all this in***
###Code
all_posts= pd.DataFrame()
for j in types:
temp1 = data_set[data_set['type']==j]['posts']
temp2 = []
for i in temp1:
temp2+=i.split('|||')
temp3 = pd.Series(temp2)
all_posts[j] = temp3
all_posts.tail()
###Output
_____no_output_____
###Markdown
Creating a function to tokenize the words
###Code
useless_words = nltk.corpus.stopwords.words("english") + list(string.punctuation)
def build_bag_of_words_features_filtered(words):
words = nltk.word_tokenize(words)
return {
word:1 for word in words \
if not word in useless_words}
###Output
_____no_output_____
###Markdown
A random check of the function
###Code
build_bag_of_words_features_filtered(all_posts['INTJ'].iloc[1])
###Output
_____no_output_____
###Markdown
Creating an array of features
###Code
features=[]
for j in types:
temp1 = all_posts[j]
temp1 = temp1.dropna() #not all the personality types have same number of files
features += [[(build_bag_of_words_features_filtered(i), j) \
for i in temp1]]
###Output
_____no_output_____
###Markdown
Because each number of personality types have different number of posts they must be splitted accordingle. Taking 80% for training and 20% for testing
###Code
split=[]
for i in range(16):
split += [len(features[i]) * 0.8]
split = np.array(split,dtype = int)
split
###Output
_____no_output_____
###Markdown
Data for training
###Code
train=[]
for i in range(16):
train += features[i][:split[i]]
###Output
_____no_output_____
###Markdown
Training the model
###Code
sentiment_classifier = NaiveBayesClassifier.train(train)
###Output
_____no_output_____
###Markdown
Testing the model on the dataset it was trained for accuracy
###Code
nltk.classify.util.accuracy(sentiment_classifier, train)*100
###Output
_____no_output_____
###Markdown
Creating the test data
###Code
test=[]
for i in range(16):
test += features[i][split[i]:]
###Output
_____no_output_____
###Markdown
Testing the model on the test dataset which it has never seen before
###Code
nltk.classify.util.accuracy(sentiment_classifier, test)*100
###Output
_____no_output_____
###Markdown
The model performs at efficieny of only 10% which is pretty bad. Hence, instead of selecting all 16 types of personalitys as a unique feature I explored the dataset further and decided to simplify it.The Myers Briggs Type Indicator (or MBTI for short) is a personality type system that divides everyone into 16 distinct personality types across 4 axis:- Introversion (I) – Extroversion (E)- Intuition (N) – Sensing (S)- Thinking (T) – Feeling (F)- Judging (J) – Perceiving (P)We will use this and create 4 classifyers to classify the person Creating a classifyer for Introversion (I) and Extroversion (E)**Note:** The details for the steps over here are same as the ones while creating the model above, hence I will only explain the changes
###Code
# Features for the bag of words model
features=[]
for j in types:
temp1 = all_posts[j]
temp1 = temp1.dropna() #not all the personality types have same number of files
if('I' in j):
features += [[(build_bag_of_words_features_filtered(i), 'introvert') \
for i in temp1]]
if('E' in j):
features += [[(build_bag_of_words_features_filtered(i), 'extrovert') \
for i in temp1]]
###Output
_____no_output_____
###Markdown
Data for training
###Code
train=[]
for i in range(16):
train += features[i][:split[i]]
###Output
_____no_output_____
###Markdown
Training the model
###Code
IntroExtro = NaiveBayesClassifier.train(train)
###Output
_____no_output_____
###Markdown
Testing the model on the dataset it was trained for accuracy
###Code
nltk.classify.util.accuracy(IntroExtro, train)*100
###Output
_____no_output_____
###Markdown
Creating the test data
###Code
test=[]
for i in range(16):
test += features[i][split[i]:]
###Output
_____no_output_____
###Markdown
Testing the model on the test dataset which it has never seen before
###Code
nltk.classify.util.accuracy(IntroExtro, test)*100
###Output
_____no_output_____
###Markdown
Seeing that this model has good somewhat good results, I shall repeat the same with the rest of the traits Creating a classifyer for Intuition (N) and Sensing (S)
###Code
# Features for the bag of words model
features=[]
for j in types:
temp1 = all_posts[j]
temp1 = temp1.dropna() #not all the personality types have same number of files
if('N' in j):
features += [[(build_bag_of_words_features_filtered(i), 'Intuition') \
for i in temp1]]
if('E' in j):
features += [[(build_bag_of_words_features_filtered(i), 'Sensing') \
for i in temp1]]
###Output
_____no_output_____
###Markdown
Data for training
###Code
train=[]
for i in range(16):
train += features[i][:split[i]]
###Output
_____no_output_____
###Markdown
Training the model
###Code
IntuitionSensing = NaiveBayesClassifier.train(train)
###Output
_____no_output_____
###Markdown
Testing the model on the dataset it was trained for accuracy
###Code
nltk.classify.util.accuracy(IntuitionSensing, train)*100
###Output
_____no_output_____
###Markdown
Creating the test data
###Code
test=[]
for i in range(16):
test += features[i][split[i]:]
###Output
_____no_output_____
###Markdown
Testing the model on the test dataset which it has never seen before
###Code
nltk.classify.util.accuracy(IntuitionSensing, test)*100
###Output
_____no_output_____
###Markdown
Creating a classifyer for Thinking (T) and Feeling (F)
###Code
# Features for the bag of words model
features=[]
for j in types:
temp1 = all_posts[j]
temp1 = temp1.dropna() #not all the personality types have same number of files
if('T' in j):
features += [[(build_bag_of_words_features_filtered(i), 'Thinking') \
for i in temp1]]
if('F' in j):
features += [[(build_bag_of_words_features_filtered(i), 'Feeling') \
for i in temp1]]
###Output
_____no_output_____
###Markdown
Data for training
###Code
train=[]
for i in range(16):
train += features[i][:split[i]]
###Output
_____no_output_____
###Markdown
Training the model
###Code
ThinkingFeeling = NaiveBayesClassifier.train(train)
###Output
_____no_output_____
###Markdown
Testing the model on the dataset it was trained for accuracy
###Code
nltk.classify.util.accuracy(ThinkingFeeling, train)*100
###Output
_____no_output_____
###Markdown
Creating the test data
###Code
test=[]
for i in range(16):
test += features[i][split[i]:]
###Output
_____no_output_____
###Markdown
Testing the model on the test dataset which it has never seen before
###Code
nltk.classify.util.accuracy(ThinkingFeeling, test)*100
###Output
_____no_output_____
###Markdown
Creating a classifyer for Judging (J) and Percieving (P)
###Code
# Features for the bag of words model
features=[]
for j in types:
temp1 = all_posts[j]
temp1 = temp1.dropna() #not all the personality types have same number of files
if('J' in j):
features += [[(build_bag_of_words_features_filtered(i), 'Judging') \
for i in temp1]]
if('P' in j):
features += [[(build_bag_of_words_features_filtered(i), 'Percieving') \
for i in temp1]]
###Output
_____no_output_____
###Markdown
Data for training
###Code
train=[]
for i in range(16):
train += features[i][:split[i]]
###Output
_____no_output_____
###Markdown
Training the model
###Code
JudgingPercieiving = NaiveBayesClassifier.train(train)
###Output
_____no_output_____
###Markdown
Testing the model on the dataset it was trained for accuracy
###Code
nltk.classify.util.accuracy(JudgingPercieiving, train)*100
###Output
_____no_output_____
###Markdown
Creating the test data
###Code
test=[]
for i in range(16):
test += features[i][split[i]:]
###Output
_____no_output_____
###Markdown
Testing the model on the test dataset which it has never seen before
###Code
nltk.classify.util.accuracy(JudgingPercieiving, test)*100
###Output
_____no_output_____
###Markdown
Summarizing the results of the models***
###Code
temp = {'train' : [81.12443979837917,70.14524215640667,80.03456948570128,79.79341109742592], 'test' : [58.20469312585358,54.46262259027357,59.41315234035509,54.40549600629061]}
results = pd.DataFrame.from_dict(temp, orient='index', columns=['Introvert - Extrovert', 'Intuition - Sensing', 'Thinking - Feeling', 'Judging - Percieiving'])
results
###Output
_____no_output_____
###Markdown
Plotting the results for better appeal
###Code
plt.figure(figsize = (12,6))
plt.bar(np.array(results.columns), height = results.loc['train'],)
plt.xlabel('Personality types', size = 14)
plt.ylabel('Number of posts available', size = 14)
plt.title('Total posts for each personality type')
labels = np.array(results.columns)
training = results.loc['train']
ind = np.arange(4)
width = 0.4
fig = plt.figure()
ax = fig.add_subplot(111)
rects1 = ax.bar(ind, training, width, color='royalblue')
testing = results.loc['test']
rects2 = ax.bar(ind+width, testing, width, color='seagreen')
fig.set_size_inches(12, 6)
fig.savefig('Results.png', dpi=200)
ax.set_xlabel('Model Classifying Trait', size = 18)
ax.set_ylabel('Accuracy Percent (%)', size = 18)
ax.set_xticks(ind + width / 2)
ax.set_xticklabels(labels)
ax.legend((rects1[0], rects2[0]), ('Tested on a known dataframe', 'Tested on an unknown dataframe'))
plt.show()
###Output
_____no_output_____
###Markdown
Testing the models to predict my trait my feeding few of my quora writingslink to my quora answers feed: https://www.quora.com/profile/Divya-Bramhecha Defining a functions that inputs the writings, tokenizes them and then predicts the output based on our earlier classifiers
###Code
def MBTI(input):
tokenize = build_bag_of_words_features_filtered(input)
ie = IntroExtro.classify(tokenize)
Is = IntuitionSensing.classify(tokenize)
tf = ThinkingFeeling.classify(tokenize)
jp = JudgingPercieiving.classify(tokenize)
mbt = ''
if(ie == 'introvert'):
mbt+='I'
if(ie == 'extrovert'):
mbt+='E'
if(Is == 'Intuition'):
mbt+='N'
if(Is == 'Sensing'):
mbt+='S'
if(tf == 'Thinking'):
mbt+='T'
if(tf == 'Feeling'):
mbt+='F'
if(jp == 'Judging'):
mbt+='J'
if(jp == 'Percieving'):
mbt+='P'
return(mbt)
###Output
_____no_output_____
###Markdown
Building another functions that takes all of my posts as input and outputs the graph showing percentage of each trait seen in each posts and sums up displaying your personality as the graph title**Note:** The input should be an array of your posts
###Code
def tellmemyMBTI(input, name, traasits=[]):
a = []
trait1 = pd.DataFrame([0,0,0,0],['I','N','T','J'],['count'])
trait2 = pd.DataFrame([0,0,0,0],['E','S','F','P'],['count'])
for i in input:
a += [MBTI(i)]
for i in a:
for j in ['I','N','T','J']:
if(j in i):
trait1.loc[j]+=1
for j in ['E','S','F','P']:
if(j in i):
trait2.loc[j]+=1
trait1 = trait1.T
trait1 = trait1*100/len(input)
trait2 = trait2.T
trait2 = trait2*100/len(input)
#Finding the personality
YourTrait = ''
for i,j in zip(trait1,trait2):
temp = max(trait1[i][0],trait2[j][0])
if(trait1[i][0]==temp):
YourTrait += i
if(trait2[j][0]==temp):
YourTrait += j
traasits +=[YourTrait]
#Plotting
labels = np.array(results.columns)
intj = trait1.loc['count']
ind = np.arange(4)
width = 0.4
fig = plt.figure()
ax = fig.add_subplot(111)
rects1 = ax.bar(ind, intj, width, color='royalblue')
esfp = trait2.loc['count']
rects2 = ax.bar(ind+width, esfp, width, color='seagreen')
fig.set_size_inches(10, 7)
ax.set_xlabel('Finding the MBTI Trait', size = 18)
ax.set_ylabel('Trait Percent (%)', size = 18)
ax.set_xticks(ind + width / 2)
ax.set_xticklabels(labels)
ax.set_yticks(np.arange(0,105, step= 10))
ax.set_title('Your Personality is '+YourTrait,size = 20)
plt.grid(True)
fig.savefig(name+'.png', dpi=200)
plt.show()
return(traasits)
###Output
_____no_output_____
###Markdown
Importing my quora answers from a text fileI copied all my answer from the link i provided before (i broke down the paragraphs as separte posts)
###Code
My_writings = open("Myquora.txt")
my_writing = My_writings.readlines()
#my_writing
my_posts = my_writing[0].split('|||')
len(my_posts)
#my_posts
###Output
_____no_output_____
###Markdown
Using the classifier to predict my personality type
###Code
trait=tellmemyMBTI(my_posts, 'Divy')
###Output
_____no_output_____
###Markdown
Concluding noteMy profile according to https://www.16personalities.com/ is INTJ.I am pretty happy that using such a basic model it was pretty close to my real profile, only 1 different. And even that difference was very close, between 10% inaccuary which pretty good.Although, I am not sure how the classifier will perform on all test cases in general. Specially, the data for some profiles was very less. Sanaya profile
###Code
My_writings = open("Sanayapoem.txt")
my_writing = My_writings.readlines()
#my_writing
my_posts = my_writing[0].split('|||')
len(my_posts)
#my_posts
trait = tellmemyMBTI(my_posts,'sanaya')
###Output
_____no_output_____
###Markdown
Valentin Pyataev
###Code
My_writings = open("Valentin pyatev.txt")
my_writing = My_writings.readlines()
#my_writing
my_posts = my_writing[0].split('|||')
len(my_posts)
#my_posts
trait=tellmemyMBTI(my_posts,'Valentin')
###Output
_____no_output_____
###Markdown
MIT gurukul people
###Code
My_writings = open("All texts.txt")
my_writing = My_writings.readlines()
a =[''];
for i in my_writing:
a[0]=a[0]+i
len(a)
my_posts = a[0].split('&&&')
len(my_posts)
#my_posts
###Output
_____no_output_____
###Markdown
Posts for each person
###Code
alls = [None]*len(my_posts)
for i in range(len(my_posts)):
alls[i] = my_posts[i].split('|||')
###Output
_____no_output_____
###Markdown
Email ID connection
###Code
Names = open("Names.txt")
names = Names.readlines()
#names
for i in range(len(names)):
names[i] = names[i].replace('@gmail.com\n','')
print(names[i])
names[len(names)-1]=names[len(names)-1].replace('@gmail.com','')
for i in range(len(alls)):
trait=tellmemyMBTI(alls[i],names[i])
trait
###Output
_____no_output_____ |
4-Machine_Learning/1-Supervisado/6-KNN/Ejercicio_k-NearestNeighbor.ipynb | ###Markdown
Ejercicio k-Nearest Neighbor App ReviewsEn este ejercicio vas a trabajar con una base de datos de reviews de una aplicación. Entre los datos podemos encontrar el texto de la review, las estrellas, así como el sentimiento del comentario (si es algo bueno o malo).El objetivo es montar un algoritmo de clasificación que prediga el rating, en función del sentimiento del comentario y la cantidad de palabras empleadas en el mismo. Para ello tendrás que utilizar un algoritmo de tipo KNN. Importamos las librerías que vamos a utilizar
###Code
import pandas as pd
import numpy as np
import seaborn as sns
import matplotlib.pyplot as plt
from matplotlib.colors import ListedColormap
import matplotlib.patches as mpatches
import seaborn as sns
%matplotlib inline
plt.rcParams['figure.figsize'] = (16, 9)
plt.style.use('ggplot')
from sklearn.model_selection import train_test_split
from sklearn.preprocessing import MinMaxScaler
from sklearn.neighbors import KNeighborsClassifier
from sklearn.metrics import classification_report
from sklearn.metrics import confusion_matrix
###Output
_____no_output_____
###Markdown
Leemos nuestro archivo de entrada
###Code
dataframe = pd.read_csv(r"data/reviews_sentiment.csv", sep=";")
dataframe.head(10)
dataframe.describe()
dataframe.hist()
print(dataframe.groupby('Star Rating').size())
sns.catplot(x='Star Rating',data=dataframe,kind="count", aspect=3) # relación de aspecto
sns.catplot(x='wordcount',data=dataframe,kind="count", aspect=3) # relación de aspecto
###Output
_____no_output_____
###Markdown
Para facilitar el ejercicio, las columnas que utilizaremos serán: wordcount con la cantidad de palabras utilizadas y sentimentValue con un valor entre -4 y 4 que indica si el comentario fue valorado como positivo o negativo Nuestras etiquetas, serán las estrellas que dieron los usuarios a la app, que son valores discretos del 1 al 5 Rápidas visualizaciones y análisis Preparamos el dataset
###Code
X = dataframe[['wordcount', 'sentimentValue']].values
y = dataframe['Star Rating'].values
X_train, X_test, y_train, y_test = train_test_split(X, y, random_state =0)
scaler = MinMaxScaler()
X_train = scaler.fit_transform(X_train)
X_test = scaler.transform(X_test)
###Output
_____no_output_____
###Markdown
Creamos el Modelo
###Code
n_neighbors = 7
knn = KNeighborsClassifier(n_neighbors)
knn.fit(X_train, y_train)
print('Accuracy of K-NN classifier on training set: {:.2f}'
.format(knn.score(X_train, y_train)))
print('Accuracy of K-NN classifier on test set: {:.2f}'
.format(knn.score(X_test, y_test)))
###Output
Accuracy of K-NN classifier on training set: 0.90
Accuracy of K-NN classifier on test set: 0.86
###Markdown
Resultados obtenidos
###Code
pred = knn.predict(X_test)
print(confusion_matrix(y_test, pred))
print(classification_report(y_test, pred))
h = .02 # step size in the mesh
# Create color maps
cmap_light = ListedColormap(['#FFAAAA', '#ffcc99', '#ffffb3','#b3ffff','#c2f0c2'])
cmap_bold = ListedColormap(['#FF0000', '#ff9933','#FFFF00','#00ffff','#00FF00'])
# we create an instance of Neighbours Classifier and fit the data.
clf = KNeighborsClassifier(n_neighbors, weights='distance')
clf.fit(X, y)
# Plot the decision boundary. For that, we will assign a color to each
# point in the mesh [x_min, x_max]x[y_min, y_max].
x_min, x_max = X[:, 0].min() - 1, X[:, 0].max() + 1 # columna 0 en x, resto y sumo 1 para verlo mejor (más margen)
y_min, y_max = X[:, 1].min() - 1, X[:, 1].max() + 1 # columna 1 en y, resto y sumo 1 para verlo mejor (más margen)
xx, yy = np.meshgrid(np.arange(x_min, x_max, h),
np.arange(y_min, y_max, h))
Z = clf.predict(np.c_[xx.ravel(), yy.ravel()]) # predice dos columnas que tienen todas las combinaciones x-y
# Put the result into a color plot
Z = Z.reshape(xx.shape)
plt.figure()
plt.pcolormesh(xx, yy, Z, cmap=cmap_light)
# Plot also the training points
plt.scatter(X[:, 0], X[:, 1], c=y, cmap=cmap_bold,
edgecolor='k', s=20)
plt.xlim(xx.min(), xx.max())
plt.ylim(yy.min(), yy.max())
patch0 = mpatches.Patch(color='#FF0000', label='1')
patch1 = mpatches.Patch(color='#ff9933', label='2')
patch2 = mpatches.Patch(color='#FFFF00', label='3')
patch3 = mpatches.Patch(color='#00ffff', label='4')
patch4 = mpatches.Patch(color='#00FF00', label='5')
plt.legend(handles=[patch0, patch1, patch2, patch3,patch4])
plt.title("5-Class classification (k = %i, weights = '%s')"
% (n_neighbors, 'distance'))
plt.show()
###Output
C:\Users\Admin\AppData\Roaming\Python\Python37\site-packages\ipykernel_launcher.py:21: MatplotlibDeprecationWarning: Auto-removal of grids by pcolor() and pcolormesh() is deprecated since 3.5 and will be removed two minor releases later; please call grid(False) first.
###Markdown
¿Cómo obtener el mejor valor de k?
###Code
k_range = range(1,20,2)
scores = []
for k in k_range:
knn = KNeighborsClassifier(n_neighbors= k)
knn.fit(X_train, y_train)
scores.append(knn.score(X_test, y_test))
plt.figure()
plt.xlabel('k')
plt.ylabel('accuracy')
plt.scatter(k_range, scores)
plt.xticks([0,5,10,15,20])
###Output
_____no_output_____
###Markdown
PrediccionesEjemplo: supongamos que nos llega una review de 5 palabras y sentimiento 1
###Code
nuevo_transformado = scaler.transform([[5, 1.0]]) # word count, sentiment value
print(clf.predict(nuevo_transformado))
print(clf.predict_proba(scaler.transform([[5,1.0]])))
knn = KNeighborsClassifier(n_neighbors= 7)
knn.fit(X_train, y_train)
print(knn.predict(nuevo_transformado))
print(knn.predict_proba(nuevo_transformado))
clf
knn.weights
###Output
_____no_output_____ |
Code for Each Epoch .ipynb | ###Markdown
* EN:If you run this code in every Epoch process, you can save your entire system's performance while doing business. I think you should because it's a good reason to wait 1 second longer per Epoch.* TR:Her Epoch işleminde bu kodu çalıştırırsanız tüm sisteminizi iş yaparken performansını kaydedebilirsiniz. Bence yapmalısınız Çünkü Her Epochda 1 saniye daha fazla beklemek için iyi bir sebep.
###Code
stats
###Output
_____no_output_____ |
notebooks/trade_demo/mock_notebooks/Part 5 - Perform analysis producting remote result.ipynb | ###Markdown
User: Data Scientist Goal:- Select Italy and Canada trade datasets- Perform a join between the two datasets on the `Commodity Code` column- Perform analysis on the merged dataset Summary:- Select Italy and Canada trade datasets- ETL the trade datasets- Merge the two datasets on the `Commodity Code` column- For each commodity calculate the export/import ratio- Fetch all the commodities where the export/import ratio exceeds 10%
###Code
import syft as sy
# Select the united nations network
un_network = sy.network[0]
# Login into the network
un_network_client = un.login(email="[email protected]", password="bazinga")
# Let's quickly check the datasets available on the network
un_network_client.datasets
# Filter and select the Canada and the Italy trade datasets
ca_trade_dataset_ptr = un_network_client.datasets["f3s9h1m"]
it_trade_dataset_ptr = un_network_client.datasets["42wk65l"]
# Let's filter out the data for the columns we desire.
required_columns = [
"Classification",
"Commodity Code",
"Commodity",
"Trade Value (US$)",
"Partner",
"Commodity Code",
"Trade Flow",
]
ca_dataset_ptr = ca_trade_dataset_ptr.select(columns=required_columns)
it_dataset_ptr = it_trade_dataset_ptr.select(columns=required_columns)
# In canada dataset filter out the rows where the `Partner` is `Italy`
ca_filtered_dataset_ptr = ca_dataset_ptr.filter(
ca_filtered_dataset_ptr["Partner"] == "Italy"
)
# Similary, in italy dataset filter out the rows where the `Partner` is `Canada`
it_filtered_dataset_ptr = it_dataset_ptr.filter(
ca_filtered_dataset_ptr["Partner"] == "Canada"
)
# Join the two datasets
merged_dataset_ptr = sympc.merge(
left=ca_filtered_dataset_ptr,
right=it_filtered_dataset_ptr,
on="Commodity Code",
how="inner",
suffixes=("_ca", "_it"),
)
merged_dataset_ptr.column_description
ca_imports_it_exports = merged_dataset_ptr.filter(
merged_dataset_ptr["Partner_ca"] == "Imports"
)
ca_export_it_imports = merged_dataset_ptr.filter(
merged_dataset_ptr["Partner_ca"] == "Exports"
)
# Select the commodities where the error rate is greater than 10%
commodities1_with_error_gt_10 = ca_imports_it_exports.filter(
(ca_imports_it_exports["Trade Value_it"] / ca_imports_it_exports["Trade Value_ca"])
> 0.1
).select(columns=["Commodity Code"])
commodities2_with_error_gt_10 = ca_export_it_imports.filter(
(ca_export_it_imports["Trade Value_ca"] / ca_export_it_imports["Trade Value_it"])
> 0.1
).select(columns=["Commodity Code"])
###Output
_____no_output_____
###Markdown
Awesome !!! We have successfully selected the commodity code where the import to export ratio is greater than 10%. Dummy data
###Code
import pandas as pd
from enum import Enum
## Dummy Data Store
dataset_store = [
{
"Name": "breast_cancer",
"Tags": ["mri", "breast cancer", "dicoms"],
"Description": "Labelled image dataset of patients suffering different types of breast cancer",
"Dtype": "ImageClassificationDataset",
"Id": "56lkw24",
"Domain": "WHO",
"Shape": "((25000, 300, 300), (25000))",
},
{
"Name": "canada_trade_data",
"Tags": ["canada", "trade", "un", "commodities"],
"Description": "This dataset represents aggregated trade statistics as reported by Canada about what it believes was imported/exported to/from its country in Feb 2021.",
"Dtype": "DataFrame",
"Id": "f3s9h1m",
"Domain": "Canada",
"Shape": "(25000, 22)",
},
{
"Name": "netherlands_trade_data",
"Tags": ["netherlands", "trade", "commodities", "export"],
"Description": "This dataset represents aggregated trade statistics as reported by Netherlands about what it believes was imported/exported to/from its country in Feb 2021.",
"Dtype": "DataFrame",
"Id": "2kf3o5d",
"Domain": "Netherlands",
"Shape": "(35000, 22)",
},
{
"Name": "italy_trade_data",
"Tags": ["italy", "trade", "un", "commodities", "export", "import"],
"Description": "This dataset represents aggregated trade statistics as reported by Italy about what it believes was imported/exported to/from its country in Feb 2021.",
"Dtype": "DataFrame",
"Id": "42wk65l",
"Domain": "Italy",
"Shape": "(30000, 22)",
},
{
"Name": "us_trade_data",
"Tags": ["us", "trade", "un", "commodities"],
"Description": "This dataset represents aggregated trade statistics as reported by United States about what it believes was imported/exported to/from its country in Feb 2021.",
"Dtype": "DataFrame",
"Id": "86pfgh1",
"Domain": "United States",
"Shape": "(40000, 22)",
},
]
dataset_store = pd.DataFrame(dataset_store)
class bcolors(Enum):
HEADER = "\033[95m"
OKBLUE = "\033[94m"
OKCYAN = "\033[96m"
OKGREEN = "\033[92m"
WARNING = "\033[93m"
FAIL = "\033[91m"
ENDC = "\033[0m"
BOLD = "\033[1m"
UNDERLINE = "\033[4m"
d={
'Column': {0: 'Classification_ca',
1: 'Commodity Code',
2: 'Commodity_ca',
3: 'Trade Value_ca',
4: 'Partner_ca',
5: 'Trade Flow_ca',
6: 'Classification_it',
7: 'Commodity_it',
8: 'Trade Value_it',
9: 'Partner_it',
10: 'Trade Flow_it'},
'Description': {0: 'Commodity Classification (HS= Harmonized System)',
1: 'HS Commodity Code',
2: 'Description',
3: 'in US dollars',
4: 'Description',
5: 'Description',
6: 'Commodity Classification (HS= Harmonized System)',
7: 'Description',
8: 'in US dollars',
9: 'Description',
10: 'Description'},
'Private': {0: True, 1: True, 2: True, 3: True, 4: False, 5: False, 6: True, 7: True, 8: True, 9: True, 10: False}}
merged_dataset_schema = pd.DataFrame.from_dict(d)
###Output
_____no_output_____ |
notebooks/tstoolbox_statistics_python.ipynb | ###Markdown
tstoolbox: StatisticsI will use the water level record for USGS site '02240000 OCKLAWAHA RIVER NEAR CONNER, FL'. Use tsgettoolbox to download the data we will be using.
###Code
from tsgettoolbox import tsgettoolbox
from tstoolbox import tstoolbox
ock_flow = tsgettoolbox.nwis(
sites="02240000", startDT="2008-01-01", endDT="2016-01-01", parameterCd="00060"
)
ock_flow.head()
ock_stage = tsgettoolbox.nwis(
sites="02240000", startDT="2008-01-01", endDT="2016-01-01", parameterCd="00065"
)
ock_stage.head()
###Output
_____no_output_____
###Markdown
The tstoolbox.rolling_window calculates the average stage over the spans listed in the 'span' argument.
###Code
r_ock_stage = tstoolbox.rolling_window(
input_ts=ock_stage, span="1,14,30,60,90,120,180,274,365", statistic="mean"
)
a_ock_stage = tstoolbox.aggregate(
input_ts=r_ock_stage, agg_interval="A", statistic="max"
)
a_ock_stage
q_max_avg_obs = tstoolbox.calculate_fdc(input_ts=a_ock_stage, sort_order="descending")
q_max_avg_obs
tstoolbox.plot(
input_ts=q_max_avg_obs,
ofilename="q_max_avg.png",
type="norm_xaxis",
xtitle="Annual Exceedence Probability (%)",
ytitle="Stage (feet)",
title="N-day Maximum Rolling Window Average Stage for 0224000",
legend_names="1,14,30,60,90,120,180,274,365",
)
###Output
_____no_output_____
###Markdown

###Code
combined = ock_flow.join(ock_stage)
tstoolbox.plot(
input_ts=combined,
type="xy",
ofilename="stage_flow.png",
legend=False,
title="Plot of Daily Stage vs Flow for 02240000",
xtitle="Flow (cfs)",
ytitle="Stage (ft)",
)
###Output
_____no_output_____ |
tutorials/2-intervention/simpsons-paradox-and-defining-causal-effects.ipynb | ###Markdown
Simpsons Paradox Installing Packages
###Code
pip install pandas
pip install pgmpy
###Output
Requirement already satisfied: pgmpy in /usr/local/lib/python3.7/dist-packages (0.1.15)
Requirement already satisfied: pyparsing in /usr/local/lib/python3.7/dist-packages (from pgmpy) (2.4.7)
Requirement already satisfied: torch in /usr/local/lib/python3.7/dist-packages (from pgmpy) (1.9.0+cu102)
Requirement already satisfied: joblib in /usr/local/lib/python3.7/dist-packages (from pgmpy) (1.0.1)
Requirement already satisfied: scipy in /usr/local/lib/python3.7/dist-packages (from pgmpy) (1.4.1)
Requirement already satisfied: pandas in /usr/local/lib/python3.7/dist-packages (from pgmpy) (1.1.5)
Requirement already satisfied: tqdm in /usr/local/lib/python3.7/dist-packages (from pgmpy) (4.41.1)
Requirement already satisfied: numpy in /usr/local/lib/python3.7/dist-packages (from pgmpy) (1.19.5)
Requirement already satisfied: statsmodels in /usr/local/lib/python3.7/dist-packages (from pgmpy) (0.10.2)
Requirement already satisfied: networkx in /usr/local/lib/python3.7/dist-packages (from pgmpy) (2.5.1)
Requirement already satisfied: scikit-learn in /usr/local/lib/python3.7/dist-packages (from pgmpy) (0.22.2.post1)
Requirement already satisfied: typing-extensions in /usr/local/lib/python3.7/dist-packages (from torch->pgmpy) (3.7.4.3)
Requirement already satisfied: pytz>=2017.2 in /usr/local/lib/python3.7/dist-packages (from pandas->pgmpy) (2018.9)
Requirement already satisfied: python-dateutil>=2.7.3 in /usr/local/lib/python3.7/dist-packages (from pandas->pgmpy) (2.8.1)
Requirement already satisfied: patsy>=0.4.0 in /usr/local/lib/python3.7/dist-packages (from statsmodels->pgmpy) (0.5.1)
Requirement already satisfied: decorator<5,>=4.3 in /usr/local/lib/python3.7/dist-packages (from networkx->pgmpy) (4.4.2)
Requirement already satisfied: six>=1.5 in /usr/local/lib/python3.7/dist-packages (from python-dateutil>=2.7.3->pandas->pgmpy) (1.15.0)
###Markdown
Importing packages
###Code
import pandas as pd
from pgmpy.models import BayesianModel as bm
from pgmpy.inference import VariableElimination
###Output
_____no_output_____
###Markdown
Read data
###Code
click_data = pd.read_csv("clickdata.csv",usecols=('X','Y','Z'))
click_data
###Output
_____no_output_____
###Markdown
Creating model
###Code
model = bm()
model.add_edges_from(
[
('Z','X'),
('X','Y'),
('Z','Y')
]
)
model.fit(click_data)
print(model.get_cpds()[0])
print(model.get_cpds()[1])
print(model.get_cpds()[2])
###Output
+-------------+------------+------------+--------------+--------------+
| X | X(engaged) | X(engaged) | X(unengaged) | X(unengaged) |
+-------------+------------+------------+--------------+--------------+
| Z | Z(old) | Z(young) | Z(old) | Z(young) |
+-------------+------------+------------+--------------+--------------+
| Y(click) | 0.075 | 0.4 | 0.05 | 0.3 |
+-------------+------------+------------+--------------+--------------+
| Y(no click) | 0.925 | 0.6 | 0.95 | 0.7 |
+-------------+------------+------------+--------------+--------------+
###Markdown
Inference
###Code
inference = VariableElimination(model)
theta_u = inference.query(['Y'],{'X': 'unengaged'})
print(theta_u)
theta_u.values[0]
theta_e = inference.query(['Y'],{'X': 'engaged'})
print(theta_e)
theta_e.values[0]
theta_e.values[0] - theta_u.values[0]
###Output
_____no_output_____
###Markdown
Updated model inference
###Code
model = model.do('X')
inference = VariableElimination(model)
gamma_u = inference.query(['Y'],{'X': 'unengaged'})
print(gamma_u)
gamma_e = inference.query(['Y'],{'X': 'engaged'})
print(gamma_e)
gamma_e.values[0] - gamma_u.values[0]
###Output
_____no_output_____ |
contours.ipynb | ###Markdown
In this notebook, I will be attempting to consolidate the many cells of code which creates the 90x180 rebinned grids in previous notebooks to (hopefull) singular functions. Then, I will try to plot land cover with contours showing cloud optical thickness and cloud top pressure first, land cover
###Code
def terminator(date):
'''
inputs: date - solar.datetime object
output: np
'''
lons2, lats2, daynight = solar.daynight_grid(dat, 2, -180, 179) # gets grid of day/night values, night = 1, day = NaN
daynight = np.delete(daynight, 90, 0) # gets daynight to be 90x180
daynight = daynight[::-1] # corrects lat/lon to same as MODIS data
return daynight
def landtypes(landfile, snowfile, landsds_name, snowsds_name, daynight, in_res = 0.05):
'''
inputs: landfile (str) - path to HDF file containing MODIS land cover data (MCD12C1)
snowfile (str) - path to HDF file containing MODIS snow cover data (MYD10C1)
landsds_name (str) - name of desired SDS from MODIS land cover file
snowsds_name (str) - name of desired SDS from MODIS snow cover file
daynight - numpy masked array (output of local function func.terminator)
in_res - resolution of input data in degrees
output - landcover - 90 x 180 numpy array of integers showing land type (from func.modismap)
'''
itdat = range(90) # used for iterating through cot and ctp data
itdat2 = range(180)
#----------SNOW DATA-----------------#
snowhdf = SD(snowfile, SDC.READ)
snowsds = snowhdf.select(snowsds_name)
snowvals = np.array(snowsds.get())
#----------LAND DATA-----------------#
landhdf = SD(landfile, SDC.READ)
landsds = landhdf.select(landsds_name)
landvals = np.array(landsds.get())
landmodes = submatrx_mode(landvals, in_res) # rebins yearly data to 2x2
snowmodes = submatrx_mode(snowvals, in_res) # "
landcover = modismap(landmodes)
for i in range(len(snowmodes[0:74])):
for j in range(len(snowmodes[i])): # this is the world above lat -60 deg.
if (snowmodes[i][j] > 0) and (snowmodes[i][j] <= 100):
landcover[i][j] = 5 # this changes snow values to value corresponding to snow in land data
for i in range(len(daynight)):
for j in range(len(daynight[i])):
if daynight[i][j] == 1.0:
landcover[i][j] = np.nan
west_edge = range(0,26)
east_edge = range(115,180)
print landcover
# makes values None outside of lon range
for i in itdat:
for j in west_edge:
landcover[i][j] = np.nan
for i in itdat:
for j in east_edge:
landcover[i][j] = np.nan
return landcover
dat = datetime(2013,5,18,21,20,0) # date of observation at time 21:20 UT
daynight = terminator(dat)
snowfile = 'MYD10C1_5.18.2013.hdf'
snowsds_name = 'Day_CMG_Snow_Cover'
landfile = 'MCD12C1.2013.hdf'
landsds_name = 'Majority_Land_Cover_Type_1'
land = landtypes(landfile, snowfile, landsds_name, snowsds_name, daynight)
print np.shape(daynight)
###Output
(90L, 180L)
###Markdown
Now, cloud opt. thickness and cloud top pressure
###Code
def rebindat(data, bins):
'''
rebins your data grid - keeping shape, but changing values
'''
new_data = copy.deepcopy(data)
for i in range(len(new_data)):
for j in range(len(new_data[i])):
if np.isnan(new_data[i][j]) != True:
num = min(bins, key=lambda x:abs(x-data[i][j]))
new_data[i][j] = num
return new_data
def cloudtypes(cloudfile, sdslist, ctpbins, cotbins, daynight, is_ctp = True):
'''
Use this function to extract data from MYD08_D3 files - particularly cloud top pressure (CTP)
and cloud optical thickness (COT) data. This function can be used to extract SDS's, but is
geared toward these two since these are the primary SDS's this Earthshine project uses.
Produces 2 x 2 degree numpy arrays of global COT and CTP for which one can choose how the
values are binned. This array can then be plotted or saved as an ascii table by the user
INPUTS: cloudfile: path to HDF file containing MODIS cloud profile data
sdslist: name of desired SDS from MODIS cloud profile file
ctpbins: list of bin edges for values of CTP
cotbins: list of bin edges for values of COT
daynight: numpy masked array (output of local function func.terminator)
is_ctp: if True, then converts units of ctp from hPa to bar. Set to False if getting
data for an SDS which is not CTP
OUTPUTS: cot: 90 x 180 numpy array containing rebinned COT data
ctp: 90 x 180 numpy array containing rebinned CTP data
'''
# opens hdf file, gets the SDS's
hdf, sds = getSDS(cloudfile, sdslist)
cot = sds[0] # cloud optical thickness
ctp = sds[1] # cloud top pressure
itdat = range(90) # used for iterating through cot and ctp data
itdat2 = range(180)
# rebins array shape of both to 2 x 2 degrees
cot = binavg(cot,1)
ctp = binavg(ctp,1)
if is_ctp == True:
ctp *= 0.001 # converts from hPa to bars
ctp = rebindat(ctp, ctpbins) # rebins ctp values to desired bins
cot = rebindat(cot, cotbins)
west_edge = range(0,26) # west edge of earth's visible disk from moon
east_edge = range(115,180) # east edge of earth's visible disk from moon
# makes values None outside of lon range
for i in itdat:
for j in west_edge:
cot[i][j] = np.nan
ctp[i][j] = np.nan
for i in itdat:
for j in east_edge:
cot[i][j] = np.nan
ctp[i][j] = np.nan
for i in range(len(daynight)):
for j in range(len(daynight[i])):
if daynight[i][j] == 1.0:
cot[i][j] = np.nan
ctp[i][j] = np.nan
return ctp, cot
cloudfile = 'MYD08_D3_5.18.13.hdf'
sdslist = ['Cloud_Optical_Thickness_Combined_Mean', 'Cloud_Top_Pressure_Mean']
ctp_bins = [0.0000003, 0.025, 0.189, 0.213, 0.238, 0.268, 0.299, 0.332,
0.372, 0.411, 0.554, 0.591, 0.628, 0.710, 0.902, 1.03]
cot_bins = [0,2,4,6,8,10,12,14,16,18,20,25,30,35,40,52,64,76,88,100]
cot_bins_crude = [0, 2, 5, 10, 15, 20, 25, 30, 35, 40, 52, 64, 76, 88, 100]
ctp, cot = ctp_cot(cloudfile, sdslist, ctp_bins, cot_bins, daynight)
ctp, cot_crude = ctp_cot(cloudfile, sdslist, ctp_bins, cot_bins_crude, daynight)
###Output
func.py:89: RuntimeWarning: Mean of empty slice
binned.append(np.nanmean(catch)) # finds mean of all values within a bin
###Markdown
Now, I try to plot land cover with ctp and/or cot contours First, make a custom colorbar so that land type plot isnt so weird.
###Code
# From matplotlib tutorial
my_cmap = ListedColormap(['xkcd:black',
'xkcd:royal blue',
'xkcd:forest green',
'xkcd:green',
'xkcd:beige',
'xkcd:white'])
'''
THESE ARE THE GOOD PLOTS READY FOR PUBLICATION
'''
fig = plt.figure(figsize=(15,15))
plt.rc('font', family='serif', weight = 545, size = 15)
m = Basemap(projection='ortho', resolution='l', lat_0=0, lon_0 = -39.8333)
# ^ to plot on a globe
m.drawcoastlines(linewidth=0.8)
m.drawparallels(np.arange(-90., 120., 30.), labels=[1, 0, 0, 0])
m.drawmeridians(np.arange(-180., 181., 45.), labels=[0, 0, 0, 1])
lat = np.arange(-90,89,2)
lon = np.arange(-180,180,2)
x, y = m(*np.meshgrid(lon, lat))
ax1 = m.pcolor(x, y, land[::-1], cmap=plt.cm.get_cmap(my_cmap,6))
plt.clim(0,5)
cbar = plt.colorbar(ax1, label='land type', orientation='vertical', ticks=range(6), shrink = 0.6)
cbar.outline.set_edgecolor('xkcd:black')
cbar.outline.set_linewidth(1)
cbar.ax.set_yticklabels(['fill', 'ocean', 'forest', 'grass', 'sand', 'ice'], {'fontsize':15})
cbar.outline.set_label({'fontsize':15})
ax2 = m.contourf(x, y, ctp[::-1], levels = ctp_bins, alpha = 0.5, cmap='Greys_r')
contour_bar = plt.colorbar(ax2, label = 'Cloud Top Pressure (bar)', orientation='vertical', shrink = 0.6)
contour_bar.outline.set_edgecolor('xkcd:black')
contour_bar.outline.set_linewidth(1)
#cax = contour_bar.ax
#contour_bar.ax.set_yticklabels(ctpbins, {'fontsize':15})
'''
cb_ax = contour_bar.ax
ax.text(1.3,0.5,r'$\tau$',rotation=90)
'''
#plt.title('2x2 Degree Land Cover of Earth as Seen from the Moon w/ Cloud Top Pressure - 5/18/13 21:20 UTC', {'fontsize':15})
#plt.savefig('landcover_ctp.pdf')
plt.show()
###Output
Warning: Cannot label parallels on Orthographic basemap'Warning: Cannot label meridians on full-disk
Geostationary, Orthographic or Azimuthal equidistant basemap
###Markdown
7-30-18I'm going to try to use gridspec to create more professional plots, using Devin's githubnotebooks as reference
###Code
from matplotlib import gridspec as gridspec
import cartopy
import cartopy.crs as ccrs
#plt.close(1)
fig = plt.figure(figsize=(7.6,8))
m = Basemap(projection='ortho', resolution='l', lat_0=0, lon_0 = -39.8333)
# ^ to plot on a globe
m.drawcoastlines()
m.drawparallels(np.arange(-90., 120., 30.), labels=[1, 0, 0, 0])
m.drawmeridians(np.arange(-180., 181., 45.), labels=[0, 0, 0, 1])
lat = np.arange(-90,89,2)
lon = np.arange(-180,180,2)
x, y = m(*np.meshgrid(lon, lat))
gs = gridspec.GridSpec(1,3, height_ratios=[1], width_ratios=[0.05,1,0.05])
#----SUBPLOT 1----#
ax2 = plt.subplot(gs[0,1])
plt1 = m.pcolor(x, y, land[::-1], ax = ax2, cmap=plt.cm.get_cmap(my_cmap,6), clim = (0,5))
#plt2 = m.contourf(x, y, ctp[::-1], levels = ctp_bins, alpha = 0.2, cmap='Greys_r')
#----SUBPLOT 2-----#
#cbax = plt.subplot(gs[0,2]) # Place it where it should be.
cbar = plt.colorbar(mappable = plt1, cax = ax2, label = 'land type', orientation='vertical', ticks=range(6))
cbar.outline.set_edgecolor('xkcd:black')
cbar.outline.set_linewidth(1)
cbar.ax.set_yticklabels(['fill', 'ocean', 'forest', 'grass', 'sand', 'ice'], {'fontsize':15})
cbar.outline.set_label({'fontsize':20})
#ax2 = plt.subplot(gs[0,0])
#----SUBPLOT 3----#
#cbax = plt.subplot(gs[0,0])
# Left
'''
cbax = plt.subplot(gs[0,0]) # Place it where it should be.
cb = plt.colorbar(cax = cbax, mappable = plt2, orientation = 'vertical', ticklocation = 'left')
cb.set_label(r'Colorbar !', labelpad=10)
'''
plt.show()
fig = plt.figure(figsize=(15,15))
plt.rc('font', family='serif', weight = 545, size = 15)
m = Basemap(projection='ortho', resolution='l', lat_0=0, lon_0 = -39.8333)
# ^ to plot on a globe
m.drawcoastlines(linewidth=0.8)
m.drawparallels(np.arange(-90., 120., 30.), labels=[1, 0, 0, 0])
m.drawmeridians(np.arange(-180., 181., 45.), labels=[0, 0, 0, 1])
lat = np.arange(-90,89,2)
lon = np.arange(-180,180,2)
x, y = m(*np.meshgrid(lon, lat))
ax1 = m.pcolor(x, y, land[::-1], cmap=plt.cm.get_cmap(my_cmap,6))
plt.clim(0,5)
cbar = plt.colorbar(ax1, label = 'land type', orientation='vertical', ticks=range(6), shrink = 0.64)
cbar.outline.set_edgecolor('xkcd:black')
cbar.outline.set_linewidth(1)
cbar.ax.set_yticklabels(['fill', 'ocean', 'forest', 'grass', 'sand', 'ice'], {'fontsize':15})
cbar.outline.set_label({'fontsize':20})
ax2 = m.contourf(x, y, ctp[::-1], levels = ctp_bins, alpha = 0.5, cmap='Greys_r')
contour_bar = plt.colorbar(ax2, label = 'Cloud Top Pressure (bar)', orientation='vertical', shrink = 0.64)
contour_bar.outline.set_edgecolor('xkcd:black')
contour_bar.outline.set_linewidth(1)
cax = contour_bar.ax
#contour_bar.ax.set_yticklabels(ctpbins, {'fontsize':15})
#pos2 = [pos1.x0 + 0.3, pos1.y0 + 0.3, pos1.width / 2.0, pos1.height / 2.0]
plt.title('2x2 Degree Land Cover of Earth as Seen from the Moon w/ Cloud Top Pressure - 5/18/13 21:20 UTC',
{'fontsize':15},
loc = 'left')
#plt.savefig('landcover_ctp.pdf')
#plt.tight_layout()
plt.show()
### from Devin's code
def set_gridspec(widths=[12], heights=[12], wspace=0, hspace=0):
fig = plt.figure(figsize=(sum(widths) + wspace * (len(widths) - 1),
sum(heights) + hspace * (len(heights) - 1)))
gs = gridspec.GridSpec(len(heights), len(widths),
height_ratios=heights, width_ratios=widths)
return fig, gs
fig, gs = set_gridspec(widths=[75], heights=[10, 10])
ax = fig.add_subplot(gs[0])
###Output
_____no_output_____
###Markdown
1.6 Micron DataNow I make the same plots of land cover/ cloud props, but with 1.6 $\mu$m data from MODIS. I choose to use the liquid cloud cloud optical thickness and cloud top pressure instead of ice cot/ctp.
###Code
sdslist_16 = ['Cloud_Optical_Thickness_16_Liquid_Mean', 'Cloud_Optical_Thickness_16_Ice_Mean']
liq_16, ice_16 = cloudtypes(cloudfile, sdslist_16, cot_bins, cot_bins, daynight, is_ctp = False)
liq_16_crude, ice_16_crude = cloudtypes(cloudfile, sdslist_16,
cot_bins_crude, cot_bins_crude, daynight, is_ctp = False)
###--------UNIQUE COMBO PLOT--------###
fig = plt.figure(figsize=(15,15))
plt.rc('font', family='serif', weight = 545, size = 15)
m = Basemap(projection='ortho', resolution='l', lat_0=0, lon_0 = -39.8333)
# ^ to plot on a globe
m.drawcoastlines(linewidth=0.8)
m.drawparallels(np.arange(-90., 120., 30.), labels=[1, 0, 0, 0])
m.drawmeridians(np.arange(-180., 181., 45.), labels=[0, 0, 0, 1])
lat = np.arange(-90,89,2)
lon = np.arange(-180,180,2)
x, y = m(*np.meshgrid(lon, lat))
ax1 = m.pcolor(x, y, land[::-1], cmap=plt.cm.get_cmap(my_cmap,6))
plt.clim(0,5)
cbar = plt.colorbar(ax1, label = 'land type', orientation='vertical', ticks=range(6), shrink = 0.6)
cbar.outline.set_edgecolor('xkcd:black')
cbar.outline.set_linewidth(1)
cbar.ax.set_yticklabels(['fill', 'ocean', 'forest', 'grass', 'sand', 'ice'], {'fontsize':15})
cbar.outline.set_label({'fontsize':20})
ax2 = m.contourf(x, y, ctp[::-1], levels = ctp_bins, alpha = 0.5, cmap='Greys_r')
contour_bar = plt.colorbar(ax2, label = 'Cloud Top Pressure (bar)', orientation='vertical', shrink = 0.6)
contour_bar.outline.set_edgecolor('xkcd:black')
contour_bar.outline.set_linewidth(1)
cax = contour_bar.ax
#contour_bar.ax.set_yticklabels(ctpbins, {'fontsize':15})
plt.title('2x2 Degree Land Cover of Earth as Seen from the Moon w/ Cloud Top Pressure - 5/18/13 21:20 UTC', {'fontsize':15})
plt.savefig('landcover_ctp.pdf')
#plt.show()
###--------SINGLE PARAMETER PLOT--------###
fig = plt.figure(figsize=(15,15))
plt.rc('font', family='serif', weight = 545, size = 15)
m = Basemap(projection='ortho', resolution='l', lat_0=0, lon_0 = -39.8333)
# ^ to plot on a globe
m.drawcoastlines(linewidth=0.8)
m.drawparallels(np.arange(-90., 120., 30.), labels=[1, 0, 0, 0])
m.drawmeridians(np.arange(-180., 181., 45.), labels=[0, 0, 0, 1])
lat = np.arange(-90,89,2)
lon = np.arange(-180,180,2)
x, y = m(*np.meshgrid(lon, lat))
ax1 = m.pcolor(x, y, ice_16[::-1],
cmap=plt.cm.get_cmap('viridis',len(cot_bins)))
plt.clim(0, len(cot_bins) - 1)
cbar = plt.colorbar(ax1,
label = r'Cloud Optical Thickness 1.6 $\mu$m (bar)',
orientation = 'vertical',
ticks=range(len(cot_bins)),
shrink = 0.6)
cbar.outline.set_edgecolor('xkcd:black')
cbar.outline.set_linewidth(1)
cbar.ax.set_yticklabels(cot_bins, {'fontsize':15})
cbar.outline.set_label({'fontsize':20})
plt.legend()
plt.title('2x2 Degree Land Cover of Earth as Seen from the Moon w/ Cloud Optical Thickness - 5/18/13 21:20 UTC', {'fontsize':15})
#plt.savefig('landcover_ctp.pdf')
plt.show()
def simplehist(dat, bins, xlabel, title):
sns.set()
fig = plt.figure( figsize = (25,15) )
plt.hist(dat, bins = bins, range = (min(bins), max(bins))) # add additional value because last bin is inclusive
plt.xlabel('Cloud Top Pressure (bar)')
plt.xticks(bins)
plt.ylabel('Counts')
plt.title(title)
plt.show()
#plt.savefig('histogram_ctp_reduced.png')
simplehist(liq_16.flatten(), cot_bins,
'COT Liquid',
'Histogram Liquid COT for 2x2 Degree Lit Earth as Seen Moon')
###Output
_____no_output_____
###Markdown
Now, a function for finding unique combinations
###Code
def unique_combos(arr1, arr2, arr3):
'''
Takes 3 arrays of the same shape, returns an array containing lists of each unique
combination of the three arrays at each place in the arrays.
INPUTS: arr1, arr2, arr3 - 2D numpy arrays
OUTPUTS: combos - list containing lists (each of length 3) of each unique combination
'''
itdat = range(len(arr1))
itdat2 = range(len(arr1[0]))
arr1_new = copy.deepcopy(arr1)
arr2_new = copy.deepcopy(arr2)
arr3_new = copy.deepcopy(arr3)
for i in itdat:
for j in itdat2:
if np.isnan(arr1_new[i][j]) == True:
arr1_new[i][j] = -9999.0
if np.isnan(arr2_new[i][j]) == True:
arr2_new[i][j] = -9999.0
if np.isnan(arr3_new[i][j]) == True:
arr3_new[i][j] = -9999.0
#print arr1_new[np.isnan(arr1_new) == True]
combo_list = [[arr1_new[i][j], arr2_new[i][j], arr3_new[i][j]]
for i in itdat
for j in itdat2]
combos = []
for i in combo_list:
if i not in combos:
combos.append(i)
return combos
combos_liq = unique_combos(land, liq_16, ctp)
combos_ice = unique_combos(land, ice_16, ctp)
combos_liq_crude = unique_combos(land, liq_16_crude, ctp)
combos_ice_crude = unique_combos(land, ice_16_crude, ctp)
print len(combos_liq)
print len(combos_ice)
print len(combos_liq_crude)
print len(combos_ice_crude)
#crude_cot = rebindat(cot, cot_bins_crude)
combos_orig = unique_combos(land, cot, ctp)
combos_crude = unique_combos(land, cot_crude, ctp)
combos_orig_redo = unique_combos(cot, ctp, land)
print len(combos_orig)
print len(combos_crude)
print len(combos_orig_redo)
print combos_orig
print ice_16[np.isnan(ice_16) != True]
###Output
[ 30. 25. 25. ..., 35. 10. 40.]
###Markdown
Here, I test the unique_combos function with various test arrays
###Code
# TEST LISTS
[1., 1., 1.]
[3., 2., 1.]
[2., 2., 2.]
[1., 2., 3.]
test1 = np.array([[1., 1., 1., 1., 1., 1., 2.], [2., 3., 2., 1., 3., 2., 1.]])
test2 = np.array([[1., 2., 3., 3., 2., 1., 1.], [1., 1., 2., 3., 1., 2., 3.]])
test3 = np.array([[1., 1., 1., 3., 2., 1., 2.], [2., 1., 1., 1., 1., 1., 1.]])
# there are 4 unique combos
print len(unique_combos(test1, test2, test3))
print test1[0][0]
ctp_copy = copy.deepcopy(ctp)
land_copy = copy.deepcopy(land)
cot_copy = copy.deepcopy(cot)
print ctp_copy[np.isnan(ctp_copy) != True]
for i in range(len(ctp_copy)):
for j in range(len(ctp_copy[i])):
if np.isnan(land_copy[i][j]) == True:
land_copy[i][j] = -9999.0
if np.isnan(cot_copy[i][j]) == True:
cot_copy[i][j] = -9999.0
if np.isnan(ctp_copy[i][j]) == True:
ctp_copy[i][j] = -9999.0
print ctp_copy[ctp_copy != -9999.]
'''
combo_list = [[land_copy[i][j], cot_copy[i][j], ctp_copy[i][j]]
for i in range(len(ctp_copy))
for j in range(len(ctp_copy[i]))]
'''
combo_list = []
for i in range(len(ctp_copy)):
for j in range(len(ctp_copy[i])):
sample = []
#combo_list.append([land_copy[i][j], cot_copy[i][j], ctp_copy[i][j]])
#print ctp_copy[i][j]
a = land_copy[i][j]
b = cot_copy[i][j]
c = ctp_copy[i][j]
sample.append(a)
sample.append(b)
sample.append(c)
combo_list.append(sample)
#print combo_list
boom = []
for i in combo_list:
if i not in boom:
boom.append(i)
print len(boom)
ascii.write(ctp.T, 'ctp_moonview.txt')
###Output
_____no_output_____ |
Lectures/Lecture6-Streams/SGD TESTING.ipynb | ###Markdown
Stochastic Gradient Descentdiscriminative learning of linear classifiers under convex loss functions such as (linear) Support Vector Machines and Logistic Regression. SGD has been successfully applied to large-scale and sparse machine learning problems often encountered in text classification and natural language processing. Given that the data is sparse, the classifiers in this module easily scale to problems with more than 10^5 training examples and more than 10^5 features.The advantages of Stochastic Gradient Descent are:Efficiency.Ease of implementation (lots of opportunities for code tuning).The disadvantages of Stochastic Gradient Descent include:SGD requires a number of hyperparameters such as the regularization parameter and the number of iterations.SGD is sensitive to feature scaling.http://scikit-learn.org/stable/modules/sgd.htmlThe class SGDClassifier implements a plain stochastic gradient descent learning routine which supports different loss functions and penalties for classification.
###Code
from sklearn.linear_model import SGDClassifier
X = [[0., 0.], [1., 1.]] #N_samples, N_features - training samples
y = [0, 1] # n_samples - target values for training the samples
clf = SGDClassifier(loss="hinge", penalty="l2")
clf.fit(X, y)
clf.predict([[2.,2.]]) # predict new value
clf.coef_ # fits a linear model to the training data , coef_ holds the model parameters
clf.intercept_ # contains the offset of the linear equation
clf.decision_function([[2., 2.]])
###Output
_____no_output_____
###Markdown
The concrete loss function can be set via the loss parameter. SGDClassifier supports the following loss functions:loss="hinge": (soft-margin) linear Support Vector Machine,loss="modified_huber": smoothed hinge loss,loss="log": logistic regression,and all regression losses below.The first two loss functions are lazy, they only update the model parameters if an example violates the margin constraint, which makes training very efficient and may result in sparser models, even when L2 penalty is used.Using loss="log" or loss="modified_huber" enables the predict_proba method, which gives a vector of probability estimates P(y|x) per sample x:
###Code
clf = SGDClassifier(loss="log").fit(X, y)
clf.predict_proba([[1., 1.]])
###Output
_____no_output_____
###Markdown
The default setting is penalty="l2". The L1 penalty leads to sparse solutions, driving most coefficients to zero. The Elastic Net solves some deficiencies of the L1 penalty in the presence of highly correlated attributes. The parameter l1_ratio controls the convex combination of L1 and L2 penalty.
###Code
%matplotlib inline
# SGD: Maximum Margin Separating hyperplan
import numpy as np
import matplotlib.pyplot as plt
from sklearn.linear_model import SGDClassifier
from sklearn.datasets.samples_generator import make_blobs
# we create 50 separable points
X, Y = make_blobs(n_samples=50, centers=2, random_state=0, cluster_std=0.60)
# fit the model
clf = SGDClassifier(loss="hinge", alpha=0.01, n_iter=200, fit_intercept=True)
clf.fit(X, Y)
# plot the line, the points, and the nearest vectors to the plane
xx = np.linspace(-1, 5, 10)
yy = np.linspace(-1, 5, 10)
X1, X2 = np.meshgrid(xx, yy)
Z = np.empty(X1.shape)
for (i, j), val in np.ndenumerate(X1):
x1 = val
x2 = X2[i, j]
p = clf.decision_function([x1, x2])
Z[i, j] = p[0]
levels = [-1.0, 0.0, 1.0]
linestyles = ['dashed', 'solid', 'dashed']
colors = 'k'
plt.contour(X1, X2, Z, levels, colors=colors, linestyles=linestyles)
plt.scatter(X[:, 0], X[:, 1], c=Y, cmap=plt.cm.Paired)
plt.axis('tight')
plt.show()
###Output
_____no_output_____ |
0 - Quick Intro to Python.ipynb | ###Markdown
Welcome to the wonderful world of PythonSo Python ha. Is that like the reptile - Python . Well, no. It's because the developers liked Monty Python's flying Circus :DSo, most programming languages can be divided into two categories: Interpreted and Compiled An interpreted language converts the code into bytecode for an 'interpreter' to 'interpret' Whereas in a complied language the compiler converts it directly to machine code. Was that a little complicated ? Amm well, let's just start with something simple. Open Python in your favourite way. I like ipython, so I'd probably type "ipython" on my terminal and start.Let's say Hello to the world
###Code
print("Hello World")
###Output
_____no_output_____
###Markdown
Voila. Wasn't that amazing. Ha.. well, wait till you finish this whole tutorial, and be ready to have your mind blown :P Python MathBTW did you know you can do calculations in python. Try this
###Code
123+123
222-89
21/3
###Output
_____no_output_____
###Markdown
Now try this.
###Code
23/3
###Output
_____no_output_____
###Markdown
Notice something. We are dividing integers, but the result is .. well, .. a float. Now let's try Modulo.
###Code
9%2
###Output
_____no_output_____
###Markdown
Check out floor division though
###Code
9//2
###Output
_____no_output_____
###Markdown
Wait. How about
###Code
9.0//2.0
###Output
_____no_output_____
###Markdown
Aha. Now try
###Code
-11.0//3
###Output
_____no_output_____
###Markdown
Exponent is a **
###Code
2**3
###Output
_____no_output_____ |
handsOn/ch5/SupportVectorMachines.ipynb | ###Markdown
Ch5: Support Vector MachinesA Popular ML model, useful for linear and non-linear classification, regression, and outlier detection.SVM's try to fit the "widest possible street" between the classes. "Large Margin Classification". The instances of the training data located on the edge of the street are the "support vectors". It is a good idea to use feature scaling (`StandardScaler`) with SVM's. Linear SVM Classification
###Code
# Try out SVM on Iris dataset
import numpy as np
from sklearn import datasets
from sklearn.pipeline import Pipeline
from sklearn.preprocessing import StandardScaler
from sklearn.svm import LinearSVC
iris = datasets.load_iris()
X = iris["data"][:, (2,3)] #petal length, petal width
y = (iris["target"] == 2).astype(np.float64) #Iris-Virginica
# Small C value, wider street but more margin violations ("soft margin classification")
# Reduce C to avoid SVM overfitting.
svm_clf = Pipeline([
("scaler", StandardScaler()),
("linear_svc", LinearSVC(C=1, loss="hinge")),
])
svm_clf.fit(X, y)
svm_clf.predict([[5.5, 1.7]])
###Output
_____no_output_____
###Markdown
Outputs binary classification, not probabilities.For Larger datasets, a good choice is the `SGDClassifier` which uses Stochastic gradient descent to train a linear SVM. Non-linear SVM ClassificationA trick for nonlinearly separable data is to add a polynomial feature. Use the `PolynomialFeatures` transformer.
###Code
from sklearn.datasets import make_moons
from sklearn.pipeline import Pipeline
from sklearn.preprocessing import PolynomialFeatures
%matplotlib inline
import matplotlib
import matplotlib.pyplot as plt
# Lets see what the Moons dataset looks like.
X,y = make_moons(n_samples=100, noise=0.15, random_state=42)
def plot_dataset(X,y,axes):
plt.plot(X[:, 0][y==0], X[:, 1][y==0], "bs")
plt.plot(X[:, 0][y==1], X[:, 1][y==1], "g^")
plt.axis(axes)
plt.grid(True, which='both')
plt.xlabel(r"$x_1$", fontsize=20)
plt.ylabel(r"$x_2$", fontsize=20, rotation=0)
plot_dataset(X, y, [-1.5, 2.5, -1, 1.5])
plt.show()
polynomial_svm_clf = Pipeline([
("poly_features", PolynomialFeatures(degree=3)),
("scaler", StandardScaler()),
("svm_clf", LinearSVC(C=10, loss="hinge"))
])
polynomial_svm_clf.fit(X,y)
#Lets see how it did.
def plot_predictions(clf, axes):
x0s = np.linspace(axes[0], axes[1], 100)
x1s = np.linspace(axes[2], axes[3], 100)
x0, x1 = np.meshgrid(x0s, x1s)
X = np.c_[x0.ravel(), x1.ravel()]
y_pred = clf.predict(X).reshape(x0.shape)
y_decision = clf.decision_function(X).reshape(x0.shape)
plt.contourf(x0, x1, y_pred, cmap=plt.cm.brg, alpha=0.2)
plt.contourf(x0, x1, y_decision, cmap=plt.cm.brg, alpha=0.1)
plot_predictions(polynomial_svm_clf, [-1.5, 2.5, -1, 1.5])
plot_dataset(X, y, [-1.5, 2.5, -1, 1.5])
plt.show()
X
y
X.shape, y.shape
###Output
_____no_output_____ |
thomson-reuters-poly-reg/thomson-reuters-poly-reg.ipynb | ###Markdown
Polynomial Regression on Stock data (Thomson Reuters)In this project, a time series data is taken as input and a polynomial regression is executed on the dataset to predict the future stock prices.
###Code
%matplotlib inline
%config InlineBackend.figure_format = 'retina'
import numpy as np
import pandas as pd
import matplotlib.pyplot as mp
###Output
_____no_output_____
###Markdown
Load and prepare the dataThis project works with a small dataset saved into a `.csv` file. Let us load that up and perform required pre-processing if any.
###Code
data_path = 'tri.csv'
data = pd.read_csv(data_path)
data.head()
data = data.sort_index(ascending=False)
data.plot(x='Date', y='Close')
data = data.sort_index()
updated_data = data.drop(['Open', 'High', 'Low', 'Volume', 'Date'], axis=1)
updated_data['Serial'] = range(len(updated_data) - 1, -1, -1)
updated_data.index = updated_data['Serial']
updated_data = updated_data.drop(['Serial'], axis=1)
###Output
_____no_output_____
###Markdown
Polynomial Regression with up to 16 degreesGenerally, the more coefficients you take, in other words, the higher the degrees, the more accurate the line fits. With 16 degrees predicted values are rather optimistic as it ends with a peak.
###Code
coeffs = np.polyfit(updated_data.index, updated_data['Close'], 16)
x_p = np.linspace(0, 260, 500)
y_p = np.polyval(coeffs, x_p)
mp.plot(updated_data.index, updated_data['Close'])
mp.plot(x_p, y_p)
mp.legend(['Actual', 'Regression'])
mp.ylim(38, 48)
###Output
_____no_output_____
###Markdown
Polynomial Regression with above 16 degreesInterestingly, the moment we increase coefficients beyond 16, for this particular dataset, it ends with a sharp decline. Therefore, it is not a predictable strategy.
###Code
coeffs = np.polyfit(updated_data.index, updated_data['Close'], 17)
x_p = np.linspace(0, 260, 500)
y_p = np.polyval(coeffs, x_p)
mp.plot(updated_data.index, updated_data['Close'])
mp.plot(x_p, y_p)
mp.legend(['Actual', 'Regression'])
mp.ylim(38, 48)
###Output
_____no_output_____ |
Dataset files and corpus creation.ipynb | ###Markdown
Creating differnet datasets using the raw data.
###Code
%load_ext autoreload
%autoreload 2
import pandas as pd
from pathlib import Path
from scripts.parser_utils import file_parser,write_repeat_files,write_weighted_files,write_max_files,create_repeat_data,\
create_weighted_data,create_max_data,create_max_weighted_and_repeated_tsv,normalize,create_corpus
cur_dir = Path.cwd()
data_dir = cur_dir / "data"
raw_data_dir = data_dir / "raw_data"
proc_data_dir = data_dir / "processed_data"
sample_file = raw_data_dir / "sample.tsv"
train_file = proc_data_dir / "train_clean.tsv"
dev_file = raw_data_dir / "te.translit.sampled.dev.tsv"
test_file = raw_data_dir / "te.translit.sampled.test.tsv"
weighted_sample_file = proc_data_dir / "weighted_sample.tsv"
max_sample_file = proc_data_dir / "max_sample.tsv"
repeat_sample_file = proc_data_dir / "repeat_sample.tsv"
weighted_dev_file = proc_data_dir / "weighted_dev.tsv"
max_dev_file = proc_data_dir / "max_dev.tsv"
repeat_dev_file = proc_data_dir / "repeat_dev.tsv"
weighted_train_file = proc_data_dir / "weighted_train.tsv"
max_train_file = proc_data_dir / "max_train.tsv"
repeat_train_file = proc_data_dir / "repeat_train.tsv"
weighted_test_file = proc_data_dir / "weighted_test.tsv"
max_test_file = proc_data_dir / "max_test.tsv"
repeat_test_file = proc_data_dir / "repeat_test.tsv"
tgt_corpus_file = proc_data_dir / "target_corpus.txt"
src_corpus_file = proc_data_dir / "source_corpus.txt"
df_sample= pd.read_csv(sample_file, sep='\t',header = None , names=["target","source", "frequency"])
df_sample.head()
with open(sample_file, 'r', encoding='utf-8') as f:
for line in f:
print (line.strip().split("\t"))
sample_dict = file_parser(sample_file)
sample_dict
normalize((1,3))
weighted_sample_data = create_weighted_data(sample_dict)
weighted_sample_data
max_sample_data = create_max_data(sample_dict)
max_sample_data
repeat_sample_data = create_repeat_data(sample_dict)
repeat_sample_data
%%time
create_max_weighted_and_repeated_tsv(sample_file,max_sample_file,weighted_sample_file, repeat_sample_file)
%%time
create_max_weighted_and_repeated_tsv(dev_file,max_dev_file,weighted_dev_file, repeat_dev_file)
create_max_weighted_and_repeated_tsv(train_file,max_train_file,weighted_train_file, repeat_train_file)
create_max_weighted_and_repeated_tsv(test_file,max_test_file,weighted_test_file, repeat_test_file)
df_weighted_train= pd.read_csv(weighted_train_file, sep='\t',header = None , names=["target","source", "weights"])
df_weighted_train.head()
df_repeat_train= pd.read_csv(repeat_train_file, sep='\t',header = None , names=["target","source"])
df_repeat_train.head()
df_max_train= pd.read_csv(max_train_file, sep='\t',header = None , names=["target","source"])
df_max_train.head()
df_train= pd.read_csv(train_file, sep='\t',header = None , names=["target","source", "frequency"])
df_train.head()
create_corpus(train_file,src_corpus_file,tgt_corpus_file)
###Output
_____no_output_____ |
examples/notebooks/morph.ipynb | ###Markdown
import libraries
###Code
from compas_vol.combinations import Morph
from compas_vol.primitives import VolSphere, VolBox
from compas.geometry import Box, Frame, Point, Sphere
import numpy as np
import meshplot as mp
from skimage.measure import marching_cubes
from compas_vol.utilities import bbox_edges
###Output
_____no_output_____
###Markdown
create volumetric object (CSG tree)
###Code
s = Sphere(Point(5, 6, 0), 9)
b = Box(Frame.worldXY(), 20, 15, 10)
vs = VolSphere(s)
vb = VolBox(b, 2.5)
u = Morph(vs, vb, 0.5)
###Output
_____no_output_____
###Markdown
workspace (dense grid)
###Code
#workspace initialization
# lower and upper bounds
lbx, ubx = -16.0, 16.0
lby, uby = -16.0, 16.0
lbz, ubz = -16.0, 16.0
# resolution(s)
nx, ny, nz = 100, 100, 100
x, y, z = np.ogrid[lbx:ubx:nx*1j, lby:uby:ny*1j, lbz:ubz:nz*1j]
#voxel dimensions
gx = (ubx-lbx)/nx
gy = (uby-lby)/ny
gz = (ubz-lbz)/nz
###Output
_____no_output_____
###Markdown
sample at discrete interval (marching cube)
###Code
dm = u.get_distance_numpy(x, y, z)
###Output
_____no_output_____
###Markdown
generate isosurface (marching cube)
###Code
v, f, n, l = marching_cubes(dm, 0, spacing=(gx, gy, gz))
v += [lbx,lby,lbz]
###Output
_____no_output_____
###Markdown
display mesh
###Code
p = mp.plot(v, f, c=np.array([0,0.57,0.82]), shading={"flat":False, "roughness":0.4, "metalness":0.01, "reflectivity":1.0})
vs,ve = bbox_edges(lbx,ubx,lby,uby,lbz,ubz)
p.add_lines(np.array(vs), np.array(ve))
###Output
_____no_output_____ |
07_2_gradient_mat_mul.ipynb | ###Markdown
7-4. Gradient of matrix multiplication Matrix-Vector Addition
###Code
X = np.array([
[1, 2, 3]
])
X
b = np.array([10, 20, 30])
b
X + b
X2 = np.array([
[1, 2, 3],
[4, 5, 6],
[7, 8, 9],
[10, 11, 12]
])
X2
X2 + b
###Output
_____no_output_____
###Markdown
Matrix-Matrix Multiplication
###Code
X = np.array([
[1.0, 2.0, 3.0],
[4.0, 5.0, 6.0]
])
X
W = np.array([
[10.0, 20.0],
[30.0, 40.0],
[50.0, 60.0]
])
W
np.dot(X, W)
np.sum(np.dot(X, W))
###Output
_____no_output_____
###Markdown
Function taking two matrices
###Code
def f(X, W):
return np.sum(np.dot(X, W))
f(X, W)
###Output
_____no_output_____
###Markdown
Iteration over Numpy Array
###Code
copyX = np.zeros_like(X)
itr = np.nditer(X, flags=['multi_index'], op_flags=['writeonly'])
while not itr.finished:
# itr[0] = it.multi_index[1] - it.multi_index[0]
print(itr[0], itr.multi_index)
print("===before==")
print(X)
itr[0] += 1
copyX[itr.multi_index] = itr[0]
print("===after==")
print(X)
itr.iternext()
###Output
1.0 (0, 0)
===before==
[[ 1. 2. 3.]
[ 4. 5. 6.]]
===after==
[[ 2. 2. 3.]
[ 4. 5. 6.]]
2.0 (0, 1)
===before==
[[ 2. 2. 3.]
[ 4. 5. 6.]]
===after==
[[ 2. 3. 3.]
[ 4. 5. 6.]]
3.0 (0, 2)
===before==
[[ 2. 3. 3.]
[ 4. 5. 6.]]
===after==
[[ 2. 3. 4.]
[ 4. 5. 6.]]
4.0 (1, 0)
===before==
[[ 2. 3. 4.]
[ 4. 5. 6.]]
===after==
[[ 2. 3. 4.]
[ 5. 5. 6.]]
5.0 (1, 1)
===before==
[[ 2. 3. 4.]
[ 5. 5. 6.]]
===after==
[[ 2. 3. 4.]
[ 5. 6. 6.]]
6.0 (1, 2)
===before==
[[ 2. 3. 4.]
[ 5. 6. 6.]]
===after==
[[ 2. 3. 4.]
[ 5. 6. 7.]]
###Markdown
Numerical Gradient of Matrices
###Code
def numerical_gradient(X, W):
h = 1e-4
dX = np.zeros_like(X)
dW = np.zeros_like(W)
itr = np.nditer(X, flags=['multi_index'], op_flags=['readwrite'])
while not itr.finished:
original = itr[0].copy()
itr[0] = original + h
v1 = f(X, W)
itr[0] = original - h
v2 = f(X, W)
dX[itr.multi_index] = (v1 - v2) / (2 * h)
X[itr.multi_index] = original
itr.iternext()
itr = np.nditer(W, flags=['multi_index'], op_flags=['readwrite'])
while not itr.finished:
original = itr[0].copy()
itr[0] = original + h
v1 = f(X, W)
itr[0] = original - h
v2 = f(X, W)
dW[itr.multi_index] = (v1 - v2) / (2 * h)
itr[0] = original
itr.iternext()
return dX, dW
###Output
_____no_output_____
###Markdown
Gradient of Matrix Multiplication
###Code
dX, dW = numerical_gradient(X, W)
print('dX')
print(dX)
print('dW')
print(dW)
X = np.array([
[1.0, 2.0, 3.0],
[4.0, 5.0, 6.0]
])
W = np.array([
[10.0, 9.0],
[8.0, 7.0],
[6.0, 5.0]
])
f(X, W)
# np.dot(X, W)
X2 = np.array([
[1.0, 2.0, 3.0],
[4.0, 5.0, 6.0]
])
W2 = np.array([
[10.0, 9.0],
[8.0, 7.0],
[6.0, 5.0]
])
f(X2, W2)
np.dot(np.ones((2,2)), W.T)
np.dot(X.T, np.ones((2,2)))
###Output
_____no_output_____ |
leo/LGD_Phase1_Stage1_Autoencoder.ipynb | ###Markdown
VAE Model
###Code
# parameters
laten_dim = 32
ker_si = 2
filters = 32
loss_fn = 'mse'
# reparameterization trick
# instead of sampling from Q(z|X), sample eps = N(0,I)
# then z = z_mean + sqrt(var)*eps
def sampling(args):
"""Reparameterization trick by sampling fr an isotropic unit Gaussian.
# Arguments:
args (tensor): mean and log of variance of Q(z|X)
# Returns:
z (tensor): sampled latent vector
"""
z_mean, z_log_var = args
batch = K.shape(z_mean)[0]
dim = K.int_shape(z_mean)[1]
# by default, random_normal has mean=0 and std=1.0
epsilon = K.random_normal(shape=(batch, dim))
return z_mean + K.exp(0.5 * z_log_var) * epsilon
input_img = Input(shape=(X_train.shape[1],X_train.shape[2],1))
x = Conv2D(filters,kernel_size=ker_si,padding='same')(input_img)
# x = Conv2D(filters*2,(2,2),padding='same',activation='relu')(x)
# x = Conv2D(filters*4,(2,2),padding='same',activation='relu')(x)
shape = K.int_shape(x)
x = Flatten()(x)
x = Dense(laten_dim*32,activation='selu')(x)
z_mean = Dense(laten_dim,name='z_mean')(x)
z_log_var = Dense(laten_dim,name='z_log_var')(x)
z = Lambda(sampling, output_shape=(laten_dim,),name='z')([z_mean,z_log_var])
encoder = Model(input_img,[z_mean,z_log_var,z],name='encoder')
encoder.summary()
latent_inputs = Input(shape=(laten_dim,), name='z_sampling')
x = Dense(shape[1] * shape[2] * shape[3], activation='selu')(latent_inputs)
x = Reshape((shape[1], shape[2], shape[3]))(x)
# x = Conv2DTranspose(filters=filters*4,kernel_size=ker_si,activation='relu',padding='same')(x)
# x = Conv2DTranspose(filters=filters*2,kernel_size=ker_si,activation='relu',padding='same')(x)
x = Conv2DTranspose(filters=filters,kernel_size=ker_si,activation='selu',padding='same')(x)
outputs = Conv2DTranspose(filters=1,kernel_size=ker_si,activation='sigmoid',padding='same',name='decoder_output')(x)
decoder = Model(latent_inputs,outputs,name='decoder')
decoder.summary()
outputs = decoder(encoder(input_img)[2])
vae = Model(input_img,outputs,name='vae')
if loss_fn == 'mse':
reconstruction_loss = mse(K.flatten(input_img), K.flatten(outputs))
else:
reconstruction_loss = binary_crossentropy(K.flatten(input_img),K.flatten(outputs))
# reconstruction_loss *= X_train.shape[1] * X_train.shape[2]
kl_loss = 1 + z_log_var - K.square(z_mean) - K.exp(z_log_var)
kl_loss = K.sum(kl_loss, axis=-1)
kl_loss *= -0.5
vae_loss = K.mean(reconstruction_loss + kl_loss)
vae.add_loss(vae_loss)
vae.compile(optimizer=Nadam())
vae.summary()
vae.fit(X_train,epochs=50 ,shuffle=True,batch_size=64)
# vae.fit_generator(batch_generator(X_train,X_train,2),samples_per_epoch=len(X_train)//2)
def generate_batch_data_random(x, y, batch_size):
"""逐步提取batch数据到显存,降低对显存的占用"""
ylen = len(y)
loopcount = ylen // batch_size
while (True):
i = random.randint(0,loopcount)
yield x[i * batch_size:(i + 1) * batch_size]
def batch_generator(X_gen,Y_gen,batch_size):
while True:
yield(X_gen[batch_size],Y_gen[batch_size])
###Output
_____no_output_____
###Markdown
CNN Autoencoder Model
###Code
input_img = Input(shape=(X_train.shape[1],X_train.shape[2],1))
x = Conv2D(64,(4,7),activation='selu',padding='same')(input_img)
x = Conv2D(32,(4,7),activation='selu',padding='same')(x)
x = Conv2D(1,(4,7),activation='selu',padding='same')(x)
shape3 = K.int_shape(x)
# x = MaxPool2D((4,11))(x)
# x = Dropout(0.25)(x)
shape = K.int_shape(x)
x = Flatten()(x)
encoder = Dense(int(shape[1]*shape[2]*shape[3]),activation='selu')(x)
# x = Dense(int(shape[1]*shape[2]*shape[3]/5),activation='relu')(x)
# x = Dropout(0.25)(x)
# encoder = Dense(shape[1]*shape[2],activation='relu')(x)
# x = Dense(int(shape[1]*shape[2]*shape[3]/5),activation='relu')(encoder)
# x = Dropout(0.25)(x)
# x = Dense(int(shape[1]*shape[2]*shape[3]),activation='relu')(x)
# x = Dropout(0.25)(x)
# x = Reshape((shape[1],shape[2],shape[3]))(x)
x = Reshape((shape[1],shape[2],shape[3]))(encoder)
# decoder = UpSampling2D((4,11))(x)
decoder = Conv2DTranspose(64,(4,7),padding='same',activation='selu')(x)
decoder = Conv2DTranspose(1,(4,7),padding='same',activation='linear')(decoder)
shape2 = K.int_shape(decoder)
autoencoder = Model(input_img,decoder)
autoencoder.summary()
X_train
autoencoder.compile(optimizer='adadelta', loss='binary_crossentropy')
autoencoder.fit(X_train,X_train)
reduce_lr = ReduceLROnPlateau(monitor='loss', factor=0.5, patience=10, min_lr=1e-4)
# autoencoder.compile(optimizer=Nadam(), loss='mse')
autoencoder.compile(optimizer=Adam(lr=0.002), loss='mse')
autoencoder.fit(X_train,X_train, epochs=500,shuffle=True,batch_size=256,callbacks=[reduce_lr])
reduce_lr = ReduceLROnPlateau(monitor='loss', factor=0.5, patience=5, min_lr=2e-4)
autoencoder.compile(optimizer=Adamax(decay=1e-6), loss='mse')
autoencoder.fit(X_train,X_train, epochs=500,shuffle=True,batch_size=256,callbacks=[reduce_lr])
autoencoder.save('model/lgd_dense_AE3.h5')
# encoder.save('model/lgd_dense_enc.h5')
###Output
_____no_output_____
###Markdown
Label Spreading
###Code
# map_path = path of 'map.pkl'
map_path = 'data/map.pkl'#sys.argv[1]
# base_path = directory of all the data (train_label.csv, X_train.npy)
base_path = 'feature/fbank2/'#sys.argv[2]
with open(map_path, 'rb') as f:
map_dict = pickle.load(f)
verified = []
unverified = []
train_label_path = os.path.join(base_path, 'train_label.csv')
Y_train = pd.read_csv(train_label_path)
for i in range(len(Y_train)):
if Y_train['manually_verified'][i] == 1:
verified.append(i)
else:
unverified.append(i)
Y_un = Y_train.loc[unverified,:]
fname_all = Y_un['fname']
fname_all = np.array(fname_all)
Y_dict = Y_un['label'].map(map_dict)
Y_dict = np.array(Y_dict)
Y_all = []
for i in Y_dict:
Y_all.append(to_categorical(i, num_classes=41))
Y_all = np.array(Y_all)
filename = os.path.join(base_path, 'fname_unverified.npy')
np.save(filename, fname_all)
filename = os.path.join(base_path, 'y_unverified.npy')
np.save(filename, Y_all)
X_train_path = os.path.join(base_path, 'X_train.npy')
X_all = np.load(X_train_path)
X_un = X_all[unverified, :]
filename = os.path.join(base_path, 'X_unverified.npy')
np.save(filename, X_un)
X_ver = X_all[verified, :]
train_label_path = os.path.join(base_path, 'train_label.csv')
Y_ver = pd.read_csv(train_label_path)
Y_ver = Y_ver.loc[verified,:]
'''
exit()
X_all = X_all[verified, :]
idx = list(range(X_all.shape[0]))
random.shuffle(idx)
xSize = math.ceil(X_all.shape[0] / num_fold)
split_X_path = os.path.join(base_path, 'X')
split_y_path = os.path.join(base_path, 'y')
if not os.path.exists(split_X_path):
os.makedirs(split_X_path)
if not os.path.exists(split_y_path):
os.makedirs(split_y_path)
for i in range(num_fold):
X = X_all[idx[i*xSize:i*xSize+xSize]]
y = Y_all[idx[i*xSize:i*xSize+xSize]]
print('Saving fold {}'.format(i+1))
print('X.shape:', X.shape)
print('y.shape:', y.shape)
filename = os.path.join(split_X_path, 'X' + str(i+1) + '.npy')
np.save(filename, X)
filename = os.path.join(split_y_path, 'y' + str(i+1) + '.npy')
np.save(filename, y)
time.sleep(1)
'''
X_un.shape , X_ver.shape
Y_ver = Y_ver['label'].map(map_dict)
Y_ver = np.array(Y_ver)
Y_un = Y_un['label'].map(map_dict)
Y_un = np.array(Y_un)
Y_un.shape , Y_ver.shape
# enc =
autoencoder.summary()
model = Model(input = autoencoder.layers[0].input, output = autoencoder.layers[5].output)
model.summary()
X_ver_dense = model.predict(X_ver)
X_un_dense = model.predict(X_un)
print(X_ver.shape , X_un.shape)
X_test = np.load('feature/fbank2/X_test.npy')
X_test_dense = model.predict(X_test)
print(X_ver.shape, len(Y_ver))
X_test_dense
X_test_dense.shape
lp_model = LabelSpreading(kernel='knn',n_jobs=-1)#,n_neighbors=3,alpha=0.05)#, max_iter=50, gamma=10, alpha=0.1,tol=0.0001)
lp_model.fit(X_ver_dense,Y_ver)
un_ans = lp_model.predict(X_un_dense)
test_ans = lp_model.predict(X_test_dense)
un_ans, test_ans
###Output
_____no_output_____
###Markdown
Save semi data and re-label
###Code
print(X_un_dense.shape)
np.save('feature/fbank2/semi/X_un_dense.npy',X_un_dense)
np.save('feature/fbank2/semi/Y_un_dense.npy',un_ans)
np.save('feature/fbank2/semi/X_test_dense.npy',X_test_dense)
np.save('feature/fbank2/semi/Y_test_dense.npy',test_ans)
###Output
_____no_output_____ |
experiments/baseline_ptn/oracle.run1/trials/2/trial.ipynb | ###Markdown
PTN TemplateThis notebook serves as a template for single dataset PTN experiments It can be run on its own by setting STANDALONE to True (do a find for "STANDALONE" to see where) But it is intended to be executed as part of a *papermill.py script. See any of the experimentes with a papermill script to get started with that workflow.
###Code
%load_ext autoreload
%autoreload 2
%matplotlib inline
import os, json, sys, time, random
import numpy as np
import torch
from torch.optim import Adam
from easydict import EasyDict
import matplotlib.pyplot as plt
from steves_models.steves_ptn import Steves_Prototypical_Network
from steves_utils.lazy_iterable_wrapper import Lazy_Iterable_Wrapper
from steves_utils.iterable_aggregator import Iterable_Aggregator
from steves_utils.ptn_train_eval_test_jig import PTN_Train_Eval_Test_Jig
from steves_utils.torch_sequential_builder import build_sequential
from steves_utils.torch_utils import get_dataset_metrics, ptn_confusion_by_domain_over_dataloader
from steves_utils.utils_v2 import (per_domain_accuracy_from_confusion, get_datasets_base_path)
from steves_utils.PTN.utils import independent_accuracy_assesment
from steves_utils.stratified_dataset.episodic_accessor import Episodic_Accessor_Factory
from steves_utils.ptn_do_report import (
get_loss_curve,
get_results_table,
get_parameters_table,
get_domain_accuracies,
)
from steves_utils.transforms import get_chained_transform
###Output
_____no_output_____
###Markdown
Required ParametersThese are allowed parameters, not defaultsEach of these values need to be present in the injected parameters (the notebook will raise an exception if they are not present)Papermill uses the cell tag "parameters" to inject the real parameters below this cell.Enable tags to see what I mean
###Code
required_parameters = {
"experiment_name",
"lr",
"device",
"seed",
"dataset_seed",
"labels_source",
"labels_target",
"domains_source",
"domains_target",
"num_examples_per_domain_per_label_source",
"num_examples_per_domain_per_label_target",
"n_shot",
"n_way",
"n_query",
"train_k_factor",
"val_k_factor",
"test_k_factor",
"n_epoch",
"patience",
"criteria_for_best",
"x_transforms_source",
"x_transforms_target",
"episode_transforms_source",
"episode_transforms_target",
"pickle_name",
"x_net",
"NUM_LOGS_PER_EPOCH",
"BEST_MODEL_PATH",
"torch_default_dtype"
}
standalone_parameters = {}
standalone_parameters["experiment_name"] = "STANDALONE PTN"
standalone_parameters["lr"] = 0.0001
standalone_parameters["device"] = "cuda"
standalone_parameters["seed"] = 1337
standalone_parameters["dataset_seed"] = 1337
standalone_parameters["num_examples_per_domain_per_label_source"]=100
standalone_parameters["num_examples_per_domain_per_label_target"]=100
standalone_parameters["n_shot"] = 3
standalone_parameters["n_query"] = 2
standalone_parameters["train_k_factor"] = 1
standalone_parameters["val_k_factor"] = 2
standalone_parameters["test_k_factor"] = 2
standalone_parameters["n_epoch"] = 100
standalone_parameters["patience"] = 10
standalone_parameters["criteria_for_best"] = "target_accuracy"
standalone_parameters["x_transforms_source"] = ["unit_power"]
standalone_parameters["x_transforms_target"] = ["unit_power"]
standalone_parameters["episode_transforms_source"] = []
standalone_parameters["episode_transforms_target"] = []
standalone_parameters["torch_default_dtype"] = "torch.float32"
standalone_parameters["x_net"] = [
{"class": "nnReshape", "kargs": {"shape":[-1, 1, 2, 256]}},
{"class": "Conv2d", "kargs": { "in_channels":1, "out_channels":256, "kernel_size":(1,7), "bias":False, "padding":(0,3), },},
{"class": "ReLU", "kargs": {"inplace": True}},
{"class": "BatchNorm2d", "kargs": {"num_features":256}},
{"class": "Conv2d", "kargs": { "in_channels":256, "out_channels":80, "kernel_size":(2,7), "bias":True, "padding":(0,3), },},
{"class": "ReLU", "kargs": {"inplace": True}},
{"class": "BatchNorm2d", "kargs": {"num_features":80}},
{"class": "Flatten", "kargs": {}},
{"class": "Linear", "kargs": {"in_features": 80*256, "out_features": 256}}, # 80 units per IQ pair
{"class": "ReLU", "kargs": {"inplace": True}},
{"class": "BatchNorm1d", "kargs": {"num_features":256}},
{"class": "Linear", "kargs": {"in_features": 256, "out_features": 256}},
]
# Parameters relevant to results
# These parameters will basically never need to change
standalone_parameters["NUM_LOGS_PER_EPOCH"] = 10
standalone_parameters["BEST_MODEL_PATH"] = "./best_model.pth"
# uncomment for CORES dataset
from steves_utils.CORES.utils import (
ALL_NODES,
ALL_NODES_MINIMUM_1000_EXAMPLES,
ALL_DAYS
)
standalone_parameters["labels_source"] = ALL_NODES
standalone_parameters["labels_target"] = ALL_NODES
standalone_parameters["domains_source"] = [1]
standalone_parameters["domains_target"] = [2,3,4,5]
standalone_parameters["pickle_name"] = "cores.stratified_ds.2022A.pkl"
# Uncomment these for ORACLE dataset
# from steves_utils.ORACLE.utils_v2 import (
# ALL_DISTANCES_FEET,
# ALL_RUNS,
# ALL_SERIAL_NUMBERS,
# )
# standalone_parameters["labels_source"] = ALL_SERIAL_NUMBERS
# standalone_parameters["labels_target"] = ALL_SERIAL_NUMBERS
# standalone_parameters["domains_source"] = [8,20, 38,50]
# standalone_parameters["domains_target"] = [14, 26, 32, 44, 56]
# standalone_parameters["pickle_name"] = "oracle.frame_indexed.stratified_ds.2022A.pkl"
# standalone_parameters["num_examples_per_domain_per_label_source"]=1000
# standalone_parameters["num_examples_per_domain_per_label_target"]=1000
# Uncomment these for Metahan dataset
# standalone_parameters["labels_source"] = list(range(19))
# standalone_parameters["labels_target"] = list(range(19))
# standalone_parameters["domains_source"] = [0]
# standalone_parameters["domains_target"] = [1]
# standalone_parameters["pickle_name"] = "metehan.stratified_ds.2022A.pkl"
# standalone_parameters["n_way"] = len(standalone_parameters["labels_source"])
# standalone_parameters["num_examples_per_domain_per_label_source"]=200
# standalone_parameters["num_examples_per_domain_per_label_target"]=100
standalone_parameters["n_way"] = len(standalone_parameters["labels_source"])
# Parameters
parameters = {
"experiment_name": "baseline_ptn_oracle.run1",
"lr": 0.001,
"device": "cuda",
"seed": 1337,
"dataset_seed": 1337,
"labels_source": [
"3123D52",
"3123D65",
"3123D79",
"3123D80",
"3123D54",
"3123D70",
"3123D7B",
"3123D89",
"3123D58",
"3123D76",
"3123D7D",
"3123EFE",
"3123D64",
"3123D78",
"3123D7E",
"3124E4A",
],
"labels_target": [
"3123D52",
"3123D65",
"3123D79",
"3123D80",
"3123D54",
"3123D70",
"3123D7B",
"3123D89",
"3123D58",
"3123D76",
"3123D7D",
"3123EFE",
"3123D64",
"3123D78",
"3123D7E",
"3124E4A",
],
"x_transforms_source": [],
"x_transforms_target": [],
"episode_transforms_source": [],
"episode_transforms_target": [],
"num_examples_per_domain_per_label_source": 1000,
"num_examples_per_domain_per_label_target": 1000,
"n_shot": 3,
"n_way": 16,
"n_query": 2,
"train_k_factor": 1,
"val_k_factor": 2,
"test_k_factor": 2,
"torch_default_dtype": "torch.float64",
"n_epoch": 50,
"patience": 3,
"criteria_for_best": "target_loss",
"x_net": [
{"class": "nnReshape", "kargs": {"shape": [-1, 1, 2, 256]}},
{
"class": "Conv2d",
"kargs": {
"in_channels": 1,
"out_channels": 256,
"kernel_size": [1, 7],
"bias": False,
"padding": [0, 3],
},
},
{"class": "ReLU", "kargs": {"inplace": True}},
{"class": "BatchNorm2d", "kargs": {"num_features": 256}},
{
"class": "Conv2d",
"kargs": {
"in_channels": 256,
"out_channels": 80,
"kernel_size": [2, 7],
"bias": True,
"padding": [0, 3],
},
},
{"class": "ReLU", "kargs": {"inplace": True}},
{"class": "BatchNorm2d", "kargs": {"num_features": 80}},
{"class": "Flatten", "kargs": {}},
{"class": "Linear", "kargs": {"in_features": 20480, "out_features": 256}},
{"class": "ReLU", "kargs": {"inplace": True}},
{"class": "BatchNorm1d", "kargs": {"num_features": 256}},
{"class": "Linear", "kargs": {"in_features": 256, "out_features": 256}},
],
"NUM_LOGS_PER_EPOCH": 10,
"BEST_MODEL_PATH": "./best_model.pth",
"pickle_name": "oracle.Run1_10kExamples_stratified_ds.2022A.pkl",
"domains_source": [8, 32, 50],
"domains_target": [14, 20, 26, 38, 44],
}
# Set this to True if you want to run this template directly
STANDALONE = False
if STANDALONE:
print("parameters not injected, running with standalone_parameters")
parameters = standalone_parameters
if not 'parameters' in locals() and not 'parameters' in globals():
raise Exception("Parameter injection failed")
#Use an easy dict for all the parameters
p = EasyDict(parameters)
supplied_keys = set(p.keys())
if supplied_keys != required_parameters:
print("Parameters are incorrect")
if len(supplied_keys - required_parameters)>0: print("Shouldn't have:", str(supplied_keys - required_parameters))
if len(required_parameters - supplied_keys)>0: print("Need to have:", str(required_parameters - supplied_keys))
raise RuntimeError("Parameters are incorrect")
###################################
# Set the RNGs and make it all deterministic
###################################
np.random.seed(p.seed)
random.seed(p.seed)
torch.manual_seed(p.seed)
torch.use_deterministic_algorithms(True)
###########################################
# The stratified datasets honor this
###########################################
torch.set_default_dtype(eval(p.torch_default_dtype))
###################################
# Build the network(s)
# Note: It's critical to do this AFTER setting the RNG
# (This is due to the randomized initial weights)
###################################
x_net = build_sequential(p.x_net)
start_time_secs = time.time()
###################################
# Build the dataset
###################################
if p.x_transforms_source == []: x_transform_source = None
else: x_transform_source = get_chained_transform(p.x_transforms_source)
if p.x_transforms_target == []: x_transform_target = None
else: x_transform_target = get_chained_transform(p.x_transforms_target)
if p.episode_transforms_source == []: episode_transform_source = None
else: raise Exception("episode_transform_source not implemented")
if p.episode_transforms_target == []: episode_transform_target = None
else: raise Exception("episode_transform_target not implemented")
eaf_source = Episodic_Accessor_Factory(
labels=p.labels_source,
domains=p.domains_source,
num_examples_per_domain_per_label=p.num_examples_per_domain_per_label_source,
iterator_seed=p.seed,
dataset_seed=p.dataset_seed,
n_shot=p.n_shot,
n_way=p.n_way,
n_query=p.n_query,
train_val_test_k_factors=(p.train_k_factor,p.val_k_factor,p.test_k_factor),
pickle_path=os.path.join(get_datasets_base_path(), p.pickle_name),
x_transform_func=x_transform_source,
example_transform_func=episode_transform_source,
)
train_original_source, val_original_source, test_original_source = eaf_source.get_train(), eaf_source.get_val(), eaf_source.get_test()
eaf_target = Episodic_Accessor_Factory(
labels=p.labels_target,
domains=p.domains_target,
num_examples_per_domain_per_label=p.num_examples_per_domain_per_label_target,
iterator_seed=p.seed,
dataset_seed=p.dataset_seed,
n_shot=p.n_shot,
n_way=p.n_way,
n_query=p.n_query,
train_val_test_k_factors=(p.train_k_factor,p.val_k_factor,p.test_k_factor),
pickle_path=os.path.join(get_datasets_base_path(), p.pickle_name),
x_transform_func=x_transform_target,
example_transform_func=episode_transform_target,
)
train_original_target, val_original_target, test_original_target = eaf_target.get_train(), eaf_target.get_val(), eaf_target.get_test()
transform_lambda = lambda ex: ex[1] # Original is (<domain>, <episode>) so we strip down to episode only
train_processed_source = Lazy_Iterable_Wrapper(train_original_source, transform_lambda)
val_processed_source = Lazy_Iterable_Wrapper(val_original_source, transform_lambda)
test_processed_source = Lazy_Iterable_Wrapper(test_original_source, transform_lambda)
train_processed_target = Lazy_Iterable_Wrapper(train_original_target, transform_lambda)
val_processed_target = Lazy_Iterable_Wrapper(val_original_target, transform_lambda)
test_processed_target = Lazy_Iterable_Wrapper(test_original_target, transform_lambda)
datasets = EasyDict({
"source": {
"original": {"train":train_original_source, "val":val_original_source, "test":test_original_source},
"processed": {"train":train_processed_source, "val":val_processed_source, "test":test_processed_source}
},
"target": {
"original": {"train":train_original_target, "val":val_original_target, "test":test_original_target},
"processed": {"train":train_processed_target, "val":val_processed_target, "test":test_processed_target}
},
})
# Some quick unit tests on the data
from steves_utils.transforms import get_average_power, get_average_magnitude
q_x, q_y, s_x, s_y, truth = next(iter(train_processed_source))
assert q_x.dtype == eval(p.torch_default_dtype)
assert s_x.dtype == eval(p.torch_default_dtype)
print("Visually inspect these to see if they line up with expected values given the transforms")
print('x_transforms_source', p.x_transforms_source)
print('x_transforms_target', p.x_transforms_target)
print("Average magnitude, source:", get_average_magnitude(q_x[0].numpy()))
print("Average power, source:", get_average_power(q_x[0].numpy()))
q_x, q_y, s_x, s_y, truth = next(iter(train_processed_target))
print("Average magnitude, target:", get_average_magnitude(q_x[0].numpy()))
print("Average power, target:", get_average_power(q_x[0].numpy()))
###################################
# Build the model
###################################
model = Steves_Prototypical_Network(x_net, device=p.device, x_shape=(2,256))
optimizer = Adam(params=model.parameters(), lr=p.lr)
###################################
# train
###################################
jig = PTN_Train_Eval_Test_Jig(model, p.BEST_MODEL_PATH, p.device)
jig.train(
train_iterable=datasets.source.processed.train,
source_val_iterable=datasets.source.processed.val,
target_val_iterable=datasets.target.processed.val,
num_epochs=p.n_epoch,
num_logs_per_epoch=p.NUM_LOGS_PER_EPOCH,
patience=p.patience,
optimizer=optimizer,
criteria_for_best=p.criteria_for_best,
)
total_experiment_time_secs = time.time() - start_time_secs
###################################
# Evaluate the model
###################################
source_test_label_accuracy, source_test_label_loss = jig.test(datasets.source.processed.test)
target_test_label_accuracy, target_test_label_loss = jig.test(datasets.target.processed.test)
source_val_label_accuracy, source_val_label_loss = jig.test(datasets.source.processed.val)
target_val_label_accuracy, target_val_label_loss = jig.test(datasets.target.processed.val)
history = jig.get_history()
total_epochs_trained = len(history["epoch_indices"])
val_dl = Iterable_Aggregator((datasets.source.original.val,datasets.target.original.val))
confusion = ptn_confusion_by_domain_over_dataloader(model, p.device, val_dl)
per_domain_accuracy = per_domain_accuracy_from_confusion(confusion)
# Add a key to per_domain_accuracy for if it was a source domain
for domain, accuracy in per_domain_accuracy.items():
per_domain_accuracy[domain] = {
"accuracy": accuracy,
"source?": domain in p.domains_source
}
# Do an independent accuracy assesment JUST TO BE SURE!
# _source_test_label_accuracy = independent_accuracy_assesment(model, datasets.source.processed.test, p.device)
# _target_test_label_accuracy = independent_accuracy_assesment(model, datasets.target.processed.test, p.device)
# _source_val_label_accuracy = independent_accuracy_assesment(model, datasets.source.processed.val, p.device)
# _target_val_label_accuracy = independent_accuracy_assesment(model, datasets.target.processed.val, p.device)
# assert(_source_test_label_accuracy == source_test_label_accuracy)
# assert(_target_test_label_accuracy == target_test_label_accuracy)
# assert(_source_val_label_accuracy == source_val_label_accuracy)
# assert(_target_val_label_accuracy == target_val_label_accuracy)
experiment = {
"experiment_name": p.experiment_name,
"parameters": dict(p),
"results": {
"source_test_label_accuracy": source_test_label_accuracy,
"source_test_label_loss": source_test_label_loss,
"target_test_label_accuracy": target_test_label_accuracy,
"target_test_label_loss": target_test_label_loss,
"source_val_label_accuracy": source_val_label_accuracy,
"source_val_label_loss": source_val_label_loss,
"target_val_label_accuracy": target_val_label_accuracy,
"target_val_label_loss": target_val_label_loss,
"total_epochs_trained": total_epochs_trained,
"total_experiment_time_secs": total_experiment_time_secs,
"confusion": confusion,
"per_domain_accuracy": per_domain_accuracy,
},
"history": history,
"dataset_metrics": get_dataset_metrics(datasets, "ptn"),
}
ax = get_loss_curve(experiment)
plt.show()
get_results_table(experiment)
get_domain_accuracies(experiment)
print("Source Test Label Accuracy:", experiment["results"]["source_test_label_accuracy"], "Target Test Label Accuracy:", experiment["results"]["target_test_label_accuracy"])
print("Source Val Label Accuracy:", experiment["results"]["source_val_label_accuracy"], "Target Val Label Accuracy:", experiment["results"]["target_val_label_accuracy"])
json.dumps(experiment)
###Output
_____no_output_____ |
Keiandra_King_3_assignment_regression_classification_3.ipynb | ###Markdown
Lambda School Data Science*Unit 2, Sprint 1, Module 3*--- Ridge Regression AssignmentWe're going back to our other **New York City** real estate dataset. Instead of predicting apartment rents, you'll predict property sales prices.But not just for condos in Tribeca...Instead, predict property sales prices for **One Family Dwellings** (`BUILDING_CLASS_CATEGORY` == `'01 ONE FAMILY DWELLINGS'`). Use a subset of the data where the **sale price was more than \\$100 thousand and less than $2 million.** The [NYC Department of Finance](https://www1.nyc.gov/site/finance/taxes/property-rolling-sales-data.page) has a glossary of property sales terms and NYC Building Class Code Descriptions. The data comes from the [NYC OpenData](https://data.cityofnewyork.us/browse?q=NYC%20calendar%20sales) portal.- [X] Do train/test split. Use data from January — March 2019 to train. Use data from April 2019 to test.- [X] Do one-hot encoding of categorical features.- [X] Do feature selection with `SelectKBest`.- [X] Do [feature scaling](https://scikit-learn.org/stable/modules/preprocessing.html).- [X] Fit a ridge regression model with multiple features.- [ ] Get mean absolute error for the test set.- [ ] As always, commit your notebook to your fork of the GitHub repo. Stretch Goals- [ ] Add your own stretch goal(s) !- [ ] Instead of `Ridge`, try `LinearRegression`. Depending on how many features you select, your errors will probably blow up! 💥- [ ] Instead of `Ridge`, try [`RidgeCV`](https://scikit-learn.org/stable/modules/generated/sklearn.linear_model.RidgeCV.html).- [ ] Learn more about feature selection: - ["Permutation importance"](https://www.kaggle.com/dansbecker/permutation-importance) - [scikit-learn's User Guide for Feature Selection](https://scikit-learn.org/stable/modules/feature_selection.html) - [mlxtend](http://rasbt.github.io/mlxtend/) library - scikit-learn-contrib libraries: [boruta_py](https://github.com/scikit-learn-contrib/boruta_py) & [stability-selection](https://github.com/scikit-learn-contrib/stability-selection) - [_Feature Engineering and Selection_](http://www.feat.engineering/) by Kuhn & Johnson.- [ ] Try [statsmodels](https://www.statsmodels.org/stable/index.html) if you’re interested in more inferential statistical approach to linear regression and feature selection, looking at p values and 95% confidence intervals for the coefficients.- [ ] Read [_An Introduction to Statistical Learning_](http://faculty.marshall.usc.edu/gareth-james/ISL/ISLR%20Seventh%20Printing.pdf), Chapters 1-3, for more math & theory, but in an accessible, readable way.- [ ] Try [scikit-learn pipelines](https://scikit-learn.org/stable/modules/compose.html).
###Code
%%capture
import sys
# If you're on Colab:
if 'google.colab' in sys.modules:
DATA_PATH = 'https://raw.githubusercontent.com/LambdaSchool/DS-Unit-2-Applied-Modeling/master/data/'
!pip install category_encoders==2.*
# If you're working locally:
else:
DATA_PATH = '../data/'
# Ignore this Numpy warning when using Plotly Express:
# FutureWarning: Method .ptp is deprecated and will be removed in a future version. Use numpy.ptp instead.
import warnings
warnings.filterwarnings(action='ignore', category=FutureWarning, module='numpy')
import pandas as pd
import pandas_profiling
# Read New York City property sales data
df = pd.read_csv(DATA_PATH+'condos/NYC_Citywide_Rolling_Calendar_Sales.csv')
# Change column names: replace spaces with underscores
df.columns = [col.replace(' ', '_') for col in df]
# SALE_PRICE was read as strings.
# Remove symbols, convert to integer
df['SALE_PRICE'] = (
df['SALE_PRICE']
.str.replace('$','')
.str.replace('-','')
.str.replace(',','')
.astype(int)
)
# BOROUGH is a numeric column, but arguably should be a categorical feature,
# so convert it from a number to a string
df['BOROUGH'] = df['BOROUGH'].astype(str)
# Reduce cardinality for NEIGHBORHOOD feature
# Get a list of the top 10 neighborhoods
top10 = df['NEIGHBORHOOD'].value_counts()[:10].index
# At locations where the neighborhood is NOT in the top 10,
# replace the neighborhood with 'OTHER'
df.loc[~df['NEIGHBORHOOD'].isin(top10), 'NEIGHBORHOOD'] = 'OTHER'
# Setting the mask(labeled as one_fam)
one_fam= ((df['BUILDING_CLASS_CATEGORY'] == '01 ONE FAMILY DWELLINGS') &
(df['SALE_PRICE']>100000) & (df['SALE_PRICE']<200000000))
df_fam= df[one_fam]
df_fam.head()
df_fam.shape
df_fam['SALE_DATE']=pd.to_datetime(df_fam['SALE_DATE'],infer_datetime_format=True)
cutoff=pd.to_datetime('2019-04-01')
train= df_fam[df_fam.SALE_DATE < cutoff]
test= df_fam[df_fam.SALE_DATE.dt.month==4]
train.dtypes
train.head()
print(train['SALE_DATE'].dt.month.unique())
print(test['SALE_DATE'].dt.month.unique())
df_fam.select_dtypes(exclude='number').columns
from sklearn.preprocessing import OneHotEncoder
onehotencoder= OneHotEncoder(categorical_features=[0])
import category_encoders as ce
encoder = ce.OneHotEncoder(use_cat_names=True)
# Subset and split again
target='SALE_PRICE'
number_column = train.select_dtypes(include='number').columns.drop(target).tolist()
categorical_column = train.select_dtypes(exclude='number').columns.tolist()
low_cardinality_catg = [col for col in categorical_column
if train[col].nunique() <= 50]
features=number_column + low_cardinality_catg
X_train= train[features]
y_train= train[target]
X_test=test[features]
y_test=test[target]
# Checking shape to verify
print(X_train.shape)
print(y_train.shape)
print(X_test.shape)
print(y_test.shape)
df_fam['NEIGHBORHOOD'].value_counts()
df_fam['LAND_SQUARE_FEET'].unique().shape
###Output
_____no_output_____
###Markdown
One Hot Encoding
###Code
# Encoding
import category_encoders as ce
encoder = ce.OneHotEncoder(use_cat_names=True)
X_train = encoder.fit_transform(X_train)
X_test= encoder.transform(X_test)
X_train.drop(['EASE-MENT','APARTMENT_NUMBER_nan'],inplace=True,axis=1)
X_test.drop(['EASE-MENT','APARTMENT_NUMBER_nan'],inplace=True,axis=1)
from sklearn.feature_selection import f_regression, SelectKBest
selector= SelectKBest(score_func=f_regression,k=10)
X_train_selected= pd.DataFrame(selector.fit_transform(X_train,y_train), columns=X_train.columns[selector.get_support()])
X_test_selected= pd.DataFrame(selector.transform(X_test), columns=X_test.columns[selector.get_support()])
from sklearn.preprocessing import StandardScaler
scaler = StandardScaler(True, True)
###Output
_____no_output_____
###Markdown
Feature Scaling
###Code
# Feature Scaling
X_train_scaler= pd.DataFrame(scaler.fit_transform(X_train_selected,y_train), columns=X_train_selected.columns)
X_test_scaler= pd.DataFrame(scaler.transform(X_test_selected), columns=X_test_selected.columns)
import warnings
warnings.filterwarnings(action='ignore', category=RuntimeWarning, module='sklearn')
from sklearn.linear_model import LinearRegression
from sklearn.metrics import mean_absolute_error
for k in range(1, len(X_train.columns)+1):
print(f'{k} features')
selector = SelectKBest(score_func=f_regression, k=k)
X_train_selected = selector.fit_transform(X_train, y_train)
X_test_selected = selector.transform(X_test)
model = LinearRegression()
model.fit(X_train_selected, y_train)
y_pred = model.predict(X_test_selected)
mae = mean_absolute_error(y_test, y_pred)
print(f'Test MAE: ${mae:,.0f} \n')
###Output
1 features
Test MAE: $290,855
2 features
Test MAE: $288,471
3 features
Test MAE: $255,484
4 features
Test MAE: $260,289
5 features
Test MAE: $258,212
6 features
Test MAE: $258,212
7 features
Test MAE: $253,793
8 features
Test MAE: $241,563
9 features
Test MAE: $244,664
10 features
Test MAE: $242,496
11 features
Test MAE: $243,042
12 features
Test MAE: $243,669
13 features
###Markdown
Ridge Regression
###Code
# Ridge Regression
from sklearn.linear_model import RidgeCV
import matplotlib.pyplot as plt
from sklearn.feature_selection import f_regression, SelectKBest
for k in range(1, len(X_train_selected.columns)+1):
print(f'{k} features')
Selector= SelectKBest(score_func=f_regression,k='k')
X_train_selected_R = selector.fit_transform(X_train_scaler, y_train)
X_test_selected_R = selector.transform(X_test_scaler)
ridge= RidgeCV()
ridge.fit(X_train_selected_R,y_train)
y_ridge_pred= ridge.predict(X_test_selected_R)
mae= mean_absolute_error(y_test,y_ridge_pred)
print(f'Test MAE: ${mae:,.0f} \n')
%matplotlib inline
coefficients = pd.Series(ridge.coef_, X_train_selected.columns)
coefficients.sort_values().plot.barh(color='grey');
print(coefficients.to_string())
###Output
BLOCK -27455.019497
GROSS_SQUARE_FEET 267405.892196
BOROUGH_1 363386.517745
NEIGHBORHOOD_OTHER -48285.703754
NEIGHBORHOOD_UPPER EAST SIDE (59-79) 206985.873039
NEIGHBORHOOD_UPPER EAST SIDE (79-96) 448891.194439
BUILDING_CLASS_AT_PRESENT_A7 63930.618292
BUILDING_CLASS_AT_PRESENT_A4 25841.136807
BUILDING_CLASS_AT_TIME_OF_SALE_A7 63930.618292
BUILDING_CLASS_AT_TIME_OF_SALE_A4 25841.136806
|
aata/groups-sage.ipynb | ###Markdown
**Important:** to view this notebook properly you will need to execute the cell above, which assumes you have an Internet connection. It should already be selected, or place your cursor anywhere above to select. Then press the "Run" button in the menu bar above (the right-pointing arrowhead), or press Shift-Enter on your keyboard. $\newcommand{\identity}{\mathrm{id}}\newcommand{\notdivide}{\nmid}\newcommand{\notsubset}{\not\subset}\newcommand{\lcm}{\operatorname{lcm}}\newcommand{\gf}{\operatorname{GF}}\newcommand{\inn}{\operatorname{Inn}}\newcommand{\aut}{\operatorname{Aut}}\newcommand{\Hom}{\operatorname{Hom}}\newcommand{\cis}{\operatorname{cis}}\newcommand{\chr}{\operatorname{char}}\newcommand{\Null}{\operatorname{Null}}\newcommand{\lt}{<}\newcommand{\gt}{>}\newcommand{\amp}{&}$ Section3.7Sage¶ Many of the groups discussed in this chapter are available for study in Sage. It is important to understand that sets that form algebraic objects (groups in this chapter) are called “parents” in Sage, and elements of these objects are called, well, “elements.” So every element belongs to a parent (in other words, is contained in some set). We can ask about properties of parents (finite? order? abelian?), and we can ask about properties of individual elements (identity? inverse?). In the following we will show you how to create some of these common groups and begin to explore their properties with Sage. SubsectionIntegers mod n
###Code
Z8 = Integers(8)
Z8
Z8.list()
a = Z8.an_element(); a
a.parent()
###Output
_____no_output_____
###Markdown
We would like to work with elements of Z8. If you were to type a 6 into a compute cell right now, what would you mean? The integer $6\text{,}$ the rational number $\frac{6}{1}\text{,}$ the real number $6.00000\text{,}$ or the complex number $6.00000+0.00000i\text{?}$ Or perhaps you really do want the integer $6$ mod $8\text{?}$ Sage really has no idea what you mean or want. To make this clear, you can “coerce” 6 into Z8 with the syntax Z8(6). Without this, Sage will treat a input number like 6 as an integer, the simplest possible interpretation in some sense. Study the following carefully, where we first work with “normal” integers and then with integers mod 8.
###Code
a = 6
a
a.parent()
b = 7
c = a + b; c
d = Z8(6)
d
d.parent()
e = Z8(7)
f = d+e; f
g = Z8(85); g
f == g
###Output
_____no_output_____
###Markdown
Z8 is a bit unusual as a first example, since it has two operations defined, both addition and multiplication, with addition forming a group, and multiplication not forming a group. Still, we can work with the additive portion, here forming the Cayley table for the addition.
###Code
Z8.addition_table(names='elements')
###Output
_____no_output_____
###Markdown
When $n$ is a prime number, the multipicative structure (excluding zero), will also form a group. The integers mod $n$ are very important, so Sage implements both addition and multiplication together. Groups of symmetries are a better example of how Sage implements groups, since there is just one operation present.
###Code
###Output
_____no_output_____
###Markdown
SubsectionGroups of symmetries¶ The symmetries of some geometric shapes are already defined in Sage, albeit with different names. They are implemented as “permutation groups” which we will begin to study carefully in Chapter 5. Sage uses integers to label vertices, starting the count at 1, instead of letters. Elements by default are printed using “cycle notation” which we will see described carefully in Chapter 5. Here is an example, with both the mathematics and Sage. For the Sage part, we create the group of symmetries and then create the symmetry $\rho_2$ with coercion, followed by outputting the element in cycle notation. Then we create just the bottom row of the notation we are using for permutations.\begin{equation*}\rho_2=\begin{pmatrix}A & B & C\\C & A & B\end{pmatrix}=\begin{pmatrix}1 & 2 & 3\\3 & 1 & 2\end{pmatrix}\end{equation*}
###Code
triangle = SymmetricGroup(3)
rho2 = triangle([3,1,2])
rho2
[rho2(x) for x in triangle.domain()]
###Output
_____no_output_____
###Markdown
The final list comprehension deserves comment. The .domain() method gives a lait of the symbols used for the permutation group triangle and then rho2 is employed with syntax like it is a function (it is a function) to create the images that would occupy the bottom row. With a double list comprehension we can list all six elements of the group in the “bottom row” format. A good exercise would be to pair up each element with its name as given in Figure 3.6.
###Code
[[a(x) for x in triangle.domain()] for a in triangle]
###Output
_____no_output_____
###Markdown
Different books, different authors, different software all have different ideas about the order in which to write multiplication of functions. This textbook builds on the idea of composition of functions, so that $fg$ is the composition $(fg)(x)=f(g(x))$ and it is natural to apply $g$ first. Sage takes the opposite view and since we write $fg\text{,}$ Sage will understand that we want to do $f$ first. Neither approach is wrong, and neither is necessarily superior, they are just different and there are good arguments for either one. When you consult other books that work with permutation groups, you want to first determine which approach it takes. (Be aware that this discussion of Sage function composition is limited to permutations only—“regular” functions in Sage compose in the order you might be familiar with from a calculus course.) The translation here between the text and Sage will be worthwhile practice. Here we will reprise the discussion at the end of Section 3.1, but reverse the order on each product to compute Sage-style and exactly mirror what the text does.
###Code
mu1 = triangle([1,3,2])
mu2 = triangle([3,2,1])
mu3 = triangle([2,1,3])
rho1 = triangle([2,3,1])
product = rho1*mu1
product == mu2
[product(x) for x in triangle.domain()]
rho1*mu1 == mu1*rho1
mu1*rho1 == mu3
###Output
_____no_output_____
###Markdown
Now that we understand that Sage does multiplication in reverse, we can compute the Cayley table for this group. Default behavior is to just name elements of a group as letters, a, b, c, \dots{} in the same order that the .list() command would produce the elements of the group. But you can also print the elements in the table as themselves (that uses cycle notation here), or you can give the elements names. We will use u as shorthand for $\mu$ and r as shorthand for $\rho\text{.}$
###Code
triangle.cayley_table()
triangle.cayley_table(names='elements')
triangle.cayley_table(names=['id','u3','r1','r2','u1','u2'])
###Output
_____no_output_____
###Markdown
You should verify that the table above is correct, just like Table 3.2 is correct. Remember that the convention is to multiply a row label times a column label, in that order. However, to do a check across the two tables, you will need to recall the difference in ordering between your textbook and Sage.
###Code
###Output
_____no_output_____
###Markdown
SubsectionQuaternions Sage implements the quaternions, but the elements are not matrices, but rather are permutations. Despite appearances the structure is identical. It should not matter which version you have in mind (matrices or permutations) if you build the Cayley table and use the default behavior of using letters to name the elements. As permutations, or as letters, can you identify $-1\text{,}$ $I\text{,}$ $J$ and $K\text{?}$
###Code
Q = QuaternionGroup()
[[a(x) for x in Q.domain()] for a in Q]
Q.cayley_table()
###Output
_____no_output_____
###Markdown
It should be fairly obvious that a is the identity element of the group ($1$), either from its behavior in the table, or from its “bottom row” representation as the first element of the list above. And if you prefer, you can ask Sage for a list of its outputs when viewed as a function.
###Code
id = Q.identity()
[id(x) for x in Q.domain()]
###Output
_____no_output_____
###Markdown
Now $-1$ should have the property that $-1\cdot -1= 1\text{.}$ We see that the identity element a is on the diagonal of the Cayley table only when we compute d*d. We can verify this easily, borrowing the fourth “bottom row” element from the list above. With this information, once we locate $I\text{,}$ we can easily compute $-I\text{,}$ and so on.
###Code
minus_one = Q([3, 4, 1, 2, 7, 8, 5, 6])
minus_one*minus_one == Q.identity()
###Output
_____no_output_____
###Markdown
See if you can pair up the letters with all eight elements of the quaternions. Be a bit careful with your names, the symbol I is used by Sage for the imaginary number $i=\sqrt{-1}$ (which we will use below), but Sage will silently let you redefine it to be anything you like. Same goes for using lower-case i in Sage. So call your elements of the quaternions something like QI, QJ, QK to avoid confusion. As we begin to work with groups it is instructive to work with the actual elements. But many properties of groups are totally independent of the order we use for multiplication, or the names or representations we use for the elements. Here are facts about the quaternions we can compute without any knowledge of just how the elements are written or multiplied.
###Code
Q.is_finite()
Q.order()
Q.is_abelian()
###Output
_____no_output_____
###Markdown
SubsectionSubgroups The best techniques for creating subgroups will come in future chapters, but we can create some groups that are naturally subgroups of other groups. Elements of the quaternions were represented by certain permutations of the integers 1 through 8. We can also build the group of all permutations of these eight integers. It gets pretty big, so do not list it unless you want a lot of output! (I dare you.)
###Code
S8 = SymmetricGroup(8)
a = S8.random_element()
[a(x) for x in S8.domain()] # random
S8.order()
###Output
_____no_output_____
###Markdown
The quaternions, Q, is a subgroup of the full group of all permutations, the symmetric group $S_8$ or S8, and Sage regards this as a property of Q.
###Code
Q.is_subgroup(S8)
###Output
_____no_output_____
###Markdown
In Sage the complex numbers are known by the name CC. We can create a list of the elements in the subgroup described in Example 3.16. Then we can verify that this set is a subgroup by examining the Cayley table, using multiplication as the operation.
###Code
H = [CC(1), CC(-1), CC(I), CC(-I)]
CC.multiplication_table(elements=H,
names=['1', '-1', 'i', '-i'])
###Output
_____no_output_____ |
yhat_image_classifier-Copy1.ipynb | ###Markdown
Importing Image Data Create Processing Functions
###Code
#setup a standard image size; this will distort some images but will get everything into the same shape
STANDARD_SIZE = (230, 170)
def img_to_matrix(filename, verbose=False):
"""
takes a filename and turns it into a numpy array of RGB pixels
"""
img = PIL.Image.open(filename)
if verbose==True:
print("changing size from %s to %s" % (str(img.size), str(STANDARD_SIZE)))
img = img.resize(STANDARD_SIZE)
#img = list(img.getdata())
#img = map(list, img)
img = np.array(img)
return img
def flatten_image(img):
"""
takes in an (m, n) numpy array and flattens it
into an array of shape (1, m * n)
"""
s = img.shape[0] * img.shape[1]
img_wide = img.reshape(1, img.size)
return img_wide[0]
filename = "/home/vm/sunset/images/sunset_1"
img = PIL.Image.open(filename)
i = np.array(img.getdata())
i.shape
from skimage import *
from skimage import novice
novice?
picture = novice.open('/home/vm/sunset/images/sunset_1')
picture.format
skimage?
###Output
_____no_output_____
###Markdown
Define the location of your data and import it
###Code
# TODO PATH TO YOUR DATA
img_dir = "/home/vm/sunset/images/"
images = [img_dir+ f for f in os.listdir(img_dir)]
labels = ["sunset" if "sunset" in f.split('/')[-1] else "sunrise" for f in images]
data = []
for image in images:
img = img_to_matrix(image)
img_flat = flatten_image(img)
#img_flat = flatten_image(image)
data.append(img_flat)
data = np.array(data)
data
###Output
_____no_output_____
###Markdown
Creating Features Define a training and test set
###Code
is_train = np.random.uniform(0, 1, len(data)) <= 0.7
y = np.where(np.array(labels)=="check", 1, 0)
train_x, train_y = data[is_train], y[is_train]
test_x, test_y = data[is_train==False], y[is_train==False]
###Output
_____no_output_____
###Markdown
RandomizedPCA to create featuresBefore we actually create our feature vectors, we're going to show a demo of RandomizedPCA in 2 dimensions. This makes it easy to plot high dimensional data
###Code
pca = PCA(svd_solver='randomized',n_components=2)
X = pca.fit_transform(data)
df = pd.DataFrame({"x": X[:, 0], "y": X[:, 1], "label":np.where(y==1, "sunset", "sunrise")})
colors = ["red", "yellow"]
for label, color in zip(df['label'].unique(), colors):
mask = df['label']==label
pl.scatter(df[mask]['x'], df[mask]['y'], c=color, label=label)
pl.legend()
X
###Output
_____no_output_____
###Markdown
RandomizedPCA in 5 dimensionsInstead of 2 dimenisons, we're going to do RandomizedPCA in 5 dimensions. This will make it a bit harder to visualize, but it will make it easier for some of the classifiers to work with the dataset.
###Code
pca = RandomizedPCA(n_components=5)
train_x = pca.fit_transform(train_x)
test_x = pca.transform(test_x)
###Output
/home/vm/anaconda3/lib/python3.5/site-packages/sklearn/utils/deprecation.py:52: DeprecationWarning: Class RandomizedPCA is deprecated; RandomizedPCA was deprecated in 0.18 and will be removed in 0.20. Use PCA(svd_solver='randomized') instead. The new implementation DOES NOT store whiten ``components_``. Apply transform to get them.
warnings.warn(msg, category=DeprecationWarning)
###Markdown
This gives our classifier a nice set of tabular data that we can then use to train the model
###Code
train_x[:5]
###Output
_____no_output_____
###Markdown
We're going to be using a K-Nearest Neighbors classifier. Based on our set of training data, we're going to caclulate which training obersvations are closest to a given test point. Whichever class has the most votes wins.
###Code
knn = KNeighborsClassifier()
knn.fit(train_x, train_y)
pd.crosstab(test_y, knn.predict(test_x), rownames=["Actual"], colnames=["Predicted"])
knn.outputs_2d_
# string_to_img(new_image)
def string_to_img(image_string):
print("called string_to_image")
#we need to decode the image from base64
image_string = base64.decodestring(image_string)
#since we're seing this as a JSON string, we use StringIO so it acts like a file
img = StringIO(image_string)
img = PIL.Image.open(img)
img = img.resize(STANDARD_SIZE)
img = list(img.getdata())
img = map(list, img)
img = np.array(img)
s = img.shape[0] * img.shape[1]
img_wide = img.reshape(1, s)
return pca.transform(img_wide[0])
def classify_image(data):
print("called classify_image")
preds = knn.predict(data)
preds = np.where(preds==1, "check", "drivers_license")
pred = preds[0]
return {"image_label": pred}
from yhat import Yhat, YhatModel, preprocess
class ImageClassifier(YhatModel):
REQUIREMENTS = [
"PIL==1.1.7"
]
def execute(self, data):
print("called execute")
img_string = data.get("image_as_base64_string", None)
if img_string is None:
return {"status": "error", "message": "data was None", "input_data": data}
else:
img = string_to_img(img_string)
pred = classify_image(img)
return pred
# authenticate
yh = Yhat("USERNAME", "YOUR API KEY", "http://cloud.yhathq.com/")
# upload model to yhat
yh.deploy("ImageClassifier", ImageClassifier, globals())
# i don't have the image data set any more
# so just some dummy data to get it to work :(
new_image = open("/Users/hernamesbarbara/Desktop/img/1-plot-iris.png", 'rb').read()
#we need to make the image JSON serializeable
new_image = base64.encodestring(new_image)
yh.predict("ImageClassifier", {"image_as_base64_string": new_image})
###Output
_____no_output_____ |
Neural_Nets_Gesture_Recognition_Final.ipynb | ###Markdown
Gesture RecognitionIn this project, we are going to build a 3D Conv model that will be able to predict the 5 gestures correctly. Import libraries to get started.
###Code
import numpy as np
import datetime
import os
import cv2
import random as rn
import tensorflow as tf
from keras import backend as K
from keras.callbacks import ModelCheckpoint, ReduceLROnPlateau
from keras.losses import categorical_crossentropy
from keras.optimizers import Adam
from keras.models import Sequential, Model
from keras.layers import Dense, GRU, Flatten, TimeDistributed, Flatten, BatchNormalization
from keras.layers import Activation, Dropout, ZeroPadding3D
from keras.layers.convolutional import Conv2D, MaxPooling3D, Conv3D, MaxPooling2D
from keras.layers.recurrent import LSTM
###Output
_____no_output_____
###Markdown
We set the random seed so that the results don't vary drastically.
###Code
np.random.seed(30)
rn.seed(30)
tf.set_random_seed(30)
train_path = './Project_data/train'
val_path = './Project_data/val'
train_doc = np.random.permutation(open('./Project_data/train.csv').readlines())
val_doc = np.random.permutation(open('./Project_data/val.csv').readlines())
###Output
_____no_output_____
###Markdown
Generator ClassThis is one of the most important part of the code. The overall structure of the generator is broken down into modules. In the generator, we are going to preprocess the images as we have images of 2 different dimensions as well as create a batch of video frames.
###Code
class DataGenerator:
def __init__(self, width=120, height=120, frames=30, channel=3,
crop = True, normalize = False, affine = False, flip = False, edge = False ):
self.width = width # X dimension of the image
self.height = height # Y dimesnion of the image
self.frames = frames # length/depth of the video frames
self.channel = channel # number of channels in images 3 for color(RGB) and 1 for Gray
self.affine = affine # augment data with affine transform of the image
self.flip = flip
self.normalize = normalize
self.edge = edge # edge detection
self.crop = crop
# Helper function to generate a random affine transform on the image
def __get_random_affine(self): # private method
dx, dy = np.random.randint(-1.7, 1.8, 2)
M = np.float32([[1, 0, dx], [0, 1, dy]])
return M
# Helper function to initialize all the batch image data and labels
def __init_batch_data(self, batch_size): # private method
batch_data = np.zeros((batch_size, self.frames, self.width, self.height, self.channel))
batch_labels = np.zeros((batch_size,5)) # batch_labels is the one hot representation of the output
return batch_data, batch_labels
def __load_batch_images(self, source_path, folder_list, batch_num, batch_size, t): # private method
batch_data,batch_labels = self.__init_batch_data(batch_size)
# We will also build a agumented batch data
if self.affine:
batch_data_aug,batch_labels_aug = self.__init_batch_data(batch_size)
if self.flip:
batch_data_flip,batch_labels_flip = self.__init_batch_data(batch_size)
#create a list of image numbers you want to use for a particular video
img_idx = [x for x in range(0, self.frames)]
for folder in range(batch_size): # iterate over the batch_size
# read all the images in the folder
imgs = sorted(os.listdir(source_path+'/'+ t[folder + (batch_num*batch_size)].split(';')[0]))
# Generate a random affine to be used in image transformation for buidling agumented data set
M = self.__get_random_affine()
# Iterate over the frames/images of a folder to read them in
for idx, item in enumerate(img_idx):
image = cv2.imread(source_path+'/'+ t[folder + (batch_num*batch_size)].strip().split(';')[0]+'/'+imgs[item], cv2.IMREAD_COLOR)
image = cv2.cvtColor(image, cv2.COLOR_BGR2RGB)
#crop the images and resize them. Note that the images are of 2 different shape
#and the conv3D will throw error if the inputs in a batch have different shapes
if self.crop:
image = self.__crop(image)
# If normalize is set normalize the image else use the raw image.
if self.normalize:
resized = self.__normalize(self.__resize(image))
else:
resized = self.__resize(image)
# If the input is edge detected image then use the sobelx, sobely and laplacian as 3 channel of the edge detected image
if self.edge:
resized = self.__edge(resized)
batch_data[folder,idx] = resized
if self.affine:
batch_data_aug[folder,idx] = self.__affine(resized, M)
if self.flip:
batch_data_flip[folder,idx] = self.__flip(resized)
batch_labels[folder, int(t[folder + (batch_num*batch_size)].strip().split(';')[2])] = 1
if self.affine:
batch_labels_aug[folder, int(t[folder + (batch_num*batch_size)].strip().split(';')[2])] = 1
if self.flip:
if int(t[folder + (batch_num*batch_size)].strip().split(';')[2])==0:
batch_labels_flip[folder, 1] = 1
elif int(t[folder + (batch_num*batch_size)].strip().split(';')[2])==1:
batch_labels_flip[folder, 0] = 1
else:
batch_labels_flip[folder, int(t[folder + (batch_num*batch_size)].strip().split(';')[2])] = 1
if self.affine:
batch_data = np.append(batch_data, batch_data_aug, axis = 0)
batch_labels = np.append(batch_labels, batch_labels_aug, axis = 0)
if self.flip:
batch_data = np.append(batch_data, batch_data_flip, axis = 0)
batch_labels = np.append(batch_labels, batch_labels_flip, axis = 0)
return batch_data, batch_labels
def generator(self, source_path, folder_list, batch_size): # public method
print( 'Source path = ', source_path, '; batch size =', batch_size)
while True:
t = np.random.permutation(folder_list)
num_batches = len(folder_list)//batch_size # calculate the number of batches
for batch in range(num_batches): # we iterate over the number of batches
# you yield the batch_data and the batch_labels, remember what does yield do
yield self.__load_batch_images(source_path, folder_list, batch, batch_size, t)
# Code for the remaining data points which are left after full batches
if (len(folder_list) != batch_size*num_batches):
batch_size = len(folder_list) - (batch_size*num_batches)
yield self.__load_batch_images(source_path, folder_list, num_batches, batch_size, t)
# Helper function to perfom affice transform on the image
def __affine(self, image, M):
return cv2.warpAffine(image, M, (image.shape[0], image.shape[1]))
# Helper function to flip the image
def __flip(self, image):
return np.flip(image,1)
# Helper function to normalise the data
def __normalize(self, image):
return image/127.5-1
# Helper function to resize the image
def __resize(self, image):
return cv2.resize(image, (self.width,self.height), interpolation = cv2.INTER_AREA)
# Helper function to crop the image
def __crop(self, image):
if image.shape[0] != image.shape[1]:
return image[0:120, 20:140]
else:
return image
# Helper function for edge detection
def __edge(self, image):
edge = np.zeros((image.shape[0], image.shape[1], image.shape[2]))
edge[:,:,0] = cv2.Laplacian(cv2.GaussianBlur(image[:,:,0],(3,3),0),cv2.CV_64F)
edge[:,:,1] = cv2.Laplacian(cv2.GaussianBlur(image[:,:,1],(3,3),0),cv2.CV_64F)
edge[:,:,2] = cv2.Laplacian(cv2.GaussianBlur(image[:,:,2],(3,3),0),cv2.CV_64F)
return edge
###Output
_____no_output_____
###Markdown
Model Class
###Code
class ModelGenerator(object):
@classmethod
def c3d1(cls, input_shape, nb_classes):
"""
Build a 3D convolutional network, based loosely on C3D.
https://arxiv.org/pdf/1412.0767.pdf
"""
# Model.
model = Sequential()
model.add(Conv3D(
8, (3,3,3), activation='relu', input_shape=input_shape
))
model.add(MaxPooling3D(pool_size=(1, 2, 2), strides=(1, 2, 2)))
model.add(Conv3D(16, (3,3,3), activation='relu'))
model.add(MaxPooling3D(pool_size=(1, 2, 2), strides=(1, 2, 2)))
model.add(Conv3D(32, (3,3,3), activation='relu'))
model.add(Conv3D(32, (3,3,3), activation='relu'))
model.add(MaxPooling3D(pool_size=(1, 2, 2), strides=(1, 2, 2)))
model.add(Conv3D(64, (2,2,2), activation='relu'))
model.add(Conv3D(64, (2,2,2), activation='relu'))
model.add(MaxPooling3D(pool_size=(1, 2, 2), strides=(1, 2, 2)))
model.add(Flatten())
model.add(Dense(512))
model.add(Dropout(0.5))
model.add(Dense(256))
model.add(Dropout(0.5))
model.add(Dense(nb_classes, activation='softmax'))
return model
@classmethod
def c3d2(cls, input_shape, nb_classes):
"""
Build a 3D convolutional network, aka C3D.
https://arxiv.org/pdf/1412.0767.pdf
"""
model = Sequential()
# 1st layer group
model.add(Conv3D(16, 3, 3, 3, activation='relu',
padding='same', name='conv1',
subsample=(1, 1, 1),
input_shape=input_shape))
model.add(MaxPooling3D(pool_size=(1, 2, 2), strides=(1, 2, 2),
border_mode='valid', name='pool1'))
# 2nd layer group
model.add(Conv3D(32, 3, 3, 3, activation='relu',
padding='same', name='conv2',
subsample=(1, 1, 1)))
model.add(MaxPooling3D(pool_size=(2, 2, 2), strides=(2, 2, 2),
border_mode='valid', name='pool2'))
# 3rd layer group
model.add(Conv3D(64, 3, 3, 3, activation='relu',
padding='same', name='conv3a',
subsample=(1, 1, 1)))
model.add(Conv3D(64, 3, 3, 3, activation='relu',
padding='same', name='conv3b',
subsample=(1, 1, 1)))
model.add(MaxPooling3D(pool_size=(2, 2, 2), strides=(2, 2, 2),
border_mode='valid', name='pool3'))
# 4th layer group
model.add(Conv3D(128, 3, 3, 3, activation='relu',
padding='same', name='conv4a',
subsample=(1, 1, 1)))
model.add(Conv3D(128, 3, 3, 3, activation='relu',
padding='same', name='conv4b',
subsample=(1, 1, 1)))
model.add(MaxPooling3D(pool_size=(2, 2, 2), strides=(2, 2, 2),
border_mode='valid', name='pool4'))
# 5th layer group
model.add(Conv3D(256, 3, 3, 3, activation='relu',
padding='same', name='conv5a',
subsample=(1, 1, 1)))
model.add(Conv3D(256, 3, 3, 3, activation='relu',
padding='same', name='conv5b',
subsample=(1, 1, 1)))
model.add(ZeroPadding3D(padding=(0, 1, 1)))
model.add(MaxPooling3D(pool_size=(2, 2, 2), strides=(2, 2, 2),
padding='valid', name='pool5'))
model.add(Flatten())
# FC layers group
model.add(Dense(512, activation='relu', name='fc6'))
model.add(Dropout(0.5))
model.add(Dense(512, activation='relu', name='fc7'))
model.add(Dropout(0.5))
model.add(Dense(nb_classes, activation='softmax'))
return model
@classmethod
def c3d3(cls, input_shape, nb_classes):
model = Sequential()
model.add(Conv3D(16, kernel_size=(3, 3, 3), input_shape=input_shape, padding='same'))
model.add(Activation('relu'))
model.add(Conv3D(16, padding="same", kernel_size=(3, 3, 3)))
model.add(Activation('relu'))
model.add(MaxPooling3D(pool_size=(3, 3, 3), padding="same"))
model.add(Dropout(0.25))
model.add(Conv3D(32, padding="same", kernel_size=(3, 3, 3)))
model.add(Activation('relu'))
model.add(Conv3D(32, padding="same", kernel_size=(3, 3, 3)))
model.add(Activation('relu'))
model.add(MaxPooling3D(pool_size=(3, 3, 3), padding="same"))
model.add(Dropout(0.25))
model.add(Conv3D(32, padding="same", kernel_size=(3, 3, 3)))
model.add(Activation('relu'))
model.add(Conv3D(32, padding="same", kernel_size=(3, 3, 3)))
model.add(Activation('relu'))
model.add(MaxPooling3D(pool_size=(3, 3, 3), padding="same"))
model.add(Dropout(0.25))
model.add(Flatten())
model.add(Dense(512, activation='relu'))
model.add(BatchNormalization())
model.add(Dropout(0.5))
model.add(Dense(nb_classes, activation='softmax'))
return model
@classmethod
def c3d4(cls, input_shape, nb_classes):
# Define model
model = Sequential()
model.add(Conv3D(8, kernel_size=(3,3,3), input_shape=input_shape, padding='same'))
model.add(BatchNormalization())
model.add(Activation('relu'))
model.add(MaxPooling3D(pool_size=(2,2,2)))
model.add(Conv3D(16, kernel_size=(3,3,3), padding='same'))
model.add(BatchNormalization())
model.add(Activation('relu'))
model.add(MaxPooling3D(pool_size=(2,2,2)))
model.add(Conv3D(32, kernel_size=(1,3,3), padding='same'))
model.add(BatchNormalization())
model.add(Activation('relu'))
model.add(MaxPooling3D(pool_size=(2,2,2)))
model.add(Conv3D(64, kernel_size=(1,3,3), padding='same'))
model.add(Activation('relu'))
model.add(Dropout(0.25))
model.add(MaxPooling3D(pool_size=(2,2,2)))
#Flatten Layers
model.add(Flatten())
model.add(Dense(256, activation='relu'))
model.add(Dropout(0.5))
model.add(Dense(128, activation='relu'))
model.add(Dropout(0.5))
#softmax layer
model.add(Dense(5, activation='softmax'))
return model
@classmethod
def c3d5(cls, input_shape, nb_classes):
# Define model
model = Sequential()
model.add(Conv3D(8, kernel_size=(3,3,3), input_shape=input_shape, padding='same'))
#model.add(BatchNormalization())
model.add(Activation('relu'))
model.add(Dropout(0.25))
model.add(MaxPooling3D(pool_size=(2,2,2)))
model.add(Conv3D(16, kernel_size=(3,3,3), padding='same'))
#model.add(BatchNormalization())
model.add(Activation('relu'))
model.add(Dropout(0.25))
model.add(MaxPooling3D(pool_size=(2,2,2)))
model.add(Conv3D(32, kernel_size=(1,3,3), padding='same'))
#model.add(BatchNormalization())
model.add(Activation('relu'))
model.add(Dropout(0.25))
model.add(MaxPooling3D(pool_size=(2,2,2)))
model.add(Conv3D(64, kernel_size=(1,3,3), padding='same'))
model.add(Activation('relu'))
model.add(Dropout(0.25))
model.add(MaxPooling3D(pool_size=(2,2,2)))
#Flatten Layers
model.add(Flatten())
model.add(Dense(256, activation='relu'))
model.add(Dropout(0.5))
model.add(Dense(128, activation='relu'))
model.add(Dropout(0.5))
#softmax layer
model.add(Dense(5, activation='softmax'))
return model
@classmethod
def lstm(cls, input_shape, nb_classes):
"""Build a simple LSTM network. We pass the extracted features from
our CNN to this model predomenently."""
# Model.
model = Sequential()
model.add(LSTM(2048, return_sequences=False,
input_shape=input_shape,
dropout=0.5))
model.add(Dense(512, activation='relu'))
model.add(Dropout(0.5))
model.add(Dense(nb_classes, activation='softmax'))
return model
@classmethod
def lrcn(cls, input_shape, nb_classes):
"""Build a CNN into RNN.
Starting version from:
https://github.com/udacity/self-driving-car/blob/master/
steering-models/community-models/chauffeur/models.py
Heavily influenced by VGG-16:
https://arxiv.org/abs/1409.1556
Also known as an LRCN:
https://arxiv.org/pdf/1411.4389.pdf
"""
model = Sequential()
model.add(TimeDistributed(Conv2D(32, (7, 7), strides=(2, 2),
activation='relu', padding='same'), input_shape=input_shape))
model.add(TimeDistributed(Conv2D(32, (3,3),
kernel_initializer="he_normal", activation='relu')))
model.add(TimeDistributed(MaxPooling2D((2, 2), strides=(2, 2))))
model.add(TimeDistributed(Conv2D(64, (3,3),
padding='same', activation='relu')))
model.add(TimeDistributed(Conv2D(64, (3,3),
padding='same', activation='relu')))
model.add(TimeDistributed(MaxPooling2D((2, 2), strides=(2, 2))))
model.add(TimeDistributed(Conv2D(128, (3,3),
padding='same', activation='relu')))
model.add(TimeDistributed(Conv2D(128, (3,3),
padding='same', activation='relu')))
model.add(TimeDistributed(MaxPooling2D((2, 2), strides=(2, 2))))
model.add(TimeDistributed(Conv2D(256, (3,3),
padding='same', activation='relu')))
model.add(TimeDistributed(Conv2D(256, (3,3),
padding='same', activation='relu')))
model.add(TimeDistributed(MaxPooling2D((2, 2), strides=(2, 2))))
model.add(TimeDistributed(Conv2D(512, (3,3),
padding='same', activation='relu')))
model.add(TimeDistributed(Conv2D(512, (3,3),
padding='same', activation='relu')))
model.add(TimeDistributed(MaxPooling2D((2, 2), strides=(2, 2))))
model.add(TimeDistributed(Flatten()))
model.add(Dropout(0.5))
model.add(LSTM(256, return_sequences=False, dropout=0.5))
model.add(Dense(nb_classes, activation='softmax'))
return model
@classmethod
def mlp(cls, input_shape, nb_classes):
"""Build a simple MLP. It uses extracted features as the input
because of the otherwise too-high dimensionality."""
# Model.
model = Sequential()
model.add(Flatten(input_shape=input_shape))
model.add(Dense(512))
model.add(Dropout(0.5))
model.add(Dense(512))
model.add(Dropout(0.5))
model.add(Dense(nb_classes, activation='softmax'))
return model
###Output
_____no_output_____
###Markdown
Trainer Function
###Code
def train(batch_size, num_epochs, model, train_generator, val_generator, optimiser=None):
curr_dt_time = datetime.datetime.now()
num_train_sequences = len(train_doc)
print('# training sequences =', num_train_sequences)
num_val_sequences = len(val_doc)
print('# validation sequences =', num_val_sequences)
print('# batch size =', batch_size)
print('# epochs =', num_epochs)
#optimizer = Adam(lr=rate)
#write your optimizer
if optimiser == None:
optimiser = Adam()
model.compile(optimizer=optimiser, loss='categorical_crossentropy', metrics=['categorical_accuracy'])
print (model.summary())
model_name = 'model_init' + '_' + str(curr_dt_time).replace(' ','').replace(':','_') + '/'
if not os.path.exists(model_name):
os.mkdir(model_name)
filepath = model_name + 'model-{epoch:05d}-{loss:.5f}-{categorical_accuracy:.5f}-{val_loss:.5f}-{val_categorical_accuracy:.5f}.h5'
checkpoint = ModelCheckpoint(filepath,
monitor='val_loss',
verbose=1,
save_best_only=False,
save_weights_only=False,
mode='auto',
period=1)
LR = ReduceLROnPlateau(monitor='val_loss', factor=0.5, patience=2, cooldown=1, verbose=1)
callbacks_list = [checkpoint, LR]
if (num_train_sequences%batch_size) == 0:
steps_per_epoch = int(num_train_sequences/batch_size)
else:
steps_per_epoch = (num_train_sequences//batch_size) + 1
if (num_val_sequences%batch_size) == 0:
validation_steps = int(num_val_sequences/batch_size)
else:
validation_steps = (num_val_sequences//batch_size) + 1
model.fit_generator(train_generator, steps_per_epoch=steps_per_epoch, epochs=num_epochs, verbose=1,
callbacks=callbacks_list, validation_data=val_generator,
validation_steps=validation_steps, class_weight=None, workers=1, initial_epoch=0)
K.clear_session()
from numba import cuda
def clear_cuda():
#cuda.select_device(0)
#cuda.close()
pass
###Output
_____no_output_____
###Markdown
Model 1 Model 1a : Resize to 120*120, Raw image input, No cropping, No normalisation, No agumentation, No flipped images, No edge detection
###Code
train_gen = DataGenerator()
val_gen = DataGenerator()
model_gen = ModelGenerator()
input_shape = (30,120,120, 3)
num_classes = 5
model = model_gen.c3d1(input_shape, num_classes)
batch_size = 20
num_epochs = 20
train_generator = train_gen.generator(train_path, train_doc, batch_size)
val_generator = val_gen.generator(val_path, val_doc, batch_size)
train(batch_size, num_epochs, model, train_generator, val_generator)
###Output
# training sequences = 663
# validation sequences = 100
# batch size = 20
# epochs = 20
_________________________________________________________________
Layer (type) Output Shape Param #
=================================================================
conv3d_1 (Conv3D) (None, 28, 118, 118, 8) 656
_________________________________________________________________
max_pooling3d_1 (MaxPooling3 (None, 28, 59, 59, 8) 0
_________________________________________________________________
conv3d_2 (Conv3D) (None, 26, 57, 57, 16) 3472
_________________________________________________________________
max_pooling3d_2 (MaxPooling3 (None, 26, 28, 28, 16) 0
_________________________________________________________________
conv3d_3 (Conv3D) (None, 24, 26, 26, 32) 13856
_________________________________________________________________
conv3d_4 (Conv3D) (None, 22, 24, 24, 32) 27680
_________________________________________________________________
max_pooling3d_3 (MaxPooling3 (None, 22, 12, 12, 32) 0
_________________________________________________________________
conv3d_5 (Conv3D) (None, 21, 11, 11, 64) 16448
_________________________________________________________________
conv3d_6 (Conv3D) (None, 20, 10, 10, 64) 32832
_________________________________________________________________
max_pooling3d_4 (MaxPooling3 (None, 20, 5, 5, 64) 0
_________________________________________________________________
flatten_1 (Flatten) (None, 32000) 0
_________________________________________________________________
dense_1 (Dense) (None, 512) 16384512
_________________________________________________________________
dropout_1 (Dropout) (None, 512) 0
_________________________________________________________________
dense_2 (Dense) (None, 256) 131328
_________________________________________________________________
dropout_2 (Dropout) (None, 256) 0
_________________________________________________________________
dense_3 (Dense) (None, 5) 1285
=================================================================
Total params: 16,612,069
Trainable params: 16,612,069
Non-trainable params: 0
_________________________________________________________________
None
Epoch 1/20
Source path = ./Project_data/val ; batch size = 20
Source path = ./Project_data/train ; batch size = 20
34/34 [==============================] - 26s 762ms/step - loss: 12.8213 - categorical_accuracy: 0.2046 - val_loss: 12.4109 - val_categorical_accuracy: 0.2300
Epoch 00001: saving model to model_init_2018-10-2121_17_53.160408/model-00001-12.73929-0.20965-12.41093-0.23000.h5
Epoch 2/20
34/34 [==============================] - 4s 115ms/step - loss: 12.9577 - categorical_accuracy: 0.1961 - val_loss: 12.4109 - val_categorical_accuracy: 0.2300
Epoch 00002: saving model to model_init_2018-10-2121_17_53.160408/model-00002-12.95768-0.19608-12.41093-0.23000.h5
Epoch 3/20
34/34 [==============================] - 4s 110ms/step - loss: 12.9577 - categorical_accuracy: 0.1961 - val_loss: 12.4109 - val_categorical_accuracy: 0.2300
Epoch 00003: saving model to model_init_2018-10-2121_17_53.160408/model-00003-12.95768-0.19608-12.41093-0.23000.h5
Epoch 00003: ReduceLROnPlateau reducing learning rate to 0.0005000000237487257.
Epoch 4/20
34/34 [==============================] - 4s 105ms/step - loss: 13.1157 - categorical_accuracy: 0.1863 - val_loss: 12.4109 - val_categorical_accuracy: 0.2300
Epoch 00004: saving model to model_init_2018-10-2121_17_53.160408/model-00004-13.11570-0.18627-12.41093-0.23000.h5
Epoch 5/20
34/34 [==============================] - 4s 113ms/step - loss: 12.7997 - categorical_accuracy: 0.2059 - val_loss: 12.4109 - val_categorical_accuracy: 0.2300
Epoch 00005: saving model to model_init_2018-10-2121_17_53.160408/model-00005-12.79966-0.20588-12.41093-0.23000.h5
Epoch 00005: ReduceLROnPlateau reducing learning rate to 0.0002500000118743628.
Epoch 6/20
34/34 [==============================] - 4s 118ms/step - loss: 12.9577 - categorical_accuracy: 0.1961 - val_loss: 12.4109 - val_categorical_accuracy: 0.2300
Epoch 00006: saving model to model_init_2018-10-2121_17_53.160408/model-00006-12.95768-0.19608-12.41093-0.23000.h5
Epoch 7/20
34/34 [==============================] - 3s 102ms/step - loss: 12.7997 - categorical_accuracy: 0.2059 - val_loss: 12.4109 - val_categorical_accuracy: 0.2300
Epoch 00007: saving model to model_init_2018-10-2121_17_53.160408/model-00007-12.79966-0.20588-12.41093-0.23000.h5
Epoch 00007: ReduceLROnPlateau reducing learning rate to 0.0001250000059371814.
Epoch 8/20
34/34 [==============================] - 4s 122ms/step - loss: 11.5355 - categorical_accuracy: 0.2843 - val_loss: 12.4109 - val_categorical_accuracy: 0.2300
Epoch 00008: saving model to model_init_2018-10-2121_17_53.160408/model-00008-11.53550-0.28431-12.41093-0.23000.h5
Epoch 9/20
34/34 [==============================] - 4s 122ms/step - loss: 12.4836 - categorical_accuracy: 0.2255 - val_loss: 12.4109 - val_categorical_accuracy: 0.2300
Epoch 00009: saving model to model_init_2018-10-2121_17_53.160408/model-00009-12.48362-0.22549-12.41093-0.23000.h5
Epoch 00009: ReduceLROnPlateau reducing learning rate to 6.25000029685907e-05.
Epoch 10/20
34/34 [==============================] - 5s 134ms/step - loss: 12.6416 - categorical_accuracy: 0.2157 - val_loss: 12.4109 - val_categorical_accuracy: 0.2300
Epoch 00010: saving model to model_init_2018-10-2121_17_53.160408/model-00010-12.64164-0.21569-12.41093-0.23000.h5
Epoch 11/20
34/34 [==============================] - 5s 138ms/step - loss: 13.1157 - categorical_accuracy: 0.1863 - val_loss: 12.4109 - val_categorical_accuracy: 0.2300
Epoch 00011: saving model to model_init_2018-10-2121_17_53.160408/model-00011-13.11571-0.18627-12.41093-0.23000.h5
Epoch 00011: ReduceLROnPlateau reducing learning rate to 3.125000148429535e-05.
Epoch 12/20
34/34 [==============================] - 5s 136ms/step - loss: 12.7997 - categorical_accuracy: 0.2059 - val_loss: 12.4109 - val_categorical_accuracy: 0.2300
Epoch 00012: saving model to model_init_2018-10-2121_17_53.160408/model-00012-12.79966-0.20588-12.41093-0.23000.h5
Epoch 13/20
34/34 [==============================] - 5s 139ms/step - loss: 13.1157 - categorical_accuracy: 0.1863 - val_loss: 12.4109 - val_categorical_accuracy: 0.2300
Epoch 00013: saving model to model_init_2018-10-2121_17_53.160408/model-00013-13.11570-0.18627-12.41093-0.23000.h5
Epoch 00013: ReduceLROnPlateau reducing learning rate to 1.5625000742147677e-05.
Epoch 14/20
34/34 [==============================] - 5s 138ms/step - loss: 13.4317 - categorical_accuracy: 0.1667 - val_loss: 12.4109 - val_categorical_accuracy: 0.2300
Epoch 00014: saving model to model_init_2018-10-2121_17_53.160408/model-00014-13.43175-0.16667-12.41093-0.23000.h5
Epoch 15/20
34/34 [==============================] - 5s 133ms/step - loss: 12.0096 - categorical_accuracy: 0.2549 - val_loss: 12.4109 - val_categorical_accuracy: 0.2300
Epoch 00015: saving model to model_init_2018-10-2121_17_53.160408/model-00015-12.00956-0.25490-12.41093-0.23000.h5
Epoch 00015: ReduceLROnPlateau reducing learning rate to 7.812500371073838e-06.
Epoch 16/20
34/34 [==============================] - 5s 137ms/step - loss: 12.7997 - categorical_accuracy: 0.2059 - val_loss: 12.4109 - val_categorical_accuracy: 0.2300
Epoch 00016: saving model to model_init_2018-10-2121_17_53.160408/model-00016-12.79966-0.20588-12.41093-0.23000.h5
Epoch 17/20
34/34 [==============================] - 5s 142ms/step - loss: 11.5355 - categorical_accuracy: 0.2843 - val_loss: 12.4109 - val_categorical_accuracy: 0.2300
Epoch 00017: saving model to model_init_2018-10-2121_17_53.160408/model-00017-11.53550-0.28431-12.41093-0.23000.h5
Epoch 00017: ReduceLROnPlateau reducing learning rate to 3.906250185536919e-06.
Epoch 18/20
###Markdown
Model 1b : Resize to 120*120, agumentation, No flipped images, No cropping, No normalisation, No edge detection
###Code
train_gen = DataGenerator(affine=True)
val_gen = DataGenerator()
model_gen = ModelGenerator()
input_shape = (30,120,120, 3)
num_classes = 5
model = model_gen.c3d1(input_shape, num_classes)
#batch_size = 20
#num_epochs = 20
train_generator = train_gen.generator(train_path, train_doc, batch_size)
val_generator = val_gen.generator(val_path, val_doc, batch_size)
train(batch_size, num_epochs, model, train_generator, val_generator)
###Output
# training sequences = 663
# validation sequences = 100
# batch size = 20
# epochs = 20
_________________________________________________________________
Layer (type) Output Shape Param #
=================================================================
conv3d_1 (Conv3D) (None, 28, 118, 118, 8) 656
_________________________________________________________________
max_pooling3d_1 (MaxPooling3 (None, 28, 59, 59, 8) 0
_________________________________________________________________
conv3d_2 (Conv3D) (None, 26, 57, 57, 16) 3472
_________________________________________________________________
max_pooling3d_2 (MaxPooling3 (None, 26, 28, 28, 16) 0
_________________________________________________________________
conv3d_3 (Conv3D) (None, 24, 26, 26, 32) 13856
_________________________________________________________________
conv3d_4 (Conv3D) (None, 22, 24, 24, 32) 27680
_________________________________________________________________
max_pooling3d_3 (MaxPooling3 (None, 22, 12, 12, 32) 0
_________________________________________________________________
conv3d_5 (Conv3D) (None, 21, 11, 11, 64) 16448
_________________________________________________________________
conv3d_6 (Conv3D) (None, 20, 10, 10, 64) 32832
_________________________________________________________________
max_pooling3d_4 (MaxPooling3 (None, 20, 5, 5, 64) 0
_________________________________________________________________
flatten_1 (Flatten) (None, 32000) 0
_________________________________________________________________
dense_1 (Dense) (None, 512) 16384512
_________________________________________________________________
dropout_1 (Dropout) (None, 512) 0
_________________________________________________________________
dense_2 (Dense) (None, 256) 131328
_________________________________________________________________
dropout_2 (Dropout) (None, 256) 0
_________________________________________________________________
dense_3 (Dense) (None, 5) 1285
=================================================================
Total params: 16,612,069
Trainable params: 16,612,069
Non-trainable params: 0
_________________________________________________________________
None
Epoch 1/20
Source path = ./Project_data/val ; batch size = 20
Source path = ./Project_data/train ; batch size = 20
34/34 [==============================] - 31s 919ms/step - loss: 13.0202 - categorical_accuracy: 0.1917 - val_loss: 13.5392 - val_categorical_accuracy: 0.1600
Epoch 00001: saving model to model_init_2018-10-2121_19_44.687027/model-00001-13.00995-0.19231-13.53920-0.16000.h5
Epoch 2/20
34/34 [==============================] - 5s 140ms/step - loss: 13.2737 - categorical_accuracy: 0.1765 - val_loss: 13.5392 - val_categorical_accuracy: 0.1600
Epoch 00002: saving model to model_init_2018-10-2121_19_44.687027/model-00002-13.27373-0.17647-13.53920-0.16000.h5
Epoch 3/20
34/34 [==============================] - 6s 173ms/step - loss: 13.7478 - categorical_accuracy: 0.1471 - val_loss: 13.5392 - val_categorical_accuracy: 0.1600
Epoch 00003: saving model to model_init_2018-10-2121_19_44.687027/model-00003-13.74779-0.14706-13.53920-0.16000.h5
Epoch 00003: ReduceLROnPlateau reducing learning rate to 0.0005000000237487257.
Epoch 4/20
34/34 [==============================] - 5s 158ms/step - loss: 13.9848 - categorical_accuracy: 0.1324 - val_loss: 13.5392 - val_categorical_accuracy: 0.1600
Epoch 00004: saving model to model_init_2018-10-2121_19_44.687027/model-00004-13.98482-0.13235-13.53920-0.16000.h5
Epoch 5/20
34/34 [==============================] - 5s 160ms/step - loss: 13.6688 - categorical_accuracy: 0.1520 - val_loss: 13.5392 - val_categorical_accuracy: 0.1600
Epoch 00005: saving model to model_init_2018-10-2121_19_44.687027/model-00005-13.66878-0.15196-13.53920-0.16000.h5
Epoch 00005: ReduceLROnPlateau reducing learning rate to 0.0002500000118743628.
Epoch 6/20
34/34 [==============================] - 5s 150ms/step - loss: 13.1157 - categorical_accuracy: 0.1863 - val_loss: 13.5392 - val_categorical_accuracy: 0.1600
Epoch 00006: saving model to model_init_2018-10-2121_19_44.687027/model-00006-13.11571-0.18627-13.53920-0.16000.h5
Epoch 7/20
34/34 [==============================] - 5s 157ms/step - loss: 12.2466 - categorical_accuracy: 0.2402 - val_loss: 13.5392 - val_categorical_accuracy: 0.1600
Epoch 00007: saving model to model_init_2018-10-2121_19_44.687027/model-00007-12.24659-0.24020-13.53920-0.16000.h5
Epoch 00007: ReduceLROnPlateau reducing learning rate to 0.0001250000059371814.
Epoch 8/20
34/34 [==============================] - 5s 153ms/step - loss: 12.4046 - categorical_accuracy: 0.2304 - val_loss: 13.5392 - val_categorical_accuracy: 0.1600
Epoch 00008: saving model to model_init_2018-10-2121_19_44.687027/model-00008-12.40461-0.23039-13.53920-0.16000.h5
Epoch 9/20
34/34 [==============================] - 5s 150ms/step - loss: 13.5108 - categorical_accuracy: 0.1618 - val_loss: 13.5392 - val_categorical_accuracy: 0.1600
Epoch 00009: saving model to model_init_2018-10-2121_19_44.687027/model-00009-13.51076-0.16176-13.53920-0.16000.h5
Epoch 00009: ReduceLROnPlateau reducing learning rate to 6.25000029685907e-05.
Epoch 10/20
34/34 [==============================] - 5s 158ms/step - loss: 12.4836 - categorical_accuracy: 0.2255 - val_loss: 13.5392 - val_categorical_accuracy: 0.1600
Epoch 00010: saving model to model_init_2018-10-2121_19_44.687027/model-00010-12.48362-0.22549-13.53920-0.16000.h5
Epoch 11/20
34/34 [==============================] - 5s 134ms/step - loss: 13.7478 - categorical_accuracy: 0.1471 - val_loss: 13.5392 - val_categorical_accuracy: 0.1600
Epoch 00011: saving model to model_init_2018-10-2121_19_44.687027/model-00011-13.74779-0.14706-13.53920-0.16000.h5
Epoch 00011: ReduceLROnPlateau reducing learning rate to 3.125000148429535e-05.
Epoch 12/20
34/34 [==============================] - 5s 137ms/step - loss: 13.5108 - categorical_accuracy: 0.1618 - val_loss: 13.5392 - val_categorical_accuracy: 0.1600
Epoch 00012: saving model to model_init_2018-10-2121_19_44.687027/model-00012-13.51076-0.16176-13.53920-0.16000.h5
Epoch 13/20
34/34 [==============================] - 5s 148ms/step - loss: 12.4046 - categorical_accuracy: 0.2304 - val_loss: 13.5392 - val_categorical_accuracy: 0.1600
Epoch 00013: saving model to model_init_2018-10-2121_19_44.687027/model-00013-12.40461-0.23039-13.53920-0.16000.h5
Epoch 00013: ReduceLROnPlateau reducing learning rate to 1.5625000742147677e-05.
Epoch 14/20
34/34 [==============================] - 5s 148ms/step - loss: 13.1157 - categorical_accuracy: 0.1863 - val_loss: 13.5392 - val_categorical_accuracy: 0.1600
Epoch 00014: saving model to model_init_2018-10-2121_19_44.687027/model-00014-13.11571-0.18627-13.53920-0.16000.h5
Epoch 15/20
34/34 [==============================] - 5s 152ms/step - loss: 12.7997 - categorical_accuracy: 0.2059 - val_loss: 13.5392 - val_categorical_accuracy: 0.1600
Epoch 00015: saving model to model_init_2018-10-2121_19_44.687027/model-00015-12.79966-0.20588-13.53920-0.16000.h5
Epoch 00015: ReduceLROnPlateau reducing learning rate to 7.812500371073838e-06.
Epoch 16/20
34/34 [==============================] - 5s 152ms/step - loss: 13.4317 - categorical_accuracy: 0.1667 - val_loss: 13.5392 - val_categorical_accuracy: 0.1600
Epoch 00016: saving model to model_init_2018-10-2121_19_44.687027/model-00016-13.43175-0.16667-13.53920-0.16000.h5
Epoch 17/20
34/34 [==============================] - 5s 150ms/step - loss: 13.0367 - categorical_accuracy: 0.1912 - val_loss: 13.5392 - val_categorical_accuracy: 0.1600
Epoch 00017: saving model to model_init_2018-10-2121_19_44.687027/model-00017-13.03669-0.19118-13.53920-0.16000.h5
Epoch 00017: ReduceLROnPlateau reducing learning rate to 3.906250185536919e-06.
Epoch 18/20
###Markdown
Model 1c : Resize to 120*120, agumentation, flipped images, No normalisation, No cropping, No edge detection
###Code
train_gen = DataGenerator(affine=True, flip=True)
val_gen = DataGenerator()
model_gen = ModelGenerator()
input_shape = (30,120,120, 3)
num_classes = 5
model = model_gen.c3d1(input_shape, num_classes)
#batch_size = 20
#num_epochs = 20
train_generator = train_gen.generator(train_path, train_doc, batch_size)
val_generator = val_gen.generator(val_path, val_doc, batch_size)
train(batch_size, num_epochs, model, train_generator, val_generator)
###Output
# training sequences = 663
# validation sequences = 100
# batch size = 20
# epochs = 20
_________________________________________________________________
Layer (type) Output Shape Param #
=================================================================
conv3d_1 (Conv3D) (None, 28, 118, 118, 8) 656
_________________________________________________________________
max_pooling3d_1 (MaxPooling3 (None, 28, 59, 59, 8) 0
_________________________________________________________________
conv3d_2 (Conv3D) (None, 26, 57, 57, 16) 3472
_________________________________________________________________
max_pooling3d_2 (MaxPooling3 (None, 26, 28, 28, 16) 0
_________________________________________________________________
conv3d_3 (Conv3D) (None, 24, 26, 26, 32) 13856
_________________________________________________________________
conv3d_4 (Conv3D) (None, 22, 24, 24, 32) 27680
_________________________________________________________________
max_pooling3d_3 (MaxPooling3 (None, 22, 12, 12, 32) 0
_________________________________________________________________
conv3d_5 (Conv3D) (None, 21, 11, 11, 64) 16448
_________________________________________________________________
conv3d_6 (Conv3D) (None, 20, 10, 10, 64) 32832
_________________________________________________________________
max_pooling3d_4 (MaxPooling3 (None, 20, 5, 5, 64) 0
_________________________________________________________________
flatten_1 (Flatten) (None, 32000) 0
_________________________________________________________________
dense_1 (Dense) (None, 512) 16384512
_________________________________________________________________
dropout_1 (Dropout) (None, 512) 0
_________________________________________________________________
dense_2 (Dense) (None, 256) 131328
_________________________________________________________________
dropout_2 (Dropout) (None, 256) 0
_________________________________________________________________
dense_3 (Dense) (None, 5) 1285
=================================================================
Total params: 16,612,069
Trainable params: 16,612,069
Non-trainable params: 0
_________________________________________________________________
None
Epoch 1/20
Source path = ./Project_data/val ; batch size = 20
Source path = ./Project_data/train ; batch size = 20
34/34 [==============================] - 37s 1s/step - loss: 12.8788 - categorical_accuracy: 0.2004 - val_loss: 12.5721 - val_categorical_accuracy: 0.2200
Epoch 00001: saving model to model_init_2018-10-2121_21_58.844546/model-00001-12.93189-0.19708-12.57211-0.22000.h5
Epoch 2/20
34/34 [==============================] - 5s 148ms/step - loss: 12.4836 - categorical_accuracy: 0.2255 - val_loss: 12.5721 - val_categorical_accuracy: 0.2200
Epoch 00002: saving model to model_init_2018-10-2121_21_58.844546/model-00002-12.48362-0.22549-12.57211-0.22000.h5
Epoch 3/20
34/34 [==============================] - 6s 175ms/step - loss: 11.6935 - categorical_accuracy: 0.2745 - val_loss: 12.5721 - val_categorical_accuracy: 0.2200
Epoch 00003: saving model to model_init_2018-10-2121_21_58.844546/model-00003-11.69352-0.27451-12.57211-0.22000.h5
Epoch 00003: ReduceLROnPlateau reducing learning rate to 0.0005000000237487257.
Epoch 4/20
34/34 [==============================] - 6s 181ms/step - loss: 13.4317 - categorical_accuracy: 0.1667 - val_loss: 12.5721 - val_categorical_accuracy: 0.2200
Epoch 00004: saving model to model_init_2018-10-2121_21_58.844546/model-00004-13.43175-0.16667-12.57211-0.22000.h5
Epoch 5/20
34/34 [==============================] - 6s 179ms/step - loss: 13.4317 - categorical_accuracy: 0.1667 - val_loss: 12.5721 - val_categorical_accuracy: 0.2200
Epoch 00005: saving model to model_init_2018-10-2121_21_58.844546/model-00005-13.43175-0.16667-12.57211-0.22000.h5
Epoch 00005: ReduceLROnPlateau reducing learning rate to 0.0002500000118743628.
Epoch 6/20
34/34 [==============================] - 6s 164ms/step - loss: 13.9058 - categorical_accuracy: 0.1373 - val_loss: 12.5721 - val_categorical_accuracy: 0.2200
Epoch 00006: saving model to model_init_2018-10-2121_21_58.844546/model-00006-13.90581-0.13725-12.57211-0.22000.h5
Epoch 7/20
34/34 [==============================] - 6s 178ms/step - loss: 13.1157 - categorical_accuracy: 0.1863 - val_loss: 12.5721 - val_categorical_accuracy: 0.2200
Epoch 00007: saving model to model_init_2018-10-2121_21_58.844546/model-00007-13.11570-0.18627-12.57211-0.22000.h5
Epoch 00007: ReduceLROnPlateau reducing learning rate to 0.0001250000059371814.
Epoch 8/20
34/34 [==============================] - 6s 183ms/step - loss: 13.2211 - categorical_accuracy: 0.1797 - val_loss: 12.5721 - val_categorical_accuracy: 0.2200
Epoch 00008: saving model to model_init_2018-10-2121_21_58.844546/model-00008-13.22105-0.17974-12.57211-0.22000.h5
Epoch 9/20
34/34 [==============================] - 6s 189ms/step - loss: 12.4836 - categorical_accuracy: 0.2255 - val_loss: 12.5721 - val_categorical_accuracy: 0.2200
Epoch 00009: saving model to model_init_2018-10-2121_21_58.844546/model-00009-12.48362-0.22549-12.57211-0.22000.h5
Epoch 00009: ReduceLROnPlateau reducing learning rate to 6.25000029685907e-05.
Epoch 10/20
34/34 [==============================] - 5s 143ms/step - loss: 12.7997 - categorical_accuracy: 0.2059 - val_loss: 12.5721 - val_categorical_accuracy: 0.2200
Epoch 00010: saving model to model_init_2018-10-2121_21_58.844546/model-00010-12.79966-0.20588-12.57211-0.22000.h5
Epoch 11/20
34/34 [==============================] - 5s 140ms/step - loss: 13.2737 - categorical_accuracy: 0.1765 - val_loss: 12.5721 - val_categorical_accuracy: 0.2200
Epoch 00011: saving model to model_init_2018-10-2121_21_58.844546/model-00011-13.27373-0.17647-12.57211-0.22000.h5
Epoch 00011: ReduceLROnPlateau reducing learning rate to 3.125000148429535e-05.
Epoch 12/20
34/34 [==============================] - 6s 172ms/step - loss: 12.9577 - categorical_accuracy: 0.1961 - val_loss: 12.5721 - val_categorical_accuracy: 0.2200
Epoch 00012: saving model to model_init_2018-10-2121_21_58.844546/model-00012-12.95768-0.19608-12.57211-0.22000.h5
Epoch 13/20
34/34 [==============================] - 6s 175ms/step - loss: 12.4309 - categorical_accuracy: 0.2288 - val_loss: 12.5721 - val_categorical_accuracy: 0.2200
Epoch 00013: saving model to model_init_2018-10-2121_21_58.844546/model-00013-12.43095-0.22876-12.57211-0.22000.h5
Epoch 00013: ReduceLROnPlateau reducing learning rate to 1.5625000742147677e-05.
Epoch 14/20
34/34 [==============================] - 6s 180ms/step - loss: 13.1157 - categorical_accuracy: 0.1863 - val_loss: 12.5721 - val_categorical_accuracy: 0.2200
Epoch 00014: saving model to model_init_2018-10-2121_21_58.844546/model-00014-13.11570-0.18627-12.57211-0.22000.h5
Epoch 15/20
34/34 [==============================] - 5s 156ms/step - loss: 13.5898 - categorical_accuracy: 0.1569 - val_loss: 12.5721 - val_categorical_accuracy: 0.2200
Epoch 00015: saving model to model_init_2018-10-2121_21_58.844546/model-00015-13.58977-0.15686-12.57211-0.22000.h5
Epoch 00015: ReduceLROnPlateau reducing learning rate to 7.812500371073838e-06.
Epoch 16/20
34/34 [==============================] - 6s 190ms/step - loss: 12.0096 - categorical_accuracy: 0.2549 - val_loss: 12.5721 - val_categorical_accuracy: 0.2200
Epoch 00016: saving model to model_init_2018-10-2121_21_58.844546/model-00016-12.00956-0.25490-12.57211-0.22000.h5
Epoch 17/20
34/34 [==============================] - 6s 178ms/step - loss: 12.4836 - categorical_accuracy: 0.2255 - val_loss: 12.5721 - val_categorical_accuracy: 0.2200
Epoch 00017: saving model to model_init_2018-10-2121_21_58.844546/model-00017-12.48362-0.22549-12.57211-0.22000.h5
Epoch 00017: ReduceLROnPlateau reducing learning rate to 3.906250185536919e-06.
Epoch 18/20
###Markdown
Model 1d : Resize to 120*120, agumentation, flipped images, normalisation, No cropping, No edge detection
###Code
train_gen = DataGenerator(affine=True, flip=True, normalize=True)
val_gen = DataGenerator()
model_gen = ModelGenerator()
input_shape = (30,120,120, 3)
num_classes = 5
model = model_gen.c3d1(input_shape, num_classes)
#batch_size = 20
#num_epochs = 20
train_generator = train_gen.generator(train_path, train_doc, batch_size)
val_generator = val_gen.generator(val_path, val_doc, batch_size)
train(batch_size, num_epochs, model, train_generator, val_generator)
###Output
# training sequences = 663
# validation sequences = 100
# batch size = 20
# epochs = 20
_________________________________________________________________
Layer (type) Output Shape Param #
=================================================================
conv3d_1 (Conv3D) (None, 28, 118, 118, 8) 656
_________________________________________________________________
max_pooling3d_1 (MaxPooling3 (None, 28, 59, 59, 8) 0
_________________________________________________________________
conv3d_2 (Conv3D) (None, 26, 57, 57, 16) 3472
_________________________________________________________________
max_pooling3d_2 (MaxPooling3 (None, 26, 28, 28, 16) 0
_________________________________________________________________
conv3d_3 (Conv3D) (None, 24, 26, 26, 32) 13856
_________________________________________________________________
conv3d_4 (Conv3D) (None, 22, 24, 24, 32) 27680
_________________________________________________________________
max_pooling3d_3 (MaxPooling3 (None, 22, 12, 12, 32) 0
_________________________________________________________________
conv3d_5 (Conv3D) (None, 21, 11, 11, 64) 16448
_________________________________________________________________
conv3d_6 (Conv3D) (None, 20, 10, 10, 64) 32832
_________________________________________________________________
max_pooling3d_4 (MaxPooling3 (None, 20, 5, 5, 64) 0
_________________________________________________________________
flatten_1 (Flatten) (None, 32000) 0
_________________________________________________________________
dense_1 (Dense) (None, 512) 16384512
_________________________________________________________________
dropout_1 (Dropout) (None, 512) 0
_________________________________________________________________
dense_2 (Dense) (None, 256) 131328
_________________________________________________________________
dropout_2 (Dropout) (None, 256) 0
_________________________________________________________________
dense_3 (Dense) (None, 5) 1285
=================================================================
Total params: 16,612,069
Trainable params: 16,612,069
Non-trainable params: 0
_________________________________________________________________
None
Epoch 1/20
Source path = ./Project_data/val ; batch size = 20
Source path = ./Project_data/train ; batch size = 20
34/34 [==============================] - 39s 1s/step - loss: 1.7442 - categorical_accuracy: 0.2198 - val_loss: 12.0548 - val_categorical_accuracy: 0.2300
Epoch 00001: saving model to model_init_2018-10-2121_24_30.405740/model-00001-1.74767-0.22524-12.05480-0.23000.h5
Epoch 2/20
34/34 [==============================] - 6s 184ms/step - loss: 1.5880 - categorical_accuracy: 0.2124 - val_loss: 12.5721 - val_categorical_accuracy: 0.2200
Epoch 00002: saving model to model_init_2018-10-2121_24_30.405740/model-00002-1.58803-0.21242-12.57211-0.22000.h5
Epoch 3/20
34/34 [==============================] - 6s 186ms/step - loss: 1.5356 - categorical_accuracy: 0.2908 - val_loss: 11.4677 - val_categorical_accuracy: 0.2300
Epoch 00003: saving model to model_init_2018-10-2121_24_30.405740/model-00003-1.53557-0.29085-11.46772-0.23000.h5
Epoch 4/20
34/34 [==============================] - 6s 189ms/step - loss: 1.6427 - categorical_accuracy: 0.2222 - val_loss: 10.6737 - val_categorical_accuracy: 0.2300
Epoch 00004: saving model to model_init_2018-10-2121_24_30.405740/model-00004-1.64266-0.22222-10.67373-0.23000.h5
Epoch 5/20
34/34 [==============================] - 7s 197ms/step - loss: 1.6390 - categorical_accuracy: 0.1242 - val_loss: 9.3224 - val_categorical_accuracy: 0.2400
Epoch 00005: saving model to model_init_2018-10-2121_24_30.405740/model-00005-1.63904-0.12418-9.32243-0.24000.h5
Epoch 6/20
34/34 [==============================] - 6s 179ms/step - loss: 1.6307 - categorical_accuracy: 0.1765 - val_loss: 9.6737 - val_categorical_accuracy: 0.2300
Epoch 00006: saving model to model_init_2018-10-2121_24_30.405740/model-00006-1.63074-0.17647-9.67374-0.23000.h5
Epoch 7/20
34/34 [==============================] - 7s 192ms/step - loss: 1.6149 - categorical_accuracy: 0.2026 - val_loss: 11.5835 - val_categorical_accuracy: 0.2300
Epoch 00007: saving model to model_init_2018-10-2121_24_30.405740/model-00007-1.61489-0.20261-11.58353-0.23000.h5
Epoch 00007: ReduceLROnPlateau reducing learning rate to 0.0005000000237487257.
Epoch 8/20
34/34 [==============================] - 6s 184ms/step - loss: 1.5933 - categorical_accuracy: 0.3301 - val_loss: 12.4109 - val_categorical_accuracy: 0.2300
Epoch 00008: saving model to model_init_2018-10-2121_24_30.405740/model-00008-1.59325-0.33007-12.41093-0.23000.h5
Epoch 9/20
34/34 [==============================] - 7s 196ms/step - loss: 1.4548 - categorical_accuracy: 0.4085 - val_loss: 12.4109 - val_categorical_accuracy: 0.2300
Epoch 00009: saving model to model_init_2018-10-2121_24_30.405740/model-00009-1.45480-0.40850-12.41093-0.23000.h5
Epoch 00009: ReduceLROnPlateau reducing learning rate to 0.0002500000118743628.
Epoch 10/20
34/34 [==============================] - 6s 164ms/step - loss: 1.2635 - categorical_accuracy: 0.3954 - val_loss: 12.4109 - val_categorical_accuracy: 0.2300
Epoch 00010: saving model to model_init_2018-10-2121_24_30.405740/model-00010-1.26352-0.39542-12.41093-0.23000.h5
Epoch 11/20
34/34 [==============================] - 7s 199ms/step - loss: 1.2204 - categorical_accuracy: 0.4510 - val_loss: 12.4109 - val_categorical_accuracy: 0.2300
Epoch 00011: saving model to model_init_2018-10-2121_24_30.405740/model-00011-1.22038-0.45098-12.41093-0.23000.h5
Epoch 00011: ReduceLROnPlateau reducing learning rate to 0.0001250000059371814.
Epoch 12/20
34/34 [==============================] - 7s 207ms/step - loss: 1.1795 - categorical_accuracy: 0.4444 - val_loss: 12.4109 - val_categorical_accuracy: 0.2300
Epoch 00012: saving model to model_init_2018-10-2121_24_30.405740/model-00012-1.17950-0.44444-12.41093-0.23000.h5
Epoch 13/20
34/34 [==============================] - 6s 181ms/step - loss: 1.2239 - categorical_accuracy: 0.4085 - val_loss: 11.8406 - val_categorical_accuracy: 0.2600
Epoch 00013: saving model to model_init_2018-10-2121_24_30.405740/model-00013-1.22386-0.40850-11.84062-0.26000.h5
Epoch 00013: ReduceLROnPlateau reducing learning rate to 6.25000029685907e-05.
Epoch 14/20
34/34 [==============================] - 6s 177ms/step - loss: 1.2276 - categorical_accuracy: 0.4542 - val_loss: 12.0886 - val_categorical_accuracy: 0.2500
Epoch 00014: saving model to model_init_2018-10-2121_24_30.405740/model-00014-1.22756-0.45425-12.08857-0.25000.h5
Epoch 15/20
34/34 [==============================] - 6s 189ms/step - loss: 1.1856 - categorical_accuracy: 0.4771 - val_loss: 11.6050 - val_categorical_accuracy: 0.2800
Epoch 00015: saving model to model_init_2018-10-2121_24_30.405740/model-00015-1.18559-0.47712-11.60503-0.28000.h5
Epoch 00015: ReduceLROnPlateau reducing learning rate to 3.125000148429535e-05.
Epoch 16/20
34/34 [==============================] - 7s 192ms/step - loss: 1.1229 - categorical_accuracy: 0.4739 - val_loss: 11.6050 - val_categorical_accuracy: 0.2800
Epoch 00016: saving model to model_init_2018-10-2121_24_30.405740/model-00016-1.12293-0.47386-11.60503-0.28000.h5
Epoch 17/20
34/34 [==============================] - 6s 186ms/step - loss: 1.0783 - categorical_accuracy: 0.5392 - val_loss: 11.9274 - val_categorical_accuracy: 0.2600
Epoch 00017: saving model to model_init_2018-10-2121_24_30.405740/model-00017-1.07835-0.53922-11.92739-0.26000.h5
Epoch 00017: ReduceLROnPlateau reducing learning rate to 1.5625000742147677e-05.
Epoch 18/20
34/34 [==============================] - 6s 188ms/step - loss: 1.1344 - categorical_accuracy: 0.5261 - val_loss: 11.9088 - val_categorical_accuracy: 0.2600
Epoch 00018: saving model to model_init_2018-10-2121_24_30.405740/model-00018-1.13436-0.52614-11.90885-0.26000.h5
Epoch 19/20
###Markdown
Model 1e : Resize to 120*120, agumentation, flipped images, normalisation, cropping, No edge detection
###Code
train_gen = DataGenerator(affine=True, flip=True, normalize=True, crop=True)
val_gen = DataGenerator()
model_gen = ModelGenerator()
input_shape = (30,120,120, 3)
num_classes = 5
model = model_gen.c3d1(input_shape, num_classes)
#batch_size = 20
#num_epochs = 20
train_generator = train_gen.generator(train_path, train_doc, batch_size)
val_generator = val_gen.generator(val_path, val_doc, batch_size)
train(batch_size, num_epochs, model, train_generator, val_generator)
###Output
# training sequences = 663
# validation sequences = 100
# batch size = 20
# epochs = 20
_________________________________________________________________
Layer (type) Output Shape Param #
=================================================================
conv3d_1 (Conv3D) (None, 28, 118, 118, 8) 656
_________________________________________________________________
max_pooling3d_1 (MaxPooling3 (None, 28, 59, 59, 8) 0
_________________________________________________________________
conv3d_2 (Conv3D) (None, 26, 57, 57, 16) 3472
_________________________________________________________________
max_pooling3d_2 (MaxPooling3 (None, 26, 28, 28, 16) 0
_________________________________________________________________
conv3d_3 (Conv3D) (None, 24, 26, 26, 32) 13856
_________________________________________________________________
conv3d_4 (Conv3D) (None, 22, 24, 24, 32) 27680
_________________________________________________________________
max_pooling3d_3 (MaxPooling3 (None, 22, 12, 12, 32) 0
_________________________________________________________________
conv3d_5 (Conv3D) (None, 21, 11, 11, 64) 16448
_________________________________________________________________
conv3d_6 (Conv3D) (None, 20, 10, 10, 64) 32832
_________________________________________________________________
max_pooling3d_4 (MaxPooling3 (None, 20, 5, 5, 64) 0
_________________________________________________________________
flatten_1 (Flatten) (None, 32000) 0
_________________________________________________________________
dense_1 (Dense) (None, 512) 16384512
_________________________________________________________________
dropout_1 (Dropout) (None, 512) 0
_________________________________________________________________
dense_2 (Dense) (None, 256) 131328
_________________________________________________________________
dropout_2 (Dropout) (None, 256) 0
_________________________________________________________________
dense_3 (Dense) (None, 5) 1285
=================================================================
Total params: 16,612,069
Trainable params: 16,612,069
Non-trainable params: 0
_________________________________________________________________
None
Epoch 1/20
Source path = ./Project_data/train ; batch size = 20
Source path = ./Project_data/val ; batch size = 20
34/34 [==============================] - 40s 1s/step - loss: 1.7331 - categorical_accuracy: 0.2205 - val_loss: 12.3378 - val_categorical_accuracy: 0.2300
Epoch 00001: saving model to model_init_2018-10-2121_27_14.268054/model-00001-1.74043-0.22323-12.33778-0.23000.h5
Epoch 2/20
34/34 [==============================] - 6s 183ms/step - loss: 1.5517 - categorical_accuracy: 0.3072 - val_loss: 12.4109 - val_categorical_accuracy: 0.2300
Epoch 00002: saving model to model_init_2018-10-2121_27_14.268054/model-00002-1.55169-0.30719-12.41093-0.23000.h5
Epoch 3/20
34/34 [==============================] - 6s 178ms/step - loss: 1.6571 - categorical_accuracy: 0.1765 - val_loss: 11.2436 - val_categorical_accuracy: 0.2400
Epoch 00003: saving model to model_init_2018-10-2121_27_14.268054/model-00003-1.65714-0.17647-11.24361-0.24000.h5
Epoch 4/20
34/34 [==============================] - 6s 182ms/step - loss: 1.6184 - categorical_accuracy: 0.1765 - val_loss: 11.1183 - val_categorical_accuracy: 0.2600
Epoch 00004: saving model to model_init_2018-10-2121_27_14.268054/model-00004-1.61836-0.17647-11.11832-0.26000.h5
Epoch 5/20
34/34 [==============================] - 7s 206ms/step - loss: 1.6157 - categorical_accuracy: 0.1961 - val_loss: 11.1666 - val_categorical_accuracy: 0.2300
Epoch 00005: saving model to model_init_2018-10-2121_27_14.268054/model-00005-1.61573-0.19608-11.16660-0.23000.h5
Epoch 6/20
34/34 [==============================] - 7s 211ms/step - loss: 1.6203 - categorical_accuracy: 0.1634 - val_loss: 11.1758 - val_categorical_accuracy: 0.2400
Epoch 00006: saving model to model_init_2018-10-2121_27_14.268054/model-00006-1.62033-0.16340-11.17583-0.24000.h5
Epoch 00006: ReduceLROnPlateau reducing learning rate to 0.0005000000237487257.
Epoch 7/20
34/34 [==============================] - 7s 191ms/step - loss: 1.6150 - categorical_accuracy: 0.1928 - val_loss: 11.4916 - val_categorical_accuracy: 0.2200
Epoch 00007: saving model to model_init_2018-10-2121_27_14.268054/model-00007-1.61502-0.19281-11.49160-0.22000.h5
Epoch 8/20
34/34 [==============================] - 7s 205ms/step - loss: 1.6206 - categorical_accuracy: 0.1765 - val_loss: 11.4358 - val_categorical_accuracy: 0.2200
Epoch 00008: saving model to model_init_2018-10-2121_27_14.268054/model-00008-1.62063-0.17647-11.43583-0.22000.h5
Epoch 00008: ReduceLROnPlateau reducing learning rate to 0.0002500000118743628.
Epoch 9/20
34/34 [==============================] - 7s 200ms/step - loss: 1.6136 - categorical_accuracy: 0.2124 - val_loss: 11.2736 - val_categorical_accuracy: 0.2300
Epoch 00009: saving model to model_init_2018-10-2121_27_14.268054/model-00009-1.61364-0.21242-11.27357-0.23000.h5
Epoch 10/20
34/34 [==============================] - 7s 193ms/step - loss: 1.6045 - categorical_accuracy: 0.2124 - val_loss: 11.2576 - val_categorical_accuracy: 0.2300
Epoch 00010: saving model to model_init_2018-10-2121_27_14.268054/model-00010-1.60449-0.21242-11.25761-0.23000.h5
Epoch 00010: ReduceLROnPlateau reducing learning rate to 0.0001250000059371814.
Epoch 11/20
34/34 [==============================] - 6s 187ms/step - loss: 1.6120 - categorical_accuracy: 0.1993 - val_loss: 11.3519 - val_categorical_accuracy: 0.2200
Epoch 00011: saving model to model_init_2018-10-2121_27_14.268054/model-00011-1.61196-0.19935-11.35191-0.22000.h5
Epoch 12/20
34/34 [==============================] - 6s 188ms/step - loss: 1.6021 - categorical_accuracy: 0.2516 - val_loss: 11.4623 - val_categorical_accuracy: 0.2200
Epoch 00012: saving model to model_init_2018-10-2121_27_14.268054/model-00012-1.60209-0.25163-11.46227-0.22000.h5
Epoch 00012: ReduceLROnPlateau reducing learning rate to 6.25000029685907e-05.
Epoch 13/20
34/34 [==============================] - 7s 195ms/step - loss: 1.6083 - categorical_accuracy: 0.2418 - val_loss: 11.4505 - val_categorical_accuracy: 0.2200
Epoch 00013: saving model to model_init_2018-10-2121_27_14.268054/model-00013-1.60830-0.24183-11.45048-0.22000.h5
Epoch 14/20
34/34 [==============================] - 7s 201ms/step - loss: 1.6194 - categorical_accuracy: 0.1373 - val_loss: 11.4397 - val_categorical_accuracy: 0.2200
Epoch 00014: saving model to model_init_2018-10-2121_27_14.268054/model-00014-1.61939-0.13725-11.43970-0.22000.h5
Epoch 00014: ReduceLROnPlateau reducing learning rate to 3.125000148429535e-05.
Epoch 15/20
34/34 [==============================] - 7s 206ms/step - loss: 1.6137 - categorical_accuracy: 0.1667 - val_loss: 11.4535 - val_categorical_accuracy: 0.2200
Epoch 00015: saving model to model_init_2018-10-2121_27_14.268054/model-00015-1.61369-0.16667-11.45353-0.22000.h5
Epoch 16/20
34/34 [==============================] - 7s 193ms/step - loss: 1.6103 - categorical_accuracy: 0.1667 - val_loss: 11.4629 - val_categorical_accuracy: 0.2200
Epoch 00016: saving model to model_init_2018-10-2121_27_14.268054/model-00016-1.61032-0.16667-11.46293-0.22000.h5
Epoch 00016: ReduceLROnPlateau reducing learning rate to 1.5625000742147677e-05.
Epoch 17/20
34/34 [==============================] - 6s 190ms/step - loss: 1.6115 - categorical_accuracy: 0.2124 - val_loss: 11.4629 - val_categorical_accuracy: 0.2200
Epoch 00017: saving model to model_init_2018-10-2121_27_14.268054/model-00017-1.61154-0.21242-11.46287-0.22000.h5
Epoch 18/20
34/34 [==============================] - 6s 190ms/step - loss: 1.5983 - categorical_accuracy: 0.2451 - val_loss: 11.4675 - val_categorical_accuracy: 0.2200
Epoch 00018: saving model to model_init_2018-10-2121_27_14.268054/model-00018-1.59826-0.24510-11.46753-0.22000.h5
Epoch 00018: ReduceLROnPlateau reducing learning rate to 7.812500371073838e-06.
Epoch 19/20
###Markdown
Model 1f : Resize to 120*120, agumentation, flipped images, normalisation, cropping, edge detection
###Code
train_gen = DataGenerator(affine=True, flip=True, normalize=True, crop=True, edge=True)
val_gen = DataGenerator()
model_gen = ModelGenerator()
input_shape = (30,120,120, 3)
num_classes = 5
model = model_gen.c3d1(input_shape, num_classes)
#batch_size = 20
#num_epochs = 20
train_generator = train_gen.generator(train_path, train_doc, batch_size)
val_generator = val_gen.generator(val_path, val_doc, batch_size)
train(batch_size, num_epochs, model, train_generator, val_generator)
###Output
# training sequences = 663
# validation sequences = 100
# batch size = 20
# epochs = 20
_________________________________________________________________
Layer (type) Output Shape Param #
=================================================================
conv3d_1 (Conv3D) (None, 28, 118, 118, 8) 656
_________________________________________________________________
max_pooling3d_1 (MaxPooling3 (None, 28, 59, 59, 8) 0
_________________________________________________________________
conv3d_2 (Conv3D) (None, 26, 57, 57, 16) 3472
_________________________________________________________________
max_pooling3d_2 (MaxPooling3 (None, 26, 28, 28, 16) 0
_________________________________________________________________
conv3d_3 (Conv3D) (None, 24, 26, 26, 32) 13856
_________________________________________________________________
conv3d_4 (Conv3D) (None, 22, 24, 24, 32) 27680
_________________________________________________________________
max_pooling3d_3 (MaxPooling3 (None, 22, 12, 12, 32) 0
_________________________________________________________________
conv3d_5 (Conv3D) (None, 21, 11, 11, 64) 16448
_________________________________________________________________
conv3d_6 (Conv3D) (None, 20, 10, 10, 64) 32832
_________________________________________________________________
max_pooling3d_4 (MaxPooling3 (None, 20, 5, 5, 64) 0
_________________________________________________________________
flatten_1 (Flatten) (None, 32000) 0
_________________________________________________________________
dense_1 (Dense) (None, 512) 16384512
_________________________________________________________________
dropout_1 (Dropout) (None, 512) 0
_________________________________________________________________
dense_2 (Dense) (None, 256) 131328
_________________________________________________________________
dropout_2 (Dropout) (None, 256) 0
_________________________________________________________________
dense_3 (Dense) (None, 5) 1285
=================================================================
Total params: 16,612,069
Trainable params: 16,612,069
Non-trainable params: 0
_________________________________________________________________
None
Epoch 1/20
Source path = ./Project_data/train ; batch size = 20
Source path = ./Project_data/val ; batch size = 20
34/34 [==============================] - 45s 1s/step - loss: 1.6431 - categorical_accuracy: 0.2217 - val_loss: 12.5721 - val_categorical_accuracy: 0.2200
Epoch 00001: saving model to model_init_2018-10-2121_30_03.823329/model-00001-1.64619-0.22725-12.57211-0.22000.h5
Epoch 2/20
34/34 [==============================] - 7s 195ms/step - loss: 1.5410 - categorical_accuracy: 0.2843 - val_loss: 11.8811 - val_categorical_accuracy: 0.2400
Epoch 00002: saving model to model_init_2018-10-2121_30_03.823329/model-00002-1.54099-0.28431-11.88110-0.24000.h5
Epoch 3/20
34/34 [==============================] - 7s 209ms/step - loss: 1.6156 - categorical_accuracy: 0.1863 - val_loss: 12.5721 - val_categorical_accuracy: 0.2200
Epoch 00003: saving model to model_init_2018-10-2121_30_03.823329/model-00003-1.61563-0.18627-12.57211-0.22000.h5
Epoch 4/20
34/34 [==============================] - 7s 200ms/step - loss: 1.5456 - categorical_accuracy: 0.2320 - val_loss: 12.4109 - val_categorical_accuracy: 0.2300
Epoch 00004: saving model to model_init_2018-10-2121_30_03.823329/model-00004-1.54556-0.23203-12.41093-0.23000.h5
Epoch 00004: ReduceLROnPlateau reducing learning rate to 0.0005000000237487257.
Epoch 5/20
34/34 [==============================] - 7s 204ms/step - loss: 1.3928 - categorical_accuracy: 0.4020 - val_loss: 12.2498 - val_categorical_accuracy: 0.2400
Epoch 00005: saving model to model_init_2018-10-2121_30_03.823329/model-00005-1.39285-0.40196-12.24975-0.24000.h5
Epoch 6/20
34/34 [==============================] - 8s 221ms/step - loss: 1.3561 - categorical_accuracy: 0.4248 - val_loss: 12.2498 - val_categorical_accuracy: 0.2400
Epoch 00006: saving model to model_init_2018-10-2121_30_03.823329/model-00006-1.35607-0.42484-12.24975-0.24000.h5
Epoch 00006: ReduceLROnPlateau reducing learning rate to 0.0002500000118743628.
Epoch 7/20
34/34 [==============================] - 7s 214ms/step - loss: 1.2937 - categorical_accuracy: 0.4183 - val_loss: 11.3834 - val_categorical_accuracy: 0.2800
Epoch 00007: saving model to model_init_2018-10-2121_30_03.823329/model-00007-1.29371-0.41830-11.38340-0.28000.h5
Epoch 8/20
34/34 [==============================] - 7s 218ms/step - loss: 1.4249 - categorical_accuracy: 0.3627 - val_loss: 12.2498 - val_categorical_accuracy: 0.2400
Epoch 00008: saving model to model_init_2018-10-2121_30_03.823329/model-00008-1.42494-0.36275-12.24975-0.24000.h5
Epoch 9/20
34/34 [==============================] - 7s 216ms/step - loss: 1.2871 - categorical_accuracy: 0.4248 - val_loss: 11.2827 - val_categorical_accuracy: 0.3000
Epoch 00009: saving model to model_init_2018-10-2121_30_03.823329/model-00009-1.28715-0.42484-11.28267-0.30000.h5
Epoch 10/20
34/34 [==============================] - 7s 202ms/step - loss: 1.1282 - categorical_accuracy: 0.5098 - val_loss: 11.2827 - val_categorical_accuracy: 0.3000
Epoch 00010: saving model to model_init_2018-10-2121_30_03.823329/model-00010-1.12815-0.50980-11.28267-0.30000.h5
Epoch 11/20
34/34 [==============================] - 7s 217ms/step - loss: 1.2406 - categorical_accuracy: 0.4739 - val_loss: 11.9274 - val_categorical_accuracy: 0.2600
Epoch 00011: saving model to model_init_2018-10-2121_30_03.823329/model-00011-1.24063-0.47386-11.92739-0.26000.h5
Epoch 00011: ReduceLROnPlateau reducing learning rate to 0.0001250000059371814.
Epoch 12/20
34/34 [==============================] - 7s 216ms/step - loss: 1.1081 - categorical_accuracy: 0.5425 - val_loss: 11.9274 - val_categorical_accuracy: 0.2600
Epoch 00012: saving model to model_init_2018-10-2121_30_03.823329/model-00012-1.10813-0.54248-11.92739-0.26000.h5
Epoch 13/20
34/34 [==============================] - 8s 222ms/step - loss: 1.1064 - categorical_accuracy: 0.5425 - val_loss: 11.2827 - val_categorical_accuracy: 0.3000
Epoch 00013: saving model to model_init_2018-10-2121_30_03.823329/model-00013-1.10638-0.54248-11.28267-0.30000.h5
Epoch 00013: ReduceLROnPlateau reducing learning rate to 6.25000029685907e-05.
Epoch 14/20
34/34 [==============================] - 7s 199ms/step - loss: 1.0275 - categorical_accuracy: 0.5490 - val_loss: 11.2827 - val_categorical_accuracy: 0.3000
Epoch 00014: saving model to model_init_2018-10-2121_30_03.823329/model-00014-1.02754-0.54902-11.28267-0.30000.h5
Epoch 15/20
34/34 [==============================] - 7s 209ms/step - loss: 1.0274 - categorical_accuracy: 0.5719 - val_loss: 11.2827 - val_categorical_accuracy: 0.3000
Epoch 00015: saving model to model_init_2018-10-2121_30_03.823329/model-00015-1.02739-0.57190-11.28267-0.30000.h5
Epoch 00015: ReduceLROnPlateau reducing learning rate to 3.125000148429535e-05.
Epoch 16/20
34/34 [==============================] - 7s 214ms/step - loss: 1.0453 - categorical_accuracy: 0.5556 - val_loss: 11.1457 - val_categorical_accuracy: 0.3000
Epoch 00016: saving model to model_init_2018-10-2121_30_03.823329/model-00016-1.04525-0.55556-11.14572-0.30000.h5
Epoch 17/20
34/34 [==============================] - 7s 195ms/step - loss: 0.9928 - categorical_accuracy: 0.6405 - val_loss: 11.2827 - val_categorical_accuracy: 0.3000
Epoch 00017: saving model to model_init_2018-10-2121_30_03.823329/model-00017-0.99281-0.64052-11.28267-0.30000.h5
Epoch 18/20
34/34 [==============================] - 7s 215ms/step - loss: 0.9497 - categorical_accuracy: 0.6111 - val_loss: 11.2827 - val_categorical_accuracy: 0.3000
Epoch 00018: saving model to model_init_2018-10-2121_30_03.823329/model-00018-0.94970-0.61111-11.28267-0.30000.h5
Epoch 00018: ReduceLROnPlateau reducing learning rate to 1.5625000742147677e-05.
Epoch 19/20
###Markdown
Model 2 Model 2a : Resize to 120*120, No agumentation, No flipped images, No normalisation, No cropping, No edge detection
###Code
train_gen = DataGenerator()
val_gen = DataGenerator()
model_gen = ModelGenerator()
input_shape = (30,120,120, 3)
num_classes = 5
model = model_gen.c3d2(input_shape, num_classes)
#batch_size = 20
#num_epochs = 20
train_generator = train_gen.generator(train_path, train_doc, batch_size)
val_generator = val_gen.generator(val_path, val_doc, batch_size)
train(batch_size, num_epochs, model, train_generator, val_generator)
###Output
/home/santhosh/anaconda3/envs/tensor36/lib/python3.6/site-packages/ipykernel_launcher.py:44: UserWarning: Update your `Conv3D` call to the Keras 2 API: `Conv3D(16, (3, 3, 3), activation="relu", padding="same", name="conv1", input_shape=(30, 120, ..., strides=(1, 1, 1))`
/home/santhosh/anaconda3/envs/tensor36/lib/python3.6/site-packages/ipykernel_launcher.py:46: UserWarning: Update your `MaxPooling3D` call to the Keras 2 API: `MaxPooling3D(pool_size=(1, 2, 2), strides=(1, 2, 2), name="pool1", padding="valid")`
/home/santhosh/anaconda3/envs/tensor36/lib/python3.6/site-packages/ipykernel_launcher.py:50: UserWarning: Update your `Conv3D` call to the Keras 2 API: `Conv3D(32, (3, 3, 3), activation="relu", padding="same", name="conv2", strides=(1, 1, 1))`
/home/santhosh/anaconda3/envs/tensor36/lib/python3.6/site-packages/ipykernel_launcher.py:52: UserWarning: Update your `MaxPooling3D` call to the Keras 2 API: `MaxPooling3D(pool_size=(2, 2, 2), strides=(2, 2, 2), name="pool2", padding="valid")`
/home/santhosh/anaconda3/envs/tensor36/lib/python3.6/site-packages/ipykernel_launcher.py:56: UserWarning: Update your `Conv3D` call to the Keras 2 API: `Conv3D(64, (3, 3, 3), activation="relu", padding="same", name="conv3a", strides=(1, 1, 1))`
/home/santhosh/anaconda3/envs/tensor36/lib/python3.6/site-packages/ipykernel_launcher.py:59: UserWarning: Update your `Conv3D` call to the Keras 2 API: `Conv3D(64, (3, 3, 3), activation="relu", padding="same", name="conv3b", strides=(1, 1, 1))`
/home/santhosh/anaconda3/envs/tensor36/lib/python3.6/site-packages/ipykernel_launcher.py:61: UserWarning: Update your `MaxPooling3D` call to the Keras 2 API: `MaxPooling3D(pool_size=(2, 2, 2), strides=(2, 2, 2), name="pool3", padding="valid")`
/home/santhosh/anaconda3/envs/tensor36/lib/python3.6/site-packages/ipykernel_launcher.py:65: UserWarning: Update your `Conv3D` call to the Keras 2 API: `Conv3D(128, (3, 3, 3), activation="relu", padding="same", name="conv4a", strides=(1, 1, 1))`
/home/santhosh/anaconda3/envs/tensor36/lib/python3.6/site-packages/ipykernel_launcher.py:68: UserWarning: Update your `Conv3D` call to the Keras 2 API: `Conv3D(128, (3, 3, 3), activation="relu", padding="same", name="conv4b", strides=(1, 1, 1))`
/home/santhosh/anaconda3/envs/tensor36/lib/python3.6/site-packages/ipykernel_launcher.py:70: UserWarning: Update your `MaxPooling3D` call to the Keras 2 API: `MaxPooling3D(pool_size=(2, 2, 2), strides=(2, 2, 2), name="pool4", padding="valid")`
/home/santhosh/anaconda3/envs/tensor36/lib/python3.6/site-packages/ipykernel_launcher.py:75: UserWarning: Update your `Conv3D` call to the Keras 2 API: `Conv3D(256, (3, 3, 3), activation="relu", padding="same", name="conv5a", strides=(1, 1, 1))`
/home/santhosh/anaconda3/envs/tensor36/lib/python3.6/site-packages/ipykernel_launcher.py:78: UserWarning: Update your `Conv3D` call to the Keras 2 API: `Conv3D(256, (3, 3, 3), activation="relu", padding="same", name="conv5b", strides=(1, 1, 1))`
###Markdown
Model 2b : Resize to 120*120, agumentation, No flipped images, No normalisation, No cropping, No edge detection
###Code
train_gen = DataGenerator(affine=True)
val_gen = DataGenerator()
model_gen = ModelGenerator()
input_shape = (30,120,120, 3)
num_classes = 5
model = model_gen.c3d2(input_shape, num_classes)
#batch_size = 20
#num_epochs = 20
train_generator = train_gen.generator(train_path, train_doc, batch_size)
val_generator = val_gen.generator(val_path, val_doc, batch_size)
train(batch_size, num_epochs, model, train_generator, val_generator)
###Output
/home/santhosh/anaconda3/envs/tensor36/lib/python3.6/site-packages/ipykernel_launcher.py:44: UserWarning: Update your `Conv3D` call to the Keras 2 API: `Conv3D(16, (3, 3, 3), activation="relu", padding="same", name="conv1", input_shape=(30, 120, ..., strides=(1, 1, 1))`
/home/santhosh/anaconda3/envs/tensor36/lib/python3.6/site-packages/ipykernel_launcher.py:46: UserWarning: Update your `MaxPooling3D` call to the Keras 2 API: `MaxPooling3D(pool_size=(1, 2, 2), strides=(1, 2, 2), name="pool1", padding="valid")`
/home/santhosh/anaconda3/envs/tensor36/lib/python3.6/site-packages/ipykernel_launcher.py:50: UserWarning: Update your `Conv3D` call to the Keras 2 API: `Conv3D(32, (3, 3, 3), activation="relu", padding="same", name="conv2", strides=(1, 1, 1))`
/home/santhosh/anaconda3/envs/tensor36/lib/python3.6/site-packages/ipykernel_launcher.py:52: UserWarning: Update your `MaxPooling3D` call to the Keras 2 API: `MaxPooling3D(pool_size=(2, 2, 2), strides=(2, 2, 2), name="pool2", padding="valid")`
/home/santhosh/anaconda3/envs/tensor36/lib/python3.6/site-packages/ipykernel_launcher.py:56: UserWarning: Update your `Conv3D` call to the Keras 2 API: `Conv3D(64, (3, 3, 3), activation="relu", padding="same", name="conv3a", strides=(1, 1, 1))`
/home/santhosh/anaconda3/envs/tensor36/lib/python3.6/site-packages/ipykernel_launcher.py:59: UserWarning: Update your `Conv3D` call to the Keras 2 API: `Conv3D(64, (3, 3, 3), activation="relu", padding="same", name="conv3b", strides=(1, 1, 1))`
/home/santhosh/anaconda3/envs/tensor36/lib/python3.6/site-packages/ipykernel_launcher.py:61: UserWarning: Update your `MaxPooling3D` call to the Keras 2 API: `MaxPooling3D(pool_size=(2, 2, 2), strides=(2, 2, 2), name="pool3", padding="valid")`
/home/santhosh/anaconda3/envs/tensor36/lib/python3.6/site-packages/ipykernel_launcher.py:65: UserWarning: Update your `Conv3D` call to the Keras 2 API: `Conv3D(128, (3, 3, 3), activation="relu", padding="same", name="conv4a", strides=(1, 1, 1))`
/home/santhosh/anaconda3/envs/tensor36/lib/python3.6/site-packages/ipykernel_launcher.py:68: UserWarning: Update your `Conv3D` call to the Keras 2 API: `Conv3D(128, (3, 3, 3), activation="relu", padding="same", name="conv4b", strides=(1, 1, 1))`
/home/santhosh/anaconda3/envs/tensor36/lib/python3.6/site-packages/ipykernel_launcher.py:70: UserWarning: Update your `MaxPooling3D` call to the Keras 2 API: `MaxPooling3D(pool_size=(2, 2, 2), strides=(2, 2, 2), name="pool4", padding="valid")`
/home/santhosh/anaconda3/envs/tensor36/lib/python3.6/site-packages/ipykernel_launcher.py:75: UserWarning: Update your `Conv3D` call to the Keras 2 API: `Conv3D(256, (3, 3, 3), activation="relu", padding="same", name="conv5a", strides=(1, 1, 1))`
/home/santhosh/anaconda3/envs/tensor36/lib/python3.6/site-packages/ipykernel_launcher.py:78: UserWarning: Update your `Conv3D` call to the Keras 2 API: `Conv3D(256, (3, 3, 3), activation="relu", padding="same", name="conv5b", strides=(1, 1, 1))`
###Markdown
Model 2c : Resize to 120*120, agumentation, flipped images, No normalisation, No cropping, No edge detection
###Code
train_gen = DataGenerator(affine=True, flip=True)
val_gen = DataGenerator()
model_gen = ModelGenerator()
input_shape = (30,120,120, 3)
num_classes = 5
model = model_gen.c3d2(input_shape, num_classes)
#batch_size = 20
#num_epochs = 20
train_generator = train_gen.generator(train_path, train_doc, batch_size)
val_generator = val_gen.generator(val_path, val_doc, batch_size)
train(batch_size, num_epochs, model, train_generator, val_generator)
###Output
/home/santhosh/anaconda3/envs/tensor36/lib/python3.6/site-packages/ipykernel_launcher.py:44: UserWarning: Update your `Conv3D` call to the Keras 2 API: `Conv3D(16, (3, 3, 3), activation="relu", padding="same", name="conv1", input_shape=(30, 120, ..., strides=(1, 1, 1))`
/home/santhosh/anaconda3/envs/tensor36/lib/python3.6/site-packages/ipykernel_launcher.py:46: UserWarning: Update your `MaxPooling3D` call to the Keras 2 API: `MaxPooling3D(pool_size=(1, 2, 2), strides=(1, 2, 2), name="pool1", padding="valid")`
/home/santhosh/anaconda3/envs/tensor36/lib/python3.6/site-packages/ipykernel_launcher.py:50: UserWarning: Update your `Conv3D` call to the Keras 2 API: `Conv3D(32, (3, 3, 3), activation="relu", padding="same", name="conv2", strides=(1, 1, 1))`
/home/santhosh/anaconda3/envs/tensor36/lib/python3.6/site-packages/ipykernel_launcher.py:52: UserWarning: Update your `MaxPooling3D` call to the Keras 2 API: `MaxPooling3D(pool_size=(2, 2, 2), strides=(2, 2, 2), name="pool2", padding="valid")`
/home/santhosh/anaconda3/envs/tensor36/lib/python3.6/site-packages/ipykernel_launcher.py:56: UserWarning: Update your `Conv3D` call to the Keras 2 API: `Conv3D(64, (3, 3, 3), activation="relu", padding="same", name="conv3a", strides=(1, 1, 1))`
/home/santhosh/anaconda3/envs/tensor36/lib/python3.6/site-packages/ipykernel_launcher.py:59: UserWarning: Update your `Conv3D` call to the Keras 2 API: `Conv3D(64, (3, 3, 3), activation="relu", padding="same", name="conv3b", strides=(1, 1, 1))`
/home/santhosh/anaconda3/envs/tensor36/lib/python3.6/site-packages/ipykernel_launcher.py:61: UserWarning: Update your `MaxPooling3D` call to the Keras 2 API: `MaxPooling3D(pool_size=(2, 2, 2), strides=(2, 2, 2), name="pool3", padding="valid")`
/home/santhosh/anaconda3/envs/tensor36/lib/python3.6/site-packages/ipykernel_launcher.py:65: UserWarning: Update your `Conv3D` call to the Keras 2 API: `Conv3D(128, (3, 3, 3), activation="relu", padding="same", name="conv4a", strides=(1, 1, 1))`
/home/santhosh/anaconda3/envs/tensor36/lib/python3.6/site-packages/ipykernel_launcher.py:68: UserWarning: Update your `Conv3D` call to the Keras 2 API: `Conv3D(128, (3, 3, 3), activation="relu", padding="same", name="conv4b", strides=(1, 1, 1))`
/home/santhosh/anaconda3/envs/tensor36/lib/python3.6/site-packages/ipykernel_launcher.py:70: UserWarning: Update your `MaxPooling3D` call to the Keras 2 API: `MaxPooling3D(pool_size=(2, 2, 2), strides=(2, 2, 2), name="pool4", padding="valid")`
/home/santhosh/anaconda3/envs/tensor36/lib/python3.6/site-packages/ipykernel_launcher.py:75: UserWarning: Update your `Conv3D` call to the Keras 2 API: `Conv3D(256, (3, 3, 3), activation="relu", padding="same", name="conv5a", strides=(1, 1, 1))`
/home/santhosh/anaconda3/envs/tensor36/lib/python3.6/site-packages/ipykernel_launcher.py:78: UserWarning: Update your `Conv3D` call to the Keras 2 API: `Conv3D(256, (3, 3, 3), activation="relu", padding="same", name="conv5b", strides=(1, 1, 1))`
###Markdown
Model 2d : Resize to 120*120, agumentation, flipped images, normalisation, No cropping, No edge detection
###Code
train_gen = DataGenerator(affine=True, flip=True, normalize=True)
val_gen = DataGenerator()
model_gen = ModelGenerator()
input_shape = (30,120,120, 3)
num_classes = 5
model = model_gen.c3d2(input_shape, num_classes)
#batch_size = 20
#num_epochs = 20
train_generator = train_gen.generator(train_path, train_doc, batch_size)
val_generator = val_gen.generator(val_path, val_doc, batch_size)
train(batch_size, num_epochs, model, train_generator, val_generator)
###Output
/home/santhosh/anaconda3/envs/tensor36/lib/python3.6/site-packages/ipykernel_launcher.py:44: UserWarning: Update your `Conv3D` call to the Keras 2 API: `Conv3D(16, (3, 3, 3), activation="relu", padding="same", name="conv1", input_shape=(30, 120, ..., strides=(1, 1, 1))`
/home/santhosh/anaconda3/envs/tensor36/lib/python3.6/site-packages/ipykernel_launcher.py:46: UserWarning: Update your `MaxPooling3D` call to the Keras 2 API: `MaxPooling3D(pool_size=(1, 2, 2), strides=(1, 2, 2), name="pool1", padding="valid")`
/home/santhosh/anaconda3/envs/tensor36/lib/python3.6/site-packages/ipykernel_launcher.py:50: UserWarning: Update your `Conv3D` call to the Keras 2 API: `Conv3D(32, (3, 3, 3), activation="relu", padding="same", name="conv2", strides=(1, 1, 1))`
/home/santhosh/anaconda3/envs/tensor36/lib/python3.6/site-packages/ipykernel_launcher.py:52: UserWarning: Update your `MaxPooling3D` call to the Keras 2 API: `MaxPooling3D(pool_size=(2, 2, 2), strides=(2, 2, 2), name="pool2", padding="valid")`
/home/santhosh/anaconda3/envs/tensor36/lib/python3.6/site-packages/ipykernel_launcher.py:56: UserWarning: Update your `Conv3D` call to the Keras 2 API: `Conv3D(64, (3, 3, 3), activation="relu", padding="same", name="conv3a", strides=(1, 1, 1))`
/home/santhosh/anaconda3/envs/tensor36/lib/python3.6/site-packages/ipykernel_launcher.py:59: UserWarning: Update your `Conv3D` call to the Keras 2 API: `Conv3D(64, (3, 3, 3), activation="relu", padding="same", name="conv3b", strides=(1, 1, 1))`
/home/santhosh/anaconda3/envs/tensor36/lib/python3.6/site-packages/ipykernel_launcher.py:61: UserWarning: Update your `MaxPooling3D` call to the Keras 2 API: `MaxPooling3D(pool_size=(2, 2, 2), strides=(2, 2, 2), name="pool3", padding="valid")`
/home/santhosh/anaconda3/envs/tensor36/lib/python3.6/site-packages/ipykernel_launcher.py:65: UserWarning: Update your `Conv3D` call to the Keras 2 API: `Conv3D(128, (3, 3, 3), activation="relu", padding="same", name="conv4a", strides=(1, 1, 1))`
/home/santhosh/anaconda3/envs/tensor36/lib/python3.6/site-packages/ipykernel_launcher.py:68: UserWarning: Update your `Conv3D` call to the Keras 2 API: `Conv3D(128, (3, 3, 3), activation="relu", padding="same", name="conv4b", strides=(1, 1, 1))`
/home/santhosh/anaconda3/envs/tensor36/lib/python3.6/site-packages/ipykernel_launcher.py:70: UserWarning: Update your `MaxPooling3D` call to the Keras 2 API: `MaxPooling3D(pool_size=(2, 2, 2), strides=(2, 2, 2), name="pool4", padding="valid")`
/home/santhosh/anaconda3/envs/tensor36/lib/python3.6/site-packages/ipykernel_launcher.py:75: UserWarning: Update your `Conv3D` call to the Keras 2 API: `Conv3D(256, (3, 3, 3), activation="relu", padding="same", name="conv5a", strides=(1, 1, 1))`
/home/santhosh/anaconda3/envs/tensor36/lib/python3.6/site-packages/ipykernel_launcher.py:78: UserWarning: Update your `Conv3D` call to the Keras 2 API: `Conv3D(256, (3, 3, 3), activation="relu", padding="same", name="conv5b", strides=(1, 1, 1))`
###Markdown
Model 2e : Resize to 120*120, agumentation, flipped images, normalisation, cropping, No edge detection
###Code
train_gen = DataGenerator(affine=True, flip=True, normalize=True, crop=True)
val_gen = DataGenerator()
model_gen = ModelGenerator()
input_shape = (30,120,120, 3)
num_classes = 5
model = model_gen.c3d2(input_shape, num_classes)
#batch_size = 20
#num_epochs = 20
train_generator = train_gen.generator(train_path, train_doc, batch_size)
val_generator = val_gen.generator(val_path, val_doc, batch_size)
train(batch_size, num_epochs, model, train_generator, val_generator)
###Output
/home/santhosh/anaconda3/envs/tensor36/lib/python3.6/site-packages/ipykernel_launcher.py:44: UserWarning: Update your `Conv3D` call to the Keras 2 API: `Conv3D(16, (3, 3, 3), activation="relu", padding="same", name="conv1", input_shape=(30, 120, ..., strides=(1, 1, 1))`
/home/santhosh/anaconda3/envs/tensor36/lib/python3.6/site-packages/ipykernel_launcher.py:46: UserWarning: Update your `MaxPooling3D` call to the Keras 2 API: `MaxPooling3D(pool_size=(1, 2, 2), strides=(1, 2, 2), name="pool1", padding="valid")`
/home/santhosh/anaconda3/envs/tensor36/lib/python3.6/site-packages/ipykernel_launcher.py:50: UserWarning: Update your `Conv3D` call to the Keras 2 API: `Conv3D(32, (3, 3, 3), activation="relu", padding="same", name="conv2", strides=(1, 1, 1))`
/home/santhosh/anaconda3/envs/tensor36/lib/python3.6/site-packages/ipykernel_launcher.py:52: UserWarning: Update your `MaxPooling3D` call to the Keras 2 API: `MaxPooling3D(pool_size=(2, 2, 2), strides=(2, 2, 2), name="pool2", padding="valid")`
/home/santhosh/anaconda3/envs/tensor36/lib/python3.6/site-packages/ipykernel_launcher.py:56: UserWarning: Update your `Conv3D` call to the Keras 2 API: `Conv3D(64, (3, 3, 3), activation="relu", padding="same", name="conv3a", strides=(1, 1, 1))`
/home/santhosh/anaconda3/envs/tensor36/lib/python3.6/site-packages/ipykernel_launcher.py:59: UserWarning: Update your `Conv3D` call to the Keras 2 API: `Conv3D(64, (3, 3, 3), activation="relu", padding="same", name="conv3b", strides=(1, 1, 1))`
/home/santhosh/anaconda3/envs/tensor36/lib/python3.6/site-packages/ipykernel_launcher.py:61: UserWarning: Update your `MaxPooling3D` call to the Keras 2 API: `MaxPooling3D(pool_size=(2, 2, 2), strides=(2, 2, 2), name="pool3", padding="valid")`
/home/santhosh/anaconda3/envs/tensor36/lib/python3.6/site-packages/ipykernel_launcher.py:65: UserWarning: Update your `Conv3D` call to the Keras 2 API: `Conv3D(128, (3, 3, 3), activation="relu", padding="same", name="conv4a", strides=(1, 1, 1))`
/home/santhosh/anaconda3/envs/tensor36/lib/python3.6/site-packages/ipykernel_launcher.py:68: UserWarning: Update your `Conv3D` call to the Keras 2 API: `Conv3D(128, (3, 3, 3), activation="relu", padding="same", name="conv4b", strides=(1, 1, 1))`
/home/santhosh/anaconda3/envs/tensor36/lib/python3.6/site-packages/ipykernel_launcher.py:70: UserWarning: Update your `MaxPooling3D` call to the Keras 2 API: `MaxPooling3D(pool_size=(2, 2, 2), strides=(2, 2, 2), name="pool4", padding="valid")`
/home/santhosh/anaconda3/envs/tensor36/lib/python3.6/site-packages/ipykernel_launcher.py:75: UserWarning: Update your `Conv3D` call to the Keras 2 API: `Conv3D(256, (3, 3, 3), activation="relu", padding="same", name="conv5a", strides=(1, 1, 1))`
/home/santhosh/anaconda3/envs/tensor36/lib/python3.6/site-packages/ipykernel_launcher.py:78: UserWarning: Update your `Conv3D` call to the Keras 2 API: `Conv3D(256, (3, 3, 3), activation="relu", padding="same", name="conv5b", strides=(1, 1, 1))`
###Markdown
Model 2f : Resize to 120*120, agumentation, flipped images, normalisation, cropping, edge detection
###Code
train_gen = DataGenerator(affine=True, flip=True, normalize=True, crop=True, edge=True)
val_gen = DataGenerator()
model_gen = ModelGenerator()
input_shape = (30,120,120, 3)
num_classes = 5
model = model_gen.c3d2(input_shape, num_classes)
#batch_size = 20
#num_epochs = 20
train_generator = train_gen.generator(train_path, train_doc, batch_size)
val_generator = val_gen.generator(val_path, val_doc, batch_size)
train(batch_size, num_epochs, model, train_generator, val_generator)
###Output
/home/santhosh/anaconda3/envs/tensor36/lib/python3.6/site-packages/ipykernel_launcher.py:44: UserWarning: Update your `Conv3D` call to the Keras 2 API: `Conv3D(16, (3, 3, 3), activation="relu", padding="same", name="conv1", input_shape=(30, 120, ..., strides=(1, 1, 1))`
/home/santhosh/anaconda3/envs/tensor36/lib/python3.6/site-packages/ipykernel_launcher.py:46: UserWarning: Update your `MaxPooling3D` call to the Keras 2 API: `MaxPooling3D(pool_size=(1, 2, 2), strides=(1, 2, 2), name="pool1", padding="valid")`
/home/santhosh/anaconda3/envs/tensor36/lib/python3.6/site-packages/ipykernel_launcher.py:50: UserWarning: Update your `Conv3D` call to the Keras 2 API: `Conv3D(32, (3, 3, 3), activation="relu", padding="same", name="conv2", strides=(1, 1, 1))`
/home/santhosh/anaconda3/envs/tensor36/lib/python3.6/site-packages/ipykernel_launcher.py:52: UserWarning: Update your `MaxPooling3D` call to the Keras 2 API: `MaxPooling3D(pool_size=(2, 2, 2), strides=(2, 2, 2), name="pool2", padding="valid")`
/home/santhosh/anaconda3/envs/tensor36/lib/python3.6/site-packages/ipykernel_launcher.py:56: UserWarning: Update your `Conv3D` call to the Keras 2 API: `Conv3D(64, (3, 3, 3), activation="relu", padding="same", name="conv3a", strides=(1, 1, 1))`
/home/santhosh/anaconda3/envs/tensor36/lib/python3.6/site-packages/ipykernel_launcher.py:59: UserWarning: Update your `Conv3D` call to the Keras 2 API: `Conv3D(64, (3, 3, 3), activation="relu", padding="same", name="conv3b", strides=(1, 1, 1))`
/home/santhosh/anaconda3/envs/tensor36/lib/python3.6/site-packages/ipykernel_launcher.py:61: UserWarning: Update your `MaxPooling3D` call to the Keras 2 API: `MaxPooling3D(pool_size=(2, 2, 2), strides=(2, 2, 2), name="pool3", padding="valid")`
/home/santhosh/anaconda3/envs/tensor36/lib/python3.6/site-packages/ipykernel_launcher.py:65: UserWarning: Update your `Conv3D` call to the Keras 2 API: `Conv3D(128, (3, 3, 3), activation="relu", padding="same", name="conv4a", strides=(1, 1, 1))`
/home/santhosh/anaconda3/envs/tensor36/lib/python3.6/site-packages/ipykernel_launcher.py:68: UserWarning: Update your `Conv3D` call to the Keras 2 API: `Conv3D(128, (3, 3, 3), activation="relu", padding="same", name="conv4b", strides=(1, 1, 1))`
/home/santhosh/anaconda3/envs/tensor36/lib/python3.6/site-packages/ipykernel_launcher.py:70: UserWarning: Update your `MaxPooling3D` call to the Keras 2 API: `MaxPooling3D(pool_size=(2, 2, 2), strides=(2, 2, 2), name="pool4", padding="valid")`
/home/santhosh/anaconda3/envs/tensor36/lib/python3.6/site-packages/ipykernel_launcher.py:75: UserWarning: Update your `Conv3D` call to the Keras 2 API: `Conv3D(256, (3, 3, 3), activation="relu", padding="same", name="conv5a", strides=(1, 1, 1))`
/home/santhosh/anaconda3/envs/tensor36/lib/python3.6/site-packages/ipykernel_launcher.py:78: UserWarning: Update your `Conv3D` call to the Keras 2 API: `Conv3D(256, (3, 3, 3), activation="relu", padding="same", name="conv5b", strides=(1, 1, 1))`
###Markdown
Model 3 Model 3a : Resize to 120*120, No agumentation, No flipped images, No normalisation, No cropping, No edge detection
###Code
train_gen = DataGenerator()
val_gen = DataGenerator()
model_gen = ModelGenerator()
input_shape = (30,120,120, 3)
num_classes = 5
model = model_gen.c3d3(input_shape, num_classes)
#batch_size = 20
#num_epochs = 20
train_generator = train_gen.generator(train_path, train_doc, batch_size)
val_generator = val_gen.generator(val_path, val_doc, batch_size)
train(batch_size, num_epochs, model, train_generator, val_generator)
###Output
# training sequences = 663
# validation sequences = 100
# batch size = 20
# epochs = 20
_________________________________________________________________
Layer (type) Output Shape Param #
=================================================================
conv3d_1 (Conv3D) (None, 30, 120, 120, 16) 1312
_________________________________________________________________
activation_1 (Activation) (None, 30, 120, 120, 16) 0
_________________________________________________________________
conv3d_2 (Conv3D) (None, 30, 120, 120, 16) 6928
_________________________________________________________________
activation_2 (Activation) (None, 30, 120, 120, 16) 0
_________________________________________________________________
max_pooling3d_1 (MaxPooling3 (None, 10, 40, 40, 16) 0
_________________________________________________________________
dropout_1 (Dropout) (None, 10, 40, 40, 16) 0
_________________________________________________________________
conv3d_3 (Conv3D) (None, 10, 40, 40, 32) 13856
_________________________________________________________________
activation_3 (Activation) (None, 10, 40, 40, 32) 0
_________________________________________________________________
conv3d_4 (Conv3D) (None, 10, 40, 40, 32) 27680
_________________________________________________________________
activation_4 (Activation) (None, 10, 40, 40, 32) 0
_________________________________________________________________
max_pooling3d_2 (MaxPooling3 (None, 4, 14, 14, 32) 0
_________________________________________________________________
dropout_2 (Dropout) (None, 4, 14, 14, 32) 0
_________________________________________________________________
conv3d_5 (Conv3D) (None, 4, 14, 14, 32) 27680
_________________________________________________________________
activation_5 (Activation) (None, 4, 14, 14, 32) 0
_________________________________________________________________
conv3d_6 (Conv3D) (None, 4, 14, 14, 32) 27680
_________________________________________________________________
activation_6 (Activation) (None, 4, 14, 14, 32) 0
_________________________________________________________________
max_pooling3d_3 (MaxPooling3 (None, 2, 5, 5, 32) 0
_________________________________________________________________
dropout_3 (Dropout) (None, 2, 5, 5, 32) 0
_________________________________________________________________
flatten_1 (Flatten) (None, 1600) 0
_________________________________________________________________
dense_1 (Dense) (None, 512) 819712
_________________________________________________________________
batch_normalization_1 (Batch (None, 512) 2048
_________________________________________________________________
dropout_4 (Dropout) (None, 512) 0
_________________________________________________________________
dense_2 (Dense) (None, 5) 2565
=================================================================
Total params: 929,461
Trainable params: 928,437
Non-trainable params: 1,024
_________________________________________________________________
None
Epoch 1/20
Source path = ./Project_data/val ; batch size = 20
Source path = ./Project_data/train ; batch size = 20
34/34 [==============================] - 26s 760ms/step - loss: 2.0576 - categorical_accuracy: 0.2892 - val_loss: 5.0192 - val_categorical_accuracy: 0.2300
Epoch 00001: saving model to model_init_2018-10-2121_48_25.184784/model-00001-2.06901-0.28808-5.01923-0.23000.h5
Epoch 2/20
34/34 [==============================] - 4s 107ms/step - loss: 2.1142 - categorical_accuracy: 0.2941 - val_loss: 9.7407 - val_categorical_accuracy: 0.2300
Epoch 00002: saving model to model_init_2018-10-2121_48_25.184784/model-00002-2.11419-0.29412-9.74067-0.23000.h5
Epoch 3/20
34/34 [==============================] - 4s 112ms/step - loss: 2.2670 - categorical_accuracy: 0.2353 - val_loss: 3.2265 - val_categorical_accuracy: 0.1600
Epoch 00003: saving model to model_init_2018-10-2121_48_25.184784/model-00003-2.26705-0.23529-3.22650-0.16000.h5
Epoch 4/20
34/34 [==============================] - 4s 123ms/step - loss: 2.0248 - categorical_accuracy: 0.3529 - val_loss: 4.2291 - val_categorical_accuracy: 0.1600
Epoch 00004: saving model to model_init_2018-10-2121_48_25.184784/model-00004-2.02481-0.35294-4.22906-0.16000.h5
Epoch 5/20
34/34 [==============================] - 4s 122ms/step - loss: 1.7502 - categorical_accuracy: 0.2941 - val_loss: 2.1105 - val_categorical_accuracy: 0.3100
Epoch 00005: saving model to model_init_2018-10-2121_48_25.184784/model-00005-1.75018-0.29412-2.11048-0.31000.h5
Epoch 6/20
34/34 [==============================] - 4s 116ms/step - loss: 2.0282 - categorical_accuracy: 0.2647 - val_loss: 8.8249 - val_categorical_accuracy: 0.1700
Epoch 00006: saving model to model_init_2018-10-2121_48_25.184784/model-00006-2.02816-0.26471-8.82490-0.17000.h5
Epoch 7/20
34/34 [==============================] - 3s 102ms/step - loss: 1.9073 - categorical_accuracy: 0.3333 - val_loss: 3.4074 - val_categorical_accuracy: 0.2100
Epoch 00007: saving model to model_init_2018-10-2121_48_25.184784/model-00007-1.90728-0.33333-3.40737-0.21000.h5
Epoch 00007: ReduceLROnPlateau reducing learning rate to 0.0005000000237487257.
Epoch 8/20
34/34 [==============================] - 3s 103ms/step - loss: 1.9111 - categorical_accuracy: 0.3235 - val_loss: 1.9341 - val_categorical_accuracy: 0.2400
Epoch 00008: saving model to model_init_2018-10-2121_48_25.184784/model-00008-1.91108-0.32353-1.93409-0.24000.h5
Epoch 9/20
34/34 [==============================] - 5s 133ms/step - loss: 1.8167 - categorical_accuracy: 0.3235 - val_loss: 1.5979 - val_categorical_accuracy: 0.2500
Epoch 00009: saving model to model_init_2018-10-2121_48_25.184784/model-00009-1.81670-0.32353-1.59789-0.25000.h5
Epoch 10/20
34/34 [==============================] - 5s 135ms/step - loss: 1.6990 - categorical_accuracy: 0.3235 - val_loss: 1.6198 - val_categorical_accuracy: 0.2800
Epoch 00010: saving model to model_init_2018-10-2121_48_25.184784/model-00010-1.69901-0.32353-1.61977-0.28000.h5
Epoch 11/20
34/34 [==============================] - 5s 137ms/step - loss: 1.7620 - categorical_accuracy: 0.3529 - val_loss: 2.0583 - val_categorical_accuracy: 0.2200
Epoch 00011: saving model to model_init_2018-10-2121_48_25.184784/model-00011-1.76203-0.35294-2.05833-0.22000.h5
Epoch 00011: ReduceLROnPlateau reducing learning rate to 0.0002500000118743628.
Epoch 12/20
34/34 [==============================] - 5s 138ms/step - loss: 1.8838 - categorical_accuracy: 0.3137 - val_loss: 2.3716 - val_categorical_accuracy: 0.2200
Epoch 00012: saving model to model_init_2018-10-2121_48_25.184784/model-00012-1.88384-0.31373-2.37165-0.22000.h5
Epoch 13/20
34/34 [==============================] - 5s 134ms/step - loss: 1.7689 - categorical_accuracy: 0.3039 - val_loss: 3.0822 - val_categorical_accuracy: 0.2300
Epoch 00013: saving model to model_init_2018-10-2121_48_25.184784/model-00013-1.76893-0.30392-3.08225-0.23000.h5
Epoch 00013: ReduceLROnPlateau reducing learning rate to 0.0001250000059371814.
Epoch 14/20
34/34 [==============================] - 5s 137ms/step - loss: 1.8690 - categorical_accuracy: 0.2745 - val_loss: 1.9421 - val_categorical_accuracy: 0.2500
Epoch 00014: saving model to model_init_2018-10-2121_48_25.184784/model-00014-1.86902-0.27451-1.94209-0.25000.h5
Epoch 15/20
34/34 [==============================] - 5s 140ms/step - loss: 1.6192 - categorical_accuracy: 0.3725 - val_loss: 1.5335 - val_categorical_accuracy: 0.3400
Epoch 00015: saving model to model_init_2018-10-2121_48_25.184784/model-00015-1.61921-0.37255-1.53347-0.34000.h5
Epoch 16/20
34/34 [==============================] - 5s 138ms/step - loss: 1.7434 - categorical_accuracy: 0.3039 - val_loss: 1.4134 - val_categorical_accuracy: 0.4200
Epoch 00016: saving model to model_init_2018-10-2121_48_25.184784/model-00016-1.74336-0.30392-1.41338-0.42000.h5
Epoch 17/20
###Markdown
Model 3b : Resize to 120*120, agumentation, No flipped images, No normalisation, No cropping, No edge detection
###Code
train_gen = DataGenerator(affine=True)
val_gen = DataGenerator()
model_gen = ModelGenerator()
input_shape = (30,120,120, 3)
num_classes = 5
model = model_gen.c3d3(input_shape, num_classes)
#batch_size = 20
#num_epochs = 20
train_generator = train_gen.generator(train_path, train_doc, batch_size)
val_generator = val_gen.generator(val_path, val_doc, batch_size)
train(batch_size, num_epochs, model, train_generator, val_generator)
###Output
# training sequences = 663
# validation sequences = 100
# batch size = 20
# epochs = 20
_________________________________________________________________
Layer (type) Output Shape Param #
=================================================================
conv3d_1 (Conv3D) (None, 30, 120, 120, 16) 1312
_________________________________________________________________
activation_1 (Activation) (None, 30, 120, 120, 16) 0
_________________________________________________________________
conv3d_2 (Conv3D) (None, 30, 120, 120, 16) 6928
_________________________________________________________________
activation_2 (Activation) (None, 30, 120, 120, 16) 0
_________________________________________________________________
max_pooling3d_1 (MaxPooling3 (None, 10, 40, 40, 16) 0
_________________________________________________________________
dropout_1 (Dropout) (None, 10, 40, 40, 16) 0
_________________________________________________________________
conv3d_3 (Conv3D) (None, 10, 40, 40, 32) 13856
_________________________________________________________________
activation_3 (Activation) (None, 10, 40, 40, 32) 0
_________________________________________________________________
conv3d_4 (Conv3D) (None, 10, 40, 40, 32) 27680
_________________________________________________________________
activation_4 (Activation) (None, 10, 40, 40, 32) 0
_________________________________________________________________
max_pooling3d_2 (MaxPooling3 (None, 4, 14, 14, 32) 0
_________________________________________________________________
dropout_2 (Dropout) (None, 4, 14, 14, 32) 0
_________________________________________________________________
conv3d_5 (Conv3D) (None, 4, 14, 14, 32) 27680
_________________________________________________________________
activation_5 (Activation) (None, 4, 14, 14, 32) 0
_________________________________________________________________
conv3d_6 (Conv3D) (None, 4, 14, 14, 32) 27680
_________________________________________________________________
activation_6 (Activation) (None, 4, 14, 14, 32) 0
_________________________________________________________________
max_pooling3d_3 (MaxPooling3 (None, 2, 5, 5, 32) 0
_________________________________________________________________
dropout_3 (Dropout) (None, 2, 5, 5, 32) 0
_________________________________________________________________
flatten_1 (Flatten) (None, 1600) 0
_________________________________________________________________
dense_1 (Dense) (None, 512) 819712
_________________________________________________________________
batch_normalization_1 (Batch (None, 512) 2048
_________________________________________________________________
dropout_4 (Dropout) (None, 512) 0
_________________________________________________________________
dense_2 (Dense) (None, 5) 2565
=================================================================
Total params: 929,461
Trainable params: 928,437
Non-trainable params: 1,024
_________________________________________________________________
None
Epoch 1/20
Source path = ./Project_data/val ; batch size = 20
Source path = ./Project_data/train ; batch size = 20
34/34 [==============================] - 33s 968ms/step - loss: 2.0259 - categorical_accuracy: 0.3245 - val_loss: 6.9701 - val_categorical_accuracy: 0.2000
Epoch 00001: saving model to model_init_2018-10-2121_50_16.316011/model-00001-2.04276-0.31599-6.97008-0.20000.h5
Epoch 2/20
34/34 [==============================] - 5s 149ms/step - loss: 1.9068 - categorical_accuracy: 0.3137 - val_loss: 5.7957 - val_categorical_accuracy: 0.1800
Epoch 00002: saving model to model_init_2018-10-2121_50_16.316011/model-00002-1.90684-0.31373-5.79573-0.18000.h5
Epoch 3/20
34/34 [==============================] - 6s 168ms/step - loss: 2.2127 - categorical_accuracy: 0.2647 - val_loss: 2.2881 - val_categorical_accuracy: 0.3900
Epoch 00003: saving model to model_init_2018-10-2121_50_16.316011/model-00003-2.21270-0.26471-2.28813-0.39000.h5
Epoch 4/20
34/34 [==============================] - 5s 160ms/step - loss: 1.8377 - categorical_accuracy: 0.3431 - val_loss: 2.8585 - val_categorical_accuracy: 0.3400
Epoch 00004: saving model to model_init_2018-10-2121_50_16.316011/model-00004-1.83770-0.34314-2.85848-0.34000.h5
Epoch 5/20
34/34 [==============================] - 5s 159ms/step - loss: 1.8096 - categorical_accuracy: 0.3971 - val_loss: 2.7257 - val_categorical_accuracy: 0.3800
Epoch 00005: saving model to model_init_2018-10-2121_50_16.316011/model-00005-1.80965-0.39706-2.72570-0.38000.h5
Epoch 00005: ReduceLROnPlateau reducing learning rate to 0.0005000000237487257.
Epoch 6/20
34/34 [==============================] - 5s 148ms/step - loss: 1.6500 - categorical_accuracy: 0.3676 - val_loss: 1.1602 - val_categorical_accuracy: 0.5200
Epoch 00006: saving model to model_init_2018-10-2121_50_16.316011/model-00006-1.64996-0.36765-1.16015-0.52000.h5
Epoch 7/20
34/34 [==============================] - 6s 172ms/step - loss: 1.7321 - categorical_accuracy: 0.3971 - val_loss: 1.5239 - val_categorical_accuracy: 0.4000
Epoch 00007: saving model to model_init_2018-10-2121_50_16.316011/model-00007-1.73214-0.39706-1.52390-0.40000.h5
Epoch 8/20
34/34 [==============================] - 6s 169ms/step - loss: 1.5334 - categorical_accuracy: 0.3971 - val_loss: 2.0541 - val_categorical_accuracy: 0.3100
Epoch 00008: saving model to model_init_2018-10-2121_50_16.316011/model-00008-1.53341-0.39706-2.05407-0.31000.h5
Epoch 00008: ReduceLROnPlateau reducing learning rate to 0.0002500000118743628.
Epoch 9/20
34/34 [==============================] - 6s 164ms/step - loss: 1.4767 - categorical_accuracy: 0.4167 - val_loss: 1.7865 - val_categorical_accuracy: 0.3200
Epoch 00009: saving model to model_init_2018-10-2121_50_16.316011/model-00009-1.47665-0.41667-1.78645-0.32000.h5
Epoch 10/20
34/34 [==============================] - 5s 153ms/step - loss: 1.6362 - categorical_accuracy: 0.4118 - val_loss: 1.5283 - val_categorical_accuracy: 0.3500
Epoch 00010: saving model to model_init_2018-10-2121_50_16.316011/model-00010-1.63617-0.41176-1.52830-0.35000.h5
Epoch 00010: ReduceLROnPlateau reducing learning rate to 0.0001250000059371814.
Epoch 11/20
34/34 [==============================] - 6s 171ms/step - loss: 1.7075 - categorical_accuracy: 0.3775 - val_loss: 1.4677 - val_categorical_accuracy: 0.3800
Epoch 00011: saving model to model_init_2018-10-2121_50_16.316011/model-00011-1.70752-0.37745-1.46773-0.38000.h5
Epoch 12/20
34/34 [==============================] - 6s 180ms/step - loss: 1.7881 - categorical_accuracy: 0.2990 - val_loss: 1.1966 - val_categorical_accuracy: 0.4500
Epoch 00012: saving model to model_init_2018-10-2121_50_16.316011/model-00012-1.78812-0.29902-1.19657-0.45000.h5
Epoch 00012: ReduceLROnPlateau reducing learning rate to 6.25000029685907e-05.
Epoch 13/20
34/34 [==============================] - 6s 162ms/step - loss: 1.3688 - categorical_accuracy: 0.4706 - val_loss: 1.1351 - val_categorical_accuracy: 0.4800
Epoch 00013: saving model to model_init_2018-10-2121_50_16.316011/model-00013-1.36883-0.47059-1.13510-0.48000.h5
Epoch 14/20
34/34 [==============================] - 5s 158ms/step - loss: 1.3979 - categorical_accuracy: 0.4510 - val_loss: 1.1015 - val_categorical_accuracy: 0.5100
Epoch 00014: saving model to model_init_2018-10-2121_50_16.316011/model-00014-1.39792-0.45098-1.10147-0.51000.h5
Epoch 15/20
34/34 [==============================] - 5s 162ms/step - loss: 1.4141 - categorical_accuracy: 0.4314 - val_loss: 1.0760 - val_categorical_accuracy: 0.5000
Epoch 00015: saving model to model_init_2018-10-2121_50_16.316011/model-00015-1.41411-0.43137-1.07603-0.50000.h5
Epoch 16/20
###Markdown
Model 3c : Resize to 120*120, agumentation, flipped images, No normalisation, No cropping, No edge detection
###Code
train_gen = DataGenerator(affine=True, flip=True)
val_gen = DataGenerator()
model_gen = ModelGenerator()
input_shape = (30,120,120, 3)
num_classes = 5
model = model_gen.c3d3(input_shape, num_classes)
batch_size = 10
#num_epochs = 20
train_generator = train_gen.generator(train_path, train_doc, batch_size)
val_generator = val_gen.generator(val_path, val_doc, batch_size)
train(batch_size, num_epochs, model, train_generator, val_generator)
###Output
# training sequences = 663
# validation sequences = 100
# batch size = 10
# epochs = 20
_________________________________________________________________
Layer (type) Output Shape Param #
=================================================================
conv3d_1 (Conv3D) (None, 30, 120, 120, 16) 1312
_________________________________________________________________
activation_1 (Activation) (None, 30, 120, 120, 16) 0
_________________________________________________________________
conv3d_2 (Conv3D) (None, 30, 120, 120, 16) 6928
_________________________________________________________________
activation_2 (Activation) (None, 30, 120, 120, 16) 0
_________________________________________________________________
max_pooling3d_1 (MaxPooling3 (None, 10, 40, 40, 16) 0
_________________________________________________________________
dropout_1 (Dropout) (None, 10, 40, 40, 16) 0
_________________________________________________________________
conv3d_3 (Conv3D) (None, 10, 40, 40, 32) 13856
_________________________________________________________________
activation_3 (Activation) (None, 10, 40, 40, 32) 0
_________________________________________________________________
conv3d_4 (Conv3D) (None, 10, 40, 40, 32) 27680
_________________________________________________________________
activation_4 (Activation) (None, 10, 40, 40, 32) 0
_________________________________________________________________
max_pooling3d_2 (MaxPooling3 (None, 4, 14, 14, 32) 0
_________________________________________________________________
dropout_2 (Dropout) (None, 4, 14, 14, 32) 0
_________________________________________________________________
conv3d_5 (Conv3D) (None, 4, 14, 14, 32) 27680
_________________________________________________________________
activation_5 (Activation) (None, 4, 14, 14, 32) 0
_________________________________________________________________
conv3d_6 (Conv3D) (None, 4, 14, 14, 32) 27680
_________________________________________________________________
activation_6 (Activation) (None, 4, 14, 14, 32) 0
_________________________________________________________________
max_pooling3d_3 (MaxPooling3 (None, 2, 5, 5, 32) 0
_________________________________________________________________
dropout_3 (Dropout) (None, 2, 5, 5, 32) 0
_________________________________________________________________
flatten_1 (Flatten) (None, 1600) 0
_________________________________________________________________
dense_1 (Dense) (None, 512) 819712
_________________________________________________________________
batch_normalization_1 (Batch (None, 512) 2048
_________________________________________________________________
dropout_4 (Dropout) (None, 512) 0
_________________________________________________________________
dense_2 (Dense) (None, 5) 2565
=================================================================
Total params: 929,461
Trainable params: 928,437
Non-trainable params: 1,024
_________________________________________________________________
None
Epoch 1/20
Source path = Source path = ./Project_data/val ; batch size = 10
./Project_data/train ; batch size = 10
67/67 [==============================] - 39s 575ms/step - loss: 2.0557 - categorical_accuracy: 0.2962 - val_loss: 2.3495 - val_categorical_accuracy: 0.2500
Epoch 00001: saving model to model_init_2018-10-2121_52_37.183439/model-00001-2.05886-0.29462-2.34949-0.25000.h5
Epoch 2/20
67/67 [==============================] - 11s 164ms/step - loss: 1.9500 - categorical_accuracy: 0.3250 - val_loss: 3.8812 - val_categorical_accuracy: 0.2100
Epoch 00002: saving model to model_init_2018-10-2121_52_37.183439/model-00002-1.95003-0.32504-3.88119-0.21000.h5
Epoch 3/20
67/67 [==============================] - 11s 167ms/step - loss: 1.8074 - categorical_accuracy: 0.3449 - val_loss: 8.3546 - val_categorical_accuracy: 0.1800
Epoch 00003: saving model to model_init_2018-10-2121_52_37.183439/model-00003-1.80737-0.34494-8.35460-0.18000.h5
Epoch 00003: ReduceLROnPlateau reducing learning rate to 0.0005000000237487257.
Epoch 4/20
67/67 [==============================] - 12s 179ms/step - loss: 1.7849 - categorical_accuracy: 0.3068 - val_loss: 3.5334 - val_categorical_accuracy: 0.1500
Epoch 00004: saving model to model_init_2018-10-2121_52_37.183439/model-00004-1.78491-0.30680-3.53335-0.15000.h5
Epoch 5/20
67/67 [==============================] - 12s 183ms/step - loss: 1.5105 - categorical_accuracy: 0.3765 - val_loss: 1.1159 - val_categorical_accuracy: 0.4700
Epoch 00005: saving model to model_init_2018-10-2121_52_37.183439/model-00005-1.51054-0.37645-1.11590-0.47000.h5
Epoch 6/20
67/67 [==============================] - 12s 180ms/step - loss: 1.2289 - categorical_accuracy: 0.4942 - val_loss: 1.8201 - val_categorical_accuracy: 0.3600
Epoch 00006: saving model to model_init_2018-10-2121_52_37.183439/model-00006-1.22891-0.49420-1.82008-0.36000.h5
Epoch 7/20
67/67 [==============================] - 12s 174ms/step - loss: 1.3360 - categorical_accuracy: 0.4594 - val_loss: 1.1516 - val_categorical_accuracy: 0.5100
Epoch 00007: saving model to model_init_2018-10-2121_52_37.183439/model-00007-1.33595-0.45937-1.15156-0.51000.h5
Epoch 00007: ReduceLROnPlateau reducing learning rate to 0.0002500000118743628.
Epoch 8/20
67/67 [==============================] - 11s 171ms/step - loss: 1.3067 - categorical_accuracy: 0.4743 - val_loss: 1.1880 - val_categorical_accuracy: 0.4800
Epoch 00008: saving model to model_init_2018-10-2121_52_37.183439/model-00008-1.30667-0.47430-1.18796-0.48000.h5
Epoch 9/20
67/67 [==============================] - 12s 178ms/step - loss: 1.1481 - categorical_accuracy: 0.5207 - val_loss: 1.0318 - val_categorical_accuracy: 0.5800
Epoch 00009: saving model to model_init_2018-10-2121_52_37.183439/model-00009-1.14806-0.52073-1.03183-0.58000.h5
Epoch 10/20
67/67 [==============================] - 12s 175ms/step - loss: 1.0384 - categorical_accuracy: 0.5804 - val_loss: 1.0895 - val_categorical_accuracy: 0.5800
Epoch 00010: saving model to model_init_2018-10-2121_52_37.183439/model-00010-1.03841-0.58043-1.08951-0.58000.h5
Epoch 11/20
67/67 [==============================] - 12s 185ms/step - loss: 1.1862 - categorical_accuracy: 0.5141 - val_loss: 0.8389 - val_categorical_accuracy: 0.6600
Epoch 00011: saving model to model_init_2018-10-2121_52_37.183439/model-00011-1.18619-0.51410-0.83886-0.66000.h5
Epoch 12/20
67/67 [==============================] - 13s 197ms/step - loss: 0.9704 - categorical_accuracy: 0.6202 - val_loss: 0.7591 - val_categorical_accuracy: 0.6600
Epoch 00012: saving model to model_init_2018-10-2121_52_37.183439/model-00012-0.97039-0.62023-0.75907-0.66000.h5
Epoch 13/20
67/67 [==============================] - 14s 204ms/step - loss: 0.9577 - categorical_accuracy: 0.6186 - val_loss: 0.7943 - val_categorical_accuracy: 0.6800
Epoch 00013: saving model to model_init_2018-10-2121_52_37.183439/model-00013-0.95767-0.61857-0.79429-0.68000.h5
Epoch 14/20
67/67 [==============================] - 14s 211ms/step - loss: 0.9854 - categorical_accuracy: 0.5904 - val_loss: 0.8224 - val_categorical_accuracy: 0.6800
Epoch 00014: saving model to model_init_2018-10-2121_52_37.183439/model-00014-0.98541-0.59038-0.82243-0.68000.h5
Epoch 00014: ReduceLROnPlateau reducing learning rate to 0.0001250000059371814.
Epoch 15/20
67/67 [==============================] - 13s 200ms/step - loss: 0.7984 - categorical_accuracy: 0.6816 - val_loss: 0.7368 - val_categorical_accuracy: 0.7200
Epoch 00015: saving model to model_init_2018-10-2121_52_37.183439/model-00015-0.79843-0.68159-0.73682-0.72000.h5
Epoch 16/20
67/67 [==============================] - 14s 212ms/step - loss: 0.8576 - categorical_accuracy: 0.6667 - val_loss: 0.6656 - val_categorical_accuracy: 0.7500
###Markdown
Model 3d : Resize to 120*120, agumentation, flipped images, normalisation, No cropping, No edge detection
###Code
train_gen = DataGenerator(affine=True, flip=True, normalize=True)
val_gen = DataGenerator()
model_gen = ModelGenerator()
input_shape = (30,120,120, 3)
num_classes = 5
model = model_gen.c3d3(input_shape, num_classes)
#batch_size = 20
#num_epochs = 20
train_generator = train_gen.generator(train_path, train_doc, batch_size)
val_generator = val_gen.generator(val_path, val_doc, batch_size)
train(batch_size, num_epochs, model, train_generator, val_generator)
###Output
# training sequences = 663
# validation sequences = 100
# batch size = 10
# epochs = 20
_________________________________________________________________
Layer (type) Output Shape Param #
=================================================================
conv3d_1 (Conv3D) (None, 30, 120, 120, 16) 1312
_________________________________________________________________
activation_1 (Activation) (None, 30, 120, 120, 16) 0
_________________________________________________________________
conv3d_2 (Conv3D) (None, 30, 120, 120, 16) 6928
_________________________________________________________________
activation_2 (Activation) (None, 30, 120, 120, 16) 0
_________________________________________________________________
max_pooling3d_1 (MaxPooling3 (None, 10, 40, 40, 16) 0
_________________________________________________________________
dropout_1 (Dropout) (None, 10, 40, 40, 16) 0
_________________________________________________________________
conv3d_3 (Conv3D) (None, 10, 40, 40, 32) 13856
_________________________________________________________________
activation_3 (Activation) (None, 10, 40, 40, 32) 0
_________________________________________________________________
conv3d_4 (Conv3D) (None, 10, 40, 40, 32) 27680
_________________________________________________________________
activation_4 (Activation) (None, 10, 40, 40, 32) 0
_________________________________________________________________
max_pooling3d_2 (MaxPooling3 (None, 4, 14, 14, 32) 0
_________________________________________________________________
dropout_2 (Dropout) (None, 4, 14, 14, 32) 0
_________________________________________________________________
conv3d_5 (Conv3D) (None, 4, 14, 14, 32) 27680
_________________________________________________________________
activation_5 (Activation) (None, 4, 14, 14, 32) 0
_________________________________________________________________
conv3d_6 (Conv3D) (None, 4, 14, 14, 32) 27680
_________________________________________________________________
activation_6 (Activation) (None, 4, 14, 14, 32) 0
_________________________________________________________________
max_pooling3d_3 (MaxPooling3 (None, 2, 5, 5, 32) 0
_________________________________________________________________
dropout_3 (Dropout) (None, 2, 5, 5, 32) 0
_________________________________________________________________
flatten_1 (Flatten) (None, 1600) 0
_________________________________________________________________
dense_1 (Dense) (None, 512) 819712
_________________________________________________________________
batch_normalization_1 (Batch (None, 512) 2048
_________________________________________________________________
dropout_4 (Dropout) (None, 512) 0
_________________________________________________________________
dense_2 (Dense) (None, 5) 2565
=================================================================
Total params: 929,461
Trainable params: 928,437
Non-trainable params: 1,024
_________________________________________________________________
None
Epoch 1/20
Source path = ./Project_data/train ; batch size = 10
Source path = ./Project_data/val ; batch size = 10
67/67 [==============================] - 43s 647ms/step - loss: 1.6757 - categorical_accuracy: 0.3925 - val_loss: 10.1544 - val_categorical_accuracy: 0.3700
Epoch 00001: saving model to model_init_2018-10-2121_57_21.165248/model-00001-1.68678-0.38964-10.15440-0.37000.h5
Epoch 2/20
67/67 [==============================] - 13s 190ms/step - loss: 1.3581 - categorical_accuracy: 0.5008 - val_loss: 10.5852 - val_categorical_accuracy: 0.3200
Epoch 00002: saving model to model_init_2018-10-2121_57_21.165248/model-00002-1.35815-0.50083-10.58518-0.32000.h5
Epoch 3/20
67/67 [==============================] - 14s 206ms/step - loss: 1.1130 - categorical_accuracy: 0.5439 - val_loss: 9.5579 - val_categorical_accuracy: 0.4000
Epoch 00003: saving model to model_init_2018-10-2121_57_21.165248/model-00003-1.11302-0.54395-9.55792-0.40000.h5
Epoch 4/20
67/67 [==============================] - 14s 213ms/step - loss: 1.0168 - categorical_accuracy: 0.5589 - val_loss: 8.3289 - val_categorical_accuracy: 0.4400
Epoch 00004: saving model to model_init_2018-10-2121_57_21.165248/model-00004-1.01678-0.55887-8.32886-0.44000.h5
Epoch 5/20
67/67 [==============================] - 13s 198ms/step - loss: 0.9039 - categorical_accuracy: 0.5937 - val_loss: 8.8274 - val_categorical_accuracy: 0.4200
Epoch 00005: saving model to model_init_2018-10-2121_57_21.165248/model-00005-0.90390-0.59370-8.82735-0.42000.h5
Epoch 6/20
67/67 [==============================] - 14s 203ms/step - loss: 0.8796 - categorical_accuracy: 0.6202 - val_loss: 9.0585 - val_categorical_accuracy: 0.4200
Epoch 00006: saving model to model_init_2018-10-2121_57_21.165248/model-00006-0.87963-0.62023-9.05852-0.42000.h5
Epoch 00006: ReduceLROnPlateau reducing learning rate to 0.0005000000237487257.
Epoch 7/20
67/67 [==============================] - 13s 197ms/step - loss: 0.7924 - categorical_accuracy: 0.6584 - val_loss: 7.9558 - val_categorical_accuracy: 0.4700
Epoch 00007: saving model to model_init_2018-10-2121_57_21.165248/model-00007-0.79239-0.65837-7.95581-0.47000.h5
Epoch 8/20
67/67 [==============================] - 14s 209ms/step - loss: 0.7410 - categorical_accuracy: 0.6816 - val_loss: 9.5097 - val_categorical_accuracy: 0.4100
Epoch 00008: saving model to model_init_2018-10-2121_57_21.165248/model-00008-0.74104-0.68159-9.50968-0.41000.h5
Epoch 9/20
67/67 [==============================] - 14s 210ms/step - loss: 0.5998 - categorical_accuracy: 0.7446 - val_loss: 8.7440 - val_categorical_accuracy: 0.4500
Epoch 00009: saving model to model_init_2018-10-2121_57_21.165248/model-00009-0.59984-0.74461-8.74402-0.45000.h5
Epoch 00009: ReduceLROnPlateau reducing learning rate to 0.0002500000118743628.
Epoch 10/20
67/67 [==============================] - 14s 205ms/step - loss: 0.6721 - categorical_accuracy: 0.7164 - val_loss: 9.2423 - val_categorical_accuracy: 0.4200
Epoch 00010: saving model to model_init_2018-10-2121_57_21.165248/model-00010-0.67213-0.71642-9.24229-0.42000.h5
Epoch 11/20
67/67 [==============================] - 14s 206ms/step - loss: 0.5822 - categorical_accuracy: 0.7363 - val_loss: 9.5097 - val_categorical_accuracy: 0.4100
Epoch 00011: saving model to model_init_2018-10-2121_57_21.165248/model-00011-0.58217-0.73632-9.50968-0.41000.h5
Epoch 00011: ReduceLROnPlateau reducing learning rate to 0.0001250000059371814.
Epoch 12/20
67/67 [==============================] - 14s 214ms/step - loss: 0.5411 - categorical_accuracy: 0.7695 - val_loss: 9.1873 - val_categorical_accuracy: 0.4300
Epoch 00012: saving model to model_init_2018-10-2121_57_21.165248/model-00012-0.54110-0.76949-9.18731-0.43000.h5
Epoch 13/20
67/67 [==============================] - 13s 199ms/step - loss: 0.6722 - categorical_accuracy: 0.7330 - val_loss: 9.0261 - val_categorical_accuracy: 0.4400
Epoch 00013: saving model to model_init_2018-10-2121_57_21.165248/model-00013-0.67224-0.73300-9.02613-0.44000.h5
Epoch 00013: ReduceLROnPlateau reducing learning rate to 6.25000029685907e-05.
Epoch 14/20
67/67 [==============================] - 13s 196ms/step - loss: 0.5676 - categorical_accuracy: 0.7562 - val_loss: 9.1883 - val_categorical_accuracy: 0.4300
Epoch 00014: saving model to model_init_2018-10-2121_57_21.165248/model-00014-0.56760-0.75622-9.18834-0.43000.h5
Epoch 15/20
67/67 [==============================] - 14s 205ms/step - loss: 0.5467 - categorical_accuracy: 0.7678 - val_loss: 9.1274 - val_categorical_accuracy: 0.4300
Epoch 00015: saving model to model_init_2018-10-2121_57_21.165248/model-00015-0.54672-0.76783-9.12738-0.43000.h5
Epoch 00015: ReduceLROnPlateau reducing learning rate to 3.125000148429535e-05.
Epoch 16/20
###Markdown
Model 3e : Resize to 120*120, agumentation, flipped images, normalisation, cropping, No edge detection
###Code
train_gen = DataGenerator(affine=True, flip=True, normalize=True, crop=True)
val_gen = DataGenerator()
model_gen = ModelGenerator()
input_shape = (30,120,120, 3)
num_classes = 5
model = model_gen.c3d3(input_shape, num_classes)
#batch_size = 20
#num_epochs = 20
train_generator = train_gen.generator(train_path, train_doc, batch_size)
val_generator = val_gen.generator(val_path, val_doc, batch_size)
train(batch_size, num_epochs, model, train_generator, val_generator)
###Output
# training sequences = 663
# validation sequences = 100
# batch size = 10
# epochs = 20
_________________________________________________________________
Layer (type) Output Shape Param #
=================================================================
conv3d_1 (Conv3D) (None, 30, 120, 120, 16) 1312
_________________________________________________________________
activation_1 (Activation) (None, 30, 120, 120, 16) 0
_________________________________________________________________
conv3d_2 (Conv3D) (None, 30, 120, 120, 16) 6928
_________________________________________________________________
activation_2 (Activation) (None, 30, 120, 120, 16) 0
_________________________________________________________________
max_pooling3d_1 (MaxPooling3 (None, 10, 40, 40, 16) 0
_________________________________________________________________
dropout_1 (Dropout) (None, 10, 40, 40, 16) 0
_________________________________________________________________
conv3d_3 (Conv3D) (None, 10, 40, 40, 32) 13856
_________________________________________________________________
activation_3 (Activation) (None, 10, 40, 40, 32) 0
_________________________________________________________________
conv3d_4 (Conv3D) (None, 10, 40, 40, 32) 27680
_________________________________________________________________
activation_4 (Activation) (None, 10, 40, 40, 32) 0
_________________________________________________________________
max_pooling3d_2 (MaxPooling3 (None, 4, 14, 14, 32) 0
_________________________________________________________________
dropout_2 (Dropout) (None, 4, 14, 14, 32) 0
_________________________________________________________________
conv3d_5 (Conv3D) (None, 4, 14, 14, 32) 27680
_________________________________________________________________
activation_5 (Activation) (None, 4, 14, 14, 32) 0
_________________________________________________________________
conv3d_6 (Conv3D) (None, 4, 14, 14, 32) 27680
_________________________________________________________________
activation_6 (Activation) (None, 4, 14, 14, 32) 0
_________________________________________________________________
max_pooling3d_3 (MaxPooling3 (None, 2, 5, 5, 32) 0
_________________________________________________________________
dropout_3 (Dropout) (None, 2, 5, 5, 32) 0
_________________________________________________________________
flatten_1 (Flatten) (None, 1600) 0
_________________________________________________________________
dense_1 (Dense) (None, 512) 819712
_________________________________________________________________
batch_normalization_1 (Batch (None, 512) 2048
_________________________________________________________________
dropout_4 (Dropout) (None, 512) 0
_________________________________________________________________
dense_2 (Dense) (None, 5) 2565
=================================================================
Total params: 929,461
Trainable params: 928,437
Non-trainable params: 1,024
_________________________________________________________________
None
Epoch 1/20
Source path = Source path = ./Project_data/train ; batch size = 10
./Project_data/val ; batch size = 10
67/67 [==============================] - 44s 651ms/step - loss: 2.1600 - categorical_accuracy: 0.2600 - val_loss: 10.2617 - val_categorical_accuracy: 0.2200
Epoch 00001: saving model to model_init_2018-10-2122_02_27.244563/model-00001-2.16378-0.26043-10.26169-0.22000.h5
Epoch 2/20
67/67 [==============================] - 13s 200ms/step - loss: 2.1479 - categorical_accuracy: 0.2587 - val_loss: 12.4109 - val_categorical_accuracy: 0.2300
Epoch 00002: saving model to model_init_2018-10-2122_02_27.244563/model-00002-2.14792-0.25871-12.41093-0.23000.h5
Epoch 3/20
67/67 [==============================] - 13s 198ms/step - loss: 1.9318 - categorical_accuracy: 0.2703 - val_loss: 11.9274 - val_categorical_accuracy: 0.2600
Epoch 00003: saving model to model_init_2018-10-2122_02_27.244563/model-00003-1.93178-0.27032-11.92739-0.26000.h5
Epoch 00003: ReduceLROnPlateau reducing learning rate to 0.0005000000237487257.
Epoch 4/20
67/67 [==============================] - 12s 181ms/step - loss: 1.7600 - categorical_accuracy: 0.3118 - val_loss: 11.9274 - val_categorical_accuracy: 0.2600
Epoch 00004: saving model to model_init_2018-10-2122_02_27.244563/model-00004-1.75999-0.31177-11.92739-0.26000.h5
Epoch 5/20
67/67 [==============================] - 14s 205ms/step - loss: 1.6809 - categorical_accuracy: 0.3167 - val_loss: 12.2498 - val_categorical_accuracy: 0.2400
Epoch 00005: saving model to model_init_2018-10-2122_02_27.244563/model-00005-1.68094-0.31675-12.24975-0.24000.h5
Epoch 00005: ReduceLROnPlateau reducing learning rate to 0.0002500000118743628.
Epoch 6/20
67/67 [==============================] - 13s 200ms/step - loss: 1.5568 - categorical_accuracy: 0.3466 - val_loss: 12.4109 - val_categorical_accuracy: 0.2300
Epoch 00006: saving model to model_init_2018-10-2122_02_27.244563/model-00006-1.55684-0.34660-12.41093-0.23000.h5
Epoch 7/20
67/67 [==============================] - 14s 215ms/step - loss: 1.4909 - categorical_accuracy: 0.4196 - val_loss: 12.2498 - val_categorical_accuracy: 0.2400
Epoch 00007: saving model to model_init_2018-10-2122_02_27.244563/model-00007-1.49093-0.41957-12.24975-0.24000.h5
Epoch 00007: ReduceLROnPlateau reducing learning rate to 0.0001250000059371814.
Epoch 8/20
67/67 [==============================] - 13s 189ms/step - loss: 1.4987 - categorical_accuracy: 0.4030 - val_loss: 12.2498 - val_categorical_accuracy: 0.2400
Epoch 00008: saving model to model_init_2018-10-2122_02_27.244563/model-00008-1.49867-0.40299-12.24975-0.24000.h5
Epoch 9/20
67/67 [==============================] - 12s 184ms/step - loss: 1.4286 - categorical_accuracy: 0.4395 - val_loss: 12.2498 - val_categorical_accuracy: 0.2400
Epoch 00009: saving model to model_init_2018-10-2122_02_27.244563/model-00009-1.42857-0.43947-12.24975-0.24000.h5
Epoch 00009: ReduceLROnPlateau reducing learning rate to 6.25000029685907e-05.
Epoch 10/20
67/67 [==============================] - 14s 209ms/step - loss: 1.4077 - categorical_accuracy: 0.4345 - val_loss: 12.2498 - val_categorical_accuracy: 0.2400
Epoch 00010: saving model to model_init_2018-10-2122_02_27.244563/model-00010-1.40767-0.43449-12.24975-0.24000.h5
Epoch 11/20
67/67 [==============================] - 14s 204ms/step - loss: 1.4748 - categorical_accuracy: 0.3964 - val_loss: 12.2498 - val_categorical_accuracy: 0.2400
Epoch 00011: saving model to model_init_2018-10-2122_02_27.244563/model-00011-1.47483-0.39635-12.24975-0.24000.h5
Epoch 00011: ReduceLROnPlateau reducing learning rate to 3.125000148429535e-05.
Epoch 12/20
67/67 [==============================] - 12s 186ms/step - loss: 1.4274 - categorical_accuracy: 0.4262 - val_loss: 12.2498 - val_categorical_accuracy: 0.2400
Epoch 00012: saving model to model_init_2018-10-2122_02_27.244563/model-00012-1.42741-0.42620-12.24975-0.24000.h5
Epoch 13/20
67/67 [==============================] - 14s 202ms/step - loss: 1.3807 - categorical_accuracy: 0.4295 - val_loss: 12.2498 - val_categorical_accuracy: 0.2400
Epoch 00013: saving model to model_init_2018-10-2122_02_27.244563/model-00013-1.38071-0.42952-12.24975-0.24000.h5
Epoch 00013: ReduceLROnPlateau reducing learning rate to 1.5625000742147677e-05.
Epoch 14/20
67/67 [==============================] - 13s 201ms/step - loss: 1.4316 - categorical_accuracy: 0.4096 - val_loss: 12.2498 - val_categorical_accuracy: 0.2400
Epoch 00014: saving model to model_init_2018-10-2122_02_27.244563/model-00014-1.43159-0.40962-12.24975-0.24000.h5
Epoch 15/20
67/67 [==============================] - 14s 212ms/step - loss: 1.4203 - categorical_accuracy: 0.4179 - val_loss: 12.2498 - val_categorical_accuracy: 0.2400
###Markdown
Model 3f : Resize to 120*120, agumentation, flipped images, normalisation, cropping, edge detection
###Code
train_gen = DataGenerator(affine=True, flip=True, normalize=True, crop=True, edge=True)
val_gen = DataGenerator()
model_gen = ModelGenerator()
input_shape = (30,120,120, 3)
num_classes = 5
model = model_gen.c3d3(input_shape, num_classes)
#batch_size = 20
#num_epochs = 20
train_generator = train_gen.generator(train_path, train_doc, batch_size)
val_generator = val_gen.generator(val_path, val_doc, batch_size)
train(batch_size, num_epochs, model, train_generator, val_generator)
###Output
# training sequences = 663
# validation sequences = 100
# batch size = 10
# epochs = 20
_________________________________________________________________
Layer (type) Output Shape Param #
=================================================================
conv3d_1 (Conv3D) (None, 30, 120, 120, 16) 1312
_________________________________________________________________
activation_1 (Activation) (None, 30, 120, 120, 16) 0
_________________________________________________________________
conv3d_2 (Conv3D) (None, 30, 120, 120, 16) 6928
_________________________________________________________________
activation_2 (Activation) (None, 30, 120, 120, 16) 0
_________________________________________________________________
max_pooling3d_1 (MaxPooling3 (None, 10, 40, 40, 16) 0
_________________________________________________________________
dropout_1 (Dropout) (None, 10, 40, 40, 16) 0
_________________________________________________________________
conv3d_3 (Conv3D) (None, 10, 40, 40, 32) 13856
_________________________________________________________________
activation_3 (Activation) (None, 10, 40, 40, 32) 0
_________________________________________________________________
conv3d_4 (Conv3D) (None, 10, 40, 40, 32) 27680
_________________________________________________________________
activation_4 (Activation) (None, 10, 40, 40, 32) 0
_________________________________________________________________
max_pooling3d_2 (MaxPooling3 (None, 4, 14, 14, 32) 0
_________________________________________________________________
dropout_2 (Dropout) (None, 4, 14, 14, 32) 0
_________________________________________________________________
conv3d_5 (Conv3D) (None, 4, 14, 14, 32) 27680
_________________________________________________________________
activation_5 (Activation) (None, 4, 14, 14, 32) 0
_________________________________________________________________
conv3d_6 (Conv3D) (None, 4, 14, 14, 32) 27680
_________________________________________________________________
activation_6 (Activation) (None, 4, 14, 14, 32) 0
_________________________________________________________________
max_pooling3d_3 (MaxPooling3 (None, 2, 5, 5, 32) 0
_________________________________________________________________
dropout_3 (Dropout) (None, 2, 5, 5, 32) 0
_________________________________________________________________
flatten_1 (Flatten) (None, 1600) 0
_________________________________________________________________
dense_1 (Dense) (None, 512) 819712
_________________________________________________________________
batch_normalization_1 (Batch (None, 512) 2048
_________________________________________________________________
dropout_4 (Dropout) (None, 512) 0
_________________________________________________________________
dense_2 (Dense) (None, 5) 2565
=================================================================
Total params: 929,461
Trainable params: 928,437
Non-trainable params: 1,024
_________________________________________________________________
None
Epoch 1/20
Source path = ./Project_data/val ; batch size = 10
Source path = ./Project_data/train ; batch size = 10
67/67 [==============================] - 49s 729ms/step - loss: 1.4047 - categorical_accuracy: 0.3756 - val_loss: 11.8905 - val_categorical_accuracy: 0.2500
Epoch 00001: saving model to model_init_2018-10-2122_07_26.449958/model-00001-1.40491-0.37607-11.89046-0.25000.h5
Epoch 2/20
67/67 [==============================] - 15s 220ms/step - loss: 1.1593 - categorical_accuracy: 0.5340 - val_loss: 8.5508 - val_categorical_accuracy: 0.4700
Epoch 00002: saving model to model_init_2018-10-2122_07_26.449958/model-00002-1.15928-0.53400-8.55077-0.47000.h5
Epoch 3/20
67/67 [==============================] - 16s 233ms/step - loss: 1.0332 - categorical_accuracy: 0.6003 - val_loss: 11.3355 - val_categorical_accuracy: 0.2800
Epoch 00003: saving model to model_init_2018-10-2122_07_26.449958/model-00003-1.03320-0.60033-11.33554-0.28000.h5
Epoch 4/20
67/67 [==============================] - 16s 243ms/step - loss: 0.9572 - categorical_accuracy: 0.6219 - val_loss: 9.9932 - val_categorical_accuracy: 0.3800
Epoch 00004: saving model to model_init_2018-10-2122_07_26.449958/model-00004-0.95720-0.62189-9.99322-0.38000.h5
Epoch 00004: ReduceLROnPlateau reducing learning rate to 0.0005000000237487257.
Epoch 5/20
67/67 [==============================] - 16s 234ms/step - loss: 0.8203 - categorical_accuracy: 0.6716 - val_loss: 10.7991 - val_categorical_accuracy: 0.3300
Epoch 00005: saving model to model_init_2018-10-2122_07_26.449958/model-00005-0.82029-0.67164-10.79913-0.33000.h5
Epoch 6/20
67/67 [==============================] - 16s 234ms/step - loss: 0.7070 - categorical_accuracy: 0.6833 - val_loss: 11.1288 - val_categorical_accuracy: 0.3000
Epoch 00006: saving model to model_init_2018-10-2122_07_26.449958/model-00006-0.70698-0.68325-11.12882-0.30000.h5
Epoch 00006: ReduceLROnPlateau reducing learning rate to 0.0002500000118743628.
Epoch 7/20
67/67 [==============================] - 15s 219ms/step - loss: 0.6941 - categorical_accuracy: 0.7098 - val_loss: 10.6840 - val_categorical_accuracy: 0.3300
Epoch 00007: saving model to model_init_2018-10-2122_07_26.449958/model-00007-0.69409-0.70978-10.68399-0.33000.h5
Epoch 8/20
67/67 [==============================] - 16s 235ms/step - loss: 0.6164 - categorical_accuracy: 0.7114 - val_loss: 11.4655 - val_categorical_accuracy: 0.2800
Epoch 00008: saving model to model_init_2018-10-2122_07_26.449958/model-00008-0.61639-0.71144-11.46548-0.28000.h5
Epoch 00008: ReduceLROnPlateau reducing learning rate to 0.0001250000059371814.
Epoch 9/20
67/67 [==============================] - 14s 215ms/step - loss: 0.5968 - categorical_accuracy: 0.7562 - val_loss: 11.9092 - val_categorical_accuracy: 0.2600
Epoch 00009: saving model to model_init_2018-10-2122_07_26.449958/model-00009-0.59678-0.75622-11.90918-0.26000.h5
Epoch 10/20
67/67 [==============================] - 15s 226ms/step - loss: 0.5238 - categorical_accuracy: 0.7910 - val_loss: 10.0091 - val_categorical_accuracy: 0.3400
Epoch 00010: saving model to model_init_2018-10-2122_07_26.449958/model-00010-0.52380-0.79104-10.00909-0.34000.h5
Epoch 00010: ReduceLROnPlateau reducing learning rate to 6.25000029685907e-05.
Epoch 11/20
67/67 [==============================] - 15s 224ms/step - loss: 0.5839 - categorical_accuracy: 0.7662 - val_loss: 10.5103 - val_categorical_accuracy: 0.3100
Epoch 00011: saving model to model_init_2018-10-2122_07_26.449958/model-00011-0.58393-0.76617-10.51028-0.31000.h5
Epoch 12/20
67/67 [==============================] - 15s 224ms/step - loss: 0.6365 - categorical_accuracy: 0.7579 - val_loss: 11.3120 - val_categorical_accuracy: 0.2900
Epoch 00012: saving model to model_init_2018-10-2122_07_26.449958/model-00012-0.63651-0.75788-11.31202-0.29000.h5
Epoch 00012: ReduceLROnPlateau reducing learning rate to 3.125000148429535e-05.
Epoch 13/20
67/67 [==============================] - 16s 232ms/step - loss: 0.5244 - categorical_accuracy: 0.7811 - val_loss: 10.6303 - val_categorical_accuracy: 0.3200
Epoch 00013: saving model to model_init_2018-10-2122_07_26.449958/model-00013-0.52441-0.78109-10.63029-0.32000.h5
Epoch 14/20
67/67 [==============================] - 15s 227ms/step - loss: 0.6042 - categorical_accuracy: 0.7645 - val_loss: 10.9256 - val_categorical_accuracy: 0.3100
Epoch 00014: saving model to model_init_2018-10-2122_07_26.449958/model-00014-0.60415-0.76451-10.92562-0.31000.h5
Epoch 00014: ReduceLROnPlateau reducing learning rate to 1.5625000742147677e-05.
Epoch 15/20
67/67 [==============================] - 15s 231ms/step - loss: 0.4564 - categorical_accuracy: 0.7960 - val_loss: 10.8879 - val_categorical_accuracy: 0.3100
###Markdown
Model 4 Model 4a : Resize to 120*120, No agumentation, No flipped images, No normalisation, No cropping, No edge detection
###Code
train_gen = DataGenerator()
val_gen = DataGenerator()
model_gen = ModelGenerator()
input_shape = (30,120,120, 3)
num_classes = 5
model = model_gen.c3d4(input_shape, num_classes)
batch_size = 90
num_epochs = 10
train_generator = train_gen.generator(train_path, train_doc, batch_size)
val_generator = val_gen.generator(val_path, val_doc, batch_size)
train(batch_size, num_epochs, model, train_generator, val_generator)
###Output
# training sequences = 663
# validation sequences = 100
# batch size = 90
# epochs = 10
_________________________________________________________________
Layer (type) Output Shape Param #
=================================================================
conv3d_1 (Conv3D) (None, 30, 120, 120, 8) 656
_________________________________________________________________
batch_normalization_1 (Batch (None, 30, 120, 120, 8) 32
_________________________________________________________________
activation_1 (Activation) (None, 30, 120, 120, 8) 0
_________________________________________________________________
max_pooling3d_1 (MaxPooling3 (None, 15, 60, 60, 8) 0
_________________________________________________________________
conv3d_2 (Conv3D) (None, 15, 60, 60, 16) 3472
_________________________________________________________________
batch_normalization_2 (Batch (None, 15, 60, 60, 16) 64
_________________________________________________________________
activation_2 (Activation) (None, 15, 60, 60, 16) 0
_________________________________________________________________
max_pooling3d_2 (MaxPooling3 (None, 7, 30, 30, 16) 0
_________________________________________________________________
conv3d_3 (Conv3D) (None, 7, 30, 30, 32) 4640
_________________________________________________________________
batch_normalization_3 (Batch (None, 7, 30, 30, 32) 128
_________________________________________________________________
activation_3 (Activation) (None, 7, 30, 30, 32) 0
_________________________________________________________________
max_pooling3d_3 (MaxPooling3 (None, 3, 15, 15, 32) 0
_________________________________________________________________
conv3d_4 (Conv3D) (None, 3, 15, 15, 64) 18496
_________________________________________________________________
activation_4 (Activation) (None, 3, 15, 15, 64) 0
_________________________________________________________________
dropout_1 (Dropout) (None, 3, 15, 15, 64) 0
_________________________________________________________________
max_pooling3d_4 (MaxPooling3 (None, 1, 7, 7, 64) 0
_________________________________________________________________
flatten_1 (Flatten) (None, 3136) 0
_________________________________________________________________
dense_1 (Dense) (None, 256) 803072
_________________________________________________________________
dropout_2 (Dropout) (None, 256) 0
_________________________________________________________________
dense_2 (Dense) (None, 128) 32896
_________________________________________________________________
dropout_3 (Dropout) (None, 128) 0
_________________________________________________________________
dense_3 (Dense) (None, 5) 645
=================================================================
Total params: 864,101
Trainable params: 863,989
Non-trainable params: 112
_________________________________________________________________
None
Epoch 1/10
Source path = ./Project_data/val ; batch size = 90
Source path = ./Project_data/train ; batch size = 90
8/8 [==============================] - 26s 3s/step - loss: 2.2082 - categorical_accuracy: 0.2345 - val_loss: 1.4782 - val_categorical_accuracy: 0.3300
Epoch 00001: saving model to model_init_2018-10-2122_54_52.925487/model-00001-2.25286-0.22926-1.47824-0.33000.h5
Epoch 2/10
8/8 [==============================] - 10s 1s/step - loss: 1.5098 - categorical_accuracy: 0.3409 - val_loss: 1.8553 - val_categorical_accuracy: 0.2000
Epoch 00002: saving model to model_init_2018-10-2122_54_52.925487/model-00002-1.50976-0.34091-1.85528-0.20000.h5
Epoch 3/10
8/8 [==============================] - 10s 1s/step - loss: 1.4082 - categorical_accuracy: 0.3788 - val_loss: 2.1802 - val_categorical_accuracy: 0.2000
Epoch 00003: saving model to model_init_2018-10-2122_54_52.925487/model-00003-1.40824-0.37879-2.18019-0.20000.h5
Epoch 00003: ReduceLROnPlateau reducing learning rate to 0.0005000000237487257.
Epoch 4/10
8/8 [==============================] - 5s 573ms/step - loss: 1.3246 - categorical_accuracy: 0.3836 - val_loss: 1.4174 - val_categorical_accuracy: 0.4000
Epoch 00004: saving model to model_init_2018-10-2122_54_52.925487/model-00004-1.39342-0.36111-1.41744-0.40000.h5
Epoch 5/10
8/8 [==============================] - 1s 98ms/step - loss: 1.5630 - categorical_accuracy: 0.2917 - val_loss: 1.3387 - val_categorical_accuracy: 0.3500
Epoch 00005: saving model to model_init_2018-10-2122_54_52.925487/model-00005-1.56302-0.29167-1.33873-0.35000.h5
Epoch 6/10
8/8 [==============================] - 1s 127ms/step - loss: 1.3304 - categorical_accuracy: 0.4167 - val_loss: 1.6484 - val_categorical_accuracy: 0.2500
Epoch 00006: saving model to model_init_2018-10-2122_54_52.925487/model-00006-1.33043-0.41667-1.64843-0.25000.h5
Epoch 7/10
8/8 [==============================] - 1s 97ms/step - loss: 2.0385 - categorical_accuracy: 0.2917 - val_loss: 1.8207 - val_categorical_accuracy: 0.2000
Epoch 00007: saving model to model_init_2018-10-2122_54_52.925487/model-00007-2.03852-0.29167-1.82072-0.20000.h5
Epoch 00007: ReduceLROnPlateau reducing learning rate to 0.0002500000118743628.
Epoch 8/10
8/8 [==============================] - 1s 100ms/step - loss: 1.4933 - categorical_accuracy: 0.3333 - val_loss: 1.6126 - val_categorical_accuracy: 0.3500
Epoch 00008: saving model to model_init_2018-10-2122_54_52.925487/model-00008-1.49334-0.33333-1.61259-0.35000.h5
Epoch 9/10
8/8 [==============================] - 1s 132ms/step - loss: 1.7015 - categorical_accuracy: 0.1667 - val_loss: 1.5275 - val_categorical_accuracy: 0.2500
Epoch 00009: saving model to model_init_2018-10-2122_54_52.925487/model-00009-1.70145-0.16667-1.52752-0.25000.h5
Epoch 00009: ReduceLROnPlateau reducing learning rate to 0.0001250000059371814.
Epoch 10/10
8/8 [==============================] - 1s 116ms/step - loss: 1.4576 - categorical_accuracy: 0.3333 - val_loss: 1.2325 - val_categorical_accuracy: 0.4000
Epoch 00010: saving model to model_init_2018-10-2122_54_52.925487/model-00010-1.45761-0.33333-1.23253-0.40000.h5
###Markdown
Model 4b : Resize to 120*120, agumentation, No flipped images, No normalisation, No cropping, No edge detection
###Code
train_gen = DataGenerator(affine=True)
val_gen = DataGenerator()
model_gen = ModelGenerator()
input_shape = (30,120,120, 3)
num_classes = 5
model = model_gen.c3d4(input_shape, num_classes)
batch_size = 50
num_epochs = 10
train_generator = train_gen.generator(train_path, train_doc, batch_size)
val_generator = val_gen.generator(val_path, val_doc, batch_size)
train(batch_size, num_epochs, model, train_generator, val_generator)
###Output
# training sequences = 663
# validation sequences = 100
# batch size = 50
# epochs = 10
_________________________________________________________________
Layer (type) Output Shape Param #
=================================================================
conv3d_5 (Conv3D) (None, 30, 120, 120, 8) 656
_________________________________________________________________
batch_normalization_4 (Batch (None, 30, 120, 120, 8) 32
_________________________________________________________________
activation_5 (Activation) (None, 30, 120, 120, 8) 0
_________________________________________________________________
max_pooling3d_5 (MaxPooling3 (None, 15, 60, 60, 8) 0
_________________________________________________________________
conv3d_6 (Conv3D) (None, 15, 60, 60, 16) 3472
_________________________________________________________________
batch_normalization_5 (Batch (None, 15, 60, 60, 16) 64
_________________________________________________________________
activation_6 (Activation) (None, 15, 60, 60, 16) 0
_________________________________________________________________
max_pooling3d_6 (MaxPooling3 (None, 7, 30, 30, 16) 0
_________________________________________________________________
conv3d_7 (Conv3D) (None, 7, 30, 30, 32) 4640
_________________________________________________________________
batch_normalization_6 (Batch (None, 7, 30, 30, 32) 128
_________________________________________________________________
activation_7 (Activation) (None, 7, 30, 30, 32) 0
_________________________________________________________________
max_pooling3d_7 (MaxPooling3 (None, 3, 15, 15, 32) 0
_________________________________________________________________
conv3d_8 (Conv3D) (None, 3, 15, 15, 64) 18496
_________________________________________________________________
activation_8 (Activation) (None, 3, 15, 15, 64) 0
_________________________________________________________________
dropout_4 (Dropout) (None, 3, 15, 15, 64) 0
_________________________________________________________________
max_pooling3d_8 (MaxPooling3 (None, 1, 7, 7, 64) 0
_________________________________________________________________
flatten_2 (Flatten) (None, 3136) 0
_________________________________________________________________
dense_4 (Dense) (None, 256) 803072
_________________________________________________________________
dropout_5 (Dropout) (None, 256) 0
_________________________________________________________________
dense_5 (Dense) (None, 128) 32896
_________________________________________________________________
dropout_6 (Dropout) (None, 128) 0
_________________________________________________________________
dense_6 (Dense) (None, 5) 645
=================================================================
Total params: 864,101
Trainable params: 863,989
Non-trainable params: 112
_________________________________________________________________
None
Epoch 1/10
Source path = ./Project_data/train ; batch size = 50
Source path = ./Project_data/val ; batch size = 50
14/14 [==============================] - 33s 2s/step - loss: 2.0553 - categorical_accuracy: 0.2291 - val_loss: 1.5846 - val_categorical_accuracy: 0.3000
Epoch 00001: saving model to model_init_2018-10-2123_00_03.869014/model-00001-2.08225-0.22700-1.58463-0.30000.h5
Epoch 2/10
14/14 [==============================] - 8s 594ms/step - loss: 1.5820 - categorical_accuracy: 0.2555 - val_loss: 1.5652 - val_categorical_accuracy: 0.4300
Epoch 00002: saving model to model_init_2018-10-2123_00_03.869014/model-00002-1.58205-0.25549-1.56520-0.43000.h5
Epoch 3/10
14/14 [==============================] - 9s 656ms/step - loss: 1.5663 - categorical_accuracy: 0.3132 - val_loss: 1.5406 - val_categorical_accuracy: 0.3900
Epoch 00003: saving model to model_init_2018-10-2123_00_03.869014/model-00003-1.56634-0.31319-1.54062-0.39000.h5
Epoch 4/10
14/14 [==============================] - 9s 640ms/step - loss: 1.5074 - categorical_accuracy: 0.3022 - val_loss: 1.4637 - val_categorical_accuracy: 0.3500
Epoch 00004: saving model to model_init_2018-10-2123_00_03.869014/model-00004-1.50738-0.30220-1.46365-0.35000.h5
Epoch 5/10
14/14 [==============================] - 9s 618ms/step - loss: 1.3264 - categorical_accuracy: 0.4341 - val_loss: 1.3242 - val_categorical_accuracy: 0.3900
Epoch 00005: saving model to model_init_2018-10-2123_00_03.869014/model-00005-1.32641-0.43407-1.32416-0.39000.h5
Epoch 6/10
14/14 [==============================] - 9s 627ms/step - loss: 1.4257 - categorical_accuracy: 0.3846 - val_loss: 1.6070 - val_categorical_accuracy: 0.3100
Epoch 00006: saving model to model_init_2018-10-2123_00_03.869014/model-00006-1.42574-0.38462-1.60701-0.31000.h5
Epoch 7/10
14/14 [==============================] - 10s 689ms/step - loss: 1.3611 - categorical_accuracy: 0.4011 - val_loss: 1.2537 - val_categorical_accuracy: 0.4800
Epoch 00007: saving model to model_init_2018-10-2123_00_03.869014/model-00007-1.36112-0.40110-1.25373-0.48000.h5
Epoch 8/10
14/14 [==============================] - 9s 619ms/step - loss: 1.2781 - categorical_accuracy: 0.4176 - val_loss: 1.2370 - val_categorical_accuracy: 0.5200
Epoch 00008: saving model to model_init_2018-10-2123_00_03.869014/model-00008-1.27811-0.41758-1.23701-0.52000.h5
Epoch 9/10
14/14 [==============================] - 9s 617ms/step - loss: 1.1630 - categorical_accuracy: 0.5220 - val_loss: 2.0248 - val_categorical_accuracy: 0.3200
Epoch 00009: saving model to model_init_2018-10-2123_00_03.869014/model-00009-1.16298-0.52198-2.02485-0.32000.h5
Epoch 10/10
14/14 [==============================] - 9s 626ms/step - loss: 1.1293 - categorical_accuracy: 0.5247 - val_loss: 1.2289 - val_categorical_accuracy: 0.5000
Epoch 00010: saving model to model_init_2018-10-2123_00_03.869014/model-00010-1.12934-0.52473-1.22895-0.50000.h5
###Markdown
Model 4c : Resize to 120*120, agumentation, flipped images, No normalisation, No cropping, No edge detection
###Code
train_gen = DataGenerator(affine=True, flip=True)
val_gen = DataGenerator()
model_gen = ModelGenerator()
input_shape = (30,120,120, 3)
num_classes = 5
model = model_gen.c3d4(input_shape, num_classes)
batch_size = 30
num_epochs = 10
train_generator = train_gen.generator(train_path, train_doc, batch_size)
val_generator = val_gen.generator(val_path, val_doc, batch_size)
train(batch_size, num_epochs, model, train_generator, val_generator)
###Output
# training sequences = 663
# validation sequences = 100
# batch size = 30
# epochs = 10
_________________________________________________________________
Layer (type) Output Shape Param #
=================================================================
conv3d_13 (Conv3D) (None, 30, 120, 120, 8) 656
_________________________________________________________________
batch_normalization_10 (Batc (None, 30, 120, 120, 8) 32
_________________________________________________________________
activation_13 (Activation) (None, 30, 120, 120, 8) 0
_________________________________________________________________
max_pooling3d_13 (MaxPooling (None, 15, 60, 60, 8) 0
_________________________________________________________________
conv3d_14 (Conv3D) (None, 15, 60, 60, 16) 3472
_________________________________________________________________
batch_normalization_11 (Batc (None, 15, 60, 60, 16) 64
_________________________________________________________________
activation_14 (Activation) (None, 15, 60, 60, 16) 0
_________________________________________________________________
max_pooling3d_14 (MaxPooling (None, 7, 30, 30, 16) 0
_________________________________________________________________
conv3d_15 (Conv3D) (None, 7, 30, 30, 32) 4640
_________________________________________________________________
batch_normalization_12 (Batc (None, 7, 30, 30, 32) 128
_________________________________________________________________
activation_15 (Activation) (None, 7, 30, 30, 32) 0
_________________________________________________________________
max_pooling3d_15 (MaxPooling (None, 3, 15, 15, 32) 0
_________________________________________________________________
conv3d_16 (Conv3D) (None, 3, 15, 15, 64) 18496
_________________________________________________________________
activation_16 (Activation) (None, 3, 15, 15, 64) 0
_________________________________________________________________
dropout_10 (Dropout) (None, 3, 15, 15, 64) 0
_________________________________________________________________
max_pooling3d_16 (MaxPooling (None, 1, 7, 7, 64) 0
_________________________________________________________________
flatten_4 (Flatten) (None, 3136) 0
_________________________________________________________________
dense_10 (Dense) (None, 256) 803072
_________________________________________________________________
dropout_11 (Dropout) (None, 256) 0
_________________________________________________________________
dense_11 (Dense) (None, 128) 32896
_________________________________________________________________
dropout_12 (Dropout) (None, 128) 0
_________________________________________________________________
dense_12 (Dense) (None, 5) 645
=================================================================
Total params: 864,101
Trainable params: 863,989
Non-trainable params: 112
_________________________________________________________________
None
Epoch 1/10
Source path = ./Project_data/train ; batch size = 30
Source path = ./Project_data/val ; batch size = 30
23/23 [==============================] - 39s 2s/step - loss: 1.8885 - categorical_accuracy: 0.2794 - val_loss: 1.4772 - val_categorical_accuracy: 0.2800
Epoch 00001: saving model to model_init_2018-10-2123_03_52.487540/model-00001-1.90538-0.27300-1.47719-0.28000.h5
Epoch 2/10
23/23 [==============================] - 3s 120ms/step - loss: 1.5493 - categorical_accuracy: 0.2899 - val_loss: 1.7572 - val_categorical_accuracy: 0.3000
Epoch 00002: saving model to model_init_2018-10-2123_03_52.487540/model-00002-1.54925-0.28986-1.75721-0.30000.h5
Epoch 3/10
23/23 [==============================] - 4s 178ms/step - loss: 1.6312 - categorical_accuracy: 0.2029 - val_loss: 1.4681 - val_categorical_accuracy: 0.2000
Epoch 00003: saving model to model_init_2018-10-2123_03_52.487540/model-00003-1.63117-0.20290-1.46810-0.20000.h5
Epoch 4/10
23/23 [==============================] - 4s 188ms/step - loss: 1.5177 - categorical_accuracy: 0.3092 - val_loss: 2.7249 - val_categorical_accuracy: 0.2500
Epoch 00004: saving model to model_init_2018-10-2123_03_52.487540/model-00004-1.51767-0.30918-2.72490-0.25000.h5
Epoch 5/10
23/23 [==============================] - 5s 220ms/step - loss: 1.5391 - categorical_accuracy: 0.2947 - val_loss: 1.5052 - val_categorical_accuracy: 0.2750
Epoch 00005: saving model to model_init_2018-10-2123_03_52.487540/model-00005-1.53908-0.29469-1.50523-0.27500.h5
Epoch 00005: ReduceLROnPlateau reducing learning rate to 0.0005000000237487257.
Epoch 6/10
23/23 [==============================] - 5s 198ms/step - loss: 1.5284 - categorical_accuracy: 0.2899 - val_loss: 1.4060 - val_categorical_accuracy: 0.3000
Epoch 00006: saving model to model_init_2018-10-2123_03_52.487540/model-00006-1.52835-0.28986-1.40602-0.30000.h5
Epoch 7/10
23/23 [==============================] - 4s 173ms/step - loss: 1.4752 - categorical_accuracy: 0.3043 - val_loss: 1.4763 - val_categorical_accuracy: 0.2750
Epoch 00007: saving model to model_init_2018-10-2123_03_52.487540/model-00007-1.47524-0.30435-1.47627-0.27500.h5
Epoch 8/10
23/23 [==============================] - 4s 162ms/step - loss: 1.4490 - categorical_accuracy: 0.3913 - val_loss: 1.3351 - val_categorical_accuracy: 0.3750
Epoch 00008: saving model to model_init_2018-10-2123_03_52.487540/model-00008-1.44901-0.39130-1.33512-0.37500.h5
Epoch 9/10
23/23 [==============================] - 5s 198ms/step - loss: 1.5220 - categorical_accuracy: 0.3188 - val_loss: 1.4831 - val_categorical_accuracy: 0.4000
Epoch 00009: saving model to model_init_2018-10-2123_03_52.487540/model-00009-1.52202-0.31884-1.48306-0.40000.h5
Epoch 10/10
23/23 [==============================] - 4s 177ms/step - loss: 1.4250 - categorical_accuracy: 0.3768 - val_loss: 1.3581 - val_categorical_accuracy: 0.4750
Epoch 00010: saving model to model_init_2018-10-2123_03_52.487540/model-00010-1.42505-0.37681-1.35807-0.47500.h5
Epoch 00010: ReduceLROnPlateau reducing learning rate to 0.0002500000118743628.
###Markdown
Model 4d : Resize to 120*120, agumentation, No flipped images, No normalisation, No cropping, No edge detection
###Code
train_gen = DataGenerator(affine=True, crop=False)
val_gen = DataGenerator(crop=False)
model_gen = ModelGenerator()
input_shape = (30,120,120, 3)
num_classes = 5
model = model_gen.c3d4(input_shape, num_classes)
batch_size = 40
num_epochs = 10
train_generator = train_gen.generator(train_path, train_doc, batch_size)
val_generator = val_gen.generator(val_path, val_doc, batch_size)
train(batch_size, num_epochs, model, train_generator, val_generator)
###Output
# training sequences = 663
# validation sequences = 100
# batch size = 40
# epochs = 10
_________________________________________________________________
Layer (type) Output Shape Param #
=================================================================
conv3d_17 (Conv3D) (None, 30, 120, 120, 8) 656
_________________________________________________________________
batch_normalization_13 (Batc (None, 30, 120, 120, 8) 32
_________________________________________________________________
activation_17 (Activation) (None, 30, 120, 120, 8) 0
_________________________________________________________________
max_pooling3d_17 (MaxPooling (None, 15, 60, 60, 8) 0
_________________________________________________________________
conv3d_18 (Conv3D) (None, 15, 60, 60, 16) 3472
_________________________________________________________________
batch_normalization_14 (Batc (None, 15, 60, 60, 16) 64
_________________________________________________________________
activation_18 (Activation) (None, 15, 60, 60, 16) 0
_________________________________________________________________
max_pooling3d_18 (MaxPooling (None, 7, 30, 30, 16) 0
_________________________________________________________________
conv3d_19 (Conv3D) (None, 7, 30, 30, 32) 4640
_________________________________________________________________
batch_normalization_15 (Batc (None, 7, 30, 30, 32) 128
_________________________________________________________________
activation_19 (Activation) (None, 7, 30, 30, 32) 0
_________________________________________________________________
max_pooling3d_19 (MaxPooling (None, 3, 15, 15, 32) 0
_________________________________________________________________
conv3d_20 (Conv3D) (None, 3, 15, 15, 64) 18496
_________________________________________________________________
activation_20 (Activation) (None, 3, 15, 15, 64) 0
_________________________________________________________________
dropout_13 (Dropout) (None, 3, 15, 15, 64) 0
_________________________________________________________________
max_pooling3d_20 (MaxPooling (None, 1, 7, 7, 64) 0
_________________________________________________________________
flatten_5 (Flatten) (None, 3136) 0
_________________________________________________________________
dense_13 (Dense) (None, 256) 803072
_________________________________________________________________
dropout_14 (Dropout) (None, 256) 0
_________________________________________________________________
dense_14 (Dense) (None, 128) 32896
_________________________________________________________________
dropout_15 (Dropout) (None, 128) 0
_________________________________________________________________
dense_15 (Dense) (None, 5) 645
=================================================================
Total params: 864,101
Trainable params: 863,989
Non-trainable params: 112
_________________________________________________________________
None
Epoch 1/10
Source path = ./Project_data/train ; batch size = 40
Source path = ./Project_data/val ; batch size = 40
17/17 [==============================] - 35s 2s/step - loss: 2.1257 - categorical_accuracy: 0.2260 - val_loss: 1.5702 - val_categorical_accuracy: 0.4400
Epoch 00001: saving model to model_init_2018-10-2123_11_50.641986/model-00001-2.13875-0.22624-1.57019-0.44000.h5
Epoch 2/10
17/17 [==============================] - 18s 1s/step - loss: 1.5261 - categorical_accuracy: 0.3184 - val_loss: 1.4787 - val_categorical_accuracy: 0.3000
Epoch 00002: saving model to model_init_2018-10-2123_11_50.641986/model-00002-1.52613-0.31841-1.47872-0.30000.h5
Epoch 3/10
17/17 [==============================] - 19s 1s/step - loss: 1.3811 - categorical_accuracy: 0.3848 - val_loss: 1.4465 - val_categorical_accuracy: 0.3500
Epoch 00003: saving model to model_init_2018-10-2123_11_50.641986/model-00003-1.38761-0.38283-1.44646-0.35000.h5
Epoch 4/10
17/17 [==============================] - 15s 903ms/step - loss: 1.3315 - categorical_accuracy: 0.4087 - val_loss: 1.4391 - val_categorical_accuracy: 0.3667
Epoch 00004: saving model to model_init_2018-10-2123_11_50.641986/model-00004-1.33148-0.40867-1.43911-0.36667.h5
Epoch 5/10
17/17 [==============================] - 16s 947ms/step - loss: 1.1736 - categorical_accuracy: 0.4947 - val_loss: 1.3551 - val_categorical_accuracy: 0.4167
Epoch 00005: saving model to model_init_2018-10-2123_11_50.641986/model-00005-1.17239-0.49521-1.35512-0.41667.h5
Epoch 6/10
17/17 [==============================] - 15s 861ms/step - loss: 1.0766 - categorical_accuracy: 0.5588 - val_loss: 1.5101 - val_categorical_accuracy: 0.3833
Epoch 00006: saving model to model_init_2018-10-2123_11_50.641986/model-00006-1.07656-0.55882-1.51011-0.38333.h5
Epoch 7/10
17/17 [==============================] - 14s 838ms/step - loss: 0.9791 - categorical_accuracy: 0.5744 - val_loss: 1.7059 - val_categorical_accuracy: 0.3500
Epoch 00007: saving model to model_init_2018-10-2123_11_50.641986/model-00007-0.97911-0.57439-1.70590-0.35000.h5
Epoch 00007: ReduceLROnPlateau reducing learning rate to 0.0005000000237487257.
Epoch 8/10
17/17 [==============================] - 15s 874ms/step - loss: 0.8199 - categorical_accuracy: 0.6592 - val_loss: 1.4384 - val_categorical_accuracy: 0.3167
Epoch 00008: saving model to model_init_2018-10-2123_11_50.641986/model-00008-0.81985-0.65917-1.43838-0.31667.h5
Epoch 9/10
17/17 [==============================] - 14s 829ms/step - loss: 0.8155 - categorical_accuracy: 0.6696 - val_loss: 1.3038 - val_categorical_accuracy: 0.4000
Epoch 00009: saving model to model_init_2018-10-2123_11_50.641986/model-00009-0.81553-0.66955-1.30381-0.40000.h5
Epoch 10/10
17/17 [==============================] - 14s 810ms/step - loss: 0.7782 - categorical_accuracy: 0.7128 - val_loss: 0.7777 - val_categorical_accuracy: 0.7000
Epoch 00010: saving model to model_init_2018-10-2123_11_50.641986/model-00010-0.77823-0.71280-0.77775-0.70000.h5
###Markdown
Model 4e : Resize to 120*120, agumentation, flipped images, normalisation, cropping, No edge detection
###Code
train_gen = DataGenerator(affine=True, flip=True, normalize=True, crop=True)
val_gen = DataGenerator()
model_gen = ModelGenerator()
input_shape = (30,120,120, 3)
num_classes = 5
model = model_gen.c3d4(input_shape, num_classes)
#batch_size = 20
#num_epochs = 20
train_generator = train_gen.generator(train_path, train_doc, batch_size)
val_generator = val_gen.generator(val_path, val_doc, batch_size)
train(batch_size, num_epochs, model, train_generator, val_generator)
###Output
# training sequences = 663
# validation sequences = 100
# batch size = 20
# epochs = 20
_________________________________________________________________
Layer (type) Output Shape Param #
=================================================================
conv3d_1 (Conv3D) (None, 30, 120, 120, 8) 656
_________________________________________________________________
batch_normalization_1 (Batch (None, 30, 120, 120, 8) 32
_________________________________________________________________
activation_1 (Activation) (None, 30, 120, 120, 8) 0
_________________________________________________________________
max_pooling3d_1 (MaxPooling3 (None, 15, 60, 60, 8) 0
_________________________________________________________________
conv3d_2 (Conv3D) (None, 15, 60, 60, 16) 3472
_________________________________________________________________
batch_normalization_2 (Batch (None, 15, 60, 60, 16) 64
_________________________________________________________________
activation_2 (Activation) (None, 15, 60, 60, 16) 0
_________________________________________________________________
max_pooling3d_2 (MaxPooling3 (None, 7, 30, 30, 16) 0
_________________________________________________________________
conv3d_3 (Conv3D) (None, 7, 30, 30, 32) 4640
_________________________________________________________________
batch_normalization_3 (Batch (None, 7, 30, 30, 32) 128
_________________________________________________________________
activation_3 (Activation) (None, 7, 30, 30, 32) 0
_________________________________________________________________
max_pooling3d_3 (MaxPooling3 (None, 3, 15, 15, 32) 0
_________________________________________________________________
conv3d_4 (Conv3D) (None, 3, 15, 15, 64) 18496
_________________________________________________________________
activation_4 (Activation) (None, 3, 15, 15, 64) 0
_________________________________________________________________
dropout_1 (Dropout) (None, 3, 15, 15, 64) 0
_________________________________________________________________
max_pooling3d_4 (MaxPooling3 (None, 1, 7, 7, 64) 0
_________________________________________________________________
flatten_1 (Flatten) (None, 3136) 0
_________________________________________________________________
dense_1 (Dense) (None, 256) 803072
_________________________________________________________________
dropout_2 (Dropout) (None, 256) 0
_________________________________________________________________
dense_2 (Dense) (None, 128) 32896
_________________________________________________________________
dropout_3 (Dropout) (None, 128) 0
_________________________________________________________________
dense_3 (Dense) (None, 5) 645
=================================================================
Total params: 864,101
Trainable params: 863,989
Non-trainable params: 112
_________________________________________________________________
None
Epoch 1/20
Source path = ./Project_data/val ; batch size = 20
Source path = ./Project_data/train ; batch size = 20
34/34 [==============================] - 42s 1s/step - loss: 1.7862 - categorical_accuracy: 0.2597 - val_loss: 12.4109 - val_categorical_accuracy: 0.2300
Epoch 00001: saving model to model_init_2018-10-2122_23_29.778726/model-00001-1.79459-0.26345-12.41093-0.23000.h5
Epoch 2/20
34/34 [==============================] - 6s 183ms/step - loss: 1.5856 - categorical_accuracy: 0.3497 - val_loss: 11.1863 - val_categorical_accuracy: 0.2900
Epoch 00002: saving model to model_init_2018-10-2122_23_29.778726/model-00002-1.58564-0.34967-11.18625-0.29000.h5
Epoch 3/20
34/34 [==============================] - 6s 184ms/step - loss: 1.5143 - categorical_accuracy: 0.3235 - val_loss: 11.9239 - val_categorical_accuracy: 0.2400
Epoch 00003: saving model to model_init_2018-10-2122_23_29.778726/model-00003-1.51434-0.32353-11.92390-0.24000.h5
Epoch 4/20
34/34 [==============================] - 7s 207ms/step - loss: 1.4596 - categorical_accuracy: 0.4379 - val_loss: 11.9732 - val_categorical_accuracy: 0.2500
Epoch 00004: saving model to model_init_2018-10-2122_23_29.778726/model-00004-1.45957-0.43791-11.97320-0.25000.h5
Epoch 00004: ReduceLROnPlateau reducing learning rate to 0.0005000000237487257.
Epoch 5/20
34/34 [==============================] - 7s 209ms/step - loss: 1.3611 - categorical_accuracy: 0.4575 - val_loss: 10.3550 - val_categorical_accuracy: 0.3500
Epoch 00005: saving model to model_init_2018-10-2122_23_29.778726/model-00005-1.36105-0.45752-10.35501-0.35000.h5
Epoch 6/20
34/34 [==============================] - 7s 205ms/step - loss: 1.1823 - categorical_accuracy: 0.5033 - val_loss: 10.3328 - val_categorical_accuracy: 0.3500
Epoch 00006: saving model to model_init_2018-10-2122_23_29.778726/model-00006-1.18229-0.50327-10.33281-0.35000.h5
Epoch 7/20
34/34 [==============================] - 7s 206ms/step - loss: 1.2272 - categorical_accuracy: 0.5261 - val_loss: 12.4109 - val_categorical_accuracy: 0.2300
Epoch 00007: saving model to model_init_2018-10-2122_23_29.778726/model-00007-1.22716-0.52614-12.41093-0.23000.h5
Epoch 8/20
34/34 [==============================] - 7s 199ms/step - loss: 1.3433 - categorical_accuracy: 0.4216 - val_loss: 10.4525 - val_categorical_accuracy: 0.3400
Epoch 00008: saving model to model_init_2018-10-2122_23_29.778726/model-00008-1.34334-0.42157-10.45253-0.34000.h5
Epoch 00008: ReduceLROnPlateau reducing learning rate to 0.0002500000118743628.
Epoch 9/20
34/34 [==============================] - 7s 206ms/step - loss: 1.1297 - categorical_accuracy: 0.5327 - val_loss: 10.7233 - val_categorical_accuracy: 0.3300
Epoch 00009: saving model to model_init_2018-10-2122_23_29.778726/model-00009-1.12970-0.53268-10.72334-0.33000.h5
Epoch 10/20
34/34 [==============================] - 7s 204ms/step - loss: 1.0739 - categorical_accuracy: 0.5882 - val_loss: 10.3622 - val_categorical_accuracy: 0.3500
Epoch 00010: saving model to model_init_2018-10-2122_23_29.778726/model-00010-1.07389-0.58824-10.36222-0.35000.h5
Epoch 00010: ReduceLROnPlateau reducing learning rate to 0.0001250000059371814.
Epoch 11/20
34/34 [==============================] - 7s 195ms/step - loss: 1.0442 - categorical_accuracy: 0.6078 - val_loss: 10.4768 - val_categorical_accuracy: 0.3500
Epoch 00011: saving model to model_init_2018-10-2122_23_29.778726/model-00011-1.04419-0.60784-10.47676-0.35000.h5
Epoch 12/20
34/34 [==============================] - 7s 191ms/step - loss: 0.9718 - categorical_accuracy: 0.5784 - val_loss: 10.3156 - val_categorical_accuracy: 0.3600
Epoch 00012: saving model to model_init_2018-10-2122_23_29.778726/model-00012-0.97175-0.57843-10.31558-0.36000.h5
Epoch 13/20
34/34 [==============================] - 7s 194ms/step - loss: 0.9057 - categorical_accuracy: 0.6699 - val_loss: 10.3156 - val_categorical_accuracy: 0.3600
Epoch 00013: saving model to model_init_2018-10-2122_23_29.778726/model-00013-0.90573-0.66993-10.31558-0.36000.h5
Epoch 14/20
34/34 [==============================] - 7s 217ms/step - loss: 1.0807 - categorical_accuracy: 0.6013 - val_loss: 10.9551 - val_categorical_accuracy: 0.3200
Epoch 00014: saving model to model_init_2018-10-2122_23_29.778726/model-00014-1.08075-0.60131-10.95511-0.32000.h5
Epoch 00014: ReduceLROnPlateau reducing learning rate to 6.25000029685907e-05.
Epoch 15/20
34/34 [==============================] - 7s 193ms/step - loss: 0.9136 - categorical_accuracy: 0.6340 - val_loss: 10.8786 - val_categorical_accuracy: 0.3200
Epoch 00015: saving model to model_init_2018-10-2122_23_29.778726/model-00015-0.91362-0.63399-10.87859-0.32000.h5
Epoch 16/20
34/34 [==============================] - 6s 187ms/step - loss: 0.9170 - categorical_accuracy: 0.6503 - val_loss: 10.6197 - val_categorical_accuracy: 0.3300
Epoch 00016: saving model to model_init_2018-10-2122_23_29.778726/model-00016-0.91705-0.65033-10.61973-0.33000.h5
Epoch 00016: ReduceLROnPlateau reducing learning rate to 3.125000148429535e-05.
Epoch 17/20
###Markdown
Model 4f : Resize to 120*120, agumentation, flipped images, normalisation, cropping, edge detection
###Code
train_gen = DataGenerator(affine=True, flip=True, normalize=True, crop=True, edge=True)
val_gen = DataGenerator()
model_gen = ModelGenerator()
input_shape = (30,120,120, 3)
num_classes = 5
model = model_gen.c3d4(input_shape, num_classes)
#batch_size = 20
#num_epochs = 20
train_generator = train_gen.generator(train_path, train_doc, batch_size)
val_generator = val_gen.generator(val_path, val_doc, batch_size)
train(batch_size, num_epochs, model, train_generator, val_generator)
### Model 4g : Resize to 120*120, agumentation, flipped images, No normalisation, cropping, edge detection
train_gen = DataGenerator(affine=True, flip=True, normalize=False, crop=True, edge=True)
val_gen = DataGenerator()
model_gen = ModelGenerator()
input_shape = (30,120,120, 3)
num_classes = 5
model = model_gen.c3d4(input_shape, num_classes)
#batch_size = 20
#num_epochs = 20
train_generator = train_gen.generator(train_path, train_doc, batch_size)
val_generator = val_gen.generator(val_path, val_doc, batch_size)
train(batch_size, num_epochs, model, train_generator, val_generator)
###Output
# training sequences = 663
# validation sequences = 100
# batch size = 20
# epochs = 20
_________________________________________________________________
Layer (type) Output Shape Param #
=================================================================
conv3d_1 (Conv3D) (None, 30, 120, 120, 8) 656
_________________________________________________________________
batch_normalization_1 (Batch (None, 30, 120, 120, 8) 32
_________________________________________________________________
activation_1 (Activation) (None, 30, 120, 120, 8) 0
_________________________________________________________________
max_pooling3d_1 (MaxPooling3 (None, 15, 60, 60, 8) 0
_________________________________________________________________
conv3d_2 (Conv3D) (None, 15, 60, 60, 16) 3472
_________________________________________________________________
batch_normalization_2 (Batch (None, 15, 60, 60, 16) 64
_________________________________________________________________
activation_2 (Activation) (None, 15, 60, 60, 16) 0
_________________________________________________________________
max_pooling3d_2 (MaxPooling3 (None, 7, 30, 30, 16) 0
_________________________________________________________________
conv3d_3 (Conv3D) (None, 7, 30, 30, 32) 4640
_________________________________________________________________
batch_normalization_3 (Batch (None, 7, 30, 30, 32) 128
_________________________________________________________________
activation_3 (Activation) (None, 7, 30, 30, 32) 0
_________________________________________________________________
max_pooling3d_3 (MaxPooling3 (None, 3, 15, 15, 32) 0
_________________________________________________________________
conv3d_4 (Conv3D) (None, 3, 15, 15, 64) 18496
_________________________________________________________________
activation_4 (Activation) (None, 3, 15, 15, 64) 0
_________________________________________________________________
dropout_1 (Dropout) (None, 3, 15, 15, 64) 0
_________________________________________________________________
max_pooling3d_4 (MaxPooling3 (None, 1, 7, 7, 64) 0
_________________________________________________________________
flatten_1 (Flatten) (None, 3136) 0
_________________________________________________________________
dense_1 (Dense) (None, 256) 803072
_________________________________________________________________
dropout_2 (Dropout) (None, 256) 0
_________________________________________________________________
dense_2 (Dense) (None, 128) 32896
_________________________________________________________________
dropout_3 (Dropout) (None, 128) 0
_________________________________________________________________
dense_3 (Dense) (None, 5) 645
=================================================================
Total params: 864,101
Trainable params: 863,989
Non-trainable params: 112
_________________________________________________________________
None
Epoch 1/20
Source path = ./Project_data/train Source path = ./Project_data/val ; batch size = 20
; batch size = 20
34/34 [==============================] - 54s 2s/step - loss: 1.8184 - categorical_accuracy: 0.2575 - val_loss: 4.9224 - val_categorical_accuracy: 0.2200
Epoch 00001: saving model to model_init_2018-10-2122_49_34.968496/model-00001-1.82500-0.25842-4.92243-0.22000.h5
Epoch 2/20
34/34 [==============================] - 9s 262ms/step - loss: 1.6118 - categorical_accuracy: 0.2810 - val_loss: 1.8029 - val_categorical_accuracy: 0.2200
Epoch 00002: saving model to model_init_2018-10-2122_49_34.968496/model-00002-1.61177-0.28105-1.80290-0.22000.h5
Epoch 3/20
34/34 [==============================] - 10s 282ms/step - loss: 1.6043 - categorical_accuracy: 0.2320 - val_loss: 3.9067 - val_categorical_accuracy: 0.2200
Epoch 00003: saving model to model_init_2018-10-2122_49_34.968496/model-00003-1.60434-0.23203-3.90674-0.22000.h5
Epoch 4/20
34/34 [==============================] - 9s 254ms/step - loss: 1.5384 - categorical_accuracy: 0.3072 - val_loss: 6.0873 - val_categorical_accuracy: 0.2200
Epoch 00004: saving model to model_init_2018-10-2122_49_34.968496/model-00004-1.53843-0.30719-6.08726-0.22000.h5
Epoch 00004: ReduceLROnPlateau reducing learning rate to 0.0005000000237487257.
Epoch 5/20
34/34 [==============================] - 9s 258ms/step - loss: 1.5152 - categorical_accuracy: 0.3105 - val_loss: 5.2582 - val_categorical_accuracy: 0.1600
Epoch 00005: saving model to model_init_2018-10-2122_49_34.968496/model-00005-1.51520-0.31046-5.25819-0.16000.h5
Epoch 6/20
34/34 [==============================] - 8s 245ms/step - loss: 1.5385 - categorical_accuracy: 0.3072 - val_loss: 7.4924 - val_categorical_accuracy: 0.2200
Epoch 00006: saving model to model_init_2018-10-2122_49_34.968496/model-00006-1.53848-0.30719-7.49241-0.22000.h5
Epoch 00006: ReduceLROnPlateau reducing learning rate to 0.0002500000118743628.
Epoch 7/20
34/34 [==============================] - 10s 281ms/step - loss: 1.4942 - categorical_accuracy: 0.3007 - val_loss: 6.8632 - val_categorical_accuracy: 0.2600
Epoch 00007: saving model to model_init_2018-10-2122_49_34.968496/model-00007-1.49417-0.30065-6.86325-0.26000.h5
Epoch 8/20
34/34 [==============================] - 10s 295ms/step - loss: 1.5006 - categorical_accuracy: 0.3529 - val_loss: 6.3283 - val_categorical_accuracy: 0.2300
Epoch 00008: saving model to model_init_2018-10-2122_49_34.968496/model-00008-1.50064-0.35294-6.32827-0.23000.h5
Epoch 00008: ReduceLROnPlateau reducing learning rate to 0.0001250000059371814.
Epoch 9/20
34/34 [==============================] - 9s 272ms/step - loss: 1.4316 - categorical_accuracy: 0.3987 - val_loss: 7.1439 - val_categorical_accuracy: 0.2300
Epoch 00009: saving model to model_init_2018-10-2122_49_34.968496/model-00009-1.43160-0.39869-7.14388-0.23000.h5
Epoch 10/20
34/34 [==============================] - 8s 249ms/step - loss: 1.4436 - categorical_accuracy: 0.3889 - val_loss: 6.9663 - val_categorical_accuracy: 0.2300
Epoch 00010: saving model to model_init_2018-10-2122_49_34.968496/model-00010-1.44356-0.38889-6.96633-0.23000.h5
Epoch 00010: ReduceLROnPlateau reducing learning rate to 6.25000029685907e-05.
Epoch 11/20
34/34 [==============================] - 9s 272ms/step - loss: 1.5062 - categorical_accuracy: 0.3693 - val_loss: 7.2006 - val_categorical_accuracy: 0.2300
Epoch 00011: saving model to model_init_2018-10-2122_49_34.968496/model-00011-1.50616-0.36928-7.20055-0.23000.h5
Epoch 12/20
34/34 [==============================] - 9s 259ms/step - loss: 1.5033 - categorical_accuracy: 0.3235 - val_loss: 7.0267 - val_categorical_accuracy: 0.2300
Epoch 00012: saving model to model_init_2018-10-2122_49_34.968496/model-00012-1.50335-0.32353-7.02673-0.23000.h5
Epoch 00012: ReduceLROnPlateau reducing learning rate to 3.125000148429535e-05.
Epoch 13/20
34/34 [==============================] - 9s 278ms/step - loss: 1.4653 - categorical_accuracy: 0.3758 - val_loss: 7.2299 - val_categorical_accuracy: 0.2300
Epoch 00013: saving model to model_init_2018-10-2122_49_34.968496/model-00013-1.46530-0.37582-7.22992-0.23000.h5
Epoch 14/20
34/34 [==============================] - 9s 252ms/step - loss: 1.5106 - categorical_accuracy: 0.3562 - val_loss: 7.0995 - val_categorical_accuracy: 0.2300
Epoch 00014: saving model to model_init_2018-10-2122_49_34.968496/model-00014-1.51057-0.35621-7.09950-0.23000.h5
Epoch 00014: ReduceLROnPlateau reducing learning rate to 1.5625000742147677e-05.
Epoch 15/20
34/34 [==============================] - 9s 265ms/step - loss: 1.4240 - categorical_accuracy: 0.3725 - val_loss: 7.0083 - val_categorical_accuracy: 0.2300
Epoch 00015: saving model to model_init_2018-10-2122_49_34.968496/model-00015-1.42399-0.37255-7.00832-0.23000.h5
Epoch 16/20
###Markdown
Model 5 Model 5a : Resize to 120*120, No agumentation, No flipped images, No normalisation, No cropping, No edge detection
###Code
train_gen = DataGenerator()
val_gen = DataGenerator()
model_gen = ModelGenerator()
input_shape = (30,120,120, 3)
num_classes = 5
model = model_gen.c3d5(input_shape, num_classes)
#batch_size = 20
#num_epochs = 20
train_generator = train_gen.generator(train_path, train_doc, batch_size)
val_generator = val_gen.generator(val_path, val_doc, batch_size)
train(batch_size, num_epochs, model, train_generator, val_generator)
###Output
# training sequences = 663
# validation sequences = 100
# batch size = 20
# epochs = 20
_________________________________________________________________
Layer (type) Output Shape Param #
=================================================================
conv3d_1 (Conv3D) (None, 30, 120, 120, 8) 656
_________________________________________________________________
activation_1 (Activation) (None, 30, 120, 120, 8) 0
_________________________________________________________________
dropout_1 (Dropout) (None, 30, 120, 120, 8) 0
_________________________________________________________________
max_pooling3d_1 (MaxPooling3 (None, 15, 60, 60, 8) 0
_________________________________________________________________
conv3d_2 (Conv3D) (None, 15, 60, 60, 16) 3472
_________________________________________________________________
activation_2 (Activation) (None, 15, 60, 60, 16) 0
_________________________________________________________________
dropout_2 (Dropout) (None, 15, 60, 60, 16) 0
_________________________________________________________________
max_pooling3d_2 (MaxPooling3 (None, 7, 30, 30, 16) 0
_________________________________________________________________
conv3d_3 (Conv3D) (None, 7, 30, 30, 32) 4640
_________________________________________________________________
activation_3 (Activation) (None, 7, 30, 30, 32) 0
_________________________________________________________________
dropout_3 (Dropout) (None, 7, 30, 30, 32) 0
_________________________________________________________________
max_pooling3d_3 (MaxPooling3 (None, 3, 15, 15, 32) 0
_________________________________________________________________
conv3d_4 (Conv3D) (None, 3, 15, 15, 64) 18496
_________________________________________________________________
activation_4 (Activation) (None, 3, 15, 15, 64) 0
_________________________________________________________________
dropout_4 (Dropout) (None, 3, 15, 15, 64) 0
_________________________________________________________________
max_pooling3d_4 (MaxPooling3 (None, 1, 7, 7, 64) 0
_________________________________________________________________
flatten_1 (Flatten) (None, 3136) 0
_________________________________________________________________
dense_1 (Dense) (None, 256) 803072
_________________________________________________________________
dropout_5 (Dropout) (None, 256) 0
_________________________________________________________________
dense_2 (Dense) (None, 128) 32896
_________________________________________________________________
dropout_6 (Dropout) (None, 128) 0
_________________________________________________________________
dense_3 (Dense) (None, 5) 645
=================================================================
Total params: 863,877
Trainable params: 863,877
Non-trainable params: 0
_________________________________________________________________
None
Epoch 1/20
Source path = ./Project_data/train ; batch size = 20
Source path = ./Project_data/val ; batch size = 20
34/34 [==============================] - 26s 769ms/step - loss: 12.6633 - categorical_accuracy: 0.2104 - val_loss: 12.7333 - val_categorical_accuracy: 0.2100
Epoch 00001: saving model to model_init_2018-10-2122_29_41.675097/model-00001-12.57732-0.21569-12.73329-0.21000.h5
Epoch 2/20
34/34 [==============================] - 4s 115ms/step - loss: 13.2737 - categorical_accuracy: 0.1765 - val_loss: 12.7333 - val_categorical_accuracy: 0.2100
Epoch 00002: saving model to model_init_2018-10-2122_29_41.675097/model-00002-13.27373-0.17647-12.73330-0.21000.h5
Epoch 3/20
34/34 [==============================] - 5s 133ms/step - loss: 12.3256 - categorical_accuracy: 0.2353 - val_loss: 12.7333 - val_categorical_accuracy: 0.2100
Epoch 00003: saving model to model_init_2018-10-2122_29_41.675097/model-00003-12.32560-0.23529-12.73330-0.21000.h5
Epoch 00003: ReduceLROnPlateau reducing learning rate to 0.0005000000237487257.
Epoch 4/20
34/34 [==============================] - 4s 127ms/step - loss: 12.3285 - categorical_accuracy: 0.2353 - val_loss: 12.4109 - val_categorical_accuracy: 0.2300
Epoch 00004: saving model to model_init_2018-10-2122_29_41.675097/model-00004-12.32855-0.23529-12.41093-0.23000.h5
Epoch 5/20
34/34 [==============================] - 4s 106ms/step - loss: 12.0096 - categorical_accuracy: 0.2549 - val_loss: 12.4109 - val_categorical_accuracy: 0.2300
Epoch 00005: saving model to model_init_2018-10-2122_29_41.675097/model-00005-12.00956-0.25490-12.41093-0.23000.h5
Epoch 6/20
34/34 [==============================] - 4s 104ms/step - loss: 12.9577 - categorical_accuracy: 0.1961 - val_loss: 12.4109 - val_categorical_accuracy: 0.2300
Epoch 00006: saving model to model_init_2018-10-2122_29_41.675097/model-00006-12.95768-0.19608-12.41093-0.23000.h5
Epoch 00006: ReduceLROnPlateau reducing learning rate to 0.0002500000118743628.
Epoch 7/20
34/34 [==============================] - 4s 112ms/step - loss: 13.1157 - categorical_accuracy: 0.1863 - val_loss: 12.4109 - val_categorical_accuracy: 0.2300
Epoch 00007: saving model to model_init_2018-10-2122_29_41.675097/model-00007-13.11570-0.18627-12.41093-0.23000.h5
Epoch 8/20
34/34 [==============================] - 4s 118ms/step - loss: 13.5775 - categorical_accuracy: 0.1569 - val_loss: 12.4109 - val_categorical_accuracy: 0.2300
Epoch 00008: saving model to model_init_2018-10-2122_29_41.675097/model-00008-13.57753-0.15686-12.41093-0.23000.h5
Epoch 00008: ReduceLROnPlateau reducing learning rate to 0.0001250000059371814.
Epoch 9/20
34/34 [==============================] - 5s 139ms/step - loss: 12.3256 - categorical_accuracy: 0.2353 - val_loss: 12.4109 - val_categorical_accuracy: 0.2300
Epoch 00009: saving model to model_init_2018-10-2122_29_41.675097/model-00009-12.32560-0.23529-12.41093-0.23000.h5
Epoch 10/20
34/34 [==============================] - 5s 144ms/step - loss: 11.3775 - categorical_accuracy: 0.2941 - val_loss: 12.4109 - val_categorical_accuracy: 0.2300
Epoch 00010: saving model to model_init_2018-10-2122_29_41.675097/model-00010-11.37748-0.29412-12.41093-0.23000.h5
Epoch 00010: ReduceLROnPlateau reducing learning rate to 6.25000029685907e-05.
Epoch 11/20
34/34 [==============================] - 5s 139ms/step - loss: 12.9577 - categorical_accuracy: 0.1961 - val_loss: 12.4109 - val_categorical_accuracy: 0.2300
Epoch 00011: saving model to model_init_2018-10-2122_29_41.675097/model-00011-12.95768-0.19608-12.41093-0.23000.h5
Epoch 12/20
34/34 [==============================] - 5s 143ms/step - loss: 13.4317 - categorical_accuracy: 0.1667 - val_loss: 12.4109 - val_categorical_accuracy: 0.2300
Epoch 00012: saving model to model_init_2018-10-2122_29_41.675097/model-00012-13.43175-0.16667-12.41093-0.23000.h5
Epoch 00012: ReduceLROnPlateau reducing learning rate to 3.125000148429535e-05.
Epoch 13/20
34/34 [==============================] - 5s 144ms/step - loss: 13.4317 - categorical_accuracy: 0.1667 - val_loss: 12.4109 - val_categorical_accuracy: 0.2300
Epoch 00013: saving model to model_init_2018-10-2122_29_41.675097/model-00013-13.43175-0.16667-12.41093-0.23000.h5
Epoch 14/20
34/34 [==============================] - 5s 141ms/step - loss: 13.2737 - categorical_accuracy: 0.1765 - val_loss: 12.4109 - val_categorical_accuracy: 0.2300
Epoch 00014: saving model to model_init_2018-10-2122_29_41.675097/model-00014-13.27373-0.17647-12.41093-0.23000.h5
Epoch 00014: ReduceLROnPlateau reducing learning rate to 1.5625000742147677e-05.
Epoch 15/20
34/34 [==============================] - 5s 141ms/step - loss: 13.7478 - categorical_accuracy: 0.1471 - val_loss: 12.4109 - val_categorical_accuracy: 0.2300
Epoch 00015: saving model to model_init_2018-10-2122_29_41.675097/model-00015-13.74779-0.14706-12.41093-0.23000.h5
Epoch 16/20
###Markdown
Model 5b : Resize to 120*120, agumentation, No flipped images, No normalisation, No cropping, No edge detection
###Code
train_gen = DataGenerator(affine=True)
val_gen = DataGenerator()
model_gen = ModelGenerator()
input_shape = (30,120,120, 3)
num_classes = 5
model = model_gen.c3d5(input_shape, num_classes)
#batch_size = 20
#num_epochs = 20
train_generator = train_gen.generator(train_path, train_doc, batch_size)
val_generator = val_gen.generator(val_path, val_doc, batch_size)
train(batch_size, num_epochs, model, train_generator, val_generator)
###Output
# training sequences = 663
# validation sequences = 100
# batch size = 20
# epochs = 20
_________________________________________________________________
Layer (type) Output Shape Param #
=================================================================
conv3d_1 (Conv3D) (None, 30, 120, 120, 8) 656
_________________________________________________________________
activation_1 (Activation) (None, 30, 120, 120, 8) 0
_________________________________________________________________
dropout_1 (Dropout) (None, 30, 120, 120, 8) 0
_________________________________________________________________
max_pooling3d_1 (MaxPooling3 (None, 15, 60, 60, 8) 0
_________________________________________________________________
conv3d_2 (Conv3D) (None, 15, 60, 60, 16) 3472
_________________________________________________________________
activation_2 (Activation) (None, 15, 60, 60, 16) 0
_________________________________________________________________
dropout_2 (Dropout) (None, 15, 60, 60, 16) 0
_________________________________________________________________
max_pooling3d_2 (MaxPooling3 (None, 7, 30, 30, 16) 0
_________________________________________________________________
conv3d_3 (Conv3D) (None, 7, 30, 30, 32) 4640
_________________________________________________________________
activation_3 (Activation) (None, 7, 30, 30, 32) 0
_________________________________________________________________
dropout_3 (Dropout) (None, 7, 30, 30, 32) 0
_________________________________________________________________
max_pooling3d_3 (MaxPooling3 (None, 3, 15, 15, 32) 0
_________________________________________________________________
conv3d_4 (Conv3D) (None, 3, 15, 15, 64) 18496
_________________________________________________________________
activation_4 (Activation) (None, 3, 15, 15, 64) 0
_________________________________________________________________
dropout_4 (Dropout) (None, 3, 15, 15, 64) 0
_________________________________________________________________
max_pooling3d_4 (MaxPooling3 (None, 1, 7, 7, 64) 0
_________________________________________________________________
flatten_1 (Flatten) (None, 3136) 0
_________________________________________________________________
dense_1 (Dense) (None, 256) 803072
_________________________________________________________________
dropout_5 (Dropout) (None, 256) 0
_________________________________________________________________
dense_2 (Dense) (None, 128) 32896
_________________________________________________________________
dropout_6 (Dropout) (None, 128) 0
_________________________________________________________________
dense_3 (Dense) (None, 5) 645
=================================================================
Total params: 863,877
Trainable params: 863,877
Non-trainable params: 0
_________________________________________________________________
None
Epoch 1/20
Source path = ./Project_data/train ; batch size = 20
Source path = ./Project_data/val ; batch size = 20
34/34 [==============================] - 32s 949ms/step - loss: 12.7285 - categorical_accuracy: 0.2090 - val_loss: 12.9711 - val_categorical_accuracy: 0.1500
Epoch 00001: saving model to model_init_2018-10-2122_31_35.281471/model-00001-12.64414-0.21418-12.97112-0.15000.h5
Epoch 2/20
34/34 [==============================] - 6s 179ms/step - loss: 12.4836 - categorical_accuracy: 0.2255 - val_loss: 12.7333 - val_categorical_accuracy: 0.2100
Epoch 00002: saving model to model_init_2018-10-2122_31_35.281471/model-00002-12.48362-0.22549-12.73330-0.21000.h5
Epoch 3/20
34/34 [==============================] - 6s 180ms/step - loss: 13.5898 - categorical_accuracy: 0.1569 - val_loss: 12.7333 - val_categorical_accuracy: 0.2100
Epoch 00003: saving model to model_init_2018-10-2122_31_35.281471/model-00003-13.58977-0.15686-12.73330-0.21000.h5
Epoch 4/20
34/34 [==============================] - 6s 177ms/step - loss: 13.6688 - categorical_accuracy: 0.1520 - val_loss: 12.7333 - val_categorical_accuracy: 0.2100
Epoch 00004: saving model to model_init_2018-10-2122_31_35.281471/model-00004-13.66878-0.15196-12.73330-0.21000.h5
Epoch 00004: ReduceLROnPlateau reducing learning rate to 0.0005000000237487257.
Epoch 5/20
34/34 [==============================] - 5s 143ms/step - loss: 13.1023 - categorical_accuracy: 0.1863 - val_loss: 12.7333 - val_categorical_accuracy: 0.2100
Epoch 00005: saving model to model_init_2018-10-2122_31_35.281471/model-00005-13.10227-0.18627-12.73330-0.21000.h5
Epoch 6/20
34/34 [==============================] - 6s 171ms/step - loss: 12.3980 - categorical_accuracy: 0.2304 - val_loss: 12.7333 - val_categorical_accuracy: 0.2100
Epoch 00006: saving model to model_init_2018-10-2122_31_35.281471/model-00006-12.39802-0.23039-12.73330-0.21000.h5
Epoch 00006: ReduceLROnPlateau reducing learning rate to 0.0002500000118743628.
Epoch 7/20
34/34 [==============================] - 5s 161ms/step - loss: 13.2521 - categorical_accuracy: 0.1765 - val_loss: 12.7333 - val_categorical_accuracy: 0.2100
Epoch 00007: saving model to model_init_2018-10-2122_31_35.281471/model-00007-13.25209-0.17647-12.73330-0.21000.h5
Epoch 8/20
34/34 [==============================] - 6s 179ms/step - loss: 12.4046 - categorical_accuracy: 0.2304 - val_loss: 12.7333 - val_categorical_accuracy: 0.2100
Epoch 00008: saving model to model_init_2018-10-2122_31_35.281471/model-00008-12.40461-0.23039-12.73330-0.21000.h5
Epoch 00008: ReduceLROnPlateau reducing learning rate to 0.0001250000059371814.
Epoch 9/20
34/34 [==============================] - 6s 185ms/step - loss: 12.4836 - categorical_accuracy: 0.2255 - val_loss: 12.7333 - val_categorical_accuracy: 0.2100
Epoch 00009: saving model to model_init_2018-10-2122_31_35.281471/model-00009-12.48362-0.22549-12.73330-0.21000.h5
Epoch 10/20
34/34 [==============================] - 6s 181ms/step - loss: 12.4836 - categorical_accuracy: 0.2255 - val_loss: 12.7333 - val_categorical_accuracy: 0.2100
Epoch 00010: saving model to model_init_2018-10-2122_31_35.281471/model-00010-12.48362-0.22549-12.73330-0.21000.h5
Epoch 00010: ReduceLROnPlateau reducing learning rate to 6.25000029685907e-05.
Epoch 11/20
34/34 [==============================] - 6s 169ms/step - loss: 12.3256 - categorical_accuracy: 0.2353 - val_loss: 12.7333 - val_categorical_accuracy: 0.2100
Epoch 00011: saving model to model_init_2018-10-2122_31_35.281471/model-00011-12.32560-0.23529-12.73330-0.21000.h5
Epoch 12/20
34/34 [==============================] - 6s 168ms/step - loss: 12.1676 - categorical_accuracy: 0.2451 - val_loss: 12.7333 - val_categorical_accuracy: 0.2100
Epoch 00012: saving model to model_init_2018-10-2122_31_35.281471/model-00012-12.16758-0.24510-12.73330-0.21000.h5
Epoch 00012: ReduceLROnPlateau reducing learning rate to 3.125000148429535e-05.
Epoch 13/20
34/34 [==============================] - 5s 157ms/step - loss: 13.5898 - categorical_accuracy: 0.1569 - val_loss: 12.7333 - val_categorical_accuracy: 0.2100
Epoch 00013: saving model to model_init_2018-10-2122_31_35.281471/model-00013-13.58977-0.15686-12.73330-0.21000.h5
Epoch 14/20
34/34 [==============================] - 6s 173ms/step - loss: 13.1947 - categorical_accuracy: 0.1814 - val_loss: 12.7333 - val_categorical_accuracy: 0.2100
Epoch 00014: saving model to model_init_2018-10-2122_31_35.281471/model-00014-13.19472-0.18137-12.73330-0.21000.h5
Epoch 00014: ReduceLROnPlateau reducing learning rate to 1.5625000742147677e-05.
Epoch 15/20
34/34 [==============================] - 6s 166ms/step - loss: 13.2737 - categorical_accuracy: 0.1765 - val_loss: 12.7333 - val_categorical_accuracy: 0.2100
Epoch 00015: saving model to model_init_2018-10-2122_31_35.281471/model-00015-13.27373-0.17647-12.73330-0.21000.h5
Epoch 16/20
###Markdown
Model 5c : Resize to 120*120, agumentation, flipped images, No normalisation, No cropping, No edge detection
###Code
train_gen = DataGenerator(affine=True, flip=True)
val_gen = DataGenerator()
model_gen = ModelGenerator()
input_shape = (30,120,120, 3)
num_classes = 5
model = model_gen.c3d5(input_shape, num_classes)
#batch_size = 20
#num_epochs = 20
train_generator = train_gen.generator(train_path, train_doc, batch_size)
val_generator = val_gen.generator(val_path, val_doc, batch_size)
train(batch_size, num_epochs, model, train_generator, val_generator)
###Output
# training sequences = 663
# validation sequences = 100
# batch size = 20
# epochs = 20
_________________________________________________________________
Layer (type) Output Shape Param #
=================================================================
conv3d_1 (Conv3D) (None, 30, 120, 120, 8) 656
_________________________________________________________________
activation_1 (Activation) (None, 30, 120, 120, 8) 0
_________________________________________________________________
dropout_1 (Dropout) (None, 30, 120, 120, 8) 0
_________________________________________________________________
max_pooling3d_1 (MaxPooling3 (None, 15, 60, 60, 8) 0
_________________________________________________________________
conv3d_2 (Conv3D) (None, 15, 60, 60, 16) 3472
_________________________________________________________________
activation_2 (Activation) (None, 15, 60, 60, 16) 0
_________________________________________________________________
dropout_2 (Dropout) (None, 15, 60, 60, 16) 0
_________________________________________________________________
max_pooling3d_2 (MaxPooling3 (None, 7, 30, 30, 16) 0
_________________________________________________________________
conv3d_3 (Conv3D) (None, 7, 30, 30, 32) 4640
_________________________________________________________________
activation_3 (Activation) (None, 7, 30, 30, 32) 0
_________________________________________________________________
dropout_3 (Dropout) (None, 7, 30, 30, 32) 0
_________________________________________________________________
max_pooling3d_3 (MaxPooling3 (None, 3, 15, 15, 32) 0
_________________________________________________________________
conv3d_4 (Conv3D) (None, 3, 15, 15, 64) 18496
_________________________________________________________________
activation_4 (Activation) (None, 3, 15, 15, 64) 0
_________________________________________________________________
dropout_4 (Dropout) (None, 3, 15, 15, 64) 0
_________________________________________________________________
max_pooling3d_4 (MaxPooling3 (None, 1, 7, 7, 64) 0
_________________________________________________________________
flatten_1 (Flatten) (None, 3136) 0
_________________________________________________________________
dense_1 (Dense) (None, 256) 803072
_________________________________________________________________
dropout_5 (Dropout) (None, 256) 0
_________________________________________________________________
dense_2 (Dense) (None, 128) 32896
_________________________________________________________________
dropout_6 (Dropout) (None, 128) 0
_________________________________________________________________
dense_3 (Dense) (None, 5) 645
=================================================================
Total params: 863,877
Trainable params: 863,877
Non-trainable params: 0
_________________________________________________________________
None
Epoch 1/20
Source path = ./Project_data/train ; batch size = 20
Source path = ./Project_data/val ; batch size = 20
34/34 [==============================] - 39s 1s/step - loss: 12.7549 - categorical_accuracy: 0.2063 - val_loss: 12.7333 - val_categorical_accuracy: 0.2100
Epoch 00001: saving model to model_init_2018-10-2122_34_00.297517/model-00001-12.80494-0.20312-12.73330-0.21000.h5
Epoch 2/20
34/34 [==============================] - 7s 194ms/step - loss: 13.0630 - categorical_accuracy: 0.1895 - val_loss: 12.7333 - val_categorical_accuracy: 0.2100
Epoch 00002: saving model to model_init_2018-10-2122_34_00.297517/model-00002-13.06303-0.18954-12.73330-0.21000.h5
Epoch 3/20
34/34 [==============================] - 6s 190ms/step - loss: 12.8523 - categorical_accuracy: 0.2026 - val_loss: 12.7333 - val_categorical_accuracy: 0.2100
Epoch 00003: saving model to model_init_2018-10-2122_34_00.297517/model-00003-12.85234-0.20261-12.73330-0.21000.h5
Epoch 00003: ReduceLROnPlateau reducing learning rate to 0.0005000000237487257.
Epoch 4/20
34/34 [==============================] - 7s 204ms/step - loss: 13.2737 - categorical_accuracy: 0.1765 - val_loss: 12.7333 - val_categorical_accuracy: 0.2100
Epoch 00004: saving model to model_init_2018-10-2122_34_00.297517/model-00004-13.27373-0.17647-12.73330-0.21000.h5
Epoch 5/20
34/34 [==============================] - 7s 215ms/step - loss: 13.2737 - categorical_accuracy: 0.1765 - val_loss: 12.7333 - val_categorical_accuracy: 0.2100
Epoch 00005: saving model to model_init_2018-10-2122_34_00.297517/model-00005-13.27373-0.17647-12.73330-0.21000.h5
Epoch 00005: ReduceLROnPlateau reducing learning rate to 0.0002500000118743628.
Epoch 6/20
34/34 [==============================] - 7s 208ms/step - loss: 12.7191 - categorical_accuracy: 0.2092 - val_loss: 12.7333 - val_categorical_accuracy: 0.2100
Epoch 00006: saving model to model_init_2018-10-2122_34_00.297517/model-00006-12.71910-0.20915-12.73330-0.21000.h5
Epoch 7/20
34/34 [==============================] - 7s 208ms/step - loss: 12.7470 - categorical_accuracy: 0.2092 - val_loss: 12.7333 - val_categorical_accuracy: 0.2100
Epoch 00007: saving model to model_init_2018-10-2122_34_00.297517/model-00007-12.74700-0.20915-12.73330-0.21000.h5
Epoch 00007: ReduceLROnPlateau reducing learning rate to 0.0001250000059371814.
Epoch 8/20
34/34 [==============================] - 7s 218ms/step - loss: 12.0096 - categorical_accuracy: 0.2549 - val_loss: 12.7333 - val_categorical_accuracy: 0.2100
Epoch 00008: saving model to model_init_2018-10-2122_34_00.297517/model-00008-12.00956-0.25490-12.73330-0.21000.h5
Epoch 9/20
34/34 [==============================] - 7s 210ms/step - loss: 13.8015 - categorical_accuracy: 0.1438 - val_loss: 12.7333 - val_categorical_accuracy: 0.2100
Epoch 00009: saving model to model_init_2018-10-2122_34_00.297517/model-00009-13.80153-0.14379-12.73330-0.21000.h5
Epoch 00009: ReduceLROnPlateau reducing learning rate to 6.25000029685907e-05.
Epoch 10/20
34/34 [==============================] - 7s 204ms/step - loss: 13.2737 - categorical_accuracy: 0.1765 - val_loss: 12.7333 - val_categorical_accuracy: 0.2100
Epoch 00010: saving model to model_init_2018-10-2122_34_00.297517/model-00010-13.27373-0.17647-12.73330-0.21000.h5
Epoch 11/20
34/34 [==============================] - 7s 218ms/step - loss: 12.6943 - categorical_accuracy: 0.2124 - val_loss: 12.7333 - val_categorical_accuracy: 0.2100
Epoch 00011: saving model to model_init_2018-10-2122_34_00.297517/model-00011-12.69432-0.21242-12.73330-0.21000.h5
Epoch 00011: ReduceLROnPlateau reducing learning rate to 3.125000148429535e-05.
Epoch 12/20
34/34 [==============================] - 7s 201ms/step - loss: 12.4836 - categorical_accuracy: 0.2255 - val_loss: 12.7333 - val_categorical_accuracy: 0.2100
Epoch 00012: saving model to model_init_2018-10-2122_34_00.297517/model-00012-12.48362-0.22549-12.73330-0.21000.h5
Epoch 13/20
34/34 [==============================] - 6s 191ms/step - loss: 12.1676 - categorical_accuracy: 0.2451 - val_loss: 12.7333 - val_categorical_accuracy: 0.2100
Epoch 00013: saving model to model_init_2018-10-2122_34_00.297517/model-00013-12.16758-0.24510-12.73330-0.21000.h5
Epoch 00013: ReduceLROnPlateau reducing learning rate to 1.5625000742147677e-05.
Epoch 14/20
34/34 [==============================] - 7s 218ms/step - loss: 12.6953 - categorical_accuracy: 0.2092 - val_loss: 12.7333 - val_categorical_accuracy: 0.2100
Epoch 00014: saving model to model_init_2018-10-2122_34_00.297517/model-00014-12.69532-0.20915-12.73330-0.21000.h5
Epoch 15/20
34/34 [==============================] - 7s 207ms/step - loss: 12.4309 - categorical_accuracy: 0.2288 - val_loss: 12.7333 - val_categorical_accuracy: 0.2100
Epoch 00015: saving model to model_init_2018-10-2122_34_00.297517/model-00015-12.43095-0.22876-12.73330-0.21000.h5
Epoch 00015: ReduceLROnPlateau reducing learning rate to 7.812500371073838e-06.
Epoch 16/20
###Markdown
Model 5d : Resize to 120*120, agumentation, flipped images, normalisation, No cropping, No edge detection
###Code
train_gen = DataGenerator(affine=True, flip=True, normalize=True)
val_gen = DataGenerator()
model_gen = ModelGenerator()
input_shape = (30,120,120, 3)
num_classes = 5
model = model_gen.c3d5(input_shape, num_classes)
#batch_size = 20
#num_epochs = 20
train_generator = train_gen.generator(train_path, train_doc, batch_size)
val_generator = val_gen.generator(val_path, val_doc, batch_size)
train(batch_size, num_epochs, model, train_generator, val_generator)
###Output
# training sequences = 663
# validation sequences = 100
# batch size = 20
# epochs = 20
_________________________________________________________________
Layer (type) Output Shape Param #
=================================================================
conv3d_1 (Conv3D) (None, 30, 120, 120, 8) 656
_________________________________________________________________
activation_1 (Activation) (None, 30, 120, 120, 8) 0
_________________________________________________________________
dropout_1 (Dropout) (None, 30, 120, 120, 8) 0
_________________________________________________________________
max_pooling3d_1 (MaxPooling3 (None, 15, 60, 60, 8) 0
_________________________________________________________________
conv3d_2 (Conv3D) (None, 15, 60, 60, 16) 3472
_________________________________________________________________
activation_2 (Activation) (None, 15, 60, 60, 16) 0
_________________________________________________________________
dropout_2 (Dropout) (None, 15, 60, 60, 16) 0
_________________________________________________________________
max_pooling3d_2 (MaxPooling3 (None, 7, 30, 30, 16) 0
_________________________________________________________________
conv3d_3 (Conv3D) (None, 7, 30, 30, 32) 4640
_________________________________________________________________
activation_3 (Activation) (None, 7, 30, 30, 32) 0
_________________________________________________________________
dropout_3 (Dropout) (None, 7, 30, 30, 32) 0
_________________________________________________________________
max_pooling3d_3 (MaxPooling3 (None, 3, 15, 15, 32) 0
_________________________________________________________________
conv3d_4 (Conv3D) (None, 3, 15, 15, 64) 18496
_________________________________________________________________
activation_4 (Activation) (None, 3, 15, 15, 64) 0
_________________________________________________________________
dropout_4 (Dropout) (None, 3, 15, 15, 64) 0
_________________________________________________________________
max_pooling3d_4 (MaxPooling3 (None, 1, 7, 7, 64) 0
_________________________________________________________________
flatten_1 (Flatten) (None, 3136) 0
_________________________________________________________________
dense_1 (Dense) (None, 256) 803072
_________________________________________________________________
dropout_5 (Dropout) (None, 256) 0
_________________________________________________________________
dense_2 (Dense) (None, 128) 32896
_________________________________________________________________
dropout_6 (Dropout) (None, 128) 0
_________________________________________________________________
dense_3 (Dense) (None, 5) 645
=================================================================
Total params: 863,877
Trainable params: 863,877
Non-trainable params: 0
_________________________________________________________________
None
Epoch 1/20
Source path = ./Project_data/val ; batch size = 20
Source path = ./Project_data/train ; batch size = 20
34/34 [==============================] - 41s 1s/step - loss: 1.6472 - categorical_accuracy: 0.2178 - val_loss: 12.4109 - val_categorical_accuracy: 0.2300
Epoch 00001: saving model to model_init_2018-10-2122_36_55.673460/model-00001-1.65032-0.21217-12.41093-0.23000.h5
Epoch 2/20
34/34 [==============================] - 6s 189ms/step - loss: 1.5888 - categorical_accuracy: 0.2614 - val_loss: 8.7788 - val_categorical_accuracy: 0.2300
Epoch 00002: saving model to model_init_2018-10-2122_36_55.673460/model-00002-1.58879-0.26144-8.77878-0.23000.h5
Epoch 3/20
34/34 [==============================] - 7s 195ms/step - loss: 1.6041 - categorical_accuracy: 0.2157 - val_loss: 4.0235 - val_categorical_accuracy: 0.2500
Epoch 00003: saving model to model_init_2018-10-2122_36_55.673460/model-00003-1.60410-0.21569-4.02345-0.25000.h5
Epoch 4/20
34/34 [==============================] - 7s 207ms/step - loss: 1.6037 - categorical_accuracy: 0.2516 - val_loss: 9.9986 - val_categorical_accuracy: 0.1800
Epoch 00004: saving model to model_init_2018-10-2122_36_55.673460/model-00004-1.60373-0.25163-9.99860-0.18000.h5
Epoch 5/20
34/34 [==============================] - 7s 219ms/step - loss: 1.5491 - categorical_accuracy: 0.2876 - val_loss: 6.2645 - val_categorical_accuracy: 0.3600
Epoch 00005: saving model to model_init_2018-10-2122_36_55.673460/model-00005-1.54908-0.28758-6.26453-0.36000.h5
Epoch 00005: ReduceLROnPlateau reducing learning rate to 0.0005000000237487257.
Epoch 6/20
34/34 [==============================] - 7s 204ms/step - loss: 1.5083 - categorical_accuracy: 0.3529 - val_loss: 8.9215 - val_categorical_accuracy: 0.3600
Epoch 00006: saving model to model_init_2018-10-2122_36_55.673460/model-00006-1.50831-0.35294-8.92153-0.36000.h5
Epoch 7/20
34/34 [==============================] - 6s 185ms/step - loss: 1.4791 - categorical_accuracy: 0.3464 - val_loss: 9.6920 - val_categorical_accuracy: 0.3900
Epoch 00007: saving model to model_init_2018-10-2122_36_55.673460/model-00007-1.47913-0.34641-9.69197-0.39000.h5
Epoch 00007: ReduceLROnPlateau reducing learning rate to 0.0002500000118743628.
Epoch 8/20
34/34 [==============================] - 7s 195ms/step - loss: 1.3545 - categorical_accuracy: 0.4183 - val_loss: 9.0794 - val_categorical_accuracy: 0.4200
Epoch 00008: saving model to model_init_2018-10-2122_36_55.673460/model-00008-1.35454-0.41830-9.07940-0.42000.h5
Epoch 9/20
34/34 [==============================] - 7s 205ms/step - loss: 1.3377 - categorical_accuracy: 0.3693 - val_loss: 7.0047 - val_categorical_accuracy: 0.5200
Epoch 00009: saving model to model_init_2018-10-2122_36_55.673460/model-00009-1.33769-0.36928-7.00467-0.52000.h5
Epoch 00009: ReduceLROnPlateau reducing learning rate to 0.0001250000059371814.
Epoch 10/20
34/34 [==============================] - 7s 215ms/step - loss: 1.2744 - categorical_accuracy: 0.4673 - val_loss: 9.6709 - val_categorical_accuracy: 0.4000
Epoch 00010: saving model to model_init_2018-10-2122_36_55.673460/model-00010-1.27441-0.46732-9.67086-0.40000.h5
Epoch 11/20
34/34 [==============================] - 8s 224ms/step - loss: 1.2480 - categorical_accuracy: 0.4542 - val_loss: 9.4313 - val_categorical_accuracy: 0.4000
Epoch 00011: saving model to model_init_2018-10-2122_36_55.673460/model-00011-1.24805-0.45425-9.43131-0.40000.h5
Epoch 00011: ReduceLROnPlateau reducing learning rate to 6.25000029685907e-05.
Epoch 12/20
34/34 [==============================] - 7s 200ms/step - loss: 1.2046 - categorical_accuracy: 0.5065 - val_loss: 9.4112 - val_categorical_accuracy: 0.4000
Epoch 00012: saving model to model_init_2018-10-2122_36_55.673460/model-00012-1.20464-0.50654-9.41120-0.40000.h5
Epoch 13/20
34/34 [==============================] - 7s 203ms/step - loss: 1.1524 - categorical_accuracy: 0.5000 - val_loss: 9.0066 - val_categorical_accuracy: 0.4200
Epoch 00013: saving model to model_init_2018-10-2122_36_55.673460/model-00013-1.15244-0.50000-9.00660-0.42000.h5
Epoch 00013: ReduceLROnPlateau reducing learning rate to 3.125000148429535e-05.
Epoch 14/20
34/34 [==============================] - 6s 189ms/step - loss: 1.2207 - categorical_accuracy: 0.4739 - val_loss: 9.3730 - val_categorical_accuracy: 0.4000
Epoch 00014: saving model to model_init_2018-10-2122_36_55.673460/model-00014-1.22073-0.47386-9.37300-0.40000.h5
Epoch 15/20
34/34 [==============================] - 7s 218ms/step - loss: 1.0922 - categorical_accuracy: 0.5294 - val_loss: 9.4957 - val_categorical_accuracy: 0.3900
Epoch 00015: saving model to model_init_2018-10-2122_36_55.673460/model-00015-1.09217-0.52941-9.49572-0.39000.h5
Epoch 00015: ReduceLROnPlateau reducing learning rate to 1.5625000742147677e-05.
Epoch 16/20
###Markdown
Model 5e : Resize to 120*120, agumentation, flipped images, normalisation, cropping, No edge detection
###Code
train_gen = DataGenerator(affine=True, flip=True, normalize=True, crop=True)
val_gen = DataGenerator()
model_gen = ModelGenerator()
input_shape = (30,120,120, 3)
num_classes = 5
model = model_gen.c3d5(input_shape, num_classes)
#batch_size = 20
#num_epochs = 20
train_generator = train_gen.generator(train_path, train_doc, batch_size)
val_generator = val_gen.generator(val_path, val_doc, batch_size)
train(batch_size, num_epochs, model, train_generator, val_generator)
###Output
# training sequences = 663
# validation sequences = 100
# batch size = 20
# epochs = 20
_________________________________________________________________
Layer (type) Output Shape Param #
=================================================================
conv3d_1 (Conv3D) (None, 30, 120, 120, 8) 656
_________________________________________________________________
activation_1 (Activation) (None, 30, 120, 120, 8) 0
_________________________________________________________________
dropout_1 (Dropout) (None, 30, 120, 120, 8) 0
_________________________________________________________________
max_pooling3d_1 (MaxPooling3 (None, 15, 60, 60, 8) 0
_________________________________________________________________
conv3d_2 (Conv3D) (None, 15, 60, 60, 16) 3472
_________________________________________________________________
activation_2 (Activation) (None, 15, 60, 60, 16) 0
_________________________________________________________________
dropout_2 (Dropout) (None, 15, 60, 60, 16) 0
_________________________________________________________________
max_pooling3d_2 (MaxPooling3 (None, 7, 30, 30, 16) 0
_________________________________________________________________
conv3d_3 (Conv3D) (None, 7, 30, 30, 32) 4640
_________________________________________________________________
activation_3 (Activation) (None, 7, 30, 30, 32) 0
_________________________________________________________________
dropout_3 (Dropout) (None, 7, 30, 30, 32) 0
_________________________________________________________________
max_pooling3d_3 (MaxPooling3 (None, 3, 15, 15, 32) 0
_________________________________________________________________
conv3d_4 (Conv3D) (None, 3, 15, 15, 64) 18496
_________________________________________________________________
activation_4 (Activation) (None, 3, 15, 15, 64) 0
_________________________________________________________________
dropout_4 (Dropout) (None, 3, 15, 15, 64) 0
_________________________________________________________________
max_pooling3d_4 (MaxPooling3 (None, 1, 7, 7, 64) 0
_________________________________________________________________
flatten_1 (Flatten) (None, 3136) 0
_________________________________________________________________
dense_1 (Dense) (None, 256) 803072
_________________________________________________________________
dropout_5 (Dropout) (None, 256) 0
_________________________________________________________________
dense_2 (Dense) (None, 128) 32896
_________________________________________________________________
dropout_6 (Dropout) (None, 128) 0
_________________________________________________________________
dense_3 (Dense) (None, 5) 645
=================================================================
Total params: 863,877
Trainable params: 863,877
Non-trainable params: 0
_________________________________________________________________
None
Epoch 1/20
Source path = ./Project_data/val ; batch size = 20
Source path = ./Project_data/train ; batch size = 20
34/34 [==============================] - 40s 1s/step - loss: 1.6260 - categorical_accuracy: 0.2016 - val_loss: 9.5488 - val_categorical_accuracy: 0.1800
Epoch 00001: saving model to model_init_2018-10-2122_39_49.545996/model-00001-1.62522-0.20664-9.54876-0.18000.h5
Epoch 2/20
34/34 [==============================] - 7s 205ms/step - loss: 1.5888 - categorical_accuracy: 0.2712 - val_loss: 10.0854 - val_categorical_accuracy: 0.2200
Epoch 00002: saving model to model_init_2018-10-2122_39_49.545996/model-00002-1.58879-0.27124-10.08535-0.22000.h5
Epoch 3/20
34/34 [==============================] - 7s 208ms/step - loss: 1.5213 - categorical_accuracy: 0.3039 - val_loss: 11.4701 - val_categorical_accuracy: 0.2300
Epoch 00003: saving model to model_init_2018-10-2122_39_49.545996/model-00003-1.52134-0.30392-11.47012-0.23000.h5
Epoch 00003: ReduceLROnPlateau reducing learning rate to 0.0005000000237487257.
Epoch 4/20
34/34 [==============================] - 6s 189ms/step - loss: 1.5586 - categorical_accuracy: 0.3301 - val_loss: 9.7992 - val_categorical_accuracy: 0.3200
Epoch 00004: saving model to model_init_2018-10-2122_39_49.545996/model-00004-1.55862-0.33007-9.79915-0.32000.h5
Epoch 5/20
34/34 [==============================] - 7s 202ms/step - loss: 1.4901 - categorical_accuracy: 0.3366 - val_loss: 12.0286 - val_categorical_accuracy: 0.2200
Epoch 00005: saving model to model_init_2018-10-2122_39_49.545996/model-00005-1.49008-0.33660-12.02862-0.22000.h5
Epoch 00005: ReduceLROnPlateau reducing learning rate to 0.0002500000118743628.
Epoch 6/20
34/34 [==============================] - 6s 179ms/step - loss: 1.4203 - categorical_accuracy: 0.3562 - val_loss: 10.3346 - val_categorical_accuracy: 0.3300
Epoch 00006: saving model to model_init_2018-10-2122_39_49.545996/model-00006-1.42031-0.35621-10.33456-0.33000.h5
Epoch 7/20
34/34 [==============================] - 7s 213ms/step - loss: 1.4565 - categorical_accuracy: 0.3693 - val_loss: 10.4382 - val_categorical_accuracy: 0.3200
Epoch 00007: saving model to model_init_2018-10-2122_39_49.545996/model-00007-1.45653-0.36928-10.43820-0.32000.h5
Epoch 00007: ReduceLROnPlateau reducing learning rate to 0.0001250000059371814.
Epoch 8/20
34/34 [==============================] - 7s 215ms/step - loss: 1.3131 - categorical_accuracy: 0.4444 - val_loss: 10.7587 - val_categorical_accuracy: 0.3000
Epoch 00008: saving model to model_init_2018-10-2122_39_49.545996/model-00008-1.31312-0.44444-10.75871-0.30000.h5
Epoch 9/20
34/34 [==============================] - 7s 203ms/step - loss: 1.3650 - categorical_accuracy: 0.4346 - val_loss: 10.6145 - val_categorical_accuracy: 0.3200
Epoch 00009: saving model to model_init_2018-10-2122_39_49.545996/model-00009-1.36505-0.43464-10.61453-0.32000.h5
Epoch 00009: ReduceLROnPlateau reducing learning rate to 6.25000029685907e-05.
Epoch 10/20
34/34 [==============================] - 7s 217ms/step - loss: 1.3558 - categorical_accuracy: 0.4216 - val_loss: 10.4348 - val_categorical_accuracy: 0.3300
Epoch 00010: saving model to model_init_2018-10-2122_39_49.545996/model-00010-1.35576-0.42157-10.43482-0.33000.h5
Epoch 11/20
34/34 [==============================] - 7s 194ms/step - loss: 1.2342 - categorical_accuracy: 0.4869 - val_loss: 10.3179 - val_categorical_accuracy: 0.3600
Epoch 00011: saving model to model_init_2018-10-2122_39_49.545996/model-00011-1.23423-0.48693-10.31792-0.36000.h5
Epoch 00011: ReduceLROnPlateau reducing learning rate to 3.125000148429535e-05.
Epoch 12/20
34/34 [==============================] - 7s 198ms/step - loss: 1.3025 - categorical_accuracy: 0.4967 - val_loss: 10.3196 - val_categorical_accuracy: 0.3600
Epoch 00012: saving model to model_init_2018-10-2122_39_49.545996/model-00012-1.30252-0.49673-10.31961-0.36000.h5
Epoch 13/20
34/34 [==============================] - 7s 220ms/step - loss: 1.2961 - categorical_accuracy: 0.4183 - val_loss: 10.3175 - val_categorical_accuracy: 0.3600
Epoch 00013: saving model to model_init_2018-10-2122_39_49.545996/model-00013-1.29606-0.41830-10.31752-0.36000.h5
Epoch 00013: ReduceLROnPlateau reducing learning rate to 1.5625000742147677e-05.
Epoch 14/20
34/34 [==============================] - 7s 203ms/step - loss: 1.2302 - categorical_accuracy: 0.4575 - val_loss: 10.3198 - val_categorical_accuracy: 0.3600
Epoch 00014: saving model to model_init_2018-10-2122_39_49.545996/model-00014-1.23017-0.45752-10.31981-0.36000.h5
Epoch 15/20
34/34 [==============================] - 7s 219ms/step - loss: 1.3058 - categorical_accuracy: 0.4477 - val_loss: 10.3255 - val_categorical_accuracy: 0.3600
Epoch 00015: saving model to model_init_2018-10-2122_39_49.545996/model-00015-1.30581-0.44771-10.32549-0.36000.h5
Epoch 00015: ReduceLROnPlateau reducing learning rate to 7.812500371073838e-06.
Epoch 16/20
###Markdown
Model 5f : Resize to 120*120, All
###Code
train_gen = DataGenerator(affine=True, flip=True, normalize=True, crop=True, edge=True)
val_gen = DataGenerator()
model_gen = ModelGenerator()
input_shape = (30,120,120, 3)
num_classes = 5
model = model_gen.c3d5(input_shape, num_classes)
#batch_size = 20
#num_epochs = 20
train_generator = train_gen.generator(train_path, train_doc, batch_size)
val_generator = val_gen.generator(val_path, val_doc, batch_size)
train(batch_size, num_epochs, model, train_generator, val_generator)
###Output
# training sequences = 663
# validation sequences = 100
# batch size = 20
# epochs = 20
_________________________________________________________________
Layer (type) Output Shape Param #
=================================================================
conv3d_1 (Conv3D) (None, 30, 120, 120, 8) 656
_________________________________________________________________
activation_1 (Activation) (None, 30, 120, 120, 8) 0
_________________________________________________________________
dropout_1 (Dropout) (None, 30, 120, 120, 8) 0
_________________________________________________________________
max_pooling3d_1 (MaxPooling3 (None, 15, 60, 60, 8) 0
_________________________________________________________________
conv3d_2 (Conv3D) (None, 15, 60, 60, 16) 3472
_________________________________________________________________
activation_2 (Activation) (None, 15, 60, 60, 16) 0
_________________________________________________________________
dropout_2 (Dropout) (None, 15, 60, 60, 16) 0
_________________________________________________________________
max_pooling3d_2 (MaxPooling3 (None, 7, 30, 30, 16) 0
_________________________________________________________________
conv3d_3 (Conv3D) (None, 7, 30, 30, 32) 4640
_________________________________________________________________
activation_3 (Activation) (None, 7, 30, 30, 32) 0
_________________________________________________________________
dropout_3 (Dropout) (None, 7, 30, 30, 32) 0
_________________________________________________________________
max_pooling3d_3 (MaxPooling3 (None, 3, 15, 15, 32) 0
_________________________________________________________________
conv3d_4 (Conv3D) (None, 3, 15, 15, 64) 18496
_________________________________________________________________
activation_4 (Activation) (None, 3, 15, 15, 64) 0
_________________________________________________________________
dropout_4 (Dropout) (None, 3, 15, 15, 64) 0
_________________________________________________________________
max_pooling3d_4 (MaxPooling3 (None, 1, 7, 7, 64) 0
_________________________________________________________________
flatten_1 (Flatten) (None, 3136) 0
_________________________________________________________________
dense_1 (Dense) (None, 256) 803072
_________________________________________________________________
dropout_5 (Dropout) (None, 256) 0
_________________________________________________________________
dense_2 (Dense) (None, 128) 32896
_________________________________________________________________
dropout_6 (Dropout) (None, 128) 0
_________________________________________________________________
dense_3 (Dense) (None, 5) 645
=================================================================
Total params: 863,877
Trainable params: 863,877
Non-trainable params: 0
_________________________________________________________________
None
Epoch 1/20
Source path = ./Project_data/train ; batch size = 20
Source path = ./Project_data/val ; batch size = 20
34/34 [==============================] - 47s 1s/step - loss: 1.6120 - categorical_accuracy: 0.1935 - val_loss: 8.1142 - val_categorical_accuracy: 0.2300
Epoch 00001: saving model to model_init_2018-10-2122_42_43.394553/model-00001-1.61146-0.19558-8.11422-0.23000.h5
Epoch 2/20
34/34 [==============================] - 7s 214ms/step - loss: 1.5764 - categorical_accuracy: 0.2582 - val_loss: 12.1885 - val_categorical_accuracy: 0.2300
Epoch 00002: saving model to model_init_2018-10-2122_42_43.394553/model-00002-1.57642-0.25817-12.18854-0.23000.h5
Epoch 3/20
34/34 [==============================] - 8s 245ms/step - loss: 1.5651 - categorical_accuracy: 0.2614 - val_loss: 12.4109 - val_categorical_accuracy: 0.2300
Epoch 00003: saving model to model_init_2018-10-2122_42_43.394553/model-00003-1.56513-0.26144-12.41093-0.23000.h5
Epoch 00003: ReduceLROnPlateau reducing learning rate to 0.0005000000237487257.
Epoch 4/20
34/34 [==============================] - 8s 244ms/step - loss: 1.5458 - categorical_accuracy: 0.3137 - val_loss: 12.4109 - val_categorical_accuracy: 0.2300
Epoch 00004: saving model to model_init_2018-10-2122_42_43.394553/model-00004-1.54578-0.31373-12.41093-0.23000.h5
Epoch 5/20
34/34 [==============================] - 8s 233ms/step - loss: 1.4490 - categorical_accuracy: 0.3529 - val_loss: 12.4109 - val_categorical_accuracy: 0.2300
Epoch 00005: saving model to model_init_2018-10-2122_42_43.394553/model-00005-1.44904-0.35294-12.41093-0.23000.h5
Epoch 00005: ReduceLROnPlateau reducing learning rate to 0.0002500000118743628.
Epoch 6/20
34/34 [==============================] - 8s 230ms/step - loss: 1.4577 - categorical_accuracy: 0.2941 - val_loss: 12.1257 - val_categorical_accuracy: 0.2300
Epoch 00006: saving model to model_init_2018-10-2122_42_43.394553/model-00006-1.45771-0.29412-12.12568-0.23000.h5
Epoch 7/20
34/34 [==============================] - 8s 232ms/step - loss: 1.4267 - categorical_accuracy: 0.3301 - val_loss: 12.4109 - val_categorical_accuracy: 0.2300
Epoch 00007: saving model to model_init_2018-10-2122_42_43.394553/model-00007-1.42673-0.33007-12.41093-0.23000.h5
Epoch 00007: ReduceLROnPlateau reducing learning rate to 0.0001250000059371814.
Epoch 8/20
34/34 [==============================] - 8s 234ms/step - loss: 1.3577 - categorical_accuracy: 0.4542 - val_loss: 12.4109 - val_categorical_accuracy: 0.2300
Epoch 00008: saving model to model_init_2018-10-2122_42_43.394553/model-00008-1.35767-0.45425-12.41093-0.23000.h5
Epoch 9/20
34/34 [==============================] - 7s 219ms/step - loss: 1.3708 - categorical_accuracy: 0.3464 - val_loss: 12.4109 - val_categorical_accuracy: 0.2300
Epoch 00009: saving model to model_init_2018-10-2122_42_43.394553/model-00009-1.37076-0.34641-12.41093-0.23000.h5
Epoch 00009: ReduceLROnPlateau reducing learning rate to 6.25000029685907e-05.
Epoch 10/20
34/34 [==============================] - 8s 230ms/step - loss: 1.3474 - categorical_accuracy: 0.3856 - val_loss: 12.4109 - val_categorical_accuracy: 0.2300
Epoch 00010: saving model to model_init_2018-10-2122_42_43.394553/model-00010-1.34743-0.38562-12.41093-0.23000.h5
Epoch 11/20
34/34 [==============================] - 7s 220ms/step - loss: 1.3115 - categorical_accuracy: 0.4314 - val_loss: 12.4109 - val_categorical_accuracy: 0.2300
Epoch 00011: saving model to model_init_2018-10-2122_42_43.394553/model-00011-1.31148-0.43137-12.41093-0.23000.h5
Epoch 00011: ReduceLROnPlateau reducing learning rate to 3.125000148429535e-05.
Epoch 12/20
34/34 [==============================] - 8s 228ms/step - loss: 1.4023 - categorical_accuracy: 0.3562 - val_loss: 12.4109 - val_categorical_accuracy: 0.2300
Epoch 00012: saving model to model_init_2018-10-2122_42_43.394553/model-00012-1.40227-0.35621-12.41093-0.23000.h5
Epoch 13/20
34/34 [==============================] - 7s 213ms/step - loss: 1.2985 - categorical_accuracy: 0.4216 - val_loss: 12.4109 - val_categorical_accuracy: 0.2300
Epoch 00013: saving model to model_init_2018-10-2122_42_43.394553/model-00013-1.29849-0.42157-12.41093-0.23000.h5
Epoch 00013: ReduceLROnPlateau reducing learning rate to 1.5625000742147677e-05.
Epoch 14/20
34/34 [==============================] - 8s 230ms/step - loss: 1.3730 - categorical_accuracy: 0.3889 - val_loss: 12.4109 - val_categorical_accuracy: 0.2300
Epoch 00014: saving model to model_init_2018-10-2122_42_43.394553/model-00014-1.37296-0.38889-12.41093-0.23000.h5
Epoch 15/20
34/34 [==============================] - 8s 227ms/step - loss: 1.3399 - categorical_accuracy: 0.3922 - val_loss: 12.4109 - val_categorical_accuracy: 0.2300
Epoch 00015: saving model to model_init_2018-10-2122_42_43.394553/model-00015-1.33991-0.39216-12.41093-0.23000.h5
Epoch 00015: ReduceLROnPlateau reducing learning rate to 7.812500371073838e-06.
Epoch 16/20
###Markdown
Model 5g : Resize to 120*120, All except normalization
###Code
train_gen = DataGenerator(affine=True, flip=True, normalize=False, crop=True, edge=True)
val_gen = DataGenerator()
model_gen = ModelGenerator()
input_shape = (30,120,120, 3)
num_classes = 5
model = model_gen.c3d5(input_shape, num_classes)
#batch_size = 20
#num_epochs = 20
train_generator = train_gen.generator(train_path, train_doc, batch_size)
val_generator = val_gen.generator(val_path, val_doc, batch_size)
train(batch_size, num_epochs, model, train_generator, val_generator)
###Output
# training sequences = 663
# validation sequences = 100
# batch size = 20
# epochs = 20
_________________________________________________________________
Layer (type) Output Shape Param #
=================================================================
conv3d_1 (Conv3D) (None, 30, 120, 120, 8) 656
_________________________________________________________________
activation_1 (Activation) (None, 30, 120, 120, 8) 0
_________________________________________________________________
dropout_1 (Dropout) (None, 30, 120, 120, 8) 0
_________________________________________________________________
max_pooling3d_1 (MaxPooling3 (None, 15, 60, 60, 8) 0
_________________________________________________________________
conv3d_2 (Conv3D) (None, 15, 60, 60, 16) 3472
_________________________________________________________________
activation_2 (Activation) (None, 15, 60, 60, 16) 0
_________________________________________________________________
dropout_2 (Dropout) (None, 15, 60, 60, 16) 0
_________________________________________________________________
max_pooling3d_2 (MaxPooling3 (None, 7, 30, 30, 16) 0
_________________________________________________________________
conv3d_3 (Conv3D) (None, 7, 30, 30, 32) 4640
_________________________________________________________________
activation_3 (Activation) (None, 7, 30, 30, 32) 0
_________________________________________________________________
dropout_3 (Dropout) (None, 7, 30, 30, 32) 0
_________________________________________________________________
max_pooling3d_3 (MaxPooling3 (None, 3, 15, 15, 32) 0
_________________________________________________________________
conv3d_4 (Conv3D) (None, 3, 15, 15, 64) 18496
_________________________________________________________________
activation_4 (Activation) (None, 3, 15, 15, 64) 0
_________________________________________________________________
dropout_4 (Dropout) (None, 3, 15, 15, 64) 0
_________________________________________________________________
max_pooling3d_4 (MaxPooling3 (None, 1, 7, 7, 64) 0
_________________________________________________________________
flatten_1 (Flatten) (None, 3136) 0
_________________________________________________________________
dense_1 (Dense) (None, 256) 803072
_________________________________________________________________
dropout_5 (Dropout) (None, 256) 0
_________________________________________________________________
dense_2 (Dense) (None, 128) 32896
_________________________________________________________________
dropout_6 (Dropout) (None, 128) 0
_________________________________________________________________
dense_3 (Dense) (None, 5) 645
=================================================================
Total params: 863,877
Trainable params: 863,877
Non-trainable params: 0
_________________________________________________________________
None
Epoch 1/20
Source path = ./Project_data/val ; batch size = 20
Source path = ./Project_data/train ; batch size = 20
34/34 [==============================] - 52s 2s/step - loss: 12.4080 - categorical_accuracy: 0.2166 - val_loss: 12.7333 - val_categorical_accuracy: 0.2100
Epoch 00001: saving model to model_init_2018-10-2122_46_00.151324/model-00001-12.44940-0.21368-12.73330-0.21000.h5
Epoch 2/20
34/34 [==============================] - 8s 242ms/step - loss: 12.4423 - categorical_accuracy: 0.2222 - val_loss: 12.7333 - val_categorical_accuracy: 0.2100
Epoch 00002: saving model to model_init_2018-10-2122_46_00.151324/model-00002-12.44231-0.22222-12.73330-0.21000.h5
Epoch 3/20
34/34 [==============================] - 8s 249ms/step - loss: 12.3783 - categorical_accuracy: 0.2320 - val_loss: 12.7333 - val_categorical_accuracy: 0.2100
Epoch 00003: saving model to model_init_2018-10-2122_46_00.151324/model-00003-12.37828-0.23203-12.73330-0.21000.h5
Epoch 00003: ReduceLROnPlateau reducing learning rate to 0.0005000000237487257.
Epoch 4/20
34/34 [==============================] - 8s 243ms/step - loss: 13.9992 - categorical_accuracy: 0.1307 - val_loss: 12.7333 - val_categorical_accuracy: 0.2100
Epoch 00004: saving model to model_init_2018-10-2122_46_00.151324/model-00004-13.99916-0.13072-12.73330-0.21000.h5
Epoch 5/20
34/34 [==============================] - 9s 254ms/step - loss: 12.5363 - categorical_accuracy: 0.2222 - val_loss: 12.7333 - val_categorical_accuracy: 0.2100
Epoch 00005: saving model to model_init_2018-10-2122_46_00.151324/model-00005-12.53630-0.22222-12.73330-0.21000.h5
Epoch 00005: ReduceLROnPlateau reducing learning rate to 0.0002500000118743628.
Epoch 6/20
34/34 [==============================] - 9s 251ms/step - loss: 13.2336 - categorical_accuracy: 0.1765 - val_loss: 12.7333 - val_categorical_accuracy: 0.2100
Epoch 00006: saving model to model_init_2018-10-2122_46_00.151324/model-00006-13.23358-0.17647-12.73330-0.21000.h5
Epoch 7/20
34/34 [==============================] - 9s 250ms/step - loss: 12.2729 - categorical_accuracy: 0.2386 - val_loss: 12.7333 - val_categorical_accuracy: 0.2100
Epoch 00007: saving model to model_init_2018-10-2122_46_00.151324/model-00007-12.27293-0.23856-12.73330-0.21000.h5
Epoch 00007: ReduceLROnPlateau reducing learning rate to 0.0001250000059371814.
Epoch 8/20
34/34 [==============================] - 9s 255ms/step - loss: 13.3791 - categorical_accuracy: 0.1699 - val_loss: 12.7333 - val_categorical_accuracy: 0.2100
Epoch 00008: saving model to model_init_2018-10-2122_46_00.151324/model-00008-13.37912-0.16993-12.73330-0.21000.h5
Epoch 9/20
34/34 [==============================] - 9s 264ms/step - loss: 12.3783 - categorical_accuracy: 0.2320 - val_loss: 12.7333 - val_categorical_accuracy: 0.2100
Epoch 00009: saving model to model_init_2018-10-2122_46_00.151324/model-00009-12.37828-0.23203-12.73330-0.21000.h5
Epoch 00009: ReduceLROnPlateau reducing learning rate to 6.25000029685907e-05.
Epoch 10/20
34/34 [==============================] - 9s 252ms/step - loss: 13.6424 - categorical_accuracy: 0.1536 - val_loss: 12.7333 - val_categorical_accuracy: 0.2100
Epoch 00010: saving model to model_init_2018-10-2122_46_00.151324/model-00010-13.64244-0.15359-12.73330-0.21000.h5
Epoch 11/20
34/34 [==============================] - 8s 229ms/step - loss: 12.3783 - categorical_accuracy: 0.2320 - val_loss: 12.7333 - val_categorical_accuracy: 0.2100
Epoch 00011: saving model to model_init_2018-10-2122_46_00.151324/model-00011-12.37828-0.23203-12.73330-0.21000.h5
Epoch 00011: ReduceLROnPlateau reducing learning rate to 3.125000148429535e-05.
Epoch 12/20
34/34 [==============================] - 8s 245ms/step - loss: 12.3525 - categorical_accuracy: 0.2320 - val_loss: 12.7333 - val_categorical_accuracy: 0.2100
Epoch 00012: saving model to model_init_2018-10-2122_46_00.151324/model-00012-12.35248-0.23203-12.73330-0.21000.h5
Epoch 13/20
34/34 [==============================] - 9s 256ms/step - loss: 13.1157 - categorical_accuracy: 0.1863 - val_loss: 12.7333 - val_categorical_accuracy: 0.2100
Epoch 00013: saving model to model_init_2018-10-2122_46_00.151324/model-00013-13.11571-0.18627-12.73330-0.21000.h5
Epoch 00013: ReduceLROnPlateau reducing learning rate to 1.5625000742147677e-05.
Epoch 14/20
34/34 [==============================] - 9s 256ms/step - loss: 12.2203 - categorical_accuracy: 0.2418 - val_loss: 12.7333 - val_categorical_accuracy: 0.2100
Epoch 00014: saving model to model_init_2018-10-2122_46_00.151324/model-00014-12.22026-0.24183-12.73330-0.21000.h5
Epoch 15/20
34/34 [==============================] - 9s 260ms/step - loss: 13.0630 - categorical_accuracy: 0.1895 - val_loss: 12.7333 - val_categorical_accuracy: 0.2100
Epoch 00015: saving model to model_init_2018-10-2122_46_00.151324/model-00015-13.06303-0.18954-12.73330-0.21000.h5
Epoch 00015: ReduceLROnPlateau reducing learning rate to 7.812500371073838e-06.
Epoch 16/20
###Markdown
Model 5h : Resize to 120*120, All except normalization
###Code
train_gen = DataGenerator(affine=True, flip=True, normalize=False, crop=True, edge=False)
val_gen = DataGenerator()
model_gen = ModelGenerator()
input_shape = (30,120,120, 3)
num_classes = 5
model = model_gen.c3d5(input_shape, num_classes)
#batch_size = 20
#num_epochs = 20
train_generator = train_gen.generator(train_path, train_doc, batch_size)
val_generator = val_gen.generator(val_path, val_doc, batch_size)
train(batch_size, num_epochs, model, train_generator, val_generator)
###Output
_____no_output_____ |
2022_KDT/2022_03/2022-03-28-coding.ipynb | ###Markdown
10.```cal1 = Calculator([1,2,3,4,5])cal1.sum() 합계cal1.avg() 평균cal2 = Calculator([6,7,8,9,10])cal2.sum() 합계cal2.avg() 평균```
###Code
# 10.
class Calculator:
def __init__(self, first, second,third,fourth,fifth):
self.first = first
self.second = second
self.third = third
self.fourth = fourth
self.fifth = fifth
def sum(self):
result = self.first + self.second + self.third + self.fourth + self.fifth
return result
def avg(self):
result = (self.first + self.second + self.third + self.fourth + self.fifth) / 5
return result
cal1 = Calculator(1,2,3,4,5)
# cal1.setdata(1,2,3,4,5)
cal1.avg()
cal2 = Calculator(6,7,8,9,10)
cal2.avg()
###Output
_____no_output_____
###Markdown
13.```DashInsert 함수는 숫자로 구성된 문자열을 입력받은 뒤 문자열 안에서 홀수가 연속되면 두 수 사이에 -를 추가하고, 짝수가 연속되면 *를 추가하는 기능을 갖고 있다. DashInsert 함수를 완성하시오.```
###Code
# # 13.
# i = 0
# n = input()
# def DashInsert(n):
# for i in range(n):
# i += 1
# if n[i] and n[i+1] % 2 == 0:
# return n[i]+"-"+n[i+1]
# elif n[i] and n[i+1] % 2 != 0:
# return n[i]+"*"+n[i+1]
# 13.
def DashInsert(n):
i = 0
n = input()
for i in range(len(n)):
i += 1
if int(n[i:]) and int(n[:i]) % 2 == 0:
return n[i]+"-"+n[i+1]
elif int(n[i:]) and int(n[:i]) % 2 != 0:
return n[i]+"*"+n[:i]
DashInsert(456793)
# n = input()
# i = 0
# def DashInsert(n):
# for i in range(n*2):
# i += 1
# if n[i] and n[i+1] % 2 == 0:
# print(n.insert(i,"-"))
# elif n[i] and n[i+1] % 2 !=0:
# print(n.insert(i,"*"))
# n = input()
# i = 0
# def DashInsert(n):
# for i in range(len(n)):
# i += 1
# if int(n[i:]) % 2 == 0 and n[i,]
DashInsert(4546793)
n = input()
def DashInsert(n):
i = 0
for i in range(n):
i += 1
if n[i] and n[i+1] % 2 == 0:
print(n[i]+"-"+n[i+1])
elif n[i] and n[i+1] % 2 != 0:
print(n[i]+"*"+n[i+1])
DashInsert(456455)
###Output
_____no_output_____
###Markdown
14. 문자열 압축하기```문자열을 입력받아 같은 문자가 연속적으로 반복되는 경우에 그 반복 횟수를 표시해 문자열을 압축하여 표시하시오.
###Code
n = input()
i = 0
for i in range (len(n)):
i += 1
if n[i] == n[i+1]:
print(n[i],n.count(n[i]),end=" ")
###Output
a 12 a 12 a 12 a 12 a 12 a 12 a 12 a 12 a 12 a 12 |
Chapter 10 - Introduction to Artificial Neural Networks with Keras/.ipynb_checkpoints/10_neural_nets_with_keras-checkpoint.ipynb | ###Markdown
**Chapter 10 – Introduction to Artificial Neural Networks with Keras**_This notebook contains all the sample code and solutions to the exercises in chapter 10._ Run in Google Colab Setup First, let's import a few common modules, ensure MatplotLib plots figures inline and prepare a function to save the figures. We also check that Python 3.5 or later is installed (although Python 2.x may work, it is deprecated so we strongly recommend you use Python 3 instead), as well as Scikit-Learn ≥0.20 and TensorFlow ≥2.0.
###Code
# Python ≥3.5 is required
import sys
assert sys.version_info >= (3, 5)
# Scikit-Learn ≥0.20 is required
import sklearn
assert sklearn.__version__ >= "0.20"
try:
# %tensorflow_version only exists in Colab.
%tensorflow_version 2.x
except Exception:
pass
# TensorFlow ≥2.0 is required
import tensorflow as tf
assert tf.__version__ >= "2.0"
# Common imports
import numpy as np
import os
# to make this notebook's output stable across runs
np.random.seed(42)
# To plot pretty figures
%matplotlib inline
import matplotlib as mpl
import matplotlib.pyplot as plt
mpl.rc('axes', labelsize=14)
mpl.rc('xtick', labelsize=12)
mpl.rc('ytick', labelsize=12)
# Where to save the figures
PROJECT_ROOT_DIR = "."
CHAPTER_ID = "ann"
IMAGES_PATH = os.path.join(PROJECT_ROOT_DIR, "images", CHAPTER_ID)
os.makedirs(IMAGES_PATH, exist_ok=True)
def save_fig(fig_id, tight_layout=True, fig_extension="png", resolution=300):
path = os.path.join(IMAGES_PATH, fig_id + "." + fig_extension)
print("Saving figure", fig_id)
if tight_layout:
plt.tight_layout()
plt.savefig(path, format=fig_extension, dpi=resolution)
# Ignore useless warnings (see SciPy issue #5998)
import warnings
warnings.filterwarnings(action="ignore", message="^internal gelsd")
###Output
_____no_output_____
###Markdown
Perceptrons **Note**: we set `max_iter` and `tol` explicitly to avoid warnings about the fact that their default value will change in future versions of Scikit-Learn.
###Code
import numpy as np
from sklearn.datasets import load_iris
from sklearn.linear_model import Perceptron
iris = load_iris()
X = iris.data[:, (2, 3)] # petal length, petal width
y = (iris.target == 0).astype(np.int)
per_clf = Perceptron(max_iter=1000, tol=1e-3, random_state=42)
per_clf.fit(X, y)
y_pred = per_clf.predict([[2, 0.5]])
y_pred
a = -per_clf.coef_[0][0] / per_clf.coef_[0][1]
b = -per_clf.intercept_ / per_clf.coef_[0][1]
axes = [0, 5, 0, 2]
x0, x1 = np.meshgrid(
np.linspace(axes[0], axes[1], 500).reshape(-1, 1),
np.linspace(axes[2], axes[3], 200).reshape(-1, 1),
)
X_new = np.c_[x0.ravel(), x1.ravel()]
y_predict = per_clf.predict(X_new)
zz = y_predict.reshape(x0.shape)
plt.figure(figsize=(10, 4))
plt.plot(X[y==0, 0], X[y==0, 1], "bs", label="Not Iris-Setosa")
plt.plot(X[y==1, 0], X[y==1, 1], "yo", label="Iris-Setosa")
plt.plot([axes[0], axes[1]], [a * axes[0] + b, a * axes[1] + b], "k-", linewidth=3)
from matplotlib.colors import ListedColormap
custom_cmap = ListedColormap(['#9898ff', '#fafab0'])
plt.contourf(x0, x1, zz, cmap=custom_cmap)
plt.xlabel("Petal length", fontsize=14)
plt.ylabel("Petal width", fontsize=14)
plt.legend(loc="lower right", fontsize=14)
plt.axis(axes)
save_fig("perceptron_iris_plot")
plt.show()
###Output
Saving figure perceptron_iris_plot
###Markdown
Activation functions
###Code
def sigmoid(z):
return 1 / (1 + np.exp(-z))
def relu(z):
return np.maximum(0, z)
def derivative(f, z, eps=0.000001):
return (f(z + eps) - f(z - eps))/(2 * eps)
z = np.linspace(-5, 5, 200)
plt.figure(figsize=(11,4))
plt.subplot(121)
plt.plot(z, np.sign(z), "r-", linewidth=1, label="Step")
plt.plot(z, sigmoid(z), "g--", linewidth=2, label="Sigmoid")
plt.plot(z, np.tanh(z), "b-", linewidth=2, label="Tanh")
plt.plot(z, relu(z), "m-.", linewidth=2, label="ReLU")
plt.grid(True)
plt.legend(loc="center right", fontsize=14)
plt.title("Activation functions", fontsize=14)
plt.axis([-5, 5, -1.2, 1.2])
plt.subplot(122)
plt.plot(z, derivative(np.sign, z), "r-", linewidth=1, label="Step")
plt.plot(0, 0, "ro", markersize=5)
plt.plot(0, 0, "rx", markersize=10)
plt.plot(z, derivative(sigmoid, z), "g--", linewidth=2, label="Sigmoid")
plt.plot(z, derivative(np.tanh, z), "b-", linewidth=2, label="Tanh")
plt.plot(z, derivative(relu, z), "m-.", linewidth=2, label="ReLU")
plt.grid(True)
#plt.legend(loc="center right", fontsize=14)
plt.title("Derivatives", fontsize=14)
plt.axis([-5, 5, -0.2, 1.2])
save_fig("activation_functions_plot")
plt.show()
def heaviside(z):
return (z >= 0).astype(z.dtype)
def mlp_xor(x1, x2, activation=heaviside):
return activation(-activation(x1 + x2 - 1.5) + activation(x1 + x2 - 0.5) - 0.5)
x1s = np.linspace(-0.2, 1.2, 100)
x2s = np.linspace(-0.2, 1.2, 100)
x1, x2 = np.meshgrid(x1s, x2s)
z1 = mlp_xor(x1, x2, activation=heaviside)
z2 = mlp_xor(x1, x2, activation=sigmoid)
plt.figure(figsize=(10,4))
plt.subplot(121)
plt.contourf(x1, x2, z1)
plt.plot([0, 1], [0, 1], "gs", markersize=20)
plt.plot([0, 1], [1, 0], "y^", markersize=20)
plt.title("Activation function: heaviside", fontsize=14)
plt.grid(True)
plt.subplot(122)
plt.contourf(x1, x2, z2)
plt.plot([0, 1], [0, 1], "gs", markersize=20)
plt.plot([0, 1], [1, 0], "y^", markersize=20)
plt.title("Activation function: sigmoid", fontsize=14)
plt.grid(True)
###Output
_____no_output_____
###Markdown
Building an Image Classifier First let's import TensorFlow and Keras.
###Code
import tensorflow as tf
from tensorflow import keras
tf.__version__
keras.__version__
###Output
_____no_output_____
###Markdown
Let's start by loading the fashion MNIST dataset. Keras has a number of functions to load popular datasets in `keras.datasets`. The dataset is already split for you between a training set and a test set, but it can be useful to split the training set further to have a validation set:
###Code
fashion_mnist = keras.datasets.fashion_mnist
(X_train_full, y_train_full), (X_test, y_test) = fashion_mnist.load_data()
###Output
_____no_output_____
###Markdown
The training set contains 60,000 grayscale images, each 28x28 pixels:
###Code
X_train_full.shape
###Output
_____no_output_____
###Markdown
Each pixel intensity is represented as a byte (0 to 255):
###Code
X_train_full.dtype
###Output
_____no_output_____
###Markdown
Let's split the full training set into a validation set and a (smaller) training set. We also scale the pixel intensities down to the 0-1 range and convert them to floats, by dividing by 255.
###Code
X_valid, X_train = X_train_full[:5000] / 255., X_train_full[5000:] / 255.
y_valid, y_train = y_train_full[:5000], y_train_full[5000:]
X_test = X_test / 255.
###Output
_____no_output_____
###Markdown
You can plot an image using Matplotlib's `imshow()` function, with a `'binary'` color map:
###Code
plt.imshow(X_train[0], cmap="binary")
plt.axis('off')
plt.show()
###Output
_____no_output_____
###Markdown
The labels are the class IDs (represented as uint8), from 0 to 9:
###Code
y_train
###Output
_____no_output_____
###Markdown
Here are the corresponding class names:
###Code
class_names = ["T-shirt/top", "Trouser", "Pullover", "Dress", "Coat",
"Sandal", "Shirt", "Sneaker", "Bag", "Ankle boot"]
###Output
_____no_output_____
###Markdown
So the first image in the training set is a coat:
###Code
class_names[y_train[0]]
###Output
_____no_output_____
###Markdown
The validation set contains 5,000 images, and the test set contains 10,000 images:
###Code
X_valid.shape
X_test.shape
###Output
_____no_output_____
###Markdown
Let's take a look at a sample of the images in the dataset:
###Code
n_rows = 4
n_cols = 10
plt.figure(figsize=(n_cols * 1.2, n_rows * 1.2))
for row in range(n_rows):
for col in range(n_cols):
index = n_cols * row + col
plt.subplot(n_rows, n_cols, index + 1)
plt.imshow(X_train[index], cmap="binary", interpolation="nearest")
plt.axis('off')
plt.title(class_names[y_train[index]], fontsize=12)
plt.subplots_adjust(wspace=0.2, hspace=0.5)
save_fig('fashion_mnist_plot', tight_layout=False)
plt.show()
model = keras.models.Sequential()
model.add(keras.layers.Flatten(input_shape=[28, 28]))
model.add(keras.layers.Dense(300, activation="relu"))
model.add(keras.layers.Dense(100, activation="relu"))
model.add(keras.layers.Dense(10, activation="softmax"))
keras.backend.clear_session()
np.random.seed(42)
tf.random.set_seed(42)
model = keras.models.Sequential([
keras.layers.Flatten(input_shape=[28, 28]),
keras.layers.Dense(300, activation="relu"),
keras.layers.Dense(100, activation="relu"),
keras.layers.Dense(10, activation="softmax")
])
model.layers
model.summary()
keras.utils.plot_model(model, "my_fashion_mnist_model.png", show_shapes=True)
hidden1 = model.layers[1]
hidden1.name
model.get_layer(hidden1.name) is hidden1
weights, biases = hidden1.get_weights()
weights
weights.shape
biases
biases.shape
model.compile(loss="sparse_categorical_crossentropy",
optimizer="sgd",
metrics=["accuracy"])
###Output
_____no_output_____
###Markdown
This is equivalent to: ```pythonmodel.compile(loss=keras.losses.sparse_categorical_crossentropy, optimizer=keras.optimizers.SGD(), metrics=[keras.metrics.sparse_categorical_accuracy])```
###Code
history = model.fit(X_train, y_train, epochs=30,
validation_data=(X_valid, y_valid))
history.params
print(history.epoch)
history.history.keys()
import pandas as pd
pd.DataFrame(history.history).plot(figsize=(8, 5))
plt.grid(True)
plt.gca().set_ylim(0, 1)
save_fig("keras_learning_curves_plot")
plt.show()
model.evaluate(X_test, y_test)
X_new = X_test[:3]
y_proba = model.predict(X_new)
y_proba.round(2)
y_pred = model.predict_classes(X_new)
y_pred
np.array(class_names)[y_pred]
y_new = y_test[:3]
y_new
plt.figure(figsize=(7.2, 2.4))
for index, image in enumerate(X_new):
plt.subplot(1, 3, index + 1)
plt.imshow(image, cmap="binary", interpolation="nearest")
plt.axis('off')
plt.title(class_names[y_test[index]], fontsize=12)
plt.subplots_adjust(wspace=0.2, hspace=0.5)
save_fig('fashion_mnist_images_plot', tight_layout=False)
plt.show()
###Output
Saving figure fashion_mnist_images_plot
###Markdown
Regression MLP Let's load, split and scale the California housing dataset (the original one, not the modified one as in chapter 2):
###Code
from sklearn.datasets import fetch_california_housing
from sklearn.model_selection import train_test_split
from sklearn.preprocessing import StandardScaler
housing = fetch_california_housing()
X_train_full, X_test, y_train_full, y_test = train_test_split(housing.data, housing.target, random_state=42)
X_train, X_valid, y_train, y_valid = train_test_split(X_train_full, y_train_full, random_state=42)
scaler = StandardScaler()
X_train = scaler.fit_transform(X_train)
X_valid = scaler.transform(X_valid)
X_test = scaler.transform(X_test)
np.random.seed(42)
tf.random.set_seed(42)
model = keras.models.Sequential([
keras.layers.Dense(30, activation="relu", input_shape=X_train.shape[1:]),
keras.layers.Dense(1)
])
model.compile(loss="mean_squared_error", optimizer=keras.optimizers.SGD(lr=1e-3))
history = model.fit(X_train, y_train, epochs=20, validation_data=(X_valid, y_valid))
mse_test = model.evaluate(X_test, y_test)
X_new = X_test[:3]
y_pred = model.predict(X_new)
plt.plot(pd.DataFrame(history.history))
plt.grid(True)
plt.gca().set_ylim(0, 1)
plt.show()
y_pred
###Output
_____no_output_____
###Markdown
Functional API Not all neural network models are simply sequential. Some may have complex topologies. Some may have multiple inputs and/or multiple outputs. For example, a Wide & Deep neural network (see [paper](https://ai.google/research/pubs/pub45413)) connects all or part of the inputs directly to the output layer.
###Code
np.random.seed(42)
tf.random.set_seed(42)
input_ = keras.layers.Input(shape=X_train.shape[1:])
hidden1 = keras.layers.Dense(30, activation="relu")(input_)
hidden2 = keras.layers.Dense(30, activation="relu")(hidden1)
concat = keras.layers.concatenate([input_, hidden2])
output = keras.layers.Dense(1)(concat)
model = keras.models.Model(inputs=[input_], outputs=[output])
model.summary()
model.compile(loss="mean_squared_error", optimizer=keras.optimizers.SGD(lr=1e-3))
history = model.fit(X_train, y_train, epochs=20,
validation_data=(X_valid, y_valid))
mse_test = model.evaluate(X_test, y_test)
y_pred = model.predict(X_new)
###Output
Train on 11610 samples, validate on 3870 samples
Epoch 1/20
11610/11610 [==============================] - 1s 76us/sample - loss: 1.2390 - val_loss: 0.6566
Epoch 2/20
11610/11610 [==============================] - 1s 60us/sample - loss: 0.6312 - val_loss: 0.6734
Epoch 3/20
11610/11610 [==============================] - 1s 59us/sample - loss: 0.5886 - val_loss: 0.5574
Epoch 4/20
11610/11610 [==============================] - 1s 58us/sample - loss: 0.5595 - val_loss: 0.5235
Epoch 5/20
11610/11610 [==============================] - 1s 58us/sample - loss: 0.5361 - val_loss: 0.5011
Epoch 6/20
11610/11610 [==============================] - 1s 60us/sample - loss: 0.5178 - val_loss: 0.5065
Epoch 7/20
11610/11610 [==============================] - 1s 59us/sample - loss: 0.5016 - val_loss: 0.4699
Epoch 8/20
11610/11610 [==============================] - 1s 58us/sample - loss: 0.4888 - val_loss: 0.4745
Epoch 9/20
11610/11610 [==============================] - 1s 60us/sample - loss: 0.4772 - val_loss: 0.4425
Epoch 10/20
11610/11610 [==============================] - 1s 60us/sample - loss: 0.4673 - val_loss: 0.4384
Epoch 11/20
11610/11610 [==============================] - 1s 58us/sample - loss: 0.4586 - val_loss: 0.4533
Epoch 12/20
11610/11610 [==============================] - 1s 58us/sample - loss: 0.4504 - val_loss: 0.4179
Epoch 13/20
11610/11610 [==============================] - 1s 58us/sample - loss: 0.4435 - val_loss: 0.4137
Epoch 14/20
11610/11610 [==============================] - 1s 58us/sample - loss: 0.4376 - val_loss: 0.4062
Epoch 15/20
11610/11610 [==============================] - 1s 60us/sample - loss: 0.4318 - val_loss: 0.4541
Epoch 16/20
11610/11610 [==============================] - 1s 58us/sample - loss: 0.4266 - val_loss: 0.3952
Epoch 17/20
11610/11610 [==============================] - 1s 58us/sample - loss: 0.4221 - val_loss: 0.3910
Epoch 18/20
11610/11610 [==============================] - 1s 58us/sample - loss: 0.4173 - val_loss: 0.4205
Epoch 19/20
11610/11610 [==============================] - 1s 58us/sample - loss: 0.4132 - val_loss: 0.3830
Epoch 20/20
11610/11610 [==============================] - 1s 58us/sample - loss: 0.4096 - val_loss: 0.3923
5160/5160 [==============================] - 0s 29us/sample - loss: 0.4042
###Markdown
What if you want to send different subsets of input features through the wide or deep paths? We will send 5 features (features 0 to 4), and 6 through the deep path (features 2 to 7). Note that 3 features will go through both (features 2, 3 and 4).
###Code
np.random.seed(42)
tf.random.set_seed(42)
input_A = keras.layers.Input(shape=[5], name="wide_input")
input_B = keras.layers.Input(shape=[6], name="deep_input")
hidden1 = keras.layers.Dense(30, activation="relu")(input_B)
hidden2 = keras.layers.Dense(30, activation="relu")(hidden1)
concat = keras.layers.concatenate([input_A, hidden2])
output = keras.layers.Dense(1, name="output")(concat)
model = keras.models.Model(inputs=[input_A, input_B], outputs=[output])
model.compile(loss="mse", optimizer=keras.optimizers.SGD(lr=1e-3))
X_train_A, X_train_B = X_train[:, :5], X_train[:, 2:]
X_valid_A, X_valid_B = X_valid[:, :5], X_valid[:, 2:]
X_test_A, X_test_B = X_test[:, :5], X_test[:, 2:]
X_new_A, X_new_B = X_test_A[:3], X_test_B[:3]
history = model.fit((X_train_A, X_train_B), y_train, epochs=20,
validation_data=((X_valid_A, X_valid_B), y_valid))
mse_test = model.evaluate((X_test_A, X_test_B), y_test)
y_pred = model.predict((X_new_A, X_new_B))
###Output
Train on 11610 samples, validate on 3870 samples
Epoch 1/20
11610/11610 [==============================] - 1s 76us/sample - loss: 1.8127 - val_loss: 2.1165
Epoch 2/20
11610/11610 [==============================] - 1s 64us/sample - loss: 0.6852 - val_loss: 0.6178
Epoch 3/20
11610/11610 [==============================] - 1s 62us/sample - loss: 0.5965 - val_loss: 0.5600
Epoch 4/20
11610/11610 [==============================] - 1s 62us/sample - loss: 0.5587 - val_loss: 0.5269
Epoch 5/20
11610/11610 [==============================] - 1s 61us/sample - loss: 0.5321 - val_loss: 0.5185
Epoch 6/20
11610/11610 [==============================] - 1s 61us/sample - loss: 0.5129 - val_loss: 0.4803
Epoch 7/20
11610/11610 [==============================] - 1s 61us/sample - loss: 0.4959 - val_loss: 0.4689
Epoch 8/20
11610/11610 [==============================] - 1s 62us/sample - loss: 0.4837 - val_loss: 0.4498
Epoch 9/20
11610/11610 [==============================] - 1s 61us/sample - loss: 0.4734 - val_loss: 0.4387
Epoch 10/20
11610/11610 [==============================] - 1s 61us/sample - loss: 0.4646 - val_loss: 0.4306
Epoch 11/20
11610/11610 [==============================] - 1s 61us/sample - loss: 0.4571 - val_loss: 0.4262
Epoch 12/20
11610/11610 [==============================] - 1s 61us/sample - loss: 0.4507 - val_loss: 0.4173
Epoch 13/20
11610/11610 [==============================] - 1s 61us/sample - loss: 0.4456 - val_loss: 0.4124
Epoch 14/20
11610/11610 [==============================] - 1s 61us/sample - loss: 0.4422 - val_loss: 0.4084
Epoch 15/20
11610/11610 [==============================] - 1s 63us/sample - loss: 0.4386 - val_loss: 0.4351
Epoch 16/20
11610/11610 [==============================] - 1s 61us/sample - loss: 0.4361 - val_loss: 0.4017
Epoch 17/20
11610/11610 [==============================] - 1s 61us/sample - loss: 0.4326 - val_loss: 0.3990
Epoch 18/20
11610/11610 [==============================] - 1s 64us/sample - loss: 0.4296 - val_loss: 0.4148
Epoch 19/20
11610/11610 [==============================] - 1s 62us/sample - loss: 0.4278 - val_loss: 0.3957
Epoch 20/20
11610/11610 [==============================] - 1s 61us/sample - loss: 0.4259 - val_loss: 0.3976
5160/5160 [==============================] - 0s 32us/sample - loss: 0.4202
###Markdown
Adding an auxiliary output for regularization:
###Code
np.random.seed(42)
tf.random.set_seed(42)
input_A = keras.layers.Input(shape=[5], name="wide_input")
input_B = keras.layers.Input(shape=[6], name="deep_input")
hidden1 = keras.layers.Dense(30, activation="relu")(input_B)
hidden2 = keras.layers.Dense(30, activation="relu")(hidden1)
concat = keras.layers.concatenate([input_A, hidden2])
output = keras.layers.Dense(1, name="main_output")(concat)
aux_output = keras.layers.Dense(1, name="aux_output")(hidden2)
model = keras.models.Model(inputs=[input_A, input_B],
outputs=[output, aux_output])
model.compile(loss=["mse", "mse"], loss_weights=[0.9, 0.1], optimizer=keras.optimizers.SGD(lr=1e-3))
history = model.fit([X_train_A, X_train_B], [y_train, y_train], epochs=20,
validation_data=([X_valid_A, X_valid_B], [y_valid, y_valid]))
total_loss, main_loss, aux_loss = model.evaluate(
[X_test_A, X_test_B], [y_test, y_test])
y_pred_main, y_pred_aux = model.predict([X_new_A, X_new_B])
###Output
5160/5160 [==============================] - 0s 43us/sample - loss: 0.4656 - main_output_loss: 0.4165 - aux_output_loss: 0.9111
###Markdown
The subclassing API
###Code
class WideAndDeepModel(keras.models.Model):
def __init__(self, units=30, activation="relu", **kwargs):
super().__init__(**kwargs)
self.hidden1 = keras.layers.Dense(units, activation=activation)
self.hidden2 = keras.layers.Dense(units, activation=activation)
self.main_output = keras.layers.Dense(1)
self.aux_output = keras.layers.Dense(1)
def call(self, inputs):
input_A, input_B = inputs
hidden1 = self.hidden1(input_B)
hidden2 = self.hidden2(hidden1)
concat = keras.layers.concatenate([input_A, hidden2])
main_output = self.main_output(concat)
aux_output = self.aux_output(hidden2)
return main_output, aux_output
model = WideAndDeepModel(30, activation="relu")
model.compile(loss="mse", loss_weights=[0.9, 0.1], optimizer=keras.optimizers.SGD(lr=1e-3))
history = model.fit((X_train_A, X_train_B), (y_train, y_train), epochs=10,
validation_data=((X_valid_A, X_valid_B), (y_valid, y_valid)))
total_loss, main_loss, aux_loss = model.evaluate((X_test_A, X_test_B), (y_test, y_test))
y_pred_main, y_pred_aux = model.predict((X_new_A, X_new_B))
model = WideAndDeepModel(30, activation="relu")
###Output
_____no_output_____
###Markdown
Saving and Restoring
###Code
np.random.seed(42)
tf.random.set_seed(42)
model = keras.models.Sequential([
keras.layers.Dense(30, activation="relu", input_shape=[8]),
keras.layers.Dense(30, activation="relu"),
keras.layers.Dense(1)
])
model.compile(loss="mse", optimizer=keras.optimizers.SGD(lr=1e-3))
history = model.fit(X_train, y_train, epochs=10, validation_data=(X_valid, y_valid))
mse_test = model.evaluate(X_test, y_test)
model.save("my_keras_model.h5")
model = keras.models.load_model("my_keras_model.h5")
model.predict(X_new)
a = os.getcwd()
print(a)
import os
import sys
model.save_weights("my_keras_weights.ckpt")
model.load_weights("my_keras_weights.ckpt")
###Output
_____no_output_____
###Markdown
Using Callbacks during Training
###Code
keras.backend.clear_session()
np.random.seed(42)
tf.random.set_seed(42)
model = keras.models.Sequential([
keras.layers.Dense(30, activation="relu", input_shape=[8]),
keras.layers.Dense(30, activation="relu"),
keras.layers.Dense(1)
])
model.compile(loss="mse", optimizer=keras.optimizers.SGD(lr=1e-3))
checkpoint_cb = keras.callbacks.ModelCheckpoint("my_keras_model.h5", save_best_only=True)
history = model.fit(X_train, y_train, epochs=10,
validation_data=(X_valid, y_valid),
callbacks=[checkpoint_cb])
model = keras.models.load_model("my_keras_model.h5") # rollback to best model
mse_test = model.evaluate(X_test, y_test)
model.compile(loss="mse", optimizer=keras.optimizers.SGD(lr=1e-3))
early_stopping_cb = keras.callbacks.EarlyStopping(patience=10,
restore_best_weights=True)
history = model.fit(X_train, y_train, epochs=100,
validation_data=(X_valid, y_valid),
callbacks=[checkpoint_cb, early_stopping_cb])
mse_test = model.evaluate(X_test, y_test)
class PrintValTrainRatioCallback(keras.callbacks.Callback):
def on_epoch_end(self, epoch, logs):
print("\nval/train: {:.2f}".format(logs["val_loss"] / logs["loss"]))
val_train_ratio_cb = PrintValTrainRatioCallback()
history = model.fit(X_train, y_train, epochs=1,
validation_data=(X_valid, y_valid),
callbacks=[val_train_ratio_cb])
###Output
Train on 11610 samples, validate on 3870 samples
11520/11610 [============================>.] - ETA: 0s - loss: 0.3265
val/train: 1.16
11610/11610 [==============================] - 1s 70us/sample - loss: 0.3256 - val_loss: 0.3789
###Markdown
TensorBoard
###Code
root_logdir = os.path.join(os.curdir, "my_logs")
def get_run_logdir():
import time
run_id = time.strftime("run_%Y_%m_%d-%H_%M_%S")
return os.path.join(root_logdir, run_id)
run_logdir = get_run_logdir()
run_logdir
keras.backend.clear_session()
np.random.seed(42)
tf.random.set_seed(42)
model = keras.models.Sequential([
keras.layers.Dense(30, activation="relu", input_shape=[8]),
keras.layers.Dense(30, activation="relu"),
keras.layers.Dense(1)
])
model.compile(loss="mse", optimizer=keras.optimizers.SGD(lr=1e-3))
tensorboard_cb = keras.callbacks.TensorBoard(run_logdir)
history = model.fit(X_train, y_train, epochs=30,
validation_data=(X_valid, y_valid),
callbacks=[checkpoint_cb, tensorboard_cb])
###Output
Train on 11610 samples, validate on 3870 samples
###Markdown
To start the TensorBoard server, one option is to open a terminal, if needed activate the virtualenv where you installed TensorBoard, go to this notebook's directory, then type:```bash$ tensorboard --logdir=./my_logs --port=6006```You can then open your web browser to [localhost:6006](http://localhost:6006) and use TensorBoard. Once you are done, press Ctrl-C in the terminal window, this will shutdown the TensorBoard server.Alternatively, you can load TensorBoard's Jupyter extension and run it like this:
###Code
%load_ext tensorboard
%tensorboard --logdir=./my_logs --port=6006
run_logdir2 = get_run_logdir()
run_logdir2
keras.backend.clear_session()
np.random.seed(42)
tf.random.set_seed(42)
model = keras.models.Sequential([
keras.layers.Dense(30, activation="relu", input_shape=[8]),
keras.layers.Dense(30, activation="relu"),
keras.layers.Dense(1)
])
model.compile(loss="mse", optimizer=keras.optimizers.SGD(lr=0.05))
tensorboard_cb = keras.callbacks.TensorBoard(run_logdir2)
history = model.fit(X_train, y_train, epochs=30,
validation_data=(X_valid, y_valid),
callbacks=[checkpoint_cb, tensorboard_cb])
###Output
_____no_output_____
###Markdown
Notice how TensorBoard now sees two runs, and you can compare the learning curves. Check out the other available logging options:
###Code
help(keras.callbacks.TensorBoard.__init__)
###Output
_____no_output_____
###Markdown
Hyperparameter Tuning
###Code
keras.backend.clear_session()
np.random.seed(42)
tf.random.set_seed(42)
def build_model(n_hidden=1, n_neurons=30, learning_rate=3e-3, input_shape=[8]):
model = keras.models.Sequential()
model.add(keras.layers.InputLayer(input_shape=input_shape))
for layer in range(n_hidden):
model.add(keras.layers.Dense(n_neurons, activation="relu"))
model.add(keras.layers.Dense(1))
optimizer = keras.optimizers.SGD(lr=learning_rate)
model.compile(loss="mse", optimizer=optimizer)
return model
keras_reg = keras.wrappers.scikit_learn.KerasRegressor(build_model)
keras_reg.fit(X_train, y_train, epochs=100,
validation_data=(X_valid, y_valid),
callbacks=[keras.callbacks.EarlyStopping(patience=10)])
mse_test = keras_reg.score(X_test, y_test)
y_pred = keras_reg.predict(X_new)
np.random.seed(42)
tf.random.set_seed(42)
###Output
_____no_output_____
###Markdown
**Warning**: the following cell crashes at the end of training. This seems to be caused by [Keras issue 13586](https://github.com/keras-team/keras/issues/13586), which was triggered by a recent change in Scikit-Learn. [Pull Request 13598](https://github.com/keras-team/keras/pull/13598) seems to fix the issue, so this problem should be resolved soon.
###Code
from scipy.stats import reciprocal
from sklearn.model_selection import RandomizedSearchCV
param_distribs = {
"n_hidden": [0, 1, 2, 3],
"n_neurons": np.arange(1, 100),
"learning_rate": reciprocal(3e-4, 3e-2),
}
rnd_search_cv = RandomizedSearchCV(keras_reg, param_distribs, n_iter=10, cv=3, verbose=2)
rnd_search_cv.fit(X_train, y_train, epochs=100,
validation_data=(X_valid, y_valid),
callbacks=[keras.callbacks.EarlyStopping(patience=10)])
rnd_search_cv.best_params_
rnd_search_cv.best_score_
rnd_search_cv.best_estimator_
rnd_search_cv.score(X_test, y_test)
model = rnd_search_cv.best_estimator_.model
model
model.evaluate(X_test, y_test)
###Output
_____no_output_____
###Markdown
Exercise solutions 1. to 9. See appendix A. 10. *Exercise: Train a deep MLP on the MNIST dataset (you can load it using `keras.datasets.mnist.load_data()`. See if you can get over 98% precision. Try searching for the optimal learning rate by using the approach presented in this chapter (i.e., by growing the learning rate exponentially, plotting the loss, and finding the point where the loss shoots up). Try adding all the bells and whistles—save checkpoints, use early stopping, and plot learning curves using TensorBoard.* Let's load the dataset:
###Code
(X_train_full, y_train_full), (X_test, y_test) = keras.datasets.mnist.load_data()
###Output
_____no_output_____
###Markdown
Just like for the Fashion MNIST dataset, the MNIST training set contains 60,000 grayscale images, each 28x28 pixels:
###Code
X_train_full.shape
###Output
_____no_output_____
###Markdown
Each pixel intensity is also represented as a byte (0 to 255):
###Code
X_train_full.dtype
###Output
_____no_output_____
###Markdown
Let's split the full training set into a validation set and a (smaller) training set. We also scale the pixel intensities down to the 0-1 range and convert them to floats, by dividing by 255, just like we did for Fashion MNIST:
###Code
X_valid, X_train = X_train_full[:5000] / 255., X_train_full[5000:] / 255.
y_valid, y_train = y_train_full[:5000], y_train_full[5000:]
X_test = X_test / 255.
###Output
_____no_output_____
###Markdown
Let's plot an image using Matplotlib's `imshow()` function, with a `'binary'` color map:
###Code
plt.imshow(X_train[0], cmap="binary")
plt.axis('off')
plt.show()
###Output
_____no_output_____
###Markdown
The labels are the class IDs (represented as uint8), from 0 to 9. Conveniently, the class IDs correspond to the digits represented in the images, so we don't need a `class_names` array:
###Code
y_train
###Output
_____no_output_____
###Markdown
The validation set contains 5,000 images, and the test set contains 10,000 images:
###Code
X_valid.shape
X_test.shape
###Output
_____no_output_____
###Markdown
Let's take a look at a sample of the images in the dataset:
###Code
n_rows = 4
n_cols = 10
plt.figure(figsize=(n_cols * 1.2, n_rows * 1.2))
for row in range(n_rows):
for col in range(n_cols):
index = n_cols * row + col
plt.subplot(n_rows, n_cols, index + 1)
plt.imshow(X_train[index], cmap="binary", interpolation="nearest")
plt.axis('off')
plt.title(y_train[index], fontsize=12)
plt.subplots_adjust(wspace=0.2, hspace=0.5)
plt.show()
###Output
_____no_output_____
###Markdown
Let's build a simple dense network and find the optimal learning rate. We will need a callback to grow the learning rate at each iteration. It will also record the learning rate and the loss at each iteration:
###Code
K = keras.backend
class ExponentialLearningRate(keras.callbacks.Callback):
def __init__(self, factor):
self.factor = factor
self.rates = []
self.losses = []
def on_batch_end(self, batch, logs):
self.rates.append(K.get_value(self.model.optimizer.lr))
self.losses.append(logs["loss"])
K.set_value(self.model.optimizer.lr, self.model.optimizer.lr * self.factor)
keras.backend.clear_session()
np.random.seed(42)
tf.random.set_seed(42)
model = keras.models.Sequential([
keras.layers.Flatten(input_shape=[28, 28]),
keras.layers.Dense(300, activation="relu"),
keras.layers.Dense(100, activation="relu"),
keras.layers.Dense(10, activation="softmax")
])
###Output
_____no_output_____
###Markdown
We will start with a small learning rate of 1e-3, and grow it by 0.5% at each iteration:
###Code
model.compile(loss="sparse_categorical_crossentropy",
optimizer=keras.optimizers.SGD(lr=1e-3),
metrics=["accuracy"])
expon_lr = ExponentialLearningRate(factor=1.005)
###Output
_____no_output_____
###Markdown
Now let's train the model for just 1 epoch:
###Code
history = model.fit(X_train, y_train, epochs=1,
validation_data=(X_valid, y_valid),
callbacks=[expon_lr])
###Output
_____no_output_____
###Markdown
We can now plot the loss as a functionof the learning rate:
###Code
plt.plot(expon_lr.rates, expon_lr.losses)
plt.gca().set_xscale('log')
plt.hlines(min(expon_lr.losses), min(expon_lr.rates), max(expon_lr.rates))
plt.axis([min(expon_lr.rates), max(expon_lr.rates), 0, expon_lr.losses[0]])
plt.xlabel("Learning rate")
plt.ylabel("Loss")
###Output
_____no_output_____
###Markdown
The loss starts shooting back up violently around 3e-1, so let's try using 2e-1 as our learning rate:
###Code
keras.backend.clear_session()
np.random.seed(42)
tf.random.set_seed(42)
model = keras.models.Sequential([
keras.layers.Flatten(input_shape=[28, 28]),
keras.layers.Dense(300, activation="relu"),
keras.layers.Dense(100, activation="relu"),
keras.layers.Dense(10, activation="softmax")
])
model.compile(loss="sparse_categorical_crossentropy",
optimizer=keras.optimizers.SGD(lr=2e-1),
metrics=["accuracy"])
run_index = 1 # increment this at every run
run_logdir = os.path.join(os.curdir, "my_mnist_logs", "run_{:03d}".format(run_index))
run_logdir
early_stopping_cb = keras.callbacks.EarlyStopping(patience=20)
checkpoint_cb = keras.callbacks.ModelCheckpoint("my_mnist_model.h5", save_best_only=True)
tensorboard_cb = keras.callbacks.TensorBoard(run_logdir)
history = model.fit(X_train, y_train, epochs=100,
validation_data=(X_valid, y_valid),
callbacks=[early_stopping_cb, checkpoint_cb, tensorboard_cb])
model = keras.models.load_model("my_mnist_model.h5") # rollback to best model
model.evaluate(X_test, y_test)
###Output
_____no_output_____
###Markdown
We got over 98% accuracy. Finally, let's look at the learning curves using TensorBoard:
###Code
%tensorboard --logdir=./my_mnist_logs --port=6006
###Output
_____no_output_____ |
additional_content/CreatingQWITables.ipynb | ###Markdown
Website Ghani, Rayid, Frauke Kreuter, Julia Lane, Adrianne Bradford, Alex Engler, Nicolas Guetta Jeanrenaud, Graham Henke, Daniela Hochfellner, Clayton Hunter, Brian Kim, Avishek Kumar, and Jonathan Morgan. Applications: Replication QWI Statistics with MO Wage Records---- Table of Contents- [Introduction](Introduction)- [Basic Concepts](Basic-Concepts)- [Python Setup](Python-Setup)- [The Six Starter Files](The-Six-Starter-Files)- [Longitudinal Linkage](Longitudinal-Linkage)- [QWI Metrics](QWI-Metrics) Introduction- Back to [Table of Contents](Table-of-Contents)This notebook presents an application of creating variables, as taught in the "Variables" notebook. Here we will generate the relevant metrics of the Quarterly Workforce Indicators (QWI) framework, for the overall Missouri economy and by business EIN. QWI is a robust method of quantifying the dynamic employment patterns of workers and firms. It was developed by the Longitudinal Employer-Household Dynamics (LEHD) program at the Census in collaboration with state employment security agencies across the US. LEHD collects administrative unemployment insurance (UI) and employer (Quarterly Census of Employment and Wages) data from the state agencies. It then standardizes and cleans the data, applies some statistical imputation procedures, calculates statistics, and finally makes aggregates available to the public and microdata available to researchers. This procedure is described in detail in "The LEHD Infrastructure Files and the Creation of the Quarterly Workforce Indicators" (Abowd et al. 2009. in "Producer Dynamics: New Evidence from Micro Data").In this notebook, we present code for turning simple Wage Records files into QWI files, as well as examples and suggestions for optimal use. > In the following steps, we will code the QWI metrics for a given quarter (2010 quarter 1). If you need these metrics for project work, the results for all quarters have been run and stored on the ADRF, in the `ada_18_uchi` schema. Basic Concepts- Back to [Table of Contents](Table-of-Contents)The QWI framework is focused on **jobs**, which are the combination of **people** and **employers**. If John works at both McDonalds and Burger King, this will generate two completely separate jobs in the QWI data. If John quits his job at McDonalds, that job will no longer exist in the QWI data. If John quits both of his jobs, he will not be observed in the QWI data at all. The Wage Records files might contain "jobs" where no money changes hands in a given quarter. From the perspective of QWI, a job only exists if it involves wages greater than one dollar.In the ADRF environment, **People** are identified by their (hashed) Social Security Number and (hashed) name. In a perfect world, tracking people over time would simply be a matter of tracking their unique SSN. However, SSNs can be corrupted by data entry errors or used by multiple people. For the time being, we do not address these issues. We only longitudinally link two jobs if they have identical SSNs.Due to the fact that people who are unemployed or out of the labor force do not appear in QWI data, these data are not suitable for calculating population-wide employment rates of the kind produced by the Bureau of Labor Statistics. However, under the assumption that people who do not appear in the Wage Records data do not have jobs, QWI data can be used to calculate employment rates and other employment statistics for a subpopulation of people with known (hashed) SSNs. In this QWI routine, **Employers** are identified by their Employer Identification Number (EIN). Other methodologies use the Unemployment Insurance Account Number (UI Account Number).Each QWI file is specific to a single **quarter**. It includes a job if and only if it existed in that quarter. It includes information from prior and later quarters, but this is only to help construct the employment dynamics. The QWI file for 2010 quarter 2 gives a complete picture of the employment dynamics for that quarter but an incomplete (and likely misleading) picture of the employment dynamics for 2010 quarter 1 (or any other quarter). The Wage Records data tell us whether a job existed in a given quarter, but without more information we cannot differentiate a job that lasted the entire quarter from one that only lasted a day. Python Setup- Back to [Table of Contents](Table-of-Contents)
###Code
# general use imports
import datetime
import glob
import inspect
import numpy
import os
import six
import warnings
import math
from itertools import izip
# pandas-related imports
import pandas as pd
import sqlalchemy
# CSV file reading-related imports
import csv
# database interaction imports
import sqlalchemy
import datetime
# to create a connection to the database, we need to pass the name of the database and host of the database
connection_string = "postgresql://10.10.2.10/appliedda"
conn = sqlalchemy.create_engine(connection_string)
## makeDataDict: A function to split a dataset into 6 quarterly datasets for use in QWI calculations
## Inputs:
## -connection: Connection to the database
## -keyYr: The year to caculate QWI stats for
## -keyQ: The quarter to calculate QWI stats for
## Output:
## -res: A dictionary with keys m4, m3, m2, m1, t, and p1 containing subsets of the longDat that condition
## on keyRy/keyQ (for entry 't'), 4 lags ('m1'-'m4'), and one lead ('p1')
def makeDataDict(connection, keyYr, keyQ):
keys = ['m4', 'm3', 'm2', 'm1', 't', 'p1']
res = {}
for i in range(0,6):
##Find the right year and quarter, with i=0 corresponding to the 4th lag
yr = int(keyYr - 1 + math.floor((keyQ+i-1)/4))
q = int(keyQ + i - 4*math.floor((keyQ+i-1)/4))
# Query the dataset on the given year and quarter, keeping only identifiers and wage as columns
query='''
SELECT ssn
, ein
, 1 as wage
FROM il_des_kcmo.il_wage
WHERE year = {} and quarter = {}
'''.format(yr, q)
res[keys[i]] = pd.read_sql(query, connection)
return res
## linkData: A function to longitudinally link the datasets supplied in the form of a dictionary output by
## makedataDict. Currently links by a deterministic left merge (where 't' is always on the left) by SSN and EIN.
## Input:
## -dataDict: A dictionary with keys m4, m3, m2, m1, t, and p1 containing subsets of the longDat that condition
## on keyRy/keyQ (for entry 't'), 4 lags ('m1'-'m4'), and one lead ('p1')
## Output:
## -res: A single dataframe with columns SSN, EIN, and wage_m4-wage_p1, the results of the
## longitudinal linkage. A job is included iff it exists in period 't'. When that job does not exist in a different
## period, the record describing that job will have a missing value in the column for that period.
def linkData(dataDict):
for time in dataDict:
dataDict[time] = dataDict[time][(dataDict[time]['wage'].notnull())]
res = dataDict['t']
for time in dataDict:
if time != 't':
##Define the suffix to add to the time period being merged in
suff = '_' + time
##Merge on iddssn, uiacctno. Keep all records in 't'. Drop unmatched records in the other period.
res = pd.merge(left=res, right=dataDict[time], on=['ssn','ein'], how='left', suffixes=('',suff))
res = res[['ssn', 'ein', 'wage', 'wage_m4', 'wage_m3', 'wage_m2', 'wage_m1', 'wage_p1']]
return res
## makeStatistics: A function to calculate QWI statistics
## (employment-stable employment, accessions and separations therefrom, etc.).
## Input:
## -linkData: A single dataframe with columns idssn, uiacctno, and wage_m4-wage_p1.
## Missing entries will be interpreted as the non-existence of a job.
## Output:
## -res: The input dataset with columns appended for each statistic.
def makeStatistics(linkData):
res = linkData
#Flow Employment
res['qwmt'] = 1*(res['wage'] > 0)
res['qwmtm4'] = 1*(res['wage_m4'] > 0)
res['qwmtm3'] = 1*(res['wage_m3'] > 0)
res['qwmtm2'] = 1*(res['wage_m2'] > 0)
res['qwmtm1'] = 1*(res['wage_m1'] > 0)
res['qwmtp1'] = 1*(res['wage_p1'] > 0)
#Beginning of Quarter Employment
res['qwbt'] = 1*((res['qwmtm1']==1) & (res['qwmt']==1))
#End of Quarter Employment
res['qwet'] = 1*((res['qwmt']==1) & (res['qwmtp1']==1))
#Full Quarter Employment
res['qwft'] = 1*((res['qwmtm1']==1) & (res['qwmt']==1) & (res['qwmtp1']==1))
#Accessions
res['qwat'] = 1*((res['qwmtm1']==0) & (res['qwmt']==1))
#Accessions to Consecutive Quarter Status
res['qwa2t'] = 1*((res['qwat']==1) & (res['qwmtp1']==1))
#Accessions to Full Quarter Status
res['qwa3t'] = 1*((res['qwmtm2']==0) & (res['qwmtm1']==1) & (res['qwmt']==1)
& (res['qwmtp1']==1))
#Separations
res['qwst'] = 1*((res['qwmt']==1) & (res['qwmtp1']==0))
#New Hires
res['qwht'] = 1*((res['qwmtm4']==0) & (res['qwmtm3']==0) & (res['qwmtm2']==0)
& (res['qwmtm1']==0) & (res['qwmt']==1))
#Recalls
res['qwrt'] = 1*((res['qwmtm1']==0) & (res['qwmt']==1) & (res['qwht']==0))
##Replace technical names by more explicit names
names_tech = (['qwmt','qwmtm4','qwmtm3','qwmtm2','qwmtm1','qwmtp1','qwbt'
,'qwet','qwft','qwat','qwa2t','qwa3t','qwst','qwht','qwrt'])
names_def =(['emp_current_qrt','emp_4qtrs_ago','emp_3qtrs_ago','emp_2qtrs_ago'
,'emp_prev_qtr', 'emp_next_qtr','emp_begin_qtr','emp_end_qtr'
,'emp_full_qtr','accessions_current', 'accessions_consecutive_qtr'
,'accessions_full_qtr','separations','new_hires','recalls'])
rn_dict = dict(izip(names_tech, names_def))
res = res.rename(index=str, columns=rn_dict)
return res
qwi_vars =(['emp_current_qrt','emp_4qtrs_ago','emp_3qtrs_ago','emp_2qtrs_ago','emp_prev_qtr'
, 'emp_next_qtr','emp_begin_qtr','emp_end_qtr','emp_full_qtr','accessions_current'
, 'accessions_consecutive_qtr','accessions_full_qtr','separations','new_hires','recalls'])
for i in range(2012,2016):
full_data = makeDataDict(conn, i, 1)
print("Downloaded " + str(i) + " data")
linked_data = linkData(full_data)
print("Linked "+ str(i) + " data")
qwi_stats = makeStatistics(linked_data)
qwi_ein_stats = qwi_stats.groupby(['ein'])[qwi_vars].mean().reset_index()
qwi_ein_count = qwi_stats.groupby(['ein']).size().reset_index()
qwi_ein_count['nb_empl'] = qwi_ein_count[0]
del qwi_ein_count[0]
qwi_ein = pd.merge(qwi_ein_count, qwi_ein_stats, on = 'ein')
table_name = 'qwi_ein_' + str(i) + "_1"
conn = sqlalchemy.create_engine(connection_string)
qwi_ein.to_sql(table_name, conn, schema = 'ada_18_uchi')
print("Uploaded " + str(i) + " data")
###Output
_____no_output_____ |
Praktikum-DataScientist-Specialist.ipynb | ###Markdown
Kementerian Keuangan RI Badan Pendidikan dan Pelatihan KeuanganPusdiklat Keuangan Umum Praktikum Data Scientist Specialist*** Perhatikan petunjuk pengisian berikut :1. Tujuan praktikum ini adalah untuk mengukur kemampuan Anda dalam melakukan pengolahan data dan mengaplikasikan metode-metode machine learning.2. Anda diperbolehkan melihat file jupyter-notebook yang telah disediakan sebelumnya atau menggunakan search engine untuk menyelesaikan soal-soal yang disediakan.3. Perhatikan petunjuk pengisian pada masing-masing bagian. Tambahan pekerjaan (step) untuk pengolahan data akan memberikan nilai bonus.4. Waktu menyelesaikan seluruh soal berikut adalah 60 menit.*** Tuliskan Nama dan NIP Anda : *** Data Science Story Project> Anda diminta oleh Kementerian Kelautan Republik sebelah sana untuk membangun model yang akan digunakan untuk mengklasifikasikan suatu spesies ikan dari berat, dan ukuran suatu ikan. > Anda diminta untuk membuat model klasifikasi yang paling akurat dari beberapa model yang ada, **paling sedikit memuat 3 model** antara lain yaitu **Logistic Regression, RandomForest, Support Vector Machine, dan/atau Multi Layer Perceptron**,. > Anda juga diminta untuk memberikan perbandingan model berdasarkan akurasi.Terdapat tahapan pada jupyter notebook yang sudah dilengkapi secara default, namun Anda diminta untuk tetap melakukan tahapan-tahapan dasar standard *machine learning* yaitu *exploratory data analysis* sebelum membangun model. Import library dan load dataset **1. Hal yang pertama kali harus dilakukan adalah meng-import *library* pandas dan numpy.**
###Code
# import libraries
import pandas as pd
import numpy as np
###Output
_____no_output_____
###Markdown
Selanjutnya, kita akan mengimport (load) data dalam bentuk file `csv` ke dalam objek bernama `df` dengan menggunakan *method* `read_csv`.
###Code
df = pd.read_csv('Fish.csv')
###Output
_____no_output_____
###Markdown
I. Exploratory Data AnalysisPada tahapan ini Anda diminta untuk meng-explor data **2. Lakukan observasi dengan menampilkan 10 data pertama !**
###Code
# tampilkan 10 data pertama
df.head(10)
###Output
_____no_output_____
###Markdown
**3. Lakukan observasi dengan menampilkan 10 data terakhir !**
###Code
# tampilkan 10 data terakhir
df.tail(10)
###Output
_____no_output_____
###Markdown
Apakah ada yang aneh?Dapat dilihat pada kolom yang pertama, ada kolom yang tidak relevan, sehingga Anda dapat menghilangkan kolom tersebut.**5. Hilangkan kolom yang tidak relevan**
###Code
# Hilangkan kolom tidak relevan
df[:]['Species':'Sex']
###Output
_____no_output_____
###Markdown
Selanjutnya, kita ingin mengetahui ada berapa baris di dalam data tersebut dengan menggunakan *method* `info`.
###Code
# panggil metode info
###Output
_____no_output_____
###Markdown
Terdapat 8 kolom dan 159 baris pada data tersebut. Metadata untuk dataset tersebut adalah:- Species name of fish- Weight of fish in Gram g- Length1 is vertical length in cm- Length2 is diagonal length in cm- Length3 is cross length in cm- Height in cm- Width is diagonal width in cm- SexDari data `info` di atas, diketahui bahwa 6 kolom adalah data *continuous* sedangkan dua kolom merupakan objek. Lanjutkan Observasi data di atas, Apakah ada data `NaN`?**6. Temukan data `NaN` dan hitung berapa jumlah record yg `NaN`** Kita akan melakukan observasi terakhir yaitu menampilkan data apa saja di dalam kolom `Species`, dan `Sex`.**7. Tampilkan seluruh data pada kolom `Species` dan `Sex` beserta jumlahnya** II. Data Pre-processingTahapan lanjutan adalah melakukan pembersihan atau organising atas data yang dimiliki.> Namun, diketahui bahwa, terdapat *feature* (kolom) terdapat nilai `NaN`, di samping nilai yang sesungguhnya.**8. Langkah apa yang akan Anda lakukan?**Tuliskan Langkah-langkah Anda di bawah ini: Jawaban Anda: **9. Lakukan Langkah Yang Anda telah jelaskan di atas** Diasumsikan bahwa data yang akan kita gunakan telah melalui serangkaian pembersihan data dan seterusnya. Tugas selanjutnya adalah membuat model untuk memprediksi Species berdasarkan atribut lainnya. Lakukan train test splitSebelum membangun model, langkah yang harus dilakukan adalah memecah target variabel dan independent variabel. **10. Lakukan metode train test split**
###Code
# Import train_test_split
# X merupakan dataset kecuali target variabel
# Y merupakan target variabel yaitu Species
# kita gunakan 70% atau 80% data training (silakan pilih)
# Creation of Train and Test dataset
# Creation of Train and validation dataset
###Output
_____no_output_____
###Markdown
Selanjutnya kita akan meng-import beberapa alat pengukuran
###Code
from sklearn import model_selection
from sklearn.metrics import accuracy_score
from sklearn.metrics import classification_report
from sklearn.metrics import confusion_matrix
###Output
_____no_output_____
###Markdown
**11. Lakukan Pembuatan model-model sesuai dengan pilihan Anda**Perhatikan bahwa Anda diminta membuat **minimal 3 model** !
###Code
# import library
# buat objek model
# fit model
# panggil fungsi predict
print ('-'*40)
print ('Accuracy score:')
print (accuracy_score(y_test, _prediction))
print ('-'*40)
print ('Confusion Matrix:')
print (confusion_matrix(y_test, _prediction))
print ('-'*40)
print ('Classification Matrix:')
print (classification_report(y_test, _prediction))
###Output
_____no_output_____ |
IoTApps/.ipynb_checkpoints/kernel-checkpoint.ipynb | ###Markdown
function code_toggle() { if (code_shown){ $('div.input').hide('500'); $('toggleButton').val('Show Code') } else { $('div.input').show('500'); $('toggleButton').val('Hide Code') } code_shown = !code_shown } $( document ).ready(function(){ code_shown=false; $('div.input').hide() });
###Code
from IPython.display import HTML
HTML('''
<script>
function code_toggle() {
if (code_shown){
$('div.input').hide('500');
$('#toggleButton').val('Show Code')
} else {
$('div.input').show('500');
$('#toggleButton').val('Hide Code')
}
code_shown = !code_shown
}
$( document ).ready(function(){
code_shown=false;
$('div.input').hide()
});
</script>
<form action="javascript:code_toggle()"><input type="submit" id="toggleButton" value="Show Code"></form>''')
%matplotlib inline
import pandas as pd
import numpy as np
from matplotlib import pyplot as plt
plt.style.use('ggplot')
import seaborn as sns # for making plots with seaborn
color = sns.color_palette()
sns.set(rc={'figure.figsize':(25,15)})
import plotly
# connected=True means it will download the latest version of plotly javascript library.
plotly.offline.init_notebook_mode(connected=True)
import plotly.graph_objs as go
import plotly.figure_factory as ff
import cufflinks as cf
import warnings
warnings.filterwarnings('ignore')
###Output
_____no_output_____
###Markdown
Sneak peek at the dataset
###Code
df = pd.read_csv('../input/googleplaystore.csv')
#print(df.dtypes)
#df.loc[df.App=='Tiny Scanner - PDF Scanner App']
# df[df.duplicated(keep='first')]
df.drop_duplicates(subset='App', inplace=True)
df = df[df['Android Ver'] != np.nan]
df = df[df['Android Ver'] != 'NaN']
df = df[df['Installs'] != 'Free']
df = df[df['Installs'] != 'Paid']
#print(len(df))
print('Number of apps in the dataset : ' , len(df))
df.sample(7)
###Output
_____no_output_____
###Markdown
Data Cleaning - Convert all app sizes to MB - Remove '+' from 'Number of Installs' to make it numeric - Convert all review text to English language using Google Translator library
###Code
# - Installs : Remove + and ,
df['Installs'] = df['Installs'].apply(lambda x: x.replace('+', '') if '+' in str(x) else x)
df['Installs'] = df['Installs'].apply(lambda x: x.replace(',', '') if ',' in str(x) else x)
df['Installs'] = df['Installs'].apply(lambda x: int(x))
#print(type(df['Installs'].values))
# - Size : Remove 'M', Replace 'k' and divide by 10^-3
#df['Size'] = df['Size'].fillna(0)
df['Size'] = df['Size'].apply(lambda x: str(x).replace('Varies with device', 'NaN') if 'Varies with device' in str(x) else x)
df['Size'] = df['Size'].apply(lambda x: str(x).replace('M', '') if 'M' in str(x) else x)
df['Size'] = df['Size'].apply(lambda x: str(x).replace(',', '') if 'M' in str(x) else x)
df['Size'] = df['Size'].apply(lambda x: float(str(x).replace('k', '')) / 1000 if 'k' in str(x) else x)
df['Size'] = df['Size'].apply(lambda x: float(x))
df['Installs'] = df['Installs'].apply(lambda x: float(x))
df['Price'] = df['Price'].apply(lambda x: str(x).replace('$', '') if '$' in str(x) else str(x))
df['Price'] = df['Price'].apply(lambda x: float(x))
df['Reviews'] = df['Reviews'].apply(lambda x: int(x))
#df['Reviews'] = df['Reviews'].apply(lambda x: 'NaN' if int(x) == 0 else int(x))
#print(df.loc[df.Size == 0.713]) #index = 3384
#df.loc[df.col1 == '']['col2']
# 0 - Free, 1 - Paid
# df['Type'] = pd.factorize(df['Type'])[0]
#print(df.dtypes)
###Output
_____no_output_____
###Markdown
Basic EDA
###Code
#print(df.dtypes)
x = df['Rating'].dropna()
y = df['Size'].dropna()
z = df['Installs'][df.Installs!=0].dropna()
p = df['Reviews'][df.Reviews!=0].dropna()
t = df['Type'].dropna()
price = df['Price']
p = sns.pairplot(pd.DataFrame(list(zip(x, y, np.log(z), np.log10(p), t, price)),
columns=['Rating','Size', 'Installs', 'Reviews', 'Type', 'Price']), hue='Type', palette="Set2")
###Output
_____no_output_____
###Markdown
This is the basic exploratory analysis to look for any evident patterns or relationships between the features. Android market breakdownWhich category has the highest share of (active) apps in the market?
###Code
number_of_apps_in_category = df['Category'].value_counts().sort_values(ascending=True)
data = [go.Pie(
labels = number_of_apps_in_category.index,
values = number_of_apps_in_category.values,
hoverinfo = 'label+value'
)]
plotly.offline.iplot(data, filename='active_category')
###Output
_____no_output_____
###Markdown
- **Family** and **Game** apps have the highest market prevelance. - Interestingly, **Tools, Business and Medical** apps are also catching up. Average rating of appsDo any apps perform really good or really bad?
###Code
data = [go.Histogram(
x = df.Rating,
xbins = {'start': 1, 'size': 0.1, 'end' :5}
)]
print('Average app rating = ', np.mean(df['Rating']))
plotly.offline.iplot(data, filename='overall_rating_distribution')
###Output
_____no_output_____
###Markdown
Generally, most apps do well with an average rating of **4.17**.Let's break this down and inspect if we have categories which perform exceptionally good or bad. App ratings across categories - One Way Anova Test
###Code
import scipy.stats as stats
f = stats.f_oneway(df.loc[df.Category == 'BUSINESS']['Rating'].dropna(),
df.loc[df.Category == 'FAMILY']['Rating'].dropna(),
df.loc[df.Category == 'GAME']['Rating'].dropna(),
df.loc[df.Category == 'PERSONALIZATION']['Rating'].dropna(),
df.loc[df.Category == 'LIFESTYLE']['Rating'].dropna(),
df.loc[df.Category == 'FINANCE']['Rating'].dropna(),
df.loc[df.Category == 'EDUCATION']['Rating'].dropna(),
df.loc[df.Category == 'MEDICAL']['Rating'].dropna(),
df.loc[df.Category == 'TOOLS']['Rating'].dropna(),
df.loc[df.Category == 'PRODUCTIVITY']['Rating'].dropna()
)
print(f)
print('\nThe p-value is extremely small, hence we reject the null hypothesis in favor of the alternate hypothesis.\n')
#temp = df.loc[df.Category.isin(['BUSINESS', 'DATING'])]
groups = df.groupby('Category').filter(lambda x: len(x) > 286).reset_index()
array = groups['Rating'].hist(by=groups['Category'], sharex=True, figsize=(20,20))
###Output
_____no_output_____
###Markdown
The average app ratings across categories is significantly different. Best performing categories
###Code
groups = df.groupby('Category').filter(lambda x: len(x) >= 170).reset_index()
#print(type(groups.item.['BUSINESS']))
print('Average rating = ', np.nanmean(list(groups.Rating)))
#print(len(groups.loc[df.Category == 'DATING']))
c = ['hsl('+str(h)+',50%'+',50%)' for h in np.linspace(0, 720, len(set(groups.Category)))]
#df_sorted = df.groupby('Category').agg({'Rating':'median'}).reset_index().sort_values(by='Rating', ascending=False)
#print(df_sorted)
layout = {'title' : 'App ratings across major categories',
'xaxis': {'tickangle':-40},
'yaxis': {'title': 'Rating'},
'plot_bgcolor': 'rgb(250,250,250)',
'shapes': [{
'type' :'line',
'x0': -.5,
'y0': np.nanmean(list(groups.Rating)),
'x1': 19,
'y1': np.nanmean(list(groups.Rating)),
'line': { 'dash': 'dashdot'}
}]
}
data = [{
'y': df.loc[df.Category==category]['Rating'],
'type':'violin',
'name' : category,
'showlegend':False,
#'marker': {'color': 'Set2'},
} for i,category in enumerate(list(set(groups.Category)))]
plotly.offline.iplot({'data': data, 'layout': layout})
###Output
_____no_output_____
###Markdown
- Almost all app categories perform decently. **Health and Fitness** and **Books and Reference** produce the highest quality apps with **50% apps having a rating greater than 4.5.** This is extremely high!- On the contrary, **50% of apps in the Dating category have a rating lesser than the average rating**. - A few **junk apps** also exist in the **Lifestyle**, **Family** and **Finance** category. Sizing Strategy - Light Vs Bulky?How do app sizes impact the app rating?
###Code
groups = df.groupby('Category').filter(lambda x: len(x) >= 50).reset_index()
# sns.set_style('ticks')
# fig, ax = plt.subplots()
# fig.set_size_inches(8, 8)
sns.set_style("darkgrid")
ax = sns.jointplot(df['Size'], df['Rating'])
#ax.set_title('Rating Vs Size')
###Output
_____no_output_____
###Markdown
Most top rated apps are optimally sized between **~2MB to ~40MB** - neither too light nor too heavy.
###Code
c = ['hsl('+str(h)+',50%'+',50%)' for h in np.linspace(0, 360, len(list(set(groups.Category))))]
subset_df = df[df.Size > 40]
groups_temp = subset_df.groupby('Category').filter(lambda x: len(x) >20)
# for category in enumerate(list(set(groups_temp.Category))):
# print (category)
data = [{
'x': groups_temp.loc[subset_df.Category==category[1]]['Rating'],
'type':'scatter',
'y' : subset_df['Size'],
'name' : str(category[1]),
'mode' : 'markers',
'showlegend': True,
#'marker': {'color':c[i]}
#'text' : df['rating'],
} for category in enumerate(['GAME', 'FAMILY'])]
layout = {'title':"Rating vs Size",
'xaxis': {'title' : 'Rating'},
'yaxis' : {'title' : 'Size (in MB)'},
'plot_bgcolor': 'rgb(0,0,0)'}
plotly.offline.iplot({'data': data, 'layout': layout})
# heavy_categories = [ 'ENTERTAINMENT', 'MEDICAL', 'DATING']
# data = [{
# 'x': groups.loc[df.Category==category]['Rating'],
# 'type':'scatter',
# 'y' : df['Size'],
# 'name' : category,
# 'mode' : 'markers',
# 'showlegend': True,
# #'text' : df['rating'],
# } for category in heavy_categories]
###Output
_____no_output_____
###Markdown
Most bulky apps ( >50MB) belong to the **Game** and **Family** category. Despite this, these bulky apps are fairly highly rated indicating that they are bulky for a purpose. Pricing Strategy - Free Vs Paid?How do app prices impact app rating?
###Code
paid_apps = df[df.Price>0]
p = sns.jointplot( "Price", "Rating", paid_apps)
###Output
_____no_output_____
###Markdown
Most top rated apps are optimally priced between **~1\$ to ~30\$**. There are only a very few apps priced above 20\$. Current pricing trend - How to price your app?
###Code
subset_df = df[df.Category.isin(['GAME', 'FAMILY', 'PHOTOGRAPHY', 'MEDICAL', 'TOOLS', 'FINANCE',
'LIFESTYLE','BUSINESS'])]
sns.set_style('darkgrid')
fig, ax = plt.subplots()
fig.set_size_inches(15, 8)
p = sns.stripplot(x="Price", y="Category", data=subset_df, jitter=True, linewidth=1)
title = ax.set_title('App pricing trend across categories')
###Output
_____no_output_____
###Markdown
**Shocking...Apps priced above 250\$ !!! ** Let's quickly examine what these junk apps are.
###Code
#print('Junk apps priced above 350$')
df[['Category', 'App']][df.Price > 200]
fig, ax = plt.subplots()
fig.set_size_inches(15, 8)
subset_df_price = subset_df[subset_df.Price<100]
p = sns.stripplot(x="Price", y="Category", data=subset_df_price, jitter=True, linewidth=1)
title = ax.set_title('App pricing trend across categories - after filtering for junk apps')
###Output
_____no_output_____
###Markdown
- Clearly, **Medical and Family apps** are the most expensive. Some medical apps extend even upto 80\$.- All other apps are priced under 30\$.- Surprisingly, **all game apps are reasonably priced below 20\$.** Distribution of paid and free apps across categories
###Code
# Stacked bar graph for top 5-10 categories - Ratio of paid and free apps
#fig, ax = plt.subplots(figsize=(15,10))
new_df = df.groupby(['Category', 'Type']).agg({'App' : 'count'}).reset_index()
#print(new_df)
# outer_group_names = df['Category'].sort_values().value_counts()[:5].index
# outer_group_values = df['Category'].sort_values().value_counts()[:5].values
outer_group_names = ['GAME', 'FAMILY', 'MEDICAL', 'TOOLS']
outer_group_values = [len(df.App[df.Category == category]) for category in outer_group_names]
a, b, c, d=[plt.cm.Blues, plt.cm.Reds, plt.cm.Greens, plt.cm.Purples]
inner_group_names = ['Paid', 'Free'] * 4
inner_group_values = []
#inner_colors = ['#58a27c','#FFD433']
for category in outer_group_names:
for t in ['Paid', 'Free']:
x = new_df[new_df.Category == category]
try:
#print(x.App[x.Type == t].values[0])
inner_group_values.append(int(x.App[x.Type == t].values[0]))
except:
#print(x.App[x.Type == t].values[0])
inner_group_values.append(0)
explode = (0.025,0.025,0.025,0.025)
# First Ring (outside)
fig, ax = plt.subplots(figsize=(10,10))
ax.axis('equal')
mypie, texts, _ = ax.pie(outer_group_values, radius=1.2, labels=outer_group_names, autopct='%1.1f%%', pctdistance=1.1,
labeldistance= 0.75, explode = explode, colors=[a(0.6), b(0.6), c(0.6), d(0.6)], textprops={'fontsize': 16})
plt.setp( mypie, width=0.5, edgecolor='black')
# Second Ring (Inside)
mypie2, _ = ax.pie(inner_group_values, radius=1.2-0.5, labels=inner_group_names, labeldistance= 0.7,
textprops={'fontsize': 12}, colors = [a(0.4), a(0.2), b(0.4), b(0.2), c(0.4), c(0.2), d(0.4), d(0.2)])
plt.setp( mypie2, width=0.5, edgecolor='black')
plt.margins(0,0)
# show it
plt.tight_layout()
plt.show()
#ax = sns.countplot(x="Category", hue="Type", data=new_df)
#df.groupby(['Category', 'Type']).count()['App'].unstack().plot(kind='bar', stacked=True, ax=ax)
#ylabel = plt.ylabel('Number of apps')
###Output
_____no_output_____
###Markdown
Distribution of free and paid apps across major categories Are paid apps downloaded as much as free apps?
###Code
trace0 = go.Box(
y=np.log10(df['Installs'][df.Type=='Paid']),
name = 'Paid',
marker = dict(
color = 'rgb(214, 12, 140)',
)
)
trace1 = go.Box(
y=np.log10(df['Installs'][df.Type=='Free']),
name = 'Free',
marker = dict(
color = 'rgb(0, 128, 128)',
)
)
layout = go.Layout(
title = "Number of downloads of paid apps Vs free apps",
yaxis= {'title': 'Number of downloads (log-scaled)'}
)
data = [trace0, trace1]
plotly.offline.iplot({'data': data, 'layout': layout})
###Output
_____no_output_____
###Markdown
**Paid apps have a relatively lower number of downloads than free apps.** However, it is not too bad. How do the sizes of paid apps and free apps vary?
###Code
temp_df = df[df.Type == 'Paid']
temp_df = temp_df[temp_df.Size > 5]
#type_groups = df.groupby('Type')
data = [{
#'x': type_groups.get_group(t)['Rating'],
'x' : temp_df['Rating'],
'type':'scatter',
'y' : temp_df['Size'],
#'name' : t,
'mode' : 'markers',
#'showlegend': True,
'text' : df['Size'],
} for t in set(temp_df.Type)]
layout = {'title':"Rating vs Size",
'xaxis': {'title' : 'Rating'},
'yaxis' : {'title' : 'Size (in MB)'},
'plot_bgcolor': 'rgb(0,0,0)'}
plotly.offline.iplot({'data': data, 'layout': layout})
###Output
_____no_output_____
###Markdown
- **Majority of the paid apps that are highly rated have small sizes.** This means that most paid apps are designed and developed to cater to specific functionalities and hence are not bulky.- **Users prefer to pay for apps that are light-weighted.** A paid app that is bulky may not perform well in the market. Exploring Correlations
###Code
#df['Installs'].corr(df['Reviews'])#df['Insta
#print(np.corrcoef(l, rating))
corrmat = df.corr()
#f, ax = plt.subplots()
p =sns.heatmap(corrmat, annot=True, cmap=sns.diverging_palette(220, 20, as_cmap=True))
df_copy = df.copy()
df_copy = df_copy[df_copy.Reviews > 10]
df_copy = df_copy[df_copy.Installs > 0]
df_copy['Installs'] = np.log10(df['Installs'])
df_copy['Reviews'] = np.log10(df['Reviews'])
sns.lmplot("Reviews", "Installs", data=df_copy)
ax = plt.gca()
_ = ax.set_title('Number of Reviews Vs Number of Downloads (Log scaled)')
###Output
_____no_output_____
###Markdown
**A moderate positive correlation of 0.63 exists between the number of reviews and number of downloads.** This means that customers tend to download a given app more if it has been reviewed by a larger number of people. This also means that many active users who download an app usually also leave back a review or feedback. So, getting your app reviewed by more people maybe a good idea to increase your app's capture in the market! Basic sentiment analysis - User reviews
###Code
reviews_df = pd.read_csv('../input/googleplaystore_user_reviews.csv')
merged_df = pd.merge(df, reviews_df, on = "App", how = "inner")
merged_df = merged_df.dropna(subset=['Sentiment', 'Translated_Review'])
grouped_sentiment_category_count = merged_df.groupby(['Category', 'Sentiment']).agg({'App': 'count'}).reset_index()
grouped_sentiment_category_sum = merged_df.groupby(['Category']).agg({'Sentiment': 'count'}).reset_index()
new_df = pd.merge(grouped_sentiment_category_count, grouped_sentiment_category_sum, on=["Category"])
#print(new_df)
new_df['Sentiment_Normalized'] = new_df.App/new_df.Sentiment_y
new_df = new_df.groupby('Category').filter(lambda x: len(x) ==3)
# new_df = new_df[new_df.Category.isin(['HEALTH_AND_FITNESS', 'GAME', 'FAMILY', 'EDUCATION', 'COMMUNICATION',
# 'ENTERTAINMENT', 'TOOLS', 'SOCIAL', 'TRAVEL_AND_LOCAL'])]
new_df
trace1 = go.Bar(
x=list(new_df.Category[::3])[6:-5],
y= new_df.Sentiment_Normalized[::3][6:-5],
name='Negative',
marker=dict(color = 'rgb(209,49,20)')
)
trace2 = go.Bar(
x=list(new_df.Category[::3])[6:-5],
y= new_df.Sentiment_Normalized[1::3][6:-5],
name='Neutral',
marker=dict(color = 'rgb(49,130,189)')
)
trace3 = go.Bar(
x=list(new_df.Category[::3])[6:-5],
y= new_df.Sentiment_Normalized[2::3][6:-5],
name='Positive',
marker=dict(color = 'rgb(49,189,120)')
)
data = [trace1, trace2, trace3]
layout = go.Layout(
title = 'Sentiment analysis',
barmode='stack',
xaxis = {'tickangle': -45},
yaxis = {'title': 'Fraction of reviews'}
)
fig = go.Figure(data=data, layout=layout)
plotly.offline.iplot({'data': data, 'layout': layout})
###Output
_____no_output_____
###Markdown
- **Health and Fitness** apps perform the best, having more than **85% positive reviews**.- On the contrary, many **Game and Social** apps perform bad leading to **50% positive and 50% negative**.
###Code
#merged_df.loc[merged_df.Type=='Free']['Sentiment_Polarity']
sns.set_style('ticks')
sns.set_style("darkgrid")
fig, ax = plt.subplots()
fig.set_size_inches(11.7, 8.27)
ax = sns.boxplot(x='Type', y='Sentiment_Polarity', data=merged_df)
title = ax.set_title('Sentiment Polarity Distribution')
###Output
_____no_output_____
###Markdown
- **Free apps receive a lot of harsh comments** which are indicated as outliers on the negative Y-axis. - **Users are more lenient and tolerant while reviewing paid apps - moderate choice of words.** They are never extremely negative while reviewing a paid app. WORDCLOUD - A quick look on reviews
###Code
from wordcloud import WordCloud
wc = WordCloud(background_color="white", max_words=200, colormap="Set2")
# generate word cloud
from nltk.corpus import stopwords
stop = stopwords.words('english')
stop = stop + ['app', 'APP' ,'ap', 'App', 'apps', 'application', 'browser', 'website', 'websites', 'chrome', 'click', 'web', 'ip', 'address',
'files', 'android', 'browse', 'service', 'use', 'one', 'download', 'email', 'Launcher']
#merged_df = merged_df.dropna(subset=['Translated_Review'])
merged_df['Translated_Review'] = merged_df['Translated_Review'].apply(lambda x: " ".join(x for x in str(x).split(' ') if x not in stop))
#print(any(merged_df.Translated_Review.isna()))
merged_df.Translated_Review = merged_df.Translated_Review.apply(lambda x: x if 'app' not in x.split(' ') else np.nan)
merged_df.dropna(subset=['Translated_Review'], inplace=True)
free = merged_df.loc[merged_df.Type=='Free']['Translated_Review'].apply(lambda x: '' if x=='nan' else x)
wc.generate(''.join(str(free)))
plt.figure(figsize=(10, 10))
plt.imshow(wc, interpolation='bilinear')
plt.axis("off")
plt.show()
###Output
_____no_output_____
###Markdown
** FREE APPS **> **Negative words: ads, bad, hate**>**Positive words: good, love, best, great**
###Code
paid = merged_df.loc[merged_df.Type=='Paid']['Translated_Review'].apply(lambda x: '' if x=='nan' else x)
wc.generate(''.join(str(paid)))
plt.figure(figsize=(10, 10))
plt.imshow(wc, interpolation='bilinear')
plt.axis("off")
plt.show()
###Output
_____no_output_____ |
.ipynb_checkpoints/Training Module_single-checkpoint.ipynb | ###Markdown
CMC Data Processing
###Code
# data_bt = pd.read_csv("cmc_btc.csv")
# data_bt['Date'] = pd.to_datetime(data_bt['Date'])
# data_bt = data_bt.sort_values(by=['Date']).reset_index(drop=True)
# data_bt.head()
# date_col = pd.to_datetime(data_bt["Date"])
# data_open = data_bt["Open*"]
# data_high = data_bt["High"]
# data_low = data_bt["Low"]
# data_close = data_bt["Close**"]
# data_vol = data_bt["Volume"]
# data_mark = data_bt["Market Cap"]
###Output
_____no_output_____
###Markdown
BITFINEX DATA PROCESSING
###Code
data_bt = pd.read_csv("BITFINEX_SPOT_BTC_USD/1DAY_2013-03-31&2018-09-20.csv")
data_bt['time_close'] = pd.to_datetime(data_bt['time_close'])
data_bt = data_bt.sort_values(by=['time_close']).reset_index(drop=True)
data_bt.head()
data_bt.dropna(inplace=True)
data_bt.reset_index(drop=True, inplace=True)
data_bt.isna().any()
date_col = pd.to_datetime(data_bt["time_close"])
data_open = data_bt["price_open"]
data_high = data_bt["price_high"]
data_low = data_bt["price_low"]
data_close = data_bt["price_close"]
data_vol = data_bt["volume_traded"]
data_mark = data_bt["market_cap"]
###Output
_____no_output_____
###Markdown
Log Transformation
###Code
log_open = np.log(data_open)
log_high = np.log(data_high)
log_low = np.log(data_low)
log_close = np.log(data_close)
log_vol = np.log(data_vol)
log_mark = np.log(data_mark)
###Output
_____no_output_____
###Markdown
STL Decompostion
###Code
log_open = pd.DataFrame(log_open)
log_open = log_open.set_index(date_col)
log_high = pd.DataFrame(log_high)
log_high = log_high.set_index(date_col)
log_low = pd.DataFrame(log_low)
log_low = log_low.set_index(date_col)
log_close = pd.DataFrame(log_close)
log_close = log_close.set_index(date_col)
log_vol = pd.DataFrame(log_vol)
log_vol = log_vol.set_index(date_col)
log_mark = pd.DataFrame(log_mark)
log_mark = log_mark.set_index(date_col)
stl_open = decompose(log_open)
stl_high = decompose(log_high)
stl_low = decompose(log_low)
stl_close = decompose(log_close)
stl_vol = decompose(log_vol)
stl_mark = decompose(log_mark)
###Output
_____no_output_____
###Markdown
Deasonal TS component
###Code
deseason_open = (stl_open.resid + stl_open.trend).iloc[:,0]
deseason_high = (stl_high.resid + stl_high.trend).iloc[:,0]
deseason_low = (stl_low.resid + stl_low.trend).iloc[:,0]
deseason_close = (stl_close.resid + stl_close.trend).iloc[:,0]
deseason_vol = (stl_vol.resid + stl_vol.trend).iloc[:,0]
deseason_mark = (stl_mark.resid + stl_mark.trend).iloc[:,0]
###Output
_____no_output_____
###Markdown
Input Normalized Window
###Code
date_col_win_in = rolling_window(date_col[0:(len(data_bt)-out_win)], in_win, 1)
date_col_win_in_exp = np.expand_dims(date_col_win_in, axis=2)
data_open_win_in = rolling_window(deseason_open[0:(len(deseason_open)-out_win)], in_win, 1)
data_open_win_in = pd.DataFrame(data_open_win_in)
norm_open_win_in = data_open_win_in.subtract(data_open_win_in.iloc[:,in_win-1], axis='index')
norm_open_win_in_exp = np.expand_dims(norm_open_win_in.values, axis=2)
data_high_win_in = rolling_window(deseason_high[0:(len(deseason_high)-out_win)], in_win, 1)
data_high_win_in = pd.DataFrame(data_high_win_in)
norm_high_win_in = data_high_win_in.subtract(data_high_win_in.iloc[:,in_win-1], axis='index')
norm_high_win_in_exp = np.expand_dims(norm_high_win_in.values, axis=2)
data_low_win_in = rolling_window(deseason_low[0:(len(deseason_low)-out_win)], in_win, 1)
data_low_win_in = pd.DataFrame(data_low_win_in)
norm_low_win_in = data_low_win_in.subtract(data_low_win_in.iloc[:,in_win-1], axis='index')
norm_low_win_in_exp = np.expand_dims(norm_low_win_in.values, axis=2)
data_close_win_in = rolling_window(deseason_close[0:(len(deseason_close)-out_win)], in_win, 1)
data_close_win_in = pd.DataFrame(data_close_win_in)
norm_close_win_in = data_close_win_in.subtract(data_close_win_in.iloc[:,in_win-1], axis='index')
norm_close_win_in_exp = np.expand_dims(norm_close_win_in.values, axis=2)
data_vol_win_in = rolling_window(deseason_vol[0:(len(deseason_vol)-out_win)], in_win, 1)
data_vol_win_in = pd.DataFrame(data_vol_win_in)
norm_vol_win_in = data_vol_win_in.subtract(data_vol_win_in.iloc[:,in_win-1], axis='index')
norm_vol_win_in_exp = np.expand_dims(norm_vol_win_in.values, axis=2)
data_mark_win_in = rolling_window(deseason_mark[0:(len(deseason_mark)-out_win)], in_win, 1)
data_mark_win_in = pd.DataFrame(data_mark_win_in)
norm_mark_win_in = data_mark_win_in.subtract(data_mark_win_in.iloc[:,in_win-1], axis='index')
norm_mark_win_in_exp = np.expand_dims(norm_mark_win_in.values, axis=2)
###Output
C:\ProgramData\Anaconda3\lib\site-packages\ipykernel\__main__.py:3: FutureWarning: Series.strides is deprecated and will be removed in a future version
app.launch_new_instance()
###Markdown
Output Normalized Window
###Code
date_col_win_out = rolling_window(date_col[in_win:len(data_bt)], out_win, 1)
date_col_win_out_exp = np.expand_dims(date_col_win_out, axis=2)
data_high_win_out = rolling_window(deseason_high[in_win:len(deseason_high)], out_win, 1)
data_high_win_out = pd.DataFrame(data_high_win_out)
norm_high_win_out = data_high_win_out.subtract(data_high_win_in.iloc[:,out_win-1], axis='index').values
#norm_high_win_out_exp = np.expand_dims(norm_high_win_out.values, axis=2)
data_low_win_out = rolling_window(deseason_low[in_win:len(deseason_high)], out_win, 1)
data_low_win_out = pd.DataFrame(data_low_win_out)
norm_low_win_out = data_low_win_out.subtract(data_low_win_in.iloc[:,out_win-1], axis='index').values
#norm_low_win_out_exp = np.expand_dims(norm_low_win_out.values, axis=2)
data_close_win_out = rolling_window(deseason_close[in_win:len(deseason_high)], out_win, 1)
data_close_win_out = pd.DataFrame(data_close_win_out)
norm_close_win_out = data_close_win_out.subtract(data_close_win_in.iloc[:,out_win-1], axis='index').values
#norm_close_win_out_exp = np.expand_dims(norm_close_win_out.values, axis=2)
###Output
C:\ProgramData\Anaconda3\lib\site-packages\ipykernel\__main__.py:3: FutureWarning: Series.strides is deprecated and will be removed in a future version
app.launch_new_instance()
###Markdown
Stacking Data for Training in High Dimension
###Code
x_all = np.dstack((norm_open_win_in_exp, norm_high_win_in_exp, norm_low_win_in_exp
,norm_close_win_in_exp, norm_vol_win_in_exp, norm_mark_win_in_exp))
x_all.shape
#y_all = np.dstack((norm_high_win_out_exp, norm_low_win_out_exp, norm_close_win_out_exp))
y_all = norm_close_win_out
y_all.shape
###Output
_____no_output_____
###Markdown
Train Validation Test Split
###Code
X_train, X_test, y_train, y_test = train_test_split(x_all, y_all, test_size=0.10,shuffle=False)
print(X_test.shape, X_train.shape)
###Output
(194, 15, 6) (1740, 15, 6)
###Markdown
Building Deep Learning Architecture using Keras
###Code
from keras import Sequential
from keras.layers import LSTM, RepeatVector, TimeDistributed, Dense, Activation,Permute,Conv1D,GaussianNoise,Dropout,regularizers,Conv2D,Reshape
from keras.optimizers import SGD, nadam,adam
from keras.callbacks import ReduceLROnPlateau,EarlyStopping
hidden_neurons = 128
nets = 256
batch_size = 16
model = Sequential()
#model.add(LSTM(batch_input_shape = (batch_size,15,6), units=hidden_neurons, stateful = False, return_sequences=False))
model.add(Conv1D(nets, 3, strides=16, padding='same', input_shape=(X_train.shape[1], X_train.shape[2])))
#model.add(Reshape((nets, -1)))
#model.add(Permute((2, 1)))
model.add(LSTM(units=hidden_neurons, stateful = False, return_sequences=False))
model.add(Dense(62))
model.add(GaussianNoise(0.005))
model.add(Dense(12,use_bias=False, bias_initializer='zeros',kernel_regularizer=regularizers.l2(0.0008)))
#model.add(TimeDistributed(Activation('linear')))
#sgd = SGD(lr=0.01, decay=0.9, momentum=0.9, nesterov=True)
optm = nadam(lr=0.01)
#model.add(Activation('linear'))
model.compile(loss='mae', optimizer=optm, metrics=['accuracy'])
model.summary()
###Output
_________________________________________________________________
Layer (type) Output Shape Param #
=================================================================
conv1d_14 (Conv1D) (None, 1, 256) 4864
_________________________________________________________________
lstm_20 (LSTM) (None, 128) 197120
_________________________________________________________________
dense_12 (Dense) (None, 62) 7998
_________________________________________________________________
gaussian_noise_8 (GaussianNo (None, 62) 0
_________________________________________________________________
dense_13 (Dense) (None, 12) 744
=================================================================
Total params: 210,726
Trainable params: 210,726
Non-trainable params: 0
_________________________________________________________________
###Markdown
Training Model
###Code
# for i in range(200):
# model.fit(X_train, y_train,
# epochs=1,
# batch_size=batch_size,
# verbose=2,
# validation_data = (X_test, y_test))
# model.reset_states()
reduce_lr = ReduceLROnPlateau(monitor='val_loss',patience=3, min_lr=0.00001,factor=0.2)
#early_stopping = EarlyStopping(monitor='val_loss', patience=5)
model.fit(X_train, y_train,
epochs=40,
batch_size=batch_size,
verbose=2,
callbacks=[reduce_lr],
validation_data = (X_test, y_test))
# Save the weights
model.save_weights('model_weights.h5')
# Save the model architecture
with open('model_architecture.json', 'w') as f:
f.write(model.to_json())
# Model reconstruction from JSON file
with open('model_architecture.json', 'r') as f:
model = model_from_json(f.read())
# Load weights into the new model
model.load_weights('model_weights.h5')
###Output
_____no_output_____
###Markdown
Carrying out Prediction on test data set
###Code
y_pred = model.predict(X_test)
y_all.shape
###Output
_____no_output_____
###Markdown
Renormalizing and seasonalizing the predictions and true labels
###Code
season_high = pd.DataFrame(rolling_window(stl_high.seasonal.iloc[:,0][in_win:len(stl_high.seasonal)], out_win, 1))
season_low = pd.DataFrame(rolling_window(stl_low.seasonal.iloc[:,0][in_win:len(stl_low.seasonal)], out_win, 1))
season_close = pd.DataFrame(rolling_window(stl_close.seasonal.iloc[:,0][in_win:len(stl_close.seasonal)], out_win, 1))
season_high_pr = pd.DataFrame(rolling_window(stl_high.seasonal.iloc[:,0][0:(len(stl_high.seasonal)-out_win)], in_win, 1))
season_high_pr.drop(season_high_pr.columns[0:(in_win-out_win)], axis=1, inplace=True)
season_high_pr.columns = np.arange(len(season_high_pr.columns))
season_low_pr = pd.DataFrame(rolling_window(stl_low.seasonal.iloc[:,0][0:(len(stl_low.seasonal)-out_win)], in_win, 1))
season_low_pr.drop(season_low_pr.columns[0:(in_win-out_win)], axis=1, inplace=True)
season_low_pr.columns = np.arange(len(season_low_pr.columns))
season_close_pr = pd.DataFrame(rolling_window(stl_close.seasonal.iloc[:,0][0:(len(stl_close.seasonal)-out_win)], in_win, 1))
season_close_pr.drop(season_close_pr.columns[0:(in_win-out_win)], axis=1, inplace=True)
season_close_pr.columns = np.arange(len(season_close_pr.columns))
X_train.shape[0]
###Output
_____no_output_____
###Markdown
Transforming Predictions
###Code
#high_pred = pd.DataFrame(y_pred[:,:,0])
#low_pred = pd.DataFrame(y_pred[:,:,1])
close_pred = pd.DataFrame(y_pred[:,0])
#high_denorm_pred = high_pred.add(data_high_win_in.iloc[X_train.shape[0]:,out_win-1].reset_index(drop=True), axis='index')
#low_denorm_pred = low_pred.add(data_low_win_in.iloc[X_train.shape[0]:,out_win-1].reset_index(drop=True), axis='index')
close_denorm_pred = close_pred.add(data_close_win_in.iloc[X_train.shape[0]:,out_win-1].reset_index(drop=True), axis='index')
#deseason_high_pred = high_denorm_pred.add(season_high_pr.iloc[X_train.shape[0]:,].reset_index(drop=True), axis='index')
#deseason_low_pred = low_denorm_pred.add(season_low_pr.iloc[X_train.shape[0]:,].reset_index(drop=True), axis='index')
deseason_close_pred = close_denorm_pred.add(season_close_pr.iloc[X_train.shape[0]:,].reset_index(drop=True), axis='index')
#pred_high = np.exp(deseason_high_pred)
#pred_low = np.exp(deseason_low_pred)
pred_close = np.exp(deseason_close_pred)
pred_close.shape
###Output
_____no_output_____
###Markdown
Transforming True Labels
###Code
#high_test = pd.DataFrame(y_test[:,:,0])
#low_test = pd.DataFrame(y_test[:,:,1])
close_test = pd.DataFrame(y_test[:,0])
#high_denorm_test = high_test.add(data_high_win_in.iloc[X_train.shape[0]:,out_win-1].reset_index(drop=True), axis='index')
#low_denorm_test = low_test.add(data_low_win_in.iloc[X_train.shape[0]:,out_win-1].reset_index(drop=True), axis='index')
close_denorm_test = close_test.add(data_close_win_in.iloc[X_train.shape[0]:,out_win-1].reset_index(drop=True), axis='index')
#deseason_high_test = high_denorm_test.add(season_high.iloc[X_train.shape[0]:,].reset_index(drop=True))
#deseason_low_test = low_denorm_test.add(season_low.iloc[X_train.shape[0]:,].reset_index(drop=True))
deseason_close_test = close_denorm_test.add(season_close.iloc[X_train.shape[0]:,].reset_index(drop=True))
#test_high = np.exp(deseason_high_test)
#test_low = np.exp(deseason_low_test)
test_close = np.exp(deseason_close_test)
from matplotlib import pyplot as plt
#X_test[1,:,1]
date_col_win_test = rolling_window(date_col[0:(len(data_bt)-out_win)], in_win, 1)[X_train.shape[0]:]
date_col_win_test = pd.DataFrame(date_col_win_test)
data_open_win_test = rolling_window(data_open[0:(len(data_open)-out_win)], in_win, 1)[X_train.shape[0]:]
data_open_win_test = pd.DataFrame(data_open_win_test)
data_high_win_test = rolling_window(data_high[0:(len(data_high)-out_win)], in_win, 1)[X_train.shape[0]:]
data_high_win_test = pd.DataFrame(data_high_win_test)
data_low_win_test = rolling_window(data_low[0:(len(data_low)-out_win)], in_win, 1)[X_train.shape[0]:]
data_low_win_test = pd.DataFrame(data_low_win_test)
data_close_win_test = rolling_window(data_close[0:(len(data_close)-out_win)], in_win, 1)[X_train.shape[0]:]
data_close_win_test = pd.DataFrame(data_close_win_test)
data_vol_win_test = rolling_window(data_vol[0:(len(data_vol)-out_win)], in_win, 1)[X_train.shape[0]:]
data_vol_win_test = pd.DataFrame(data_vol_win_test)
data_mark_win_test = rolling_window(data_mark[0:(len(data_mark)-out_win)], in_win, 1)[X_train.shape[0]:]
data_mark_win_test = pd.DataFrame(data_mark_win_test)
date_col_win_pred = pd.DataFrame(date_col_win_out[X_train.shape[0]:])
date_col_win_pred.shape
print(type(data_high_win_test))
data_high_win_test.shape
%matplotlib qt
for index,row in pred_close.iterrows():
plt.figure()
plt.plot(date_col_win_pred.iloc[index,:],pred_close.iloc[index,:],color='r',marker = '.')
plt.plot(date_col_win_test.iloc[index,:],data_close_win_test.iloc[index,:],color='g',marker = '.')
plt.plot(date_col_win_pred.iloc[index,:],test_close.iloc[index,:],color='b',marker = '.')
if index == 5:
break
plt.show()
error_high = []
error_low = []
error_close = []
# for index,row in pred_high.iterrows():
# error_high.append(smape(test_high.iloc[index,:], pred_high.iloc[index,:]))
# for index,row in pred_low.iterrows():
# error_low.append(smape(test_low.iloc[index,:], pred_low.iloc[index,:]))
for index,row in pred_close.iterrows():
error_close.append(smape(test_close.iloc[index,:], pred_close.iloc[index,:]))
###Output
_____no_output_____
###Markdown
SMAPE ERROR
###Code
# print("High prediciton SMAPE score : ",sum(error_high)/len(error_high))
# print("Low prediciton SMAPE score : ",sum(error_low)/len(error_low))
print("Close prediciton SMAPE score : ",sum(error_close)/len(error_close))
#print("Overall SMAPE score : ",((sum(error_high)/len(error_high)) + (sum(error_low)/len(error_low)) + (sum(error_close)/len(error_close)))/3)
close_pred_check = data_close_win_test.iloc[:,in_win-1]-pred_close.iloc[:,0]
close_true_check = data_close_win_test.iloc[:,in_win-1]-test_close.iloc[:,0]
# high_pred_check = data_high_win_test.iloc[:,in_win-1]-pred_high.iloc[:,0]
# high_true_check = data_high_win_test.iloc[:,in_win-1]-test_high.iloc[:,0]
# low_pred_check = data_low_win_test.iloc[:,in_win-1]-pred_low.iloc[:,0]
# low_true_check = data_low_win_test.iloc[:,in_win-1]-test_low.iloc[:,0]
###Output
_____no_output_____
###Markdown
F-Score , Precision Recall, True Positive Rate & True Negative Rate
###Code
close_tp = 0
close_tn = 0
close_fp = 0
close_fn = 0
# high_tp = 0
# high_tn = 0
# high_fp = 0
# high_fn = 0
# low_tp = 0
# low_tn = 0
# low_fp = 0
# low_fn = 0
for i in range(0,len(close_pred_check)):
if(close_true_check[i] >= 0 and close_pred_check[i] >= 0):
close_tp += 1
if(close_true_check[i] >= 0 and close_pred_check[i] < 0):
close_tn += 1
if(close_true_check[i] < 0 and close_pred_check[i] < 0):
close_fp += 1
if(close_true_check[i] < 0 and close_pred_check[i] >= 0):
close_fn += 1
# if(high_true_check[i] >= 0 and high_pred_check[i] >= 0):
# high_tp += 1
# if(high_true_check[i] >= 0 and high_pred_check[i] < 0):
# high_tn += 1
# if(high_true_check[i] < 0 and high_pred_check[i] < 0):
# high_fp += 1
# if(high_true_check[i] < 0 and high_pred_check[i] >= 0):
# high_fn += 1
# if(low_true_check[i] >= 0 and low_pred_check[i] >= 0):
# low_tp += 1
# if(low_true_check[i] >= 0 and low_pred_check[i] < 0):
# low_tn += 1
# if(low_true_check[i] < 0 and low_pred_check[i] < 0):
# low_fp += 1
# if(low_true_check[i] < 0 and low_pred_check[i] >= 0):
# low_fn += 1
def precision(tp,fp):
return(tp/(tp + fp))
def recall(tp,fn):
return(tp/(tp + fn))
def f_score(precision,recall):
return(2*((precision * recall)/(precision + recall)))
def tp_rate(tp,fn):
return(tp/(tp+fn))
def fp_rate(fp,tn):
return(fp/(fp+tn))
def Accuracy(TP,TN,FP,FN):
return (TP + TN) / (TP + TN + FP + FN)
###Output
_____no_output_____
###Markdown
Close
###Code
close_precision = [precision(close_tp,close_fp) , precision(close_tn,close_fn)]
close_recall = [recall(close_tp,close_fn) , recall(close_tn,close_fp)]
close_f_score = [f_score(close_precision[0],close_recall[0]) , f_score(close_precision[1],close_recall[1])]
close_tp_rate = [tp_rate(close_tp,close_fn), tp_rate(close_tn,close_fp)]
close_fp_rate = [fp_rate(close_fp,close_tn) , fp_rate(close_fn,close_tp)]
close_Accuracy = [Accuracy(close_tp,close_tn,close_fp,close_fn), Accuracy(close_tn,close_tp,close_fn,close_fp)]
print("close precision : ",close_precision)
print("close recall : ",close_recall)
print("close f_score : ",close_f_score)
print("close tp_rate : ",close_tp_rate)
print("close fp_rate : ",close_fp_rate)
print("close Accuracy : ",close_Accuracy)
###Output
close precision : [0.3563218390804598, 0.6355140186915887]
close recall : [0.44285714285714284, 0.5483870967741935]
close f_score : [0.3949044585987261, 0.5887445887445887]
close tp_rate : [0.44285714285714284, 0.5483870967741935]
close fp_rate : [0.45161290322580644, 0.5571428571428572]
close Accuracy : [0.5103092783505154, 0.5103092783505154]
###Markdown
High
###Code
# high_precision = [precision(high_tp,high_fp) , precision(high_tn,high_fn)]
# high_recall = [recall(high_tp,high_fn) , recall(high_tn,high_fp)]
# high_f_score = [f_score(high_precision[0],high_recall[0]) , f_score(high_precision[1],high_recall[1])]
# high_tp_rate = [tp_rate(high_tp,high_fn), tp_rate(high_tn,high_fp)]
# high_fp_rate = [fp_rate(high_fp,high_tn) , fp_rate(high_fn,high_tp)]
# high_Accuracy = [Accuracy(high_tp,high_tn,high_fp,high_fn), Accuracy(high_tn,high_tp,high_fn,high_fp)]
# print("high precision : ",high_precision)
# print("high recall : ",high_recall)
# print("high f_score : ",high_f_score)
# print("high tp_rate : ",high_tp_rate)
# print("high fp_rate : ",high_fp_rate)
# print("high Accuracy : ",high_Accuracy)
###Output
_____no_output_____
###Markdown
Low
###Code
# low_precision = [precision(low_tp,low_fp) , precision(low_tn,low_fn)]
# low_recall = [recall(low_tp,low_fn) , recall(low_tn,low_fp)]
# low_f_score = [f_score(low_precision[0],low_recall[0]) , f_score(low_precision[1],low_recall[1])]
# low_tp_rate = [tp_rate(low_tp,low_fn), tp_rate(low_tn,low_fp)]
# low_fp_rate = [fp_rate(low_fp,low_tn) , fp_rate(low_fn,low_tp)]
# low_Accuracy = [Accuracy(low_tp,low_tn,low_fp,low_fn), Accuracy(low_tn,low_tp,low_fn,low_fp)]
# print("low precision : ",low_precision)
# print("low recall : ",low_recall)
# print("low f_score : ",low_f_score)
# print("low tp_rate : ",low_tp_rate)
# print("low fp_rate : ",low_fp_rate)
# print("low Accuracy ,: ",low_Accuracy)
###Output
_____no_output_____ |
data collecting process.ipynb | ###Markdown
Google Scholar Assignment วัตถุประสงค์ :* เพื่อรวบรวมข้อมูลเกี่ยวกับงานวิจัยของมหาวิทยาลัยธรรมศาสตร์* เพื่อฝึกฝนกระบวนการรวบรวมข้อมูล ได้แก่ การค้นหา, การดึงข้อมูล, การเปลี่ยนแปลงและจัดเก็บข้อมูล บทนำ:Google Scholar เป็นบริการที่ช่วยผู้ใช้ค้นหาและรับข้อมูลเอกสารงานวิจัยสิทธิบัตรและทรัพย์สินทางปัญญาประเภทอื่นๆ URL ที่นำมาใช้ในงาน:* Affiliation Query - https://scholar.google.com/citations?view_op=view_org&hl=en&org=10241031385301082500 * User Query - https://scholar.google.com/citations?hl=en&user=N5APA98AAAAJ ผลที่คาดหวัง:ตารางไฟล์ CSV ประกอบไปด้วย 2 ตาราง* ตาราง “Author” ประกอบด้วยนักวิจัยทั้งหมดในมหาวิทยาลัยธรรมศาสตร์* ตาราง “Paper” ประกอบด้วยเอกสารทั้งหมดของมหาวิทยาลัยธรรมศาสตร์ตัวอย่างตาราง ศึกษาโครงสร้างข้อมูลของผู้แต่งและเอกสารจาก Google Scholar tree data structure ของ Author Table tree data structure ของ Paper Table รวบรวมข้อมูล ดึงข้อมูลและสร้างตาราง Author1. เรียกใช้ SeleniumLibrary ซึ้งเป็นไลบรารีการทดสอบเว็บสำหรับ Robot Framework เพื่อเข้าหน้า web siteเมื่อเรียกคีย์เวิร์ด Open BrowserหรือCreate WebDriver จะสร้างอินสแตนซ์ Selenium WebDriver ใหม่โดยใช้ Selenium WebDriver API ในเงื่อนไขของ SeleniumLibrary เบราว์เซอร์ใหม่จะถูกสร้างขึ้น เป็นไปได้ที่จะเริ่มเบราว์เซอร์อิสระหลายตัว (อินสแตนซ์ Selenium Webdriver) พร้อมกันโดยเรียกOpen BrowserหรือCreate WebDriverหลาย ๆ ครั้ง โดยปกติเบราว์เซอร์เหล่านี้จะไม่เชื่อมโยงกันและไม่แชร์ข้อมูลเช่นคุกกี้เซสชันหรือโปรไฟล์ โดยปกติเมื่อเบราว์เซอร์เริ่มทำงานมันจะสร้างหน้าต่างเดียวที่แสดงให้ผู้ใช้เห็น
###Code
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.common.keys import Keys
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
from selenium import webdriver
from webdriver_manager.chrome import ChromeDriverManager
PATH='./chromedriver'
driver = webdriver.Chrome(ChromeDriverManager().install())
#1 open homepage
driver.get("https://scholar.google.com/")
print(driver.title)
#2 enter search box
search_box=driver.find_element_by_css_selector('input#gs_hdr_tsi.gs_in_txt.gs_in_ac')
search_box.send_keys("Thammasat University",Keys.ENTER)
#3 click thammasat university
driver.implicitly_wait(3)
driver.find_element_by_css_selector('div.gs_ob_inst_r')\
.find_element_by_css_selector('a').click()
###Output
_____no_output_____
###Markdown
2. สร้าง dataframe เปล่าเพื่อรอเก็บข้อมูล
###Code
#4 create dataframe
import pandas as pd
df=pd.DataFrame(
{
'user_ID':['N5APA98AAAAJ'],
'name':['Wasit Limprasert'],
'affiliation':['Thammasat university']
}
)
df
###Output
_____no_output_____
###Markdown
3. เก็บข้อมูลจากหน้า web site และนำข้อมูลเข้าตาราง dataframe ที่สร้างไว้
###Code
#5 for every author, get detail and save to df
while 1:
for i in driver.find_elements(By.CSS_SELECTOR,'div.gs_ai_t'):
author=i.find_element_by_css_selector('a')
aff=i.find_element_by_css_selector('div.gs_ai_aff')
print(
author.get_attribute('href').split('=')[-1],
author.text,
aff.text
)
df = df.append(
{
'user_ID': author.get_attribute('href').split('=')[-1],
'name': author.text,
'affiliation': aff.text
}
, ignore_index=True
)
driver.implicitly_wait(3)
x=driver.find_element_by_css_selector('#gsc_authors_bottom_pag > div > button.gs_btnPR.gs_in_ib.gs_btn_half.gs_btn_lsb.gs_btn_srt.gsc_pgn_pnx')
x.click()
df.tail(20)
###Output
_____no_output_____
###Markdown
4. ลบข้อมูลซำ้ออก
###Code
#6 delete column Duplicate
df = df.drop_duplicates(subset="name")
df
###Output
_____no_output_____
###Markdown
5. เก็บข้อมูลเป็นตาราง dataframe และ save ข้อมูลเป็นไฟล์ csv
###Code
#7 store df into csv file
df.to_csv('Authors Table.csv')
###Output
_____no_output_____
###Markdown
______________________________________________________________________________________________ ดึงข้อมูลและสร้างตาราง Paper1. อ่านไฟล์ Authors Table
###Code
#1 read file aurhor csv
author = pd.read_csv("Authors Table.csv")
###Output
_____no_output_____
###Markdown
2. เข้าถึงหน้า web site
###Code
#2 open homepage
PATH='./chromedriver'
driver = webdriver.Chrome(ChromeDriverManager().install())
row = 0
###Output
====== WebDriver manager ======
Current google-chrome version is 90.0.4430
Get LATEST driver version for 90.0.4430
Driver [/Users/nichaaa/.wdm/drivers/chromedriver/mac64/90.0.4430.24/chromedriver] found in cache
###Markdown
3. สร้างตาราง dataframe เปล่าเพื่อใช้เก็บข้อมูล
###Code
#3 Create dataframe
paper = pd.DataFrame(columns=['title','authors','publication_date','description','cite_by'])
###Output
_____no_output_____
###Markdown
4. เก็บข้อมูลจากหน้า web site และนำข้อมูลเข้าตาราง dataframe ที่สร้างไว้
###Code
#4 for every paper, get detail and save to df
import time
for usr_id in author['user_ID']:
driver.get('https://scholar.google.com/citations?hl=en&user='+usr_id)
a=driver.find_elements_by_class_name('gs_btnPD')
while a[0].is_enabled()==True:
driver.find_element_by_class_name('gs_btnPD').click()
time.sleep(5)
link = driver.find_elements_by_class_name('gsc_a_at')
link = [i.get_attribute('data-href') for i in link]
for url in link:
driver.get('https://scholar.google.com/'+url)
try :
table = driver.find_element_by_id('gsc_vcd_table')
b = table.find_elements_by_class_name('gs_scl')
title =''
Au = ''
PD = ''
Des = ''
cited = ''
for i in b:
title = driver.find_elements_by_class_name('gsc_vcd_title_link')[0].text
if i.find_elements_by_class_name('gsc_vcd_field')[0].text == 'Authors':
Au = i.find_elements_by_class_name('gsc_vcd_value')[0].text
elif i.find_elements_by_class_name('gsc_vcd_field')[0].text == 'Publication date':
PD =i.find_elements_by_class_name('gsc_vcd_value')[0].text
elif i.find_elements_by_class_name('gsc_vcd_field')[0].text == 'Description':
Des =i.find_elements_by_class_name('gsc_vcd_value')[0].text
elif i.find_elements_by_class_name('gsc_vcd_field')[0].text == 'Total citations':
cited =i.find_elements_by_css_selector('a')[0].text.replace('Cited by ','')
paper.loc[row]=[title,Au,PD,Des,cited]
row += 1
except :
pass
print('row : ',row)
time.sleep(5)
paper
###Output
_____no_output_____
###Markdown
5. ปรับตาราง dataframe ที่ได้ให้อยู่ในรูป 1NF
###Code
#5 prepare data to 1NF
p_paper = pd.DataFrame(columns=['title','authors','publication_date','description','cite_by'])
rows = 0
for index, row in paper.iterrows():
for token in row['authors'].split(','):
p_paper.loc[rows]=[row['title'],token,row['publication_date'],row['description'],row['cite_by']]
rows +=1
p_paper
###Output
_____no_output_____
###Markdown
6. เก็บข้อมูลเป็นตาราง dataframe และ save ข้อมูลเป็นไฟล์ csv
###Code
#7 store df into csv file
p_paper.to_csv('Paper Table.csv')
###Output
_____no_output_____
###Markdown
______________________________________________________________________________________________ จัดเก็บข้อมูลผลลัพธ์ลงใน Google Sheet1. Author Table https://docs.google.com/spreadsheets/d/1FWIKhQtJ-zHsSLC11Hb4nSul-pfbac9YHvSr3awpNeM/edit?usp=sharing2. Paper Table https://docs.google.com/spreadsheets/d/13Ni68sgyL7kdQNYt6nSRnE4QTcXIkSKK_X8TIUCI4iA/edit?usp=sharing ___ นำข้อมูลไป visualization https://datastudio.google.com/reporting/878faf9a-8fa9-465a-ac8b-1f16ee47d827 ___ รายงานปัญหา
###Code
import requests
url = "https://scholar.google.com/citations?hl=en&user=N5APA98AAAAJ"
r =requests.get(url)
text = r.text
text
from bs4 import BeautifulSoup
soup = BeautifulSoup(r.text, 'html.parser')
titles = []
authors = []
publications_date = []
cite = []
title = soup.find_all('td', {'class':'gsc_a_t'})
for title in title:
title = str(title).split('<td class="gsc_a_t"><a class="gsc_a_at"')
title = title[1]
title = title.split(' href="javascript:void(0)">')
title = title[1]
title = str(title).split('</a>')
title_f = title[0]
titles.append(title_f)
author = title[1]
author = author.split('<div class="gs_gray">')
author = author[1]
author = author.split('</div>')
author = author[0]
authors.append(author)
publication_date = soup.find_all('td', {'gsc_a_y'})
for year in publication_date:
year = str(year).split('<td class="gsc_a_y"><span class="gsc_a_h gsc_a_hc gs_ibl">')
year = year[1]
year = year.split('</span></td>')
year = year[0]
publications_date.append(year)
cite_by = soup.find_all('a', {'class':'gsc_a_ac gs_ibl'})
for cit in cite_by:
cit = str(cit).split('<a class="gsc_a_ac gs_ibl"')
cit =cit[1].split('>')
cit = cit[1].split('</a')
cit = cit[0]
cite.append(cit)
import pandas as pd
df = pd.DataFrame({
'title': titles,
'authors': authors,
'publication_date': publication_date,
'description':'',
'cite_by': cite_by
})
df
df.to_csv('Paper Table.csv')
###Output
_____no_output_____ |
Basic-Tutorials/handson202107-vcp/JupyterNotebook_Introduction.ipynb | ###Markdown
JupyterNotebook入門所要時間:30分JupyterNotebookの基本操作と、NIIクラウド運用チームによるプラグイン拡張の一部について、お話します。 操作対象となるNotebookを準備する 新規作成  Notebook一覧表示画面の右上のプルダウンメニュー(赤丸)から、「Python3」(青丸)を選ぶことで、  新しいNotebookを作成できます。Notebookのタイトル部分(赤丸)をクリックすると、Notebookの名前を変更できます。保存ボタン(青丸)でNotebookをファイルとして保存できます。作成・保存したNotebookは、Notebook一覧表示画面で確認できます。Notebookの名前に拡張子「.ipynb」が付いてファイル保存されるので、文字化けの可能性のある環境では注意してください。 Notebookのインポート・エクスポートPC上にファイルとして持っている.ipynbファイルを、Notebook一覧表示画面のファイルリスト部分にドラッグアンドドロップすることで、Notebookをインポートすることができます。  Notebook一覧表示画面で、エクスポートしたいNotebookのチェックボックスを付け(赤丸)、Notebookが使われている状態の場合は、実行中のセルがないか確認の上、「Shutdown」(青丸)で使用を中止し、  「Download」(赤丸)で、.ipynb形式でエクスポートできます。 基本操作 Pythonのコードを実行する
###Code
import math
for i in range(3) :
print(math.sin(i))
###Output
0.0
0.8414709848078965
0.9092974268256817
###Markdown
セルに実行したいコードを書いて「Shift」+「Enter」で実行できます。実行結果がセルの下に表示されます。下のセルに「math.cos」と入力してから、「Shift」+「Tab」を押してみてください。
###Code
math.cos(29)
###Output
_____no_output_____
###Markdown
Docstringの参照が行えます。コードを書いている途中で調べたい場合に便利でしょう。 なお、「Shift」に続けて 「Tab」を2回押すと、より詳細な内容が表示されます。下のセルに「math.t」まで入力してから「Tab」を押してみてください。
###Code
math.t
###Output
_____no_output_____
###Markdown
コード補完が行えます。 Linuxコマンドを実行する
###Code
!ls
###Output
JupyterNotebook_Introduction.ipynb
###Markdown
「!」に続けてLinuxコマンドを書くと、そのコマンドが実行できます。コマンドの終了コードを反転させる「!」を併せて使いたい場合は、「! ! cmd」と書くことができます。スペースの入れ方に注意してください。 マークダウンでドキュメントを書くセルを選択した状態で、ツールバーのセルのタイプ(赤丸)を操作すると、セルのタイプを切り替えることができます。実行できる「Code」セルから、ドキュメントを書く「Markdown」セルに変更すると、そのセルにGitHubマークダウンの書式でドキュメントが書けるようになります。下の「Code」セルを「Markdown」セルに変更して、実行(「Shift」+「Enter」)してみてください。 > 引用 > 引用>> 多重引用```コードを書く場合は「```」で囲む```水平線を入れる---- リスト1 - リスト1_1 - リスト1_1_1 - リスト1_1_2 - リスト1_2- リスト2- リスト3 マジックコマンドを使う「%」で始まるマジックコマンドを使うことができます。有効なマジックコマンドの一覧は「%lsmagic」で確認できます。
###Code
%lsmagic
###Output
_____no_output_____
###Markdown
例えば、lsコマンドでファイルの一覧を確認することができます。
###Code
%alias
###Output
Total number of aliases: 12
###Markdown
個々のコマンドについては、セルに入力してから「Shift」+「Tab」で調べることができます。(Tabを2回続けると詳細情報) 目次の表示/非表示ツールバーの目次ボタン(赤丸)を操作すると、目次の表示状態を切り替えることができます。目次が表示されていない方がセルの表示領域が広くなりますが、目次の見出しをクリックすることでジャンプすることができるので、適時、切り替えながら作業すると良いでしょう。 NII拡張について NIIクラウド運用チームでは、JupyterNotebookを使った情報システムの構築・運用手法「Literate Computing for Reproducible Infrastructure(再構築可能なインフラのための文芸的コンピューティング、LC4RIと省略)」を提唱・実践しています。LC4RIでは、そのユースケースに合わせてJupyterNotebookを拡張して使っており、オンデマンド構築サービスで用いるJupyterNotebookにも採用されています。ここでは、NII拡張について、本日のハンズオンを行う上で知っておいた方が良いものを紹介します。 凍結システム運用では同じ操作を複数回実施してはいけない場合が多くあります。そこで、実行したセルを誤って再実行しないように「凍結」するプラグインを開発し、使用しています。凍結されたセルを再実行したい場合には、そのセルを選択し、凍結解除を行うボタン(赤丸)を操作して、凍結を解除した後、実行が可能となります。 色による実行状態の表示実行セルの左側に、セルの実行状態に応じて、色を付けています。- 青:実行中- 緑:実行成功- 赤:実行失敗色は、Notebookをリロードしたり、一度Notebookを閉じて立ち上げなおすと消えます。 畳み込み表示とまとめ実行 見出しの左側にある△を操作することで表示を畳み込むことができます。畳み込み表示部の実行ボタン(赤丸)を押すことで、畳み込まれた範囲に含まれる実行セルを順番にまとめて実行することができます。畳み込み表示部の実行ボタンの下に並んだ□が、個々の実行セルを表しており、色や凍結マークで実行状態を表示するようになっています。 実行結果の保存コードセルの過去の実行結果をピン止めして複数、タブ状に保存しておくことができます。通常の実行結果は、再実行により、上書きされて消えてしまいますが、この仕組みによって、過去の実行結果と今の実行結果を比較できます。「実行したら、こんな結果になるハズだよ」と、お手本として使うことができます。複数残すこともできるので、成功だけでなく、エラーの結果を得られた時にも残しておいて、「こうなったら成功だけど、こうなったら失敗しているよ。失敗の場合は……」と、マークダウンの説明と組み合わせて使うと効果的です。- 保存:「ピン」アイコンをクリックする度に、タブ状に保存されます- 比較:比較したいタブを選択して「互い違いの2本の矢印」アイコンをクリックすると、2つの結果のdiffが表示されます
###Code
!date
###Output
_____no_output_____
###Markdown
実行結果は Cell > Current Outputs > Clear もしくは Cell > All Output > Clear で消すことができます。 ただし、ピン止めしている実行結果は消えません。ピン止めしている実行結果は、タブごとに × を押して消してください。 また、フリーズしている場合は実行結果を消すことはできません。一度フリーズを解除してから消すようにしてください。 処理が実行される仕組み カーネル[How IPython and Jupyter Notebook work](https://jupyter.readthedocs.io/en/latest/architecture/how_jupyter_ipython_work.html)ユーザがブラウザを操作してJupyterNotebook(上の図ではNotebookServer)上でNotebookを開くと、セル内に書かれた処理を実行するための「カーネル」が生成され、ブラウザからセルの実行を指示すると、処理は全てカーネルに送られて実行され、その結果がブラウザに戻されます。カーネルは、標準として用意されているIPythonの他にも、[さまざまな環境](https://github.com/jupyter/jupyter/wiki/Jupyter-kernels)を使うことができます。IPythonのカーネルは、Pythonの対話型環境ですので、生成されて以降に実行された変数や関数の定義を蓄積・更新していきます。「実行された」順で積み重なっていくので、Notebook上に書かれたセルの順序とは関係ありません。但し、その2つの順序が一致することも多いでしょう。JupyterNotebookは、1つのNotebookに対しては、1つのカーネルしか生成しません。別々のPCのブラウザ上で同じNotebookを開いたとしても、全て同じカーネルを共有することになります。異なるNotebookに対しては、異なるカーネルが生成されるので、Notebookをまたがって変数を共有することはできません。Notebookをまたがって変数を共有するためには、ファイル経由などの方法を考えなければなりません。 カーネルに関わる操作Notebookを開くと、対応するカーネルが存在しない場合、そのNotebookに設定された種類のカーネルを自動的に生成し、セルの実行が可能な状態になりますが、明示的にカーネルを操作することもできます。  Interruptいわゆる「Ctrl」+「C」です。時間のかかる処理を中断したい時などに、カーネルに対して、現在の処理実行を中断するようにリクエストします。 Restart現在使用中のカーネルを破棄し、新しいカーネルを生成します。使っていたカーネルが保持していた変数や関数の定義は失われます。さまざまな操作によって、カーネル内の状態が把握できなくなって、イチからやり直したい場合に、使うことができます。Restart後に、続けて、セルに出力を全て消したり、先頭から全てのセルを実行(凍結済みのセルはスキップされることに注意)する操作も用意されています。 Reconnect何らかの理由で、NotebookServerとカーネルの接続がおかしくなった場合に、明示的に再接続を指示できます。Reconnectで正常な状態に復帰できなかった場合は、Restartを使うことになるでしょう。 Shutdown現在使用中のカーネルを停止します。Notebookのエクスポート手順にあったShutdownも同じ意味の操作です。使い終わったNotebookを再利用する可能性がある場合、大量のNotebookがあって大量のカーネルが生成される可能性がある場合は、使い終わったNotebookをShutdownする運用が良いでしょう。 Notebook一覧表示画面のRunningタブ(赤丸)で、サーバ上で実行中のプロセス一覧が表示できます。ここで、ShutdownしたいプロセスのShutdownボタン(青丸)を押して、Shutdownすることもできます。 ターミナルを使う(非推奨) 先に見たように、Notebookを使って作業を行うことで、セルでLinuxコマンドを実行でき、その結果を残しておけるので、ターミナルによるCLI操作を必要とすることは基本的にありません。むしろ、ターミナルでは、ドキュメントとして実行結果が残らないので、Notebookで作業する方が良いでしょう。但し、パスワードなど、ドキュメントとして残ると困るものを扱うなど、例外的にターミナルが使いたい場合もあります。JupyterNotebookには、直接、ターミナルを使う機能もあります。Notebook一覧表示画面を操作して、「New」-「Terminal」(赤丸)から、ターミナルを開くことができます。 ターミナルは、サーバ上でJupyterNotebookを実行しているユーザのシェルとして開きます。使い終わったら、exitで停止するか、先に紹介したNotebook一覧表示画面のRunningタブから停止させることができます。 その他 ファイルの移動やコピーファイル一覧の画面でファイルを選択し、まとめてコピーや移動ができます。 ただし、Runningの状態のNotebookを選択すると移動はできません。(Moveのボタンが表示されなくなります)。その場合は該当するNotebookをShutdownしてください。 ショートカットHelpよりショートカットの一覧を確認できます(Keyboard Shortcuts)。また、自分用にショートカットをカスタマイズすることも可能です(Edit Keyboard Shortcuts)。 セルの状態には、入力モード(セルの左端のバーが緑色)とコマンドモード(セルの左端のバーが青色)があります。入力モード、コマンドモードのそれぞれで使用できるショートカットが異なるので注意してください。 ツールバー 1. Notebook の保存。File > Save and Checkpoint と同じ。File > Revert to Checkpoint で直前に保存した状態に戻せます。1. 現在のセルの下にセルを追加。コマンドモードで b を押す、Insert > Insert Cell Below と同じです。1. セルの カット(削除)。ただしフリーズした状態では削除できません。1. セルの コピー。1. セルのペースト。1. フォーカスがあたっているセルの上下移動。コマンドモードで 矢印の上下キーでも移動できます。1. セルを実行して、次のセルのフィーカスを移す。Shift + Enter と同じです。1. 実行中の処理を中断します。Kernel > Interrupt と同じです。1. カーネルを再起動します。Kernel > Restart と同じです。1. カーネルを再起動し、セルをすべて実行します。 Kernel > Restart & Run All と同じです。1. セルの種類を選択します。 - Code コードを実行できます - Markdown Markdown形式で文章を入力できます - Raw NBConvert 入力したものがそのまま出力されます1. ショートカットの一覧を表示します。Help > Keyboard Shortcuts でも確認できます1. 選択中のセルを編集禁止にしたり、解除したりします。編集禁止にするとセルがクリーム色に色が付き、編集・削除ができなくなります。編集禁止でも実行はできます。1. 選択中のセルをフリーズさせます。編集・削除・実行ができなくなります。1. 選択中のセルのフリーズを解除します。1. 選択中のセル以降、そのセルがあるセクションの終わりまでをフリーズ解除します。1. 選択中のセル以降、Notebookの最後までのセルをすべてフリーズ解除します。1. 目次の表示/非表示を切り替えます。1. セルの実行結果をピン止めします。1. セルの実行結果のピン止めが複数ある場合に、最も新しいものを1つだけ残して残りを削除します。 Markdownよく使うMarkdownの記法の例を紹介します。$$ で囲うと mathjax で数式を記述することもできます。以下のセルは Raw NBConvert 形式になっていますが、Markdown に変更して実行すると、結果を参照できます。
###Code
# 見出し1
## 見出し2
### 見出し3
#### 見出し4
##### 見出し5
普通の文章。改行させたい場合には、行末にスペースを2つ入れてください。
この行のうしろにはスペースが2つはいっており改行されます。
この行の後ろは改行されません。
最後の行
*強調* _強調_ は、イタリックで表示されます。
**強調** __強調__ は、太字で表示されます。
`バッククウォートはコードの表記です`
```
複数行にわたる場合には、
バッククウォートを3つつなげて囲ってください
```
> 引用
>> 引用の中の引用
順序なしリストは - もしくは * とスペースを行頭に
- 順序なしリストのアイテム 1
- サブアイテム。タブでインデント
* 順序なしリストのアイテム 2
順序付きリストは、数字 + ピリオド + スペース を行頭に。
1. 順序ありリストのアイテム
1. 順序ありリストのサブアイテム
1. 順序ありリストのサブアイテム
1. 順序ありリストのアイテム 2
リンクの書き方
[https://cloud.gakunin.jp/ocs/]
[タイトルつきリンク](https://cloud.gakunin.jp/ocs/)
水平線は ハイフンやアスタリスクを3つ以上並べる。間にスペースが入ってもよい。
---
* * *
ダラーを2つで囲うと mathjax の数式が書けます。ただし、セルを分けてください。
$$
|\boldsymbol{a}| = \sqrt{a_0^2+a_1^2}
$$
###Output
_____no_output_____ |
FEEG6016 Simulation and Modelling/07-Finite-Elements-Lab-1.ipynb | ###Markdown
Finite Elements Lab 1 Worksheet
###Code
from IPython.core.display import HTML
css_file = 'https://raw.githubusercontent.com/ngcm/training-public/master/ipython_notebook_styles/ngcmstyle.css'
HTML(url=css_file)
###Output
_____no_output_____
###Markdown
1d problem The temperature $T$ of a bar of length $1$ at equilibrium satisfies$$ \partial_{xx} T + f(x) = 0.$$The heat source is given by $f(x)$. We'll hold the temperature fixed at the right edge by setting $T(1) = 0$. We'll allow heat to conduct through the left edge by setting $\partial_x T(0) = 0$. Dealing with the second derivative is a problem, so we want to remove some derivatives from the formulation. Introducing a *weighting* function $w(x)$. Multiply the equation by $w$ and integrate by parts to get$$ \left[ \partial_x T(x) w(x) \right]_0^1 - \int_0^1 \text{d}x \, \partial_x T(x) \partial_x w(x) = - \int_0^1 \text{d}x \, f(x) w(x).$$Putting in the boundary conditions gives$$ \int_0^1 \text{d}x \, \partial_x T(x) \partial_x w(x) = \int_0^1 \text{d}x \, w(x) f(x).$$This is the **weak form**. It can be proved that solutions of the *strong* form (with two derivatives) are also solutions of the weak form, and vice versa. Function representation To get an approximate solution we split the domain into intervals, or elements. To start we'll choose $[0, 1/2]$ and $[1/2, 1]$, which means there are nodes at $\{ 0, 1/2, 1 \}$. We want to represent all unknown functions ($T, w$) on these elements. We'll introduce some *shape* or *basis* functions $N_A(x)$ and write, for example, $$ T(x) = \sum_A T_A N_A(x).$$Here $A$ is a counter associated with the nodes, so here $A = \{ 0, 1, 2 \}$: the nodes are labelled $x_A$. The shape function $N_A(x)$ is $1$ at the associated node, and $0$ at all other nodes. If we choose the shape functions to be piecewise linear then the three shape functions therefore look like$$\begin{align} N_0(x) &= \begin{cases} 1 - 2 x, & 0 \le x \le 1/2 \\ 0, & 1/2 \le x \le 1, \end{cases}, \\ N_1(x) &= \begin{cases} 2 x, & 0 \le x \le 1/2 \\ 2 - 2 x, & 1/2 \le x \le 1, \end{cases}, \\ N_2(x) &= \begin{cases} 0, & 0 \le x \le 1/2 \\ 2 x - 1, & 1/2 \le x \le 1. \end{cases}\end{align}$$
###Code
%matplotlib inline
import numpy
from matplotlib import pyplot
from matplotlib import rcParams
rcParams['font.family'] = 'serif'
rcParams['font.size'] = 16
rcParams['figure.figsize'] = (12,6)
x = numpy.linspace(0, 1, 1000)
N0 = numpy.where(x <= 0.5, 1-2*x, numpy.zeros_like(x))
N1 = numpy.where(x <= 0.5, 2*x, 2-2*x)
N2 = numpy.where(x <= 0.5, numpy.zeros_like(x), 2*x-1)
pyplot.figure()
pyplot.plot(x, N0, label=r"$N_0$")
pyplot.plot(x, N1, label=r"$N_1$")
pyplot.plot(x, N2, label=r"$N_2$")
pyplot.xlabel(r"$x$")
pyplot.ylim(-0.1, 1.1)
pyplot.legend();
###Output
_____no_output_____
###Markdown
With this definition of the shape functions, the coefficients are given exactly as (e.g.) $T_A = T(x_A)$. **NOTE**: because we have imposed the boundary condition $T(1) = 0$ we must have that $T_2 \equiv 0$, so we do not need to calculate $T_2$.We can now write out the weak form of the problem as$$ \sum_A \sum_B T_A w_B \int_0^1 \text{d}x \, \partial_x N_A(x) \partial_x N_B(x) = \sum_B w_B \int_0^1 \text{d}x \, N_A(x) f(x).$$This has to be true for *any* choice of weight function, so for *any* coefficients $w_B$. This is true only if$$ \sum_A T_A \int_0^1 \text{d}x \, \partial_x N_A(x) \partial_x N_B(x) = \int_0^1 \text{d}x \, N_A(x) f(x).$$This can be written as a matrix problem:$$ K {\bf T} = {\bf F}.$$The coefficients of the **stiffness matrix** $K$ are given by$$ K_{AB} = \int_0^1 \text{d}x \, \partial_x N_A(x) \partial_x N_B(x).$$The coefficients of the **force vector** $F$ are given by$$ F_{A} = \int_0^1 \text{d}x \, N_A(x) f(x).$$ Tasks 1. Compute (analytically) the coefficients of the stiffness matrix given the shape functions above.2. Compute (analytically) the coefficients of the force vector when $f(x) = x$.3. Solve (numerically) for $T_A$ and plot the resulting solution for $T(x)$. Compare against the exact solution $T(x) = (1 - x^3) / 6)$. The element viewpoint This works surprisingly well, but it's clearly going to get very complex when the number of elements (or nodes) gets large as we try to improve accuracy. To work systematically with large numbers of elements, we need to consider one at a time.First, note that in any element there's only two shape functions that are not zero: those that correspond to the nodes at the edge of the element. We'll call these shape functions $N_1$ and $N_2$.Second, note that by doing a coordinate transformation, we can take *any* element from the interval $[ x_A, x_{A+1} ]$ to the interval $[ \xi_1, \xi_2 ]$ where we fix (by convention) $\xi_1 = -1$ and $\xi_2 = 1$. We have$$\begin{align} \xi(x) &= \frac{2 x - x_A - x_{A+1}}{x_{A+1} - x_{A}}, \\ x(\xi) &= \frac{(x_{A+1} - x_{A}) \xi + x_A + x_{A+1}}{2}.\end{align}$$We can now write the shape functions in terms of the *reference coordinates* $\xi$ as$$ N_a(\xi) = \tfrac{1}{2} \left( 1 + \xi_a \xi \right), \qquad a = 1, 2.$$As above we needed the derivatives of the shape function when computing coefficients, we note that$$ \partial_{\xi} N_a = \frac{(-1)^a}{2}, \qquad a = 1, 2.$$We will also need to change coefficients of vectors which will require a Jacobian, which needs derivatives of the coordinate transformation. This needs$$\begin{align} \partial_x \xi &= \frac{2}{x_{A+1} - x_{A}}, \\ \partial_{\xi} x &= \frac{(x_{A+1} - x_{A})}{2}.\end{align}$$ With this information we can compute the stiffness matrix $k^e_{ab}$ for the single element $e$. This gives$$\begin{align} k^e_{ab} &= \int_{x_A}^{x_{A+1}} \text{d}x \, \partial_x N_a \partial_x N_b \\ &= \int_{-1}^{+1} \text{d}\xi \, \partial_{\xi} x \, \partial_{x} N_a \partial_{x} N_b \\ &= \int_{-1}^{+1} \text{d}\xi \, \left(\partial_{\xi} x\right)^{-1} \, \partial_{\xi} N_a \partial_{\xi} N_b \\ &= \frac{(-1)^{a+b}}{x_{A+1} - x_A}.\end{align}$$This is incredibly useful: there's no need to do any integrals at all. The element force vector is given by$$\begin{align} f^e_{a} &= \int_{x_A}^{x_{A+1}} \text{d}x \, N_a f(x) + \text{boundary terms} & = \int_{x_A}^{x_{A+1}} \text{d}x \, N_a f(x).\end{align}$$This is specific to our choice of boundary conditions: normally there would be extra terms.To evaluate this it's convenient to write $f(x) = \sum_a f_a N_a$ within the element, where $f_a = f(x(\xi_a))$. With the simple shape functions we have here, we get$$\begin{equation} f^e_{a} = \frac{x_{A+1} - x_{A}}{6} \begin{pmatrix} 2 f_1 + f_2 \\ f_1 + 2 f_2 \end{pmatrix}.\end{equation}$$ Linking elements to equations In the end we want to construct a linear (matrix) equation to give us the solution $T_A$ at all nodes $A$ where it isn't enforced by the boundary conditions (which in the problem above is all nodes except the right-hand boundary). We note that each *interior* node is linked to two elements, so contributions from the element matrix may affect more than one equation. To keep track of this, we construct the **location matrix** or **location array** $LM$ which, given the node number $a = 1, 2$ and the element number $e$ returns the associated equation number.In our case we have$$ A = LM(a, e) = \begin{cases} e & a = 1 \\ e+1 & a = 2 \end{cases}$$for $e = 0, 1, \dots, N_{\text{elements}}-1$. For the final element we have to change things, as the final node is not to be included. Here we'll set the equation number $A$ to be $-1$.
###Code
Nelements = 4
LM = numpy.zeros((2, Nelements))
for e in range(Nelements):
LM[0, e] = e
LM[1, e] = e+1
LM[1, -1] = -1 # Right hand node of final element is not considered thanks to BC.
###Output
_____no_output_____ |
Jupyter Notebook/ETL Pipeline Preparation.ipynb | ###Markdown
ETL Pipeline PreparationFollow the instructions below to help you create your ETL pipeline. 1. Import libraries and load datasets.- Import Python libraries- Load `messages.csv` into a dataframe and inspect the first few lines.- Load `categories.csv` into a dataframe and inspect the first few lines.
###Code
# import libraries
import pandas as pd
import sqlalchemy as db
# load messages dataset
messages = pd.read_csv('messages.csv')
# Set index to id column for convieneince of concat and merge across dataframe
messages.set_index('id',inplace=True)
messages.head()
# load categories dataset
categories = pd.read_csv('categories.csv')
# Set index to id column for convieneince of concat and merge across dataframe
categories.set_index('id',inplace=True)
categories.head()
###Output
_____no_output_____
###Markdown
2. Merge datasets.- Merge the messages and categories datasets using the common id- Assign this combined dataset to `df`, which will be cleaned in the following steps
###Code
# merge datasets
df = pd.merge(messages, categories, on='id')
df.head()
###Output
_____no_output_____
###Markdown
3. Split `categories` into separate category columns.- Split the values in the `categories` column on the `;` character so that each value becomes a separate column. You'll find [this method](https://pandas.pydata.org/pandas-docs/version/0.23/generated/pandas.Series.str.split.html) very helpful! Make sure to set `expand=True`.- Use the first row of categories dataframe to create column names for the categories data.- Rename columns of `categories` with new column names.
###Code
# create a dataframe of the 36 individual category columns
categories = categories['categories'].str.split(';',expand=True)
categories.head()
# select the first row of the categories dataframe
row = categories.iloc[0]
# use this row to extract a list of new column names for categories.
# one way is to apply a lambda function that takes everything
# up to the second to last character of each string with slicing
category_colnames = row.apply(lambda a : a[:-2])
print(category_colnames)
# rename the columns of `categories`
categories.columns = category_colnames
categories.head()
###Output
_____no_output_____
###Markdown
4. Convert category values to just numbers 0 or 1.- Iterate through the category columns in df to keep only the last character of each string (the 1 or 0). For example, `related-0` becomes `0`, `related-1` becomes `1`. Convert the string to a numeric value.- You can perform [normal string actions on Pandas Series](https://pandas.pydata.org/pandas-docs/stable/text.htmlindexing-with-str), like indexing, by including `.str` after the Series. You may need to first convert the Series to be of type string, which you can do with `astype(str)`.
###Code
for column in categories:
# set each value to be the last character of the string
categories[column] = categories[column].str.extract(r'([0-9])')
# convert column from string to numeric
categories[column] = categories[column].astype(int)
categories.head()
###Output
_____no_output_____
###Markdown
5. Replace `categories` column in `df` with new category columns.- Drop the categories column from the df dataframe since it is no longer needed.- Concatenate df and categories data frames.
###Code
# drop the original categories column from `df`
df = df.drop(columns=['categories'])
df.head()
# concatenate the original dataframe with the new `categories` dataframe
df=pd.merge(df,categories,on='id',how='outer')
###Output
_____no_output_____
###Markdown
6. Remove duplicates.- Check how many duplicates are in this dataset.- Drop the duplicates.- Confirm duplicates were removed.
###Code
# check number of duplicates
df[df.duplicated()==True].shape[0]
# drop duplicates
df.drop_duplicates(keep='first', inplace=True)
# check number of duplicates
df[df.duplicated()].shape[0]
###Output
_____no_output_____
###Markdown
7. Save the clean dataset into an sqlite database.You can do this with pandas [`to_sql` method](https://pandas.pydata.org/pandas-docs/stable/generated/pandas.DataFrame.to_sql.html) combined with the SQLAlchemy library. Remember to import SQLAlchemy's `create_engine` in the first cell of this notebook to use it below.
###Code
engine = db.create_engine('sqlite:///CategorisedMessages.db')
df.to_sql('CategorisedMessages', engine, index=False)
###Output
_____no_output_____
###Markdown
8. Use this notebook to complete `etl_pipeline.py`Use the template file attached in the Resources folder to write a script that runs the steps above to create a database based on new datasets specified by the user. Alternatively, you can complete `etl_pipeline.py` in the classroom on the `Project Workspace IDE` coming later.
###Code
import sys
import pandas as pd
import sqlalchemy as db
def load_data(messages_filepath, categories_filepath):
# Load message file
# Set index to id column for convieneince of concat and merge across dataframe
messages = pd.read_csv(messages_filepath)
messages.set_index('id',inplace=True)
# Load categories file
categories = pd.read_csv(categories_filepath)
# Set index to id column for convieneince of concat and merge across dataframe
categories.set_index('id',inplace=True)
# create a dataframe of the 36 individual category columns
categories = categories['categories'].str.split(';',expand=True)
for column in categories:
# set each value to be the last character of the string
categories[column] = categories[column].str.extract(r'([0-9])')
# convert column from string to numeric
categories[column] = categories[column].astype(int)
df = pd.merge(messages, categories, on='id')
return df
def clean_data(df):
return df.drop_duplicates(keep='first')
def save_data(df, database_filename):
if database_filename.find('.db')>=0:
engine = db.create_engine('sqlite:///'+database_filename)
df.to_sql('CategorisedMessages', engine, index=False)
else:
print('Invalid database filename (need name.db) : '+database_filename)
def main():
if len(sys.argv) == 4:
messages_filepath, categories_filepath, database_filepath = sys.argv[1:]
print('Loading data...\n MESSAGES: {}\n CATEGORIES: {}'
.format(messages_filepath, categories_filepath))
df = load_data(messages_filepath, categories_filepath)
print('Cleaning data...')
df = clean_data(df)
print('Saving data...\n DATABASE: {}'.format(database_filepath))
save_data(df, database_filepath)
print('Cleaned data saved to database!')
else:
print('Please provide the filepaths of the messages and categories '\
'datasets as the first and second argument respectively, as '\
'well as the filepath of the database to save the cleaned data '\
'to as the third argument. \n\nExample: python process_data.py '\
'disaster_messages.csv disaster_categories.csv '\
'DisasterResponse.db')
if __name__ == '__main__':
main()
###Output
Loading data...
MESSAGES: messages.csv
CATEGORIES: categories.csv
Cleaning data...
Saving data...
DATABASE: CategorisedMessages.db
Cleaned data saved to database!
|
March/Week9/60.ipynb | ###Markdown
Product of Array Except Self[原题](https://mp.weixin.qq.com/s/YVOFraWQLvz8cPRHIkwOqg) QuestionYou are given an array of integers. Return an array of the same size where the element at each index is the product of all the elements in the original array except for the element at that index.```textInput: [1, 2, 3, 4, 5]Output: [120, 60, 40, 30, 24]```**Note:** *You cannot use division in this porblem.*
###Code
def products(nums):
''' Main Algorithm '''
# Production begins from head.
prefix = []
for elem in nums:
if prefix != []:
prefix.append(prefix[-1] * elem)
else:
prefix.append(elem)
# Production begins from tail.
suffix = []
for elem in nums[-1::-1]:
if suffix != []:
suffix.append(suffix[-1] * elem)
else:
suffix.append(elem)
suffix = suffix[-1::-1]
# Generate result.
result = []
for i in range(len(nums)):
if i == 0:
result.append(suffix[i + 1])
elif i == len(nums) - 1:
result.append(prefix[i - 1])
else:
result.append(prefix[i - 1] * suffix[i + 1])
return result
''' Test Scripts '''
print(products([1, 2, 3, 4, 5]))
###Output
[120, 60, 40, 30, 24]
|
week-2/3-data-visualization/4. Fancy plots/B. Fibonaccci Sequence.ipynb | ###Markdown
La sucesión de Fibonaccci *Por: Martin Vuelta ([zodiacfireworks](https://github.com/zodiacfireworks))* *Email:* `[email protected]` 1. Introducción Los números de Fibonacci son aquellos pertenecientes a la secuencia de números $\{F_n\}_{n=1}^{\infty}$ definida por la relación lineal de recurrencia:$$F_n = F_{n-1} + F_{n-2}$$con $F_{1} = F_{2} = 1$. Como resultado de dicha definicion, comúnmente se asigna el valor $0$ al termino $F_{0}$ 2. Implementación en Python 2.1. Algoritmo Recursivo
###Code
def RecursiveFibonacci(n):
if n < 2:
return n
return RecursiveFibonacci(n-1) + RecursiveFibonacci(n-2)
for n in range(0, 11):
print("Término {0:>2d} -> {1:}".format(n, RecursiveFibonacci(n)))
%timeit -n 10 RecursiveFibonacci(30)
###Output
_____no_output_____
###Markdown
2.2. Algoritmo Iterativo
###Code
def IterativeFibonacci(n):
fib = [0, 1]
if n < 2:
return fib[n]
for i in range(1, n):
fib = [fib[1], fib[0] + fib[1]]
return fib[1]
for n in range(0, 11):
print("Término {0:>2d} -> {1:}".format(n, IterativeFibonacci(n)))
%timeit -n 10 IterativeFibonacci(30)
###Output
_____no_output_____
###Markdown
2.3. Algoritmo Divide y vencerás
###Code
def DCFibonacci(n):
fib = [0, 1]
if n < 2:
return fib[n]
i = n - 1
b, a = c, d = fib
while i > 0:
if i % 2 != 0:
a, b = d * b + c * a, d * (b + a) + c * b
c, d = c * c + d * d, d * (2 * c + d)
i = i//2
return a + b
for n in range(0, 11):
print("Término {0:>2d} -> {1:}".format(n, DCFibonacci(n)))
%timeit -n 10 DCFibonacci(30)
###Output
_____no_output_____
###Markdown
2.4. Memoization Dado que en las siguientes secciones de este trabajo calcularemos a menudo los términos de la serie de Fibonacci, es conveniente emplear una tecnica que nos permita almacenar los valores ya calculados y consultarlos antes de calcular nuevamente. Para ello haremos uso de la tecnica de optimizacion Memoization mediante el siguiente decorador.
###Code
class Memoize:
def __init__(self, f):
self.f = f
self.memo = {}
def __call__(self, *args):
if args not in self.memo.keys():
self.memo[args] = self.f(*args)
return self.memo[args]
###Output
_____no_output_____
###Markdown
2.4.1. Memoization del algoritmo recursivo
###Code
MRFibonacci = Memoize(RecursiveFibonacci)
%timeit -n 10 MRFibonacci(30)
###Output
_____no_output_____
###Markdown
2.4.2. Memoization del algoritmo iterativo
###Code
MIFibonacci = Memoize(IterativeFibonacci)
%timeit -n 10 MIFibonacci(30)
###Output
_____no_output_____
###Markdown
2.4.3. Memoization del algoritmo D&C
###Code
MDCFibonacci = Memoize(DCFibonacci)
%timeit -n 10 MDCFibonacci(30)
###Output
_____no_output_____
###Markdown
2.5. Implementación final En vista de los tiempos de ejecución anteriores finalmente elegimos la implementacion con el algoritmo D&C optimizada.
###Code
Fibonacci = Memoize(DCFibonacci)
###Output
_____no_output_____
###Markdown
3. Tabulación de la Sucesión de Fibonacci Como aplicación de la implementación realizada vamos a tabular algunos de los valores de la sucesión de Fibonacci.
###Code
from IPython.display import HTML
from IPython.display import display_html
from uuid import uuid4
table_template = """<style>
#fibonacci_{uuid:} {{width: 250px;border: 0 none;}}
#fibonacci_{uuid:} * {{border: 0 none;text-align: center;}}
#fibonacci_{uuid:} caption {{margin: 0 -5rem 0.5rem;border: 0 none;}}
#fibonacci_{uuid:} tr:first-child, #fibonacci_{uuid:} tr:last-child {{border-bottom: 1px solid black;}}
#fibonacci_{uuid:} tr:first-child {{color: #fafafa;background-color: #212121;}}
#fibonacci_{uuid:} tr:nth-child(2n) {{background-color: #dedede;}}
#fibonacci_{uuid:} tr td:first-child, #fibonacci_{uuid:} tr th:first-child {{width: 50px;border-right: 1px solid #212121;}}
</style><center><table id="fibonacci_{uuid:}">
<caption>Primeros 30 términos de la Sucesión de Fibonacci</caption>
<tr><th>$n$</th><th nowrap="nowrap">$Fibonacci[n]$</th></tr>
{0:}</table></center>
"""
table_content = "".join(
["<tr><td>${n:}$</td><td>${fn:}$</td></tr>".format(
n=n,
fn=Fibonacci(n)
) for n in range(1,31)]
)
table = table_template.format(table_content, uuid=uuid4().hex)
display_html(HTML(table))
###Output
_____no_output_____
###Markdown
3.1. Representación Binaria La representación binaria de los números de Fibonacci la obtenemos de la siguiente forma.
###Code
def BinaryFibonacci(n):
return '{0:b}'.format(Fibonacci(n))
from IPython.display import HTML
from IPython.display import display_html
from uuid import uuid4
table_template = """<style>
#fibonacci_{uuid:} {{width: 250px;border: 0 none;}}
#fibonacci_{uuid:} * {{border: 0 none;text-align: center;}}
#fibonacci_{uuid:} caption {{margin: 0 -5rem 0.5rem;border: 0 none;}}
#fibonacci_{uuid:} tr:first-child, #fibonacci_{uuid:} tr:last-child {{border-bottom: 1px solid black;}}
#fibonacci_{uuid:} tr:first-child {{color: #fafafa;background-color: #212121;}}
#fibonacci_{uuid:} tr:nth-child(2n) {{background-color: #dedede;}}
#fibonacci_{uuid:} tr td:first-child, #fibonacci_{uuid:} tr th:first-child {{width: 50px;border-right: 1px solid #212121;}}
</style><center><table id="fibonacci_{uuid:}">
<caption>Primeros 30 términos de la Sucesión de Fibonacci en forma binaria</caption>
<tr><th>$n$</th><th nowrap="nowrap">$Fibonacci[n]$</th></tr>
{0:}</table></center>
"""
table_content = "".join(
["<tr><td>${n:}$</td><td>${fn:}$</td></tr>".format(
n=n,
fn=BinaryFibonacci(n)
) for n in range(1,31)]
)
table = table_template.format(table_content, uuid=uuid4().hex)
display_html(HTML(table))
###Output
_____no_output_____
###Markdown
4. Expresión general y extensión a los reales 4.1. Expresión general La ecuación de recurrencia que define a la suceción de Fibonacci es de la forma$$ x_n = A\,x_{n-1} + B\,x_{n-2}\,;\,n \geq 3 $$entonces la forma cerrada de $F_n$ esta dada por$$ F_n=\frac{\alpha^n-\beta^n}{\alpha-\beta}, $$donde $\alpha$ y $\beta$ son las raices de la ecuación $x^2 = A\,x+B$. Para la secuencia de Fibonacci $A=B=1$, entonces la ecuación anterior convierte en$$ x^2 - x - 1 = 0 $$cuyas raices son$$ x=\frac{1}{2}\left(1\pm\sqrt{5}\right). $$Por tanto la forma cerrada de $F_{n}$ es$$ F_n=\frac{\left(1+\sqrt{5}\right)^n - \left(1-\sqrt{5}\right)^n}{2^{n}\sqrt{5}}. $$Otras representaciones simbólicas de la expresión general de la sucesión de Fibonacci se pueden obtener manipulando la expresion anterior teniendo en cuenta que$$ \phi=\frac{1}{2}\left(1+\sqrt{5}\right). $$es el número áureo. Reemplazando en la expresión de $F_n$ obtenida anteriormente obtenemos $F_n$ en funcion de $\phi$ como$$ F_n=\frac{\phi^n - \left(-\phi\right)^{-n}}{\sqrt{5}}. $$ 4.2. Cálculo por redondeo En la expresión obtenida anteriromente, podemos observar que$$ \left|\frac{\left(-\phi\right)^{-n}}{\sqrt{5}}\right| < \frac{1}{2} \,;\, \forall n \geq 0, $$entonces podemos expresar $F_n$ mediante la funcion de redondeo $\left[x\right]$$$ F_n = \left[\frac{\phi^n}{\sqrt{5}}\right] $$
###Code
from math import *
def RoundFibonacci(n):
phi = 0.5 * (1 + sqrt(5))
return round((phi**n) / sqrt(5))
for n in range(0, 11):
print("Término {0:>2d} -> {1:}".format(n, RoundFibonacci(n)))
%timeit -n 10 RoundFibonacci(30)
from IPython.display import HTML
from IPython.display import display_html
from uuid import uuid4
table_template = """<style>
#fibonacci_{uuid:} {{width: 600px;border: 0 none;}}
#fibonacci_{uuid:} * {{border: 0 none;text-align: center;}}
#fibonacci_{uuid:} caption {{margin: 0 -5rem 0.5rem;border: 0 none;}}
#fibonacci_{uuid:} tr:first-child, #fibonacci_{uuid:} tr:last-child {{border-bottom: 1px solid black;}}
#fibonacci_{uuid:} tr:first-child {{color: #fafafa;background-color: #212121;}}
#fibonacci_{uuid:} tr:nth-child(2n) {{background-color: #dedede;}}
#fibonacci_{uuid:} tr td:first-child, #fibonacci_{uuid:} tr th:first-child {{width: 50px;border-right: 1px solid #212121;}}
</style><center><table id="fibonacci_{uuid:}">
<caption>Términos 60 - 80 de la Sucesión de Fibonacci</caption>
<tr><th>$n$</th><th>$Fibonacci[n]$</th><th>$RoundFibonacci[n]$</th><th>$Difference[n]$</th></tr>
{0:}</table></center>
"""
table_content = "".join(
["<tr><td>${n:}$</td><td>${fn:}$</td><td {attr}>${rfn:}$</td><td {attr}>${diff:}$</td></tr>".format(
n=n,
fn=Fibonacci(n),
rfn=RoundFibonacci(n),
diff=Fibonacci(n) - RoundFibonacci(n),
attr="style=\"color:#f44336;\"" if RoundFibonacci(n) != Fibonacci(n) else None
) for n in range(60,81)]
)
table = table_template.format(table_content, uuid=uuid4().hex)
display_html(HTML(table))
###Output
_____no_output_____
###Markdown
Como se puede ver en la tabla anterior el cálculo por redondeo nos da el valor exacto hasta el término 70 de la sucesión, sin embargo, luego este término, el error de cálculo crece conforme lo hace $n$. 4. Extensiones 4.1. Extensión a los enteros Por medio de la expresión obtenida para $F_n$ en función de $\phi$, se puede extender $F_n$ para $n < 0$ mediante$$F_{-n} = (-1)^{n+1}F_{n}$$ 4.2. Extensión a los reales La expresion de $F_n$ en funcion de $\phi$ tambien nos permite extenderla $F_n$ a los reales mediante la identidad $\mathrm{e}^{\pi i}=-1$ dando origen a$$ F_{\nu}=\frac{1}{\sqrt{5}}\left(\phi^{\nu} - \left(\frac{1}{\phi}\right)^{\nu}\cos\left(\nu\pi\right)\right). $$La función $F_{\nu}$ tiene sus ceros en los puntos en los cuales se cumple la condicion$$ \phi^{2\nu} = \cos\left(\nu\pi\right). $$ 4.3. Extensión de la implementación en Python Con las observaciones anteriores extenderemos la definicion de nuestra función Fibonacci.
###Code
from math import *
def NMFibonacci(x):
if isinstance(x, int):
if x < 0:
x = abs(x)
return (-1)**(x+1) * DCFibonacci(x)
return DCFibonacci(x)
phi = 0.5*(1 + sqrt(5))
return (phi**x - cos(x*pi) * (phi)**(-x))/sqrt(5)
Fibonacci = Memoize(NMFibonacci)
for n in range(0, 11):
print("Término {0:>2d} -> {1:}".format(n, Fibonacci(n)))
%timeit -n 10 Fibonacci(30)
###Output
_____no_output_____
###Markdown
5. Gráficos La secuencia de Fibonacci se encuentra en múltiples configuraciones biológicas, donde aparecen números consecutivos de la sucesión, como en la distribución de las ramas de los árboles, la distribución de las hojas en un tallo, los frutos de la piña tropical, las flores de la alcachofa, en las piñas de las coníferas, o en el "árbol genealógico" de las abejas melíferas.En esta sección veremos algunos ejemplos gráficos que hacen uso de la serie de Fibonacci. 5.1. Ajustes generales para todos los gráficos
###Code
%matplotlib inline
# Ajustes generales para los gráficos
# Para poder usar 'usetex=True' es necesario haber instalado latex
from matplotlib.font_manager import *
from matplotlib.collections import *
from matplotlib.patches import *
from matplotlib.pylab import *
import gc
ioff()
rc('lines', linewidth=1)
rc('font', family='serif')
font_title = FontProperties(size=18)
font_label = FontProperties(size=14, style='italic')
font_ticks = FontProperties(size=12)
font_legend = FontProperties(size=13)
###Output
_____no_output_____
###Markdown
5.2. Gráficos Discretos 5.2.1. Sucesión de Fibonacci
###Code
fig = figure(figsize=(9, 6), frameon=False)
axs = fig.add_subplot('111')
x = range(1,11)
y = [Fibonacci(n) for n in x]
markerline, stemlines, baseline = axs.stem(x, y)
setp(baseline, 'color', '#64dd17')
setp(markerline, 'color', '#64dd17')
for stemline in stemlines:
setp(stemline, 'color', '#64dd17')
axs.set_axisbelow(True)
axs.set_facecolor('#fafafa')
axs.tick_params(length=0, width=0)
axs.spines['top'].set_color('#fafafa')
axs.spines['right'].set_color('#fafafa')
axs.spines['bottom'].set_color('#212121')
axs.spines['left'].set_color('#212121')
axs.set_xlim(0, 11)
axs.set_xticks(range(0,12))
axs.set_xticklabels(range(0,12), fontproperties=font_ticks)
axs.set_xlabel("n", fontproperties=font_label, labelpad=10)
axs.set_ylim(0, 60)
axs.set_yticks(range(0,70,10))
axs.set_yticklabels(range(0,70,10), fontproperties=font_ticks)
axs.set_ylabel("Fibonacci[n]", fontproperties=font_label, labelpad=10)
axs.set_title("Sucesión de Fibonacci", fontproperties=font_title, y=1.025)
axs.grid(linestyle='--', color='#dedede')
draw()
tight_layout()
show()
fig.clf()
close()
gc.collect();
###Output
_____no_output_____
###Markdown
5.2.2. Sucesión de Fibonacci módulo 10
###Code
fig = figure(figsize=(11.75, 6), frameon=False)
axs = fig.add_subplot('111')
x = range(1,200)
y = [Fibonacci(n)%10 for n in x]
axs.plot(
x,
y,
linestyle='--',
linewidth=1,
color='#212121',
marker='o',
markerfacecolor='#ffc107',
markeredgecolor='#ffc107')
axs.plot(
[60, 60],
[0, 10],
linestyle='-',
linewidth=1,
color='#f44336')
axs.plot(
[120, 120],
[0, 10],
linestyle='-',
linewidth=1,
color='#f44336')
axs.plot(
[180, 180],
[0, 10],
linestyle='-',
linewidth=1,
color='#f44336')
axs.set_axisbelow(True)
axs.set_facecolor('#fafafa')
axs.tick_params(length=0, width=0)
axs.spines['top'].set_color('#fafafa')
axs.spines['right'].set_color('#fafafa')
axs.spines['bottom'].set_color('#212121')
axs.spines['left'].set_color('#212121')
axs.set_xlim(0, 200)
axs.set_xticks(range(0,201,10))
axs.set_xticklabels(range(0,201,10), fontproperties=font_ticks)
axs.set_xlabel("n", fontproperties=font_label, labelpad=10)
axs.set_ylim(0, 10)
axs.set_yticks(range(0,11,1))
axs.set_yticklabels(range(0,11,1), fontproperties=font_ticks)
axs.set_ylabel("Fibonacci[n] % 10", fontproperties=font_label, labelpad=10)
axs.set_title("Sucesión de Fibonacci módulo 10", fontproperties=font_title, y=1.025)
axs.grid(linestyle='--', color='#dedede')
draw()
tight_layout()
show()
fig.clf()
close()
gc.collect();
###Output
_____no_output_____
###Markdown
5.2.3. Sucesión de Fibonacci módulo n
###Code
fig = figure(figsize=(11.75, 6), frameon=False)
axs = fig.add_subplot('111')
x = range(1,200)
y = [Fibonacci(n)%n for n in x]
axs.plot(
x,
y,
linestyle='--',
linewidth=1,
color='#212121',
marker='o',
markerfacecolor='#ffc107',
markeredgecolor='#ffc107')
axs.set_axisbelow(True)
axs.set_facecolor('#fafafa')
axs.tick_params(length=0, width=0)
axs.spines['top'].set_color('#fafafa')
axs.spines['right'].set_color('#fafafa')
axs.spines['bottom'].set_color('#212121')
axs.spines['left'].set_color('#212121')
axs.set_xlim(0, 200)
axs.set_xticks(range(0,201,10))
axs.set_xticklabels(range(0,201,10), fontproperties=font_ticks)
axs.set_xlabel("n", fontproperties=font_label, labelpad=10)
axs.set_ylim(0, 200)
axs.set_yticks(range(0,201,20))
axs.set_yticklabels(range(0,201,20), fontproperties=font_ticks)
axs.set_ylabel("Fibonacci[n] % n", fontproperties=font_label, labelpad=10)
axs.set_title("Sucesión de Fibonacci módulo n", fontproperties=font_title, y=1.025)
axs.grid(linestyle='--', color='#dedede')
draw()
tight_layout()
show()
fig.clf()
close()
gc.collect();
###Output
_____no_output_____
###Markdown
5.2.4. Extensión a los números negativos
###Code
fig = figure(figsize=(12, 8), frameon=False)
axs = fig.add_subplot('111')
x = range(-10,11)
y = [Fibonacci(n) for n in x]
markerline, stemlines, baseline = axs.stem(x, y)
setp(baseline, 'color', '#64dd17')
setp(markerline, 'color', '#64dd17')
for stemline in stemlines:
setp(stemline, 'color', '#64dd17')
axs.set_axisbelow(True)
axs.set_facecolor('#fafafa')
axs.tick_params(length=0, width=0)
axs.spines['top'].set_color('#fafafa')
axs.spines['right'].set_color('#fafafa')
axs.spines['bottom'].set_color('#212121')
axs.spines['left'].set_color('#212121')
axs.set_xlim(-11, 11)
axs.set_xticks(range(-11,12))
axs.set_xticklabels(range(-11,12), fontproperties=font_ticks)
axs.set_xlabel("n", fontproperties=font_label, labelpad=10)
axs.set_ylim(-60, 60)
axs.set_yticks(range(-60,70,10))
axs.set_yticklabels(range(-60,70,10), fontproperties=font_ticks)
axs.set_ylabel("Fibonacci[n]", fontproperties=font_label, labelpad=10)
axs.set_title("Extensión de la sucesión de Fibonacci a los enteros", fontproperties=font_title, y=1.025)
axs.grid(linestyle='--', color='#dedede')
draw()
tight_layout()
show()
fig.clf()
close()
gc.collect();
###Output
_____no_output_____
###Markdown
5.2.5. Representación en forma binaria
###Code
fig = figure(figsize=(9, 6), frameon=False)
axs = fig.add_subplot('111')
BinaryFibonacciMatrix = [[int(d) for d in "{0:0>600s}".format(BinaryFibonacci(n))] for n in range (1, 600)]
BinaryFibonacciMatrix = array(BinaryFibonacciMatrix ,dtype=int)
BinaryFibonacciMatrix = BinaryFibonacciMatrix.transpose()
mask = [i for i, row in enumerate(BinaryFibonacciMatrix) if not any(row)]
BinaryFibonacciMatrix = delete(BinaryFibonacciMatrix, mask, 0)
axs.imshow(BinaryFibonacciMatrix, cmap='Greys')
axs.set_title(
"Gráfico binario de la Suceción de Fibonacci",
fontproperties=font_title,
horizontalalignment='left',
verticalalignment='top',
x=0,
y=1,
)
axs.axis('off')
draw()
tight_layout()
show()
###Output
_____no_output_____
###Markdown
5.3. Gráficos Continuos 5.3.1. Función de Fibonacci
###Code
fig = figure(figsize=(12, 8), frameon=False)
axs = fig.add_subplot('111')
x = linspace(-10,10,500)
y = [Fibonacci(nu) for nu in x]
axs.plot(
x,
y,
linestyle='--',
color='#212121',
label="Función de Fibonacci")
x = linspace(-10,10,21)
y = [Fibonacci(nu) for nu in x]
markerline, stemlines, baseline = axs.stem(
x,
y,
label="Sucesión de Fibonacci")
setp(baseline, 'color', '#ffc107')
setp(markerline, 'color', '#ffc107')
for stemline in stemlines:
setp(stemline, 'color', '#ffc107')
axs.set_axisbelow(True)
axs.set_facecolor('#fafafa')
axs.tick_params(length=0, width=0)
axs.spines['top'].set_color('#fafafa')
axs.spines['right'].set_color('#fafafa')
axs.spines['bottom'].set_color('#212121')
axs.spines['left'].set_color('#212121')
axs.set_xlim(-11, 11)
axs.set_xticks(range(-11,12))
axs.set_xticklabels(range(-11,12), fontproperties=font_ticks)
axs.set_xlabel("x", fontproperties=font_label, labelpad=10)
axs.set_ylim(-60, 60)
axs.set_yticks(range(-60,70,10))
axs.set_yticklabels(range(-60,70,10), fontproperties=font_ticks)
axs.set_ylabel("Fibonacci[x]", fontproperties=font_label, labelpad=10)
axs.set_title("Función de Fibonacci", fontproperties=font_title, y=1.025)
axs.grid(linestyle='--', color='#dedede')
axs.legend(loc=4, prop=font_legend, title='Leyenda')
draw()
tight_layout()
show()
fig.clf()
close()
gc.collect();
###Output
_____no_output_____
###Markdown
5.3.2. Los ceros de la Función de Fibonacci
###Code
from scipy.optimize import fsolve
fig = figure(figsize=(12, 8), frameon=False)
axs = fig.add_subplot('111')
x = linspace(-10,10,500)
y = [Fibonacci(nu) for nu in x]
axs.plot(
x,
y,
linestyle='--',
color='#212121',
label="Función de Fibonacci")
x = [fsolve(NMFibonacci, nu + 0.5) for nu in linspace(-10,0,11)]
y = [0 for nu in x]
axs.plot(
x,
y,
linewidth=0,
marker='o',
markerfacecolor='#f44336',
markeredgecolor='#f44336',
label="Ceros de la función de Fibonacci")
axs.set_axisbelow(True)
axs.set_facecolor('#fafafa')
axs.tick_params(length=0, width=0)
axs.spines['top'].set_color('#fafafa')
axs.spines['right'].set_color('#fafafa')
axs.spines['bottom'].set_color('#212121')
axs.spines['left'].set_color('#212121')
axs.set_xlim(-11, 11)
axs.set_xticks(range(-11,12))
axs.set_xticklabels(range(-11,12), fontproperties=font_ticks)
axs.set_xlabel("x", fontproperties=font_label, labelpad=10)
axs.set_ylim(-60, 60)
axs.set_yticks(range(-60,70,10))
axs.set_yticklabels(range(-60,70,10), fontproperties=font_ticks)
axs.set_ylabel("Fibonacci[x]", fontproperties=font_label, labelpad=10)
axs.set_title("Función de Fibonacci", fontproperties=font_title, y=1.025)
axs.grid(linestyle='--', color='#dedede')
axs.legend(loc=4, prop=font_legend, title='Leyenda')
draw()
tight_layout()
show()
fig.clf()
close()
gc.collect();
###Output
_____no_output_____
###Markdown
5.4. Gráficos Geométricos 5.4.1. La espiral de Fibonacci
###Code
fig = figure(figsize=[12, 8], frameon=False)
axs = fig.add_subplot('111')
origin = array([0, 0])
center = origin
theta = array([270, 0])
n_max=10
for n in range(1,n_max+1):
theta = theta + 90
order = (n - 1) % 4 + 1
fib_n = Fibonacci(n)
radius = fib_n
height = fib_n
width = fib_n
if order == 1:
origin = origin + [-Fibonacci(n-2), Fibonacci(n-1)]
center = center + [-Fibonacci(n-2), 0]
elif order == 2:
origin = origin + [-Fibonacci(n), -Fibonacci(n-2)]
center = center + [0, -Fibonacci(n-2) ]
elif order == 3:
origin = origin + [0, -Fibonacci(n)]
center = center + [Fibonacci(n-2) , 0]
elif order == 4:
origin = origin + [Fibonacci(n-1), 0]
center = center + [0, Fibonacci(n-2)]
# Add a rectangle
rectangle = Rectangle(origin, height, width, linewidth=0, color="#212121")
axs.add_patch(rectangle)
# Add a wedge
wedge = Wedge(center, radius, theta1=theta[0] , theta2=theta[1], color="#ffa000")
axs.add_patch(wedge)
text(
*(origin + [0.5*height, 0.5*width]),
"{0:}".format(fib_n),
horizontalalignment='center',
verticalalignment='center',
family='serif',
size=n*2,
color='#fafafa',
)
axs.set_title("La espiral de fibonacci",
fontproperties=font_title,
horizontalalignment='center',
verticalalignment='center',
x=0.50,
y=0.95,
)
axs.set_facecolor('#ff0000')
axs.axis('equal')
axs.axis('off')
axs.grid('off')
draw()
tight_layout()
show()
fig.clf()
close()
gc.collect();
###Output
_____no_output_____
###Markdown
5.4.2. Modelo de Vogel para la distribución de semillas girasol Para este gráfico necesitaremos el denominado Ángulo de oro que viene dado por$$ \psi = \frac{2\pi}{\phi^2} \approx 137.507764^\circ .$$Teniendo en cuenta las propiedades del número áureo $\phi$, podemos expresar $\psi$ como$$ \psi = 2\pi\left(2-\phi\right)$$que es la representación que emplearemos para calcular $\psi$ en este gráfico.
###Code
X = 0
Y = 1
discs=500
colors=34
divider=100
lower=0
upper=100
columns=4
rows=25
scale=4
fig = figure(figsize=[columns*scale, rows*scale], frameon=False)
for index, delta in enumerate([n * pi / divider for n in range(lower, upper)]):
axs = fig.add_subplot(rows, columns, index+1)
origin = array([0, 0])
phi = (1 + (5 ** 0.5)) / 2.0
psi = (2 * pi) + (2 - phi)
delta = psi + delta
theta = 0
k = 0.2
radius = k * (1 + (5 ** 0.5)) / 4.0
color_step = 100 / colors
color_cycle = [(n % colors) * color_step for n in range(discs)]
patches = []
for n in range(1, discs+1):
r = k * (n ** 0.5)
theta = theta + delta
x = origin[X] + (r * cos(theta))
y = origin[Y] + (r * sin(theta))
disc = Circle((x, y), radius)
patches.append(disc)
patches = PatchCollection(patches, cmap=matplotlib.cm.jet, alpha=1.0)
patches.set_array(array(color_cycle))
axs.add_collection(patches)
axs.set_xticks([])
axs.set_yticks([])
axs.set_facecolor('#fafafa')
axs.spines['top'].set_linewidth(3)
axs.spines['top'].set_color('#212121')
axs.spines['right'].set_linewidth(0)
axs.spines['right'].set_color('#fafafa')
axs.spines['bottom'].set_linewidth(0)
axs.spines['bottom'].set_color('#fafafa')
axs.spines['left'].set_linewidth(0)
axs.spines['left'].set_color('#fafafa')
axs.set_title("Delta: {0: >5.2f}PI rad".format((index + lower)/divider, divider),
fontproperties=font_title,
horizontalalignment='left',
verticalalignment='bottom',
x=0,
y=1,
)
axs.axis('equal')
draw()
tight_layout()
show()
fig.clf()
close()
gc.collect();
###Output
_____no_output_____ |
electrochem/data_loading.ipynb | ###Markdown
Data Loading
###Code
import os
os.chdir('electrochemistry_sean_mcintosh/electrochem')
!ls
import numpy as np
import pandas as pd
import matplotlib.pyplot as plt
import pickle
from pathlib import Path
import seaborn as sns
from tqdm.notebook import trange, tqdm
import wandb
sns.set()
DATA_DIR = Path('../data')
# Do not read in 'Mix 1' sheet, as that has been updated in 'mix_1_updated.xlsx'
sheet_names = ['Seawater - No Heavy Metals', 'Copper', 'Cadmium', 'Lead']
xcel = pd.read_excel(DATA_DIR / 'main.xlsx', sheet_name=sheet_names)
# Read in updated mix sheet
mix = pd.read_excel(DATA_DIR / 'mix_1_updated.xlsx')
seawater = xcel['Seawater - No Heavy Metals']
copper = xcel['Copper']
cadmium = xcel['Cadmium']
lead = xcel['Lead']
seawater['label'] = 'Sw'
seawater = seawater.drop(['Unnamed: 0', 'Unnamed: 1', 'Unnamed: 2'], axis=1)
seawater.head(3)
copper['label'] = 'Cu'
cadmium['label'] = 'Cd'
lead['label'] = 'Pb'
lead.columns
copper = copper.drop(['Unnamed: 0', 'Unnamed: 1', 'Concentration'], axis=1)
cadmium = cadmium.drop(['Unnamed: 0', 'Analyte', 'Concentration'], axis=1)
lead = lead.drop(['Unnamed: 0', 'Analyte', 'Concentration'], axis=1)
dfs = [copper, cadmium, lead, seawater]
for df in dfs:
print(df.shape)
df = pd.concat(dfs, ignore_index=True)
df.label.value_counts(normalize=True)
df.head()
df.to_csv(DATA_DIR / 'four_class_dataset.csv')
X = df.iloc[:, :-1].values
y = df.iloc[:, -1].values
df.shape
###Output
_____no_output_____ |
notebooks/SST_diurnal_cycle.ipynb | ###Markdown
SST data as a function of MJO, monsoon
###Code
import xarray
import pandas
sst_dataset = xarray.open_dataset('/home/rjackson/data/IMOS_aggregation_20181128T154948Z.nc')
sst_dataset
sst_dataset.sea_surface_temperature.mean(axis=0).plot()
###Output
_____no_output_____ |
notebook/fun_07_01.ipynb | ###Markdown
说明```"车辆登入":{ "数据采集时间":"6", "登入流水号":"2", "ICCID":"20", "可充电储能子系统数n":"1", "可充电储能系统编码长度m":"1", *"可充电储能系统编码":"n*m"[[],[],...],}``` 导入工具包和公共函数
###Code
import Ipynb_importer
from public_fun import *
import sys
import globalVar as glv
###Output
_____no_output_____
###Markdown
类主体
###Code
class fun_07_01(object):
def __init__(self, data):
self.cf = [6, 2, 20, 1, 1]
self.cf_a = hexlist2(self.cf)
self.n = hex2dec(data[self.cf_a[3]:self.cf_a[4]])
self.m = hex2dec(data[self.cf_a[4]:self.cf_a[5]])
self.cf.append(self.n*self.m)
self.cf_a = hexlist2(self.cf)
self.o = data[0:self.cf_a[-1]]
self.list_o = [
"数据采集时间",
"登入流水号",
"ICCID",
"可充电储能子系统数",
"可充电储能系统编码长度",
"可充电储能系统编码",
]
self.oj = list2dict(self.o, self.list_o, self.cf_a)
self.oj2 = {'车辆登入': self.oj}
self.ol = pd.DataFrame([self.oj]).reindex(columns=self.list_o)
self.pj = {
"数据采集时间":get_datetime(self.oj['数据采集时间']),
"登入流水号":hex2dec(self.oj['登入流水号']),
"ICCID":hex2str(self.oj['ICCID']),
"可充电储能子系统数":hex2dec(self.oj['可充电储能子系统数']),
"可充电储能系统编码长度":hex2dec(self.oj['可充电储能系统编码长度']),
"可充电储能系统编码":fun_07_01.fun_07_01_06(self.oj['可充电储能系统编码'], self.oj['可充电储能子系统数'], self.oj['可充电储能系统编码长度']),
}
self.pj2 = {'车辆登入': self.pj}
self.pl = pd.DataFrame([self.pj]).reindex(columns=self.list_o)
self.next = data[len(self.o):]
self.nextMark = data[len(self.o):len(self.o)+2]
# glv.set_value('data_f', self.next)
def fun_07_01_06(data, n, m):
if m=='00':
return "NA"
else :
n = hex2dec(n)
m = hex2dec(m) * 2
output = []
for i in range(n):
output_unit = hex2str(data[i * m: i* m +m])
output.append(output_unit)
return output
###Output
_____no_output_____
###Markdown
执行
###Code
#
data = "130c1e0d371700033839383630363137303330303831303036303531010c313137333831333033383430dc"
fun_07_01 = fun_07_01(data)
###Output
{'数据采集时间': '130c1e0d3717', '登入流水号': '0003', 'ICCID': '3839383630363137303330303831303036303531', '可充电储能子系统数': '01', '可充电储能系统编码长度': '0c', '可充电储能系统编码': '313137333831333033383430'}
###Markdown
结果
###Code
fun_07_01.oj
fun_07_01.ol
fun_07_01.pj
fun_07_01.pl
fun_07_01.cf
fun_07_01.cf_a
###Output
_____no_output_____ |
tf_quant_finance/experimental/dev/Exact_Gaussian_HJM_WIP.ipynb | ###Markdown
Copyright 2021 Google LLC.Licensed under the Apache License, Version 2.0 (the "License");
###Code
#@title Licensed under the Apache License, Version 2.0 (the "License"); { display-mode: "form" }
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# https://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
!pip install tf-quant-finance
!pip install QuantLib-Python
import tensorflow as tf
import numpy as np
# tf.compat.v1.disable_eager_execution()
from typing import Any, Dict, List, Tuple, Union, Optional
import dataclasses
from tf_quant_finance import datetime as dates
from tf_quant_finance.models import generic_ito_process
from tf_quant_finance.math import piecewise
from tf_quant_finance.math import random
from tf_quant_finance.math import gradient
from tf_quant_finance.models import euler_sampling
from tf_quant_finance.models import utils
from tf_quant_finance.models.hjm import quasi_gaussian_hjm
from tf_quant_finance.math.interpolation.linear import interpolate
from tf_quant_finance.math.pde import fd_solvers
import matplotlib.pyplot as plt
###Output
_____no_output_____
###Markdown
HJM Model
###Code
class GaussianHJM(quasi_gaussian_hjm.QuasiGaussianHJM):
r"""Gaussian HJM model for term-structure modeling.
Heath-Jarrow-Morton (HJM) model for the interest rate term-structre
modelling specifies the dynamics of the instantaneus forward rate `f(t,T)`
with maturity `T` at time `t` as follows:
```None
df(t,T) = mu(t,T) dt + sum_i sigma_i(t, T) * dW_i(t),
1 <= i <= n,
```
where `mu(t,T)` and `sigma_i(t,T)` denote the drift and volatility
for the forward rate and `W_i` are Brownian motions with instantaneous
correlation `Rho`. The model above represents an `n-factor` HJM model.
The Gaussian HJM model assumes that the volatility `sigma_i(t,T)` is a
deterministic function of time (t). Under the risk-neutral measure, the
drift `mu(t,T)` is computed as
```
mu(t,T) = sum_i sigma_i(t,T) int_t^T sigma_(t,u) du
```
Using the separability condition, the HJM model above can be formulated as
the following Markovian model:
```None
sigma(t,T) = sigma(t) * h(T) (Separability condition)
```
A common choice for the function h(t) is `h(t) = exp(-kt)`. Using the above
parameterization of sigma(t,T), we obtain the following Markovian
formulation of the HJM model [1]:
```None
HJM Model
dx_i(t) = (sum_j [y_ij(t)] - k_i * x_i(t)) dt + sigma_i(t) dW_i
dy_ij(t) = (rho_ij * sigma_i(t)*sigma_j(t) - (k_i + k_j) * y_ij(t)) dt
r(t) = sum_i x_i(t) + f(0, t)
```
where `x` is an `n`-dimensional vector and `y` is an `nxn` dimensional
matrix. For Gaussian HJM model, the quantity `y_ij(t)` can be computed
analytically as follows:
```None
y_ij(t) = rho_ij * exp(-k_i * t) * exp(-k_j * t) *
int_0^t exp((k_i+k_j) * s) * sigma_i(s) * sigma_j(s) ds
```
The Gaussian HJM class implements the model outlined above by simulating the
state `x(t)` while analytically computing `y(t)`.
The price at time `t` of a zero-coupon bond maturing at `T` is given by
(Ref. [1]):
```None
P(t,T) = P(0,T) / P(0,t) *
exp(-x(t) * G(t,T) - 0.5 * y(t) * G(t,T)^2)
```
The HJM model implementation supports constant mean-reversion rate `k` and
`sigma(t)` can be an arbitrary function of `t`. We use Euler discretization
to simulate the HJM model.
#### Example. Simulate a 4-factor HJM process.
```python
import numpy as np
import tensorflow.compat.v2 as tf
import tf_quant_finance as tff
dtype = tf.float64
def discount_fn(x):
return 0.01 * tf.ones_like(x, dtype=dtype)
process = tff.models.hjm.GaussianHJM(
dim=4,
mean_reversion=[0.03, 0.01, 0.02, 0.005], # constant mean-reversion
volatility=[0.01, 0.011, 0.015, 0.008], # constant volatility
initial_discount_rate_fn=discount_fn,
dtype=dtype)
times = np.array([0.1, 1.0, 2.0, 3.0])
short_rate_paths, discount_paths, _, _ = process.sample_paths(
times,
num_samples=100000,
time_step=0.1,
random_type=tff.math.random.RandomType.STATELESS_ANTITHETIC,
seed=[1, 2],
skip=1000000)
```
#### References:
[1]: Leif B. G. Andersen and Vladimir V. Piterbarg. Interest Rate Modeling.
Volume II: Term Structure Models.
"""
def __init__(self,
dim,
mean_reversion,
volatility,
initial_discount_rate_fn,
corr_matrix=None,
dtype=None,
name=None):
"""Initializes the HJM model.
Args:
dim: A Python scalar which corresponds to the number of factors comprising
the model.
mean_reversion: A real positive `Tensor` of shape `[dim]`. Corresponds to
the mean reversion rate of each factor.
volatility: A real positive `Tensor` of the same `dtype` and shape as
`mean_reversion` or a callable with the following properties: (a) The
callable should accept a scalar `Tensor` `t` and returns a 1-D
`Tensor` of shape `[dim]`. The function returns instantaneous
volatility `sigma(t)`. When `volatility` is specified is a real
`Tensor`, each factor is assumed to have a constant instantaneous
volatility. Corresponds to the instantaneous volatility of each
factor.
initial_discount_rate_fn: A Python callable that accepts expiry time as a
real `Tensor` of the same `dtype` as `mean_reversion` and returns a
`Tensor` of shape `input_shape`. Corresponds to the zero coupon bond
yield at the present time for the input expiry time.
corr_matrix: A `Tensor` of shape `[dim, dim]` and the same `dtype` as
`mean_reversion`. Corresponds to the correlation matrix `Rho`.
dtype: The default dtype to use when converting values to `Tensor`s.
Default value: `None` which means that default dtypes inferred by
TensorFlow are used.
name: Python string. The name to give to the ops created by this class.
Default value: `None` which maps to the default name
`gaussian_hjm_model`.
"""
self._name = name or 'gaussian_hjm_model'
with tf.name_scope(self._name):
self._dtype = dtype or None
self._dim = dim
self._factors = dim
def _instant_forward_rate_fn(t):
t = tf.convert_to_tensor(t, dtype=self._dtype)
def _log_zero_coupon_bond(x):
r = tf.convert_to_tensor(
initial_discount_rate_fn(x), dtype=self._dtype)
return -r * x
rate = -gradient.fwd_gradient(
_log_zero_coupon_bond,
t,
use_gradient_tape=True,
unconnected_gradients=tf.UnconnectedGradients.ZERO)
return rate
def _initial_discount_rate_fn(t):
return tf.convert_to_tensor(
initial_discount_rate_fn(t), dtype=self._dtype)
self._instant_forward_rate_fn = _instant_forward_rate_fn
self._initial_discount_rate_fn = _initial_discount_rate_fn
self._mean_reversion = tf.convert_to_tensor(
mean_reversion, dtype=dtype, name='mean_reversion')
self._batch_shape = []
self._batch_rank = 0
# Setup volatility
if callable(volatility):
self._volatility = volatility
else:
volatility = tf.convert_to_tensor(volatility, dtype=dtype)
jump_locations = [[]] * dim
volatility = tf.expand_dims(volatility, axis=-1)
self._volatility = piecewise.PiecewiseConstantFunc(
jump_locations=jump_locations, values=volatility, dtype=dtype)
if corr_matrix is None:
corr_matrix = tf.eye(dim, dim, dtype=self._dtype)
self._rho = tf.convert_to_tensor(corr_matrix, dtype=dtype, name='rho')
self._sqrt_rho = tf.linalg.cholesky(self._rho)
# Volatility function
def _vol_fn(t, state):
"""Volatility function of Gaussian-HJM."""
del state
volatility = self._volatility(tf.expand_dims(t, -1)) # shape=(dim, 1)
print(t, volatility)
return self._sqrt_rho * volatility
# Drift function
def _drift_fn(t, state):
"""Drift function of Gaussian-HJM."""
x = state
# shape = [self._factors, self._factors]
y = self.state_y(tf.expand_dims(t, axis=-1))[..., 0]
drift = tf.math.reduce_sum(y, axis=-1) - self._mean_reversion * x
return drift
self._exact_discretization_setup(dim)
super(quasi_gaussian_hjm.QuasiGaussianHJM,
self).__init__(dim, _drift_fn, _vol_fn, dtype, self._name)
def sample_paths(self,
times,
num_samples,
time_step=None,
num_time_steps=None,
random_type=None,
seed=None,
skip=0,
use_euler_sampling=False,
name=None):
"""Returns a sample of short rate paths from the HJM process.
For the Gaussian HJM model, the distribution of short rate and spot discount
curve can be computed analytically. By default the method uses the
analytical distribution to generate sample paths for short rate. Euler
sampling can be enabled through an input flag.
Args:
times: A real positive `Tensor` of shape `(num_times,)`. The times at
which the path points are to be evaluated.
num_samples: Positive scalar `int32` `Tensor`. The number of paths to
draw.
time_step: Scalar real `Tensor`. Maximal distance between time grid points
in Euler scheme. Used only when Euler scheme is applied.
Default value: `None`.
num_time_steps: An optional Scalar integer `Tensor` - a total number of
time steps performed by the algorithm. The maximal distance betwen
points in grid is bounded by
`times[-1] / (num_time_steps - times.shape[0])`.
Either this or `time_step` should be supplied.
Default value: `None`.
random_type: Enum value of `RandomType`. The type of (quasi)-random
number generator to use to generate the paths.
Default value: `None` which maps to the standard pseudo-random numbers.
seed: Seed for the random number generator. The seed is
only relevant if `random_type` is one of
`[STATELESS, PSEUDO, HALTON_RANDOMIZED, PSEUDO_ANTITHETIC,
STATELESS_ANTITHETIC]`. For `PSEUDO`, `PSEUDO_ANTITHETIC` and
`HALTON_RANDOMIZED` the seed should be an Python integer. For
`STATELESS` and `STATELESS_ANTITHETIC `must be supplied as an integer
`Tensor` of shape `[2]`.
Default value: `None` which means no seed is set.
skip: `int32` 0-d `Tensor`. The number of initial points of the Sobol or
Halton sequence to skip. Used only when `random_type` is 'SOBOL',
'HALTON', or 'HALTON_RANDOMIZED', otherwise ignored.
Default value: `0`.
use_euler_sampling: An optional Python boolean to indicate if simulations
are performed using Euler sampling.
Default value: `False` indicating exact sampling to be used.
name: Python string. The name to give this op.
Default value: `sample_paths`.
Returns:
A tuple containing four elements.
* The first element is a `Tensor` of
shape `[num_samples, num_times]` containing the simulated short rate
paths.
* The second element is a `Tensor` of shape
`[num_samples, num_times]` containing the simulated discount factor
paths.
* The third element is a `Tensor` of shape
`[num_samples, num_times, dim]` conating the simulated values of the
state variable `x`
* The fourth element is a `Tensor` of shape
`[num_samples, num_times, dim^2]` conating the simulated values of the
state variable `y`.
Raises:
ValueError:
(a) If `times` has rank different from `1`.
(b) If Euler scheme is used by times is not supplied.
"""
name = name or self._name + '_sample_path'
with tf.name_scope(name):
times = tf.convert_to_tensor(times, self._dtype)
if times.shape.rank != 1:
raise ValueError('`times` should be a rank 1 Tensor. '
'Rank is {} instead.'.format(times.shape.rank))
return self._sample_paths(
times, time_step, num_time_steps, num_samples, random_type, skip,
seed, use_euler_sampling)
def state_y(self, t):
"""Computes the state variable `y(t)` for tha Gaussian HJM Model.
For Gaussian HJM model, the state parameter y(t), can be analytically
computed as follows:
y_ij(t) = exp(-k_i * t) * exp(-k_j * t) * (
int_0^t [exp(k_i * u) * exp(k_j * u) * rho_ij *
sigma_i(u) * sigma_j(u)] * du)
Args:
t: A rank 1 real `Tensor` of shape `[num_times]` specifying the time `t`.
Returns:
A real `Tensor` of shape [self._factors, self._factors, num_times]
containing the computed y_ij(t).
"""
t = tf.convert_to_tensor(t, dtype=self._dtype)
# t_shape = tf.shape(t)
# t = tf.broadcast_to(t, tf.concat([[self._dim], t_shape], axis=0))
time_index = tf.searchsorted(self._jump_locations, t)
# create a matrix k2(i,j) = k(i) + k(j)
mr2 = tf.expand_dims(self._mean_reversion, axis=-1)
# Add a dimension corresponding to `num_times`
mr2 = tf.expand_dims(mr2 + tf.transpose(mr2), axis=-1)
def _integrate_volatility_squared(vol, l_limit, u_limit):
# create sigma2_ij = sigma_i * sigma_j
vol = tf.expand_dims(vol, axis=-2)
vol_squared = tf.expand_dims(self._rho, axis=-1) * (
vol * tf.transpose(vol, perm=[1, 0, 2]))
return vol_squared / mr2 * (tf.math.exp(mr2 * u_limit) - tf.math.exp(
mr2 * l_limit))
is_constant_vol = tf.math.equal(tf.shape(self._jump_values_vol)[-1], 0)
v_squared_between_vol_knots = tf.cond(
is_constant_vol,
lambda: tf.zeros(shape=(self._dim, self._dim, 0), dtype=self._dtype),
lambda: _integrate_volatility_squared( # pylint: disable=g-long-lambda
self._jump_values_vol, self._padded_knots, self._jump_locations))
v_squared_at_vol_knots = tf.concat([
tf.zeros((self._dim, self._dim, 1), dtype=self._dtype),
utils.cumsum_using_matvec(v_squared_between_vol_knots)
], axis=-1)
vn = tf.concat([self._zero_padding, self._jump_locations], axis=0)
v_squared_t = _integrate_volatility_squared(
self._volatility(t), tf.gather(vn, time_index), t)
v_squared_t += tf.gather(v_squared_at_vol_knots, time_index, batch_dims=-1)
return tf.math.exp(-mr2 * t) * v_squared_t
def discount_bond_price(self, state, times, maturities, name=None):
"""Returns zero-coupon bond prices `P(t,T)` conditional on `x(t)`.
Args:
state: A `Tensor` of real dtype and shape compatible with
`(num_times, dim)` specifying the state `x(t)`.
times: A `Tensor` of real dtype and shape `(num_times,)`. The time `t`
at which discount bond prices are computed.
maturities: A `Tensor` of real dtype and shape `(num_times,)`. The time
to maturity of the discount bonds.
name: Str. The name to give this op.
Default value: `discount_bond_prices`.
Returns:
A `Tensor` of real dtype and the same shape as `(num_times,)`
containing the price of zero-coupon bonds.
"""
name = name or self._name + '_discount_bond_prices'
with tf.name_scope(name):
x_t = tf.convert_to_tensor(state, self._dtype)
times = tf.convert_to_tensor(times, self._dtype)
maturities = tf.convert_to_tensor(maturities, self._dtype)
# Flatten it because `PiecewiseConstantFunction` expects the first
# dimension to be broadcastable to [dim]
input_shape_times = tf.shape(times)
# The shape of `mean_reversion` will is `[dim]`
mean_reversion = self._mean_reversion
y_t = self.state_y(times)
y_t = tf.reshape(tf.transpose(y_t), tf.concat(
[input_shape_times, [self._dim, self._dim]], axis=0))
# Shape=(1, 1, num_times)
values = self._bond_reconstitution(
times, maturities, mean_reversion, x_t, y_t, 1,
tf.shape(times)[0])
return values[0][0]
def _sample_paths(self, times, time_step, num_time_steps, num_samples,
random_type, skip, seed, use_euler_sampling=False):
"""Returns a sample of paths from the process."""
initial_state = tf.zeros((self._dim,), dtype=self._dtype)
if use_euler_sampling:
rate_paths, discount_factor_paths, paths = self._sample_paths_from_euler(
times, time_step, num_time_steps, num_samples, random_type, skip,
seed, initial_state)
else:
rate_paths, discount_factor_paths, paths = self._sample_paths_from_exact(
times, time_step, num_time_steps, num_samples, random_type,
skip, seed, initial_state)
y_paths = self.state_y(times) # shape=(dim, dim, num_times)
y_paths = tf.reshape(
y_paths, tf.concat([[self._dim**2], tf.shape(times)], axis=0))
# shape=(num_samples, num_times, dim**2)
y_paths = tf.repeat(tf.expand_dims(tf.transpose(
y_paths), axis=0), num_samples, axis=0)
return (rate_paths, discount_factor_paths, paths, y_paths)
def _sample_paths_from_euler(
self, times, time_step, num_time_steps, num_samples, random_type, skip,
seed, initial_state):
"""Returns a sample of paths from the process using Euler sampling."""
# Note that we need a finer simulation grid (determnied by `dt`) to compute
# discount factors accurately. The `times` input might not be granular
# enough for accurate calculations.
time_step_internal = time_step
if num_time_steps is not None:
num_time_steps = tf.convert_to_tensor(num_time_steps, dtype=tf.int32,
name='num_time_steps')
time_step_internal = times[-1] / tf.cast(
num_time_steps, dtype=self._dtype)
times, _, time_indices = utils.prepare_grid(
times=times, time_step=time_step_internal, dtype=self._dtype,
num_time_steps=num_time_steps)
# Add zeros as a starting location
dt = times[1:] - times[:-1]
# Shape = (num_samples, num_times, nfactors)
paths = euler_sampling.sample(
self._dim,
self._drift_fn,
self._volatility_fn,
times,
num_time_steps=num_time_steps,
num_samples=num_samples,
initial_state=initial_state,
random_type=random_type,
seed=seed,
time_step=time_step,
skip=skip)
f_0_t = self._instant_forward_rate_fn(times) # shape=(num_times,)
rate_paths = tf.math.reduce_sum(
paths, axis=-1) + f_0_t # shape=(num_samples, num_times)
discount_factor_paths = tf.math.exp(-rate_paths[:, :-1] * dt)
discount_factor_paths = tf.concat(
[tf.ones((num_samples, 1), dtype=self._dtype), discount_factor_paths],
axis=1) # shape=(num_samples, num_times)
discount_factor_paths = utils.cumprod_using_matvec(discount_factor_paths)
return (tf.gather(rate_paths, time_indices, axis=1),
tf.gather(discount_factor_paths, time_indices, axis=1),
tf.gather(paths, time_indices, axis=1))
def _sample_paths_from_exact(
self, times, time_step, num_time_steps, num_samples, random_type, skip,
seed, initial_state):
"""Returns a sample of paths from the process using exact sampling."""
num_requested_times = tf.shape(times)[0]
# Add zeros as a starting location
times = tf.concat([[0.], times], axis=0)
keep_mask = tf.cast(tf.concat([[0], tf.ones((num_requested_times), dtype=tf.int32)], axis=0), tf.bool)
dt = times[1:] - times[:-1]
if dt.shape.is_fully_defined():
steps_num = dt.shape.as_list()[-1]
else:
steps_num = tf.shape(dt)[-1]
# TODO(b/148133811): Re-enable Sobol test when TF 2.2 is released.
if random_type == random.RandomType.SOBOL:
raise ValueError('Sobol sequence for sample paths is temporarily '
'unsupported when `time_step` or `times` have a '
'non-constant value')
# We generate `dim + 1` draws with an additonal draw for discount factor
normal_draws = utils.generate_mc_normal_draws(
num_normal_draws=self._dim + 1, num_time_steps=steps_num,
num_sample_paths=num_samples, random_type=random_type,
seed=seed,
dtype=self._dtype, skip=skip)
exp_x_t = self._conditional_mean_x(times)
var_x_t = self._conditional_variance_x(times)
log_df_double_integral = self._discount_factor_double_integral(times)
y = self.state_y(times)
cov_x_log_df2 = self._cov_between_x_and_log_df(times)
cov_x_log_df = -cov_x_log_df2# tf.zeros_like(cov_x_log_df2)
cond_fn = lambda i, *args: i < steps_num
def body_fn(i, written_count, current_x, current_log_df, x_paths, log_df_paths):
"""Simulate HJM process to the next time point."""
normals = normal_draws[i]
# Update log discount factor I(t) = -int_0^t sum(x_i(t))
# G(t_i, t_i+1)
capital_g = (1. - tf.math.exp(
-self._mean_reversion * (times[i+1] - times[i]))) / self._mean_reversion
y_times_g_squared = tf.math.reduce_sum(tf.linalg.matvec(y[..., i], capital_g) * capital_g)
cov_matrix = tf.concat(
[var_x_t[..., i], tf.expand_dims(cov_x_log_df[..., i], axis=0)],
axis=0)
vv = 2 * log_df_double_integral[i:i+1] - y_times_g_squared
cov_matrix = tf.concat([cov_matrix, tf.expand_dims(tf.concat([cov_x_log_df[..., i], vv], axis=0),axis=-1)], axis=1)
sigma = tf.math.sqrt(tf.linalg.diag_part(cov_matrix))
sigma_ij = tf.expand_dims(sigma, axis=0) * tf.expand_dims(sigma, axis=1)
corr_matrix = tf.math.divide_no_nan(cov_matrix, sigma_ij)
try:
sqrt_corr = _get_valid_sqrt_matrix(corr_matrix)
except:
sqrt_corr = tf.zeros_like(corr_matrix)
sqrt_cov = tf.math.sqrt(sigma_ij) * sqrt_corr
try:
sqrt_cov = _get_valid_sqrt_matrix(cov_matrix)
except:
sqrt_cov = tf.zeros_like(corr_matrix)
print(times[i], times[i+1], sqrt_cov)
normals = tf.linalg.matvec(sqrt_cov, normals)
normals_x = normals[..., :-1]
normals_df = normals[..., -1]
vol_x_t = tf.math.sqrt(tf.nn.relu(tf.transpose(var_x_t)[i]))
# If numerically `vol_x_t == 0`, the gradient of `vol_x_t` becomes `NaN`.
# To prevent this, we explicitly set `vol_x_t` to zero tensor at zero
# values so that the gradient is set to zero at this values.
vol_x_t = tf.where(vol_x_t > 0.0, vol_x_t, 0.0)
next_x = (tf.math.exp(-self._mean_reversion * dt[i])
* current_x
+ tf.transpose(exp_x_t)[i]
+ normals_x)
# Update `rate_paths`
x_paths = utils.maybe_update_along_axis(
tensor=x_paths,
do_update=keep_mask[i + 1],
ind=written_count,
axis=1,
new_tensor=tf.expand_dims(next_x, axis=1))
next_log_df = (current_log_df -
tf.math.reduce_sum(current_x * capital_g, axis=-1) -
log_df_double_integral[i] + normals_df)
# print(2 * log_df_double_integral[i] - y_times_g_squared, times[i])
log_df_paths = utils.maybe_update_along_axis(
tensor=log_df_paths,
do_update=keep_mask[i + 1],
ind=written_count,
axis=1,
new_tensor=tf.expand_dims(next_log_df, axis=1))
written_count += tf.cast(keep_mask[i + 1], dtype=tf.int32)
return (i + 1, written_count, next_x, next_log_df, x_paths, log_df_paths)
x_paths = tf.zeros(
(num_samples, num_requested_times, self._factors), dtype=self._dtype)
log_df_paths = tf.zeros(
(num_samples, num_requested_times), dtype=self._dtype)
written_count = tf.cast(keep_mask[0], dtype=tf.int32)
initial_x = tf.zeros((num_samples, self._factors), dtype=self._dtype)
initial_log_df = tf.zeros((num_samples,), dtype=self._dtype)
_, _, _, _, x_paths, log_df_paths = tf.while_loop(
cond_fn, body_fn, (0, written_count, initial_x, initial_log_df, x_paths, log_df_paths),
maximum_iterations=steps_num,
swap_memory=True)
f_0_t = self._instant_forward_rate_fn(times[1:]) # shape=(num_times,)
rate_paths = tf.math.reduce_sum(
x_paths, axis=-1) + f_0_t # shape=(num_samples, num_times)
p_0_t = self._initial_discount_rate_fn(times[1:])
discount_factor_paths = tf.math.exp(log_df_paths - p_0_t * times[1:])
return (rate_paths, discount_factor_paths, x_paths)
def _conditional_mean_x(self, t):
"""Computes the drift term in [1], Eq. 10.39."""
time_index = tf.searchsorted(self._jump_locations, t)
vn = tf.concat([self._zero_padding, self._jump_locations], axis=0)
zero_padding_2d = tf.zeros((self._factors, self._factors, 1), dtype=self._dtype)
y_at_vol_knots = tf.concat([zero_padding_2d, self.state_y(self._jump_locations)], axis=-1)
# Add a training dimension so that we broadcast along `t`
mr = tf.expand_dims(self._mean_reversion, axis=-1)
# create a matrix k2(i,j) = k(i) + k(j)
mr2 = tf.expand_dims(self._mean_reversion, axis=-1)
# Add a dimension corresponding to `num_times`
mr2 = tf.expand_dims(mr2 + tf.transpose(mr2), axis=-1)
ex_between_vol_knots = self._ex_integral(self._padded_knots,
self._jump_locations,
self._jump_values_vol,
mr, mr2,
y_at_vol_knots[:, :, :-1])
ex_at_vol_knots = tf.concat(
[zero_padding_2d,
utils.cumsum_using_matvec(ex_between_vol_knots)], axis=-1)
c = tf.gather(y_at_vol_knots, time_index, axis=-1)
sigma_t = self._volatility(t)
exp_x_t = self._ex_integral(
tf.gather(vn, time_index, axis=-1), t, sigma_t, mr, mr2, c)
exp_x_t = exp_x_t + tf.gather(ex_at_vol_knots, time_index, axis=-1)
exp_x_t = tf.math.reduce_sum(exp_x_t, axis=1)
exp_x_t = (exp_x_t[:, 1:] - exp_x_t[:, :-1]) * tf.math.exp(-mr * t[1:])
return exp_x_t
def _ex_integral(self, t0, t, vol, k, k2, y_t0):
"""Function computes the integral for the drift calculation."""
# Computes int_t0^t (exp(k*s)*y(s)) ds,
# where y(s)=y(t0) + int_t0^s exp(-k2*(s-u)) vol(u)^2 du."""
vol = tf.expand_dims(vol, axis=-2)
vol_squared = tf.expand_dims(self._rho, axis=-1) * (
vol * tf.transpose(vol, perm=[1, 0, 2]))
value = (
tf.math.exp(k * t) - tf.math.exp(k * t0) + tf.math.exp(k2 * t0) *
(tf.math.exp(-k * t) - tf.math.exp(-k * t0)))
print(t0.shape, t.shape, vol.shape, k.shape, y_t0.shape)
value = value * vol_squared / (k2 * k) + y_t0 * (tf.math.exp(-k * t0) -
tf.math.exp(-k * t)) / k
return value
def _conditional_variance_x(self, t):
"""Computes the variance of x(t), see [1], Eq. 10.41."""
# Add a trailing dimension so that we broadcast along `t`
mr = tf.expand_dims(self._mean_reversion, axis=-1)
# create a matrix k2(i,j) = k(i) + k(j)
mr2 = tf.expand_dims(self._mean_reversion, axis=-1)
# Add a dimension corresponding to `num_times`
mr2 = tf.expand_dims(mr2 + tf.transpose(mr2), axis=-1)
def _integrate_volatility_squared(vol, l_limit, u_limit):
# create sigma2_ij = sigma_i * sigma_j
vol = tf.expand_dims(vol, axis=-2)
vol_squared = tf.expand_dims(self._rho, axis=-1) * (
vol * tf.transpose(vol, perm=[1, 0, 2]))
return vol_squared / mr2 * (tf.math.exp(mr2 * u_limit) - tf.math.exp(
mr2 * l_limit))
var_x_between_vol_knots = _integrate_volatility_squared(
self._jump_values_vol, self._padded_knots, self._jump_locations)
zero_padding_2d = tf.zeros((self._factors, self._factors, 1), dtype=self._dtype)
varx_at_vol_knots = tf.concat(
[zero_padding_2d,
utils.cumsum_using_matvec(var_x_between_vol_knots)], axis=-1)
time_index = tf.searchsorted(self._jump_locations, t)
vn = tf.concat([self._zero_padding, self._jump_locations], axis=0)
var_x_t = _integrate_volatility_squared(
self._volatility(t),tf.gather(vn, time_index), t)
var_x_t = var_x_t + tf.gather(varx_at_vol_knots, time_index, axis=-1)
var_x_t = (var_x_t[:, :, 1:] - var_x_t[:, :, :-1]) * tf.math.exp(
-mr2 * t[1:])
# return tf.math.reduce_sum(var_x_t, axis=-2)
return var_x_t
def _i_double_integral(self, t0, t, vol, ki, kj, k2, y_t0):
"""Double integral for discount factor calculations."""
# Computes int_t0^t (exp(k*s)*y(s)) ds,
# where y(s)=y(t0) + int_t0^s exp(-k2*(s-u)) vol(u)^2 du."""
vol = tf.expand_dims(vol, axis=-2)
vol_squared = tf.expand_dims(self._rho, axis=-1) * (
vol * tf.transpose(vol, perm=[1, 0, 2]))
value = -(1.0 - tf.math.exp(-ki * (t - t0))) / (kj * ki)
value += (1.0 - tf.math.exp(-k2 * (t - t0))) / (kj * k2)
value += (t - t0) / ki
value -= (1.0 - tf.math.exp(-ki * (t - t0))) / (ki * ki)
value = value * vol_squared / k2
#value += y_t0 * ((t - t0) - (1.0 - tf.math.exp(-ki * (t - t0))) / ki) / ki
value2 = tf.math.exp(-kj*t0) * (tf.math.exp(-ki*t0) - tf.math.exp(-ki*t)) / ki
value2 -= (tf.math.exp(-k2*t0) - tf.math.exp(-k2*t)) / k2
value2 = value2 * tf.math.exp(k2*t0) * y_t0 / kj
return value + value2
def _discount_factor_double_integral(self, t):
"""Computes the double integral in Eq 10.42 and 10.43 in Ref. [1]."""
knots = tf.sort(tf.concat([t, self._jump_locations], axis=0))
time_index = tf.searchsorted(knots, t)
vn = tf.concat([self._zero_padding, knots], axis=0)
zero_padding_2d = tf.zeros((self._factors, self._factors, 1), dtype=self._dtype)
y_at_knots = tf.concat([zero_padding_2d, self.state_y(knots)], axis=-1)
mr = tf.expand_dims(self._mean_reversion, axis=-1)
# Add a dimension corresponding to `num_times`
mri = tf.expand_dims(mr, axis=-1)
mrj = tf.expand_dims(tf.transpose(mr), axis=-1)
# create a matrix k2(i,j) = k(i) + k(j)
mr2 = mri + mrj
int_between_knots = self._i_double_integral(
vn[:-1], knots, self._volatility(vn)[:, :-1], mri, mrj, mr2,
y_at_knots[:, :, :-1])
int_at_knots = utils.cumsum_using_matvec(int_between_knots)
int_t = tf.gather(int_at_knots, time_index, axis=-1)
int_t = tf.math.reduce_sum(int_t, axis=[0, 1])
print(tf.reduce_sum(int_between_knots, axis=[0, 1]))
return (int_t[1:] - int_t[:-1])
def _cov_between_x_and_log_df(self, t):
"""Computes covariance between state `x` and log(df) (Eq. )"""
knots = tf.sort(tf.concat([t, self._jump_locations], axis=0))
time_index = tf.searchsorted(knots, t)
vn = tf.concat([self._zero_padding, knots], axis=0)
zero_padding_2d = tf.zeros((self._factors, self._factors, 1), dtype=self._dtype)
mr = tf.expand_dims(self._mean_reversion, axis=-1)
# Add a dimension corresponding to `num_times`
mri = tf.expand_dims(mr, axis=-1)
mrj = tf.expand_dims(tf.transpose(mr), axis=-1)
# create a matrix k2(i,j) = k(i) + k(j)
mr2 = mri + mrj
def _integrate(vol, t0, t1):
# create sigma2_ij = sigma_i * sigma_j
vol = tf.expand_dims(vol, axis=-2)
vol_squared = tf.expand_dims(self._rho, axis=-1) * (
vol * tf.transpose(vol, perm=[1, 0, 2]))
value = -tf.math.exp(mr2 * t0) * (tf.math.exp(-mrj * t0) - tf.math.exp(-mrj * t1)) / mrj
value += (tf.math.exp(mri * t1) - tf.math.exp(mri * t0)) / mri
value = value * vol_squared * tf.math.exp(-mri * t1) / mr2
return value
cov_between_knots = _integrate(
self._volatility(vn)[:, :-1], vn[:-1], knots)
cov_at_knots = utils.cumsum_using_matvec(cov_between_knots)
cov_t = tf.gather(cov_at_knots, time_index, axis=-1)
cov_t = (cov_t[:, :, 1:] - cov_t[:, :, :-1])
return tf.math.reduce_sum(cov_t, axis=-2)
def _exact_discretization_setup(self, dim):
"""Initial setup for efficient computations."""
self._zero_padding = tf.constant([0.0], dtype=self._dtype)
self._jump_locations = tf.sort(tf.reshape(
self._volatility.jump_locations(), [-1]))
self._jump_values_vol = self._volatility(self._jump_locations)
self._padded_knots = tf.concat(
[self._zero_padding, self._jump_locations[:-1]], axis=0)
def _get_valid_sqrt_matrix(rho):
"""Returns a matrix L such that rho = LL^T."""
e, v = tf.linalg.eigh(rho)
def _psd_true():
return tf.linalg.cholesky(rho)
def _psd_false():
print(tf.math.real(e))
realv = tf.math.real(v)
adjusted_e = tf.linalg.diag(tf.maximum(tf.math.real(e), 1e-14))
return tf.matmul(realv, tf.math.sqrt(adjusted_e))
return tf.cond(
tf.math.reduce_any(tf.less(tf.math.real(e), 1e-14)), _psd_false, _psd_true)
###Output
_____no_output_____
###Markdown
model definition
###Code
dtype = np.float64
rate_fn = lambda x: 0.01 * tf.ones_like(x, dtype=dtype)
fn = piecewise.PiecewiseConstantFunc([[0.5, 1.0]], [[0.01, 0.02, 0.01]], dtype=dtype)
fn2 = piecewise.PiecewiseConstantFunc(
[[0.5, 2.0], [0.5, 2.0]], [[0.005, 0.01, 0.015], [0.005, 0.01, 0.015]], dtype=dtype)
fn3 = piecewise.PiecewiseConstantFunc(
[[0.5, 1.0], [0.5, 1.0]], [[0.01, 0.008, 0.005], [0.005, 0.008, 0.005]], dtype=dtype)
def vol_fn(t, r):
return fn([t]) * tf.math.abs(r)**0.5
model = GaussianHJM(dim=2,
mean_reversion=[0.5, 0.5],
volatility=[0.01, 0.01],
initial_discount_rate_fn=rate_fn,
corr_matrix=[[1.0, 0.5], [0.5, 1.0]],
dtype=dtype
)
model2 = GaussianHJM(dim=2,
mean_reversion=[0.15, 0.03],
volatility=[0.015, 0.01],
initial_discount_rate_fn=rate_fn,
# corr_matrix=[[1.0, 0.5], [0.5, 1.0]],
dtype=dtype
)
model1 = GaussianHJM(dim=1,
mean_reversion=[0.03],
volatility=fn,
initial_discount_rate_fn=rate_fn,
corr_matrix=None,
dtype=dtype
)
###Output
_____no_output_____
###Markdown
Test E(x) Test double integral
###Code
from scipy.integrate import quad
k = 0.015
v = 0.01
def _intfun1(x, t0):
xt = tf.convert_to_tensor([x], dtype=dtype)
return np.exp(k*x) * tf.reduce_sum(model.state_y(xt)).numpy()
yt0 = tf.math.reduce_sum(model.state_y([t0])).numpy()
ff = lambda u: 2*np.exp(-2*k*(x - u))*v**2
aa, _ = quad(ff, t0, x)
return np.exp(k*x) *(yt0 + aa)
def _intfun2(x, t0):
val = quad(_intfun1, t0, x, args=(t0))
return np.exp(-k*x) * val[0]
for l in range(0, 10):
u = l + 1
print(l,u, quad(_intfun2, l, u, args=(l)))
from scipy.integrate import quad
k = 0.015
v = 0.01
def _intfun1(x, t0, t):
xt = tf.convert_to_tensor([x], dtype=dtype)
return np.exp(-k*t) * np.exp(k*x) * tf.reduce_sum(model.state_y(xt), axis=[1, 2]).numpy()[0]
for l in range(0, 10):
u = l + 1
print(l,u, quad(_intfun1, l, u, args=(l, u)))
tt = tf.convert_to_tensor(np.arange(0, 11), dtype=dtype)
model._conditional_mean_x(tt)
###Output
0 1 (5.48858072538982e-05, 6.093548693841622e-19)
1 2 (0.00011430185068066105, 1.2690054638293652e-18)
2 3 (0.00013615979153311673, 1.5116773558822763e-18)
3 4 (0.00014420087859907658, 1.6009513559187182e-18)
4 5 (0.0001471590292153128, 1.6337934251632635e-18)
5 6 (0.0001482472720109147, 1.6458753472438602e-18)
6 7 (0.00014864761416241959, 1.6503200379871472e-18)
7 8 (0.0001487948918093926, 1.651955148333968e-18)
8 9 (0.00014884907222785805, 1.6525566718146095e-18)
9 10 (0.00014886900408992554, 1.6527779599365194e-18)
(1,) (0,) (2, 1, 0) (2, 1) (2, 2, 0)
(11,) (11,) (2, 1, 11) (2, 1) (2, 2, 11)
###Markdown
Test covariance
###Code
from scipy.integrate import quad
k = 0.015
v = 0.01
def _intfun1(x, t0):
return np.exp(2*k*x)
def _intfun2(x, t0, t):
val = quad(_intfun1, t0, x, args=(t0))
return np.exp(-k*x) * val[0] * v**2 * np.exp(-k*t)
for l in range(0, 10):
u = l + 1
print(l, u, quad(_intfun2, l, u, args=(l, u)))
###Output
_____no_output_____
###Markdown
Test rate sims
###Code
dt_0 = 0.05
times_0 = np.arange(dt_0, 10 + dt_0, dt_0, dtype=np.float64)# np.array([0., 0.5, 1.0, 10])
curve_times = np.array([0., 0.5, 1.0, 5.0, 10.0])
num_samples = 100000
rpaths_0, paths_0, xn_0, _ = model.sample_paths(times=times_0,
num_samples=num_samples,
random_type=random.RandomType.STATELESS_ANTITHETIC,
seed=[0,0],
time_step=dt_0,
use_euler_sampling=True)
dt = 1.0
times = np.arange(dt, 10 + dt, dt, dtype=np.float64)# np.array([0., 0.5, 1.0, 10])
curve_times = np.array([0., 0.5, 1.0, 5.0, 10.0])
num_samples = 100000
rpaths, paths, xn, _ = model.sample_paths(times=times,
num_samples=num_samples,
random_type=random.RandomType.STATELESS_ANTITHETIC,
seed=[0,0],
time_step=0.1,
use_euler_sampling=False)
t = tf.convert_to_tensor([0, 5, 10], dtype=dtype)
# model._conditional_variance_x(t)
# model._discount_factor_double_integral(times)
model._cov_between_x_and_log_df(times)
paths.shape, rpaths_0.shape, rpaths.shape
import matplotlib.pyplot as plt
dt = np.concatenate([[0], times_0[1:] - times_0[:-1]], axis=0)
p_sim = np.mean(np.exp(-np.cumsum(rpaths_0 * dt, axis=1)), axis=0)
p_sim_df = tf.math.reduce_mean(paths, axis=0)
p_true = np.exp(-times * rate_fn(times))
plt.plot(times_0, p_sim, label='E(e(-rdt))')
plt.plot(times, p_sim_df, label='E(df)')
plt.plot(times, p_true, label='P(0,t)')
plt.legend()
plt.show()
p_true, p_sim_df
dt = np.concatenate([[0], times_0[1:] - times_0[:-1]], axis=0)
p_sim = np.std(np.exp(-np.cumsum(rpaths_0*dt, axis=1)), axis=0)
p_sim_df = tf.math.reduce_std(paths, axis=0)
plt.plot(times_0, p_sim, label='std(e(-rdt))')
plt.plot(times, p_sim_df, label='std(df)')
plt.legend()
plt.show()
dt = np.concatenate([[0], times[1:] - times[:-1]], axis=0)
rdf = -np.cumsum(rpaths*dt, axis=1)
df = np.log(paths)
p_sim, p_sim_df = (np.zeros((df.shape[1])), np.zeros((df.shape[1])))
for i, _ in enumerate(p_sim):
p_sim[i] = np.corrcoef(rdf[:, i], xn[:, i, 1])[0][1]
p_sim_df[i] = np.corrcoef(df[:, i], xn[:, i, 1])[0][1]
plt.plot(times, p_sim, label='std(e(-rdt))')
plt.plot(times, p_sim_df, label='std(df)')
plt.legend()
plt.show()
dt = np.concatenate([[0], times[1:] - times[:-1]], axis=0)
rdf = -np.cumsum(rpaths*dt, axis=1)
df = np.log(paths)
p_sim, p_sim_df = (np.zeros((df.shape[1])), np.zeros((df.shape[1])))
for i, _ in enumerate(p_sim):
p_sim[i] = np.corrcoef(rdf[:, i], xn[:, i, 1])[0][1]
p_sim_df[i] = np.corrcoef(df[:, i], xn[:, i, 1])[0][1]
plt.plot(times, p_sim, label='std(e(-rdt))')
plt.plot(times, p_sim_df, label='std(df)')
plt.legend()
plt.show()
idx = 50
np.cov(pp[:, idx] - pp[:, idx-1], xn[:, idx, 0].numpy() - xn[:, idx-1, 0].numpy())
###Output
_____no_output_____
###Markdown
Test Bond sims
###Code
p_t_tau, _, _ = model.sample_discount_curve_paths(
times=times,
curve_times=curve_times,
num_samples=num_samples,
time_step=0.1)
print(p_t_tau.shape)
p_t_tau.shape
def bond_std(t, T, v, k):
eT = np.exp(-k*T)
et = np.exp(k*t)
#return np.sqrt(v**2/k**2 *(t-eT**2*(et**2-1.)/2/k+2*eT*(et - 1)/k))
val = v/k * (1. - eT*et) * np.sqrt((1.-1./et/et)/k/2)
print (val)
return val
d = tf.math.reduce_std(tf.math.log(p_t_tau), axis=0)
tidx = 3
plt.plot(d[:,tidx])
plt.plot(bond_std(times[tidx], curve_times + times[tidx], 0.01, 0.03))
d = tf.math.reduce_std(tf.math.log(p_t_tau), axis=0)
tidx = 2
plt.plot(d[:,tidx])
plt.plot(bond_std(times[tidx], curve_times + times[tidx],
np.sqrt(0.005**2+0.008**2+2*0.5*0.005*0.008), 0.03))
aa = tf.convert_to_tensor([1,2,3])
tf.reverse(tf.expand_dims(aa, axis=0), [1])[0]
###Output
_____no_output_____
###Markdown
Discount bond price
###Code
model.state_y([5.0]), model2.state_y([5.0])
print(['%0.16f'% x for x in np.array([1, 2])])
###Output
['1.0000000000000000', '2.0000000000000000']
###Markdown
Swaption pricing
###Code
import enum
from tf_quant_finance.math import pde
from tf_quant_finance.models import utils
from tf_quant_finance.models import valuation_method as vm
from tf_quant_finance.models.hjm import gaussian_hjm
from tf_quant_finance.models.hjm import quasi_gaussian_hjm
from tf_quant_finance.models.hjm import swaption_util
@enum.unique
class ValuationMethod(enum.Enum):
"""Swaption valuation methods.
* `ANALYTIC`: Analytic valuation.
* `MONTE_CARLO`: Valuation using Monte carlo simulations.
* `FINITE_DIFFERENCE`: Valuation using finite difference.
"""
ANALYTIC = 1
MONTE_CARLO = 2
FINITE_DIFFERENCE = 3
# Points smaller than this are merged together in FD time grid
_PDE_TIME_GRID_TOL = 1e-7
def price(*,
expiries,
fixed_leg_payment_times,
fixed_leg_daycount_fractions,
fixed_leg_coupon,
reference_rate_fn,
num_hjm_factors,
mean_reversion,
volatility,
times=None,
time_step=None,
num_time_steps=None,
curve_times=None,
corr_matrix=None,
notional=None,
is_payer_swaption=None,
valuation_method=vm.ValuationMethod.MONTE_CARLO,
num_samples=1,
random_type=None,
seed=None,
skip=0,
time_step_finite_difference=None,
num_time_steps_finite_difference=None,
num_grid_points_finite_difference=101,
dtype=None,
name=None):
"""Calculates the price of European swaptions using the HJM model.
A European Swaption is a contract that gives the holder an option to enter a
swap contract at a future date at a prespecified fixed rate. A swaption that
grants the holder the right to pay fixed rate and receive floating rate is
called a payer swaption while the swaption that grants the holder the right to
receive fixed and pay floating payments is called the receiver swaption.
Typically the start date (or the inception date) of the swap coincides with
the expiry of the swaption. Mid-curve swaptions are currently not supported
(b/160061740).
This implementation uses the HJM model to numerically value the swaption via
Monte-Carlo. For more information on the formulation of the HJM model, see
quasi_gaussian_hjm.py.
#### Example
````python
import numpy as np
import tensorflow.compat.v2 as tf
import tf_quant_finance as tff
dtype = tf.float64
# Price 1y x 1y swaption with quarterly payments using Monte Carlo
# simulations.
expiries = np.array([1.0])
fixed_leg_payment_times = np.array([1.25, 1.5, 1.75, 2.0])
fixed_leg_daycount_fractions = 0.25 * np.ones_like(fixed_leg_payment_times)
fixed_leg_coupon = 0.011 * np.ones_like(fixed_leg_payment_times)
zero_rate_fn = lambda x: 0.01 * tf.ones_like(x, dtype=dtype)
mean_reversion = [0.03]
volatility = [0.02]
price = tff.models.hjm.swaption_price(
expiries=expiries,
fixed_leg_payment_times=fixed_leg_payment_times,
fixed_leg_daycount_fractions=fixed_leg_daycount_fractions,
fixed_leg_coupon=fixed_leg_coupon,
reference_rate_fn=zero_rate_fn,
notional=100.,
num_hjm_factors=1,
mean_reversion=mean_reversion,
volatility=volatility,
valuation_method=tff.model.ValuationMethod.MONTE_CARLO,
num_samples=500000,
time_step=0.1,
random_type=tff.math.random.RandomType.STATELESS_ANTITHETIC,
seed=[1, 2])
# Expected value: [[0.716]]
````
#### References:
[1]: D. Brigo, F. Mercurio. Interest Rate Models-Theory and Practice.
Second Edition. 2007. Section 6.7, page 237.
Args:
expiries: A real `Tensor` of any shape and dtype. The time to expiration of
the swaptions. The shape of this input determines the number (and shape)
of swaptions to be priced and the shape of the output.
fixed_leg_payment_times: A real `Tensor` of the same dtype as `expiries`.
The payment times for each payment in the fixed leg. The shape of this
input should be `expiries.shape + [n]` where `n` denotes the number of
fixed payments in each leg. The `fixed_leg_payment_times` should be
greater-than or equal-to the corresponding expiries.
fixed_leg_daycount_fractions: A real `Tensor` of the same dtype and
compatible shape as `fixed_leg_payment_times`. The daycount fractions for
each payment in the fixed leg.
fixed_leg_coupon: A real `Tensor` of the same dtype and compatible shape as
`fixed_leg_payment_times`. The fixed rate for each payment in the fixed
leg.
reference_rate_fn: A Python callable that accepts expiry time as a real
`Tensor` and returns a `Tensor` of shape `input_shape +
[num_hjm_factors]`. Returns the continuously compounded zero rate at the
present time for the input expiry time.
num_hjm_factors: A Python scalar which corresponds to the number of factors
in the HJM model to be used for pricing.
mean_reversion: A real positive `Tensor` of shape `[num_hjm_factors]`.
Corresponds to the mean reversion rate of each factor.
volatility: A real positive `Tensor` of the same `dtype` and shape as
`mean_reversion` or a callable with the following properties: (a) The
callable should accept a scalar `Tensor` `t` and a 1-D `Tensor` `r(t)`
of shape `[num_samples]` and returns a 2-D `Tensor` of shape
`[num_samples, num_hjm_factors]`. The variable `t` stands for time and
`r(t)` is the short rate at time `t`. The function returns the
instantaneous volatility `sigma(t) = sigma(t, r(t))`. When `volatility`
is specified as a real `Tensor`, each factor is assumed to have a
constant instantaneous volatility and the model is effectively a
Gaussian HJM model. Corresponds to the instantaneous volatility of each
factor.
times: An optional rank 1 `Tensor` of increasing positive real values. The
times at which Monte Carlo simulations are performed. Relevant when
swaption valuation is done using Monte Calro simulations.
Default value: `None` in which case simulation times are computed based
on either `time_step` or `num_time_steps` inputs.
time_step: Optional scalar real `Tensor`. Maximal distance between time
grid points in Euler scheme. Relevant when Euler scheme is used for
simulation. This input or `num_time_steps` are required when valuation
method is Monte Carlo.
Default Value: `None`.
num_time_steps: An optional scalar integer `Tensor` - a total number of
time steps during Monte Carlo simulations. The maximal distance betwen
points in grid is bounded by
`times[-1] / (num_time_steps - times.shape[0])`.
Either this or `time_step` should be supplied when the valuation method
is Monte Carlo.
Default value: `None`.
curve_times: An optional rank 1 `Tensor` of positive real values. The
maturities at which spot discount curve is computed during simulations.
Default value: `None` in which case `curve_times` is computed based on
swaption expities and `fixed_leg_payments_times` inputs.
corr_matrix: A `Tensor` of shape `[num_hjm_factors, num_hjm_factors]` and
the same `dtype` as `mean_reversion`. Specifies the correlation between
HJM factors.
Default value: `None` in which case the factors are assumed to be
uncorrelated.
notional: An optional `Tensor` of same dtype and compatible shape as
`strikes`specifying the notional amount for the underlying swaps.
Default value: None in which case the notional is set to 1.
is_payer_swaption: A boolean `Tensor` of a shape compatible with `expiries`.
Indicates whether the swaption is a payer (if True) or a receiver (if
False) swaption. If not supplied, payer swaptions are assumed.
valuation_method: An enum of type `ValuationMethod` specifying
the method to be used for swaption valuation. Currently the valuation is
supported using `MONTE_CARLO` and `FINITE_DIFFERENCE` methods. Valuation
using finite difference is only supported for Gaussian HJM models, i.e.
for models with constant mean-reversion rate and time-dependent
volatility.
Default value: `ValuationMethod.MONTE_CARLO`, in which case
swaption valuation is done using Monte Carlo simulations.
num_samples: Positive scalar `int32` `Tensor`. The number of simulation
paths during Monte-Carlo valuation. This input is ignored during analytic
valuation.
Default value: The default value is 1.
random_type: Enum value of `RandomType`. The type of (quasi)-random number
generator to use to generate the simulation paths. This input is relevant
only for Monte-Carlo valuation and ignored during analytic valuation.
Default value: `None` which maps to the standard pseudo-random numbers.
seed: Seed for the random number generator. The seed is only relevant if
`random_type` is one of `[STATELESS, PSEUDO, HALTON_RANDOMIZED,
PSEUDO_ANTITHETIC, STATELESS_ANTITHETIC]`. For `PSEUDO`,
`PSEUDO_ANTITHETIC` and `HALTON_RANDOMIZED` the seed should be an Python
integer. For `STATELESS` and `STATELESS_ANTITHETIC` must be supplied as
an integer `Tensor` of shape `[2]`. This input is relevant only for
Monte-Carlo valuation and ignored during analytic valuation.
Default value: `None` which means no seed is set.
skip: `int32` 0-d `Tensor`. The number of initial points of the Sobol or
Halton sequence to skip. Used only when `random_type` is 'SOBOL',
'HALTON', or 'HALTON_RANDOMIZED', otherwise ignored.
Default value: `0`.
time_step_finite_difference: Optional scalar real `Tensor`. Spacing between
time grid points in finite difference discretization. This input is only
relevant for valuation using finite difference.
Default value: `None`. If `num_time_steps_finite_difference` is also
unspecified then a `time_step` corresponding to 100 discretization steps
is used.
num_time_steps_finite_difference: Optional scalar real `Tensor`. Number of
time grid points in finite difference discretization. This input is only
relevant for valuation using finite difference.
Default value: `None`. If `time_step_finite_difference` is also
unspecified, then 100 time steps are used.
num_grid_points_finite_difference: Optional scalar real `Tensor`. Number of
spatial grid points per dimension. Currently, we construct an uniform grid
for spatial discretization. This input is only relevant for valuation
using finite difference.
Default value: 101.
dtype: The default dtype to use when converting values to `Tensor`s.
Default value: `None` which means that default dtypes inferred by
TensorFlow are used.
name: Python string. The name to give to the ops created by this function.
Default value: `None` which maps to the default name `hjm_swaption_price`.
Returns:
A `Tensor` of real dtype and shape expiries.shape + [1]
containing the computed swaption prices. For swaptions that have reset in
the past (expiries<0), the function sets the corresponding option prices to
0.0.
"""
# TODO(b/160061740): Extend the functionality to support mid-curve swaptions.
name = name or 'hjm_swaption_price'
with tf.name_scope(name):
expiries = tf.convert_to_tensor(expiries, dtype=dtype, name='expiries')
dtype = dtype or expiries.dtype
fixed_leg_payment_times = tf.convert_to_tensor(
fixed_leg_payment_times, dtype=dtype, name='fixed_leg_payment_times')
fixed_leg_daycount_fractions = tf.convert_to_tensor(
fixed_leg_daycount_fractions,
dtype=dtype,
name='fixed_leg_daycount_fractions')
fixed_leg_coupon = tf.convert_to_tensor(
fixed_leg_coupon, dtype=dtype, name='fixed_leg_coupon')
notional = tf.convert_to_tensor(notional, dtype=dtype, name='notional')
notional = tf.expand_dims(
tf.broadcast_to(notional, expiries.shape), axis=-1)
if is_payer_swaption is None:
is_payer_swaption = True
is_payer_swaption = tf.convert_to_tensor(
is_payer_swaption, dtype=tf.bool, name='is_payer_swaption')
output_shape = expiries.shape.as_list() + [1]
# Add a dimension corresponding to multiple cashflows in a swap
if expiries.shape.rank == fixed_leg_payment_times.shape.rank - 1:
expiries = tf.expand_dims(expiries, axis=-1)
elif expiries.shape.rank < fixed_leg_payment_times.shape.rank - 1:
raise ValueError('Swaption expiries not specified for all swaptions '
'in the batch. Expected rank {} but received {}.'.format(
fixed_leg_payment_times.shape.rank - 1,
expiries.shape.rank))
# Expected shape: batch_shape + [m], where m is the number of fixed leg
# payments per underlying swap. This is the same as
# fixed_leg_payment_times.shape
#
# We need to explicitly use tf.repeat because we need to price
# batch_shape + [m] bond options with different strikes along the last
# dimension.
expiries = tf.repeat(
expiries, tf.shape(fixed_leg_payment_times)[-1], axis=-1)
if valuation_method == vm.ValuationMethod.FINITE_DIFFERENCE:
model = gaussian_hjm.GaussianHJM(
num_hjm_factors,
mean_reversion=mean_reversion,
volatility=volatility,
initial_discount_rate_fn=reference_rate_fn,
corr_matrix=corr_matrix,
dtype=dtype)
batch_shape = expiries.shape.as_list()[:-1] or [1]
return _bermudan_swaption_fd(
batch_shape,
model,
# Add a dimension to denote ONE exercise date
tf.expand_dims(expiries, axis=-2),
fixed_leg_payment_times,
fixed_leg_daycount_fractions,
fixed_leg_coupon,
notional,
is_payer_swaption,
time_step_finite_difference,
num_time_steps_finite_difference,
num_grid_points_finite_difference,
name + '_fd',
dtype)
elif valuation_method == vm.ValuationMethod.MONTE_CARLO:
# Monte-Carlo pricing
model = quasi_gaussian_hjm.QuasiGaussianHJM(
num_hjm_factors,
mean_reversion=mean_reversion,
volatility=volatility,
initial_discount_rate_fn=reference_rate_fn,
corr_matrix=corr_matrix,
dtype=dtype)
return _european_swaption_mc(
output_shape, model, expiries, fixed_leg_payment_times,
fixed_leg_daycount_fractions, fixed_leg_coupon, notional,
is_payer_swaption, times, time_step, num_time_steps, curve_times,
num_samples, random_type, skip, seed, dtype, name + '_mc')
else:
raise ValueError('Swaption Valuation using {} is not supported'.format(
str(valuation_method)))
def _european_swaption_mc(output_shape, model, expiries,
fixed_leg_payment_times, fixed_leg_daycount_fractions,
fixed_leg_coupon, notional, is_payer_swaption, times,
time_step, num_time_steps, curve_times, num_samples,
random_type, skip, seed, dtype, name):
"""Price European swaptions using Monte-Carlo."""
with tf.name_scope(name):
if (times is None) and (time_step is None) and (num_time_steps is None):
raise ValueError(
'One of `times`, `time_step` or `num_time_steps` must be '
'provided for simulation based swaption valuation.')
def _sample_discount_curve_path_fn(times, curve_times, num_samples):
p_t_tau, r_t, df = model.sample_discount_curve_paths(
times=times,
curve_times=curve_times,
num_samples=num_samples,
random_type=random_type,
time_step=time_step,
num_time_steps=num_time_steps,
seed=seed,
skip=skip)
p_t_tau = tf.expand_dims(p_t_tau, axis=-1)
r_t = tf.expand_dims(r_t, axis=-1)
df = tf.expand_dims(df, axis=-1)
return p_t_tau, r_t, df
payoff_discount_factors, payoff_bond_price = (
swaption_util.discount_factors_and_bond_prices_from_samples(
expiries=expiries,
payment_times=fixed_leg_payment_times,
sample_discount_curve_paths_fn=_sample_discount_curve_path_fn,
num_samples=num_samples,
times=times,
curve_times=curve_times,
dtype=dtype))
# Add an axis corresponding to `dim`
fixed_leg_pv = tf.expand_dims(
fixed_leg_coupon * fixed_leg_daycount_fractions,
axis=-1) * payoff_bond_price
# Sum fixed coupon payments within each swap.
# Here, axis=-2 is the payments axis - i.e. summing over all payments; and
# the last axis is the `dim` axis, as explained in comment above
# `fixed_leg_pv` (Note that for HJM the dim of this axis is 1 always).
fixed_leg_pv = tf.math.reduce_sum(fixed_leg_pv, axis=-2)
float_leg_pv = 1.0 - payoff_bond_price[..., -1, :]
payoff_swap = payoff_discount_factors[..., -1, :] * (
float_leg_pv - fixed_leg_pv)
payoff_swap = tf.where(is_payer_swaption, payoff_swap, -1.0 * payoff_swap)
payoff_swaption = tf.math.maximum(payoff_swap, 0.0)
option_value = tf.reshape(
tf.math.reduce_mean(payoff_swaption, axis=0), output_shape)
return notional * option_value
def _bermudan_swaption_fd(batch_shape, model, exercise_times,
fixed_leg_payment_times, fixed_leg_daycount_fractions,
fixed_leg_coupon, notional, is_payer_swaption,
time_step_fd, num_time_steps_fd, num_grid_points_fd,
name, dtype):
"""Price Bermudan swaptions using finite difference."""
with tf.name_scope(name):
dim = model.dim()
x_min = -0.5
x_max = 0.5
# grid.shape = (num_grid_points,2)
grid = pde.grids.uniform_grid(
minimums=[x_min] * dim,
maximums=[x_max] * dim,
sizes=[num_grid_points_fd] * dim,
dtype=dtype)
# TODO(b/186876306): Remove dynamic shapes.
pde_time_grid, pde_time_grid_dt = _create_pde_time_grid(
exercise_times, time_step_fd, num_time_steps_fd, dtype)
maturities, unique_maturities, maturities_shape = (
_create_termstructure_maturities(fixed_leg_payment_times))
num_maturities = tf.shape(unique_maturities)[-1]
x_meshgrid = _coord_grid_to_mesh_grid(grid)
meshgrid_shape = tf.shape(x_meshgrid)
broadcasted_maturities = tf.expand_dims(unique_maturities, axis=0)
num_grid_points = tf.math.reduce_prod(meshgrid_shape[1:])
shape_to_broadcast = tf.concat(
[meshgrid_shape, [num_maturities]], axis=0)
# Reshape `state_x`, `maturities` to (num_grid_points, num_maturities)
state_x = tf.expand_dims(x_meshgrid, axis=-1)
state_x = tf.broadcast_to(state_x, shape_to_broadcast)
broadcasted_maturities = tf.broadcast_to(
broadcasted_maturities, shape_to_broadcast[1:])
def _get_swap_payoff(payoff_time):
broadcasted_exercise_times = tf.broadcast_to(
payoff_time, shape_to_broadcast[1:])
# Zero-coupon bond curve
zcb_curve = model.discount_bond_price(
tf.transpose(
tf.reshape(
state_x, [dim, num_grid_points * num_maturities])),
tf.reshape(broadcasted_exercise_times, [-1]),
tf.reshape(broadcasted_maturities, [-1]))
zcb_curve = tf.reshape(
zcb_curve, [num_grid_points, num_maturities])
maturities_index = tf.searchsorted(
unique_maturities, tf.reshape(maturities, [-1]))
zcb_curve = tf.gather(zcb_curve, maturities_index, axis=-1)
# zcb_curve.shape = [num_grid_points] + [maturities_shape]
zcb_curve = tf.reshape(
zcb_curve, tf.concat([[num_grid_points], maturities_shape], axis=0))
# Shape after reduce_sum =
# (num_grid_points, batch_shape)
fixed_leg = tf.math.reduce_sum(
fixed_leg_coupon * fixed_leg_daycount_fractions * zcb_curve, axis=-1)
float_leg = 1.0 - zcb_curve[..., -1]
payoff_swap = float_leg - fixed_leg
payoff_swap = tf.where(is_payer_swaption, payoff_swap, -payoff_swap)
return tf.reshape(tf.transpose(
payoff_swap), tf.concat([batch_shape, meshgrid_shape[1:]], axis=0))
def _get_index(t, tensor_to_search):
t = tf.expand_dims(t, axis=-1)
index = tf.searchsorted(tensor_to_search, t - _PDE_TIME_GRID_TOL, 'right')
y = tf.gather(tensor_to_search, index)
return tf.where(tf.math.abs(t - y) < _PDE_TIME_GRID_TOL, index, -1)[0]
sum_x_meshgrid = tf.math.reduce_sum(x_meshgrid, axis=0)
def _is_exercise_time(t):
return tf.reduce_any(tf.where(
tf.math.abs(exercise_times[..., -1] - t) < _PDE_TIME_GRID_TOL,
True, False), axis=-1)
def _discounting_fn(t, grid):
del grid
f_0_t = (model._instant_forward_rate_fn(t)) # pylint: disable=protected-access
return sum_x_meshgrid + f_0_t
def _final_value():
t = pde_time_grid[-1]
payoff_swap = tf.nn.relu(_get_swap_payoff(t))
is_ex_time = _is_exercise_time(t)
return tf.where(tf.reshape(is_ex_time, tf.concat(
[batch_shape, [1] * dim], axis=0)), payoff_swap, 0.0)
def _values_transform_fn(t, grid, value_grid):
zero = tf.zeros_like(value_grid)
is_ex_time = _is_exercise_time(t)
def _at_least_one_swaption_pays():
payoff_swap = tf.nn.relu(_get_swap_payoff(t))
return tf.where(tf.reshape(is_ex_time, tf.concat(
[batch_shape, [1] * dim], axis=0)), payoff_swap, zero)
v_star = tf.cond(tf.math.reduce_any(is_ex_time),
_at_least_one_swaption_pays, lambda: zero)
return grid, tf.maximum(value_grid, v_star)
# TODO(b/186876306): Use piecewise constant func here.
def _pde_time_step(t):
index = _get_index(t, pde_time_grid)
cond = lambda i, dt: tf.math.logical_and(dt < _PDE_TIME_GRID_TOL, i > -1)
def _fn(i, dt):
del dt
return i + 1, pde_time_grid_dt[i + 1]
_, dt = tf.while_loop(cond, _fn, (index, pde_time_grid_dt[index]))
return dt
# Use default boundary conditions, d^2V/dx_i^2 = 0
boundary_conditions = [(None, None) for i in range(dim)]
# res[0] contains the swaption prices.
# res[0].shape = batch_shape + [num_grid_points] * dim
res = model.fd_solver_backward(
pde_time_grid[-1],
0.0,
grid,
values_grid=_final_value(),
time_step=_pde_time_step,
boundary_conditions=boundary_conditions,
values_transform_fn=_values_transform_fn,
discounting=_discounting_fn,
dtype=dtype)
idx = tf.searchsorted(
tf.convert_to_tensor(grid),
tf.expand_dims(tf.convert_to_tensor([0.0] * dim, dtype=dtype), axis=-1))
# idx.shape = (dim, 1)
idx = tf.squeeze(idx) if dim > 1 else tf.expand_dims(idx, axis=-1)
option_value = res[0]
for i in range(dim - 1, -1, -1):
option_value = tf.gather(option_value, idx[i], axis=-1)
# output_shape = batch_shape + [1]
return notional * tf.expand_dims(
tf.reshape(option_value, batch_shape), axis=-1)
def _coord_grid_to_mesh_grid(coord_grid):
if len(coord_grid) == 1:
return tf.expand_dims(coord_grid[0], 0)
x_meshgrid = tf.stack(values=tf.meshgrid(*coord_grid, indexing='ij'), axis=-1)
perm = [len(coord_grid)] + list(range(len(coord_grid)))
return tf.transpose(x_meshgrid, perm=perm)
def _create_pde_time_grid(exercise_times, time_step_fd, num_time_steps_fd,
dtype):
"""Create PDE time grid."""
with tf.name_scope('create_pde_time_grid'):
exercise_times, _ = tf.unique(tf.reshape(exercise_times, shape=[-1]))
if num_time_steps_fd is not None:
num_time_steps_fd = tf.convert_to_tensor(
num_time_steps_fd, dtype=tf.int32, name='num_time_steps_fd')
time_step_fd = tf.math.reduce_max(exercise_times) / tf.cast(
num_time_steps_fd, dtype=dtype)
if time_step_fd is None and num_time_steps_fd is None:
num_time_steps_fd = 100
pde_time_grid, _, _ = utils.prepare_grid(
times=exercise_times,
time_step=time_step_fd,
dtype=dtype,
num_time_steps=num_time_steps_fd)
pde_time_grid_dt = pde_time_grid[1:] - pde_time_grid[:-1]
pde_time_grid_dt = tf.concat([[100.0], pde_time_grid_dt], axis=-1)
return pde_time_grid, pde_time_grid_dt
def _create_termstructure_maturities(fixed_leg_payment_times):
"""Create maturities needed for termstructure simulations."""
with tf.name_scope('create_termstructure_maturities'):
maturities = fixed_leg_payment_times
maturities_shape = tf.shape(maturities)
# We should eventually remove tf.unique, but keeping it for now because
# PDE solvers are not xla compatible in TFF currently.
unique_maturities, _ = tf.unique(tf.reshape(maturities, shape=[-1]))
unique_maturities = tf.sort(unique_maturities, name='sort_maturities')
return maturities, unique_maturities, maturities_shape
###Output
_____no_output_____
###Markdown
Example
###Code
swaption_price = price
dtype = tf.float64
expiries = np.array([1.002739726, 2.002739726])
fixed_leg_payment_times = np.array([[1.249315068, 1.498630137, 1.750684932, 2.002739726],
[2.249315068, 2.498630137, 2.750684932, 3.002739726]])
fixed_leg_daycount_fractions = np.array([[0.2465753425, 0.2493150685, 0.2520547945, 0.2520547945],
[0.2465753425, 0.2493150685, 0.2520547945, 0.2520547945]])
fixed_leg_coupon = 0.011 * np.ones_like(fixed_leg_payment_times)
zero_rate_fn = lambda x: 0.01 * tf.ones_like(x, dtype=dtype)
mu = [0.03, 0.15]
vol = [0.01, 0.015]
hjm_price = swaption_price(
expiries=expiries,
fixed_leg_payment_times=fixed_leg_payment_times,
fixed_leg_daycount_fractions=fixed_leg_daycount_fractions,
fixed_leg_coupon=fixed_leg_coupon,
reference_rate_fn=zero_rate_fn,
notional=100.,
num_hjm_factors=len(mu),
mean_reversion=mu,
volatility=vol,
# corr_matrix=[[1, 0.0], [0.0, 1]],
valuation_method=vm.ValuationMethod.FINITE_DIFFERENCE,
time_step_finite_difference=0.05,
num_grid_points_finite_difference=251,
time_step=0.05,
num_samples=1000000,
dtype=dtype)
hjm_price
###Output
_____no_output_____
###Markdown
Using quantlib
###Code
import QuantLib as ql
from QuantLib import *
frequency_enum, settle_date = 4, Date(1, 1, 2020)
expiry_date = Date(1,1,2022)
midcurve_expiry_date = Date(1,7,2020)
maturity_date = Date(1, 1, 2023)
face_amount = 100.0
settlement_days = 0
fixing_days = 0
calendar = NullCalendar()
todays_date = Date(1,1,2020)
Settings.instance().evaluationDate = todays_date
spotDates = [ql.Date(1, 1, 2020), ql.Date(1, 1, 2021), ql.Date(1, 1, 2022), ql.Date(1, 1, 2030)]
spotRates = [0.01, 0.01, 0.01, 0.01]
dayCount = ql.Actual365Fixed()
calendar = ql.UnitedStates()
interpolation = ql.Linear()
compounding = ql.Compounded
compoundingFrequency = ql.Continuous
spotCurve = ql.ZeroCurve(spotDates, spotRates, dayCount)
spotCurveHandle = ql.YieldTermStructureHandle(spotCurve)
index = IborIndex('USD Libor', Period(3, Months), settlement_days, USDCurrency(), NullCalendar(),
Unadjusted, False, Actual365Fixed(), spotCurveHandle)
#index.addFixing(ql.Date(15,1,2021), 0.006556)
schedule = Schedule(expiry_date,
maturity_date, Period(frequency_enum),
NullCalendar(),
Unadjusted, Unadjusted,
DateGeneration.Forward, False)
strike = 0.011
ibor_leg = ql.IborLeg([face_amount], schedule, index)
fixed_leg = ql.FixedRateLeg(schedule, Actual365Fixed(), [face_amount], [strike])
swap = ql.VanillaSwap(ql.VanillaSwap.Payer, face_amount, schedule, strike, Actual365Fixed(), schedule, index, 0.0, Actual365Fixed())
exercise_date = ql.EuropeanExercise(expiry_date)
swaption = ql.Swaption(swap, exercise_date)
# price using hull-white
model = ql.HullWhite(spotCurveHandle, a=0.03, sigma=0.02)
engine = ql.JamshidianSwaptionEngine(model, spotCurveHandle)
swaption.setPricingEngine(engine)
print("price using HW", swaption.NPV())
# price using G2
model = ql.G2(spotCurveHandle, a=0.03, sigma=0.01, b=0.15, eta=0.015, rho=0.0)
engine = ql.FdG2SwaptionEngine(model, 20, 500, 500)
swaption.setPricingEngine(engine)
print("price using G2", swaption.NPV())
# additional debugging
swap.setPricingEngine(ql.DiscountingSwapEngine(spotCurveHandle))
swap.fairRate()
###Output
price using HW 1.007148261731575
price using G2 0.8029012153434956
###Markdown
Example - Time dependent vol
###Code
swaption_price = price
dtype = tf.float64
expiries = np.array([1.002739726, 1.002739726])
fixed_leg_payment_times = np.array([[1.249315068, 1.498630137, 1.750684932, 2.002739726],
[1.249315068, 1.498630137, 1.750684932, 2.002739726]])
fixed_leg_daycount_fractions = np.array([[0.2465753425, 0.2493150685, 0.2520547945, 0.2520547945],
[0.2465753425, 0.2493150685, 0.2520547945, 0.2520547945]])
fixed_leg_coupon = 0.011 * np.ones_like(fixed_leg_payment_times)
zero_rate_fn = lambda x: 0.01 * tf.ones_like(x, dtype=dtype)
mu = [0.03, 0.15]
vol = piecewise.PiecewiseConstantFunc(
[[0.5],[0.5]], [[0.01, 0.015], [0.015, 0.02]], dtype=dtype)
hjm_price = swaption_price(
expiries=[expiries[0]],
fixed_leg_payment_times=fixed_leg_payment_times[0],
fixed_leg_daycount_fractions=fixed_leg_daycount_fractions[0],
fixed_leg_coupon=fixed_leg_coupon[0],
reference_rate_fn=zero_rate_fn,
notional=100.,
num_hjm_factors=len(mu),
mean_reversion=mu,
volatility=vol,
# corr_matrix=[[1, 0.0], [0.0, 1]],
valuation_method=ValuationMethod.MONTE_CARLO,
time_step_finite_difference=0.05,
num_grid_points_finite_difference=501,
time_step=0.05,
num_samples=2000000,
dtype=dtype)
hjm_price
vol([0.50001])
aa = tf.ones((2,3,4))
tf.reduce_sum(aa, axis=[0, 1])
###Output
_____no_output_____ |
book/tutorials/Annolid_on_Detectron2_Tutorial_3_Evaluate_our_model.ipynb | ###Markdown
Annolid on Detectron2 Tutorial 3 : Evaluating the modelThis is modified from the official colab tutorial of detectron2. Here, we will * Evaluate our previously trained model.You can make a copy of this tutorial by "File -> Open in playground mode" and play with it yourself. __DO NOT__ request access to this tutorial.
###Code
# Is running in colab or in jupyter-notebook
try:
import google.colab
IN_COLAB = True
except:
IN_COLAB = False
# install dependencies:
!pip install pyyaml==5.3
import torch, torchvision
TORCH_VERSION = ".".join(torch.__version__.split(".")[:2])
CUDA_VERSION = torch.__version__.split("+")[-1]
print("torch: ", TORCH_VERSION, "; cuda: ", CUDA_VERSION)
# Install detectron2 that matches the above pytorch version
# See https://detectron2.readthedocs.io/tutorials/install.html for instructions
!pip install detectron2 -f https://dl.fbaipublicfiles.com/detectron2/wheels/$CUDA_VERSION/torch$TORCH_VERSION/index.html
# If there is not yet a detectron2 release that matches the given torch + CUDA version, you need to install a different pytorch.
# exit(0) # After installation, you may need to "restart runtime" in Colab. This line can also restart runtime
# import some common libraries
import json
import os
import cv2
import random
import glob
import numpy as np
if IN_COLAB:
from google.colab.patches import cv2_imshow
import matplotlib.pyplot as plt
%matplotlib inline
# Setup detectron2 logger
import detectron2
from detectron2.utils.logger import setup_logger
setup_logger()
# import some common detectron2 utilities
from detectron2 import model_zoo
from detectron2.engine import DefaultPredictor
from detectron2.config import get_cfg
from detectron2.utils.visualizer import Visualizer
from detectron2.data import MetadataCatalog, DatasetCatalog
# is there a gpu
if torch.cuda.is_available():
GPU = True
print('gpu available')
else:
GPU = False
print('no gpu')
###Output
gpu available
###Markdown
Upload a labeled dataset.The following code is expecting the dataset in the COCO format to be in a ***.zip*** file. For example: ```sample_dataset.zip``` \Note: please make sure there is no white space in your file path if you encounter file not found issues.
###Code
!pip install gdown
!gdown --id 1fUXCLnoJ5SwXg54mj0NBKGzidsV8ALVR
if IN_COLAB:
dataset = '/content/novelctrlk6_8_coco_dataset.zip'
else:
dataset = 'novelctrlk6_8_coco_dataset.zip'
if IN_COLAB:
!unzip $dataset -d /content/
else:
#TODO generalize this
!unzip -o $dataset -d .
DATASET_NAME = DATASET_DIR = f"{dataset.replace('.zip','')}"
from detectron2.data.datasets import register_coco_instances
from detectron2.data import get_detection_dataset_dicts
from detectron2.data.datasets import builtin_meta
register_coco_instances(f"{DATASET_NAME}_train", {}, f"{DATASET_DIR}/train/annotations.json", f"{DATASET_DIR}/train/")
register_coco_instances(f"{DATASET_NAME}_valid", {}, f"{DATASET_DIR}/valid/annotations.json", f"{DATASET_DIR}/valid/")
_dataset_metadata = MetadataCatalog.get(f"{DATASET_NAME}_train")
_dataset_metadata.thing_colors = [cc['color'] for cc in builtin_meta.COCO_CATEGORIES]
dataset_dicts = get_detection_dataset_dicts([f"{DATASET_NAME}_train"])
NUM_CLASSES = len(_dataset_metadata.thing_classes)
print(f"{NUM_CLASSES} Number of classes in the dataset")
###Output
7 Number of classes in the dataset
###Markdown
Inference & evaluation using the trained modelNow, let's run inference with the trained model on the validation dataset. First, let's create a predictor using the model we just trained:
###Code
if GPU:
!nvidia-smi
from detectron2.engine import DefaultTrainer
cfg = get_cfg()
if GPU:
pass
else:
cfg.MODEL.DEVICE='cpu'
cfg.merge_from_file(model_zoo.get_config_file("COCO-InstanceSegmentation/mask_rcnn_R_50_FPN_3x.yaml"))
cfg.DATASETS.TRAIN = (f"{DATASET_NAME}_train",)
cfg.DATASETS.TEST = ()
cfg.DATALOADER.NUM_WORKERS = 2 #@param
cfg.DATALOADER.SAMPLER_TRAIN = "RepeatFactorTrainingSampler"
cfg.DATALOADER.REPEAT_THRESHOLD = 0.3
cfg.MODEL.WEIGHTS = model_zoo.get_checkpoint_url("COCO-InstanceSegmentation/mask_rcnn_R_50_FPN_3x.yaml") # Let training initialize from model zoo
cfg.SOLVER.IMS_PER_BATCH = 4 #@param
cfg.SOLVER.BASE_LR = 0.0025 #@param # pick a good LR
cfg.SOLVER.MAX_ITER = 3000 #@param # 300 iterations seems good enough for 100 frames dataset; you will need to train longer for a practical dataset
cfg.SOLVER.CHECKPOINT_PERIOD = 1000 #@param
cfg.MODEL.ROI_HEADS.BATCH_SIZE_PER_IMAGE = 32 #@param # faster, and good enough for this toy dataset (default: 512)
cfg.MODEL.ROI_HEADS.NUM_CLASSES = NUM_CLASSES # (see https://detectron2.readthedocs.io/tutorials/datasets.html#update-the-config-for-new-datasets)
os.makedirs(cfg.OUTPUT_DIR, exist_ok=True)
trainer = DefaultTrainer(cfg)
trainer.resume_or_load(resume=False)
# Inference should use the config with parameters that are used in training
# cfg now already contains everything we've set previously.
# We simply update the weights with the newly trained ones to perform inference:
cfg.MODEL.WEIGHTS = os.path.join(cfg.OUTPUT_DIR, "model_final.pth") # path to the model we just trained
# set a custom testing threshold
cfg.MODEL.ROI_HEADS.SCORE_THRESH_TEST = 0.15 #@param {type: "slider", min:0.0, max:1.0, step: 0.01}
predictor = DefaultPredictor(cfg)
###Output
_____no_output_____
###Markdown
Then, we randomly select several samples to visualize the prediction results.
###Code
from detectron2.utils.visualizer import ColorMode
dataset_dicts = get_detection_dataset_dicts([f"{DATASET_NAME}_valid"])
for d in random.sample(dataset_dicts, 4):
im = cv2.imread(d["file_name"])
outputs = predictor(im) # format is documented at https://detectron2.readthedocs.io/tutorials/models.html#model-output-format
v = Visualizer(im[:, :, ::-1],
metadata=_dataset_metadata,
scale=0.5,
instance_mode=ColorMode.SEGMENTATION # remove the colors of unsegmented pixels. This option is only available for segmentation models
)
out = v.draw_instance_predictions(outputs["instances"].to("cpu"))
if IN_COLAB:
cv2_imshow(out.get_image()[:, :, ::-1])
else:
plt.imshow(out.get_image()[:, :, ::-1])
plt.show()
###Output
[32m[01/24 14:31:55 d2.data.datasets.coco]: [0mLoaded 60 images in COCO format from novelctrlk6_8_coco_dataset/valid/annotations.json
[32m[01/24 14:31:55 d2.data.build]: [0mRemoved 0 images with no usable annotations. 60 images left.
[32m[01/24 14:31:55 d2.data.build]: [0mDistribution of instances among all 7 categories:
[36m| category | #instances | category | #instances | category | #instances |
|:------------:|:-------------|:----------:|:-------------|:----------:|:-------------|
| _background_ | 0 | nose | 60 | left_ear | 60 |
| right_ear | 60 | tail_base | 60 | mouse | 60 |
| centroid | 0 | | | | |
| total | 300 | | | | |[0m
###Markdown
A more robust way to evaluate the model is to use a metric called Average Precision (AP) already implemented in the detectron2 package. If you want more precision on what the AP is, you can take a look [here](https://scikit-learn.org/stable/modules/generated/sklearn.metrics.average_precision_score.htmlsklearn.metrics.average_precision_score) and [here](https://en.wikipedia.org/w/index.php?title=Information_retrieval&oldid=793358396Average_precision). TODO: expand on how to interpret AP
###Code
from detectron2.evaluation import COCOEvaluator, inference_on_dataset
from detectron2.data import build_detection_test_loader
if IN_COLAB:
evaluator = COCOEvaluator(f"{DATASET_NAME}_valid", cfg, False, output_dir="/content/eval_output/")
else:
evaluator = COCOEvaluator(f"{DATASET_NAME}_valid", cfg, False, output_dir="eval_output/")
val_loader = build_detection_test_loader(cfg, f"{DATASET_NAME}_valid")
print(inference_on_dataset(predictor.model, val_loader, evaluator))
# another equivalent way to evaluate the model is to use `trainer.test`
###Output
[5m[31mWARNING[0m [32m[01/24 14:31:56 d2.evaluation.coco_evaluation]: [0mCOCO Evaluator instantiated using config, this is deprecated behavior. Please pass in explicit arguments instead.
[32m[01/24 14:31:56 d2.data.datasets.coco]: [0mLoaded 60 images in COCO format from novelctrlk6_8_coco_dataset/valid/annotations.json
[32m[01/24 14:31:56 d2.data.dataset_mapper]: [0m[DatasetMapper] Augmentations used in inference: [ResizeShortestEdge(short_edge_length=(800, 800), max_size=1333, sample_style='choice')]
[32m[01/24 14:31:56 d2.data.common]: [0mSerializing 60 elements to byte tensors and concatenating them all ...
[32m[01/24 14:31:56 d2.data.common]: [0mSerialized dataset takes 0.15 MiB
[32m[01/24 14:31:56 d2.evaluation.evaluator]: [0mStart inference on 60 batches
[32m[01/24 14:31:57 d2.evaluation.evaluator]: [0mInference done 11/60. Dataloading: 0.0008 s/iter. Inference: 0.0523 s/iter. Eval: 0.0180 s/iter. Total: 0.0712 s/iter. ETA=0:00:03
[32m[01/24 14:32:01 d2.evaluation.evaluator]: [0mTotal inference time: 0:00:03.823960 (0.069527 s / iter per device, on 1 devices)
[32m[01/24 14:32:01 d2.evaluation.evaluator]: [0mTotal inference pure compute time: 0:00:02 (0.051859 s / iter per device, on 1 devices)
[32m[01/24 14:32:01 d2.evaluation.coco_evaluation]: [0mPreparing results for COCO format ...
[32m[01/24 14:32:01 d2.evaluation.coco_evaluation]: [0mSaving results to eval_output/coco_instances_results.json
[32m[01/24 14:32:01 d2.evaluation.coco_evaluation]: [0mEvaluating predictions with unofficial COCO API...
Loading and preparing results...
DONE (t=0.00s)
creating index...
index created!
[32m[01/24 14:32:01 d2.evaluation.fast_eval_api]: [0mEvaluate annotation type *bbox*
[32m[01/24 14:32:01 d2.evaluation.fast_eval_api]: [0mCOCOeval_opt.evaluate() finished in 0.02 seconds.
[32m[01/24 14:32:01 d2.evaluation.fast_eval_api]: [0mAccumulating evaluation results...
[32m[01/24 14:32:01 d2.evaluation.fast_eval_api]: [0mCOCOeval_opt.accumulate() finished in 0.01 seconds.
Average Precision (AP) @[ IoU=0.50:0.95 | area= all | maxDets=100 ] = 0.429
Average Precision (AP) @[ IoU=0.50 | area= all | maxDets=100 ] = 0.823
Average Precision (AP) @[ IoU=0.75 | area= all | maxDets=100 ] = 0.407
Average Precision (AP) @[ IoU=0.50:0.95 | area= small | maxDets=100 ] = 0.348
Average Precision (AP) @[ IoU=0.50:0.95 | area=medium | maxDets=100 ] = 0.796
Average Precision (AP) @[ IoU=0.50:0.95 | area= large | maxDets=100 ] = -1.000
Average Recall (AR) @[ IoU=0.50:0.95 | area= all | maxDets= 1 ] = 0.499
Average Recall (AR) @[ IoU=0.50:0.95 | area= all | maxDets= 10 ] = 0.530
Average Recall (AR) @[ IoU=0.50:0.95 | area= all | maxDets=100 ] = 0.530
Average Recall (AR) @[ IoU=0.50:0.95 | area= small | maxDets=100 ] = 0.464
Average Recall (AR) @[ IoU=0.50:0.95 | area=medium | maxDets=100 ] = 0.797
Average Recall (AR) @[ IoU=0.50:0.95 | area= large | maxDets=100 ] = -1.000
[32m[01/24 14:32:01 d2.evaluation.coco_evaluation]: [0mEvaluation results for bbox:
| AP | AP50 | AP75 | APs | APm | APl |
|:------:|:------:|:------:|:------:|:------:|:-----:|
| 42.881 | 82.250 | 40.677 | 34.808 | 79.604 | nan |
[32m[01/24 14:32:01 d2.evaluation.coco_evaluation]: [0mSome metrics cannot be computed and is shown as NaN.
[32m[01/24 14:32:01 d2.evaluation.coco_evaluation]: [0mPer-category bbox AP:
| category | AP | category | AP | category | AP |
|:-------------|:-------|:-----------|:-------|:-----------|:-------|
| _background_ | nan | nose | 37.467 | left_ear | 34.991 |
| right_ear | 26.474 | tail_base | 40.300 | mouse | 75.175 |
| centroid | nan | | | | |
Loading and preparing results...
DONE (t=0.00s)
creating index...
index created!
[32m[01/24 14:32:01 d2.evaluation.fast_eval_api]: [0mEvaluate annotation type *segm*
[32m[01/24 14:32:01 d2.evaluation.fast_eval_api]: [0mCOCOeval_opt.evaluate() finished in 0.02 seconds.
[32m[01/24 14:32:01 d2.evaluation.fast_eval_api]: [0mAccumulating evaluation results...
[32m[01/24 14:32:01 d2.evaluation.fast_eval_api]: [0mCOCOeval_opt.accumulate() finished in 0.01 seconds.
Average Precision (AP) @[ IoU=0.50:0.95 | area= all | maxDets=100 ] = 0.138
Average Precision (AP) @[ IoU=0.50 | area= all | maxDets=100 ] = 0.207
Average Precision (AP) @[ IoU=0.75 | area= all | maxDets=100 ] = 0.190
Average Precision (AP) @[ IoU=0.50:0.95 | area= small | maxDets=100 ] = 0.001
Average Precision (AP) @[ IoU=0.50:0.95 | area=medium | maxDets=100 ] = 0.689
Average Precision (AP) @[ IoU=0.50:0.95 | area= large | maxDets=100 ] = -1.000
Average Recall (AR) @[ IoU=0.50:0.95 | area= all | maxDets= 1 ] = 0.145
Average Recall (AR) @[ IoU=0.50:0.95 | area= all | maxDets= 10 ] = 0.145
Average Recall (AR) @[ IoU=0.50:0.95 | area= all | maxDets=100 ] = 0.145
Average Recall (AR) @[ IoU=0.50:0.95 | area= small | maxDets=100 ] = 0.004
Average Recall (AR) @[ IoU=0.50:0.95 | area=medium | maxDets=100 ] = 0.712
Average Recall (AR) @[ IoU=0.50:0.95 | area= large | maxDets=100 ] = -1.000
[32m[01/24 14:32:01 d2.evaluation.coco_evaluation]: [0mEvaluation results for segm:
| AP | AP50 | AP75 | APs | APm | APl |
|:------:|:------:|:------:|:-----:|:------:|:-----:|
| 13.838 | 20.664 | 18.990 | 0.083 | 68.859 | nan |
[32m[01/24 14:32:01 d2.evaluation.coco_evaluation]: [0mSome metrics cannot be computed and is shown as NaN.
[32m[01/24 14:32:01 d2.evaluation.coco_evaluation]: [0mPer-category segm AP:
| category | AP | category | AP | category | AP |
|:-------------|:------|:-----------|:------|:-----------|:-------|
| _background_ | nan | nose | 0.000 | left_ear | 0.000 |
| right_ear | 0.025 | tail_base | 0.307 | mouse | 68.859 |
| centroid | nan | | | | |
OrderedDict([('bbox', {'AP': 42.881449697137505, 'AP50': 82.2501044835862, 'AP75': 40.67677036400211, 'APs': 34.80797160883607, 'APm': 79.60396039603961, 'APl': nan, 'AP-_background_': nan, 'AP-nose': 37.46745432692759, 'AP-left_ear': 34.99076354765347, 'AP-right_ear': 26.474155569306223, 'AP-tail_base': 40.29951299145698, 'AP-mouse': 75.17536205034328, 'AP-centroid': nan}), ('segm', {'AP': 13.838099650346003, 'AP50': 20.663994970925664, 'AP75': 18.99009900990099, 'APs': 0.082999371365708, 'APm': 68.85850076626717, 'APl': nan, 'AP-_background_': nan, 'AP-nose': 0.0, 'AP-left_ear': 0.0, 'AP-right_ear': 0.024752475247524754, 'AP-tail_base': 0.3072450102153072, 'AP-mouse': 68.85850076626717, 'AP-centroid': nan})])
|
notebooks/stephan_notebooks/MH_notebooks/01_Check_dataloader.ipynb | ###Markdown
Sanity checks for the dataloaderS.Rasp developed the dataloader, the pytorch tool to wrap all the data laoding, preparation and batch generation... **Special focus should be given on th patches and times**
###Code
%load_ext autoreload
%autoreload 2
import xarray as xr
import numpy as np
import cartopy.crs as ccrs
import matplotlib.pyplot as plt
import cartopy.feature as cfeature
states_provinces = cfeature.NaturalEarthFeature(
category='cultural',
name='admin_1_states_provinces_lines',
scale='50m',
facecolor='none')
import hvplot.xarray
import cartopy.crs as crs
# add src directory
import sys
sys.path.append('..')
from src.dataloader import *
###Output
_____no_output_____
###Markdown
General functionality of data loader
###Code
ds = TiggeMRMSDataset(
tigge_dir='/datadrive/tigge/32km/',
tigge_vars=['total_precipitation'],
mrms_dir='/datadrive/mrms/4km/RadarOnly_QPE_06H/',
rq_fn='/datadrive/mrms/4km/RadarQuality.nc',
# const_fn='/datadrive/tigge/32km/constants.nc',
# const_vars=['orog', 'lsm'],
val_days=7,
split='train',
tp_log=1
)
print(ds)
idx= 1200 # which patch index to use
X, y = ds[idx] # X: tigge, y : radar
plt.pcolor(X[0])
plt.colorbar()
plt.figure()
plt.pcolor(y[0])
plt.colorbar()
###Output
_____no_output_____
###Markdown
Check TIGGE data
###Code
tigge_tp = xr.open_mfdataset('/datadrive/tigge/32km/total_precipitation/*.nc').tp.diff('lead_time')
tigge_tp
tigge_tp.
###Output
_____no_output_____
###Markdown
Compare Time steps: the time-idx value corresponds to the time given by the overlap_times array. Note that the leadtime needs to be subtracted. Given the different precip scalings, the two images are similar! --> Time seems to be alright between manually loaded and ds.tigge data
###Code
idx= 3140 # which patch index to use
from matplotlib.patches import Rectangle
tigge_sel = tigge_tp.sel(lead_time=np.timedelta64(12, 'h'))
t_idx, lat_idx, lon_idx = ds.idxs[idx] # take indices from selected patch idx
fig = plt.figure(figsize=[25,10])
tigge_sel = tigge_sel.sel(init_time=ds.overlap_times[t_idx] - np.timedelta64(12, 'h'))
tigge_sel.plot(cmap='viridis', levels=np.arange(0,20))
patch_lat = tigge_sel.lat.max().values+0.32
patch_lon = tigge_sel.lon.min().values
# Create Rectangle patches
delta = ds.patch_size/100 # patch size in deg
for i in range(0,12):
for j in range(0,9):
xy = np.array((patch_lon+i*(delta+0.32),patch_lat-(j+1)*(delta+0.32)))
rect = Rectangle(xy ,delta, delta , linewidth=1,edgecolor='r',facecolor='none')
fig.gca().add_patch(rect)
fig.gca().annotate('(%i,%i)'%(i,j), xy+delta/2, color='w', weight='bold',
fontsize=16, ha='center', va='center')
from matplotlib.patches import Rectangle
fig = plt.figure(figsize=[25,10])
ds.tigge.isel(valid_time=t_idx,variable=0).plot()
patch_lat = tigge_sel.lat.max().values +0.32
patch_lon = tigge_sel.lon.min().values
# Create Rectangle patches
delta = ds.patch_size/100 # patch size in deg
for i in range(0,12):
for j in range(0,9):
xy = np.array((patch_lon+i*(delta+0.32),patch_lat-(j+1)*(delta+0.32)))
rect = Rectangle(xy ,delta, +delta , linewidth=1,edgecolor='r',facecolor='none')
fig.gca().add_patch(rect)
fig.gca().annotate('(%i,%i)'%(i,j), xy+delta/2, color='w', weight='bold',
fontsize=16, ha='center', va='center')
###Output
_____no_output_____
###Markdown
Now compare ds.tigge with ds[patch_idx]
###Code
idx= 3590 # which patch index to use
test = ds.tigge.isel(
valid_time=t_idx,
lat=slice(0 * ds.patch_tigge, (0+1) * ds.patch_tigge + ds.pad_tigge*2),
lon=slice(0 * ds.patch_tigge, (0+1) * ds.patch_tigge + ds.pad_tigge*2)
)
test.isel(lat=0).plot()
test = ds.tigge.isel(
valid_time=t_idx,
lat=slice(1 * ds.patch_tigge, (1+1) * ds.patch_tigge + ds.pad_tigge*2),
lon=slice(0 * ds.patch_tigge, (0+1) * ds.patch_tigge + ds.pad_tigge*2)
)
test.isel(lat=-1).plot()
from matplotlib.patches import Rectangle
fig = plt.figure(figsize=[25,10])
t_idx, lat_idx, lon_idx = ds.idxs[idx] # take indices from selected patch idx
ds.tigge.isel(valid_time=t_idx,variable=0).plot()
patch_lat = tigge_sel.lat.max().values+0.32
patch_lon = tigge_sel.lon.min().values
# Create Rectangle patches
delta = ds.patch_size/100 # patch size in deg
for lat_idx,lon_idx in ds.idxs[:,1:3]:
test = ds.tigge.isel(
valid_time=t_idx,
lat=slice(lat_idx * ds.patch_tigge, (lat_idx+1) * ds.patch_tigge + ds.pad_tigge*2),
lon=slice(lon_idx * ds.patch_tigge, (lon_idx+1) * ds.patch_tigge + ds.pad_tigge*2)
)
#print(j)
#print(test.lat.max('lat').values)
xy = np.array([test.lon.min('lon'),test.lat.min('lat').values])
rect = Rectangle(xy ,delta, delta , linewidth=1,edgecolor='r',facecolor='none')
fig.gca().add_patch(rect)
fig.gca().annotate('(%i,%i)'%(lon_idx,lat_idx), xy+delta/2, color='w', weight='bold',
fontsize=16, ha='center', va='center')
plt.ylim([20,51])
from matplotlib.patches import Rectangle
fig = plt.figure(figsize=[25,10])
t_idx, lat_idx, lon_idx = ds.idxs[idx] # take indices from selected patch idx
ds.tigge.isel(valid_time=t_idx,variable=0).plot()
patch_lat = tigge_sel.lat.max().values+0.32
patch_lon = tigge_sel.lon.min().values
pad_tigge = 2
# Create Rectangle patches
delta = ds.patch_size/100 +pad_tigge*2*0.32 # patch size in deg
for lat_idx,lon_idx in ds.idxs[:,1:3]:
test = ds.tigge.isel(
valid_time=t_idx,
lat=slice(lat_idx * ds.patch_tigge, (lat_idx+1) * ds.patch_tigge + pad_tigge*2),
lon=slice(lon_idx * ds.patch_tigge, (lon_idx+1) * ds.patch_tigge + pad_tigge*2)
)
#print(j)
#print(test.lat.max('lat').values)
xy = np.array([test.lon.min('lon'),test.lat.min('lat').values])
rect = Rectangle(xy ,delta, delta, linewidth=1,edgecolor='r',facecolor='none')
fig.gca().add_patch(rect)
fig.gca().annotate('(%i,%i)'%(lon_idx,lat_idx), xy+delta/2, color='w', weight='bold',
fontsize=16, ha='center', va='center')
plt.ylim([20,51])
from matplotlib.patches import Rectangle
fig = plt.figure(figsize=[25,10])
t_idx, lat_idx, lon_idx = ds.idxs[idx] # take indices from selected patch idx
ds.tigge.isel(valid_time=t_idx,variable=0).plot()
patch_lat = tigge_sel.lat.max().values+0.32
patch_lon = tigge_sel.lon.min().values
# Create Rectangle patches
delta = ds.patch_size/100 # patch size in deg
for i in range(0,10):
for j in range(0,5):
test = ds.tigge.isel(
valid_time=t_idx,
lat=slice(j * ds.patch_tigge, (j+1) * ds.patch_tigge + ds.pad_tigge*2),
lon=slice(i * ds.patch_tigge, (i+1) * ds.patch_tigge + ds.pad_tigge*2)
)
#print(j)
#print(test.lat.max('lat').values)
xy = np.array([test.lon.min('lon'),test.lat.min('lat').values])
print(xy)
rect = Rectangle(xy ,delta, delta , linewidth=1,edgecolor='r',facecolor='none')
fig.gca().add_patch(rect)
fig.gca().annotate('(%i,%i)'%(i,j), xy+delta/2, color='w', weight='bold',
fontsize=16, ha='center', va='center')
np.array([test.lon.min('lon'),test.lat.min('lat').values])[0]
test = ds.tigge.isel(
valid_time=t_idx,
lat=slice(j * ds.patch_tigge, (j+1) * ds.patch_tigge + ds.pad_tigge*2),
lon=slice(i * ds.patch_tigge, (i+1) * ds.patch_tigge + ds.pad_tigge*2)
)
test.plot()
ds. xy = np.array((test.lon.min('lon').values), test.lat.min('lat').values)
test = ds.tigge.isel(valid_time=t_idx,variable=0).sel(lon=slice(xy[0],xy[0]+delta), lat = slice(xy[1]+delta, xy[1]))
test.plot(levels=np.arange(0,1,.05))
X,_ = ds[idx]
plt.pcolor(X[0])
X = ds.tigge.isel(
valid_time=t_idx,
lat=slice(lat_idx * self.patch_tigge, (lat_idx+1) * self.patch_tigge + self.pad_tigge*2),
lon=slice(lon_idx * self.patch_tigge, (lon_idx+1) * self.patch_tigge + self.pad_tigge*2)
).values
tigge_tp
###Output
_____no_output_____
###Markdown
Check MRMS Radar data
###Code
idx=3590
t_idx, lat_idx,lon_idx = ds.idxs[idx]
X,y = ds[idx]
plt.pcolor(y[0])
ds.mrms.isel(time=t_idx).plot()
fig = plt.figure(figsize=[25,10])
ds.mrms.isel(time=t_idx).plot()
# Create Rectangle patches
delta = ds.patch_size/100 # patch size in deg
for lat_idx,lon_idx in ds.idxs[:,1:3]:
test = ds.mrms.isel(
time=t_idx,
lat=slice(lat_idx * ds.patch_mrms, (lat_idx+1) * ds.patch_mrms + ds.pad_mrms*2),
lon=slice(lon_idx * ds.patch_mrms, (lon_idx+1) * ds.patch_mrms + ds.pad_mrms*2)
)
#print(j)
#print(test.lat.max('lat').values)
xy = np.array([test.lon.min('lon'),test.lat.min('lat').values])
rect = Rectangle(xy ,delta, delta , linewidth=1,edgecolor='r',facecolor='none')
fig.gca().add_patch(rect)
fig.gca().annotate('(%i,%i)'%(lon_idx,lat_idx), xy+delta/2, color='w', weight='bold',
fontsize=16, ha='center', va='center')
plt.ylim([20,51])
lon_idx
lat_idx
###Output
_____no_output_____ |
Day 13/13_4_Simple_Sentiment_Analysis.ipynb | ###Markdown
Reference: https://github.com/bentrevett/pytorch-sentiment-analysis 1 - Simple Sentiment AnalysisIn this series we'll be building a machine learning model to detect sentiment (i.e. detect if a sentence is positive or negative) using PyTorch and TorchText. This will be done on movie reviews, using the [IMDb dataset](http://ai.stanford.edu/~amaas/data/sentiment/).In this first notebook, we'll start very simple to understand the general concepts whilst not really caring about good results. Further notebooks will build on this knowledge and we'll actually get good results. IntroductionWe'll be using a **recurrent neural network** (RNN) as they are commonly used in analysing sequences. An RNN takes in sequence of words, $X=\{x_1, ..., x_T\}$, one at a time, and produces a _hidden state_, $h$, for each word. We use the RNN _recurrently_ by feeding in the current word $x_t$ as well as the hidden state from the previous word, $h_{t-1}$, to produce the next hidden state, $h_t$. $$h_t = \text{RNN}(x_t, h_{t-1})$$Once we have our final hidden state, $h_T$, (from feeding in the last word in the sequence, $x_T$) we feed it through a linear layer, $f$, (also known as a fully connected layer), to receive our predicted sentiment, $\hat{y} = f(h_T)$.Below shows an example sentence, with the RNN predicting zero, which indicates a negative sentiment. The RNN is shown in orange and the linear layer shown in silver. Note that we use the same RNN for every word, i.e. it has the same parameters. The initial hidden state, $h_0$, is a tensor initialized to all zeros. **Note:** some layers and steps have been omitted from the diagram, but these will be explained later. Preparing DataOne of the main concepts of TorchText is the `Field`. These define how your data should be processed. In our sentiment classification task the data consists of both the raw string of the review and the sentiment, either "pos" or "neg".The parameters of a `Field` specify how the data should be processed. We use the `TEXT` field to define how the review should be processed, and the `LABEL` field to process the sentiment. Our `TEXT` field has `tokenize='spacy'` as an argument. This defines that the "tokenization" (the act of splitting the string into discrete "tokens") should be done using the [spaCy](https://spacy.io) tokenizer. If no `tokenize` argument is passed, the default is simply splitting the string on spaces. We also need to specify a `tokenizer_language` which tells torchtext which spaCy model to use. We use the `en_core_web_sm` model which has to be downloaded with `python -m spacy download en_core_web_sm` before you run this notebook!`LABEL` is defined by a `LabelField`, a special subset of the `Field` class specifically used for handling labels. We will explain the `dtype` argument later.For more on `Fields`, go [here](https://github.com/pytorch/text/blob/master/torchtext/data/field.py).We also set the random seeds for reproducibility.
###Code
!pip install torchtext==0.9.0
import torch
from torchtext.legacy import data
SEED = 1234
torch.manual_seed(SEED)
torch.backends.cudnn.deterministic = True
TEXT = data.Field(tokenize = 'spacy',
tokenizer_language = 'en_core_web_sm')
LABEL = data.LabelField(dtype = torch.float)
###Output
_____no_output_____
###Markdown
Another handy feature of TorchText is that it has support for common datasets used in natural language processing (NLP). The following code automatically downloads the IMDb dataset and splits it into the canonical train/test splits as `torchtext.datasets` objects. It process the data using the `Fields` we have previously defined. The IMDb dataset consists of 50,000 movie reviews, each marked as being a positive or negative review.
###Code
from torchtext.legacy import datasets
train_data, test_data = datasets.IMDB.splits(TEXT, LABEL)
###Output
downloading aclImdb_v1.tar.gz
###Markdown
We can see how many examples are in each split by checking their length.
###Code
print(f'Number of training examples: {len(train_data)}')
print(f'Number of testing examples: {len(test_data)}')
###Output
Number of training examples: 25000
Number of testing examples: 25000
###Markdown
We can also check an example.
###Code
print(vars(train_data.examples[0]))
###Output
{'text': ['This', 'movie', 'is', 'the', 'first', 'of', 'Miikes', 'triad', 'society', 'trilogy', ',', 'and', 'the', 'trilogy', 'kicks', 'of', 'to', 'a', 'great', 'start', '.', 'The', 'movies', 'in', 'the', 'trilogy', 'are', 'only', 'connected', 'thematically', ',', 'and', 'these', 'themes', 'are', 'actually', 'apparent', 'in', 'all', 'his', 'films', ',', 'if', 'you', 'look', 'close', 'enough', '.', 'Shinjuku', 'Triad', 'Society', 'is', 'about', 'a', 'cop', 'trying', 'to', 'prevent', 'his', 'kid', 'brother', 'from', 'getting', 'too', 'involved', 'with', 'a', 'rather', 'extreme', 'gang', 'of', 'outsiders', ',', 'struggling', 'their', 'way', 'to', 'the', 'top', 'of', 'Tokyos', 'yakuza', '.', 'The', 'kid', 'brother', 'is', 'a', 'lawyer', ',', 'and', 'the', 'triad', 'gang', 'is', 'becoming', 'increasingly', 'in', 'need', 'of', 'one', ',', 'as', 'the', 'movie', 'progresses', '.', 'The', 'movie', 'takes', 'place', 'in', 'a', 'very', 'harsh', 'environment', ',', 'and', 'is', 'therefore', 'pretty', 'violent', 'and', 'tough', '.', 'Miike', 'has', 'done', 'worse', ',', 'but', 'since', 'this', 'is', 'a', 'serious', 'movie', 'it', 'hits', 'you', 'very', 'hard', '.', 'As', 'usual', 'there', 'is', 'also', 'a', 'lot', 'of', 'perverted', 'sex', ',', 'mostly', 'homosexual', 'in', 'this', 'one', '.', 'The', 'movie', 'is', 'in', 'many', 'ways', 'a', 'typical', 'gangster', 'movie', ',', 'but', 'with', 'a', 'great', 'drive', 'and', 'true', 'grittiness', '.', 'If', 'you', "'ve", 'only', 'seen', 'Miikes', 'far', '-', 'out', 'movies', '(', 'Ichi', 'the', 'killer', ',', 'Fudoh', 'etc', '.', ')', 'this', 'is', 'worth', 'checking', 'out', 'since', 'it', 'is', 'sort', 'of', 'a', 'compromise', 'between', 'his', 'aggressive', 'over', '-', 'the', '-', 'top', 'style', 'displayed', 'in', 'those', 'movies', 'and', 'his', 'more', 'serious', 'side', ',', 'as', 'seen', 'in', 'the', 'other', 'films', 'of', 'the', 'trilogy', '.', 'And', 'as', 'always', 'with', 'Miike', ',', 'there', 'are', 'at', 'least', 'two', 'scenes', 'in', 'this', 'that', 'you', "'ll", 'NEVER', 'forget', '(', 'see', 'it', 'and', 'figure', 'out', 'which', 'ones', 'for', 'yourself).<br', '/><br', '/>8/10'], 'label': 'pos'}
###Markdown
The IMDb dataset only has train/test splits, so we need to create a validation set. We can do this with the `.split()` method. By default this splits 70/30, however by passing a `split_ratio` argument, we can change the ratio of the split, i.e. a `split_ratio` of 0.8 would mean 80% of the examples make up the training set and 20% make up the validation set. We also pass our random seed to the `random_state` argument, ensuring that we get the same train/validation split each time.
###Code
import random
train_data, valid_data = train_data.split(random_state = random.seed(SEED))
###Output
_____no_output_____
###Markdown
Again, we'll view how many examples are in each split.
###Code
print(f'Number of training examples: {len(train_data)}')
print(f'Number of validation examples: {len(valid_data)}')
print(f'Number of testing examples: {len(test_data)}')
###Output
Number of training examples: 17500
Number of validation examples: 7500
Number of testing examples: 25000
###Markdown
Next, we have to build a _vocabulary_. This is a effectively a look up table where every unique word in your data set has a corresponding _index_ (an integer).We do this as our machine learning model cannot operate on strings, only numbers. Each _index_ is used to construct a _one-hot_ vector for each word. A one-hot vector is a vector where all of the elements are 0, except one, which is 1, and dimensionality is the total number of unique words in your vocabulary, commonly denoted by $V$.The number of unique words in our training set is over 100,000, which means that our one-hot vectors will have over 100,000 dimensions! This will make training slow and possibly won't fit onto your GPU (if you're using one). There are two ways effectively cut down our vocabulary, we can either only take the top $n$ most common words or ignore words that appear less than $m$ times. We'll do the former, only keeping the top 25,000 words.What do we do with words that appear in examples but we have cut from the vocabulary? We replace them with a special _unknown_ or `` token. For example, if the sentence was "This film is great and I love it" but the word "love" was not in the vocabulary, it would become "This film is great and I `` it".The following builds the vocabulary, only keeping the most common `max_size` tokens.
###Code
MAX_VOCAB_SIZE = 25_000
TEXT.build_vocab(train_data, max_size = MAX_VOCAB_SIZE)
LABEL.build_vocab(train_data)
###Output
_____no_output_____
###Markdown
Why do we only build the vocabulary on the training set? When testing any machine learning system you do not want to look at the test set in any way. We do not include the validation set as we want it to reflect the test set as much as possible.
###Code
print(f"Unique tokens in TEXT vocabulary: {len(TEXT.vocab)}")
print(f"Unique tokens in LABEL vocabulary: {len(LABEL.vocab)}")
###Output
Unique tokens in TEXT vocabulary: 25002
Unique tokens in LABEL vocabulary: 2
###Markdown
Why is the vocab size 25002 and not 25000? One of the addition tokens is the `` token and the other is a `` token.When we feed sentences into our model, we feed a _batch_ of them at a time, i.e. more than one at a time, and all sentences in the batch need to be the same size. Thus, to ensure each sentence in the batch is the same size, any shorter than the longest within the batch are padded.We can also view the most common words in the vocabulary and their frequencies.
###Code
print(TEXT.vocab.freqs.most_common(20))
###Output
[('the', 203522), (',', 193141), ('.', 166396), ('a', 109712), ('and', 109710), ('of', 100686), ('to', 93968), ('is', 76710), ('in', 61305), ('I', 54268), ('it', 53492), ('that', 49245), ('"', 44030), ("'s", 43462), ('this', 42390), ('-', 37048), ('/><br', 35651), ('was', 35208), ('as', 30388), ('with', 30134)]
###Markdown
We can also see the vocabulary directly using either the `stoi` (**s**tring **to** **i**nt) or `itos` (**i**nt **to** **s**tring) method.
###Code
print(TEXT.vocab.itos[:10])
###Output
['<unk>', '<pad>', 'the', ',', '.', 'a', 'and', 'of', 'to', 'is']
###Markdown
We can also check the labels, ensuring 0 is for negative and 1 is for positive.
###Code
print(LABEL.vocab.stoi)
###Output
defaultdict(None, {'neg': 0, 'pos': 1})
###Markdown
The final step of preparing the data is creating the iterators. We iterate over these in the training/evaluation loop, and they return a batch of examples (indexed and converted into tensors) at each iteration.We'll use a `BucketIterator` which is a special type of iterator that will return a batch of examples where each example is of a similar length, minimizing the amount of padding per example.We also want to place the tensors returned by the iterator on the GPU (if you're using one). PyTorch handles this using `torch.device`, we then pass this device to the iterator.
###Code
BATCH_SIZE = 64
device = torch.device('cuda' if torch.cuda.is_available() else 'cpu')
train_iterator, valid_iterator, test_iterator = data.BucketIterator.splits(
(train_data, valid_data, test_data),
batch_size = BATCH_SIZE,
device = device)
###Output
_____no_output_____
###Markdown
Build the ModelThe next stage is building the model that we'll eventually train and evaluate. There is a small amount of boilerplate code when creating models in PyTorch, note how our `RNN` class is a sub-class of `nn.Module` and the use of `super`.Within the `__init__` we define the _layers_ of the module. Our three layers are an _embedding_ layer, our RNN, and a _linear_ layer. All layers have their parameters initialized to random values, unless explicitly specified.The embedding layer is used to transform our sparse one-hot vector (sparse as most of the elements are 0) into a dense embedding vector (dense as the dimensionality is a lot smaller and all the elements are real numbers). This embedding layer is simply a single fully connected layer. As well as reducing the dimensionality of the input to the RNN, there is the theory that words which have similar impact on the sentiment of the review are mapped close together in this dense vector space. For more information about word embeddings, see [here](https://monkeylearn.com/blog/word-embeddings-transform-text-numbers/).The RNN layer is our RNN which takes in our dense vector and the previous hidden state $h_{t-1}$, which it uses to calculate the next hidden state, $h_t$.Finally, the linear layer takes the final hidden state and feeds it through a fully connected layer, $f(h_T)$, transforming it to the correct output dimension.The `forward` method is called when we feed examples into our model.Each batch, `text`, is a tensor of size _**[sentence length, batch size]**_. That is a batch of sentences, each having each word converted into a one-hot vector. You may notice that this tensor should have another dimension due to the one-hot vectors, however PyTorch conveniently stores a one-hot vector as it's index value, i.e. the tensor representing a sentence is just a tensor of the indexes for each token in that sentence. The act of converting a list of tokens into a list of indexes is commonly called *numericalizing*.The input batch is then passed through the embedding layer to get `embedded`, which gives us a dense vector representation of our sentences. `embedded` is a tensor of size _**[sentence length, batch size, embedding dim]**_.`embedded` is then fed into the RNN. In some frameworks you must feed the initial hidden state, $h_0$, into the RNN, however in PyTorch, if no initial hidden state is passed as an argument it defaults to a tensor of all zeros.The RNN returns 2 tensors, `output` of size _**[sentence length, batch size, hidden dim]**_ and `hidden` of size _**[1, batch size, hidden dim]**_. `output` is the concatenation of the hidden state from every time step, whereas `hidden` is simply the final hidden state. We verify this using the `assert` statement. Note the `squeeze` method, which is used to remove a dimension of size 1. Finally, we feed the last hidden state, `hidden`, through the linear layer, `fc`, to produce a prediction.
###Code
import torch.nn as nn
class RNN(nn.Module):
def __init__(self, input_dim, embedding_dim, hidden_dim, output_dim):
super().__init__()
self.embedding = nn.Embedding(input_dim, embedding_dim)
self.rnn = nn.RNN(embedding_dim, hidden_dim)
self.fc = nn.Linear(hidden_dim, output_dim)
def forward(self, text):
#text = [sent len, batch size]
embedded = self.embedding(text)
#embedded = [sent len, batch size, emb dim]
output, hidden = self.rnn(embedded)
#output = [sent len, batch size, hid dim]
#hidden = [1, batch size, hid dim]
assert torch.equal(output[-1,:,:], hidden.squeeze(0))
return self.fc(hidden.squeeze(0))
###Output
_____no_output_____
###Markdown
We now create an instance of our RNN class. The input dimension is the dimension of the one-hot vectors, which is equal to the vocabulary size. The embedding dimension is the size of the dense word vectors. This is usually around 50-250 dimensions, but depends on the size of the vocabulary.The hidden dimension is the size of the hidden states. This is usually around 100-500 dimensions, but also depends on factors such as on the vocabulary size, the size of the dense vectors and the complexity of the task.The output dimension is usually the number of classes, however in the case of only 2 classes the output value is between 0 and 1 and thus can be 1-dimensional, i.e. a single scalar real number.
###Code
INPUT_DIM = len(TEXT.vocab)
EMBEDDING_DIM = 100
HIDDEN_DIM = 256
OUTPUT_DIM = 1
model = RNN(INPUT_DIM, EMBEDDING_DIM, HIDDEN_DIM, OUTPUT_DIM)
###Output
_____no_output_____
###Markdown
Let's also create a function that will tell us how many trainable parameters our model has so we can compare the number of parameters across different models.
###Code
def count_parameters(model):
return sum(p.numel() for p in model.parameters() if p.requires_grad)
print(f'The model has {count_parameters(model):,} trainable parameters')
###Output
The model has 2,592,105 trainable parameters
###Markdown
Train the Model Now we'll set up the training and then train the model.First, we'll create an optimizer. This is the algorithm we use to update the parameters of the module. Here, we'll use _stochastic gradient descent_ (SGD). The first argument is the parameters will be updated by the optimizer, the second is the learning rate, i.e. how much we'll change the parameters by when we do a parameter update.
###Code
import torch.optim as optim
optimizer = optim.SGD(model.parameters(), lr=1e-3)
###Output
_____no_output_____
###Markdown
Next, we'll define our loss function. In PyTorch this is commonly called a criterion. The loss function here is _binary cross entropy with logits_. Our model currently outputs an unbound real number. As our labels are either 0 or 1, we want to restrict the predictions to a number between 0 and 1. We do this using the _sigmoid_ or _logit_ functions. We then use this this bound scalar to calculate the loss using binary cross entropy. The `BCEWithLogitsLoss` criterion carries out both the sigmoid and the binary cross entropy steps.
###Code
criterion = nn.BCEWithLogitsLoss()
###Output
_____no_output_____
###Markdown
Using `.to`, we can place the model and the criterion on the GPU (if we have one).
###Code
model = model.to(device)
criterion = criterion.to(device)
###Output
_____no_output_____
###Markdown
Our criterion function calculates the loss, however we have to write our function to calculate the accuracy. This function first feeds the predictions through a sigmoid layer, squashing the values between 0 and 1, we then round them to the nearest integer. This rounds any value greater than 0.5 to 1 (a positive sentiment) and the rest to 0 (a negative sentiment).We then calculate how many rounded predictions equal the actual labels and average it across the batch.
###Code
def binary_accuracy(preds, y):
"""
Returns accuracy per batch, i.e. if you get 8/10 right, this returns 0.8, NOT 8
"""
#round predictions to the closest integer
rounded_preds = torch.round(torch.sigmoid(preds))
correct = (rounded_preds == y).float() #convert into float for division
acc = correct.sum() / len(correct)
return acc
###Output
_____no_output_____
###Markdown
The `train` function iterates over all examples, one batch at a time. `model.train()` is used to put the model in "training mode", which turns on _dropout_ and _batch normalization_. Although we aren't using them in this model, it's good practice to include it.For each batch, we first zero the gradients. Each parameter in a model has a `grad` attribute which stores the gradient calculated by the `criterion`. PyTorch does not automatically remove (or "zero") the gradients calculated from the last gradient calculation, so they must be manually zeroed.We then feed the batch of sentences, `batch.text`, into the model. Note, you do not need to do `model.forward(batch.text)`, simply calling the model works. The `squeeze` is needed as the predictions are initially size _**[batch size, 1]**_, and we need to remove the dimension of size 1 as PyTorch expects the predictions input to our criterion function to be of size _**[batch size]**_.The loss and accuracy are then calculated using our predictions and the labels, `batch.label`, with the loss being averaged over all examples in the batch.We calculate the gradient of each parameter with `loss.backward()`, and then update the parameters using the gradients and optimizer algorithm with `optimizer.step()`.The loss and accuracy is accumulated across the epoch, the `.item()` method is used to extract a scalar from a tensor which only contains a single value.Finally, we return the loss and accuracy, averaged across the epoch. The `len` of an iterator is the number of batches in the iterator.You may recall when initializing the `LABEL` field, we set `dtype=torch.float`. This is because TorchText sets tensors to be `LongTensor`s by default, however our criterion expects both inputs to be `FloatTensor`s. Setting the `dtype` to be `torch.float`, did this for us. The alternative method of doing this would be to do the conversion inside the `train` function by passing `batch.label.float()` instad of `batch.label` to the criterion.
###Code
def train(model, iterator, optimizer, criterion):
epoch_loss = 0
epoch_acc = 0
model.train()
for batch in iterator:
optimizer.zero_grad()
predictions = model(batch.text).squeeze(1)
loss = criterion(predictions, batch.label)
acc = binary_accuracy(predictions, batch.label)
loss.backward()
optimizer.step()
epoch_loss += loss.item()
epoch_acc += acc.item()
return epoch_loss / len(iterator), epoch_acc / len(iterator)
###Output
_____no_output_____
###Markdown
`evaluate` is similar to `train`, with a few modifications as you don't want to update the parameters when evaluating.`model.eval()` puts the model in "evaluation mode", this turns off _dropout_ and _batch normalization_. Again, we are not using them in this model, but it is good practice to include them.No gradients are calculated on PyTorch operations inside the `with no_grad()` block. This causes less memory to be used and speeds up computation.The rest of the function is the same as `train`, with the removal of `optimizer.zero_grad()`, `loss.backward()` and `optimizer.step()`, as we do not update the model's parameters when evaluating.
###Code
def evaluate(model, iterator, criterion):
epoch_loss = 0
epoch_acc = 0
model.eval()
with torch.no_grad():
for batch in iterator:
predictions = model(batch.text).squeeze(1)
loss = criterion(predictions, batch.label)
acc = binary_accuracy(predictions, batch.label)
epoch_loss += loss.item()
epoch_acc += acc.item()
return epoch_loss / len(iterator), epoch_acc / len(iterator)
###Output
_____no_output_____
###Markdown
We'll also create a function to tell us how long an epoch takes to compare training times between models.
###Code
import time
def epoch_time(start_time, end_time):
elapsed_time = end_time - start_time
elapsed_mins = int(elapsed_time / 60)
elapsed_secs = int(elapsed_time - (elapsed_mins * 60))
return elapsed_mins, elapsed_secs
###Output
_____no_output_____
###Markdown
We then train the model through multiple epochs, an epoch being a complete pass through all examples in the training and validation sets.At each epoch, if the validation loss is the best we have seen so far, we'll save the parameters of the model and then after training has finished we'll use that model on the test set.
###Code
N_EPOCHS = 5
best_valid_loss = float('inf')
for epoch in range(N_EPOCHS):
start_time = time.time()
train_loss, train_acc = train(model, train_iterator, optimizer, criterion)
valid_loss, valid_acc = evaluate(model, valid_iterator, criterion)
end_time = time.time()
epoch_mins, epoch_secs = epoch_time(start_time, end_time)
if valid_loss < best_valid_loss:
best_valid_loss = valid_loss
torch.save(model.state_dict(), 'tut1-model.pt')
print(f'Epoch: {epoch+1:02} | Epoch Time: {epoch_mins}m {epoch_secs}s')
print(f'\tTrain Loss: {train_loss:.3f} | Train Acc: {train_acc*100:.2f}%')
print(f'\t Val. Loss: {valid_loss:.3f} | Val. Acc: {valid_acc*100:.2f}%')
###Output
Epoch: 01 | Epoch Time: 0m 46s
Train Loss: 0.694 | Train Acc: 50.29%
Val. Loss: 0.697 | Val. Acc: 49.20%
Epoch: 02 | Epoch Time: 0m 46s
Train Loss: 0.693 | Train Acc: 50.03%
Val. Loss: 0.697 | Val. Acc: 49.40%
Epoch: 03 | Epoch Time: 0m 45s
Train Loss: 0.693 | Train Acc: 49.83%
Val. Loss: 0.697 | Val. Acc: 50.39%
Epoch: 04 | Epoch Time: 0m 45s
Train Loss: 0.693 | Train Acc: 49.87%
Val. Loss: 0.697 | Val. Acc: 48.83%
Epoch: 05 | Epoch Time: 0m 45s
Train Loss: 0.693 | Train Acc: 50.09%
Val. Loss: 0.697 | Val. Acc: 50.64%
###Markdown
You may have noticed the loss is not really decreasing and the accuracy is poor. This is due to several issues with the model which we'll improve in the next notebook.Finally, the metric we actually care about, the test loss and accuracy, which we get from our parameters that gave us the best validation loss.
###Code
model.load_state_dict(torch.load('tut1-model.pt'))
test_loss, test_acc = evaluate(model, test_iterator, criterion)
print(f'Test Loss: {test_loss:.3f} | Test Acc: {test_acc*100:.2f}%')
###Output
Test Loss: 0.709 | Test Acc: 47.52%
|
homework/HW_ExtraPractice_2.ipynb | ###Markdown
HW Addendum 2 Imports
###Code
import numpy as np
import pandas as pd
import matplotlib.pyplot as plt
import seaborn as sns
import statsmodels.api as sm
from sklearn.linear_model import LinearRegression, Lasso, Ridge, LassoCV, RidgeCV
from statsmodels.tsa.stattools import adfuller
import yfinance as yf
from scipy import stats
from arch import arch_model
from arch.univariate import GARCH, EWMAVariance
sns.set(font_scale = 1.2, rc={'figure.figsize':(12,8)})
import warnings
warnings.filterwarnings('ignore')
###Output
_____no_output_____
###Markdown
Data
###Code
df = pd.read_excel('../data/treasury_rates.xls').dropna()
df = df.set_index('Date')
df.head()
df2 = yf.download("TSLA AAPL MSFT XOM NFLX PYPL T WMT F C")['Adj Close'].dropna()
returns = df2.pct_change().dropna().loc['2016-01':'2021-06']
returns.head()
###Output
[*********************100%***********************] 10 of 10 completed
###Markdown
1 Regression 1. Run the following OLS regression for all data points and report the $R^{2}$. Let us exclude $y_{t−1}^{1yr}$ as a factor to better examine how OLS and LASSO regression models behave.
###Code
### Make a regression function that outputs parameters and r-squared
def reg(x,y,intercept):
if intercept == True:
x = sm.add_constant(x)
run_reg = sm.OLS(y,x).fit()
output_df = run_reg.params.to_frame('Parameters')
output_df.loc[r'$R^{2}$'] = run_reg.rsquared
return output_df
### Shift our regressors
x = df[df.columns[1:]].shift(1).dropna()
### Make the dataframes the same size so we can use the regression package
y = df['1 year'].iloc[1:].to_frame('1 year')
reg(x, y, True)
###Output
_____no_output_____
###Markdown
2. Why might this $R^{2}$ be misleading when assessing our ability to forecast the 1 year treasury?Treasury yields are not stationary processes. The level of a treasury yield tomorrow is highly dependent on the treasury yield level today, and the $R^{2}$ reflects this, not whether we can forecast where the yield *moves*. 3. Run LASSO regression for the same regressand and regresssors with a parameter of 0.05. Report the $\alpha$, $\beta$'s, and the $R^{2}$
###Code
model_lasso = Lasso(alpha=.05).fit(x,y)
lasso_res = pd.DataFrame(data = model_lasso.coef_, index = x.columns, columns = ['Parameters'])
lasso_res.loc['Intercept'] = model_lasso.intercept_
round(lasso_res, 3)
print('Lasso r-squared: ' + str(round(model_lasso.score(x,y),3)))
###Output
Lasso r-squared: 0.975
###Markdown
1.4 What would you expect the non-diagonal entries of the covariance matrix of the regressors to look like? Do you think it makes more sense to use the OLS or LASSO model here? - Treasury yields of different tenors are highly correlated, so we have non-zero values in the non-diagonal entries of the covariance matrix. We can suspect this by the magnitude of the regressors and $R^{2}$ in the OLS model vs. the LASSO model, and also by what the securities themselves are (e.g. part of the 2 year treasury yield is based off of what the 1 year yield is). - It makes sense to use LASSO here, as it deals with the multicollinearity exhibited by the OLS regression. The OLS assumption of independent regressors fails in this case.
###Code
x.corr()
x.cov()
###Output
_____no_output_____
###Markdown
2 1. Conduct and interpret the Augmented Dickey Fuller Test for the $y^{1yr}$ timeseries and conclude whether it is a stationary process. (*hint: see the stationarity jupyter notebook*)
###Code
ADF = adfuller(df['1 year'].dropna())
ADF_res = pd.DataFrame(data = [ADF[0],ADF[1]], index = ['Test statistic', 'p-value'], columns = ['ADF Test Results'])
round(ADF_res,3)
###Output
_____no_output_____
###Markdown
At $\alpha = 0.05$ we fail to reject the null hypothesis of non-stationarity. 2.
###Code
delta_yield = df['1 year'].diff().dropna().to_frame(r'$\Delta y^{1 yr}$')
delta_yield.head()
ADF2 = adfuller(df['1 year'].diff().dropna())
ADF_res2 = pd.DataFrame(data = [ADF2[0],ADF2[1]], index = ['Test statistic', 'p-value'], columns = ['ADF Test Results'])
round(ADF_res2,3)
###Output
_____no_output_____
###Markdown
At $\alpha = 0.05$ we reject the null hypothesis of non-stationarity. **3.** Differencing works. With respect to detrending, we have to consider the following question:- Are we confident on a trend when it comes to yields? And as an extension of this question:- While yields have decreased in past years, do we expect this to continue? **It's hard to say where yields will be in the future.**- Is it rational to say in a future time period yields will be very negative? **No, we can think about yields as being bounded.** Yields are more cyclical, and differencing makes much more sense here than detrending. 3 1. Fit an Expanding Series volatility model for the $\Delta y^{1yr}$ data that starts when $t = 40$
###Code
var = (delta_yield.shift(1)**2).expanding(min_periods = 40).mean()
var.dropna().head()
###Output
_____no_output_____
###Markdown
2. Fit a GARCH(1,1) model for $\Delta y^{1yr}$. When forecasting use the initial parameter $\sigma = 0.015$
###Code
GARCH = arch_model(delta_yield, vol='Garch', p=1, o=0, q=1, dist='Normal')
GARCH_model = GARCH.fit()
GARCH_model.params
var['GARCH'] = None
### Initialize with the given parameter
var.iloc[0,1:] = 0.015**2
### Simulate the rest of the period using the initial variance given
for i in range(1, len(var)):
### Forecast variance by plugging the GARCH and IGARCH parameters into their respective equations
var['GARCH'].iloc[i] = (GARCH_model.params['omega'] + var['GARCH'].iloc[i-1] * GARCH_model.params['beta[1]'] + GARCH_model.params['alpha[1]']*(delta_yield.iloc[i-1]**2))[0]
var = var.dropna()
var.head()
###Output
_____no_output_____
###Markdown
3. Forecast and plot $\sigma_{t}$
###Code
(var**.5).plot()
plt.ylabel(r'$\sigma$')
plt.title('Volatility Forecast')
plt.show()
###Output
_____no_output_____
###Markdown
4. Given only the volatility prediction plot, would you expect high volatility in the bond markets in 2020? What about in 2021? Which model do you think performed better when it came to predicting daily volatility? Given by how much the expanding volatility prediction jumps over the course of 2020, we expect high volatility in the bond markets for the first part of 2020. The subsequent consistent decrease of the expanding prediction suggests much lower volatility in latter part of 2020 and all of 2021. The GARCH prediction suggests the same scenario. GARCH volatility predictions are high for the first part of 2020 and then very low in the later stages of 2020 and in 2021. We can be confident GARCH performed much better, as it will excel in time periods where volatility clusters. In the first half of 2020 we have high volatility for an extended period of time, and then from the second half of 2020 onwards we have seen low volatility. As GARCH adjusts predictions based on recent vol, it will predict significantly more accurate in time periods such as this. We expect these movements not just in the treasury bond markets but also in the bond market as a whole to some degree, due to the fact that part of the yields of many bonds can be attributed to a risk free return (the treasury yield). Forward Step Regression
###Code
y = returns['TSLA'].to_frame('TSLA')
x = returns.copy().drop(columns = 'TSLA')
x.head()
###Output
_____no_output_____
###Markdown
1. Build an algorithm to select our final 5 factor model and report the coefficients
###Code
coefs = []
securities = list(x.columns)
### Stop when we have 5 coefficients
while len(coefs) < 5:
### Set an initial max R2
max_r2 = ('Initial', -np.inf)
### Loop through the securities that are not coefficients yet
for security in securities:
### Temporary regression to run and check R2
temp_coefs = coefs + [security]
### Run the regression and grab R2
x_current = sm.add_constant(x[temp_coefs])
r2 = sm.OLS(y, x_current).fit().rsquared
### Keep track of the current max R2 and temporary coefficient
if r2 > max_r2[1]:
max_r2 = (security, r2)
### When we have a coefficient to add:
### We remove it from the coefficients we want to test and add it to the coefficient list
securities.remove(max_r2[0])
coefs.append(max_r2[0])
x_current = sm.add_constant(x[coefs])
sm.OLS(y, x_current).fit().params.to_frame('Parameters')
###Output
_____no_output_____
###Markdown
2. What security is most correlated to TSLA? Can we know this from our final model?
###Code
returns.corr().drop(index = 'TSLA')['TSLA'].to_frame('TSLA').sort_values('TSLA', ascending = False)
###Output
_____no_output_____
###Markdown
MSFT is the highest correlated to TSLA.
###Code
sm.OLS(y, x_current).fit().summary()
###Output
_____no_output_____
###Markdown
We do not know this from running our final 5 factor regression. As can seen from the above regression summary we cannot conclude that MSFT is the most correlated to TSLA. We know that MSFT is that most correlated to TSLA because it is the first coefficient we select. We know that in a univariate regression to explain the variation of TSLA, MSFT had the highest correlation, as that is the methodology for our model. 3. Report $R^{2}$ of the final model
###Code
print('Final Model R-squared: ' + str(round(sm.OLS(y, x_current).fit().rsquared, 3)))
###Output
Final Model R-squared: 0.231
###Markdown
4. What may be some potential complications of this model? - Number of coefficients & multicollinearity: We arbitrarily set a number of coefficients without doing any analysis to see if that is the appropriate amount of coefficients. For example, perhaps a model with 2 factors or 6 factors would perform better. Furthermore, our coefficients are correlated to each other and our covariance matrix is not robust as statsmodels warns us. We do not account for multicollinearity. - We are just fitting our model in sample. We do no cross validation or out of sample testing.
###Code
round(returns.corr(), 2)
###Output
_____no_output_____ |
Space_Y_002_SpaceX_Data_Collecting.ipynb | ###Markdown
**SpaceX Falcon 9 first stage Landing Prediction** Lab 1: Collecting the data Estimated time needed: **45** minutes In this capstone, we will predict if the Falcon 9 first stage will land successfully. SpaceX advertises Falcon 9 rocket launches on its website with a cost of 62 million dollars; other providers cost upward of 165 million dollars each, much of the savings is because SpaceX can reuse the first stage. Therefore if we can determine if the first stage will land, we can determine the cost of a launch. This information can be used if an alternate company wants to bid against SpaceX for a rocket launch. In this lab, you will collect and make sure the data is in the correct format from an API. The following is an example of a successful and launch.  Several examples of an unsuccessful landing are shown here: 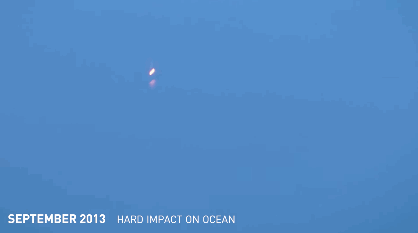 Most unsuccessful landings are planned. Space X performs a controlled landing in the oceans. Objectives In this lab, you will make a get request to the SpaceX API. You will also do some basic data wrangling and formating.* Request to the SpaceX API* Clean the requested data *** Import Libraries and Define Auxiliary Functions We will import the following libraries into the lab
###Code
# Requests allows us to make HTTP requests which we will use to get data from an API
import requests
# Pandas is a software library written for the Python programming language for data manipulation and analysis.
import pandas as pd
# NumPy is a library for the Python programming language, adding support for large, multi-dimensional arrays and matrices, along with a large collection of high-level mathematical functions to operate on these arrays
import numpy as np
# Datetime is a library that allows us to represent dates
import datetime
# Setting this option will print all collumns of a dataframe
pd.set_option('display.max_columns', None)
# Setting this option will print all of the data in a feature
pd.set_option('display.max_colwidth', None)
###Output
_____no_output_____
###Markdown
Below we will define a series of helper functions that will help us use the API to extract information using identification numbers in the launch data.From the rocket column we would like to learn the booster name.
###Code
# Takes the dataset and uses the rocket column to call the API and append the data to the list
def getBoosterVersion(data):
for x in data['rocket']:
response = requests.get("https://api.spacexdata.com/v4/rockets/"+str(x)).json()
BoosterVersion.append(response['name'])
###Output
_____no_output_____
###Markdown
From the launchpad we would like to know the name of the launch site being used, the logitude, and the latitude.
###Code
# Takes the dataset and uses the launchpad column to call the API and append the data to the list
def getLaunchSite(data):
for x in data['launchpad']:
response = requests.get("https://api.spacexdata.com/v4/launchpads/"+str(x)).json()
Longitude.append(response['longitude'])
Latitude.append(response['latitude'])
LaunchSite.append(response['name'])
###Output
_____no_output_____
###Markdown
From the payload we would like to learn the mass of the payload and the orbit that it is going to.
###Code
# Takes the dataset and uses the payloads column to call the API and append the data to the lists
def getPayloadData(data):
for load in data['payloads']:
response = requests.get("https://api.spacexdata.com/v4/payloads/"+load).json()
PayloadMass.append(response['mass_kg'])
Orbit.append(response['orbit'])
###Output
_____no_output_____
###Markdown
From cores we would like to learn the outcome of the landing, the type of the landing, number of flights with that core, whether gridfins were used, wheter the core is reused, wheter legs were used, the landing pad used, the block of the core which is a number used to seperate version of cores, the number of times this specific core has been reused, and the serial of the core.
###Code
# Takes the dataset and uses the cores column to call the API and append the data to the lists
def getCoreData(data):
for core in data['cores']:
if core['core'] != None:
response = requests.get("https://api.spacexdata.com/v4/cores/"+core['core']).json()
Block.append(response['block'])
ReusedCount.append(response['reuse_count'])
Serial.append(response['serial'])
else:
Block.append(None)
ReusedCount.append(None)
Serial.append(None)
Outcome.append(str(core['landing_success'])+' '+str(core['landing_type']))
Flights.append(core['flight'])
GridFins.append(core['gridfins'])
Reused.append(core['reused'])
Legs.append(core['legs'])
LandingPad.append(core['landpad'])
###Output
_____no_output_____
###Markdown
Now let's start requesting rocket launch data from SpaceX API with the following URL:
###Code
spacex_url="https://api.spacexdata.com/v4/launches/past"
response = requests.get(spacex_url)
###Output
_____no_output_____
###Markdown
Check the content of the response
###Code
print(response.content[:5000])
###Output
b'[{"fairings":{"reused":false,"recovery_attempt":false,"recovered":false,"ships":[]},"links":{"patch":{"small":"https://images2.imgbox.com/3c/0e/T8iJcSN3_o.png","large":"https://images2.imgbox.com/40/e3/GypSkayF_o.png"},"reddit":{"campaign":null,"launch":null,"media":null,"recovery":null},"flickr":{"small":[],"original":[]},"presskit":null,"webcast":"https://www.youtube.com/watch?v=0a_00nJ_Y88","youtube_id":"0a_00nJ_Y88","article":"https://www.space.com/2196-spacex-inaugural-falcon-1-rocket-lost-launch.html","wikipedia":"https://en.wikipedia.org/wiki/DemoSat"},"static_fire_date_utc":"2006-03-17T00:00:00.000Z","static_fire_date_unix":1142553600,"net":false,"window":0,"rocket":"5e9d0d95eda69955f709d1eb","success":false,"failures":[{"time":33,"altitude":null,"reason":"merlin engine failure"}],"details":"Engine failure at 33 seconds and loss of vehicle","crew":[],"ships":[],"capsules":[],"payloads":["5eb0e4b5b6c3bb0006eeb1e1"],"launchpad":"5e9e4502f5090995de566f86","flight_number":1,"name":"FalconSat","date_utc":"2006-03-24T22:30:00.000Z","date_unix":1143239400,"date_local":"2006-03-25T10:30:00+12:00","date_precision":"hour","upcoming":false,"cores":[{"core":"5e9e289df35918033d3b2623","flight":1,"gridfins":false,"legs":false,"reused":false,"landing_attempt":false,"landing_success":null,"landing_type":null,"landpad":null}],"auto_update":true,"tbd":false,"launch_library_id":null,"id":"5eb87cd9ffd86e000604b32a"},{"fairings":{"reused":false,"recovery_attempt":false,"recovered":false,"ships":[]},"links":{"patch":{"small":"https://images2.imgbox.com/4f/e3/I0lkuJ2e_o.png","large":"https://images2.imgbox.com/be/e7/iNqsqVYM_o.png"},"reddit":{"campaign":null,"launch":null,"media":null,"recovery":null},"flickr":{"small":[],"original":[]},"presskit":null,"webcast":"https://www.youtube.com/watch?v=Lk4zQ2wP-Nc","youtube_id":"Lk4zQ2wP-Nc","article":"https://www.space.com/3590-spacex-falcon-1-rocket-fails-reach-orbit.html","wikipedia":"https://en.wikipedia.org/wiki/DemoSat"},"static_fire_date_utc":null,"static_fire_date_unix":null,"net":false,"window":0,"rocket":"5e9d0d95eda69955f709d1eb","success":false,"failures":[{"time":301,"altitude":289,"reason":"harmonic oscillation leading to premature engine shutdown"}],"details":"Successful first stage burn and transition to second stage, maximum altitude 289 km, Premature engine shutdown at T+7 min 30 s, Failed to reach orbit, Failed to recover first stage","crew":[],"ships":[],"capsules":[],"payloads":["5eb0e4b6b6c3bb0006eeb1e2"],"launchpad":"5e9e4502f5090995de566f86","flight_number":2,"name":"DemoSat","date_utc":"2007-03-21T01:10:00.000Z","date_unix":1174439400,"date_local":"2007-03-21T13:10:00+12:00","date_precision":"hour","upcoming":false,"cores":[{"core":"5e9e289ef35918416a3b2624","flight":1,"gridfins":false,"legs":false,"reused":false,"landing_attempt":false,"landing_success":null,"landing_type":null,"landpad":null}],"auto_update":true,"tbd":false,"launch_library_id":null,"id":"5eb87cdaffd86e000604b32b"},{"fairings":{"reused":false,"recovery_attempt":false,"recovered":false,"ships":[]},"links":{"patch":{"small":"https://images2.imgbox.com/3d/86/cnu0pan8_o.png","large":"https://images2.imgbox.com/4b/bd/d8UxLh4q_o.png"},"reddit":{"campaign":null,"launch":null,"media":null,"recovery":null},"flickr":{"small":[],"original":[]},"presskit":null,"webcast":"https://www.youtube.com/watch?v=v0w9p3U8860","youtube_id":"v0w9p3U8860","article":"http://www.spacex.com/news/2013/02/11/falcon-1-flight-3-mission-summary","wikipedia":"https://en.wikipedia.org/wiki/Trailblazer_(satellite)"},"static_fire_date_utc":null,"static_fire_date_unix":null,"net":false,"window":0,"rocket":"5e9d0d95eda69955f709d1eb","success":false,"failures":[{"time":140,"altitude":35,"reason":"residual stage-1 thrust led to collision between stage 1 and stage 2"}],"details":"Residual stage 1 thrust led to collision between stage 1 and stage 2","crew":[],"ships":[],"capsules":[],"payloads":["5eb0e4b6b6c3bb0006eeb1e3","5eb0e4b6b6c3bb0006eeb1e4"],"launchpad":"5e9e4502f5090995de566f86","flight_number":3,"name":"Trailblazer","date_utc":"2008-08-03T03:34:00.000Z","date_unix":1217734440,"date_local":"2008-08-03T15:34:00+12:00","date_precision":"hour","upcoming":false,"cores":[{"core":"5e9e289ef3591814873b2625","flight":1,"gridfins":false,"legs":false,"reused":false,"landing_attempt":false,"landing_success":null,"landing_type":null,"landpad":null}],"auto_update":true,"tbd":false,"launch_library_id":null,"id":"5eb87cdbffd86e000604b32c"},{"fairings":{"reused":false,"recovery_attempt":false,"recovered":false,"ships":[]},"links":{"patch":{"small":"https://images2.imgbox.com/e9/c9/T8CfiSYb_o.png","large":"https://images2.imgbox.com/e0/a7/FNjvKlXW_o.png"},"reddit":{"campaign":null,"launch":null,"media":null,"recovery":null},"flickr":{"small":[],"original":[]},"presskit":null,"webcast":"https://www.youtube.com/watch?v=dLQ2tZEH6G0","youtube_id":"dLQ2tZEH6G0","article":"https://en.wikipedia.org/wiki/Ratsat","wikipedia":"https://en.wikipedia.'
###Markdown
You should see the response contains massive information about SpaceX launches. Next, let's try to discover some more relevant information for this project. Task 1: Request and parse the SpaceX launch data using the GET request To make the requested JSON results more consistent, we will use the following static response object for this project:
###Code
static_json_url='https://cf-courses-data.s3.us.cloud-object-storage.appdomain.cloud/IBM-DS0321EN-SkillsNetwork/datasets/API_call_spacex_api.json'
###Output
_____no_output_____
###Markdown
We should see that the request was successfull with the 200 status response code
###Code
response.status_code
###Output
_____no_output_____
###Markdown
Now we decode the response content as a Json using .json() and turn it into a Pandas dataframe using .json_normalize()
###Code
# Use json_normalize meethod to convert the json result into a dataframe
data = pd.json_normalize(response.json())
###Output
_____no_output_____
###Markdown
Using the dataframe data print the first 5 rows
###Code
# Get the head of the dataframe
data.head()
###Output
_____no_output_____
###Markdown
You will notice that a lot of the data are IDs. For example the rocket column has no information about the rocket just an identification number.We will now use the API again to get information about the launches using the IDs given for each launch. Specifically we will be using columns rocket, payloads, launchpad, and cores.
###Code
# Lets take a subset of our dataframe keeping only the features we want and the flight number, and date_utc.
data = data[['rocket', 'payloads', 'launchpad', 'cores', 'flight_number', 'date_utc']]
# We will remove rows with multiple cores because those are falcon rockets with 2 extra rocket boosters and rows that have multiple payloads in a single rocket.
data = data[data['cores'].map(len)==1]
data = data[data['payloads'].map(len)==1]
# Since payloads and cores are lists of size 1 we will also extract the single value in the list and replace the feature.
data['cores'] = data['cores'].map(lambda x : x[0])
data['payloads'] = data['payloads'].map(lambda x : x[0])
# We also want to convert the date_utc to a datetime datatype and then extracting the date leaving the time
data['date'] = pd.to_datetime(data['date_utc']).dt.date
# Using the date we will restrict the dates of the launches
data = data[data['date'] <= datetime.date(2020, 11, 13)]
###Output
_____no_output_____
###Markdown
* From the rocket we would like to learn the booster name* From the payload we would like to learn the mass of the payload and the orbit that it is going to* From the launchpad we would like to know the name of the launch site being used, the longitude, and the latitude.* From cores we would like to learn the outcome of the landing, the type of the landing, number of flights with that core, whether gridfins were used, whether the core is reused, whether legs were used, the landing pad used, the block of the core which is a number used to seperate version of cores, the number of times this specific core has been reused, and the serial of the core.The data from these requests will be stored in lists and will be used to create a new dataframe.
###Code
#Global variables
BoosterVersion = []
PayloadMass = []
Orbit = []
LaunchSite = []
Outcome = []
Flights = []
GridFins = []
Reused = []
Legs = []
LandingPad = []
Block = []
ReusedCount = []
Serial = []
Longitude = []
Latitude = []
###Output
_____no_output_____
###Markdown
These functions will apply the outputs globally to the above variables. Let's take a looks at BoosterVersion variable. Before we apply getBoosterVersion the list is empty:
###Code
BoosterVersion
###Output
_____no_output_____
###Markdown
Now, let's apply getBoosterVersion function method to get the booster version
###Code
# Call getBoosterVersion
getBoosterVersion(data)
###Output
_____no_output_____
###Markdown
the list has now been update
###Code
BoosterVersion[0:5]
###Output
_____no_output_____
###Markdown
we can apply the rest of the functions here:
###Code
# Call getLaunchSite
getLaunchSite(data)
# Call getPayloadData
getPayloadData(data)
# Call getCoreData
getCoreData(data)
###Output
_____no_output_____
###Markdown
Finally lets construct our dataset using the data we have obtained. We will combine the columns into a dictionary.
###Code
launch_dict = {'FlightNumber': list(data['flight_number']),
'Date': list(data['date']),
'BoosterVersion':BoosterVersion,
'PayloadMass':PayloadMass,
'Orbit':Orbit,
'LaunchSite':LaunchSite,
'Outcome':Outcome,
'Flights':Flights,
'GridFins':GridFins,
'Reused':Reused,
'Legs':Legs,
'LandingPad':LandingPad,
'Block':Block,
'ReusedCount':ReusedCount,
'Serial':Serial,
'Longitude': Longitude,
'Latitude': Latitude}
###Output
_____no_output_____
###Markdown
Then, we need to create a Pandas data frame from the dictionary launch_dict.
###Code
# Create a data from launch_dict
df = pd.DataFrame.from_dict(launch_dict)
###Output
_____no_output_____
###Markdown
Show the summary of the dataframe
###Code
# Show the head of the dataframe
df.head()
###Output
_____no_output_____
###Markdown
Task 2: Filter the dataframe to only include `Falcon 9` launches Finally we will remove the Falcon 1 launches keeping only the Falcon 9 launches. Filter the data dataframe using the BoosterVersion column to only keep the Falcon 9 launches. Save the filtered data to a new dataframe called data_falcon9.
###Code
data_falcon9 = df.loc[df.BoosterVersion == 'Falcon 9'].copy()
data_falcon9.shape
# Hint data['BoosterVersion']!='Falcon 1'
data_falcon9 = df.loc[df['BoosterVersion']!='Falcon 1'].reset_index(drop=True)
###Output
_____no_output_____
###Markdown
Now that we have removed some values we should reset the FlgihtNumber column
###Code
data_falcon9.loc[:,('FlightNumber')] = data_falcon9.index
data_falcon9.FlightNumber = data_falcon9.FlightNumber + 1
data_falcon9
###Output
_____no_output_____
###Markdown
Data Wrangling We can see below that some of the rows are missing values in our dataset.
###Code
data_falcon9.isnull().sum()
###Output
_____no_output_____
###Markdown
Before we can continue we must deal with these missing values. The LandingPad column will retain None values to represent when landing pads were not used. Task 3: Dealing with Missing Values Calculate below the mean for the PayloadMass using the .mean(). Then use the mean and the .replace() function to replace `np.nan` values in the data with the mean you calculated.
###Code
# Calculate the mean value of PayloadMass column
PayloadMass_mean = data_falcon9.PayloadMass.mean()
# Replace the np.nan values with its mean value
data_falcon9.loc[data_falcon9.PayloadMass.isna(), 'PayloadMass'] = PayloadMass_mean
###Output
_____no_output_____
###Markdown
You should see the number of missing values of the PayLoadMass change to zero. Now we should have no missing values in our dataset except for in LandingPad. We can now export it to a CSV for the next section,but to make the answers consistent, in the next lab we will provide data in a pre-selected date range.
###Code
# data_falcon9.to_csv('dataset_part\_1.csv', index=False)
###Output
_____no_output_____ |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.