Datasets:
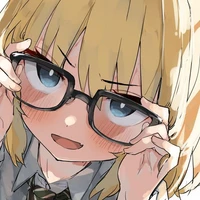
add data processing scripts and ffhq dataset; implement image-label mapping and visualization
548d832
import os | |
import sys | |
import pickle | |
from PIL import Image | |
import random | |
import matplotlib.pyplot as plt | |
# load the data(ffhq_data.pkl) | |
with open("ffhq_data.pkl", "rb") as f: | |
data = pickle.load(f) | |
# 假設 data 已經從 ffhq_data.pkl 載入 | |
# 隨機抽取 5 張圖片 | |
selected_data = random.sample(data, 5) | |
# 建立 1x5 的子圖區域,調整畫布大小以避免文字重疊 | |
fig, axes = plt.subplots(1, 5, figsize=(20, 4)) | |
for ax, item in zip(axes, selected_data): | |
img = item['img'] # PIL 圖片物件 | |
label = item['label'] # 標籤字典 | |
# 分離 headPose 與其他標籤資料 | |
head_pose = label.get("headPose", None) | |
other_labels = {k: v for k, v in label.items() if k != "headPose"} | |
# 顯示圖片 | |
ax.imshow(img) | |
ax.axis('off') | |
# 格式化 headPose 文字(若存在),並以多行呈現 | |
if head_pose: | |
head_pose_text = "headPose:\n" + "\n".join([f"{k}: {v}" for k, v in head_pose.items()]) | |
else: | |
head_pose_text = "" | |
# 格式化其他標籤文字 | |
other_text = "\n".join([f"{k}: {v}" for k, v in other_labels.items()]) | |
# 將 headPose 文字顯示在圖片上方(利用 ax.set_title) | |
if head_pose_text: | |
ax.set_title(head_pose_text, fontsize=10, pad=10) | |
# 將其他標籤文字顯示在圖片下方 | |
ax.text(0.5, -0.1, other_text, transform=ax.transAxes, fontsize=10, | |
ha='center', va='top') | |
plt.tight_layout() | |
plt.savefig("output.png", bbox_inches='tight') |