modelId
stringlengths 4
112
| sha
stringlengths 40
40
| lastModified
stringlengths 24
24
| tags
sequence | pipeline_tag
stringclasses 29
values | private
bool 1
class | author
stringlengths 2
38
⌀ | config
null | id
stringlengths 4
112
| downloads
float64 0
36.8M
⌀ | likes
float64 0
712
⌀ | library_name
stringclasses 17
values | readme
stringlengths 0
186k
| embedding
sequence |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|
ai4bharat/IndicBARTSS | 4b2669d25bc24a46ad2501c2b759451b7a4a1a26 | 2022-03-15T05:48:12.000Z | [
"pytorch",
"mbart",
"text2text-generation",
"arxiv:2109.02903",
"transformers",
"autotrain_compatible"
] | text2text-generation | false | ai4bharat | null | ai4bharat/IndicBARTSS | 2,151 | 2 | transformers | IndicBARTSS is a multilingual, sequence-to-sequence pre-trained model focusing on Indic languages and English. It currently supports 11 Indian languages and is based on the mBART architecture. You can use IndicBARTSS model to build natural language generation applications for Indian languages by finetuning the model with supervised training data for tasks like machine translation, summarization, question generation, etc. Some salient features of the IndicBARTSS are:
<ul>
<li >Supported languages: Assamese, Bengali, Gujarati, Hindi, Marathi, Odiya, Punjabi, Kannada, Malayalam, Tamil, Telugu and English. Not all of these languages are supported by mBART50 and mT5. </li>
<li >The model is much smaller than the mBART and mT5(-base) models, so less computationally expensive for finetuning and decoding. </li>
<li> Trained on large Indic language corpora (452 million sentences and 9 billion tokens) which also includes Indian English content. </li>
<li> Unlike ai4bharat/IndicBART each language is written in its own script so you do not need to perform any script mapping to/from Devanagari. </li>
</ul>
You can read more about IndicBARTSS in this <a href="https://arxiv.org/abs/2109.02903">paper</a>.
For detailed documentation, look here: https://github.com/AI4Bharat/indic-bart/ and https://indicnlp.ai4bharat.org/indic-bart/
# Pre-training corpus
We used the <a href="https://indicnlp.ai4bharat.org/corpora/">IndicCorp</a> data spanning 12 languages with 452 million sentences (9 billion tokens). The model was trained using the text-infilling objective used in mBART.
# Usage:
```
from transformers import MBartForConditionalGeneration, AutoModelForSeq2SeqLM
from transformers import AlbertTokenizer, AutoTokenizer
tokenizer = AutoTokenizer.from_pretrained("ai4bharat/IndicBARTSS", do_lower_case=False, use_fast=False, keep_accents=True)
# Or use tokenizer = AlbertTokenizer.from_pretrained("ai4bharat/IndicBARTSS", do_lower_case=False, use_fast=False, keep_accents=True)
model = AutoModelForSeq2SeqLM.from_pretrained("ai4bharat/IndicBARTSS")
# Or use model = MBartForConditionalGeneration.from_pretrained("ai4bharat/IndicBARTSS")
# Some initial mapping
bos_id = tokenizer._convert_token_to_id_with_added_voc("<s>")
eos_id = tokenizer._convert_token_to_id_with_added_voc("</s>")
pad_id = tokenizer._convert_token_to_id_with_added_voc("<pad>")
# To get lang_id use any of ['<2as>', '<2bn>', '<2en>', '<2gu>', '<2hi>', '<2kn>', '<2ml>', '<2mr>', '<2or>', '<2pa>', '<2ta>', '<2te>']
# First tokenize the input and outputs. The format below is how IndicBARTSS was trained so the input should be "Sentence </s> <2xx>" where xx is the language code. Similarly, the output should be "<2yy> Sentence </s>".
inp = tokenizer("I am a boy </s> <2en>", add_special_tokens=False, return_tensors="pt", padding=True).input_ids # tensor([[ 466, 1981, 80, 25573, 64001, 64004]])
out = tokenizer("<2hi> मैं एक लड़का हूँ </s>", add_special_tokens=False, return_tensors="pt", padding=True).input_ids # tensor([[64006, 942, 43, 32720, 8384, 64001]])
model_outputs=model(input_ids=inp, decoder_input_ids=out[:,0:-1], labels=out[:,1:])
# For loss
model_outputs.loss ## This is not label smoothed.
# For logits
model_outputs.logits
# For generation. Pardon the messiness. Note the decoder_start_token_id.
model.eval() # Set dropouts to zero
model_output=model.generate(inp, use_cache=True, num_beams=4, max_length=20, min_length=1, early_stopping=True, pad_token_id=pad_id, bos_token_id=bos_id, eos_token_id=eos_id, decoder_start_token_id=tokenizer._convert_token_to_id_with_added_voc("<2en>"))
# Decode to get output strings
decoded_output=tokenizer.decode(model_output[0], skip_special_tokens=True, clean_up_tokenization_spaces=False)
print(decoded_output) # I am a boy
# What if we mask?
inp = tokenizer("I am [MASK] </s> <2en>", add_special_tokens=False, return_tensors="pt", padding=True).input_ids
model_output=model.generate(inp, use_cache=True, num_beams=4, max_length=20, min_length=1, early_stopping=True, pad_token_id=pad_id, bos_token_id=bos_id, eos_token_id=eos_id, decoder_start_token_id=tokenizer._convert_token_to_id_with_added_voc("<2en>"))
decoded_output=tokenizer.decode(model_output[0], skip_special_tokens=True, clean_up_tokenization_spaces=False)
print(decoded_output) # I am happy
inp = tokenizer("मैं [MASK] हूँ </s> <2hi>", add_special_tokens=False, return_tensors="pt", padding=True).input_ids
model_output=model.generate(inp, use_cache=True, num_beams=4, max_length=20, min_length=1, early_stopping=True, pad_token_id=pad_id, bos_token_id=bos_id, eos_token_id=eos_id, decoder_start_token_id=tokenizer._convert_token_to_id_with_added_voc("<2en>"))
decoded_output=tokenizer.decode(model_output[0], skip_special_tokens=True, clean_up_tokenization_spaces=False)
print(decoded_output) # मैं जानता हूँ
inp = tokenizer("मला [MASK] पाहिजे </s> <2mr>", add_special_tokens=False, return_tensors="pt", padding=True).input_ids
model_output=model.generate(inp, use_cache=True, num_beams=4, max_length=20, min_length=1, early_stopping=True, pad_token_id=pad_id, bos_token_id=bos_id, eos_token_id=eos_id, decoder_start_token_id=tokenizer._convert_token_to_id_with_added_voc("<2en>"))
decoded_output=tokenizer.decode(model_output[0], skip_special_tokens=True, clean_up_tokenization_spaces=False)
print(decoded_output) # मला ओळखलं पाहिजे
```
# Notes:
1. This is compatible with the latest version of transformers but was developed with version 4.3.2 so consider using 4.3.2 if possible.
2. While I have only shown how to get logits and loss and how to generate outputs, you can do pretty much everything the MBartForConditionalGeneration class can do as in https://huggingface.co/docs/transformers/model_doc/mbart#transformers.MBartForConditionalGeneration
3. Note that the tokenizer I have used is based on sentencepiece and not BPE. Therefore, I used the AlbertTokenizer class and not the MBartTokenizer class.
# Fine-tuning on a downstream task
1. If you wish to fine-tune this model, then you can do so using the <a href="https://github.com/prajdabre/yanmtt">YANMTT</a> toolkit, following the instructions <a href="https://github.com/AI4Bharat/indic-bart ">here</a>.
2. (Untested) Alternatively, you may use the official huggingface scripts for <a href="https://github.com/huggingface/transformers/tree/master/examples/pytorch/translation">translation</a> and <a href="https://github.com/huggingface/transformers/tree/master/examples/pytorch/summarization">summarization</a>.
# Contributors
<ul>
<li> Raj Dabre </li>
<li> Himani Shrotriya </li>
<li> Anoop Kunchukuttan </li>
<li> Ratish Puduppully </li>
<li> Mitesh M. Khapra </li>
<li> Pratyush Kumar </li>
</ul>
# Paper
If you use IndicBARTSS, please cite the following paper:
```
@misc{dabre2021indicbart,
title={IndicBART: A Pre-trained Model for Natural Language Generation of Indic Languages},
author={Raj Dabre and Himani Shrotriya and Anoop Kunchukuttan and Ratish Puduppully and Mitesh M. Khapra and Pratyush Kumar},
year={2021},
eprint={2109.02903},
archivePrefix={arXiv},
primaryClass={cs.CL}
}
```
# License
The model is available under the MIT License. | [
-0.05840491130948067,
-0.0971253290772438,
0.027299297973513603,
-0.05429568514227867,
-0.026637300848960876,
0.022271329537034035,
-0.10089723765850067,
0.02821085788309574,
0.03767402097582817,
-0.016876831650733948,
-0.00884261354804039,
-0.0740542784333229,
0.0491076223552227,
-0.022409532219171524,
0.06983725726604462,
0.022273452952504158,
-0.007336476817727089,
0.01934920810163021,
-0.034091055393218994,
-0.10390757769346237,
0.06381461024284363,
0.05524540692567825,
-0.011475186794996262,
-0.039712853729724884,
0.04742669686675072,
0.01618480309844017,
0.03615204244852066,
-0.06677787750959396,
0.038119833916425705,
-0.04161613807082176,
0.055203016847372055,
0.02832677587866783,
0.023685868829488754,
0.026905573904514313,
-0.08851195126771927,
-0.04248904436826706,
-0.021343380212783813,
0.039033472537994385,
0.03647761419415474,
-0.0315832793712616,
-0.07450319826602936,
-0.001927845412865281,
-0.0026789195835590363,
0.015376750379800797,
0.11904140561819077,
-0.04553904011845589,
-0.10364268720149994,
0.029356343671679497,
-0.061075691133737564,
0.018315929919481277,
-0.09264234453439713,
0.03651436045765877,
0.04448145255446434,
0.007183059584349394,
-0.025484884157776833,
-0.16929370164871216,
-0.05763368308544159,
-0.006686049047857523,
0.03064591810107231,
-0.0028813856188207865,
-0.023068631067872047,
-0.01733757182955742,
-0.06273724138736725,
0.07967706024646759,
0.004368811845779419,
0.00400654599070549,
0.0530758872628212,
0.05545993894338608,
0.04102844372391701,
-0.033647824078798294,
-0.02706369385123253,
-0.05264916270971298,
0.02394532971084118,
0.11683057993650436,
-0.008369644172489643,
0.04710690677165985,
0.08476996421813965,
0.0019032839918509126,
-0.024487819522619247,
-0.05559663102030754,
-0.009966121055185795,
0.09677054733037949,
0.13108307123184204,
-0.006786115001887083,
-0.0005502357380464673,
-0.023469150066375732,
0.012343821115791798,
0.11795546859502792,
-0.0028519246261566877,
0.0040937974117696285,
0.05756591632962227,
-0.025971828028559685,
0.013599648140370846,
0.03323016315698624,
0.027105819433927536,
0.05407457798719406,
-0.004635162185877562,
-0.04074717313051224,
-0.034228164702653885,
0.0352935791015625,
0.03997843712568283,
0.038951046764850616,
-0.02148222178220749,
-0.03258388862013817,
-0.13646550476551056,
-0.06335955858230591,
0.08262713253498077,
-0.033655934035778046,
0.022833898663520813,
-0.04692975431680679,
0.02776837721467018,
-0.019163629040122032,
-0.06765683740377426,
-0.020468933507800102,
0.010237090289592743,
-0.023167645558714867,
-0.001581060467287898,
-0.0044369082897901535,
0.07662175595760345,
0.006160714663565159,
-0.12243498861789703,
0.044150788336992264,
-0.006657202262431383,
0.043508131057024,
-0.06499209254980087,
-0.051100682467222214,
-0.022372432053089142,
3.14128714535583e-33,
0.0037702969275414944,
-0.01986922137439251,
-0.043244462460279465,
-0.02149149775505066,
-0.037634894251823425,
-0.07425426691770554,
-0.008459752425551414,
-0.01057754922658205,
-0.04778014495968819,
-0.05392369627952576,
0.0443744957447052,
-0.008396925404667854,
-0.054779037833213806,
0.041019849479198456,
0.0812125951051712,
-0.02143937721848488,
0.005112580023705959,
0.0032396886963397264,
0.03343391790986061,
0.00767887756228447,
0.008504187688231468,
0.05172960087656975,
0.0934862494468689,
-0.009891725145280361,
0.04721662029623985,
0.0744871124625206,
0.16247498989105225,
-0.05564303696155548,
-0.021024497225880623,
0.032226793467998505,
-0.05106654763221741,
-0.05396385118365288,
-0.024326032027602196,
-0.014862457290291786,
-0.04505838453769684,
0.007892526686191559,
-0.02734082005918026,
-0.027607498690485954,
-0.018200252205133438,
0.007223861292004585,
-0.02650987170636654,
-0.0029446862172335386,
-0.04714305326342583,
0.005135309416800737,
-0.013038706965744495,
-0.0009172147838398814,
-0.02336118184030056,
0.04857730492949486,
-0.025239456444978714,
0.014362625777721405,
-0.045696768909692764,
0.09383152425289154,
-0.042194679379463196,
0.0057082888670265675,
0.07990395277738571,
0.08776693791151047,
0.011586503125727177,
-0.008427157066762447,
0.022498849779367447,
-0.01896638423204422,
0.02318466082215309,
-0.022982366383075714,
0.06058218702673912,
0.001048840000294149,
0.08350639045238495,
-0.00965418852865696,
-0.03168507292866707,
0.026194419711828232,
0.085500568151474,
-0.04953218251466751,
-0.03322508558630943,
-0.04639710858464241,
0.025458937510848045,
0.05399378016591072,
-0.029174622148275375,
0.01442805491387844,
0.026959128677845,
-0.025651033967733383,
-0.005707037169486284,
0.07240753620862961,
0.012571228668093681,
-0.009568244218826294,
0.016904786229133606,
-0.06673324108123779,
0.05728689581155777,
-0.014097359031438828,
0.0000816149331512861,
0.013981136493384838,
-0.037232525646686554,
-0.04955585300922394,
0.062023505568504333,
-0.010807255282998085,
0.023522965610027313,
0.045905355364084244,
0.01794569380581379,
-4.048115282799976e-33,
-0.006533443462103605,
-0.006548680830746889,
-0.05981966853141785,
0.05868011713027954,
-0.08326797932386398,
-0.07946081459522247,
-0.0023313334677368402,
0.08052578568458557,
-0.03667239844799042,
0.0439273975789547,
-0.013948754407465458,
-0.02254808321595192,
0.07712724059820175,
-0.011456454172730446,
0.06582463532686234,
-0.007209246978163719,
0.005706766154617071,
0.008640622720122337,
0.0140038738027215,
0.0534343495965004,
-0.02549293264746666,
0.05320601910352707,
-0.08591809868812561,
-0.007956097833812237,
-0.0126586202532053,
0.05219118669629097,
-0.09001807868480682,
0.0014624384930357337,
-0.0652349516749382,
-0.041601117700338364,
0.03787035122513771,
0.028433561325073242,
-0.024137768894433975,
-0.031813353300094604,
-0.054925642907619476,
-0.013188608922064304,
0.06205657869577408,
-0.026815690100193024,
-0.0701136440038681,
0.11979647725820541,
0.0438200905919075,
0.11087450385093689,
-0.04820513725280762,
-0.08383439481258392,
-0.01871705800294876,
0.00020062287512701005,
-0.04511271044611931,
-0.025589486584067345,
0.06656350195407867,
-0.070426344871521,
0.06953798979520798,
0.0030826041474938393,
0.006799252238124609,
-0.06317088752985,
0.054230790585279465,
-0.09468274563550949,
-0.013185541145503521,
0.012112847529351711,
-0.05495579168200493,
-0.09848231077194214,
-0.1036481261253357,
-0.05836586654186249,
0.13784366846084595,
-0.07599897682666779,
0.07262419909238815,
-0.007830438204109669,
-0.0028279514517635107,
0.015230396762490273,
-0.013778739608824253,
-0.07639934867620468,
0.01630227267742157,
-0.08639821410179138,
-0.010362546890974045,
-0.012998681515455246,
-0.031207436695694923,
0.03380134329199791,
0.039848316460847855,
-0.0845177099108696,
-0.0442349910736084,
-0.08048581331968307,
0.007746138144284487,
0.023132754489779472,
0.044351547956466675,
-0.006085276138037443,
0.01361026894301176,
0.004269350320100784,
0.04941888898611069,
0.01154640968888998,
0.010237800888717175,
0.04666372761130333,
0.036900319159030914,
0.09040289372205734,
0.07799341529607773,
0.11676246672868729,
-0.02999696508049965,
-4.518026841537903e-8,
-0.018921880051493645,
-0.06073511764407158,
-0.10012602806091309,
0.061517808586359024,
-0.06274446845054626,
-0.07831926643848419,
-0.06332366168498993,
-0.02058197371661663,
0.013489984907209873,
-0.037904176861047745,
-0.02070068009197712,
-0.029728930443525314,
-0.07220825552940369,
0.04225683584809303,
0.041588328778743744,
0.005361753515899181,
0.05040321871638298,
0.029463665559887886,
0.028565624728798866,
-0.06027927249670029,
0.04268472269177437,
0.01898757554590702,
0.05041428282856941,
0.09588634967803955,
-0.018121810629963875,
0.005591197405010462,
-0.0758100375533104,
-0.03511304408311844,
0.09135894477367401,
-0.04077455401420593,
-0.04642188549041748,
0.043863486498594284,
0.017737234011292458,
-0.07513837516307831,
0.043553225696086884,
0.04343133047223091,
0.03011244907975197,
-0.024510610848665237,
0.030953189358115196,
0.06771314144134521,
0.08237103372812271,
-0.022963490337133408,
-0.04526916518807411,
0.008760705590248108,
0.0088396817445755,
-0.053255077451467514,
-0.05285883694887161,
-0.028053870424628258,
-0.03144514560699463,
-0.09011255204677582,
-0.0741540789604187,
-0.014225422404706478,
-0.0028493732679635286,
-0.00042666762601584196,
0.05818115174770355,
0.07554758340120316,
-0.06741760671138763,
-0.020378507673740387,
0.1209733784198761,
0.043516431003808975,
0.004669609945267439,
0.02544267475605011,
0.021387549117207527,
-0.02851998247206211
] |
zjukg/OntoProtein | d27d23e56e1b565958a5016eaf82847fa08a427a | 2022-04-12T14:42:54.000Z | [
"pytorch",
"bert",
"fill-mask",
"protein",
"dataset:ProteinKG25",
"transformers",
"protein language model",
"autotrain_compatible"
] | fill-mask | false | zjukg | null | zjukg/OntoProtein | 2,150 | 3 | transformers | ---
language: protein
tags:
- protein language model
datasets:
- ProteinKG25
widget:
- text: "D L I P T S S K L V V [MASK] D T S L Q V K K A F F A L V T"
---
# OntoProtein model
Pretrained model on protein sequences using masked language modeling (MLM) and knowledge embedding (KE) objective objective. It was introduced in [this paper](https://openreview.net/pdf?id=yfe1VMYAXa4) and first released in [this repository](https://github.com/zjunlp/OntoProtein). This model is trained on uppercase amino acids: it only works with capital letter amino acids.
## Model description
OntoProtein is the first general framework that makes use of structure in GO (Gene Ontology) into protein pre-training models. We construct a novel large-scale knowledge graph that consists of GO and its related proteins, and gene annotation texts or protein sequences describe all nodes in the graph. We propose novel contrastive learning with knowledge-aware negative sampling to jointly optimize the knowledge graph and protein embedding during pre-training.
### BibTeX entry and citation info
```bibtex
@article{zhang2022ontoprotein,
title={OntoProtein: Protein Pretraining With Gene Ontology Embedding},
author={Zhang, Ningyu and Bi, Zhen and Liang, Xiaozhuan and Cheng, Siyuan and Hong, Haosen and Deng, Shumin and Lian, Jiazhang and Zhang, Qiang and Chen, Huajun},
journal={arXiv preprint arXiv:2201.11147},
year={2022}
}
```
| [
-0.06640700995922089,
-0.11526701599359512,
-0.023672766983509064,
0.014363388530910015,
0.016232436522841454,
0.04524272680282593,
0.011677848175168037,
0.026764053851366043,
0.0066252765245735645,
-0.03422898054122925,
0.014869536273181438,
-0.034928224980831146,
0.05607284978032112,
0.14798906445503235,
-0.019055040553212166,
0.07581619918346405,
0.07013801485300064,
0.06009924039244652,
-0.03962375596165657,
-0.0766763687133789,
0.06350214034318924,
0.0815623477101326,
0.0365266390144825,
-0.03990890085697174,
-0.04295553267002106,
-0.03043113835155964,
-0.04281298816204071,
-0.047038283199071884,
0.022583644837141037,
0.014741063117980957,
0.026878898963332176,
-0.04774293676018715,
0.053362663835287094,
0.04083995148539543,
-0.009994958527386189,
0.07063081860542297,
-0.06283536553382874,
0.005810330621898174,
0.019816311076283455,
0.009472844190895557,
-0.006155930459499359,
0.03373408690094948,
-0.00711318152025342,
0.049146756529808044,
0.059675924479961395,
0.07405520230531693,
-0.03992670774459839,
0.024755725637078285,
0.04419313743710518,
0.05392134189605713,
-0.11363445222377777,
-0.09709855169057846,
-0.06575067341327667,
0.011603300459682941,
0.047287531197071075,
0.052215661853551865,
-0.06184079125523567,
-0.05752161890268326,
-0.02499757707118988,
-0.052361153066158295,
-0.00954697746783495,
-0.09381367266178131,
-0.004564951173961163,
-0.05209898576140404,
-0.0076025789603590965,
-0.03720086067914963,
0.007605958264321089,
0.08613867312669754,
-0.015124375000596046,
0.06832181662321091,
0.01597822643816471,
0.07138049602508545,
-0.020342526957392693,
0.06608323752880096,
-0.04782070592045784,
0.09133661538362503,
0.01730724237859249,
0.0273332130163908,
0.02101823315024376,
-0.0987330749630928,
0.04743129760026932,
0.06119110807776451,
0.06868616491556168,
0.06683339923620224,
0.05179484933614731,
0.015318429097533226,
0.01612832583487034,
0.046861521899700165,
-0.008977117948234081,
0.008625572547316551,
-0.02183002047240734,
-0.11452370136976242,
0.07831547409296036,
-0.015777407214045525,
0.023842718452215195,
0.01250846590846777,
0.056416578590869904,
-0.04546310380101204,
-0.030852917581796646,
0.07995794713497162,
-0.0025374186225235462,
0.03586984798312187,
0.027083296328783035,
-0.08175522089004517,
-0.01613057218492031,
-0.0017917741788551211,
-0.060016363859176636,
0.008589853532612324,
0.07228481769561768,
-0.08672325313091278,
0.00556191336363554,
0.10407233238220215,
0.03312582150101662,
-0.019063662737607956,
0.015115680173039436,
0.018712138757109642,
0.07406062632799149,
-0.06781931966543198,
0.03880113363265991,
0.042934805154800415,
-0.04764806851744652,
0.036398548632860184,
0.04613328352570534,
0.03102869540452957,
-0.028016377240419388,
-0.015706855803728104,
-0.08322162926197052,
1.1120448391847467e-33,
0.0743563324213028,
0.019171694293618202,
0.06278179585933685,
0.017062004655599594,
-0.0026955706998705864,
-0.04199327901005745,
-0.003764060325920582,
0.04557325690984726,
-0.06767084449529648,
-0.045188046991825104,
0.004615066573023796,
-0.031787700951099396,
-0.007545033935457468,
0.02569754607975483,
-0.024517744779586792,
-0.006357010919600725,
-0.014697614125907421,
0.01785981096327305,
-0.033231474459171295,
-0.03112841583788395,
0.021414656192064285,
0.03111674264073372,
-0.004009857773780823,
0.006876446306705475,
0.00482437526807189,
0.04175678640604019,
0.029628733173012733,
-0.12378007173538208,
-0.007636892609298229,
0.016104169189929962,
-0.17233625054359436,
0.002045699628069997,
0.005856485106050968,
0.03212238848209381,
0.02185489982366562,
-0.06860329210758209,
0.0137329725548625,
-0.09744377434253693,
0.008828891441226006,
-0.05927376449108124,
0.006242471747100353,
0.03129630535840988,
-0.04146592691540718,
-0.06122419983148575,
-0.031105125322937965,
-0.046973202377557755,
0.038655154407024384,
-0.04384082928299904,
0.02621861919760704,
-0.036328673362731934,
0.06773845106363297,
-0.037686821073293686,
0.016191832721233368,
-0.038470447063446045,
-0.0669993981719017,
0.030124755576252937,
-0.022275852039456367,
0.08070441335439682,
0.061778321862220764,
0.03724437952041626,
-0.043668266385793686,
-0.03548041731119156,
0.047929417341947556,
0.05812889337539673,
0.030806023627519608,
0.012416179291903973,
-0.12496115267276764,
-0.0054710679687559605,
0.03273891285061836,
0.02482018433511257,
-0.008093217387795448,
0.010974033735692501,
0.0013300558784976602,
-0.04434534162282944,
-0.006670703645795584,
0.0025600530207157135,
-0.03431226685643196,
-0.0825321227312088,
-0.05841892212629318,
0.10138723999261856,
0.003018143819645047,
0.0024844652507454157,
-0.10922566056251526,
-0.10419520735740662,
-0.07515139132738113,
-0.08270333707332611,
0.1176915243268013,
-0.10541291534900665,
0.019280482083559036,
-0.06510434299707413,
0.0016303384909406304,
-0.043716538697481155,
-0.04928047955036163,
0.03751731291413307,
0.04817427694797516,
-1.9344115034704033e-33,
0.016351308673620224,
-0.014977023005485535,
-0.005191665142774582,
0.025292659178376198,
-0.006396343931555748,
-0.09072546660900116,
0.04858947917819023,
0.08565693348646164,
-0.02477118745446205,
-0.01178252138197422,
0.026471570134162903,
-0.11226662248373032,
-0.020096980035305023,
-0.055473219603300095,
0.026540607213974,
0.03514708951115608,
-0.06514066457748413,
0.07798773050308228,
0.023573948070406914,
0.08564884215593338,
-0.07947107404470444,
0.002888381015509367,
-0.1067974716424942,
0.07962116599082947,
0.04928796738386154,
0.09903883188962936,
-0.013750632293522358,
0.11442703753709793,
-0.024266868829727173,
0.03078315407037735,
-0.056161776185035706,
0.025340568274259567,
-0.041549164801836014,
-0.009749448858201504,
-0.1020258218050003,
0.040181972086429596,
0.01946958899497986,
-0.03257311135530472,
0.04778105765581131,
-0.030197927728295326,
0.04914683848619461,
0.05772635340690613,
-0.02491547353565693,
0.007459008600562811,
-0.0013453696155920625,
-0.016401205211877823,
-0.0802144929766655,
-0.008594002574682236,
0.061346009373664856,
-0.08158580213785172,
0.022587446495890617,
-0.05072818696498871,
-0.01592388190329075,
-0.05568496510386467,
-0.05256021395325661,
-0.09064942598342896,
0.01108789723366499,
0.005177656654268503,
-0.018663084134459496,
-0.01587466336786747,
-0.04037957638502121,
-0.06273914873600006,
0.009444103576242924,
0.042971134185791016,
-0.03385741636157036,
-0.02870548702776432,
-0.012956852093338966,
0.012682587839663029,
0.009702564217150211,
-0.03291987627744675,
-0.04897970333695412,
0.034532226622104645,
0.05876747891306877,
0.03518472611904144,
0.08826924115419388,
-0.0448196716606617,
0.003593884175643325,
-0.004602106288075447,
-0.041147343814373016,
-0.08958397805690765,
-0.041505273431539536,
-0.03520648181438446,
0.006729678716510534,
0.04679475352168083,
0.1137879341840744,
0.0666108950972557,
0.04061832278966904,
0.09277820587158203,
0.04428449645638466,
0.03732108697295189,
-0.049989376217126846,
-0.04174521937966347,
-0.06186063215136528,
0.11388745158910751,
0.019145285710692406,
-4.3250476977618746e-8,
-0.05349825322628021,
-0.03092813305556774,
0.0341460257768631,
-0.002362629398703575,
0.04626955837011337,
-0.008937451057136059,
-0.04416273161768913,
0.06368717551231384,
-0.019534103572368622,
-0.0178051944822073,
0.07199201732873917,
0.042565543204545975,
-0.08624773472547531,
-0.05564284697175026,
-0.05479880049824715,
0.054991740733385086,
-0.03562668338418007,
0.02542039565742016,
0.031394194811582565,
0.035920556634664536,
0.032549820840358734,
0.01536193210631609,
0.021267710253596306,
0.0475209504365921,
0.0780070573091507,
-0.09024856239557266,
-0.032877691090106964,
0.08965587615966797,
0.06312209367752075,
-0.0366714708507061,
-0.06372625380754471,
-0.031122447922825813,
-0.009321813471615314,
-0.02195669338107109,
0.03144139423966408,
0.04597083106637001,
0.028723478317260742,
-0.07212186604738235,
-0.03526904806494713,
-0.007450438570231199,
0.0608365461230278,
0.0654403418302536,
-0.0636218786239624,
-0.035024844110012054,
0.03817470371723175,
-0.0101632010191679,
-0.05467892438173294,
0.010040785185992718,
0.0276927649974823,
-0.004234231077134609,
0.026273181661963463,
-0.03175193443894386,
-0.00372262648306787,
0.010532883927226067,
-0.0013122223317623138,
0.03875505179166794,
-0.055135201662778854,
-0.030450239777565002,
0.030749041587114334,
-0.021117515861988068,
0.007263760082423687,
0.002596143167465925,
0.06309526413679123,
0.0337660014629364
] |
prajjwal1/bert-medium-mnli | 82e4a3118f63cba6e97875aa1b7e6a674a193063 | 2021-10-05T17:56:07.000Z | [
"pytorch",
"jax",
"bert",
"text-classification",
"arxiv:1908.08962",
"arxiv:2110.01518",
"transformers"
] | text-classification | false | prajjwal1 | null | prajjwal1/bert-medium-mnli | 2,149 | null | transformers | The following model is a Pytorch pre-trained model obtained from converting Tensorflow checkpoint found in the [official Google BERT repository](https://github.com/google-research/bert). These BERT variants were introduced in the paper [Well-Read Students Learn Better: On the Importance of Pre-training Compact Models](https://arxiv.org/abs/1908.08962). These models are trained on MNLI.
If you use the model, please consider citing the paper
```
@misc{bhargava2021generalization,
title={Generalization in NLI: Ways (Not) To Go Beyond Simple Heuristics},
author={Prajjwal Bhargava and Aleksandr Drozd and Anna Rogers},
year={2021},
eprint={2110.01518},
archivePrefix={arXiv},
primaryClass={cs.CL}
}
```
Original Implementation and more info can be found in [this Github repository](https://github.com/prajjwal1/generalize_lm_nli).
```
MNLI: 75.86%
MNLI-mm: 77.03%
```
These models are trained for 4 epochs.
[@prajjwal_1](https://twitter.com/prajjwal_1)
| [
-0.09788774698972702,
-0.06514044851064682,
0.04305513948202133,
0.053854115307331085,
0.026423117145895958,
0.050810474902391434,
-0.0007797605358064175,
0.016668150201439857,
-0.04501102864742279,
-0.012287932448089123,
0.006685130298137665,
0.038580089807510376,
0.021358292549848557,
0.01878148689866066,
-0.0023792090360075235,
0.03674514591693878,
-0.0009333660709671676,
-0.03087114356458187,
-0.059926100075244904,
-0.02405935898423195,
0.024990027770400047,
0.05209911987185478,
-0.02203434146940708,
-0.061362676322460175,
0.029483946040272713,
-0.08549626916646957,
-0.039729952812194824,
-0.03715015575289726,
0.10267771780490875,
0.05506744235754013,
0.061975762248039246,
0.0463872030377388,
0.04730004444718361,
0.0722469687461853,
0.0635097473859787,
0.08549873530864716,
-0.04004219174385071,
0.01351358462125063,
0.07104308903217316,
0.03724750131368637,
0.0003359560505487025,
-0.016006097197532654,
-0.02558239735662937,
-0.057748906314373016,
0.08689751476049423,
-0.06968552619218826,
-0.014961841516196728,
-0.04987373203039169,
-0.06442078202962875,
-0.03191566839814186,
-0.07537708431482315,
0.003502520965412259,
0.06949708610773087,
-0.0013229715405032039,
-0.021989313885569572,
0.0033531063236296177,
-0.027425946667790413,
-0.037609271705150604,
-0.00906334351748228,
-0.1473996341228485,
-0.12541252374649048,
-0.06768559664487839,
-0.07162073999643326,
-0.040180668234825134,
-0.03598414734005928,
0.07183584570884705,
-0.025315215811133385,
0.010802967473864555,
0.10925733298063278,
-0.007170251104980707,
-0.019552193582057953,
0.07190949469804764,
-0.03021315298974514,
0.040888331830501556,
0.0806264728307724,
-0.03482760116457939,
0.11244107782840729,
0.04007010534405708,
0.009653953835368156,
-0.09457726031541824,
-0.00700289336964488,
0.024561993777751923,
0.1179293766617775,
-0.01812363788485527,
0.038288310170173645,
0.04023471474647522,
0.010189363732933998,
0.00491721136495471,
0.10049524158239365,
-0.08056795597076416,
0.03640131279826164,
-0.04239637032151222,
-0.04626257345080376,
0.027595313265919685,
0.04128534719347954,
0.0506657212972641,
0.08899658918380737,
-0.040257588028907776,
0.013736427761614323,
0.10200124979019165,
0.07672547549009323,
0.023269737139344215,
0.047827765345573425,
-0.05542147532105446,
0.06616295129060745,
0.019845232367515564,
0.07390538603067398,
0.015656625851988792,
0.04626477509737015,
-0.04610814154148102,
0.042757272720336914,
0.011558320373296738,
0.012027928605675697,
-0.02429303713142872,
0.009131155908107758,
-0.0024075873661786318,
-0.03446345031261444,
0.007040876429527998,
-0.009704191237688065,
0.06115623936057091,
-0.019774578511714935,
-0.004150899592787027,
0.010371407493948936,
0.03690893203020096,
-0.05551765859127045,
-0.04847989231348038,
-0.0706876739859581,
7.466289755150011e-33,
0.020133117213845253,
0.026498615741729736,
-0.0030499056447297335,
-0.025648823007941246,
0.006507859565317631,
-0.0028629109729081392,
0.05314359441399574,
-0.06207125261425972,
0.03708501160144806,
-0.05941545218229294,
-0.07189537584781647,
0.013163677416741848,
-0.08291475474834442,
0.0753445252776146,
-0.035245563834905624,
-0.0022813265677541494,
-0.11505432426929474,
0.060231249779462814,
0.06526103615760803,
0.050657693296670914,
0.08094123750925064,
0.015442661941051483,
-0.006225495599210262,
-0.13206134736537933,
-0.03686416149139404,
0.0505230613052845,
0.1307280957698822,
-0.03660650923848152,
-0.045845530927181244,
0.05068325996398926,
-0.1274682581424713,
0.024932803586125374,
-0.030553115531802177,
-0.002432215726003051,
-0.025467535480856895,
0.02629098854959011,
-0.07744420319795609,
-0.037865471094846725,
-0.0022636842913925648,
-0.08490670472383499,
-0.027288740500807762,
0.11159767955541611,
-0.031549081206321716,
-0.017503106966614723,
-0.020481634885072708,
-0.04349208623170853,
0.023337071761488914,
0.02151145040988922,
0.023607907816767693,
-0.051832415163517,
-0.026202650740742683,
-0.004159054718911648,
-0.08511428534984589,
-0.07675091922283173,
-0.01575930416584015,
-0.04055444896221161,
0.0764862671494484,
0.07344821840524673,
0.07268871366977692,
0.04188734292984009,
0.06231238692998886,
0.022938130423426628,
0.013279172591865063,
0.10787027329206467,
0.06958235800266266,
-0.04518388211727142,
-0.048487283289432526,
-0.007443455513566732,
0.057593122124671936,
-0.017251327633857727,
-0.03924453258514404,
-0.04154811054468155,
-0.009896961972117424,
0.013934449292719364,
0.027389397844672203,
-0.08069821447134018,
0.046590015292167664,
-0.06783363968133926,
-0.023314088582992554,
0.001349254627712071,
0.0008446259307675064,
0.021814294159412384,
-0.08719299733638763,
-0.028128229081630707,
-0.10532345622777939,
-0.07368111610412598,
0.03936494514346123,
-0.09040196985006332,
-0.027083348482847214,
-0.03801574185490608,
0.026187235489487648,
-0.05398950353264809,
0.03874717280268669,
0.052201949059963226,
-0.08065443485975266,
-5.9998238183007446e-33,
0.044262826442718506,
0.054612983018159866,
-0.029677344486117363,
0.02387051284313202,
-0.056101903319358826,
-0.0574805773794651,
0.05710882321000099,
0.0888059213757515,
-0.021789316087961197,
-0.007561128586530685,
0.005784717388451099,
-0.006904765497893095,
0.025622963905334473,
0.0008066225564107299,
0.06716152280569077,
0.004406440071761608,
0.04506547749042511,
0.001370099140331149,
0.029448920860886574,
0.03752446919679642,
0.04013121873140335,
-0.009132146835327148,
-0.12795917689800262,
0.02619091421365738,
-0.05156804621219635,
0.0616430938243866,
-0.05691858381032944,
0.050009842962026596,
-0.06551534682512283,
0.008551704697310925,
-0.03525305539369583,
-0.0204540453851223,
-0.0513736717402935,
0.046991679817438126,
0.00324030639603734,
0.045505035668611526,
-0.0005776170874014497,
-0.007955657318234444,
0.031245816498994827,
0.027462631464004517,
0.07383367419242859,
-0.0048749507404863834,
-0.025615764781832695,
-0.03641674667596817,
0.01420630794018507,
0.030361568555235863,
-0.09353630244731903,
-0.02911841869354248,
0.02796829864382744,
-0.07337943464517593,
-0.030169857665896416,
-0.021200386807322502,
-0.05208074674010277,
-0.06387083232402802,
-0.040594879537820816,
-0.06331337243318558,
0.06504765152931213,
-0.06299376487731934,
0.000684865633957088,
-0.0036391408648341894,
-0.07262835651636124,
-0.03420674428343773,
0.026926428079605103,
0.011294356547296047,
-0.04497559368610382,
0.009466365911066532,
-0.020355138927698135,
0.07607854157686234,
-0.04775537922978401,
0.03878059238195419,
-0.03322484344244003,
0.001450072624720633,
0.038880035281181335,
0.013573347590863705,
-0.024823669344186783,
0.014214077033102512,
0.034969571977853775,
-0.06314109265804291,
-0.004403316415846348,
-0.11270622164011002,
-0.04744882136583328,
-0.06531617790460587,
0.008901884779334068,
0.08111008256673813,
0.024111973121762276,
0.0695621594786644,
0.04921707138419151,
0.019059941172599792,
0.04435368627309799,
0.030731331557035446,
0.005113172344863415,
-0.023417461663484573,
0.038813769817352295,
0.07407662272453308,
0.004901112522929907,
-6.667697505235992e-8,
-0.09160012751817703,
0.02322857826948166,
-0.04168491065502167,
0.06844652444124222,
-0.03773044794797897,
-0.047478146851062775,
-0.060606732964515686,
0.013459974899888039,
-0.009561608545482159,
0.014405442401766777,
0.029360022395849228,
0.05230507254600525,
-0.03140542283654213,
-0.01409412082284689,
0.015814408659934998,
0.0945654958486557,
-0.0245362501591444,
0.021901486441493034,
-0.035811565816402435,
-0.02771449275314808,
0.0610172264277935,
-0.005129834171384573,
0.0236892718821764,
-0.0158474612981081,
0.04630585387349129,
-0.06987912952899933,
0.004676796495914459,
0.1094369888305664,
-0.03116499073803425,
-0.01876765303313732,
-0.04897104948759079,
0.062371667474508286,
-0.05771437659859657,
0.027952980250120163,
0.1018626019358635,
0.11040100455284119,
-0.019263487309217453,
-0.02817624621093273,
0.03264090046286583,
0.03568077087402344,
0.0003892612876370549,
0.06507185846567154,
-0.06816139817237854,
-0.019395457580685616,
0.07614446431398392,
0.00004911017094855197,
-0.07554464042186737,
-0.059764232486486435,
0.02497064508497715,
0.015768712386488914,
0.03550311177968979,
-0.032954953610897064,
0.007485062815248966,
0.0404963493347168,
-0.02028428018093109,
0.04519294947385788,
-0.09147068113088608,
-0.0027313458267599344,
0.01635180227458477,
-0.013664265163242817,
0.024192672222852707,
0.07293985784053802,
0.005031263921409845,
0.04888756945729256
] |
sentence-transformers/all-MiniLM-L12-v1 | c8f1d5b49a00a0b0025e540ceca2c38101fc926f | 2021-08-30T20:01:21.000Z | [
"pytorch",
"bert",
"en",
"arxiv:1904.06472",
"arxiv:2102.07033",
"arxiv:2104.08727",
"arxiv:1704.05179",
"arxiv:1810.09305",
"sentence-transformers",
"feature-extraction",
"sentence-similarity",
"license:apache-2.0"
] | sentence-similarity | false | sentence-transformers | null | sentence-transformers/all-MiniLM-L12-v1 | 2,148 | 2 | sentence-transformers | ---
pipeline_tag: sentence-similarity
tags:
- sentence-transformers
- feature-extraction
- sentence-similarity
language: en
license: apache-2.0
---
# all-MiniLM-L12-v1
This is a [sentence-transformers](https://www.SBERT.net) model: It maps sentences & paragraphs to a 384 dimensional dense vector space and can be used for tasks like clustering or semantic search.
## Usage (Sentence-Transformers)
Using this model becomes easy when you have [sentence-transformers](https://www.SBERT.net) installed:
```
pip install -U sentence-transformers
```
Then you can use the model like this:
```python
from sentence_transformers import SentenceTransformer
sentences = ["This is an example sentence", "Each sentence is converted"]
model = SentenceTransformer('sentence-transformers/all-MiniLM-L12-v1')
embeddings = model.encode(sentences)
print(embeddings)
```
## Usage (HuggingFace Transformers)
Without [sentence-transformers](https://www.SBERT.net), you can use the model like this: First, you pass your input through the transformer model, then you have to apply the right pooling-operation on-top of the contextualized word embeddings.
```python
from transformers import AutoTokenizer, AutoModel
import torch
import torch.nn.functional as F
#Mean Pooling - Take attention mask into account for correct averaging
def mean_pooling(model_output, attention_mask):
token_embeddings = model_output[0] #First element of model_output contains all token embeddings
input_mask_expanded = attention_mask.unsqueeze(-1).expand(token_embeddings.size()).float()
return torch.sum(token_embeddings * input_mask_expanded, 1) / torch.clamp(input_mask_expanded.sum(1), min=1e-9)
# Sentences we want sentence embeddings for
sentences = ['This is an example sentence', 'Each sentence is converted']
# Load model from HuggingFace Hub
tokenizer = AutoTokenizer.from_pretrained('sentence-transformers/all-MiniLM-L12-v1')
model = AutoModel.from_pretrained('sentence-transformers/all-MiniLM-L12-v1')
# Tokenize sentences
encoded_input = tokenizer(sentences, padding=True, truncation=True, return_tensors='pt')
# Compute token embeddings
with torch.no_grad():
model_output = model(**encoded_input)
# Perform pooling
sentence_embeddings = mean_pooling(model_output, encoded_input['attention_mask'])
# Normalize embeddings
sentence_embeddings = F.normalize(sentence_embeddings, p=2, dim=1)
print("Sentence embeddings:")
print(sentence_embeddings)
```
## Evaluation Results
For an automated evaluation of this model, see the *Sentence Embeddings Benchmark*: [https://seb.sbert.net](https://seb.sbert.net?model_name=sentence-transformers/all-MiniLM-L12-v1)
------
## Background
The project aims to train sentence embedding models on very large sentence level datasets using a self-supervised
contrastive learning objective. We used the pretrained [`microsoft/MiniLM-L12-H384-uncased`](https://huggingface.co/microsoft/MiniLM-L12-H384-uncased) model and fine-tuned in on a
1B sentence pairs dataset. We use a contrastive learning objective: given a sentence from the pair, the model should predict which out of a set of randomly sampled other sentences, was actually paired with it in our dataset.
We developped this model during the
[Community week using JAX/Flax for NLP & CV](https://discuss.huggingface.co/t/open-to-the-community-community-week-using-jax-flax-for-nlp-cv/7104),
organized by Hugging Face. We developped this model as part of the project:
[Train the Best Sentence Embedding Model Ever with 1B Training Pairs](https://discuss.huggingface.co/t/train-the-best-sentence-embedding-model-ever-with-1b-training-pairs/7354). We benefited from efficient hardware infrastructure to run the project: 7 TPUs v3-8, as well as intervention from Googles Flax, JAX, and Cloud team member about efficient deep learning frameworks.
## Intended uses
Our model is intented to be used as a sentence and short paragraph encoder. Given an input text, it ouptuts a vector which captures
the semantic information. The sentence vector may be used for information retrieval, clustering or sentence similarity tasks.
By default, input text longer than 128 word pieces is truncated.
## Training procedure
### Pre-training
We use the pretrained [`microsoft/MiniLM-L12-H384-uncased`](https://huggingface.co/microsoft/MiniLM-L12-H384-uncased). Please refer to the model card for more detailed information about the pre-training procedure.
### Fine-tuning
We fine-tune the model using a contrastive objective. Formally, we compute the cosine similarity from each possible sentence pairs from the batch.
We then apply the cross entropy loss by comparing with true pairs.
#### Hyper parameters
We trained ou model on a TPU v3-8. We train the model during 540k steps using a batch size of 1024 (128 per TPU core).
We use a learning rate warm up of 500. The sequence length was limited to 128 tokens. We used the AdamW optimizer with
a 2e-5 learning rate. The full training script is accessible in this current repository: `train_script.py`.
#### Training data
We use the concatenation from multiple datasets to fine-tune our model. The total number of sentence pairs is above 1 billion sentences.
We sampled each dataset given a weighted probability which configuration is detailed in the `data_config.json` file.
| Dataset | Paper | Number of training tuples |
|--------------------------------------------------------|:----------------------------------------:|:--------------------------:|
| [Reddit comments (2015-2018)](https://github.com/PolyAI-LDN/conversational-datasets/tree/master/reddit) | [paper](https://arxiv.org/abs/1904.06472) | 726,484,430 |
| [S2ORC](https://github.com/allenai/s2orc) Citation pairs (Abstracts) | [paper](https://aclanthology.org/2020.acl-main.447/) | 116,288,806 |
| [WikiAnswers](https://github.com/afader/oqa#wikianswers-corpus) Duplicate question pairs | [paper](https://doi.org/10.1145/2623330.2623677) | 77,427,422 |
| [PAQ](https://github.com/facebookresearch/PAQ) (Question, Answer) pairs | [paper](https://arxiv.org/abs/2102.07033) | 64,371,441 |
| [S2ORC](https://github.com/allenai/s2orc) Citation pairs (Titles) | [paper](https://aclanthology.org/2020.acl-main.447/) | 52,603,982 |
| [S2ORC](https://github.com/allenai/s2orc) (Title, Abstract) | [paper](https://aclanthology.org/2020.acl-main.447/) | 41,769,185 |
| [Stack Exchange](https://huggingface.co/datasets/flax-sentence-embeddings/stackexchange_xml) (Title, Body) pairs | - | 25,316,456 |
| [MS MARCO](https://microsoft.github.io/msmarco/) triplets | [paper](https://doi.org/10.1145/3404835.3462804) | 9,144,553 |
| [GOOAQ: Open Question Answering with Diverse Answer Types](https://github.com/allenai/gooaq) | [paper](https://arxiv.org/pdf/2104.08727.pdf) | 3,012,496 |
| [Yahoo Answers](https://www.kaggle.com/soumikrakshit/yahoo-answers-dataset) (Title, Answer) | [paper](https://proceedings.neurips.cc/paper/2015/hash/250cf8b51c773f3f8dc8b4be867a9a02-Abstract.html) | 1,198,260 |
| [Code Search](https://huggingface.co/datasets/code_search_net) | - | 1,151,414 |
| [COCO](https://cocodataset.org/#home) Image captions | [paper](https://link.springer.com/chapter/10.1007%2F978-3-319-10602-1_48) | 828,395|
| [SPECTER](https://github.com/allenai/specter) citation triplets | [paper](https://doi.org/10.18653/v1/2020.acl-main.207) | 684,100 |
| [Yahoo Answers](https://www.kaggle.com/soumikrakshit/yahoo-answers-dataset) (Question, Answer) | [paper](https://proceedings.neurips.cc/paper/2015/hash/250cf8b51c773f3f8dc8b4be867a9a02-Abstract.html) | 681,164 |
| [Yahoo Answers](https://www.kaggle.com/soumikrakshit/yahoo-answers-dataset) (Title, Question) | [paper](https://proceedings.neurips.cc/paper/2015/hash/250cf8b51c773f3f8dc8b4be867a9a02-Abstract.html) | 659,896 |
| [SearchQA](https://huggingface.co/datasets/search_qa) | [paper](https://arxiv.org/abs/1704.05179) | 582,261 |
| [Eli5](https://huggingface.co/datasets/eli5) | [paper](https://doi.org/10.18653/v1/p19-1346) | 325,475 |
| [Flickr 30k](https://shannon.cs.illinois.edu/DenotationGraph/) | [paper](https://transacl.org/ojs/index.php/tacl/article/view/229/33) | 317,695 |
| [Stack Exchange](https://huggingface.co/datasets/flax-sentence-embeddings/stackexchange_xml) Duplicate questions (titles) | | 304,525 |
| AllNLI ([SNLI](https://nlp.stanford.edu/projects/snli/) and [MultiNLI](https://cims.nyu.edu/~sbowman/multinli/) | [paper SNLI](https://doi.org/10.18653/v1/d15-1075), [paper MultiNLI](https://doi.org/10.18653/v1/n18-1101) | 277,230 |
| [Stack Exchange](https://huggingface.co/datasets/flax-sentence-embeddings/stackexchange_xml) Duplicate questions (bodies) | | 250,519 |
| [Stack Exchange](https://huggingface.co/datasets/flax-sentence-embeddings/stackexchange_xml) Duplicate questions (titles+bodies) | | 250,460 |
| [Sentence Compression](https://github.com/google-research-datasets/sentence-compression) | [paper](https://www.aclweb.org/anthology/D13-1155/) | 180,000 |
| [Wikihow](https://github.com/pvl/wikihow_pairs_dataset) | [paper](https://arxiv.org/abs/1810.09305) | 128,542 |
| [Altlex](https://github.com/chridey/altlex/) | [paper](https://aclanthology.org/P16-1135.pdf) | 112,696 |
| [Quora Question Triplets](https://quoradata.quora.com/First-Quora-Dataset-Release-Question-Pairs) | - | 103,663 |
| [Simple Wikipedia](https://cs.pomona.edu/~dkauchak/simplification/) | [paper](https://www.aclweb.org/anthology/P11-2117/) | 102,225 |
| [Natural Questions (NQ)](https://ai.google.com/research/NaturalQuestions) | [paper](https://transacl.org/ojs/index.php/tacl/article/view/1455) | 100,231 |
| [SQuAD2.0](https://rajpurkar.github.io/SQuAD-explorer/) | [paper](https://aclanthology.org/P18-2124.pdf) | 87,599 |
| [TriviaQA](https://huggingface.co/datasets/trivia_qa) | - | 73,346 |
| **Total** | | **1,124,818,467** | | [
-0.030681096017360687,
-0.034574031829833984,
0.005059215240180492,
0.030977435410022736,
0.03437228128314018,
0.06355078518390656,
-0.06853145360946655,
0.011841836385428905,
0.019633395597338676,
-0.08080363273620605,
0.05672736093401909,
-0.018334997817873955,
0.04258238524198532,
0.04904390126466751,
0.06345119327306747,
0.03198466822504997,
0.02896079234778881,
0.1105802059173584,
-0.06819241493940353,
-0.12342889606952667,
0.11967078596353531,
0.12316497415304184,
0.005652649328112602,
0.03863738849759102,
-0.022140946239233017,
0.08604201674461365,
-0.041076596826314926,
-0.012194438837468624,
0.026146212592720985,
-0.01473234873265028,
0.03311622142791748,
-0.02040284126996994,
-0.05656511336565018,
0.06475784629583359,
0.06195022165775299,
0.08561667799949646,
-0.009048442356288433,
0.014484823681414127,
-0.003876354545354843,
-0.08422430604696274,
0.004798105917870998,
-0.03911951556801796,
-0.05752352625131607,
-0.0308152474462986,
0.021906569600105286,
-0.074110247194767,
-0.1066836416721344,
-0.029628660529851913,
-0.024125853553414345,
-0.029296278953552246,
-0.08454254269599915,
0.02807544730603695,
0.015118565410375595,
0.07744501531124115,
0.004428069572895765,
0.03167876601219177,
0.036860350519418716,
-0.006008625496178865,
0.01990346796810627,
-0.12606693804264069,
-0.08600804954767227,
-0.025265857577323914,
0.018771817907691002,
0.005042460281401873,
-0.053839631378650665,
-0.031977612525224686,
0.04778430983424187,
0.013690273277461529,
0.021516580134630203,
0.019192706793546677,
-0.09273598343133926,
0.04198628291487694,
-0.0606045126914978,
-0.04706030339002609,
-0.05132941156625748,
0.008290654979646206,
0.09857857972383499,
0.0029699034057557583,
0.03779437020421028,
0.03788270428776741,
-0.006952796597033739,
-0.08867282420396805,
0.03489968925714493,
0.09212449938058853,
0.002909609582275152,
-0.07620204240083694,
-0.009780802763998508,
-0.029692716896533966,
-0.005255661904811859,
-0.023000365123152733,
-0.08656913042068481,
-0.10565128922462463,
0.018455103039741516,
-0.0286366268992424,
0.024099625647068024,
0.0311879925429821,
-0.022581156343221664,
-0.0028533036820590496,
0.04278160631656647,
0.048056043684482574,
0.03610639646649361,
0.034968312829732895,
0.06357628107070923,
-0.10946519672870636,
-0.028561202809214592,
0.03705495968461037,
-0.03706301003694534,
-0.01799837313592434,
0.04735482111573219,
-0.12148608267307281,
0.02774425968527794,
0.01249329000711441,
-0.012329202145338058,
-0.02835162542760372,
0.08899901062250137,
-0.05532897263765335,
0.03501014783978462,
-0.03240127116441727,
-0.004564796108752489,
0.11057674139738083,
-0.029388412833213806,
0.06121610105037689,
-0.011238937266170979,
0.02170109562575817,
-0.0042672352865338326,
-0.01272512972354889,
0.006192715838551521,
1.059855668031996e-34,
-0.002573879901319742,
-0.01129430253058672,
-0.003503393614664674,
-0.02631901390850544,
0.03349895775318146,
0.03151022270321846,
0.045275166630744934,
0.06236164644360542,
-0.09268652647733688,
-0.036336109042167664,
-0.047972992062568665,
0.02422199584543705,
-0.030874459072947502,
0.06087740138173103,
0.004214375279843807,
-0.015198428183794022,
-0.028659885749220848,
-0.05226510763168335,
0.06322092562913895,
0.013006185181438923,
0.013905973173677921,
0.022472448647022247,
0.007072678301483393,
-0.03224100545048714,
-0.10419504344463348,
-0.03152947500348091,
0.06207387521862984,
-0.08928138017654419,
-0.07450155168771744,
0.005920162424445152,
-0.07001426815986633,
0.017924228683114052,
-0.01495598815381527,
0.015739131718873978,
-0.005634428467601538,
-0.005624788347631693,
0.045766301453113556,
-0.011230330914258957,
-0.03815308213233948,
-0.09057783335447311,
-0.031120087951421738,
0.028474604710936546,
-0.01996702514588833,
-0.07490155845880508,
-0.0024395142681896687,
-0.001883524120785296,
0.04540098085999489,
0.02032635174691677,
0.09374450892210007,
0.0010935644386336207,
0.09620632976293564,
-0.007366634439677,
-0.011270681396126747,
-0.05175922065973282,
0.02573411539196968,
0.008938327431678772,
0.04635174199938774,
0.027873052284121513,
0.09560549259185791,
-0.012109457515180111,
0.022452056407928467,
-0.023778829723596573,
0.04202685505151749,
0.030100226402282715,
0.10837683081626892,
-0.020391106605529785,
0.07545935362577438,
0.03593993932008743,
-0.0035866706166416407,
0.04862953722476959,
-0.04152040556073189,
0.025506844744086266,
-0.04164333641529083,
0.009119927883148193,
0.05661088228225708,
-0.029874423518776894,
-0.009370156563818455,
-0.08720140159130096,
-0.020543020218610764,
0.08376803249120712,
-0.03918002173304558,
-0.028065189719200134,
0.07230114191770554,
-0.0500350259244442,
-0.009824471548199654,
-0.042697686702013016,
0.025887655094265938,
-0.03330428525805473,
0.0668414905667305,
-0.05652777850627899,
0.011413516476750374,
-0.013473241589963436,
0.006394999101758003,
0.028917424380779266,
0.06737586110830307,
-2.2805367334249874e-33,
0.02431890368461609,
0.027019895613193512,
-0.062420301139354706,
0.03923801705241203,
-0.00879931915551424,
-0.05669547989964485,
0.04393541440367699,
0.0682361051440239,
0.01221569161862135,
-0.011827666312456131,
-0.05063878744840622,
-0.021886320784687996,
0.08575866371393204,
-0.09138131141662598,
0.07666699588298798,
0.06971149891614914,
-0.02534038946032524,
0.0434773787856102,
0.06033758074045181,
0.059792786836624146,
0.018557004630565643,
0.06012256443500519,
-0.11119448393583298,
0.057839829474687576,
-0.013099335134029388,
-0.025177402421832085,
-0.01218267809599638,
-0.009473376907408237,
-0.00794658437371254,
-0.052774880081415176,
-0.0030668252147734165,
-0.00024468504125252366,
-0.04259953647851944,
-0.026677878573536873,
-0.13926297426223755,
0.011618262156844139,
-0.03644319623708725,
-0.014122525230050087,
0.044061046093702316,
0.051615532487630844,
0.021122103556990623,
0.08323066681623459,
-0.03081665001809597,
-0.0022780413273721933,
-0.01726391725242138,
-0.005598658695816994,
-0.06716887652873993,
-0.0815420001745224,
0.013900192454457283,
0.0013691746862605214,
-0.028562044724822044,
0.0453132800757885,
-0.1433895081281662,
0.04226766526699066,
-0.0502188466489315,
-0.07150313258171082,
-0.052316416054964066,
-0.014233987778425217,
-0.0971955806016922,
-0.06455500423908234,
-0.060847435146570206,
-0.029926011338829994,
0.011503778398036957,
-0.06223960965871811,
0.06588014960289001,
-0.04218120872974396,
-0.013063193298876286,
0.026395348832011223,
-0.03887183219194412,
-0.04235139861702919,
0.013458059169352055,
-0.02429322898387909,
0.011923998594284058,
0.046985771507024765,
0.04734012112021446,
-0.057550590485334396,
-0.013286399655044079,
0.008496006950736046,
-0.020891882479190826,
-0.0660652369260788,
0.04633740335702896,
-0.027223501354455948,
0.008355013094842434,
-0.04259961470961571,
0.03454345092177391,
-0.0389859713613987,
0.026088126003742218,
0.08325536549091339,
-0.010200814343988895,
0.03539995104074478,
-0.009363300167024136,
-0.0029375653248280287,
0.005048607010394335,
0.050550609827041626,
0.044319164007902145,
-4.836777378613988e-8,
-0.0856255441904068,
-0.03741379454731941,
-0.06278376281261444,
0.06712214648723602,
-0.09188741445541382,
-0.020231904461979866,
0.0613568015396595,
0.07523474842309952,
-0.06687352061271667,
-0.005089998245239258,
0.018809694796800613,
0.02426060475409031,
-0.07824481278657913,
0.015087008476257324,
-0.04657561704516411,
0.1294330507516861,
-0.01108280848711729,
0.039222195744514465,
0.03805393725633621,
0.004618870560079813,
0.007556607946753502,
0.005059939809143543,
-0.036302559077739716,
0.03313523903489113,
-0.009852400980889797,
0.01873091049492359,
-0.026201125234365463,
0.012833764776587486,
0.012446936219930649,
-0.010675443336367607,
-0.021995211020112038,
0.013886954635381699,
-0.034434229135513306,
-0.061524465680122375,
0.006797173526138067,
0.041857246309518814,
0.0538073405623436,
-0.05881528928875923,
0.03630978614091873,
0.08165331184864044,
0.04913736879825592,
0.037445757538080215,
-0.13736702501773834,
-0.01846916601061821,
0.10539713501930237,
0.019891779869794846,
0.0008424305124208331,
-0.07638360559940338,
0.04651637375354767,
0.02919742837548256,
0.09010912477970123,
-0.07989683747291565,
-0.019285470247268677,
-0.029794706031680107,
0.024610061198472977,
0.03704182058572769,
0.02933189831674099,
-0.021682340651750565,
0.08435172587633133,
-0.06592670828104019,
0.060331448912620544,
0.105305977165699,
0.10624610632658005,
-0.08441467583179474
] |
shahrukhx01/roberta-base-boolq | 87b8505e8f651d5aadedb50ea6737871a45a83b8 | 2022-06-02T08:36:14.000Z | [
"pytorch",
"roberta",
"text-classification",
"en",
"transformers",
"boolean-qa"
] | text-classification | false | shahrukhx01 | null | shahrukhx01/roberta-base-boolq | 2,147 | null | transformers | ---
language: "en"
tags:
- boolean-qa
widget:
- text: "Is Berlin the smallest city of Germany? <s> Berlin is the capital and largest city of Germany by both area and population. Its 3.8 million inhabitants make it the European Union's most populous city, according to the population within city limits "
---
# Labels Map
LABEL_0 => **"NO"** <br/>
LABEL_1 => **"YES"**
```python
from transformers import (
AutoModelForSequenceClassification,
AutoTokenizer,
)
model = AutoModelForSequenceClassification.from_pretrained("shahrukhx01/roberta-base-boolq")
model.to(device)
#model.push_to_hub("roberta-base-boolq")
tokenizer = AutoTokenizer.from_pretrained("shahrukhx01/roberta-base-boolq")
def predict(question, passage):
sequence = tokenizer.encode_plus(question, passage, return_tensors="pt")['input_ids'].to(device)
logits = model(sequence)[0]
probabilities = torch.softmax(logits, dim=1).detach().cpu().tolist()[0]
proba_yes = round(probabilities[1], 2)
proba_no = round(probabilities[0], 2)
print(f"Question: {question}, Yes: {proba_yes}, No: {proba_no}")
passage = """Berlin is the capital and largest city of Germany by both area and population. Its 3.8 million inhabitants make it the European Union's most populous city,
according to the population within city limits."""
question = "Is Berlin the smallest city of Germany?"
predict(s_question, passage)
```
| [
-0.04027774930000305,
0.0010535010369494557,
-0.02835865132510662,
0.03511268272995949,
-0.02613994851708412,
0.0933423638343811,
0.02112245373427868,
0.029584145173430443,
-0.0344892218708992,
-0.061691172420978546,
-0.005555383861064911,
-0.14769375324249268,
0.015224738977849483,
0.05613432824611664,
-0.018821772187948227,
0.029872102662920952,
-0.03723062574863434,
-0.07522287964820862,
-0.08834198862314224,
-0.10468229651451111,
0.09289880841970444,
0.08480438590049744,
0.05149258300662041,
0.042738478630781174,
0.0908060148358345,
-0.05171860381960869,
0.0276752021163702,
0.03988660126924515,
0.0011542036663740873,
0.05691375955939293,
-0.012145576998591423,
0.04114999622106552,
0.022509654983878136,
0.08171739429235458,
0.07744364440441132,
-0.008435823954641819,
-0.06786265969276428,
-0.017232514917850494,
0.01385580562055111,
-0.040330156683921814,
-0.0013805038761347532,
-0.10233370214700699,
-0.029561465606093407,
-0.013366948813199997,
0.04394974559545517,
0.03467810899019241,
-0.003291614819318056,
0.02536587044596672,
-0.03448930382728577,
-0.02915419079363346,
0.0005409153527580202,
0.029807770624756813,
0.006263419054448605,
0.031161490827798843,
-0.04884945601224899,
0.017884934321045876,
0.009979979135096073,
-0.030037328600883484,
0.02162940613925457,
-0.054782234132289886,
-0.07275441288948059,
-0.06592977792024612,
-0.020782627165317535,
-0.003846237435936928,
-0.02285674586892128,
-0.041829295456409454,
0.00008564243034925312,
0.04694554582238197,
0.015495351515710354,
0.02859623357653618,
-0.011241779662668705,
-0.00469589326530695,
-0.007218217011541128,
0.03344927355647087,
0.14801962673664093,
-0.04513357952237129,
0.016834143549203873,
0.024323517456650734,
0.02468983829021454,
-0.04149900749325752,
-0.032518427819013596,
-0.08029653131961823,
0.04812172055244446,
0.07191143184900284,
0.01575954258441925,
-0.013912048190832138,
0.000055441934819100425,
0.030465492978692055,
-0.012818604707717896,
0.021829206496477127,
0.009480143897235394,
-0.06468266248703003,
0.00913246814161539,
0.033910028636455536,
-0.027151834219694138,
0.06179843842983246,
0.0389750711619854,
-0.0461714006960392,
0.021641943603754044,
0.048282112926244736,
-0.024879224598407745,
0.005890398286283016,
0.023418039083480835,
-0.02088252268731594,
0.002106694970279932,
-0.07033564150333405,
0.04899153113365173,
0.014177870005369186,
-0.009813185781240463,
-0.06316886842250824,
-0.012964650057256222,
-0.04058903083205223,
0.03005354106426239,
0.0004959421348758042,
-0.007414212450385094,
0.04839874058961868,
0.061518363654613495,
0.05174931511282921,
-0.0582277849316597,
0.029910804703831673,
0.04703843593597412,
0.0037387132178992033,
-0.07380115985870361,
0.05787689983844757,
-0.02474190667271614,
0.008042490109801292,
-0.06263277679681778,
4.3526340738781976e-33,
0.005035127513110638,
0.006080036051571369,
0.10029349476099014,
0.06824842095375061,
-0.08932900428771973,
0.007456699851900339,
-0.06509550660848618,
0.09572555869817734,
0.0006722479010932148,
-0.001606740988790989,
-0.03399215638637543,
0.03291124477982521,
-0.0345340333878994,
-0.000876009464263916,
-0.0296881552785635,
-0.002388342982158065,
-0.054073087871074677,
0.012416178360581398,
-0.0532926507294178,
0.05450486019253731,
0.0832551047205925,
0.020032867789268494,
-0.0412064753472805,
-0.06254716962575912,
-0.03549954667687416,
0.014448922127485275,
0.06276919692754745,
0.008002759888768196,
-0.007547872141003609,
-0.003864178666844964,
-0.15922407805919647,
-0.025516510009765625,
-0.015490434132516384,
-0.061228152364492416,
-0.016842307522892952,
0.033776044845581055,
0.01725030317902565,
-0.03593660891056061,
-0.08561142534017563,
-0.12091485410928726,
-0.03707681968808174,
0.005654462147504091,
-0.036780111491680145,
-0.07544408738613129,
-0.0029391988646239042,
-0.03436892852187157,
0.0022505815140902996,
-0.0483078807592392,
0.05024569854140282,
0.01974925957620144,
0.09935969859361649,
-0.036267150193452835,
-0.08654599636793137,
-0.03799799084663391,
0.04610332474112511,
0.04658203199505806,
0.07676036655902863,
0.04612173140048981,
0.0849020779132843,
-0.021060261875391006,
-0.031967394053936005,
-0.011277991347014904,
0.044348880648612976,
0.015197302214801311,
0.08209676295518875,
0.026705896481871605,
0.04171103984117508,
0.0260888934135437,
0.047177936881780624,
0.03421910107135773,
-0.029979288578033447,
-0.06772315502166748,
-0.02806798927485943,
0.007120566442608833,
0.0504298135638237,
-0.009440407156944275,
-0.0017971652559936047,
-0.03454233333468437,
-0.05884482339024544,
-0.031851496547460556,
-0.025448253378272057,
-0.03224480152130127,
-0.05602148547768593,
-0.015217282809317112,
0.040025744587183,
-0.030486201867461205,
0.07637680321931839,
-0.05918999761343002,
-0.06133851036429405,
-0.05601129308342934,
-0.023085633292794228,
0.0176802147179842,
-0.010825521312654018,
0.034895241260528564,
-0.05659961700439453,
-6.690107896566178e-33,
0.037842243909835815,
-0.0028974160086363554,
-0.04893992468714714,
-0.029114287346601486,
-0.019368870183825493,
-0.09784656018018723,
0.03489382192492485,
0.10684360563755035,
0.03777216747403145,
-0.038914408534765244,
0.019841134548187256,
-0.10622942447662354,
0.10571126639842987,
0.0013040662743151188,
0.057677481323480606,
0.014669684693217278,
0.0009808177128434181,
-0.02461385726928711,
-0.04335515573620796,
0.09963851422071457,
-0.07534735649824142,
0.04806830734014511,
-0.13596193492412567,
-0.03919290378689766,
-0.1221931204199791,
0.0025281584821641445,
-0.03479264676570892,
0.07485618442296982,
0.032018132507801056,
-0.02279146946966648,
-0.09222835302352905,
-0.07628842443227768,
-0.016991935670375824,
0.035609595477581024,
-0.052928898483514786,
0.0003374735824763775,
0.05633101984858513,
-0.03989499434828758,
-0.06575661897659302,
0.0828140527009964,
0.02913491614162922,
0.011939479038119316,
-0.13158492743968964,
0.07795305550098419,
-0.029757576063275337,
-0.014107439666986465,
0.013905372470617294,
-0.008890416473150253,
0.024540435522794724,
-0.028129393234848976,
0.12622524797916412,
0.05650299787521362,
-0.1294531673192978,
0.019875511527061462,
-0.02975393645465374,
0.03139343857765198,
0.06017472594976425,
-0.06185508146882057,
0.0056512802839279175,
-0.032453592866659164,
-0.05257430300116539,
0.009770282544195652,
0.07542598992586136,
-0.053207289427518845,
0.03227229788899422,
-0.09547895193099976,
-0.06333193182945251,
0.033003807067871094,
0.059483859688043594,
-0.02063470520079136,
0.0490574948489666,
0.046766217797994614,
0.0031929227989166975,
-0.0045100850984454155,
0.017642993479967117,
0.009524520486593246,
-0.00038843927904963493,
-0.011560386046767235,
0.02953162044286728,
-0.0237235389649868,
-0.10335340350866318,
0.0164178516715765,
0.06628704816102982,
0.14954137802124023,
-0.042388997972011566,
-0.01678250916302204,
0.10128143429756165,
0.08949435502290726,
0.03511422127485275,
0.05895879864692688,
-0.022017737850546837,
0.049552202224731445,
0.0007454543374478817,
0.11247671395540237,
-0.07448233664035797,
-5.769267730215688e-8,
-0.08457180857658386,
0.05029333382844925,
-0.043707769364118576,
0.06801524013280869,
-0.03917510434985161,
-0.03624618798494339,
0.004209122620522976,
0.021571364253759384,
-0.04717621952295303,
0.01227184385061264,
-0.044968873262405396,
0.026089150458574295,
-0.09291628003120422,
0.0012006089091300964,
-0.01504442747682333,
0.03992559015750885,
-0.025229137390851974,
0.05515215918421745,
-0.018756546080112457,
-0.00040420840377919376,
0.024643808603286743,
0.003771201241761446,
-0.0524786151945591,
-0.00808035396039486,
0.028302470222115517,
0.0015606586821377277,
-0.05249718949198723,
0.07163532078266144,
-0.008465803228318691,
-0.035324957221746445,
0.0283979382365942,
-0.037870824337005615,
-0.021334368735551834,
-0.012167638167738914,
-0.07168781757354736,
0.08161208778619766,
0.037181127816438675,
-0.03224166855216026,
0.04159560054540634,
0.023696916177868843,
0.022640490904450417,
0.008439095690846443,
-0.18268465995788574,
0.004902479704469442,
0.00015402499411720783,
-0.06552673131227493,
0.019124113023281097,
-0.08822862058877945,
0.03610982373356819,
0.03239789232611656,
-0.03638989105820656,
-0.04619283229112625,
-0.01868601143360138,
-0.02013770304620266,
0.037012241780757904,
0.013971107080578804,
-0.009931736625730991,
0.0018840571865439415,
-0.014040919952094555,
0.05132352188229561,
0.045620113611221313,
0.022865358740091324,
0.026538152247667313,
0.003267076797783375
] |
artemnech/enrut5-base | 13523cbed3ee1390197d050cc52b0e6f9aa3ea45 | 2022-07-25T05:17:35.000Z | [
"pytorch",
"mt5",
"text2text-generation",
"ru",
"en",
"transformers",
"russian",
"license:mit",
"autotrain_compatible"
] | text2text-generation | false | artemnech | null | artemnech/enrut5-base | 2,146 | null | transformers | ---
language: ["ru", "en"]
tags:
- russian
license: mit
widget:
- text: "translate ru to en: Интересный момент. Модель не видела русских диалогов, но может их понимать"
---
This pruned model of mt5-base [google/mt5-base](https://huggingface.co/google/mt5-base) with only some Rusian and English embeddings left.
The model has been fine-tuned for several tasks:
* translation (opus100 dataset)
* dialog (daily dialog dataset)
How to use:
```
# !pip install transformers sentencepiece
from transformers import AutoModelForSeq2SeqLM, AutoTokenizer, T5Tokenizer
import torch
model_name = 'artemnech/enrut5-base'
model = AutoModelForSeq2SeqLM.from_pretrained(model_name)
tokenizer = AutoTokenizer.from_pretrained(model_name)
def generate(text, **kwargs):
model.eval()
inputs = tokenizer(text, return_tensors='pt')
with torch.no_grad():
hypotheses = model.generate(**inputs, **kwargs)
return tokenizer.decode(hypotheses[0], skip_special_tokens=True)
print(generate('translate ru to en: Интересный момент. Модель не видела русских диалогов, но может их понимать', num_beams=4,))
# The Model didn't see Russian dialogues, but can understand them.
print(generate("translate en to ru: The Model didn't see Russian dialogues, but can understand them.", num_beams=4,))
# Модель не видела русских диалога, но может понимать их.
print(generate('dialog: user1>>: Hello', num_beams=2))
# Hi
print(generate('dialog: user1>>: Hello user2>>: Hi user1>>: Would you like to drink something?', num_beams=2))
# I'd like to drink a cup of coffee.
#An interesting point. The model has not seen Russian dialogues, but can understand them
print(generate('dialog: user1>>: Привет'))
# Hi
print(generate('dialog: user1>>: Привет user2>>: Hi user1>>: Хочешь выпить что-нибудь?', num_beams=2))
# I'd like to have a cup of coffee.
```
| [
-0.11572178453207016,
-0.05912119150161743,
0.04978984594345093,
0.00022305546735879034,
0.026459282264113426,
-0.026849456131458282,
0.006122882477939129,
0.031718190759420395,
-0.017213841900229454,
-0.06695273518562317,
-0.04727241024374962,
-0.018260424956679344,
0.021225137636065483,
0.05479608476161957,
0.016268856823444366,
-0.005450261291116476,
0.020337969064712524,
0.01869138702750206,
-0.07393017411231995,
-0.07910186052322388,
0.07278598099946976,
0.02153554931282997,
0.08556373417377472,
0.050819892436265945,
0.08736161887645721,
0.024240178987383842,
-0.019849926233291626,
0.024887608364224434,
0.06551014631986618,
-0.016863718628883362,
0.014495236799120903,
0.06354588270187378,
-0.03308751434087753,
0.0143894637003541,
0.01583228074014187,
0.1422407031059265,
-0.05850088223814964,
-0.058364883065223694,
-0.05644557252526283,
0.024495815858244896,
0.023151591420173645,
-0.07393690943717957,
-0.04859836772084236,
-0.014848601073026657,
0.07965470105409622,
-0.02864428609609604,
-0.04668641462922096,
-0.028640011325478554,
0.027694696560502052,
0.0020352057181298733,
-0.07851462811231613,
-0.03069395013153553,
-0.00595508236438036,
0.05141162499785423,
-0.04198487475514412,
-0.04328916221857071,
0.0038971586618572474,
-0.058506421744823456,
0.03919275477528572,
-0.04098643735051155,
-0.07226286083459854,
-0.006586721632629633,
-0.052383363246917725,
-0.003750778269022703,
-0.07978443801403046,
0.052053552120923996,
0.04447658360004425,
0.03581074997782707,
0.024609766900539398,
0.05735437199473381,
-0.014265906065702438,
0.031954891979694366,
-0.036341018974781036,
0.060010477900505066,
-0.021793583407998085,
-0.06769804656505585,
0.05578389763832092,
-0.04791213572025299,
-0.015169638209044933,
-0.05807240679860115,
0.027640264481306076,
0.010782097466289997,
0.04874686896800995,
0.04267645999789238,
0.015419737435877323,
-0.03165217116475105,
0.03796028718352318,
0.03131163492798805,
0.023957833647727966,
-0.027075951918959618,
-0.01402890495955944,
-0.05686157941818237,
0.040665093809366226,
0.05927387252449989,
-0.02554016187787056,
0.026799071580171585,
-0.046384990215301514,
0.04600274935364723,
-0.08360306918621063,
0.05135343223810196,
-0.0023503517732024193,
0.024991974234580994,
0.02313893288373947,
0.006468970328569412,
-0.0999767929315567,
-0.04653356224298477,
-0.04175242781639099,
0.054693397134542465,
-0.053466279059648514,
-0.11528269201517105,
0.023088594898581505,
-0.04151708632707596,
-0.020464785397052765,
-0.036336757242679596,
0.06405987590551376,
-0.0388864241540432,
-0.030810002237558365,
-0.0322042740881443,
-0.03937597945332527,
0.05549679696559906,
-0.012269118800759315,
0.07226911932229996,
-0.06180272996425629,
0.015937233343720436,
-0.01635812595486641,
-0.03262017294764519,
-0.016846859827637672,
8.789367421718037e-33,
0.05267973244190216,
0.0673794224858284,
0.019608451053500175,
0.06682420521974564,
-0.05778013914823532,
0.02761421725153923,
-0.013565856032073498,
0.02800583280622959,
-0.12291454523801804,
0.006631303112953901,
-0.03515766188502312,
0.06612617522478104,
-0.0836162194609642,
-0.008786700665950775,
-0.003584763500839472,
0.01339415181428194,
0.02897348254919052,
0.028882397338747978,
0.03478933125734329,
0.06677356362342834,
0.09168080985546112,
0.10903310775756836,
-0.046112094074487686,
-0.028431303799152374,
-0.03527529165148735,
0.0993281751871109,
0.05583519488573074,
-0.11759984493255615,
-0.02571704052388668,
0.03238493576645851,
-0.13224488496780396,
-0.01744401827454567,
-0.0020731838885694742,
0.05162941291928291,
-0.01076486986130476,
-0.07663898169994354,
-0.028688030317425728,
-0.01504118274897337,
-0.04636359214782715,
-0.03998264670372009,
0.02311505377292633,
-0.009817682206630707,
-0.06070323660969734,
-0.00852245558053255,
0.001857949304394424,
0.01544099673628807,
0.0484263114631176,
0.012604783289134502,
0.05005587264895439,
-0.0008042527479119599,
0.04492802172899246,
0.04521631821990013,
-0.005258623044937849,
0.01751074567437172,
-0.004655067343264818,
0.012394550256431103,
0.05065467208623886,
0.025535374879837036,
0.06294766068458557,
-0.04269634559750557,
0.04599371179938316,
-0.0006254990585148335,
0.06841220706701279,
0.07351192831993103,
0.11324600875377655,
-0.019384467974305153,
-0.0028004420455545187,
-0.019215522333979607,
0.04337135702371597,
0.02916402369737625,
-0.109012670814991,
-0.0061637223698198795,
0.02516426332294941,
0.14439038932323456,
0.03239677473902702,
-0.045126982033252716,
0.004203128162771463,
-0.07611825317144394,
-0.040815070271492004,
0.08691725879907608,
-0.0803544893860817,
-0.0352671779692173,
0.032468583434820175,
-0.01474417932331562,
0.005182890687137842,
0.024508846923708916,
0.12206760793924332,
-0.09047591686248779,
-0.02348448522388935,
-0.0016371090896427631,
-0.04857064038515091,
-0.030199484899640083,
-0.02878868393599987,
0.003897671587765217,
-0.05246701091527939,
-8.453081997834797e-33,
0.12103038281202316,
-0.04997720941901207,
-0.06600962579250336,
0.05723312869668007,
-0.10823962837457657,
-0.033373020589351654,
0.09568426758050919,
0.07611081004142761,
0.029660208150744438,
0.05450071021914482,
0.09838079661130905,
-0.06682240217924118,
0.03172718361020088,
-0.014331427402794361,
0.09510105103254318,
-0.041923947632312775,
0.028897661715745926,
-0.016126830130815506,
-0.014559888280928135,
0.028867021203041077,
-0.050202175974845886,
0.08405954390764236,
-0.13416193425655365,
0.058850906789302826,
-0.027162710204720497,
0.0281385388225317,
0.01930035836994648,
-0.00609624246135354,
0.0005515397060662508,
-0.03718234971165657,
-0.04736229032278061,
-0.032528407871723175,
-0.059207506477832794,
0.028012175112962723,
-0.06360913068056107,
0.06644030660390854,
0.00355758354999125,
-0.009246738627552986,
-0.026922602206468582,
0.07928231358528137,
0.027255747467279434,
0.06207781285047531,
-0.03072148747742176,
0.015360474586486816,
-0.028053613379597664,
-0.0343356691300869,
-0.07262276858091354,
-0.004799465648829937,
0.012884418480098248,
-0.07901545614004135,
0.0541045218706131,
0.018856903538107872,
-0.12125243991613388,
-0.057535964995622635,
-0.003408192889764905,
-0.0806204304099083,
0.01277647539973259,
-0.09811297804117203,
-0.021152283996343613,
-0.05857550725340843,
-0.023494642227888107,
-0.02686237171292305,
0.04833673685789108,
-0.12175091356039047,
-0.0306917205452919,
0.005032129120081663,
0.023334501311182976,
-0.005444460082799196,
0.041984979063272476,
-0.035654887557029724,
0.04253530502319336,
-0.024418151006102562,
0.09108072519302368,
0.012802796438336372,
0.03369557857513428,
0.03986348584294319,
-0.037889644503593445,
0.04438244551420212,
0.06752055883407593,
-0.056812040507793427,
-0.04764324054121971,
-0.04000907018780708,
0.02496473304927349,
0.01979161985218525,
-0.009294318035244942,
-0.007958527654409409,
-0.014115258120000362,
0.09759210050106049,
0.10975825786590576,
0.00382817629724741,
0.0064820777624845505,
0.016278164461255074,
-0.009429195895791054,
0.14454570412635803,
0.008009025827050209,
-5.5916515862008964e-8,
-0.04800458252429962,
0.0372513085603714,
-0.03686771169304848,
0.03620821610093117,
-0.05262824520468712,
-0.04146554693579674,
-0.004350495990365744,
-0.00264935614541173,
0.00837579183280468,
0.03810090944170952,
-0.011581262573599815,
-0.04058157280087471,
-0.07835149019956589,
0.006291377358138561,
-0.05306187644600868,
0.07768749445676804,
0.01714012771844864,
0.1147303432226181,
0.011885538697242737,
-0.04311475157737732,
0.03807542845606804,
-0.004491542931646109,
0.02834118902683258,
-0.08452638238668442,
-0.02868509106338024,
-0.023032840341329575,
-0.033313170075416565,
0.019803566858172417,
0.0304577574133873,
-0.0531039796769619,
-0.010377204976975918,
-0.03267316520214081,
-0.046764809638261795,
0.0025532643776386976,
0.017498627305030823,
0.06382040679454803,
0.008464908227324486,
-0.0014072690391913056,
0.033753231167793274,
0.03768274188041687,
0.07066471129655838,
-0.023012176156044006,
-0.08591093868017197,
-0.014330164529383183,
0.007350754924118519,
0.011144833639264107,
-0.037012021988630295,
-0.08478997647762299,
0.04213634505867958,
0.009577019140124321,
0.04556252807378769,
-0.016244417056441307,
-0.06238679587841034,
0.07828251272439957,
0.03877924755215645,
0.03065286949276924,
0.03925967961549759,
-0.11642886698246002,
-0.020179232582449913,
0.0006900078733451664,
-0.05925660952925682,
0.006190468557178974,
-0.007479703053832054,
-0.030484266579151154
] |
whaleloops/phrase-bert | 6f68f4dc2d28aadefa038c79023dc7dfd51f6495 | 2021-11-03T15:04:02.000Z | [
"pytorch",
"bert",
"feature-extraction",
"arxiv:2109.06304",
"sentence-transformers",
"sentence-similarity",
"transformers"
] | sentence-similarity | false | whaleloops | null | whaleloops/phrase-bert | 2,144 | 5 | sentence-transformers | ---
pipeline_tag: sentence-similarity
tags:
- sentence-transformers
- feature-extraction
- sentence-similarity
- transformers
---
# whaleloops/phrase-bert
This is the official repository for the EMNLP 2021 long paper [Phrase-BERT: Improved Phrase Embeddings from BERT with an Application to Corpus Exploration](https://arxiv.org/abs/2109.06304). We provide [code](https://github.com/sf-wa-326/phrase-bert-topic-model) for training and evaluating Phrase-BERT in addition to the datasets used in the paper.
## Usage (Sentence-Transformers)
Using this model becomes easy when you have [sentence-transformers](https://www.SBERT.net) installed:
```
pip install -U sentence-transformers
```
Our model is tested on pytorch=1.9.0, tranformers=4.8.1, sentence-tranformers = 2.1.0 TODO
Then you can use the model like this:
```python
from sentence_transformers import SentenceTransformer
phrase_list = [ 'play an active role', 'participate actively', 'active lifestyle']
model = SentenceTransformer('whaleloops/phrase-bert')
phrase_embs = model.encode( phrase_list )
[p1, p2, p3] = phrase_embs
```
As in sentence-BERT, the default output is a list of numpy arrays:
````
for phrase, embedding in zip(phrase_list, phrase_embs):
print("Phrase:", phrase)
print("Embedding:", embedding)
print("")
````
An example of computing the dot product of phrase embeddings:
````
import numpy as np
print(f'The dot product between phrase 1 and 2 is: {np.dot(p1, p2)}')
print(f'The dot product between phrase 1 and 3 is: {np.dot(p1, p3)}')
print(f'The dot product between phrase 2 and 3 is: {np.dot(p2, p3)}')
````
An example of computing cosine similarity of phrase embeddings:
````
import torch
from torch import nn
cos_sim = nn.CosineSimilarity(dim=0)
print(f'The cosine similarity between phrase 1 and 2 is: {cos_sim( torch.tensor(p1), torch.tensor(p2))}')
print(f'The cosine similarity between phrase 1 and 3 is: {cos_sim( torch.tensor(p1), torch.tensor(p3))}')
print(f'The cosine similarity between phrase 2 and 3 is: {cos_sim( torch.tensor(p2), torch.tensor(p3))}')
````
The output should look like:
````
The dot product between phrase 1 and 2 is: 218.43600463867188
The dot product between phrase 1 and 3 is: 165.48483276367188
The dot product between phrase 2 and 3 is: 160.51708984375
The cosine similarity between phrase 1 and 2 is: 0.8142536282539368
The cosine similarity between phrase 1 and 3 is: 0.6130303144454956
The cosine similarity between phrase 2 and 3 is: 0.584893524646759
````
## Evaluation
Given the lack of a unified phrase embedding evaluation benchmark, we collect the following five phrase semantics evaluation tasks, which are described further in our paper:
* Turney [[Download](https://storage.googleapis.com/phrase-bert/turney/data.txt) ]
* BiRD [[Download](https://storage.googleapis.com/phrase-bert/bird/data.txt)]
* PPDB [[Download](https://storage.googleapis.com/phrase-bert/ppdb/examples.json)]
* PPDB-filtered [[Download](https://storage.googleapis.com/phrase-bert/ppdb_exact/examples.json)]
* PAWS-short [[Download Train-split](https://storage.googleapis.com/phrase-bert/paws_short/train_examples.json) ] [[Download Dev-split](https://storage.googleapis.com/phrase-bert/paws_short/dev_examples.json) ] [[Download Test-split](https://storage.googleapis.com/phrase-bert/paws_short/test_examples.json) ]
Change `config/model_path.py` with the model path according to your directories and
* For evaluation on Turney, run `python eval_turney.py`
* For evaluation on BiRD, run `python eval_bird.py`
* for evaluation on PPDB / PPDB-filtered / PAWS-short, run `eval_ppdb_paws.py` with:
````
nohup python -u eval_ppdb_paws.py \
--full_run_mode \
--task <task-name> \
--data_dir <input-data-dir> \
--result_dir <result-storage-dr> \
>./output.txt 2>&1 &
````
## Train your own Phrase-BERT
If you would like to go beyond using the pre-trained Phrase-BERT model, you may train your own Phrase-BERT using data from the domain you are interested in. Please refer to
`phrase-bert/phrase_bert_finetune.py`
The datasets we used to fine-tune Phrase-BERT are here: [training data csv file](https://storage.googleapis.com/phrase-bert/phrase-bert-ft-data/pooled_context_para_triples_p%3D0.8_train.csv) and [validation data csv file](https://storage.googleapis.com/phrase-bert/phrase-bert-ft-data/pooled_context_para_triples_p%3D0.8_valid.csv).
To re-produce the trained Phrase-BERT, please run:
export INPUT_DATA_PATH=<directory-of-phrasebert-finetuning-data>
export TRAIN_DATA_FILE=<training-data-filename.csv>
export VALID_DATA_FILE=<validation-data-filename.csv>
export INPUT_MODEL_PATH=bert-base-nli-stsb-mean-tokens
export OUTPUT_MODEL_PATH=<directory-of-saved-model>
python -u phrase_bert_finetune.py \
--input_data_path $INPUT_DATA_PATH \
--train_data_file $TRAIN_DATA_FILE \
--valid_data_file $VALID_DATA_FILE \
--input_model_path $INPUT_MODEL_PATH \
--output_model_path $OUTPUT_MODEL_PATH
## Citation:
Please cite us if you find this useful:
````
@inproceedings{phrasebertwang2021,
author={Shufan Wang and Laure Thompson and Mohit Iyyer},
Booktitle = {Empirical Methods in Natural Language Processing},
Year = "2021",
Title={Phrase-BERT: Improved Phrase Embeddings from BERT with an Application to Corpus Exploration}
}
````
| [
-0.11470529437065125,
-0.04245885834097862,
0.049170512706041336,
0.01923442631959915,
0.021510593593120575,
0.04200662299990654,
0.0030415314249694347,
0.06264961510896683,
-0.005018340423703194,
-0.014323093928396702,
0.005926281213760376,
-0.003933824133127928,
0.017738226801156998,
0.08297602087259293,
0.05417389050126076,
0.10007882863283157,
0.043217312544584274,
0.03366667032241821,
-0.0605522021651268,
-0.0757230743765831,
0.10671312361955643,
0.1016741693019867,
0.011399016715586185,
-0.0011657157447189093,
0.02477249503135681,
0.015936480835080147,
-0.048739396035671234,
-0.017916541546583176,
0.06000015139579773,
0.07335887104272842,
0.07302821427583694,
-0.0007769392686896026,
-0.03564458340406418,
0.05907699465751648,
0.054209448397159576,
0.06701178103685379,
-0.023664137348532677,
0.0009312169859185815,
0.013382858596742153,
0.006991820875555277,
0.04000001400709152,
-0.022837458178400993,
-0.08076567202806473,
-0.017210636287927628,
0.06179240718483925,
-0.04688677936792374,
-0.08821945637464523,
-0.015726864337921143,
-0.005061674863100052,
-0.01124524511396885,
-0.07964320480823517,
0.0012408738257363439,
0.03839176520705223,
0.08290223777294159,
-0.023831814527511597,
0.05518350750207901,
0.0446171835064888,
-0.05266984552145004,
0.011107387952506542,
-0.14210355281829834,
-0.10705319792032242,
-0.03715401515364647,
-0.005474083125591278,
-0.02838457003235817,
-0.07116284966468811,
0.015798749402165413,
-0.0573900006711483,
0.01982148177921772,
0.010076697915792465,
0.039259862154722214,
-0.05852202698588371,
0.07185214012861252,
-0.04002268612384796,
-0.023629415780305862,
-0.04950210452079773,
-0.06179587543010712,
0.10539929568767548,
-0.005539515521377325,
0.03865203633904457,
-0.05751026049256325,
-0.0162053145468235,
-0.055368538945913315,
0.08511198312044144,
0.014853473752737045,
0.03793655335903168,
-0.030358225107192993,
0.018243523314595222,
-0.029942262917757034,
-0.02636735513806343,
-0.00980602391064167,
-0.041173093020915985,
-0.15056416392326355,
0.06082894280552864,
-0.03406115993857384,
0.049317412078380585,
0.008971413597464561,
-0.002876884303987026,
-0.03236812353134155,
0.021205056458711624,
0.03091345727443695,
0.013347890228033066,
0.06413491070270538,
0.019019970670342445,
-0.1405269056558609,
0.03247249126434326,
0.06246359646320343,
-0.019645271822810173,
-0.026660220697522163,
0.06312549859285355,
-0.10129070281982422,
0.001568682724609971,
0.01388978585600853,
0.009001148864626884,
-0.02751728892326355,
0.07031023502349854,
-0.07296282052993774,
-0.011060455814003944,
-0.03570052236318588,
-0.0020281567703932524,
0.12233629822731018,
0.009446394629776478,
0.03515345975756645,
-0.027860188856720924,
0.05095313489437103,
-0.045829739421606064,
-0.014049993827939034,
0.032054271548986435,
-1.4158171683913221e-34,
-0.004009705036878586,
-0.003878842806443572,
-0.01071967463940382,
-0.03414403274655342,
0.0067866044119000435,
0.020786458626389503,
0.011844296008348465,
0.02371007204055786,
-0.053078439086675644,
-0.02654324099421501,
-0.07837332785129547,
0.01702909730374813,
-0.07966093719005585,
0.05258161947131157,
-0.018413284793496132,
-0.011479535140097141,
-0.05290616303682327,
0.024105112999677658,
0.06995923072099686,
0.050797462463378906,
0.0362091138958931,
0.04481958970427513,
0.005894507747143507,
-0.07127635180950165,
-0.044706106185913086,
-0.016920706257224083,
0.06042119860649109,
-0.06287328153848648,
-0.022507313638925552,
0.02242698334157467,
-0.10432232171297073,
0.05767533555626869,
0.013264509849250317,
0.018281465396285057,
-0.03639036789536476,
-0.03249365836381912,
-0.0023100306279957294,
-0.03256615996360779,
-0.013818039558827877,
-0.04475168138742447,
-0.04106969013810158,
0.028164172545075417,
-0.012723375111818314,
-0.06474485248327255,
0.019739149138331413,
-0.008527014404535294,
0.058886270970106125,
0.011706299148499966,
0.11935000121593475,
0.023905480280518532,
0.06098980829119682,
-0.018750488758087158,
-0.05662858858704567,
-0.010738059878349304,
0.023344630375504494,
-0.05776415765285492,
0.05279449746012688,
0.010799223557114601,
0.11707406491041183,
-0.0032115033827722073,
0.007822049781680107,
0.012347610667347908,
0.054753270000219345,
-0.028498442843556404,
0.08860141783952713,
0.0024432518985122442,
0.04005584865808487,
0.07841653376817703,
-0.03069416992366314,
0.044503211975097656,
-0.014725897461175919,
0.003332003252580762,
-0.07794686406850815,
0.016031980514526367,
0.058533478528261185,
-0.03924708068370819,
0.002876468701288104,
-0.0701976791024208,
-0.05590726062655449,
0.07489880919456482,
-0.03479485586285591,
-0.03671391308307648,
0.009740992449223995,
-0.03815164789557457,
-0.031787972897291183,
-0.005296293646097183,
0.04738239943981171,
-0.06897597759962082,
0.024980634450912476,
-0.06040063872933388,
0.028451163321733475,
-0.042105041444301605,
-0.027883119881153107,
0.02894452214241028,
0.016061319038271904,
-1.759393435273419e-33,
0.03168488293886185,
0.030354822054505348,
-0.08348025381565094,
0.0513666607439518,
-0.01864718459546566,
-0.08888082951307297,
0.006920659448951483,
0.11245322972536087,
0.016985278576612473,
-0.035110682249069214,
-0.07098269462585449,
-0.06802746653556824,
-0.0397757887840271,
-0.06665505468845367,
0.1136072501540184,
0.04764426499605179,
-0.06632595509290695,
0.0909605398774147,
0.024771353229880333,
0.040231965482234955,
0.0033614151179790497,
-0.035760387778282166,
-0.10792567580938339,
0.06620021164417267,
-0.028162743896245956,
0.01121450774371624,
-0.02903546392917633,
-0.01477794535458088,
0.016769012436270714,
-0.03288900852203369,
-0.062433257699012756,
0.04083886742591858,
-0.06843126565217972,
-0.00016859531751833856,
-0.10324255377054214,
0.03393344208598137,
0.0028850631788372993,
-0.038275763392448425,
0.05203177034854889,
-0.011131907813251019,
0.06029142066836357,
0.07585892826318741,
-0.006277814973145723,
0.008668346330523491,
-0.05654704570770264,
0.009312966838479042,
-0.11628025025129318,
-0.06608448922634125,
0.03580353781580925,
-0.02249835431575775,
0.002540646819397807,
0.011076166294515133,
-0.10592103004455566,
0.02500002831220627,
-0.09749442338943481,
-0.09442191570997238,
0.00018693305901251733,
-0.04126225411891937,
-0.12757866084575653,
-0.047352295368909836,
-0.03600189834833145,
0.0043747322633862495,
0.08289880305528641,
-0.04308832809329033,
0.05621427670121193,
-0.025853285565972328,
-0.002053376752883196,
0.04640154913067818,
-0.017528867349028587,
-0.02812942862510681,
-0.030889613553881645,
-0.025071965530514717,
0.04112876579165459,
0.029586967080831528,
0.01319351140409708,
0.02493894100189209,
0.023221492767333984,
-0.04998958855867386,
-0.014397564344108105,
-0.051114436239004135,
0.013163073919713497,
-0.04736120253801346,
0.011933131143450737,
-0.00004599714884534478,
-0.04971034452319145,
0.016356736421585083,
0.035136982798576355,
0.051403023302555084,
-0.02083049900829792,
0.040814466774463654,
0.005373646505177021,
-0.03014707937836647,
0.006850733887404203,
0.09336492419242859,
0.0510978177189827,
-5.032992689280036e-8,
-0.062116630375385284,
0.005717922002077103,
-0.044300030916929245,
0.038024112582206726,
-0.09650630503892899,
-0.014476151205599308,
-0.004826321266591549,
0.0761149451136589,
-0.060904454439878464,
-0.03728574514389038,
0.028148680925369263,
0.06764869391918182,
-0.08695662021636963,
0.019858134910464287,
-0.026023365557193756,
0.11168178915977478,
-0.04177936911582947,
0.021822957322001457,
0.04893431439995766,
-0.0027009029872715473,
-0.0027514284010976553,
0.02438022382557392,
0.007710671983659267,
0.028223169967532158,
0.004163224715739489,
0.011439234018325806,
-0.03128151595592499,
0.08827470242977142,
-0.009940709918737411,
0.023825986310839653,
0.036238349974155426,
0.0012696172343567014,
-0.10335887223482132,
0.006811026018112898,
0.10431737452745438,
0.047396037727594376,
0.009917045012116432,
-0.06379851698875427,
0.012653201818466187,
0.0673130601644516,
0.056197576224803925,
0.03832637891173363,
-0.10950600355863571,
0.0019391090609133244,
0.12457282841205597,
0.009922313503921032,
-0.00005152601806912571,
-0.0328652560710907,
0.026877105236053467,
-0.0017023371765390038,
0.061733316630125046,
-0.061797354370355606,
-0.05340127274394035,
-0.04328617826104164,
-0.0018981730099767447,
0.032513782382011414,
-0.048765067011117935,
-0.023612651973962784,
0.07413514703512192,
-0.054648928344249725,
0.01629376783967018,
0.08920247852802277,
0.09847357124090195,
0.007072666194289923
] |
michaelrglass/albert-base-rci-wikisql-col | d51bdace09428c72213107d0fe12709c1d7d5d2f | 2021-06-16T15:58:03.000Z | [
"pytorch",
"albert",
"text-classification",
"transformers"
] | text-classification | false | michaelrglass | null | michaelrglass/albert-base-rci-wikisql-col | 2,143 | null | transformers | Entry not found | [
0.0461147278547287,
-0.038838207721710205,
-0.01049656979739666,
-0.03682169318199158,
0.011261860840022564,
0.013094935566186905,
0.0019101888174191117,
-0.013979103416204453,
0.027092741802334785,
-0.015212527476251125,
0.017284274101257324,
-0.08189476281404495,
0.03817418962717056,
-0.04920130595564842,
0.021389011293649673,
-0.015245908871293068,
-0.03203780576586723,
-0.1245758980512619,
0.03150877356529236,
0.032381657510995865,
-0.060957908630371094,
0.05409295856952667,
-0.025087490677833557,
0.01568586938083172,
0.028129950165748596,
-0.04710396006703377,
-0.018688226118683815,
0.013785239309072495,
-0.04001208767294884,
0.01173911802470684,
-0.04317743331193924,
0.05500618368387222,
0.004543041344732046,
0.02973111905157566,
0.14852192997932434,
0.02658126689493656,
0.02907961793243885,
-0.05169107764959335,
0.05803573504090309,
-0.07732241600751877,
-0.017637968063354492,
-0.04219653457403183,
0.041807834059000015,
0.023620979860424995,
0.021563321352005005,
0.016478516161441803,
-0.0021814992651343346,
-0.06400240957736969,
0.06393089145421982,
0.019599027931690216,
-0.08565037697553635,
0.00934905931353569,
-0.008718925528228283,
-0.028583496809005737,
-0.07310017943382263,
0.09416428208351135,
0.001759322709403932,
0.06184990331530571,
0.011840506456792355,
-0.035997264087200165,
0.08358278125524521,
-0.02619801089167595,
0.03736566752195358,
-0.028206506744027138,
-0.07454850524663925,
-0.08883563429117203,
-0.06279942393302917,
-0.008695344440639019,
0.014119276776909828,
-0.0825355276465416,
0.0649217739701271,
-0.00223911227658391,
-0.14716917276382446,
0.07743025571107864,
-0.03548373281955719,
-0.055201586335897446,
0.006981803569942713,
-0.012166670523583889,
0.055111464112997055,
-0.007116836030036211,
-0.023175746202468872,
-0.005835152696818113,
-0.09185640513896942,
0.055196937173604965,
0.034148022532463074,
0.03835180774331093,
0.038685429841279984,
-0.025987252593040466,
0.017804903909564018,
0.022428328171372414,
0.025005368515849113,
-0.10761535167694092,
-0.048001550137996674,
-0.04343584179878235,
0.012374646961688995,
-0.019502125680446625,
0.029218152165412903,
0.0842173621058464,
-0.011719699949026108,
0.09283553808927536,
-0.007015465293079615,
-0.03543110564351082,
-0.06936459988355637,
0.09425332397222519,
-0.010958523489534855,
-0.00805904995650053,
0.004974212497472763,
-0.0031528924591839314,
0.06105927750468254,
-0.03964288905262947,
-0.03619541600346565,
-0.019901901483535767,
0.07134733349084854,
0.039514873176813126,
-0.012729483656585217,
-0.006646515801548958,
-0.04746140539646149,
-0.014432490803301334,
-0.05157482624053955,
0.09506245702505112,
-0.049747664481401443,
-0.04591796174645424,
-0.008965466171503067,
-0.0325421579182148,
-0.08626784384250641,
-0.06624380499124527,
0.02538885548710823,
-4.303924894057984e-33,
0.01133066974580288,
0.0033434738870710135,
-0.002155609894543886,
0.04871906340122223,
-0.023564351722598076,
-0.07933273911476135,
0.0600903145968914,
0.02335330657660961,
-0.03844716399908066,
-0.020433755591511726,
-0.06952055543661118,
-0.03235611692070961,
0.0062485747039318085,
0.064804308116436,
-0.03201229125261307,
0.061689723283052444,
0.0417000837624073,
-0.00761845987290144,
0.03340127319097519,
-0.047770582139492035,
0.00887306872755289,
-0.04066338762640953,
-0.010506896302103996,
0.0106519665569067,
0.021333497017621994,
0.12854498624801636,
-0.009705503471195698,
0.010055632330477238,
-0.017507633194327354,
0.006515394430607557,
0.06334009766578674,
-0.057817306369543076,
0.013668818399310112,
-0.020286159589886665,
0.05430467426776886,
-0.023184705525636673,
0.0828516036272049,
0.0005449643940664828,
-0.10372652113437653,
-0.07634282112121582,
-0.005381610710173845,
-0.039263784885406494,
0.0006114727002568543,
-0.013281986117362976,
0.07119110971689224,
0.043696220964193344,
0.03168422728776932,
0.04338686540722847,
0.05728672817349434,
0.0832006186246872,
-0.07961414009332657,
0.015234283171594143,
0.017002005130052567,
0.047004107385873795,
-0.09794387966394424,
0.004990279674530029,
-0.07062993198633194,
-0.028000490739941597,
-0.04018733277916908,
-0.0702052190899849,
0.011351344175636768,
0.06020182743668556,
-0.03297270089387894,
0.09396500885486603,
0.03417910635471344,
-0.019825750961899757,
-0.034690454602241516,
-0.013036907650530338,
0.05896938592195511,
-0.012359356507658958,
-0.017275206744670868,
-0.07982361316680908,
0.02059139870107174,
0.06737419217824936,
0.04176458343863487,
-0.04978838190436363,
-0.05877475067973137,
-0.06289287656545639,
-0.03354167565703392,
-0.03871942684054375,
0.009898529388010502,
-0.05514208599925041,
-0.11629002541303635,
-0.011855563148856163,
0.10663620382547379,
0.037354156374931335,
-0.0065480442717671394,
-0.051189567893743515,
0.06663123518228531,
0.01874656230211258,
0.032841797918081284,
0.041593004018068314,
-0.06879369914531708,
0.04216769337654114,
-0.01628219522535801,
5.4139394340936695e-34,
0.05697013810276985,
-0.006972255185246468,
0.015711724758148193,
-0.17956365644931793,
0.02320219948887825,
0.007923615165054798,
-0.008062449283897877,
0.0074974060989916325,
0.07391711324453354,
0.0309313777834177,
0.060510627925395966,
0.058605875819921494,
0.09515274316072464,
-0.002282935893163085,
0.001603541080839932,
0.07024981826543808,
0.012629246339201927,
0.07425693422555923,
-0.038426291197538376,
0.01861148327589035,
0.030608950182795525,
-0.02449394389986992,
0.021528491750359535,
-0.003039651783183217,
-0.03676343336701393,
0.03130284696817398,
0.07998586446046829,
0.010451192036271095,
-0.07930229604244232,
-0.013543923385441303,
0.018781835213303566,
0.05168003588914871,
-0.07191970944404602,
0.15783067047595978,
0.026191607117652893,
0.01262354850769043,
0.08218053728342056,
-0.029807550832629204,
-0.07528624683618546,
-0.04250097647309303,
0.017244765534996986,
0.04411793500185013,
0.03708017244935036,
0.009233047254383564,
-0.040271829813718796,
0.022496428340673447,
0.02495843544602394,
0.07633638381958008,
0.005147108342498541,
0.013892097398638725,
0.05610476806759834,
-0.06684739887714386,
0.05862557515501976,
-0.020688841119408607,
0.05377643182873726,
0.06718500703573227,
0.005329249892383814,
-0.01388032827526331,
0.029931528493762016,
0.009508464485406876,
-0.045173756778240204,
0.11534366756677628,
-0.06510116159915924,
0.05117698386311531,
-0.0026125339791178703,
-0.08554837852716446,
-0.03784770518541336,
0.0804959163069725,
0.011298024095594883,
-0.07695550471544266,
-0.04868878796696663,
0.02515520341694355,
0.06252261996269226,
-0.04509226232767105,
-0.01246943511068821,
0.028559505939483643,
-0.030573077499866486,
0.05066261067986488,
-0.08187384903430939,
0.04469604790210724,
0.0034051244147121906,
0.04145054519176483,
-0.021858664229512215,
-0.06112268194556236,
-0.00908052921295166,
-0.05903250351548195,
0.0259539932012558,
0.059690944850444794,
-0.07613514363765717,
-0.03720718249678612,
-0.036316655576229095,
0.07058046013116837,
-0.008224100805819035,
0.041961874812841415,
-0.0285952128469944,
-1.496900736697171e-8,
-0.0014124972512945533,
0.03401879221200943,
-0.040338415652513504,
0.04116074740886688,
0.0935964286327362,
-0.05115952715277672,
0.0008746005478315055,
-0.03389839455485344,
-0.00567849725484848,
-0.010686947964131832,
-0.04789939522743225,
-0.04820054769515991,
-0.02011880651116371,
-0.03209094703197479,
-0.04211259260773659,
-0.10229527950286865,
-0.07819421589374542,
-0.031228765845298767,
-0.02154778689146042,
-0.04960230365395546,
0.08087796717882156,
-0.07801242172718048,
0.06919731199741364,
-0.04999840259552002,
0.03687043860554695,
0.03889009356498718,
-0.049989692866802216,
-0.04254625365138054,
-0.04606937617063522,
0.08682432025671005,
-0.031148413196206093,
0.11826753616333008,
0.034102488309144974,
-0.0208592489361763,
-0.0205202866345644,
0.027134142816066742,
0.09741277992725372,
0.051608603447675705,
0.013477512635290623,
-0.13649295270442963,
-0.022304272279143333,
0.02385953813791275,
0.038732077926397324,
-0.09249968826770782,
-0.04549082741141319,
0.054220106452703476,
0.01160438358783722,
0.051190607249736786,
0.07713303714990616,
-0.022097084671258926,
-0.06127818301320076,
-0.01857956498861313,
0.006740490905940533,
-0.00496308971196413,
0.024095389991998672,
0.0736224576830864,
-0.003481915919110179,
-0.0699305310845375,
-0.006629763171076775,
-0.0598808117210865,
0.05297163128852844,
-0.02902800403535366,
-0.027858933433890343,
-0.01287526823580265
] |
jjzha/jobspanbert-base-cased | 0565591b92a2f8da7094dbf05c3ec6e2b93c0987 | 2022-07-26T08:15:15.000Z | [
"pytorch",
"bert",
"en",
"transformers",
"continuous pretraining",
"job postings",
"JobSpanBERT"
] | null | false | jjzha | null | jjzha/jobspanbert-base-cased | 2,140 | null | transformers | ---
language:
- en
tags:
- continuous pretraining
- job postings
- JobSpanBERT
---
# JobSpanBERT
This is the JobSpanBERT model from:
Mike Zhang, Kristian Nørgaard Jensen, Sif Dam Sonniks, and Barbara Plank. __SkillSpan: Hard and Soft Skill Extraction from Job Postings__. Proceedings of the 2022 Conference of the North American Chapter of the Association for Computational Linguistics: Human Language Technologies.
This model is continuously pre-trained from a spanbert-base-cased checkpoint (which can also be found in our repository) on ~3.2M sentences from job postings. More information can be found in the paper.
If you use this model, please cite the following paper:
```
@inproceedings{zhang-etal-2022-skillspan,
title = "{S}kill{S}pan: Hard and Soft Skill Extraction from {E}nglish Job Postings",
author = "Zhang, Mike and
Jensen, Kristian N{\o}rgaard and
Sonniks, Sif and
Plank, Barbara",
booktitle = "Proceedings of the 2022 Conference of the North American Chapter of the Association for Computational Linguistics: Human Language Technologies",
month = jul,
year = "2022",
address = "Seattle, United States",
publisher = "Association for Computational Linguistics",
url = "https://aclanthology.org/2022.naacl-main.366",
pages = "4962--4984",
abstract = "Skill Extraction (SE) is an important and widely-studied task useful to gain insights into labor market dynamics. However, there is a lacuna of datasets and annotation guidelines; available datasets are few and contain crowd-sourced labels on the span-level or labels from a predefined skill inventory. To address this gap, we introduce SKILLSPAN, a novel SE dataset consisting of 14.5K sentences and over 12.5K annotated spans. We release its respective guidelines created over three different sources annotated for hard and soft skills by domain experts. We introduce a BERT baseline (Devlin et al., 2019). To improve upon this baseline, we experiment with language models that are optimized for long spans (Joshi et al., 2020; Beltagy et al., 2020), continuous pre-training on the job posting domain (Han and Eisenstein, 2019; Gururangan et al., 2020), and multi-task learning (Caruana, 1997). Our results show that the domain-adapted models significantly outperform their non-adapted counterparts, and single-task outperforms multi-task learning.",
}
``` | [
-0.05598774924874306,
-0.05565176159143448,
0.03357158228754997,
0.015421109274029732,
0.005415663123130798,
0.023664088919758797,
0.05156513303518295,
-0.05692017450928688,
-0.0102796396240592,
-0.04219409078359604,
0.03475611284375191,
0.04258475452661514,
0.06996939331293106,
0.01257933396846056,
-0.0013045337982475758,
0.044166531413793564,
0.04916468262672424,
0.012058485299348831,
-0.062291547656059265,
-0.09932954609394073,
0.05502225086092949,
0.03364217281341553,
0.02708386816084385,
0.01463782787322998,
0.04906556010246277,
-0.025906655937433243,
-0.03758813068270683,
-0.018200716003775597,
0.08247169107198715,
-0.020677650347352028,
-0.034557756036520004,
0.05077458918094635,
0.021099330857396126,
0.07570933550596237,
0.026409238576889038,
0.11507215350866318,
-0.042185939848423004,
-0.01876671425998211,
0.0394335575401783,
-0.008910325355827808,
-0.012430379167199135,
-0.06748054176568985,
0.010286415927112103,
-0.00858611986041069,
0.08637319505214691,
0.019549783319234848,
-0.025236885994672775,
0.006928915623575449,
-0.11340835690498352,
-0.05916562303900719,
-0.07128031551837921,
-0.06725358963012695,
0.14388757944107056,
0.06169223040342331,
0.01566365920007229,
0.022714585065841675,
0.017791030928492546,
0.027985600754618645,
0.01598198525607586,
-0.02089715003967285,
-0.07502919435501099,
-0.04871046915650368,
-0.07478711754083633,
-0.011397065594792366,
0.037162020802497864,
0.0026259382721036673,
-0.08330297470092773,
0.007699719630181789,
-0.05554758757352829,
0.046143487095832825,
-0.06235511228442192,
0.009235837496817112,
-0.07272930443286896,
0.06014289706945419,
0.033742647618055344,
-0.015761684626340866,
0.03135247528553009,
0.01333524752408266,
0.023539436981081963,
-0.1020951196551323,
0.03609198331832886,
-0.07213281095027924,
0.020138410851359367,
-0.015001491643488407,
0.0006050250958651304,
0.017800137400627136,
0.03278479352593422,
0.014156414195895195,
0.09064953029155731,
-0.01597338356077671,
-0.016304882243275642,
-0.06779064983129501,
0.008202052675187588,
0.01813550479710102,
-0.07834137231111526,
0.0028953966684639454,
-0.01894962042570114,
0.10152465105056763,
-0.039399027824401855,
0.05301593616604805,
0.05459548905491829,
0.005334882996976376,
0.03133506327867508,
-0.01895894855260849,
-0.05593378096818924,
-0.059939198195934296,
-0.005712694022804499,
0.09259936958551407,
-0.007902830839157104,
-0.0254812054336071,
-0.021154556423425674,
0.015392987057566643,
-0.06186642497777939,
0.015348010696470737,
0.0010599000379443169,
0.006378531455993652,
0.016661573201417923,
0.0019969837740063667,
0.042597342282533646,
0.08569572865962982,
0.0023266319185495377,
0.0891658216714859,
-0.0029946190770715475,
-0.05539475008845329,
-0.043742403388023376,
-0.03694693744182587,
0.0036721392534673214,
4.907780382837224e-33,
0.07843972742557526,
0.07887952774763107,
-0.010495925322175026,
0.03952965512871742,
-0.017142891883850098,
-0.04972311481833458,
-0.044794704765081406,
0.05436941236257553,
0.048177123069763184,
-0.023567823693156242,
-0.04727133736014366,
0.02043311856687069,
-0.013290674425661564,
0.06726931780576706,
-0.01650886796414852,
0.001122923451475799,
0.028232600539922714,
0.003452690551057458,
0.015375109389424324,
0.027421120554208755,
0.08250803500413895,
0.04133540764451027,
-0.03883899748325348,
-0.015691274777054787,
-0.016287514939904213,
0.02118079364299774,
0.05139797553420067,
-0.08785709738731384,
-0.05462417006492615,
0.0470488965511322,
-0.08512169867753983,
0.0031982557848095894,
-0.04508237913250923,
0.01877320557832718,
-0.0008011428290046751,
0.018106261268258095,
-0.004484521225094795,
-0.037122692912817,
0.04492011293768883,
-0.02784378081560135,
-0.050842221826314926,
0.019456416368484497,
0.08967192471027374,
-0.03298125043511391,
-0.0574038065969944,
-0.05140737444162369,
0.029861366376280785,
-0.015182546339929104,
0.003316171932965517,
0.07179596275091171,
0.0708826556801796,
-0.002825358184054494,
0.019912075251340866,
-0.054078955203294754,
-0.004966953303664923,
0.09685809165239334,
0.061313800513744354,
0.030233515426516533,
0.021618176251649857,
0.0766926184296608,
0.0020311204716563225,
-0.023378783836960793,
0.022995376959443092,
0.03274206444621086,
0.08875320851802826,
-0.016483034938573837,
-0.0669097974896431,
0.04621654003858566,
0.13586370646953583,
-0.034012049436569214,
-0.05081807076931,
-0.028328100219368935,
-0.025222087278962135,
-0.006438558921217918,
0.027197761461138725,
-0.05600395426154137,
0.019161006435751915,
-0.10914924740791321,
-0.019587576389312744,
0.08380318433046341,
0.005135863553732634,
-0.05062531307339668,
-0.029012851417064667,
-0.0328545942902565,
0.021233079954981804,
-0.035856496542692184,
0.054983511567115784,
-0.09088770300149918,
0.0347290113568306,
0.013372831046581268,
0.02599175274372101,
-0.04402494803071022,
0.01969991996884346,
0.05123237892985344,
-0.04198312386870384,
-5.691249939054171e-33,
-0.00552188279107213,
0.023095469921827316,
-0.10482335835695267,
0.07506958395242691,
0.016455577686429024,
-0.07320371270179749,
0.13539624214172363,
0.10133086144924164,
-0.010126810520887375,
-0.03639347851276398,
-0.010746654123067856,
-0.05909901112318039,
0.020617593079805374,
0.0291023850440979,
-0.0030734864994883537,
-0.03088921122252941,
-0.009021501988172531,
0.03765149414539337,
0.023585565388202667,
0.09294089674949646,
0.03951141983270645,
-0.027032623067498207,
-0.11322060972452164,
0.07970104366540909,
0.04342280700802803,
0.033215951174497604,
0.0148477079346776,
0.037499941885471344,
-0.06986385583877563,
0.08067229390144348,
-0.05131823197007179,
-0.022503234446048737,
-0.03911026939749718,
0.0565790981054306,
-0.10535068064928055,
-0.04006390646100044,
-0.05121351778507233,
0.004304422065615654,
0.03307056427001953,
0.06322718411684036,
0.031599510461091995,
-0.004790385253727436,
-0.0785367414355278,
-0.017396407201886177,
-0.04791446775197983,
-0.0316016748547554,
-0.10624368488788605,
-0.05157720297574997,
-0.044072601944208145,
0.017307955771684647,
0.022606901824474335,
0.042473092675209045,
-0.09678328037261963,
0.020312989130616188,
-0.07625400274991989,
-0.10257052630186081,
0.04398225620388985,
-0.12842440605163574,
-0.07603581249713898,
-0.010711060836911201,
-0.05947502702474594,
0.06533670425415039,
0.04977934807538986,
-0.007799998391419649,
0.09225916862487793,
-0.122822105884552,
-0.008849798701703548,
0.03233174607157707,
-0.046385668218135834,
-0.09802491217851639,
0.09121674299240112,
0.006986682768911123,
-0.030704861506819725,
0.007613162975758314,
-0.06810746341943741,
-0.04595319926738739,
0.007504885550588369,
-0.04222770780324936,
-0.014224443584680557,
-0.04433593526482582,
-0.09650479257106781,
-0.0629432275891304,
0.025083787739276886,
0.06916630268096924,
0.00026459372020326555,
0.06885171681642532,
-0.02853439189493656,
0.00040882721077650785,
0.03028997778892517,
-0.03216230496764183,
-0.013187237083911896,
0.023356949910521507,
-0.02940448559820652,
0.06254695355892181,
-0.10053818672895432,
-6.414413888933268e-8,
-0.08910912275314331,
-0.016035383567214012,
-0.040152084082365036,
0.023321112617850304,
0.03885515779256821,
-0.04961665719747543,
-0.018147775903344154,
0.022207682952284813,
0.014178403653204441,
-0.0413762629032135,
-0.019042616710066795,
0.05423976480960846,
-0.023634282872080803,
-0.08670033514499664,
0.0256053376942873,
0.08765175193548203,
0.04242897033691406,
0.03824916109442711,
-0.06331916898488998,
-0.06952542066574097,
0.07386960834264755,
0.055543676018714905,
-0.03419129177927971,
-0.03351937234401703,
0.018894707784056664,
-0.009096214547753334,
-0.07776028662919998,
0.11257510632276535,
0.003582478268072009,
0.0022880504839122295,
-0.010234592482447624,
-0.007110831327736378,
-0.05548635497689247,
-0.030958684161305428,
0.07178928703069687,
0.07327147573232651,
0.03883320465683937,
-0.11701097339391708,
-0.013951338827610016,
0.054405950009822845,
0.02923567220568657,
0.010536612942814827,
-0.06484529376029968,
-0.022688541561365128,
0.0944422036409378,
-0.00536387600004673,
-0.052275776863098145,
-0.0212105643004179,
0.03645854815840721,
-0.01731843873858452,
0.05572674050927162,
-0.008159722201526165,
-0.047942861914634705,
0.03628968447446823,
0.042186152189970016,
0.02485402673482895,
0.031404029577970505,
-0.08379030227661133,
0.006671524606645107,
0.017595261335372925,
0.04032259061932564,
0.0545109361410141,
0.06764189153909683,
-0.0034674175549298525
] |
Laggrif/DialoGPT-medium-Luke | 36fcfc7f3d7209dcb7a349804a7a6f5dab2ddd94 | 2022-06-21T17:50:07.000Z | [
"pytorch",
"gpt2",
"text-generation",
"transformers",
"conversational"
] | conversational | false | Laggrif | null | Laggrif/DialoGPT-medium-Luke | 2,138 | null | transformers | ---
tags:
- conversational
---
# Luke DialoGPT Model | [
-0.0526893176138401,
-0.0894097313284874,
0.05479353293776512,
-0.0293112862855196,
0.010608881711959839,
0.009249537251889706,
0.10537885129451752,
0.03502672165632248,
0.10639014095067978,
-0.01961355097591877,
0.009214483201503754,
-0.013150538317859173,
0.03444003313779831,
0.012775237672030926,
0.0396619588136673,
-0.0007137682987377048,
-0.014399743638932705,
-0.06745891273021698,
-0.023374294862151146,
0.033646292984485626,
0.005280607845634222,
0.14833825826644897,
0.031009720638394356,
0.025535419583320618,
0.000935589661821723,
0.0349755585193634,
-0.042661383748054504,
-0.034270431846380234,
0.05768926069140434,
-0.014739257283508778,
0.05357423797249794,
0.06516434252262115,
0.0376121923327446,
0.002690446563065052,
-0.055041611194610596,
-0.003547523869201541,
0.006839607376605272,
0.04339976981282234,
0.050900865346193314,
-0.0011980986455455422,
-0.06371702998876572,
-0.005351820960640907,
-0.03946700692176819,
-0.00604829890653491,
0.06098208576440811,
-0.04506806284189224,
-0.059215690940618515,
-0.024177704006433487,
0.00997361820191145,
0.048387277871370316,
-0.09319135546684265,
-0.03621356934309006,
0.007168231066316366,
0.09274788200855255,
-0.008571036159992218,
0.04680420085787773,
-0.0615130178630352,
-0.08317676186561584,
0.07475356757640839,
0.012447904795408249,
-0.058252181857824326,
0.01861867681145668,
-0.09669565409421921,
0.047370657324790955,
0.0010304073803126812,
0.0727267861366272,
-0.07219858467578888,
0.015450715087354183,
-0.03309013321995735,
0.08548319339752197,
-0.0037370752543210983,
0.02785654179751873,
0.05902979150414467,
-0.03518078476190567,
-0.02043243497610092,
-0.015051278285682201,
0.013489753939211369,
-0.014066913165152073,
0.06554282456636429,
-0.0003985466610174626,
0.0017604733584448695,
-0.11191651970148087,
0.016616106033325195,
0.01091669499874115,
-0.021902404725551605,
-0.04650679603219032,
-0.007727142423391342,
-0.05814417451620102,
0.020407136529684067,
0.020873958244919777,
-0.08849147707223892,
-0.09963588416576385,
0.06213408708572388,
0.03216971084475517,
-0.027526186779141426,
0.014342619106173515,
-0.04554985836148262,
-0.09174438565969467,
-0.042556215077638626,
0.10788771510124207,
-0.013571163639426231,
0.06373988091945648,
0.0503225140273571,
-0.07542797178030014,
0.004124489612877369,
0.03155837953090668,
-0.003917742986232042,
0.002465061144903302,
0.045089393854141235,
-0.037222400307655334,
-0.013844575732946396,
-0.045803457498550415,
0.01035788469016552,
-0.03279595822095871,
0.08562345057725906,
-0.07970194518566132,
0.08569809049367905,
-0.03517930209636688,
0.023025983944535255,
-0.012367719784379005,
-0.019537555053830147,
0.004160820972174406,
-0.022238323464989662,
-0.022647427394986153,
-0.020082995295524597,
-0.02995426207780838,
-0.042308010160923004,
-2.7289571552079733e-33,
0.10587260872125626,
-0.009339005686342716,
0.07939033955335617,
0.08419574052095413,
0.04419710114598274,
0.05739142373204231,
-0.07549930363893509,
-0.06134985387325287,
-0.006019668187946081,
-0.03206055611371994,
-0.028253352269530296,
-0.08377210050821304,
-0.07907312363386154,
0.0631401464343071,
0.0033153691329061985,
0.050371356308460236,
-0.09079482406377792,
0.005117482505738735,
-0.016943132504820824,
-0.06414962559938431,
-0.026626400649547577,
0.06178291514515877,
0.011701553128659725,
0.06890431046485901,
0.116502545773983,
0.062464114278554916,
0.0915885791182518,
-0.057565875351428986,
-0.036462485790252686,
0.06637521833181381,
-0.05276785418391228,
0.045279961079359055,
-0.027804534882307053,
0.012671501375734806,
0.013604114763438702,
0.04920767620205879,
-0.03666403517127037,
-0.007471953984349966,
0.028648318722844124,
-0.08328387141227722,
-0.02952684462070465,
-0.017000621184706688,
-0.025673866271972656,
-0.039701566100120544,
-0.026283126324415207,
0.05243980512022972,
0.009451182559132576,
0.041022516787052155,
0.010799562558531761,
0.055320240557193756,
-0.025390708819031715,
0.04851160943508148,
-0.025563683360815048,
-0.0604332834482193,
-0.001372873899526894,
-0.05431791767477989,
-0.004896410275250673,
0.07107667624950409,
0.014895042404532433,
-0.0048537482507526875,
-0.0020748514216393232,
0.0058206114917993546,
0.04672528803348541,
-0.058548230677843094,
0.07603945583105087,
0.047567736357450485,
-0.07699110358953476,
-0.0548115074634552,
0.025833427906036377,
-0.05269067734479904,
-0.06867892295122147,
0.019004452973604202,
-0.00645454041659832,
0.03784600645303726,
-0.010735183954238892,
0.020301369950175285,
-0.04767220467329025,
-0.08254074305295944,
0.06121357902884483,
0.04193812981247902,
-0.05653974413871765,
-0.10842923074960709,
-0.035784199833869934,
-0.0010965430410578847,
0.0003011144290212542,
-0.07185345143079758,
0.04809162765741348,
-0.06053617224097252,
0.013443728908896446,
-0.008765616454184055,
0.06138652190566063,
0.022061483934521675,
-0.037264131009578705,
0.02338133193552494,
-0.082307830452919,
5.433437028282973e-34,
-0.03583727031946182,
-0.011135163716971874,
-0.10356228798627853,
0.09991397708654404,
0.03280539810657501,
-0.06939071416854858,
0.017517821863293648,
0.13391011953353882,
0.04069456085562706,
-0.040729936212301254,
-0.043564628809690475,
0.05534830316901207,
-0.034628041088581085,
-0.000024147977455868386,
0.12301640212535858,
-0.0005336266476660967,
0.04823155328631401,
-0.05460255593061447,
-0.011930957436561584,
0.019374236464500427,
0.11647932976484299,
-0.002493214560672641,
-0.13496658205986023,
0.002784022130072117,
-0.01041165366768837,
-0.004853151272982359,
-0.05185827612876892,
0.08459313958883286,
0.09521501511335373,
-0.05282492935657501,
-0.08520771563053131,
0.027624329552054405,
-0.015070146881043911,
-0.05099678412079811,
-0.023666732013225555,
0.02097335085272789,
0.06066349148750305,
0.004547464195638895,
0.015818139538168907,
0.042617782950401306,
0.06005597114562988,
-0.04577859118580818,
-0.008766957558691502,
-0.04581109434366226,
-0.01341517735272646,
-0.05355963855981827,
-0.0202105063945055,
-0.05875985696911812,
-0.0782180055975914,
0.0007938285125419497,
0.007175665348768234,
-0.038268737494945526,
-0.02978977933526039,
-0.027619346976280212,
-0.07914897054433823,
-0.02736695669591427,
0.0040484643541276455,
-0.016312861815094948,
0.02981739491224289,
0.006216596346348524,
-0.03163247928023338,
-0.05698630213737488,
0.04695127159357071,
-0.01981458067893982,
0.01653103157877922,
-0.07381957024335861,
0.028601180762052536,
0.0007323076715692878,
0.017602941021323204,
-0.037369515746831894,
0.06517615169286728,
-0.01821717619895935,
-0.0029928646981716156,
0.03252057731151581,
0.0725775957107544,
-0.00275333016179502,
-0.021245330572128296,
-0.06855858862400055,
0.02335568331182003,
-0.08709560334682465,
-0.026384852826595306,
-0.020114056766033173,
0.04652481898665428,
0.0866672545671463,
0.02560841664671898,
0.05313611775636673,
0.026942534372210503,
0.0788472592830658,
0.003434441750869155,
0.02447705715894699,
0.007259608246386051,
-0.009445317089557648,
0.03156520426273346,
0.06813231110572815,
-0.031050710007548332,
-2.2690860745910868e-8,
-0.09099132567644119,
-0.02760530449450016,
-0.024584874510765076,
0.039453912526369095,
0.03888306766748428,
0.03573960065841675,
0.05891590192914009,
0.04605509713292122,
-0.0007312737870961428,
0.0012003821320831776,
0.07648446410894394,
0.10752055048942566,
-0.0007955793989822268,
0.03373293578624725,
-0.03562712296843529,
0.05395315960049629,
-0.06258240342140198,
0.01814737543463707,
-0.02808569185435772,
-0.03836102411150932,
0.07535266131162643,
-0.018994558602571487,
-0.015604202635586262,
0.08145580440759659,
0.056440453976392746,
-0.05942760780453682,
-0.08234068006277084,
0.10083857923746109,
-0.10237855464220047,
0.06104230135679245,
-0.014345947653055191,
0.10640992224216461,
-0.13020065426826477,
-0.06103232130408287,
-0.08512687683105469,
0.014924733899533749,
0.003179488703608513,
-0.04485630616545677,
0.025152286514639854,
-0.011256908066570759,
0.02945082075893879,
0.03687401860952377,
-0.0985434278845787,
-0.004129305947571993,
0.08615656197071075,
0.015797534957528114,
0.03103627637028694,
-0.07229790836572647,
-0.017681928351521492,
-0.06707017868757248,
-0.03405211493372917,
0.0019694699440151453,
0.017780082300305367,
-0.0011022606631740928,
-0.024024562910199165,
-0.013622754253447056,
-0.003740692511200905,
0.02060490846633911,
0.057125382125377655,
-0.012460929341614246,
0.061712443828582764,
0.05702982470393181,
-0.009655366651713848,
-0.05280081927776337
] |
GroNLP/gpt2-small-italian | 9a5b0043f33d9adacd23d53e3a8e9c70f71febc9 | 2021-05-21T09:58:53.000Z | [
"pytorch",
"tf",
"jax",
"gpt2",
"text-generation",
"it",
"arxiv:2012.05628",
"transformers",
"adaption",
"recycled",
"gpt2-small"
] | text-generation | false | GroNLP | null | GroNLP/gpt2-small-italian | 2,136 | null | transformers | ---
language: it
tags:
- adaption
- recycled
- gpt2-small
pipeline_tag: text-generation
---
# GPT-2 recycled for Italian (small)
[Wietse de Vries](https://www.semanticscholar.org/author/Wietse-de-Vries/144611157) •
[Malvina Nissim](https://www.semanticscholar.org/author/M.-Nissim/2742475)
## Model description
This model is based on the small OpenAI GPT-2 ([`gpt2`](https://huggingface.co/gpt2)) model.
For details, check out our paper on [arXiv](https://arxiv.org/abs/2012.05628) and the code on [Github](https://github.com/wietsedv/gpt2-recycle).
## Related models
### Dutch
- [`gpt2-small-dutch-embeddings`](https://huggingface.co/GroNLP/gpt2-small-dutch-embeddings): Small model size with only retrained lexical embeddings.
- [`gpt2-small-dutch`](https://huggingface.co/GroNLP/gpt2-small-dutch): Small model size with retrained lexical embeddings and additional fine-tuning of the full model. (**Recommended**)
- [`gpt2-medium-dutch-embeddings`](https://huggingface.co/GroNLP/gpt2-medium-dutch-embeddings): Medium model size with only retrained lexical embeddings.
### Italian
- [`gpt2-small-italian-embeddings`](https://huggingface.co/GroNLP/gpt2-small-italian-embeddings): Small model size with only retrained lexical embeddings.
- [`gpt2-small-italian`](https://huggingface.co/GroNLP/gpt2-small-italian): Small model size with retrained lexical embeddings and additional fine-tuning of the full model. (**Recommended**)
- [`gpt2-medium-italian-embeddings`](https://huggingface.co/GroNLP/gpt2-medium-italian-embeddings): Medium model size with only retrained lexical embeddings.
## How to use
```python
from transformers import pipeline
pipe = pipeline("text-generation", model="GroNLP/gpt2-small-italian")
```
```python
from transformers import AutoTokenizer, AutoModel, TFAutoModel
tokenizer = AutoTokenizer.from_pretrained("GroNLP/gpt2-small-italian")
model = AutoModel.from_pretrained("GroNLP/gpt2-small-italian") # PyTorch
model = TFAutoModel.from_pretrained("GroNLP/gpt2-small-italian") # Tensorflow
```
## BibTeX entry
```bibtex
@misc{devries2020good,
title={As good as new. How to successfully recycle English GPT-2 to make models for other languages},
author={Wietse de Vries and Malvina Nissim},
year={2020},
eprint={2012.05628},
archivePrefix={arXiv},
primaryClass={cs.CL}
}
```
| [
-0.11205121874809265,
-0.012354469858109951,
-0.020820504054427147,
0.02239690162241459,
0.06895915418863297,
-0.07969732582569122,
-0.027879701927304268,
0.0686274841427803,
-0.007760918233543634,
-0.0899244025349617,
0.000037691912439186126,
-0.00284908851608634,
-0.043342225253582,
0.05863972380757332,
-0.024696212261915207,
0.007106631062924862,
-0.008107306435704231,
0.06675665080547333,
-0.024381617084145546,
-0.010808021761476994,
0.033447474241256714,
0.017796199768781662,
0.06339248269796371,
0.018569236621260643,
0.03303966298699379,
0.020792122930288315,
0.028940588235855103,
0.006634693592786789,
0.04988918825984001,
-0.029871374368667603,
0.03507004305720329,
0.06732909381389618,
-0.040817879140377045,
0.0412314236164093,
0.002856082748621702,
0.08817464113235474,
-0.01672433689236641,
-0.0286265816539526,
-0.061865296214818954,
-0.022004539147019386,
0.06785380095243454,
-0.01246094610542059,
-0.01765824481844902,
0.007710270117968321,
0.08517578989267349,
-0.01924184337258339,
-0.021021807566285133,
-0.034952472895383835,
-0.18947453796863556,
-0.040063027292490005,
0.01966319978237152,
-0.05115027725696564,
-0.02426634356379509,
0.038239337503910065,
0.029141541570425034,
0.006480146199464798,
-0.03635337948799133,
-0.07954370230436325,
-0.006088973488658667,
-0.021439779549837112,
0.06257493793964386,
-0.008340015076100826,
-0.11292358487844467,
0.00402724277228117,
-0.01081128604710102,
0.06096179783344269,
0.012101462110877037,
0.04497166723012924,
-0.015012962743639946,
-0.0292158592492342,
-0.0438440702855587,
0.01534857228398323,
-0.08789608627557755,
0.0005318408948369324,
0.021938402205705643,
0.07432691007852554,
0.049063362181186676,
0.006782192271202803,
-0.024409493431448936,
-0.039096783846616745,
0.04739852249622345,
0.03433727100491524,
0.09187152236700058,
-0.04563506692647934,
-0.0035872231237590313,
0.01503452006727457,
0.07002156972885132,
0.02197963558137417,
0.049854785203933716,
-0.02696089632809162,
-0.04554472118616104,
0.04558802768588066,
0.07855235785245895,
0.032687339931726456,
-0.006780683994293213,
-0.037393201142549515,
0.0330669991672039,
0.012536752037703991,
-0.04681890830397606,
0.08137331902980804,
0.020622344687581062,
0.05426938459277153,
0.02011610008776188,
0.02656150422990322,
-0.07305063307285309,
-0.0376385934650898,
-0.017006760463118553,
0.03730718791484833,
-0.008300704881548882,
-0.02565460279583931,
0.01844591461122036,
-0.013806450180709362,
-0.007372591644525528,
-0.06510064005851746,
-0.024110132828354836,
0.02358103357255459,
-0.045349497348070145,
-0.02504807710647583,
0.004766352940350771,
0.062386203557252884,
-0.09787119925022125,
0.03231121227145195,
-0.02885979227721691,
-0.0969659686088562,
-0.025179952383041382,
0.07406994700431824,
-0.055445533245801926,
3.201367396310327e-33,
0.05795983597636223,
0.072576142847538,
-0.016333656385540962,
0.020416686311364174,
0.06684286147356033,
0.04364960268139839,
-0.00041501439409330487,
-0.10105424374341965,
-0.02370475046336651,
-0.08272106945514679,
-0.01715785823762417,
0.02129075862467289,
-0.09829457104206085,
0.043134938925504684,
0.01312334556132555,
-0.05024297535419464,
-0.020816927775740623,
0.08432038128376007,
0.03136688470840454,
0.01910294219851494,
0.04093330726027489,
0.14795076847076416,
-0.017323093488812447,
-0.03145401179790497,
0.008526962250471115,
0.024452682584524155,
0.006561994086951017,
-0.08825592696666718,
-0.01218319684267044,
0.03471718356013298,
-0.043314334005117416,
0.005378709174692631,
-0.0035811616107821465,
0.04167468845844269,
-0.042254239320755005,
-0.031047021970152855,
-0.041719093918800354,
-0.1220078319311142,
0.026446782052516937,
-0.06841905415058136,
0.068113312125206,
0.03748564422130585,
0.07109460979700089,
-0.07918136566877365,
-0.03473077714443207,
-0.06600194424390793,
0.048873964697122574,
0.025989409536123276,
-0.015903452411293983,
0.016266200691461563,
-0.0177537240087986,
0.060471706092357635,
-0.08564111590385437,
0.034731391817331314,
-0.022175809368491173,
0.010126297362148762,
0.04302690178155899,
0.017084671184420586,
0.10989031940698624,
0.05208325386047363,
-0.008143511600792408,
0.05953556299209595,
0.062336016446352005,
0.013553161174058914,
0.04066247493028641,
0.09578082710504532,
-0.054769501090049744,
0.02023959718644619,
0.04485497251152992,
0.07145530730485916,
-0.06354192644357681,
0.03825930133461952,
-0.01601267419755459,
0.035622380673885345,
0.09380359947681427,
-0.04925772547721863,
0.019822556525468826,
-0.10451031476259232,
0.014889881946146488,
0.0057134986855089664,
-0.13878871500492096,
-0.015234633348882198,
0.013083439320325851,
-0.02954000234603882,
-0.07811111211776733,
-0.10119003057479858,
0.024817142635583878,
-0.08933987468481064,
0.0018109324155375361,
-0.010232744738459587,
-0.04375665634870529,
-0.05954710766673088,
-0.06262598186731339,
-0.032702021300792694,
0.055754467844963074,
-3.0877191331048894e-33,
0.08110249042510986,
-0.021533280611038208,
-0.008847828954458237,
0.1003909781575203,
-0.043630775064229965,
-0.14202457666397095,
0.008320213295519352,
0.10483671724796295,
0.01677592098712921,
-0.033468641340732574,
0.03944046050310135,
0.02480223961174488,
0.09279792755842209,
0.0335979200899601,
0.10487724095582962,
-0.06869321316480637,
0.014143862761557102,
-0.09016616642475128,
0.047874875366687775,
0.06128005310893059,
0.048137836158275604,
0.004528477322310209,
-0.11938270181417465,
0.01961682178080082,
-0.0036057177931070328,
0.03145948052406311,
0.01272554136812687,
-0.05906018242239952,
0.05193629115819931,
-0.051744163036346436,
-0.016996972262859344,
0.018594713881611824,
-0.05562012642621994,
0.019057128578424454,
-0.05639071762561798,
0.02476874366402626,
0.09013084322214127,
0.06513852626085281,
-0.03599221259355545,
0.03515453264117241,
0.02432326041162014,
-0.008407180197536945,
-0.025730885565280914,
0.031009817495942116,
-0.03905108571052551,
0.03297895938158035,
-0.022122565656900406,
0.01213311031460762,
-0.014504059217870235,
-0.09757304936647415,
0.031929146498441696,
0.015498603694140911,
-0.0405111201107502,
0.0346708670258522,
-0.0262150801718235,
-0.07791391760110855,
0.07531265169382095,
-0.02356773614883423,
-0.11723380535840988,
-0.006772016640752554,
-0.00200302479788661,
-0.06376946717500687,
0.03171583265066147,
-0.043362807482481,
-0.006780441850423813,
-0.1000048816204071,
-0.04112166911363602,
-0.027817625552415848,
0.029202500358223915,
-0.017221393063664436,
0.04910654574632645,
-0.031725671142339706,
0.00881209596991539,
0.026062730699777603,
0.01781078428030014,
-0.024200424551963806,
0.01064752135425806,
0.013888955116271973,
0.09287925064563751,
-0.04898638650774956,
-0.022673912346363068,
0.023999415338039398,
0.05951809510588646,
0.027186697348952293,
0.10262025147676468,
-0.08295261114835739,
0.01590091548860073,
0.022459901869297028,
0.01764390617609024,
0.05152465030550957,
-0.05446596071124077,
0.10225459188222885,
0.002804012503474951,
0.11923506110906601,
-0.010844185948371887,
-5.185985330058429e-8,
-0.0480765774846077,
-0.04851033538579941,
-0.032634034752845764,
0.09864836186170578,
-0.10182902216911316,
-0.08302383869886398,
-0.005803620908409357,
0.01991288550198078,
-0.004109142813831568,
0.0077090151607990265,
0.008733406662940979,
0.012505173683166504,
-0.058446891605854034,
-0.06570305675268173,
0.0033234120346605778,
0.08099089562892914,
0.010507547296583652,
0.032437365502119064,
-0.06150703877210617,
0.00763903371989727,
-0.06540579348802567,
0.029585514217615128,
0.04362509772181511,
-0.041379738599061966,
-0.03516823425889015,
-0.03698260337114334,
-0.006303003989160061,
0.019430937245488167,
0.02121219038963318,
-0.018501155078411102,
0.06185544654726982,
0.02971312589943409,
-0.0070960186421871185,
0.046740997582674026,
0.0060888552106916904,
0.017713842913508415,
-0.02697952650487423,
-0.015281849540770054,
0.047761667519807816,
-0.026915479451417923,
0.09605255722999573,
-0.03259860724210739,
-0.07984990626573563,
-0.00010504922101972625,
0.08281373232603073,
0.00977332703769207,
-0.06886535882949829,
-0.08894144743680954,
0.006708852481096983,
0.02538258023560047,
-0.03254443779587746,
0.012755978852510452,
-0.03379008546471596,
0.03727586939930916,
0.01877729222178459,
0.0421019084751606,
-0.03574177995324135,
-0.011284888722002506,
0.05480074882507324,
0.01369891595095396,
0.015931319445371628,
0.016379699110984802,
0.05849003791809082,
0.020860474556684494
] |
hf-internal-testing/tiny-random-unispeech | a90315fed34d4891a62a539637210f2bdd30e68f | 2022-01-26T13:56:35.000Z | [
"pytorch",
"unispeech",
"audio-classification",
"transformers"
] | audio-classification | false | hf-internal-testing | null | hf-internal-testing/tiny-random-unispeech | 2,136 | null | transformers | Entry not found | [
0.0461147278547287,
-0.038838207721710205,
-0.01049656979739666,
-0.03682169318199158,
0.011261860840022564,
0.013094935566186905,
0.0019101888174191117,
-0.013979103416204453,
0.027092741802334785,
-0.015212527476251125,
0.017284274101257324,
-0.08189476281404495,
0.03817418962717056,
-0.04920130595564842,
0.021389011293649673,
-0.015245908871293068,
-0.03203780576586723,
-0.1245758980512619,
0.03150877356529236,
0.032381657510995865,
-0.060957908630371094,
0.05409295856952667,
-0.025087490677833557,
0.01568586938083172,
0.028129950165748596,
-0.04710396006703377,
-0.018688226118683815,
0.013785239309072495,
-0.04001208767294884,
0.01173911802470684,
-0.04317743331193924,
0.05500618368387222,
0.004543041344732046,
0.02973111905157566,
0.14852192997932434,
0.02658126689493656,
0.02907961793243885,
-0.05169107764959335,
0.05803573504090309,
-0.07732241600751877,
-0.017637968063354492,
-0.04219653457403183,
0.041807834059000015,
0.023620979860424995,
0.021563321352005005,
0.016478516161441803,
-0.0021814992651343346,
-0.06400240957736969,
0.06393089145421982,
0.019599027931690216,
-0.08565037697553635,
0.00934905931353569,
-0.008718925528228283,
-0.028583496809005737,
-0.07310017943382263,
0.09416428208351135,
0.001759322709403932,
0.06184990331530571,
0.011840506456792355,
-0.035997264087200165,
0.08358278125524521,
-0.02619801089167595,
0.03736566752195358,
-0.028206506744027138,
-0.07454850524663925,
-0.08883563429117203,
-0.06279942393302917,
-0.008695344440639019,
0.014119276776909828,
-0.0825355276465416,
0.0649217739701271,
-0.00223911227658391,
-0.14716917276382446,
0.07743025571107864,
-0.03548373281955719,
-0.055201586335897446,
0.006981803569942713,
-0.012166670523583889,
0.055111464112997055,
-0.007116836030036211,
-0.023175746202468872,
-0.005835152696818113,
-0.09185640513896942,
0.055196937173604965,
0.034148022532463074,
0.03835180774331093,
0.038685429841279984,
-0.025987252593040466,
0.017804903909564018,
0.022428328171372414,
0.025005368515849113,
-0.10761535167694092,
-0.048001550137996674,
-0.04343584179878235,
0.012374646961688995,
-0.019502125680446625,
0.029218152165412903,
0.0842173621058464,
-0.011719699949026108,
0.09283553808927536,
-0.007015465293079615,
-0.03543110564351082,
-0.06936459988355637,
0.09425332397222519,
-0.010958523489534855,
-0.00805904995650053,
0.004974212497472763,
-0.0031528924591839314,
0.06105927750468254,
-0.03964288905262947,
-0.03619541600346565,
-0.019901901483535767,
0.07134733349084854,
0.039514873176813126,
-0.012729483656585217,
-0.006646515801548958,
-0.04746140539646149,
-0.014432490803301334,
-0.05157482624053955,
0.09506245702505112,
-0.049747664481401443,
-0.04591796174645424,
-0.008965466171503067,
-0.0325421579182148,
-0.08626784384250641,
-0.06624380499124527,
0.02538885548710823,
-4.303924894057984e-33,
0.01133066974580288,
0.0033434738870710135,
-0.002155609894543886,
0.04871906340122223,
-0.023564351722598076,
-0.07933273911476135,
0.0600903145968914,
0.02335330657660961,
-0.03844716399908066,
-0.020433755591511726,
-0.06952055543661118,
-0.03235611692070961,
0.0062485747039318085,
0.064804308116436,
-0.03201229125261307,
0.061689723283052444,
0.0417000837624073,
-0.00761845987290144,
0.03340127319097519,
-0.047770582139492035,
0.00887306872755289,
-0.04066338762640953,
-0.010506896302103996,
0.0106519665569067,
0.021333497017621994,
0.12854498624801636,
-0.009705503471195698,
0.010055632330477238,
-0.017507633194327354,
0.006515394430607557,
0.06334009766578674,
-0.057817306369543076,
0.013668818399310112,
-0.020286159589886665,
0.05430467426776886,
-0.023184705525636673,
0.0828516036272049,
0.0005449643940664828,
-0.10372652113437653,
-0.07634282112121582,
-0.005381610710173845,
-0.039263784885406494,
0.0006114727002568543,
-0.013281986117362976,
0.07119110971689224,
0.043696220964193344,
0.03168422728776932,
0.04338686540722847,
0.05728672817349434,
0.0832006186246872,
-0.07961414009332657,
0.015234283171594143,
0.017002005130052567,
0.047004107385873795,
-0.09794387966394424,
0.004990279674530029,
-0.07062993198633194,
-0.028000490739941597,
-0.04018733277916908,
-0.0702052190899849,
0.011351344175636768,
0.06020182743668556,
-0.03297270089387894,
0.09396500885486603,
0.03417910635471344,
-0.019825750961899757,
-0.034690454602241516,
-0.013036907650530338,
0.05896938592195511,
-0.012359356507658958,
-0.017275206744670868,
-0.07982361316680908,
0.02059139870107174,
0.06737419217824936,
0.04176458343863487,
-0.04978838190436363,
-0.05877475067973137,
-0.06289287656545639,
-0.03354167565703392,
-0.03871942684054375,
0.009898529388010502,
-0.05514208599925041,
-0.11629002541303635,
-0.011855563148856163,
0.10663620382547379,
0.037354156374931335,
-0.0065480442717671394,
-0.051189567893743515,
0.06663123518228531,
0.01874656230211258,
0.032841797918081284,
0.041593004018068314,
-0.06879369914531708,
0.04216769337654114,
-0.01628219522535801,
5.4139394340936695e-34,
0.05697013810276985,
-0.006972255185246468,
0.015711724758148193,
-0.17956365644931793,
0.02320219948887825,
0.007923615165054798,
-0.008062449283897877,
0.0074974060989916325,
0.07391711324453354,
0.0309313777834177,
0.060510627925395966,
0.058605875819921494,
0.09515274316072464,
-0.002282935893163085,
0.001603541080839932,
0.07024981826543808,
0.012629246339201927,
0.07425693422555923,
-0.038426291197538376,
0.01861148327589035,
0.030608950182795525,
-0.02449394389986992,
0.021528491750359535,
-0.003039651783183217,
-0.03676343336701393,
0.03130284696817398,
0.07998586446046829,
0.010451192036271095,
-0.07930229604244232,
-0.013543923385441303,
0.018781835213303566,
0.05168003588914871,
-0.07191970944404602,
0.15783067047595978,
0.026191607117652893,
0.01262354850769043,
0.08218053728342056,
-0.029807550832629204,
-0.07528624683618546,
-0.04250097647309303,
0.017244765534996986,
0.04411793500185013,
0.03708017244935036,
0.009233047254383564,
-0.040271829813718796,
0.022496428340673447,
0.02495843544602394,
0.07633638381958008,
0.005147108342498541,
0.013892097398638725,
0.05610476806759834,
-0.06684739887714386,
0.05862557515501976,
-0.020688841119408607,
0.05377643182873726,
0.06718500703573227,
0.005329249892383814,
-0.01388032827526331,
0.029931528493762016,
0.009508464485406876,
-0.045173756778240204,
0.11534366756677628,
-0.06510116159915924,
0.05117698386311531,
-0.0026125339791178703,
-0.08554837852716446,
-0.03784770518541336,
0.0804959163069725,
0.011298024095594883,
-0.07695550471544266,
-0.04868878796696663,
0.02515520341694355,
0.06252261996269226,
-0.04509226232767105,
-0.01246943511068821,
0.028559505939483643,
-0.030573077499866486,
0.05066261067986488,
-0.08187384903430939,
0.04469604790210724,
0.0034051244147121906,
0.04145054519176483,
-0.021858664229512215,
-0.06112268194556236,
-0.00908052921295166,
-0.05903250351548195,
0.0259539932012558,
0.059690944850444794,
-0.07613514363765717,
-0.03720718249678612,
-0.036316655576229095,
0.07058046013116837,
-0.008224100805819035,
0.041961874812841415,
-0.0285952128469944,
-1.496900736697171e-8,
-0.0014124972512945533,
0.03401879221200943,
-0.040338415652513504,
0.04116074740886688,
0.0935964286327362,
-0.05115952715277672,
0.0008746005478315055,
-0.03389839455485344,
-0.00567849725484848,
-0.010686947964131832,
-0.04789939522743225,
-0.04820054769515991,
-0.02011880651116371,
-0.03209094703197479,
-0.04211259260773659,
-0.10229527950286865,
-0.07819421589374542,
-0.031228765845298767,
-0.02154778689146042,
-0.04960230365395546,
0.08087796717882156,
-0.07801242172718048,
0.06919731199741364,
-0.04999840259552002,
0.03687043860554695,
0.03889009356498718,
-0.049989692866802216,
-0.04254625365138054,
-0.04606937617063522,
0.08682432025671005,
-0.031148413196206093,
0.11826753616333008,
0.034102488309144974,
-0.0208592489361763,
-0.0205202866345644,
0.027134142816066742,
0.09741277992725372,
0.051608603447675705,
0.013477512635290623,
-0.13649295270442963,
-0.022304272279143333,
0.02385953813791275,
0.038732077926397324,
-0.09249968826770782,
-0.04549082741141319,
0.054220106452703476,
0.01160438358783722,
0.051190607249736786,
0.07713303714990616,
-0.022097084671258926,
-0.06127818301320076,
-0.01857956498861313,
0.006740490905940533,
-0.00496308971196413,
0.024095389991998672,
0.0736224576830864,
-0.003481915919110179,
-0.0699305310845375,
-0.006629763171076775,
-0.0598808117210865,
0.05297163128852844,
-0.02902800403535366,
-0.027858933433890343,
-0.01287526823580265
] |
mrsinghania/asr-question-detection | 90b29f15265e6819044d484039b1ae9ca683342d | 2021-09-21T06:44:23.000Z | [
"pytorch",
"bert",
"text-classification",
"transformers"
] | text-classification | false | mrsinghania | null | mrsinghania/asr-question-detection | 2,136 | 2 | transformers | <i>Question vs Statement classifier</i> trained on more than 7k samples which were coming from spoken data in an interview setting
<b>Code for using in Transformers:</b>
from transformers import AutoTokenizer, AutoModelForSequenceClassification
tokenizer = AutoTokenizer.from_pretrained("mrsinghania/asr-question-detection")
model = AutoModelForSequenceClassification.from_pretrained("mrsinghania/asr-question-detection") | [
-0.06994037330150604,
0.021035272628068924,
-0.04242785647511482,
0.04690863937139511,
-0.015079021453857422,
0.020538851618766785,
0.018428444862365723,
0.028734387829899788,
-0.0365055613219738,
-0.08027040213346481,
-0.055534277111291885,
-0.09425815939903259,
-0.026135282590985298,
-0.028871480375528336,
-0.014969364739954472,
0.016330834478139877,
0.017589882016181946,
-0.056620508432388306,
-0.09342555701732635,
-0.08298061788082123,
0.04163229838013649,
0.11854005604982376,
0.06716598570346832,
0.07609681785106659,
0.061842985451221466,
-0.0323706790804863,
0.027317877858877182,
-0.01512688584625721,
0.08863634616136551,
0.03291149437427521,
-0.0017353249713778496,
0.018432358279824257,
0.03318661078810692,
0.12141896039247513,
-0.027515456080436707,
0.00693716574460268,
-0.04924030229449272,
0.03717353940010071,
0.02398710697889328,
-0.02080518566071987,
0.007747516501694918,
-0.08656870573759079,
-0.024499811232089996,
-0.053285714238882065,
0.03499767184257507,
-0.05986279994249344,
-0.060028042644262314,
0.028406741097569466,
0.02754782885313034,
-0.019745375961065292,
-0.09132065623998642,
-0.024844978004693985,
0.006647518370300531,
0.06300806254148483,
-0.08785552531480789,
-0.021642664447426796,
0.009240850806236267,
-0.001286364160478115,
-0.013527458533644676,
0.03989957645535469,
-0.1131359115242958,
-0.07701314240694046,
0.01960049569606781,
0.052400682121515274,
-0.07269926369190216,
-0.019443295896053314,
0.0011449585435912013,
0.009741542860865593,
0.058902692049741745,
-0.04256190359592438,
-0.05444807559251785,
0.07436531782150269,
0.032139912247657776,
0.09471534937620163,
0.06460786610841751,
0.03507635369896889,
-0.0035123296547681093,
-0.04183870926499367,
0.07964416593313217,
-0.04440882056951523,
0.03172922134399414,
-0.08395495265722275,
0.03889156132936478,
0.04842235893011093,
0.07988908141851425,
-0.021127974614501,
-0.009657267481088638,
0.010023967362940311,
-0.056448064744472504,
-0.016363391652703285,
-0.041213005781173706,
-0.11524907499551773,
0.016051650047302246,
0.03628293424844742,
-0.02019139938056469,
0.054659195244312286,
-0.04320515692234039,
0.010489674285054207,
0.0613584890961647,
0.01072701346129179,
0.011111527681350708,
-0.004969645757228136,
-0.01066967286169529,
0.013299916870892048,
-0.07729315757751465,
-0.053811658173799515,
0.02554410882294178,
-0.02783898264169693,
0.05233803391456604,
-0.03517621010541916,
0.002497493289411068,
0.02408258430659771,
0.031047850847244263,
-0.05944286286830902,
0.03374739736318588,
0.044435128569602966,
-0.012648272328078747,
0.022409824654459953,
-0.0548020601272583,
0.069724902510643,
-0.0167850349098444,
0.02837495319545269,
-0.05870168283581734,
0.0024332241155207157,
0.07890520989894867,
-0.03549644351005554,
-0.03896896168589592,
7.861410875343815e-33,
-0.017695650458335876,
0.026272060349583626,
0.0489693246781826,
0.01727164164185524,
-0.06037236005067825,
0.004222580697387457,
0.0026675236877053976,
0.05642283335328102,
0.06294938176870346,
-0.03678160533308983,
-0.019814033061265945,
0.0007047919789329171,
-0.014686609618365765,
0.026584995910525322,
-0.01977439969778061,
0.011425523087382317,
-0.08532311767339706,
-0.002277837134897709,
-0.04526950418949127,
0.011881017126142979,
0.11151953786611557,
0.0018297693459317088,
0.005987129174172878,
0.03949906677007675,
0.01837640255689621,
0.004775443580001593,
0.05758900195360184,
-0.0467112697660923,
-0.009683940559625626,
0.00008821923984214664,
-0.13591179251670837,
-0.0872822254896164,
-0.01137517485767603,
-0.015341714955866337,
0.0660107210278511,
0.026425352320075035,
0.07998186349868774,
-0.019295042380690575,
-0.07040972262620926,
-0.08232678472995758,
-0.023062918335199356,
-0.0008307982352562249,
0.05609261617064476,
-0.013181619346141815,
-0.03843251243233681,
-0.017533589154481888,
-0.05229566991329193,
-0.011505001224577427,
0.008359771221876144,
0.046464692801237106,
0.0012929099611938,
-0.024977434426546097,
-0.07032003998756409,
-0.0040347762405872345,
0.09839726984500885,
0.0014601375441998243,
0.06941850483417511,
0.00837444793432951,
0.0754474475979805,
0.03595415875315666,
-0.026304231956601143,
-0.01710863970220089,
0.04713529348373413,
0.028202340006828308,
0.0925227627158165,
-0.029847808182239532,
-0.017133772373199463,
0.01138900127261877,
0.04275413602590561,
0.014634151011705399,
0.010288065299391747,
-0.04822351783514023,
-0.03408604860305786,
-0.015744363889098167,
-0.02525714784860611,
0.025464536622166634,
-0.03402704745531082,
0.03823172673583031,
-0.01424828078597784,
0.017881281673908234,
0.01850905828177929,
-0.013347811065614223,
-0.005417036823928356,
-0.05965954437851906,
-0.0029188296757638454,
0.030056802555918694,
0.08866465091705322,
-0.0712861567735672,
0.03190401941537857,
-0.01987217739224434,
0.012727354653179646,
0.05123727396130562,
-0.031077386811375618,
-0.009620945900678635,
0.008375514298677444,
-9.925468752792362e-33,
0.01787329837679863,
0.0717330053448677,
-0.0741218626499176,
0.09051962941884995,
-0.03191603720188141,
0.0038106886204332113,
0.06498855352401733,
0.1512586772441864,
-0.01787658780813217,
-0.030442943796515465,
0.057394132018089294,
-0.0570511631667614,
0.08311353623867035,
-0.044967591762542725,
0.03745283931493759,
0.021183930337429047,
-0.034017499536275864,
0.03798660263419151,
0.05235534533858299,
0.11820364743471146,
-0.03692323714494705,
0.12837444245815277,
-0.09688209742307663,
0.002067331923171878,
-0.1158921867609024,
-0.012733166106045246,
-0.07466722279787064,
0.05588085204362869,
0.033174630254507065,
-0.0018747097346931696,
-0.04529457539319992,
-0.014011320658028126,
-0.038046132773160934,
-0.009782717563211918,
-0.07477512210607529,
-0.0035471338778734207,
0.05725989490747452,
-0.09142793715000153,
0.0026386750396341085,
0.11058894544839859,
0.06543044745922089,
0.0015864226734265685,
-0.12684881687164307,
0.009642777033150196,
-0.06225096434354782,
-0.10650947690010071,
-0.06381313502788544,
-0.03286726027727127,
0.07433486729860306,
-0.01595713384449482,
0.02059752121567726,
-0.026377379894256592,
-0.0025505004450678825,
0.0019272490171715617,
-0.040438469499349594,
-0.04030286520719528,
0.0288603026419878,
-0.04168028384447098,
-0.03529207035899162,
-0.020046021789312363,
-0.033364783972501755,
0.04629550129175186,
0.042180173099040985,
-0.12485212832689285,
0.015973959118127823,
-0.026848381385207176,
-0.02358967997133732,
0.028237666934728622,
0.02769147977232933,
-0.01910199224948883,
-0.04356600344181061,
0.02442016638815403,
0.04120718315243721,
0.00018127770454157144,
-0.01525166630744934,
-0.010190434753894806,
-0.08689620345830917,
-0.05284487456083298,
-0.031890302896499634,
-0.036273159086704254,
-0.08646294474601746,
-0.03379512578248978,
0.04092957824468613,
0.14104506373405457,
-0.05043558403849602,
0.08696827292442322,
0.04353814199566841,
0.05134256184101105,
0.03114341013133526,
0.017582913860678673,
0.018039440736174583,
0.017652148380875587,
0.022706512361764908,
0.01243678294122219,
-0.05161568894982338,
-5.0675794227572624e-8,
-0.05923568084836006,
0.05980922654271126,
0.024414721876382828,
-0.01382029615342617,
0.035979487001895905,
-0.08589192479848862,
-0.09668659418821335,
0.06756541132926941,
-0.054312847554683685,
0.03183974698185921,
-0.017986517399549484,
-0.004022806417196989,
-0.04900623485445976,
-0.002502691699191928,
0.011754737235605717,
0.03098924458026886,
0.012675294652581215,
0.07337528467178345,
0.005544215440750122,
-0.1529151052236557,
0.0683048740029335,
-0.009167639538645744,
0.0003696483909152448,
0.06689095497131348,
-0.0016142023960128427,
0.040491025894880295,
0.011513855308294296,
0.07216981798410416,
-0.06053376570343971,
-0.02545815519988537,
-0.06851290911436081,
0.017831094563007355,
-0.09122540056705475,
-0.07250344753265381,
0.0456172451376915,
0.07187595963478088,
0.01746705174446106,
-0.07612448185682297,
-0.013488070107996464,
0.029550746083259583,
-0.02811218611896038,
-0.03904999792575836,
-0.10885364562273026,
0.0007915960159152746,
-0.0013741027796640992,
0.00854069646447897,
-0.05272301658987999,
-0.12421932816505432,
-0.00011632480891421437,
-0.042266830801963806,
-0.008389842696487904,
-0.014548195526003838,
-0.017781313508749008,
0.009158573113381863,
0.07574355602264404,
0.03908030688762665,
-0.020210085436701775,
-0.04357659071683884,
-0.09131982177495956,
-0.009601944126188755,
0.05031930282711983,
0.07283147424459457,
-0.014993628486990929,
-0.030180759727954865
] |
ktrapeznikov/biobert_v1.1_pubmed_squad_v2 | 351a8218e59777dcb0a1b454ead77a0c39014bc5 | 2021-05-19T21:10:03.000Z | [
"pytorch",
"jax",
"bert",
"question-answering",
"transformers",
"autotrain_compatible"
] | question-answering | false | ktrapeznikov | null | ktrapeznikov/biobert_v1.1_pubmed_squad_v2 | 2,135 | 1 | transformers | ### Model
**[`monologg/biobert_v1.1_pubmed`](https://huggingface.co/monologg/biobert_v1.1_pubmed)** fine-tuned on **[`SQuAD V2`](https://rajpurkar.github.io/SQuAD-explorer/)** using **[`run_squad.py`](https://github.com/huggingface/transformers/blob/master/examples/question-answering/run_squad.py)**
This model is cased.
### Training Parameters
Trained on 4 NVIDIA GeForce RTX 2080 Ti 11Gb
```bash
BASE_MODEL=monologg/biobert_v1.1_pubmed
python run_squad.py \
--version_2_with_negative \
--model_type albert \
--model_name_or_path $BASE_MODEL \
--output_dir $OUTPUT_MODEL \
--do_eval \
--do_lower_case \
--train_file $SQUAD_DIR/train-v2.0.json \
--predict_file $SQUAD_DIR/dev-v2.0.json \
--per_gpu_train_batch_size 18 \
--per_gpu_eval_batch_size 64 \
--learning_rate 3e-5 \
--num_train_epochs 3.0 \
--max_seq_length 384 \
--doc_stride 128 \
--save_steps 2000 \
--threads 24 \
--warmup_steps 550 \
--gradient_accumulation_steps 1 \
--fp16 \
--logging_steps 50 \
--do_train
```
### Evaluation
Evaluation on the dev set. I did not sweep for best threshold.
| | val |
|-------------------|-------------------|
| exact | 75.97068980038743 |
| f1 | 79.37043950121722 |
| total | 11873.0 |
| HasAns_exact | 74.13967611336032 |
| HasAns_f1 | 80.94892513460755 |
| HasAns_total | 5928.0 |
| NoAns_exact | 77.79646761984861 |
| NoAns_f1 | 77.79646761984861 |
| NoAns_total | 5945.0 |
| best_exact | 75.97068980038743 |
| best_exact_thresh | 0.0 |
| best_f1 | 79.37043950121729 |
| best_f1_thresh | 0.0 |
### Usage
See [huggingface documentation](https://huggingface.co/transformers/model_doc/bert.html#bertforquestionanswering). Training on `SQuAD V2` allows the model to score if a paragraph contains an answer:
```python
start_scores, end_scores = model(input_ids)
span_scores = start_scores.softmax(dim=1).log()[:,:,None] + end_scores.softmax(dim=1).log()[:,None,:]
ignore_score = span_scores[:,0,0] #no answer scores
```
| [
-0.03852738440036774,
-0.012833156622946262,
-0.05821830406785011,
0.01829357072710991,
0.045406524091959,
-0.015766242519021034,
-0.049227308481931686,
0.12100313603878021,
-0.04791204631328583,
-0.06379124522209167,
0.02770586684346199,
-0.057590898126363754,
0.011363998986780643,
0.05232508108019829,
0.0374700129032135,
0.0040505980141460896,
-0.000007222120530059328,
-0.042246997356414795,
-0.08558723330497742,
0.0001755682606017217,
0.0566791370511055,
0.02793613262474537,
0.07320982962846756,
-0.005897833965718746,
-0.07585974782705307,
-0.060195598751306534,
-0.004572051577270031,
0.0016880277544260025,
-0.034502219408750534,
-0.04586450010538101,
0.013212982565164566,
0.07407817989587784,
-0.003446376882493496,
0.06196177750825882,
0.07595277577638626,
0.10690340399742126,
-0.0141562195494771,
-0.06650324910879135,
-0.04014063626527786,
0.017147257924079895,
0.03348616138100624,
-0.037145283073186874,
0.003985641989856958,
-0.0010805053170770407,
0.04639801010489464,
-0.03509549796581268,
-0.03184550628066063,
-0.017827996984124184,
0.05287801846861839,
-0.05398176610469818,
-0.07913941890001297,
-0.0974029004573822,
-0.00019416243594605476,
0.028061527758836746,
-0.003003225428983569,
0.029256537556648254,
-0.04093579575419426,
-0.04411199316382408,
-0.049387361854314804,
-0.07001090049743652,
-0.04170863330364227,
-0.013152423314750195,
-0.06744777411222458,
-0.01628352701663971,
-0.04292896017432213,
-0.033001113682985306,
-0.016450626775622368,
0.02834238111972809,
0.025824718177318573,
0.03156454116106033,
-0.04752493277192116,
0.024540476500988007,
-0.04317774996161461,
-0.030213557183742523,
-0.047972820699214935,
0.03433744236826897,
0.06857132166624069,
0.014303784817457199,
0.05661627650260925,
-0.15232586860656738,
-0.04049057513475418,
-0.09228958934545517,
0.02779049053788185,
0.010736553929746151,
0.05385540425777435,
0.011457192711532116,
0.07811469584703445,
-0.0024645612575113773,
0.03360382840037346,
0.013370084576308727,
-0.019848773255944252,
-0.019999586045742035,
0.07532966136932373,
0.036776985973119736,
0.025236105546355247,
0.0695880800485611,
0.03479169309139252,
0.0408489890396595,
-0.050368014723062515,
0.10354185849428177,
-0.015732239931821823,
-0.024056127294898033,
0.07246638834476471,
0.0910470187664032,
-0.024030309170484543,
-0.016791867092251778,
0.00968210119754076,
0.05052721127867699,
0.004004190675914288,
-0.05983149632811546,
0.02243851311504841,
0.03328900411725044,
0.017145903781056404,
0.005163491237908602,
0.054500192403793335,
0.07041060924530029,
0.008062108419835567,
0.05655830726027489,
-0.11818896234035492,
0.09498143941164017,
0.038107842206954956,
-0.045154400169849396,
-0.013864122331142426,
0.009314213879406452,
0.04017418995499611,
-0.036468058824539185,
-0.032007522881031036,
1.3057545532567515e-33,
0.09442710131406784,
-0.05580845847725868,
0.0643375888466835,
0.03345843032002449,
0.05736006796360016,
0.06574149429798126,
-0.01628933474421501,
0.08150435984134674,
-0.055896759033203125,
0.08815367519855499,
-0.12327920645475388,
0.008465655148029327,
-0.014864412136375904,
0.05148770660161972,
-0.013121278956532478,
-0.03893230855464935,
-0.06255260109901428,
0.07686885446310043,
0.005391180515289307,
0.07346980273723602,
0.15782193839550018,
0.03310738876461983,
-0.06760723143815994,
-0.015849372372031212,
-0.005986666772514582,
0.017918966710567474,
-0.007581792771816254,
-0.04594434052705765,
0.01837834343314171,
0.04029405117034912,
-0.07946278154850006,
-0.0612700954079628,
-0.076254703104496,
0.008958861231803894,
-0.0448685884475708,
-0.040229979902505875,
-0.04021921381354332,
-0.03276129439473152,
0.007689408492296934,
-0.00278931250795722,
-0.006325636524707079,
0.01661776192486286,
-0.020985571667551994,
-0.11275674402713776,
-0.028801532462239265,
-0.06541161239147186,
-0.014624467119574547,
0.00024409028992522508,
-0.023788893595337868,
0.03928103670477867,
0.008737437427043915,
-0.023876940831542015,
-0.013097620569169521,
-0.06252796202898026,
-0.018797840923070908,
0.013304934836924076,
0.017155198380351067,
0.06689164787530899,
0.025113224983215332,
0.01814238540828228,
0.07688917219638824,
0.019089536741375923,
-0.028270790353417397,
0.03287266939878464,
0.04212311655282974,
0.012120419181883335,
-0.03972260653972626,
-0.046328190714120865,
0.048269666731357574,
0.03450977802276611,
-0.07907695323228836,
-0.0285408403724432,
-0.004767736420035362,
-0.0010271896608173847,
0.10055163502693176,
-0.07671710848808289,
0.010662198998034,
-0.00452245119959116,
-0.057615675032138824,
-0.07621019333600998,
-0.06106298416852951,
0.0634404793381691,
-0.05855594947934151,
-0.04313158243894577,
0.004083266016095877,
-0.009314978495240211,
0.012991562485694885,
-0.047579988837242126,
-0.09705346077680588,
-0.03270336240530014,
-0.06872168928384781,
-0.02534092217683792,
-0.07730960845947266,
-0.00917807687073946,
-0.009172032587230206,
-2.092385270922449e-33,
0.05356530472636223,
-0.007589799351990223,
-0.026848744601011276,
0.019565008580684662,
0.06715402007102966,
-0.019901560619473457,
0.06631386280059814,
0.04321404919028282,
-0.009258564561605453,
-0.02088744193315506,
0.01813570037484169,
-0.005272742360830307,
0.002697567455470562,
-0.026667887344956398,
0.04201463982462883,
0.0017000421648845077,
-0.08216425776481628,
-0.08319403976202011,
-0.004673046059906483,
0.02207004837691784,
-0.035144511610269547,
0.09680350869894028,
-0.046758051961660385,
0.029390519484877586,
-0.03601885586977005,
0.05493231117725372,
0.013258151710033417,
0.07987261563539505,
0.06457990407943726,
0.02557545155286789,
-0.05855975300073624,
0.02737315557897091,
-0.10410375893115997,
0.06936542689800262,
-0.031774118542671204,
0.0767439529299736,
0.012842428870499134,
0.0017657841090112925,
0.010067264549434185,
0.13477806746959686,
0.05138115584850311,
0.03332281857728958,
-0.09850979596376419,
0.016658157110214233,
0.000558210420422256,
-0.028188228607177734,
-0.0016120767686516047,
-0.06941613554954529,
0.01356307789683342,
-0.05743271857500076,
0.016782548278570175,
-0.027582377195358276,
-0.07731295377016068,
0.058381956070661545,
-0.07143617421388626,
-0.08738387376070023,
0.019919930025935173,
-0.04632028937339783,
-0.0359470471739769,
-0.019873425364494324,
0.025844434276223183,
0.014438728801906109,
-0.019552579149603844,
-0.0660589337348938,
-0.07274385541677475,
-0.06219784542918205,
-0.08021900057792664,
0.03270655870437622,
0.05489800497889519,
0.025473732501268387,
-0.016949094831943512,
0.007438453380018473,
0.0993732139468193,
0.029353810474276543,
-0.002278066473081708,
0.04619032144546509,
-0.05904413387179375,
-0.06238970905542374,
0.02857012115418911,
0.020855039358139038,
-0.08989283442497253,
-0.024828573688864708,
0.06864183396100998,
0.09777428209781647,
-0.041970234364271164,
0.07222326844930649,
0.06876222044229507,
0.1505616158246994,
0.0428420789539814,
-0.03388088569045067,
0.04676945134997368,
0.014500029385089874,
0.10576191544532776,
0.14430847764015198,
-0.006514676380902529,
-5.7119013519013606e-8,
0.02024717628955841,
0.021318020299077034,
0.050116077065467834,
0.07797821611166,
-0.03331923112273216,
-0.026987949386239052,
-0.06321859359741211,
0.007370366249233484,
0.06403013318777084,
0.024836214259266853,
0.028586527332663536,
0.014460636302828789,
-0.04812327027320862,
0.0022081476636230946,
0.00976293720304966,
0.05484989285469055,
-0.04918227344751358,
0.05460432544350624,
-0.06795396655797958,
-0.061110347509384155,
-0.015934426337480545,
-0.014847159385681152,
-0.03656558692455292,
-0.01894461363554001,
0.0598551407456398,
-0.054288044571876526,
-0.0669938400387764,
-0.030921384692192078,
-0.10004869848489761,
0.012371030636131763,
0.008153903298079967,
0.029013680294156075,
-0.01690654084086418,
-0.0017593030352145433,
0.007952048443257809,
0.06567342579364777,
0.04868590086698532,
-0.023039646446704865,
0.024930639192461967,
0.02317594364285469,
0.03861679509282112,
0.00006941586616449058,
-0.07191824913024902,
-0.006523482501506805,
0.11049740016460419,
-0.02042047493159771,
0.04040832445025444,
-0.0902586504817009,
-0.00481391791254282,
-0.019364850595593452,
0.01226005982607603,
0.046448562294244766,
-0.07425935566425323,
0.07610805332660675,
-0.009945888072252274,
0.04572351649403572,
-0.06759769469499588,
-0.02622460573911667,
-0.02607891894876957,
0.05051189288496971,
-0.04168092831969261,
-0.025248069316148758,
0.00467647984623909,
-0.025204991921782494
] |
aware-ai/wav2vec2-xls-r-1b-5gram-german | 4bfed40b06b3286744027db0cf211efdfb1c7aa6 | 2022-06-01T13:33:48.000Z | [
"pytorch",
"wav2vec2",
"automatic-speech-recognition",
"de",
"dataset:common_voice",
"transformers",
"audio",
"speech",
"hf-asr-leaderboard",
"license:apache-2.0",
"model-index"
] | automatic-speech-recognition | false | aware-ai | null | aware-ai/wav2vec2-xls-r-1b-5gram-german | 2,127 | 1 | transformers | ---
language: de
datasets:
- common_voice
metrics:
- wer
- cer
tags:
- audio
- automatic-speech-recognition
- speech
- hf-asr-leaderboard
license: apache-2.0
model-index:
- name: wav2vec2-xls-r-1b-5gram-german with LM by Florian Zimmermeister @A\\Ware
results:
- task:
name: Speech Recognition
type: automatic-speech-recognition
dataset:
name: Common Voice de
type: common_voice
args: de
metrics:
- name: Test WER
type: wer
value: 4.382541642219636
- name: Test CER
type: cer
value: 1.6235493024026488
- task:
name: Speech Recognition
type: automatic-speech-recognition
dataset:
name: Common Voice 8 de
type: mozilla-foundation/common_voice_8_0
args: de
metrics:
- name: Test WER
type: wer
value: 4.382541642219636
- name: Test CER
type: cer
value: 1.6235493024026488
---
## Evaluation
The model can be evaluated as follows on the German test data of Common Voice.
```python
import torch
from transformers import AutoModelForCTC, AutoProcessor
from unidecode import unidecode
import re
from datasets import load_dataset, load_metric
import datasets
counter = 0
wer_counter = 0
cer_counter = 0
device = "cuda" if torch.cuda.is_available() else "cpu"
special_chars = [["Ä"," AE "], ["Ö"," OE "], ["Ü"," UE "], ["ä"," ae "], ["ö"," oe "], ["ü"," ue "]]
def clean_text(sentence):
for special in special_chars:
sentence = sentence.replace(special[0], special[1])
sentence = unidecode(sentence)
for special in special_chars:
sentence = sentence.replace(special[1], special[0])
sentence = re.sub("[^a-zA-Z0-9öäüÖÄÜ ,.!?]", " ", sentence)
return sentence
def main(model_id):
print("load model")
model = AutoModelForCTC.from_pretrained(model_id).to(device)
print("load processor")
processor = AutoProcessor.from_pretrained(processor_id)
print("load metrics")
wer = load_metric("wer")
cer = load_metric("cer")
ds = load_dataset("mozilla-foundation/common_voice_8_0","de")
ds = ds["test"]
ds = ds.cast_column(
"audio", datasets.features.Audio(sampling_rate=16_000)
)
def calculate_metrics(batch):
global counter, wer_counter, cer_counter
resampled_audio = batch["audio"]["array"]
input_values = processor(resampled_audio, return_tensors="pt", sampling_rate=16_000).input_values
with torch.no_grad():
logits = model(input_values.to(device)).logits.cpu().numpy()[0]
decoded = processor.decode(logits)
pred = decoded.text.lower()
ref = clean_text(batch["sentence"]).lower()
wer_result = wer.compute(predictions=[pred], references=[ref])
cer_result = cer.compute(predictions=[pred], references=[ref])
counter += 1
wer_counter += wer_result
cer_counter += cer_result
if counter % 100 == True:
print(f"WER: {(wer_counter/counter)*100} | CER: {(cer_counter/counter)*100}")
return batch
ds.map(calculate_metrics, remove_columns=ds.column_names)
print(f"WER: {(wer_counter/counter)*100} | CER: {(cer_counter/counter)*100}")
model_id = "flozi00/wav2vec2-xls-r-1b-5gram-german"
main(model_id)
``` | [
-0.06033414974808693,
-0.07789084315299988,
-0.015358850359916687,
-0.09364037215709686,
-0.01063525676727295,
-0.0013783758040517569,
-0.020906507968902588,
-0.017267417162656784,
-0.01630781777203083,
-0.07726844400167465,
0.03709043562412262,
-0.1764192134141922,
-0.041393641382455826,
-0.013449118472635746,
-0.014339607208967209,
-0.05838965252041817,
-0.027245672419667244,
-0.08300626277923584,
-0.022534212097525597,
-0.06838708370923996,
0.007970456965267658,
0.12284433096647263,
0.02058033086359501,
-0.018410595133900642,
0.06460974365472794,
0.003334958804771304,
-0.06980296224355698,
0.025915799662470818,
-0.009958423674106598,
-0.04957756772637367,
0.14230121672153473,
0.06380414962768555,
0.12155414372682571,
0.034387487918138504,
0.041311901062726974,
-0.001732839155010879,
0.015185325406491756,
-0.05746595934033394,
-0.030209051445126534,
-0.037675969302654266,
-0.038317978382110596,
0.013535118661820889,
0.015829773619771004,
-0.06130477041006088,
-0.031089115887880325,
-0.03987155482172966,
-0.11621446162462234,
0.00024992250837385654,
-0.02419346012175083,
0.045532725751399994,
-0.055858954787254333,
0.014785255305469036,
0.042508091777563095,
0.05901310592889786,
-0.07222352176904678,
0.011061606928706169,
-0.0006881604203954339,
0.07885410636663437,
0.05458185449242592,
0.011133749969303608,
-0.056046415120363235,
-0.028501540422439575,
-0.036371130496263504,
0.007601223420351744,
-0.06598042696714401,
-0.02240941673517227,
-0.006482305470854044,
-0.05906521528959274,
-0.022894836962223053,
0.03168223798274994,
-0.09917153418064117,
0.052853669971227646,
0.008645775727927685,
0.06853063404560089,
0.046585772186517715,
-0.018107835203409195,
0.003086486365646124,
-0.0463079959154129,
0.07313434034585953,
-0.09033636748790741,
-0.023178236559033394,
-0.046606022864580154,
-0.0030871666967868805,
0.004705739673227072,
0.08160080760717392,
-0.007731888443231583,
0.0025711229536682367,
-0.042265333235263824,
-0.018709903582930565,
-0.05300774797797203,
-0.08083610981702805,
-0.05221181362867355,
-0.061569467186927795,
0.1056680902838707,
0.0027052592486143112,
0.07792773097753525,
0.08982755988836288,
0.11313264071941376,
0.034283168613910675,
0.06113863363862038,
-0.013809886761009693,
-0.05809324234724045,
0.006279794033616781,
-0.0025723937433212996,
-0.029082298278808594,
-0.03538516163825989,
0.024661367759108543,
0.04225054755806923,
0.03815164789557457,
-0.0722137838602066,
0.006278474349528551,
-0.01435956172645092,
-0.024321023374795914,
-0.0516250841319561,
0.03703363984823227,
-0.0029333392158150673,
-0.04063393920660019,
-0.049944665282964706,
0.01851760782301426,
0.020947549492120743,
-0.0336742140352726,
-0.00634723948314786,
-0.04181254282593727,
-0.00962886307388544,
0.02463875524699688,
0.03300437331199646,
-0.0022250309120863676,
1.14223256267142e-32,
0.016850970685482025,
-0.010853404179215431,
0.0249025821685791,
0.009370814077556133,
0.004459977615624666,
-0.05791642144322395,
-0.06043826416134834,
0.07773049175739288,
-0.02843690663576126,
-0.002466680249199271,
-0.03634455427527428,
-0.0310964398086071,
-0.08502647280693054,
0.024429650977253914,
0.03499086946249008,
0.050667162984609604,
0.02853410691022873,
-0.01309621799737215,
-0.05165249481797218,
-0.018321115523576736,
0.14057618379592896,
-0.001679514884017408,
0.04453137516975403,
-0.020429575815796852,
0.08714711666107178,
0.07032204419374466,
0.07031421363353729,
-0.06684672832489014,
0.0023178004194051027,
0.02570641040802002,
-0.04106983542442322,
-0.05539052560925484,
0.0037810583598911762,
-0.032588113099336624,
0.05865878239274025,
0.026535945013165474,
0.03799651935696602,
0.07254400849342346,
-0.07380062341690063,
-0.09183349460363388,
0.06164786219596863,
0.025102101266384125,
-0.022737501189112663,
-0.03434276953339577,
-0.0024190943222492933,
-0.06766142696142197,
-0.04134245961904526,
-0.0036205437500029802,
0.03974694758653641,
0.05559660121798515,
-0.06732095777988434,
0.005170547403395176,
-0.04766067862510681,
0.022943181917071342,
0.003898223862051964,
0.0037589254789054394,
0.06934180855751038,
0.05851590633392334,
-0.00750320591032505,
-0.00964419450610876,
-0.01843259111046791,
0.02136194333434105,
-0.005418168846517801,
-0.02088771015405655,
0.11293289810419083,
-0.07863562554121017,
-0.016983339563012123,
-0.012492690235376358,
0.014630720019340515,
0.02038636803627014,
-0.022512884810566902,
-0.059658825397491455,
0.11447324603796005,
0.07463444024324417,
0.008946763351559639,
0.018400713801383972,
-0.004363234154880047,
-0.025473112240433693,
0.00539236469194293,
0.030946878716349602,
-0.03860863298177719,
0.03143468126654625,
-0.02437814511358738,
-0.06335728615522385,
-0.007382705807685852,
-0.027499478310346603,
0.019332675263285637,
-0.07086677104234695,
-0.014083856716752052,
0.0286048986017704,
-0.04602369666099548,
0.08402291685342789,
-0.09462092071771622,
-0.025765487924218178,
-0.10029018670320511,
-1.3484170636047325e-32,
-0.048428475856781006,
0.11480488628149033,
-0.0010496992617845535,
0.09512361139059067,
0.024971311911940575,
0.0028850403614342213,
0.11460523307323456,
0.07852523028850555,
0.045438192784786224,
0.005219311453402042,
0.04656336084008217,
-0.0734761506319046,
0.09762690216302872,
-0.03057830221951008,
0.08509672433137894,
0.044403575360774994,
-0.04525664076209068,
0.004855838138610125,
0.02841239422559738,
0.07826364040374756,
0.007379031740128994,
0.10781903564929962,
-0.017878757789731026,
0.05837889760732651,
-0.08990616351366043,
-0.043020524084568024,
-0.06977040320634842,
0.020352615043520927,
0.018068784847855568,
-0.03998927026987076,
-0.028801484033465385,
0.029467467218637466,
-0.12377332150936127,
0.03852519392967224,
-0.007836987264454365,
-0.04593149572610855,
0.0055852425284683704,
0.004779960494488478,
-0.005260069854557514,
0.049692943692207336,
0.06812889873981476,
0.049050092697143555,
-0.09357918053865433,
-0.07691050320863724,
0.06015745550394058,
-0.06934254616498947,
0.01194214727729559,
0.0006345988367684186,
-0.022454453632235527,
-0.04436756670475006,
0.06042255461215973,
-0.012980717234313488,
0.003901675809174776,
0.028714260086417198,
-0.02172483503818512,
-0.012459367513656616,
-0.00039928738260641694,
-0.05323118343949318,
-0.05046061798930168,
-0.0075773983262479305,
0.028031503781676292,
0.0013285479508340359,
-0.08861379325389862,
-0.05202966183423996,
0.0590539425611496,
0.039687760174274445,
-0.05292829871177673,
0.006127428729087114,
0.031560640782117844,
-0.02956654131412506,
-0.021448634564876556,
-0.024589942768216133,
-0.00011768485273933038,
-0.02920965477824211,
-0.034722667187452316,
-0.03486350551247597,
-0.14675842225551605,
-0.04835300147533417,
-0.02442268095910549,
-0.043263211846351624,
0.00425291433930397,
0.028119059279561043,
0.08440224081277847,
0.023464618250727654,
0.043041642755270004,
0.06874028593301773,
-0.027979305014014244,
0.022109033539891243,
0.0008618419524282217,
0.011011551134288311,
-0.02691693603992462,
0.059263914823532104,
-0.03715645894408226,
0.06766918301582336,
0.011024052277207375,
-6.349542758243842e-8,
-0.06792864203453064,
-0.002959988545626402,
-0.0355207733809948,
-0.047051429748535156,
0.015765825286507607,
-0.11650709062814713,
-0.035151880234479904,
-0.008494400419294834,
0.01266663707792759,
-0.039210401475429535,
0.027379034087061882,
-0.029003525152802467,
-0.09855218976736069,
0.05305548757314682,
0.010254761204123497,
-0.04560087248682976,
-0.06076988950371742,
0.1273432821035385,
-0.03572867438197136,
-0.0992429256439209,
0.06764746457338333,
0.012211850844323635,
0.027701634913682938,
0.011601568199694157,
0.01859854906797409,
-0.02796793356537819,
0.00938748475164175,
0.07213275134563446,
0.004643611144274473,
-0.018987705931067467,
-0.05132841318845749,
0.08006227016448975,
-0.02630241960287094,
-0.07794199138879776,
0.07031639665365219,
0.024663036689162254,
-0.04338639974594116,
0.015860607847571373,
-0.021443987265229225,
0.06039798632264137,
0.029196709394454956,
0.09119307994842529,
-0.10129949450492859,
0.0284566693007946,
0.04582881182432175,
-0.023978395387530327,
-0.02247699163854122,
-0.055645618587732315,
0.06185358762741089,
-0.026471508666872978,
0.024938063696026802,
-0.0038874123711138964,
-0.006697623524814844,
-0.003547206288203597,
0.025614086538553238,
0.03866396099328995,
-0.007666279096156359,
0.012108889408409595,
0.020009344443678856,
-0.03932805359363556,
0.08145353943109512,
-0.033222347497940063,
-0.03662586212158203,
-0.024279965087771416
] |
readerbench/RoBERT-large | 2677d2cc3bc009380161e71eda03515abfb5feb4 | 2021-05-20T04:07:47.000Z | [
"pytorch",
"tf",
"jax",
"bert",
"ro",
"transformers"
] | null | false | readerbench | null | readerbench/RoBERT-large | 2,125 | null | transformers | Model card for RoBERT-large
---
language:
- ro
---
# RoBERT-large
## Pretrained BERT model for Romanian
Pretrained model on Romanian language using a masked language modeling (MLM) and next sentence prediction (NSP) objective.
It was introduced in this [paper](https://www.aclweb.org/anthology/2020.coling-main.581/). Three BERT models were released: RoBERT-small, RoBERT-base and **RoBERT-large**, all versions uncased.
| Model | Weights | L | H | A | MLM accuracy | NSP accuracy |
|----------------|:---------:|:------:|:------:|:------:|:--------------:|:--------------:|
| RoBERT-small | 19M | 12 | 256 | 8 | 0.5363 | 0.9687 |
| RoBERT-base | 114M | 12 | 768 | 12 | 0.6511 | 0.9802 |
| *RoBERT-large* | *341M* | *24* | *1024* | *24* | *0.6929* | *0.9843* |
All models are available:
* [RoBERT-small](https://huggingface.co/readerbench/RoBERT-small)
* [RoBERT-base](https://huggingface.co/readerbench/RoBERT-base)
* [RoBERT-large](https://huggingface.co/readerbench/RoBERT-large)
#### How to use
```python
# tensorflow
from transformers import AutoModel, AutoTokenizer, TFAutoModel
tokenizer = AutoTokenizer.from_pretrained("readerbench/RoBERT-large")
model = TFAutoModel.from_pretrained("readerbench/RoBERT-large")
inputs = tokenizer("exemplu de propoziție", return_tensors="tf")
outputs = model(inputs)
# pytorch
from transformers import AutoModel, AutoTokenizer, AutoModel
tokenizer = AutoTokenizer.from_pretrained("readerbench/RoBERT-large")
model = AutoModel.from_pretrained("readerbench/RoBERT-large")
inputs = tokenizer("exemplu de propoziție", return_tensors="pt")
outputs = model(**inputs)
```
## Training data
The model is trained on the following compilation of corpora. Note that we present the statistics after the cleaning process.
| Corpus | Words | Sentences | Size (GB)|
|-----------|:---------:|:---------:|:--------:|
| Oscar | 1.78B | 87M | 10.8 |
| RoTex | 240M | 14M | 1.5 |
| RoWiki | 50M | 2M | 0.3 |
| **Total** | **2.07B** | **103M** | **12.6** |
## Downstream performance
### Sentiment analysis
We report Macro-averaged F1 score (in %)
| Model | Dev | Test |
|------------------|:--------:|:--------:|
| multilingual-BERT| 68.96 | 69.57 |
| XLM-R-base | 71.26 | 71.71 |
| BERT-base-ro | 70.49 | 71.02 |
| RoBERT-small | 66.32 | 66.37 |
| RoBERT-base | 70.89 | 71.61 |
| *RoBERT-large* | **72.48**| **72.11**|
### Moldavian vs. Romanian Dialect and Cross-dialect Topic identification
We report results on [VarDial 2019](https://sites.google.com/view/vardial2019/campaign) Moldavian vs. Romanian Cross-dialect Topic identification Challenge, as Macro-averaged F1 score (in %).
| Model | Dialect Classification | MD to RO | RO to MD |
|-------------------|:----------------------:|:--------:|:--------:|
| 2-CNN + SVM | 93.40 | 65.09 | 75.21 |
| Char+Word SVM | 96.20 | 69.08 | 81.93 |
| BiGRU | 93.30 | **70.10**| 80.30 |
| multilingual-BERT | 95.34 | 68.76 | 78.24 |
| XLM-R-base | 96.28 | 69.93 | 82.28 |
| BERT-base-ro | 96.20 | 69.93 | 78.79 |
| RoBERT-small | 95.67 | 69.01 | 80.40 |
| RoBERT-base | 97.39 | 68.30 | 81.09 |
| *RoBERT-large* | **97.78** | *69.91* | **83.65**|
### Diacritics Restoration
Challenge can be found [here](https://diacritics-challenge.speed.pub.ro/). We report results on the official test set, as accuracies in %.
| Model | word level | char level |
|-----------------------------|:----------:|:----------:|
| BiLSTM | 99.42 | - |
| CharCNN | 98.40 | 99.65 |
| CharCNN + multilingual-BERT | 99.72 | 99.94 |
| CharCNN + XLM-R-base | 99.76 | **99.95** |
| CharCNN + BERT-base-ro | **99.79** | **99.95** |
| CharCNN + RoBERT-small | 99.73 | 99.94 |
| CharCNN + RoBERT-base | 99.78 | **99.95** |
| *CharCNN + RoBERT-large* | *99.76* | **99.95** |
### BibTeX entry and citation info
```bibtex
@inproceedings{masala2020robert,
title={RoBERT--A Romanian BERT Model},
author={Masala, Mihai and Ruseti, Stefan and Dascalu, Mihai},
booktitle={Proceedings of the 28th International Conference on Computational Linguistics},
pages={6626--6637},
year={2020}
}
```
| [
-0.06179095432162285,
-0.06221776455640793,
-0.01094813458621502,
0.03353476524353027,
-0.01905711740255356,
0.1390560120344162,
-0.041893042623996735,
0.04355796426534653,
0.01320213358849287,
-0.022508300840854645,
-0.006025333888828754,
-0.0714028999209404,
-0.0008968995534814894,
0.037207599729299545,
-0.05912485718727112,
0.01393017079681158,
0.05360272899270058,
0.017394617199897766,
-0.058811090886592865,
-0.0759880542755127,
0.06016137823462486,
0.07955130934715271,
0.0156317837536335,
-0.03735547140240669,
0.018994569778442383,
-0.030405445024371147,
-0.0665738582611084,
-0.10043008625507355,
0.07064306735992432,
0.03448254242539406,
-0.0027339530643075705,
-0.02551327459514141,
0.08653666824102402,
0.09352360665798187,
-0.0483834370970726,
-0.04318511113524437,
-0.06422889977693558,
0.032144807279109955,
0.011811870150268078,
-0.011722560971975327,
-0.06886514276266098,
-0.004044665489345789,
-0.03486185520887375,
0.015112608671188354,
0.11219368875026703,
-0.05409667268395424,
-0.049213506281375885,
0.04485459253191948,
-0.07262292504310608,
0.005380390211939812,
-0.09481389075517654,
-0.024722756817936897,
0.031006205826997757,
0.03063059225678444,
-0.06612393260002136,
-0.033529166132211685,
-0.022621456533670425,
-0.032787639647722244,
-0.004814290441572666,
-0.03693637251853943,
-0.06696119904518127,
-0.010494668036699295,
-0.09208331257104874,
0.02315836027264595,
-0.02386074885725975,
0.023086920380592346,
-0.08955138176679611,
0.01324448361992836,
-0.032140325754880905,
0.05165102705359459,
-0.001708613708615303,
-0.001278931275010109,
0.008998829871416092,
0.01515865232795477,
-0.018234295770525932,
-0.02323116362094879,
0.10453271865844727,
-0.005010821390897036,
0.024387212470173836,
-0.02189316414296627,
0.03081141971051693,
-0.017472120001912117,
0.052276697009801865,
-0.03245506435632706,
0.081447072327137,
0.007090349681675434,
0.03877534344792366,
-0.028822269290685654,
-0.04808054119348526,
-0.0338425487279892,
-0.012729395180940628,
-0.11568049341440201,
0.028245681896805763,
0.014435365796089172,
0.0032076502684503794,
0.02820412628352642,
0.007832009345293045,
-0.019144779071211815,
-0.06254512816667557,
0.0955992043018341,
0.01901761069893837,
0.04476071894168854,
0.004576170351356268,
-0.05843508243560791,
0.007225853390991688,
0.012126547284424305,
0.062155432999134064,
-0.02094632014632225,
0.047638699412345886,
-0.060955699533224106,
0.050588663667440414,
0.0009722675895318389,
-0.02374972216784954,
-0.04822962358593941,
0.0624319426715374,
-0.0026052952744066715,
0.012300679460167885,
-0.024265721440315247,
0.006877006031572819,
0.12223509699106216,
-0.04571572318673134,
0.016818642616271973,
0.03396563604474068,
0.04549460858106613,
-0.05575776845216751,
-0.016317902132868767,
0.042943548411130905,
-3.218401044137711e-33,
-0.03904679790139198,
-0.01712862402200699,
-0.023232905194163322,
-0.04431227594614029,
-0.014863218180835247,
-0.014875506050884724,
0.04858127236366272,
-0.001319601316936314,
-0.007473750039935112,
-0.0159937534481287,
-0.028662804514169693,
-0.04972658306360245,
-0.08490883558988571,
0.0950252041220665,
-0.03970559686422348,
0.036648306995630264,
0.012049161829054356,
0.02191721275448799,
-0.00799756869673729,
-0.0040793269872665405,
0.05884367600083351,
0.0875263437628746,
0.02556321583688259,
-0.0798095315694809,
0.05148027092218399,
0.06488008052110672,
0.06202273443341255,
-0.137164905667305,
-0.018061039969325066,
0.034407489001750946,
-0.1122351586818695,
0.044645290821790695,
-0.05306423455476761,
-0.007834970951080322,
-0.01981712132692337,
-0.01184995286166668,
0.002427708823233843,
-0.06929516792297363,
0.003636944340541959,
-0.03779256343841553,
0.016195032745599747,
0.030689075589179993,
0.029229240491986275,
-0.07619073241949081,
-0.05669194087386131,
-0.027114590629935265,
-0.01562810316681862,
0.006276722997426987,
-0.010291416198015213,
-0.013273855671286583,
0.05928317829966545,
0.01215451117604971,
-0.14245304465293884,
0.02031216211616993,
0.026208363473415375,
-0.03778031840920448,
0.026077184826135635,
0.03303245082497597,
0.08101744204759598,
0.020910369232296944,
0.017164014279842377,
-0.03488844633102417,
0.016121208667755127,
0.047720763832330704,
0.00870935432612896,
-0.046052735298871994,
-0.07891026139259338,
-0.03625154495239258,
0.041687168180942535,
0.013577647507190704,
-0.03823772817850113,
-0.022107640281319618,
0.036624133586883545,
0.05407366901636124,
-0.01106531172990799,
0.014035899192094803,
0.025943197309970856,
-0.04508782550692558,
-0.010459898971021175,
0.047253094613552094,
-0.017090553417801857,
0.01974492147564888,
-0.014242615550756454,
-0.07840494811534882,
-0.07181106507778168,
-0.02851984091103077,
0.08052115887403488,
-0.030234094709157944,
-0.002669601235538721,
0.0029269095975905657,
0.018074525520205498,
0.005218732636421919,
0.04729023575782776,
0.02721436135470867,
-0.0324195921421051,
-1.486387260017843e-33,
-0.027555711567401886,
0.07568056881427765,
-0.039439938962459564,
0.031662747263908386,
-0.030140213668346405,
-0.09933499991893768,
-0.01498393528163433,
0.11277492344379425,
-0.011209211312234402,
-0.006130308378487825,
-0.005627525504678488,
-0.0699298232793808,
0.048940107226371765,
-0.010660527274012566,
0.11514800041913986,
-0.013373317196965218,
0.025690371170639992,
0.013148840516805649,
0.04188993200659752,
0.03274358808994293,
0.056950416415929794,
0.028620369732379913,
-0.124695785343647,
0.06310166418552399,
-0.016651371493935585,
-0.006462567485868931,
-0.0177149698138237,
0.040229201316833496,
-0.07950390875339508,
-0.013737635686993599,
-0.059999629855155945,
0.0046621947549283504,
-0.03670012205839157,
0.04324774071574211,
-0.08929548412561417,
0.10536175221204758,
0.0042665922082960606,
-0.010819588787853718,
-0.02809726446866989,
0.10854407399892807,
0.0708143562078476,
0.0014584858436137438,
-0.0876140221953392,
0.015114196576178074,
-0.020880773663520813,
-0.010869242250919342,
-0.07736928761005402,
-0.05616005137562752,
0.08479244261980057,
-0.10308364778757095,
0.005990954581648111,
-0.0053737410344183445,
-0.06241414695978165,
-0.037248533219099045,
-0.08388780057430267,
-0.039100226014852524,
0.007199496030807495,
-0.09663694351911545,
-0.008256361819803715,
-0.0007849510293453932,
-0.042589183896780014,
-0.025168348103761673,
0.0654197707772255,
-0.10198815912008286,
0.038394007831811905,
-0.02444619871675968,
-0.030738389119505882,
0.11499647051095963,
0.0059278132393956184,
-0.03181334212422371,
-0.02139594592154026,
-0.02754770591855049,
0.04814211279153824,
0.10634713619947433,
-0.04262440279126167,
-0.002564852125942707,
0.033624086529016495,
-0.05596790462732315,
0.005125907715409994,
-0.08242100477218628,
-0.08418550342321396,
-0.06227680668234825,
0.04870020970702171,
0.06994375586509705,
0.037788812071084976,
0.08616286516189575,
0.05638287588953972,
-0.03609505668282509,
-0.016191700473427773,
0.07403495162725449,
-0.03552085533738136,
0.05130722373723984,
0.07404235005378723,
0.004725569859147072,
-0.02247561700642109,
-5.290647564493156e-8,
-0.04670465365052223,
-0.02003532275557518,
-0.031631942838430405,
0.06393515318632126,
-0.03242321312427521,
-0.05893160030245781,
-0.057412777096033096,
-0.0649784728884697,
-0.07436561584472656,
-0.036188285797834396,
0.02356937900185585,
0.042203910648822784,
-0.054362814873456955,
-0.008840157650411129,
-0.01679905876517296,
0.043182291090488434,
-0.025877567008137703,
0.016432922333478928,
0.025222864001989365,
-0.07877115905284882,
0.07681334763765335,
0.04979710653424263,
0.029336590319871902,
-0.0029573668725788593,
-0.009995324537158012,
-0.04196980968117714,
-0.029751375317573547,
0.11486618220806122,
-0.018145481124520302,
0.07229488343000412,
0.0013223731657490134,
0.06926654279232025,
-0.0525677427649498,
0.027199728414416313,
0.08953705430030823,
0.09123186767101288,
0.011637210845947266,
-0.04743950068950653,
-0.018534163013100624,
0.027401208877563477,
0.07659629732370377,
0.000425651523983106,
-0.06236489117145538,
0.0006954986019991338,
0.08104957640171051,
-0.004909939598292112,
-0.004631855990737677,
-0.19653907418251038,
0.00789815653115511,
-0.05902252346277237,
0.03715413808822632,
-0.026409320533275604,
-0.01762033812701702,
0.0969930961728096,
0.0725056454539299,
0.01559508964419365,
-0.03847290948033333,
-0.03078259900212288,
-0.0011418898357078433,
-0.04662153497338295,
0.00958932377398014,
0.05287711322307587,
-0.012550212442874908,
0.07041394710540771
] |
IDEA-CCNL/Erlangshen-Roberta-110M-Sentiment | 21ff55d0bd2f7904d2a5380165ac2fd6d0d74b81 | 2022-05-27T07:59:44.000Z | [
"pytorch",
"bert",
"text-classification",
"zh",
"transformers",
"NLU",
"Sentiment",
"Chinese",
"license:apache-2.0"
] | text-classification | false | IDEA-CCNL | null | IDEA-CCNL/Erlangshen-Roberta-110M-Sentiment | 2,124 | 4 | transformers | ---
language:
- zh
license: apache-2.0
tags:
- bert
- NLU
- Sentiment
- Chinese
inference: true
widget:
- text: "今天心情不好"
---
# Erlangshen-Roberta-110M-Semtiment, model (Chinese),one model of [Fengshenbang-LM](https://github.com/IDEA-CCNL/Fengshenbang-LM).
We collect 8 sentiment datasets in the Chinese domain for finetune, with a total of 227347 samples. Our model is mainly based on [roberta](https://huggingface.co/hfl/chinese-roberta-wwm-ext)
## Usage
```python
from transformers import BertForSequenceClassification
from transformers import BertTokenizer
import torch
tokenizer=BertTokenizer.from_pretrained('IDEA-CCNL/Erlangshen-Roberta-110M-Sentiment')
model=BertForSequenceClassification.from_pretrained('IDEA-CCNL/Erlangshen-Roberta-110M-Sentiment')
text='今天心情不好'
output=model(torch.tensor([tokenizer.encode(text)]))
print(torch.nn.functional.softmax(output.logits,dim=-1))
```
## Scores on downstream chinese tasks
| Model | ASAP-SENT | ASAP-ASPECT | ChnSentiCorp |
| :--------: | :-----: | :----: | :-----: |
| Erlangshen-Roberta-110M-Sentiment | 97.77 | 97.31 | 96.61 |
| Erlangshen-Roberta-330M-Sentiment | 97.9 | 97.51 | 96.66 |
| Erlangshen-MegatronBert-1.3B-Sentiment | 98.1 | 97.8 | 97 |
## Citation
If you find the resource is useful, please cite the following website in your paper.
```
@misc{Fengshenbang-LM,
title={Fengshenbang-LM},
author={IDEA-CCNL},
year={2021},
howpublished={\url{https://github.com/IDEA-CCNL/Fengshenbang-LM}},
}
``` | [
-0.08709655702114105,
-0.0722990334033966,
0.07701954245567322,
0.041991714388132095,
0.03149540349841118,
0.03384114429354668,
0.025520043447613716,
0.0040841554291546345,
0.04542120173573494,
-0.02865923009812832,
0.03528405725955963,
-0.09324324876070023,
0.013453560881316662,
-0.012478427961468697,
0.05564378947019577,
0.07974134385585785,
0.012300804257392883,
-0.04634853079915047,
-0.15248017013072968,
-0.0949406772851944,
0.03764059394598007,
0.050695568323135376,
0.055521782487630844,
-0.007598993368446827,
0.015838004648685455,
-0.05443115532398224,
-0.01298938412219286,
0.018508898094296455,
0.09384230524301529,
0.005115598440170288,
0.0196062158793211,
0.14168226718902588,
-0.03356187790632248,
0.08738093078136444,
0.026232177391648293,
0.07818195968866348,
-0.06648901849985123,
-0.04045693948864937,
0.02811119332909584,
0.048198916018009186,
0.016869941726326942,
0.036569975316524506,
0.017824651673436165,
-0.06320077180862427,
0.0742897167801857,
-0.016086051240563393,
-0.0018130082171410322,
-0.052397359162569046,
-0.006876520812511444,
-0.022518333047628403,
-0.053778935223817825,
0.04323159530758858,
-0.01413756888359785,
0.0864938348531723,
-0.05500520393252373,
-0.05680961161851883,
0.011803319677710533,
-0.02932504378259182,
0.03207942470908165,
-0.05921201407909393,
-0.09671512246131897,
-0.023452920839190483,
-0.013243473134934902,
-0.04714931547641754,
-0.07400970906019211,
-0.02669498138129711,
-0.08967149257659912,
0.06003411114215851,
0.04118582233786583,
0.03089805319905281,
0.0004255702951923013,
0.0386984720826149,
-0.02780815213918686,
0.07744553685188293,
-0.004905744455754757,
-0.019787821918725967,
0.07766765356063843,
-0.03031807951629162,
0.04033433273434639,
-0.1551591008901596,
-0.026260126382112503,
-0.03261682763695717,
0.11006978154182434,
0.02303212881088257,
0.10485435277223587,
-0.044590264558792114,
0.008788722567260265,
0.052986208349466324,
-0.02578381821513176,
0.027775876224040985,
-0.01571471430361271,
-0.042463310062885284,
0.0676548033952713,
0.04018223285675049,
0.010300783440470695,
0.0895296186208725,
0.022795358672738075,
-0.028614407405257225,
-0.039811860769987106,
0.12770593166351318,
0.026664014905691147,
0.10081527382135391,
0.03567621111869812,
-0.06707307696342468,
-0.026821419596672058,
-0.023556308820843697,
0.014207292348146439,
0.06499475240707397,
0.020765135064721107,
-0.10020697861909866,
0.004790873266756535,
0.016073904931545258,
0.0023505371063947678,
-0.025822512805461884,
0.053941380232572556,
-0.03149028867483139,
0.01054401509463787,
0.004310715477913618,
-0.041538506746292114,
0.057785436511039734,
-0.002352913608774543,
0.03218885883688927,
-0.0024672949220985174,
0.01528833992779255,
-0.03382920101284981,
0.001681973342783749,
-0.035493403673172,
5.4331591340715986e-33,
0.060234300792217255,
0.05887254327535629,
0.027698349207639694,
0.001829526387155056,
-0.03466029465198517,
0.00588569650426507,
0.005902073346078396,
0.0318867564201355,
-0.09349159151315689,
-0.003283114405348897,
-0.06388513743877411,
0.027886684983968735,
-0.08120609819889069,
0.04499848186969757,
-0.010710093192756176,
-0.01752765104174614,
-0.05355601757764816,
-0.004259494598954916,
0.025053732097148895,
0.015683535486459732,
0.07487459480762482,
0.035212986171245575,
-0.02018670178949833,
-0.10632380843162537,
-0.08987262845039368,
0.030252542346715927,
0.09252644330263138,
-0.026631509885191917,
-0.045670006424188614,
0.02403958886861801,
-0.08100535720586777,
0.0692591518163681,
0.023736268281936646,
0.022755905985832214,
0.003943201620131731,
-0.023332076147198677,
0.02766716480255127,
-0.008063621819019318,
0.014072118327021599,
-0.07984081655740738,
0.013011584989726543,
0.06723234802484512,
-0.029061315581202507,
-0.029430633410811424,
-0.03352788835763931,
0.023261399939656258,
-0.014403657987713814,
-0.05618448927998543,
0.07940855622291565,
0.04788776487112045,
0.014839738607406616,
0.018748769536614418,
-0.005831862334161997,
0.06187065690755844,
-0.05199002847075462,
-0.02139476127922535,
0.044613540172576904,
-0.01301787793636322,
0.09079091995954514,
-0.014922942966222763,
0.0030018133111298084,
-0.03644498810172081,
0.0035326185170561075,
-0.010371629148721695,
0.08005746454000473,
0.021205145865678787,
-0.02378387376666069,
0.01831744611263275,
-0.026689069345593452,
-0.006636634003371,
-0.0317823551595211,
-0.0357397124171257,
0.0052692280150949955,
-0.040322814136743546,
0.02311897464096546,
-0.07759358733892441,
-0.01629401370882988,
-0.07493416219949722,
-0.042160484939813614,
0.03435703366994858,
-0.013660157099366188,
-0.01934286765754223,
-0.02864779904484749,
-0.01882382109761238,
-0.043684687465429306,
-0.014822149649262428,
0.05677805840969086,
-0.056548718363046646,
-0.026840588077902794,
-0.010563547722995281,
-0.009539785794913769,
-0.01803002320230007,
0.01782285049557686,
-0.014916900545358658,
-0.08297692984342575,
-5.198322546084168e-33,
-0.036993518471717834,
0.054300498217344284,
-0.06355956941843033,
0.06230736896395683,
0.012893631123006344,
-0.10957425087690353,
0.04250045493245125,
0.11724637448787689,
-0.03851621225476265,
-0.02547513321042061,
0.07937134057283401,
-0.08576592803001404,
0.01230724435299635,
-0.003022683784365654,
0.04712042212486267,
0.028528792783617973,
0.0170523002743721,
0.07345625013113022,
0.01955166645348072,
0.056013379245996475,
-0.03601839393377304,
0.027108553797006607,
-0.14398330450057983,
0.07755143940448761,
-0.03770091012120247,
0.11421110481023788,
-0.02199467271566391,
0.00657745823264122,
0.029043104499578476,
-0.01573438197374344,
-0.06091455742716789,
0.009062341414391994,
-0.0748249962925911,
0.039179477840662,
-0.10632787644863129,
-0.01731591671705246,
-0.03998640552163124,
-0.04776474088430405,
-0.04335327446460724,
0.06734654307365417,
0.07409562915563583,
-0.009857839904725552,
-0.06356562674045563,
0.045437704771757126,
-0.025920558720827103,
-0.019214866682887077,
-0.06646479666233063,
-0.04102574288845062,
0.020267199724912643,
-0.005478565115481615,
0.010510454885661602,
0.014821049757301807,
-0.06536663323640823,
0.04418748989701271,
-0.02977500483393669,
-0.07789453119039536,
0.03377482295036316,
-0.0860808789730072,
-0.04743761569261551,
-0.04738900065422058,
-0.06565798819065094,
0.025761129334568977,
-0.009188272058963776,
-0.03962419927120209,
0.012068229727447033,
-0.011001314967870712,
0.03944242000579834,
0.0582684651017189,
0.014505993574857712,
-0.047167107462882996,
0.05001578852534294,
0.07860090583562851,
0.016416067257523537,
-0.031885311007499695,
-0.0033380857203155756,
0.02159205451607704,
-0.021304255351424217,
-0.020847873762249947,
0.007687569595873356,
-0.0490771047770977,
-0.07257981598377228,
-0.017260806635022163,
0.03335781767964363,
-0.018792981281876564,
-0.04535520821809769,
-0.015161515213549137,
0.008136213757097721,
0.07933314889669418,
0.008176441304385662,
0.01817847415804863,
0.0001360794121865183,
-0.011907591484487057,
0.037415143102407455,
0.08838909864425659,
0.049269188195466995,
-5.147917647718714e-8,
-0.11537602543830872,
-0.043757256120443344,
-0.06019112095236778,
0.07028158009052277,
-0.08378533273935318,
-0.02506924420595169,
-0.022388532757759094,
0.08804191648960114,
-0.01322236843407154,
-0.016459405422210693,
0.0787673071026802,
0.04197169095277786,
-0.1390567123889923,
0.027984915301203728,
-0.0189979150891304,
0.01040048711001873,
-0.014689026400446892,
0.09302748739719391,
0.0013698493130505085,
-0.031146340072155,
0.02748328447341919,
0.05026846379041672,
0.059917278587818146,
-0.06348513066768646,
0.029399700462818146,
-0.011244229972362518,
-0.06551595777273178,
0.08171786367893219,
-0.04113375395536423,
-0.026441683992743492,
-0.0008249770035035908,
-0.025780795142054558,
-0.08909159153699875,
-0.03612127900123596,
0.02487444132566452,
0.06069111451506615,
-0.06476162374019623,
-0.080284483730793,
0.020959148183465004,
-0.001314549706876278,
0.04624095559120178,
0.032660070806741714,
-0.11076541244983673,
0.0010113705648109317,
0.11533752083778381,
-0.07499057799577713,
-0.0064829266630113125,
-0.09433696419000626,
0.11583013087511063,
0.042505741119384766,
-0.002667458029463887,
-0.019497808068990707,
-0.022772688418626785,
-0.00266881356947124,
-0.01677589677274227,
0.04429478570818901,
-0.0626695305109024,
0.000997783150523901,
0.001259782467968762,
-0.00559414504095912,
0.022608047351241112,
-0.024318834766745567,
-0.030689725652337074,
-0.004394433926790953
] |
facebook/contriever-msmarco | abe8c1493371369031bcb1e02acb754cf4e162fa | 2022-06-25T17:19:59.000Z | [
"pytorch",
"bert",
"arxiv:2112.09118",
"transformers",
"feature-extraction"
] | feature-extraction | false | facebook | null | facebook/contriever-msmarco | 2,121 | null | transformers | ---
tags:
- feature-extraction
pipeline_tag: feature-extraction
---
This model is the finetuned version of the pre-trained contriever model available here https://huggingface.co/facebook/contriever, following the approach described in [Towards Unsupervised Dense Information Retrieval with Contrastive Learning](https://arxiv.org/abs/2112.09118). The associated GitHub repository is available here https://github.com/facebookresearch/contriever.
## Usage (HuggingFace Transformers)
Using the model directly available in HuggingFace transformers requires to add a mean pooling operation to obtain a sentence embedding.
```python
import torch
from transformers import AutoTokenizer, AutoModel
tokenizer = AutoTokenizer.from_pretrained('facebook/contriever-msmarco')
model = AutoModel.from_pretrained('facebook/contriever-msmarco')
sentences = [
"Where was Marie Curie born?",
"Maria Sklodowska, later known as Marie Curie, was born on November 7, 1867.",
"Born in Paris on 15 May 1859, Pierre Curie was the son of Eugène Curie, a doctor of French Catholic origin from Alsace."
]
# Apply tokenizer
inputs = tokenizer(sentences, padding=True, truncation=True, return_tensors='pt')
# Compute token embeddings
outputs = model(**inputs)
# Mean pooling
def mean_pooling(token_embeddings, mask):
token_embeddings = token_embeddings.masked_fill(~mask[..., None].bool(), 0.)
sentence_embeddings = token_embeddings.sum(dim=1) / mask.sum(dim=1)[..., None]
return sentence_embeddings
embeddings = mean_pooling(outputs[0], inputs['attention_mask'])
``` | [
-0.05973246321082115,
-0.003820281010121107,
0.029406532645225525,
0.0905199944972992,
0.07488294690847397,
-0.0025456889998167753,
-0.07535018771886826,
-0.027842730283737183,
-0.030140535905957222,
-0.11075305193662643,
0.015893571078777313,
-0.06382659822702408,
0.005084634758532047,
0.02924598753452301,
0.021726587787270546,
0.06124576926231384,
0.04308748617768288,
0.05372457578778267,
-0.10365509241819382,
-0.044556982815265656,
0.014212891459465027,
0.0618288591504097,
0.06363773345947266,
-0.01711195893585682,
0.05988481640815735,
-0.006267381366342306,
-0.07676096260547638,
-0.06027543172240257,
0.12273148447275162,
0.023486167192459106,
0.06782689690589905,
0.019589493051171303,
-0.04232793673872948,
0.12180294096469879,
-0.014355478808283806,
0.0956914871931076,
-0.0712757259607315,
0.020438557490706444,
-0.022354908287525177,
-0.049190111458301544,
0.04612572118639946,
-0.0384150967001915,
-0.03911715745925903,
0.010211185552179813,
0.08897639811038971,
0.029296372085809708,
-0.06852216273546219,
0.010324811562895775,
-0.017275525256991386,
-0.024368438869714737,
-0.09588289260864258,
-0.06896712630987167,
0.011638735421001911,
0.08038900792598724,
-0.020673617720603943,
0.05261966958642006,
-0.009236904792487621,
-0.08622511476278305,
0.022971244528889656,
-0.060383543372154236,
-0.02964545600116253,
-0.05784027278423309,
-0.006520417984575033,
-0.016633987426757812,
-0.04646681621670723,
-0.006082342937588692,
-0.0024563453625887632,
0.04211803153157234,
0.04667705297470093,
0.02207338809967041,
0.03383035957813263,
0.052966900169849396,
0.002981300000101328,
0.02957092598080635,
0.055005114525556564,
0.030485253781080246,
0.03438505902886391,
0.01314765214920044,
0.03928392753005028,
-0.03410657122731209,
0.026747751981019974,
-0.06241634860634804,
0.0904872715473175,
0.011839414946734905,
0.03317759931087494,
-0.019855372607707977,
-0.023296790197491646,
-0.05159248039126396,
-0.09378111362457275,
0.048664458096027374,
-0.07823753356933594,
-0.07570245862007141,
-0.040043387562036514,
-0.022252172231674194,
-0.07498311996459961,
-0.03743719682097435,
-0.021195748820900917,
0.018750200048089027,
-0.05693698301911354,
0.10182555019855499,
-0.02277960628271103,
0.035050664097070694,
0.046410322189331055,
-0.0059526292607188225,
-0.04882621765136719,
-0.032089006155729294,
-0.009215258061885834,
0.0018231736030429602,
0.022571764886379242,
-0.03690069168806076,
0.029714860022068024,
-0.0041998764500021935,
-0.03281587362289429,
-0.06272697448730469,
0.04092320054769516,
-0.024785980582237244,
0.030442018061876297,
-0.019454680383205414,
0.10902965068817139,
0.04079660773277283,
0.03745940327644348,
0.04108394309878349,
-0.012620833702385426,
-0.029695305973291397,
-0.011727487668395042,
-0.04977954179048538,
-0.04244084283709526,
4.810493898969279e-33,
0.012313836254179478,
0.04794301465153694,
0.02413245663046837,
0.010846429504454136,
-0.02614043653011322,
0.04374151676893234,
-0.020360765978693962,
-0.03170480579137802,
-0.0642765462398529,
-0.006449935492128134,
-0.03400498628616333,
0.06725995987653732,
-0.03695603087544441,
0.029830940067768097,
-0.04579107463359833,
-0.04900166764855385,
-0.01264398917555809,
-0.03587545454502106,
-0.007730776909738779,
0.04841351509094238,
0.01837366819381714,
-0.006066167261451483,
-0.0510617159307003,
-0.05838076025247574,
-0.1240493580698967,
-0.04921010509133339,
0.02103998139500618,
0.015497029758989811,
0.005319278687238693,
0.017230002209544182,
-0.10732722282409668,
0.04976295307278633,
0.022930437698960304,
0.004552875645458698,
0.04481050744652748,
-0.029582064598798752,
0.034237176179885864,
-0.039426542818546295,
-0.04479024186730385,
-0.06610524654388428,
-0.004996518138796091,
0.053203076124191284,
-0.006842518225312233,
-0.1303243637084961,
-0.06593921780586243,
0.03621191158890724,
0.014433721080422401,
-0.048233889043331146,
0.034631576389074326,
0.02227560617029667,
0.07569048553705215,
-0.012027701362967491,
-0.04505616053938866,
0.006595301907509565,
-0.022723263129591942,
0.029025612398982048,
0.06302068382501602,
0.03584887832403183,
0.0677775964140892,
-0.03799128532409668,
-0.005326960701495409,
-0.003993976395577192,
0.11556986719369888,
0.011354085989296436,
0.03310827538371086,
0.03862980380654335,
0.04971633851528168,
0.11016219854354858,
-0.010537412948906422,
-0.023763015866279602,
-0.04340323060750961,
0.043232955038547516,
-0.06398317962884903,
-0.07131194323301315,
0.007417564280331135,
-0.03929271176457405,
0.04716255143284798,
-0.04502962902188301,
0.007168476935476065,
0.03334726393222809,
0.023175165057182312,
0.0011745874071493745,
0.027922596782445908,
-0.006600608583539724,
-0.058492328971624374,
-0.02484525553882122,
0.056019991636276245,
-0.09478265047073364,
0.009240684099495411,
-0.04033010080456734,
-0.011388462036848068,
0.005330926273018122,
0.04191030189394951,
-0.06980199366807938,
-0.03187105059623718,
-5.4237158736726647e-33,
0.09942507743835449,
0.011940127238631248,
-0.00676887109875679,
-0.03482755273580551,
0.015517793595790863,
-0.04679400846362114,
0.04988811910152435,
0.09702622890472412,
-0.026419486850500107,
-0.08647586405277252,
0.03212989866733551,
-0.07649710029363632,
-0.018321800976991653,
-0.0864575058221817,
0.06651214510202408,
0.06127188727259636,
-0.00021707976702600718,
0.035501524806022644,
-0.0017165469471365213,
0.05298948287963867,
-0.030745159834623337,
0.03756706416606903,
-0.14330169558525085,
0.07025782763957977,
-0.03755364567041397,
0.03297669440507889,
0.02858630008995533,
0.03211278095841408,
0.05089893937110901,
-0.0678400993347168,
0.0018137552542611957,
-0.023580437526106834,
-0.06805245578289032,
0.03625794127583504,
-0.1311781406402588,
0.013366492465138435,
-0.009372875094413757,
0.02194567210972309,
-0.0018296249909326434,
0.00020956608932465315,
0.09745222330093384,
0.05750429630279541,
-0.13635337352752686,
0.08035822212696075,
-0.017766054719686508,
0.02407274954020977,
-0.06718626618385315,
-0.011881135404109955,
0.017362849786877632,
0.06601868569850922,
0.018939990550279617,
-0.02727700211107731,
-0.08797433972358704,
0.01190093718469143,
-0.108094222843647,
-0.08835781365633011,
0.08445975929498672,
-0.02674141153693199,
-0.04752110317349434,
-0.005570659879595041,
-0.05176600068807602,
-0.03138837590813637,
-0.07218824326992035,
-0.08485827594995499,
0.031950294971466064,
-0.06805721670389175,
-0.04588516801595688,
0.036447152495384216,
-0.04703271761536598,
0.008281458169221878,
0.005498568993061781,
0.057897962629795074,
0.01647099480032921,
0.02474099211394787,
0.034882303327322006,
0.06269794702529907,
0.038862451910972595,
-0.027004901319742203,
-0.0033205028157681227,
-0.01567704789340496,
-0.11429527401924133,
-0.021766413003206253,
0.037484992295503616,
0.08283054083585739,
0.02400645986199379,
0.001466575195081532,
0.003208798123523593,
0.04565777629613876,
0.02535570040345192,
-0.03646683692932129,
-0.03180473670363426,
0.012808163650333881,
0.011246005073189735,
0.06339666992425919,
0.023382220417261124,
-5.61181145997125e-8,
-0.11237289756536484,
-0.03016063943505287,
-0.0792553648352623,
0.005548399407416582,
-0.07077696919441223,
0.01520825456827879,
0.026058170944452286,
0.009998978115618229,
-0.0331464558839798,
-0.006222131662070751,
-0.048577308654785156,
0.048236045986413956,
-0.035959165543317795,
-0.024843238294124603,
-0.03714042156934738,
0.05100330337882042,
0.024112287908792496,
0.058118972927331924,
-0.014660459011793137,
0.014034067280590534,
0.009582621976733208,
0.00620298134163022,
0.025201819837093353,
-0.015060216188430786,
0.0498526506125927,
-0.04831637442111969,
-0.08793078362941742,
0.047937046736478806,
-0.054387710988521576,
0.004919559229165316,
0.007940304465591908,
0.018160440027713776,
0.027770549058914185,
-0.04862569272518158,
0.07413262873888016,
0.08424071222543716,
0.02930956520140171,
-0.13801933825016022,
-0.013282615691423416,
0.04165521636605263,
0.09869620203971863,
0.08189351856708527,
-0.06650137156248093,
-0.01649557054042816,
0.002514186315238476,
0.03703151270747185,
0.07191222161054611,
-0.06169021874666214,
0.08750637620687485,
0.08013111352920532,
0.025632187724113464,
-0.01862473599612713,
-0.09921509027481079,
0.05056420713663101,
0.022415995597839355,
-0.010322274640202522,
0.02327340468764305,
0.07871776819229126,
0.0790623128414154,
0.045454978942871094,
0.06882309913635254,
0.03365930914878845,
0.06097203865647316,
0.020306991413235664
] |
textattack/distilbert-base-cased-CoLA | 73fd8dc841293aab1caea98581bb57481c87ff55 | 2020-06-09T16:45:43.000Z | [
"pytorch",
"distilbert",
"text-classification",
"transformers"
] | text-classification | false | textattack | null | textattack/distilbert-base-cased-CoLA | 2,120 | null | transformers | Entry not found | [
0.0461147278547287,
-0.038838207721710205,
-0.01049656979739666,
-0.03682169318199158,
0.011261860840022564,
0.013094935566186905,
0.0019101888174191117,
-0.013979103416204453,
0.027092741802334785,
-0.015212527476251125,
0.017284274101257324,
-0.08189476281404495,
0.03817418962717056,
-0.04920130595564842,
0.021389011293649673,
-0.015245908871293068,
-0.03203780576586723,
-0.1245758980512619,
0.03150877356529236,
0.032381657510995865,
-0.060957908630371094,
0.05409295856952667,
-0.025087490677833557,
0.01568586938083172,
0.028129950165748596,
-0.04710396006703377,
-0.018688226118683815,
0.013785239309072495,
-0.04001208767294884,
0.01173911802470684,
-0.04317743331193924,
0.05500618368387222,
0.004543041344732046,
0.02973111905157566,
0.14852192997932434,
0.02658126689493656,
0.02907961793243885,
-0.05169107764959335,
0.05803573504090309,
-0.07732241600751877,
-0.017637968063354492,
-0.04219653457403183,
0.041807834059000015,
0.023620979860424995,
0.021563321352005005,
0.016478516161441803,
-0.0021814992651343346,
-0.06400240957736969,
0.06393089145421982,
0.019599027931690216,
-0.08565037697553635,
0.00934905931353569,
-0.008718925528228283,
-0.028583496809005737,
-0.07310017943382263,
0.09416428208351135,
0.001759322709403932,
0.06184990331530571,
0.011840506456792355,
-0.035997264087200165,
0.08358278125524521,
-0.02619801089167595,
0.03736566752195358,
-0.028206506744027138,
-0.07454850524663925,
-0.08883563429117203,
-0.06279942393302917,
-0.008695344440639019,
0.014119276776909828,
-0.0825355276465416,
0.0649217739701271,
-0.00223911227658391,
-0.14716917276382446,
0.07743025571107864,
-0.03548373281955719,
-0.055201586335897446,
0.006981803569942713,
-0.012166670523583889,
0.055111464112997055,
-0.007116836030036211,
-0.023175746202468872,
-0.005835152696818113,
-0.09185640513896942,
0.055196937173604965,
0.034148022532463074,
0.03835180774331093,
0.038685429841279984,
-0.025987252593040466,
0.017804903909564018,
0.022428328171372414,
0.025005368515849113,
-0.10761535167694092,
-0.048001550137996674,
-0.04343584179878235,
0.012374646961688995,
-0.019502125680446625,
0.029218152165412903,
0.0842173621058464,
-0.011719699949026108,
0.09283553808927536,
-0.007015465293079615,
-0.03543110564351082,
-0.06936459988355637,
0.09425332397222519,
-0.010958523489534855,
-0.00805904995650053,
0.004974212497472763,
-0.0031528924591839314,
0.06105927750468254,
-0.03964288905262947,
-0.03619541600346565,
-0.019901901483535767,
0.07134733349084854,
0.039514873176813126,
-0.012729483656585217,
-0.006646515801548958,
-0.04746140539646149,
-0.014432490803301334,
-0.05157482624053955,
0.09506245702505112,
-0.049747664481401443,
-0.04591796174645424,
-0.008965466171503067,
-0.0325421579182148,
-0.08626784384250641,
-0.06624380499124527,
0.02538885548710823,
-4.303924894057984e-33,
0.01133066974580288,
0.0033434738870710135,
-0.002155609894543886,
0.04871906340122223,
-0.023564351722598076,
-0.07933273911476135,
0.0600903145968914,
0.02335330657660961,
-0.03844716399908066,
-0.020433755591511726,
-0.06952055543661118,
-0.03235611692070961,
0.0062485747039318085,
0.064804308116436,
-0.03201229125261307,
0.061689723283052444,
0.0417000837624073,
-0.00761845987290144,
0.03340127319097519,
-0.047770582139492035,
0.00887306872755289,
-0.04066338762640953,
-0.010506896302103996,
0.0106519665569067,
0.021333497017621994,
0.12854498624801636,
-0.009705503471195698,
0.010055632330477238,
-0.017507633194327354,
0.006515394430607557,
0.06334009766578674,
-0.057817306369543076,
0.013668818399310112,
-0.020286159589886665,
0.05430467426776886,
-0.023184705525636673,
0.0828516036272049,
0.0005449643940664828,
-0.10372652113437653,
-0.07634282112121582,
-0.005381610710173845,
-0.039263784885406494,
0.0006114727002568543,
-0.013281986117362976,
0.07119110971689224,
0.043696220964193344,
0.03168422728776932,
0.04338686540722847,
0.05728672817349434,
0.0832006186246872,
-0.07961414009332657,
0.015234283171594143,
0.017002005130052567,
0.047004107385873795,
-0.09794387966394424,
0.004990279674530029,
-0.07062993198633194,
-0.028000490739941597,
-0.04018733277916908,
-0.0702052190899849,
0.011351344175636768,
0.06020182743668556,
-0.03297270089387894,
0.09396500885486603,
0.03417910635471344,
-0.019825750961899757,
-0.034690454602241516,
-0.013036907650530338,
0.05896938592195511,
-0.012359356507658958,
-0.017275206744670868,
-0.07982361316680908,
0.02059139870107174,
0.06737419217824936,
0.04176458343863487,
-0.04978838190436363,
-0.05877475067973137,
-0.06289287656545639,
-0.03354167565703392,
-0.03871942684054375,
0.009898529388010502,
-0.05514208599925041,
-0.11629002541303635,
-0.011855563148856163,
0.10663620382547379,
0.037354156374931335,
-0.0065480442717671394,
-0.051189567893743515,
0.06663123518228531,
0.01874656230211258,
0.032841797918081284,
0.041593004018068314,
-0.06879369914531708,
0.04216769337654114,
-0.01628219522535801,
5.4139394340936695e-34,
0.05697013810276985,
-0.006972255185246468,
0.015711724758148193,
-0.17956365644931793,
0.02320219948887825,
0.007923615165054798,
-0.008062449283897877,
0.0074974060989916325,
0.07391711324453354,
0.0309313777834177,
0.060510627925395966,
0.058605875819921494,
0.09515274316072464,
-0.002282935893163085,
0.001603541080839932,
0.07024981826543808,
0.012629246339201927,
0.07425693422555923,
-0.038426291197538376,
0.01861148327589035,
0.030608950182795525,
-0.02449394389986992,
0.021528491750359535,
-0.003039651783183217,
-0.03676343336701393,
0.03130284696817398,
0.07998586446046829,
0.010451192036271095,
-0.07930229604244232,
-0.013543923385441303,
0.018781835213303566,
0.05168003588914871,
-0.07191970944404602,
0.15783067047595978,
0.026191607117652893,
0.01262354850769043,
0.08218053728342056,
-0.029807550832629204,
-0.07528624683618546,
-0.04250097647309303,
0.017244765534996986,
0.04411793500185013,
0.03708017244935036,
0.009233047254383564,
-0.040271829813718796,
0.022496428340673447,
0.02495843544602394,
0.07633638381958008,
0.005147108342498541,
0.013892097398638725,
0.05610476806759834,
-0.06684739887714386,
0.05862557515501976,
-0.020688841119408607,
0.05377643182873726,
0.06718500703573227,
0.005329249892383814,
-0.01388032827526331,
0.029931528493762016,
0.009508464485406876,
-0.045173756778240204,
0.11534366756677628,
-0.06510116159915924,
0.05117698386311531,
-0.0026125339791178703,
-0.08554837852716446,
-0.03784770518541336,
0.0804959163069725,
0.011298024095594883,
-0.07695550471544266,
-0.04868878796696663,
0.02515520341694355,
0.06252261996269226,
-0.04509226232767105,
-0.01246943511068821,
0.028559505939483643,
-0.030573077499866486,
0.05066261067986488,
-0.08187384903430939,
0.04469604790210724,
0.0034051244147121906,
0.04145054519176483,
-0.021858664229512215,
-0.06112268194556236,
-0.00908052921295166,
-0.05903250351548195,
0.0259539932012558,
0.059690944850444794,
-0.07613514363765717,
-0.03720718249678612,
-0.036316655576229095,
0.07058046013116837,
-0.008224100805819035,
0.041961874812841415,
-0.0285952128469944,
-1.496900736697171e-8,
-0.0014124972512945533,
0.03401879221200943,
-0.040338415652513504,
0.04116074740886688,
0.0935964286327362,
-0.05115952715277672,
0.0008746005478315055,
-0.03389839455485344,
-0.00567849725484848,
-0.010686947964131832,
-0.04789939522743225,
-0.04820054769515991,
-0.02011880651116371,
-0.03209094703197479,
-0.04211259260773659,
-0.10229527950286865,
-0.07819421589374542,
-0.031228765845298767,
-0.02154778689146042,
-0.04960230365395546,
0.08087796717882156,
-0.07801242172718048,
0.06919731199741364,
-0.04999840259552002,
0.03687043860554695,
0.03889009356498718,
-0.049989692866802216,
-0.04254625365138054,
-0.04606937617063522,
0.08682432025671005,
-0.031148413196206093,
0.11826753616333008,
0.034102488309144974,
-0.0208592489361763,
-0.0205202866345644,
0.027134142816066742,
0.09741277992725372,
0.051608603447675705,
0.013477512635290623,
-0.13649295270442963,
-0.022304272279143333,
0.02385953813791275,
0.038732077926397324,
-0.09249968826770782,
-0.04549082741141319,
0.054220106452703476,
0.01160438358783722,
0.051190607249736786,
0.07713303714990616,
-0.022097084671258926,
-0.06127818301320076,
-0.01857956498861313,
0.006740490905940533,
-0.00496308971196413,
0.024095389991998672,
0.0736224576830864,
-0.003481915919110179,
-0.0699305310845375,
-0.006629763171076775,
-0.0598808117210865,
0.05297163128852844,
-0.02902800403535366,
-0.027858933433890343,
-0.01287526823580265
] |
SEBIS/legal_t5_small_trans_fr_en | 2940039b5f8da8f9a6f3c09be0c9667be9d7a9a9 | 2021-06-23T09:52:57.000Z | [
"pytorch",
"jax",
"t5",
"text2text-generation",
"French English",
"dataset:dcep europarl jrc-acquis",
"transformers",
"translation French English model",
"autotrain_compatible"
] | text2text-generation | false | SEBIS | null | SEBIS/legal_t5_small_trans_fr_en | 2,119 | null | transformers |
---
language: French English
tags:
- translation French English model
datasets:
- dcep europarl jrc-acquis
widget:
- text: "quels montants ont été attribués et quelles sommes ont été effectivement utilisées dans chaque État membre? 4."
---
# legal_t5_small_trans_fr_en model
Model on translating legal text from French to English. It was first released in
[this repository](https://github.com/agemagician/LegalTrans). This model is trained on three parallel corpus from jrc-acquis, europarl and dcep.
## Model description
legal_t5_small_trans_fr_en is based on the `t5-small` model and was trained on a large corpus of parallel text. This is a smaller model, which scales the baseline model of t5 down by using `dmodel = 512`, `dff = 2,048`, 8-headed attention, and only 6 layers each in the encoder and decoder. This variant has about 60 million parameters.
## Intended uses & limitations
The model could be used for translation of legal texts from French to English.
### How to use
Here is how to use this model to translate legal text from French to English in PyTorch:
```python
from transformers import AutoTokenizer, AutoModelWithLMHead, TranslationPipeline
pipeline = TranslationPipeline(
model=AutoModelWithLMHead.from_pretrained("SEBIS/legal_t5_small_trans_fr_en"),
tokenizer=AutoTokenizer.from_pretrained(pretrained_model_name_or_path = "SEBIS/legal_t5_small_trans_fr_en", do_lower_case=False,
skip_special_tokens=True),
device=0
)
fr_text = "quels montants ont été attribués et quelles sommes ont été effectivement utilisées dans chaque État membre? 4."
pipeline([fr_text], max_length=512)
```
## Training data
The legal_t5_small_trans_fr_en model was trained on [JRC-ACQUIS](https://wt-public.emm4u.eu/Acquis/index_2.2.html), [EUROPARL](https://www.statmt.org/europarl/), and [DCEP](https://ec.europa.eu/jrc/en/language-technologies/dcep) dataset consisting of 5 Million parallel texts.
## Training procedure
The model was trained on a single TPU Pod V3-8 for 250K steps in total, using sequence length 512 (batch size 4096). It has a total of approximately 220M parameters and was trained using the encoder-decoder architecture. The optimizer used is AdaFactor with inverse square root learning rate schedule for pre-training.
### Preprocessing
An unigram model trained with 88M lines of text from the parallel corpus (of all possible language pairs) to get the vocabulary (with byte pair encoding), which is used with this model.
### Pretraining
## Evaluation results
When the model is used for translation test dataset, achieves the following results:
Test results :
| Model | BLEU score |
|:-----:|:-----:|
| legal_t5_small_trans_fr_en | 51.44|
### BibTeX entry and citation info
> Created by [Ahmed Elnaggar/@Elnaggar_AI](https://twitter.com/Elnaggar_AI) | [LinkedIn](https://www.linkedin.com/in/prof-ahmed-elnaggar/)
| [
-0.025606190785765648,
-0.05472900718450546,
-0.013601262122392654,
-0.05703667923808098,
0.04314785823225975,
0.0399705208837986,
-0.026793818920850754,
0.07951846718788147,
0.10081396251916885,
-0.006382662802934647,
0.04180935025215149,
-0.03271105885505676,
0.017123233526945114,
-0.03732677921652794,
-0.056459009647369385,
-0.054997868835926056,
0.01638522371649742,
0.03963484242558479,
-0.14073798060417175,
-0.07936036586761475,
0.027576223015785217,
0.037695132195949554,
0.06063934043049812,
0.008102437481284142,
0.07111417502164841,
-0.025301221758127213,
-0.08831824362277985,
-0.022166170179843903,
0.01786058582365513,
-0.031249936670064926,
0.011419159360229969,
0.07693306356668472,
-0.04987361282110214,
0.08718958497047424,
-0.030631551519036293,
0.002386132488027215,
-0.046215880662202835,
-0.05590737611055374,
0.03686283528804779,
0.028828447684645653,
-0.007546086795628071,
-0.030903035774827003,
-0.04401577636599541,
0.010208112187683582,
0.10373090952634811,
-0.00573750352486968,
-0.048195671290159225,
0.051299240440130234,
-0.04489605873823166,
-0.015137088485062122,
-0.11090052127838135,
-0.032824188470840454,
0.004482359625399113,
0.06122219190001488,
-0.06383863836526871,
-0.06911461055278778,
-0.0017473542829975486,
-0.007930165156722069,
0.05701562389731407,
0.00023641657026018947,
-0.060335922986269,
-0.010197962634265423,
-0.06907551735639572,
0.048093151301145554,
-0.022904351353645325,
-0.029258091002702713,
0.007907162420451641,
0.011807992123067379,
-0.020254390314221382,
-0.014555374160408974,
-0.05402694642543793,
0.02610819786787033,
0.003966088406741619,
0.0856272354722023,
0.02519225887954235,
-0.0062265219166874886,
0.02056320197880268,
0.022508056834340096,
0.062418702989816666,
-0.16851802170276642,
0.011063394136726856,
0.01124575175344944,
0.04166683182120323,
-0.032134126871824265,
0.036721426993608475,
-0.06487889587879181,
0.01789344660937786,
0.03719937056303024,
0.12964849174022675,
0.005184561479836702,
0.02927582897245884,
-0.015324839390814304,
0.0342385470867157,
0.05234835669398308,
-0.06270338594913483,
0.02441275864839554,
0.02911955490708351,
0.05547286570072174,
-0.05787239596247673,
0.08343413472175598,
0.06648220866918564,
0.07995044440031052,
0.0921974927186966,
0.014040748588740826,
-0.07933877408504486,
-0.05242424085736275,
0.07337445765733719,
0.04442344605922699,
0.017378337681293488,
-0.14524149894714355,
-0.0012104922207072377,
0.024488581344485283,
-0.02802344039082527,
-0.05943518504500389,
0.057061828672885895,
0.025882937014102936,
-0.00738033652305603,
-0.07474313676357269,
0.013000966981053352,
0.0068546780385077,
-0.07642898708581924,
0.05329824984073639,
-0.039912838488817215,
-0.06572956591844559,
0.003502747043967247,
-0.01063764188438654,
-0.07956091314554214,
5.0299260008311676e-33,
0.024521637707948685,
0.1047961488366127,
0.017513040453195572,
0.08346796780824661,
-0.0343744158744812,
-0.030296294018626213,
-0.05898726359009743,
0.06527677923440933,
-0.08424540609121323,
0.016079723834991455,
-0.07863064110279083,
-0.021867750212550163,
-0.06767091155052185,
0.09689280390739441,
0.021850617602467537,
-0.04184131324291229,
0.05066181346774101,
0.004991546738892794,
-0.008351336233317852,
0.05045889690518379,
0.1443195939064026,
-0.0013030257541686296,
0.044962309300899506,
0.004319299012422562,
-0.0041417828761041164,
0.07718504965305328,
0.027710089460015297,
-0.08208286017179489,
-0.054862067103385925,
0.06303815543651581,
-0.12569983303546906,
-0.010337761603295803,
0.006054915487766266,
0.009848705492913723,
0.04829634726047516,
-0.026501070708036423,
0.04059109836816788,
-0.021604616194963455,
0.01785150170326233,
-0.04166237264871597,
0.02426694706082344,
0.022180233150720596,
0.049363378435373306,
-0.02486814744770527,
-0.034668587148189545,
-0.010177331045269966,
-0.03344796970486641,
-0.05837760865688324,
-0.018316220492124557,
0.01261071301996708,
0.03893526270985603,
-0.013801123946905136,
-0.03702498972415924,
-0.04929666966199875,
0.06700541824102402,
0.1283424347639084,
-0.0482323095202446,
0.05432841554284096,
0.012641304172575474,
0.06375781446695328,
0.01659517176449299,
0.009093119762837887,
0.0231331717222929,
0.0743168294429779,
0.11377923935651779,
0.0455603264272213,
-0.11362271755933762,
-0.009027605876326561,
0.10439830273389816,
-0.02037646435201168,
-0.08566273748874664,
0.027063541114330292,
0.042901813983917236,
0.04378825053572655,
0.09004152566194534,
0.009404651820659637,
0.004355776589363813,
-0.07952798902988434,
0.012228394858539104,
-0.027838192880153656,
-0.10470666736364365,
-0.00601828983053565,
-0.04070340096950531,
-0.016078412532806396,
-0.028424030169844627,
-0.03899417445063591,
0.07163537293672562,
-0.052555885165929794,
0.02254766784608364,
0.008303230628371239,
0.0009415175300091505,
-0.053495652973651886,
-0.047589804977178574,
-0.03899458423256874,
0.017955569550395012,
-5.011387720234731e-33,
-0.02898075059056282,
-0.026029733940958977,
-0.08678677678108215,
0.04297225549817085,
-0.040585558861494064,
-0.05876097455620766,
-0.007121299393475056,
0.10574765503406525,
0.0005390067235566676,
-0.06502620130777359,
0.03331724926829338,
-0.09523217380046844,
0.05740971490740776,
-0.01951150968670845,
0.06232088804244995,
-0.044910088181495667,
0.03277646750211716,
-0.02481946162879467,
0.08767570555210114,
0.1073656901717186,
0.007552372291684151,
0.00405703904107213,
-0.08885233104228973,
0.045529041439294815,
-0.020677635446190834,
0.04897112026810646,
-0.04320752993226051,
0.04069065302610397,
0.025518298149108887,
-0.0038946266286075115,
-0.060055144131183624,
-0.020765451714396477,
-0.008089425973594189,
0.05157047510147095,
-0.0862840786576271,
0.025217745453119278,
0.017305515706539154,
-0.012648012489080429,
-0.028837330639362335,
0.045159902423620224,
0.04176928848028183,
0.01940479874610901,
-0.05663039907813072,
-0.04523063078522682,
-0.04480491206049919,
0.02140248939394951,
-0.11591742187738419,
-0.05520504340529442,
0.033865585923194885,
-0.06484048813581467,
0.07732044160366058,
0.013287421315908432,
-0.0673849806189537,
0.011533019132912159,
-0.014161154627799988,
-0.07760360836982727,
0.0026468306314200163,
-0.06731078773736954,
-0.05044570192694664,
-0.06307335197925568,
-0.0023514183703809977,
0.02653234638273716,
-0.01414372306317091,
-0.06301825493574142,
0.06372914463281631,
-0.030069956555962563,
-0.06031838059425354,
0.04238857701420784,
0.009770194999873638,
-0.041841719299554825,
0.10422973334789276,
-0.08800310641527176,
0.005191461183130741,
-0.025884799659252167,
-0.012208758853375912,
-0.07987045496702194,
-0.01016196794807911,
-0.03587307035923004,
-0.004169776104390621,
-0.029357055202126503,
-0.04695422947406769,
-0.049761466681957245,
-0.003324968973174691,
0.05677180737257004,
0.018429137766361237,
0.048587825149297714,
-0.009754368104040623,
0.02371545508503914,
0.05118529871106148,
0.04538259655237198,
0.031768955290317535,
0.06036052852869034,
0.0008773705922067165,
0.09397459030151367,
-0.017575355246663094,
-5.520809409631511e-8,
-0.04696130380034447,
0.01875200867652893,
-0.059040945023298264,
0.055728666484355927,
-0.02490198239684105,
-0.060372721403837204,
-0.06186997890472412,
0.014318233355879784,
-0.05140566825866699,
0.0029609412886202335,
0.030441801995038986,
0.03150253742933273,
-0.03348853439092636,
-0.0131145678460598,
-0.01995910331606865,
0.05462586134672165,
0.013648301362991333,
0.037337079644203186,
-0.0031339460983872414,
0.0024801616091281176,
0.0020910196471959352,
0.05332198739051819,
0.02195940911769867,
-0.049544427543878555,
0.04950600489974022,
-0.049773238599300385,
-0.057176340371370316,
0.04803215339779854,
0.01332765817642212,
-0.08332021534442902,
-0.016380557790398598,
0.04990800842642784,
-0.03930355980992317,
-0.01576007343828678,
0.03618653491139412,
0.04275145381689072,
-0.01070618350058794,
-0.0044000823982059956,
0.019394109025597572,
0.06760598719120026,
0.0970497652888298,
-0.03971020132303238,
-0.07949722558259964,
0.0038481249939650297,
0.08198989927768707,
0.0011390340514481068,
-0.025188051164150238,
-0.08753806352615356,
0.08804921060800552,
-0.022817643359303474,
0.015034024603664875,
0.02108551748096943,
-0.0009147559758275747,
0.03159679099917412,
-0.01331156026571989,
0.05437494441866875,
0.02330845408141613,
-0.029049022123217583,
0.002680770121514797,
0.004241349175572395,
0.01676667481660843,
-0.006684620399028063,
0.006594719830900431,
-0.03128521516919136
] |
dbmdz/convbert-base-turkish-cased | 6d9b09e4e6f249c477aac7b73f3bcf9aa78ed1a8 | 2021-03-15T23:29:04.000Z | [
"pytorch",
"tf",
"convbert",
"feature-extraction",
"tr",
"arxiv:2008.02496",
"transformers",
"license:mit"
] | feature-extraction | false | dbmdz | null | dbmdz/convbert-base-turkish-cased | 2,119 | null | transformers | ---
language: tr
license: mit
---
# 🤗 + 📚 dbmdz Turkish ConvBERT model
In this repository the MDZ Digital Library team (dbmdz) at the Bavarian State
Library open sources a cased ConvBERT model for Turkish 🎉
# 🇹🇷 ConvBERTurk
ConvBERTurk is a community-driven cased ConvBERT model for Turkish.
In addition to the BERT and ELECTRA based models, we also trained a ConvBERT model. The ConvBERT architecture is presented
in the ["ConvBERT: Improving BERT with Span-based Dynamic Convolution"](https://arxiv.org/abs/2008.02496) paper.
We follow a different training procedure: instead of using a two-phase approach, that pre-trains the model for 90% with 128
sequence length and 10% with 512 sequence length, we pre-train the model with 512 sequence length for 1M steps on a v3-32 TPU.
## Stats
The current version of the model is trained on a filtered and sentence
segmented version of the Turkish [OSCAR corpus](https://traces1.inria.fr/oscar/),
a recent Wikipedia dump, various [OPUS corpora](http://opus.nlpl.eu/) and a
special corpus provided by [Kemal Oflazer](http://www.andrew.cmu.edu/user/ko/).
The final training corpus has a size of 35GB and 44,04,976,662 tokens.
Thanks to Google's TensorFlow Research Cloud (TFRC) we could train a cased model
on a TPU v3-32!
## Usage
With Transformers >= 4.3 our cased ConvBERT model can be loaded like:
```python
from transformers import AutoModel, AutoTokenizer
model_name = "dbmdz/convbert-base-turkish-cased"
tokenizer = AutoTokenizer.from_pretrained(model_name)
model = AutoModel.from_pretrained(model_name)
```
## Results
For results on PoS tagging, NER and Question Answering downstream tasks, please refer to
[this repository](https://github.com/stefan-it/turkish-bert).
# Huggingface model hub
All models are available on the [Huggingface model hub](https://huggingface.co/dbmdz).
# Contact (Bugs, Feedback, Contribution and more)
For questions about our DBMDZ BERT models in general, just open an issue
[here](https://github.com/dbmdz/berts/issues/new) 🤗
# Acknowledgments
Thanks to [Kemal Oflazer](http://www.andrew.cmu.edu/user/ko/) for providing us
additional large corpora for Turkish. Many thanks to Reyyan Yeniterzi for providing
us the Turkish NER dataset for evaluation.
Research supported with Cloud TPUs from Google's TensorFlow Research Cloud (TFRC).
Thanks for providing access to the TFRC ❤️
Thanks to the generous support from the [Hugging Face](https://huggingface.co/) team,
it is possible to download both cased and uncased models from their S3 storage 🤗
| [
-0.12887485325336456,
-0.0630696639418602,
0.04466338828206062,
-0.02069954387843609,
-0.02033671922981739,
0.036361560225486755,
-0.0796685591340065,
0.021850204095244408,
0.017036043107509613,
-0.03186916559934616,
-0.06118922308087349,
0.02171807549893856,
0.034395404160022736,
-0.02171330526471138,
-0.020923566073179245,
-0.02230929024517536,
0.047088783234357834,
-0.006568666081875563,
-0.022388681769371033,
-0.035337403416633606,
0.03255194425582886,
0.04529915377497673,
0.04053796827793121,
-0.05167793482542038,
0.032822027802467346,
-0.05675508826971054,
0.004883793648332357,
-0.04064051806926727,
0.10777068883180618,
-0.031580887734889984,
0.08576931804418564,
0.06592987477779388,
-0.0068426476791501045,
0.06518381834030151,
-0.06147076189517975,
0.03938765823841095,
-0.007245290093123913,
-0.06450781971216202,
-0.013495632447302341,
0.019587481394410133,
0.0360441654920578,
0.015811625868082047,
0.004440524149686098,
0.046217743307352066,
0.06599223613739014,
-0.04022694379091263,
-0.028392285108566284,
0.014154868200421333,
-0.044191282242536545,
0.05081939697265625,
-0.10927548259496689,
-0.023772165179252625,
0.03748658299446106,
0.11151059716939926,
-0.020592665299773216,
0.023342492058873177,
0.027129046618938446,
0.056008145213127136,
-0.010111388750374317,
-0.04624178633093834,
-0.04452555254101753,
-0.01946501061320305,
-0.10198064893484116,
-0.05758417025208473,
-0.06416446715593338,
-0.014854680746793747,
-0.04702714830636978,
0.08417164534330368,
0.02511591836810112,
0.05094193294644356,
-0.029237480834126472,
0.047573693096637726,
-0.029747676104307175,
0.04552140831947327,
-0.02975362166762352,
-0.011937647126615047,
0.12058155983686447,
-0.045435868203639984,
0.09105336666107178,
-0.12700490653514862,
-0.004443770740181208,
-0.021689530462026596,
0.04999755695462227,
-0.1267940104007721,
0.07489289343357086,
-0.040164608508348465,
-0.022738385945558548,
0.007986106909811497,
0.07565708458423615,
0.022001920267939568,
0.059763744473457336,
0.007871920242905617,
0.048175591975450516,
-0.03326365724205971,
0.053678594529628754,
0.08858068287372589,
0.03732436150312424,
0.04375986382365227,
0.05453641712665558,
0.10463140904903412,
0.05749032646417618,
0.053537517786026,
0.03028612583875656,
0.02403084561228752,
-0.014640036039054394,
-0.04858509451150894,
0.10700874030590057,
-0.0032342125196009874,
-0.020371031016111374,
-0.053806401789188385,
0.034822843968868256,
-0.019900521263480186,
0.10440826416015625,
-0.0831671878695488,
0.008008461445569992,
0.04157262295484543,
-0.0140488026663661,
-0.03642093017697334,
0.09437073767185211,
-0.04759508743882179,
-0.0032867533154785633,
0.002548163291066885,
0.025515882298350334,
0.07105199992656708,
0.006275380030274391,
-0.017520828172564507,
-0.02957100421190262,
5.431752214270458e-33,
-0.014950530603528023,
0.008593490347266197,
-0.008776108734309673,
-0.001565449987538159,
-0.021942831575870514,
-0.008993735536932945,
-0.013836048543453217,
-0.0011338993208482862,
-0.10247856378555298,
0.015377325005829334,
-0.096502885222435,
0.0009242663509212434,
-0.07820581644773483,
0.049718696624040604,
0.03731979429721832,
0.0004047703987453133,
-0.03835320845246315,
0.044343169778585434,
-0.06605152040719986,
0.04466257989406586,
0.08454665541648865,
0.04030207172036171,
0.017315568402409554,
-0.058493148535490036,
0.019152993336319923,
0.05594843626022339,
0.06032487750053406,
-0.026482682675123215,
-0.05955268442630768,
0.0585489459335804,
-0.0330483578145504,
0.04131939634680748,
-0.09677613526582718,
0.02286383882164955,
-0.048128847032785416,
-0.04170072451233864,
-0.038385774940252304,
-0.021141229197382927,
-0.04624073952436447,
-0.06085291877388954,
-0.002024911344051361,
0.02908153459429741,
-0.004922168329358101,
-0.030221546068787575,
-0.10609178990125656,
-0.02685067243874073,
0.07035280764102936,
-0.047669243067502975,
-0.028286634013056755,
-0.03116738609969616,
0.08570448309183121,
0.03840329870581627,
-0.10701952129602432,
-0.04751742631196976,
-0.007537460420280695,
0.04864391312003136,
0.03350979834794998,
0.04486605152487755,
0.10567010939121246,
0.09390002489089966,
0.0012878794223070145,
-0.019126934930682182,
0.03941837698221207,
0.051680270582437515,
0.036686141043901443,
-0.03317418694496155,
-0.034710563719272614,
0.04360940307378769,
0.08107923716306686,
-0.005989613011479378,
-0.00507179694250226,
-0.022293586283922195,
-0.028226127848029137,
-0.03365428373217583,
0.030991824343800545,
-0.050494637340307236,
0.06530610471963882,
-0.1347890943288803,
-0.10437966883182526,
-0.0017934844363480806,
-0.03408437222242355,
0.02302597463130951,
-0.045878294855356216,
-0.025455830618739128,
-0.009805440902709961,
-0.0012734857155010104,
0.029598983004689217,
-0.08297505229711533,
-0.0569472536444664,
-0.08870291709899902,
-0.007419710047543049,
-0.019308632239699364,
0.0156694408506155,
0.042971350252628326,
-0.004407098051160574,
-4.6901173999733224e-33,
0.03434854745864868,
0.018519142642617226,
-0.07197146117687225,
0.031748514622449875,
-0.07965336740016937,
-0.058355920016765594,
0.03042869083583355,
0.10072159022092819,
0.008187252096831799,
-0.058221619576215744,
0.0006698116194456816,
-0.10748486965894699,
0.008867784403264523,
-0.011430004611611366,
0.08403221517801285,
-0.023044971749186516,
-0.011354034766554832,
-0.0048017301596701145,
0.0022579894866794348,
0.04714708775281906,
0.060321226716041565,
-0.0008408111170865595,
-0.09411460161209106,
0.03500988334417343,
-0.06560418754816055,
0.01782236434519291,
-0.01871890015900135,
0.07898224890232086,
0.0013494255254045129,
0.016973815858364105,
-0.04524482041597366,
-0.01960160955786705,
-0.02424711175262928,
0.05741385743021965,
-0.11185774207115173,
0.014379044063389301,
0.07418067008256912,
-0.01989651843905449,
-0.021181141957640648,
0.05234035104513168,
0.14260804653167725,
0.030783291906118393,
-0.0694955512881279,
0.03001077100634575,
-0.03785988315939903,
-0.010016177780926228,
-0.06119375675916672,
0.019723784178495407,
-0.0056677162647247314,
-0.09855636954307556,
0.02848910354077816,
-0.03402523696422577,
-0.030831411480903625,
-0.003199174301698804,
0.004315156023949385,
-0.01789039559662342,
-0.04388338699936867,
-0.016609186306595802,
0.01830773428082466,
-0.0322386734187603,
-0.052720021456480026,
-0.006601914297789335,
0.02076805755496025,
-0.09364673495292664,
0.10590570420026779,
0.01790618523955345,
-0.04937909170985222,
0.07611242681741714,
-0.007887796498835087,
0.012310605496168137,
-0.031181855127215385,
0.0096669290214777,
-0.020207390189170837,
0.04170970991253853,
-0.012086907401680946,
0.003991822712123394,
0.002491367980837822,
-0.04899044707417488,
-0.017114687711000443,
-0.011351347900927067,
-0.10260624438524246,
-0.03602919727563858,
0.019406430423259735,
0.029724054038524628,
0.014424648135900497,
0.08870219439268112,
0.03956117853522301,
0.04717874899506569,
0.04367722198367119,
0.06638145446777344,
-0.05819793418049812,
0.05657001584768295,
0.029307929798960686,
0.10060806572437286,
-0.003496748860925436,
-5.582040785157005e-8,
-0.052323099225759506,
0.04041755944490433,
-0.050661250948905945,
0.05205400288105011,
-0.0039407191798090935,
-0.04827237129211426,
-0.07525602728128433,
0.03632684424519539,
-0.023797599598765373,
-0.0233237873762846,
0.03890557959675789,
0.03710462152957916,
-0.07449917495250702,
0.013842115178704262,
-0.05603950843214989,
0.1231967881321907,
-0.056136541068553925,
-0.009534441865980625,
-0.036541134119033813,
0.034913696348667145,
0.038184113800525665,
0.09187359362840652,
0.02788614295423031,
0.020765816792845726,
-0.03712844476103783,
-0.027971774339675903,
-0.06379203498363495,
0.103336401283741,
0.037490151822566986,
-0.04192856699228287,
-0.04889945313334465,
0.08040590584278107,
-0.058873988687992096,
0.007052520290017128,
-0.013358109630644321,
0.04962075501680374,
-0.012774985283613205,
-0.035785794258117676,
0.002701082732528448,
0.033543724566698074,
0.10436411947011948,
0.011943437159061432,
-0.05129339545965195,
-0.022363724187016487,
-0.017121994867920876,
0.006882849149405956,
-0.025873538106679916,
-0.10261514782905579,
0.05525730922818184,
0.03380497545003891,
0.021252427250146866,
0.019311653450131416,
-0.04061438515782356,
0.06398283690214157,
0.03262816742062569,
-0.011174614541232586,
-0.07062274217605591,
-0.025768624618649483,
0.038682520389556885,
0.03323294594883919,
0.04378538578748703,
-0.01719418354332447,
0.04026104137301445,
-0.012827100232243538
] |
valurank/distilroberta-bias | c1e4a2773522c3acc929a7b2c9af2b7e4137b96d | 2022-06-08T20:44:39.000Z | [
"pytorch",
"roberta",
"text-classification",
"en",
"dataset:valurank/wikirev-bias",
"transformers",
"license:other"
] | text-classification | false | valurank | null | valurank/distilroberta-bias | 2,114 | null | transformers | ---
license: other
language: en
datasets:
- valurank/wikirev-bias
---
# DistilROBERTA fine-tuned for bias detection
This model is based on [distilroberta-base](https://huggingface.co/distilroberta-base) pretrained weights, with a classification head fine-tuned to classify text into 2 categories (neutral, biased).
## Training data
The dataset used to fine-tune the model is [wikirev-bias](https://huggingface.co/datasets/valurank/wikirev-bias), extracted from English wikipedia revisions, see https://github.com/rpryzant/neutralizing-bias for details on the WNC wiki edits corpus.
## Inputs
Similar to its base model, this model accepts inputs with a maximum length of 512 tokens.
| [
-0.02942579798400402,
-0.055089376866817474,
-0.05088803172111511,
0.00426904484629631,
0.014935962855815887,
0.008648215793073177,
0.04608653858304024,
0.019209766760468483,
0.016845911741256714,
0.0024407831951975822,
-0.005859118886291981,
0.016711082309484482,
0.018617970868945122,
-0.018605995923280716,
-0.038371823728084564,
0.027219949290156364,
0.06092679500579834,
0.02184324339032173,
-0.13056422770023346,
-0.04242958128452301,
0.03857755288481712,
0.029071763157844543,
0.05023648217320442,
0.003810772905126214,
0.0068854112178087234,
-0.022388774901628494,
-0.028359729796648026,
0.04007924720644951,
0.02051699534058571,
-0.05086209625005722,
0.009869884699583054,
-0.005987865384668112,
0.06893840432167053,
0.03777097538113594,
-0.11348361521959305,
0.02618243359029293,
-0.0702110081911087,
-0.02558698132634163,
0.014316285960376263,
0.07090701907873154,
0.01572526805102825,
0.013258005492389202,
-0.028815336525440216,
0.1103590577840805,
0.07986986637115479,
0.030084513127803802,
-0.060322873294353485,
0.027236655354499817,
-0.1136067733168602,
0.033809516578912735,
-0.032156698405742645,
-0.04281909391283989,
0.002833946608006954,
0.14503659307956696,
-0.06173618137836456,
-0.0849343091249466,
-0.012777108699083328,
0.11847910284996033,
0.03001151792705059,
0.012262220494449139,
0.01216017547994852,
-0.04264940693974495,
-0.0784449502825737,
-0.005033663008362055,
-0.05496663972735405,
-0.05150065943598747,
-0.008812577463686466,
0.060242339968681335,
0.027010370045900345,
-0.06323429942131042,
0.00917692482471466,
0.04413799196481705,
-0.033451635390520096,
0.0519215352833271,
0.04443679377436638,
0.02544216439127922,
0.11106173694133759,
0.005921319127082825,
0.07264702022075653,
-0.11143036931753159,
0.01691044308245182,
-0.015450014732778072,
0.08664824068546295,
-0.03544467315077782,
0.08685897290706635,
-0.0032359932083636522,
-0.07890639454126358,
0.04319777339696884,
0.029170947149395943,
0.055527977645397186,
0.022092780098319054,
-0.02173663303256035,
0.026716038584709167,
0.0056803105399012566,
-0.002402589423581958,
0.05297568067908287,
-0.0563029907643795,
0.013288895599544048,
-0.024426547810435295,
0.08685603737831116,
-0.00021787453442811966,
-0.031224384903907776,
-0.0713401809334755,
0.049860332161188126,
-0.047022271901369095,
-0.08658656477928162,
0.09182742238044739,
0.03305558115243912,
-0.02083474025130272,
-0.09050227701663971,
-0.015380777418613434,
-0.034979529678821564,
0.0008299120818264782,
-0.029999755322933197,
-0.007922870106995106,
0.03242190182209015,
0.023805053904652596,
0.027511216700077057,
-0.019309187307953835,
-0.0126127228140831,
-0.029991822317242622,
-0.0638866275548935,
-0.0066661830060184,
-0.039367128163576126,
-0.017423853278160095,
-0.020433608442544937,
-0.05953517556190491,
3.053028825444185e-33,
0.08484362810850143,
0.06744445115327835,
-0.05819988250732422,
0.00431109918281436,
-0.03402627632021904,
-0.0701412782073021,
-0.03018934838473797,
-0.018149640411138535,
-0.11514674127101898,
0.05139300972223282,
0.003803243627771735,
0.07228739559650421,
-0.04283098503947258,
0.01903955079615116,
0.015663733705878258,
-0.06215611472725868,
-0.02412017621099949,
0.09607122093439102,
0.007929650135338306,
0.051518764346838,
0.04763753339648247,
0.06662745773792267,
0.02225346677005291,
-0.06967148929834366,
-0.05594956874847412,
0.019466590136289597,
-0.00046715670032426715,
-0.003682653186842799,
-0.04146350920200348,
0.03858231380581856,
-0.0948922410607338,
-0.02071339450776577,
0.018816571682691574,
-0.013386688195168972,
-0.0070278760977089405,
-0.059994280338287354,
-0.0604557991027832,
-0.01786688156425953,
0.04467553272843361,
-0.052572183310985565,
0.051176127046346664,
-0.0019482043571770191,
0.11264385282993317,
-0.0014784488594159484,
0.01160250324755907,
-0.01365911215543747,
-0.0069668907672166824,
-0.06613807380199432,
0.028042543679475784,
0.01863628625869751,
-0.0019080133643001318,
0.055222880095243454,
0.037715472280979156,
0.014025654643774033,
-0.021334977820515633,
0.10435493290424347,
0.030637379735708237,
0.05097534880042076,
0.07359693199396133,
0.06103525683283806,
-0.006419561803340912,
0.08962836861610413,
0.03114798292517662,
-0.02085588499903679,
-0.0015685608377680182,
-0.007112998981028795,
-0.061255503445863724,
-0.06422450393438339,
-0.010487359017133713,
0.04432925954461098,
0.0034556519240140915,
-0.044982027262449265,
-0.0887090265750885,
0.0278097502887249,
-0.020401563495397568,
-0.0025355303660035133,
0.024807536974549294,
0.0004845825023949146,
-0.06923464685678482,
-0.034343671053647995,
-0.00023696156858932227,
0.0039077079854905605,
0.011759517714381218,
-0.04550518840551376,
-0.11496049910783768,
0.023496272042393684,
-0.02309412881731987,
-0.12530885636806488,
0.02397344261407852,
0.012113305740058422,
-0.03176136687397957,
0.07735715061426163,
-0.0700354278087616,
-0.06549516320228577,
-0.005634004715830088,
-3.237268095810393e-33,
-0.0022548509296029806,
0.03349248319864273,
-0.0708218663930893,
0.10113575309515,
-0.0606551393866539,
-0.002273878548294306,
-0.010514420457184315,
0.04059924930334091,
0.04472547024488449,
0.03797568008303642,
0.11015637964010239,
-0.06949707865715027,
0.009270242415368557,
0.022120889276266098,
-0.016279684379696846,
-0.019362550228834152,
0.007237321697175503,
0.0009681285009719431,
0.004437972325831652,
0.008164995349943638,
0.10176029056310654,
0.03801281750202179,
-0.10270127654075623,
0.04026562720537186,
-0.010318455286324024,
0.02204250730574131,
0.04044671729207039,
0.03607476130127907,
0.009441602043807507,
0.014820382930338383,
-0.13543114066123962,
-0.04050428047776222,
-0.011492634192109108,
-0.003976432606577873,
-0.03283261880278587,
-0.02437911368906498,
0.029291782528162003,
0.009379374794661999,
-0.055650752037763596,
0.06227247416973114,
0.05678465589880943,
0.03951924294233322,
-0.04031885415315628,
-0.017512092366814613,
-0.030782224610447884,
-0.060700684785842896,
-0.0834793746471405,
0.04952457174658775,
0.018701283261179924,
-0.01540079154074192,
0.06996279209852219,
0.049020200967788696,
-0.043908536434173584,
0.13213224709033966,
0.00787695124745369,
-0.07217384874820709,
0.02288724109530449,
0.01027708314359188,
0.05000055208802223,
0.03778472915291786,
-0.029812173917889595,
-0.008149387314915657,
-0.08950889110565186,
-0.04725447669625282,
0.020610835403203964,
-0.0017010580049827695,
-0.027793334797024727,
-0.034644048660993576,
0.05011163279414177,
0.024291837587952614,
0.07262388616800308,
-0.012835646979510784,
0.011242842301726341,
-0.030481377616524696,
-0.10085241496562958,
-0.0020694108679890633,
0.015404527075588703,
-0.04525841772556305,
-0.04535989090800285,
0.013492136262357235,
0.0011429899604991078,
-0.016029689460992813,
0.0852321907877922,
0.0013507961994037032,
0.051511190831661224,
0.037809062749147415,
-0.01965540647506714,
-0.0034093984868377447,
-0.042168520390987396,
0.02519666962325573,
-0.007523819804191589,
-0.05355697125196457,
0.04008151963353157,
0.02027725987136364,
-0.04258381947875023,
-4.985959023429132e-8,
-0.0661003440618515,
-0.0675402507185936,
-0.09910780191421509,
0.08742086589336395,
-0.08763650059700012,
-0.08783315122127533,
0.02628372609615326,
-0.06254648417234421,
-0.06399773061275482,
0.022358136251568794,
0.030838927254080772,
0.04257485643029213,
-0.028848858550190926,
-0.019942080602049828,
0.06464403122663498,
0.07896888256072998,
0.012747452594339848,
0.07589332014322281,
-0.00689360685646534,
0.06334389001131058,
0.09334924072027206,
0.032734837383031845,
0.0222842488437891,
-0.09138017147779465,
0.04484972357749939,
-0.024511605501174927,
-0.0342266708612442,
0.032290250062942505,
0.032923031598329544,
-0.07688393443822861,
0.002643600571900606,
0.06524275243282318,
-0.030058985576033592,
-0.09855718910694122,
0.06643415242433548,
0.12215043604373932,
-0.1045256033539772,
-0.02528325654566288,
-0.034715622663497925,
0.0887334868311882,
-0.004542239010334015,
0.0600406639277935,
-0.05053197965025902,
0.01132581289857626,
-0.03540334478020668,
-0.005433158949017525,
0.010333947837352753,
-0.0008545891614630818,
-0.011534915305674076,
-0.004016229882836342,
0.09670275449752808,
0.02483978681266308,
0.01885301060974598,
-0.01507629919797182,
-0.0726688876748085,
0.04384029284119606,
0.02642907202243805,
-0.042403366416692734,
-0.06309284269809723,
0.037893228232860565,
0.06827680766582489,
0.018782049417495728,
0.07695728540420532,
-0.10971008986234665
] |
fhswf/bert_de_ner | 97b17ba2e2bfe2e9d1b8d6e348cb60e0e82fc0b4 | 2021-05-19T16:49:54.000Z | [
"pytorch",
"tf",
"jax",
"bert",
"token-classification",
"de",
"dataset:germeval_14",
"transformers",
"German",
"NER",
"license:cc-by-sa-4.0",
"autotrain_compatible"
] | token-classification | false | fhswf | null | fhswf/bert_de_ner | 2,113 | 2 | transformers | ---
language: de
license: cc-by-sa-4.0
datasets:
- germeval_14
tags:
- German
- de
- NER
---
# BERT-DE-NER
## What is it?
This is a German BERT model fine-tuned for named entity recognition.
## Base model & training
This model is based on [bert-base-german-dbmdz-cased](https://huggingface.co/bert-base-german-dbmdz-cased) and has been fine-tuned
for NER on the training data from [GermEval2014](https://sites.google.com/site/germeval2014ner).
## Model results
The results on the test data from GermEval2014 are (entities only):
| Precision | Recall | F1-Score |
|----------:|-------:|---------:|
| 0.817 | 0.842 | 0.829 |
## How to use
```Python
>>> from transformers import pipeline
>>> classifier = pipeline('ner', model="fhswf/bert_de_ner")
>>> classifier('Von der Organisation „medico international“ hieß es, die EU entziehe sich seit vielen Jahren der Verantwortung für die Menschen an ihren Außengrenzen.')
[{'word': 'med', 'score': 0.9996621608734131, 'entity': 'B-ORG', 'index': 6},
{'word': '##ico', 'score': 0.9995362162590027, 'entity': 'I-ORG', 'index': 7},
{'word': 'international',
'score': 0.9996932744979858,
'entity': 'I-ORG',
'index': 8},
{'word': 'eu', 'score': 0.9997008442878723, 'entity': 'B-ORG', 'index': 14}]
```
| [
-0.1450337916612625,
0.0011599728604778647,
0.016816282644867897,
-0.011684613302350044,
-0.011903299018740654,
0.00879985187202692,
-0.015275263227522373,
0.06008461117744446,
-0.02085556462407112,
-0.04426681995391846,
0.019751595333218575,
-0.058505307883024216,
0.03231952339410782,
0.0667620599269867,
0.03934632986783981,
0.06324216723442078,
0.017521968111395836,
-0.03693562000989914,
-0.08278564363718033,
-0.07232990115880966,
0.0367068387567997,
0.11701085418462753,
0.018856605514883995,
-0.06264292448759079,
0.03001677617430687,
-0.08491818606853485,
-0.041090480983257294,
-0.025934752076864243,
0.06283702701330185,
0.010388032533228397,
0.0471218079328537,
0.04126085713505745,
-0.01346429344266653,
0.10982829332351685,
0.04868793115019798,
0.056781888008117676,
-0.02347508817911148,
-0.028485096991062164,
0.000030180966859916225,
0.0573771633207798,
0.018600931391119957,
-0.03353559225797653,
-0.03637336567044258,
-0.02237313613295555,
0.024968180805444717,
0.06358840316534042,
-0.07118305563926697,
-0.043452825397253036,
-0.031274281442165375,
-0.03409523516893387,
-0.10155423730611801,
0.01533117238432169,
0.055215269327163696,
0.09374126046895981,
0.008769485168159008,
0.04733992740511894,
0.029005955904722214,
-0.09995109587907791,
-0.05868249386548996,
-0.08638838678598404,
-0.08737541735172272,
-0.03298277035355568,
-0.03257261961698532,
-0.048592232167720795,
-0.11071914434432983,
0.04025640711188316,
-0.013488668017089367,
-0.004337646998465061,
0.018719151616096497,
-0.012402091175317764,
-0.011459953151643276,
-0.03880753740668297,
0.024640588089823723,
0.023900577798485756,
0.013369771651923656,
-0.05023892596364021,
0.06920912116765976,
-0.012847798876464367,
0.07349392771720886,
-0.1063196137547493,
-0.019548939540982246,
0.014742324128746986,
0.025818005204200745,
0.01379257533699274,
0.1032024621963501,
-0.014601449482142925,
0.05055639520287514,
-0.0634295791387558,
0.008957924321293831,
0.02010932005941868,
-0.04918333888053894,
-0.12102977186441422,
0.06523195654153824,
0.01126718707382679,
0.0018499717116355896,
0.051344383507966995,
0.07401593774557114,
-0.0035522575490176678,
0.014178876765072346,
0.05029768869280815,
-0.03777443245053291,
0.05802450701594353,
0.01872481405735016,
-0.009457321837544441,
-0.04044539853930473,
0.00965159758925438,
0.02712775208055973,
0.046518873423337936,
0.04206918179988861,
-0.040262944996356964,
0.023753631860017776,
0.018562892451882362,
0.016734983772039413,
-0.0931374803185463,
0.0033513789530843496,
-0.06403972953557968,
-0.01727237179875374,
-0.0036336116027086973,
-0.04797257483005524,
0.002645969158038497,
0.04805921018123627,
-0.015341110527515411,
-0.031274840235710144,
0.06169912591576576,
-0.0015237239422276616,
0.07384799420833588,
-0.04019440338015556,
3.034207875190574e-33,
0.019862450659275055,
-0.0292463768273592,
0.020308412611484528,
-0.015485621057450771,
-0.057905469089746475,
0.041635170578956604,
-0.02545541152358055,
0.07150489091873169,
-0.05743161588907242,
0.02531568333506584,
-0.0845726877450943,
0.02165396325290203,
-0.07356160134077072,
0.017319951206445694,
-0.06240218132734299,
0.04237476736307144,
-0.008314955048263073,
0.0014412258751690388,
-0.0033593543339520693,
0.05741250514984131,
0.11887213587760925,
0.057432930916547775,
0.014771953225135803,
-0.06482835859060287,
-0.026690324768424034,
0.01935308426618576,
0.028803611174225807,
-0.06637132167816162,
-0.06683768332004547,
0.013020242564380169,
-0.06342894583940506,
-0.025419088080525398,
0.011853724718093872,
0.053923074156045914,
-0.0012523450423032045,
0.020928790792822838,
0.002638602163642645,
-0.024209363386034966,
-0.031576007604599,
-0.06184585392475128,
0.007335027679800987,
0.08493602275848389,
0.021235905587673187,
-0.06268013268709183,
-0.03551776334643364,
0.024407552555203438,
0.028477758169174194,
-0.032242242246866226,
0.04680041968822479,
0.03232945129275322,
0.03804272040724754,
0.014642956666648388,
-0.10661505907773972,
0.05562096834182739,
0.03356334567070007,
0.014482011087238789,
0.04475795477628708,
0.04854712262749672,
0.049524810165166855,
-0.007296225056052208,
-0.05459042638540268,
0.02721392549574375,
0.008267439901828766,
-0.013888335786759853,
0.10368423163890839,
-0.06769917905330658,
-0.014559721574187279,
0.010779539123177528,
-0.010335494764149189,
0.022317681461572647,
-0.015674665570259094,
0.011635640636086464,
0.007526572328060865,
0.07837198674678802,
0.016530774533748627,
-0.04061588644981384,
0.02826661802828312,
-0.09886397421360016,
-0.003423372283577919,
0.005719567649066448,
0.018780112266540527,
0.027219397947192192,
-0.07072874158620834,
-0.04449373483657837,
-0.04279659315943718,
-0.008267327211797237,
0.0072075119242072105,
-0.07892660796642303,
-0.04663769528269768,
-0.027896441519260406,
0.006027061026543379,
-0.027585433796048164,
-0.031724922358989716,
-0.026981472969055176,
-0.10979699343442917,
-4.382060370899126e-33,
0.010590489022433758,
0.011219081468880177,
-0.09668184816837311,
0.02384554035961628,
-0.049654271453619,
-0.07539264112710953,
0.07061393558979034,
0.10133211314678192,
-0.04604138806462288,
-0.028288010507822037,
0.04900887981057167,
-0.043489158153533936,
0.027049630880355835,
-0.06522595137357712,
0.09053352475166321,
0.04339887946844101,
-0.07439661771059036,
-0.028900528326630592,
-0.020860949531197548,
0.07979279011487961,
0.009570729918777943,
0.07718803733587265,
-0.14607925713062286,
0.03399296849966049,
-0.058473970741033554,
0.06222105398774147,
-0.02203153818845749,
0.07103011012077332,
0.02379642426967621,
0.0021990081295371056,
-0.04350573942065239,
0.037959951907396317,
-0.041450001299381256,
0.03680099546909332,
-0.052510567009449005,
-0.03673313930630684,
0.017283009365200996,
-0.04055744782090187,
-0.017799878492951393,
-0.052951522171497345,
0.005146485287696123,
0.0840277373790741,
-0.09826033562421799,
0.07646847516298294,
-0.00973267387598753,
0.013157153502106667,
-0.09228106588125229,
-0.026701468974351883,
0.09686283767223358,
-0.047385718673467636,
0.016132008284330368,
-0.04693928733468056,
-0.09082648903131485,
-0.016627108678221703,
-0.06196160614490509,
-0.07741809636354446,
0.04915574938058853,
-0.10361544042825699,
-0.038990918546915054,
0.018148666247725487,
0.003153572091832757,
0.0623321607708931,
0.04109984263777733,
-0.001547692110762,
-0.039424601942300797,
0.027153966948390007,
-0.05621704086661339,
0.08721587806940079,
0.004466851241886616,
-0.03727714717388153,
-0.01416846178472042,
0.02329345978796482,
0.07660380005836487,
-0.0547235831618309,
-0.021547602489590645,
-0.009640064090490341,
-0.031689271330833435,
-0.01780400238931179,
0.01662914641201496,
-0.01708432473242283,
-0.03768272325396538,
-0.05019545182585716,
0.02645377442240715,
0.08456406742334366,
-0.030632296577095985,
0.07800614833831787,
0.05317347124218941,
0.06030355393886566,
0.024183986708521843,
0.000419927469920367,
-0.04250518977642059,
0.035640496760606766,
-0.02725614234805107,
0.13337329030036926,
0.04266432300209999,
-5.8682893211425835e-8,
-0.07829982042312622,
0.030656050890684128,
0.007888298481702805,
-0.0019349526846781373,
-0.023489564657211304,
-0.03732415288686752,
-0.060411155223846436,
0.04490939900279045,
-0.027977094054222107,
0.02291862480342388,
0.04789786413311958,
0.059027038514614105,
-0.160806804895401,
-0.016651790589094162,
0.046684879809617996,
0.06877397745847702,
-0.017434800043702126,
0.0779663473367691,
-0.01629718951880932,
-0.007148479111492634,
-0.00005042965494794771,
0.02275410294532776,
-0.006649096962064505,
-0.04869748651981354,
-0.014628903940320015,
-0.044677332043647766,
-0.026688775047659874,
0.09218285232782364,
0.02782120555639267,
-0.0524790994822979,
-0.028132135048508644,
0.07138990610837936,
0.004699853714555502,
0.04600115865468979,
0.0944119542837143,
0.04606315866112709,
0.012910104356706142,
-0.030055642127990723,
0.0036869505420327187,
0.01923658326268196,
0.07378605008125305,
0.06713476777076721,
-0.1036805808544159,
0.025302104651927948,
0.018677830696105957,
-0.019897690042853355,
-0.008422662504017353,
-0.09625117480754852,
0.0398702472448349,
0.06131383776664734,
0.06596748530864716,
-0.044080425053834915,
-0.05844727158546448,
0.09262026846408844,
-0.07591841369867325,
0.03668595850467682,
-0.06885890662670135,
-0.03654320538043976,
-0.02396848425269127,
0.017171723768115044,
0.006193622946739197,
-0.026516113430261612,
0.032376017421483994,
0.09979848563671112
] |
ixa-ehu/SciBERT-SQuAD-QuAC | df352e10c506e443875447c166a679b6a5ee34e9 | 2021-06-29T22:55:53.000Z | [
"pytorch",
"bert",
"question-answering",
"en",
"arxiv:1808.07036",
"transformers",
"autotrain_compatible"
] | question-answering | false | ixa-ehu | null | ixa-ehu/SciBERT-SQuAD-QuAC | 2,110 | null | transformers | ---
language: en
---
# SciBERT-SQuAD-QuAC
This is the [SciBERT language representation model](https://huggingface.co/allenai/scibert_scivocab_uncased) fine tuned for Question Answering. SciBERT is a pre-trained language model based on BERT that has been trained on a large corpus of scientific text. When fine tuning for Question Answering we combined [SQuAD2.0](https://www.aclweb.org/anthology/P18-2124/) and [QuAC](https://arxiv.org/abs/1808.07036) datasets.
If using this model, please cite the following paper:
```
@inproceedings{otegi-etal-2020-automatic,
title = "Automatic Evaluation vs. User Preference in Neural Textual {Q}uestion{A}nswering over {COVID}-19 Scientific Literature",
author = "Otegi, Arantxa and
Campos, Jon Ander and
Azkune, Gorka and
Soroa, Aitor and
Agirre, Eneko",
booktitle = "Proceedings of the 1st Workshop on {NLP} for {COVID}-19 (Part 2) at {EMNLP} 2020",
month = dec,
year = "2020",
address = "Online",
publisher = "Association for Computational Linguistics",
url = "https://www.aclweb.org/anthology/2020.nlpcovid19-2.15",
doi = "10.18653/v1/2020.nlpcovid19-2.15",
}
```
| [
-0.06901852041482925,
-0.058135416358709335,
-0.01078801229596138,
0.04283185675740242,
0.014553511515259743,
0.05824289843440056,
0.017340855672955513,
0.09025508910417557,
0.037824418395757675,
0.00008996385440696031,
0.0035636054817587137,
-0.03186975419521332,
0.029077300801873207,
0.02993241883814335,
-0.04408359155058861,
0.026729576289653778,
0.0834074467420578,
-0.05751585587859154,
-0.07293263077735901,
-0.012903672643005848,
0.00012173839058959857,
0.04604025557637215,
0.12330760061740875,
-0.03792106732726097,
0.03815753012895584,
-0.06229732930660248,
-0.06661317497491837,
0.0010439350735396147,
0.017377378419041634,
0.04875272884964943,
0.05008934438228607,
0.09468656033277512,
0.022984886541962624,
0.062334123998880386,
-0.002259777393192053,
0.05337768793106079,
-0.04521683230996132,
-0.07192505896091461,
0.031224317848682404,
0.0339946448802948,
-0.07794985920190811,
-0.06238473206758499,
-0.003633475862443447,
0.03976404666900635,
0.1007971242070198,
-0.01833534613251686,
-0.08611445873975754,
-0.00855463556945324,
-0.0031173480674624443,
0.005275609903037548,
-0.12726588547229767,
-0.03408128768205643,
0.05990979075431824,
0.024250878021121025,
-0.009052008390426636,
0.010092326439917088,
-0.008835525251924992,
0.00019927180255763233,
-0.013732275925576687,
-0.06445197016000748,
-0.06192615255713463,
-0.09813179075717926,
-0.09327863156795502,
0.04722234979271889,
-0.0022712352219969034,
-0.0018218006007373333,
-0.03570877015590668,
0.0035208447370678186,
-0.06610766798257828,
0.02014615386724472,
-0.045564647763967514,
0.05158409848809242,
0.007467709947377443,
0.10557402670383453,
0.041355982422828674,
0.03029271960258484,
0.07147552818059921,
-0.019609615206718445,
0.10562647134065628,
-0.12235711514949799,
0.014635373838245869,
-0.046717606484889984,
0.028119897469878197,
0.0012852853396907449,
0.09280329197645187,
-0.00010850306716747582,
0.04422484338283539,
0.017977172508835793,
0.008850856684148312,
0.07749365270137787,
-0.02382197417318821,
-0.059813473373651505,
0.11532679200172424,
-0.031552355736494064,
-0.009471067227423191,
0.07565069198608398,
0.06022424250841141,
-0.014865027740597725,
-0.024024074897170067,
0.05861341208219528,
0.05552436038851738,
0.04132663831114769,
-0.023659395053982735,
-0.013729579746723175,
-0.05417266860604286,
-0.09015221148729324,
0.023331059142947197,
0.025697749108076096,
0.015637869015336037,
-0.1010943055152893,
-0.036074135452508926,
0.025061143562197685,
-0.03348793834447861,
0.002169255632907152,
0.03242452070116997,
-0.02426687255501747,
0.10891003161668777,
0.06035347282886505,
-0.009385311044752598,
0.026827767491340637,
-0.05287191644310951,
-0.029822075739502907,
-0.0065840077586472034,
0.006247972138226032,
0.037505581974983215,
0.024151746183633804,
-0.04921097308397293,
5.015793987340391e-33,
0.07266617566347122,
0.05028748884797096,
-0.0034360988065600395,
0.009521127678453922,
-0.03868936747312546,
0.005258424207568169,
-0.038462989032268524,
0.01759485900402069,
-0.07842908054590225,
-0.05137663334608078,
-0.04153253883123398,
0.053001828491687775,
-0.0049014524556696415,
0.03720174357295036,
-0.015680523589253426,
0.03054451197385788,
-0.06537118554115295,
0.024990040808916092,
-0.05099652707576752,
-0.027275528758764267,
0.08755061030387878,
0.06512804329395294,
0.06576027721166611,
-0.030346881598234177,
0.01841943897306919,
-0.040176596492528915,
0.02226630225777626,
-0.08553826063871384,
-0.06209893897175789,
0.04225527495145798,
-0.12776699662208557,
-0.011824398301541805,
-0.025682616978883743,
0.021420061588287354,
0.011653165332973003,
-0.04728546738624573,
0.007126720622181892,
-0.033223800361156464,
0.041622985154390335,
0.021909285336732864,
0.07431846112012863,
0.03993174061179161,
0.08507014811038971,
-0.019273366779088974,
-0.039380285888910294,
-0.00888929795473814,
0.017998766154050827,
0.03171705827116966,
0.002864065347239375,
-0.03116421401500702,
0.00437333295121789,
0.007705588825047016,
-0.04114563763141632,
-0.017282700166106224,
0.06798209249973297,
0.06032814830541611,
0.019821858033537865,
0.04597492516040802,
-0.026574132964015007,
-0.016064897179603577,
0.0655427798628807,
0.07907362282276154,
0.06518587470054626,
0.0065411836840212345,
0.029333192855119705,
0.0008391712326556444,
-0.03707985579967499,
0.00909416377544403,
0.03800288960337639,
0.07043065875768661,
-0.017908602952957153,
0.0038882873486727476,
-0.056265704333782196,
0.04572875425219536,
0.03351902589201927,
-0.03456276282668114,
0.011792230419814587,
-0.07123135030269623,
0.027928125113248825,
0.026478391140699387,
0.015313825570046902,
-0.015075309202075005,
-0.00904568750411272,
-0.050095416605472565,
-0.06991229206323624,
-0.06356919556856155,
0.009202641434967518,
-0.04858602583408356,
0.016718363389372826,
-0.07239807397127151,
0.07409384846687317,
-0.10068535804748535,
0.0035816021263599396,
-0.019197478890419006,
-0.07853911072015762,
-4.732675805602859e-33,
-0.03550553694367409,
-0.07134586572647095,
-0.07615657150745392,
0.07722105085849762,
-0.012255373410880566,
-0.0569213330745697,
0.004783547017723322,
0.04522019624710083,
0.03345140814781189,
-0.04607982933521271,
0.05066501349210739,
-0.04718766361474991,
0.03850648179650307,
-0.05700775235891342,
0.056532349437475204,
-0.040949638932943344,
-0.0723058357834816,
-0.0644955188035965,
-0.006917272228747606,
0.06063671410083771,
-0.013892000541090965,
0.0019346887711435556,
-0.035468313843011856,
0.06418201327323914,
0.011094342917203903,
0.044798944145441055,
0.012922306545078754,
0.028674719855189323,
-0.03323015198111534,
0.005186343565583229,
-0.03566717728972435,
-0.027076415717601776,
-0.08574675768613815,
-0.03136393055319786,
-0.037181612104177475,
0.09471778571605682,
0.0535995177924633,
-0.06355340778827667,
-0.03331856429576874,
0.12009155005216599,
0.021748796105384827,
0.07538386434316635,
-0.11495897173881531,
-0.021540112793445587,
-0.03769627586007118,
0.004196351859718561,
-0.10536400973796844,
-0.04975072667002678,
-0.03761148452758789,
0.022697070613503456,
0.016463054344058037,
0.020952872931957245,
-0.03684038296341896,
0.02550004981458187,
-0.08907066285610199,
-0.07626155763864517,
0.019064322113990784,
-0.03189340978860855,
-0.03175738453865051,
0.05575399845838547,
-0.02529818005859852,
0.04967145249247551,
-0.029326865449547768,
0.008240332826972008,
-0.0055109248496592045,
-0.04917418956756592,
-0.029902968555688858,
0.09813512116670609,
0.023746542632579803,
-0.07720097154378891,
0.06378747522830963,
-0.13495750725269318,
0.01959805004298687,
0.008176769129931927,
-0.0022435046266764402,
0.028952931985259056,
0.025426842272281647,
-0.08124198019504547,
0.03128486126661301,
-0.036162637174129486,
-0.057825956493616104,
0.007663023192435503,
0.06118588149547577,
0.10131505876779556,
0.017735304310917854,
0.05223136767745018,
-0.04059615731239319,
0.07524228096008301,
-0.02464020438492298,
0.042021531611680984,
0.058980222791433334,
-0.05540647730231285,
0.00866248644888401,
0.10519278049468994,
-0.05298943817615509,
-6.516202688544581e-8,
-0.010229193605482578,
0.023071009665727615,
-0.008290794678032398,
0.08108539879322052,
0.013594143092632294,
-0.07148587703704834,
-0.0814819484949112,
0.02033965475857258,
-0.04160086438059807,
0.021001847460865974,
0.017134029418230057,
0.0546683669090271,
-0.04588925093412399,
-0.04890477657318115,
0.01616809330880642,
0.10039585083723068,
0.015098470263183117,
0.047215674072504044,
-0.04326315596699715,
-0.03654177859425545,
0.08814167231321335,
0.051073186099529266,
-0.04590578377246857,
-0.05274587869644165,
0.028595928102731705,
0.02308981865644455,
-0.14301516115665436,
0.026806335896253586,
-0.025805536657571793,
-0.005224677734076977,
-0.03262832760810852,
-0.004095448646694422,
-0.09283451735973358,
-0.026145078241825104,
0.07267099618911743,
0.08529718965291977,
-0.022162189707159996,
-0.08752641826868057,
0.015033051371574402,
0.07746198028326035,
0.0737384632229805,
0.03740730136632919,
-0.07049459964036942,
0.04138309881091118,
0.07066148519515991,
0.027254333719611168,
-0.0032031473238021135,
-0.08308529853820801,
0.07083586603403091,
-0.02220379002392292,
0.06684765219688416,
-0.037168268114328384,
-0.03802787885069847,
0.05232224613428116,
-0.04478336125612259,
0.04055071994662285,
-0.05696208029985428,
-0.015286040492355824,
0.012421296909451485,
-0.010877064429223537,
0.013773469254374504,
0.032156817615032196,
0.027042368426918983,
-0.0059589664451777935
] |
hf-internal-testing/tiny-random-blenderbot | 9432cd260adf10352afc43e7080b154ca0313105 | 2021-09-17T19:25:13.000Z | [
"pytorch",
"tf",
"blenderbot",
"transformers"
] | null | false | hf-internal-testing | null | hf-internal-testing/tiny-random-blenderbot | 2,106 | null | transformers | Entry not found | [
0.0461147278547287,
-0.038838207721710205,
-0.01049656979739666,
-0.03682169318199158,
0.011261860840022564,
0.013094935566186905,
0.0019101888174191117,
-0.013979103416204453,
0.027092741802334785,
-0.015212527476251125,
0.017284274101257324,
-0.08189476281404495,
0.03817418962717056,
-0.04920130595564842,
0.021389011293649673,
-0.015245908871293068,
-0.03203780576586723,
-0.1245758980512619,
0.03150877356529236,
0.032381657510995865,
-0.060957908630371094,
0.05409295856952667,
-0.025087490677833557,
0.01568586938083172,
0.028129950165748596,
-0.04710396006703377,
-0.018688226118683815,
0.013785239309072495,
-0.04001208767294884,
0.01173911802470684,
-0.04317743331193924,
0.05500618368387222,
0.004543041344732046,
0.02973111905157566,
0.14852192997932434,
0.02658126689493656,
0.02907961793243885,
-0.05169107764959335,
0.05803573504090309,
-0.07732241600751877,
-0.017637968063354492,
-0.04219653457403183,
0.041807834059000015,
0.023620979860424995,
0.021563321352005005,
0.016478516161441803,
-0.0021814992651343346,
-0.06400240957736969,
0.06393089145421982,
0.019599027931690216,
-0.08565037697553635,
0.00934905931353569,
-0.008718925528228283,
-0.028583496809005737,
-0.07310017943382263,
0.09416428208351135,
0.001759322709403932,
0.06184990331530571,
0.011840506456792355,
-0.035997264087200165,
0.08358278125524521,
-0.02619801089167595,
0.03736566752195358,
-0.028206506744027138,
-0.07454850524663925,
-0.08883563429117203,
-0.06279942393302917,
-0.008695344440639019,
0.014119276776909828,
-0.0825355276465416,
0.0649217739701271,
-0.00223911227658391,
-0.14716917276382446,
0.07743025571107864,
-0.03548373281955719,
-0.055201586335897446,
0.006981803569942713,
-0.012166670523583889,
0.055111464112997055,
-0.007116836030036211,
-0.023175746202468872,
-0.005835152696818113,
-0.09185640513896942,
0.055196937173604965,
0.034148022532463074,
0.03835180774331093,
0.038685429841279984,
-0.025987252593040466,
0.017804903909564018,
0.022428328171372414,
0.025005368515849113,
-0.10761535167694092,
-0.048001550137996674,
-0.04343584179878235,
0.012374646961688995,
-0.019502125680446625,
0.029218152165412903,
0.0842173621058464,
-0.011719699949026108,
0.09283553808927536,
-0.007015465293079615,
-0.03543110564351082,
-0.06936459988355637,
0.09425332397222519,
-0.010958523489534855,
-0.00805904995650053,
0.004974212497472763,
-0.0031528924591839314,
0.06105927750468254,
-0.03964288905262947,
-0.03619541600346565,
-0.019901901483535767,
0.07134733349084854,
0.039514873176813126,
-0.012729483656585217,
-0.006646515801548958,
-0.04746140539646149,
-0.014432490803301334,
-0.05157482624053955,
0.09506245702505112,
-0.049747664481401443,
-0.04591796174645424,
-0.008965466171503067,
-0.0325421579182148,
-0.08626784384250641,
-0.06624380499124527,
0.02538885548710823,
-4.303924894057984e-33,
0.01133066974580288,
0.0033434738870710135,
-0.002155609894543886,
0.04871906340122223,
-0.023564351722598076,
-0.07933273911476135,
0.0600903145968914,
0.02335330657660961,
-0.03844716399908066,
-0.020433755591511726,
-0.06952055543661118,
-0.03235611692070961,
0.0062485747039318085,
0.064804308116436,
-0.03201229125261307,
0.061689723283052444,
0.0417000837624073,
-0.00761845987290144,
0.03340127319097519,
-0.047770582139492035,
0.00887306872755289,
-0.04066338762640953,
-0.010506896302103996,
0.0106519665569067,
0.021333497017621994,
0.12854498624801636,
-0.009705503471195698,
0.010055632330477238,
-0.017507633194327354,
0.006515394430607557,
0.06334009766578674,
-0.057817306369543076,
0.013668818399310112,
-0.020286159589886665,
0.05430467426776886,
-0.023184705525636673,
0.0828516036272049,
0.0005449643940664828,
-0.10372652113437653,
-0.07634282112121582,
-0.005381610710173845,
-0.039263784885406494,
0.0006114727002568543,
-0.013281986117362976,
0.07119110971689224,
0.043696220964193344,
0.03168422728776932,
0.04338686540722847,
0.05728672817349434,
0.0832006186246872,
-0.07961414009332657,
0.015234283171594143,
0.017002005130052567,
0.047004107385873795,
-0.09794387966394424,
0.004990279674530029,
-0.07062993198633194,
-0.028000490739941597,
-0.04018733277916908,
-0.0702052190899849,
0.011351344175636768,
0.06020182743668556,
-0.03297270089387894,
0.09396500885486603,
0.03417910635471344,
-0.019825750961899757,
-0.034690454602241516,
-0.013036907650530338,
0.05896938592195511,
-0.012359356507658958,
-0.017275206744670868,
-0.07982361316680908,
0.02059139870107174,
0.06737419217824936,
0.04176458343863487,
-0.04978838190436363,
-0.05877475067973137,
-0.06289287656545639,
-0.03354167565703392,
-0.03871942684054375,
0.009898529388010502,
-0.05514208599925041,
-0.11629002541303635,
-0.011855563148856163,
0.10663620382547379,
0.037354156374931335,
-0.0065480442717671394,
-0.051189567893743515,
0.06663123518228531,
0.01874656230211258,
0.032841797918081284,
0.041593004018068314,
-0.06879369914531708,
0.04216769337654114,
-0.01628219522535801,
5.4139394340936695e-34,
0.05697013810276985,
-0.006972255185246468,
0.015711724758148193,
-0.17956365644931793,
0.02320219948887825,
0.007923615165054798,
-0.008062449283897877,
0.0074974060989916325,
0.07391711324453354,
0.0309313777834177,
0.060510627925395966,
0.058605875819921494,
0.09515274316072464,
-0.002282935893163085,
0.001603541080839932,
0.07024981826543808,
0.012629246339201927,
0.07425693422555923,
-0.038426291197538376,
0.01861148327589035,
0.030608950182795525,
-0.02449394389986992,
0.021528491750359535,
-0.003039651783183217,
-0.03676343336701393,
0.03130284696817398,
0.07998586446046829,
0.010451192036271095,
-0.07930229604244232,
-0.013543923385441303,
0.018781835213303566,
0.05168003588914871,
-0.07191970944404602,
0.15783067047595978,
0.026191607117652893,
0.01262354850769043,
0.08218053728342056,
-0.029807550832629204,
-0.07528624683618546,
-0.04250097647309303,
0.017244765534996986,
0.04411793500185013,
0.03708017244935036,
0.009233047254383564,
-0.040271829813718796,
0.022496428340673447,
0.02495843544602394,
0.07633638381958008,
0.005147108342498541,
0.013892097398638725,
0.05610476806759834,
-0.06684739887714386,
0.05862557515501976,
-0.020688841119408607,
0.05377643182873726,
0.06718500703573227,
0.005329249892383814,
-0.01388032827526331,
0.029931528493762016,
0.009508464485406876,
-0.045173756778240204,
0.11534366756677628,
-0.06510116159915924,
0.05117698386311531,
-0.0026125339791178703,
-0.08554837852716446,
-0.03784770518541336,
0.0804959163069725,
0.011298024095594883,
-0.07695550471544266,
-0.04868878796696663,
0.02515520341694355,
0.06252261996269226,
-0.04509226232767105,
-0.01246943511068821,
0.028559505939483643,
-0.030573077499866486,
0.05066261067986488,
-0.08187384903430939,
0.04469604790210724,
0.0034051244147121906,
0.04145054519176483,
-0.021858664229512215,
-0.06112268194556236,
-0.00908052921295166,
-0.05903250351548195,
0.0259539932012558,
0.059690944850444794,
-0.07613514363765717,
-0.03720718249678612,
-0.036316655576229095,
0.07058046013116837,
-0.008224100805819035,
0.041961874812841415,
-0.0285952128469944,
-1.496900736697171e-8,
-0.0014124972512945533,
0.03401879221200943,
-0.040338415652513504,
0.04116074740886688,
0.0935964286327362,
-0.05115952715277672,
0.0008746005478315055,
-0.03389839455485344,
-0.00567849725484848,
-0.010686947964131832,
-0.04789939522743225,
-0.04820054769515991,
-0.02011880651116371,
-0.03209094703197479,
-0.04211259260773659,
-0.10229527950286865,
-0.07819421589374542,
-0.031228765845298767,
-0.02154778689146042,
-0.04960230365395546,
0.08087796717882156,
-0.07801242172718048,
0.06919731199741364,
-0.04999840259552002,
0.03687043860554695,
0.03889009356498718,
-0.049989692866802216,
-0.04254625365138054,
-0.04606937617063522,
0.08682432025671005,
-0.031148413196206093,
0.11826753616333008,
0.034102488309144974,
-0.0208592489361763,
-0.0205202866345644,
0.027134142816066742,
0.09741277992725372,
0.051608603447675705,
0.013477512635290623,
-0.13649295270442963,
-0.022304272279143333,
0.02385953813791275,
0.038732077926397324,
-0.09249968826770782,
-0.04549082741141319,
0.054220106452703476,
0.01160438358783722,
0.051190607249736786,
0.07713303714990616,
-0.022097084671258926,
-0.06127818301320076,
-0.01857956498861313,
0.006740490905940533,
-0.00496308971196413,
0.024095389991998672,
0.0736224576830864,
-0.003481915919110179,
-0.0699305310845375,
-0.006629763171076775,
-0.0598808117210865,
0.05297163128852844,
-0.02902800403535366,
-0.027858933433890343,
-0.01287526823580265
] |
JamesStratford/PLord-bot-DialoGPT-medium | a1f8100aa348ae0b41363d6089d81529e0ac3484 | 2022-07-08T01:37:51.000Z | [
"pytorch",
"gpt2",
"text-generation",
"transformers",
"conversational"
] | conversational | false | JamesStratford | null | JamesStratford/PLord-bot-DialoGPT-medium | 2,105 | null | transformers | ---
tags:
- conversational
---
# PlordBot - medium | [
-0.04313283413648605,
-0.04160225763916969,
0.04087880998849869,
-0.045091647654771805,
0.07174453139305115,
-0.041430581361055374,
0.0829135999083519,
0.03301629424095154,
0.02080393396317959,
-0.00828311126679182,
0.01817755587399006,
0.033393509685993195,
0.013027019798755646,
-0.015865495428442955,
0.018745267763733864,
0.08349945396184921,
0.006186053156852722,
0.04667951539158821,
-0.031178662553429604,
0.041237059980630875,
0.033772487193346024,
0.09600096940994263,
0.05562791973352432,
-0.0022842923644930124,
0.032405633479356766,
0.06388604640960693,
-0.054884035140275955,
0.03347901627421379,
0.05087852478027344,
-0.04990902543067932,
0.01380529347807169,
0.07669062912464142,
0.03483236953616142,
0.08160898089408875,
0.005929930135607719,
0.05389407277107239,
0.018466847017407417,
-0.018030911684036255,
-0.018547365441918373,
0.0152271818369627,
-0.00762847950682044,
-0.01812729611992836,
-0.0496022067964077,
-0.010462392121553421,
-0.03605033457279205,
-0.0055463616736233234,
-0.06591929495334625,
0.027924221009016037,
-0.0643249899148941,
0.03438003733754158,
-0.09674236923456192,
-0.06215880438685417,
-0.03462904691696167,
0.1047007367014885,
0.030642611905932426,
0.009925343096256256,
-0.06723286956548691,
-0.04542845860123634,
0.09204604476690292,
-0.03448764607310295,
-0.09020587801933289,
-0.008489192463457584,
-0.035563696175813675,
0.018451817333698273,
-0.005261031445115805,
0.009178921580314636,
-0.07630787789821625,
0.0016043306095525622,
-0.07036980986595154,
0.10531698167324066,
0.06269706785678864,
0.03335189074277878,
0.07016286998987198,
0.03700166940689087,
0.003932265564799309,
-0.04463569074869156,
-0.023946786299347878,
-0.0189229603856802,
-0.019094539806246758,
0.0190241988748312,
-0.05902250483632088,
-0.06341154128313065,
-0.02644648589193821,
0.03636057302355766,
-0.00762128084897995,
-0.06220085918903351,
0.005618833005428314,
0.009782206267118454,
-0.01793617196381092,
-0.00032808579271659255,
-0.033817414194345474,
-0.0777154341340065,
0.02570684440433979,
0.059262312948703766,
-0.03511837497353554,
0.04706582427024841,
-0.05140673741698265,
-0.08806908130645752,
-0.053650036454200745,
0.12109383195638657,
0.015257788822054863,
0.007915554568171501,
0.0008745508384890854,
-0.07500199228525162,
-0.03857987001538277,
-0.010211032815277576,
-0.007494102697819471,
0.09243235737085342,
0.00925231259316206,
-0.01551008690148592,
-0.02356821298599243,
-0.05237602815032005,
0.00493909465149045,
-0.020904112607240677,
0.07803452759981155,
-0.02911968342959881,
0.0495564229786396,
0.030489329248666763,
0.06021743640303612,
-0.07368896156549454,
0.017409512773156166,
0.029519394040107727,
-0.07962227612733841,
-0.05593256279826164,
0.031155619770288467,
-0.0027531636878848076,
-0.00860453862696886,
-2.8926713758538627e-33,
0.06331020593643188,
-0.0333343930542469,
0.03956687077879906,
0.060348134487867355,
0.011538404040038586,
0.07450229674577713,
-0.05916151404380798,
-0.039875876158475876,
-0.01995883882045746,
0.02399887517094612,
0.05076562985777855,
0.03448718041181564,
-0.09862754493951797,
0.05844118818640709,
0.03554575890302658,
-0.08776186406612396,
0.05111958086490631,
0.04426843672990799,
-0.02777218259871006,
-0.00557135883718729,
0.005403218790888786,
0.045995257794857025,
-0.00999747309833765,
0.06982173770666122,
0.03793514892458916,
0.04786751791834831,
0.012801125645637512,
-0.14346542954444885,
0.06381922215223312,
0.037601910531520844,
-0.07381734251976013,
0.013090967200696468,
-0.02316577546298504,
0.015048859640955925,
-0.01851554587483406,
0.027041254565119743,
-0.05316038802266121,
-0.012964760884642601,
-0.034430913627147675,
-0.046351730823516846,
-0.012797934003174305,
-0.0448620542883873,
-0.12304449826478958,
-0.08014700561761856,
0.0648949146270752,
0.016647567972540855,
0.00305448891595006,
0.03979835286736488,
0.03765561431646347,
-0.030301684513688087,
-0.053205445408821106,
0.08519619703292847,
0.07681815326213837,
0.0043378411792218685,
-0.02651546150445938,
-0.06391116976737976,
-0.019455788657069206,
0.03257804736495018,
-0.02978668361902237,
-0.08876491338014603,
0.02342873439192772,
0.014751886948943138,
0.08701319992542267,
-0.06478018313646317,
0.10436258465051651,
-0.04467591643333435,
-0.03367778658866882,
0.013828293420374393,
0.04806333780288696,
-0.01921556517481804,
-0.04344015195965767,
0.11011387407779694,
-0.05583088472485542,
0.048086244612932205,
-0.028172580525279045,
-0.0355127714574337,
-0.035845715552568436,
-0.019603600725531578,
0.08246272802352905,
0.03877878561615944,
-0.07700517028570175,
-0.07079043984413147,
-0.04077892750501633,
-0.06151978671550751,
-0.05164239555597305,
-0.01784391887485981,
-0.027099767699837685,
-0.07059749960899353,
0.07139880955219269,
0.0009629568085074425,
-0.012687748298048973,
-0.0010961440857499838,
-0.043474409729242325,
-0.009047236293554306,
-0.11157678067684174,
1.0740609432898323e-33,
-0.03445877879858017,
-0.05311722308397293,
-0.029002543538808823,
0.13520529866218567,
-0.005849218461662531,
-0.01981060393154621,
-0.03505171090364456,
0.13648852705955505,
-0.021724224090576172,
0.04112900048494339,
-0.07460111379623413,
-0.01946892961859703,
-0.053881675004959106,
-0.021937986835837364,
0.13972386717796326,
0.08721643686294556,
-0.010320466943085194,
-0.02035439759492874,
0.035142358392477036,
-0.02029038406908512,
0.03684871271252632,
-0.003119342029094696,
-0.09010401368141174,
0.08944611251354218,
0.056877363473176956,
-0.006127126980572939,
0.04530029743909836,
0.06195075809955597,
0.03634311631321907,
-0.04159870743751526,
0.029789503663778305,
0.03780251368880272,
0.025441735982894897,
-0.04863794520497322,
0.03383972868323326,
-0.03373942896723747,
-0.0019403640180826187,
0.04954298958182335,
0.014099223539233208,
0.07743095606565475,
0.05813658982515335,
0.03002803772687912,
0.010772084817290306,
-0.048058636486530304,
-0.026022523641586304,
-0.06840336322784424,
-0.03163925185799599,
-0.05980297550559044,
-0.03339596465229988,
0.020500652492046356,
0.023997459560632706,
0.004072265699505806,
-0.002563609043136239,
-0.16912735998630524,
-0.03591238334774971,
-0.02276846580207348,
-0.023406216874718666,
-0.02748725563287735,
-0.059865664690732956,
-0.040014754980802536,
-0.05246802046895027,
-0.08874092251062393,
-0.02711201272904873,
0.010101770982146263,
0.04088839143514633,
0.006915257312357426,
-0.009949376806616783,
-0.007277755998075008,
-0.04665271192789078,
-0.05895695090293884,
0.13660039007663727,
-0.014903857372701168,
-0.039945561438798904,
0.050385482609272,
0.029298286885023117,
0.020337536931037903,
0.03019564598798752,
-0.0025098947808146477,
0.02522548846900463,
-0.01441190391778946,
-0.02331802435219288,
-0.014164146035909653,
-0.0018464932218194008,
0.08584216237068176,
-0.003211260074749589,
0.05025819316506386,
0.05795006826519966,
0.014712341129779816,
-0.08288362622261047,
0.011810963042080402,
0.00355873117223382,
-0.050288956612348557,
0.0674135610461235,
0.05189491808414459,
-0.05234120413661003,
-2.4953299870844603e-8,
-0.03894346207380295,
-0.11586662381887436,
-0.03727314621210098,
0.06024365499615669,
0.014339235611259937,
0.015810180455446243,
0.029930641874670982,
-0.033794887363910675,
-0.08233433961868286,
-0.01337473001331091,
0.046747904270887375,
0.026622537523508072,
0.0131310373544693,
0.05292865261435509,
0.11242596060037613,
0.034408070147037506,
0.00783493835479021,
-0.036073457449674606,
-0.03190802410244942,
-0.1103157103061676,
0.024918880313634872,
-0.04045123979449272,
-0.04475599154829979,
0.08351725339889526,
0.0007543300744146109,
0.04622289538383484,
-0.034268271178007126,
0.07365845143795013,
-0.008705602958798409,
0.10088662058115005,
-0.0035546335857361555,
0.05150110647082329,
-0.09449728578329086,
-0.005881614983081818,
-0.052803944796323776,
0.02730274200439453,
0.0051611377857625484,
-0.045266710221767426,
0.007166053168475628,
-0.036327797919511795,
0.015325046144425869,
0.06061559170484543,
0.056887026876211166,
-0.04885935038328171,
0.0649125874042511,
-0.04149846360087395,
-0.04497548192739487,
-0.0798642635345459,
-0.016346033662557602,
-0.058122459799051285,
-0.05668391287326813,
-0.0034402674064040184,
0.029785223305225372,
0.020852504298090935,
0.038247525691986084,
0.059048064053058624,
0.032019153237342834,
0.003650030354037881,
0.006689437665045261,
0.002281665103510022,
0.07600861042737961,
0.10169439762830734,
0.02954547107219696,
-0.0006524836062453687
] |
ktrapeznikov/gpt2-medium-topic-news | d079f5fb6ab7eaf5a38dc2a72bd708a60879d23c | 2021-05-23T06:18:56.000Z | [
"pytorch",
"jax",
"gpt2",
"text-generation",
"en",
"transformers"
] | text-generation | false | ktrapeznikov | null | ktrapeznikov/gpt2-medium-topic-news | 2,101 | 1 | transformers | ---
language:
- en
thumbnail:
widget:
- text: "topic: climate article:"
---
# GPT2-medium-topic-news
## Model description
GPT2-medium fine tuned on a large news corpus conditioned on a topic
## Intended uses & limitations
#### How to use
To generate a news article text conditioned on a topic, prompt model with:
`topic: climate article:`
The following tags were used during training:
`arts law international science business politics disaster world conflict football sport sports artanddesign environment music film lifeandstyle business health commentisfree books technology media education politics travel stage uk society us money culture religion science news tv fashion uk australia cities global childrens sustainable global voluntary housing law local healthcare theguardian`
Zero shot generation works pretty well as long as `topic` is a single word and not too specific.
```python
device = "cuda:0"
tokenizer = AutoTokenizer.from_pretrained("ktrapeznikov/gpt2-medium-topic-news")
model = AutoModelWithLMHead.from_pretrained("ktrapeznikov/gpt2-medium-topic-news")
model.to(device)
topic = "climate"
prompt = tokenizer(f"topic: {topic} article:", return_tensors="pt")
out = model.generate(prompt["input_ids"].to(device), do_sample=True,max_length=500, early_stopping=True, top_p=.9)
print(tokenizer.decode(list(out.cpu()[0])))
```
## Training data
## Training procedure
| [
0.009488138370215893,
0.0065520526841282845,
-0.025096198543906212,
0.07207126170396805,
0.10381163656711578,
0.012702981941401958,
0.015589464455842972,
0.00575883686542511,
-0.02551005221903324,
-0.007964757271111012,
-0.04055188596248627,
-0.07502543181180954,
0.061210304498672485,
0.05674389749765396,
0.07635200023651123,
-0.005854147486388683,
0.006633337587118149,
-0.013100913725793362,
-0.08392887562513351,
-0.046790711581707,
0.006799222901463509,
0.1235072985291481,
0.07493072748184204,
0.040782079100608826,
-0.02939329855144024,
-0.041229233145713806,
-0.023949595168232918,
-0.019068587571382523,
-0.033576007932424545,
0.03426738083362579,
0.024836814031004906,
0.045061953365802765,
-0.025277772918343544,
0.08005135506391525,
0.051618218421936035,
0.04687198996543884,
-0.0741216242313385,
-0.009535633958876133,
-0.05710316821932793,
0.038197048008441925,
0.04721023142337799,
-0.04976831004023552,
-0.04219986870884895,
0.0027467762120068073,
0.04161704704165459,
-0.002885950729250908,
-0.07015284150838852,
0.006187022663652897,
-0.01642766036093235,
0.014994822442531586,
-0.10155262798070908,
-0.0919560045003891,
0.012157452292740345,
-0.00427278783172369,
0.009930236265063286,
0.013433193787932396,
-0.026030054315924644,
-0.015071903355419636,
0.04115820676088333,
-0.11447448283433914,
-0.026510436087846756,
-0.021207217127084732,
-0.1282014101743698,
-0.007611769717186689,
-0.0002011714968830347,
-0.038783784955739975,
-0.025113031268119812,
0.1268962025642395,
0.031232506036758423,
0.003425436792895198,
-0.01788819208741188,
0.11760100722312927,
0.029643269255757332,
0.08173341304063797,
-0.003256851341575384,
-0.05982309207320213,
-0.01618618331849575,
-0.02093225158751011,
0.03184029459953308,
-0.03934626281261444,
0.07004331052303314,
-0.07088952511548996,
0.03852178901433945,
-0.04285694286227226,
0.04348580166697502,
-0.07467565685510635,
0.017662975937128067,
0.006881895009428263,
0.026981612667441368,
0.04225040227174759,
-0.03846622258424759,
-0.03855802118778229,
0.15932589769363403,
0.0016665186267346144,
0.018406778573989868,
0.03627524524927139,
0.04148183763027191,
-0.07775271683931351,
-0.010751810856163502,
0.04567906633019447,
0.028903312981128693,
-0.07023303955793381,
-0.029468713328242302,
0.051532015204429626,
-0.03049449622631073,
-0.11037097871303558,
-0.05167493224143982,
0.0017118671676144004,
-0.036544203758239746,
0.01047645602375269,
-0.007142489776015282,
0.06745579838752747,
-0.04858258366584778,
-0.04793421924114227,
0.06667101383209229,
0.056658241897821426,
0.05409734696149826,
-0.03112952411174774,
0.026898019015789032,
0.0674879401922226,
-0.08693525940179825,
0.02184356562793255,
-0.06227043643593788,
0.009958039969205856,
0.005502647720277309,
-0.0004351568641141057,
-0.00981956347823143,
1.2701504456750768e-32,
0.035437390208244324,
-0.012948586605489254,
-0.002290565986186266,
0.0713779628276825,
-0.019581254571676254,
0.039718613028526306,
-0.07636535912752151,
-0.06217338889837265,
0.016747698187828064,
-0.09813268482685089,
-0.007926709949970245,
0.09483583271503448,
-0.08435095846652985,
0.0763787105679512,
0.02741973288357258,
-0.03955693170428276,
-0.08784037828445435,
0.05720079317688942,
-0.02594960853457451,
0.05522531270980835,
0.012100516818463802,
0.03334914892911911,
-0.016898222267627716,
-0.019239794462919235,
-0.06029589846730232,
0.0691618099808693,
0.051068928092718124,
-0.07071541994810104,
0.01120743341743946,
0.034729134291410446,
-0.08638709783554077,
-0.021689385175704956,
-0.0025801712181419134,
0.033963289111852646,
-0.007820484228432178,
0.004503276199102402,
0.0012463132152333856,
-0.04550952464342117,
0.023645050823688507,
-0.06227818876504898,
-0.026761572808027267,
-0.01889919303357601,
0.03677893429994583,
-0.10048747062683105,
0.009560995735228062,
0.014741136692464352,
0.012861308641731739,
0.025187013670802116,
-0.03207812085747719,
0.03242645412683487,
0.0254532303661108,
-0.013550221920013428,
0.011185119859874249,
-0.02418617345392704,
-0.02917446568608284,
0.05929120257496834,
0.04527118057012558,
-0.010175504721701145,
0.05283467099070549,
-0.10696178674697876,
0.03640696033835411,
0.025540119037032127,
0.10893899202346802,
-0.01376871857792139,
0.10279928147792816,
0.04428223893046379,
0.019101852551102638,
0.07532569020986557,
0.011540552601218224,
0.018089119344949722,
-0.024458294734358788,
-0.021352648735046387,
-0.05164705589413643,
-0.0036021831911057234,
0.0467122346162796,
0.024734295904636383,
0.0042083910666406155,
-0.089357890188694,
-0.11139824986457825,
0.07169760763645172,
-0.04067840054631233,
0.0014124445151537657,
0.0024497387930750847,
-0.07132549583911896,
-0.004182968754321337,
-0.06028362736105919,
0.058949194848537445,
-0.0548764169216156,
-0.0029384936206042767,
-0.0504123717546463,
-0.05604509264230728,
-0.011436198838055134,
0.007047116290777922,
0.04651431366801262,
-0.026241036131978035,
-1.4282725090863184e-32,
0.03415021300315857,
-0.05478615313768387,
-0.00997766200453043,
0.03143489733338356,
0.055193230509757996,
-0.04327267408370972,
-0.02737882360816002,
0.008682243525981903,
0.06679307669401169,
-0.021100936457514763,
0.04462733119726181,
-0.09287756681442261,
-0.090053491294384,
0.002417664509266615,
0.059116292744874954,
-0.03830664977431297,
-0.06991376727819443,
0.046654343605041504,
-0.04447666183114052,
0.07902225106954575,
-0.08028317987918854,
-0.03215324878692627,
-0.15774983167648315,
0.07345358282327652,
0.0009619955671951175,
-0.03109915927052498,
0.03326764330267906,
0.02916664630174637,
0.03906740993261337,
0.03668172284960747,
-0.07304582744836807,
0.06491599231958389,
-0.06868213415145874,
0.03146210312843323,
-0.056114595383405685,
0.02182876132428646,
0.0747719332575798,
-0.07591444253921509,
-0.014185115694999695,
0.046661000698804855,
0.13283035159111023,
0.0013479443732649088,
-0.05600273981690407,
-0.003131620120257139,
-0.08535599708557129,
0.001764540676958859,
-0.024604232981801033,
0.023257730528712273,
0.044988229870796204,
-0.014965836890041828,
0.06683275103569031,
-0.07377282530069351,
-0.024297185242176056,
0.00994040071964264,
-0.08192918449640274,
-0.03036336414515972,
-0.00831137876957655,
-0.05967749282717705,
-0.0959310531616211,
-0.01733669824898243,
-0.0637846365571022,
-0.01148079615086317,
-0.014073807746171951,
-0.10771017521619797,
-0.0017442228272557259,
-0.15344366431236267,
-0.053764067590236664,
0.009961357340216637,
-0.009892771951854229,
0.010134226642549038,
-0.031025908887386322,
0.0515412911772728,
0.021881019696593285,
-0.07657312601804733,
-0.03147785738110542,
0.0893687829375267,
-0.02709575928747654,
0.0023553550709038973,
0.03341865539550781,
-0.010676993988454342,
0.00025268096942454576,
0.04969426989555359,
0.008525731973350048,
0.030653435736894608,
0.06652796268463135,
0.01902846060693264,
-0.0023836668115109205,
0.08411276340484619,
-0.01871579699218273,
0.00876529049128294,
0.022372622042894363,
-0.05488405376672745,
0.0580771341919899,
0.13879112899303436,
-0.08812198787927628,
-6.881000302882967e-8,
-0.026240242645144463,
-0.028721584007143974,
-0.036252461373806,
0.06395704299211502,
-0.07309965044260025,
0.00767397228628397,
-0.02091323211789131,
-0.08507980406284332,
-0.0018874173983931541,
-0.0033422918058931828,
-0.04257836192846298,
-0.012820418924093246,
-0.060689814388751984,
0.01929948478937149,
-0.04850860685110092,
0.014830980449914932,
-0.05083595588803291,
0.02765987440943718,
0.0014258221490308642,
-0.06061160936951637,
0.03961741924285889,
-0.07361102104187012,
-0.018786631524562836,
0.0026557394303381443,
0.03828426077961922,
0.038259465247392654,
-0.06532659381628036,
0.0011314692674204707,
0.03420571610331535,
-0.014049521647393703,
0.004365027882158756,
-0.052473410964012146,
-0.12264098972082138,
0.009067694656550884,
0.040084224194288254,
0.04935987666249275,
0.13236553966999054,
-0.04107099771499634,
-0.0066778529435396194,
-0.02152564562857151,
0.03696108236908913,
0.0005410690209828317,
-0.06753835827112198,
-0.0051073371432721615,
0.02023840881884098,
0.009953799657523632,
-0.031754158437252045,
-0.0056160083040595055,
0.022520780563354492,
0.025119779631495476,
-0.06564047932624817,
-0.03990660980343819,
0.021120542660355568,
-0.014254343695938587,
0.013633846305310726,
0.05982503667473793,
0.00558189582079649,
0.054307419806718826,
-0.004668236710131168,
-0.01138350646942854,
0.04581489786505699,
0.004231890197843313,
0.007121887058019638,
0.03757820278406143
] |
Qishuai/distilbert_punctuator_en | 3b5050a2775440ef59b76082ad75eb9574973ad3 | 2021-12-13T14:47:49.000Z | [
"pytorch",
"distilbert",
"token-classification",
"transformers",
"autotrain_compatible"
] | token-classification | false | Qishuai | null | Qishuai/distilbert_punctuator_en | 2,098 | 5 | transformers | # Punctuator for Uncased English
The model is fine-tuned based on `DistilBertForTokenClassification` for adding punctuations to plain text (uncased English)
## Usage
```python
from transformers import DistilBertForTokenClassification, DistilBertTokenizerFast
model = DistilBertForTokenClassification.from_pretrained("Qishuai/distilbert_punctuator_en")
tokenizer = DistilBertTokenizerFast.from_pretrained("Qishuai/distilbert_punctuator_en")
```
## Model Overview
### Training data
Combination of following three dataset:
- BBC news: From BBC news website corresponding to stories in five topical areas from 2004-2005. [Reference](https://www.kaggle.com/hgultekin/bbcnewsarchive)
- News articles: 20000 samples of short news articles scraped from Hindu, Indian times and Guardian between Feb 2017 and Aug 2017 [Reference](https://www.kaggle.com/sunnysai12345/news-summary?select=news_summary_more.csv)
- Ted talks: transcripts of over 4,000 TED talks between 2004 and 2019 [Reference](https://www.kaggle.com/miguelcorraljr/ted-ultimate-dataset)
### Model Performance
- Validation with 500 samples of dataset scraped from https://www.thenews.com.pk website. [Reference](https://www.kaggle.com/asad1m9a9h6mood/news-articles)
- Metrics Report:
| | precision | recall | f1-score | support |
|:--------------:|:---------:|:------:|:--------:|:-------:|
| COMMA | 0.66 | 0.55 | 0.60 | 7064 |
| EXLAMATIONMARK | 1.00 | 0.00 | 0.00 | 5 |
| PERIOD | 0.73 | 0.63 | 0.68 | 6573 |
| QUESTIONMARK | 0.54 | 0.41 | 0.47 | 17 |
| micro avg | 0.69 | 0.59 | 0.64 | 13659 |
| macro avg | 0.73 | 0.40 | 0.44 | 13659 |
| weighted avg | 0.69 | 0.59 | 0.64 | 13659 |
- Validation with 86 news ted talks of 2020 which are not included in training dataset [Reference](https://www.kaggle.com/thegupta/ted-talk)
- Metrics Report:
| | precision | recall | f1-score | support |
|:--------------:|:---------:|:------:|:--------:|:-------:|
| COMMA | 0.71 | 0.56 | 0.63 | 10712 |
| EXLAMATIONMARK | 0.45 | 0.07 | 0.12 | 75 |
| PERIOD | 0.75 | 0.65 | 0.70 | 7921 |
| QUESTIONMARK | 0.73 | 0.67 | 0.70 | 827 |
| micro avg | 0.73 | 0.60 | 0.66 | 19535 |
| macro avg | 0.66 | 0.49 | 0.53 | 19535 |
| weighted avg | 0.73 | 0.60 | 0.66 | 19535 |
| [
-0.01037026010453701,
-0.033465802669525146,
0.05477744713425636,
0.05313171446323395,
-0.02260603755712509,
0.02112329564988613,
-0.043683670461177826,
0.03406726196408272,
-0.05485139787197113,
-0.07472700625658035,
0.029058843851089478,
-0.023424115031957626,
0.004317182116210461,
0.031640782952308655,
0.0653376579284668,
0.01747065596282482,
-0.019138429313898087,
0.03012252226471901,
-0.10531596094369888,
-0.13009105622768402,
0.10954268276691437,
0.09027141332626343,
0.044241972267627716,
0.009995629079639912,
0.07175282388925552,
0.003090647980570793,
-0.010976468212902546,
-0.02484527789056301,
0.012991614639759064,
-0.017419960349798203,
-0.03506689891219139,
-0.01604013703763485,
0.004660430364310741,
0.019403917714953423,
0.02015119232237339,
0.03143494948744774,
0.0216892771422863,
0.0011136371176689863,
0.0755605474114418,
0.03290528059005737,
0.03108828142285347,
-0.06485140323638916,
-0.005863697733730078,
-0.006618998479098082,
0.07159898430109024,
0.03852887451648712,
-0.05352042242884636,
0.02113310806453228,
-0.044203463941812515,
-0.005788684822618961,
-0.09033311903476715,
0.01725267805159092,
0.029945462942123413,
0.0668158084154129,
-0.07413802295923233,
-0.037846583873033524,
-0.034062135964632034,
0.007947313599288464,
0.08592166006565094,
-0.10457161068916321,
-0.09779887646436691,
0.009093397296965122,
-0.036831241101026535,
-0.006647706497460604,
-0.024878883734345436,
-0.025053974241018295,
0.025167062878608704,
-0.011472229845821857,
0.06635689735412598,
0.0020968387834727764,
-0.06818034499883652,
0.0469491221010685,
-0.018264010548591614,
0.05384247004985809,
-0.007992442697286606,
0.016208689659833908,
0.04114553704857826,
0.013172455132007599,
0.0034320943523198366,
-0.07741987705230713,
0.03302410617470741,
-0.06507448852062225,
0.06048193573951721,
0.042131658643484116,
0.06662541627883911,
-0.08569679409265518,
-0.035827912390232086,
-0.032209061086177826,
0.014952827244997025,
-0.0373716875910759,
0.013524120673537254,
-0.09011460095643997,
0.06964921951293945,
0.05137774348258972,
-0.028995219618082047,
0.035030510276556015,
-0.017447149381041527,
0.041172273457050323,
-0.035500749945640564,
0.07850010693073273,
-0.023630613461136818,
-0.041073866188526154,
-0.01998966373503208,
-0.020123369991779327,
-0.11539293825626373,
-0.07090173661708832,
-0.034196168184280396,
-0.02948145568370819,
0.026165656745433807,
-0.0705396831035614,
0.015856564044952393,
0.0208680909126997,
-0.03319142013788223,
-0.06458357721567154,
0.026137905195355415,
-0.004412371199578047,
-0.06260063499212265,
-0.0372631773352623,
0.046138275414705276,
0.10185449570417404,
-0.043198708444833755,
0.07379471510648727,
-0.016721457242965698,
0.03228343278169632,
-0.04182913899421692,
-0.02527402527630329,
-0.008560101501643658,
1.2041135975029793e-32,
0.0099291130900383,
0.07367070764303207,
0.0032752908300608397,
0.014236590825021267,
-0.07372807711362839,
-0.02335251308977604,
-0.07028175890445709,
0.0015961048193275928,
-0.011011283844709396,
-0.06647927314043045,
-0.017396626994013786,
0.06889340281486511,
-0.08013462275266647,
-0.034201350063085556,
-0.057745080441236496,
-0.01018909178674221,
0.004867915529757738,
0.046709004789590836,
0.03117583505809307,
0.02796405926346779,
0.08401673287153244,
0.039381034672260284,
0.04992522671818733,
-0.057575248181819916,
-0.05009520426392555,
0.03448475897312164,
0.11371692270040512,
-0.06957786530256271,
-0.025543726980686188,
0.04170050844550133,
-0.046694375574588776,
-0.03415064513683319,
0.006988445296883583,
-0.03672192990779877,
0.033393535763025284,
-0.038786038756370544,
0.023098846897482872,
-0.06852616369724274,
-0.004443929065018892,
-0.05435610190033913,
-0.046435870230197906,
0.011391833424568176,
0.020232750102877617,
-0.028517691418528557,
-0.013460438698530197,
0.058408912271261215,
0.011094183661043644,
-0.007778963539749384,
0.027492202818393707,
0.0010422342456877232,
-0.023958107456564903,
0.012607939541339874,
-0.04643522575497627,
-0.04768461361527443,
0.030247781425714493,
0.00638560438528657,
0.10587527602910995,
-0.01683902181684971,
0.07627677172422409,
-0.024021785706281662,
0.037454187870025635,
0.07231815159320831,
0.06217150762677193,
-0.03234159201383591,
0.02995740808546543,
0.029779311269521713,
-0.01237453706562519,
0.03463466092944145,
-0.015571883879601955,
0.04369252920150757,
-0.05598407983779907,
-0.048061802983284,
-0.022397581487894058,
0.049548376351594925,
0.0007714733947068453,
-0.026957442983984947,
0.0016269467305392027,
-0.08904791623353958,
-0.03069508634507656,
0.016439087688922882,
0.010652742348611355,
-0.027567807585000992,
0.03018311597406864,
-0.0383571982383728,
-0.022990047931671143,
-0.045890651643276215,
0.035606835037469864,
-0.06689074635505676,
0.03810925409197807,
-0.06816067546606064,
-0.0014136639656499028,
0.03534829244017601,
-0.06594467163085938,
0.0342135950922966,
0.020906204357743263,
-1.240134785174005e-32,
-0.018037671223282814,
0.05494866147637367,
-0.07729953527450562,
0.106864333152771,
-0.009608710184693336,
-0.04792175069451332,
0.033425744622945786,
0.17845234274864197,
0.08215361088514328,
-0.022145578637719154,
0.08098112791776657,
-0.07136962562799454,
0.00376828177832067,
-0.009619674645364285,
0.00807638093829155,
0.05863474681973457,
0.010177246294915676,
0.06050807982683182,
-0.02591467835009098,
0.0651346817612648,
-0.009391509927809238,
0.030121201649308205,
-0.2140626758337021,
0.044294118881225586,
-0.008161372505128384,
0.026555221527814865,
0.019722478464245796,
-0.017003988847136497,
0.007367778569459915,
-0.014882486313581467,
-0.09377909451723099,
-0.028759580105543137,
-0.03947870433330536,
0.0012793190544471145,
-0.1625465452671051,
0.02587370201945305,
-0.0007836624863557518,
-0.06137208268046379,
-0.02376028336584568,
0.05754859372973442,
0.04144391417503357,
0.054018691182136536,
-0.03968869522213936,
0.01711283251643181,
-0.026867907494306564,
-0.045518532395362854,
-0.03847271949052811,
0.009242016822099686,
0.07375913113355637,
0.020943868905305862,
0.06969606876373291,
0.04584401473402977,
-0.0828685611486435,
-0.03213915228843689,
-0.017083700746297836,
-0.07483354210853577,
0.0022885713260620832,
-0.07514284551143646,
-0.08431538194417953,
-0.05257532373070717,
-0.09310557693243027,
0.0289005134254694,
0.021109694615006447,
-0.091886006295681,
0.013463011011481285,
-0.08908410370349884,
0.011512565426528454,
-0.001967823598533869,
0.05971977859735489,
-0.0686010867357254,
0.0809050127863884,
-0.023109862580895424,
0.029844556003808975,
-0.07832350581884384,
-0.048798367381095886,
0.07073269784450531,
0.003418167820200324,
0.022791322320699692,
0.002371457638218999,
0.010484595783054829,
-0.06474647670984268,
-0.004673718940466642,
0.05645447596907616,
0.07507066428661346,
0.04341539368033409,
0.07459285855293274,
-0.008271940983831882,
0.09165742993354797,
0.03655947744846344,
0.051260560750961304,
0.009820148348808289,
0.035424165427684784,
-0.0000767121382523328,
0.12486853450536728,
0.005149968434125185,
-6.835224297674358e-8,
-0.042332474142313004,
-0.020857375115156174,
-0.08537635207176208,
0.08177405595779419,
-0.012775388546288013,
-0.024780506268143654,
-0.029159681871533394,
0.010113014839589596,
-0.038554541766643524,
0.0037469095550477505,
-0.012099772691726685,
0.005794151686131954,
-0.12066036462783813,
-0.01226745918393135,
-0.04488950967788696,
0.09825391322374344,
0.025741448625922203,
0.129271000623703,
-0.02310539223253727,
-0.02487645484507084,
0.06766261160373688,
0.02565525472164154,
0.039603717625141144,
-0.031120916828513145,
0.011739236302673817,
0.011464934796094894,
-0.08079761266708374,
0.012227579951286316,
-0.01918650232255459,
-0.022723913192749023,
-0.018844155594706535,
-0.003495878539979458,
-0.08133427053689957,
-0.08752042800188065,
-0.04749034345149994,
0.06934751570224762,
0.002714922185987234,
0.023425482213497162,
-0.0028478645253926516,
0.0724894180893898,
0.044687796384096146,
-0.049317505210638046,
-0.07140600681304932,
0.06307340413331985,
0.07490284740924835,
0.006147780921310186,
-0.05262726917862892,
-0.10362725704908371,
0.027133041992783546,
0.016181396320462227,
0.04206457361578941,
-0.07031658291816711,
0.010842083021998405,
0.0013623738195747137,
-0.012458899058401585,
0.053512055426836014,
-0.0004528673889581114,
-0.03888823837041855,
-0.057958878576755524,
-0.009551714174449444,
0.046806834638118744,
-0.05158368870615959,
0.037505701184272766,
0.009524400345981121
] |
johngiorgi/declutr-base | 3a644f1c78aae97f6e7ed0e2463bcbbaef2e7383 | 2022-03-11T14:47:09.000Z | [
"pytorch",
"jax",
"roberta",
"fill-mask",
"arxiv:2006.03659",
"transformers",
"autotrain_compatible"
] | fill-mask | false | johngiorgi | null | johngiorgi/declutr-base | 2,095 | 1 | transformers | # DeCLUTR-base
## Model description
The "DeCLUTR-base" model from our paper: [DeCLUTR: Deep Contrastive Learning for Unsupervised Textual Representations](https://arxiv.org/abs/2006.03659).
## Intended uses & limitations
The model is intended to be used as a universal sentence encoder, similar to [Google's Universal Sentence Encoder](https://tfhub.dev/google/universal-sentence-encoder/4) or [Sentence Transformers](https://github.com/UKPLab/sentence-transformers).
#### How to use
Please see [our repo](https://github.com/JohnGiorgi/DeCLUTR) for full details. A simple example is shown below.
##### With [SentenceTransformers](https://www.sbert.net/)
```python
from scipy.spatial.distance import cosine
from sentence_transformers import SentenceTransformer
# Load the model
model = SentenceTransformer("johngiorgi/declutr-base")
# Prepare some text to embed
texts = [
"A smiling costumed woman is holding an umbrella.",
"A happy woman in a fairy costume holds an umbrella.",
]
# Embed the text
embeddings = model.encode(texts)
# Compute a semantic similarity via the cosine distance
semantic_sim = 1 - cosine(embeddings[0], embeddings[1])
```
##### With 🤗 Transformers
```python
import torch
from scipy.spatial.distance import cosine
from transformers import AutoModel, AutoTokenizer
# Load the model
tokenizer = AutoTokenizer.from_pretrained("johngiorgi/declutr-base")
model = AutoModel.from_pretrained("johngiorgi/declutr-base")
# Prepare some text to embed
text = [
"A smiling costumed woman is holding an umbrella.",
"A happy woman in a fairy costume holds an umbrella.",
]
inputs = tokenizer(text, padding=True, truncation=True, return_tensors="pt")
# Embed the text
with torch.no_grad():
sequence_output = model(**inputs)[0]
# Mean pool the token-level embeddings to get sentence-level embeddings
embeddings = torch.sum(
sequence_output * inputs["attention_mask"].unsqueeze(-1), dim=1
) / torch.clamp(torch.sum(inputs["attention_mask"], dim=1, keepdims=True), min=1e-9)
# Compute a semantic similarity via the cosine distance
semantic_sim = 1 - cosine(embeddings[0], embeddings[1])
```
### BibTeX entry and citation info
```bibtex
@article{Giorgi2020DeCLUTRDC,
title={DeCLUTR: Deep Contrastive Learning for Unsupervised Textual Representations},
author={John M Giorgi and Osvald Nitski and Gary D. Bader and Bo Wang},
journal={ArXiv},
year={2020},
volume={abs/2006.03659}
}
``` | [
-0.044719502329826355,
-0.08998658508062363,
0.009725366719067097,
-0.010423085652291775,
-0.023786641657352448,
0.007124846335500479,
-0.1138182058930397,
0.037695642560720444,
0.010254251770675182,
-0.050631068646907806,
0.035369839519262314,
-0.03548182547092438,
0.017322812229394913,
0.08099794387817383,
-0.018332118168473244,
0.013525412417948246,
0.0756882056593895,
0.09545853734016418,
-0.08296389132738113,
-0.12401261180639267,
0.11432559043169022,
0.05552910640835762,
-0.010147251188755035,
-0.004502265714108944,
0.0818028450012207,
0.0518467016518116,
-0.0010615497594699264,
-0.0046846140176057816,
0.047496091574430466,
-0.039546336978673935,
0.026287587359547615,
-0.0521966926753521,
0.04080749303102493,
0.10398080945014954,
0.025209149345755577,
0.06055748090147972,
-0.015328977257013321,
-0.03482922539114952,
-0.0011637614807114005,
0.010469792410731316,
-0.0030424222350120544,
0.006246513687074184,
0.0016816871939226985,
0.027278216555714607,
0.08256618678569794,
-0.034501660615205765,
-0.11003478616476059,
0.0003766674781218171,
-0.009065713733434677,
-0.05047405883669853,
-0.014010710641741753,
0.07781525701284409,
-0.007237260229885578,
0.08446834981441498,
-0.06368663161993027,
0.010913134552538395,
0.008519835770130157,
-0.008982192724943161,
-0.023813990876078606,
-0.1184462457895279,
-0.05424676090478897,
-0.014329127036035061,
-0.04722447693347931,
-0.04941534623503685,
-0.01998569257557392,
0.022747907787561417,
0.004660502541810274,
0.015164719894528389,
-0.030887532979249954,
0.06240968033671379,
-0.04607096314430237,
0.03727688267827034,
0.015966031700372696,
0.013220760971307755,
-0.04438527300953865,
0.016665130853652954,
0.07136833667755127,
0.002353869378566742,
0.04670734703540802,
-0.05130472406744957,
0.06539200991392136,
0.004886926151812077,
0.0835162028670311,
0.06060412898659706,
0.07704188674688339,
-0.03865484148263931,
-0.05541844666004181,
0.02318933792412281,
-0.015490338206291199,
0.03520234674215317,
-0.05004602670669556,
-0.13441090285778046,
0.020481016486883163,
-0.010748487897217274,
-0.017079031094908714,
0.010769343003630638,
0.002612958662211895,
-0.010362938046455383,
-0.04980744794011116,
0.006289453711360693,
0.02392769418656826,
0.0018765224376693368,
0.039619218558073044,
-0.10949239879846573,
-0.058715999126434326,
-0.014784635975956917,
0.04378106817603111,
-0.019075604155659676,
0.11007930338382721,
-0.12409821152687073,
-0.04355815798044205,
0.0006806859746575356,
-0.08511435240507126,
-0.0012000385904684663,
0.06662951409816742,
-0.016329895704984665,
0.03322349488735199,
-0.027065444737672806,
0.03689459711313248,
-0.010319255292415619,
0.015249048359692097,
0.011186630465090275,
-0.047345176339149475,
0.06876470893621445,
-0.0022303189616650343,
-0.07996626198291779,
-0.0409151054918766,
4.737306886003114e-33,
-0.008507530204951763,
0.0353081189095974,
0.013223573565483093,
0.019699277356266975,
0.007746294606477022,
0.052394308149814606,
-0.0791591927409172,
0.05954204499721527,
-0.09474173933267593,
0.010845187120139599,
-0.05530587583780289,
0.08143483102321625,
-0.05534522607922554,
0.07366988062858582,
-0.021996162831783295,
-0.025665439665317535,
-0.007336392533034086,
-0.02278286963701248,
-0.02496429532766342,
-0.00957054365426302,
0.03193731606006622,
0.11691123247146606,
-0.045393671840429306,
-0.04135202616453171,
-0.12674276530742645,
-0.012782045640051365,
0.03585607931017876,
-0.049036905169487,
-0.02521846629679203,
0.020372506231069565,
-0.1419585645198822,
0.010803398676216602,
0.020273784175515175,
0.03866559639573097,
0.06930309534072876,
-0.03084728494286537,
-0.0242313239723444,
-0.009312047623097897,
0.019017238169908524,
-0.0652124360203743,
0.0060498095117509365,
0.05449439957737923,
-0.04372057691216469,
-0.04919213429093361,
-0.030396632850170135,
0.018036630004644394,
0.03558595851063728,
-0.06398585438728333,
0.04148366302251816,
-0.0018329296726733446,
0.06092340871691704,
0.040272071957588196,
-0.09933124482631683,
-0.09346392005681992,
0.02228389121592045,
0.020587341859936714,
0.0796201080083847,
0.06042985990643501,
0.06808532029390335,
-0.040798112750053406,
0.03972150757908821,
0.029933316633105278,
0.062226247042417526,
0.030685218051075935,
0.05528244003653526,
0.019855014979839325,
-0.0518735907971859,
0.006297457497566938,
-0.023054761812090874,
0.05523671209812164,
-0.08180174231529236,
0.04036027938127518,
-0.06606581807136536,
0.037793777883052826,
0.025162989273667336,
-0.002615587320178747,
0.05901787057518959,
-0.0031674166675657034,
-0.040531255304813385,
0.01200571283698082,
-0.015641992911696434,
0.018329598009586334,
0.00872566644102335,
-0.10690254718065262,
-0.08097189664840698,
0.004956556484103203,
0.045818910002708435,
-0.07817629724740982,
-0.012624348513782024,
-0.0328153595328331,
0.014055807143449783,
-0.035674694925546646,
0.0011849267175421119,
-0.01558142900466919,
0.0727899894118309,
-7.20763985566683e-33,
0.024839306250214577,
0.0412401482462883,
-0.06373048573732376,
0.04631955921649933,
-0.06375768035650253,
-0.03896933048963547,
0.046420060098171234,
0.07801593840122223,
0.07397668063640594,
-0.008389730006456375,
0.014291107654571533,
-0.015193966217339039,
0.03837580233812332,
-0.018393488600850105,
0.10230529308319092,
0.08323805779218674,
-0.005812431685626507,
0.06822103261947632,
-0.03394841030240059,
0.0006152569549158216,
0.01741287112236023,
0.1263795793056488,
-0.132792666554451,
-0.007429463788866997,
-0.029109638184309006,
0.03251554071903229,
-0.050288889557123184,
0.027745980769395828,
0.01423716638237238,
-0.0645613819360733,
-0.0767960473895073,
0.028234217315912247,
-0.011358595453202724,
0.006413287948817015,
-0.1538967341184616,
0.026498524472117424,
-0.012985303066670895,
-0.05923767015337944,
-0.04624741151928902,
0.0003540634934324771,
0.07989432662725449,
0.016827547922730446,
-0.02053235098719597,
0.04907124862074852,
0.02131711132824421,
0.0019545843824744225,
-0.08139882236719131,
-0.06277619302272797,
0.059436675161123276,
0.036777105182409286,
-0.006391406524926424,
0.002931619295850396,
-0.11519861966371536,
0.01682206243276596,
-0.038053303956985474,
-0.015446232631802559,
-0.016016419976949692,
-0.052973825484514236,
-0.0018696587067097425,
0.008958028629422188,
-0.05560237541794777,
-0.02351406402885914,
-0.005809797905385494,
-0.07228124141693115,
0.023585502058267593,
-0.057514168322086334,
-0.01597149297595024,
0.024982621893286705,
0.03343333676457405,
-0.06577868014574051,
0.03333773463964462,
-0.02684895694255829,
0.01730637438595295,
0.01646493934094906,
0.029728488996624947,
-0.028922559693455696,
-0.04135887697339058,
0.006215989589691162,
-0.10565730929374695,
-0.016813714057207108,
-0.02561025321483612,
-0.04114210978150368,
-0.002194908680394292,
0.047582365572452545,
0.04799932241439819,
0.011272369883954525,
0.004242969676852226,
0.07916224002838135,
-0.026979370042681694,
0.027007373049855232,
-0.034421972930431366,
-0.032476652413606644,
0.0399743877351284,
0.06326018273830414,
0.020101193338632584,
-5.723611806729423e-8,
-0.10251256078481674,
-0.011908645741641521,
-0.03997280076146126,
0.013444304466247559,
-0.019885506480932236,
-0.06370456516742706,
0.0031579991336911917,
-0.01307633239775896,
-0.06518152356147766,
-0.004297330044209957,
0.02798810973763466,
-0.017200713977217674,
-0.06525909900665283,
-0.024390336126089096,
-0.05193581059575081,
0.15783119201660156,
0.025263575837016106,
-0.005334966816008091,
0.06028928607702255,
0.06820210069417953,
0.04412563517689705,
0.022237030789256096,
-0.012136454693973064,
0.03881767392158508,
-0.05238371714949608,
0.04626668617129326,
-0.0576922744512558,
0.0816749781370163,
-0.00995862577110529,
0.012052861973643303,
0.0543670691549778,
0.05820760130882263,
-0.0037939869798719883,
-0.057293906807899475,
-0.0605493001639843,
0.05621061474084854,
0.025739213451743126,
-0.01971183717250824,
0.03224365785717964,
0.04047174006700516,
0.03524456173181534,
0.028859928250312805,
-0.13635751605033875,
0.08398924767971039,
0.05205755680799484,
0.04422241449356079,
0.05667402967810631,
-0.08718425780534744,
-0.016299795359373093,
0.02465566247701645,
0.03421752527356148,
-0.03771545737981796,
-0.040830712765455246,
0.009629190899431705,
0.06977218389511108,
-0.030081452801823616,
0.029565731063485146,
-0.014798750169575214,
-0.021004078909754753,
-0.004272107500582933,
0.012252841144800186,
0.10181985795497894,
0.057962458580732346,
-0.012663849629461765
] |
LeBenchmark/wav2vec2-FR-7K-large | 970d57910b508c27e9cafd52b781fee76cebfc8b | 2021-11-23T17:54:37.000Z | [
"pytorch",
"wav2vec2",
"feature-extraction",
"fr",
"transformers",
"license:apache-2.0"
] | feature-extraction | false | LeBenchmark | null | LeBenchmark/wav2vec2-FR-7K-large | 2,092 | 3 | transformers | ---
language: "fr"
thumbnail:
tags:
- wav2vec2
license: "apache-2.0"
---
# LeBenchmark: wav2vec2 large model trained on 7K hours of French speech
LeBenchmark provides an ensemble of pretrained wav2vec2 models on different French datasets containing spontaneous, read, and broadcasted speech. For more information on the different benchmarks that can be used to evaluate the wav2vec2 models, please refer to our paper at: [Task Agnostic and Task Specific Self-Supervised Learning from Speech with LeBenchmark](https://openreview.net/pdf?id=TSvj5dmuSd)
## Model and data descriptions
We release four different models that can be found under our HuggingFace organization. Two different wav2vec2 architectures *Base* and *Large* are coupled with our small (1K), medium (3K), and large (7K) corpus. A larger one should come later. In short:
- [wav2vec2-FR-7K-large](https://huggingface.co/LeBenchmark/wav2vec2-FR-7K-large): Large wav2vec2 trained on 7.6K hours of French speech (1.8K Males / 1.0K Females / 4.8K unknown).
- [wav2vec2-FR-7K-base](https://huggingface.co/LeBenchmark/wav2vec2-FR-7K-base): Base wav2vec2 trained on 7.6K hours of French speech (1.8K Males / 1.0K Females / 4.8K unknown).
- [wav2vec2-FR-3K-large](https://huggingface.co/LeBenchmark/wav2vec2-FR-3K-large): Large wav2vec2 trained on 2.9K hours of French speech (1.8K Males / 1.0K Females / 0.1K unknown).
- [wav2vec2-FR-3K-base](https://huggingface.co/LeBenchmark/wav2vec2-FR-3K-base): Base wav2vec2 trained on 2.9K hours of French speech (1.8K Males / 1.0K Females / 0.1K unknown).
- [wav2vec2-FR-2.6K-base](https://huggingface.co/LeBenchmark/wav2vec2-FR-2.6K-base): Base wav2vec2 trained on 2.6K hours of French speech (**no spontaneous speech**).
- [wav2vec2-FR-1K-large](https://huggingface.co/LeBenchmark/wav2vec2-FR-1K-large): Large wav2vec2 trained on 1K hours of French speech (0.5K Males / 0.5K Females).
- [wav2vec2-FR-1K-base](https://huggingface.co/LeBenchmark/wav2vec2-FR-1K-base): Base wav2vec2 trained on 1K hours of French speech (0.5K Males / 0.5K Females).
## Intended uses & limitations
Pretrained wav2vec2 models are distributed under the Apache-2.0 license. Hence, they can be reused extensively without strict limitations. However, benchmarks and data may be linked to corpora that are not completely open-sourced.
## Fine-tune with Fairseq for ASR with CTC
As our wav2vec2 models were trained with Fairseq, then can be used in the different tools that they provide to fine-tune the model for ASR with CTC. The full procedure has been nicely summarized in [this blogpost](https://huggingface.co/blog/fine-tune-wav2vec2-english).
Please note that due to the nature of CTC, speech-to-text results aren't expected to be state-of-the-art. Moreover, future features might appear depending on the involvement of Fairseq and HuggingFace on this part.
## Integrate to SpeechBrain for ASR, Speaker, Source Separation ...
Pretrained wav2vec models recently gained in popularity. At the same time, [SpeechBrain toolkit](https://speechbrain.github.io) came out, proposing a new and simpler way of dealing with state-of-the-art speech & deep-learning technologies.
While it currently is in beta, SpeechBrain offers two different ways of nicely integrating wav2vec2 models that were trained with Fairseq i.e our LeBenchmark models!
1. Extract wav2vec2 features on-the-fly (with a frozen wav2vec2 encoder) to be combined with any speech-related architecture. Examples are: E2E ASR with CTC+Att+Language Models; Speaker Recognition or Verification, Source Separation ...
2. *Experimental:* To fully benefit from wav2vec2, the best solution remains to fine-tune the model while you train your downstream task. This is very simply allowed within SpeechBrain as just a flag needs to be turned on. Thus, our wav2vec2 models can be fine-tuned while training your favorite ASR pipeline or Speaker Recognizer.
**If interested, simply follow this [tutorial](https://colab.research.google.com/drive/17Hu1pxqhfMisjkSgmM2CnZxfqDyn2hSY?usp=sharing)**
## Referencing LeBenchmark
```
@article{Evain2021LeBenchmarkAR,
title={LeBenchmark: A Reproducible Framework for Assessing Self-Supervised Representation Learning from Speech},
author={Sol{\`e}ne Evain and Ha Nguyen and Hang Le and Marcely Zanon Boito and Salima Mdhaffar and Sina Alisamir and Ziyi Tong and N. Tomashenko and Marco Dinarelli and Titouan Parcollet and A. Allauzen and Y. Est{\`e}ve and B. Lecouteux and F. Portet and S. Rossato and F. Ringeval and D. Schwab and L. Besacier},
journal={ArXiv},
year={2021},
volume={abs/2104.11462}
}
```
| [
-0.050674133002758026,
-0.10285968333482742,
0.042683012783527374,
-0.04666152596473694,
0.0782104954123497,
0.04967045038938522,
-0.05681654065847397,
-0.021900855004787445,
-0.0017419015057384968,
-0.031061338260769844,
-0.04434194415807724,
-0.09681036323308945,
0.003902298165485263,
-0.0036174084525555372,
-0.018546218052506447,
-0.03945721313357353,
0.09988021850585938,
0.00261093070730567,
-0.04887958616018295,
-0.03868670389056206,
0.0428551584482193,
0.05395449325442314,
0.10365675389766693,
-0.053223010152578354,
0.10772323608398438,
0.022077148780226707,
-0.09770087897777557,
0.032603416591882706,
0.08294111490249634,
-0.030493592843413353,
0.10269500315189362,
0.010504115372896194,
0.038199298083782196,
0.011264310218393803,
-0.04497375711798668,
-0.03964495658874512,
0.04230177402496338,
-0.025159472599625587,
-0.014635968022048473,
0.016100795939564705,
-0.027974393218755722,
0.051053717732429504,
-0.04288056865334511,
-0.01103055290877819,
0.031951144337654114,
-0.01606590300798416,
-0.04020330682396889,
0.013337488286197186,
-0.09886712580919266,
0.008883805014193058,
-0.06499266624450684,
-0.04874412342905998,
-0.005101209972053766,
0.11533133685588837,
-0.10017290711402893,
0.03628694266080856,
-0.0064854128286242485,
0.04143550246953964,
0.03963453322649002,
0.045925941318273544,
-0.0313536673784256,
-0.0941900834441185,
-0.041614629328250885,
-0.009014680981636047,
0.016323938965797424,
-0.01068267785012722,
-0.0461413636803627,
0.025008607655763626,
0.018648436293005943,
0.02500198595225811,
-0.08437076210975647,
0.06064143031835556,
0.030193546786904335,
0.03862761706113815,
0.09593255072832108,
0.009396987035870552,
0.044144708663225174,
0.026626750826835632,
0.06349413841962814,
-0.127881720662117,
-0.007741022389382124,
-0.0626806914806366,
0.05652390420436859,
-0.045163001865148544,
0.09986606240272522,
-0.021325303241610527,
0.041128192096948624,
0.0045835101045668125,
-0.028754476457834244,
-0.036577608436346054,
-0.02282659523189068,
-0.024888930842280388,
-0.007313563954085112,
0.026556948199868202,
0.006084072403609753,
0.050436101853847504,
0.05768078565597534,
0.06413231045007706,
-0.02977452427148819,
0.06448094546794891,
0.027704672887921333,
-0.04829668998718262,
-0.023065686225891113,
-0.06299613416194916,
0.003478002268821001,
-0.05663007125258446,
-0.026036109775304794,
0.048001307994127274,
0.044148582965135574,
-0.09841834008693695,
0.032634276896715164,
0.0429459810256958,
-0.0324283093214035,
0.0009259347571060061,
0.056917980313301086,
0.025340162217617035,
-0.031058134511113167,
-0.06716777384281158,
0.05433114618062973,
0.016316421329975128,
-0.0670456811785698,
-0.02368731051683426,
0.010437926277518272,
-0.015605452470481396,
-0.03648960590362549,
-0.004788604099303484,
-0.06555810570716858,
7.720128944002453e-33,
0.048662614077329636,
0.08516676723957062,
-0.008298791013658047,
-0.034749843180179596,
-0.029765505343675613,
-0.056386351585388184,
-0.039931830018758774,
0.04044226184487343,
-0.02880091778934002,
-0.027307113632559776,
-0.03567168861627579,
0.018872642889618874,
-0.01610667072236538,
0.08557846397161484,
0.035103410482406616,
0.012842047959566116,
-0.05653343349695206,
0.005254419054836035,
-0.008638686500489712,
-0.004483778029680252,
0.17963235080242157,
0.035862069576978683,
0.05611088126897812,
-0.0026686182245612144,
0.08517862111330032,
0.010688398033380508,
0.07154194265604019,
-0.07350841164588928,
-0.03909585624933243,
0.06802130490541458,
-0.078246109187603,
-0.07566920667886734,
0.00641428679227829,
-0.008441387675702572,
-0.015113015659153461,
0.010066529735922813,
0.011029348708689213,
-0.02404584176838398,
-0.03485977649688721,
-0.1072060689330101,
0.01830332726240158,
-0.006518235430121422,
0.030502799898386,
-0.04389577358961105,
-0.06585641950368881,
-0.09974513947963715,
-0.0027033966034650803,
0.032916467636823654,
-0.004656381905078888,
-0.04196691885590553,
-0.005857858806848526,
-0.0027248014230281115,
-0.05389789119362831,
0.0218204315751791,
-0.006132996175438166,
0.029164453968405724,
0.084036685526371,
0.025976646691560745,
0.0219376552850008,
0.035059235990047455,
0.0229507926851511,
0.006505555473268032,
0.030075836926698685,
0.01734137162566185,
0.05712631717324257,
-0.016002999618649483,
-0.07048407942056656,
0.021333543583750725,
0.031797412782907486,
-0.05177977681159973,
0.0027110630180686712,
-0.004085168708115816,
0.06745099276304245,
-0.007979737594723701,
0.054606880992650986,
-0.030655918642878532,
0.05576227605342865,
-0.08996912837028503,
-0.029383622109889984,
0.03795977309346199,
-0.02144153043627739,
0.02170855738222599,
-0.03808608651161194,
-0.10273934155702591,
-0.09023384749889374,
-0.09735328704118729,
0.012704283930361271,
-0.1478261798620224,
-0.08435443043708801,
0.03981423377990723,
-0.05639299750328064,
0.033641450107097626,
0.011462377384305,
-0.00039469925104640424,
-0.04549025371670723,
-8.279673071201493e-33,
-0.0030272353906184435,
0.10038774460554123,
-0.05127999931573868,
0.10739139467477798,
0.014439408667385578,
-0.004155809059739113,
0.057647671550512314,
0.11661618202924728,
-0.0497477687895298,
-0.06846828758716583,
0.048144999891519547,
-0.08704957365989685,
0.07002654671669006,
-0.013186592608690262,
0.023806093260645866,
-0.04359707981348038,
-0.015569374896585941,
0.002518234308809042,
0.1157039999961853,
0.0591580867767334,
0.056950606405735016,
0.0023697379510849714,
-0.007607220206409693,
0.08306398242712021,
-0.01733633130788803,
-0.0043692318722605705,
-0.03091476298868656,
0.014270010404288769,
-0.01507195457816124,
-0.012045665644109249,
-0.08665674924850464,
0.020942773669958115,
-0.06361086666584015,
-0.017477620393037796,
-0.022834274917840958,
0.05523758754134178,
-0.02513005957007408,
-0.05716128647327423,
-0.015254740603268147,
0.057644642889499664,
0.03500639274716377,
0.04172001779079437,
-0.0750017762184143,
-0.07147753983736038,
0.010102763772010803,
-0.02546946331858635,
-0.09330398589372635,
0.03591885417699814,
0.014338119886815548,
-0.022373715415596962,
0.055803388357162476,
-0.013471544720232487,
-0.05270484834909439,
0.05166688561439514,
-0.04118935763835907,
-0.013446992263197899,
-0.003094941610470414,
-0.07353803515434265,
-0.041014447808265686,
-0.0017368737608194351,
-0.025568243116140366,
0.0014096411177888513,
-0.05748960003256798,
-0.03172965347766876,
0.060284681618213654,
-0.003673091297969222,
-0.05539041757583618,
0.0326969213783741,
0.036997269839048386,
0.004805436357855797,
0.006322285160422325,
-0.04804817959666252,
0.013205121271312237,
0.02865407057106495,
-0.0696728527545929,
-0.0426790788769722,
-0.019537845626473427,
-0.05522245913743973,
-0.06385699659585953,
-0.06952562928199768,
-0.049753040075302124,
0.030038021504878998,
0.042138468474149704,
-0.010984188877046108,
0.07739918678998947,
0.1255478709936142,
0.013848170638084412,
-0.019778123125433922,
-0.018638476729393005,
0.03869747370481491,
0.0003201178915333003,
0.09469705820083618,
-0.01571567915380001,
0.028824154287576675,
0.042394839227199554,
-5.5025626721771914e-8,
-0.051563289016485214,
0.0017637477722018957,
0.026378141716122627,
0.0005843472317792475,
-0.02802939899265766,
-0.11938348412513733,
-0.029987258836627007,
0.030705634504556656,
-0.02952890284359455,
0.005081062205135822,
0.04245352745056152,
-0.007564544677734375,
-0.027339834719896317,
0.01917428709566593,
0.0013688368489965796,
0.06592311710119247,
0.02365913800895214,
0.0752764567732811,
-0.03127042576670647,
-0.11932250112295151,
0.04487205669283867,
0.022523637861013412,
0.05187305063009262,
-0.002726857317611575,
0.031487077474594116,
-0.03032159060239792,
-0.03459281846880913,
0.06312082707881927,
0.01118483953177929,
0.005269959568977356,
-0.05247077718377113,
0.03704530745744705,
-0.024413563311100006,
-0.08318261802196503,
0.0318562276661396,
0.07717075198888779,
-0.08628548681735992,
-0.017296766862273216,
-0.014263888821005821,
0.10980869829654694,
0.06291808187961578,
0.08089203387498856,
-0.06975821405649185,
-0.003101406618952751,
0.09682463109493256,
-0.04018609970808029,
-0.008395083248615265,
-0.09213822335004807,
0.04934123903512955,
0.014456016942858696,
0.014649834483861923,
0.006445345468819141,
-0.044050127267837524,
0.00006612862489419058,
0.0763295441865921,
0.07846754789352417,
-0.07750308513641357,
-0.026539888232946396,
0.04097713902592659,
0.011930804699659348,
0.05817066878080368,
0.022360386326909065,
-0.04384595528244972,
0.014823522418737411
] |
hustvl/yolos-small | 5f960fd774250e41a01086ccbbf5e44d9d603c14 | 2022-06-27T08:37:45.000Z | [
"pytorch",
"yolos",
"object-detection",
"dataset:coco",
"arxiv:2106.00666",
"transformers",
"vision",
"license:apache-2.0"
] | object-detection | false | hustvl | null | hustvl/yolos-small | 2,089 | 10 | transformers | ---
license: apache-2.0
tags:
- object-detection
- vision
datasets:
- coco
widget:
- src: https://huggingface.co/datasets/mishig/sample_images/resolve/main/savanna.jpg
example_title: Savanna
- src: https://huggingface.co/datasets/mishig/sample_images/resolve/main/football-match.jpg
example_title: Football Match
- src: https://huggingface.co/datasets/mishig/sample_images/resolve/main/airport.jpg
example_title: Airport
---
# YOLOS (small-sized) model
YOLOS model fine-tuned on COCO 2017 object detection (118k annotated images). It was introduced in the paper [You Only Look at One Sequence: Rethinking Transformer in Vision through Object Detection](https://arxiv.org/abs/2106.00666) by Fang et al. and first released in [this repository](https://github.com/hustvl/YOLOS).
Disclaimer: The team releasing YOLOS did not write a model card for this model so this model card has been written by the Hugging Face team.
## Model description
YOLOS is a Vision Transformer (ViT) trained using the DETR loss. Despite its simplicity, a base-sized YOLOS model is able to achieve 42 AP on COCO validation 2017 (similar to DETR and more complex frameworks such as Faster R-CNN).
The model is trained using a "bipartite matching loss": one compares the predicted classes + bounding boxes of each of the N = 100 object queries to the ground truth annotations, padded up to the same length N (so if an image only contains 4 objects, 96 annotations will just have a "no object" as class and "no bounding box" as bounding box). The Hungarian matching algorithm is used to create an optimal one-to-one mapping between each of the N queries and each of the N annotations. Next, standard cross-entropy (for the classes) and a linear combination of the L1 and generalized IoU loss (for the bounding boxes) are used to optimize the parameters of the model.
## Intended uses & limitations
You can use the raw model for object detection. See the [model hub](https://huggingface.co/models?search=hustvl/yolos) to look for all available YOLOS models.
### How to use
Here is how to use this model:
```python
from transformers import YolosFeatureExtractor, YolosForObjectDetection
from PIL import Image
import requests
url = 'http://images.cocodataset.org/val2017/000000039769.jpg'
image = Image.open(requests.get(url, stream=True).raw)
feature_extractor = YolosFeatureExtractor.from_pretrained('hustvl/yolos-small')
model = YolosForObjectDetection.from_pretrained('hustvl/yolos-small')
inputs = feature_extractor(images=image, return_tensors="pt")
outputs = model(**inputs)
# model predicts bounding boxes and corresponding COCO classes
logits = outputs.logits
bboxes = outputs.pred_boxes
```
Currently, both the feature extractor and model support PyTorch.
## Training data
The YOLOS model was pre-trained on [ImageNet-1k](https://huggingface.co/datasets/imagenet2012) and fine-tuned on [COCO 2017 object detection](https://cocodataset.org/#download), a dataset consisting of 118k/5k annotated images for training/validation respectively.
### Training
The model was pre-trained for 200 epochs on ImageNet-1k and fine-tuned for 150 epochs on COCO.
## Evaluation results
This model achieves an AP (average precision) of **36.1** on COCO 2017 validation. For more details regarding evaluation results, we refer to table 1 of the original paper.
### BibTeX entry and citation info
```bibtex
@article{DBLP:journals/corr/abs-2106-00666,
author = {Yuxin Fang and
Bencheng Liao and
Xinggang Wang and
Jiemin Fang and
Jiyang Qi and
Rui Wu and
Jianwei Niu and
Wenyu Liu},
title = {You Only Look at One Sequence: Rethinking Transformer in Vision through
Object Detection},
journal = {CoRR},
volume = {abs/2106.00666},
year = {2021},
url = {https://arxiv.org/abs/2106.00666},
eprinttype = {arXiv},
eprint = {2106.00666},
timestamp = {Fri, 29 Apr 2022 19:49:16 +0200},
biburl = {https://dblp.org/rec/journals/corr/abs-2106-00666.bib},
bibsource = {dblp computer science bibliography, https://dblp.org}
}
``` | [
-0.06679151952266693,
0.004586847964674234,
0.05742426961660385,
-0.018529441207647324,
0.14868931472301483,
-0.05666124075651169,
-0.013755842112004757,
0.008189009502530098,
0.01832091435790062,
-0.02930036000907421,
0.03650052845478058,
-0.0765552893280983,
-0.03984060510993004,
0.05477694049477577,
0.0062383185140788555,
0.01712675392627716,
-0.014147322624921799,
0.07314404845237732,
-0.055236514657735825,
-0.008121367543935776,
-0.007835940457880497,
-0.006074044853448868,
0.047118376940488815,
-0.03698059171438217,
-0.06553015857934952,
0.004440357908606529,
-0.047118090093135834,
-0.05213635787367821,
0.0016093801241368055,
-0.09786371141672134,
-0.027984438464045525,
0.052797239273786545,
0.02898332290351391,
0.091064453125,
-0.023419825360178947,
0.04612109810113907,
0.054056622087955475,
-0.03232774883508682,
-0.04660061001777649,
-0.007795026991516352,
0.01050516776740551,
0.00991534162312746,
0.04421822354197502,
-0.03074042685329914,
0.03911949694156647,
0.03278704732656479,
0.027748923748731613,
-0.058556921780109406,
0.018583694472908974,
-0.05360358580946922,
-0.08091621845960617,
-0.05149625241756439,
-0.04040553420782089,
0.09608066082000732,
0.005331950727850199,
0.026097716763615608,
-0.0278035756200552,
-0.07031681388616562,
0.07005879282951355,
0.012082519009709358,
0.035704758018255234,
0.02538757212460041,
-0.02920401655137539,
0.00011918481322936714,
-0.024054525420069695,
-0.07749461382627487,
0.018582453951239586,
-0.06864835321903229,
0.06351307779550552,
-0.01082524098455906,
-0.015698205679655075,
0.04015444591641426,
0.054643310606479645,
0.011352543719112873,
-0.01191793940961361,
0.03705794736742973,
0.0692487359046936,
0.013034740462899208,
0.08911815285682678,
-0.13977007567882538,
0.01559087261557579,
-0.009567614644765854,
0.07401257753372192,
-0.019898729398846626,
0.06521416455507278,
0.007325183134526014,
-0.11088575422763824,
0.019190005958080292,
-0.031520918011665344,
0.0009341969271190464,
-0.029101546853780746,
-0.07274913042783737,
-0.02138056792318821,
0.05773112550377846,
-0.050039783120155334,
-0.0665363296866417,
0.021208660677075386,
-0.011245445348322392,
-0.03285212814807892,
0.1067056730389595,
-0.0005654914421029389,
-0.056807562708854675,
0.10870067030191422,
0.05117712542414665,
0.0282736849039793,
-0.0034874596167355776,
0.011304403655230999,
0.07700259238481522,
0.056949716061353683,
0.009630703367292881,
0.007407160475850105,
0.005445593502372503,
-0.04839874058961868,
-0.0234232060611248,
-0.07621343433856964,
0.011810198426246643,
-0.04250818490982056,
0.03505980223417282,
-0.02547306939959526,
-0.01651903986930847,
0.004424587357789278,
-0.036961495876312256,
-0.019378850236535072,
-0.054475802928209305,
0.008277368731796741,
-0.04039108380675316,
-0.04547314718365669,
3.6540734133570214e-33,
0.027183586731553078,
-0.012250439263880253,
0.0002588499628473073,
-0.00847987737506628,
0.025286348536610603,
-0.041674353182315826,
-0.01708371378481388,
0.04638688638806343,
-0.0984315276145935,
0.05015037581324577,
-0.04291842132806778,
0.002285181311890483,
-0.06101221963763237,
0.05233067274093628,
0.040191978216171265,
-0.0902075469493866,
-0.03662663325667381,
0.013831164687871933,
-0.04004334285855293,
0.06498764455318451,
-0.011381830088794231,
-0.024786297231912613,
-0.015981266275048256,
-0.036033764481544495,
-0.03223070129752159,
0.10042990744113922,
0.014403779990971088,
-0.032121967524290085,
0.027315134182572365,
0.03998429700732231,
0.025459332391619682,
0.07764710485935211,
0.006358632817864418,
0.03390837833285332,
-0.005432303994894028,
-0.07218990474939346,
0.024786461144685745,
-0.024393541738390923,
-0.07184619456529617,
-0.05290238931775093,
-0.0046716900542378426,
0.07039698958396912,
-0.026483241468667984,
-0.08655443787574768,
0.01743553951382637,
0.026684986427426338,
0.035412728786468506,
0.0580722950398922,
-0.005150629673153162,
0.09040794521570206,
0.04284309223294258,
0.006729291286319494,
-0.09872977435588837,
-0.06417258828878403,
-0.005595340393483639,
-0.0021678342018276453,
0.015607373788952827,
0.01183579582720995,
-0.0006540809408761561,
0.023934639990329742,
-0.01562490314245224,
-0.04052746295928955,
-0.022605817764997482,
0.014310124330222607,
-0.017615772783756256,
0.002047727582976222,
-0.005626793950796127,
0.00684231286868453,
-0.043330054730176926,
0.05251670628786087,
-0.03989777714014053,
0.05223242938518524,
-0.02014220505952835,
-0.042794328182935715,
0.034671954810619354,
-0.041210826486349106,
0.022166835144162178,
0.039002858102321625,
-0.07539507746696472,
0.026553435251116753,
-0.09826754778623581,
0.06811502575874329,
0.07224775850772858,
-0.014344558119773865,
-0.052467502653598785,
0.05372041091322899,
0.06058655306696892,
0.0020414090249687433,
-0.04723915457725525,
0.035065628588199615,
0.008137532509863377,
0.08916117250919342,
-0.0727214589715004,
-0.0342639721930027,
-0.048854511231184006,
-3.775471490412239e-33,
0.05313943326473236,
0.04305008053779602,
-0.05823977664113045,
-0.00009764847345650196,
-0.02331985905766487,
-0.021084459498524666,
0.06289629638195038,
0.11098223924636841,
0.034610096365213394,
-0.07745017111301422,
0.04446462541818619,
-0.04335867986083031,
-0.02491348795592785,
-0.04773290082812309,
0.00971356499940157,
-0.06537485867738724,
-0.03431777283549309,
-0.09389394521713257,
-0.07662073522806168,
0.02928386628627777,
0.0449359230697155,
0.10793488472700119,
-0.0799596831202507,
-0.03188371658325195,
-0.08235393464565277,
0.0771137923002243,
0.014489790424704552,
0.08386736363172531,
-0.01807500049471855,
-0.034412845969200134,
-0.00416716281324625,
-0.02984681911766529,
-0.03797674551606178,
0.0401490293443203,
-0.021445035934448242,
-0.017789263278245926,
0.015559236519038677,
-0.03495216369628906,
-0.06394880264997482,
0.023049376904964447,
0.06743930280208588,
0.0584908165037632,
-0.14029498398303986,
0.04107341915369034,
-0.05186110734939575,
-0.06160234659910202,
0.009030373767018318,
0.059879809617996216,
0.03140905126929283,
0.015223289839923382,
-0.04388609156012535,
-0.06279244273900986,
-0.16742870211601257,
0.0033041732385754585,
-0.05502355471253395,
0.03832508623600006,
0.06009453162550926,
0.00783238373696804,
-0.020880701020359993,
0.008546411991119385,
0.01783137395977974,
-0.0077778249979019165,
-0.11872265487909317,
-0.03937671333551407,
0.046732522547245026,
0.032406073063611984,
-0.03579559922218323,
-0.016533268615603447,
0.07639841735363007,
0.05379829555749893,
0.062492843717336655,
0.030097542330622673,
0.015175078064203262,
-0.01308699045330286,
0.009988000616431236,
-0.014065471477806568,
0.015022329054772854,
-0.000832721998449415,
0.07844949513673782,
-0.01638791710138321,
-0.06782054156064987,
-0.0159755926579237,
0.06747075170278549,
0.1449117213487625,
0.08604201674461365,
0.12025852501392365,
0.0033352356404066086,
0.004978467710316181,
0.022128183394670486,
0.04306325688958168,
-0.029185257852077484,
0.003305260092020035,
0.037454959005117416,
0.13142135739326477,
0.033482085913419724,
-5.925172530396594e-8,
-0.08753668516874313,
0.046505946666002274,
-0.09429202973842621,
-0.03722809627652168,
-0.06709554046392441,
-0.0005361753283068538,
0.012834900990128517,
0.04628362879157066,
-0.024526841938495636,
-0.005841113161295652,
0.02076331339776516,
0.05533311888575554,
-0.06788107752799988,
0.07795605063438416,
0.01906345970928669,
-0.050241321325302124,
-0.04609951004385948,
0.05325551703572273,
-0.0908169075846672,
0.02435285784304142,
-0.06666424125432968,
-0.019493835046887398,
0.025052523240447044,
0.007225788198411465,
-0.0023370643611997366,
-0.04916628450155258,
-0.11010751873254776,
0.04541908577084541,
-0.027080226689577103,
-0.05097498744726181,
-0.06895910203456879,
0.07447463274002075,
0.03927452117204666,
-0.03505828231573105,
0.0732969120144844,
0.06303782016038895,
-0.059276726096868515,
-0.01349326130002737,
0.006901073269546032,
-0.03794148564338684,
0.05487445741891861,
-0.056183017790317535,
-0.04156259819865227,
0.019309036433696747,
0.051896095275878906,
0.011806869879364967,
0.0983087345957756,
-0.09520160406827927,
-0.03453606739640236,
0.04976283386349678,
0.06440486758947372,
-0.012220256961882114,
-0.04697399213910103,
0.06498965620994568,
0.02522752620279789,
-0.02334911935031414,
0.024505294859409332,
-0.08982868492603302,
0.013647849671542645,
0.0883297473192215,
0.06391073763370514,
-0.030151275917887688,
-0.027106724679470062,
-0.010648754425346851
] |
hyunwoongko/kobart | b5a881942b2536ed7851752a77d7da36d58f2e49 | 2022-04-11T01:19:27.000Z | [
"pytorch",
"bart",
"text2text-generation",
"ko",
"transformers",
"license:mit",
"autotrain_compatible"
] | text2text-generation | false | hyunwoongko | null | hyunwoongko/kobart | 2,083 | 1 | transformers | ---
language: ko
tags:
- bart
license: mit
---
## KoBART-base-v2
With the addition of chatting data, the model is trained to handle the semantics of sequences longer than KoBART.
```python
from transformers import PreTrainedTokenizerFast, BartModel
tokenizer = PreTrainedTokenizerFast.from_pretrained('hyunwoongko/kobart')
model = BartModel.from_pretrained('hyunwoongko/kobart')
```
### Performance
NSMC
- acc. : 0.901
### hyunwoongko/kobart
- Added bos/eos post processor
- Removed token_type_ids
| [
-0.12081006914377213,
-0.0069268848747015,
0.025432558730244637,
-0.020224617794156075,
-0.017781516537070274,
-0.029650287702679634,
-0.009337184019386768,
0.0648166835308075,
0.005995850544422865,
-0.07163005322217941,
0.04287656024098396,
-0.040475111454725266,
-0.018176954239606857,
-0.028717784211039543,
0.07442307472229004,
0.01346746739000082,
-0.009047677740454674,
0.0025442689657211304,
-0.0373699925839901,
-0.08536656200885773,
0.07542728632688522,
0.03627076745033264,
-0.0004310652439016849,
0.044886596500873566,
0.042845938354730606,
-0.03010137937963009,
0.004150177352130413,
-0.03191051632165909,
0.04426125809550285,
0.012609857134521008,
0.03639949858188629,
0.07003969699144363,
0.02998024970293045,
0.048839692026376724,
-0.037615254521369934,
0.061738718301057816,
-0.0014609773643314838,
-0.07187295705080032,
0.05768517032265663,
-0.0012859496055170894,
0.02892722561955452,
0.008070211857557297,
-0.021589472889900208,
0.01480577141046524,
0.033785462379455566,
0.008421213366091251,
-0.03199447691440582,
-0.043948788195848465,
-0.04449324309825897,
-0.05130908265709877,
-0.0682227835059166,
-0.01638595387339592,
0.05527687817811966,
0.08251506835222244,
-0.018306788057088852,
-0.0046896906569600105,
-0.06302992254495621,
0.012115097604691982,
0.12274270504713058,
-0.05652613192796707,
-0.0422002449631691,
-0.003837308846414089,
0.0013353530084714293,
0.05530943349003792,
-0.0671619176864624,
-0.037610575556755066,
0.06079818680882454,
0.019800478592514992,
0.039856549352407455,
0.04711635410785675,
-0.010404709726572037,
0.016063077375292778,
0.009244304150342941,
0.11910530924797058,
0.07218720018863678,
-0.05160030350089073,
0.08906758576631546,
-0.003286150051280856,
0.015441606752574444,
-0.11476263403892517,
-0.011743144132196903,
-0.07229078561067581,
-0.011987599544227123,
0.025431787595152855,
-0.012340139597654343,
-0.040817633271217346,
-0.02999073453247547,
-0.028291825205087662,
0.04001913592219353,
0.04735228046774864,
-0.04965030401945114,
-0.09504663199186325,
0.056205809116363525,
-0.013573752716183662,
-0.079244464635849,
0.04692280292510986,
0.025328030809760094,
0.09213956445455551,
-0.07064475119113922,
0.1026974692940712,
0.010627157986164093,
0.07540710270404816,
0.0277725700289011,
0.017167380079627037,
-0.015021521598100662,
-0.05334373190999031,
0.0083461944013834,
0.018263962119817734,
-0.007362297736108303,
0.03995862603187561,
0.06235288456082344,
-0.06901004910469055,
0.0017576911486685276,
-0.055387143045663834,
0.035438574850559235,
0.07962267100811005,
-0.047843579202890396,
0.006296846549957991,
-0.03292739763855934,
0.10512176156044006,
0.07002757489681244,
-0.0276127140969038,
-0.029387131333351135,
0.014222190715372562,
-0.08647321909666061,
0.005837603472173214,
0.01473532896488905,
5.580383189356367e-33,
-0.01792745105922222,
-0.01672755554318428,
0.050900425761938095,
0.025678982958197594,
-0.024568375200033188,
-0.05283365398645401,
-0.0374651663005352,
-0.0019969558343291283,
-0.08808159828186035,
-0.055805787444114685,
-0.06705015897750854,
0.05540004000067711,
-0.08271031081676483,
0.06227993220090866,
0.00048708400572650135,
-0.032911136746406555,
-0.03649510070681572,
0.03504929691553116,
0.08868958801031113,
0.03702967241406441,
0.11701598763465881,
-0.028224926441907883,
-0.05012715980410576,
0.001977210631594062,
0.013140633702278137,
0.04671309515833855,
0.04761321470141411,
-0.12618745863437653,
-0.029850268736481667,
0.06984012573957443,
-0.0684535801410675,
0.04101261496543884,
-0.01847255416214466,
0.03314953297376633,
-0.03697700798511505,
-0.025361543521285057,
0.024517858400940895,
-0.09201478213071823,
-0.030926814302802086,
-0.15231071412563324,
-0.012959320098161697,
0.017112739384174347,
-0.07516211271286011,
-0.02717873826622963,
-0.057878997176885605,
-0.04560540243983269,
0.005128368269652128,
0.006373682990670204,
0.0695759579539299,
0.07589104771614075,
0.0005830711452290416,
-0.007219995371997356,
-0.033047452569007874,
0.05145702883601189,
0.0322926789522171,
0.0002400442463112995,
0.06456798315048218,
0.02807406149804592,
0.0694146603345871,
-0.0025039645843207836,
-0.01550831738859415,
0.045635830610990524,
0.04929053783416748,
0.05201225355267525,
0.07914993911981583,
-0.037807758897542953,
-0.035854946821928024,
-0.006533370353281498,
0.005115497391670942,
-0.060547225177288055,
-0.08484189212322235,
-0.06163956969976425,
-0.04800007492303848,
0.008758132345974445,
-0.01616610400378704,
-0.06328818947076797,
-0.004519662819802761,
-0.058256398886442184,
-0.13156536221504211,
0.029080942273139954,
-0.019125401973724365,
-0.032620977610349655,
-0.031254034489393234,
-0.005755688063800335,
0.04343191906809807,
-0.053131185472011566,
0.06396439671516418,
0.009336750954389572,
-0.060980647802352905,
-0.022087136283516884,
-0.0040803151205182076,
-0.025225885212421417,
0.002277305582538247,
0.026437409222126007,
-0.05463059991598129,
-6.947461813527578e-33,
0.0022007818333804607,
0.057433389127254486,
-0.015403293073177338,
0.026955479755997658,
-0.01568767800927162,
-0.029183190315961838,
0.05184045806527138,
0.13640213012695312,
-0.04971718788146973,
-0.006607407238334417,
0.006163895595818758,
-0.13268133997917175,
0.006117352284491062,
-0.052182167768478394,
0.12547434866428375,
0.02741502970457077,
-0.045523352921009064,
0.012604924850165844,
0.0034859618172049522,
0.01809491217136383,
0.025382041931152344,
0.05949072167277336,
-0.14205649495124817,
0.08325419574975967,
-0.040548041462898254,
0.06024211272597313,
-0.022370390594005585,
0.09597991406917572,
0.023408975452184677,
0.0018766849534586072,
-0.041615311056375504,
-0.0008406464476138353,
-0.04323931783437729,
0.060274265706539154,
-0.047552455216646194,
-0.016022440046072006,
0.04265090450644493,
0.0488552562892437,
-0.025345927104353905,
0.011859710328280926,
0.0877302885055542,
0.03145122528076172,
-0.10273117572069168,
0.03146137297153473,
-0.011800169944763184,
-0.020868081599473953,
-0.017049239948391914,
-0.050282761454582214,
0.083527110517025,
-0.06408890336751938,
0.0659901425242424,
0.022464986890554428,
-0.07057465612888336,
-0.04063558578491211,
-0.06673986464738846,
-0.029500262811779976,
0.05950965732336044,
0.004342384170740843,
-0.038875043392181396,
-0.11448778957128525,
-0.041788071393966675,
-0.08163920044898987,
0.07310955971479416,
-0.038918379694223404,
0.031876496970653534,
-0.10318701714277267,
-0.03583923354744911,
0.005175756756216288,
0.014686883427202702,
-0.07973426580429077,
0.0027854852378368378,
0.0270791444927454,
0.025637909770011902,
-0.029774677008390427,
0.000021300411390257068,
0.007579723838716745,
-0.05768485367298126,
-0.010797123424708843,
0.02041313610970974,
-0.012146864086389542,
-0.117097407579422,
-0.003639375790953636,
0.10890588164329529,
0.11586187034845352,
-0.023019786924123764,
0.026000358164310455,
0.0520298071205616,
0.07824308425188065,
0.06598889827728271,
-0.021007966250181198,
0.016302872449159622,
0.03979228064417839,
0.007326054852455854,
0.08650940656661987,
-0.004629983566701412,
-5.021266957783155e-8,
-0.022061387076973915,
-0.10175414383411407,
-0.046622201800346375,
0.07048894464969635,
0.02066129632294178,
0.012645356357097626,
-0.06381914764642715,
0.01117241382598877,
-0.026553725823760033,
-0.04608387500047684,
0.0005767134134657681,
0.046993374824523926,
-0.04428529366850853,
0.018474208191037178,
0.059249576181173325,
0.015575251542031765,
-0.04309528321027756,
0.09072897583246231,
-0.053597331047058105,
-0.04106384515762329,
-0.014719897881150246,
-0.02795247547328472,
0.009317103773355484,
-0.005878838244825602,
-0.022835558280348778,
0.006430891342461109,
-0.0028690171893686056,
0.09778359532356262,
-0.005984625779092312,
-0.0527828074991703,
-0.07405263185501099,
0.001081165042705834,
-0.0748610645532608,
-0.00555403484031558,
-0.005726037546992302,
0.03921182453632355,
0.0015669104177504778,
-0.045999735593795776,
-0.029827609658241272,
0.07399794459342957,
-0.044697392731904984,
-0.026878900825977325,
-0.11831720173358917,
0.020196402445435524,
0.0537821389734745,
0.026549672707915306,
-0.023943757638335228,
-0.011705618351697922,
0.02770640328526497,
-0.007343416567891836,
-0.050612371414899826,
-0.019247395917773247,
-0.055654559284448624,
-0.022194579243659973,
-0.01927300915122032,
0.041729021817445755,
-0.05997693911194801,
-0.04367661848664284,
-0.0036008793395012617,
0.02502472884953022,
0.03233495354652405,
0.020404765382409096,
0.06003916263580322,
0.011087439954280853
] |
richielleisart/Childe | 5da9de8d7f9e9cfde2c126b6ac6531b3ddff606a | 2022-01-19T18:52:50.000Z | [
"pytorch",
"gpt2",
"text-generation",
"transformers",
"conversational"
] | conversational | false | richielleisart | null | richielleisart/Childe | 2,080 | null | transformers | ---
tags:
- conversational
---
# Childe Chatbot Model | [
-0.08342590183019638,
-0.01777823083102703,
0.04956606775522232,
0.03118353709578514,
0.047361504286527634,
-0.08925030380487442,
0.08672982454299927,
0.012996003031730652,
0.0705133005976677,
-0.012105018831789494,
0.0010526645928621292,
-0.02044222690165043,
-0.004734580870717764,
0.04789955914020538,
0.07216192036867142,
0.06365247815847397,
0.05846608430147171,
-0.03514132276177406,
-0.008041972294449806,
-0.04475462809205055,
0.0179605595767498,
0.10268010199069977,
0.06439937651157379,
0.01193477027118206,
-0.0005066842422820628,
0.0707617998123169,
-0.022742513567209244,
-0.0009815257508307695,
0.04205695912241936,
-0.008337927050888538,
0.04860885441303253,
0.05701566860079765,
0.044379085302352905,
0.07985548675060272,
-0.013880645856261253,
0.0396929495036602,
0.03670274466276169,
-0.013096727430820465,
0.03231976553797722,
0.014024506323039532,
-0.0489455908536911,
-0.0011231827083975077,
-0.09395167231559753,
-0.0690259337425232,
-0.004895724821835756,
-0.02979600802063942,
-0.09589990973472595,
-0.035736147314310074,
-0.00502714654430747,
0.030305147171020508,
-0.08677757531404495,
-0.04728717729449272,
0.03280142322182655,
0.13927756249904633,
-0.04639167711138725,
0.04616974666714668,
-0.06777554005384445,
-0.0765509232878685,
0.11400686949491501,
0.01203970517963171,
-0.049876268953084946,
0.007357686758041382,
-0.04433467984199524,
0.010219712741672993,
-0.04578985646367073,
-0.004383676219731569,
-0.058512959629297256,
0.0017066745785996318,
-0.005545696243643761,
0.05652330070734024,
0.014522210694849491,
0.03465273976325989,
0.03494110330939293,
0.0669131726026535,
-0.013649395667016506,
0.019479546695947647,
-0.005523153115063906,
-0.028696462512016296,
0.034473076462745667,
-0.04979288578033447,
-0.11021257191896439,
-0.06837634742259979,
0.040918782353401184,
-0.03771364688873291,
-0.011926167644560337,
0.0005848607397638261,
0.021875562146306038,
-0.008608253672719002,
-0.014251855202019215,
0.02617807500064373,
-0.07365155965089798,
-0.07682190835475922,
0.12126260250806808,
0.09929309785366058,
-0.022279147058725357,
-0.00967106968164444,
0.009932163171470165,
-0.05044565722346306,
-0.0860336497426033,
0.062485795468091965,
0.013287621550261974,
0.03353692218661308,
0.03221924602985382,
-0.024952350184321404,
0.01711174100637436,
-0.025019660592079163,
0.012290273793041706,
0.025663968175649643,
0.019257154315710068,
-0.03922499716281891,
-0.06558003276586533,
-0.048955224454402924,
0.06066935136914253,
-0.012132962234318256,
0.06907011568546295,
-0.08041231334209442,
0.10635032504796982,
0.00602645892649889,
0.010713246650993824,
-0.020605823025107384,
0.11555544286966324,
0.0070497398264706135,
-0.046690717339515686,
-0.014738543890416622,
0.023307088762521744,
-0.022669311612844467,
-0.07273226231336594,
-2.0225915795393218e-33,
0.0656004250049591,
-0.0014419492799788713,
0.039851557463407516,
0.11773498356342316,
0.00790238194167614,
0.10836848616600037,
-0.032919805496931076,
-0.00047175533836707473,
-0.03597363457083702,
-0.019953038543462753,
-0.05617978796362877,
-0.04716712236404419,
-0.06078973039984703,
-0.014158680103719234,
0.03127771243453026,
-0.023198220878839493,
-0.06429125368595123,
0.03521563112735748,
0.024568693712353706,
0.02380252629518509,
-0.04042962193489075,
0.059885282069444656,
0.018346382305026054,
0.11954393982887268,
0.08373171836137772,
0.1439063847064972,
0.03397022932767868,
-0.09164534509181976,
-0.0022964882664382458,
0.03879236429929733,
-0.06082872673869133,
0.017435453832149506,
-0.03798740729689598,
0.03152284026145935,
-0.01719454862177372,
0.01309578400105238,
0.02021992951631546,
-0.04256522282958031,
-0.038306739181280136,
-0.03884422406554222,
-0.060570769011974335,
-0.06265228241682053,
-0.06033945083618164,
-0.10641515254974365,
0.007274631876498461,
-0.0012659822823479772,
-0.030764464288949966,
-0.02227337658405304,
0.032529011368751526,
-0.004849460907280445,
-0.012748966924846172,
0.0879993662238121,
-0.024809885770082474,
-0.007692351471632719,
-0.032029781490564346,
-0.04563721641898155,
-0.04251252859830856,
0.08074133098125458,
-0.0034935197327286005,
-0.07756298780441284,
0.012339073233306408,
-0.06017376855015755,
0.02703671343624592,
-0.09736540913581848,
0.06349632889032364,
-0.02190697379410267,
0.015858184546232224,
0.022099602967500687,
0.02306239679455757,
-0.036495957523584366,
-0.019151177257299423,
0.09777660667896271,
-0.0381673239171505,
0.030776074156165123,
-0.06603645533323288,
-0.016124235466122627,
0.004704839084297419,
-0.06190748140215874,
0.06001059338450432,
0.022520888596773148,
-0.08870386332273483,
-0.08434221893548965,
-0.030593475326895714,
-0.0521228089928627,
-0.0027343991678208113,
-0.09792134910821915,
0.009300070814788342,
-0.05130400508642197,
-0.018511388450860977,
0.021162165328860283,
-0.040987156331539154,
0.008079223334789276,
-0.051601167768239975,
0.026973500847816467,
-0.059172045439481735,
3.627755564210049e-34,
0.032253555953502655,
-0.023975001648068428,
-0.08937975019216537,
0.061808984726667404,
0.00021271109289955348,
-0.07042139023542404,
0.03954631835222244,
0.09601259976625443,
0.006101618520915508,
-0.0028962884098291397,
-0.061843082308769226,
-0.014948321506381035,
-0.03391087427735329,
-0.01450586598366499,
0.10562287271022797,
0.07550168037414551,
0.01742600090801716,
-0.05517175421118736,
0.050002023577690125,
-0.023026157170534134,
0.036447811871767044,
0.0645132064819336,
-0.13709361851215363,
0.05950004979968071,
0.07668891549110413,
-0.030139096081256866,
-0.04296833649277687,
0.05263037979602814,
0.0813538208603859,
0.002207805635407567,
0.005424292758107185,
0.03702962398529053,
0.06598500162363052,
0.0032602997962385416,
0.04171281307935715,
-0.015638191252946854,
-0.002343716798350215,
-0.0033806192222982645,
0.025260936468839645,
0.0054510533809661865,
0.0688881203532219,
-0.019296806305646896,
-0.03690072521567345,
0.02348029613494873,
-0.021200045943260193,
-0.022215649485588074,
-0.03473195433616638,
-0.011179603636264801,
-0.06023170426487923,
0.028091756626963615,
0.023546775802969933,
-0.038570985198020935,
-0.036891061812639236,
-0.12722636759281158,
-0.08076708018779755,
-0.007727603893727064,
0.056767914444208145,
0.005970808677375317,
-0.018154935911297798,
0.0015168034005910158,
-0.0028919570613652468,
-0.08217357099056244,
0.03532002121210098,
0.0037278018426150084,
-0.03434498608112335,
-0.05188244208693504,
-0.07268615812063217,
0.04367247223854065,
-0.00037583938683383167,
-0.060326021164655685,
0.153590589761734,
0.024404970929026604,
-0.014438840560615063,
0.04942543804645538,
0.0363161526620388,
-0.05178557336330414,
0.01618023030459881,
0.024607088416814804,
0.06538240611553192,
-0.04366676136851311,
-0.06749279797077179,
-0.008016654290258884,
0.04483357071876526,
0.04491153359413147,
0.03436700999736786,
-0.022383293136954308,
0.00751018151640892,
0.059769243001937866,
-0.04943397268652916,
0.00402828911319375,
0.04107936844229698,
-0.030038518831133842,
-0.00006890160875627771,
0.039428018033504486,
-0.03209582343697548,
-2.1084646917302052e-8,
-0.02074485458433628,
-0.09756335616111755,
-0.009194891899824142,
0.059880081564188004,
0.05520738661289215,
-0.006380544975399971,
0.006915569771081209,
0.012540247291326523,
-0.04225858300924301,
0.0007672336068935692,
0.02783939242362976,
0.06379061937332153,
-0.00915510393679142,
0.019687119871377945,
0.04314728453755379,
0.01839836873114109,
-0.05260644480586052,
0.011036364361643791,
0.00990042369812727,
-0.010463406331837177,
0.0980464518070221,
0.031923603266477585,
-0.06265126168727875,
0.08323924243450165,
-0.03418256714940071,
-0.05783955007791519,
-0.063123919069767,
0.07914601266384125,
-0.09212241321802139,
0.025788895785808563,
-0.00556894252076745,
0.03658032789826393,
-0.06655891984701157,
0.018860213458538055,
0.006959681864827871,
-0.0064959656447172165,
-0.095746248960495,
-0.025706207379698753,
-0.02433391474187374,
0.03263940289616585,
0.045856453478336334,
0.05234919860959053,
-0.021708955988287926,
-0.0637887716293335,
0.07312465459108353,
-0.0014228866202756763,
-0.05602685734629631,
-0.12110962718725204,
0.004072874318808317,
-0.03086153231561184,
-0.06443466991186142,
-0.0475088469684124,
0.02247135527431965,
-0.020099394023418427,
0.047346461564302444,
0.015244878828525543,
0.040803246200084686,
0.0010493180016055703,
0.015932966023683548,
0.04295290261507034,
0.07020635902881622,
0.12250029295682907,
0.05388657748699188,
0.006952969823032618
] |
nvidia/mit-b5 | 9707ed6bec8a37b67fc9b6d03fe6fbb0e8020f76 | 2022-07-29T13:15:56.000Z | [
"pytorch",
"tf",
"segformer",
"image-classification",
"dataset:imagenet_1k",
"arxiv:2105.15203",
"transformers",
"vision",
"license:apache-2.0"
] | image-classification | false | nvidia | null | nvidia/mit-b5 | 2,076 | null | transformers | ---
license: apache-2.0
tags:
- vision
datasets:
- imagenet_1k
widget:
- src: https://huggingface.co/datasets/hf-internal-testing/fixtures_ade20k/resolve/main/ADE_val_00000001.jpg
example_title: House
- src: https://huggingface.co/datasets/hf-internal-testing/fixtures_ade20k/resolve/main/ADE_val_00000002.jpg
example_title: Castle
---
# SegFormer (b5-sized) encoder pre-trained-only
SegFormer encoder fine-tuned on Imagenet-1k. It was introduced in the paper [SegFormer: Simple and Efficient Design for Semantic Segmentation with Transformers](https://arxiv.org/abs/2105.15203) by Xie et al. and first released in [this repository](https://github.com/NVlabs/SegFormer).
Disclaimer: The team releasing SegFormer did not write a model card for this model so this model card has been written by the Hugging Face team.
## Model description
SegFormer consists of a hierarchical Transformer encoder and a lightweight all-MLP decode head to achieve great results on semantic segmentation benchmarks such as ADE20K and Cityscapes. The hierarchical Transformer is first pre-trained on ImageNet-1k, after which a decode head is added and fine-tuned altogether on a downstream dataset.
This repository only contains the pre-trained hierarchical Transformer, hence it can be used for fine-tuning purposes.
## Intended uses & limitations
You can use the model for fine-tuning of semantic segmentation. See the [model hub](https://huggingface.co/models?other=segformer) to look for fine-tuned versions on a task that interests you.
### How to use
Here is how to use this model to classify an image of the COCO 2017 dataset into one of the 1,000 ImageNet classes:
```python
from transformers import SegformerFeatureExtractor, SegformerForImageClassification
from PIL import Image
import requests
url = "http://images.cocodataset.org/val2017/000000039769.jpg"
image = Image.open(requests.get(url, stream=True).raw)
feature_extractor = SegformerFeatureExtractor.from_pretrained("nvidia/mit-b5")
model = SegformerForImageClassification.from_pretrained("nvidia/mit-b5")
inputs = feature_extractor(images=image, return_tensors="pt")
outputs = model(**inputs)
logits = outputs.logits
# model predicts one of the 1000 ImageNet classes
predicted_class_idx = logits.argmax(-1).item()
print("Predicted class:", model.config.id2label[predicted_class_idx])
```
For more code examples, we refer to the [documentation](https://huggingface.co/transformers/model_doc/segformer.html#).
### BibTeX entry and citation info
```bibtex
@article{DBLP:journals/corr/abs-2105-15203,
author = {Enze Xie and
Wenhai Wang and
Zhiding Yu and
Anima Anandkumar and
Jose M. Alvarez and
Ping Luo},
title = {SegFormer: Simple and Efficient Design for Semantic Segmentation with
Transformers},
journal = {CoRR},
volume = {abs/2105.15203},
year = {2021},
url = {https://arxiv.org/abs/2105.15203},
eprinttype = {arXiv},
eprint = {2105.15203},
timestamp = {Wed, 02 Jun 2021 11:46:42 +0200},
biburl = {https://dblp.org/rec/journals/corr/abs-2105-15203.bib},
bibsource = {dblp computer science bibliography, https://dblp.org}
}
```
| [
-0.08144903182983398,
0.01023835502564907,
0.042061638087034225,
-0.024326853454113007,
0.07283542305231094,
-0.13717906177043915,
-0.01180750411003828,
-0.03150502219796181,
-0.08676953613758087,
-0.09427668154239655,
0.01944034919142723,
-0.06613258272409439,
0.0055740694515407085,
0.0019551333971321583,
0.03838624805212021,
0.08383958041667938,
-0.012067459523677826,
0.1248125359416008,
-0.08841819316148758,
-0.03575419634580612,
0.0015964561607688665,
0.016946325078606606,
0.00044648293987847865,
-0.05711384117603302,
0.03142648562788963,
-0.019211813807487488,
-0.03702438250184059,
0.006592562887817621,
0.01782643236219883,
-0.04827704653143883,
0.08936082571744919,
0.04898204654455185,
0.010360383428633213,
0.06982708722352982,
0.012770644389092922,
0.08390799909830093,
0.015147385187447071,
-0.0022078638430684805,
-0.06766558438539505,
0.012475837953388691,
-0.004848418291658163,
0.0029721001628786325,
-0.03870968520641327,
-0.05635969340801239,
-0.003886660560965538,
-0.03008401207625866,
0.019256675615906715,
-0.04687303304672241,
-0.060047734528779984,
-0.02243081107735634,
-0.04250388219952583,
-0.02848154492676258,
-0.029838286340236664,
0.06534148752689362,
0.0016394015401601791,
0.016611715778708458,
-0.004458597395569086,
-0.020881090313196182,
0.016775446012616158,
0.06243768334388733,
-0.021566512063145638,
0.01329526212066412,
0.014960304833948612,
-0.0025464631617069244,
-0.0788002759218216,
-0.011012694798409939,
0.14510920643806458,
-0.06586936116218567,
0.027061481028795242,
-0.06723170727491379,
-0.04605657979846001,
-0.001721890876069665,
-0.0011603744933381677,
-0.000870854826644063,
-0.02387259714305401,
0.05739641189575195,
0.018359344452619553,
0.012037244625389576,
0.09394960105419159,
-0.14363066852092743,
0.04917752742767334,
-0.032274141907691956,
0.039538390934467316,
0.03595392033457756,
0.07750554382801056,
0.023188717663288116,
-0.031715139746665955,
0.013551127165555954,
-0.04255210980772972,
0.08575768768787384,
-0.0035774772986769676,
-0.0751640796661377,
-0.049700718373060226,
0.018980853259563446,
0.031017497181892395,
-0.056754786521196365,
-0.0017451932653784752,
-0.05141132324934006,
-0.01945577748119831,
0.09473669528961182,
-0.051735006272792816,
-0.01443160604685545,
0.019857104867696762,
0.05122130736708641,
0.012545445933938026,
-0.0501762330532074,
0.024043550714850426,
0.08504996448755264,
0.04364928975701332,
-0.04067318141460419,
0.03789404034614563,
0.020502986386418343,
0.009835883043706417,
-0.13366307318210602,
0.0064151594415307045,
0.01931120455265045,
-0.042139388620853424,
0.004426427651196718,
0.07737120240926743,
-0.027206478640437126,
-0.02040611207485199,
0.02742018736898899,
-0.10587654262781143,
0.004307424649596214,
0.07045116275548935,
-0.01520761102437973,
-0.13225118815898895,
4.6556981906972766e-33,
0.07567203044891357,
0.0352594368159771,
0.0519808866083622,
-0.015019080601632595,
-0.023095807060599327,
-0.016851922497153282,
-0.02031131461262703,
0.027635211125016212,
-0.08556041866540909,
-0.03218039497733116,
-0.04420628771185875,
-0.0241678599268198,
0.004866597708314657,
0.11115720868110657,
0.054127342998981476,
-0.12757806479930878,
-0.01603773981332779,
0.010053672827780247,
-0.04614477977156639,
0.0357474610209465,
0.009438097476959229,
-0.012271908111870289,
-0.055403754115104675,
0.05065997689962387,
-0.12455322593450546,
-0.005349030718207359,
0.014897781424224377,
-0.025386804714798927,
0.057338789105415344,
0.01764543727040291,
-0.08423863351345062,
-0.005681012291461229,
0.08079119026660919,
0.011944527737796307,
0.013927889987826347,
-0.06646811217069626,
0.003868195228278637,
-0.0008663471089676023,
-0.05325326323509216,
-0.045596253126859665,
0.035591304302215576,
0.0720815360546112,
0.03854447230696678,
-0.0613153837621212,
-0.04660041630268097,
0.0035858035553246737,
-0.015839209780097008,
0.075782909989357,
0.004346764180809259,
0.013790858909487724,
0.07481760531663895,
0.0012518229195848107,
-0.015919944271445274,
-0.04062943160533905,
0.021521348506212234,
-0.00048472563503310084,
0.04183996468782425,
-0.012915320694446564,
0.013888521119952202,
0.07862451672554016,
-0.040951501578092575,
-0.02831573784351349,
0.024005603045225143,
0.017715737223625183,
-0.015823446214199066,
-0.020014997571706772,
0.04617694765329361,
-0.04881758987903595,
-0.09917346388101578,
-0.02970171347260475,
-0.052381038665771484,
0.08508031815290451,
0.00733846053481102,
-0.035696424543857574,
0.06632627546787262,
0.009602370671927929,
-0.02379775047302246,
0.014562204480171204,
-0.10165834426879883,
0.033761341124773026,
-0.1165553480386734,
0.10694379359483719,
0.03353949263691902,
-0.030905302613973618,
-0.04186420515179634,
-0.025833506137132645,
0.04928402602672577,
-0.03840005770325661,
0.00174740853253752,
-0.004763658624142408,
0.017960863187909126,
-0.040157124400138855,
-0.018626054748892784,
-0.046835631132125854,
-0.05257420614361763,
-3.551866383604931e-33,
0.049795810133218765,
0.04272934049367905,
-0.04636111482977867,
0.07394656538963318,
-0.07252296805381775,
-0.002599956002086401,
0.11803840100765228,
0.13427072763442993,
0.00813672412186861,
-0.06219000369310379,
0.05332479625940323,
0.040829624980688095,
0.005562352482229471,
-0.09479882568120956,
0.015883242711424828,
-0.026362545788288116,
-0.01603672280907631,
-0.05056421831250191,
0.008638858795166016,
0.06323408335447311,
0.0390324741601944,
0.12502172589302063,
-0.01929657906293869,
0.061490487307310104,
-0.03851708024740219,
0.06546447426080704,
-0.031226560473442078,
0.05184205248951912,
-0.030699143186211586,
-0.01716569997370243,
-0.0010871326085180044,
-0.0662965252995491,
-0.01933646947145462,
0.010505936108529568,
-0.03465614095330238,
0.03293401375412941,
0.06300602853298187,
-0.024161141365766525,
-0.06314633786678314,
0.013062190264463425,
-0.004889644216746092,
0.013220896013081074,
-0.03301858901977539,
0.11434426158666611,
-0.02943125180900097,
-0.03408704698085785,
-0.01804105006158352,
0.022570494562387466,
-0.007305428851395845,
0.04500667378306389,
-0.050851158797740936,
0.03338494524359703,
-0.10893111675977707,
-0.01799624413251877,
-0.03669595345854759,
-0.036834318190813065,
0.004275018349289894,
-0.043857309967279434,
-0.03207874670624733,
0.056958604604005814,
0.015476727858185768,
-0.019990159198641777,
-0.035037633031606674,
0.00461161183193326,
-0.005358564667403698,
0.030587289482355118,
-0.04588271304965019,
-0.04025471210479736,
0.0002126651379512623,
-0.0059396871365606785,
0.05869722738862038,
0.012539653107523918,
0.026360685005784035,
0.04610924422740936,
0.051190175116062164,
-0.04888865724205971,
0.0012716561323031783,
0.02105538733303547,
0.01877962425351143,
-0.019575875252485275,
-0.06817696988582611,
-0.006231353152543306,
0.03335370495915413,
0.11217577010393143,
0.05221504345536232,
0.037160031497478485,
0.009088702499866486,
0.02978089638054371,
0.06537862122058868,
-0.029184741899371147,
-0.05836266651749611,
-0.009406141005456448,
0.03650849312543869,
0.1502891331911087,
0.07306802272796631,
-6.177935318874006e-8,
-0.06503969430923462,
0.023973282426595688,
-0.07025089114904404,
-0.06583869457244873,
-0.02323160134255886,
-0.06173599138855934,
0.05937446653842926,
0.08599009364843369,
-0.01775727979838848,
-0.017450422048568726,
0.07419075071811676,
0.022343602031469345,
-0.12760567665100098,
0.028059743344783783,
0.02753170020878315,
-0.002770340535789728,
0.0894622877240181,
0.05067175254225731,
-0.046264275908470154,
-0.025374600663781166,
-0.06151258572936058,
0.009500403888523579,
-0.06467290222644806,
-0.04213562235236168,
0.05199321731925011,
-0.05018439516425133,
-0.030671337619423866,
0.04467495158314705,
0.054534051567316055,
-0.032162927091121674,
-0.01367371529340744,
0.01979505643248558,
0.057114433497190475,
-0.03055041842162609,
0.0366123802959919,
0.07346359640359879,
-0.11371123045682907,
-0.015070146881043911,
0.004889794159680605,
-0.002619426930323243,
0.04130427539348602,
-0.04236350208520889,
-0.029683256521821022,
-0.03024309314787388,
0.0811406746506691,
0.016848517581820488,
0.04188487306237221,
-0.03277009353041649,
0.0005373635212890804,
0.031106604263186455,
0.07263354957103729,
0.013385635800659657,
-0.04099644720554352,
0.0718187689781189,
0.03607851266860962,
-0.08051615953445435,
0.026562826707959175,
-0.020298143848776817,
0.0871710553765297,
0.07312358915805817,
0.005846165120601654,
0.033715687692165375,
-0.014369366690516472,
0.011005549691617489
] |
microsoft/deberta-v2-xlarge-mnli | 5272422ce68b8d61766079390b96b033a64414d2 | 2021-05-21T20:08:15.000Z | [
"pytorch",
"deberta-v2",
"text-classification",
"en",
"arxiv:2006.03654",
"transformers",
"deberta",
"deberta-mnli",
"license:mit"
] | text-classification | false | microsoft | null | microsoft/deberta-v2-xlarge-mnli | 2,075 | 2 | transformers | ---
language: en
tags:
- deberta
- deberta-mnli
tasks: mnli
thumbnail: https://huggingface.co/front/thumbnails/microsoft.png
license: mit
widget:
- text: "[CLS] I love you. [SEP] I like you. [SEP]"
---
## DeBERTa: Decoding-enhanced BERT with Disentangled Attention
[DeBERTa](https://arxiv.org/abs/2006.03654) improves the BERT and RoBERTa models using disentangled attention and enhanced mask decoder. It outperforms BERT and RoBERTa on majority of NLU tasks with 80GB training data.
Please check the [official repository](https://github.com/microsoft/DeBERTa) for more details and updates.
This the DeBERTa V2 xlarge model fine-tuned with MNLI task, 24 layers, 1536 hidden size. Total parameters 900M.
### Fine-tuning on NLU tasks
We present the dev results on SQuAD 1.1/2.0 and several GLUE benchmark tasks.
| Model | SQuAD 1.1 | SQuAD 2.0 | MNLI-m/mm | SST-2 | QNLI | CoLA | RTE | MRPC | QQP |STS-B |
|---------------------------|-----------|-----------|-------------|-------|------|------|--------|-------|-------|------|
| | F1/EM | F1/EM | Acc | Acc | Acc | MCC | Acc |Acc/F1 |Acc/F1 |P/S |
| BERT-Large | 90.9/84.1 | 81.8/79.0 | 86.6/- | 93.2 | 92.3 | 60.6 | 70.4 | 88.0/- | 91.3/- |90.0/- |
| RoBERTa-Large | 94.6/88.9 | 89.4/86.5 | 90.2/- | 96.4 | 93.9 | 68.0 | 86.6 | 90.9/- | 92.2/- |92.4/- |
| XLNet-Large | 95.1/89.7 | 90.6/87.9 | 90.8/- | 97.0 | 94.9 | 69.0 | 85.9 | 90.8/- | 92.3/- |92.5/- |
| [DeBERTa-Large](https://huggingface.co/microsoft/deberta-large)<sup>1</sup> | 95.5/90.1 | 90.7/88.0 | 91.3/91.1| 96.5|95.3| 69.5| 91.0| 92.6/94.6| 92.3/- |92.8/92.5 |
| [DeBERTa-XLarge](https://huggingface.co/microsoft/deberta-xlarge)<sup>1</sup> | -/- | -/- | 91.5/91.2| 97.0 | - | - | 93.1 | 92.1/94.3 | - |92.9/92.7|
| [DeBERTa-V2-XLarge](https://huggingface.co/microsoft/deberta-v2-xlarge)<sup>1</sup>|95.8/90.8| 91.4/88.9|91.7/91.6| **97.5**| 95.8|71.1|**93.9**|92.0/94.2|92.3/89.8|92.9/92.9|
|**[DeBERTa-V2-XXLarge](https://huggingface.co/microsoft/deberta-v2-xxlarge)<sup>1,2</sup>**|**96.1/91.4**|**92.2/89.7**|**91.7/91.9**|97.2|**96.0**|**72.0**| 93.5| **93.1/94.9**|**92.7/90.3** |**93.2/93.1** |
--------
#### Notes.
- <sup>1</sup> Following RoBERTa, for RTE, MRPC, STS-B, we fine-tune the tasks based on [DeBERTa-Large-MNLI](https://huggingface.co/microsoft/deberta-large-mnli), [DeBERTa-XLarge-MNLI](https://huggingface.co/microsoft/deberta-xlarge-mnli), [DeBERTa-V2-XLarge-MNLI](https://huggingface.co/microsoft/deberta-v2-xlarge-mnli), [DeBERTa-V2-XXLarge-MNLI](https://huggingface.co/microsoft/deberta-v2-xxlarge-mnli). The results of SST-2/QQP/QNLI/SQuADv2 will also be slightly improved when start from MNLI fine-tuned models, however, we only report the numbers fine-tuned from pretrained base models for those 4 tasks.
- <sup>2</sup> To try the **XXLarge** model with **[HF transformers](https://huggingface.co/transformers/main_classes/trainer.html)**, you need to specify **--sharded_ddp**
```bash
cd transformers/examples/text-classification/
export TASK_NAME=mrpc
python -m torch.distributed.launch --nproc_per_node=8 run_glue.py --model_name_or_path microsoft/deberta-v2-xxlarge \\
--task_name $TASK_NAME --do_train --do_eval --max_seq_length 128 --per_device_train_batch_size 4 \\
--learning_rate 3e-6 --num_train_epochs 3 --output_dir /tmp/$TASK_NAME/ --overwrite_output_dir --sharded_ddp --fp16
```
### Citation
If you find DeBERTa useful for your work, please cite the following paper:
``` latex
@inproceedings{
he2021deberta,
title={DEBERTA: DECODING-ENHANCED BERT WITH DISENTANGLED ATTENTION},
author={Pengcheng He and Xiaodong Liu and Jianfeng Gao and Weizhu Chen},
booktitle={International Conference on Learning Representations},
year={2021},
url={https://openreview.net/forum?id=XPZIaotutsD}
}
```
| [
-0.11069395393133163,
-0.08945441246032715,
0.03497905284166336,
0.00196813209913671,
0.0229331087321043,
-0.01818605698645115,
-0.010388246737420559,
0.02470458298921585,
-0.018931392580270767,
0.03592129051685333,
0.013637084513902664,
-0.007165652699768543,
0.0002688775130081922,
0.01668824814260006,
0.04203568398952484,
0.10769359022378922,
0.11147819459438324,
-0.004799175076186657,
-0.13016177713871002,
-0.010431419126689434,
0.07888037711381912,
-0.010717717930674553,
0.0399203822016716,
-0.06847000122070312,
0.07608090341091156,
-0.014685031026601791,
-0.053682129830121994,
-0.06170407310128212,
0.048394959419965744,
-0.005310468841344118,
0.041461046785116196,
0.014912917278707027,
0.040568653494119644,
0.10973318666219711,
0.01579328626394272,
0.11200705170631409,
-0.020642664283514023,
-0.038166336715221405,
0.05095181241631508,
0.009914222173392773,
-0.058669015765190125,
0.08545678108930588,
-0.039405014365911484,
-0.049351371824741364,
0.05533093586564064,
-0.07110393792390823,
-0.013087318278849125,
-0.014993465505540371,
-0.030114253982901573,
-0.022010494023561478,
-0.09994622319936752,
-0.010996636003255844,
0.06155776605010033,
0.05111014097929001,
-0.003052211133763194,
-0.002278879750519991,
-0.005473962984979153,
-0.012602477334439754,
-0.03394477069377899,
-0.028491603210568428,
-0.0505559928715229,
-0.05663283169269562,
-0.03699847310781479,
-0.006518007256090641,
-0.020655812695622444,
0.0006827001343481243,
-0.015605091117322445,
-0.01804606430232525,
0.01407308503985405,
-0.02306704968214035,
-0.017239520326256752,
0.042942628264427185,
-0.028665747493505478,
-0.005812095012515783,
0.035448819398880005,
0.0038868116680532694,
0.04351154714822769,
-0.02745075896382332,
0.04434782639145851,
-0.13140127062797546,
0.04924706742167473,
-0.005021002143621445,
0.09133397042751312,
0.007458101958036423,
0.12286702543497086,
-0.008385466411709785,
0.025221852585673332,
0.0065148514695465565,
-0.02552284486591816,
-0.05195331573486328,
-0.046080537140369415,
-0.07043607532978058,
0.03861421346664429,
0.03467271849513054,
0.028694549575448036,
0.005374196916818619,
0.04900703206658363,
-0.007859023287892342,
-0.07343096286058426,
0.09604516625404358,
-0.01452245656400919,
0.02151457965373993,
-0.006101027596741915,
-0.039923813194036484,
-0.016785426065325737,
-0.024297550320625305,
0.11120723932981491,
0.03855794668197632,
0.005221696104854345,
-0.07352207601070404,
0.03447825834155083,
0.00211623078212142,
-0.0019718962721526623,
-0.013563917949795723,
0.02916853129863739,
-0.03861797973513603,
0.08933597803115845,
0.00847918726503849,
0.06296122074127197,
0.0160471610724926,
0.03162660822272301,
-0.02574898488819599,
-0.032972659915685654,
-0.012532510794699192,
-0.00575297512114048,
0.021887177601456642,
-0.015487384982407093,
4.723168627698599e-33,
0.056400470435619354,
0.04890439286828041,
-0.006077706813812256,
0.00602714903652668,
-0.009060973301529884,
-0.01617150753736496,
0.0068857092410326,
0.003330874489620328,
-0.04653312638401985,
0.012203707359731197,
-0.09490533918142319,
0.03399750962853432,
-0.057588763535022736,
0.10244100540876389,
-0.010503541678190231,
-0.03897282853722572,
-0.008162476122379303,
0.07127968966960907,
0.029976477846503258,
0.05976862087845802,
0.0924636647105217,
0.04647912085056305,
-0.01736726611852646,
-0.06942194700241089,
-0.029559947550296783,
0.019701387733221054,
0.1360614150762558,
-0.09060362726449966,
-0.03333911672234535,
0.04941025376319885,
-0.15835650265216827,
0.0157428327947855,
-0.022869599983096123,
0.02554037980735302,
0.0034343248698860407,
-0.03896990790963173,
-0.06373412907123566,
-0.036796245723962784,
0.03493940457701683,
-0.022437704727053642,
0.012895992957055569,
0.06975363194942474,
-0.026677697896957397,
-0.053110796958208084,
-0.053205087780952454,
0.013988029211759567,
0.050407081842422485,
-0.02152593433856964,
0.02812720090150833,
-0.007846805267035961,
0.08080394566059113,
0.03809605911374092,
-0.09598950296640396,
-0.06146413832902908,
-0.00010384607594460249,
-0.058971524238586426,
0.13567912578582764,
0.07226593047380447,
0.10462437570095062,
0.0764397531747818,
0.015334569849073887,
-0.07893218100070953,
0.014940822497010231,
0.02632020227611065,
0.0785919651389122,
-0.031832076609134674,
-0.060820069164037704,
0.0027783033438026905,
0.018019279465079308,
-0.0158116165548563,
-0.0723089948296547,
-0.024542253464460373,
0.058565277606248856,
-0.015497565269470215,
0.04042024165391922,
-0.02020856738090515,
0.05159134790301323,
-0.095041923224926,
-0.0015106152277439833,
-0.001907003577798605,
-0.007013273425400257,
0.03784210607409477,
-0.06976748257875443,
-0.072773277759552,
-0.08902294188737869,
-0.06181436404585838,
0.02729816362261772,
-0.09418981522321701,
-0.023149341344833374,
0.02082771249115467,
0.016690729185938835,
-0.014355823397636414,
-0.022191304713487625,
-0.020417535677552223,
-0.03307567909359932,
-2.929071293111044e-33,
-0.023186249658465385,
0.04340533912181854,
-0.1019270122051239,
0.043054237961769104,
-0.026832086965441704,
-0.0002639681624714285,
0.01827685721218586,
0.1117931678891182,
0.025545740500092506,
-0.0010409173555672169,
0.04155060276389122,
-0.07783721387386322,
-0.020194942131638527,
0.010854127816855907,
-0.0014118183171376586,
0.05195723474025726,
0.06792924553155899,
0.009353578090667725,
-0.023829014971852303,
-0.04149381071329117,
0.017524171620607376,
0.05988927185535431,
0.005832731258124113,
0.043422847986221313,
-0.00736737996339798,
0.07075686752796173,
-0.03240106627345085,
0.05355878919363022,
0.005335692316293716,
-0.04627300426363945,
-0.024117378517985344,
0.008686195127665997,
-0.04601721093058586,
0.013483408838510513,
-0.06001873314380646,
0.05662619695067406,
-0.022022832185029984,
-0.011905928142368793,
-0.028135890141129494,
0.02555888146162033,
0.06762930005788803,
-0.049809958785772324,
-0.02990417182445526,
0.08027464151382446,
0.022060701623558998,
-0.06316795200109482,
-0.09972697496414185,
-0.09425968676805496,
-0.04519137367606163,
0.08131924271583557,
0.023325858637690544,
-0.004190854262560606,
-0.11295370012521744,
-0.021344659850001335,
-0.06205642968416214,
-0.07074335962533951,
0.043609246611595154,
-0.06330713629722595,
-0.004320378415286541,
0.0022085262462496758,
-0.0038518079090863466,
0.0153739545494318,
0.005657600238919258,
0.045680154114961624,
-0.011054350063204765,
0.018646974116563797,
0.030359884724020958,
0.00554583640769124,
0.002905026078224182,
0.048027828335762024,
0.04680145904421806,
-0.04440834000706673,
0.032691583037376404,
0.028801506385207176,
-0.0021952029783278704,
0.03970162197947502,
-0.017342770472168922,
-0.07564350962638855,
-0.0488792285323143,
-0.06184225156903267,
-0.08800704777240753,
-0.0524689145386219,
0.021009273827075958,
0.06340602785348892,
0.023569568991661072,
0.11604097485542297,
0.039837323129177094,
0.042898159474134445,
-0.04815521091222763,
0.01984167844057083,
-0.022956607863307,
-0.013294192962348461,
0.016277693212032318,
0.07563761621713638,
0.03389924392104149,
-5.0243393445725815e-8,
-0.08880047500133514,
0.01943020708858967,
-0.06584850698709488,
-0.0012655461905524135,
-0.07370530813932419,
-0.09442795813083649,
-0.07166825234889984,
0.06335504353046417,
0.018745185807347298,
-0.00732297683134675,
0.12161696702241898,
0.029235316440463066,
-0.11611414700746536,
0.030731575563549995,
0.06758258491754532,
0.0761796310544014,
0.0237172432243824,
0.03276576101779938,
-0.008213397115468979,
-0.05623120069503784,
0.06651309132575989,
0.035070180892944336,
0.007652658503502607,
-0.03876035660505295,
0.007682169787585735,
-0.046466246247291565,
-0.06350376456975937,
0.11332139372825623,
0.024804051965475082,
-0.03075558878481388,
0.03217804804444313,
0.0645592212677002,
-0.05126536637544632,
-0.05372363328933716,
0.027881259098649025,
0.024898072704672813,
-0.006549658253788948,
-0.0399150624871254,
-0.03001454472541809,
0.06481634825468063,
0.07326559722423553,
0.03972134366631508,
-0.08384232968091965,
0.0022425311617553234,
0.07942907512187958,
0.01753813773393631,
-0.00007490663847420365,
-0.10663294792175293,
0.03446267917752266,
-0.014250273816287518,
0.055943358689546585,
-0.053039487451314926,
-0.03722366690635681,
0.0982694923877716,
-0.032068125903606415,
0.029707007110118866,
-0.0373808890581131,
-0.010109822265803814,
0.04554405435919762,
0.030528763309121132,
-0.010623874142765999,
0.024790359660983086,
-0.048284485936164856,
0.00968837644904852
] |
hf-internal-testing/tiny-random-longformer | 5690941b3c077e091b13b5f992b42e2ead18b35d | 2021-09-17T19:24:34.000Z | [
"pytorch",
"tf",
"longformer",
"transformers"
] | null | false | hf-internal-testing | null | hf-internal-testing/tiny-random-longformer | 2,070 | 1 | transformers | Entry not found | [
0.0461147278547287,
-0.038838207721710205,
-0.01049656979739666,
-0.03682169318199158,
0.011261860840022564,
0.013094935566186905,
0.0019101888174191117,
-0.013979103416204453,
0.027092741802334785,
-0.015212527476251125,
0.017284274101257324,
-0.08189476281404495,
0.03817418962717056,
-0.04920130595564842,
0.021389011293649673,
-0.015245908871293068,
-0.03203780576586723,
-0.1245758980512619,
0.03150877356529236,
0.032381657510995865,
-0.060957908630371094,
0.05409295856952667,
-0.025087490677833557,
0.01568586938083172,
0.028129950165748596,
-0.04710396006703377,
-0.018688226118683815,
0.013785239309072495,
-0.04001208767294884,
0.01173911802470684,
-0.04317743331193924,
0.05500618368387222,
0.004543041344732046,
0.02973111905157566,
0.14852192997932434,
0.02658126689493656,
0.02907961793243885,
-0.05169107764959335,
0.05803573504090309,
-0.07732241600751877,
-0.017637968063354492,
-0.04219653457403183,
0.041807834059000015,
0.023620979860424995,
0.021563321352005005,
0.016478516161441803,
-0.0021814992651343346,
-0.06400240957736969,
0.06393089145421982,
0.019599027931690216,
-0.08565037697553635,
0.00934905931353569,
-0.008718925528228283,
-0.028583496809005737,
-0.07310017943382263,
0.09416428208351135,
0.001759322709403932,
0.06184990331530571,
0.011840506456792355,
-0.035997264087200165,
0.08358278125524521,
-0.02619801089167595,
0.03736566752195358,
-0.028206506744027138,
-0.07454850524663925,
-0.08883563429117203,
-0.06279942393302917,
-0.008695344440639019,
0.014119276776909828,
-0.0825355276465416,
0.0649217739701271,
-0.00223911227658391,
-0.14716917276382446,
0.07743025571107864,
-0.03548373281955719,
-0.055201586335897446,
0.006981803569942713,
-0.012166670523583889,
0.055111464112997055,
-0.007116836030036211,
-0.023175746202468872,
-0.005835152696818113,
-0.09185640513896942,
0.055196937173604965,
0.034148022532463074,
0.03835180774331093,
0.038685429841279984,
-0.025987252593040466,
0.017804903909564018,
0.022428328171372414,
0.025005368515849113,
-0.10761535167694092,
-0.048001550137996674,
-0.04343584179878235,
0.012374646961688995,
-0.019502125680446625,
0.029218152165412903,
0.0842173621058464,
-0.011719699949026108,
0.09283553808927536,
-0.007015465293079615,
-0.03543110564351082,
-0.06936459988355637,
0.09425332397222519,
-0.010958523489534855,
-0.00805904995650053,
0.004974212497472763,
-0.0031528924591839314,
0.06105927750468254,
-0.03964288905262947,
-0.03619541600346565,
-0.019901901483535767,
0.07134733349084854,
0.039514873176813126,
-0.012729483656585217,
-0.006646515801548958,
-0.04746140539646149,
-0.014432490803301334,
-0.05157482624053955,
0.09506245702505112,
-0.049747664481401443,
-0.04591796174645424,
-0.008965466171503067,
-0.0325421579182148,
-0.08626784384250641,
-0.06624380499124527,
0.02538885548710823,
-4.303924894057984e-33,
0.01133066974580288,
0.0033434738870710135,
-0.002155609894543886,
0.04871906340122223,
-0.023564351722598076,
-0.07933273911476135,
0.0600903145968914,
0.02335330657660961,
-0.03844716399908066,
-0.020433755591511726,
-0.06952055543661118,
-0.03235611692070961,
0.0062485747039318085,
0.064804308116436,
-0.03201229125261307,
0.061689723283052444,
0.0417000837624073,
-0.00761845987290144,
0.03340127319097519,
-0.047770582139492035,
0.00887306872755289,
-0.04066338762640953,
-0.010506896302103996,
0.0106519665569067,
0.021333497017621994,
0.12854498624801636,
-0.009705503471195698,
0.010055632330477238,
-0.017507633194327354,
0.006515394430607557,
0.06334009766578674,
-0.057817306369543076,
0.013668818399310112,
-0.020286159589886665,
0.05430467426776886,
-0.023184705525636673,
0.0828516036272049,
0.0005449643940664828,
-0.10372652113437653,
-0.07634282112121582,
-0.005381610710173845,
-0.039263784885406494,
0.0006114727002568543,
-0.013281986117362976,
0.07119110971689224,
0.043696220964193344,
0.03168422728776932,
0.04338686540722847,
0.05728672817349434,
0.0832006186246872,
-0.07961414009332657,
0.015234283171594143,
0.017002005130052567,
0.047004107385873795,
-0.09794387966394424,
0.004990279674530029,
-0.07062993198633194,
-0.028000490739941597,
-0.04018733277916908,
-0.0702052190899849,
0.011351344175636768,
0.06020182743668556,
-0.03297270089387894,
0.09396500885486603,
0.03417910635471344,
-0.019825750961899757,
-0.034690454602241516,
-0.013036907650530338,
0.05896938592195511,
-0.012359356507658958,
-0.017275206744670868,
-0.07982361316680908,
0.02059139870107174,
0.06737419217824936,
0.04176458343863487,
-0.04978838190436363,
-0.05877475067973137,
-0.06289287656545639,
-0.03354167565703392,
-0.03871942684054375,
0.009898529388010502,
-0.05514208599925041,
-0.11629002541303635,
-0.011855563148856163,
0.10663620382547379,
0.037354156374931335,
-0.0065480442717671394,
-0.051189567893743515,
0.06663123518228531,
0.01874656230211258,
0.032841797918081284,
0.041593004018068314,
-0.06879369914531708,
0.04216769337654114,
-0.01628219522535801,
5.4139394340936695e-34,
0.05697013810276985,
-0.006972255185246468,
0.015711724758148193,
-0.17956365644931793,
0.02320219948887825,
0.007923615165054798,
-0.008062449283897877,
0.0074974060989916325,
0.07391711324453354,
0.0309313777834177,
0.060510627925395966,
0.058605875819921494,
0.09515274316072464,
-0.002282935893163085,
0.001603541080839932,
0.07024981826543808,
0.012629246339201927,
0.07425693422555923,
-0.038426291197538376,
0.01861148327589035,
0.030608950182795525,
-0.02449394389986992,
0.021528491750359535,
-0.003039651783183217,
-0.03676343336701393,
0.03130284696817398,
0.07998586446046829,
0.010451192036271095,
-0.07930229604244232,
-0.013543923385441303,
0.018781835213303566,
0.05168003588914871,
-0.07191970944404602,
0.15783067047595978,
0.026191607117652893,
0.01262354850769043,
0.08218053728342056,
-0.029807550832629204,
-0.07528624683618546,
-0.04250097647309303,
0.017244765534996986,
0.04411793500185013,
0.03708017244935036,
0.009233047254383564,
-0.040271829813718796,
0.022496428340673447,
0.02495843544602394,
0.07633638381958008,
0.005147108342498541,
0.013892097398638725,
0.05610476806759834,
-0.06684739887714386,
0.05862557515501976,
-0.020688841119408607,
0.05377643182873726,
0.06718500703573227,
0.005329249892383814,
-0.01388032827526331,
0.029931528493762016,
0.009508464485406876,
-0.045173756778240204,
0.11534366756677628,
-0.06510116159915924,
0.05117698386311531,
-0.0026125339791178703,
-0.08554837852716446,
-0.03784770518541336,
0.0804959163069725,
0.011298024095594883,
-0.07695550471544266,
-0.04868878796696663,
0.02515520341694355,
0.06252261996269226,
-0.04509226232767105,
-0.01246943511068821,
0.028559505939483643,
-0.030573077499866486,
0.05066261067986488,
-0.08187384903430939,
0.04469604790210724,
0.0034051244147121906,
0.04145054519176483,
-0.021858664229512215,
-0.06112268194556236,
-0.00908052921295166,
-0.05903250351548195,
0.0259539932012558,
0.059690944850444794,
-0.07613514363765717,
-0.03720718249678612,
-0.036316655576229095,
0.07058046013116837,
-0.008224100805819035,
0.041961874812841415,
-0.0285952128469944,
-1.496900736697171e-8,
-0.0014124972512945533,
0.03401879221200943,
-0.040338415652513504,
0.04116074740886688,
0.0935964286327362,
-0.05115952715277672,
0.0008746005478315055,
-0.03389839455485344,
-0.00567849725484848,
-0.010686947964131832,
-0.04789939522743225,
-0.04820054769515991,
-0.02011880651116371,
-0.03209094703197479,
-0.04211259260773659,
-0.10229527950286865,
-0.07819421589374542,
-0.031228765845298767,
-0.02154778689146042,
-0.04960230365395546,
0.08087796717882156,
-0.07801242172718048,
0.06919731199741364,
-0.04999840259552002,
0.03687043860554695,
0.03889009356498718,
-0.049989692866802216,
-0.04254625365138054,
-0.04606937617063522,
0.08682432025671005,
-0.031148413196206093,
0.11826753616333008,
0.034102488309144974,
-0.0208592489361763,
-0.0205202866345644,
0.027134142816066742,
0.09741277992725372,
0.051608603447675705,
0.013477512635290623,
-0.13649295270442963,
-0.022304272279143333,
0.02385953813791275,
0.038732077926397324,
-0.09249968826770782,
-0.04549082741141319,
0.054220106452703476,
0.01160438358783722,
0.051190607249736786,
0.07713303714990616,
-0.022097084671258926,
-0.06127818301320076,
-0.01857956498861313,
0.006740490905940533,
-0.00496308971196413,
0.024095389991998672,
0.0736224576830864,
-0.003481915919110179,
-0.0699305310845375,
-0.006629763171076775,
-0.0598808117210865,
0.05297163128852844,
-0.02902800403535366,
-0.027858933433890343,
-0.01287526823580265
] |
CAMeL-Lab/bert-base-arabic-camelbert-mix-sentiment | 6ef16021f303c8a2bac02fd5af16601593e665d2 | 2021-10-17T12:09:14.000Z | [
"pytorch",
"tf",
"bert",
"text-classification",
"ar",
"arxiv:2103.06678",
"transformers",
"license:apache-2.0"
] | text-classification | false | CAMeL-Lab | null | CAMeL-Lab/bert-base-arabic-camelbert-mix-sentiment | 2,064 | 2 | transformers | ---
language:
- ar
license: apache-2.0
widget:
- text: "أنا بخير"
---
# CAMeLBERT Mix SA Model
## Model description
**CAMeLBERT Mix SA Model** is a Sentiment Analysis (SA) model that was built by fine-tuning the [CAMeLBERT Mix](https://huggingface.co/CAMeL-Lab/bert-base-arabic-camelbert-mix/) model.
For the fine-tuning, we used the [ASTD](https://aclanthology.org/D15-1299.pdf), [ArSAS](http://lrec-conf.org/workshops/lrec2018/W30/pdf/22_W30.pdf), and [SemEval](https://aclanthology.org/S17-2088.pdf) datasets.
Our fine-tuning procedure and the hyperparameters we used can be found in our paper *"[The Interplay of Variant, Size, and Task Type in Arabic Pre-trained Language Models](https://arxiv.org/abs/2103.06678)."* Our fine-tuning code can be found [here](https://github.com/CAMeL-Lab/CAMeLBERT).
## Intended uses
You can use the CAMeLBERT Mix SA model directly as part of our [CAMeL Tools](https://github.com/CAMeL-Lab/camel_tools) SA component (*recommended*) or as part of the transformers pipeline.
#### How to use
To use the model with the [CAMeL Tools](https://github.com/CAMeL-Lab/camel_tools) SA component:
```python
>>> from camel_tools.sentiment import SentimentAnalyzer
>>> sa = SentimentAnalyzer("CAMeL-Lab/bert-base-arabic-camelbert-mix-sentiment")
>>> sentences = ['أنا بخير', 'أنا لست بخير']
>>> sa.predict(sentences)
>>> ['positive', 'negative']
```
You can also use the SA model directly with a transformers pipeline:
```python
>>> from transformers import pipeline
>>> sa = pipeline('sentiment-analysis', model='CAMeL-Lab/bert-base-arabic-camelbert-mix-sentiment')
>>> sentences = ['أنا بخير', 'أنا لست بخير']
>>> sa(sentences)
[{'label': 'positive', 'score': 0.9616648554801941},
{'label': 'negative', 'score': 0.9779177904129028}]
```
*Note*: to download our models, you would need `transformers>=3.5.0`.
Otherwise, you could download the models manually.
## Citation
```bibtex
@inproceedings{inoue-etal-2021-interplay,
title = "The Interplay of Variant, Size, and Task Type in {A}rabic Pre-trained Language Models",
author = "Inoue, Go and
Alhafni, Bashar and
Baimukan, Nurpeiis and
Bouamor, Houda and
Habash, Nizar",
booktitle = "Proceedings of the Sixth Arabic Natural Language Processing Workshop",
month = apr,
year = "2021",
address = "Kyiv, Ukraine (Online)",
publisher = "Association for Computational Linguistics",
abstract = "In this paper, we explore the effects of language variants, data sizes, and fine-tuning task types in Arabic pre-trained language models. To do so, we build three pre-trained language models across three variants of Arabic: Modern Standard Arabic (MSA), dialectal Arabic, and classical Arabic, in addition to a fourth language model which is pre-trained on a mix of the three. We also examine the importance of pre-training data size by building additional models that are pre-trained on a scaled-down set of the MSA variant. We compare our different models to each other, as well as to eight publicly available models by fine-tuning them on five NLP tasks spanning 12 datasets. Our results suggest that the variant proximity of pre-training data to fine-tuning data is more important than the pre-training data size. We exploit this insight in defining an optimized system selection model for the studied tasks.",
}
``` | [
-0.07104498893022537,
-0.05099228024482727,
0.04781763255596161,
0.05118279159069061,
-0.04921862855553627,
0.03468291833996773,
0.045174747705459595,
-0.028838014230132103,
0.012287568300962448,
-0.013280537910759449,
0.030459383502602577,
-0.061211880296468735,
0.06807728856801987,
-0.04698414355516434,
0.039403513073921204,
0.004191813059151173,
0.10704003274440765,
-0.040582336485385895,
-0.1053663119673729,
-0.05543765053153038,
0.049167219549417496,
0.07744160294532776,
0.059980589896440506,
0.021994054317474365,
-0.002900769468396902,
-0.027907423675060272,
-0.04651282727718353,
-0.007162650115787983,
0.050122447311878204,
0.012796296738088131,
0.003236629068851471,
0.0879555493593216,
0.008839888498187065,
0.09589648991823196,
-0.03521716594696045,
0.08617547899484634,
-0.028245773166418076,
0.0010724276071414351,
0.05792837589979172,
0.07928454130887985,
-0.014456963166594505,
-0.03785812854766846,
0.016990188509225845,
-0.011247131042182446,
0.04766423627734184,
-0.06327860802412033,
-0.04330369457602501,
-0.0023363761138170958,
-0.025620145723223686,
0.05723489448428154,
-0.0981605127453804,
0.015552597120404243,
0.009419484995305538,
-0.033687032759189606,
-0.017874334007501602,
-0.12022005021572113,
-0.01950102671980858,
-0.011321134865283966,
0.013721696101129055,
-0.07209538668394089,
-0.05919482931494713,
-0.0606786273419857,
-0.06106007844209671,
-0.020206555724143982,
-0.03710930794477463,
-0.04966915398836136,
0.030920857563614845,
-0.04032212495803833,
0.0016629075398668647,
-0.009488733485341072,
-0.04614323377609253,
0.018399227410554886,
-0.018067387863993645,
0.07474827766418457,
-0.05815847963094711,
0.007038270588964224,
0.10021290928125381,
-0.07500465214252472,
-0.006010718643665314,
-0.03654327243566513,
-0.005915895104408264,
0.02176966518163681,
0.06053760275244713,
-0.03063773363828659,
0.10131991654634476,
-0.06390052288770676,
0.03273883834481239,
0.00935703981667757,
0.03584972769021988,
0.035826314240694046,
0.013633697293698788,
-0.04788009449839592,
0.045513927936553955,
-0.006528536789119244,
0.07109536975622177,
0.007934052497148514,
0.053640738129615784,
-0.014987927861511707,
-0.06381868571043015,
0.0825830027461052,
0.043714914470911026,
0.024461112916469574,
0.05643771216273308,
0.014235126785933971,
0.002712789224460721,
-0.04777961224317551,
-0.05704541504383087,
0.04152403771877289,
0.005659549031406641,
-0.15418162941932678,
-0.07793257385492325,
-0.030751999467611313,
-0.01644524559378624,
-0.039681095629930496,
0.025883732363581657,
0.01610706001520157,
-0.015504604205489159,
-0.07814700156450272,
-0.009598443284630775,
0.015610253438353539,
-0.021860778331756592,
-0.03661705553531647,
0.05134272947907448,
-0.016227737069129944,
-0.020264359191060066,
0.039223141968250275,
-0.030712256208062172,
1.1244327818091126e-34,
0.03746136277914047,
0.01366027444601059,
-0.003138404106721282,
0.002127915620803833,
-0.07204645872116089,
0.004138131160289049,
-0.04534147307276726,
0.03372780606150627,
-0.07735779881477356,
-0.047699056565761566,
-0.055347736924886703,
0.0456908755004406,
-0.05642475560307503,
0.06663481146097183,
0.030662715435028076,
-0.030985526740550995,
-0.027331126853823662,
-0.012809228152036667,
0.008730992674827576,
-0.04139077290892601,
0.07196509838104248,
0.06449010968208313,
0.036042552441358566,
-0.022188415750861168,
-0.06333872675895691,
-0.03128448873758316,
0.1269954890012741,
-0.05356968194246292,
-0.0645793229341507,
0.07069247215986252,
-0.10655640810728073,
0.0016745657194405794,
-0.03732464089989662,
-0.0031108150724321604,
-0.05069451034069061,
-0.04948112368583679,
-0.12396218627691269,
-0.008928894065320492,
0.038092371076345444,
-0.06321805715560913,
-0.013822613283991814,
0.06993386894464493,
0.10343969613313675,
-0.006665610242635012,
-0.056764405220746994,
-0.013300493359565735,
0.038128383457660675,
0.04664158821105957,
0.053029004484415054,
0.018205052241683006,
0.00981227122247219,
0.06894069910049438,
0.03336171805858612,
0.012060972861945629,
-0.013469483703374863,
0.02290758676826954,
0.08087252080440521,
0.029438717290759087,
-0.029243694618344307,
0.03760698810219765,
0.03989231586456299,
-0.018219847232103348,
0.025997065007686615,
-0.021278314292430878,
0.033219289034605026,
0.015308304689824581,
-0.02897319756448269,
0.01623428985476494,
0.052683185786008835,
-0.056657515466213226,
0.02162293531000614,
0.040737319737672806,
0.04199214279651642,
0.07587485015392303,
0.005731832701712847,
-0.02345145307481289,
0.02339223399758339,
-0.05833910033106804,
-0.020026978105306625,
-0.02473909594118595,
-0.011846665292978287,
0.08244593441486359,
0.05271004140377045,
-0.033162765204906464,
-0.10882139950990677,
-0.07483604550361633,
0.08574006706476212,
-0.031725119799375534,
-0.00575431389734149,
-0.02692369930446148,
-0.01633639633655548,
0.06302011013031006,
-0.025829268619418144,
-0.019701141864061356,
0.0429992638528347,
-1.66344370888755e-33,
0.005602515302598476,
-0.023976828902959824,
-0.09897257387638092,
0.06968414783477783,
-0.06334761530160904,
-0.05747535452246666,
0.14976243674755096,
0.1323564499616623,
-0.010919717140495777,
-0.020039966329932213,
0.03708109259605408,
-0.0499805323779583,
0.027873549610376358,
-0.052251096814870834,
0.03988093137741089,
-0.03569571673870087,
-0.049829088151454926,
0.005682501941919327,
0.05400059372186661,
0.042901165783405304,
0.01783294603228569,
0.015115833841264248,
-0.07006806135177612,
0.03930580988526344,
0.020719630643725395,
-0.015675459057092667,
-0.02359425649046898,
0.01386992260813713,
-0.04509929195046425,
0.07173233479261398,
-0.001901407027617097,
0.02934844046831131,
-0.04392578825354576,
0.06330644339323044,
-0.08160784840583801,
-0.024412428960204124,
0.01657729782164097,
-0.04614952951669693,
-0.0704987496137619,
0.08639209717512131,
0.08786418288946152,
0.051993221044540405,
-0.029094865545630455,
0.0009907088242471218,
0.03707612305879593,
0.05653470754623413,
-0.048827674239873886,
-0.01698020100593567,
-0.014090544544160366,
-0.1455419957637787,
0.0029137046076357365,
0.034020937979221344,
-0.025696491822600365,
0.018208252266049385,
0.00967650767415762,
-0.0450715646147728,
0.01776566542685032,
-0.08796316385269165,
-0.03864974156022072,
-0.04927501082420349,
-0.0801645889878273,
-0.011740355752408504,
0.06588030606508255,
-0.10984203219413757,
-0.010421487502753735,
-0.08671025931835175,
0.018095947802066803,
-0.045950997620821,
0.04239869862794876,
0.004003122914582491,
0.016221195459365845,
-0.024798432365059853,
-0.024164684116840363,
-0.04076709598302841,
0.028465479612350464,
-0.019443636760115623,
-0.017059482634067535,
0.005688696634024382,
-0.019299017265439034,
-0.07665639370679855,
-0.031610988080501556,
0.01559544075280428,
0.0043563819490373135,
0.05087980628013611,
0.0022138701751828194,
0.08919969946146011,
0.024774398654699326,
0.0862017422914505,
-0.06210745871067047,
0.06441051512956619,
-0.03048485703766346,
-0.024375736713409424,
0.002389675471931696,
0.06739016622304916,
0.011457964777946472,
-5.278835146782512e-8,
-0.08121879398822784,
0.010726772248744965,
-0.011933532543480396,
0.07740911841392517,
-0.018112478777766228,
0.03480321541428566,
-0.0332968644797802,
0.010696266777813435,
0.007591868285089731,
0.05122207850217819,
0.0424799881875515,
0.06955200433731079,
-0.08150836080312729,
0.005794052965939045,
-0.06652770191431046,
0.022908128798007965,
0.017361516132950783,
0.056431353092193604,
-0.006105236243456602,
-0.035400278866291046,
0.07221729308366776,
0.043989744037389755,
0.001445137313567102,
-0.012060279957950115,
0.036268409341573715,
-0.002658131532371044,
-0.0960858091711998,
0.04636721312999725,
0.005755406338721514,
-0.017523422837257385,
0.025232572108507156,
-0.010791206732392311,
-0.0666608065366745,
-0.08207231760025024,
0.01689365692436695,
0.06423576921224594,
-0.07417193800210953,
0.0051384977996349335,
0.045961957424879074,
0.059745993465185165,
0.09041480720043182,
-0.001645807409659028,
-0.10787911713123322,
-0.007512963376939297,
0.14214776456356049,
0.03260063752532005,
-0.041373275220394135,
-0.07589240372180939,
-0.06021237373352051,
0.020149365067481995,
0.12885119020938873,
-0.027237998321652412,
0.0023657912388443947,
0.08624017238616943,
0.029751192778348923,
-0.0018492669332772493,
-0.05468001961708069,
-0.0951952338218689,
0.03008594736456871,
0.04472961276769638,
0.08397585898637772,
0.017985675483942032,
-0.04529568552970886,
0.012954038567841053
] |
flax-sentence-embeddings/multi-QA_v1-mpnet-asymmetric-A | dce2aa07b3e1e5c91c2f411c5534b399462f7b16 | 2021-07-25T21:33:06.000Z | [
"pytorch",
"mpnet",
"fill-mask",
"arxiv:2102.07033",
"arxiv:2104.08727",
"sentence-transformers",
"feature-extraction",
"sentence-similarity"
] | sentence-similarity | false | flax-sentence-embeddings | null | flax-sentence-embeddings/multi-QA_v1-mpnet-asymmetric-A | 2,064 | 1 | sentence-transformers | ---
pipeline_tag: sentence-similarity
tags:
- sentence-transformers
- feature-extraction
- sentence-similarity
---
# multi-QA_v1-mpnet-asymmetric-A
## Model Description
SentenceTransformers is a set of models and frameworks that enable training and generating sentence embeddings from given data. The generated sentence embeddings can be utilized for Clustering, Semantic Search and other tasks. We used two separate pretrained [mpnet-base](https://huggingface.co/microsoft/mpnet-base) models and trained them using contrastive learning objective. Question and answer pairs from StackExchange and other datasets were used as training data to make the model robust to Question / Answer embedding similarity.
We developed this model during the
[Community week using JAX/Flax for NLP & CV](https://discuss.huggingface.co/t/open-to-the-community-community-week-using-jax-flax-for-nlp-cv/7104),
organized by Hugging Face. We developed this model as part of the project:
[Train the Best Sentence Embedding Model Ever with 1B Training Pairs](https://discuss.huggingface.co/t/train-the-best-sentence-embedding-model-ever-with-1b-training-pairs/7354). We benefited from efficient hardware infrastructure to run the project: 7 TPUs v3-8, as well
as assistance from Google’s Flax, JAX, and Cloud team members about efficient deep learning frameworks.
## Intended uses
This model set is intended to be used as a sentence encoder for a search engine. Given an input sentence, it ouptuts a vector which captures
the sentence semantic information. The sentence vector may be used for semantic-search, clustering or sentence similarity tasks.
Two models should be used on conjunction for Semantic Search purposes.
1. [multi-QA_v1-mpnet-asymmetric-Q](https://huggingface.co/flax-sentence-embeddings/multi-QA_v1-mpnet-asymmetric-Q) - Model to encode Questions
1. [multi-QA_v1-mpnet-asymmetric-A](https://huggingface.co/flax-sentence-embeddings/multi-QA_v1-mpnet-asymmetric-A) - Model to encode Answers
## How to use
Here is how to use this model to get the features of a given text using [SentenceTransformers](https://github.com/UKPLab/sentence-transformers) library:
```python
from sentence_transformers import SentenceTransformer
model_Q = SentenceTransformer('flax-sentence-embeddings/multi-QA_v1-mpnet-asymmetric-Q')
model_A = SentenceTransformer('flax-sentence-embeddings/multi-QA_v1-mpnet-asymmetric-A')
question = "Replace me by any question you'd like."
question_embbedding = model_Q.encode(text)
answer = "Replace me by any answer you'd like."
answer_embbedding = model_A.encode(text)
answer_likeliness = cosine_similarity(question_embedding, answer_embedding)
```
# Training procedure
## Pre-training
We use the pretrained [`Mpnet-base`](https://huggingface.co/microsoft/mpnet-base). Please refer to the model
card for more detailed information about the pre-training procedure.
## Fine-tuning
We fine-tune the model using a contrastive objective. Formally, we compute the cosine similarity from each possible sentence pairs from the batch.
We then apply the cross entropy loss by comparing with true pairs.
### Hyper parameters
We trained on model on a TPU v3-8. We train the model during 80k steps using a batch size of 1024 (128 per TPU core).
We use a learning rate warm up of 500. The sequence length was limited to 128 tokens. We used the AdamW optimizer with
a 2e-5 learning rate. The full training script is accessible in this current repository.
### Training data
We used the concatenation from multiple Stackexchange Question-Answer datasets to fine-tune our model. MSMARCO, NQ & other question-answer datasets were also used.
| Dataset | Paper | Number of training tuples |
|:--------------------------------------------------------:|:----------------------------------------:|:--------------------------:|
| [Stack Exchange QA - Title & Answer](https://huggingface.co/datasets/flax-sentence-embeddings/stackexchange_title_best_voted_answer_jsonl) | - | 4,750,619 |
| [Stack Exchange](https://huggingface.co/datasets/flax-sentence-embeddings/stackexchange_title_body_jsonl) | - | 364,001 |
| [TriviaqQA](https://huggingface.co/datasets/trivia_qa) | - | 73,346 |
| [SQuAD2.0](https://rajpurkar.github.io/SQuAD-explorer/) | [paper](https://aclanthology.org/P18-2124.pdf) | 87,599 |
| [Quora Question Pairs](https://quoradata.quora.com/First-Quora-Dataset-Release-Question-Pairs) | - | 103,663 |
| [Eli5](https://huggingface.co/datasets/eli5) | [paper](https://doi.org/10.18653/v1/p19-1346) | 325,475 |
| [PAQ](https://github.com/facebookresearch/PAQ) | [paper](https://arxiv.org/abs/2102.07033) | 64,371,441 |
| [WikiAnswers](https://github.com/afader/oqa#wikianswers-corpus) | [paper](https://doi.org/10.1145/2623330.2623677) | 77,427,422 |
| [MS MARCO](https://microsoft.github.io/msmarco/) | [paper](https://doi.org/10.1145/3404835.3462804) | 9,144,553 |
| [GOOAQ: Open Question Answering with Diverse Answer Types](https://github.com/allenai/gooaq) | [paper](https://arxiv.org/pdf/2104.08727.pdf) | 3,012,496 |
| [Yahoo Answers](https://www.kaggle.com/soumikrakshit/yahoo-answers-dataset) Question/Answer | [paper](https://proceedings.neurips.cc/paper/2015/hash/250cf8b51c773f3f8dc8b4be867a9a02-Abstract.html) | 681,164 |
| SearchQA | - | 582,261 |
| [Natural Questions (NQ)](https://ai.google.com/research/NaturalQuestions) | [paper](https://transacl.org/ojs/index.php/tacl/article/view/1455) | 100,231 | | [
-0.05941617861390114,
-0.09878981858491898,
-0.03278364613652229,
0.061484500765800476,
0.07150906324386597,
0.054682034999132156,
-0.06952426582574844,
0.022128066048026085,
-0.02004602551460266,
-0.10085403174161911,
0.014421924017369747,
-0.03631352260708809,
0.043370962142944336,
0.11276673525571823,
-0.007059652358293533,
0.055554669350385666,
0.10986382514238358,
0.03913488611578941,
-0.09896381944417953,
-0.013386857695877552,
0.01700841821730137,
0.0627388283610344,
0.06918708980083466,
0.02337798662483692,
-0.008346982300281525,
0.06703004986047745,
0.004940365441143513,
-0.02082282491028309,
0.02441643550992012,
0.0030713556334376335,
0.06281193345785141,
-0.018408311530947685,
0.00967686902731657,
0.09132105112075806,
0.014163634739816189,
0.10159224271774292,
-0.03454877808690071,
0.03312935680150986,
-0.03085370548069477,
-0.0833761915564537,
-0.046731799840927124,
-0.003429027507081628,
-0.01919868215918541,
0.025166988372802734,
0.08361242711544037,
-0.04203730449080467,
-0.07861022651195526,
-0.02788163162767887,
0.023320084437727928,
0.04748386889696121,
-0.07626532763242722,
-0.0071290940977633,
-0.027486471459269524,
0.07267771661281586,
-0.03372419998049736,
0.057747963815927505,
-0.0554821640253067,
-0.011945907026529312,
-0.008830873295664787,
-0.100086510181427,
-0.047038909047842026,
-0.06934987753629684,
-0.013913419097661972,
0.02422276698052883,
-0.03756437450647354,
-0.05304666608572006,
-0.024037400260567665,
0.03506462648510933,
0.031350407749414444,
-0.008213511668145657,
-0.05397611856460571,
0.07973424345254898,
-0.012715683318674564,
0.02193077839910984,
-0.06412830948829651,
0.11100677400827408,
0.08126495033502579,
-0.004044676199555397,
0.03410828858613968,
-0.03331182152032852,
0.012848573736846447,
-0.038949742913246155,
0.046417221426963806,
0.009587094187736511,
0.07036096602678299,
0.019961843267083168,
0.01581910252571106,
-0.003719527740031481,
-0.057001303881406784,
-0.02223280631005764,
-0.1167929396033287,
-0.003964947536587715,
0.09798070043325424,
-0.06786566972732544,
0.03985898196697235,
-0.026076195761561394,
0.020495085045695305,
-0.06309475749731064,
-0.011965871788561344,
0.13023705780506134,
0.039746105670928955,
0.06675159931182861,
0.04373691603541374,
-0.07222632318735123,
-0.02089543454349041,
0.0033256616443395615,
-0.041528452187776566,
0.007317536976188421,
0.020610395818948746,
-0.1267387717962265,
0.02198982611298561,
0.0736188143491745,
-0.027807947248220444,
-0.041895389556884766,
0.07521793246269226,
-0.014635908417403698,
0.03405666723847389,
-0.01908211037516594,
-0.010763914324343204,
0.08237365633249283,
-0.05255449563264847,
0.039141833782196045,
-0.003537136595696211,
-0.009502310305833817,
0.04843674227595329,
-0.05661594495177269,
-0.025681938976049423,
1.2333703661979998e-33,
0.06248995661735535,
0.025383729487657547,
0.05104048550128937,
0.0028701445553451777,
0.057761989533901215,
-0.021933456882834435,
0.003458993975073099,
0.05745426192879677,
-0.06123267859220505,
-0.03971873223781586,
-0.0037615890614688396,
0.04960531368851662,
0.0012835148954764009,
0.062270671129226685,
0.003372078761458397,
-0.05588975176215172,
-0.09873859584331512,
-0.034140873700380325,
-0.025163574144244194,
-0.007994990795850754,
-0.00735607510432601,
-0.03416607901453972,
0.015982074663043022,
0.01861017756164074,
-0.0738934576511383,
-0.04663177579641342,
0.09982097893953323,
-0.07490827143192291,
-0.001635421416722238,
0.013628392480313778,
-0.1003633588552475,
-0.014447255060076714,
-0.01544704008847475,
0.006016951519995928,
0.007159320171922445,
0.005302156321704388,
0.019936716184020042,
-0.10918296873569489,
0.00043466719216667116,
-0.06297741830348969,
-0.04831111431121826,
0.032544899731874466,
0.01589333452284336,
-0.07796508073806763,
-0.021574601531028748,
-0.01628325693309307,
0.0046711149625480175,
-0.06279010325670242,
0.025487536564469337,
-0.0357632152736187,
0.10499044507741928,
-0.04100404679775238,
-0.012798981741070747,
-0.058187589049339294,
-0.012362044304609299,
0.001862820121459663,
0.022539447993040085,
0.05704647675156593,
0.05213485285639763,
0.03783769905567169,
0.02683265507221222,
-0.07307406514883041,
0.030828827992081642,
0.016829615458846092,
0.013937203213572502,
0.027517782524228096,
0.009947801940143108,
0.08881373703479767,
0.040352173149585724,
0.029877178370952606,
0.022217772901058197,
0.023333938792347908,
-0.013256530277431011,
-0.02137642540037632,
0.06679821759462357,
0.04719678685069084,
0.008121456019580364,
-0.07795070111751556,
-0.004603642039000988,
0.06638167798519135,
-0.037259891629219055,
-0.008657784201204777,
0.004280540160834789,
-0.09089068323373795,
-0.06669709831476212,
-0.055615976452827454,
0.03839438781142235,
-0.11194249242544174,
0.07788946479558945,
0.01696542277932167,
0.02946162223815918,
-0.007265214342623949,
0.013922298327088356,
0.01030901912599802,
0.11045483499765396,
-2.9639389810325775e-34,
-0.032706018537282944,
0.010810424573719501,
-0.11453085392713547,
0.03315065801143646,
0.0042084213346242905,
-0.02643662318587303,
0.015503628179430962,
0.08822491765022278,
-0.034721847623586655,
-0.03448662534356117,
-0.027354521676898003,
-0.02156243845820427,
0.02215520106256008,
-0.052649401128292084,
0.004244902636855841,
0.04606647044420242,
0.03738836944103241,
0.05317626893520355,
0.046227362006902695,
0.12415055930614471,
-0.0008581136935390532,
0.06694330275058746,
-0.11285004764795303,
0.09079660475254059,
0.007065270561724901,
0.046665407717227936,
0.004846741911023855,
-0.006434650160372257,
-0.026084361597895622,
-0.04827963188290596,
-0.02797926776111126,
0.0052910237573087215,
-0.045263756066560745,
-0.10353951156139374,
-0.10548023134469986,
0.05271722376346588,
-0.0031122337095439434,
-0.05975071340799332,
-0.00225509749725461,
0.04484080895781517,
0.029383547604084015,
-0.010166403837502003,
-0.0565197691321373,
-0.005758782383054495,
-0.0647999718785286,
-0.005644127260893583,
-0.10057099163532257,
-0.09916974604129791,
0.0385536253452301,
0.031957414001226425,
-0.027685590088367462,
0.0030471310019493103,
-0.12079031020402908,
0.010390223935246468,
-0.03392742946743965,
-0.0813048705458641,
-0.000013701342140848283,
0.007610459346324205,
-0.019786270335316658,
0.026064440608024597,
-0.0000871035226737149,
-0.009786790236830711,
0.03488204628229141,
-0.00527331605553627,
0.07828264683485031,
-0.08104170113801956,
0.02169645205140114,
0.028801709413528442,
-0.059427790343761444,
-0.009007674641907215,
-0.015720482915639877,
0.008942273445427418,
0.04598315805196762,
0.049174822866916656,
0.03700072318315506,
-0.08219476044178009,
0.02765076607465744,
-0.019911816343665123,
-0.03527120500802994,
-0.08873596042394638,
0.033373601734638214,
-0.03169647976756096,
-0.004301225300878286,
0.04303870350122452,
0.05530873313546181,
0.031334176659584045,
0.08627890050411224,
0.05812457948923111,
0.011544044129550457,
0.06907772272825241,
-0.0044668978080153465,
-0.037681061774492264,
0.0025312325451523066,
0.046083204448223114,
-0.012981748208403587,
-4.590953039951273e-8,
-0.07937952131032944,
-0.013745100237429142,
-0.07436152547597885,
0.04670410975813866,
-0.08517569303512573,
-0.03650471940636635,
0.02425742894411087,
0.02197221852838993,
-0.030720889568328857,
-0.01598394103348255,
0.04662654176354408,
0.05733426660299301,
-0.03745352104306221,
-0.018185459077358246,
-0.04135112464427948,
0.10800379514694214,
-0.003688968252390623,
0.009519820101559162,
0.043414462357759476,
-0.031315065920352936,
0.05984274670481682,
0.06162552908062935,
-0.008961052633821964,
0.07031967490911484,
0.07918160408735275,
0.03012591414153576,
-0.06587952375411987,
0.08523012697696686,
0.05738356336951256,
-0.019848017022013664,
-0.003796821692958474,
0.010845507495105267,
-0.05812589451670647,
-0.07236111164093018,
0.014988039620220661,
0.06416931003332138,
0.033362261950969696,
-0.05658328905701637,
-0.007257090415805578,
0.049893464893102646,
0.05281231552362442,
0.07380609214305878,
-0.06446636468172073,
-0.03284931927919388,
0.11449264734983444,
0.07297425717115402,
-0.0324438251554966,
-0.037198103964328766,
0.027177808806300163,
-0.054960161447525024,
0.007432160433381796,
-0.06959261745214462,
-0.014713022857904434,
0.014409756287932396,
0.07180524617433548,
-0.014832502231001854,
-0.01061595045030117,
-0.0038757254369556904,
0.09864235669374466,
-0.019040148705244064,
0.04031343013048172,
0.052107490599155426,
0.04267431050539017,
-0.010957919992506504
] |
transfaeries/DialoGPT-medium-Discord-1.0 | fdd1f5fa445bd30233a0a2d854d89741fad3fa80 | 2021-09-02T04:19:36.000Z | [
"pytorch",
"gpt2",
"text-generation",
"transformers",
"conversational"
] | conversational | false | transfaeries | null | transfaeries/DialoGPT-medium-Discord-1.0 | 2,062 | null | transformers | ---
tags:
- conversational
---
# Discord Model Medium 7 epochs | [
-0.03739439323544502,
-0.06000816449522972,
0.05572880432009697,
0.018031371757388115,
0.03370935842394829,
-0.04772641137242317,
0.06766233593225479,
-0.016146164387464523,
0.025703784078359604,
-0.06150631606578827,
0.00934563297778368,
-0.08359815180301666,
-0.04436887428164482,
0.012471102178096771,
-0.02483672834932804,
-0.014034261927008629,
-0.018552793189883232,
-0.10439291596412659,
-0.057988241314888,
-0.04345516115427017,
-0.04429006949067116,
0.037509944289922714,
0.011217688210308552,
0.051422104239463806,
0.07372531294822693,
-0.05008037015795708,
-0.03119824454188347,
0.005935290362685919,
0.053845375776290894,
-0.03397221863269806,
0.03386411815881729,
0.048163335770368576,
0.03747135028243065,
0.09564096480607986,
-0.019775420427322388,
0.05158447101712227,
0.024985486641526222,
-0.019924212247133255,
0.00854637660086155,
0.0512530617415905,
0.03750580549240112,
-0.054958075284957886,
0.019504722207784653,
0.04094085842370987,
0.04299870505928993,
-0.043061066418886185,
-0.06353065371513367,
-0.010330624878406525,
-0.06406663358211517,
0.06488561630249023,
-0.04659784585237503,
-0.027118464931845665,
0.014997430145740509,
0.15402096509933472,
0.00880367960780859,
0.022083330899477005,
-0.046720970422029495,
-0.005583718419075012,
0.046204715967178345,
-0.05867142975330353,
-0.05768295377492905,
-0.09086275100708008,
-0.03609631955623627,
-0.010523414239287376,
-0.02682444080710411,
0.01171019859611988,
-0.015812629833817482,
0.08385008573532104,
-0.008783151395618916,
0.0811857208609581,
0.015578780323266983,
0.04246902838349342,
-0.05270298197865486,
0.02890905737876892,
-0.0009242405649274588,
0.05147133022546768,
-0.02958354726433754,
0.0007068578852340579,
0.019652368500828743,
-0.08092880249023438,
0.05086405575275421,
-0.03772246465086937,
0.004755100235342979,
-0.06863992661237717,
0.041455794125795364,
-0.07020452618598938,
-0.013720927760004997,
0.00851407554000616,
-0.06204924359917641,
-0.03001483902335167,
-0.05783265456557274,
0.038311250507831573,
0.013326853513717651,
0.0444355309009552,
-0.03868136182427406,
0.10037114471197128,
0.002653462579473853,
0.012541676871478558,
-0.01806660182774067,
0.1390809863805771,
-0.023717796429991722,
0.04440468177199364,
-0.03689827769994736,
-0.000046456676500383765,
0.004792109597474337,
0.0290912464261055,
0.016998425126075745,
0.07541581988334656,
0.02427133172750473,
-0.026519829407334328,
0.004424653481692076,
0.01877000369131565,
0.01490661222487688,
-0.04615958780050278,
0.1514836996793747,
-0.027193108573555946,
0.05292602628469467,
0.012628347612917423,
0.09177123755216599,
0.014105274342000484,
0.006171875633299351,
0.03071603737771511,
-0.005753684788942337,
-0.05149751529097557,
-0.06251786649227142,
0.0466538704931736,
-0.013985611498355865,
-9.665193732369168e-34,
0.05894612893462181,
0.0008830504375509918,
-0.05168524757027626,
0.09168614447116852,
0.032660484313964844,
0.005159351509064436,
-0.05551590025424957,
-0.027410276234149933,
-0.07089637219905853,
-0.06756306439638138,
-0.07111840695142746,
-0.016363415867090225,
0.02656850963830948,
-0.023902935907244682,
0.029779905453324318,
-0.06510588526725769,
0.047689031809568405,
0.017770862206816673,
0.03060602769255638,
-0.06620168685913086,
0.013481433503329754,
-0.018224649131298065,
-0.052669741213321686,
0.05623302236199379,
0.07724356651306152,
0.08103588223457336,
0.011828185059130192,
-0.0628378614783287,
0.015499195083975792,
0.03592918440699577,
-0.0600765086710453,
0.06338661164045334,
0.00980442576110363,
0.0732259526848793,
0.043928198516368866,
0.03031911328434944,
0.015928367152810097,
-0.004308763891458511,
0.01658168062567711,
-0.10722963511943817,
-0.019746776670217514,
-0.035790737718343735,
-0.04465951398015022,
-0.12061101943254471,
-0.05959303304553032,
-0.011431808583438396,
0.034789085388183594,
-0.05197529122233391,
0.021023793146014214,
-0.029106225818395615,
-0.019040105864405632,
0.028370682150125504,
0.04490427300333977,
-0.01065783016383648,
0.020972106605768204,
-0.057181596755981445,
0.037480678409338,
0.03572104498744011,
0.016186388209462166,
-0.018477588891983032,
0.08765985816717148,
-0.01720697060227394,
0.05576193332672119,
-0.07664711773395538,
0.0479077473282814,
0.05220409110188484,
-0.10255443304777145,
0.03200703114271164,
0.03357082977890968,
-0.025202905759215355,
-0.05192917212843895,
0.06755401939153671,
-0.05689023807644844,
-0.019051307812333107,
0.011827214621007442,
-0.04860720783472061,
-0.014180516824126244,
-0.05455328896641731,
0.03661182150244713,
0.07630020380020142,
-0.08155391365289688,
-0.08100325614213943,
-0.12341131269931793,
-0.015138440765440464,
-0.04508691281080246,
-0.05530396103858948,
0.04617638513445854,
-0.058183833956718445,
-0.01403919979929924,
0.01542083453387022,
-0.053013838827610016,
0.04626164212822914,
0.03794700652360916,
0.02384728379547596,
-0.07182405143976212,
3.882149875677892e-35,
0.015371011570096016,
0.04793276637792587,
-0.07362497597932816,
0.10499518364667892,
0.037700995802879333,
-0.034901104867458344,
0.05058019980788231,
0.15067797899246216,
-0.03360549360513687,
0.009250175207853317,
0.0747581422328949,
-0.025810780003666878,
-0.08710047602653503,
-0.09084372222423553,
0.10729732364416122,
-0.02081114798784256,
0.04771741107106209,
-0.06313763558864594,
0.04903625324368477,
-0.020801769569516182,
0.0374162420630455,
-0.023957539349794388,
-0.1257554441690445,
0.04711844399571419,
0.06507978588342667,
-0.020453596487641335,
0.04062514752149582,
0.04117967560887337,
0.032835133373737335,
-0.036537054926157,
-0.006775812245905399,
-0.026797156780958176,
-0.018325135111808777,
-0.015123935416340828,
-0.018625307828187943,
0.04093380644917488,
0.07572849839925766,
0.012907423079013824,
-0.014622841961681843,
-0.07397227734327316,
0.06372332572937012,
0.0254572294652462,
-0.08865558356046677,
0.04501447454094887,
0.012789878994226456,
0.03831856697797775,
-0.030787399038672447,
0.011941480450332165,
-0.06982647627592087,
-0.008128711022436619,
0.11861997842788696,
-0.06570016592741013,
0.009179627522826195,
-0.026746446266770363,
-0.11644180864095688,
-0.00011063341662520543,
-0.020981188863515854,
-0.0043420675210654736,
-0.010553419589996338,
-0.0018389140022918582,
-0.03670622408390045,
-0.0921853557229042,
-0.002316768281161785,
-0.03455596789717674,
0.06244221702218056,
-0.05743943899869919,
-0.0723186582326889,
0.05715401843190193,
-0.06230105832219124,
-0.00868936162441969,
0.12091423571109772,
0.030074167996644974,
-0.09432988613843918,
0.050936512649059296,
0.025453153997659683,
-0.09425017237663269,
-0.020108535885810852,
-0.05943010374903679,
0.011746944859623909,
-0.02601262740790844,
-0.07800579071044922,
0.02396930195391178,
-0.0012447470799088478,
0.08664705604314804,
0.017146989703178406,
0.01893327571451664,
0.06369494646787643,
0.0668669119477272,
-0.01685810461640358,
-0.02641858160495758,
0.009552303701639175,
-0.06610148400068283,
-0.03826376423239708,
0.07806077599525452,
-0.0422089658677578,
-2.46788633972983e-8,
-0.07498063147068024,
-0.03229716420173645,
-0.023823929950594902,
0.01661892607808113,
0.07408395409584045,
0.03858484327793121,
0.014969859272241592,
0.004061793442815542,
0.044277336448431015,
0.02909695729613304,
0.06734231114387512,
0.01841902546584606,
0.0032281633466482162,
0.046491868793964386,
-0.007352646440267563,
-0.008512584492564201,
-0.061835579574108124,
0.06115664169192314,
-0.01346312090754509,
-0.07735282927751541,
0.0751735121011734,
0.013495242223143578,
-0.039146844297647476,
0.01749177649617195,
0.038970962166786194,
-0.012783471494913101,
-0.002758769551292062,
0.12730097770690918,
-0.06606914103031158,
-0.0667201355099678,
-0.012301215901970863,
0.004347479436546564,
-0.05302441865205765,
0.006214942317456007,
-0.06526603549718857,
0.08803331106901169,
-0.0777195617556572,
-0.02188391424715519,
0.026403607800602913,
0.013139274902641773,
0.03148980438709259,
-0.010603859089314938,
-0.036568593233823776,
-0.027434788644313812,
-0.01344282552599907,
-0.0014665273483842611,
-0.045137178152799606,
-0.010883171111345291,
0.021661434322595596,
0.015935303643345833,
-0.005783747881650925,
0.019464507699012756,
0.042136985808610916,
0.047212760895490646,
0.046427302062511444,
-0.05156468600034714,
0.07733213156461716,
0.00006927437789272517,
0.07287062704563141,
-0.009445804171264172,
0.11084549129009247,
0.022295959293842316,
-0.06432489305734634,
-0.03279854729771614
] |
hf-internal-testing/tiny-random-t5-v1.1 | 95197e7dc6c034b9ae97b124952afb5e15ed0fb2 | 2021-11-02T21:08:45.000Z | [
"pytorch",
"tf",
"t5",
"transformers"
] | null | false | hf-internal-testing | null | hf-internal-testing/tiny-random-t5-v1.1 | 2,060 | null | transformers | Entry not found | [
0.0461147278547287,
-0.038838207721710205,
-0.01049656979739666,
-0.03682169318199158,
0.011261860840022564,
0.013094935566186905,
0.0019101888174191117,
-0.013979103416204453,
0.027092741802334785,
-0.015212527476251125,
0.017284274101257324,
-0.08189476281404495,
0.03817418962717056,
-0.04920130595564842,
0.021389011293649673,
-0.015245908871293068,
-0.03203780576586723,
-0.1245758980512619,
0.03150877356529236,
0.032381657510995865,
-0.060957908630371094,
0.05409295856952667,
-0.025087490677833557,
0.01568586938083172,
0.028129950165748596,
-0.04710396006703377,
-0.018688226118683815,
0.013785239309072495,
-0.04001208767294884,
0.01173911802470684,
-0.04317743331193924,
0.05500618368387222,
0.004543041344732046,
0.02973111905157566,
0.14852192997932434,
0.02658126689493656,
0.02907961793243885,
-0.05169107764959335,
0.05803573504090309,
-0.07732241600751877,
-0.017637968063354492,
-0.04219653457403183,
0.041807834059000015,
0.023620979860424995,
0.021563321352005005,
0.016478516161441803,
-0.0021814992651343346,
-0.06400240957736969,
0.06393089145421982,
0.019599027931690216,
-0.08565037697553635,
0.00934905931353569,
-0.008718925528228283,
-0.028583496809005737,
-0.07310017943382263,
0.09416428208351135,
0.001759322709403932,
0.06184990331530571,
0.011840506456792355,
-0.035997264087200165,
0.08358278125524521,
-0.02619801089167595,
0.03736566752195358,
-0.028206506744027138,
-0.07454850524663925,
-0.08883563429117203,
-0.06279942393302917,
-0.008695344440639019,
0.014119276776909828,
-0.0825355276465416,
0.0649217739701271,
-0.00223911227658391,
-0.14716917276382446,
0.07743025571107864,
-0.03548373281955719,
-0.055201586335897446,
0.006981803569942713,
-0.012166670523583889,
0.055111464112997055,
-0.007116836030036211,
-0.023175746202468872,
-0.005835152696818113,
-0.09185640513896942,
0.055196937173604965,
0.034148022532463074,
0.03835180774331093,
0.038685429841279984,
-0.025987252593040466,
0.017804903909564018,
0.022428328171372414,
0.025005368515849113,
-0.10761535167694092,
-0.048001550137996674,
-0.04343584179878235,
0.012374646961688995,
-0.019502125680446625,
0.029218152165412903,
0.0842173621058464,
-0.011719699949026108,
0.09283553808927536,
-0.007015465293079615,
-0.03543110564351082,
-0.06936459988355637,
0.09425332397222519,
-0.010958523489534855,
-0.00805904995650053,
0.004974212497472763,
-0.0031528924591839314,
0.06105927750468254,
-0.03964288905262947,
-0.03619541600346565,
-0.019901901483535767,
0.07134733349084854,
0.039514873176813126,
-0.012729483656585217,
-0.006646515801548958,
-0.04746140539646149,
-0.014432490803301334,
-0.05157482624053955,
0.09506245702505112,
-0.049747664481401443,
-0.04591796174645424,
-0.008965466171503067,
-0.0325421579182148,
-0.08626784384250641,
-0.06624380499124527,
0.02538885548710823,
-4.303924894057984e-33,
0.01133066974580288,
0.0033434738870710135,
-0.002155609894543886,
0.04871906340122223,
-0.023564351722598076,
-0.07933273911476135,
0.0600903145968914,
0.02335330657660961,
-0.03844716399908066,
-0.020433755591511726,
-0.06952055543661118,
-0.03235611692070961,
0.0062485747039318085,
0.064804308116436,
-0.03201229125261307,
0.061689723283052444,
0.0417000837624073,
-0.00761845987290144,
0.03340127319097519,
-0.047770582139492035,
0.00887306872755289,
-0.04066338762640953,
-0.010506896302103996,
0.0106519665569067,
0.021333497017621994,
0.12854498624801636,
-0.009705503471195698,
0.010055632330477238,
-0.017507633194327354,
0.006515394430607557,
0.06334009766578674,
-0.057817306369543076,
0.013668818399310112,
-0.020286159589886665,
0.05430467426776886,
-0.023184705525636673,
0.0828516036272049,
0.0005449643940664828,
-0.10372652113437653,
-0.07634282112121582,
-0.005381610710173845,
-0.039263784885406494,
0.0006114727002568543,
-0.013281986117362976,
0.07119110971689224,
0.043696220964193344,
0.03168422728776932,
0.04338686540722847,
0.05728672817349434,
0.0832006186246872,
-0.07961414009332657,
0.015234283171594143,
0.017002005130052567,
0.047004107385873795,
-0.09794387966394424,
0.004990279674530029,
-0.07062993198633194,
-0.028000490739941597,
-0.04018733277916908,
-0.0702052190899849,
0.011351344175636768,
0.06020182743668556,
-0.03297270089387894,
0.09396500885486603,
0.03417910635471344,
-0.019825750961899757,
-0.034690454602241516,
-0.013036907650530338,
0.05896938592195511,
-0.012359356507658958,
-0.017275206744670868,
-0.07982361316680908,
0.02059139870107174,
0.06737419217824936,
0.04176458343863487,
-0.04978838190436363,
-0.05877475067973137,
-0.06289287656545639,
-0.03354167565703392,
-0.03871942684054375,
0.009898529388010502,
-0.05514208599925041,
-0.11629002541303635,
-0.011855563148856163,
0.10663620382547379,
0.037354156374931335,
-0.0065480442717671394,
-0.051189567893743515,
0.06663123518228531,
0.01874656230211258,
0.032841797918081284,
0.041593004018068314,
-0.06879369914531708,
0.04216769337654114,
-0.01628219522535801,
5.4139394340936695e-34,
0.05697013810276985,
-0.006972255185246468,
0.015711724758148193,
-0.17956365644931793,
0.02320219948887825,
0.007923615165054798,
-0.008062449283897877,
0.0074974060989916325,
0.07391711324453354,
0.0309313777834177,
0.060510627925395966,
0.058605875819921494,
0.09515274316072464,
-0.002282935893163085,
0.001603541080839932,
0.07024981826543808,
0.012629246339201927,
0.07425693422555923,
-0.038426291197538376,
0.01861148327589035,
0.030608950182795525,
-0.02449394389986992,
0.021528491750359535,
-0.003039651783183217,
-0.03676343336701393,
0.03130284696817398,
0.07998586446046829,
0.010451192036271095,
-0.07930229604244232,
-0.013543923385441303,
0.018781835213303566,
0.05168003588914871,
-0.07191970944404602,
0.15783067047595978,
0.026191607117652893,
0.01262354850769043,
0.08218053728342056,
-0.029807550832629204,
-0.07528624683618546,
-0.04250097647309303,
0.017244765534996986,
0.04411793500185013,
0.03708017244935036,
0.009233047254383564,
-0.040271829813718796,
0.022496428340673447,
0.02495843544602394,
0.07633638381958008,
0.005147108342498541,
0.013892097398638725,
0.05610476806759834,
-0.06684739887714386,
0.05862557515501976,
-0.020688841119408607,
0.05377643182873726,
0.06718500703573227,
0.005329249892383814,
-0.01388032827526331,
0.029931528493762016,
0.009508464485406876,
-0.045173756778240204,
0.11534366756677628,
-0.06510116159915924,
0.05117698386311531,
-0.0026125339791178703,
-0.08554837852716446,
-0.03784770518541336,
0.0804959163069725,
0.011298024095594883,
-0.07695550471544266,
-0.04868878796696663,
0.02515520341694355,
0.06252261996269226,
-0.04509226232767105,
-0.01246943511068821,
0.028559505939483643,
-0.030573077499866486,
0.05066261067986488,
-0.08187384903430939,
0.04469604790210724,
0.0034051244147121906,
0.04145054519176483,
-0.021858664229512215,
-0.06112268194556236,
-0.00908052921295166,
-0.05903250351548195,
0.0259539932012558,
0.059690944850444794,
-0.07613514363765717,
-0.03720718249678612,
-0.036316655576229095,
0.07058046013116837,
-0.008224100805819035,
0.041961874812841415,
-0.0285952128469944,
-1.496900736697171e-8,
-0.0014124972512945533,
0.03401879221200943,
-0.040338415652513504,
0.04116074740886688,
0.0935964286327362,
-0.05115952715277672,
0.0008746005478315055,
-0.03389839455485344,
-0.00567849725484848,
-0.010686947964131832,
-0.04789939522743225,
-0.04820054769515991,
-0.02011880651116371,
-0.03209094703197479,
-0.04211259260773659,
-0.10229527950286865,
-0.07819421589374542,
-0.031228765845298767,
-0.02154778689146042,
-0.04960230365395546,
0.08087796717882156,
-0.07801242172718048,
0.06919731199741364,
-0.04999840259552002,
0.03687043860554695,
0.03889009356498718,
-0.049989692866802216,
-0.04254625365138054,
-0.04606937617063522,
0.08682432025671005,
-0.031148413196206093,
0.11826753616333008,
0.034102488309144974,
-0.0208592489361763,
-0.0205202866345644,
0.027134142816066742,
0.09741277992725372,
0.051608603447675705,
0.013477512635290623,
-0.13649295270442963,
-0.022304272279143333,
0.02385953813791275,
0.038732077926397324,
-0.09249968826770782,
-0.04549082741141319,
0.054220106452703476,
0.01160438358783722,
0.051190607249736786,
0.07713303714990616,
-0.022097084671258926,
-0.06127818301320076,
-0.01857956498861313,
0.006740490905940533,
-0.00496308971196413,
0.024095389991998672,
0.0736224576830864,
-0.003481915919110179,
-0.0699305310845375,
-0.006629763171076775,
-0.0598808117210865,
0.05297163128852844,
-0.02902800403535366,
-0.027858933433890343,
-0.01287526823580265
] |
M-CLIP/M-BERT-Distil-40 | ff20c09c1a088589cb65a169d165b5ddcbe792ca | 2021-03-21T15:39:15.000Z | [
"pytorch",
"distilbert",
"feature-extraction",
"transformers"
] | feature-extraction | false | M-CLIP | null | M-CLIP/M-BERT-Distil-40 | 2,056 | 1 | transformers | <br />
<p align="center">
<h1 align="center">M-BERT Distil 40</h1>
<p align="center">
<a href="https://github.com/FreddeFrallan/Multilingual-CLIP/tree/main/Model%20Cards/M-BERT%20Distil%2040">Github Model Card</a>
</p>
</p>
## Usage
To use this model along with the original CLIP vision encoder you need to download the code and additional linear weights from the [Multilingual-CLIP Github](https://github.com/FreddeFrallan/Multilingual-CLIP).
Once this is done, you can load and use the model with the following code
```python
from src import multilingual_clip
model = multilingual_clip.load_model('M-BERT-Distil-40')
embeddings = model(['Älgen är skogens konung!', 'Wie leben Eisbären in der Antarktis?', 'Вы знали, что все белые медведи левши?'])
print(embeddings.shape)
# Yields: torch.Size([3, 640])
```
<!-- ABOUT THE PROJECT -->
## About
A [distilbert-base-multilingual](https://huggingface.co/distilbert-base-multilingual-cased) tuned to match the embedding space for [40 languages](https://github.com/FreddeFrallan/Multilingual-CLIP/blob/main/Model%20Cards/M-BERT%20Distil%2040/Fine-Tune-Languages.md), to the embedding space of the CLIP text encoder which accompanies the Res50x4 vision encoder. <br>
A full list of the 100 languages used during pre-training can be found [here](https://github.com/google-research/bert/blob/master/multilingual.md#list-of-languages), and a list of the 40 languages used during fine-tuning can be found in [SupportedLanguages.md](Fine-Tune-Languages.md).
Training data pairs was generated by sampling 40k sentences for each language from the combined descriptions of [GCC](https://ai.google.com/research/ConceptualCaptions/) + [MSCOCO](https://cocodataset.org/#home) + [VizWiz](https://vizwiz.org/tasks-and-datasets/image-captioning/), and translating them into the corresponding language.
All translation was done using the [AWS translate service](https://aws.amazon.com/translate/), the quality of these translations have currently not been analyzed, but one can assume the quality varies between the 40 languages.
## Evaluation
[These results can be viewed at Github](https://github.com/FreddeFrallan/Multilingual-CLIP/tree/main/Model%20Cards/M-BERT%20Distil%2040). <br>
A non-rigorous qualitative evaluation shows that for the languages French, German, Spanish, Russian, Swedish and Greek it seemingly yields respectable results for most instances. The exception being that Greeks are apparently unable to recognize happy persons. <br>
When testing on Kannada, a language which was included during pre-training but not fine-tuning, it performed close to random
| [
-0.08732310682535172,
-0.10284658521413803,
0.005678316578269005,
-0.0322919636964798,
-0.007392597384750843,
0.01713128574192524,
-0.038218703120946884,
0.05018702894449234,
0.010684877634048462,
-0.04977305978536606,
-0.0050992644391953945,
-0.06435437500476837,
-0.024379689246416092,
0.06960180401802063,
-0.009267345070838928,
0.08532190322875977,
-0.03711985796689987,
0.11214273422956467,
-0.0718383714556694,
-0.023692164570093155,
0.1375589370727539,
-0.02093980275094509,
0.027554133906960487,
-0.022145116701722145,
0.04245688393712044,
-0.006907847244292498,
0.004530159290879965,
0.020698508247733116,
0.08199302107095718,
0.022732315585017204,
0.08183316886425018,
-0.03303099796175957,
0.10738107562065125,
0.10610892623662949,
0.0039354851469397545,
0.04551124572753906,
-0.07423185557126999,
-0.06037086248397827,
0.05861132964491844,
0.037055931985378265,
-0.04873548448085785,
0.07348275184631348,
-0.0665152445435524,
-0.08855053037405014,
0.009509815834462643,
-0.046886418014764786,
-0.048559803515672684,
0.061592936515808105,
-0.018902810290455818,
-0.017788119614124298,
-0.07086050510406494,
0.000557132821995765,
0.00212857942096889,
-0.0033718349877744913,
-0.03658992052078247,
-0.0192415788769722,
0.050714872777462006,
-0.08335667848587036,
0.029835207387804985,
-0.12780901789665222,
-0.02087578736245632,
0.017373336479067802,
0.028794273734092712,
0.03568632900714874,
-0.08002545684576035,
0.04438220337033272,
-0.05933709442615509,
0.06299644708633423,
-0.05103538557887077,
-0.011897473596036434,
-0.05948735401034355,
-0.029751121997833252,
0.0016750349896028638,
-0.021154101938009262,
0.003128733951598406,
-0.07209462672472,
0.10733816772699356,
-0.049977898597717285,
0.006381163839250803,
-0.040386661887168884,
0.06325875967741013,
-0.011943095363676548,
0.11836790293455124,
0.03711449354887009,
0.08689644932746887,
-0.011414271779358387,
0.015671415254473686,
0.023573601618409157,
0.02485618367791176,
-0.04848241060972214,
-0.04099101573228836,
-0.06988324970006943,
-0.023824015632271767,
-0.0009403172880411148,
0.09264767915010452,
0.007623761426657438,
0.004681472200900316,
-0.03450299799442291,
-0.039683375507593155,
0.0375199131667614,
0.025592688471078873,
-0.004064074717462063,
0.054522570222616196,
-0.08467162400484085,
0.021284600719809532,
0.04555479809641838,
0.07669254392385483,
0.017048969864845276,
0.0396575853228569,
-0.08361269533634186,
0.007177852559834719,
-0.0027453226502984762,
0.02558111771941185,
-0.06431645154953003,
0.04999230429530144,
0.0002622461470309645,
0.002481874544173479,
-0.02051960676908493,
0.034633759409189224,
-0.00008624500333098695,
-0.003939976450055838,
-0.020824171602725983,
-0.0642966628074646,
0.012845714576542377,
-0.02156340517103672,
0.007702882867306471,
0.013949464075267315,
6.659954997099648e-33,
-0.04086354747414589,
0.024558305740356445,
-0.020246805623173714,
-0.04501945897936821,
0.005863700527697802,
0.010452893562614918,
0.013231243938207626,
0.005305953789502382,
-0.06787475943565369,
-0.10112036019563675,
-0.04739649221301079,
-0.03282523155212402,
-0.060995664447546005,
0.06694693118333817,
-0.08392958343029022,
0.004815325140953064,
0.01124547515064478,
0.07624354213476181,
0.060726527124643326,
0.08681757748126984,
0.02845793589949608,
0.023989245295524597,
-0.01568857952952385,
-0.05167660117149353,
-0.005352407693862915,
0.015074948780238628,
0.030547427013516426,
-0.05770443007349968,
-0.00039151415694504976,
0.019818494096398354,
-0.054662760347127914,
0.0010636039078235626,
0.008108899928629398,
-0.04909580573439598,
-0.03182203322649002,
-0.00024350905732717365,
0.038311075419187546,
0.02354463003575802,
-0.02645125240087509,
-0.0067102909088134766,
0.03391867130994797,
0.0862249806523323,
-0.03234515339136124,
-0.06655246019363403,
-0.04752277955412865,
-0.008506356738507748,
0.04287225753068924,
0.08727870881557465,
-0.019731301814317703,
0.05540046468377113,
0.04456934332847595,
-0.012879220768809319,
-0.09712056815624237,
-0.09437171369791031,
-0.0027109035290777683,
-0.03684357926249504,
0.03826863318681717,
-0.0010308540659025311,
0.11341666430234909,
-0.02344544790685177,
-0.03913578763604164,
-0.016702665016055107,
0.08050746470689774,
-0.02389366738498211,
0.1275366246700287,
-0.0572892501950264,
-0.004325002897530794,
-0.003944460302591324,
-0.034328002482652664,
0.08259468525648117,
-0.08669745177030563,
0.0017930662725120783,
-0.016624851152300835,
0.009065890684723854,
-0.01874447800219059,
-0.010619983077049255,
-0.031239069998264313,
-0.06357667595148087,
-0.06631290912628174,
0.0666271299123764,
-0.008377151563763618,
0.025939950719475746,
0.01940341293811798,
-0.10639362782239914,
-0.02942209504544735,
0.010893534868955612,
0.0720151886343956,
-0.07710716128349304,
-0.0008239233866333961,
-0.01074201986193657,
0.032660432159900665,
-0.08812788873910904,
-0.036677759140729904,
0.01504424586892128,
0.014015769585967064,
-5.9825723366764736e-33,
0.022387156262993813,
0.1153518483042717,
0.01323442067950964,
0.058243196457624435,
-0.05444982647895813,
-0.03508753329515457,
0.03170457109808922,
0.14172515273094177,
-0.04085354879498482,
-0.010889342986047268,
-0.016699273139238358,
-0.07594440877437592,
-0.03097471594810486,
-0.020349539816379547,
0.06172257289290428,
0.02410493604838848,
0.02439242973923683,
0.11368127167224884,
-0.028843283653259277,
-0.0014821081422269344,
0.0554184764623642,
-0.046005409210920334,
-0.05013393983244896,
0.06820584088563919,
-0.040734853595495224,
0.10337290167808533,
-0.02031010203063488,
0.0006073979893699288,
0.026692228391766548,
-0.04822813719511032,
-0.0535709448158741,
0.023927468806505203,
-0.05270235985517502,
0.0769965872168541,
-0.09215442091226578,
0.08336760103702545,
-0.00742523605003953,
0.026639284566044807,
-0.008815471082925797,
-0.020769694820046425,
0.051182836294174194,
0.019391315057873726,
-0.05072504281997681,
0.03173600137233734,
-0.027701176702976227,
-0.0615294948220253,
-0.027903079986572266,
0.04784445837140083,
-0.027936043217778206,
-0.05253361165523529,
-0.06468011438846588,
-0.023627515882253647,
-0.06955431401729584,
0.02132462151348591,
-0.06947090476751328,
-0.006014928221702576,
0.00501290475949645,
-0.047256406396627426,
-0.048984888941049576,
-0.04609740898013115,
-0.07988570630550385,
-0.04328298568725586,
0.007307862397283316,
-0.060943976044654846,
0.02986249513924122,
-0.011606285348534584,
-0.004863932263106108,
0.08033978193998337,
-0.06607324630022049,
-0.00781523808836937,
-0.00860203243792057,
0.013017093762755394,
0.0833687037229538,
0.04061531648039818,
0.03937723487615585,
0.03803231939673424,
-0.057937681674957275,
0.007450312841683626,
0.02525092475116253,
0.006413079332560301,
-0.05705932155251503,
-0.07860931754112244,
0.05107380822300911,
0.04456913098692894,
-0.019508369266986847,
0.07613116502761841,
0.00970802828669548,
0.03235717490315437,
0.011700115166604519,
0.01198186818510294,
-0.04417005181312561,
0.055551882833242416,
0.06025759130716324,
0.08669114857912064,
0.08785074204206467,
-5.404166714129133e-8,
-0.08294656127691269,
-0.025940977036952972,
-0.01184093113988638,
0.02304232493042946,
-0.11146830022335052,
-0.052211467176675797,
-0.02506665140390396,
-0.03910243138670921,
-0.014733394607901573,
0.0025746054016053677,
0.04797973856329918,
0.020250331610441208,
-0.08045308291912079,
0.06809934228658676,
-0.014343494549393654,
0.07708622515201569,
0.006497896276414394,
0.05397410690784454,
0.0416078045964241,
0.014005990698933601,
0.04699249193072319,
-0.029062265530228615,
0.061018895357847214,
0.02377062290906906,
0.007207710761576891,
-0.05029823258519173,
-0.08161468803882599,
0.0576479434967041,
0.03144339472055435,
-0.005853019189089537,
0.025104945525527,
0.052952684462070465,
-0.07199832051992416,
-0.015277779661118984,
0.030251607298851013,
-0.038165848702192307,
-0.10855703800916672,
-0.01689106412231922,
-0.011164556257426739,
0.06603329628705978,
0.1405671238899231,
0.002159283496439457,
-0.029079940170049667,
-0.030255088582634926,
0.10121002048254013,
0.0019372694659978151,
0.03483014553785324,
-0.07468930631875992,
0.04032004997134209,
0.0028817374259233475,
0.031359121203422546,
-0.09049282968044281,
-0.034588102251291275,
0.06472288817167282,
-0.061703868210315704,
-0.045739077031612396,
-0.02898797020316124,
0.012040427885949612,
0.08054103702306747,
-0.00560848880559206,
-0.038395944982767105,
0.03636925667524338,
-0.011591508984565735,
0.010431655682623386
] |
voidful/albert_chinese_base | 549e8a023d81bd68e70cf3e2b4aa621e145695ed | 2021-08-03T05:02:21.000Z | [
"pytorch",
"albert",
"fill-mask",
"zh",
"transformers",
"autotrain_compatible"
] | fill-mask | false | voidful | null | voidful/albert_chinese_base | 2,054 | 4 | transformers | ---
language: zh
pipeline_tag: fill-mask
widget:
- text: "今天[MASK]情很好"
---
# albert_chinese_base
This a albert_chinese_base model from [Google's github](https://github.com/google-research/ALBERT)
converted by huggingface's [script](https://github.com/huggingface/transformers/blob/master/src/transformers/convert_albert_original_tf_checkpoint_to_pytorch.py)
## Notice
*Support AutoTokenizer*
Since sentencepiece is not used in albert_chinese_base model
you have to call BertTokenizer instead of AlbertTokenizer !!!
we can eval it using an example on MaskedLM
由於 albert_chinese_base 模型沒有用 sentencepiece
用AlbertTokenizer會載不進詞表,因此需要改用BertTokenizer !!!
我們可以跑MaskedLM預測來驗證這個做法是否正確
## Justify (驗證有效性)
```python
from transformers import AutoTokenizer, AlbertForMaskedLM
import torch
from torch.nn.functional import softmax
pretrained = 'voidful/albert_chinese_base'
tokenizer = AutoTokenizer.from_pretrained(pretrained)
model = AlbertForMaskedLM.from_pretrained(pretrained)
inputtext = "今天[MASK]情很好"
maskpos = tokenizer.encode(inputtext, add_special_tokens=True).index(103)
input_ids = torch.tensor(tokenizer.encode(inputtext, add_special_tokens=True)).unsqueeze(0) # Batch size 1
outputs = model(input_ids, labels=input_ids)
loss, prediction_scores = outputs[:2]
logit_prob = softmax(prediction_scores[0, maskpos],dim=-1).data.tolist()
predicted_index = torch.argmax(prediction_scores[0, maskpos]).item()
predicted_token = tokenizer.convert_ids_to_tokens([predicted_index])[0]
print(predicted_token, logit_prob[predicted_index])
```
Result: `感 0.36333346366882324`
| [
-0.08486413210630417,
0.047565918415784836,
0.04070831090211868,
0.009689551778137684,
-0.007355625741183758,
0.06383921951055527,
-0.005943899042904377,
0.04361318051815033,
0.01197945885360241,
-0.05198395997285843,
0.02936236746609211,
-0.1411629319190979,
0.01193983294069767,
0.022436846047639847,
0.10053405165672302,
0.06601488590240479,
0.009796923957765102,
0.0618637353181839,
-0.07617328315973282,
-0.07695389539003372,
0.07949873059988022,
0.02291364036500454,
0.033151205629110336,
-0.032426610589027405,
0.02445964515209198,
0.03475543111562729,
-0.051980335265398026,
-0.01454776618629694,
0.12779411673545837,
0.07041701674461365,
-0.037561118602752686,
-0.016856981441378593,
0.01852353662252426,
0.06819330155849457,
0.07526915520429611,
0.07637285441160202,
-0.013551570475101471,
0.043085649609565735,
0.03285500779747963,
-0.007428031414747238,
0.043069325387477875,
0.025885233655571938,
-0.048886582255363464,
-0.04408513009548187,
0.07227561622858047,
0.014241309836506844,
-0.02793145924806595,
-0.009599258191883564,
-0.1035156175494194,
-0.021158969029784203,
-0.06440023332834244,
-0.03301234915852547,
0.07107079029083252,
0.03911010921001434,
-0.037961848080158234,
0.06869149953126907,
0.016304533928632736,
-0.046956587582826614,
0.02843409776687622,
-0.05454579368233681,
-0.10903868079185486,
-0.012323805131018162,
0.01805015467107296,
0.01867865025997162,
-0.08000487089157104,
0.020885581150650978,
-0.05298694223165512,
0.019714079797267914,
-0.0008435705676674843,
0.09625030308961868,
-0.051503099501132965,
-0.04463163763284683,
-0.01435706578195095,
-0.025968756526708603,
-0.02566678076982498,
-0.01907782256603241,
0.08405300974845886,
-0.08158212900161743,
0.007608628366142511,
-0.006144761107861996,
0.008371100760996342,
-0.04334083944559097,
0.0710047110915184,
0.07503586262464523,
0.008963917382061481,
-0.019622264429926872,
-0.029000552371144295,
-0.04423382133245468,
0.00011514028301462531,
0.01725929044187069,
-0.04728947579860687,
-0.0764077752828598,
0.07086824625730515,
0.03626804053783417,
0.013195513747632504,
-0.008639533072710037,
-0.006514823064208031,
0.002402179641649127,
-0.04899618774652481,
0.0711938887834549,
0.0098008643835783,
-0.014730258844792843,
-0.01097271777689457,
-0.07690154761075974,
-0.0044402373023331165,
0.032338857650756836,
-0.05348923057317734,
-0.044335998594760895,
0.048811230808496475,
-0.029985833913087845,
0.046967513859272,
-0.08722499758005142,
-0.02556717023253441,
-0.08667416125535965,
0.025667771697044373,
-0.013047736138105392,
0.00694251898676157,
-0.043023526668548584,
0.015987951308488846,
0.05009118467569351,
0.05907650291919708,
0.017198147252202034,
-0.03551790490746498,
0.030670229345560074,
-0.03321005403995514,
-0.034643229097127914,
0.05989990755915642,
-1.4344209506553936e-33,
0.003639353672042489,
0.04826824367046356,
0.025986023247241974,
0.002220765221863985,
-0.024973884224891663,
-0.008971250616014004,
0.017607782036066055,
0.03421170637011528,
-0.08360283076763153,
-0.021508069708943367,
0.006840068846940994,
0.019658854231238365,
-0.1298397034406662,
0.015633298084139824,
-0.11159093677997589,
-0.015074823051691055,
-0.027306165546178818,
0.047641098499298096,
0.027203494682908058,
0.048492103815078735,
0.08013438433408737,
0.027072977274656296,
-0.03781326860189438,
-0.04452897980809212,
-0.0735604539513588,
0.04152309149503708,
0.06554088741540909,
-0.11889511346817017,
-0.03881753236055374,
0.021441055461764336,
-0.07075301557779312,
0.027066323906183243,
-0.013598158955574036,
0.013487286865711212,
-0.0650758221745491,
-0.036912061274051666,
-0.021384110674262047,
-0.04665813595056534,
-0.040555231273174286,
-0.057848792523145676,
-0.037243809551000595,
0.0201861634850502,
-0.03169667348265648,
-0.06728468090295792,
-0.023874185979366302,
0.01647838205099106,
-0.00786990113556385,
0.006251565646380186,
0.08367367833852768,
-0.004265882074832916,
0.024855978786945343,
0.025475643575191498,
-0.054259270429611206,
0.031427234411239624,
0.033641621470451355,
-0.06486330181360245,
-0.005917803850024939,
0.03997243195772171,
0.049260854721069336,
-0.04163260757923126,
-0.005661506671458483,
-0.03567681461572647,
0.06005329266190529,
0.08427824079990387,
0.06781284511089325,
0.036675065755844116,
-0.018516741693019867,
-0.04106596112251282,
-0.037659671157598495,
0.012460682541131973,
-0.06589581072330475,
0.01655193790793419,
-0.053790781646966934,
0.024232851341366768,
0.013496276922523975,
-0.06455216556787491,
-0.015162074938416481,
-0.036351922899484634,
-0.038257528096437454,
0.01564949005842209,
-0.06431552767753601,
-0.006295257713645697,
0.014305885881185532,
-0.026992864906787872,
-0.055348411202430725,
-0.05865734815597534,
0.05248504504561424,
-0.011074735783040524,
0.021621743217110634,
-0.0352606326341629,
-0.013299248181283474,
-0.09015485644340515,
0.008548201993107796,
-0.011613617651164532,
-0.07185454666614532,
-1.455786938014031e-33,
0.009637976065278053,
0.019626716151833534,
-0.06073921173810959,
0.037025272846221924,
0.004824398551136255,
-0.05433656647801399,
0.027507444843649864,
0.10425816476345062,
0.00454676104709506,
0.006686485838145018,
-0.03410406783223152,
-0.02363506332039833,
-0.015319467522203922,
-0.028822388499975204,
0.03199866786599159,
0.008879235945641994,
0.004794781096279621,
0.07842785865068436,
0.020349064841866493,
0.03743014857172966,
0.05833571404218674,
0.007385448087006807,
-0.1449204981327057,
0.04091670736670494,
-0.05437466874718666,
0.07387556880712509,
0.003208167152479291,
-0.0028002904728055,
0.05518772825598717,
0.02837192825973034,
-0.04719778522849083,
0.055012088268995285,
-0.031427279114723206,
0.1118488758802414,
-0.07190799713134766,
0.014301340095698833,
-0.03635832667350769,
-0.03096678853034973,
-0.03300119563937187,
-0.013633789494633675,
0.02645125985145569,
-0.003951411694288254,
-0.058202847838401794,
0.06171863153576851,
-0.01790107972919941,
-0.03460269793868065,
-0.0733291283249855,
-0.042377062141895294,
0.02201819233596325,
-0.061773061752319336,
-0.005434258840978146,
-0.015486691147089005,
-0.07891114801168442,
-0.023860231041908264,
-0.08839567750692368,
0.027785154059529305,
0.017409393563866615,
-0.08783578872680664,
-0.05479530990123749,
-0.059006355702877045,
-0.05063460022211075,
-0.030258366838097572,
0.07448701560497284,
-0.06844133883714676,
0.002904796041548252,
-0.04088125005364418,
0.07167775928974152,
0.02925843745470047,
0.021266235038638115,
-0.06567719578742981,
0.08994163572788239,
0.07177745550870895,
0.04911765828728676,
0.08128534257411957,
0.03646225854754448,
0.08322485536336899,
0.07533732801675797,
-0.05386888235807419,
-0.04177350923418999,
-0.012583854608237743,
-0.08390694856643677,
-0.02670062705874443,
0.09110243618488312,
0.09265969693660736,
-0.007824921049177647,
-0.014219729229807854,
0.013602817431092262,
0.08354891091585159,
-0.007750878110527992,
0.053346194326877594,
-0.050828173756599426,
0.020413102582097054,
0.008241058327257633,
0.09542293101549149,
0.04595058783888817,
-5.0604025858547175e-8,
-0.05922660231590271,
-0.04340086132287979,
-0.06211479753255844,
0.011885486543178558,
-0.13889150321483612,
-0.019886063411831856,
-0.02161424234509468,
-0.05255334824323654,
-0.028357328847050667,
-0.09159036725759506,
0.028566405177116394,
0.043512120842933655,
-0.05713295936584473,
0.03372012823820114,
-0.028389746323227882,
0.043017297983169556,
-0.07031060755252838,
0.045788493007421494,
-0.0040056598372757435,
-0.01091404352337122,
-0.08233005553483963,
0.03530963137745857,
0.03695838525891304,
-0.10898872464895248,
-0.044496651738882065,
0.033978015184402466,
-0.09717084467411041,
0.12171761691570282,
-0.05443357303738594,
-0.0013176779029890895,
0.04252547025680542,
0.006143311504274607,
-0.00926838256418705,
0.04504133760929108,
0.0015987645601853728,
0.020342795178294182,
0.02858593314886093,
-0.12506404519081116,
0.015215789899230003,
0.03293416276574135,
0.09089107066392899,
-0.006864781025797129,
-0.10433131456375122,
0.02174295112490654,
0.11080846190452576,
0.024835944175720215,
0.01866115629673004,
-0.08324545621871948,
0.029798928648233414,
0.09683873504400253,
0.033999379724264145,
-0.04871075600385666,
-0.07695139944553375,
-0.007554312236607075,
-0.015099259093403816,
0.02737065777182579,
-0.011722179129719734,
0.015997860580682755,
0.043335579335689545,
0.020321568474173546,
0.020131515339016914,
0.08791416138410568,
0.08546097576618195,
0.0062320660799741745
] |
M-CLIP/XLM-Roberta-Large-Vit-B-32 | cfb9f55a6aad08a948167a8360fc11bce171d941 | 2022-06-02T23:23:21.000Z | [
"pytorch",
"tf",
"M-CLIP",
"multilingual",
"transformers"
] | null | false | M-CLIP | null | M-CLIP/XLM-Roberta-Large-Vit-B-32 | 2,052 | null | transformers | ---
language: multilingual
---
## Multilingual-clip: XLM-Roberta-Large-Vit-B-32
Multilingual-CLIP extends OpenAI's English text encoders to multiple other languages. This model *only* contains the multilingual text encoder. The corresponding image model `ViT-B-32` can be retrieved via instructions found on OpenAI's [CLIP repository on Github](https://github.com/openai/CLIP). We provide a usage example below.
## Requirements
To use both the multilingual text encoder and corresponding image encoder, we need to install the packages [`multilingual-clip`](https://github.com/FreddeFrallan/Multilingual-CLIP) and [`clip`](https://github.com/openai/CLIP).
```
pip install multilingual-clip
pip install git+https://github.com/openai/CLIP.git
```
## Usage
Extracting embeddings from the text encoder can be done in the following way:
```python
from multilingual_clip import pt_multilingual_clip
import transformers
texts = [
'Three blind horses listening to Mozart.',
'Älgen är skogens konung!',
'Wie leben Eisbären in der Antarktis?',
'Вы знали, что все белые медведи левши?'
]
model_name = 'M-CLIP/XLM-Roberta-Large-Vit-B-32'
# Load Model & Tokenizer
model = pt_multilingual_clip.MultilingualCLIP.from_pretrained(model_name)
tokenizer = transformers.AutoTokenizer.from_pretrained(model_name)
embeddings = model.forward(texts, tokenizer)
print("Text features shape:", embeddings.shape)
```
Extracting embeddings from the corresponding image encoder:
```python
import torch
import clip
import requests
from PIL import Image
device = "cuda" if torch.cuda.is_available() else "cpu"
model, preprocess = clip.load("ViT-B/32", device=device)
url = "http://images.cocodataset.org/val2017/000000039769.jpg"
image = Image.open(requests.get(url, stream=True).raw)
image = preprocess(image).unsqueeze(0).to(device)
with torch.no_grad():
image_features = model.encode_image(image)
print("Image features shape:", image_features.shape)
```
## Evaluation results
None of the M-CLIP models have been extensivly evaluated, but testing them on Txt2Img retrieval on the humanly translated MS-COCO dataset, we see the following **R@10** results:
| Name | En | De | Es | Fr | Zh | It | Pl | Ko | Ru | Tr | Jp |
| ----------------------------------|:-----: |:-----: |:-----: |:-----: | :-----: |:-----: |:-----: |:-----: |:-----: |:-----: |:-----: |
| [OpenAI CLIP Vit-B/32](https://github.com/openai/CLIP)| 90.3 | - | - | - | - | - | - | - | - | - | - |
| [OpenAI CLIP Vit-L/14](https://github.com/openai/CLIP)| 91.8 | - | - | - | - | - | - | - | - | - | - |
| [OpenCLIP ViT-B-16+-](https://github.com/openai/CLIP)| 94.3 | - | - | - | - | - | - | - | - | - | - |
| [LABSE Vit-L/14](https://huggingface.co/M-CLIP/LABSE-Vit-L-14)| 91.6 | 89.6 | 89.5 | 89.9 | 88.9 | 90.1 | 89.8 | 80.8 | 85.5 | 89.8 | 73.9 |
| [XLM-R Large Vit-B/32](https://huggingface.co/M-CLIP/XLM-Roberta-Large-Vit-B-32)| 91.8 | 88.7 | 89.1 | 89.4 | 89.3 | 89.8| 91.4 | 82.1 | 86.1 | 88.8 | 81.0 |
| [XLM-R Vit-L/14](https://huggingface.co/M-CLIP/XLM-Roberta-Large-Vit-L-14)| 92.4 | 90.6 | 91.0 | 90.0 | 89.7 | 91.1 | 91.3 | 85.2 | 85.8 | 90.3 | 81.9 |
| [XLM-R Large Vit-B/16+](https://huggingface.co/M-CLIP/XLM-Roberta-Large-Vit-B-16Plus)| **95.0** | **93.0** | **93.6** | **93.1** | **94.0** | **93.1** | **94.4** | **89.0** | **90.0** | **93.0** | **84.2** |
## Training/Model details
Further details about the model training and data can be found in the [model card](https://github.com/FreddeFrallan/Multilingual-CLIP/blob/main/larger_mclip.md). | [
-0.03681173548102379,
-0.06648742407560349,
-0.0462019257247448,
-0.05530110374093056,
0.03595270588994026,
-0.02202083170413971,
-0.07926861196756363,
0.01737033575773239,
0.045329634100198746,
-0.08139368146657944,
0.06330745667219162,
-0.11164659261703491,
-0.008179321885108948,
0.07272990047931671,
0.08337252587080002,
0.026162493973970413,
-0.0024961349554359913,
0.11429019272327423,
-0.056838519871234894,
-0.1032315045595169,
0.04229311645030975,
0.008803490549325943,
0.08514396846294403,
-0.015608681365847588,
0.05443580821156502,
0.0012595681473612785,
-0.05222022905945778,
0.0037965408992022276,
0.06280232220888138,
-0.006194825749844313,
0.07646936178207397,
0.035275667905807495,
0.04662451893091202,
0.06881053000688553,
0.02944500558078289,
0.09898074716329575,
-0.055405233055353165,
-0.1109343096613884,
-0.020201046019792557,
-0.023664794862270355,
-0.005893790628761053,
0.04251883178949356,
0.006543224677443504,
-0.09875664114952087,
0.04036162421107292,
-0.00894517544656992,
-0.010444443672895432,
-0.0010040062479674816,
-0.017295485362410545,
-0.040858808904886246,
-0.10187958925962448,
-0.01634540595114231,
-0.03420599550008774,
0.033273328095674515,
0.037780534476041794,
-0.05377824604511261,
0.03234946355223656,
-0.015237828716635704,
-0.031325649470090866,
0.011648726649582386,
-0.05690668150782585,
0.013425435870885849,
-0.019166219979524612,
0.05702955648303032,
-0.06458065658807755,
0.027882039546966553,
0.04335735738277435,
0.01941109634935856,
-0.038252729922533035,
-0.061432965099811554,
-0.0489928312599659,
-0.06751486659049988,
-0.0611700713634491,
-0.01234691496938467,
-0.018577706068754196,
0.01288660615682602,
0.03226698562502861,
0.030491190031170845,
-0.03171510249376297,
-0.06946248561143875,
0.05591638758778572,
-0.0031752544455230236,
0.07416973263025284,
0.023111317306756973,
0.05688965320587158,
0.0016708328621461987,
0.017190121114253998,
0.06092118099331856,
0.05421964451670647,
0.007698503788560629,
-0.009760124608874321,
-0.06919670104980469,
0.02684822492301464,
0.07089813798666,
0.03445640578866005,
-0.03703814744949341,
-0.011507156305015087,
0.07721777260303497,
-0.04568478837609291,
0.027593905106186867,
-0.06229375675320625,
-0.07154024392366409,
0.0830015167593956,
0.03066597506403923,
-0.03459406644105911,
0.03656795620918274,
0.06827615201473236,
0.03926248848438263,
0.039490580558776855,
-0.08342768251895905,
0.05805424600839615,
-0.03197142109274864,
0.017202220857143402,
-0.04152227193117142,
0.028505519032478333,
0.05554571747779846,
0.005991695914417505,
-0.06759345531463623,
0.05448810011148453,
0.05906073749065399,
-0.08460590243339539,
-0.0384853258728981,
-0.003923952113837004,
-0.028725862503051758,
0.010049661621451378,
0.019275857135653496,
0.0012412333162501454,
5.076607820238198e-33,
0.04259462654590607,
0.01996573433279991,
0.03750359266996384,
0.006316490471363068,
0.03474436700344086,
0.013055715709924698,
0.019936444237828255,
-0.03256191685795784,
-0.09819211065769196,
-0.094231016933918,
-0.028110239654779434,
-0.0022609864827245474,
-0.07035746425390244,
0.11799934506416321,
-0.015689225867390633,
0.008174180053174496,
-0.009002283215522766,
0.016044359654188156,
0.05975771322846413,
0.10500913113355637,
0.1045868992805481,
0.02532305382192135,
-0.03178495541214943,
0.004502614960074425,
-0.04527999460697174,
-0.0021437183022499084,
0.10044603794813156,
-0.13854026794433594,
0.019675543531775475,
0.0052597965113818645,
-0.05091625824570656,
-0.0042480891570448875,
0.050860773772001266,
0.030414175242185593,
-0.029708316549658775,
-0.024453775957226753,
-0.02690182439982891,
0.021442865952849388,
-0.08529835194349289,
-0.012358914129436016,
0.0906331017613411,
0.06664904206991196,
-0.023648018017411232,
-0.03003394789993763,
0.028870951384305954,
-0.04200278967618942,
-0.041435301303863525,
0.07433383911848068,
-0.02802257426083088,
0.028329918161034584,
0.06601826846599579,
0.0339595302939415,
-0.0785415768623352,
-0.036571986973285675,
0.03407997265458107,
-0.01711771823465824,
0.06279958039522171,
0.01434476301074028,
0.059918902814388275,
0.0031751745846122503,
-0.06275417655706406,
0.004080569837242365,
0.07498078048229218,
0.052553340792655945,
0.13675466179847717,
0.0164599921554327,
-0.039046965539455414,
-0.03813636675477028,
-0.017823772504925728,
0.05141211301088333,
-0.10179080069065094,
0.025344839319586754,
-0.008536855690181255,
-0.01828899420797825,
0.02312440611422062,
-0.016996849328279495,
-0.07236699014902115,
-0.09973086416721344,
0.028816713020205498,
0.023729905486106873,
-0.05056900531053543,
0.030228672549128532,
0.04093049094080925,
-0.03393642604351044,
-0.07652673125267029,
0.028632935136556625,
0.062103379517793655,
-0.03903555870056152,
0.02277141995728016,
0.004783273208886385,
0.04583483561873436,
0.01237547118216753,
-0.03588443621993065,
-0.06205383315682411,
0.03997030854225159,
-3.914998629093014e-33,
0.059564609080553055,
0.04609180986881256,
-0.0175540279597044,
0.09609360992908478,
-0.04853435233235359,
-0.05851500481367111,
0.04277905076742172,
0.04480110853910446,
0.01381111890077591,
-0.044183433055877686,
0.031443484127521515,
-0.051430508494377136,
0.10193654149770737,
0.002145528793334961,
0.018736757338047028,
-0.05692818760871887,
0.01723630167543888,
0.010170107707381248,
0.021015044301748276,
0.03424689918756485,
-0.061106517910957336,
-0.002880154876038432,
0.012629115954041481,
0.025987429544329643,
-0.03650137409567833,
0.055493783205747604,
0.002424195408821106,
-0.011394459754228592,
-0.014060907065868378,
-0.023019343614578247,
-0.025776240974664688,
0.030567266047000885,
-0.1039680615067482,
0.03261733427643776,
-0.05023682117462158,
0.09673264622688293,
0.006000503432005644,
0.03530719503760338,
0.010624161921441555,
0.033475521951913834,
0.07944895327091217,
0.0034923048224300146,
-0.08286161720752716,
-0.0024135620333254337,
-0.06435558944940567,
-0.007657863665372133,
-0.0339297316968441,
0.03596332669258118,
-0.06532756239175797,
-0.05402044206857681,
-0.024592304602265358,
-0.01728670485317707,
-0.09409337490797043,
0.016087640076875687,
-0.001826609717682004,
-0.047372303903102875,
0.03088372014462948,
-0.03553784638643265,
-0.07051771879196167,
-0.09666689485311508,
-0.04056428000330925,
-0.02589637227356434,
0.01820496656000614,
-0.07012373208999634,
0.012347287498414516,
-0.04933419078588486,
-0.017946844920516014,
0.006105921696871519,
-0.003647857578471303,
-0.06888503581285477,
0.027250545099377632,
-0.04998326674103737,
0.08252720534801483,
0.04922109097242355,
0.06713706254959106,
0.03772002086043358,
-0.08977462351322174,
0.020346034318208694,
0.010366602800786495,
-0.008225846104323864,
-0.07456449419260025,
-0.029317650943994522,
0.037684883922338486,
0.059098020195961,
0.0487271212041378,
0.03925497084856033,
-0.009421514347195625,
0.03195979446172714,
0.09557867795228958,
-0.0019446532242000103,
-0.003343636402860284,
0.07501331716775894,
0.01641576923429966,
0.06503409892320633,
0.10858024656772614,
-4.855551338778241e-8,
-0.03897816687822342,
-0.06756915152072906,
-0.13134750723838806,
0.021361837163567543,
-0.057937141507864,
-0.022626429796218872,
-0.017193587496876717,
0.03447222709655762,
0.018787115812301636,
0.035246241837739944,
0.03142198547720909,
-0.010885193012654781,
-0.11559586971998215,
-0.007150862365961075,
-0.0182422436773777,
0.08661048114299774,
0.04535596817731857,
0.08307220786809921,
0.02621425874531269,
0.037285540252923965,
-0.019799236208200455,
-0.024839673191308975,
0.061377957463264465,
-0.06087353453040123,
-0.07629120349884033,
-0.02808019518852234,
-0.08177706599235535,
0.028149478137493134,
0.015841791406273842,
-0.07392407953739166,
0.08528449386358261,
-0.011641056276857853,
-0.043624021112918854,
-0.05317182093858719,
-0.027992036193609238,
-0.043031707406044006,
-0.0046558924950659275,
-0.015476725064218044,
0.08534540235996246,
0.022103989496827126,
0.08531539887189865,
-0.04348747059702873,
-0.09050901234149933,
-0.033652421087026596,
0.041448406875133514,
-0.04434501379728317,
-0.001498978934250772,
-0.08456913381814957,
-0.034846674650907516,
0.0383487343788147,
0.034074973315000534,
-0.06350582093000412,
0.02836924046278,
0.10964952409267426,
0.030455756932497025,
-0.03406306356191635,
-0.0005474732024595141,
-0.005889940541237593,
0.10347718745470047,
0.03783321753144264,
-0.04062718525528908,
-0.024255013093352318,
0.018219996243715286,
-0.024699801579117775
] |
textattack/roberta-base-MNLI | 6f2e633322381bc5897405e417ec531ea3633a3f | 2021-05-20T22:06:43.000Z | [
"pytorch",
"jax",
"roberta",
"text-classification",
"transformers"
] | text-classification | false | textattack | null | textattack/roberta-base-MNLI | 2,037 | 1 | transformers | Entry not found | [
0.0461147278547287,
-0.038838207721710205,
-0.01049656979739666,
-0.03682169318199158,
0.011261860840022564,
0.013094935566186905,
0.0019101888174191117,
-0.013979103416204453,
0.027092741802334785,
-0.015212527476251125,
0.017284274101257324,
-0.08189476281404495,
0.03817418962717056,
-0.04920130595564842,
0.021389011293649673,
-0.015245908871293068,
-0.03203780576586723,
-0.1245758980512619,
0.03150877356529236,
0.032381657510995865,
-0.060957908630371094,
0.05409295856952667,
-0.025087490677833557,
0.01568586938083172,
0.028129950165748596,
-0.04710396006703377,
-0.018688226118683815,
0.013785239309072495,
-0.04001208767294884,
0.01173911802470684,
-0.04317743331193924,
0.05500618368387222,
0.004543041344732046,
0.02973111905157566,
0.14852192997932434,
0.02658126689493656,
0.02907961793243885,
-0.05169107764959335,
0.05803573504090309,
-0.07732241600751877,
-0.017637968063354492,
-0.04219653457403183,
0.041807834059000015,
0.023620979860424995,
0.021563321352005005,
0.016478516161441803,
-0.0021814992651343346,
-0.06400240957736969,
0.06393089145421982,
0.019599027931690216,
-0.08565037697553635,
0.00934905931353569,
-0.008718925528228283,
-0.028583496809005737,
-0.07310017943382263,
0.09416428208351135,
0.001759322709403932,
0.06184990331530571,
0.011840506456792355,
-0.035997264087200165,
0.08358278125524521,
-0.02619801089167595,
0.03736566752195358,
-0.028206506744027138,
-0.07454850524663925,
-0.08883563429117203,
-0.06279942393302917,
-0.008695344440639019,
0.014119276776909828,
-0.0825355276465416,
0.0649217739701271,
-0.00223911227658391,
-0.14716917276382446,
0.07743025571107864,
-0.03548373281955719,
-0.055201586335897446,
0.006981803569942713,
-0.012166670523583889,
0.055111464112997055,
-0.007116836030036211,
-0.023175746202468872,
-0.005835152696818113,
-0.09185640513896942,
0.055196937173604965,
0.034148022532463074,
0.03835180774331093,
0.038685429841279984,
-0.025987252593040466,
0.017804903909564018,
0.022428328171372414,
0.025005368515849113,
-0.10761535167694092,
-0.048001550137996674,
-0.04343584179878235,
0.012374646961688995,
-0.019502125680446625,
0.029218152165412903,
0.0842173621058464,
-0.011719699949026108,
0.09283553808927536,
-0.007015465293079615,
-0.03543110564351082,
-0.06936459988355637,
0.09425332397222519,
-0.010958523489534855,
-0.00805904995650053,
0.004974212497472763,
-0.0031528924591839314,
0.06105927750468254,
-0.03964288905262947,
-0.03619541600346565,
-0.019901901483535767,
0.07134733349084854,
0.039514873176813126,
-0.012729483656585217,
-0.006646515801548958,
-0.04746140539646149,
-0.014432490803301334,
-0.05157482624053955,
0.09506245702505112,
-0.049747664481401443,
-0.04591796174645424,
-0.008965466171503067,
-0.0325421579182148,
-0.08626784384250641,
-0.06624380499124527,
0.02538885548710823,
-4.303924894057984e-33,
0.01133066974580288,
0.0033434738870710135,
-0.002155609894543886,
0.04871906340122223,
-0.023564351722598076,
-0.07933273911476135,
0.0600903145968914,
0.02335330657660961,
-0.03844716399908066,
-0.020433755591511726,
-0.06952055543661118,
-0.03235611692070961,
0.0062485747039318085,
0.064804308116436,
-0.03201229125261307,
0.061689723283052444,
0.0417000837624073,
-0.00761845987290144,
0.03340127319097519,
-0.047770582139492035,
0.00887306872755289,
-0.04066338762640953,
-0.010506896302103996,
0.0106519665569067,
0.021333497017621994,
0.12854498624801636,
-0.009705503471195698,
0.010055632330477238,
-0.017507633194327354,
0.006515394430607557,
0.06334009766578674,
-0.057817306369543076,
0.013668818399310112,
-0.020286159589886665,
0.05430467426776886,
-0.023184705525636673,
0.0828516036272049,
0.0005449643940664828,
-0.10372652113437653,
-0.07634282112121582,
-0.005381610710173845,
-0.039263784885406494,
0.0006114727002568543,
-0.013281986117362976,
0.07119110971689224,
0.043696220964193344,
0.03168422728776932,
0.04338686540722847,
0.05728672817349434,
0.0832006186246872,
-0.07961414009332657,
0.015234283171594143,
0.017002005130052567,
0.047004107385873795,
-0.09794387966394424,
0.004990279674530029,
-0.07062993198633194,
-0.028000490739941597,
-0.04018733277916908,
-0.0702052190899849,
0.011351344175636768,
0.06020182743668556,
-0.03297270089387894,
0.09396500885486603,
0.03417910635471344,
-0.019825750961899757,
-0.034690454602241516,
-0.013036907650530338,
0.05896938592195511,
-0.012359356507658958,
-0.017275206744670868,
-0.07982361316680908,
0.02059139870107174,
0.06737419217824936,
0.04176458343863487,
-0.04978838190436363,
-0.05877475067973137,
-0.06289287656545639,
-0.03354167565703392,
-0.03871942684054375,
0.009898529388010502,
-0.05514208599925041,
-0.11629002541303635,
-0.011855563148856163,
0.10663620382547379,
0.037354156374931335,
-0.0065480442717671394,
-0.051189567893743515,
0.06663123518228531,
0.01874656230211258,
0.032841797918081284,
0.041593004018068314,
-0.06879369914531708,
0.04216769337654114,
-0.01628219522535801,
5.4139394340936695e-34,
0.05697013810276985,
-0.006972255185246468,
0.015711724758148193,
-0.17956365644931793,
0.02320219948887825,
0.007923615165054798,
-0.008062449283897877,
0.0074974060989916325,
0.07391711324453354,
0.0309313777834177,
0.060510627925395966,
0.058605875819921494,
0.09515274316072464,
-0.002282935893163085,
0.001603541080839932,
0.07024981826543808,
0.012629246339201927,
0.07425693422555923,
-0.038426291197538376,
0.01861148327589035,
0.030608950182795525,
-0.02449394389986992,
0.021528491750359535,
-0.003039651783183217,
-0.03676343336701393,
0.03130284696817398,
0.07998586446046829,
0.010451192036271095,
-0.07930229604244232,
-0.013543923385441303,
0.018781835213303566,
0.05168003588914871,
-0.07191970944404602,
0.15783067047595978,
0.026191607117652893,
0.01262354850769043,
0.08218053728342056,
-0.029807550832629204,
-0.07528624683618546,
-0.04250097647309303,
0.017244765534996986,
0.04411793500185013,
0.03708017244935036,
0.009233047254383564,
-0.040271829813718796,
0.022496428340673447,
0.02495843544602394,
0.07633638381958008,
0.005147108342498541,
0.013892097398638725,
0.05610476806759834,
-0.06684739887714386,
0.05862557515501976,
-0.020688841119408607,
0.05377643182873726,
0.06718500703573227,
0.005329249892383814,
-0.01388032827526331,
0.029931528493762016,
0.009508464485406876,
-0.045173756778240204,
0.11534366756677628,
-0.06510116159915924,
0.05117698386311531,
-0.0026125339791178703,
-0.08554837852716446,
-0.03784770518541336,
0.0804959163069725,
0.011298024095594883,
-0.07695550471544266,
-0.04868878796696663,
0.02515520341694355,
0.06252261996269226,
-0.04509226232767105,
-0.01246943511068821,
0.028559505939483643,
-0.030573077499866486,
0.05066261067986488,
-0.08187384903430939,
0.04469604790210724,
0.0034051244147121906,
0.04145054519176483,
-0.021858664229512215,
-0.06112268194556236,
-0.00908052921295166,
-0.05903250351548195,
0.0259539932012558,
0.059690944850444794,
-0.07613514363765717,
-0.03720718249678612,
-0.036316655576229095,
0.07058046013116837,
-0.008224100805819035,
0.041961874812841415,
-0.0285952128469944,
-1.496900736697171e-8,
-0.0014124972512945533,
0.03401879221200943,
-0.040338415652513504,
0.04116074740886688,
0.0935964286327362,
-0.05115952715277672,
0.0008746005478315055,
-0.03389839455485344,
-0.00567849725484848,
-0.010686947964131832,
-0.04789939522743225,
-0.04820054769515991,
-0.02011880651116371,
-0.03209094703197479,
-0.04211259260773659,
-0.10229527950286865,
-0.07819421589374542,
-0.031228765845298767,
-0.02154778689146042,
-0.04960230365395546,
0.08087796717882156,
-0.07801242172718048,
0.06919731199741364,
-0.04999840259552002,
0.03687043860554695,
0.03889009356498718,
-0.049989692866802216,
-0.04254625365138054,
-0.04606937617063522,
0.08682432025671005,
-0.031148413196206093,
0.11826753616333008,
0.034102488309144974,
-0.0208592489361763,
-0.0205202866345644,
0.027134142816066742,
0.09741277992725372,
0.051608603447675705,
0.013477512635290623,
-0.13649295270442963,
-0.022304272279143333,
0.02385953813791275,
0.038732077926397324,
-0.09249968826770782,
-0.04549082741141319,
0.054220106452703476,
0.01160438358783722,
0.051190607249736786,
0.07713303714990616,
-0.022097084671258926,
-0.06127818301320076,
-0.01857956498861313,
0.006740490905940533,
-0.00496308971196413,
0.024095389991998672,
0.0736224576830864,
-0.003481915919110179,
-0.0699305310845375,
-0.006629763171076775,
-0.0598808117210865,
0.05297163128852844,
-0.02902800403535366,
-0.027858933433890343,
-0.01287526823580265
] |
philschmid/MiniLM-L6-H384-uncased-sst2 | 0c0ecdc39368f87291727ec084111e89e30b45b2 | 2021-09-24T09:53:36.000Z | [
"pytorch",
"bert",
"text-classification",
"transformers"
] | text-classification | false | philschmid | null | philschmid/MiniLM-L6-H384-uncased-sst2 | 2,034 | null | transformers | Entry not found | [
0.0461147278547287,
-0.038838207721710205,
-0.01049656979739666,
-0.03682169318199158,
0.011261860840022564,
0.013094935566186905,
0.0019101888174191117,
-0.013979103416204453,
0.027092741802334785,
-0.015212527476251125,
0.017284274101257324,
-0.08189476281404495,
0.03817418962717056,
-0.04920130595564842,
0.021389011293649673,
-0.015245908871293068,
-0.03203780576586723,
-0.1245758980512619,
0.03150877356529236,
0.032381657510995865,
-0.060957908630371094,
0.05409295856952667,
-0.025087490677833557,
0.01568586938083172,
0.028129950165748596,
-0.04710396006703377,
-0.018688226118683815,
0.013785239309072495,
-0.04001208767294884,
0.01173911802470684,
-0.04317743331193924,
0.05500618368387222,
0.004543041344732046,
0.02973111905157566,
0.14852192997932434,
0.02658126689493656,
0.02907961793243885,
-0.05169107764959335,
0.05803573504090309,
-0.07732241600751877,
-0.017637968063354492,
-0.04219653457403183,
0.041807834059000015,
0.023620979860424995,
0.021563321352005005,
0.016478516161441803,
-0.0021814992651343346,
-0.06400240957736969,
0.06393089145421982,
0.019599027931690216,
-0.08565037697553635,
0.00934905931353569,
-0.008718925528228283,
-0.028583496809005737,
-0.07310017943382263,
0.09416428208351135,
0.001759322709403932,
0.06184990331530571,
0.011840506456792355,
-0.035997264087200165,
0.08358278125524521,
-0.02619801089167595,
0.03736566752195358,
-0.028206506744027138,
-0.07454850524663925,
-0.08883563429117203,
-0.06279942393302917,
-0.008695344440639019,
0.014119276776909828,
-0.0825355276465416,
0.0649217739701271,
-0.00223911227658391,
-0.14716917276382446,
0.07743025571107864,
-0.03548373281955719,
-0.055201586335897446,
0.006981803569942713,
-0.012166670523583889,
0.055111464112997055,
-0.007116836030036211,
-0.023175746202468872,
-0.005835152696818113,
-0.09185640513896942,
0.055196937173604965,
0.034148022532463074,
0.03835180774331093,
0.038685429841279984,
-0.025987252593040466,
0.017804903909564018,
0.022428328171372414,
0.025005368515849113,
-0.10761535167694092,
-0.048001550137996674,
-0.04343584179878235,
0.012374646961688995,
-0.019502125680446625,
0.029218152165412903,
0.0842173621058464,
-0.011719699949026108,
0.09283553808927536,
-0.007015465293079615,
-0.03543110564351082,
-0.06936459988355637,
0.09425332397222519,
-0.010958523489534855,
-0.00805904995650053,
0.004974212497472763,
-0.0031528924591839314,
0.06105927750468254,
-0.03964288905262947,
-0.03619541600346565,
-0.019901901483535767,
0.07134733349084854,
0.039514873176813126,
-0.012729483656585217,
-0.006646515801548958,
-0.04746140539646149,
-0.014432490803301334,
-0.05157482624053955,
0.09506245702505112,
-0.049747664481401443,
-0.04591796174645424,
-0.008965466171503067,
-0.0325421579182148,
-0.08626784384250641,
-0.06624380499124527,
0.02538885548710823,
-4.303924894057984e-33,
0.01133066974580288,
0.0033434738870710135,
-0.002155609894543886,
0.04871906340122223,
-0.023564351722598076,
-0.07933273911476135,
0.0600903145968914,
0.02335330657660961,
-0.03844716399908066,
-0.020433755591511726,
-0.06952055543661118,
-0.03235611692070961,
0.0062485747039318085,
0.064804308116436,
-0.03201229125261307,
0.061689723283052444,
0.0417000837624073,
-0.00761845987290144,
0.03340127319097519,
-0.047770582139492035,
0.00887306872755289,
-0.04066338762640953,
-0.010506896302103996,
0.0106519665569067,
0.021333497017621994,
0.12854498624801636,
-0.009705503471195698,
0.010055632330477238,
-0.017507633194327354,
0.006515394430607557,
0.06334009766578674,
-0.057817306369543076,
0.013668818399310112,
-0.020286159589886665,
0.05430467426776886,
-0.023184705525636673,
0.0828516036272049,
0.0005449643940664828,
-0.10372652113437653,
-0.07634282112121582,
-0.005381610710173845,
-0.039263784885406494,
0.0006114727002568543,
-0.013281986117362976,
0.07119110971689224,
0.043696220964193344,
0.03168422728776932,
0.04338686540722847,
0.05728672817349434,
0.0832006186246872,
-0.07961414009332657,
0.015234283171594143,
0.017002005130052567,
0.047004107385873795,
-0.09794387966394424,
0.004990279674530029,
-0.07062993198633194,
-0.028000490739941597,
-0.04018733277916908,
-0.0702052190899849,
0.011351344175636768,
0.06020182743668556,
-0.03297270089387894,
0.09396500885486603,
0.03417910635471344,
-0.019825750961899757,
-0.034690454602241516,
-0.013036907650530338,
0.05896938592195511,
-0.012359356507658958,
-0.017275206744670868,
-0.07982361316680908,
0.02059139870107174,
0.06737419217824936,
0.04176458343863487,
-0.04978838190436363,
-0.05877475067973137,
-0.06289287656545639,
-0.03354167565703392,
-0.03871942684054375,
0.009898529388010502,
-0.05514208599925041,
-0.11629002541303635,
-0.011855563148856163,
0.10663620382547379,
0.037354156374931335,
-0.0065480442717671394,
-0.051189567893743515,
0.06663123518228531,
0.01874656230211258,
0.032841797918081284,
0.041593004018068314,
-0.06879369914531708,
0.04216769337654114,
-0.01628219522535801,
5.4139394340936695e-34,
0.05697013810276985,
-0.006972255185246468,
0.015711724758148193,
-0.17956365644931793,
0.02320219948887825,
0.007923615165054798,
-0.008062449283897877,
0.0074974060989916325,
0.07391711324453354,
0.0309313777834177,
0.060510627925395966,
0.058605875819921494,
0.09515274316072464,
-0.002282935893163085,
0.001603541080839932,
0.07024981826543808,
0.012629246339201927,
0.07425693422555923,
-0.038426291197538376,
0.01861148327589035,
0.030608950182795525,
-0.02449394389986992,
0.021528491750359535,
-0.003039651783183217,
-0.03676343336701393,
0.03130284696817398,
0.07998586446046829,
0.010451192036271095,
-0.07930229604244232,
-0.013543923385441303,
0.018781835213303566,
0.05168003588914871,
-0.07191970944404602,
0.15783067047595978,
0.026191607117652893,
0.01262354850769043,
0.08218053728342056,
-0.029807550832629204,
-0.07528624683618546,
-0.04250097647309303,
0.017244765534996986,
0.04411793500185013,
0.03708017244935036,
0.009233047254383564,
-0.040271829813718796,
0.022496428340673447,
0.02495843544602394,
0.07633638381958008,
0.005147108342498541,
0.013892097398638725,
0.05610476806759834,
-0.06684739887714386,
0.05862557515501976,
-0.020688841119408607,
0.05377643182873726,
0.06718500703573227,
0.005329249892383814,
-0.01388032827526331,
0.029931528493762016,
0.009508464485406876,
-0.045173756778240204,
0.11534366756677628,
-0.06510116159915924,
0.05117698386311531,
-0.0026125339791178703,
-0.08554837852716446,
-0.03784770518541336,
0.0804959163069725,
0.011298024095594883,
-0.07695550471544266,
-0.04868878796696663,
0.02515520341694355,
0.06252261996269226,
-0.04509226232767105,
-0.01246943511068821,
0.028559505939483643,
-0.030573077499866486,
0.05066261067986488,
-0.08187384903430939,
0.04469604790210724,
0.0034051244147121906,
0.04145054519176483,
-0.021858664229512215,
-0.06112268194556236,
-0.00908052921295166,
-0.05903250351548195,
0.0259539932012558,
0.059690944850444794,
-0.07613514363765717,
-0.03720718249678612,
-0.036316655576229095,
0.07058046013116837,
-0.008224100805819035,
0.041961874812841415,
-0.0285952128469944,
-1.496900736697171e-8,
-0.0014124972512945533,
0.03401879221200943,
-0.040338415652513504,
0.04116074740886688,
0.0935964286327362,
-0.05115952715277672,
0.0008746005478315055,
-0.03389839455485344,
-0.00567849725484848,
-0.010686947964131832,
-0.04789939522743225,
-0.04820054769515991,
-0.02011880651116371,
-0.03209094703197479,
-0.04211259260773659,
-0.10229527950286865,
-0.07819421589374542,
-0.031228765845298767,
-0.02154778689146042,
-0.04960230365395546,
0.08087796717882156,
-0.07801242172718048,
0.06919731199741364,
-0.04999840259552002,
0.03687043860554695,
0.03889009356498718,
-0.049989692866802216,
-0.04254625365138054,
-0.04606937617063522,
0.08682432025671005,
-0.031148413196206093,
0.11826753616333008,
0.034102488309144974,
-0.0208592489361763,
-0.0205202866345644,
0.027134142816066742,
0.09741277992725372,
0.051608603447675705,
0.013477512635290623,
-0.13649295270442963,
-0.022304272279143333,
0.02385953813791275,
0.038732077926397324,
-0.09249968826770782,
-0.04549082741141319,
0.054220106452703476,
0.01160438358783722,
0.051190607249736786,
0.07713303714990616,
-0.022097084671258926,
-0.06127818301320076,
-0.01857956498861313,
0.006740490905940533,
-0.00496308971196413,
0.024095389991998672,
0.0736224576830864,
-0.003481915919110179,
-0.0699305310845375,
-0.006629763171076775,
-0.0598808117210865,
0.05297163128852844,
-0.02902800403535366,
-0.027858933433890343,
-0.01287526823580265
] |
lidiya/bart-base-samsum | eeb19117db15f1388c7188cb455e7a98af647792 | 2022-07-20T14:56:27.000Z | [
"pytorch",
"bart",
"text2text-generation",
"en",
"dataset:samsum",
"transformers",
"seq2seq",
"summarization",
"license:apache-2.0",
"model-index",
"autotrain_compatible"
] | summarization | false | lidiya | null | lidiya/bart-base-samsum | 2,030 | 1 | transformers | ---
language: en
tags:
- bart
- seq2seq
- summarization
license: apache-2.0
datasets:
- samsum
widget:
- text: "Jeff: Can I train a \U0001F917 Transformers model on Amazon SageMaker? \n\
Philipp: Sure you can use the new Hugging Face Deep Learning Container. \nJeff:\
\ ok.\nJeff: and how can I get started? \nJeff: where can I find documentation?\
\ \nPhilipp: ok, ok you can find everything here. https://huggingface.co/blog/the-partnership-amazon-sagemaker-and-hugging-face\n"
model-index:
- name: bart-base-samsum
results:
- task:
name: Abstractive Text Summarization
type: abstractive-text-summarization
dataset:
name: 'SAMSum Corpus: A Human-annotated Dialogue Dataset for Abstractive Summarization'
type: samsum
metrics:
- name: Validation ROUGE-1
type: rouge-1
value: 46.6619
- name: Validation ROUGE-2
type: rouge-2
value: 23.3285
- name: Validation ROUGE-L
type: rouge-l
value: 39.4811
- name: Test ROUGE-1
type: rouge-1
value: 44.9932
- name: Test ROUGE-2
type: rouge-2
value: 21.7286
- name: Test ROUGE-L
type: rouge-l
value: 38.1921
- task:
type: summarization
name: Summarization
dataset:
name: samsum
type: samsum
config: samsum
split: test
metrics:
- name: ROUGE-1
type: rouge
value: 45.0148
verified: true
- name: ROUGE-2
type: rouge
value: 21.6861
verified: true
- name: ROUGE-L
type: rouge
value: 38.1728
verified: true
- name: ROUGE-LSUM
type: rouge
value: 41.2794
verified: true
- name: loss
type: loss
value: 1.597476601600647
verified: true
- name: gen_len
type: gen_len
value: 17.6606
verified: true
---
## `bart-base-samsum`
This model was obtained by fine-tuning `facebook/bart-base` on Samsum dataset.
## Usage
```python
from transformers import pipeline
summarizer = pipeline("summarization", model="lidiya/bart-base-samsum")
conversation = '''Jeff: Can I train a 🤗 Transformers model on Amazon SageMaker?
Philipp: Sure you can use the new Hugging Face Deep Learning Container.
Jeff: ok.
Jeff: and how can I get started?
Jeff: where can I find documentation?
Philipp: ok, ok you can find everything here. https://huggingface.co/blog/the-partnership-amazon-sagemaker-and-hugging-face
'''
summarizer(conversation)
```
## Training procedure
- Colab notebook: https://colab.research.google.com/drive/1RInRjLLso9E2HG_xjA6j8JO3zXzSCBRF?usp=sharing
## Results
| key | value |
| --- | ----- |
| eval_rouge1 | 46.6619 |
| eval_rouge2 | 23.3285 |
| eval_rougeL | 39.4811 |
| eval_rougeLsum | 43.0482 |
| test_rouge1 | 44.9932 |
| test_rouge2 | 21.7286 |
| test_rougeL | 38.1921 |
| test_rougeLsum | 41.2672 |
| [
-0.10074169188737869,
-0.04047642648220062,
0.003605861682444811,
-0.005681260488927364,
0.03903839364647865,
0.04243213310837746,
-0.05308918654918671,
0.00017262752226088196,
-0.03246826305985451,
-0.010472914204001427,
0.031036755070090294,
-0.0805387943983078,
0.029658988118171692,
-0.05514615774154663,
0.06239739805459976,
0.024031147360801697,
0.0011508641764521599,
-0.017195003107190132,
-0.07197105884552002,
0.013436510227620602,
0.05383699759840965,
-0.010064875707030296,
0.0030612004920840263,
-0.020716657862067223,
-0.008753279224038124,
0.039200276136398315,
-0.030040502548217773,
0.04727207124233246,
0.08700932562351227,
-0.05460205301642418,
0.030695520341396332,
0.02648855373263359,
0.09443247318267822,
0.04589562118053436,
-0.023024018853902817,
0.13590900599956512,
0.0013975946931168437,
-0.01599280908703804,
-0.0551883727312088,
-0.022083301097154617,
-0.057284966111183167,
-0.03389487788081169,
-0.056422557681798935,
-0.044513702392578125,
0.0905175432562828,
-0.03822726756334305,
-0.07231709361076355,
-0.05439240485429764,
0.011622251011431217,
-0.028327403590083122,
-0.1710282564163208,
-0.06924772262573242,
0.0029814704321324825,
0.021536849439144135,
0.005533964838832617,
0.05914228782057762,
-0.01582464762032032,
-0.019786378368735313,
-0.0035041612572968006,
-0.0665721744298935,
0.052063263952732086,
-0.03608229383826256,
-0.025451775640249252,
0.05030064657330513,
-0.01000046357512474,
0.01150920893996954,
0.0006777099915780127,
0.07464227080345154,
0.024225564673542976,
-0.038270607590675354,
-0.10678084939718246,
-0.053029704838991165,
-0.026785483583807945,
0.020807022228837013,
0.0029650419019162655,
0.0212467722594738,
0.0017584034940227866,
-0.01551024429500103,
0.014649211429059505,
-0.08842745423316956,
-0.035415906459093094,
0.047116611152887344,
-0.010936734266579151,
0.04632864519953728,
-0.025745995342731476,
0.02076566405594349,
0.08090290427207947,
0.004656576551496983,
-0.009485728107392788,
0.008135639131069183,
-0.021784583106637,
-0.07231743633747101,
0.03220006078481674,
-0.017146065831184387,
-0.0238101314753294,
0.05226223170757294,
0.003405866678804159,
0.009561853483319283,
0.015026349574327469,
0.10012952983379364,
0.011136961169540882,
-0.008360370062291622,
0.024779899045825005,
-0.06093038618564606,
-0.06499544531106949,
-0.030530070886015892,
-0.05610234662890434,
0.04578665643930435,
0.046493057161569595,
-0.06566991657018661,
0.026241518557071686,
-0.009347797371447086,
-0.08859221637248993,
-0.05919709801673889,
0.029807178303599358,
-0.030071604996919632,
0.010991629213094711,
-0.07533708214759827,
0.044422298669815063,
0.005466949660331011,
0.037905920296907425,
0.00048007420264184475,
0.05500209704041481,
-0.026195921003818512,
-0.049586959183216095,
-0.01076523493975401,
-0.03316693380475044,
5.553358941572947e-33,
0.06528294086456299,
0.005624639336019754,
-0.026642784476280212,
0.06287162750959396,
0.0788826271891594,
-0.05421524494886398,
-0.014727777801454067,
0.03590315207839012,
-0.059874553233385086,
-0.028453931212425232,
0.023050157353281975,
0.06743685901165009,
-0.040977708995342255,
0.05534486845135689,
-0.04816855862736702,
-0.065221406519413,
-0.023577962070703506,
0.004106106236577034,
0.02419838309288025,
-0.008194522932171822,
0.050388552248477936,
-0.041235316544771194,
0.017005423083901405,
-0.0038257925771176815,
0.005048448219895363,
0.09512753039598465,
0.0941687673330307,
-0.04662192240357399,
0.019400129094719887,
0.025861889123916626,
-0.02173985168337822,
-0.048197999596595764,
-0.019667815417051315,
-0.01759340986609459,
0.04158081114292145,
0.01269574649631977,
-0.027362681925296783,
-0.041392721235752106,
0.036969419568777084,
-0.043691765516996384,
0.00037524965591728687,
0.019216103479266167,
0.026531629264354706,
-0.07099878787994385,
-0.030965568497776985,
0.01704372465610504,
0.05611424520611763,
-0.010638481937348843,
0.10484511405229568,
-0.015383018180727959,
-0.057113587856292725,
-0.018842194229364395,
0.045346975326538086,
-0.011743023991584778,
-0.01700720004737377,
-0.014704256318509579,
0.0006120670004747808,
0.05727371573448181,
-0.01295510958880186,
-0.02134520560503006,
-0.03598802909255028,
0.04000081121921539,
0.03085302747786045,
0.023067165166139603,
0.019307123497128487,
0.028311965987086296,
-0.0656377524137497,
0.01979483850300312,
0.08395696431398392,
-0.022647734731435776,
-0.05204210802912712,
0.052995309233665466,
0.04165290296077728,
0.06187049299478531,
0.006409475579857826,
-0.04357011243700981,
-0.0018364849966019392,
-0.0440070666372776,
-0.027789458632469177,
0.024979187175631523,
-0.13727809488773346,
0.06362276524305344,
0.03089652583003044,
0.024856096133589745,
-0.07593942433595657,
-0.047035571187734604,
0.09332588315010071,
0.011872109957039356,
-0.0013650571927428246,
-0.026145976036787033,
-0.01406495738774538,
0.04571161046624184,
-0.05069877952337265,
-0.045795440673828125,
-0.014259208925068378,
-6.378781891170741e-33,
0.03481627255678177,
0.03254309669137001,
-0.11296682059764862,
0.006604500114917755,
0.027394277974963188,
0.03313407674431801,
0.03270183876156807,
0.12007129937410355,
-0.0036321026273071766,
-0.05018627271056175,
0.05136542394757271,
-0.03904404118657112,
0.04299924895167351,
-0.04710124060511589,
-0.00015589536633342505,
0.0022451758850365877,
-0.027131743729114532,
-0.06415779888629913,
-0.036009419709444046,
0.03386214002966881,
-0.035095881670713425,
0.06348845362663269,
-0.08721274137496948,
0.016655826941132545,
0.07058420777320862,
0.000671190966386348,
0.04158226028084755,
0.03813925012946129,
-0.007994433864951134,
-0.0007624007412232459,
0.08128155767917633,
-0.02407173439860344,
-0.10995062440633774,
0.05444199591875076,
-0.10086887329816818,
-0.055979982018470764,
0.02477974072098732,
-0.0073256706818938255,
-0.04846355691552162,
0.016341587528586388,
0.14298464357852936,
-0.012740852311253548,
-0.038199927657842636,
-0.022509777918457985,
-0.05232760310173035,
0.018664121627807617,
-0.10184997320175171,
-0.04567813128232956,
-0.0310042817145586,
-0.08669055253267288,
0.03532766178250313,
-0.055107954889535904,
-0.06440113484859467,
-0.07495427876710892,
-0.0737338662147522,
-0.017716970294713974,
0.10084530711174011,
0.019405432045459747,
-0.040782563388347626,
-0.02536560222506523,
-0.07290948927402496,
-0.004497390240430832,
-0.015736505389213562,
-0.020153585821390152,
0.0009070406085811555,
-0.06684453040361404,
-0.019408756867051125,
-0.049093157052993774,
-0.04264729470014572,
-0.06903038918972015,
0.013811303302645683,
0.02737387642264366,
0.052541621029376984,
0.04451792687177658,
0.07119747996330261,
0.00006802835559938103,
0.009419810026884079,
-0.0372321754693985,
0.0329580120742321,
-0.06139988824725151,
0.008871638216078281,
-0.048027295619249344,
0.08340837061405182,
0.0774792954325676,
0.05462609604001045,
0.07882601767778397,
-0.03620751574635506,
0.14730830490589142,
-0.02363765984773636,
0.02157820761203766,
-0.1314571648836136,
0.04533714801073074,
-0.033477019518613815,
0.14059248566627502,
-0.0026220043655484915,
-5.450625550906807e-8,
-0.08061232417821884,
-0.029905330389738083,
0.006580989342182875,
0.021244358271360397,
-0.08327889442443848,
0.043354522436857224,
0.024972250685095787,
-0.018045885488390923,
-0.0009870530338957906,
0.006862764246761799,
-0.002330449875444174,
0.006822660565376282,
-0.0843873918056488,
0.020950887352228165,
-0.046622272580862045,
0.06443885713815689,
0.0034198754001408815,
0.13534098863601685,
-0.03545315936207771,
-0.11472772806882858,
0.029774315655231476,
0.01115364208817482,
0.026738811284303665,
-0.04356289654970169,
0.07133802026510239,
0.003701520152390003,
-0.06933838129043579,
0.11168289929628372,
-0.03866241127252579,
-0.07901447266340256,
0.024230338633060455,
0.03220296651124954,
0.015493696555495262,
-0.031113190576434135,
0.07547859102487564,
0.06614089757204056,
-0.030949359759688377,
-0.01891172118484974,
0.032443832606077194,
0.07750142365694046,
0.06398815661668777,
0.09211000800132751,
-0.1075373962521553,
0.0007059643394313753,
0.09110400825738907,
0.02400187961757183,
-0.028330467641353607,
-0.057518370449543,
0.007726656273007393,
0.05941952392458916,
0.030639488250017166,
-0.08387162536382675,
0.00746125727891922,
0.036204781383275986,
-0.03881797567009926,
0.08773503452539444,
0.009319953620433807,
-0.03147150203585625,
0.10139315575361252,
0.025782782584428787,
0.04157981276512146,
0.05218612030148506,
-0.02092810720205307,
0.056818392127752304
] |
akdeniz27/roberta-large-cuad | 32cd27aa93ae12e576f214c40c558bdcc5081220 | 2021-11-14T08:43:30.000Z | [
"pytorch",
"roberta",
"question-answering",
"en",
"dataset:cuad",
"transformers",
"autotrain_compatible"
] | question-answering | false | akdeniz27 | null | akdeniz27/roberta-large-cuad | 2,024 | null | transformers | ---
language: en
datasets:
- cuad
---
# RoBERTa Large Model fine-tuned with CUAD dataset
This model is the fine-tuned version of "RoBERTa Large"
using CUAD dataset https://huggingface.co/datasets/cuad
Link for model checkpoint: https://github.com/TheAtticusProject/cuad
For the use of the model with CUAD: https://github.com/marshmellow77/cuad-demo
and https://huggingface.co/spaces/akdeniz27/contract-understanding-atticus-dataset-demo | [
-0.06537282466888428,
-0.054273571819067,
-0.03968266770243645,
-0.07697393000125885,
-0.03899088501930237,
0.01574712246656418,
-0.05326983705163002,
-0.004687028471380472,
-0.04908955097198486,
0.03284201771020889,
-0.005614298861473799,
-0.039843328297138214,
0.012881170026957989,
-0.0926748588681221,
-0.06678672134876251,
-0.02838195487856865,
0.03988778218626976,
0.013089578598737717,
-0.046217869967222214,
-0.02561514638364315,
-0.07099425047636032,
0.03785065934062004,
0.014318186789751053,
0.002742449287325144,
0.028432238847017288,
-0.003643463831394911,
0.006586459930986166,
-0.00498203095048666,
0.041062358766794205,
-0.07452050596475601,
0.01812843233346939,
0.0599234513938427,
-0.006007977295666933,
0.07284167408943176,
0.02882976271212101,
0.10919967293739319,
-0.0824226662516594,
-0.04794028773903847,
-0.08209051191806793,
-0.07103142142295837,
0.06870551407337189,
0.05037838965654373,
0.03467097505927086,
0.02133181132376194,
0.016809457913041115,
-0.09839451313018799,
0.014040084555745125,
0.03796766698360443,
-0.002958783647045493,
-0.02950288914144039,
-0.05743103846907616,
-0.06442819535732269,
-0.013598215766251087,
0.0802796259522438,
0.023244358599185944,
-0.029061222448945045,
-0.01631239242851734,
-0.09204038232564926,
-0.05685122311115265,
-0.045496102422475815,
-0.006146488711237907,
-0.053186461329460144,
-0.11568959802389145,
-0.02497926354408264,
0.045085906982421875,
0.02135205827653408,
-0.027285993099212646,
0.04758777841925621,
0.03203263878822327,
-0.015028119087219238,
0.0061837551183998585,
0.029283206909894943,
-0.0264561977237463,
0.016836445778608322,
0.012279137969017029,
0.07395543903112411,
0.06153527647256851,
0.05397791415452957,
0.09690793603658676,
-0.11944360285997391,
-0.035747770220041275,
0.0161498561501503,
0.027472982183098793,
-0.041599493473768234,
0.0328349694609642,
-0.0134124755859375,
0.02422296069562435,
0.06253809481859207,
0.07091379165649414,
-0.0467011034488678,
0.08662065863609314,
0.08009321987628937,
0.022220872342586517,
-0.03298531100153923,
-0.11427591741085052,
0.02339259721338749,
0.038060758262872696,
0.005091584753245115,
-0.038275547325611115,
0.047429993748664856,
-0.021431852132081985,
0.11530061811208725,
0.03554486855864525,
0.03549155220389366,
-0.07013841718435287,
-0.04791109263896942,
0.09281928837299347,
0.0695456713438034,
-0.0738501325249672,
-0.015445713885128498,
0.02509707398712635,
0.030214611440896988,
-0.021199794486165047,
0.0270469319075346,
0.09894466400146484,
-0.008166221901774406,
-0.030049242079257965,
-0.06917188316583633,
-0.009031795896589756,
-0.002552070189267397,
-0.03198016434907913,
-0.020956775173544884,
-0.016987215727567673,
-0.0015919089782983065,
-0.05222351476550102,
0.07235090434551239,
-0.013880296610295773,
2.556603602096517e-33,
0.06702032685279846,
0.040338143706321716,
0.025499729439616203,
0.0055481791496276855,
0.08245120942592621,
0.02037787437438965,
-0.08211849629878998,
-0.024274438619613647,
-0.052893806248903275,
0.049510855227708817,
-0.10204586386680603,
0.015765642747282982,
-0.07668686658143997,
0.04726455360651016,
0.017062416300177574,
-0.014803650788962841,
-0.041495390236377716,
0.04355918988585472,
-0.012249836698174477,
0.02778460830450058,
0.08576271682977676,
0.06369733810424805,
-0.032601647078990936,
-0.02017521671950817,
0.03365028649568558,
0.03774759918451309,
0.06548578292131424,
0.011919945478439331,
-0.049197327345609665,
0.04097184166312218,
-0.03645047917962074,
0.06260932236909866,
-0.014455139636993408,
0.00042480669799260795,
0.027600904926657677,
-0.0077315992675721645,
-0.06738965958356857,
-0.019048430025577545,
0.007023146841675043,
-0.03381650149822235,
0.07698102295398712,
-0.0077217319048941135,
-0.002309092553332448,
-0.019607484340667725,
-0.04843656346201897,
-0.061167165637016296,
0.05315041169524193,
0.017214734107255936,
0.045003265142440796,
-0.06723884493112564,
0.06463391333818436,
0.04195333272218704,
-0.023772727698087692,
-0.03215561807155609,
-0.01995476521551609,
0.02619621902704239,
0.11771111190319061,
0.012852037325501442,
0.036976445466279984,
0.09188437461853027,
0.03391909599304199,
-0.040581442415714264,
0.037813350558280945,
0.03319333493709564,
0.05225798860192299,
0.010330289602279663,
-0.10670650750398636,
-0.03126287832856178,
0.0666496530175209,
0.06746742874383926,
-0.07233162224292755,
0.0022132967133075,
0.04430035501718521,
0.052878689020872116,
0.02035384438931942,
-0.05195801705121994,
0.009823770262300968,
-0.04784921929240227,
-0.05822466313838959,
-0.002246580319479108,
-0.04866757243871689,
0.020988311618566513,
-0.045050859451293945,
-0.01577751152217388,
-0.028517520055174828,
-0.10958131402730942,
0.024755744263529778,
-0.02941242977976799,
-0.06284128129482269,
-0.05201026052236557,
-0.005871844477951527,
0.10023567825555801,
0.02086709253489971,
-0.042375996708869934,
-0.05480535700917244,
-2.7601573466726893e-33,
0.019758086651563644,
-0.03065846487879753,
-0.006396437995135784,
0.0249570794403553,
0.007104517892003059,
-0.010252509266138077,
-0.021841468289494514,
0.09468919038772583,
0.0008000268135219812,
-0.03822818398475647,
0.05286356061697006,
-0.054878052324056625,
0.0572517104446888,
-0.07707107067108154,
0.09319168329238892,
-0.05546867102384567,
0.04159315302968025,
-0.10251860320568085,
0.007068876177072525,
0.02198655903339386,
-0.046330634504556656,
0.0008987589972093701,
-0.0018830093322321773,
0.018968993797898293,
-0.03486519679427147,
-0.0475679486989975,
0.0032691943924874067,
0.08171786367893219,
-0.013018365949392319,
-0.009021816775202751,
0.031825751066207886,
-0.07555341720581055,
-0.02423744834959507,
0.04593924805521965,
-0.10923555493354797,
0.023920278996229172,
0.12205315381288528,
-0.009243488311767578,
-0.012188298627734184,
0.01656004972755909,
0.12904009222984314,
0.04053330793976784,
-0.10570169985294342,
0.007862130180001259,
-0.017027998343110085,
-0.026539167389273643,
-0.11743529886007309,
0.03811707720160484,
-0.1023920401930809,
-0.01787877269089222,
0.06301249563694,
-0.0016211768379434943,
0.028015531599521637,
0.03526860475540161,
0.043154485523700714,
-0.03597574681043625,
0.05173460394144058,
-0.07732594013214111,
0.061530422419309616,
0.02801509015262127,
-0.058007702231407166,
-0.041668590158224106,
-0.05253469571471214,
-0.024359874427318573,
0.06839076429605484,
-0.08283038437366486,
-0.040760040283203125,
-0.001781390281394124,
-0.029485926032066345,
0.06445670872926712,
0.016890710219740868,
-0.03985561430454254,
-0.01247331127524376,
0.08266330510377884,
-0.0054842280223965645,
-0.02735939621925354,
-0.04568108171224594,
-0.04651926830410957,
0.05344162508845329,
-0.042884185910224915,
-0.06432493031024933,
0.013146327808499336,
0.08524816483259201,
0.08314765989780426,
0.05514579266309738,
0.08527267724275589,
0.04428951442241669,
0.05112725868821144,
0.03656237944960594,
0.053873833268880844,
-0.050378669053316116,
-0.06053338944911957,
-0.030263859778642654,
0.07699046283960342,
-0.014968425035476685,
-4.336069636678985e-8,
-0.04301605746150017,
0.014011654071509838,
-0.019947201013565063,
0.008035213686525822,
-0.018291177228093147,
-0.03211961314082146,
-0.008234452456235886,
0.03187539801001549,
0.01063451450318098,
0.07083213329315186,
0.034670501947402954,
0.015531765297055244,
-0.03629826381802559,
-0.002629652852192521,
-0.0360398106276989,
0.08966755121946335,
0.04815615713596344,
0.01857382245361805,
-0.06354453414678574,
0.021705182269215584,
-0.001401327783241868,
0.11229926347732544,
0.0007915259920991957,
-0.005271878559142351,
0.06045927479863167,
0.00709073431789875,
-0.0644124299287796,
0.11892620474100113,
-0.013309184461832047,
-0.041286151856184006,
-0.010844769887626171,
-0.031307462602853775,
0.09475112706422806,
-0.06058894470334053,
-0.04270952567458153,
0.09758415818214417,
0.05286993458867073,
-0.028799938037991524,
0.03582002967596054,
-0.007684053387492895,
0.04951269552111626,
0.029102008789777756,
-0.08622054010629654,
-0.048524513840675354,
0.013727035373449326,
0.0032127434387803078,
0.00432767765596509,
-0.030010830610990524,
0.010886380448937416,
0.11396956443786621,
-0.018839316442608833,
0.014513346366584301,
-0.019977757707238197,
-0.0017994687659665942,
-0.05466597154736519,
-0.04419591650366783,
-0.0846414789557457,
-0.04096679762005806,
0.0842675268650055,
-0.0057164402678608894,
0.07803503423929214,
-0.047830965369939804,
-0.045389678329229355,
0.000950827612541616
] |
deepset/tapas-large-nq-hn-reader | 3b9b9fcfd1789686d05a3b63d8492ac162c7d9fc | 2022-01-23T14:58:08.000Z | [
"pytorch",
"tapas",
"en",
"transformers",
"license:apache-2.0"
] | null | false | deepset | null | deepset/tapas-large-nq-hn-reader | 2,024 | null | transformers | ---
language: en
tags:
- tapas
license: apache-2.0
---
This model contains the converted PyTorch checkpoint of the original Tensorflow model available in the [TaPas repository](https://github.com/google-research/tapas/blob/master/DENSE_TABLE_RETRIEVER.md#reader-models).
It is described in Herzig et al.'s (2021) [paper](https://aclanthology.org/2021.naacl-main.43/) _Open Domain Question Answering over Tables via Dense Retrieval_.
This model has 2 versions which can be used differing only in the table scoring head.
The default one has an adapted table scoring head in order to be able to generate probabilities out of the logits.
The other (non-default) version corredponds to the original checkpoint from the TaPas repository and can be accessed setting `revision="original"`.
# Usage
## In Haystack
If you want to use this model for question-answering over tables, you can load it in [Haystack](https://github.com/deepset-ai/haystack/):
```python
from haystack.nodes import TableReader
table_reader = TableReader(model_name_or_path="deepset/tapas-large-nq-hn-reader")
```
| [
-0.05595678091049194,
-0.1580241620540619,
-0.059637390077114105,
0.00029897800413891673,
0.007156631909310818,
-0.057767804712057114,
-0.02009437419474125,
-0.003179040038958192,
0.005680174566805363,
0.013105287216603756,
-0.015977278351783752,
-0.022349895909428596,
0.013213252648711205,
0.008397658355534077,
-0.031945787370204926,
0.009477567858994007,
0.026224926114082336,
0.018898682668805122,
-0.0648600235581398,
-0.01651942916214466,
-0.0142646050080657,
0.0317545123398304,
0.0612495094537735,
-0.006845772732049227,
0.039242301136255264,
-0.01861213892698288,
-0.02881815657019615,
-0.009964258410036564,
0.028475511819124222,
-0.03219882398843765,
0.026799743995070457,
0.06926562637090683,
-0.086898073554039,
0.08816179633140564,
-0.029768705368041992,
0.015091928653419018,
-0.03783028945326805,
-0.029236456379294395,
-0.003516442608088255,
-0.03945884853601456,
0.01749943383038044,
-0.052259232848882675,
-0.00011627610365394503,
0.007446314208209515,
0.034903500229120255,
-0.049792323261499405,
-0.08737727999687195,
-0.04772390425205231,
0.026328910142183304,
0.03399310261011124,
-0.0685596838593483,
0.02360554039478302,
0.02856888435781002,
0.07169336080551147,
0.009385433048009872,
0.03462250903248787,
-0.022507846355438232,
-0.02839280478656292,
-0.025645365938544273,
-0.02770569920539856,
-0.10740850865840912,
-0.062164194881916046,
-0.007783098611980677,
0.02973446622490883,
0.019376704469323158,
0.013843297958374023,
-0.046424977481365204,
0.0010572337778285146,
0.0728379637002945,
-0.03258192911744118,
-0.019401030614972115,
-0.010150196962058544,
-0.00509309908375144,
0.021971305832266808,
0.011668349616229534,
0.01272581610828638,
0.09913951903581619,
0.030697457492351532,
0.013532809913158417,
-0.012712398543953896,
-0.01427936740219593,
-0.025890707969665527,
0.08576073497533798,
-0.0171036459505558,
0.03799011558294296,
0.001636662520468235,
0.05045194551348686,
0.045183125883340836,
0.08615114539861679,
-0.03949790075421333,
-0.00048445863649249077,
0.006442606914788485,
0.0631607249379158,
-0.04405159875750542,
0.03965940698981285,
0.04839257523417473,
0.08729049563407898,
-0.01279269065707922,
-0.03339144214987755,
0.07488860189914703,
0.04978407546877861,
-0.016952402889728546,
0.0022142468951642513,
-0.03380822762846947,
-0.01174616813659668,
-0.011481554247438908,
-0.0066890413872897625,
0.04752874746918678,
0.0009549098904244602,
-0.03883315995335579,
0.04322867467999458,
0.07654543966054916,
0.0725664272904396,
-0.08668684214353561,
-0.00705769332125783,
-0.031017253175377846,
-0.06359007209539413,
-0.013470591977238655,
0.05721253529191017,
0.00675638671964407,
-0.06286630034446716,
0.02573423646390438,
-0.02447345294058323,
-0.019955873489379883,
0.007050985936075449,
0.04629432037472725,
-0.08032375574111938,
1.7048275385212255e-33,
0.031606849282979965,
-0.002851179800927639,
0.05871099606156349,
-0.08440452069044113,
0.07025895267724991,
0.015567043796181679,
0.05893182381987572,
0.023073215037584305,
0.00956304743885994,
-0.019013404846191406,
-0.06614261865615845,
0.045126207172870636,
-0.05656137317419052,
-0.030247069895267487,
0.006047315429896116,
0.03399166464805603,
-0.11111915111541748,
0.02841152250766754,
-0.01789766177535057,
0.06023658439517021,
0.08135205507278442,
-0.007076920010149479,
0.0007139131193980575,
0.07869662344455719,
0.0658048465847969,
0.025774894282221794,
0.029514959082007408,
-0.010583631694316864,
-0.015139280818402767,
0.04302389174699783,
-0.054644424468278885,
-0.055647533386945724,
-0.08527807146310806,
-0.08126845955848694,
0.005116501823067665,
-0.01017534825950861,
0.005862893536686897,
-0.019930372014641762,
-0.03916185721755028,
-0.09104125201702118,
0.03108830191195011,
0.042917124927043915,
-0.009485256858170033,
-0.047781802713871,
-0.12614208459854126,
-0.05395787954330444,
-0.023405911400914192,
0.07646428048610687,
0.00542089669033885,
-0.05028363689780235,
0.015214669518172741,
-0.06088357791304588,
-0.060128021985292435,
-0.028554538264870644,
0.013879033736884594,
-0.04167453572154045,
0.0018235360039398074,
-0.0013570385053753853,
0.07523225247859955,
0.10272201150655746,
0.06088091433048248,
-0.0338619165122509,
0.0005217964644543827,
0.014433963224291801,
0.06519340723752975,
0.050839073956012726,
-0.10419227927923203,
0.011225592344999313,
0.11640054732561111,
0.01591089367866516,
-0.05440462753176689,
0.019186895340681076,
-0.04379463195800781,
0.05055380240082741,
0.03869333490729332,
-0.07667209208011627,
-0.0030199545435607433,
-0.07408138364553452,
0.06471212953329086,
-0.015517945401370525,
-0.021350279450416565,
0.04463973641395569,
-0.0762711688876152,
0.0060364301316440105,
-0.09604426473379135,
-0.018910694867372513,
0.01811188831925392,
-0.08251774311065674,
-0.05440528318285942,
-0.06934953480958939,
-0.002330974442884326,
-0.015647925436496735,
0.04448719695210457,
-0.03993535786867142,
-0.06642244011163712,
-1.0952839427809518e-33,
-0.02266417071223259,
-0.04567214474081993,
-0.11424096673727036,
0.08806216716766357,
0.01792168989777565,
-0.06478629261255264,
0.06487180292606354,
0.0516355000436306,
0.04604683443903923,
-0.06717793643474579,
0.022185562178492546,
0.006564109120517969,
0.01855546422302723,
-0.03398105502128601,
0.1049303188920021,
-0.032332658767700195,
-0.03200612962245941,
-0.05774986743927002,
-0.03921179473400116,
0.09347183257341385,
-0.041918233036994934,
0.04308096691966057,
-0.030007055029273033,
0.04023659974336624,
0.013995913788676262,
-0.007310920394957066,
-0.0264479611068964,
0.014818036928772926,
0.002105678664520383,
-0.029442600905895233,
-0.01827339269220829,
-0.00005126693577039987,
-0.02144291251897812,
-0.013923564925789833,
-0.06861431896686554,
0.011842024512588978,
0.08952973783016205,
-0.08106772601604462,
-0.011292102746665478,
0.0934998095035553,
0.046921540051698685,
0.06309444457292557,
-0.03156868740916252,
0.06234198436141014,
-0.022955838590860367,
0.07491789758205414,
-0.08954083174467087,
0.06383448839187622,
-0.05078232288360596,
-0.04928179830312729,
0.049247633665800095,
-0.06448366492986679,
0.012490471825003624,
0.036165013909339905,
-0.0154134351760149,
0.0013887147651985288,
0.052132878452539444,
-0.031460609287023544,
-0.03201289474964142,
0.005575967952609062,
-0.04853092133998871,
0.05889549106359482,
0.025553863495588303,
0.027294611558318138,
0.036036230623722076,
0.0084956344217062,
-0.04045199230313301,
0.036892205476760864,
-0.08013779670000076,
0.021927891299128532,
-0.07673540711402893,
-0.06763242185115814,
0.08021692931652069,
0.04864601790904999,
0.11601398140192032,
0.032179635018110275,
-0.02700016088783741,
0.005444135516881943,
0.023764124140143394,
-0.12701810896396637,
-0.08049247413873672,
-0.010731630958616734,
0.07884985953569412,
0.06697829067707062,
0.08854828774929047,
0.01509201992303133,
0.059288475662469864,
0.0023299609310925007,
0.012313634157180786,
0.057975783944129944,
-0.024393390864133835,
-0.07961799204349518,
-0.09327003359794617,
0.1266258805990219,
0.0076243760995566845,
-5.8163415417311626e-8,
-0.08828294277191162,
0.10154125839471817,
-0.08107831329107285,
0.051030442118644714,
0.020030122250318527,
-0.012760897167026997,
0.030042365193367004,
0.07662717252969742,
0.0020213276147842407,
-0.007296738214790821,
0.04438644275069237,
0.049063414335250854,
-0.06060551479458809,
-0.016062147915363312,
0.010939042083919048,
0.13240572810173035,
0.056332532316446304,
0.08097446709871292,
-0.050706710666418076,
-0.027384735643863678,
0.04690871760249138,
0.04194179177284241,
-0.025075864046812057,
0.023223700001835823,
0.028828775510191917,
0.04003790020942688,
-0.06166394054889679,
0.10674112290143967,
0.0019117745105177164,
-0.054488226771354675,
0.006476365961134434,
-0.0030090962536633015,
0.020832430571317673,
-0.03228374943137169,
0.079962819814682,
0.10097147524356842,
-0.008417187258601189,
-0.023934565484523773,
0.017740851268172264,
0.12208814918994904,
-0.006502529140561819,
0.03067205101251602,
-0.054048147052526474,
-0.046962764114141464,
-0.0014110972406342626,
-0.00570718152448535,
-0.0859561637043953,
-0.04725532606244087,
0.032437656074762344,
0.03397002071142197,
-0.0014336099848151207,
-0.0508248545229435,
-0.051582422107458115,
0.05557119846343994,
-0.007168502081185579,
-0.0008085277513600886,
-0.013354456052184105,
-0.06523165851831436,
0.030856076627969742,
-0.017941290512681007,
0.16831600666046143,
0.014131181873381138,
0.01318478025496006,
0.06148405373096466
] |
facebook/wav2vec2-large-robust | 2493a2c576276145c3e066d9243b0e391fab673a | 2021-11-05T12:45:27.000Z | [
"pytorch",
"wav2vec2",
"pretraining",
"en",
"dataset:libri_light",
"dataset:common_voice",
"dataset:switchboard",
"dataset:fisher",
"arxiv:2104.01027",
"transformers",
"speech",
"license:apache-2.0"
] | null | false | facebook | null | facebook/wav2vec2-large-robust | 2,021 | 9 | transformers | ---
language: en
datasets:
- libri_light
- common_voice
- switchboard
- fisher
tags:
- speech
license: apache-2.0
---
# Wav2Vec2-Large-Robust
[Facebook's Wav2Vec2](https://ai.facebook.com/blog/wav2vec-20-learning-the-structure-of-speech-from-raw-audio/)
The large model pretrained on 16kHz sampled speech audio.
Speech datasets from multiple domains were used to pretrain the model:
- [Libri-Light](https://github.com/facebookresearch/libri-light): open-source audio books from the LibriVox project; clean, read-out audio data
- [CommonVoice](https://huggingface.co/datasets/common_voice): crowd-source collected audio data; read-out text snippets
- [Switchboard](https://catalog.ldc.upenn.edu/LDC97S62): telephone speech corpus; noisy telephone data
- [Fisher](https://catalog.ldc.upenn.edu/LDC2004T19): conversational telephone speech; noisy telephone data
When using the model make sure that your speech input is also sampled at 16Khz.
**Note**: This model does not have a tokenizer as it was pretrained on audio alone. In order to use this model **speech recognition**, a tokenizer should be created and the model should be fine-tuned on labeled text data. Check out [this blog](https://huggingface.co/blog/fine-tune-wav2vec2-english) for more in-detail explanation of how to fine-tune the model.
[Paper Robust Wav2Vec2](https://arxiv.org/abs/2104.01027)
Authors: Wei-Ning Hsu, Anuroop Sriram, Alexei Baevski, Tatiana Likhomanenko, Qiantong Xu, Vineel Pratap, Jacob Kahn, Ann Lee, Ronan Collobert, Gabriel Synnaeve, Michael Auli
**Abstract**
Self-supervised learning of speech representations has been a very active research area but most work is focused on a single domain such as read audio books for which there exist large quantities of labeled and unlabeled data. In this paper, we explore more general setups where the domain of the unlabeled data for pre-training data differs from the domain of the labeled data for fine-tuning, which in turn may differ from the test data domain. Our experiments show that using target domain data during pre-training leads to large performance improvements across a variety of setups. On a large-scale competitive setup, we show that pre-training on unlabeled in-domain data reduces the gap between models trained on in-domain and out-of-domain labeled data by 66%-73%. This has obvious practical implications since it is much easier to obtain unlabeled target domain data than labeled data. Moreover, we find that pre-training on multiple domains improves generalization performance on domains not seen during training. Code and models will be made available at this https URL.
The original model can be found under https://github.com/pytorch/fairseq/tree/master/examples/wav2vec#wav2vec-20.
# Usage
See [this notebook](https://colab.research.google.com/drive/1FjTsqbYKphl9kL-eILgUc-bl4zVThL8F?usp=sharing) for more information on how to fine-tune the model.
| [
-0.11485451459884644,
-0.17325398325920105,
-0.017741020768880844,
-0.03250914067029953,
-0.002429984277114272,
-0.0013032082933932543,
-0.06846262514591217,
-0.0421626903116703,
-0.038179781287908554,
-0.040125660598278046,
0.003664658172056079,
-0.006724386941641569,
-0.046876952052116394,
-0.03297920525074005,
-0.007760441862046719,
0.0015597825404256582,
0.08309445530176163,
0.04157296195626259,
-0.005458292085677385,
-0.03375859931111336,
0.06350641697645187,
0.06033943593502045,
0.04984022676944733,
-0.013049301691353321,
0.0947079136967659,
0.0510130450129509,
-0.013293344527482986,
-0.015895066782832146,
0.08246447890996933,
-0.027518434450030327,
0.08719465136528015,
0.06101482734084129,
0.10171660035848618,
-0.044144727289676666,
-0.06461286544799805,
-0.0004358711012173444,
0.03078310191631317,
-0.012594391591846943,
-0.004160972777754068,
0.0237279050052166,
0.013099486008286476,
0.02964833192527294,
-0.0027373728808015585,
-0.008444329723715782,
-0.040299635380506516,
-0.00010331368685001507,
-0.020960697904229164,
-0.02750401757657528,
-0.05517829582095146,
0.013590864837169647,
-0.0369902066886425,
-0.06906147301197052,
0.052009206265211105,
0.11328960955142975,
-0.06233180686831474,
-0.023286109790205956,
0.026905618607997894,
0.04351544752717018,
0.07254811376333237,
0.047634731978178024,
-0.06118228659033775,
-0.005021481774747372,
-0.040830086916685104,
0.016595270484685898,
-0.05036041513085365,
0.03926390781998634,
-0.02888251096010208,
0.05686359480023384,
0.07563485205173492,
-0.07397395372390747,
-0.09948674589395523,
0.08768125623464584,
0.07243546098470688,
0.07288014143705368,
0.03038088232278824,
-0.0014582183212041855,
0.10212177783250809,
-0.01026954222470522,
0.03474368155002594,
-0.12178865820169449,
0.01930975168943405,
-0.04709862545132637,
0.05711505562067032,
-0.016742147505283356,
0.050662703812122345,
-0.024733226746320724,
0.0018662576330825686,
0.02207041345536709,
-0.057912759482860565,
-0.04155552387237549,
-0.07494424283504486,
0.022497154772281647,
0.042896971106529236,
0.03023107536137104,
0.00021143302728887647,
0.08256667107343674,
-0.0224754735827446,
-0.01635616272687912,
0.054084572941064835,
0.05204015597701073,
0.025668084621429443,
0.0055429525673389435,
0.016804514452815056,
-0.004769619088619947,
-0.06725781410932541,
-0.12602517008781433,
-0.005890564061701298,
0.08087421208620071,
0.019535832107067108,
0.021117225289344788,
0.04232874512672424,
0.03600737452507019,
-0.05023445934057236,
-0.08099614828824997,
0.08573030680418015,
-0.04478956386446953,
-0.03451858088374138,
-0.059255704283714294,
0.02648373693227768,
0.0836046040058136,
-0.09237293154001236,
0.006184793543070555,
-0.020273404195904732,
0.01141990628093481,
0.043812111020088196,
-0.02729622647166252,
-0.02312081679701805,
7.952351526427242e-34,
0.0635932981967926,
0.050548721104860306,
-0.043101321905851364,
0.048075489699840546,
0.012161177583038807,
-0.09004002809524536,
-0.009733128361403942,
-0.02386224828660488,
-0.04796478524804115,
0.024070391431450844,
0.016318878158926964,
0.04427218437194824,
-0.08218587189912796,
0.08567310869693756,
0.007750953081995249,
-0.001959713874384761,
-0.05423794686794281,
0.007484173867851496,
0.02983805537223816,
0.02487110160291195,
0.08440355956554413,
0.01596241444349289,
0.09116705507040024,
0.06366626918315887,
0.09047393500804901,
0.06295912712812424,
0.043972864747047424,
-0.043152324855327606,
0.03498813882470131,
0.04582381621003151,
-0.08376780152320862,
-0.053594257682561874,
0.03998396918177605,
-0.026213068515062332,
0.061417724937200546,
0.04265053942799568,
-0.0005440646200440824,
-0.07278058677911758,
-0.05626009777188301,
-0.08829547464847565,
0.033142510801553726,
0.016648493707180023,
0.052146535366773605,
-0.06642329692840576,
-0.0661153569817543,
-0.03733370825648308,
-0.04433311149477959,
0.009104778990149498,
0.0018469878705218434,
0.003275812603533268,
0.00025516594178043306,
-0.003583722747862339,
-0.09696445614099503,
0.00796284805983305,
-0.027923153713345528,
-0.029211705550551414,
0.036122847348451614,
0.021735932677984238,
0.055814195424318314,
0.0604233518242836,
-0.006621420383453369,
0.07420685887336731,
0.040524765849113464,
-0.002231447957456112,
0.0408245250582695,
-0.01764853484928608,
-0.018228286877274513,
-0.01894538477063179,
-0.015422755852341652,
-0.08138067275285721,
-0.04624062404036522,
-0.04895194619894028,
0.030407872051000595,
0.008318501524627209,
0.009043561294674873,
0.02764531597495079,
-0.0074499486945569515,
-0.08141490072011948,
-0.010750574059784412,
0.05840194225311279,
-0.01733303628861904,
0.007005461025983095,
-0.039622146636247635,
-0.06803707033395767,
-0.028641939163208008,
-0.019257793202996254,
-0.004970394540578127,
-0.1227516308426857,
-0.08189065754413605,
-0.03398723155260086,
-0.03210565447807312,
0.04017721489071846,
-0.053604066371917725,
-0.02999320812523365,
-0.057401277124881744,
-1.9058719700003767e-33,
-0.01413178164511919,
0.09388335049152374,
-0.004041074775159359,
0.11161413043737411,
0.01913919858634472,
0.021000145003199577,
0.08791746944189072,
0.08871609717607498,
0.020235348492860794,
-0.029379645362496376,
0.022429179400205612,
-0.07243414968252182,
0.07370981574058533,
-0.04887717589735985,
0.05989369377493858,
-0.026143744587898254,
0.019509289413690567,
-0.04314231500029564,
0.03490252420306206,
0.024238577112555504,
0.032893601804971695,
0.0047615449875593185,
-0.012623651884496212,
0.04963093250989914,
-0.04796146601438522,
-0.01421324536204338,
-0.08204053342342377,
0.04562285542488098,
-0.016231941059231758,
-0.004177709575742483,
-0.06750714778900146,
-0.011903184466063976,
-0.03938004747033119,
-0.03109074756503105,
-0.060788773000240326,
0.035664089024066925,
0.06025759130716324,
0.04231790080666542,
-0.008202482014894485,
-0.04945145547389984,
0.10128992050886154,
0.061614274978637695,
-0.041700903326272964,
-0.1348937600851059,
-0.03422040492296219,
-0.04343819245696068,
-0.17250174283981323,
0.03356289863586426,
-0.005304307676851749,
-0.05666636303067207,
0.04748229309916496,
0.016206223517656326,
0.01987464912235737,
0.014686636626720428,
-0.015187421813607216,
-0.06235256791114807,
0.026468632742762566,
0.004246565047651529,
0.030323335900902748,
0.014808603562414646,
-0.05299019813537598,
-0.013858198188245296,
-0.0822620764374733,
-0.01456590835005045,
0.006259085610508919,
0.03472311794757843,
0.009585094638168812,
-0.005159776657819748,
0.02399682253599167,
-0.009755722247064114,
0.023862585425376892,
-0.025708286091685295,
0.02024938352406025,
0.0035922820679843426,
-0.09832869470119476,
0.00033119527506642044,
-0.07123076170682907,
-0.037490539252758026,
-0.04889308288693428,
-0.03855384513735771,
0.0367405079305172,
0.018650144338607788,
0.06174567714333534,
0.0602232851088047,
0.11938333511352539,
0.0753713920712471,
0.028584174811840057,
-0.047110989689826965,
0.0027135408017784357,
0.00763760507106781,
-0.06472043693065643,
0.04676099866628647,
-0.003964108880609274,
0.024009274318814278,
0.005971904844045639,
-4.832400080090338e-8,
-0.07171275466680527,
0.03480156883597374,
0.04284104332327843,
-0.03251929208636284,
-0.017078742384910583,
-0.10782843828201294,
0.02324756048619747,
0.07306328415870667,
-0.009556537494063377,
0.0407087467610836,
0.06901838630437851,
-0.014907739125192165,
0.004366849083453417,
0.055957041680812836,
0.0138119887560606,
0.06935662776231766,
0.010826083831489086,
0.016510700806975365,
-0.025141730904579163,
-0.11130884289741516,
0.050548993051052094,
0.06438802927732468,
0.03749161958694458,
0.008828145451843739,
0.12458769977092743,
-0.025885554030537605,
-0.001889158971607685,
0.05059623718261719,
0.026915976777672768,
-0.05547347664833069,
-0.059464309364557266,
0.05272431671619415,
0.0036839800886809826,
-0.08880715817213058,
0.058006804436445236,
0.025149598717689514,
-0.09662539511919022,
-0.03563597425818443,
-0.02848409116268158,
0.05237884819507599,
0.002837663283571601,
0.0698816180229187,
-0.0904683843255043,
-0.022317832335829735,
0.06471718102693558,
0.009647593833506107,
-0.04688630253076553,
-0.06354911625385284,
0.004294820129871368,
0.046343863010406494,
-0.009579666890203953,
0.07233067601919174,
-0.022777942940592766,
-0.03185249865055084,
0.04260025545954704,
0.05831030011177063,
-0.03486984223127365,
-0.00456378934904933,
0.018759101629257202,
0.019653983414173126,
-0.011230789124965668,
0.011050974018871784,
-0.03574956953525543,
0.031928058713674545
] |
hf-internal-testing/tiny-random-deberta | 449491e17107f61f2e8df35a0e20a55e9c4afd3c | 2021-09-17T19:22:32.000Z | [
"pytorch",
"tf",
"deberta",
"transformers"
] | null | false | hf-internal-testing | null | hf-internal-testing/tiny-random-deberta | 2,019 | null | transformers | Entry not found | [
0.0461147278547287,
-0.038838207721710205,
-0.01049656979739666,
-0.03682169318199158,
0.011261860840022564,
0.013094935566186905,
0.0019101888174191117,
-0.013979103416204453,
0.027092741802334785,
-0.015212527476251125,
0.017284274101257324,
-0.08189476281404495,
0.03817418962717056,
-0.04920130595564842,
0.021389011293649673,
-0.015245908871293068,
-0.03203780576586723,
-0.1245758980512619,
0.03150877356529236,
0.032381657510995865,
-0.060957908630371094,
0.05409295856952667,
-0.025087490677833557,
0.01568586938083172,
0.028129950165748596,
-0.04710396006703377,
-0.018688226118683815,
0.013785239309072495,
-0.04001208767294884,
0.01173911802470684,
-0.04317743331193924,
0.05500618368387222,
0.004543041344732046,
0.02973111905157566,
0.14852192997932434,
0.02658126689493656,
0.02907961793243885,
-0.05169107764959335,
0.05803573504090309,
-0.07732241600751877,
-0.017637968063354492,
-0.04219653457403183,
0.041807834059000015,
0.023620979860424995,
0.021563321352005005,
0.016478516161441803,
-0.0021814992651343346,
-0.06400240957736969,
0.06393089145421982,
0.019599027931690216,
-0.08565037697553635,
0.00934905931353569,
-0.008718925528228283,
-0.028583496809005737,
-0.07310017943382263,
0.09416428208351135,
0.001759322709403932,
0.06184990331530571,
0.011840506456792355,
-0.035997264087200165,
0.08358278125524521,
-0.02619801089167595,
0.03736566752195358,
-0.028206506744027138,
-0.07454850524663925,
-0.08883563429117203,
-0.06279942393302917,
-0.008695344440639019,
0.014119276776909828,
-0.0825355276465416,
0.0649217739701271,
-0.00223911227658391,
-0.14716917276382446,
0.07743025571107864,
-0.03548373281955719,
-0.055201586335897446,
0.006981803569942713,
-0.012166670523583889,
0.055111464112997055,
-0.007116836030036211,
-0.023175746202468872,
-0.005835152696818113,
-0.09185640513896942,
0.055196937173604965,
0.034148022532463074,
0.03835180774331093,
0.038685429841279984,
-0.025987252593040466,
0.017804903909564018,
0.022428328171372414,
0.025005368515849113,
-0.10761535167694092,
-0.048001550137996674,
-0.04343584179878235,
0.012374646961688995,
-0.019502125680446625,
0.029218152165412903,
0.0842173621058464,
-0.011719699949026108,
0.09283553808927536,
-0.007015465293079615,
-0.03543110564351082,
-0.06936459988355637,
0.09425332397222519,
-0.010958523489534855,
-0.00805904995650053,
0.004974212497472763,
-0.0031528924591839314,
0.06105927750468254,
-0.03964288905262947,
-0.03619541600346565,
-0.019901901483535767,
0.07134733349084854,
0.039514873176813126,
-0.012729483656585217,
-0.006646515801548958,
-0.04746140539646149,
-0.014432490803301334,
-0.05157482624053955,
0.09506245702505112,
-0.049747664481401443,
-0.04591796174645424,
-0.008965466171503067,
-0.0325421579182148,
-0.08626784384250641,
-0.06624380499124527,
0.02538885548710823,
-4.303924894057984e-33,
0.01133066974580288,
0.0033434738870710135,
-0.002155609894543886,
0.04871906340122223,
-0.023564351722598076,
-0.07933273911476135,
0.0600903145968914,
0.02335330657660961,
-0.03844716399908066,
-0.020433755591511726,
-0.06952055543661118,
-0.03235611692070961,
0.0062485747039318085,
0.064804308116436,
-0.03201229125261307,
0.061689723283052444,
0.0417000837624073,
-0.00761845987290144,
0.03340127319097519,
-0.047770582139492035,
0.00887306872755289,
-0.04066338762640953,
-0.010506896302103996,
0.0106519665569067,
0.021333497017621994,
0.12854498624801636,
-0.009705503471195698,
0.010055632330477238,
-0.017507633194327354,
0.006515394430607557,
0.06334009766578674,
-0.057817306369543076,
0.013668818399310112,
-0.020286159589886665,
0.05430467426776886,
-0.023184705525636673,
0.0828516036272049,
0.0005449643940664828,
-0.10372652113437653,
-0.07634282112121582,
-0.005381610710173845,
-0.039263784885406494,
0.0006114727002568543,
-0.013281986117362976,
0.07119110971689224,
0.043696220964193344,
0.03168422728776932,
0.04338686540722847,
0.05728672817349434,
0.0832006186246872,
-0.07961414009332657,
0.015234283171594143,
0.017002005130052567,
0.047004107385873795,
-0.09794387966394424,
0.004990279674530029,
-0.07062993198633194,
-0.028000490739941597,
-0.04018733277916908,
-0.0702052190899849,
0.011351344175636768,
0.06020182743668556,
-0.03297270089387894,
0.09396500885486603,
0.03417910635471344,
-0.019825750961899757,
-0.034690454602241516,
-0.013036907650530338,
0.05896938592195511,
-0.012359356507658958,
-0.017275206744670868,
-0.07982361316680908,
0.02059139870107174,
0.06737419217824936,
0.04176458343863487,
-0.04978838190436363,
-0.05877475067973137,
-0.06289287656545639,
-0.03354167565703392,
-0.03871942684054375,
0.009898529388010502,
-0.05514208599925041,
-0.11629002541303635,
-0.011855563148856163,
0.10663620382547379,
0.037354156374931335,
-0.0065480442717671394,
-0.051189567893743515,
0.06663123518228531,
0.01874656230211258,
0.032841797918081284,
0.041593004018068314,
-0.06879369914531708,
0.04216769337654114,
-0.01628219522535801,
5.4139394340936695e-34,
0.05697013810276985,
-0.006972255185246468,
0.015711724758148193,
-0.17956365644931793,
0.02320219948887825,
0.007923615165054798,
-0.008062449283897877,
0.0074974060989916325,
0.07391711324453354,
0.0309313777834177,
0.060510627925395966,
0.058605875819921494,
0.09515274316072464,
-0.002282935893163085,
0.001603541080839932,
0.07024981826543808,
0.012629246339201927,
0.07425693422555923,
-0.038426291197538376,
0.01861148327589035,
0.030608950182795525,
-0.02449394389986992,
0.021528491750359535,
-0.003039651783183217,
-0.03676343336701393,
0.03130284696817398,
0.07998586446046829,
0.010451192036271095,
-0.07930229604244232,
-0.013543923385441303,
0.018781835213303566,
0.05168003588914871,
-0.07191970944404602,
0.15783067047595978,
0.026191607117652893,
0.01262354850769043,
0.08218053728342056,
-0.029807550832629204,
-0.07528624683618546,
-0.04250097647309303,
0.017244765534996986,
0.04411793500185013,
0.03708017244935036,
0.009233047254383564,
-0.040271829813718796,
0.022496428340673447,
0.02495843544602394,
0.07633638381958008,
0.005147108342498541,
0.013892097398638725,
0.05610476806759834,
-0.06684739887714386,
0.05862557515501976,
-0.020688841119408607,
0.05377643182873726,
0.06718500703573227,
0.005329249892383814,
-0.01388032827526331,
0.029931528493762016,
0.009508464485406876,
-0.045173756778240204,
0.11534366756677628,
-0.06510116159915924,
0.05117698386311531,
-0.0026125339791178703,
-0.08554837852716446,
-0.03784770518541336,
0.0804959163069725,
0.011298024095594883,
-0.07695550471544266,
-0.04868878796696663,
0.02515520341694355,
0.06252261996269226,
-0.04509226232767105,
-0.01246943511068821,
0.028559505939483643,
-0.030573077499866486,
0.05066261067986488,
-0.08187384903430939,
0.04469604790210724,
0.0034051244147121906,
0.04145054519176483,
-0.021858664229512215,
-0.06112268194556236,
-0.00908052921295166,
-0.05903250351548195,
0.0259539932012558,
0.059690944850444794,
-0.07613514363765717,
-0.03720718249678612,
-0.036316655576229095,
0.07058046013116837,
-0.008224100805819035,
0.041961874812841415,
-0.0285952128469944,
-1.496900736697171e-8,
-0.0014124972512945533,
0.03401879221200943,
-0.040338415652513504,
0.04116074740886688,
0.0935964286327362,
-0.05115952715277672,
0.0008746005478315055,
-0.03389839455485344,
-0.00567849725484848,
-0.010686947964131832,
-0.04789939522743225,
-0.04820054769515991,
-0.02011880651116371,
-0.03209094703197479,
-0.04211259260773659,
-0.10229527950286865,
-0.07819421589374542,
-0.031228765845298767,
-0.02154778689146042,
-0.04960230365395546,
0.08087796717882156,
-0.07801242172718048,
0.06919731199741364,
-0.04999840259552002,
0.03687043860554695,
0.03889009356498718,
-0.049989692866802216,
-0.04254625365138054,
-0.04606937617063522,
0.08682432025671005,
-0.031148413196206093,
0.11826753616333008,
0.034102488309144974,
-0.0208592489361763,
-0.0205202866345644,
0.027134142816066742,
0.09741277992725372,
0.051608603447675705,
0.013477512635290623,
-0.13649295270442963,
-0.022304272279143333,
0.02385953813791275,
0.038732077926397324,
-0.09249968826770782,
-0.04549082741141319,
0.054220106452703476,
0.01160438358783722,
0.051190607249736786,
0.07713303714990616,
-0.022097084671258926,
-0.06127818301320076,
-0.01857956498861313,
0.006740490905940533,
-0.00496308971196413,
0.024095389991998672,
0.0736224576830864,
-0.003481915919110179,
-0.0699305310845375,
-0.006629763171076775,
-0.0598808117210865,
0.05297163128852844,
-0.02902800403535366,
-0.027858933433890343,
-0.01287526823580265
] |
Helsinki-NLP/opus-mt-ur-en | c803d32b6f7a3a7a8cb1ba91d2947de0009f8cdc | 2020-08-21T14:42:51.000Z | [
"pytorch",
"marian",
"text2text-generation",
"ur",
"en",
"transformers",
"translation",
"license:apache-2.0",
"autotrain_compatible"
] | translation | false | Helsinki-NLP | null | Helsinki-NLP/opus-mt-ur-en | 2,017 | 1 | transformers | ---
language:
- ur
- en
tags:
- translation
license: apache-2.0
---
### urd-eng
* source group: Urdu
* target group: English
* OPUS readme: [urd-eng](https://github.com/Helsinki-NLP/Tatoeba-Challenge/tree/master/models/urd-eng/README.md)
* model: transformer-align
* source language(s): urd
* target language(s): eng
* model: transformer-align
* pre-processing: normalization + SentencePiece (spm32k,spm32k)
* download original weights: [opus-2020-06-17.zip](https://object.pouta.csc.fi/Tatoeba-MT-models/urd-eng/opus-2020-06-17.zip)
* test set translations: [opus-2020-06-17.test.txt](https://object.pouta.csc.fi/Tatoeba-MT-models/urd-eng/opus-2020-06-17.test.txt)
* test set scores: [opus-2020-06-17.eval.txt](https://object.pouta.csc.fi/Tatoeba-MT-models/urd-eng/opus-2020-06-17.eval.txt)
## Benchmarks
| testset | BLEU | chr-F |
|-----------------------|-------|-------|
| Tatoeba-test.urd.eng | 23.2 | 0.435 |
### System Info:
- hf_name: urd-eng
- source_languages: urd
- target_languages: eng
- opus_readme_url: https://github.com/Helsinki-NLP/Tatoeba-Challenge/tree/master/models/urd-eng/README.md
- original_repo: Tatoeba-Challenge
- tags: ['translation']
- languages: ['ur', 'en']
- src_constituents: {'urd'}
- tgt_constituents: {'eng'}
- src_multilingual: False
- tgt_multilingual: False
- prepro: normalization + SentencePiece (spm32k,spm32k)
- url_model: https://object.pouta.csc.fi/Tatoeba-MT-models/urd-eng/opus-2020-06-17.zip
- url_test_set: https://object.pouta.csc.fi/Tatoeba-MT-models/urd-eng/opus-2020-06-17.test.txt
- src_alpha3: urd
- tgt_alpha3: eng
- short_pair: ur-en
- chrF2_score: 0.435
- bleu: 23.2
- brevity_penalty: 0.975
- ref_len: 12029.0
- src_name: Urdu
- tgt_name: English
- train_date: 2020-06-17
- src_alpha2: ur
- tgt_alpha2: en
- prefer_old: False
- long_pair: urd-eng
- helsinki_git_sha: 480fcbe0ee1bf4774bcbe6226ad9f58e63f6c535
- transformers_git_sha: 2207e5d8cb224e954a7cba69fa4ac2309e9ff30b
- port_machine: brutasse
- port_time: 2020-08-21-14:41 | [
-0.08449462801218033,
-0.002718463074415922,
-0.009004054591059685,
-0.043939508497714996,
-0.020228758454322815,
0.022150268778204918,
-0.04535124823451042,
-0.0463683046400547,
0.04170478507876396,
0.0004827983502764255,
0.03506169468164444,
-0.05356161668896675,
-0.0060081337578594685,
-0.021728994324803352,
-0.006575449835509062,
0.01945064589381218,
-0.04578618332743645,
0.0809946283698082,
-0.06257764250040054,
-0.05633089318871498,
0.03672134131193161,
0.050689250230789185,
0.04251185059547424,
0.014965242706239223,
0.06749455630779266,
0.07399937510490417,
-0.06008954346179962,
0.003147828858345747,
0.04725780710577965,
-0.05883566290140152,
0.028085270896553993,
0.04173721373081207,
0.005391017533838749,
0.03977133706212044,
0.06729929149150848,
0.08314783126115799,
-0.047550179064273834,
-0.07097518444061279,
0.018781524151563644,
0.01621638983488083,
0.011857706122100353,
0.02706156298518181,
-0.0066688754595816135,
-0.021926814690232277,
0.02590709738433361,
0.03308345749974251,
-0.025057202205061913,
0.04361472278833389,
-0.016884196549654007,
-0.02593955025076866,
-0.1501632183790207,
-0.025053204968571663,
0.003714008955284953,
0.05701051279902458,
-0.0329691581428051,
0.022579142823815346,
0.029668543487787247,
0.007954435423016548,
0.03295539692044258,
-0.07906193286180496,
-0.10701628774404526,
0.019487101584672928,
-0.07642925530672073,
0.027235297486186028,
0.003239615121856332,
-0.012599929235875607,
0.03988583758473396,
0.04545995965600014,
-0.05841154232621193,
0.04710477590560913,
-0.051762424409389496,
-0.008691267110407352,
-0.015127965249121189,
0.0919293463230133,
-0.04039740562438965,
0.01180268730968237,
0.041759345680475235,
-0.04538458585739136,
-0.0017576342215761542,
-0.08291391283273697,
0.006205422338098288,
-0.05488920956850052,
0.06504710763692856,
0.007829256355762482,
0.08021147549152374,
0.011318366974592209,
0.021857254207134247,
0.005322093144059181,
0.018347477540373802,
0.04591943696141243,
0.01395587157458067,
-0.015029571019113064,
0.04030607268214226,
0.03837186470627785,
0.0000227990767598385,
0.035565465688705444,
0.03261388838291168,
0.05185871571302414,
-0.03907368704676628,
0.1034308671951294,
0.042410917580127716,
-0.0009262232924811542,
0.06386405974626541,
-0.043073397129774094,
-0.10280943661928177,
-0.055538516491651535,
0.03559516370296478,
0.045638538897037506,
-0.006124464329332113,
-0.06576959043741226,
0.016516203060746193,
-0.035672999918460846,
-0.015823479741811752,
-0.08568088710308075,
0.012142656370997429,
-0.06539535522460938,
0.008497873321175575,
-0.023041794076561928,
-0.00452376576140523,
0.02906663343310356,
-0.06516704708337784,
0.025450890883803368,
-0.038937535136938095,
0.031640246510505676,
-0.04465339332818985,
-0.03754084184765816,
-0.0054899356327950954,
2.3813267414713597e-33,
0.05215999856591225,
0.01859435997903347,
-0.024611322209239006,
0.027730528265237808,
-0.03740900754928589,
-0.01976104825735092,
-0.020452264696359634,
-0.011034074239432812,
-0.09956984966993332,
-0.05068967863917351,
-0.044990312308073044,
-0.04283794015645981,
-0.10075836628675461,
0.010059121996164322,
-0.05853469297289848,
-0.019161151722073555,
0.023709887638688087,
0.0376754105091095,
0.017341163009405136,
0.039435673505067825,
0.03980733081698418,
0.029585786163806915,
0.015715541318058968,
-0.05233513563871384,
-0.05889147147536278,
0.0635649636387825,
0.05834447965025902,
-0.12043515592813492,
-0.08765513449907303,
0.027669817209243774,
-0.06039755418896675,
-0.00926037784665823,
-0.0478614866733551,
-0.04820600524544716,
0.01428607665002346,
-0.060139965265989304,
0.0331643782556057,
-0.058781251311302185,
-0.10551579296588898,
-0.05998876318335533,
-0.04524269700050354,
0.01216853503137827,
0.025384288281202316,
-0.04036465287208557,
0.031032763421535492,
0.01648816093802452,
-0.007203131914138794,
0.025314347818493843,
0.07201412320137024,
0.03136545047163963,
0.007182147353887558,
0.022115373983979225,
-0.052224237471818924,
0.008024325594305992,
-0.00372372311539948,
0.07006402313709259,
0.058251846581697464,
-0.02269643172621727,
0.04447650909423828,
0.046768587082624435,
0.026264581829309464,
-0.007424081210047007,
0.039121951907873154,
-0.017003007233142853,
0.1383540779352188,
-0.022530166432261467,
-0.04400429502129555,
-0.07575014233589172,
0.06530784070491791,
0.038384996354579926,
-0.09530819952487946,
-0.05552210658788681,
0.08664082735776901,
0.11611379683017731,
0.04197849705815315,
-0.02741698920726776,
-0.01932820864021778,
-0.0782378688454628,
0.0025655922945588827,
-0.0024075915571302176,
-0.10335015505552292,
0.01635110192000866,
0.04323333874344826,
-0.06757935136556625,
-0.012238851748406887,
-0.019824285060167313,
0.04643544554710388,
-0.09255412966012955,
0.00008467486622976139,
0.009284202009439468,
0.03504569083452225,
0.05145133659243584,
-0.057451434433460236,
-0.02363477647304535,
0.017488226294517517,
-2.852630732186994e-33,
0.037026915699243546,
0.015058922581374645,
-0.07163167744874954,
0.07891567051410675,
-0.021984942257404327,
-0.0752871111035347,
0.03550620749592781,
0.13174425065517426,
0.060416001826524734,
0.041544124484062195,
0.058887314051389694,
-0.09497162699699402,
0.008415477350354195,
-0.0572822205722332,
0.07124848663806915,
-0.02818003110587597,
0.06802400201559067,
0.009089307859539986,
0.041686512529850006,
0.08370911329984665,
-0.0028321179561316967,
0.10166525095701218,
-0.08401993662118912,
0.09658350795507431,
0.01461524236947298,
0.0006554964347742498,
-0.016080345958471298,
0.0565248541533947,
0.016696106642484665,
-0.010183087550103664,
0.02688516117632389,
-0.025188816711306572,
-0.11412596702575684,
0.041099291294813156,
-0.10769858956336975,
0.050436291843652725,
0.004131239373236895,
0.05527403950691223,
0.03457462415099144,
0.09686947613954544,
0.051160864531993866,
0.04371832683682442,
-0.05851224064826965,
-0.017863623797893524,
0.016552714630961418,
-0.046131353825330734,
-0.04880056530237198,
0.019752074033021927,
-0.023911653086543083,
-0.07548077404499054,
0.026265528053045273,
0.016615064814686775,
-0.011609319597482681,
-0.06624805927276611,
0.0384221151471138,
-0.07502913475036621,
-0.026834022253751755,
-0.11983513087034225,
-0.07057885080575943,
-0.03618834167718887,
-0.016802430152893066,
0.048214998096227646,
-0.0011620329460129142,
-0.09637149423360825,
0.04611344262957573,
-0.0077537246979773045,
0.04557514190673828,
0.019332503899931908,
-0.0035353652201592922,
0.007634059526026249,
0.0033025380689650774,
-0.05323117598891258,
0.015474967658519745,
0.033130038529634476,
0.026127472519874573,
-0.08583138138055801,
-0.038455016911029816,
0.0818725973367691,
0.07477074861526489,
-0.0549502968788147,
-0.05103396624326706,
0.0332624688744545,
0.010925594717264175,
0.04683341830968857,
0.08007488399744034,
0.08499963581562042,
0.020144859328866005,
0.01229886058717966,
0.030092837288975716,
0.03215653449296951,
0.025499023497104645,
0.04925728961825371,
0.04243425652384758,
0.1279008686542511,
0.01581789366900921,
-5.3544471967370555e-8,
-0.10627534985542297,
-0.03145698457956314,
-0.13029475510120392,
0.04591509699821472,
-0.04787096381187439,
-0.046716563403606415,
-0.04612931236624718,
-0.030872683972120285,
-0.03170210123062134,
-0.06837017089128494,
-0.009482033550739288,
-0.014900514855980873,
-0.08741434663534164,
0.03789087012410164,
-0.034116145223379135,
0.05037609115242958,
-0.029842689633369446,
0.0760873481631279,
-0.04896572604775429,
-0.07635807245969772,
0.06046811863780022,
0.05871368572115898,
0.007574613206088543,
-0.021890124306082726,
-0.0012899149442091584,
0.0379566065967083,
-0.061725519597530365,
0.044295042753219604,
0.018725838512182236,
0.009284439496695995,
0.02185862325131893,
0.023814158514142036,
-0.04207903891801834,
-0.059881117194890976,
0.03113614022731781,
0.08744761347770691,
0.00021841403213329613,
0.001399530447088182,
0.02499730885028839,
0.08409540355205536,
0.08478569984436035,
0.042075470089912415,
-0.06393376737833023,
0.010383539833128452,
0.026007164269685745,
-0.04018016159534454,
-0.06590467691421509,
-0.07312056422233582,
0.02056560292840004,
-0.07094766199588776,
0.11056412756443024,
-0.03724407032132149,
-0.025364384055137634,
0.03347191959619522,
0.03571685776114464,
0.027111932635307312,
0.05421685427427292,
-0.04077371209859848,
0.020821604877710342,
0.015145641751587391,
0.10566822439432144,
-0.00987569522112608,
-0.029392359778285027,
0.004137938376516104
] |
aychang/roberta-base-imdb | cb6bcadd0540b61c9623bd6295d51ac445ceb135 | 2021-05-20T14:25:56.000Z | [
"pytorch",
"jax",
"roberta",
"text-classification",
"en",
"dataset:imdb",
"transformers",
"license:mit"
] | text-classification | false | aychang | null | aychang/roberta-base-imdb | 2,017 | 1 | transformers | ---
language:
- en
thumbnail:
tags:
- text-classification
license: mit
datasets:
- imdb
metrics:
---
# IMDB Sentiment Task: roberta-base
## Model description
A simple base roBERTa model trained on the "imdb" dataset.
## Intended uses & limitations
#### How to use
##### Transformers
```python
# Load model and tokenizer
from transformers import AutoModelForSequenceClassification, AutoTokenizer
model = AutoModelForQuestionAnswering.from_pretrained(model_name)
tokenizer = AutoTokenizer.from_pretrained(model_name)
# Use pipeline
from transformers import pipeline
model_name = "aychang/roberta-base-imdb"
nlp = pipeline("sentiment-analysis", model=model_name, tokenizer=model_name)
results = nlp(["I didn't really like it because it was so terrible.", "I love how easy it is to watch and get good results."])
```
##### AdaptNLP
```python
from adaptnlp import EasySequenceClassifier
model_name = "aychang/roberta-base-imdb"
texts = ["I didn't really like it because it was so terrible.", "I love how easy it is to watch and get good results."]
classifer = EasySequenceClassifier
results = classifier.tag_text(text=texts, model_name_or_path=model_name, mini_batch_size=2)
```
#### Limitations and bias
This is minimal language model trained on a benchmark dataset.
## Training data
IMDB https://huggingface.co/datasets/imdb
## Training procedure
#### Hardware
One V100
#### Hyperparameters and Training Args
```python
from transformers import TrainingArguments
training_args = TrainingArguments(
output_dir='./models',
overwrite_output_dir=False,
num_train_epochs=2,
per_device_train_batch_size=8,
per_device_eval_batch_size=8,
warmup_steps=500,
weight_decay=0.01,
evaluation_strategy="steps",
logging_dir='./logs',
fp16=False,
eval_steps=800,
save_steps=300000
)
```
## Eval results
```
{'epoch': 2.0,
'eval_accuracy': 0.94668,
'eval_f1': array([0.94603457, 0.94731017]),
'eval_loss': 0.2578844428062439,
'eval_precision': array([0.95762642, 0.93624502]),
'eval_recall': array([0.93472, 0.95864]),
'eval_runtime': 244.7522,
'eval_samples_per_second': 102.144}
```
| [
-0.11160767823457718,
-0.06180599331855774,
-0.012959432788193226,
0.0666927620768547,
-0.018593059852719307,
0.047591518610715866,
0.025127286091446877,
0.09364642202854156,
-0.028776507824659348,
-0.022726155817508698,
0.006121745333075523,
-0.06142342835664749,
0.019239453598856926,
0.029337015002965927,
-0.012794687412679195,
0.05002308636903763,
0.0369383841753006,
-0.013017305172979832,
-0.14709262549877167,
-0.08662605285644531,
0.051144253462553024,
0.05931995064020157,
0.02497032843530178,
-0.001804581144824624,
0.05667820945382118,
0.008071563206613064,
-0.04991075396537781,
0.01649608090519905,
0.0026177363470196724,
0.007393497042357922,
-0.021725576370954514,
0.003210037015378475,
-0.015142418444156647,
0.09129995852708817,
0.03451581671833992,
0.08537671715021133,
-0.01876785047352314,
-0.0049316235817968845,
0.017835067585110664,
-0.04784766584634781,
0.022748978808522224,
-0.03798003867268562,
-0.021942278370261192,
-0.029811875894665718,
0.008664583787322044,
-0.10110220313072205,
-0.005688315257430077,
-0.02884346805512905,
0.050267238169908524,
-0.056791599839925766,
-0.13119567930698395,
0.0024723177775740623,
-0.026390111073851585,
-0.010830569081008434,
-0.04441864788532257,
0.019764769822359085,
0.044478558003902435,
-0.01738659292459488,
0.004621187224984169,
-0.0930260419845581,
-0.04087294638156891,
-0.07043927907943726,
-0.037323128432035446,
-0.01393816713243723,
-0.02811015211045742,
-0.09718991070985794,
-0.05278988927602768,
0.047421105206012726,
0.05802477151155472,
0.019673483446240425,
-0.07037424296140671,
-0.011446303687989712,
-0.0454549603164196,
0.05262093245983124,
0.0335410013794899,
-0.03239414840936661,
0.07575129717588425,
0.0056373197585344315,
0.0363466702401638,
-0.05304422974586487,
-0.05034434050321579,
-0.07286471128463745,
0.07759951055049896,
0.06766781955957413,
0.05305108055472374,
-0.04031871631741524,
0.027314411476254463,
0.054695043712854385,
0.018116841092705727,
-0.037956856191158295,
-0.0640418604016304,
-0.07701484858989716,
0.02194708026945591,
-0.0009018367272801697,
0.01617608591914177,
0.07274825125932693,
-0.0293869748711586,
-0.026872292160987854,
-0.03017505444586277,
0.11840872466564178,
-0.0019048862159252167,
0.03760343790054321,
0.030901987105607986,
-0.03057960607111454,
-0.02553943358361721,
-0.00853559747338295,
0.007624057121574879,
0.034299202263355255,
0.024783194065093994,
-0.06827817857265472,
0.018814902752637863,
0.03341839462518692,
-0.0689045786857605,
-0.005344736389815807,
0.11062280833721161,
0.022949377074837685,
0.018521547317504883,
-0.025730691850185394,
-0.047106578946113586,
0.0992291271686554,
0.030898304656147957,
0.013968887738883495,
-0.039114173501729965,
0.058485157787799835,
0.012204843573272228,
0.04450540617108345,
-0.010027873329818249,
6.128717875894362e-33,
-0.019650965929031372,
-0.009306329302489758,
0.07743145525455475,
0.011860387399792671,
-0.03378548100590706,
-0.00109862070530653,
-0.01343199796974659,
0.03644351661205292,
-0.06577592343091965,
0.005197616759687662,
-0.03434600681066513,
0.06367810070514679,
-0.052070338279008865,
0.006497231312096119,
-0.08925914019346237,
-0.020008957013487816,
-0.10536106675863266,
-0.0033896295353770256,
0.07054682821035385,
0.06893925368785858,
0.07959295064210892,
0.03043520450592041,
-0.03959040716290474,
-0.05191744491457939,
-0.07042287290096283,
-0.015385785140097141,
0.08013714849948883,
-0.05024828761816025,
-0.07329662144184113,
0.0248713418841362,
-0.07561881095170975,
0.005638581234961748,
-0.005164249800145626,
-0.04350831359624863,
-0.023839034140110016,
-0.0658627524971962,
0.011983158998191357,
0.007638180162757635,
-0.01580783724784851,
-0.11121073365211487,
-0.04480794444680214,
0.06413315236568451,
-0.049284566193819046,
-0.03352481499314308,
0.011454332619905472,
0.051430221647024155,
-0.012107967399060726,
0.024219252169132233,
0.05760399252176285,
0.02919834293425083,
0.0711405873298645,
-0.01629060134291649,
0.015586438588798046,
0.037608783692121506,
-0.024272499606013298,
0.07049153745174408,
0.06647814065217972,
0.06170300394296646,
0.11005236208438873,
-0.09315983951091766,
-0.0007637758390046656,
-0.01892816834151745,
0.01399246882647276,
-0.009768001735210419,
0.10749373584985733,
0.0085605438798666,
0.044199202209711075,
0.01426498219370842,
0.04686257243156433,
0.07157931476831436,
-0.0835200697183609,
-0.04297006502747536,
-0.032554034143686295,
0.004775515291839838,
0.08906935155391693,
-0.03316063806414604,
0.014063697308301926,
-0.055036723613739014,
-0.0419338084757328,
0.03762778267264366,
0.04233691468834877,
-0.006420224439352751,
-0.01490561943501234,
-0.07589590549468994,
-0.044554393738508224,
-0.025593625381588936,
0.035684484988451004,
-0.0806872621178627,
-0.05296702682971954,
-0.028061164543032646,
0.002017986262217164,
0.09406761825084686,
-0.035897381603717804,
0.03815511241555214,
-0.04433535784482956,
-7.044667849500889e-33,
0.07768594473600388,
0.01447584480047226,
-0.03397093713283539,
0.006243868265300989,
0.03981664404273033,
-0.04303712770342827,
-0.007862730883061886,
0.04958426579833031,
0.016692332923412323,
-0.0052057490684092045,
0.050096191465854645,
-0.10028500109910965,
0.0026053457986563444,
-0.0035319344606250525,
0.053060561418533325,
-0.006784922908991575,
-0.039615239948034286,
-0.025126708671450615,
-0.00248247804120183,
0.06293614208698273,
-0.10904315859079361,
0.11548430472612381,
-0.12178415060043335,
0.041482068598270416,
-0.08143797516822815,
0.024306604638695717,
-0.029813870787620544,
0.07680365443229675,
0.025743603706359863,
-0.054210178554058075,
-0.002146500162780285,
0.002197316149249673,
-0.06901150196790695,
-0.003566419007256627,
-0.08262251317501068,
0.042596228420734406,
0.014054559171199799,
-0.08864761143922806,
-0.031093880534172058,
0.09385859966278076,
0.10686791688203812,
0.06768838316202164,
-0.094144307076931,
0.01125700119882822,
-0.038844361901283264,
-0.034979235380887985,
0.018681302666664124,
-0.019695540890097618,
-0.04330267757177353,
-0.011016554199159145,
0.027529679238796234,
-0.01309998519718647,
-0.10102009028196335,
0.05481433868408203,
-0.03906476870179176,
-0.053196173161268234,
0.10801123827695847,
-0.06188623607158661,
-0.05994095280766487,
0.041089531034231186,
-0.06848873198032379,
0.008330515585839748,
0.0068182493560016155,
-0.058749567717313766,
0.0030331816524267197,
-0.0728958323597908,
-0.028615692630410194,
-0.007820717990398407,
-0.04592079669237137,
0.052164558321237564,
0.016108373180031776,
0.0074674938805401325,
0.06380023062229156,
-0.01893302984535694,
0.09275776892900467,
0.06945805251598358,
-0.04886016622185707,
-0.007157057989388704,
-0.04494956135749817,
-0.06518455594778061,
-0.1027890220284462,
-0.022700738161802292,
0.03475439175963402,
0.07151881605386734,
-0.028828680515289307,
0.022855296730995178,
0.07308802753686905,
0.1216786801815033,
0.02956969663500786,
-0.0056031132116913795,
0.015495090745389462,
-0.0026367439422756433,
0.06621478497982025,
0.07623124122619629,
0.03793006017804146,
-5.342450037915114e-8,
-0.0740908682346344,
-0.009914999827742577,
-0.0226737093180418,
0.05861286446452141,
-0.05472751334309578,
0.0243903249502182,
-0.03848506882786751,
0.07981782406568527,
-0.03566461428999901,
0.023846838623285294,
0.007581021636724472,
-0.012396940030157566,
-0.10805149376392365,
0.055254995822906494,
-0.01584484428167343,
0.04907483235001564,
0.023331336677074432,
0.08869269490242004,
-0.012369468808174133,
-0.02260814607143402,
0.00730849476531148,
0.01998768001794815,
0.008443753235042095,
-0.04585868865251541,
-0.00899585708975792,
-0.008590090088546276,
-0.03906618058681488,
0.06077299639582634,
-0.040280360728502274,
0.00028406447381712496,
0.028123803436756134,
0.027876749634742737,
-0.03447616845369339,
-0.04906190186738968,
-0.03961459547281265,
0.039043642580509186,
0.06676448881626129,
-0.09939195960760117,
-0.02181994915008545,
0.020548291504383087,
0.0016879300819709897,
0.10062640905380249,
-0.13203635811805725,
-0.014210447669029236,
0.02632567472755909,
-0.023556848987936974,
0.03280603513121605,
-0.08753474056720734,
0.0312807634472847,
0.06366641819477081,
0.0014394845347851515,
-0.06467482447624207,
-0.06379648298025131,
-0.0026876144111156464,
-0.005854110699146986,
-0.0161189753562212,
0.01792890951037407,
-0.023555707186460495,
0.010787438601255417,
0.021853674203157425,
0.02573978900909424,
0.027419840916991234,
0.019608628004789352,
-0.03434105962514877
] |
stas/pegasus-cnn_dailymail-tiny-random | 600d1e9bb307c4c4c7361688317e80fc2612bc5c | 2021-07-01T05:33:00.000Z | [
"pytorch",
"pegasus",
"text2text-generation",
"transformers",
"autotrain_compatible"
] | text2text-generation | false | stas | null | stas/pegasus-cnn_dailymail-tiny-random | 2,015 | null | transformers | This is a tiny random pegasus-cnn_dailymail model used for testing.
See `make-pegasus-cnn_dailymail-tiny-random.py` for how it was created.
| [
-0.10069649666547775,
0.0402926430106163,
-0.01991148293018341,
0.047542788088321686,
0.07618647813796997,
-0.13899047672748566,
0.03711189329624176,
-0.03854881972074509,
0.0337371788918972,
-0.04584392160177231,
0.03367296978831291,
0.06628968566656113,
-0.07178644090890884,
0.036509912461042404,
-0.11461460590362549,
0.03923725336790085,
0.006049045827239752,
-0.054390668869018555,
-0.03299683704972267,
0.027842193841934204,
-0.026792991906404495,
0.05290089175105095,
0.07169313728809357,
0.10415131598711014,
0.004868445452302694,
-0.04314802959561348,
-0.01709357649087906,
-0.0037030722014606,
0.037823449820280075,
-0.10501118749380112,
0.11189965903759003,
0.05590673163533211,
-0.0036770645529031754,
0.050647519528865814,
0.030471792444586754,
0.008902547881007195,
0.010648488067090511,
-0.028429556638002396,
0.009665307588875294,
0.004942681640386581,
0.08433006703853607,
-0.07263271510601044,
0.0383710153400898,
-0.0031070357654243708,
-0.015214352868497372,
0.06024980545043945,
0.001439599902369082,
-0.034361012279987335,
0.04258222132921219,
-0.003448018804192543,
-0.003628771984949708,
-0.07951929420232773,
-0.09993697702884674,
0.024072159081697464,
0.006842413451522589,
0.01944727450609207,
-0.0507931187748909,
-0.02574813924729824,
0.017378950491547585,
-0.03462378680706024,
0.03263016417622566,
0.04972384497523308,
0.0008771218126639724,
0.031315285712480545,
0.0027299458160996437,
-0.02551380917429924,
-0.07669246196746826,
-0.06858629733324051,
0.10557848960161209,
-0.14982189238071442,
-0.013011667877435684,
0.0023800726048648357,
-0.004156004171818495,
0.09209339320659637,
0.024608150124549866,
-0.020757419988512993,
0.07240380346775055,
-0.04044230654835701,
0.11418591439723969,
0.03596547618508339,
-0.019078480079770088,
-0.022504692897200584,
-0.0038765461649745703,
0.04303251951932907,
0.08020813018083572,
-0.024591289460659027,
0.020854134112596512,
0.04474082961678505,
0.05471734330058098,
-0.08448167890310287,
-0.07065795361995697,
0.03196737542748451,
-0.06878852099180222,
0.04163920879364014,
-0.0827401727437973,
-0.002291383920237422,
-0.01900060847401619,
-0.02999131940305233,
-0.07952649146318436,
0.12887008488178253,
0.06131177395582199,
-0.03685881942510605,
0.12526312470436096,
-0.005434267222881317,
0.027477655559778214,
-0.04916076734662056,
-0.006270875688642263,
-0.024332556873559952,
-0.007110548205673695,
-0.04590023308992386,
0.010135435499250889,
0.030313685536384583,
-0.009489934891462326,
-0.03519432991743088,
0.05222484469413757,
-0.06360185891389847,
-0.11297279596328735,
0.07313546538352966,
0.006401898339390755,
0.04443066567182541,
0.006505480036139488,
-0.017758529633283615,
-0.056299708783626556,
-0.11917147040367126,
-0.041122373193502426,
0.027252282947301865,
-0.04222923517227173,
-1.3257565079909543e-33,
0.021304382011294365,
-0.0013364689657464623,
0.01143589522689581,
-0.003480107756331563,
0.08220425993204117,
-0.018171515315771103,
0.0286893080919981,
0.040468502789735794,
-0.07765387743711472,
-0.01318084541708231,
-0.051239509135484695,
-0.03136978670954704,
0.0015680466312915087,
0.11157157272100449,
-0.042424242943525314,
-0.09505519270896912,
0.0020737573504447937,
0.0154647221788764,
0.033084265887737274,
-0.03953345865011215,
-0.010786126367747784,
0.05691711977124214,
-0.02868444286286831,
-0.06895621120929718,
0.04010992497205734,
-0.018499715253710747,
0.02790447697043419,
-0.04099172353744507,
0.006388970650732517,
0.07054797559976578,
0.02497701533138752,
0.05359545722603798,
-0.007793134544044733,
0.05466712638735771,
0.004207099787890911,
-0.001703006448224187,
-0.045149579644203186,
-0.09678523987531662,
-0.033738721162080765,
0.029895607382059097,
0.008934969082474709,
-0.023676350712776184,
0.0002773393061943352,
-0.0431167408823967,
-0.07009248435497284,
0.04440247267484665,
0.04964546486735344,
0.05746083706617355,
0.00906253419816494,
-0.04870512709021568,
0.02861880138516426,
-0.02888864278793335,
0.011194528080523014,
-0.04658687487244606,
-0.04968957602977753,
-0.01923072710633278,
0.07030369341373444,
-0.008348432369530201,
0.043132852762937546,
-0.007907303981482983,
-0.02194978855550289,
0.005151304416358471,
-0.07151240110397339,
0.02486570179462433,
0.046813055872917175,
-0.03216543421149254,
-0.004179247189313173,
-0.014282910153269768,
0.007703850977122784,
0.07491305470466614,
0.05578789860010147,
-0.013461213558912277,
-0.026385769248008728,
0.0044359066523611546,
0.020157696679234505,
-0.06600527465343475,
0.020634658634662628,
-0.00875493697822094,
-0.053259797394275665,
0.04259606450796127,
0.014409148134291172,
-0.016745494678616524,
0.038127560168504715,
-0.05431101843714714,
-0.12328258901834488,
0.051314350217580795,
-0.003253000555559993,
-0.011254509910941124,
-0.03931129723787308,
0.016801834106445312,
-0.05337335169315338,
-0.0655682161450386,
0.016831425949931145,
0.024065261706709862,
-0.033479899168014526,
9.72454793189104e-34,
-0.07902710884809494,
0.00746555021032691,
-0.05569947138428688,
0.05270599201321602,
0.02078324556350708,
0.009572505950927734,
0.025898784399032593,
0.08948235213756561,
-0.03570648282766342,
0.039952363818883896,
0.04905903711915016,
-0.01278811413794756,
0.028401795774698257,
-0.017297353595495224,
0.10389617085456848,
0.019346389919519424,
-0.018535621464252472,
-0.11092258989810944,
0.006295242812484503,
-0.01279879454523325,
-0.062423333525657654,
0.06951376795768738,
-0.040083304047584534,
0.05897102877497673,
0.007806804496794939,
0.00312510272487998,
0.04069577530026436,
0.03266848623752594,
0.0688127800822258,
-0.037060510367155075,
-0.126140296459198,
-0.03141690418124199,
0.011576148681342602,
0.03148535266518593,
0.0033666419330984354,
0.03739805519580841,
0.06589242815971375,
-0.10427549481391907,
0.02550903707742691,
-0.043419983237981796,
0.03622234985232353,
0.06104821711778641,
0.016087090596556664,
0.02197645977139473,
-0.0480540432035923,
0.034556806087493896,
-0.021663593128323555,
0.08182217925786972,
0.01014777086675167,
-0.018709490075707436,
-0.004425495397299528,
0.012138905003666878,
0.000558346975594759,
0.04204576835036278,
-0.07390907406806946,
0.08390834182500839,
-0.06879064440727234,
0.02167677879333496,
0.08109252154827118,
0.021147524937987328,
-0.015038980171084404,
-0.08274517953395844,
-0.04655919224023819,
-0.017448486760258675,
-0.010494397953152657,
-0.004954999312758446,
-0.056914858520030975,
-0.007098443806171417,
-0.04455544054508209,
0.06097828969359398,
0.10236074030399323,
0.03833019733428955,
-0.003999911714345217,
-0.05397043004631996,
-0.03923134133219719,
-0.04171064868569374,
-0.027842702344059944,
0.001996975392103195,
0.015205305069684982,
0.01715199463069439,
0.024396987631917,
-0.10179460048675537,
0.05698370561003685,
0.022121364250779152,
0.06129087135195732,
-0.07862650603055954,
0.0010242513380944729,
0.06723365932703018,
-0.005846406798809767,
0.07897509634494781,
0.01525599230080843,
0.05492938309907913,
-0.045767661184072495,
0.10178030282258987,
0.009243356995284557,
-2.3355317679829568e-8,
0.05527760833501816,
-0.05313863605260849,
0.0026462050154805183,
0.048402246087789536,
0.0012027538614347577,
0.0005803031381219625,
0.05231282487511635,
-0.00888031255453825,
0.039033208042383194,
-0.01631111651659012,
-0.022447245195508003,
-0.013264067471027374,
-0.07019946724176407,
-0.0007374773267656565,
-0.01379560586065054,
0.03302888572216034,
-0.0895840972661972,
0.07311797142028809,
-0.006742806173861027,
-0.027723224833607674,
0.10099964588880539,
0.000966991763561964,
0.04059021174907684,
-0.03732352703809738,
-0.0366273857653141,
0.04303183779120445,
0.02149207703769207,
0.10387393832206726,
-0.0052603078074753284,
-0.05155038833618164,
-0.03613210469484329,
0.02075246162712574,
-0.035520173609256744,
-0.07352553308010101,
-0.05262522026896477,
0.15137939155101776,
-0.07770801335573196,
-0.06390548497438431,
0.03761952370405197,
-0.003361316630616784,
0.08100306987762451,
-0.05127030983567238,
0.08178820461034775,
-0.007338931784033775,
-0.018029039725661278,
0.01511016208678484,
-0.00988916214555502,
-0.14308691024780273,
0.041916947811841965,
0.005623942706733942,
0.032472193241119385,
0.00605853833258152,
0.0035148528404533863,
0.0604381300508976,
-0.10221727192401886,
0.012255517765879631,
0.04110074043273926,
-0.07611444592475891,
-0.009586812928318977,
-0.004642031621187925,
-0.01992121897637844,
0.06902318447828293,
0.026592636480927467,
-0.011606358923017979
] |
hf-internal-testing/tiny-random-big_bird | 0ab074a1d464a4cc6846332560f1f2abca400a71 | 2022-03-25T17:49:02.000Z | [
"pytorch",
"big_bird",
"transformers"
] | null | false | hf-internal-testing | null | hf-internal-testing/tiny-random-big_bird | 2,010 | null | transformers | Entry not found | [
0.0461147278547287,
-0.038838207721710205,
-0.01049656979739666,
-0.03682169318199158,
0.011261860840022564,
0.013094935566186905,
0.0019101888174191117,
-0.013979103416204453,
0.027092741802334785,
-0.015212527476251125,
0.017284274101257324,
-0.08189476281404495,
0.03817418962717056,
-0.04920130595564842,
0.021389011293649673,
-0.015245908871293068,
-0.03203780576586723,
-0.1245758980512619,
0.03150877356529236,
0.032381657510995865,
-0.060957908630371094,
0.05409295856952667,
-0.025087490677833557,
0.01568586938083172,
0.028129950165748596,
-0.04710396006703377,
-0.018688226118683815,
0.013785239309072495,
-0.04001208767294884,
0.01173911802470684,
-0.04317743331193924,
0.05500618368387222,
0.004543041344732046,
0.02973111905157566,
0.14852192997932434,
0.02658126689493656,
0.02907961793243885,
-0.05169107764959335,
0.05803573504090309,
-0.07732241600751877,
-0.017637968063354492,
-0.04219653457403183,
0.041807834059000015,
0.023620979860424995,
0.021563321352005005,
0.016478516161441803,
-0.0021814992651343346,
-0.06400240957736969,
0.06393089145421982,
0.019599027931690216,
-0.08565037697553635,
0.00934905931353569,
-0.008718925528228283,
-0.028583496809005737,
-0.07310017943382263,
0.09416428208351135,
0.001759322709403932,
0.06184990331530571,
0.011840506456792355,
-0.035997264087200165,
0.08358278125524521,
-0.02619801089167595,
0.03736566752195358,
-0.028206506744027138,
-0.07454850524663925,
-0.08883563429117203,
-0.06279942393302917,
-0.008695344440639019,
0.014119276776909828,
-0.0825355276465416,
0.0649217739701271,
-0.00223911227658391,
-0.14716917276382446,
0.07743025571107864,
-0.03548373281955719,
-0.055201586335897446,
0.006981803569942713,
-0.012166670523583889,
0.055111464112997055,
-0.007116836030036211,
-0.023175746202468872,
-0.005835152696818113,
-0.09185640513896942,
0.055196937173604965,
0.034148022532463074,
0.03835180774331093,
0.038685429841279984,
-0.025987252593040466,
0.017804903909564018,
0.022428328171372414,
0.025005368515849113,
-0.10761535167694092,
-0.048001550137996674,
-0.04343584179878235,
0.012374646961688995,
-0.019502125680446625,
0.029218152165412903,
0.0842173621058464,
-0.011719699949026108,
0.09283553808927536,
-0.007015465293079615,
-0.03543110564351082,
-0.06936459988355637,
0.09425332397222519,
-0.010958523489534855,
-0.00805904995650053,
0.004974212497472763,
-0.0031528924591839314,
0.06105927750468254,
-0.03964288905262947,
-0.03619541600346565,
-0.019901901483535767,
0.07134733349084854,
0.039514873176813126,
-0.012729483656585217,
-0.006646515801548958,
-0.04746140539646149,
-0.014432490803301334,
-0.05157482624053955,
0.09506245702505112,
-0.049747664481401443,
-0.04591796174645424,
-0.008965466171503067,
-0.0325421579182148,
-0.08626784384250641,
-0.06624380499124527,
0.02538885548710823,
-4.303924894057984e-33,
0.01133066974580288,
0.0033434738870710135,
-0.002155609894543886,
0.04871906340122223,
-0.023564351722598076,
-0.07933273911476135,
0.0600903145968914,
0.02335330657660961,
-0.03844716399908066,
-0.020433755591511726,
-0.06952055543661118,
-0.03235611692070961,
0.0062485747039318085,
0.064804308116436,
-0.03201229125261307,
0.061689723283052444,
0.0417000837624073,
-0.00761845987290144,
0.03340127319097519,
-0.047770582139492035,
0.00887306872755289,
-0.04066338762640953,
-0.010506896302103996,
0.0106519665569067,
0.021333497017621994,
0.12854498624801636,
-0.009705503471195698,
0.010055632330477238,
-0.017507633194327354,
0.006515394430607557,
0.06334009766578674,
-0.057817306369543076,
0.013668818399310112,
-0.020286159589886665,
0.05430467426776886,
-0.023184705525636673,
0.0828516036272049,
0.0005449643940664828,
-0.10372652113437653,
-0.07634282112121582,
-0.005381610710173845,
-0.039263784885406494,
0.0006114727002568543,
-0.013281986117362976,
0.07119110971689224,
0.043696220964193344,
0.03168422728776932,
0.04338686540722847,
0.05728672817349434,
0.0832006186246872,
-0.07961414009332657,
0.015234283171594143,
0.017002005130052567,
0.047004107385873795,
-0.09794387966394424,
0.004990279674530029,
-0.07062993198633194,
-0.028000490739941597,
-0.04018733277916908,
-0.0702052190899849,
0.011351344175636768,
0.06020182743668556,
-0.03297270089387894,
0.09396500885486603,
0.03417910635471344,
-0.019825750961899757,
-0.034690454602241516,
-0.013036907650530338,
0.05896938592195511,
-0.012359356507658958,
-0.017275206744670868,
-0.07982361316680908,
0.02059139870107174,
0.06737419217824936,
0.04176458343863487,
-0.04978838190436363,
-0.05877475067973137,
-0.06289287656545639,
-0.03354167565703392,
-0.03871942684054375,
0.009898529388010502,
-0.05514208599925041,
-0.11629002541303635,
-0.011855563148856163,
0.10663620382547379,
0.037354156374931335,
-0.0065480442717671394,
-0.051189567893743515,
0.06663123518228531,
0.01874656230211258,
0.032841797918081284,
0.041593004018068314,
-0.06879369914531708,
0.04216769337654114,
-0.01628219522535801,
5.4139394340936695e-34,
0.05697013810276985,
-0.006972255185246468,
0.015711724758148193,
-0.17956365644931793,
0.02320219948887825,
0.007923615165054798,
-0.008062449283897877,
0.0074974060989916325,
0.07391711324453354,
0.0309313777834177,
0.060510627925395966,
0.058605875819921494,
0.09515274316072464,
-0.002282935893163085,
0.001603541080839932,
0.07024981826543808,
0.012629246339201927,
0.07425693422555923,
-0.038426291197538376,
0.01861148327589035,
0.030608950182795525,
-0.02449394389986992,
0.021528491750359535,
-0.003039651783183217,
-0.03676343336701393,
0.03130284696817398,
0.07998586446046829,
0.010451192036271095,
-0.07930229604244232,
-0.013543923385441303,
0.018781835213303566,
0.05168003588914871,
-0.07191970944404602,
0.15783067047595978,
0.026191607117652893,
0.01262354850769043,
0.08218053728342056,
-0.029807550832629204,
-0.07528624683618546,
-0.04250097647309303,
0.017244765534996986,
0.04411793500185013,
0.03708017244935036,
0.009233047254383564,
-0.040271829813718796,
0.022496428340673447,
0.02495843544602394,
0.07633638381958008,
0.005147108342498541,
0.013892097398638725,
0.05610476806759834,
-0.06684739887714386,
0.05862557515501976,
-0.020688841119408607,
0.05377643182873726,
0.06718500703573227,
0.005329249892383814,
-0.01388032827526331,
0.029931528493762016,
0.009508464485406876,
-0.045173756778240204,
0.11534366756677628,
-0.06510116159915924,
0.05117698386311531,
-0.0026125339791178703,
-0.08554837852716446,
-0.03784770518541336,
0.0804959163069725,
0.011298024095594883,
-0.07695550471544266,
-0.04868878796696663,
0.02515520341694355,
0.06252261996269226,
-0.04509226232767105,
-0.01246943511068821,
0.028559505939483643,
-0.030573077499866486,
0.05066261067986488,
-0.08187384903430939,
0.04469604790210724,
0.0034051244147121906,
0.04145054519176483,
-0.021858664229512215,
-0.06112268194556236,
-0.00908052921295166,
-0.05903250351548195,
0.0259539932012558,
0.059690944850444794,
-0.07613514363765717,
-0.03720718249678612,
-0.036316655576229095,
0.07058046013116837,
-0.008224100805819035,
0.041961874812841415,
-0.0285952128469944,
-1.496900736697171e-8,
-0.0014124972512945533,
0.03401879221200943,
-0.040338415652513504,
0.04116074740886688,
0.0935964286327362,
-0.05115952715277672,
0.0008746005478315055,
-0.03389839455485344,
-0.00567849725484848,
-0.010686947964131832,
-0.04789939522743225,
-0.04820054769515991,
-0.02011880651116371,
-0.03209094703197479,
-0.04211259260773659,
-0.10229527950286865,
-0.07819421589374542,
-0.031228765845298767,
-0.02154778689146042,
-0.04960230365395546,
0.08087796717882156,
-0.07801242172718048,
0.06919731199741364,
-0.04999840259552002,
0.03687043860554695,
0.03889009356498718,
-0.049989692866802216,
-0.04254625365138054,
-0.04606937617063522,
0.08682432025671005,
-0.031148413196206093,
0.11826753616333008,
0.034102488309144974,
-0.0208592489361763,
-0.0205202866345644,
0.027134142816066742,
0.09741277992725372,
0.051608603447675705,
0.013477512635290623,
-0.13649295270442963,
-0.022304272279143333,
0.02385953813791275,
0.038732077926397324,
-0.09249968826770782,
-0.04549082741141319,
0.054220106452703476,
0.01160438358783722,
0.051190607249736786,
0.07713303714990616,
-0.022097084671258926,
-0.06127818301320076,
-0.01857956498861313,
0.006740490905940533,
-0.00496308971196413,
0.024095389991998672,
0.0736224576830864,
-0.003481915919110179,
-0.0699305310845375,
-0.006629763171076775,
-0.0598808117210865,
0.05297163128852844,
-0.02902800403535366,
-0.027858933433890343,
-0.01287526823580265
] |
stas/tiny-m2m_100 | 4df2a26e27b5f4823e2e797424de47f14c2e1b27 | 2022-04-29T23:57:25.000Z | [
"pytorch",
"m2m_100",
"text2text-generation",
"en",
"transformers",
"testing",
"license:apache-2.0",
"autotrain_compatible"
] | text2text-generation | false | stas | null | stas/tiny-m2m_100 | 2,009 | null | transformers | ---
language:
- en
thumbnail:
tags:
- testing
license: apache-2.0
---
# Tiny M2M100 model
This is a tiny model that is used in the `transformers` test suite. It doesn't do anything useful beyond functional testing.
Do not try to use it for anything that requires quality.
The model is indeed 4MB in size.
You can see how it was created [here](https://huggingface.co/stas/tiny-m2m_100/blob/main/m2m-make-tiny-model.py)
If you're looking for the real model, please go to [https://huggingface.co/facebook/m2m100_418M](https://huggingface.co/facebook/m2m100_418M).
| [
-0.06283226609230042,
0.0664181187748909,
-0.010833976790308952,
0.021445905789732933,
0.074300616979599,
-0.08732432126998901,
-0.1254691779613495,
0.05449266359210014,
-0.107417993247509,
0.0025695424992591143,
0.10799375176429749,
-0.02743741124868393,
0.03798001632094383,
0.00909283384680748,
-0.02114800736308098,
0.053301356732845306,
0.05518873408436775,
-0.017070388421416283,
-0.048467956483364105,
0.001121018547564745,
0.02281026914715767,
0.009327908977866173,
0.014792573638260365,
-0.025211002677679062,
-0.006761318538337946,
-0.007963591255247593,
-0.0027885609306395054,
0.029576200991868973,
0.051274508237838745,
-0.12292520701885223,
0.07471103221178055,
0.11913695931434631,
-0.004292456898838282,
0.02659882977604866,
0.12401683628559113,
0.05669669061899185,
0.025228627026081085,
-0.045839592814445496,
-0.011866191402077675,
-0.0412861704826355,
0.08915621787309647,
-0.021606087684631348,
0.07029861956834793,
-0.09885143488645554,
0.013686789199709892,
-0.0004198556416667998,
0.05257517844438553,
-0.026859372854232788,
-0.02880723774433136,
-0.1109791025519371,
-0.009156184270977974,
-0.06185867637395859,
-0.04119940474629402,
0.03662587329745293,
-0.011056695133447647,
-0.09163836389780045,
-0.014736276119947433,
-0.05204147845506668,
-0.05427452549338341,
0.04126580432057381,
0.001542121870443225,
0.07402181625366211,
-0.02665211632847786,
0.027759946882724762,
0.027969883754849434,
0.06271866708993912,
0.054294388741254807,
-0.0790708139538765,
-0.00582317216321826,
-0.05749642103910446,
0.033642515540122986,
-0.05282297357916832,
0.012135046534240246,
0.07507968693971634,
-0.010353543795645237,
-0.040902551263570786,
0.07133302837610245,
0.05668580159544945,
0.05499635636806488,
0.032625969499349594,
-0.013625449500977993,
-0.0740419328212738,
0.013349964283406734,
-0.0321359820663929,
0.024197358638048172,
0.03123691864311695,
0.04850028455257416,
0.015248182229697704,
-0.0035938022192567587,
-0.06387573480606079,
-0.04950427636504173,
0.015258928760886192,
-0.11238671094179153,
0.07679209113121033,
-0.02791331335902214,
0.006014329381287098,
0.00688217394053936,
0.000802624796051532,
-0.08388719707727432,
0.10650420188903809,
0.07331874221563339,
0.01323260460048914,
0.09623398631811142,
0.026872610673308372,
-0.0026525696739554405,
0.016382206231355667,
0.036280885338783264,
0.07862700521945953,
0.04738108441233635,
0.024681834504008293,
0.042876891791820526,
-0.011220676824450493,
-0.0856483206152916,
-0.0214503463357687,
0.04129654914140701,
-0.10847173631191254,
0.010038974694907665,
-0.023932306095957756,
-0.019765419885516167,
0.01226513460278511,
0.02401013858616352,
0.04107855632901192,
-0.05215372517704964,
-0.09284761548042297,
-0.006584677845239639,
0.09566035866737366,
-0.06102279946208,
1.3392664278307564e-33,
0.059199053794145584,
0.06334033608436584,
0.025201668962836266,
-0.004712214693427086,
0.09236981719732285,
0.03900143504142761,
0.07539112865924835,
0.048981741070747375,
-0.03108026646077633,
-0.01080814003944397,
-0.050742827355861664,
-0.013505592942237854,
-0.038692083209753036,
0.06746503710746765,
-0.05322842299938202,
-0.08000610023736954,
0.0040170736610889435,
-0.023153765127062798,
0.009533480741083622,
0.03716116026043892,
0.006590251810848713,
-0.013024557381868362,
-0.011365590617060661,
-0.04518936574459076,
-0.018396930769085884,
0.01378901768475771,
0.07587756216526031,
-0.04891170561313629,
0.02758961170911789,
0.05542782321572304,
-0.07006170600652695,
0.03683176264166832,
0.00033775405609048903,
-0.07479356229305267,
-0.02419460564851761,
-0.05461650341749191,
-0.10144711285829544,
-0.0744229406118393,
-0.08722642064094543,
-0.11052700877189636,
0.0011599039426073432,
0.013003057800233364,
-0.07372099161148071,
-0.02619396708905697,
-0.08104799687862396,
-0.04182500019669533,
0.0368197076022625,
-0.03324376419186592,
0.035835254937410355,
-0.03758877143263817,
0.027636991813778877,
0.017568202689290047,
-0.04875807464122772,
-0.010314653627574444,
0.011602370999753475,
0.021597011014819145,
0.10429747402667999,
0.0144322095438838,
0.009854668751358986,
-0.03119400516152382,
-0.007418102119117975,
0.011817429214715958,
0.02568710595369339,
0.07951889932155609,
0.1012992262840271,
0.010790395550429821,
0.001885525300167501,
-0.000152128966874443,
0.006064622197300196,
0.008427854627370834,
0.006683467887341976,
-0.04396329075098038,
0.025210319086909294,
0.017529670149087906,
0.04861350357532501,
-0.1143312007188797,
0.054200056940317154,
-0.034950435161590576,
-0.03374166041612625,
0.007204002235084772,
0.019240280613303185,
-0.002289267722517252,
0.06412486732006073,
-0.04413279891014099,
-0.053421974182128906,
-0.0922991931438446,
0.09127645194530487,
-0.04028146341443062,
0.0067418962717056274,
-0.04508903622627258,
0.006334456615149975,
0.04451119154691696,
0.004356019198894501,
-0.058537330478429794,
-0.06296343356370926,
-1.3836911144132154e-33,
0.017559705302119255,
0.05632994323968887,
-0.013710008934140205,
0.1089765727519989,
-0.03473532944917679,
-0.07887095957994461,
0.05705004557967186,
0.1895528882741928,
-0.07790482044219971,
0.01375906728208065,
0.057231493294239044,
-0.02768300473690033,
0.034012556076049805,
-0.10236230492591858,
0.08378855139017105,
-0.025525948032736778,
0.014271494932472706,
-0.1291714310646057,
0.09370208531618118,
0.00229838490486145,
0.021871039643883705,
0.09661778062582016,
0.045532774180173874,
0.011550196446478367,
-0.07473783195018768,
0.0020682034082710743,
-0.001659195520915091,
-0.015599200502038002,
0.003565185470506549,
-0.03347298130393028,
-0.044012390077114105,
0.018357422202825546,
-0.05900413542985916,
0.023557238280773163,
-0.028519684448838234,
-0.03622796759009361,
0.014826291240751743,
0.017353059723973274,
0.05413510650396347,
0.02398361638188362,
0.021088913083076477,
0.031680770218372345,
-0.04140201210975647,
0.019165359437465668,
-0.044431280344724655,
-0.04491276666522026,
0.030220894142985344,
-0.04667352885007858,
0.06952332705259323,
-0.020409129559993744,
0.02433270402252674,
0.01904587261378765,
-0.03082146681845188,
0.024483755230903625,
-0.024788789451122284,
-0.04921523109078407,
-0.05304516851902008,
0.00536351790651679,
0.008862863294780254,
-0.006956224329769611,
-0.03296231850981712,
-0.07893659919500351,
-0.09188707172870636,
-0.04807678610086441,
-0.0067348056472837925,
0.019513355568051338,
-0.04998160898685455,
-0.03759758919477463,
-0.001170401694253087,
0.06837120652198792,
0.05552159622311592,
0.038434673100709915,
0.06374293565750122,
0.04153713956475258,
0.01619899272918701,
-0.05260901525616646,
-0.05453427881002426,
-0.005800427868962288,
0.10742687433958054,
-0.066475048661232,
-0.010827675461769104,
0.011448092758655548,
-0.024222616106271744,
-0.010976153425872326,
0.026544999331235886,
-0.04769335314631462,
0.0007540728547610343,
0.09396103024482727,
-0.022106247022747993,
0.03763182461261749,
-0.029252270236611366,
0.09097245335578918,
-0.017063841223716736,
0.05002850666642189,
0.02609165571630001,
-5.0828830922000634e-8,
0.02100585773587227,
0.007908309809863567,
-0.0056144725531339645,
-0.008540989831089973,
-0.07867763191461563,
-0.033997971564531326,
0.023413142189383507,
-0.0065374793484807014,
0.06839805096387863,
0.06158558651804924,
0.014648745767772198,
0.001989237265661359,
-0.1249287948012352,
0.07050637900829315,
-0.0193564984947443,
-0.009344102814793587,
-0.029442088678479195,
0.03172741085290909,
-0.046107497066259384,
-0.028810817748308182,
-0.010824453085660934,
0.024740474298596382,
0.02649099752306938,
-0.04499317333102226,
0.04009188711643219,
0.03608981519937515,
-0.0037148380652070045,
0.08941172063350677,
-0.0717809647321701,
-0.05533640459179878,
-0.006608814001083374,
0.051396433264017105,
-0.052623748779296875,
-0.05823181942105293,
-0.03028411976993084,
0.07011653482913971,
-0.0379040353000164,
0.032862115651369095,
0.05073193460702896,
-0.01747780479490757,
0.04519009217619896,
-0.02131834253668785,
-0.03875080496072769,
-0.006876983679831028,
0.0065108719281852245,
-0.04758140817284584,
-0.017487362027168274,
-0.081653892993927,
-0.059266217052936554,
0.02498715929687023,
0.044513504952192307,
-0.020885329693555832,
-0.056648433208465576,
0.020327575504779816,
-0.06233281269669533,
-0.017669452354311943,
0.036560721695423126,
-0.02269653230905533,
-0.0028188819997012615,
0.05325343459844589,
0.08465182781219482,
0.02954411692917347,
0.004947321489453316,
0.02688022330403328
] |
hf-internal-testing/tiny-random-mpnet | 490e676cf9e1714ddd21f9169dc14652e9a9e7f4 | 2021-09-17T19:25:01.000Z | [
"pytorch",
"tf",
"mpnet",
"transformers"
] | null | false | hf-internal-testing | null | hf-internal-testing/tiny-random-mpnet | 2,008 | null | transformers | Entry not found | [
0.0461147278547287,
-0.038838207721710205,
-0.01049656979739666,
-0.03682169318199158,
0.011261860840022564,
0.013094935566186905,
0.0019101888174191117,
-0.013979103416204453,
0.027092741802334785,
-0.015212527476251125,
0.017284274101257324,
-0.08189476281404495,
0.03817418962717056,
-0.04920130595564842,
0.021389011293649673,
-0.015245908871293068,
-0.03203780576586723,
-0.1245758980512619,
0.03150877356529236,
0.032381657510995865,
-0.060957908630371094,
0.05409295856952667,
-0.025087490677833557,
0.01568586938083172,
0.028129950165748596,
-0.04710396006703377,
-0.018688226118683815,
0.013785239309072495,
-0.04001208767294884,
0.01173911802470684,
-0.04317743331193924,
0.05500618368387222,
0.004543041344732046,
0.02973111905157566,
0.14852192997932434,
0.02658126689493656,
0.02907961793243885,
-0.05169107764959335,
0.05803573504090309,
-0.07732241600751877,
-0.017637968063354492,
-0.04219653457403183,
0.041807834059000015,
0.023620979860424995,
0.021563321352005005,
0.016478516161441803,
-0.0021814992651343346,
-0.06400240957736969,
0.06393089145421982,
0.019599027931690216,
-0.08565037697553635,
0.00934905931353569,
-0.008718925528228283,
-0.028583496809005737,
-0.07310017943382263,
0.09416428208351135,
0.001759322709403932,
0.06184990331530571,
0.011840506456792355,
-0.035997264087200165,
0.08358278125524521,
-0.02619801089167595,
0.03736566752195358,
-0.028206506744027138,
-0.07454850524663925,
-0.08883563429117203,
-0.06279942393302917,
-0.008695344440639019,
0.014119276776909828,
-0.0825355276465416,
0.0649217739701271,
-0.00223911227658391,
-0.14716917276382446,
0.07743025571107864,
-0.03548373281955719,
-0.055201586335897446,
0.006981803569942713,
-0.012166670523583889,
0.055111464112997055,
-0.007116836030036211,
-0.023175746202468872,
-0.005835152696818113,
-0.09185640513896942,
0.055196937173604965,
0.034148022532463074,
0.03835180774331093,
0.038685429841279984,
-0.025987252593040466,
0.017804903909564018,
0.022428328171372414,
0.025005368515849113,
-0.10761535167694092,
-0.048001550137996674,
-0.04343584179878235,
0.012374646961688995,
-0.019502125680446625,
0.029218152165412903,
0.0842173621058464,
-0.011719699949026108,
0.09283553808927536,
-0.007015465293079615,
-0.03543110564351082,
-0.06936459988355637,
0.09425332397222519,
-0.010958523489534855,
-0.00805904995650053,
0.004974212497472763,
-0.0031528924591839314,
0.06105927750468254,
-0.03964288905262947,
-0.03619541600346565,
-0.019901901483535767,
0.07134733349084854,
0.039514873176813126,
-0.012729483656585217,
-0.006646515801548958,
-0.04746140539646149,
-0.014432490803301334,
-0.05157482624053955,
0.09506245702505112,
-0.049747664481401443,
-0.04591796174645424,
-0.008965466171503067,
-0.0325421579182148,
-0.08626784384250641,
-0.06624380499124527,
0.02538885548710823,
-4.303924894057984e-33,
0.01133066974580288,
0.0033434738870710135,
-0.002155609894543886,
0.04871906340122223,
-0.023564351722598076,
-0.07933273911476135,
0.0600903145968914,
0.02335330657660961,
-0.03844716399908066,
-0.020433755591511726,
-0.06952055543661118,
-0.03235611692070961,
0.0062485747039318085,
0.064804308116436,
-0.03201229125261307,
0.061689723283052444,
0.0417000837624073,
-0.00761845987290144,
0.03340127319097519,
-0.047770582139492035,
0.00887306872755289,
-0.04066338762640953,
-0.010506896302103996,
0.0106519665569067,
0.021333497017621994,
0.12854498624801636,
-0.009705503471195698,
0.010055632330477238,
-0.017507633194327354,
0.006515394430607557,
0.06334009766578674,
-0.057817306369543076,
0.013668818399310112,
-0.020286159589886665,
0.05430467426776886,
-0.023184705525636673,
0.0828516036272049,
0.0005449643940664828,
-0.10372652113437653,
-0.07634282112121582,
-0.005381610710173845,
-0.039263784885406494,
0.0006114727002568543,
-0.013281986117362976,
0.07119110971689224,
0.043696220964193344,
0.03168422728776932,
0.04338686540722847,
0.05728672817349434,
0.0832006186246872,
-0.07961414009332657,
0.015234283171594143,
0.017002005130052567,
0.047004107385873795,
-0.09794387966394424,
0.004990279674530029,
-0.07062993198633194,
-0.028000490739941597,
-0.04018733277916908,
-0.0702052190899849,
0.011351344175636768,
0.06020182743668556,
-0.03297270089387894,
0.09396500885486603,
0.03417910635471344,
-0.019825750961899757,
-0.034690454602241516,
-0.013036907650530338,
0.05896938592195511,
-0.012359356507658958,
-0.017275206744670868,
-0.07982361316680908,
0.02059139870107174,
0.06737419217824936,
0.04176458343863487,
-0.04978838190436363,
-0.05877475067973137,
-0.06289287656545639,
-0.03354167565703392,
-0.03871942684054375,
0.009898529388010502,
-0.05514208599925041,
-0.11629002541303635,
-0.011855563148856163,
0.10663620382547379,
0.037354156374931335,
-0.0065480442717671394,
-0.051189567893743515,
0.06663123518228531,
0.01874656230211258,
0.032841797918081284,
0.041593004018068314,
-0.06879369914531708,
0.04216769337654114,
-0.01628219522535801,
5.4139394340936695e-34,
0.05697013810276985,
-0.006972255185246468,
0.015711724758148193,
-0.17956365644931793,
0.02320219948887825,
0.007923615165054798,
-0.008062449283897877,
0.0074974060989916325,
0.07391711324453354,
0.0309313777834177,
0.060510627925395966,
0.058605875819921494,
0.09515274316072464,
-0.002282935893163085,
0.001603541080839932,
0.07024981826543808,
0.012629246339201927,
0.07425693422555923,
-0.038426291197538376,
0.01861148327589035,
0.030608950182795525,
-0.02449394389986992,
0.021528491750359535,
-0.003039651783183217,
-0.03676343336701393,
0.03130284696817398,
0.07998586446046829,
0.010451192036271095,
-0.07930229604244232,
-0.013543923385441303,
0.018781835213303566,
0.05168003588914871,
-0.07191970944404602,
0.15783067047595978,
0.026191607117652893,
0.01262354850769043,
0.08218053728342056,
-0.029807550832629204,
-0.07528624683618546,
-0.04250097647309303,
0.017244765534996986,
0.04411793500185013,
0.03708017244935036,
0.009233047254383564,
-0.040271829813718796,
0.022496428340673447,
0.02495843544602394,
0.07633638381958008,
0.005147108342498541,
0.013892097398638725,
0.05610476806759834,
-0.06684739887714386,
0.05862557515501976,
-0.020688841119408607,
0.05377643182873726,
0.06718500703573227,
0.005329249892383814,
-0.01388032827526331,
0.029931528493762016,
0.009508464485406876,
-0.045173756778240204,
0.11534366756677628,
-0.06510116159915924,
0.05117698386311531,
-0.0026125339791178703,
-0.08554837852716446,
-0.03784770518541336,
0.0804959163069725,
0.011298024095594883,
-0.07695550471544266,
-0.04868878796696663,
0.02515520341694355,
0.06252261996269226,
-0.04509226232767105,
-0.01246943511068821,
0.028559505939483643,
-0.030573077499866486,
0.05066261067986488,
-0.08187384903430939,
0.04469604790210724,
0.0034051244147121906,
0.04145054519176483,
-0.021858664229512215,
-0.06112268194556236,
-0.00908052921295166,
-0.05903250351548195,
0.0259539932012558,
0.059690944850444794,
-0.07613514363765717,
-0.03720718249678612,
-0.036316655576229095,
0.07058046013116837,
-0.008224100805819035,
0.041961874812841415,
-0.0285952128469944,
-1.496900736697171e-8,
-0.0014124972512945533,
0.03401879221200943,
-0.040338415652513504,
0.04116074740886688,
0.0935964286327362,
-0.05115952715277672,
0.0008746005478315055,
-0.03389839455485344,
-0.00567849725484848,
-0.010686947964131832,
-0.04789939522743225,
-0.04820054769515991,
-0.02011880651116371,
-0.03209094703197479,
-0.04211259260773659,
-0.10229527950286865,
-0.07819421589374542,
-0.031228765845298767,
-0.02154778689146042,
-0.04960230365395546,
0.08087796717882156,
-0.07801242172718048,
0.06919731199741364,
-0.04999840259552002,
0.03687043860554695,
0.03889009356498718,
-0.049989692866802216,
-0.04254625365138054,
-0.04606937617063522,
0.08682432025671005,
-0.031148413196206093,
0.11826753616333008,
0.034102488309144974,
-0.0208592489361763,
-0.0205202866345644,
0.027134142816066742,
0.09741277992725372,
0.051608603447675705,
0.013477512635290623,
-0.13649295270442963,
-0.022304272279143333,
0.02385953813791275,
0.038732077926397324,
-0.09249968826770782,
-0.04549082741141319,
0.054220106452703476,
0.01160438358783722,
0.051190607249736786,
0.07713303714990616,
-0.022097084671258926,
-0.06127818301320076,
-0.01857956498861313,
0.006740490905940533,
-0.00496308971196413,
0.024095389991998672,
0.0736224576830864,
-0.003481915919110179,
-0.0699305310845375,
-0.006629763171076775,
-0.0598808117210865,
0.05297163128852844,
-0.02902800403535366,
-0.027858933433890343,
-0.01287526823580265
] |
hf-internal-testing/tiny-electra | 7479c5defabc4a550d08c170f7f4fb0b0e6be19b | 2021-07-16T01:27:58.000Z | [
"pytorch",
"electra",
"fill-mask",
"transformers",
"autotrain_compatible"
] | fill-mask | false | hf-internal-testing | null | hf-internal-testing/tiny-electra | 2,005 | null | transformers | This is a tiny-electra random model to be used for basic testing.
| [
-0.03501860052347183,
0.03153549134731293,
-0.07524871081113815,
0.061836693435907364,
0.04573119431734085,
-0.08133674412965775,
0.01244229357689619,
0.011787359602749348,
0.0000867993658175692,
0.05535188317298889,
0.0648735910654068,
-0.010716596618294716,
0.015677087008953094,
0.0010169469751417637,
-0.11600378900766373,
-0.02944428287446499,
0.032986804842948914,
-0.04179530218243599,
-0.020813466981053352,
0.035072244703769684,
0.03754914551973343,
0.003755660494789481,
-0.023382490500807762,
-0.031548600643873215,
-0.03275161609053612,
-0.0821942612528801,
0.03119158372282982,
0.0922740176320076,
0.029894711449742317,
-0.12236928194761276,
0.09551212191581726,
0.033652689307928085,
0.05415322631597519,
-0.014422748237848282,
0.00711292726919055,
-0.025845583528280258,
-0.022444281727075577,
-0.031825002282857895,
-0.0339006669819355,
0.05637427419424057,
0.011470010504126549,
-0.0834401473402977,
0.07669080793857574,
0.0229780375957489,
0.047452282160520554,
-0.04337143525481224,
-0.05702045559883118,
-0.052229464054107666,
-0.01305905357003212,
-0.018892357125878334,
0.036789409816265106,
-0.060668110847473145,
-0.08380342274904251,
0.007317143492400646,
0.03470602631568909,
-0.08473135530948639,
-0.015115339308977127,
-0.11787135154008865,
-0.008481397293508053,
0.0038700809236615896,
0.06847703456878662,
0.005699884612113237,
-0.09122633188962936,
0.060603730380535126,
0.04611140117049217,
0.011871139518916607,
-0.004667986650019884,
-0.03157634288072586,
0.05678008869290352,
-0.14379917085170746,
-0.07456054538488388,
-0.013459380716085434,
-0.0016644794959574938,
0.09085343778133392,
0.03091975674033165,
0.0033727807458490133,
-0.01118948683142662,
-0.03456279635429382,
0.02697504684329033,
0.07853702455759048,
-0.12686949968338013,
-0.06961346417665482,
-0.04684227332472801,
0.004264296032488346,
0.0032340586185455322,
0.06848103553056717,
0.09962970018386841,
0.002053268486633897,
0.030131282284855843,
-0.02342185191810131,
-0.008578390814363956,
0.09232437610626221,
-0.03754830360412598,
0.002181800315156579,
-0.03668593615293503,
0.03686477616429329,
0.03233599290251732,
-0.07245977967977524,
0.012863974086940289,
0.08163013309240341,
0.05597630888223648,
0.022554943338036537,
0.06623609364032745,
0.014026825316250324,
-0.08083142340183258,
-0.10964877903461456,
0.09370870888233185,
-0.04606081172823906,
0.03744938597083092,
-0.02268959768116474,
-0.02129235677421093,
0.021607305854558945,
-0.026906874030828476,
0.01744704134762287,
0.006616718601435423,
-0.04375883564352989,
-0.08574134111404419,
0.026438619941473007,
-0.01650124415755272,
0.06783618777990341,
0.05668003857135773,
0.02004944533109665,
-0.07230407744646072,
-0.05165386572480202,
0.003728253301233053,
0.05558684095740318,
-0.011670702137053013,
-2.8654818241773586e-33,
0.04865003377199173,
0.00805780477821827,
0.013645470142364502,
0.06254998594522476,
0.08734729886054993,
0.055995240807533264,
-0.010398667305707932,
0.03755765035748482,
0.0019412949914112687,
0.06594132632017136,
-0.05389416217803955,
0.06683453172445297,
-0.008523142896592617,
0.06970030814409256,
-0.006980265490710735,
-0.024099698290228844,
-0.06046171858906746,
0.046888597309589386,
-0.0038516023196280003,
0.030485263094305992,
0.02561798319220543,
-0.002734073204919696,
0.031476590782403946,
-0.07620441913604736,
0.06225012242794037,
0.03797101974487305,
0.031189320608973503,
-0.012884828262031078,
-0.0028074586298316717,
0.057072870433330536,
-0.02816598117351532,
0.06433863192796707,
-0.10286534577608109,
0.0008293476421386003,
-0.0324653759598732,
0.036598626524209976,
-0.007415255531668663,
-0.032436590641736984,
-0.02619699016213417,
-0.030888373032212257,
0.02771337889134884,
-0.027994658797979355,
0.051649078726768494,
0.010675666853785515,
-0.031162617728114128,
-0.01500824373215437,
0.02363884449005127,
-0.0004651751078199595,
0.07766719907522202,
-0.0031495762523263693,
-0.009341913275420666,
-0.027063563466072083,
0.016402214765548706,
-0.006138352211564779,
-0.00981680117547512,
0.13235649466514587,
0.010260005481541157,
0.018268026411533356,
-0.030467649921774864,
0.05793016403913498,
-0.065088652074337,
0.05919535085558891,
-0.025060482323169708,
0.01756203919649124,
0.014438540674746037,
0.08027365058660507,
-0.0703921765089035,
-0.14495833218097687,
0.08370603621006012,
0.031506218016147614,
0.01973767764866352,
-0.013205558061599731,
0.025084389373660088,
0.0036139588337391615,
-0.014248854480683804,
-0.03035976178944111,
0.021151449531316757,
0.021618051454424858,
-0.001966361654922366,
-0.041312411427497864,
-0.0033462238498032093,
-0.07080540806055069,
-0.0031057647429406643,
-0.09211941063404083,
-0.1082182452082634,
-0.031115448102355003,
-0.01923321560025215,
-0.00958364736288786,
-0.0977439358830452,
-0.06090417876839638,
0.03809957206249237,
0.021286122500896454,
-0.0009663496748544276,
-0.032695669680833817,
0.019184768199920654,
7.910512192732623e-34,
-0.09593638777732849,
0.0010716773103922606,
0.019865551963448524,
0.11153340339660645,
0.08850078284740448,
-0.0380234532058239,
0.0028388455975800753,
0.041533321142196655,
-0.0040226406417787075,
0.06094569340348244,
0.03571508452296257,
0.03374040126800537,
0.08053933829069138,
-0.02841844968497753,
0.0883592963218689,
0.042385444045066833,
-0.025071484968066216,
-0.10110513865947723,
0.05236368253827095,
0.027200566604733467,
-0.004438404459506273,
0.12439672648906708,
-0.057824961841106415,
-0.05673102289438248,
-0.0022600842639803886,
0.01868012174963951,
-0.04168166220188141,
-0.03796660527586937,
0.000532798352651298,
-0.02214009314775467,
-0.11942470073699951,
-0.01525757648050785,
0.004492557607591152,
0.03126073256134987,
-0.049420759081840515,
-0.05636783689260483,
0.10949664562940598,
-0.04572497680783272,
0.04329827427864075,
-0.04359675571322441,
0.018544888123869896,
0.06400059163570404,
0.03068201057612896,
-0.012923983857035637,
0.013140806928277016,
0.029094582423567772,
0.023511936888098717,
0.021939830854535103,
0.05818270146846771,
0.02105584554374218,
0.0025240725371986628,
-0.017909441143274307,
-0.07140558958053589,
0.02648693136870861,
-0.04474623501300812,
0.004766231868416071,
-0.08136669546365738,
0.04149813577532768,
-0.013925251550972462,
0.08774853497743607,
-0.02241567149758339,
-0.036991801112890244,
0.007182118482887745,
0.02532920427620411,
-0.05711476877331734,
-0.014616968110203743,
0.009030311368405819,
-0.01974649354815483,
-0.010069403797388077,
0.0634203851222992,
0.10742273181676865,
0.05946098268032074,
0.024006934836506844,
-0.05286959558725357,
-0.027047453448176384,
-0.14568325877189636,
-0.0560046024620533,
-0.04245482012629509,
-0.009987901896238327,
-0.036849670112133026,
-0.008059894666075706,
-0.004800619091838598,
-0.002698146039620042,
-0.014395286329090595,
0.012324294075369835,
-0.05255004018545151,
0.02130402810871601,
0.01890440471470356,
0.0008472225163131952,
0.07678169012069702,
-0.055556558072566986,
0.11005036532878876,
0.03851092979311943,
0.010280878283083439,
-0.05878547951579094,
-2.0255185972928302e-8,
0.07875022292137146,
-0.050510477274656296,
0.06596582382917404,
0.027321424335241318,
-0.02242131344974041,
0.018923914059996605,
0.002030714647844434,
-0.06027798727154732,
-0.005281882360577583,
-0.07372169196605682,
0.08362428843975067,
0.032573260366916656,
-0.01888127624988556,
0.021774694323539734,
0.0368863046169281,
0.006180077791213989,
-0.0006208158447407186,
0.05847739428281784,
-0.050299305468797684,
-0.0022149747237563133,
-0.0033360281959176064,
0.05523398518562317,
0.04660116136074066,
-0.008632712066173553,
0.03210264816880226,
0.007703233044594526,
-0.007085510063916445,
0.08409503847360611,
0.025116903707385063,
-0.09610365331172943,
-0.011902212165296078,
0.04752753674983978,
0.0006832325598224998,
-0.002582396613433957,
-0.061617154628038406,
0.16438962519168854,
-0.032022155821323395,
-0.02767755091190338,
0.023361727595329285,
-0.006418323144316673,
-0.0047886427491903305,
-0.10417301952838898,
-0.004629411268979311,
-0.0008276287699118257,
-0.04745645076036453,
-0.02894662693142891,
-0.05122434347867966,
-0.16332796216011047,
0.019556090235710144,
-0.017618723213672638,
0.002849276876077056,
-0.010065109468996525,
-0.04939025267958641,
-0.03617147356271744,
-0.034582048654556274,
0.07857736945152283,
-0.012623089365661144,
-0.015048986300826073,
-0.006621593609452248,
-0.009720888920128345,
0.051579054445028305,
0.054997142404317856,
-0.03193158283829689,
-0.01751052401959896
] |
hf-internal-testing/tiny-random-funnel | ec246a681806cada4b3c073569afba96f7ac8eb8 | 2021-09-17T19:25:04.000Z | [
"pytorch",
"tf",
"funnel",
"transformers"
] | null | false | hf-internal-testing | null | hf-internal-testing/tiny-random-funnel | 2,002 | null | transformers | Entry not found | [
0.0461147278547287,
-0.038838207721710205,
-0.01049656979739666,
-0.03682169318199158,
0.011261860840022564,
0.013094935566186905,
0.0019101888174191117,
-0.013979103416204453,
0.027092741802334785,
-0.015212527476251125,
0.017284274101257324,
-0.08189476281404495,
0.03817418962717056,
-0.04920130595564842,
0.021389011293649673,
-0.015245908871293068,
-0.03203780576586723,
-0.1245758980512619,
0.03150877356529236,
0.032381657510995865,
-0.060957908630371094,
0.05409295856952667,
-0.025087490677833557,
0.01568586938083172,
0.028129950165748596,
-0.04710396006703377,
-0.018688226118683815,
0.013785239309072495,
-0.04001208767294884,
0.01173911802470684,
-0.04317743331193924,
0.05500618368387222,
0.004543041344732046,
0.02973111905157566,
0.14852192997932434,
0.02658126689493656,
0.02907961793243885,
-0.05169107764959335,
0.05803573504090309,
-0.07732241600751877,
-0.017637968063354492,
-0.04219653457403183,
0.041807834059000015,
0.023620979860424995,
0.021563321352005005,
0.016478516161441803,
-0.0021814992651343346,
-0.06400240957736969,
0.06393089145421982,
0.019599027931690216,
-0.08565037697553635,
0.00934905931353569,
-0.008718925528228283,
-0.028583496809005737,
-0.07310017943382263,
0.09416428208351135,
0.001759322709403932,
0.06184990331530571,
0.011840506456792355,
-0.035997264087200165,
0.08358278125524521,
-0.02619801089167595,
0.03736566752195358,
-0.028206506744027138,
-0.07454850524663925,
-0.08883563429117203,
-0.06279942393302917,
-0.008695344440639019,
0.014119276776909828,
-0.0825355276465416,
0.0649217739701271,
-0.00223911227658391,
-0.14716917276382446,
0.07743025571107864,
-0.03548373281955719,
-0.055201586335897446,
0.006981803569942713,
-0.012166670523583889,
0.055111464112997055,
-0.007116836030036211,
-0.023175746202468872,
-0.005835152696818113,
-0.09185640513896942,
0.055196937173604965,
0.034148022532463074,
0.03835180774331093,
0.038685429841279984,
-0.025987252593040466,
0.017804903909564018,
0.022428328171372414,
0.025005368515849113,
-0.10761535167694092,
-0.048001550137996674,
-0.04343584179878235,
0.012374646961688995,
-0.019502125680446625,
0.029218152165412903,
0.0842173621058464,
-0.011719699949026108,
0.09283553808927536,
-0.007015465293079615,
-0.03543110564351082,
-0.06936459988355637,
0.09425332397222519,
-0.010958523489534855,
-0.00805904995650053,
0.004974212497472763,
-0.0031528924591839314,
0.06105927750468254,
-0.03964288905262947,
-0.03619541600346565,
-0.019901901483535767,
0.07134733349084854,
0.039514873176813126,
-0.012729483656585217,
-0.006646515801548958,
-0.04746140539646149,
-0.014432490803301334,
-0.05157482624053955,
0.09506245702505112,
-0.049747664481401443,
-0.04591796174645424,
-0.008965466171503067,
-0.0325421579182148,
-0.08626784384250641,
-0.06624380499124527,
0.02538885548710823,
-4.303924894057984e-33,
0.01133066974580288,
0.0033434738870710135,
-0.002155609894543886,
0.04871906340122223,
-0.023564351722598076,
-0.07933273911476135,
0.0600903145968914,
0.02335330657660961,
-0.03844716399908066,
-0.020433755591511726,
-0.06952055543661118,
-0.03235611692070961,
0.0062485747039318085,
0.064804308116436,
-0.03201229125261307,
0.061689723283052444,
0.0417000837624073,
-0.00761845987290144,
0.03340127319097519,
-0.047770582139492035,
0.00887306872755289,
-0.04066338762640953,
-0.010506896302103996,
0.0106519665569067,
0.021333497017621994,
0.12854498624801636,
-0.009705503471195698,
0.010055632330477238,
-0.017507633194327354,
0.006515394430607557,
0.06334009766578674,
-0.057817306369543076,
0.013668818399310112,
-0.020286159589886665,
0.05430467426776886,
-0.023184705525636673,
0.0828516036272049,
0.0005449643940664828,
-0.10372652113437653,
-0.07634282112121582,
-0.005381610710173845,
-0.039263784885406494,
0.0006114727002568543,
-0.013281986117362976,
0.07119110971689224,
0.043696220964193344,
0.03168422728776932,
0.04338686540722847,
0.05728672817349434,
0.0832006186246872,
-0.07961414009332657,
0.015234283171594143,
0.017002005130052567,
0.047004107385873795,
-0.09794387966394424,
0.004990279674530029,
-0.07062993198633194,
-0.028000490739941597,
-0.04018733277916908,
-0.0702052190899849,
0.011351344175636768,
0.06020182743668556,
-0.03297270089387894,
0.09396500885486603,
0.03417910635471344,
-0.019825750961899757,
-0.034690454602241516,
-0.013036907650530338,
0.05896938592195511,
-0.012359356507658958,
-0.017275206744670868,
-0.07982361316680908,
0.02059139870107174,
0.06737419217824936,
0.04176458343863487,
-0.04978838190436363,
-0.05877475067973137,
-0.06289287656545639,
-0.03354167565703392,
-0.03871942684054375,
0.009898529388010502,
-0.05514208599925041,
-0.11629002541303635,
-0.011855563148856163,
0.10663620382547379,
0.037354156374931335,
-0.0065480442717671394,
-0.051189567893743515,
0.06663123518228531,
0.01874656230211258,
0.032841797918081284,
0.041593004018068314,
-0.06879369914531708,
0.04216769337654114,
-0.01628219522535801,
5.4139394340936695e-34,
0.05697013810276985,
-0.006972255185246468,
0.015711724758148193,
-0.17956365644931793,
0.02320219948887825,
0.007923615165054798,
-0.008062449283897877,
0.0074974060989916325,
0.07391711324453354,
0.0309313777834177,
0.060510627925395966,
0.058605875819921494,
0.09515274316072464,
-0.002282935893163085,
0.001603541080839932,
0.07024981826543808,
0.012629246339201927,
0.07425693422555923,
-0.038426291197538376,
0.01861148327589035,
0.030608950182795525,
-0.02449394389986992,
0.021528491750359535,
-0.003039651783183217,
-0.03676343336701393,
0.03130284696817398,
0.07998586446046829,
0.010451192036271095,
-0.07930229604244232,
-0.013543923385441303,
0.018781835213303566,
0.05168003588914871,
-0.07191970944404602,
0.15783067047595978,
0.026191607117652893,
0.01262354850769043,
0.08218053728342056,
-0.029807550832629204,
-0.07528624683618546,
-0.04250097647309303,
0.017244765534996986,
0.04411793500185013,
0.03708017244935036,
0.009233047254383564,
-0.040271829813718796,
0.022496428340673447,
0.02495843544602394,
0.07633638381958008,
0.005147108342498541,
0.013892097398638725,
0.05610476806759834,
-0.06684739887714386,
0.05862557515501976,
-0.020688841119408607,
0.05377643182873726,
0.06718500703573227,
0.005329249892383814,
-0.01388032827526331,
0.029931528493762016,
0.009508464485406876,
-0.045173756778240204,
0.11534366756677628,
-0.06510116159915924,
0.05117698386311531,
-0.0026125339791178703,
-0.08554837852716446,
-0.03784770518541336,
0.0804959163069725,
0.011298024095594883,
-0.07695550471544266,
-0.04868878796696663,
0.02515520341694355,
0.06252261996269226,
-0.04509226232767105,
-0.01246943511068821,
0.028559505939483643,
-0.030573077499866486,
0.05066261067986488,
-0.08187384903430939,
0.04469604790210724,
0.0034051244147121906,
0.04145054519176483,
-0.021858664229512215,
-0.06112268194556236,
-0.00908052921295166,
-0.05903250351548195,
0.0259539932012558,
0.059690944850444794,
-0.07613514363765717,
-0.03720718249678612,
-0.036316655576229095,
0.07058046013116837,
-0.008224100805819035,
0.041961874812841415,
-0.0285952128469944,
-1.496900736697171e-8,
-0.0014124972512945533,
0.03401879221200943,
-0.040338415652513504,
0.04116074740886688,
0.0935964286327362,
-0.05115952715277672,
0.0008746005478315055,
-0.03389839455485344,
-0.00567849725484848,
-0.010686947964131832,
-0.04789939522743225,
-0.04820054769515991,
-0.02011880651116371,
-0.03209094703197479,
-0.04211259260773659,
-0.10229527950286865,
-0.07819421589374542,
-0.031228765845298767,
-0.02154778689146042,
-0.04960230365395546,
0.08087796717882156,
-0.07801242172718048,
0.06919731199741364,
-0.04999840259552002,
0.03687043860554695,
0.03889009356498718,
-0.049989692866802216,
-0.04254625365138054,
-0.04606937617063522,
0.08682432025671005,
-0.031148413196206093,
0.11826753616333008,
0.034102488309144974,
-0.0208592489361763,
-0.0205202866345644,
0.027134142816066742,
0.09741277992725372,
0.051608603447675705,
0.013477512635290623,
-0.13649295270442963,
-0.022304272279143333,
0.02385953813791275,
0.038732077926397324,
-0.09249968826770782,
-0.04549082741141319,
0.054220106452703476,
0.01160438358783722,
0.051190607249736786,
0.07713303714990616,
-0.022097084671258926,
-0.06127818301320076,
-0.01857956498861313,
0.006740490905940533,
-0.00496308971196413,
0.024095389991998672,
0.0736224576830864,
-0.003481915919110179,
-0.0699305310845375,
-0.006629763171076775,
-0.0598808117210865,
0.05297163128852844,
-0.02902800403535366,
-0.027858933433890343,
-0.01287526823580265
] |
Helsinki-NLP/opus-mt-en-sv | 13d9f7f708dd86e1edf61f0cd438298267b83850 | 2021-09-09T21:39:27.000Z | [
"pytorch",
"rust",
"marian",
"text2text-generation",
"en",
"sv",
"transformers",
"translation",
"license:apache-2.0",
"autotrain_compatible"
] | translation | false | Helsinki-NLP | null | Helsinki-NLP/opus-mt-en-sv | 1,998 | 1 | transformers | ---
tags:
- translation
license: apache-2.0
---
### opus-mt-en-sv
* source languages: en
* target languages: sv
* OPUS readme: [en-sv](https://github.com/Helsinki-NLP/OPUS-MT-train/blob/master/models/en-sv/README.md)
* dataset: opus
* model: transformer-align
* pre-processing: normalization + SentencePiece
* download original weights: [opus-2020-02-26.zip](https://object.pouta.csc.fi/OPUS-MT-models/en-sv/opus-2020-02-26.zip)
* test set translations: [opus-2020-02-26.test.txt](https://object.pouta.csc.fi/OPUS-MT-models/en-sv/opus-2020-02-26.test.txt)
* test set scores: [opus-2020-02-26.eval.txt](https://object.pouta.csc.fi/OPUS-MT-models/en-sv/opus-2020-02-26.eval.txt)
## Benchmarks
| testset | BLEU | chr-F |
|-----------------------|-------|-------|
| Tatoeba.en.sv | 60.1 | 0.736 |
| [
-0.05733996257185936,
-0.026217835023999214,
0.018503934144973755,
-0.012865296564996243,
0.0037398573476821184,
0.08888401836156845,
-0.06012595444917679,
0.030412517488002777,
0.02650635875761509,
-0.011737650260329247,
0.00891454890370369,
-0.04083113372325897,
-0.08312101662158966,
-0.029162190854549408,
-0.033798396587371826,
-0.0075004249811172485,
-0.034235820174217224,
0.08051542192697525,
-0.06830327212810516,
-0.01909932680428028,
0.03672266751527786,
0.028705336153507233,
0.030521303415298462,
-0.012708690017461777,
0.11048060655593872,
0.08588480204343796,
-0.10123922675848007,
-0.008989302441477776,
0.09408511966466904,
-0.0441548153758049,
-0.007414121180772781,
-0.0011271584080532193,
0.059314992278814316,
0.07993704825639725,
0.03926882520318031,
0.07574246823787689,
-0.011569298803806305,
-0.06934447586536407,
-0.03505153954029083,
0.05365534499287605,
0.03827643766999245,
0.053413134068250656,
-0.04589304327964783,
-0.006094362586736679,
0.0435447171330452,
0.020542053505778313,
-0.08648787438869476,
0.016512690111994743,
0.014723648317158222,
0.0010612531332299113,
-0.11947041749954224,
-0.017031831666827202,
0.023840727284550667,
0.07174751907587051,
-0.07941726595163345,
0.035376306623220444,
0.055552005767822266,
-0.0035458458587527275,
0.0680321678519249,
-0.022282611578702927,
-0.1248168870806694,
-0.03534495830535889,
-0.09759995341300964,
-0.000018266251572640613,
-0.010307385586202145,
-0.019212273880839348,
0.01501528825610876,
0.06096666306257248,
-0.06540637463331223,
0.05790381878614426,
-0.01192146260291338,
-0.00594476331025362,
0.003884296864271164,
0.05981786921620369,
-0.01377585157752037,
0.039596572518348694,
0.008215396665036678,
-0.0694739818572998,
0.0009614856098778546,
-0.08041852712631226,
0.008734402246773243,
-0.056914471089839935,
0.05703411251306534,
0.0008102222927846014,
0.07774956524372101,
0.011935974471271038,
0.018842114135622978,
-0.001183255109935999,
-0.014891121536493301,
0.04628335312008858,
-0.07083084434270859,
-0.043075576424598694,
-0.0004544729890767485,
0.012397653423249722,
0.0060692597180604935,
0.0572156198322773,
0.01599133014678955,
0.06438834965229034,
0.02042330615222454,
0.06253992766141891,
0.026800910010933876,
0.010824681259691715,
0.06914796680212021,
-0.04337283596396446,
-0.10537111014127731,
-0.025934353470802307,
0.06140593811869621,
0.04812313988804817,
0.0029607422184199095,
-0.08706263452768326,
0.019983965903520584,
-0.027895716950297356,
-0.020017409697175026,
-0.08393633365631104,
0.031068192794919014,
-0.05637199804186821,
0.013307387009263039,
-0.01173231191933155,
-0.015753503888845444,
0.04919538274407387,
-0.03851527348160744,
-0.013763802126049995,
-0.02548707276582718,
0.008141864091157913,
-0.04691184684634209,
-0.05399065092206001,
0.023645954206585884,
8.31248717967569e-34,
0.05022300034761429,
-0.030464667826890945,
-0.024610400199890137,
-0.00043127575190737844,
-0.06971555203199387,
-0.011033378541469574,
-0.034090664237737656,
0.02717456966638565,
-0.11182677000761032,
-0.0014321244088932872,
-0.013591323979198933,
-0.013961863704025745,
-0.08343179523944855,
0.019637595862150192,
-0.022892262786626816,
0.017927436158061028,
0.07686670869588852,
0.016649939119815826,
0.029210170730948448,
0.02903961017727852,
0.08145651966333389,
0.04654150456190109,
-0.007197648286819458,
-0.041566457599401474,
-0.0498504675924778,
0.050852179527282715,
0.02021351270377636,
-0.11830894649028778,
-0.12205364555120468,
0.01651395671069622,
-0.09839661419391632,
0.029213691130280495,
-0.013918107375502586,
0.01508075837045908,
-0.0069982195273041725,
-0.019060833379626274,
-0.002693123882636428,
-0.005621030926704407,
-0.047176457941532135,
-0.09294277429580688,
-0.002424401929602027,
0.011522646062076092,
-0.01744142547249794,
-0.05954161658883095,
0.03379439190030098,
0.01856314018368721,
0.0009905846090987325,
0.008605648763477802,
0.10523494333028793,
0.0024502133019268513,
0.008123919367790222,
0.06283087283372879,
-0.05528442934155464,
0.004973942879587412,
0.034536343067884445,
0.11508549004793167,
0.0765155702829361,
0.013185769319534302,
0.019467003643512726,
0.03527981415390968,
0.06933695822954178,
0.032862477004528046,
0.02210930921137333,
0.011447291821241379,
0.10499569028615952,
-0.01850229687988758,
-0.03402069956064224,
-0.07462755590677261,
0.06940875202417374,
0.039257872849702835,
-0.14163067936897278,
-0.04802917316555977,
0.06501121819019318,
0.0819903016090393,
0.05573343485593796,
-0.022939227521419525,
-0.027686186134815216,
-0.024221526458859444,
-0.01959657296538353,
-0.020897461101412773,
-0.07057557255029678,
0.017077481374144554,
-0.0010119711514562368,
-0.024258827790617943,
-0.02500239759683609,
-0.0027463152073323727,
0.04975736141204834,
-0.07027111202478409,
-0.04278341308236122,
0.00040383345913141966,
0.044984765350818634,
0.04948042705655098,
-0.09487809985876083,
-0.010065140202641487,
-0.007503151893615723,
-1.549094006162458e-33,
0.0860731452703476,
0.017262516543269157,
-0.05247551575303078,
0.07170981168746948,
-0.026122525334358215,
-0.06546612828969955,
-0.0011655710404738784,
0.11471722275018692,
0.0483442023396492,
0.051387909799814224,
0.0719359815120697,
-0.14453226327896118,
0.026392001658678055,
-0.07345808297395706,
0.06951995939016342,
-0.04517272487282753,
-0.009838469326496124,
0.0515676848590374,
0.027269715443253517,
0.024956580251455307,
0.014997970312833786,
0.09043712168931961,
-0.018832115456461906,
0.0939713716506958,
-0.0005589217180386186,
-0.0240783654153347,
-0.011372996494174004,
0.07640894502401352,
-0.0033985082991421223,
-0.00856047309935093,
0.0035929863806813955,
-0.003396125975996256,
-0.10814815759658813,
-0.01769261807203293,
-0.06922831386327744,
0.04689301922917366,
0.02489318512380123,
0.035680368542671204,
0.035970866680145264,
0.0653262510895729,
0.057099033147096634,
0.07072703540325165,
-0.04521647095680237,
-0.032769110053777695,
0.014033057726919651,
-0.03256602585315704,
0.011452832259237766,
0.008140301331877708,
0.0008033349877223372,
-0.08585818856954575,
0.023025143891572952,
-0.0003118524618912488,
-0.08310036361217499,
-0.010134603828191757,
-0.008220835588872433,
-0.07676286995410919,
-0.017729850485920906,
-0.13388365507125854,
-0.05010807141661644,
-0.022418353706598282,
-0.015006289817392826,
0.030588248744606972,
-0.0438365712761879,
-0.06912010908126831,
0.03147801384329796,
-0.0012659018393605947,
0.039950188249349594,
0.008855660445988178,
0.016889745369553566,
0.05894661322236061,
-0.02637922577559948,
-0.05814644321799278,
0.07087266445159912,
0.09146378934383392,
0.0019240730907768011,
-0.05019286274909973,
-0.041109684854745865,
0.03383777290582657,
0.055256832391023636,
-0.07964544743299484,
-0.025428669527173042,
0.018149150535464287,
0.011325359344482422,
0.043049126863479614,
0.1126742735505104,
0.1106194481253624,
0.02598787099123001,
-0.0034613963216543198,
-0.008945813402533531,
0.07130618393421173,
0.022110210731625557,
0.021438095718622208,
0.018766136839985847,
0.11184791475534439,
-0.00715851504355669,
-4.965154687397444e-8,
-0.09687219560146332,
0.004911581985652447,
-0.11086437851190567,
0.04993084445595741,
-0.04268181324005127,
-0.0735488161444664,
-0.06410493701696396,
-0.025382474064826965,
-0.05324063077569008,
-0.02238025888800621,
-0.009494544938206673,
0.020167654380202293,
-0.07326023280620575,
-0.010446717962622643,
-0.042614471167325974,
0.01707579381763935,
-0.015074197202920914,
0.09150262176990509,
-0.02712886966764927,
-0.03016963228583336,
0.05590159073472023,
0.04955258220434189,
0.03865884616971016,
-0.06863176077604294,
-0.005780661012977362,
0.0033925827592611313,
-0.042007509618997574,
0.035013724118471146,
0.01829158514738083,
0.008446168154478073,
0.0402509942650795,
0.043193407356739044,
-0.015930937603116035,
-0.09050533175468445,
0.05273185670375824,
0.06264108419418335,
-0.0013672715285792947,
-0.03149295598268509,
-0.013339225202798843,
0.07080470770597458,
0.09172149002552032,
0.03995486721396446,
-0.10449466854333878,
0.026216302067041397,
0.027038903906941414,
-0.024427779018878937,
-0.0579257495701313,
-0.03149574622511864,
0.034507088363170624,
-0.06438866257667542,
0.07882561534643173,
-0.06385833770036697,
-0.05978259816765785,
0.02806621417403221,
0.03441471979022026,
0.007334426511079073,
0.06708201766014099,
-0.007877635769546032,
0.007801218889653683,
-0.014044053852558136,
0.048198550939559937,
-0.024124780669808388,
-0.021421222016215324,
-0.016138724982738495
] |
hf-internal-testing/tiny-random-prophetnet | d5071e4655fd0413b0e71405a91dfb4280e31b81 | 2021-09-17T19:24:57.000Z | [
"pytorch",
"prophetnet",
"transformers"
] | null | false | hf-internal-testing | null | hf-internal-testing/tiny-random-prophetnet | 1,998 | null | transformers | Entry not found | [
0.0461147278547287,
-0.038838207721710205,
-0.01049656979739666,
-0.03682169318199158,
0.011261860840022564,
0.013094935566186905,
0.0019101888174191117,
-0.013979103416204453,
0.027092741802334785,
-0.015212527476251125,
0.017284274101257324,
-0.08189476281404495,
0.03817418962717056,
-0.04920130595564842,
0.021389011293649673,
-0.015245908871293068,
-0.03203780576586723,
-0.1245758980512619,
0.03150877356529236,
0.032381657510995865,
-0.060957908630371094,
0.05409295856952667,
-0.025087490677833557,
0.01568586938083172,
0.028129950165748596,
-0.04710396006703377,
-0.018688226118683815,
0.013785239309072495,
-0.04001208767294884,
0.01173911802470684,
-0.04317743331193924,
0.05500618368387222,
0.004543041344732046,
0.02973111905157566,
0.14852192997932434,
0.02658126689493656,
0.02907961793243885,
-0.05169107764959335,
0.05803573504090309,
-0.07732241600751877,
-0.017637968063354492,
-0.04219653457403183,
0.041807834059000015,
0.023620979860424995,
0.021563321352005005,
0.016478516161441803,
-0.0021814992651343346,
-0.06400240957736969,
0.06393089145421982,
0.019599027931690216,
-0.08565037697553635,
0.00934905931353569,
-0.008718925528228283,
-0.028583496809005737,
-0.07310017943382263,
0.09416428208351135,
0.001759322709403932,
0.06184990331530571,
0.011840506456792355,
-0.035997264087200165,
0.08358278125524521,
-0.02619801089167595,
0.03736566752195358,
-0.028206506744027138,
-0.07454850524663925,
-0.08883563429117203,
-0.06279942393302917,
-0.008695344440639019,
0.014119276776909828,
-0.0825355276465416,
0.0649217739701271,
-0.00223911227658391,
-0.14716917276382446,
0.07743025571107864,
-0.03548373281955719,
-0.055201586335897446,
0.006981803569942713,
-0.012166670523583889,
0.055111464112997055,
-0.007116836030036211,
-0.023175746202468872,
-0.005835152696818113,
-0.09185640513896942,
0.055196937173604965,
0.034148022532463074,
0.03835180774331093,
0.038685429841279984,
-0.025987252593040466,
0.017804903909564018,
0.022428328171372414,
0.025005368515849113,
-0.10761535167694092,
-0.048001550137996674,
-0.04343584179878235,
0.012374646961688995,
-0.019502125680446625,
0.029218152165412903,
0.0842173621058464,
-0.011719699949026108,
0.09283553808927536,
-0.007015465293079615,
-0.03543110564351082,
-0.06936459988355637,
0.09425332397222519,
-0.010958523489534855,
-0.00805904995650053,
0.004974212497472763,
-0.0031528924591839314,
0.06105927750468254,
-0.03964288905262947,
-0.03619541600346565,
-0.019901901483535767,
0.07134733349084854,
0.039514873176813126,
-0.012729483656585217,
-0.006646515801548958,
-0.04746140539646149,
-0.014432490803301334,
-0.05157482624053955,
0.09506245702505112,
-0.049747664481401443,
-0.04591796174645424,
-0.008965466171503067,
-0.0325421579182148,
-0.08626784384250641,
-0.06624380499124527,
0.02538885548710823,
-4.303924894057984e-33,
0.01133066974580288,
0.0033434738870710135,
-0.002155609894543886,
0.04871906340122223,
-0.023564351722598076,
-0.07933273911476135,
0.0600903145968914,
0.02335330657660961,
-0.03844716399908066,
-0.020433755591511726,
-0.06952055543661118,
-0.03235611692070961,
0.0062485747039318085,
0.064804308116436,
-0.03201229125261307,
0.061689723283052444,
0.0417000837624073,
-0.00761845987290144,
0.03340127319097519,
-0.047770582139492035,
0.00887306872755289,
-0.04066338762640953,
-0.010506896302103996,
0.0106519665569067,
0.021333497017621994,
0.12854498624801636,
-0.009705503471195698,
0.010055632330477238,
-0.017507633194327354,
0.006515394430607557,
0.06334009766578674,
-0.057817306369543076,
0.013668818399310112,
-0.020286159589886665,
0.05430467426776886,
-0.023184705525636673,
0.0828516036272049,
0.0005449643940664828,
-0.10372652113437653,
-0.07634282112121582,
-0.005381610710173845,
-0.039263784885406494,
0.0006114727002568543,
-0.013281986117362976,
0.07119110971689224,
0.043696220964193344,
0.03168422728776932,
0.04338686540722847,
0.05728672817349434,
0.0832006186246872,
-0.07961414009332657,
0.015234283171594143,
0.017002005130052567,
0.047004107385873795,
-0.09794387966394424,
0.004990279674530029,
-0.07062993198633194,
-0.028000490739941597,
-0.04018733277916908,
-0.0702052190899849,
0.011351344175636768,
0.06020182743668556,
-0.03297270089387894,
0.09396500885486603,
0.03417910635471344,
-0.019825750961899757,
-0.034690454602241516,
-0.013036907650530338,
0.05896938592195511,
-0.012359356507658958,
-0.017275206744670868,
-0.07982361316680908,
0.02059139870107174,
0.06737419217824936,
0.04176458343863487,
-0.04978838190436363,
-0.05877475067973137,
-0.06289287656545639,
-0.03354167565703392,
-0.03871942684054375,
0.009898529388010502,
-0.05514208599925041,
-0.11629002541303635,
-0.011855563148856163,
0.10663620382547379,
0.037354156374931335,
-0.0065480442717671394,
-0.051189567893743515,
0.06663123518228531,
0.01874656230211258,
0.032841797918081284,
0.041593004018068314,
-0.06879369914531708,
0.04216769337654114,
-0.01628219522535801,
5.4139394340936695e-34,
0.05697013810276985,
-0.006972255185246468,
0.015711724758148193,
-0.17956365644931793,
0.02320219948887825,
0.007923615165054798,
-0.008062449283897877,
0.0074974060989916325,
0.07391711324453354,
0.0309313777834177,
0.060510627925395966,
0.058605875819921494,
0.09515274316072464,
-0.002282935893163085,
0.001603541080839932,
0.07024981826543808,
0.012629246339201927,
0.07425693422555923,
-0.038426291197538376,
0.01861148327589035,
0.030608950182795525,
-0.02449394389986992,
0.021528491750359535,
-0.003039651783183217,
-0.03676343336701393,
0.03130284696817398,
0.07998586446046829,
0.010451192036271095,
-0.07930229604244232,
-0.013543923385441303,
0.018781835213303566,
0.05168003588914871,
-0.07191970944404602,
0.15783067047595978,
0.026191607117652893,
0.01262354850769043,
0.08218053728342056,
-0.029807550832629204,
-0.07528624683618546,
-0.04250097647309303,
0.017244765534996986,
0.04411793500185013,
0.03708017244935036,
0.009233047254383564,
-0.040271829813718796,
0.022496428340673447,
0.02495843544602394,
0.07633638381958008,
0.005147108342498541,
0.013892097398638725,
0.05610476806759834,
-0.06684739887714386,
0.05862557515501976,
-0.020688841119408607,
0.05377643182873726,
0.06718500703573227,
0.005329249892383814,
-0.01388032827526331,
0.029931528493762016,
0.009508464485406876,
-0.045173756778240204,
0.11534366756677628,
-0.06510116159915924,
0.05117698386311531,
-0.0026125339791178703,
-0.08554837852716446,
-0.03784770518541336,
0.0804959163069725,
0.011298024095594883,
-0.07695550471544266,
-0.04868878796696663,
0.02515520341694355,
0.06252261996269226,
-0.04509226232767105,
-0.01246943511068821,
0.028559505939483643,
-0.030573077499866486,
0.05066261067986488,
-0.08187384903430939,
0.04469604790210724,
0.0034051244147121906,
0.04145054519176483,
-0.021858664229512215,
-0.06112268194556236,
-0.00908052921295166,
-0.05903250351548195,
0.0259539932012558,
0.059690944850444794,
-0.07613514363765717,
-0.03720718249678612,
-0.036316655576229095,
0.07058046013116837,
-0.008224100805819035,
0.041961874812841415,
-0.0285952128469944,
-1.496900736697171e-8,
-0.0014124972512945533,
0.03401879221200943,
-0.040338415652513504,
0.04116074740886688,
0.0935964286327362,
-0.05115952715277672,
0.0008746005478315055,
-0.03389839455485344,
-0.00567849725484848,
-0.010686947964131832,
-0.04789939522743225,
-0.04820054769515991,
-0.02011880651116371,
-0.03209094703197479,
-0.04211259260773659,
-0.10229527950286865,
-0.07819421589374542,
-0.031228765845298767,
-0.02154778689146042,
-0.04960230365395546,
0.08087796717882156,
-0.07801242172718048,
0.06919731199741364,
-0.04999840259552002,
0.03687043860554695,
0.03889009356498718,
-0.049989692866802216,
-0.04254625365138054,
-0.04606937617063522,
0.08682432025671005,
-0.031148413196206093,
0.11826753616333008,
0.034102488309144974,
-0.0208592489361763,
-0.0205202866345644,
0.027134142816066742,
0.09741277992725372,
0.051608603447675705,
0.013477512635290623,
-0.13649295270442963,
-0.022304272279143333,
0.02385953813791275,
0.038732077926397324,
-0.09249968826770782,
-0.04549082741141319,
0.054220106452703476,
0.01160438358783722,
0.051190607249736786,
0.07713303714990616,
-0.022097084671258926,
-0.06127818301320076,
-0.01857956498861313,
0.006740490905940533,
-0.00496308971196413,
0.024095389991998672,
0.0736224576830864,
-0.003481915919110179,
-0.0699305310845375,
-0.006629763171076775,
-0.0598808117210865,
0.05297163128852844,
-0.02902800403535366,
-0.027858933433890343,
-0.01287526823580265
] |
hf-internal-testing/tiny-random-mobilebert | 1f919a6d77ef448d41e0de29f79f854ace43bc4c | 2021-09-17T19:24:24.000Z | [
"pytorch",
"tf",
"mobilebert",
"transformers"
] | null | false | hf-internal-testing | null | hf-internal-testing/tiny-random-mobilebert | 1,996 | null | transformers | Entry not found | [
0.0461147278547287,
-0.038838207721710205,
-0.01049656979739666,
-0.03682169318199158,
0.011261860840022564,
0.013094935566186905,
0.0019101888174191117,
-0.013979103416204453,
0.027092741802334785,
-0.015212527476251125,
0.017284274101257324,
-0.08189476281404495,
0.03817418962717056,
-0.04920130595564842,
0.021389011293649673,
-0.015245908871293068,
-0.03203780576586723,
-0.1245758980512619,
0.03150877356529236,
0.032381657510995865,
-0.060957908630371094,
0.05409295856952667,
-0.025087490677833557,
0.01568586938083172,
0.028129950165748596,
-0.04710396006703377,
-0.018688226118683815,
0.013785239309072495,
-0.04001208767294884,
0.01173911802470684,
-0.04317743331193924,
0.05500618368387222,
0.004543041344732046,
0.02973111905157566,
0.14852192997932434,
0.02658126689493656,
0.02907961793243885,
-0.05169107764959335,
0.05803573504090309,
-0.07732241600751877,
-0.017637968063354492,
-0.04219653457403183,
0.041807834059000015,
0.023620979860424995,
0.021563321352005005,
0.016478516161441803,
-0.0021814992651343346,
-0.06400240957736969,
0.06393089145421982,
0.019599027931690216,
-0.08565037697553635,
0.00934905931353569,
-0.008718925528228283,
-0.028583496809005737,
-0.07310017943382263,
0.09416428208351135,
0.001759322709403932,
0.06184990331530571,
0.011840506456792355,
-0.035997264087200165,
0.08358278125524521,
-0.02619801089167595,
0.03736566752195358,
-0.028206506744027138,
-0.07454850524663925,
-0.08883563429117203,
-0.06279942393302917,
-0.008695344440639019,
0.014119276776909828,
-0.0825355276465416,
0.0649217739701271,
-0.00223911227658391,
-0.14716917276382446,
0.07743025571107864,
-0.03548373281955719,
-0.055201586335897446,
0.006981803569942713,
-0.012166670523583889,
0.055111464112997055,
-0.007116836030036211,
-0.023175746202468872,
-0.005835152696818113,
-0.09185640513896942,
0.055196937173604965,
0.034148022532463074,
0.03835180774331093,
0.038685429841279984,
-0.025987252593040466,
0.017804903909564018,
0.022428328171372414,
0.025005368515849113,
-0.10761535167694092,
-0.048001550137996674,
-0.04343584179878235,
0.012374646961688995,
-0.019502125680446625,
0.029218152165412903,
0.0842173621058464,
-0.011719699949026108,
0.09283553808927536,
-0.007015465293079615,
-0.03543110564351082,
-0.06936459988355637,
0.09425332397222519,
-0.010958523489534855,
-0.00805904995650053,
0.004974212497472763,
-0.0031528924591839314,
0.06105927750468254,
-0.03964288905262947,
-0.03619541600346565,
-0.019901901483535767,
0.07134733349084854,
0.039514873176813126,
-0.012729483656585217,
-0.006646515801548958,
-0.04746140539646149,
-0.014432490803301334,
-0.05157482624053955,
0.09506245702505112,
-0.049747664481401443,
-0.04591796174645424,
-0.008965466171503067,
-0.0325421579182148,
-0.08626784384250641,
-0.06624380499124527,
0.02538885548710823,
-4.303924894057984e-33,
0.01133066974580288,
0.0033434738870710135,
-0.002155609894543886,
0.04871906340122223,
-0.023564351722598076,
-0.07933273911476135,
0.0600903145968914,
0.02335330657660961,
-0.03844716399908066,
-0.020433755591511726,
-0.06952055543661118,
-0.03235611692070961,
0.0062485747039318085,
0.064804308116436,
-0.03201229125261307,
0.061689723283052444,
0.0417000837624073,
-0.00761845987290144,
0.03340127319097519,
-0.047770582139492035,
0.00887306872755289,
-0.04066338762640953,
-0.010506896302103996,
0.0106519665569067,
0.021333497017621994,
0.12854498624801636,
-0.009705503471195698,
0.010055632330477238,
-0.017507633194327354,
0.006515394430607557,
0.06334009766578674,
-0.057817306369543076,
0.013668818399310112,
-0.020286159589886665,
0.05430467426776886,
-0.023184705525636673,
0.0828516036272049,
0.0005449643940664828,
-0.10372652113437653,
-0.07634282112121582,
-0.005381610710173845,
-0.039263784885406494,
0.0006114727002568543,
-0.013281986117362976,
0.07119110971689224,
0.043696220964193344,
0.03168422728776932,
0.04338686540722847,
0.05728672817349434,
0.0832006186246872,
-0.07961414009332657,
0.015234283171594143,
0.017002005130052567,
0.047004107385873795,
-0.09794387966394424,
0.004990279674530029,
-0.07062993198633194,
-0.028000490739941597,
-0.04018733277916908,
-0.0702052190899849,
0.011351344175636768,
0.06020182743668556,
-0.03297270089387894,
0.09396500885486603,
0.03417910635471344,
-0.019825750961899757,
-0.034690454602241516,
-0.013036907650530338,
0.05896938592195511,
-0.012359356507658958,
-0.017275206744670868,
-0.07982361316680908,
0.02059139870107174,
0.06737419217824936,
0.04176458343863487,
-0.04978838190436363,
-0.05877475067973137,
-0.06289287656545639,
-0.03354167565703392,
-0.03871942684054375,
0.009898529388010502,
-0.05514208599925041,
-0.11629002541303635,
-0.011855563148856163,
0.10663620382547379,
0.037354156374931335,
-0.0065480442717671394,
-0.051189567893743515,
0.06663123518228531,
0.01874656230211258,
0.032841797918081284,
0.041593004018068314,
-0.06879369914531708,
0.04216769337654114,
-0.01628219522535801,
5.4139394340936695e-34,
0.05697013810276985,
-0.006972255185246468,
0.015711724758148193,
-0.17956365644931793,
0.02320219948887825,
0.007923615165054798,
-0.008062449283897877,
0.0074974060989916325,
0.07391711324453354,
0.0309313777834177,
0.060510627925395966,
0.058605875819921494,
0.09515274316072464,
-0.002282935893163085,
0.001603541080839932,
0.07024981826543808,
0.012629246339201927,
0.07425693422555923,
-0.038426291197538376,
0.01861148327589035,
0.030608950182795525,
-0.02449394389986992,
0.021528491750359535,
-0.003039651783183217,
-0.03676343336701393,
0.03130284696817398,
0.07998586446046829,
0.010451192036271095,
-0.07930229604244232,
-0.013543923385441303,
0.018781835213303566,
0.05168003588914871,
-0.07191970944404602,
0.15783067047595978,
0.026191607117652893,
0.01262354850769043,
0.08218053728342056,
-0.029807550832629204,
-0.07528624683618546,
-0.04250097647309303,
0.017244765534996986,
0.04411793500185013,
0.03708017244935036,
0.009233047254383564,
-0.040271829813718796,
0.022496428340673447,
0.02495843544602394,
0.07633638381958008,
0.005147108342498541,
0.013892097398638725,
0.05610476806759834,
-0.06684739887714386,
0.05862557515501976,
-0.020688841119408607,
0.05377643182873726,
0.06718500703573227,
0.005329249892383814,
-0.01388032827526331,
0.029931528493762016,
0.009508464485406876,
-0.045173756778240204,
0.11534366756677628,
-0.06510116159915924,
0.05117698386311531,
-0.0026125339791178703,
-0.08554837852716446,
-0.03784770518541336,
0.0804959163069725,
0.011298024095594883,
-0.07695550471544266,
-0.04868878796696663,
0.02515520341694355,
0.06252261996269226,
-0.04509226232767105,
-0.01246943511068821,
0.028559505939483643,
-0.030573077499866486,
0.05066261067986488,
-0.08187384903430939,
0.04469604790210724,
0.0034051244147121906,
0.04145054519176483,
-0.021858664229512215,
-0.06112268194556236,
-0.00908052921295166,
-0.05903250351548195,
0.0259539932012558,
0.059690944850444794,
-0.07613514363765717,
-0.03720718249678612,
-0.036316655576229095,
0.07058046013116837,
-0.008224100805819035,
0.041961874812841415,
-0.0285952128469944,
-1.496900736697171e-8,
-0.0014124972512945533,
0.03401879221200943,
-0.040338415652513504,
0.04116074740886688,
0.0935964286327362,
-0.05115952715277672,
0.0008746005478315055,
-0.03389839455485344,
-0.00567849725484848,
-0.010686947964131832,
-0.04789939522743225,
-0.04820054769515991,
-0.02011880651116371,
-0.03209094703197479,
-0.04211259260773659,
-0.10229527950286865,
-0.07819421589374542,
-0.031228765845298767,
-0.02154778689146042,
-0.04960230365395546,
0.08087796717882156,
-0.07801242172718048,
0.06919731199741364,
-0.04999840259552002,
0.03687043860554695,
0.03889009356498718,
-0.049989692866802216,
-0.04254625365138054,
-0.04606937617063522,
0.08682432025671005,
-0.031148413196206093,
0.11826753616333008,
0.034102488309144974,
-0.0208592489361763,
-0.0205202866345644,
0.027134142816066742,
0.09741277992725372,
0.051608603447675705,
0.013477512635290623,
-0.13649295270442963,
-0.022304272279143333,
0.02385953813791275,
0.038732077926397324,
-0.09249968826770782,
-0.04549082741141319,
0.054220106452703476,
0.01160438358783722,
0.051190607249736786,
0.07713303714990616,
-0.022097084671258926,
-0.06127818301320076,
-0.01857956498861313,
0.006740490905940533,
-0.00496308971196413,
0.024095389991998672,
0.0736224576830864,
-0.003481915919110179,
-0.0699305310845375,
-0.006629763171076775,
-0.0598808117210865,
0.05297163128852844,
-0.02902800403535366,
-0.027858933433890343,
-0.01287526823580265
] |
hf-internal-testing/tiny-random-squeezebert | da3eaaeb3b2fa22836d34097046f192db387e961 | 2021-09-17T19:25:10.000Z | [
"pytorch",
"squeezebert",
"transformers"
] | null | false | hf-internal-testing | null | hf-internal-testing/tiny-random-squeezebert | 1,995 | null | transformers | Entry not found | [
0.0461147278547287,
-0.038838207721710205,
-0.01049656979739666,
-0.03682169318199158,
0.011261860840022564,
0.013094935566186905,
0.0019101888174191117,
-0.013979103416204453,
0.027092741802334785,
-0.015212527476251125,
0.017284274101257324,
-0.08189476281404495,
0.03817418962717056,
-0.04920130595564842,
0.021389011293649673,
-0.015245908871293068,
-0.03203780576586723,
-0.1245758980512619,
0.03150877356529236,
0.032381657510995865,
-0.060957908630371094,
0.05409295856952667,
-0.025087490677833557,
0.01568586938083172,
0.028129950165748596,
-0.04710396006703377,
-0.018688226118683815,
0.013785239309072495,
-0.04001208767294884,
0.01173911802470684,
-0.04317743331193924,
0.05500618368387222,
0.004543041344732046,
0.02973111905157566,
0.14852192997932434,
0.02658126689493656,
0.02907961793243885,
-0.05169107764959335,
0.05803573504090309,
-0.07732241600751877,
-0.017637968063354492,
-0.04219653457403183,
0.041807834059000015,
0.023620979860424995,
0.021563321352005005,
0.016478516161441803,
-0.0021814992651343346,
-0.06400240957736969,
0.06393089145421982,
0.019599027931690216,
-0.08565037697553635,
0.00934905931353569,
-0.008718925528228283,
-0.028583496809005737,
-0.07310017943382263,
0.09416428208351135,
0.001759322709403932,
0.06184990331530571,
0.011840506456792355,
-0.035997264087200165,
0.08358278125524521,
-0.02619801089167595,
0.03736566752195358,
-0.028206506744027138,
-0.07454850524663925,
-0.08883563429117203,
-0.06279942393302917,
-0.008695344440639019,
0.014119276776909828,
-0.0825355276465416,
0.0649217739701271,
-0.00223911227658391,
-0.14716917276382446,
0.07743025571107864,
-0.03548373281955719,
-0.055201586335897446,
0.006981803569942713,
-0.012166670523583889,
0.055111464112997055,
-0.007116836030036211,
-0.023175746202468872,
-0.005835152696818113,
-0.09185640513896942,
0.055196937173604965,
0.034148022532463074,
0.03835180774331093,
0.038685429841279984,
-0.025987252593040466,
0.017804903909564018,
0.022428328171372414,
0.025005368515849113,
-0.10761535167694092,
-0.048001550137996674,
-0.04343584179878235,
0.012374646961688995,
-0.019502125680446625,
0.029218152165412903,
0.0842173621058464,
-0.011719699949026108,
0.09283553808927536,
-0.007015465293079615,
-0.03543110564351082,
-0.06936459988355637,
0.09425332397222519,
-0.010958523489534855,
-0.00805904995650053,
0.004974212497472763,
-0.0031528924591839314,
0.06105927750468254,
-0.03964288905262947,
-0.03619541600346565,
-0.019901901483535767,
0.07134733349084854,
0.039514873176813126,
-0.012729483656585217,
-0.006646515801548958,
-0.04746140539646149,
-0.014432490803301334,
-0.05157482624053955,
0.09506245702505112,
-0.049747664481401443,
-0.04591796174645424,
-0.008965466171503067,
-0.0325421579182148,
-0.08626784384250641,
-0.06624380499124527,
0.02538885548710823,
-4.303924894057984e-33,
0.01133066974580288,
0.0033434738870710135,
-0.002155609894543886,
0.04871906340122223,
-0.023564351722598076,
-0.07933273911476135,
0.0600903145968914,
0.02335330657660961,
-0.03844716399908066,
-0.020433755591511726,
-0.06952055543661118,
-0.03235611692070961,
0.0062485747039318085,
0.064804308116436,
-0.03201229125261307,
0.061689723283052444,
0.0417000837624073,
-0.00761845987290144,
0.03340127319097519,
-0.047770582139492035,
0.00887306872755289,
-0.04066338762640953,
-0.010506896302103996,
0.0106519665569067,
0.021333497017621994,
0.12854498624801636,
-0.009705503471195698,
0.010055632330477238,
-0.017507633194327354,
0.006515394430607557,
0.06334009766578674,
-0.057817306369543076,
0.013668818399310112,
-0.020286159589886665,
0.05430467426776886,
-0.023184705525636673,
0.0828516036272049,
0.0005449643940664828,
-0.10372652113437653,
-0.07634282112121582,
-0.005381610710173845,
-0.039263784885406494,
0.0006114727002568543,
-0.013281986117362976,
0.07119110971689224,
0.043696220964193344,
0.03168422728776932,
0.04338686540722847,
0.05728672817349434,
0.0832006186246872,
-0.07961414009332657,
0.015234283171594143,
0.017002005130052567,
0.047004107385873795,
-0.09794387966394424,
0.004990279674530029,
-0.07062993198633194,
-0.028000490739941597,
-0.04018733277916908,
-0.0702052190899849,
0.011351344175636768,
0.06020182743668556,
-0.03297270089387894,
0.09396500885486603,
0.03417910635471344,
-0.019825750961899757,
-0.034690454602241516,
-0.013036907650530338,
0.05896938592195511,
-0.012359356507658958,
-0.017275206744670868,
-0.07982361316680908,
0.02059139870107174,
0.06737419217824936,
0.04176458343863487,
-0.04978838190436363,
-0.05877475067973137,
-0.06289287656545639,
-0.03354167565703392,
-0.03871942684054375,
0.009898529388010502,
-0.05514208599925041,
-0.11629002541303635,
-0.011855563148856163,
0.10663620382547379,
0.037354156374931335,
-0.0065480442717671394,
-0.051189567893743515,
0.06663123518228531,
0.01874656230211258,
0.032841797918081284,
0.041593004018068314,
-0.06879369914531708,
0.04216769337654114,
-0.01628219522535801,
5.4139394340936695e-34,
0.05697013810276985,
-0.006972255185246468,
0.015711724758148193,
-0.17956365644931793,
0.02320219948887825,
0.007923615165054798,
-0.008062449283897877,
0.0074974060989916325,
0.07391711324453354,
0.0309313777834177,
0.060510627925395966,
0.058605875819921494,
0.09515274316072464,
-0.002282935893163085,
0.001603541080839932,
0.07024981826543808,
0.012629246339201927,
0.07425693422555923,
-0.038426291197538376,
0.01861148327589035,
0.030608950182795525,
-0.02449394389986992,
0.021528491750359535,
-0.003039651783183217,
-0.03676343336701393,
0.03130284696817398,
0.07998586446046829,
0.010451192036271095,
-0.07930229604244232,
-0.013543923385441303,
0.018781835213303566,
0.05168003588914871,
-0.07191970944404602,
0.15783067047595978,
0.026191607117652893,
0.01262354850769043,
0.08218053728342056,
-0.029807550832629204,
-0.07528624683618546,
-0.04250097647309303,
0.017244765534996986,
0.04411793500185013,
0.03708017244935036,
0.009233047254383564,
-0.040271829813718796,
0.022496428340673447,
0.02495843544602394,
0.07633638381958008,
0.005147108342498541,
0.013892097398638725,
0.05610476806759834,
-0.06684739887714386,
0.05862557515501976,
-0.020688841119408607,
0.05377643182873726,
0.06718500703573227,
0.005329249892383814,
-0.01388032827526331,
0.029931528493762016,
0.009508464485406876,
-0.045173756778240204,
0.11534366756677628,
-0.06510116159915924,
0.05117698386311531,
-0.0026125339791178703,
-0.08554837852716446,
-0.03784770518541336,
0.0804959163069725,
0.011298024095594883,
-0.07695550471544266,
-0.04868878796696663,
0.02515520341694355,
0.06252261996269226,
-0.04509226232767105,
-0.01246943511068821,
0.028559505939483643,
-0.030573077499866486,
0.05066261067986488,
-0.08187384903430939,
0.04469604790210724,
0.0034051244147121906,
0.04145054519176483,
-0.021858664229512215,
-0.06112268194556236,
-0.00908052921295166,
-0.05903250351548195,
0.0259539932012558,
0.059690944850444794,
-0.07613514363765717,
-0.03720718249678612,
-0.036316655576229095,
0.07058046013116837,
-0.008224100805819035,
0.041961874812841415,
-0.0285952128469944,
-1.496900736697171e-8,
-0.0014124972512945533,
0.03401879221200943,
-0.040338415652513504,
0.04116074740886688,
0.0935964286327362,
-0.05115952715277672,
0.0008746005478315055,
-0.03389839455485344,
-0.00567849725484848,
-0.010686947964131832,
-0.04789939522743225,
-0.04820054769515991,
-0.02011880651116371,
-0.03209094703197479,
-0.04211259260773659,
-0.10229527950286865,
-0.07819421589374542,
-0.031228765845298767,
-0.02154778689146042,
-0.04960230365395546,
0.08087796717882156,
-0.07801242172718048,
0.06919731199741364,
-0.04999840259552002,
0.03687043860554695,
0.03889009356498718,
-0.049989692866802216,
-0.04254625365138054,
-0.04606937617063522,
0.08682432025671005,
-0.031148413196206093,
0.11826753616333008,
0.034102488309144974,
-0.0208592489361763,
-0.0205202866345644,
0.027134142816066742,
0.09741277992725372,
0.051608603447675705,
0.013477512635290623,
-0.13649295270442963,
-0.022304272279143333,
0.02385953813791275,
0.038732077926397324,
-0.09249968826770782,
-0.04549082741141319,
0.054220106452703476,
0.01160438358783722,
0.051190607249736786,
0.07713303714990616,
-0.022097084671258926,
-0.06127818301320076,
-0.01857956498861313,
0.006740490905940533,
-0.00496308971196413,
0.024095389991998672,
0.0736224576830864,
-0.003481915919110179,
-0.0699305310845375,
-0.006629763171076775,
-0.0598808117210865,
0.05297163128852844,
-0.02902800403535366,
-0.027858933433890343,
-0.01287526823580265
] |
Helsinki-NLP/opus-mt-tc-big-en-tr | dc016b6b79636a066052e581101c734ca5934667 | 2022-06-01T13:01:51.000Z | [
"pytorch",
"marian",
"text2text-generation",
"en",
"tr",
"transformers",
"translation",
"opus-mt-tc",
"license:cc-by-4.0",
"model-index",
"autotrain_compatible"
] | translation | false | Helsinki-NLP | null | Helsinki-NLP/opus-mt-tc-big-en-tr | 1,995 | 1 | transformers | ---
language:
- en
- tr
tags:
- translation
- opus-mt-tc
license: cc-by-4.0
model-index:
- name: opus-mt-tc-big-en-tr
results:
- task:
name: Translation eng-tur
type: translation
args: eng-tur
dataset:
name: flores101-devtest
type: flores_101
args: eng tur devtest
metrics:
- name: BLEU
type: bleu
value: 31.4
- task:
name: Translation eng-tur
type: translation
args: eng-tur
dataset:
name: newsdev2016
type: newsdev2016
args: eng-tur
metrics:
- name: BLEU
type: bleu
value: 21.9
- task:
name: Translation eng-tur
type: translation
args: eng-tur
dataset:
name: tatoeba-test-v2021-08-07
type: tatoeba_mt
args: eng-tur
metrics:
- name: BLEU
type: bleu
value: 42.3
- task:
name: Translation eng-tur
type: translation
args: eng-tur
dataset:
name: newstest2016
type: wmt-2016-news
args: eng-tur
metrics:
- name: BLEU
type: bleu
value: 23.4
- task:
name: Translation eng-tur
type: translation
args: eng-tur
dataset:
name: newstest2017
type: wmt-2017-news
args: eng-tur
metrics:
- name: BLEU
type: bleu
value: 25.4
- task:
name: Translation eng-tur
type: translation
args: eng-tur
dataset:
name: newstest2018
type: wmt-2018-news
args: eng-tur
metrics:
- name: BLEU
type: bleu
value: 22.6
---
# opus-mt-tc-big-en-tr
Neural machine translation model for translating from English (en) to Turkish (tr).
This model is part of the [OPUS-MT project](https://github.com/Helsinki-NLP/Opus-MT), an effort to make neural machine translation models widely available and accessible for many languages in the world. All models are originally trained using the amazing framework of [Marian NMT](https://marian-nmt.github.io/), an efficient NMT implementation written in pure C++. The models have been converted to pyTorch using the transformers library by huggingface. Training data is taken from [OPUS](https://opus.nlpl.eu/) and training pipelines use the procedures of [OPUS-MT-train](https://github.com/Helsinki-NLP/Opus-MT-train).
* Publications: [OPUS-MT – Building open translation services for the World](https://aclanthology.org/2020.eamt-1.61/) and [The Tatoeba Translation Challenge – Realistic Data Sets for Low Resource and Multilingual MT](https://aclanthology.org/2020.wmt-1.139/) (Please, cite if you use this model.)
```
@inproceedings{tiedemann-thottingal-2020-opus,
title = "{OPUS}-{MT} {--} Building open translation services for the World",
author = {Tiedemann, J{\"o}rg and Thottingal, Santhosh},
booktitle = "Proceedings of the 22nd Annual Conference of the European Association for Machine Translation",
month = nov,
year = "2020",
address = "Lisboa, Portugal",
publisher = "European Association for Machine Translation",
url = "https://aclanthology.org/2020.eamt-1.61",
pages = "479--480",
}
@inproceedings{tiedemann-2020-tatoeba,
title = "The Tatoeba Translation Challenge {--} Realistic Data Sets for Low Resource and Multilingual {MT}",
author = {Tiedemann, J{\"o}rg},
booktitle = "Proceedings of the Fifth Conference on Machine Translation",
month = nov,
year = "2020",
address = "Online",
publisher = "Association for Computational Linguistics",
url = "https://aclanthology.org/2020.wmt-1.139",
pages = "1174--1182",
}
```
## Model info
* Release: 2022-02-25
* source language(s): eng
* target language(s): tur
* model: transformer-big
* data: opusTCv20210807+bt ([source](https://github.com/Helsinki-NLP/Tatoeba-Challenge))
* tokenization: SentencePiece (spm32k,spm32k)
* original model: [opusTCv20210807+bt_transformer-big_2022-02-25.zip](https://object.pouta.csc.fi/Tatoeba-MT-models/eng-tur/opusTCv20210807+bt_transformer-big_2022-02-25.zip)
* more information released models: [OPUS-MT eng-tur README](https://github.com/Helsinki-NLP/Tatoeba-Challenge/tree/master/models/eng-tur/README.md)
## Usage
A short example code:
```python
from transformers import MarianMTModel, MarianTokenizer
src_text = [
"I know Tom didn't want to eat that.",
"On Sundays, we would get up early and go fishing."
]
model_name = "pytorch-models/opus-mt-tc-big-en-tr"
tokenizer = MarianTokenizer.from_pretrained(model_name)
model = MarianMTModel.from_pretrained(model_name)
translated = model.generate(**tokenizer(src_text, return_tensors="pt", padding=True))
for t in translated:
print( tokenizer.decode(t, skip_special_tokens=True) )
# expected output:
# Tom'un bunu yemek istemediğini biliyorum.
# Pazar günleri erkenden kalkıp balık tutmaya giderdik.
```
You can also use OPUS-MT models with the transformers pipelines, for example:
```python
from transformers import pipeline
pipe = pipeline("translation", model="Helsinki-NLP/opus-mt-tc-big-en-tr")
print(pipe("I know Tom didn't want to eat that."))
# expected output: Tom'un bunu yemek istemediğini biliyorum.
```
## Benchmarks
* test set translations: [opusTCv20210807+bt_transformer-big_2022-02-25.test.txt](https://object.pouta.csc.fi/Tatoeba-MT-models/eng-tur/opusTCv20210807+bt_transformer-big_2022-02-25.test.txt)
* test set scores: [opusTCv20210807+bt_transformer-big_2022-02-25.eval.txt](https://object.pouta.csc.fi/Tatoeba-MT-models/eng-tur/opusTCv20210807+bt_transformer-big_2022-02-25.eval.txt)
* benchmark results: [benchmark_results.txt](benchmark_results.txt)
* benchmark output: [benchmark_translations.zip](benchmark_translations.zip)
| langpair | testset | chr-F | BLEU | #sent | #words |
|----------|---------|-------|-------|-------|--------|
| eng-tur | tatoeba-test-v2021-08-07 | 0.68726 | 42.3 | 13907 | 84364 |
| eng-tur | flores101-devtest | 0.62829 | 31.4 | 1012 | 20253 |
| eng-tur | newsdev2016 | 0.58947 | 21.9 | 1001 | 15958 |
| eng-tur | newstest2016 | 0.57624 | 23.4 | 3000 | 50782 |
| eng-tur | newstest2017 | 0.58858 | 25.4 | 3007 | 51977 |
| eng-tur | newstest2018 | 0.57848 | 22.6 | 3000 | 53731 |
## Acknowledgements
The work is supported by the [European Language Grid](https://www.european-language-grid.eu/) as [pilot project 2866](https://live.european-language-grid.eu/catalogue/#/resource/projects/2866), by the [FoTran project](https://www.helsinki.fi/en/researchgroups/natural-language-understanding-with-cross-lingual-grounding), funded by the European Research Council (ERC) under the European Union’s Horizon 2020 research and innovation programme (grant agreement No 771113), and the [MeMAD project](https://memad.eu/), funded by the European Union’s Horizon 2020 Research and Innovation Programme under grant agreement No 780069. We are also grateful for the generous computational resources and IT infrastructure provided by [CSC -- IT Center for Science](https://www.csc.fi/), Finland.
## Model conversion info
* transformers version: 4.16.2
* OPUS-MT git hash: 3405783
* port time: Wed Apr 13 18:11:39 EEST 2022
* port machine: LM0-400-22516.local
| [
-0.027986979112029076,
-0.03167511522769928,
-0.0033575885463505983,
0.0027195753064006567,
0.026706408709287643,
-0.012718847952783108,
0.02529796026647091,
-0.026579199358820915,
0.027986768633127213,
-0.021980924531817436,
0.00827842392027378,
-0.163065567612648,
-0.02755395881831646,
-0.026454510167241096,
-0.018146811053156853,
-0.04358651116490364,
-0.04521859064698219,
-0.029275916516780853,
-0.07922603189945221,
-0.11339493095874786,
0.014955463819205761,
0.08683536946773529,
0.03218516334891319,
0.011351463384926319,
0.030843498185276985,
0.00789207685738802,
-0.1179218515753746,
0.003485017688944936,
-0.016013972461223602,
-0.07390624284744263,
-0.007445275317877531,
0.10778167843818665,
-0.002141897566616535,
0.038432005792856216,
0.07348674535751343,
0.1100495383143425,
-0.06634164601564407,
-0.0350036695599556,
-0.014066116884350777,
-0.030149592086672783,
0.002615937264636159,
0.01341820228844881,
0.07376554608345032,
-0.05485406517982483,
0.03554701805114746,
-0.06849592924118042,
-0.06588739901781082,
-0.058366674929857254,
0.037006277590990067,
0.04909554123878479,
-0.13829630613327026,
0.043826598674058914,
-0.05309535562992096,
0.04919777065515518,
0.03401516377925873,
0.011459378525614738,
-0.07683129608631134,
-0.010937361046671867,
0.08834794163703918,
-0.05085603892803192,
-0.05863119289278984,
-0.015999704599380493,
-0.0639122873544693,
0.0020295651629567146,
-0.028846489265561104,
-0.025083158165216446,
0.00043527749949134886,
0.042619504034519196,
-0.046782903373241425,
0.07462530583143234,
-0.002437159651890397,
0.045678190886974335,
-0.06592818349599838,
0.08582020550966263,
0.0017232035752385855,
0.02809666283428669,
0.010800374671816826,
-0.010631941258907318,
0.03940858319401741,
-0.10349807888269424,
-0.025295112282037735,
-0.02012750878930092,
0.011399672366678715,
-0.01591394655406475,
0.04893948510289192,
-0.02846105955541134,
0.054895490407943726,
-0.008620287291705608,
0.01650688238441944,
-0.010480789467692375,
0.013350863009691238,
-0.015941325575113297,
-0.012604320421814919,
0.0776282474398613,
0.0034604135435074568,
0.0008342875516973436,
0.0029367131646722555,
0.07241680473089218,
0.014193267561495304,
0.08192698657512665,
-0.0032153711654245853,
0.045017555356025696,
0.03535999357700348,
0.029163895174860954,
-0.07871899753808975,
-0.012500028125941753,
0.03031829185783863,
0.043442174792289734,
-0.01609855145215988,
-0.030021077021956444,
0.003395967883989215,
0.031708840280771255,
0.005740190856158733,
-0.04897806793451309,
0.0176395196467638,
-0.01720833219587803,
-0.030758628621697426,
-0.031505655497312546,
0.007910395972430706,
0.0860326886177063,
-0.01831263117492199,
-0.0031062988564372063,
-0.03820699080824852,
-0.02764885686337948,
-0.06939013302326202,
-0.0031825422775000334,
0.006718805525451899,
1.1498012034543801e-32,
0.07653703540563583,
-0.004336502403020859,
0.07007759809494019,
-0.025370532646775246,
0.01250851433724165,
-0.025849442929029465,
-0.06709180772304535,
0.05915834382176399,
-0.10032331198453903,
-0.001921023242175579,
-0.07374368607997894,
-0.01410975493490696,
-0.10007819533348083,
0.0058073303662240505,
0.048486482352018356,
-0.03292011097073555,
0.0036710838321596384,
0.025310475379228592,
-0.03026479110121727,
0.050517670810222626,
0.060629211366176605,
-0.015111476182937622,
-0.02143450640141964,
-0.04387066513299942,
0.003848320571705699,
0.06109493225812912,
0.0437876358628273,
-0.07254815846681595,
-0.08399713784456253,
0.04792589321732521,
-0.06755109131336212,
0.019939441233873367,
-0.044366367161273956,
0.010823325254023075,
0.006887252442538738,
-0.06609226763248444,
0.0005996658583171666,
-0.0008266547811217606,
-0.01645359955728054,
-0.003204731270670891,
0.004076886456459761,
0.04548560082912445,
-0.08839491009712219,
-0.03238259628415108,
0.008575295098125935,
-0.02267151139676571,
0.03653206676244736,
-0.02181849256157875,
0.053725916892290115,
0.0192842036485672,
-0.02720741555094719,
0.0016723546432331204,
-0.036413948982954025,
-0.0317629873752594,
-0.018962042406201363,
0.03892180696129799,
0.051918186247348785,
0.0627615749835968,
0.0098638366907835,
0.02050926350057125,
-0.009082367643713951,
0.03786304220557213,
0.03650152310729027,
0.006714909803122282,
0.09837640076875687,
-0.0010085083777084947,
-0.000021750101950601675,
-0.025918763130903244,
0.06541416049003601,
-0.012844597920775414,
-0.04510369896888733,
-0.08465045690536499,
0.05489141866564751,
0.06763970851898193,
0.1397312432527542,
-0.014971481636166573,
0.012872911058366299,
-0.0654413253068924,
-0.005329070147126913,
0.015661325305700302,
-0.0606716014444828,
0.011191820725798607,
-0.011142013594508171,
-0.06067289784550667,
-0.009779202751815319,
-0.020839925855398178,
0.06181424856185913,
-0.04060646891593933,
-0.04064740985631943,
-0.020194003358483315,
-0.04808276146650314,
0.07782337814569473,
-0.09125904738903046,
-0.03780463710427284,
-0.0829806700348854,
-1.1306190458272805e-32,
0.0062921177595853806,
0.04625711590051651,
-0.004614903125911951,
0.0313383974134922,
0.004999659024178982,
-0.08374829590320587,
0.10490182787179947,
0.05600385740399361,
0.11123908311128616,
0.02049649879336357,
0.051305510103702545,
-0.12481710314750671,
0.036476071923971176,
-0.0380854494869709,
0.05603990703821182,
-0.013487451709806919,
0.006020266562700272,
-0.021105995401740074,
-0.05366230010986328,
0.07056572288274765,
-0.07273901998996735,
0.0938025563955307,
-0.06368837505578995,
0.08819890022277832,
-0.02150004729628563,
0.007095520384609699,
-0.025499887764453888,
0.016543740406632423,
0.027094915509223938,
-0.062485963106155396,
0.006977941375225782,
-0.01290410291403532,
-0.11494681984186172,
0.05176973715424538,
-0.07417552918195724,
0.0032692730892449617,
0.03819212689995766,
0.0017167461337521672,
-0.02152777649462223,
0.14575091004371643,
0.05944281816482544,
0.08489912748336792,
-0.0708928257226944,
-0.036892764270305634,
0.011855843476951122,
-0.013754191808402538,
0.014572955667972565,
0.02889254502952099,
-0.04068486765027046,
-0.0752076581120491,
0.09965207427740097,
0.045699987560510635,
-0.0786939412355423,
-0.017705386504530907,
0.018492309376597404,
-0.0806533619761467,
-0.007867608219385147,
-0.1270216852426529,
-0.11091002076864243,
-0.06552762538194656,
0.018965182825922966,
-0.024213077500462532,
0.0036661347839981318,
-0.049705229699611664,
0.1015942394733429,
0.028098059818148613,
-0.00006112203118391335,
-0.034287042915821075,
-0.00047630866174586117,
0.015333186835050583,
0.014261338859796524,
-0.051879093050956726,
0.029394205659627914,
-0.017718585208058357,
0.045536644756793976,
-0.0006566533702425659,
-0.07289719581604004,
-0.016840778291225433,
0.07424396276473999,
-0.02272152341902256,
-0.08877184242010117,
0.04734509065747261,
0.08172757923603058,
0.019489288330078125,
-0.023871323093771935,
0.06481542438268661,
-0.008360403589904308,
0.07754845172166824,
0.03781166300177574,
0.06540917605161667,
-0.019402548670768738,
-0.044357363134622574,
0.0076790121383965015,
0.12333008646965027,
-0.010703678242862225,
-6.228386695283916e-8,
-0.056365858763456345,
-0.009465239942073822,
-0.12628397345542908,
0.06657064706087112,
-0.039193328469991684,
-0.015649445354938507,
-0.06066722422838211,
0.0013812134275212884,
0.04535182565450668,
-0.03500109165906906,
0.026152867823839188,
-0.004332997370511293,
-0.08711820095777512,
0.025949200615286827,
0.017357362434267998,
-0.02776765078306198,
-0.02145479992032051,
0.11058901250362396,
-0.025456884875893593,
-0.06426352262496948,
0.05517084151506424,
0.05189172178506851,
-0.004136556759476662,
-0.04465089738368988,
0.04173542186617851,
-0.019889602437615395,
-0.026502320542931557,
0.027999484911561012,
0.03474272042512894,
0.00042221948388032615,
0.053079038858413696,
-0.039111677557229996,
-0.03240811824798584,
-0.057774078100919724,
0.07834666222333908,
0.023244069889187813,
-0.010828856378793716,
0.033326275646686554,
0.01778707094490528,
0.06725253164768219,
0.0403192900121212,
0.046061038970947266,
-0.09885360300540924,
0.04389570280909538,
0.01253118459135294,
-0.03773673251271248,
-0.06100030615925789,
0.01996324583888054,
0.041243840008974075,
-0.07344275712966919,
0.03391018509864807,
-0.07625768333673477,
-0.05595686286687851,
-0.0019439877942204475,
0.06319296360015869,
0.032763008028268814,
0.021591980010271072,
0.0019389452645555139,
-0.0027382373809814453,
-0.07771018147468567,
0.08158725500106812,
-0.03401469066739082,
-0.09139243513345718,
-0.0320136584341526
] |
hf-internal-testing/tiny-layoutlm | 7bc6366344bf3e7363a5e0e2f4fdd3087ab68e4a | 2021-08-04T04:33:04.000Z | [
"pytorch",
"layoutlm",
"fill-mask",
"transformers",
"autotrain_compatible"
] | fill-mask | false | hf-internal-testing | null | hf-internal-testing/tiny-layoutlm | 1,992 | null | transformers | This is a tiny-layoutlm random model to be used for basic testing.
| [
-0.03318405523896217,
0.0012343220878392458,
-0.07536199688911438,
0.0014319210313260555,
0.014109555631875992,
-0.0634647086262703,
-0.014394184574484825,
-0.01187045220285654,
0.0069629424251616,
0.011518323794007301,
0.06769188493490219,
-0.0023834644816815853,
0.05398649722337723,
0.006080150604248047,
-0.11198654770851135,
-0.043609391897916794,
0.03979593515396118,
-0.014882856979966164,
-0.03473272547125816,
0.012141297571361065,
-0.01717432029545307,
0.019509905949234962,
-0.025610817596316338,
-0.0063265105709433556,
-0.02091010846197605,
-0.06226439028978348,
0.07435228675603867,
0.06334151327610016,
0.06253356486558914,
-0.10538532584905624,
0.08568593859672546,
0.07708388566970825,
0.06827837973833084,
0.02790968492627144,
0.052872154861688614,
-0.03585398197174072,
-0.03986323997378349,
-0.006604828406125307,
-0.006966238841414452,
0.028111563995480537,
-0.04290612041950226,
-0.06135588139295578,
0.076189324259758,
0.04440850019454956,
0.03382571414113045,
0.005247427150607109,
-0.07580521702766418,
-0.06926772743463516,
-0.03936469554901123,
-0.008900225162506104,
-0.010195931419730186,
-0.03977265954017639,
-0.06731283664703369,
-0.01512584276497364,
0.017803682014346123,
-0.042924780398607254,
-0.008263788186013699,
-0.0877036526799202,
-0.04229407012462616,
0.05791078507900238,
0.04826287552714348,
-0.015413089655339718,
-0.05948527529835701,
0.061493586748838425,
0.05428450182080269,
0.07026541233062744,
-0.033678166568279266,
-0.013624027371406555,
0.045024774968624115,
-0.13228167593479156,
-0.08965794742107391,
0.006442916113883257,
0.009985986165702343,
0.10393153131008148,
0.059971362352371216,
-0.002757210750132799,
-0.04786556214094162,
-0.06723368167877197,
0.04710371047258377,
-0.017627723515033722,
-0.11305058002471924,
-0.023314738646149635,
0.010068015195429325,
0.041044287383556366,
0.03743699938058853,
0.0439995676279068,
0.09088148921728134,
0.04343879967927933,
0.02533509023487568,
-0.0048330845311284065,
-0.015140905976295471,
0.08836447447538376,
-0.06380189955234528,
0.021481933072209358,
-0.08569582551717758,
0.04138382896780968,
0.05970973148941994,
-0.11803388595581055,
-0.007732028141617775,
0.0812426432967186,
0.033515557646751404,
-0.018740298226475716,
0.12951454520225525,
0.011486977338790894,
-0.00990112405270338,
-0.09604383260011673,
0.08825619518756866,
-0.0522223599255085,
0.028866305947303772,
-0.05246049165725708,
-0.018145252019166946,
0.03893425688147545,
-0.06698814779520035,
-0.0031982806976884604,
-0.018952149897813797,
-0.10232043266296387,
-0.04490627720952034,
0.014336276799440384,
-0.0058431476354599,
0.04342436417937279,
0.056171804666519165,
0.0404573529958725,
-0.08713573962450027,
-0.06967293471097946,
-0.048027053475379944,
0.038587406277656555,
-0.007200327236205339,
-2.360956342726571e-33,
0.047714512795209885,
-0.00398619519546628,
-0.019894862547516823,
0.0890006348490715,
0.11287137120962143,
0.044002894312143326,
-0.00971592590212822,
0.022316593676805496,
-0.00046509585808962584,
0.03498026356101036,
0.0010246700840070844,
-0.02391897328197956,
-0.026361413300037384,
0.08548688888549805,
0.03073832392692566,
-0.03203701972961426,
-0.040140122175216675,
0.10377375036478043,
-0.029184211045503616,
0.016858641058206558,
0.022334666922688484,
0.01069451030343771,
0.021978529170155525,
-0.08412621915340424,
0.05649914965033531,
0.06448538601398468,
0.0024488007184118032,
-0.0061989822424948215,
-0.004201582167297602,
0.05310634523630142,
-0.05068754404783249,
0.05642734467983246,
-0.10006444901227951,
-0.006924056448042393,
-0.009647014550864697,
0.005732821300625801,
-0.0026096461806446314,
-0.03011990152299404,
-0.03336043283343315,
-0.02737361565232277,
-0.007282678037881851,
-0.053035981953144073,
0.027753259986639023,
-0.04222765192389488,
-0.048236578702926636,
-0.012945994734764099,
0.016767116263508797,
0.014358626678586006,
0.058442432433366776,
-0.04682460054755211,
0.01853475160896778,
0.017879635095596313,
0.037219755351543427,
-0.025410864502191544,
-0.012337742373347282,
0.026641152799129486,
0.032606761902570724,
0.0046071759425103664,
-0.01091225165873766,
0.10704806447029114,
-0.05552608519792557,
0.04154875501990318,
-0.05488951504230499,
0.03806711733341217,
0.014960577711462975,
0.02749808132648468,
-0.07152828574180603,
-0.17061443626880646,
0.07970163226127625,
0.04843365028500557,
0.029922176152467728,
-0.0023705651983618736,
0.0005511559429578483,
0.037894513458013535,
-0.06552314013242722,
-0.026404473930597305,
0.010532468557357788,
-0.004747261758893728,
-0.052810680121183395,
-0.018932223320007324,
-0.017709510400891304,
-0.025200363248586655,
-0.0035321912728250027,
-0.10356905311346054,
-0.1175520122051239,
-0.036152396351099014,
0.02641826868057251,
-0.0554284006357193,
-0.09609292447566986,
-0.08927319198846817,
-0.01632019504904747,
-0.0053239855915308,
0.03001469559967518,
0.010500342585146427,
0.04616227000951767,
7.274842041183874e-34,
-0.11753882467746735,
0.032999467104673386,
0.027950037270784378,
0.09395494312047958,
0.04687255248427391,
-0.04147868603467941,
0.03725219517946243,
0.04787065088748932,
0.012726168148219585,
0.051597412675619125,
0.004441327415406704,
0.047333668917417526,
0.017043253406882286,
-0.02440822310745716,
0.03523138538002968,
0.0398077555000782,
-0.006186674349009991,
-0.10126906633377075,
0.030398186296224594,
0.011106587946414948,
0.01638253778219223,
0.11788105964660645,
-0.09878220409154892,
-0.01830233447253704,
0.010235436260700226,
0.04156108945608139,
-0.013332098722457886,
0.0375269316136837,
0.006326044909656048,
0.017016280442476273,
-0.08509556204080582,
-0.02033464051783085,
0.015509874559938908,
-0.0025675345677882433,
-0.0003560149052646011,
-0.023801852017641068,
0.08082840591669083,
-0.012445768341422081,
0.011002453044056892,
-0.02447778917849064,
-0.002758831949904561,
0.031419165432453156,
-0.015951281413435936,
0.025521960109472275,
-0.016913708299398422,
0.04852579906582832,
0.0394783578813076,
0.0010432401904836297,
0.052936770021915436,
-0.010037699714303017,
-0.015504103153944016,
0.04627273976802826,
-0.03224792703986168,
-0.0017457903595641255,
-0.07656901329755783,
0.033388152718544006,
-0.1292300671339035,
0.02312132529914379,
0.019843734800815582,
0.05799457058310509,
-0.03718588501214981,
-0.02666494995355606,
-0.0173113364726305,
0.026038426905870438,
-0.014940072782337666,
-0.01422030571848154,
-0.03362421318888664,
-0.07554532587528229,
-0.04163643717765808,
0.06455931812524796,
0.07164648920297623,
0.06633193045854568,
0.02607349492609501,
0.007195749320089817,
0.014833459630608559,
-0.15351158380508423,
-0.0001446988753741607,
-0.014824788086116314,
0.008295158855617046,
0.017131786793470383,
0.017529336735606194,
-0.0697018951177597,
0.020611559972167015,
0.007440756540745497,
0.013232029043138027,
-0.0405438095331192,
0.014181981794536114,
0.05688364803791046,
0.02975742518901825,
0.06575821340084076,
-0.07401331514120102,
0.09996472299098969,
0.026372363790869713,
0.04528648406267166,
-0.04350852593779564,
-2.0624961294402056e-8,
0.048095449805259705,
-0.09161525964736938,
0.04450240358710289,
0.01450835820287466,
-0.03276071324944496,
0.049854643642902374,
0.02354777231812477,
-0.04572976008057594,
-0.018987352028489113,
-0.06682020425796509,
0.03563378378748894,
0.03718213364481926,
-0.09036702662706375,
0.037246499210596085,
0.015414698049426079,
0.0294790156185627,
-0.02813219092786312,
0.04989713057875633,
-0.0491945743560791,
-0.0262227151542902,
0.0071798572316765785,
0.04792073369026184,
0.06174562871456146,
-0.04495185241103172,
-0.01993047632277012,
0.011165859177708626,
0.004644801374524832,
0.09553325176239014,
0.021647723391652107,
-0.08636597543954849,
0.04748916253447533,
0.02910204790532589,
0.04402901977300644,
0.019573470577597618,
-0.019922038540244102,
0.20505249500274658,
-0.05470672622323036,
0.01709340512752533,
0.008265708573162556,
-0.00022161667584441602,
-0.020605988800525665,
-0.09354467689990997,
-0.0053907353430986404,
0.01133069209754467,
-0.004588003270328045,
-0.016102883964776993,
-0.03033267892897129,
-0.11080849915742874,
0.050052717328071594,
-0.04454699158668518,
-0.020472394302487373,
0.008341453969478607,
-0.04132016748189926,
-0.012685125693678856,
-0.02768346294760704,
0.09131579101085663,
0.03547540679574013,
-0.008644286543130875,
0.04473497346043587,
-0.001493514166213572,
0.011568427085876465,
0.0898667648434639,
-0.023697897791862488,
-0.033647969365119934
] |
hf-internal-testing/tiny-random-gpt_neo | b95a8110971dfc560caa02c286c3b8aa0118941a | 2021-09-17T19:25:26.000Z | [
"pytorch",
"gpt_neo",
"transformers"
] | null | false | hf-internal-testing | null | hf-internal-testing/tiny-random-gpt_neo | 1,992 | null | transformers | Entry not found | [
0.0461147278547287,
-0.038838207721710205,
-0.01049656979739666,
-0.03682169318199158,
0.011261860840022564,
0.013094935566186905,
0.0019101888174191117,
-0.013979103416204453,
0.027092741802334785,
-0.015212527476251125,
0.017284274101257324,
-0.08189476281404495,
0.03817418962717056,
-0.04920130595564842,
0.021389011293649673,
-0.015245908871293068,
-0.03203780576586723,
-0.1245758980512619,
0.03150877356529236,
0.032381657510995865,
-0.060957908630371094,
0.05409295856952667,
-0.025087490677833557,
0.01568586938083172,
0.028129950165748596,
-0.04710396006703377,
-0.018688226118683815,
0.013785239309072495,
-0.04001208767294884,
0.01173911802470684,
-0.04317743331193924,
0.05500618368387222,
0.004543041344732046,
0.02973111905157566,
0.14852192997932434,
0.02658126689493656,
0.02907961793243885,
-0.05169107764959335,
0.05803573504090309,
-0.07732241600751877,
-0.017637968063354492,
-0.04219653457403183,
0.041807834059000015,
0.023620979860424995,
0.021563321352005005,
0.016478516161441803,
-0.0021814992651343346,
-0.06400240957736969,
0.06393089145421982,
0.019599027931690216,
-0.08565037697553635,
0.00934905931353569,
-0.008718925528228283,
-0.028583496809005737,
-0.07310017943382263,
0.09416428208351135,
0.001759322709403932,
0.06184990331530571,
0.011840506456792355,
-0.035997264087200165,
0.08358278125524521,
-0.02619801089167595,
0.03736566752195358,
-0.028206506744027138,
-0.07454850524663925,
-0.08883563429117203,
-0.06279942393302917,
-0.008695344440639019,
0.014119276776909828,
-0.0825355276465416,
0.0649217739701271,
-0.00223911227658391,
-0.14716917276382446,
0.07743025571107864,
-0.03548373281955719,
-0.055201586335897446,
0.006981803569942713,
-0.012166670523583889,
0.055111464112997055,
-0.007116836030036211,
-0.023175746202468872,
-0.005835152696818113,
-0.09185640513896942,
0.055196937173604965,
0.034148022532463074,
0.03835180774331093,
0.038685429841279984,
-0.025987252593040466,
0.017804903909564018,
0.022428328171372414,
0.025005368515849113,
-0.10761535167694092,
-0.048001550137996674,
-0.04343584179878235,
0.012374646961688995,
-0.019502125680446625,
0.029218152165412903,
0.0842173621058464,
-0.011719699949026108,
0.09283553808927536,
-0.007015465293079615,
-0.03543110564351082,
-0.06936459988355637,
0.09425332397222519,
-0.010958523489534855,
-0.00805904995650053,
0.004974212497472763,
-0.0031528924591839314,
0.06105927750468254,
-0.03964288905262947,
-0.03619541600346565,
-0.019901901483535767,
0.07134733349084854,
0.039514873176813126,
-0.012729483656585217,
-0.006646515801548958,
-0.04746140539646149,
-0.014432490803301334,
-0.05157482624053955,
0.09506245702505112,
-0.049747664481401443,
-0.04591796174645424,
-0.008965466171503067,
-0.0325421579182148,
-0.08626784384250641,
-0.06624380499124527,
0.02538885548710823,
-4.303924894057984e-33,
0.01133066974580288,
0.0033434738870710135,
-0.002155609894543886,
0.04871906340122223,
-0.023564351722598076,
-0.07933273911476135,
0.0600903145968914,
0.02335330657660961,
-0.03844716399908066,
-0.020433755591511726,
-0.06952055543661118,
-0.03235611692070961,
0.0062485747039318085,
0.064804308116436,
-0.03201229125261307,
0.061689723283052444,
0.0417000837624073,
-0.00761845987290144,
0.03340127319097519,
-0.047770582139492035,
0.00887306872755289,
-0.04066338762640953,
-0.010506896302103996,
0.0106519665569067,
0.021333497017621994,
0.12854498624801636,
-0.009705503471195698,
0.010055632330477238,
-0.017507633194327354,
0.006515394430607557,
0.06334009766578674,
-0.057817306369543076,
0.013668818399310112,
-0.020286159589886665,
0.05430467426776886,
-0.023184705525636673,
0.0828516036272049,
0.0005449643940664828,
-0.10372652113437653,
-0.07634282112121582,
-0.005381610710173845,
-0.039263784885406494,
0.0006114727002568543,
-0.013281986117362976,
0.07119110971689224,
0.043696220964193344,
0.03168422728776932,
0.04338686540722847,
0.05728672817349434,
0.0832006186246872,
-0.07961414009332657,
0.015234283171594143,
0.017002005130052567,
0.047004107385873795,
-0.09794387966394424,
0.004990279674530029,
-0.07062993198633194,
-0.028000490739941597,
-0.04018733277916908,
-0.0702052190899849,
0.011351344175636768,
0.06020182743668556,
-0.03297270089387894,
0.09396500885486603,
0.03417910635471344,
-0.019825750961899757,
-0.034690454602241516,
-0.013036907650530338,
0.05896938592195511,
-0.012359356507658958,
-0.017275206744670868,
-0.07982361316680908,
0.02059139870107174,
0.06737419217824936,
0.04176458343863487,
-0.04978838190436363,
-0.05877475067973137,
-0.06289287656545639,
-0.03354167565703392,
-0.03871942684054375,
0.009898529388010502,
-0.05514208599925041,
-0.11629002541303635,
-0.011855563148856163,
0.10663620382547379,
0.037354156374931335,
-0.0065480442717671394,
-0.051189567893743515,
0.06663123518228531,
0.01874656230211258,
0.032841797918081284,
0.041593004018068314,
-0.06879369914531708,
0.04216769337654114,
-0.01628219522535801,
5.4139394340936695e-34,
0.05697013810276985,
-0.006972255185246468,
0.015711724758148193,
-0.17956365644931793,
0.02320219948887825,
0.007923615165054798,
-0.008062449283897877,
0.0074974060989916325,
0.07391711324453354,
0.0309313777834177,
0.060510627925395966,
0.058605875819921494,
0.09515274316072464,
-0.002282935893163085,
0.001603541080839932,
0.07024981826543808,
0.012629246339201927,
0.07425693422555923,
-0.038426291197538376,
0.01861148327589035,
0.030608950182795525,
-0.02449394389986992,
0.021528491750359535,
-0.003039651783183217,
-0.03676343336701393,
0.03130284696817398,
0.07998586446046829,
0.010451192036271095,
-0.07930229604244232,
-0.013543923385441303,
0.018781835213303566,
0.05168003588914871,
-0.07191970944404602,
0.15783067047595978,
0.026191607117652893,
0.01262354850769043,
0.08218053728342056,
-0.029807550832629204,
-0.07528624683618546,
-0.04250097647309303,
0.017244765534996986,
0.04411793500185013,
0.03708017244935036,
0.009233047254383564,
-0.040271829813718796,
0.022496428340673447,
0.02495843544602394,
0.07633638381958008,
0.005147108342498541,
0.013892097398638725,
0.05610476806759834,
-0.06684739887714386,
0.05862557515501976,
-0.020688841119408607,
0.05377643182873726,
0.06718500703573227,
0.005329249892383814,
-0.01388032827526331,
0.029931528493762016,
0.009508464485406876,
-0.045173756778240204,
0.11534366756677628,
-0.06510116159915924,
0.05117698386311531,
-0.0026125339791178703,
-0.08554837852716446,
-0.03784770518541336,
0.0804959163069725,
0.011298024095594883,
-0.07695550471544266,
-0.04868878796696663,
0.02515520341694355,
0.06252261996269226,
-0.04509226232767105,
-0.01246943511068821,
0.028559505939483643,
-0.030573077499866486,
0.05066261067986488,
-0.08187384903430939,
0.04469604790210724,
0.0034051244147121906,
0.04145054519176483,
-0.021858664229512215,
-0.06112268194556236,
-0.00908052921295166,
-0.05903250351548195,
0.0259539932012558,
0.059690944850444794,
-0.07613514363765717,
-0.03720718249678612,
-0.036316655576229095,
0.07058046013116837,
-0.008224100805819035,
0.041961874812841415,
-0.0285952128469944,
-1.496900736697171e-8,
-0.0014124972512945533,
0.03401879221200943,
-0.040338415652513504,
0.04116074740886688,
0.0935964286327362,
-0.05115952715277672,
0.0008746005478315055,
-0.03389839455485344,
-0.00567849725484848,
-0.010686947964131832,
-0.04789939522743225,
-0.04820054769515991,
-0.02011880651116371,
-0.03209094703197479,
-0.04211259260773659,
-0.10229527950286865,
-0.07819421589374542,
-0.031228765845298767,
-0.02154778689146042,
-0.04960230365395546,
0.08087796717882156,
-0.07801242172718048,
0.06919731199741364,
-0.04999840259552002,
0.03687043860554695,
0.03889009356498718,
-0.049989692866802216,
-0.04254625365138054,
-0.04606937617063522,
0.08682432025671005,
-0.031148413196206093,
0.11826753616333008,
0.034102488309144974,
-0.0208592489361763,
-0.0205202866345644,
0.027134142816066742,
0.09741277992725372,
0.051608603447675705,
0.013477512635290623,
-0.13649295270442963,
-0.022304272279143333,
0.02385953813791275,
0.038732077926397324,
-0.09249968826770782,
-0.04549082741141319,
0.054220106452703476,
0.01160438358783722,
0.051190607249736786,
0.07713303714990616,
-0.022097084671258926,
-0.06127818301320076,
-0.01857956498861313,
0.006740490905940533,
-0.00496308971196413,
0.024095389991998672,
0.0736224576830864,
-0.003481915919110179,
-0.0699305310845375,
-0.006629763171076775,
-0.0598808117210865,
0.05297163128852844,
-0.02902800403535366,
-0.027858933433890343,
-0.01287526823580265
] |
hf-internal-testing/tiny-random-led | 2774e58f25d3fdda4c0d86b140cca8e049ee6a9f | 2021-09-17T19:24:21.000Z | [
"pytorch",
"tf",
"led",
"transformers"
] | null | false | hf-internal-testing | null | hf-internal-testing/tiny-random-led | 1,992 | null | transformers | Entry not found | [
0.0461147278547287,
-0.038838207721710205,
-0.01049656979739666,
-0.03682169318199158,
0.011261860840022564,
0.013094935566186905,
0.0019101888174191117,
-0.013979103416204453,
0.027092741802334785,
-0.015212527476251125,
0.017284274101257324,
-0.08189476281404495,
0.03817418962717056,
-0.04920130595564842,
0.021389011293649673,
-0.015245908871293068,
-0.03203780576586723,
-0.1245758980512619,
0.03150877356529236,
0.032381657510995865,
-0.060957908630371094,
0.05409295856952667,
-0.025087490677833557,
0.01568586938083172,
0.028129950165748596,
-0.04710396006703377,
-0.018688226118683815,
0.013785239309072495,
-0.04001208767294884,
0.01173911802470684,
-0.04317743331193924,
0.05500618368387222,
0.004543041344732046,
0.02973111905157566,
0.14852192997932434,
0.02658126689493656,
0.02907961793243885,
-0.05169107764959335,
0.05803573504090309,
-0.07732241600751877,
-0.017637968063354492,
-0.04219653457403183,
0.041807834059000015,
0.023620979860424995,
0.021563321352005005,
0.016478516161441803,
-0.0021814992651343346,
-0.06400240957736969,
0.06393089145421982,
0.019599027931690216,
-0.08565037697553635,
0.00934905931353569,
-0.008718925528228283,
-0.028583496809005737,
-0.07310017943382263,
0.09416428208351135,
0.001759322709403932,
0.06184990331530571,
0.011840506456792355,
-0.035997264087200165,
0.08358278125524521,
-0.02619801089167595,
0.03736566752195358,
-0.028206506744027138,
-0.07454850524663925,
-0.08883563429117203,
-0.06279942393302917,
-0.008695344440639019,
0.014119276776909828,
-0.0825355276465416,
0.0649217739701271,
-0.00223911227658391,
-0.14716917276382446,
0.07743025571107864,
-0.03548373281955719,
-0.055201586335897446,
0.006981803569942713,
-0.012166670523583889,
0.055111464112997055,
-0.007116836030036211,
-0.023175746202468872,
-0.005835152696818113,
-0.09185640513896942,
0.055196937173604965,
0.034148022532463074,
0.03835180774331093,
0.038685429841279984,
-0.025987252593040466,
0.017804903909564018,
0.022428328171372414,
0.025005368515849113,
-0.10761535167694092,
-0.048001550137996674,
-0.04343584179878235,
0.012374646961688995,
-0.019502125680446625,
0.029218152165412903,
0.0842173621058464,
-0.011719699949026108,
0.09283553808927536,
-0.007015465293079615,
-0.03543110564351082,
-0.06936459988355637,
0.09425332397222519,
-0.010958523489534855,
-0.00805904995650053,
0.004974212497472763,
-0.0031528924591839314,
0.06105927750468254,
-0.03964288905262947,
-0.03619541600346565,
-0.019901901483535767,
0.07134733349084854,
0.039514873176813126,
-0.012729483656585217,
-0.006646515801548958,
-0.04746140539646149,
-0.014432490803301334,
-0.05157482624053955,
0.09506245702505112,
-0.049747664481401443,
-0.04591796174645424,
-0.008965466171503067,
-0.0325421579182148,
-0.08626784384250641,
-0.06624380499124527,
0.02538885548710823,
-4.303924894057984e-33,
0.01133066974580288,
0.0033434738870710135,
-0.002155609894543886,
0.04871906340122223,
-0.023564351722598076,
-0.07933273911476135,
0.0600903145968914,
0.02335330657660961,
-0.03844716399908066,
-0.020433755591511726,
-0.06952055543661118,
-0.03235611692070961,
0.0062485747039318085,
0.064804308116436,
-0.03201229125261307,
0.061689723283052444,
0.0417000837624073,
-0.00761845987290144,
0.03340127319097519,
-0.047770582139492035,
0.00887306872755289,
-0.04066338762640953,
-0.010506896302103996,
0.0106519665569067,
0.021333497017621994,
0.12854498624801636,
-0.009705503471195698,
0.010055632330477238,
-0.017507633194327354,
0.006515394430607557,
0.06334009766578674,
-0.057817306369543076,
0.013668818399310112,
-0.020286159589886665,
0.05430467426776886,
-0.023184705525636673,
0.0828516036272049,
0.0005449643940664828,
-0.10372652113437653,
-0.07634282112121582,
-0.005381610710173845,
-0.039263784885406494,
0.0006114727002568543,
-0.013281986117362976,
0.07119110971689224,
0.043696220964193344,
0.03168422728776932,
0.04338686540722847,
0.05728672817349434,
0.0832006186246872,
-0.07961414009332657,
0.015234283171594143,
0.017002005130052567,
0.047004107385873795,
-0.09794387966394424,
0.004990279674530029,
-0.07062993198633194,
-0.028000490739941597,
-0.04018733277916908,
-0.0702052190899849,
0.011351344175636768,
0.06020182743668556,
-0.03297270089387894,
0.09396500885486603,
0.03417910635471344,
-0.019825750961899757,
-0.034690454602241516,
-0.013036907650530338,
0.05896938592195511,
-0.012359356507658958,
-0.017275206744670868,
-0.07982361316680908,
0.02059139870107174,
0.06737419217824936,
0.04176458343863487,
-0.04978838190436363,
-0.05877475067973137,
-0.06289287656545639,
-0.03354167565703392,
-0.03871942684054375,
0.009898529388010502,
-0.05514208599925041,
-0.11629002541303635,
-0.011855563148856163,
0.10663620382547379,
0.037354156374931335,
-0.0065480442717671394,
-0.051189567893743515,
0.06663123518228531,
0.01874656230211258,
0.032841797918081284,
0.041593004018068314,
-0.06879369914531708,
0.04216769337654114,
-0.01628219522535801,
5.4139394340936695e-34,
0.05697013810276985,
-0.006972255185246468,
0.015711724758148193,
-0.17956365644931793,
0.02320219948887825,
0.007923615165054798,
-0.008062449283897877,
0.0074974060989916325,
0.07391711324453354,
0.0309313777834177,
0.060510627925395966,
0.058605875819921494,
0.09515274316072464,
-0.002282935893163085,
0.001603541080839932,
0.07024981826543808,
0.012629246339201927,
0.07425693422555923,
-0.038426291197538376,
0.01861148327589035,
0.030608950182795525,
-0.02449394389986992,
0.021528491750359535,
-0.003039651783183217,
-0.03676343336701393,
0.03130284696817398,
0.07998586446046829,
0.010451192036271095,
-0.07930229604244232,
-0.013543923385441303,
0.018781835213303566,
0.05168003588914871,
-0.07191970944404602,
0.15783067047595978,
0.026191607117652893,
0.01262354850769043,
0.08218053728342056,
-0.029807550832629204,
-0.07528624683618546,
-0.04250097647309303,
0.017244765534996986,
0.04411793500185013,
0.03708017244935036,
0.009233047254383564,
-0.040271829813718796,
0.022496428340673447,
0.02495843544602394,
0.07633638381958008,
0.005147108342498541,
0.013892097398638725,
0.05610476806759834,
-0.06684739887714386,
0.05862557515501976,
-0.020688841119408607,
0.05377643182873726,
0.06718500703573227,
0.005329249892383814,
-0.01388032827526331,
0.029931528493762016,
0.009508464485406876,
-0.045173756778240204,
0.11534366756677628,
-0.06510116159915924,
0.05117698386311531,
-0.0026125339791178703,
-0.08554837852716446,
-0.03784770518541336,
0.0804959163069725,
0.011298024095594883,
-0.07695550471544266,
-0.04868878796696663,
0.02515520341694355,
0.06252261996269226,
-0.04509226232767105,
-0.01246943511068821,
0.028559505939483643,
-0.030573077499866486,
0.05066261067986488,
-0.08187384903430939,
0.04469604790210724,
0.0034051244147121906,
0.04145054519176483,
-0.021858664229512215,
-0.06112268194556236,
-0.00908052921295166,
-0.05903250351548195,
0.0259539932012558,
0.059690944850444794,
-0.07613514363765717,
-0.03720718249678612,
-0.036316655576229095,
0.07058046013116837,
-0.008224100805819035,
0.041961874812841415,
-0.0285952128469944,
-1.496900736697171e-8,
-0.0014124972512945533,
0.03401879221200943,
-0.040338415652513504,
0.04116074740886688,
0.0935964286327362,
-0.05115952715277672,
0.0008746005478315055,
-0.03389839455485344,
-0.00567849725484848,
-0.010686947964131832,
-0.04789939522743225,
-0.04820054769515991,
-0.02011880651116371,
-0.03209094703197479,
-0.04211259260773659,
-0.10229527950286865,
-0.07819421589374542,
-0.031228765845298767,
-0.02154778689146042,
-0.04960230365395546,
0.08087796717882156,
-0.07801242172718048,
0.06919731199741364,
-0.04999840259552002,
0.03687043860554695,
0.03889009356498718,
-0.049989692866802216,
-0.04254625365138054,
-0.04606937617063522,
0.08682432025671005,
-0.031148413196206093,
0.11826753616333008,
0.034102488309144974,
-0.0208592489361763,
-0.0205202866345644,
0.027134142816066742,
0.09741277992725372,
0.051608603447675705,
0.013477512635290623,
-0.13649295270442963,
-0.022304272279143333,
0.02385953813791275,
0.038732077926397324,
-0.09249968826770782,
-0.04549082741141319,
0.054220106452703476,
0.01160438358783722,
0.051190607249736786,
0.07713303714990616,
-0.022097084671258926,
-0.06127818301320076,
-0.01857956498861313,
0.006740490905940533,
-0.00496308971196413,
0.024095389991998672,
0.0736224576830864,
-0.003481915919110179,
-0.0699305310845375,
-0.006629763171076775,
-0.0598808117210865,
0.05297163128852844,
-0.02902800403535366,
-0.027858933433890343,
-0.01287526823580265
] |
hf-internal-testing/tiny-random-deberta-v2 | 924b47948998e199d88e95e1df46ab125e0f325a | 2021-09-17T19:23:17.000Z | [
"pytorch",
"tf",
"deberta-v2",
"transformers"
] | null | false | hf-internal-testing | null | hf-internal-testing/tiny-random-deberta-v2 | 1,991 | null | transformers | Entry not found | [
0.0461147278547287,
-0.038838207721710205,
-0.01049656979739666,
-0.03682169318199158,
0.011261860840022564,
0.013094935566186905,
0.0019101888174191117,
-0.013979103416204453,
0.027092741802334785,
-0.015212527476251125,
0.017284274101257324,
-0.08189476281404495,
0.03817418962717056,
-0.04920130595564842,
0.021389011293649673,
-0.015245908871293068,
-0.03203780576586723,
-0.1245758980512619,
0.03150877356529236,
0.032381657510995865,
-0.060957908630371094,
0.05409295856952667,
-0.025087490677833557,
0.01568586938083172,
0.028129950165748596,
-0.04710396006703377,
-0.018688226118683815,
0.013785239309072495,
-0.04001208767294884,
0.01173911802470684,
-0.04317743331193924,
0.05500618368387222,
0.004543041344732046,
0.02973111905157566,
0.14852192997932434,
0.02658126689493656,
0.02907961793243885,
-0.05169107764959335,
0.05803573504090309,
-0.07732241600751877,
-0.017637968063354492,
-0.04219653457403183,
0.041807834059000015,
0.023620979860424995,
0.021563321352005005,
0.016478516161441803,
-0.0021814992651343346,
-0.06400240957736969,
0.06393089145421982,
0.019599027931690216,
-0.08565037697553635,
0.00934905931353569,
-0.008718925528228283,
-0.028583496809005737,
-0.07310017943382263,
0.09416428208351135,
0.001759322709403932,
0.06184990331530571,
0.011840506456792355,
-0.035997264087200165,
0.08358278125524521,
-0.02619801089167595,
0.03736566752195358,
-0.028206506744027138,
-0.07454850524663925,
-0.08883563429117203,
-0.06279942393302917,
-0.008695344440639019,
0.014119276776909828,
-0.0825355276465416,
0.0649217739701271,
-0.00223911227658391,
-0.14716917276382446,
0.07743025571107864,
-0.03548373281955719,
-0.055201586335897446,
0.006981803569942713,
-0.012166670523583889,
0.055111464112997055,
-0.007116836030036211,
-0.023175746202468872,
-0.005835152696818113,
-0.09185640513896942,
0.055196937173604965,
0.034148022532463074,
0.03835180774331093,
0.038685429841279984,
-0.025987252593040466,
0.017804903909564018,
0.022428328171372414,
0.025005368515849113,
-0.10761535167694092,
-0.048001550137996674,
-0.04343584179878235,
0.012374646961688995,
-0.019502125680446625,
0.029218152165412903,
0.0842173621058464,
-0.011719699949026108,
0.09283553808927536,
-0.007015465293079615,
-0.03543110564351082,
-0.06936459988355637,
0.09425332397222519,
-0.010958523489534855,
-0.00805904995650053,
0.004974212497472763,
-0.0031528924591839314,
0.06105927750468254,
-0.03964288905262947,
-0.03619541600346565,
-0.019901901483535767,
0.07134733349084854,
0.039514873176813126,
-0.012729483656585217,
-0.006646515801548958,
-0.04746140539646149,
-0.014432490803301334,
-0.05157482624053955,
0.09506245702505112,
-0.049747664481401443,
-0.04591796174645424,
-0.008965466171503067,
-0.0325421579182148,
-0.08626784384250641,
-0.06624380499124527,
0.02538885548710823,
-4.303924894057984e-33,
0.01133066974580288,
0.0033434738870710135,
-0.002155609894543886,
0.04871906340122223,
-0.023564351722598076,
-0.07933273911476135,
0.0600903145968914,
0.02335330657660961,
-0.03844716399908066,
-0.020433755591511726,
-0.06952055543661118,
-0.03235611692070961,
0.0062485747039318085,
0.064804308116436,
-0.03201229125261307,
0.061689723283052444,
0.0417000837624073,
-0.00761845987290144,
0.03340127319097519,
-0.047770582139492035,
0.00887306872755289,
-0.04066338762640953,
-0.010506896302103996,
0.0106519665569067,
0.021333497017621994,
0.12854498624801636,
-0.009705503471195698,
0.010055632330477238,
-0.017507633194327354,
0.006515394430607557,
0.06334009766578674,
-0.057817306369543076,
0.013668818399310112,
-0.020286159589886665,
0.05430467426776886,
-0.023184705525636673,
0.0828516036272049,
0.0005449643940664828,
-0.10372652113437653,
-0.07634282112121582,
-0.005381610710173845,
-0.039263784885406494,
0.0006114727002568543,
-0.013281986117362976,
0.07119110971689224,
0.043696220964193344,
0.03168422728776932,
0.04338686540722847,
0.05728672817349434,
0.0832006186246872,
-0.07961414009332657,
0.015234283171594143,
0.017002005130052567,
0.047004107385873795,
-0.09794387966394424,
0.004990279674530029,
-0.07062993198633194,
-0.028000490739941597,
-0.04018733277916908,
-0.0702052190899849,
0.011351344175636768,
0.06020182743668556,
-0.03297270089387894,
0.09396500885486603,
0.03417910635471344,
-0.019825750961899757,
-0.034690454602241516,
-0.013036907650530338,
0.05896938592195511,
-0.012359356507658958,
-0.017275206744670868,
-0.07982361316680908,
0.02059139870107174,
0.06737419217824936,
0.04176458343863487,
-0.04978838190436363,
-0.05877475067973137,
-0.06289287656545639,
-0.03354167565703392,
-0.03871942684054375,
0.009898529388010502,
-0.05514208599925041,
-0.11629002541303635,
-0.011855563148856163,
0.10663620382547379,
0.037354156374931335,
-0.0065480442717671394,
-0.051189567893743515,
0.06663123518228531,
0.01874656230211258,
0.032841797918081284,
0.041593004018068314,
-0.06879369914531708,
0.04216769337654114,
-0.01628219522535801,
5.4139394340936695e-34,
0.05697013810276985,
-0.006972255185246468,
0.015711724758148193,
-0.17956365644931793,
0.02320219948887825,
0.007923615165054798,
-0.008062449283897877,
0.0074974060989916325,
0.07391711324453354,
0.0309313777834177,
0.060510627925395966,
0.058605875819921494,
0.09515274316072464,
-0.002282935893163085,
0.001603541080839932,
0.07024981826543808,
0.012629246339201927,
0.07425693422555923,
-0.038426291197538376,
0.01861148327589035,
0.030608950182795525,
-0.02449394389986992,
0.021528491750359535,
-0.003039651783183217,
-0.03676343336701393,
0.03130284696817398,
0.07998586446046829,
0.010451192036271095,
-0.07930229604244232,
-0.013543923385441303,
0.018781835213303566,
0.05168003588914871,
-0.07191970944404602,
0.15783067047595978,
0.026191607117652893,
0.01262354850769043,
0.08218053728342056,
-0.029807550832629204,
-0.07528624683618546,
-0.04250097647309303,
0.017244765534996986,
0.04411793500185013,
0.03708017244935036,
0.009233047254383564,
-0.040271829813718796,
0.022496428340673447,
0.02495843544602394,
0.07633638381958008,
0.005147108342498541,
0.013892097398638725,
0.05610476806759834,
-0.06684739887714386,
0.05862557515501976,
-0.020688841119408607,
0.05377643182873726,
0.06718500703573227,
0.005329249892383814,
-0.01388032827526331,
0.029931528493762016,
0.009508464485406876,
-0.045173756778240204,
0.11534366756677628,
-0.06510116159915924,
0.05117698386311531,
-0.0026125339791178703,
-0.08554837852716446,
-0.03784770518541336,
0.0804959163069725,
0.011298024095594883,
-0.07695550471544266,
-0.04868878796696663,
0.02515520341694355,
0.06252261996269226,
-0.04509226232767105,
-0.01246943511068821,
0.028559505939483643,
-0.030573077499866486,
0.05066261067986488,
-0.08187384903430939,
0.04469604790210724,
0.0034051244147121906,
0.04145054519176483,
-0.021858664229512215,
-0.06112268194556236,
-0.00908052921295166,
-0.05903250351548195,
0.0259539932012558,
0.059690944850444794,
-0.07613514363765717,
-0.03720718249678612,
-0.036316655576229095,
0.07058046013116837,
-0.008224100805819035,
0.041961874812841415,
-0.0285952128469944,
-1.496900736697171e-8,
-0.0014124972512945533,
0.03401879221200943,
-0.040338415652513504,
0.04116074740886688,
0.0935964286327362,
-0.05115952715277672,
0.0008746005478315055,
-0.03389839455485344,
-0.00567849725484848,
-0.010686947964131832,
-0.04789939522743225,
-0.04820054769515991,
-0.02011880651116371,
-0.03209094703197479,
-0.04211259260773659,
-0.10229527950286865,
-0.07819421589374542,
-0.031228765845298767,
-0.02154778689146042,
-0.04960230365395546,
0.08087796717882156,
-0.07801242172718048,
0.06919731199741364,
-0.04999840259552002,
0.03687043860554695,
0.03889009356498718,
-0.049989692866802216,
-0.04254625365138054,
-0.04606937617063522,
0.08682432025671005,
-0.031148413196206093,
0.11826753616333008,
0.034102488309144974,
-0.0208592489361763,
-0.0205202866345644,
0.027134142816066742,
0.09741277992725372,
0.051608603447675705,
0.013477512635290623,
-0.13649295270442963,
-0.022304272279143333,
0.02385953813791275,
0.038732077926397324,
-0.09249968826770782,
-0.04549082741141319,
0.054220106452703476,
0.01160438358783722,
0.051190607249736786,
0.07713303714990616,
-0.022097084671258926,
-0.06127818301320076,
-0.01857956498861313,
0.006740490905940533,
-0.00496308971196413,
0.024095389991998672,
0.0736224576830864,
-0.003481915919110179,
-0.0699305310845375,
-0.006629763171076775,
-0.0598808117210865,
0.05297163128852844,
-0.02902800403535366,
-0.027858933433890343,
-0.01287526823580265
] |
lincoln/mbart-mlsum-automatic-summarization | 17a3a2e474932a90e664ef2c75c5e46ef964fc1a | 2021-09-07T08:21:55.000Z | [
"pytorch",
"tf",
"mbart",
"text2text-generation",
"fr",
"dataset:MLSUM",
"arxiv:2004.14900",
"transformers",
"summarization",
"bart",
"license:mit",
"autotrain_compatible"
] | summarization | false | lincoln | null | lincoln/mbart-mlsum-automatic-summarization | 1,991 | 3 | transformers | ---
language:
- fr
license: mit
datasets:
- MLSUM
pipeline_tag: "summarization"
widget:
- text: « La veille de l’ouverture, je vais faire venir un coach pour les salariés qui reprendront le travail. Cela va me coûter 300 euros, mais après des mois d’oisiveté obligatoire, la reprise n’est pas simple. Certains sont au chômage partiel depuis mars 2020 », raconte Alain Fontaine, propriétaire du restaurant Le Mesturet, dans le quartier de la Bourse, à Paris. Cette date d’ouverture, désormais, il la connaît. Emmanuel Macron a, en effet, donné le feu vert pour un premier accueil des clients en terrasse, mercredi 19 mai. M. Fontaine imagine même faire venir un orchestre ce jour-là pour fêter l’événement. Il lui reste toutefois à construire sa terrasse. Il pensait que les ouvriers passeraient samedi 1er mai pour l’installer, mais, finalement, le rendez-vous a été décalé. Pour l’instant, le tas de bois est entreposé dans la salle de restaurant qui n’a plus accueilli de convives depuis le 29 octobre 2020, quand le couperet de la fermeture administrative est tombé.M. Fontaine, président de l’Association française des maîtres restaurateurs, ne manquera pas de concurrents prêts à profiter de ce premier temps de réouverture des bars et restaurants. Même si le couvre-feu limite le service à 21 heures. D’autant que la Mairie de Paris vient d’annoncer le renouvellement des terrasses éphémères installées en 2020 et leur gratuité jusqu’à la fin de l’été.
tags:
- summarization
- mbart
- bart
---
# Résumé automatique d'article de presses
Ce modèles est basé sur le modèle [`facebook/mbart-large-50`](https://huggingface.co/facebook/mbart-large-50) et été fine-tuné en utilisant des articles de presse issus de la base de données MLSUM. L'hypothèse à été faite que les chapeaux des articles faisaient de bon résumés de référence.
## Entrainement
Nous avons testé deux architecture de modèles (T5 et BART) avec des textes en entrée de 512 ou 1024 tokens. Finallement c'est le modèle BART avec 512 tokens qui à été retenu.
Il a été entrainé sur 2 epochs (~700K articles) sur une Tesla V100 (32 heures d'entrainement).
## Résultats

Nous avons comparé notre modèle (`mbart-large-512-full` sur le graphique) à deux références:
* MBERT qui correspond aux performances du modèle entrainé par l'équipe à l'origine de la base d'articles MLSUM
* Barthez qui est un autre modèle basé sur des articles de presses issus de la base de données OrangeSum
On voit que le score de novelty (cf papier MLSUM) de notre modèle n'est pas encore comparable à ces deux références et encore moins à une production humaine néanmoins les résumés générés sont dans l'ensemble de bonne qualité.
## Utilisation
```python
from transformers import AutoModelForSeq2SeqLM, AutoTokenizer
from transformers import SummarizationPipeline
model_name = 'lincoln/mbart-mlsum-automatic-summarization'
loaded_tokenizer = AutoTokenizer.from_pretrained(model_name)
loaded_model = AutoModelForSeq2SeqLM.from_pretrained(model_name)
nlp = SummarizationPipeline(model=loaded_model, tokenizer=loaded_tokenizer)
nlp("""
« La veille de l’ouverture, je vais faire venir un coach pour les salariés qui reprendront le travail.
Cela va me coûter 300 euros, mais après des mois d’oisiveté obligatoire, la reprise n’est pas simple.
Certains sont au chômage partiel depuis mars 2020 », raconte Alain Fontaine, propriétaire du restaurant Le Mesturet,
dans le quartier de la Bourse, à Paris. Cette date d’ouverture, désormais, il la connaît. Emmanuel Macron a, en effet,
donné le feu vert pour un premier accueil des clients en terrasse, mercredi 19 mai. M. Fontaine imagine même faire venir un orchestre ce jour-là pour fêter l’événement.
Il lui reste toutefois à construire sa terrasse. Il pensait que les ouvriers passeraient samedi 1er mai pour l’installer, mais, finalement, le rendez-vous a été décalé.
Pour l’instant, le tas de bois est entreposé dans la salle de restaurant qui n’a plus accueilli de convives depuis le 29 octobre 2020,
quand le couperet de la fermeture administrative est tombé.M. Fontaine, président de l’Association française des maîtres restaurateurs,
ne manquera pas de concurrents prêts à profiter de ce premier temps de réouverture des bars et restaurants. Même si le couvre-feu limite le service à 21 heures.
D’autant que la Mairie de Paris vient d’annoncer le renouvellement des terrasses éphémères installées en 2020 et leur gratuité jusqu’à la fin de l’été.
""")
```
## Citation
```bibtex
@article{scialom2020mlsum,
title={MLSUM: The Multilingual Summarization Corpus},
author={Thomas Scialom and Paul-Alexis Dray and Sylvain Lamprier and Benjamin Piwowarski and Jacopo Staiano},
year={2020},
eprint={2004.14900},
archivePrefix={arXiv},
primaryClass={cs.CL}
}
``` | [
-0.033752623945474625,
0.07255825400352478,
-0.023322252556681633,
-0.04990364611148834,
0.042897630482912064,
0.017792681232094765,
0.03042132407426834,
0.029856493696570396,
0.1065765917301178,
0.002893788507208228,
-0.0016870573163032532,
-0.08872511237859726,
-0.0003398474946152419,
-0.011296681128442287,
-0.07410614937543869,
-0.1277349293231964,
-0.017978861927986145,
0.06834549456834793,
-0.008600043132901192,
0.03432133421301842,
0.04887932911515236,
0.007072777487337589,
-0.013504592701792717,
0.033030543476343155,
0.004904580302536488,
-0.012431644834578037,
-0.08891063183546066,
0.028765320777893066,
-0.03862280026078224,
0.008043562062084675,
0.007202232722193003,
0.04494320973753929,
-0.012015455402433872,
0.03592512384057045,
0.05004033446311951,
-0.015006395988166332,
-0.0013957031769677997,
-0.0372711606323719,
-0.021053140982985497,
0.07066706568002701,
-0.0013175884960219264,
-0.030027491971850395,
-0.108932264149189,
-0.0074822804890573025,
-0.05222902074456215,
-0.0029134959913790226,
0.04351815581321716,
0.031122546643018723,
-0.055553652346134186,
0.03935263305902481,
-0.07973439246416092,
0.04688938707113266,
-0.011464105919003487,
-0.06585927307605743,
-0.04983906075358391,
0.005292147863656282,
-0.022812610492110252,
-0.026079699397087097,
0.08167075365781784,
-0.004341410472989082,
-0.0904681459069252,
-0.07292434573173523,
-0.014306544326245785,
0.0382436141371727,
0.015658285468816757,
-0.0491362027823925,
-0.027705298736691475,
0.009060664102435112,
-0.11427761614322662,
0.010544469580054283,
0.004890462849289179,
-0.03717684745788574,
-0.03827530890703201,
0.002382767852395773,
0.04971502348780632,
0.09783855825662613,
-0.01939898543059826,
-0.01629273034632206,
0.009959748014807701,
-0.18050990998744965,
0.030162665992975235,
-0.07602947950363159,
0.036667969077825546,
0.025930901989340782,
0.00510575482621789,
-0.021158110350370407,
0.03836394101381302,
0.01129857823252678,
0.15699248015880585,
-0.027778958901762962,
-0.024452704936265945,
0.034856610000133514,
-0.08026082068681717,
0.03972958028316498,
0.013576430268585682,
0.004920301493257284,
-0.021866871044039726,
-0.022537628188729286,
0.04165364056825638,
0.06932394206523895,
0.0003409830096643418,
0.021640902385115623,
0.014505496248602867,
0.02305293269455433,
-0.03373687341809273,
-0.06343705207109451,
0.04546188935637474,
-0.016762904822826385,
-0.06016647443175316,
0.0078208576887846,
0.02591334655880928,
-0.01354814413934946,
-0.021131183952093124,
-0.05193625018000603,
-0.04093721881508827,
-0.013240888714790344,
-0.009915914386510849,
-0.1192697063088417,
0.03359353542327881,
-0.06138068065047264,
0.01462441124022007,
-0.015532609075307846,
-0.0742039754986763,
-0.009883360005915165,
-0.05742965638637543,
0.04354741796851158,
0.07882927358150482,
1.55520929511742e-32,
0.009455162100493908,
0.011774679645895958,
0.0019180140225216746,
0.003788161324337125,
0.04687145724892616,
0.016023803502321243,
-0.025899291038513184,
0.08116403967142105,
-0.010177114978432655,
-0.015485871583223343,
0.01645250990986824,
-0.03641856089234352,
-0.1019689291715622,
0.0209075715392828,
0.00909015815705061,
-0.020267987623810768,
0.14593449234962463,
0.01357800979167223,
-0.023180607706308365,
-0.008656552992761135,
0.03602404147386551,
0.01565270870923996,
0.11233248561620712,
-0.00776993902400136,
0.058740098029375076,
0.021790293976664543,
-0.023489467799663544,
-0.08242771029472351,
-0.017109496518969536,
0.0417332649230957,
0.0189240500330925,
-0.0450371578335762,
0.027655592188239098,
-0.018747398629784584,
0.02339920960366726,
-0.036953315138816833,
-0.03021874837577343,
0.011313258670270443,
-0.007630136329680681,
-0.0023846414405852556,
-0.010458528995513916,
0.007577782031148672,
0.004867223557084799,
-0.10857242345809937,
-0.05724690854549408,
0.08520641922950745,
0.042176175862550735,
0.07194823771715164,
0.08911225944757462,
-0.01502201147377491,
0.013211075216531754,
0.00613403832539916,
-0.0530080571770668,
-0.09795740246772766,
0.03518866375088692,
-0.035823091864585876,
-0.08910001069307327,
-0.013653679750859737,
-0.017714813351631165,
-0.032701194286346436,
-0.0813450813293457,
0.03588326275348663,
-0.002936032135039568,
0.04053131118416786,
0.026649978011846542,
0.023718388751149178,
-0.0275419969111681,
0.08086206018924713,
0.15006905794143677,
-0.06470511108636856,
-0.05312514677643776,
0.05490688607096672,
0.0797162726521492,
0.044413093477487564,
0.06900046020746231,
0.07903485745191574,
0.028445033356547356,
0.017482761293649673,
-0.05434345081448555,
0.005683104041963816,
-0.026010360568761826,
-0.02095366269350052,
0.0030330666340887547,
-0.006801581010222435,
-0.008202262222766876,
0.035637810826301575,
0.05030260235071182,
0.053718410432338715,
0.0385904461145401,
-0.05803363025188446,
-0.013084071688354015,
-0.03145524859428406,
-0.041982460767030716,
-0.05287650600075722,
-0.04740860313177109,
-1.5883964393770103e-32,
-0.029381148517131805,
0.06509874761104584,
-0.06632696092128754,
0.09110262244939804,
-0.011167583055794239,
0.010983464308083057,
-0.0295440424233675,
0.05216981843113899,
0.05216280743479729,
-0.05208110064268112,
-0.04942627623677254,
-0.07330159097909927,
0.019553489983081818,
-0.060694243758916855,
-0.05162244290113449,
0.054044920951128006,
-0.051901064813137054,
-0.09268985688686371,
-0.004327582661062479,
0.023930387571454048,
-0.048572007566690445,
-0.008403223007917404,
0.04262828454375267,
0.007713093888014555,
0.005229312460869551,
0.009280222468078136,
0.045884206891059875,
0.035033218562603,
-0.077791228890419,
-0.013858240097761154,
0.04029802978038788,
0.005811342969536781,
-0.012823458760976791,
0.020110072568058968,
-0.03126148134469986,
-0.031130952760577202,
0.04452253505587578,
0.03177685663104057,
-0.02718524821102619,
0.17594189941883087,
0.03249211609363556,
-0.043813906610012054,
-0.012440384365618229,
-0.04416372999548912,
0.0013917280593886971,
-0.04116569831967354,
0.02311091311275959,
-0.11605042219161987,
0.015436658635735512,
-0.03466109186410904,
0.031397607177495956,
-0.016359364613890648,
-0.11372646689414978,
-0.010148313827812672,
-0.005775779020041227,
0.0067421505227684975,
0.07051853090524673,
0.00005452726691146381,
-0.05227929726243019,
-0.10303511470556259,
0.023743819445371628,
0.08375759422779083,
-0.027740895748138428,
-0.028697922825813293,
0.08128849416971207,
-0.03395357355475426,
-0.03611653670668602,
-0.1144610270857811,
-0.021094098687171936,
0.034614041447639465,
0.08218751847743988,
-0.05394255742430687,
-0.005524656269699335,
-0.016785819083452225,
-0.06153807416558266,
0.12049150466918945,
0.07536039501428604,
0.020812269300222397,
-0.024975595995783806,
0.09693046659231186,
-0.11760535836219788,
-0.05604685842990875,
0.04007399082183838,
0.035870444029569626,
0.051005441695451736,
0.02926141396164894,
-0.08274602890014648,
-0.019340820610523224,
-0.014811706729233265,
0.009500741958618164,
-0.05122829228639603,
-0.017212796956300735,
0.03753145784139633,
0.028458042070269585,
0.021014763042330742,
-7.493624565313439e-8,
-0.01955220103263855,
-0.031655941158533096,
-0.12128458172082901,
0.03504645451903343,
0.025935098528862,
-0.15169604122638702,
0.03578728064894676,
-0.007883063517510891,
-0.008862953633069992,
0.028511755168437958,
0.03668283298611641,
-0.021344570443034172,
-0.0524628721177578,
-0.016703179106116295,
-0.0289914533495903,
0.072222501039505,
-0.0021329973824322224,
0.026351094245910645,
-0.057344675064086914,
0.005639081355184317,
-0.023621736094355583,
0.03038371354341507,
-0.05548076704144478,
-0.12487038969993591,
0.0016480869380757213,
-0.04664852097630501,
-0.06766925752162933,
-0.010348566807806492,
0.002553696744143963,
-0.039239220321178436,
0.06616011261940002,
-0.00608594948425889,
0.05698367953300476,
-0.07704668492078781,
-0.013697831891477108,
-0.045561566948890686,
0.013439860194921494,
-0.022249020636081696,
0.003278035204857588,
0.03324452415108681,
0.12701937556266785,
0.030657382681965828,
-0.02107313647866249,
-0.013364064507186413,
0.09520910680294037,
0.005684949923306704,
-0.020826075226068497,
0.03278593346476555,
0.0033780091907829046,
0.06706222146749496,
-0.06709572672843933,
0.023288128897547722,
0.044618505984544754,
-0.004890720825642347,
0.058896955102682114,
-0.03970281779766083,
-0.009990965947508812,
-0.017505399882793427,
0.013910551555454731,
0.013658649288117886,
0.04506003484129906,
0.02748308889567852,
-0.03730788454413414,
-0.08870074152946472
] |
hf-internal-testing/tiny-random-camembert | 8fa65c628a3f475b1ed4e8dff6adf09db1b6bb83 | 2022-07-27T10:07:32.000Z | [
"pytorch",
"camembert",
"feature-extraction",
"transformers"
] | feature-extraction | false | hf-internal-testing | null | hf-internal-testing/tiny-random-camembert | 1,990 | null | transformers | Entry not found | [
0.0461147278547287,
-0.038838207721710205,
-0.01049656979739666,
-0.03682169318199158,
0.011261860840022564,
0.013094935566186905,
0.0019101888174191117,
-0.013979103416204453,
0.027092741802334785,
-0.015212527476251125,
0.017284274101257324,
-0.08189476281404495,
0.03817418962717056,
-0.04920130595564842,
0.021389011293649673,
-0.015245908871293068,
-0.03203780576586723,
-0.1245758980512619,
0.03150877356529236,
0.032381657510995865,
-0.060957908630371094,
0.05409295856952667,
-0.025087490677833557,
0.01568586938083172,
0.028129950165748596,
-0.04710396006703377,
-0.018688226118683815,
0.013785239309072495,
-0.04001208767294884,
0.01173911802470684,
-0.04317743331193924,
0.05500618368387222,
0.004543041344732046,
0.02973111905157566,
0.14852192997932434,
0.02658126689493656,
0.02907961793243885,
-0.05169107764959335,
0.05803573504090309,
-0.07732241600751877,
-0.017637968063354492,
-0.04219653457403183,
0.041807834059000015,
0.023620979860424995,
0.021563321352005005,
0.016478516161441803,
-0.0021814992651343346,
-0.06400240957736969,
0.06393089145421982,
0.019599027931690216,
-0.08565037697553635,
0.00934905931353569,
-0.008718925528228283,
-0.028583496809005737,
-0.07310017943382263,
0.09416428208351135,
0.001759322709403932,
0.06184990331530571,
0.011840506456792355,
-0.035997264087200165,
0.08358278125524521,
-0.02619801089167595,
0.03736566752195358,
-0.028206506744027138,
-0.07454850524663925,
-0.08883563429117203,
-0.06279942393302917,
-0.008695344440639019,
0.014119276776909828,
-0.0825355276465416,
0.0649217739701271,
-0.00223911227658391,
-0.14716917276382446,
0.07743025571107864,
-0.03548373281955719,
-0.055201586335897446,
0.006981803569942713,
-0.012166670523583889,
0.055111464112997055,
-0.007116836030036211,
-0.023175746202468872,
-0.005835152696818113,
-0.09185640513896942,
0.055196937173604965,
0.034148022532463074,
0.03835180774331093,
0.038685429841279984,
-0.025987252593040466,
0.017804903909564018,
0.022428328171372414,
0.025005368515849113,
-0.10761535167694092,
-0.048001550137996674,
-0.04343584179878235,
0.012374646961688995,
-0.019502125680446625,
0.029218152165412903,
0.0842173621058464,
-0.011719699949026108,
0.09283553808927536,
-0.007015465293079615,
-0.03543110564351082,
-0.06936459988355637,
0.09425332397222519,
-0.010958523489534855,
-0.00805904995650053,
0.004974212497472763,
-0.0031528924591839314,
0.06105927750468254,
-0.03964288905262947,
-0.03619541600346565,
-0.019901901483535767,
0.07134733349084854,
0.039514873176813126,
-0.012729483656585217,
-0.006646515801548958,
-0.04746140539646149,
-0.014432490803301334,
-0.05157482624053955,
0.09506245702505112,
-0.049747664481401443,
-0.04591796174645424,
-0.008965466171503067,
-0.0325421579182148,
-0.08626784384250641,
-0.06624380499124527,
0.02538885548710823,
-4.303924894057984e-33,
0.01133066974580288,
0.0033434738870710135,
-0.002155609894543886,
0.04871906340122223,
-0.023564351722598076,
-0.07933273911476135,
0.0600903145968914,
0.02335330657660961,
-0.03844716399908066,
-0.020433755591511726,
-0.06952055543661118,
-0.03235611692070961,
0.0062485747039318085,
0.064804308116436,
-0.03201229125261307,
0.061689723283052444,
0.0417000837624073,
-0.00761845987290144,
0.03340127319097519,
-0.047770582139492035,
0.00887306872755289,
-0.04066338762640953,
-0.010506896302103996,
0.0106519665569067,
0.021333497017621994,
0.12854498624801636,
-0.009705503471195698,
0.010055632330477238,
-0.017507633194327354,
0.006515394430607557,
0.06334009766578674,
-0.057817306369543076,
0.013668818399310112,
-0.020286159589886665,
0.05430467426776886,
-0.023184705525636673,
0.0828516036272049,
0.0005449643940664828,
-0.10372652113437653,
-0.07634282112121582,
-0.005381610710173845,
-0.039263784885406494,
0.0006114727002568543,
-0.013281986117362976,
0.07119110971689224,
0.043696220964193344,
0.03168422728776932,
0.04338686540722847,
0.05728672817349434,
0.0832006186246872,
-0.07961414009332657,
0.015234283171594143,
0.017002005130052567,
0.047004107385873795,
-0.09794387966394424,
0.004990279674530029,
-0.07062993198633194,
-0.028000490739941597,
-0.04018733277916908,
-0.0702052190899849,
0.011351344175636768,
0.06020182743668556,
-0.03297270089387894,
0.09396500885486603,
0.03417910635471344,
-0.019825750961899757,
-0.034690454602241516,
-0.013036907650530338,
0.05896938592195511,
-0.012359356507658958,
-0.017275206744670868,
-0.07982361316680908,
0.02059139870107174,
0.06737419217824936,
0.04176458343863487,
-0.04978838190436363,
-0.05877475067973137,
-0.06289287656545639,
-0.03354167565703392,
-0.03871942684054375,
0.009898529388010502,
-0.05514208599925041,
-0.11629002541303635,
-0.011855563148856163,
0.10663620382547379,
0.037354156374931335,
-0.0065480442717671394,
-0.051189567893743515,
0.06663123518228531,
0.01874656230211258,
0.032841797918081284,
0.041593004018068314,
-0.06879369914531708,
0.04216769337654114,
-0.01628219522535801,
5.4139394340936695e-34,
0.05697013810276985,
-0.006972255185246468,
0.015711724758148193,
-0.17956365644931793,
0.02320219948887825,
0.007923615165054798,
-0.008062449283897877,
0.0074974060989916325,
0.07391711324453354,
0.0309313777834177,
0.060510627925395966,
0.058605875819921494,
0.09515274316072464,
-0.002282935893163085,
0.001603541080839932,
0.07024981826543808,
0.012629246339201927,
0.07425693422555923,
-0.038426291197538376,
0.01861148327589035,
0.030608950182795525,
-0.02449394389986992,
0.021528491750359535,
-0.003039651783183217,
-0.03676343336701393,
0.03130284696817398,
0.07998586446046829,
0.010451192036271095,
-0.07930229604244232,
-0.013543923385441303,
0.018781835213303566,
0.05168003588914871,
-0.07191970944404602,
0.15783067047595978,
0.026191607117652893,
0.01262354850769043,
0.08218053728342056,
-0.029807550832629204,
-0.07528624683618546,
-0.04250097647309303,
0.017244765534996986,
0.04411793500185013,
0.03708017244935036,
0.009233047254383564,
-0.040271829813718796,
0.022496428340673447,
0.02495843544602394,
0.07633638381958008,
0.005147108342498541,
0.013892097398638725,
0.05610476806759834,
-0.06684739887714386,
0.05862557515501976,
-0.020688841119408607,
0.05377643182873726,
0.06718500703573227,
0.005329249892383814,
-0.01388032827526331,
0.029931528493762016,
0.009508464485406876,
-0.045173756778240204,
0.11534366756677628,
-0.06510116159915924,
0.05117698386311531,
-0.0026125339791178703,
-0.08554837852716446,
-0.03784770518541336,
0.0804959163069725,
0.011298024095594883,
-0.07695550471544266,
-0.04868878796696663,
0.02515520341694355,
0.06252261996269226,
-0.04509226232767105,
-0.01246943511068821,
0.028559505939483643,
-0.030573077499866486,
0.05066261067986488,
-0.08187384903430939,
0.04469604790210724,
0.0034051244147121906,
0.04145054519176483,
-0.021858664229512215,
-0.06112268194556236,
-0.00908052921295166,
-0.05903250351548195,
0.0259539932012558,
0.059690944850444794,
-0.07613514363765717,
-0.03720718249678612,
-0.036316655576229095,
0.07058046013116837,
-0.008224100805819035,
0.041961874812841415,
-0.0285952128469944,
-1.496900736697171e-8,
-0.0014124972512945533,
0.03401879221200943,
-0.040338415652513504,
0.04116074740886688,
0.0935964286327362,
-0.05115952715277672,
0.0008746005478315055,
-0.03389839455485344,
-0.00567849725484848,
-0.010686947964131832,
-0.04789939522743225,
-0.04820054769515991,
-0.02011880651116371,
-0.03209094703197479,
-0.04211259260773659,
-0.10229527950286865,
-0.07819421589374542,
-0.031228765845298767,
-0.02154778689146042,
-0.04960230365395546,
0.08087796717882156,
-0.07801242172718048,
0.06919731199741364,
-0.04999840259552002,
0.03687043860554695,
0.03889009356498718,
-0.049989692866802216,
-0.04254625365138054,
-0.04606937617063522,
0.08682432025671005,
-0.031148413196206093,
0.11826753616333008,
0.034102488309144974,
-0.0208592489361763,
-0.0205202866345644,
0.027134142816066742,
0.09741277992725372,
0.051608603447675705,
0.013477512635290623,
-0.13649295270442963,
-0.022304272279143333,
0.02385953813791275,
0.038732077926397324,
-0.09249968826770782,
-0.04549082741141319,
0.054220106452703476,
0.01160438358783722,
0.051190607249736786,
0.07713303714990616,
-0.022097084671258926,
-0.06127818301320076,
-0.01857956498861313,
0.006740490905940533,
-0.00496308971196413,
0.024095389991998672,
0.0736224576830864,
-0.003481915919110179,
-0.0699305310845375,
-0.006629763171076775,
-0.0598808117210865,
0.05297163128852844,
-0.02902800403535366,
-0.027858933433890343,
-0.01287526823580265
] |
microsoft/DialogRPT-human-vs-rand | 7206b425c2c016dd5533e2a99e665ba3546e5ce0 | 2021-05-23T09:18:07.000Z | [
"pytorch",
"gpt2",
"text-classification",
"arxiv:2009.06978",
"transformers"
] | text-classification | false | microsoft | null | microsoft/DialogRPT-human-vs-rand | 1,986 | 1 | transformers | # Demo
Please try this [➤➤➤ Colab Notebook Demo (click me!)](https://colab.research.google.com/drive/1cAtfkbhqsRsT59y3imjR1APw3MHDMkuV?usp=sharing)
| Context | Response | `human_vs_rand` score |
| :------ | :------- | :------------: |
| I love NLP! | He is a great basketball player. | 0.027 |
| I love NLP! | Can you tell me how it works? | 0.754 |
| I love NLP! | Me too! | 0.631 |
The `human_vs_rand` score predicts how likely the response is corresponding to the given context, rather than a random response.
# DialogRPT-human-vs-rand
### Dialog Ranking Pretrained Transformers
> How likely a dialog response is upvoted 👍 and/or gets replied 💬?
This is what [**DialogRPT**](https://github.com/golsun/DialogRPT) is learned to predict.
It is a set of dialog response ranking models proposed by [Microsoft Research NLP Group](https://www.microsoft.com/en-us/research/group/natural-language-processing/) trained on 100 + millions of human feedback data.
It can be used to improve existing dialog generation model (e.g., [DialoGPT](https://huggingface.co/microsoft/DialoGPT-medium)) by re-ranking the generated response candidates.
Quick Links:
* [EMNLP'20 Paper](https://arxiv.org/abs/2009.06978/)
* [Dataset, training, and evaluation](https://github.com/golsun/DialogRPT)
* [Colab Notebook Demo](https://colab.research.google.com/drive/1cAtfkbhqsRsT59y3imjR1APw3MHDMkuV?usp=sharing)
We considered the following tasks and provided corresponding pretrained models.
|Task | Description | Pretrained model |
| :------------- | :----------- | :-----------: |
| **Human feedback** | **given a context and its two human responses, predict...**|
| `updown` | ... which gets more upvotes? | [model card](https://huggingface.co/microsoft/DialogRPT-updown) |
| `width`| ... which gets more direct replies? | [model card](https://huggingface.co/microsoft/DialogRPT-width) |
| `depth`| ... which gets longer follow-up thread? | [model card](https://huggingface.co/microsoft/DialogRPT-depth) |
| **Human-like** (human vs fake) | **given a context and one human response, distinguish it with...** |
| `human_vs_rand`| ... a random human response | this model |
| `human_vs_machine`| ... a machine generated response | [model card](https://huggingface.co/microsoft/DialogRPT-human-vs-machine) |
### Contact:
Please create an issue on [our repo](https://github.com/golsun/DialogRPT)
### Citation:
```
@inproceedings{gao2020dialogrpt,
title={Dialogue Response RankingTraining with Large-Scale Human Feedback Data},
author={Xiang Gao and Yizhe Zhang and Michel Galley and Chris Brockett and Bill Dolan},
year={2020},
booktitle={EMNLP}
}
```
| [
-0.08221784979104996,
-0.0684313178062439,
0.0354909747838974,
0.014281258918344975,
0.05558816343545914,
-0.01630459725856781,
0.09241504967212677,
0.0011068449821323156,
0.10466919839382172,
0.004171146545559168,
-0.0003360148402862251,
-0.08933521807193756,
0.028311708942055702,
-0.003218272468075156,
0.02992299757897854,
-0.005410661455243826,
0.04857548698782921,
-0.06310442090034485,
-0.11697313189506531,
-0.0348455086350441,
0.05563023313879967,
0.021985497325658798,
0.03347264975309372,
-0.04283558204770088,
0.03443322703242302,
-0.0644475445151329,
-0.035721633583307266,
0.03349905088543892,
-0.014801401644945145,
-0.017367858439683914,
0.020878102630376816,
0.027263488620519638,
0.026840919628739357,
0.020940745249390602,
-0.08488579839468002,
0.02100054733455181,
-0.03650104999542236,
-0.03689122572541237,
-0.027800101786851883,
-0.0010308728087693453,
-0.07135618478059769,
-0.040774617344141006,
-0.007548022083938122,
0.039502568542957306,
0.050651103258132935,
0.01295418106019497,
-0.12244158238172531,
-0.05086817592382431,
-0.05791210010647774,
0.056658193469047546,
-0.11907152831554413,
0.045566316694021225,
0.04881839081645012,
0.0860443264245987,
-0.02030320093035698,
0.09070145338773727,
-0.01883075013756752,
0.01297321729362011,
0.03986728563904762,
-0.08995191752910614,
-0.053388312458992004,
-0.08934955298900604,
-0.06274755299091339,
0.004021496511995792,
0.020939724519848824,
-0.007814685814082623,
-0.06613602489233017,
0.0009622123907320201,
0.02208840847015381,
-0.007796318270266056,
-0.04187360033392906,
0.02086968906223774,
-0.02585112303495407,
0.042570412158966064,
0.023609457537531853,
-0.006305923219770193,
-0.019612930715084076,
-0.0775662437081337,
0.05304809659719467,
-0.018776042386889458,
0.01526129525154829,
-0.027679400518536568,
0.09490375965833664,
0.07523688673973083,
0.01198893878608942,
-0.030890733003616333,
0.05385482311248779,
0.08369550108909607,
-0.018488341942429543,
0.03348896652460098,
-0.04813123121857643,
-0.032338228076696396,
0.023369386792182922,
0.050827931612730026,
-0.01869904436171055,
0.13240177929401398,
-0.051241934299468994,
-0.11870100349187851,
-0.009124460630118847,
0.07396029680967331,
0.023237649351358414,
0.0313393771648407,
0.002093386836349964,
-0.1149929091334343,
-0.03838060423731804,
-0.005810142494738102,
-0.03759429231286049,
0.03402059152722359,
0.06093292683362961,
-0.011841902509331703,
-0.015399190597236156,
0.01575327478349209,
0.02347872592508793,
0.06048709899187088,
0.016383066773414612,
-0.03416530787944794,
0.0033624405041337013,
0.02683139033615589,
0.004038217943161726,
0.025755958631634712,
0.030540650710463524,
0.048060342669487,
-0.05512501671910286,
-0.00347709353081882,
0.004724323749542236,
0.00599618861451745,
0.07775022089481354,
3.902464185893402e-33,
0.09483656287193298,
-0.04165010154247284,
0.0314006432890892,
0.02298044227063656,
0.0403917022049427,
-0.005270292982459068,
-0.00634436309337616,
0.03453987091779709,
-0.07639895379543304,
-0.027086686342954636,
-0.02776925265789032,
0.019733484834432602,
-0.04306144267320633,
0.05283856391906738,
-0.0335841029882431,
0.032754380255937576,
-0.0837305337190628,
-0.00731669133529067,
-0.054319117218256,
0.028064262121915817,
0.05415525659918785,
0.10093763470649719,
0.010013016872107983,
-0.02578006125986576,
0.07458851486444473,
-0.010502289980649948,
0.008721016347408295,
-0.05052857846021652,
-0.05638738349080086,
0.03666555881500244,
-0.112519770860672,
0.050959713757038116,
-0.13428747653961182,
-0.020640017464756966,
0.014534199610352516,
-0.03977213799953461,
-0.03692752867937088,
-0.022327160462737083,
-0.1135190799832344,
-0.05554993078112602,
-0.05133695900440216,
0.008269054815173149,
-0.027062997221946716,
-0.03778774291276932,
-0.07934603095054626,
-0.014195257797837257,
-0.038816899061203,
0.012059845961630344,
0.010423709638416767,
0.0370243638753891,
0.02857103757560253,
0.015159624628722668,
0.0269404798746109,
0.01575382426381111,
0.039579056203365326,
-0.018511470407247543,
0.033764999359846115,
0.06878864765167236,
0.01517970860004425,
0.00878774095326662,
0.047960180789232254,
0.005874982103705406,
0.04983924701809883,
0.007205243222415447,
0.02354881912469864,
0.04227307811379433,
-0.05588584393262863,
-0.027767617255449295,
0.11210322380065918,
-0.006847540382295847,
0.03584005683660507,
0.013611426576972008,
0.007061840500682592,
0.0472419448196888,
-0.06585060805082321,
0.027531297877430916,
0.01744663715362549,
-0.0008907688315957785,
0.03815843164920807,
0.018082918599247932,
-0.01613306999206543,
-0.044015899300575256,
-0.054764747619628906,
-0.08839242905378342,
0.004296967759728432,
-0.010797667317092419,
0.03902335837483406,
-0.09772249311208725,
-0.019155114889144897,
-0.00207308866083622,
-0.004972267895936966,
0.011619006283581257,
-0.08038200438022614,
0.023837454617023468,
-0.053750526160001755,
-5.602177956646566e-33,
-0.07893002778291702,
0.08089738339185715,
-0.031180929392576218,
0.04160970821976662,
0.002098098862916231,
-0.021105803549289703,
0.07961685210466385,
0.03909328207373619,
0.11849266290664673,
-0.01365391444414854,
-0.044708434492349625,
0.0109470896422863,
0.0367155484855175,
0.014732959680259228,
0.12765580415725708,
0.02872631698846817,
-0.0005840944941155612,
0.03044773079454899,
-0.06273189932107925,
0.055137619376182556,
0.03066224604845047,
0.10647870600223541,
-0.1612749546766281,
0.05479443073272705,
-0.02811247855424881,
-0.015591044910252094,
0.0008437010110355914,
0.03597075864672661,
0.025045320391654968,
-0.06606753915548325,
0.0037860695738345385,
0.055991411209106445,
-0.06006782501935959,
0.03443816676735878,
-0.027643967419862747,
-0.028836067765951157,
0.021725540980696678,
-0.05606852471828461,
0.009443148970603943,
0.04336109012365341,
0.07485811412334442,
-0.010363884270191193,
-0.056213751435279846,
-0.045973554253578186,
-0.0018558208830654621,
0.01472934428602457,
-0.020656300708651543,
-0.0856395959854126,
-0.01440014410763979,
-0.005222196690738201,
0.014992667362093925,
0.0034127982798963785,
-0.0705009251832962,
0.0537673644721508,
-0.07048096507787704,
-0.045201316475868225,
0.015401397831737995,
-0.051586639136075974,
-0.0030904498416930437,
-0.000250151235377416,
-0.03818097338080406,
0.04188523441553116,
0.03540883958339691,
0.009994682855904102,
0.006139433477073908,
-0.04176672548055649,
-0.003205850487574935,
-0.06846696883440018,
0.04935236647725105,
-0.0514088049530983,
0.03852672874927521,
0.0235530287027359,
0.12399992346763611,
-0.046665605157613754,
0.08855552226305008,
0.011555107310414314,
-0.017585361376404762,
-0.011721765622496605,
-0.03598259761929512,
-0.06666233390569687,
-0.04078086465597153,
0.0587032251060009,
0.057313837110996246,
0.0281258262693882,
-0.0028635927010327578,
0.03711472451686859,
0.02057710662484169,
0.09909451007843018,
0.0676782876253128,
-0.007246689405292273,
0.012118221260607243,
0.043390654027462006,
-0.009921746328473091,
0.08971000462770462,
0.0015660729259252548,
-5.733873109647902e-8,
-0.06048666313290596,
0.009257769212126732,
-0.03436711058020592,
0.1105896532535553,
-0.027660559862852097,
0.06881681084632874,
0.00930835586041212,
-0.017072536051273346,
-0.034163929522037506,
-0.07765676826238632,
0.08281008154153824,
0.035485271364450455,
-0.032901689410209656,
-0.03605104610323906,
0.0012338509550318122,
0.03683366999030113,
-0.06003119423985481,
0.03633110597729683,
-0.03242754563689232,
-0.012260505929589272,
0.10816782712936401,
-0.004060198552906513,
-0.023314764723181725,
0.07101254165172577,
-0.05955023691058159,
0.016294118016958237,
-0.10406143963336945,
0.05608370155096054,
-0.11483635753393173,
-0.08814556151628494,
-0.0014521072153002024,
0.01713910512626171,
-0.05678202211856842,
-0.05053733289241791,
0.022740403190255165,
0.09214646369218826,
0.06425457447767258,
-0.0951923057436943,
0.03238676115870476,
-0.011471232399344444,
0.031044239178299904,
-0.054507650434970856,
-0.11292225867509842,
0.003981805872172117,
0.05016225576400757,
-0.023013070225715637,
-0.027509408071637154,
-0.1253870576620102,
0.03267550840973854,
-0.027346748858690262,
0.012734884396195412,
-0.06725525110960007,
-0.052066922187805176,
-0.01679779402911663,
0.06727834790945053,
-0.009495023638010025,
-0.015467406250536442,
0.0068668886087834835,
0.00042178796138614416,
0.01363879069685936,
0.11327002197504044,
0.062235523015260696,
-0.03297409415245056,
-0.049579918384552
] |
dbmdz/bert-base-italian-uncased | d91243bae3a97a72691e9a6bfdf5d9f8fa4be9e4 | 2021-05-19T15:00:42.000Z | [
"pytorch",
"tf",
"jax",
"bert",
"fill-mask",
"it",
"dataset:wikipedia",
"transformers",
"license:mit",
"autotrain_compatible"
] | fill-mask | false | dbmdz | null | dbmdz/bert-base-italian-uncased | 1,984 | 2 | transformers | ---
language: it
license: mit
datasets:
- wikipedia
---
# 🤗 + 📚 dbmdz BERT and ELECTRA models
In this repository the MDZ Digital Library team (dbmdz) at the Bavarian State
Library open sources Italian BERT and ELECTRA models 🎉
# Italian BERT
The source data for the Italian BERT model consists of a recent Wikipedia dump and
various texts from the [OPUS corpora](http://opus.nlpl.eu/) collection. The final
training corpus has a size of 13GB and 2,050,057,573 tokens.
For sentence splitting, we use NLTK (faster compared to spacy).
Our cased and uncased models are training with an initial sequence length of 512
subwords for ~2-3M steps.
For the XXL Italian models, we use the same training data from OPUS and extend
it with data from the Italian part of the [OSCAR corpus](https://traces1.inria.fr/oscar/).
Thus, the final training corpus has a size of 81GB and 13,138,379,147 tokens.
Note: Unfortunately, a wrong vocab size was used when training the XXL models.
This explains the mismatch of the "real" vocab size of 31102, compared to the
vocab size specified in `config.json`. However, the model is working and all
evaluations were done under those circumstances.
See [this issue](https://github.com/dbmdz/berts/issues/7) for more information.
The Italian ELECTRA model was trained on the "XXL" corpus for 1M steps in total using a batch
size of 128. We pretty much following the ELECTRA training procedure as used for
[BERTurk](https://github.com/stefan-it/turkish-bert/tree/master/electra).
## Model weights
Currently only PyTorch-[Transformers](https://github.com/huggingface/transformers)
compatible weights are available. If you need access to TensorFlow checkpoints,
please raise an issue!
| Model | Downloads
| ---------------------------------------------------- | ---------------------------------------------------------------------------------------------------------------
| `dbmdz/bert-base-italian-cased` | [`config.json`](https://cdn.huggingface.co/dbmdz/bert-base-italian-cased/config.json) • [`pytorch_model.bin`](https://cdn.huggingface.co/dbmdz/bert-base-italian-cased/pytorch_model.bin) • [`vocab.txt`](https://cdn.huggingface.co/dbmdz/bert-base-italian-cased/vocab.txt)
| `dbmdz/bert-base-italian-uncased` | [`config.json`](https://cdn.huggingface.co/dbmdz/bert-base-italian-uncased/config.json) • [`pytorch_model.bin`](https://cdn.huggingface.co/dbmdz/bert-base-italian-uncased/pytorch_model.bin) • [`vocab.txt`](https://cdn.huggingface.co/dbmdz/bert-base-italian-uncased/vocab.txt)
| `dbmdz/bert-base-italian-xxl-cased` | [`config.json`](https://cdn.huggingface.co/dbmdz/bert-base-italian-xxl-cased/config.json) • [`pytorch_model.bin`](https://cdn.huggingface.co/dbmdz/bert-base-italian-xxl-cased/pytorch_model.bin) • [`vocab.txt`](https://cdn.huggingface.co/dbmdz/bert-base-italian-xxl-cased/vocab.txt)
| `dbmdz/bert-base-italian-xxl-uncased` | [`config.json`](https://cdn.huggingface.co/dbmdz/bert-base-italian-xxl-uncased/config.json) • [`pytorch_model.bin`](https://cdn.huggingface.co/dbmdz/bert-base-italian-xxl-uncased/pytorch_model.bin) • [`vocab.txt`](https://cdn.huggingface.co/dbmdz/bert-base-italian-xxl-uncased/vocab.txt)
| `dbmdz/electra-base-italian-xxl-cased-discriminator` | [`config.json`](https://s3.amazonaws.com/models.huggingface.co/bert/dbmdz/electra-base-italian-xxl-cased-discriminator/config.json) • [`pytorch_model.bin`](https://cdn.huggingface.co/dbmdz/electra-base-italian-xxl-cased-discriminator/pytorch_model.bin) • [`vocab.txt`](https://cdn.huggingface.co/dbmdz/electra-base-italian-xxl-cased-discriminator/vocab.txt)
| `dbmdz/electra-base-italian-xxl-cased-generator` | [`config.json`](https://s3.amazonaws.com/models.huggingface.co/bert/dbmdz/electra-base-italian-xxl-cased-generator/config.json) • [`pytorch_model.bin`](https://cdn.huggingface.co/dbmdz/electra-base-italian-xxl-cased-generator/pytorch_model.bin) • [`vocab.txt`](https://cdn.huggingface.co/dbmdz/electra-base-italian-xxl-cased-generator/vocab.txt)
## Results
For results on downstream tasks like NER or PoS tagging, please refer to
[this repository](https://github.com/stefan-it/italian-bertelectra).
## Usage
With Transformers >= 2.3 our Italian BERT models can be loaded like:
```python
from transformers import AutoModel, AutoTokenizer
model_name = "dbmdz/bert-base-italian-cased"
tokenizer = AutoTokenizer.from_pretrained(model_name)
model = AutoModel.from_pretrained(model_name)
```
To load the (recommended) Italian XXL BERT models, just use:
```python
from transformers import AutoModel, AutoTokenizer
model_name = "dbmdz/bert-base-italian-xxl-cased"
tokenizer = AutoTokenizer.from_pretrained(model_name)
model = AutoModel.from_pretrained(model_name)
```
To load the Italian XXL ELECTRA model (discriminator), just use:
```python
from transformers import AutoModel, AutoTokenizer
model_name = "dbmdz/electra-base-italian-xxl-cased-discriminator"
tokenizer = AutoTokenizer.from_pretrained(model_name)
model = AutoModelWithLMHead.from_pretrained(model_name)
```
# Huggingface model hub
All models are available on the [Huggingface model hub](https://huggingface.co/dbmdz).
# Contact (Bugs, Feedback, Contribution and more)
For questions about our BERT/ELECTRA models just open an issue
[here](https://github.com/dbmdz/berts/issues/new) 🤗
# Acknowledgments
Research supported with Cloud TPUs from Google's TensorFlow Research Cloud (TFRC).
Thanks for providing access to the TFRC ❤️
Thanks to the generous support from the [Hugging Face](https://huggingface.co/) team,
it is possible to download both cased and uncased models from their S3 storage 🤗
| [
-0.12498454004526138,
-0.07147540897130966,
0.04052244871854782,
0.006542092654854059,
0.020640812814235687,
0.04376183822751045,
-0.03273192048072815,
0.06505201011896133,
0.04264163225889206,
-0.0211293026804924,
0.00739902351051569,
-0.004474298097193241,
-0.022958645597100258,
0.03814525157213211,
0.014773232862353325,
0.04743432253599167,
-0.00021307790302671492,
0.03318164497613907,
-0.07638270407915115,
-0.013923517428338528,
0.05248960480093956,
0.03583260625600815,
0.050060249865055084,
-0.04940240830183029,
0.056047163903713226,
0.00044328131480142474,
-0.05043663829565048,
-0.04728589206933975,
0.059585314244031906,
-0.007592213340103626,
0.05404745414853096,
0.013073126785457134,
0.04950361326336861,
0.07780371606349945,
0.046797510236501694,
0.04504270851612091,
-0.009540412575006485,
-0.02298252284526825,
0.014746108092367649,
0.013288036920130253,
0.020289553329348564,
0.0399797186255455,
0.007611314300447702,
0.06601886451244354,
0.13683778047561646,
0.008140509948134422,
-0.06348061561584473,
0.028384512290358543,
-0.033145029097795486,
0.000465056044049561,
-0.09794432669878006,
0.02288021892309189,
0.025172358378767967,
0.1866016983985901,
-0.0334286093711853,
-0.0077613783068954945,
-0.007005505729466677,
0.02708148956298828,
-0.023638185113668442,
-0.04852624610066414,
-0.08648895472288132,
-0.03566216304898262,
-0.09802451729774475,
-0.03323129191994667,
-0.05655867978930473,
-0.007545363157987595,
-0.05167776718735695,
0.03606130927801132,
0.002982419915497303,
0.056965772062540054,
-0.04200463742017746,
0.058904409408569336,
-0.04948803409934044,
0.09705120325088501,
-0.07016117125749588,
0.015208118595182896,
0.09047840535640717,
-0.014605803415179253,
0.014933556318283081,
-0.14106401801109314,
0.032952211797237396,
-0.010509386658668518,
0.07723858207464218,
-0.026626726612448692,
0.04051816835999489,
-0.051385100930929184,
0.0500471256673336,
0.03749948740005493,
-0.029595790430903435,
0.030541136860847473,
-0.012098812498152256,
-0.05849812552332878,
0.08572371304035187,
-0.0164277795702219,
-0.013928684405982494,
0.013208271004259586,
0.047656286507844925,
0.011752328835427761,
0.028121566399931908,
0.10674791038036346,
0.05763169005513191,
0.061564765870571136,
0.018778512254357338,
-0.0583847314119339,
-0.03705809637904167,
-0.028425035998225212,
0.05771791189908981,
0.004840111825615168,
0.03436916694045067,
-0.04585037752985954,
0.007311003282666206,
0.06415402889251709,
-0.020468782633543015,
-0.05652232840657234,
0.04329171031713486,
-0.018596110865473747,
-0.03904455155134201,
-0.0038676259573549032,
0.06760073453187943,
0.10170800238847733,
-0.06940634548664093,
0.012994434684515,
-0.007726691663265228,
0.02597210183739662,
-0.0246561411768198,
-0.020375125110149384,
0.007415780331939459,
3.339119171179375e-33,
0.02810736931860447,
0.046207528561353683,
-0.027390167117118835,
-0.02636856958270073,
-0.04590104520320892,
0.021456874907016754,
-0.003578181378543377,
0.0299234502017498,
-0.08262316137552261,
0.014378078281879425,
-0.0993434339761734,
0.010736505500972271,
-0.06027475371956825,
0.03270807862281799,
0.020982161164283752,
0.025327695533633232,
-0.012232779525220394,
0.04799799993634224,
-0.0101996548473835,
0.0175267793238163,
0.06441860646009445,
0.001666825613938272,
0.02756473794579506,
-0.033563219010829926,
-0.020698804408311844,
-0.022855494171380997,
0.07631576806306839,
-0.09802012145519257,
-0.07846327126026154,
0.05603728070855141,
-0.15476278960704803,
0.010744299739599228,
-0.015284011140465736,
0.06527296453714371,
0.030740441754460335,
0.002980019198730588,
-0.047394003719091415,
-0.05857335403561592,
0.026679961010813713,
-0.06831510365009308,
-0.03413095697760582,
0.023328131064772606,
0.028735069558024406,
-0.02107648178935051,
-0.05709505453705788,
0.001064305892214179,
-0.025943245738744736,
-0.019602226093411446,
0.044968053698539734,
-0.029835505411028862,
0.08666768670082092,
0.01970306783914566,
-0.06678209453821182,
0.01609126292169094,
0.050415702164173126,
0.05125335976481438,
0.04267434403300285,
0.012459445744752884,
0.08477797359228134,
0.05411247909069061,
0.035219255834817886,
0.013550910167396069,
0.06379558891057968,
0.08726520091295242,
0.046761732548475266,
0.024728579446673393,
-0.10480578988790512,
0.010140450671315193,
0.03675716370344162,
-0.03127247095108032,
-0.0650620311498642,
-0.054758068174123764,
0.031182508915662766,
0.03386343643069267,
0.021408366039395332,
-0.034747425466775894,
0.07002581655979156,
-0.11546274274587631,
-0.05723569169640541,
0.0376538448035717,
0.005707211792469025,
-0.05849055200815201,
-0.012307226657867432,
-0.08249643445014954,
-0.057030562311410904,
-0.02180338464677334,
0.03398485109210014,
-0.06406387686729431,
-0.02829717844724655,
-0.0182411577552557,
0.009446290321648121,
-0.042463984340429306,
-0.03959795460104942,
-0.017405696213245392,
-0.00960163027048111,
-2.7392329962150756e-33,
-0.0011624033795669675,
0.019448790699243546,
-0.07094529271125793,
0.01313756313174963,
-0.06057184562087059,
-0.0871473178267479,
-0.02745390310883522,
0.16888895630836487,
-0.000990270054899156,
-0.006795684807002544,
0.014399449340999126,
-0.08510465174913406,
0.04631973057985306,
-0.05133597552776337,
0.01641441509127617,
-0.005971778649836779,
0.0074147554114460945,
0.05585873872041702,
0.059886395931243896,
0.08367715030908585,
0.0346740297973156,
-0.012075232341885567,
-0.09548452496528625,
0.06974640488624573,
-0.06030629202723503,
0.06298307329416275,
-0.05769311264157295,
0.0516727939248085,
-0.014756576158106327,
0.012797743082046509,
-0.05703878402709961,
-0.01385444588959217,
-0.06168140098452568,
-0.022115081548690796,
-0.08276398479938507,
-0.013722565956413746,
0.023327240720391273,
-0.005703356117010117,
0.023792143911123276,
0.043543264269828796,
0.063088059425354,
0.01587376557290554,
-0.048171982169151306,
0.04586717486381531,
0.005787954665720463,
-0.05177084356546402,
-0.14608506858348846,
-0.01948508247733116,
0.03178272396326065,
-0.037448134273290634,
0.00936514139175415,
0.009839586913585663,
-0.04391953721642494,
0.004269181750714779,
-0.0023104758001863956,
-0.12209293991327286,
-0.026864560320973396,
-0.0781085267663002,
-0.0685475617647171,
-0.019762925803661346,
-0.044346023350954056,
0.01982296071946621,
0.009836806915700436,
-0.0021994588896632195,
0.05324501171708107,
-0.06760197132825851,
-0.031352125108242035,
0.039677347987890244,
-0.02063819020986557,
-0.0051642428152263165,
-0.010285119526088238,
-0.020513949915766716,
0.012521807104349136,
0.07105756551027298,
-0.062192659825086594,
0.030875666067004204,
0.0054783569648861885,
-0.07574820518493652,
-0.022833630442619324,
-0.04968776926398277,
-0.020647794008255005,
-0.0022600076626986265,
0.05071530491113663,
0.03967204689979553,
0.0054221320897340775,
0.09751056879758835,
0.05506902560591698,
0.004654203541576862,
-0.007884598337113857,
0.04310626536607742,
-0.002460829447954893,
0.045461177825927734,
0.052081409841775894,
0.09528554230928421,
-0.00194712751545012,
-5.297954430716345e-8,
-0.035455428063869476,
0.0515703521668911,
-0.02789660170674324,
0.06134089082479477,
-0.020066654309630394,
-0.09035556018352509,
-0.07825298607349396,
0.08924761414527893,
0.012308508157730103,
-0.06035691872239113,
0.06578279286623001,
0.07355862855911255,
-0.10284532606601715,
0.003650607308372855,
-0.049144014716148376,
0.07591896504163742,
0.0037915457505732775,
-0.006680874619632959,
0.005442341323941946,
0.049888283014297485,
0.06265541911125183,
0.07253765314817429,
0.045494671911001205,
-0.02475634589791298,
0.0309684369713068,
-0.05210667848587036,
-0.03201683983206749,
0.1147666871547699,
-0.009377646259963512,
-0.05991372838616371,
-0.017156168818473816,
0.02436886541545391,
-0.08233232796192169,
-0.03305939957499504,
0.034850459545850754,
0.09879845380783081,
-0.030211301520466805,
-0.08478494733572006,
-0.02950950525701046,
-0.014486029744148254,
0.08360416442155838,
-0.008824186399579048,
-0.05558478832244873,
-0.0003103356284555048,
0.05851684883236885,
-0.05116251856088638,
-0.026308393105864525,
-0.09283085912466049,
0.05670680105686188,
-0.020705122500658035,
0.036290042102336884,
-0.022483404725790024,
-0.0466264933347702,
-0.009814205579459667,
0.049709856510162354,
0.06106751784682274,
-0.07387179881334305,
0.013170256279408932,
0.023372014984488487,
-0.014098810032010078,
-0.011001862585544586,
-0.03597194701433182,
-0.005817764438688755,
0.032177265733480453
] |
MoritzLaurer/DeBERTa-v3-large-mnli-fever-anli-ling-wanli | 1b7b1b212ea53c7a64546076569fbb01c3df8fbd | 2022-07-28T16:24:07.000Z | [
"pytorch",
"deberta-v2",
"text-classification",
"en",
"dataset:multi_nli",
"dataset:anli",
"dataset:fever",
"dataset:lingnli",
"dataset:alisawuffles/WANLI",
"arxiv:2104.07179",
"arxiv:2111.09543",
"transformers",
"zero-shot-classification",
"license:mit",
"model-index"
] | zero-shot-classification | false | MoritzLaurer | null | MoritzLaurer/DeBERTa-v3-large-mnli-fever-anli-ling-wanli | 1,980 | 4 | transformers | ---
language:
- en
tags:
- text-classification
- zero-shot-classification
license: mit
metrics:
- accuracy
datasets:
- multi_nli
- anli
- fever
- lingnli
- alisawuffles/WANLI
pipeline_tag: zero-shot-classification
#- text-classification
#widget:
#- text: "I first thought that I really liked the movie, but upon second thought it was actually disappointing. [SEP] The movie was not good."
model-index: # info: https://github.com/huggingface/hub-docs/blame/main/modelcard.md
- name: DeBERTa-v3-large-mnli-fever-anli-ling-wanli
results:
- task:
type: text-classification # Required. Example: automatic-speech-recognition
name: Natural Language Inference # Optional. Example: Speech Recognition
dataset:
type: multi_nli # Required. Example: common_voice. Use dataset id from https://hf.co/datasets
name: MultiNLI-matched # Required. A pretty name for the dataset. Example: Common Voice (French)
split: validation_matched # Optional. Example: test
metrics:
- type: accuracy # Required. Example: wer. Use metric id from https://hf.co/metrics
value: 0,912 # Required. Example: 20.90
#name: # Optional. Example: Test WER
verified: false # Optional. If true, indicates that evaluation was generated by Hugging Face (vs. self-reported).
- task:
type: text-classification # Required. Example: automatic-speech-recognition
name: Natural Language Inference # Optional. Example: Speech Recognition
dataset:
type: multi_nli # Required. Example: common_voice. Use dataset id from https://hf.co/datasets
name: MultiNLI-mismatched # Required. A pretty name for the dataset. Example: Common Voice (French)
split: validation_mismatched # Optional. Example: test
metrics:
- type: accuracy # Required. Example: wer. Use metric id from https://hf.co/metrics
value: 0,908 # Required. Example: 20.90
#name: # Optional. Example: Test WER
verified: false # Optional. If true, indicates that evaluation was generated by Hugging Face (vs. self-reported).
- task:
type: text-classification # Required. Example: automatic-speech-recognition
name: Natural Language Inference # Optional. Example: Speech Recognition
dataset:
type: anli # Required. Example: common_voice. Use dataset id from https://hf.co/datasets
name: ANLI-all # Required. A pretty name for the dataset. Example: Common Voice (French)
split: test_r1+test_r2+test_r3 # Optional. Example: test
metrics:
- type: accuracy # Required. Example: wer. Use metric id from https://hf.co/metrics
value: 0,702 # Required. Example: 20.90
#name: # Optional. Example: Test WER
verified: false # Optional. If true, indicates that evaluation was generated by Hugging Face (vs. self-reported).
- task:
type: text-classification # Required. Example: automatic-speech-recognition
name: Natural Language Inference # Optional. Example: Speech Recognition
dataset:
type: anli # Required. Example: common_voice. Use dataset id from https://hf.co/datasets
name: ANLI-r3 # Required. A pretty name for the dataset. Example: Common Voice (French)
split: test_r3 # Optional. Example: test
metrics:
- type: accuracy # Required. Example: wer. Use metric id from https://hf.co/metrics
value: 0,64 # Required. Example: 20.90
#name: # Optional. Example: Test WER
verified: false # Optional. If true, indicates that evaluation was generated by Hugging Face (vs. self-reported).
- task:
type: text-classification # Required. Example: automatic-speech-recognition
name: Natural Language Inference # Optional. Example: Speech Recognition
dataset:
type: alisawuffles/WANLI # Required. Example: common_voice. Use dataset id from https://hf.co/datasets
name: WANLI # Required. A pretty name for the dataset. Example: Common Voice (French)
split: test # Optional. Example: test
metrics:
- type: accuracy # Required. Example: wer. Use metric id from https://hf.co/metrics
value: 0,77 # Required. Example: 20.90
#name: # Optional. Example: Test WER
verified: false # Optional. If true, indicates that evaluation was generated by Hugging Face (vs. self-reported).
- task:
type: text-classification # Required. Example: automatic-speech-recognition
name: Natural Language Inference # Optional. Example: Speech Recognition
dataset:
type: lingnli # Required. Example: common_voice. Use dataset id from https://hf.co/datasets
name: LingNLI # Required. A pretty name for the dataset. Example: Common Voice (French)
split: test # Optional. Example: test
metrics:
- type: accuracy # Required. Example: wer. Use metric id from https://hf.co/metrics
value: 0,87 # Required. Example: 20.90
#name: # Optional. Example: Test WER
verified: false # Optional. If true, indicates that evaluation was generated by Hugging Face (vs. self-reported).
---
# DeBERTa-v3-large-mnli-fever-anli-ling-wanli
## Model description
This model was fine-tuned on the [MultiNLI](https://huggingface.co/datasets/multi_nli), [Fever-NLI](https://github.com/easonnie/combine-FEVER-NSMN/blob/master/other_resources/nli_fever.md), Adversarial-NLI ([ANLI](https://huggingface.co/datasets/anli)), [LingNLI](https://arxiv.org/pdf/2104.07179.pdf) and [WANLI](https://huggingface.co/datasets/alisawuffles/WANLI) datasets, which comprise 885 242 NLI hypothesis-premise pairs. This model is the best performing NLI model on the Hugging Face Hub as of 06.06.22 and can be used for zero-shot classification. It significantly outperforms all other large models on the [ANLI benchmark](https://github.com/facebookresearch/anli).
The foundation model is [DeBERTa-v3-large from Microsoft](https://huggingface.co/microsoft/deberta-v3-large). DeBERTa-v3 combines several recent innovations compared to classical Masked Language Models like BERT, RoBERTa etc., see the [paper](https://arxiv.org/abs/2111.09543)
## Intended uses & limitations
#### How to use the model
```python
from transformers import AutoTokenizer, AutoModelForSequenceClassification
import torch
device = torch.device("cuda") if torch.cuda.is_available() else torch.device("cpu")
model_name = "MoritzLaurer/DeBERTa-v3-large-mnli-fever-anli-ling-wanli"
tokenizer = AutoTokenizer.from_pretrained(model_name)
model = AutoModelForSequenceClassification.from_pretrained(model_name)
premise = "I first thought that I liked the movie, but upon second thought it was actually disappointing."
hypothesis = "The movie was not good."
input = tokenizer(premise, hypothesis, truncation=True, return_tensors="pt")
output = model(input["input_ids"].to(device)) # device = "cuda:0" or "cpu"
prediction = torch.softmax(output["logits"][0], -1).tolist()
label_names = ["entailment", "neutral", "contradiction"]
prediction = {name: round(float(pred) * 100, 1) for pred, name in zip(prediction, label_names)}
print(prediction)
```
### Training data
DeBERTa-v3-large-mnli-fever-anli-ling-wanli was trained on the [MultiNLI](https://huggingface.co/datasets/multi_nli), [Fever-NLI](https://github.com/easonnie/combine-FEVER-NSMN/blob/master/other_resources/nli_fever.md), Adversarial-NLI ([ANLI](https://huggingface.co/datasets/anli)), [LingNLI](https://arxiv.org/pdf/2104.07179.pdf) and [WANLI](https://huggingface.co/datasets/alisawuffles/WANLI) datasets, which comprise 885 242 NLI hypothesis-premise pairs. Note that [SNLI](https://huggingface.co/datasets/snli) was explicitly excluded due to quality issues with the dataset. More data does not necessarily make for better NLI models.
### Training procedure
DeBERTa-v3-large-mnli-fever-anli-ling-wanli was trained using the Hugging Face trainer with the following hyperparameters. Note that longer training with more epochs hurt performance in my tests (overfitting).
```
training_args = TrainingArguments(
num_train_epochs=4, # total number of training epochs
learning_rate=5e-06,
per_device_train_batch_size=16, # batch size per device during training
gradient_accumulation_steps=2, # doubles the effective batch_size to 32, while decreasing memory requirements
per_device_eval_batch_size=64, # batch size for evaluation
warmup_ratio=0.06, # number of warmup steps for learning rate scheduler
weight_decay=0.01, # strength of weight decay
fp16=True # mixed precision training
)
```
### Eval results
The model was evaluated using the test sets for MultiNLI, ANLI, LingNLI, WANLI and the dev set for Fever-NLI. The metric used is accuracy.
The model achieves state-of-the-art performance on each dataset. Surprisingly, it outperforms the previous [state-of-the-art on ANLI](https://github.com/facebookresearch/anli) (ALBERT-XXL) by 8,3%. I assume that this is because ANLI was created to fool masked language models like RoBERTa (or ALBERT), while DeBERTa-v3 uses a better pre-training objective (RTD), disentangled attention and I fine-tuned it on higher quality NLI data.
|Datasets|mnli_test_m|mnli_test_mm|anli_test|anli_test_r3|ling_test|wanli_test|
| :---: | :---: | :---: | :---: | :---: | :---: | :---: |
|Accuracy|0.912|0.908|0.702|0.64|0.87|0.77|
|Speed (text/sec, A100 GPU)|696.0|697.0|488.0|425.0|828.0|980.0|
## Limitations and bias
Please consult the original DeBERTa-v3 paper and literature on different NLI datasets for more information on the training data and potential biases. The model will reproduce statistical patterns in the training data.
## Citation
If you use this model, please cite: Laurer, Moritz, Wouter van Atteveldt, Andreu Salleras Casas, and Kasper Welbers. 2022. ‘Less Annotating, More Classifying – Addressing the Data Scarcity Issue of Supervised Machine Learning with Deep Transfer Learning and BERT - NLI’. Preprint, June. Open Science Framework. https://osf.io/74b8k.
### Ideas for cooperation or questions?
If you have questions or ideas for cooperation, contact me at m{dot}laurer{at}vu{dot}nl or [LinkedIn](https://www.linkedin.com/in/moritz-laurer/)
### Debugging and issues
Note that DeBERTa-v3 was released on 06.12.21 and older versions of HF Transformers seem to have issues running the model (e.g. resulting in an issue with the tokenizer). Using Transformers>=4.13 might solve some issues.
| [
-0.10130587220191956,
-0.075241819024086,
-0.013113987632095814,
-0.025018131360411644,
0.07778599858283997,
0.05180315300822258,
-0.024484779685735703,
0.0042124222964048386,
0.03419836238026619,
-0.10865657031536102,
0.04373316839337349,
-0.07330653071403503,
-0.009922200813889503,
0.01630418375134468,
-0.02493094839155674,
-0.014354174956679344,
0.04775156453251839,
-0.023299146443605423,
-0.0866948738694191,
-0.06551526486873627,
0.036861956119537354,
0.12020102888345718,
0.07921408861875534,
0.042192377150058746,
-0.01740843430161476,
-0.00944508332759142,
-0.024277864024043083,
0.05081075802445412,
-0.02972331829369068,
-0.03601200506091118,
0.0270856823772192,
0.11168907582759857,
0.020426273345947266,
0.02021598070859909,
0.017811985686421394,
0.025431763380765915,
-0.029017839580774307,
-0.030460339039564133,
-0.03938259556889534,
0.012201757170259953,
0.017666470259428024,
-0.012636744417250156,
0.05547269061207771,
-0.035878539085388184,
0.06455942988395691,
-0.04141198471188545,
-0.13160797953605652,
-0.057241275906562805,
0.02228955179452896,
0.009307881817221642,
-0.1294085681438446,
-0.016720028594136238,
0.023248573765158653,
0.06312388926744461,
-0.06095816567540169,
-0.04507382586598396,
0.0006116767763160169,
-0.03251082822680473,
0.01780514419078827,
-0.028715943917632103,
0.009931232780218124,
-0.05051204934716225,
-0.01061389409005642,
0.02664896659553051,
0.026581568643450737,
-0.002473903354257345,
-0.014905272983014584,
-0.014432879164814949,
0.025130121037364006,
0.03239878639578819,
-0.03598504513502121,
0.06365619599819183,
0.07207303494215012,
0.00044926785631105304,
-0.0027224072255194187,
-0.02058217115700245,
-0.0009111214894801378,
0.02662012353539467,
0.04152929037809372,
-0.09564941376447678,
-0.0036800773814320564,
-0.05899535119533539,
0.05270896852016449,
-0.015506642870604992,
0.052959296852350235,
-0.026291633024811745,
0.009795169346034527,
0.01769506186246872,
-0.016057444736361504,
-0.016982771456241608,
-0.08281929790973663,
-0.03149157017469406,
0.08974071592092514,
0.021000050008296967,
0.015844134613871574,
0.0640973150730133,
-0.014156742952764034,
0.028555821627378464,
-0.048096831887960434,
0.0860631987452507,
-0.06658738851547241,
-0.052808452397584915,
-0.01316750980913639,
-0.026864785701036453,
-0.003970892634242773,
-0.04572910815477371,
0.08064483851194382,
0.0013111622538417578,
0.056550659239292145,
-0.09307368099689484,
-0.023320088163018227,
-0.015190012753009796,
-0.045836467295885086,
-0.08934830129146576,
0.04122884199023247,
-0.008213086985051632,
0.0027775838971138,
-0.03095499612390995,
0.018141131848096848,
0.05681731924414635,
-0.036758601665496826,
-0.0032938828226178885,
-0.045694999396800995,
-0.077986940741539,
0.002583189867436886,
-0.0052038440480828285,
-0.058112237602472305,
2.8302761357122157e-33,
0.09283210337162018,
0.05141240358352661,
0.03498391434550285,
0.0029217195697128773,
0.034294940531253815,
-0.09071364998817444,
-0.1097055971622467,
0.002394870389252901,
-0.08663354068994522,
-0.012330652214586735,
-0.07371285557746887,
0.015163077041506767,
-0.10391995310783386,
0.025998175144195557,
-0.000011887025721080136,
-0.022046798840165138,
-0.050147514790296555,
0.04966820403933525,
0.03403544798493385,
0.03771317005157471,
0.0759163573384285,
0.028877360746264458,
0.0218028724193573,
-0.059741728007793427,
-0.014498081989586353,
0.08442997187376022,
0.05725269019603729,
-0.06349048018455505,
0.000058079160226043314,
0.04118497297167778,
-0.09818416833877563,
0.02358139678835869,
0.07907243818044662,
-0.040459420531988144,
0.04739702492952347,
-0.03885261341929436,
-0.12500502169132233,
0.01510975044220686,
-0.005577231757342815,
-0.03162997588515282,
-0.013944689184427261,
0.041173648089170456,
-0.09687766432762146,
-0.048957377672195435,
-0.047672245651483536,
0.030903125181794167,
-0.018274132162332535,
-0.017008500173687935,
0.04697100818157196,
0.06660269945859909,
0.03462772071361542,
-0.04000423848628998,
-0.0011193660320714116,
-0.027691973373293877,
-0.06647631525993347,
0.030539274215698242,
0.02042798139154911,
0.020020518451929092,
0.049250368028879166,
-0.01804381050169468,
0.007892046123743057,
-0.02658982016146183,
-0.026410706341266632,
0.006090909708291292,
0.05503574758768082,
0.06898283213376999,
0.007822327315807343,
0.01742684468626976,
0.054494958370923996,
-0.008898315951228142,
-0.0865502879023552,
-0.012595012784004211,
0.0037515268195420504,
0.05077719688415527,
0.08916565775871277,
0.010533520020544529,
-0.02942475490272045,
-0.11452789604663849,
-0.06572368741035461,
0.08518102765083313,
-0.06819798797369003,
-0.044733013957738876,
0.012723595835268497,
-0.049772173166275024,
-0.05163600295782089,
-0.03848377615213394,
0.015440220013260841,
-0.06288275122642517,
0.04742005094885826,
-0.032561931759119034,
-0.016880186274647713,
0.035813577473163605,
-0.04032035917043686,
0.0005015071365050972,
0.004460697527974844,
-3.984802422380718e-33,
0.020975597202777863,
-0.004163156263530254,
-0.07646646350622177,
0.019363466650247574,
0.00805276446044445,
0.009745120070874691,
0.033968351781368256,
0.11858850717544556,
0.11140590906143188,
0.021280651912093163,
0.07400957494974136,
-0.01854904368519783,
-0.00952118169516325,
-0.024595651775598526,
0.025265326723456383,
0.04551650583744049,
0.025174656882882118,
-0.042776573449373245,
0.01692504622042179,
0.08601304888725281,
0.06867201626300812,
0.01728859543800354,
-0.10100586712360382,
0.03194280341267586,
-0.05132315307855606,
0.06743372976779938,
0.00005055987276136875,
0.03507581725716591,
-0.001028505270369351,
-0.07767243683338165,
-0.01639488898217678,
-0.0355154313147068,
-0.08218271285295486,
-0.00004720540891867131,
-0.10721627622842789,
0.053083330392837524,
0.019370341673493385,
-0.04138341173529625,
-0.03558092936873436,
0.06975989788770676,
0.06544613838195801,
0.04283270239830017,
-0.1436316967010498,
0.019321974366903305,
-0.005072321277111769,
-0.061615705490112305,
-0.023952605202794075,
0.04395586624741554,
-0.013341404497623444,
-0.0341741219162941,
-0.020949603989720345,
0.005266835913062096,
-0.06537336111068726,
-0.007101231254637241,
-0.018850557506084442,
-0.07086025923490524,
0.0249044056981802,
-0.06582657247781754,
-0.04035838693380356,
0.06617303192615509,
-0.038654595613479614,
0.0065021519549191,
-0.04981827363371849,
-0.022896122187376022,
0.003531542606651783,
0.019181378185749054,
-0.004507931414991617,
-0.027178434655070305,
0.0570959746837616,
-0.004954512696713209,
0.038122180849313736,
0.03820527344942093,
-0.01819346845149994,
0.0007328112842515111,
-0.014951026067137718,
0.0001673976075835526,
-0.06149931997060776,
0.005069802049547434,
-0.009646642953157425,
-0.04952764883637428,
0.001565161976031959,
-0.05326894298195839,
0.04184070602059364,
0.03819374367594719,
0.13247545063495636,
0.1029905155301094,
0.05370127409696579,
0.044294897466897964,
0.030861305072903633,
0.01455654762685299,
-0.01826540194451809,
0.03882129490375519,
0.026198148727416992,
0.15973567962646484,
-0.004736847709864378,
-5.579185824444721e-8,
-0.04305500537157059,
0.017845112830400467,
-0.054847847670316696,
0.019075490534305573,
-0.07553663849830627,
-0.07535701245069504,
-0.04140353947877884,
0.06780490279197693,
0.042392730712890625,
-0.009034681133925915,
0.05159813165664673,
0.04539671167731285,
-0.1048576757311821,
0.037921082228422165,
-0.04086805880069733,
0.03496198356151581,
0.022192195057868958,
0.14484022557735443,
-0.018542267382144928,
-0.014096192084252834,
0.04489303380250931,
-0.022210005670785904,
0.04651691019535065,
-0.07495730370283127,
0.0659223347902298,
-0.05686398223042488,
-0.03729720041155815,
0.03618432581424713,
0.0012937452411279082,
-0.06849569082260132,
-0.014609032310545444,
0.05357636883854866,
-0.08347959071397781,
-0.04491144046187401,
0.011776650324463844,
0.06627393513917923,
0.00024131972168106586,
-0.02742314524948597,
0.0041688792407512665,
0.02476215921342373,
0.0781344622373581,
0.09695327281951904,
-0.06700310856103897,
-0.03189047798514366,
0.07850615680217743,
0.03114476054906845,
0.020451588556170464,
-0.10448368638753891,
0.07719668745994568,
-0.0016002415213733912,
0.005807155277580023,
0.01482232753187418,
-0.08166863024234772,
0.09801987558603287,
0.06695781648159027,
0.012922899797558784,
0.021890681236982346,
0.02621966414153576,
0.011618363671004772,
-0.008310558274388313,
0.09602658450603485,
-0.02538982778787613,
0.019926823675632477,
0.0329098179936409
] |
kamalkraj/bioelectra-base-discriminator-pubmed | b08ce00d5a23e2682a20e9d33356730530bdecd1 | 2021-09-07T13:52:16.000Z | [
"pytorch",
"electra",
"pretraining",
"transformers"
] | null | false | kamalkraj | null | kamalkraj/bioelectra-base-discriminator-pubmed | 1,978 | 3 | transformers | ## BioELECTRA:Pretrained Biomedical text Encoder using Discriminators
Recent advancements in pretraining strategies in NLP have shown a significant improvement in the performance of models on various text mining tasks. In this paper, we introduce BioELECTRA, a biomedical domain-specific language encoder model that adapts ELECTRA (Clark et al., 2020) for the Biomedical domain. BioELECTRA outperforms the previous models and achieves state of the art (SOTA) on all the 13 datasets in BLURB benchmark and on all the 4 Clinical datasets from BLUE Benchmark across 7 NLP tasks. BioELECTRA pretrained on PubMed and PMC full text articles performs very well on Clinical datasets as well. BioELECTRA achieves new SOTA 86.34%(1.39% accuracy improvement) on MedNLI and 64% (2.98% accuracy improvement) on PubMedQA dataset.
For a detailed description and experimental results, please refer to our paper [BioELECTRA:Pretrained Biomedical text Encoder using Discriminators](https://www.aclweb.org/anthology/2021.bionlp-1.16/).
Cite our paper using below citation
```
@inproceedings{kanakarajan-etal-2021-bioelectra,
title = "{B}io{ELECTRA}:Pretrained Biomedical text Encoder using Discriminators",
author = "Kanakarajan, Kamal raj and
Kundumani, Bhuvana and
Sankarasubbu, Malaikannan",
booktitle = "Proceedings of the 20th Workshop on Biomedical Language Processing",
month = jun,
year = "2021",
address = "Online",
publisher = "Association for Computational Linguistics",
url = "https://aclanthology.org/2021.bionlp-1.16",
doi = "10.18653/v1/2021.bionlp-1.16",
pages = "143--154",
abstract = "Recent advancements in pretraining strategies in NLP have shown a significant improvement in the performance of models on various text mining tasks. We apply {`}replaced token detection{'} pretraining technique proposed by ELECTRA and pretrain a biomedical language model from scratch using biomedical text and vocabulary. We introduce BioELECTRA, a biomedical domain-specific language encoder model that adapts ELECTRA for the Biomedical domain. WE evaluate our model on the BLURB and BLUE biomedical NLP benchmarks. BioELECTRA outperforms the previous models and achieves state of the art (SOTA) on all the 13 datasets in BLURB benchmark and on all the 4 Clinical datasets from BLUE Benchmark across 7 different NLP tasks. BioELECTRA pretrained on PubMed and PMC full text articles performs very well on Clinical datasets as well. BioELECTRA achieves new SOTA 86.34{\%}(1.39{\%} accuracy improvement) on MedNLI and 64{\%} (2.98{\%} accuracy improvement) on PubMedQA dataset.",
}
```
## How to use the discriminator in `transformers`
```python
from transformers import ElectraForPreTraining, ElectraTokenizerFast
import torch
discriminator = ElectraForPreTraining.from_pretrained("kamalkraj/bioelectra-base-discriminator-pubmed")
tokenizer = ElectraTokenizerFast.from_pretrained("kamalkraj/bioelectra-base-discriminator-pubmed")
sentence = "The quick brown fox jumps over the lazy dog"
fake_sentence = "The quick brown fox fake over the lazy dog"
fake_tokens = tokenizer.tokenize(fake_sentence)
fake_inputs = tokenizer.encode(fake_sentence, return_tensors="pt")
discriminator_outputs = discriminator(fake_inputs)
predictions = torch.round((torch.sign(discriminator_outputs[0]) + 1) / 2)
[print("%7s" % token, end="") for token in fake_tokens]
[print("%7s" % int(prediction), end="") for prediction in predictions[0].tolist()]
``` | [
-0.048747170716524124,
-0.04893111437559128,
-0.015514216385781765,
-0.03868996724486351,
-0.004656575154513121,
0.015299046412110329,
-0.031510304659605026,
0.006487579550594091,
0.013422035612165928,
-0.015993250533938408,
-0.0350366085767746,
-0.01311967521905899,
-0.03418135643005371,
0.03687208890914917,
-0.009974954649806023,
0.048269160091876984,
0.03937417268753052,
0.023297958076000214,
-0.08805263787508011,
-0.028495503589510918,
0.05981229618191719,
0.08828601241111755,
0.07127442210912704,
0.0591689832508564,
0.05469048395752907,
-0.008966343477368355,
-0.004947359673678875,
-0.007438819855451584,
-0.027663689106702805,
0.015052870847284794,
0.057905759662389755,
0.029994668439030647,
0.09426417946815491,
0.03942694514989853,
-0.09002995491027832,
0.01068020612001419,
-0.06005021557211876,
0.002421562559902668,
0.015133972279727459,
-0.03344067558646202,
0.01915709488093853,
-0.025661488994956017,
0.016714224591851234,
0.07885856181383133,
0.094540074467659,
-0.028855200856924057,
-0.025195615366101265,
-0.007258655037730932,
0.03882303088903427,
0.01484126690775156,
-0.08108720183372498,
-0.0022962403018027544,
0.03725811839103699,
0.0980892926454544,
-0.04480045661330223,
0.026541415601968765,
0.0066390675492584705,
-0.0382826067507267,
-0.052636872977018356,
-0.03454998508095741,
-0.025161487981677055,
-0.07937739789485931,
0.02835587039589882,
-0.008850052952766418,
0.004350640345364809,
-0.07269241660833359,
0.038948904722929,
-0.015059649012982845,
-0.04135024920105934,
-0.026012727990746498,
0.003596816211938858,
0.05769554525613785,
0.006586230825632811,
0.12522293627262115,
-0.0016390591626986861,
0.052588801831007004,
0.07929012924432755,
0.03242895379662514,
0.0923914685845375,
-0.11810814589262009,
0.026395158842206,
-0.030058208853006363,
0.06455506384372711,
0.05233479663729668,
0.08849865943193436,
-0.026754505932331085,
0.05129091441631317,
0.03160376474261284,
-0.10493212938308716,
0.02432529255747795,
0.01636185683310032,
-0.1059616208076477,
0.1408795863389969,
-0.04496970400214195,
-0.08828329294919968,
0.015237826853990555,
-0.006284519098699093,
-0.002410969464108348,
0.018696511164307594,
-0.012692305259406567,
-0.015251610428094864,
0.00650166068226099,
-0.06742959469556808,
-0.05295579880475998,
-0.08978328108787537,
-0.07041069865226746,
0.0904431864619255,
0.011601871810853481,
0.08324392884969711,
-0.022651957347989082,
0.04253785312175751,
0.04521860554814339,
-0.02817101590335369,
0.026628464460372925,
0.032538700848817825,
0.05845779553055763,
0.007724709343165159,
-0.03141391649842262,
0.08583728969097137,
0.054823216050863266,
-0.11859945207834244,
-0.02716081589460373,
-0.01798304170370102,
0.012474656105041504,
0.008149358443915844,
0.01392007153481245,
-0.04600091278553009,
5.740467152839131e-33,
0.08548460900783539,
0.029209505766630173,
0.007621414493769407,
-0.034706976264715195,
-0.0170849971473217,
-0.023549329489469528,
-0.051736604422330856,
-0.02439485862851143,
-0.07481879740953445,
-0.06802892684936523,
-0.09149911999702454,
-0.025105368345975876,
0.0067794183269143105,
0.06293052434921265,
0.04490029066801071,
-0.03491179272532463,
-0.12755639851093292,
-0.013256537728011608,
0.01343111228197813,
0.019184408709406853,
0.06339436024427414,
0.0016293764347210526,
-0.02364908903837204,
0.0036961243022233248,
-0.01711912639439106,
0.03385253995656967,
-0.02300284057855606,
-0.058204326778650284,
-0.004918698687106371,
0.0390762984752655,
-0.12228763103485107,
-0.0026522206608206034,
0.04327738657593727,
-0.015567746013402939,
0.08212099224328995,
-0.02429693192243576,
0.036394666880369186,
0.007718491833657026,
-0.010705985128879547,
-0.0034327509347349405,
-0.037123847752809525,
0.04576700180768967,
0.05139230191707611,
-0.059523265808820724,
0.00026073356275446713,
-0.028735266998410225,
-0.0600719079375267,
0.038944121450185776,
0.020439840853214264,
0.03707255423069,
0.03871726244688034,
-0.02663593925535679,
-0.019260697066783905,
-0.0062150112353265285,
0.009537051431834698,
0.027213238179683685,
-0.008897754363715649,
0.012042243964970112,
0.09441708028316498,
0.06613460183143616,
0.06466583907604218,
0.0542912483215332,
0.0498664565384388,
0.02436014637351036,
0.03873521462082863,
-0.0347413532435894,
0.008827585726976395,
0.01725638099014759,
-0.035573456436395645,
0.025887032970786095,
-0.0055341762490570545,
0.040107257664203644,
0.00040339125553146005,
-0.011751147918403149,
0.03638159856200218,
0.06711773574352264,
0.0030346259009093046,
-0.08592862635850906,
-0.07959814369678497,
0.02024340257048607,
0.0009617017931304872,
-0.012761203572154045,
-0.04587724432349205,
-0.0617191381752491,
-0.006193534936755896,
-0.06499894708395004,
0.0097924480214715,
-0.0455133356153965,
-0.0510682687163353,
0.0036193602718412876,
0.0020197720732539892,
0.004302012734115124,
0.0030299799982458353,
-0.026643168181180954,
0.012882180511951447,
-5.602993455852449e-33,
-0.036983098834753036,
0.013432254083454609,
-0.03355947136878967,
0.07891395688056946,
0.008026398718357086,
-0.02910449169576168,
0.015481241047382355,
0.05907292664051056,
0.03916321322321892,
-0.047512706369161606,
0.07137112319469452,
-0.029433606192469597,
0.03657536953687668,
-0.006329938769340515,
0.03827708587050438,
0.013539721257984638,
-0.09719154983758926,
0.06017640233039856,
-0.040451034903526306,
0.08221365511417389,
0.008026707917451859,
0.06660216301679611,
-0.11945311725139618,
0.07760652154684067,
0.024523332715034485,
0.04004354029893875,
-0.04442431777715683,
0.08217008411884308,
-0.01504739560186863,
-0.1333765834569931,
-0.061461057513952255,
0.025121277198195457,
-0.11126445233821869,
-0.04968144744634628,
-0.08190622925758362,
0.01323104090988636,
0.03713707998394966,
-0.07914196699857712,
0.030796192586421967,
0.017432864755392075,
0.08971191942691803,
0.04587980732321739,
-0.1421416699886322,
0.023657694458961487,
-0.020135080441832542,
-0.050267480313777924,
-0.16766300797462463,
-0.009066306985914707,
0.06964356452226639,
0.0717427060008049,
-0.01934918388724327,
0.0027890498749911785,
-0.046735890209674835,
0.05554012954235077,
0.027769122272729874,
-0.17476217448711395,
-0.05139787867665291,
-0.04516681283712387,
-0.06824254989624023,
-0.0015540296444669366,
-0.09856569021940231,
0.03730475530028343,
0.040746886283159256,
0.028626734390854836,
0.011659528128802776,
-0.10782201588153839,
0.040774669498205185,
0.02719959430396557,
-0.030480114743113518,
0.0019317013211548328,
0.019099444150924683,
-0.04244891181588173,
-0.017228471115231514,
0.05172694846987724,
0.027836475521326065,
-0.026732955127954483,
-0.002089005196467042,
-0.03689580783247948,
-0.05738614872097969,
-0.02381497621536255,
0.03970490023493767,
0.013440822251141071,
0.0013174673076719046,
0.025506997480988503,
0.05997597053647041,
0.10136914253234863,
0.012760650366544724,
0.006810190621763468,
0.00977540947496891,
0.04447334632277489,
-0.03526165708899498,
0.04584905132651329,
0.005822503007948399,
0.023145468905568123,
-0.08372804522514343,
-4.50887895908636e-8,
-0.01959838904440403,
-0.04240763559937477,
-0.06654133647680283,
0.019836677238345146,
-0.033606257289648056,
-0.05928324908018112,
-0.08783889561891556,
0.09797456860542297,
-0.011916824616491795,
-0.07405474781990051,
0.0887230709195137,
0.014967404305934906,
-0.11444579809904099,
-0.048019591718912125,
0.031224094331264496,
0.04624580219388008,
0.043414488434791565,
0.04066905379295349,
0.02703481912612915,
-0.07947428524494171,
0.021382370963692665,
0.02235407382249832,
0.018903275951743126,
0.010072524659335613,
0.05070485547184944,
-0.03254159539937973,
-0.07364804297685623,
0.0018729671137407422,
0.041663989424705505,
-0.056638892740011215,
0.003016319591552019,
0.019668344408273697,
0.04487599804997444,
-0.02649368718266487,
0.005876142531633377,
0.03660508990287781,
0.027674691751599312,
-0.02468123659491539,
-0.05239778384566307,
0.039110034704208374,
0.08566399663686752,
0.003540431149303913,
-0.09664841741323471,
-0.03661542013287544,
0.03462190926074982,
-0.050480738282203674,
0.037139441817998886,
-0.017223967239260674,
0.03977663815021515,
-0.11132653802633286,
-0.008469498716294765,
-0.029190003871917725,
0.0019394728587940335,
0.014764802530407906,
0.04952642694115639,
0.09575806558132172,
-0.023362746462225914,
-0.005701456684619188,
0.04772565886378288,
-0.020611407235264778,
0.03249702230095863,
0.007466966286301613,
-0.0072782584466040134,
0.011241558939218521
] |
mbartolo/roberta-large-synqa | 1ae8322fd562c2b2193a7d2b8d0887177b616d62 | 2022-07-25T23:36:39.000Z | [
"pytorch",
"roberta",
"question-answering",
"en",
"dataset:adversarial_qa",
"dataset:mbartolo/synQA",
"dataset:squad",
"arxiv:2002.00293",
"arxiv:2104.08678",
"transformers",
"license:apache-2.0",
"model-index",
"autotrain_compatible"
] | question-answering | false | mbartolo | null | mbartolo/roberta-large-synqa | 1,973 | null | transformers | ---
language:
- en
tags:
- question-answering
license: apache-2.0
datasets:
- adversarial_qa
- mbartolo/synQA
- squad
metrics:
- exact_match
- f1
model-index:
- name: mbartolo/roberta-large-synqa
results:
- task:
type: question-answering
name: Question Answering
dataset:
name: squad
type: squad
config: plain_text
split: validation
metrics:
- name: Exact Match
type: exact_match
value: 89.6529
verified: true
- name: F1
type: f1
value: 94.8172
verified: true
- task:
type: question-answering
name: Question Answering
dataset:
name: adversarial_qa
type: adversarial_qa
config: adversarialQA
split: validation
metrics:
- name: Exact Match
type: exact_match
value: 55.3333
verified: true
- name: F1
type: f1
value: 66.7464
verified: true
---
# Model Overview
This is a RoBERTa-Large QA Model trained from https://huggingface.co/roberta-large in two stages. First, it is trained on synthetic adversarial data generated using a BART-Large question generator on Wikipedia passages from SQuAD, and then it is trained on SQuAD and AdversarialQA (https://arxiv.org/abs/2002.00293) in a second stage of fine-tuning.
# Data
Training data: SQuAD + AdversarialQA
Evaluation data: SQuAD + AdversarialQA
# Training Process
Approx. 1 training epoch on the synthetic data and 2 training epochs on the manually-curated data.
# Additional Information
Please refer to https://arxiv.org/abs/2104.08678 for full details. | [
-0.10828176885843277,
-0.040744077414274216,
-0.04300656169652939,
0.024325594305992126,
0.03482784330844879,
-0.000036329405702417716,
-0.03341180831193924,
-0.03643177077174187,
0.018875807523727417,
-0.016250116750597954,
0.01248828787356615,
-0.11017307639122009,
-0.0170422475785017,
0.03166010603308678,
-0.022669797763228416,
0.027704237028956413,
0.04750446975231171,
-0.05450354143977165,
-0.13918252289295197,
-0.026985833421349525,
-0.0020455101039260626,
0.03608481585979462,
0.07656878978013992,
-0.00818209070712328,
-0.0049974448047578335,
-0.03605823218822479,
-0.0658096969127655,
0.046652019023895264,
-0.04865624010562897,
-0.07245822250843048,
-0.005544228479266167,
0.09483584016561508,
-0.0005185077898204327,
0.041380178183317184,
0.01845838874578476,
0.11472912132740021,
-0.02576921321451664,
-0.04521254822611809,
0.05491139367222786,
-0.03780422732234001,
-0.020616980269551277,
-0.08592887222766876,
-0.028594940900802612,
-0.011046208441257477,
0.06740779429674149,
-0.08117420971393585,
-0.05067085102200508,
-0.03973397985100746,
0.04786418005824089,
-0.024369243532419205,
-0.12686876952648163,
-0.013425005599856377,
-0.008242152631282806,
0.0885460153222084,
-0.007579449098557234,
0.0038178812246769667,
-0.04095767065882683,
-0.013370370492339134,
-0.011669902130961418,
0.0028000869788229465,
-0.04067130386829376,
-0.015020082704722881,
-0.09542118012905121,
-0.00749337300658226,
-0.038070231676101685,
-0.0626559928059578,
-0.06899066269397736,
-0.035975582897663116,
0.00932550709694624,
0.022820910438895226,
0.0023691938258707523,
0.004865327849984169,
-0.06389815360307693,
0.04470742866396904,
0.018217751756310463,
0.029933324083685875,
-0.014129147864878178,
0.043855808675289154,
0.045048125088214874,
-0.11111515760421753,
0.02243768982589245,
-0.04005768895149231,
0.011921768076717854,
0.019543610513210297,
0.043737877160310745,
0.003137212945148349,
-0.01906477101147175,
0.05245957151055336,
0.023043327033519745,
0.006179213058203459,
-0.05457013472914696,
0.00470264395698905,
0.014437886886298656,
0.03460894152522087,
0.0393906831741333,
0.0006668050773441792,
0.01625842973589897,
0.046472951769828796,
-0.04830983653664589,
0.11598605662584305,
0.010054218582808971,
0.013238177634775639,
-0.004101229831576347,
-0.0011931753251701593,
0.006598273292183876,
0.0019066232489421964,
0.03397933766245842,
0.12873144447803497,
0.03972281143069267,
-0.05601341649889946,
0.03809422627091408,
0.0234671700745821,
-0.0026758864987641573,
-0.0043808650225400925,
0.021713024005293846,
0.0568009614944458,
-0.013260805048048496,
0.08672020584344864,
-0.06697050482034683,
0.060567475855350494,
0.016158299520611763,
0.004297312349081039,
0.02280421182513237,
-0.042628925293684006,
0.0490000918507576,
0.005666377954185009,
-0.05783688649535179,
1.0357464396087491e-32,
0.09388609230518341,
0.0259252842515707,
0.08395852148532867,
0.01408479269593954,
-0.011879597790539265,
0.04314953088760376,
-0.058111172169446945,
0.05852517485618591,
-0.07864293456077576,
0.02902097813785076,
-0.053150858730077744,
0.015142884105443954,
-0.05907128006219864,
0.01995457150042057,
0.035413436591625214,
0.028545334935188293,
-0.04123327508568764,
-0.018513517454266548,
-0.06809806823730469,
0.02024378813803196,
0.10985088348388672,
-0.020036980509757996,
-0.029693342745304108,
0.005659925751388073,
0.026042239740490913,
0.0020553034264594316,
0.06614551693201065,
-0.020046664401888847,
-0.052507828921079636,
0.04132074490189552,
-0.15500907599925995,
-0.007157475221902132,
-0.05429136008024216,
-0.05015242099761963,
-0.0019581844098865986,
-0.01916470378637314,
-0.04390912503004074,
-0.06851164251565933,
-0.018359001725912094,
-0.01796608790755272,
0.010313840582966805,
0.025498993694782257,
-0.001988001400604844,
-0.044149238616228104,
-0.06229960173368454,
-0.05131912976503372,
-0.00659949192777276,
0.022243380546569824,
0.02740527130663395,
-0.00633164681494236,
-0.012377139180898666,
-0.0243814829736948,
0.002865996677428484,
-0.03344235569238663,
-0.09592041373252869,
0.05953838303685188,
0.0728021040558815,
0.1400618553161621,
-0.0331549309194088,
0.039331335574388504,
0.024097774177789688,
-0.08389299362897873,
-0.08121968805789948,
0.02214697375893593,
-0.020634565502405167,
-0.013167877681553364,
-0.054802946746349335,
0.025052040815353394,
0.08035195618867874,
0.09968205541372299,
-0.013598484918475151,
0.004600186366587877,
0.050596099346876144,
0.08111272752285004,
0.06199335679411888,
-0.12066398561000824,
0.036605313420295715,
-0.004197787027806044,
-0.0011607939377427101,
0.010418771766126156,
-0.04008759185671806,
0.04559611901640892,
-0.014442535117268562,
-0.016455141827464104,
-0.10368390381336212,
-0.01735704019665718,
0.03812697157263756,
-0.05244995653629303,
-0.06856958568096161,
-0.018949970602989197,
-0.0415072925388813,
0.054749127477407455,
-0.03928279131650925,
-0.04548916220664978,
-0.05810605734586716,
-1.0210388012280545e-32,
0.022569607943296432,
0.04339877888560295,
-0.03283511847257614,
0.023859379813075066,
0.07172466069459915,
-0.004022506065666676,
0.03882381692528725,
0.14611874520778656,
-0.007009698543697596,
0.02361837960779667,
0.0603070892393589,
-0.007540406193584204,
0.050890300422906876,
-0.09900403022766113,
0.013091092929244041,
-0.029596582055091858,
-0.0634668841958046,
-0.06510752439498901,
-0.00037686683936044574,
0.046738699078559875,
-0.017234794795513153,
0.0588211864233017,
0.02622995898127556,
0.0670081228017807,
-0.0011708798119798303,
-0.03361694514751434,
0.013103820383548737,
0.07812431454658508,
0.0011084212455898523,
0.005581504199653864,
0.028509004041552544,
-0.01345419604331255,
-0.1279359757900238,
-0.02494092844426632,
-0.031962327659130096,
-0.002924496540799737,
0.041833873838186264,
-0.0210280641913414,
-0.03243853896856308,
0.14908826351165771,
0.04260535165667534,
0.01915927045047283,
-0.09598319977521896,
0.03587917238473892,
-0.02941424399614334,
-0.036026015877723694,
0.00828272569924593,
-0.04303798824548721,
-0.041760291904211044,
-0.04155610874295235,
0.05005276948213577,
0.013242055661976337,
-0.08718540519475937,
0.056553713977336884,
0.008841775357723236,
-0.07681726664304733,
-0.016195783391594887,
-0.015617725439369678,
-0.04947894811630249,
0.007949280552566051,
0.004592674318701029,
0.05182250961661339,
-0.054675258696079254,
0.021997181698679924,
0.12704263627529144,
-0.021155226975679398,
-0.0096041951328516,
0.026589933782815933,
-0.004416920244693756,
0.029093043878674507,
0.025378553196787834,
-0.07742711156606674,
0.06139068678021431,
0.06661944836378098,
0.06264711171388626,
-0.04227684438228607,
-0.07224173098802567,
-0.021548403427004814,
0.053512975573539734,
-0.0403202660381794,
-0.027359483763575554,
-0.04113394021987915,
0.05298083275556564,
0.09803646057844162,
0.008530162274837494,
0.07224562019109726,
0.0655585452914238,
0.11477893590927124,
-0.040030188858509064,
0.07779642194509506,
0.015446318313479424,
-0.034452732652425766,
0.025246383622288704,
0.061329908668994904,
-0.05075358226895332,
-6.11080182011392e-8,
-0.0356399342417717,
0.04560481011867523,
-0.06337771564722061,
0.022151395678520203,
-0.04866885766386986,
-0.020776253193616867,
-0.057002078741788864,
0.04890693351626396,
0.0165614802390337,
0.02766389213502407,
0.014932851307094097,
0.0026833955198526382,
-0.0903024896979332,
0.01482873409986496,
0.000807849457487464,
0.009467161260545254,
0.01751229539513588,
0.09565849602222443,
-0.05145290121436119,
-0.05108008533716202,
0.06513310223817825,
0.003246396780014038,
-0.047049928456544876,
0.0030863878782838583,
0.09063633531332016,
-0.032399486750364304,
-0.13349628448486328,
0.11099141836166382,
-0.04579649120569229,
0.0345706008374691,
-0.013087133876979351,
-0.05792047455906868,
0.004595163278281689,
-0.06086087226867676,
0.013634410686790943,
0.061334311962127686,
-0.05692644417285919,
-0.05260257050395012,
0.026663001626729965,
0.048896413296461105,
0.046388059854507446,
0.028368357568979263,
-0.05314774438738823,
-0.018771400675177574,
0.03270480036735535,
0.000029491882742149755,
-0.024494750425219536,
-0.06477352231740952,
0.015174368396401405,
-0.010941989719867706,
0.031592581421136856,
-0.07760366052389145,
0.012078278698027134,
0.06072327867150307,
-0.03877237066626549,
0.0011414902983233333,
-0.0033089080825448036,
0.006107674445956945,
0.11495094001293182,
-0.05072380602359772,
0.10688996315002441,
-0.042354971170425415,
-0.032014988362789154,
-0.007169483229517937
] |
sberbank-ai/ruT5-large | 4d14102f32e730d68b1950bfaeb7a4988c978737 | 2021-09-28T15:56:17.000Z | [
"pytorch",
"t5",
"text2text-generation",
"ru",
"transformers",
"PyTorch",
"Transformers",
"autotrain_compatible"
] | text2text-generation | false | sberbank-ai | null | sberbank-ai/ruT5-large | 1,962 | 7 | transformers | ---
language:
- ru
tags:
- PyTorch
- Transformers
thumbnail: "https://github.com/sberbank-ai/model-zoo"
---
# ruT5-large
Model was trained by [SberDevices](https://sberdevices.ru/) team.
* Task: `text2text generation`
* Type: `encoder-decoder`
* Tokenizer: `bpe`
* Dict size: `32 101 `
* Num Parameters: `737 M`
* Training Data Volume `300 GB`
| [
-0.041847895830869675,
-0.09142593294382095,
-0.05420286953449249,
0.051295213401317596,
0.0757187157869339,
-0.041045431047677994,
-0.033653076738119125,
0.06798464059829712,
-0.0075158895924687386,
-0.05592004954814911,
-0.03139244019985199,
-0.023706773295998573,
-0.05522305518388748,
0.04466718062758446,
0.017395297065377235,
0.050788763910532,
0.0034064624924212694,
-0.007678435184061527,
-0.06796122342348099,
-0.09994935989379883,
0.04929539933800697,
-0.007440513931214809,
0.04073783755302429,
0.0309353768825531,
0.017218368127942085,
0.06382116675376892,
-0.020032525062561035,
-0.05724194645881653,
0.03930230066180229,
-0.07474236190319061,
0.0636274442076683,
-0.0016506981337442994,
0.05510428920388222,
0.01016190741211176,
0.05656282976269722,
0.06666490435600281,
-0.033025745302438736,
-0.08385857939720154,
0.021075477823615074,
-0.014776960015296936,
0.05102873593568802,
-0.01361861452460289,
-0.04768283665180206,
-0.018742362037301064,
0.1256440430879593,
0.014311720617115498,
-0.03986850008368492,
-0.028998665511608124,
0.07109769433736801,
-0.08012843877077103,
-0.0875445157289505,
-0.013993442989885807,
-0.016216635704040527,
-0.004195621237158775,
-0.00779516389593482,
-0.021395964547991753,
0.03818304464221001,
-0.06256483495235443,
0.046141572296619415,
-0.021133698523044586,
0.011103956028819084,
0.02377191372215748,
0.01375838927924633,
-0.011216322891414165,
-0.05743744969367981,
0.014064175076782703,
0.019185423851013184,
0.02467644028365612,
0.02801072783768177,
-0.01936967298388481,
0.03415900468826294,
-0.01277151145040989,
-0.10881493985652924,
0.021080803126096725,
0.017155511304736137,
-0.07077986001968384,
0.04809613898396492,
0.05207027867436409,
0.028775859624147415,
-0.07396416366100311,
-0.02215414121747017,
-0.08541452884674072,
0.0669088363647461,
0.00002626275090733543,
-0.010701621882617474,
0.021129600703716278,
0.016437167301774025,
0.02493288181722164,
0.0024554452393203974,
0.0017164436867460608,
-0.003259857650846243,
-0.12640292942523956,
0.042208943516016006,
0.04069750756025314,
-0.08645222336053848,
0.028351599350571632,
-0.044408779591321945,
-0.012325339019298553,
-0.03648877888917923,
0.060780689120292664,
-0.032265931367874146,
-0.015253179706633091,
0.06929130107164383,
0.028115369379520416,
-0.04838333651423454,
-0.042495641857385635,
0.01888340339064598,
0.0678839161992073,
0.029918309301137924,
-0.035907089710235596,
0.09859780967235565,
-0.005834640469402075,
-0.016271919012069702,
0.04100266471505165,
0.08317552506923676,
0.02855747938156128,
-0.03585420921444893,
-0.0051773833110928535,
-0.004867746960371733,
0.09539378434419632,
0.007023208774626255,
0.02890719287097454,
-0.10389307141304016,
0.0012062094174325466,
-0.0928412526845932,
0.0017869345610961318,
-0.048512887209653854,
3.909074872248839e-33,
0.004474370740354061,
-0.0413326658308506,
0.03205857425928116,
0.03515003249049187,
0.02632591314613819,
0.017050761729478836,
-0.04168856889009476,
0.02034963294863701,
-0.029716918244957924,
-0.004817910958081484,
-0.04453670233488083,
-0.05799984559416771,
-0.035723984241485596,
0.054556429386138916,
0.03245433419942856,
-0.045189984142780304,
-0.044192053377628326,
0.03808089345693588,
0.018448391929268837,
0.053173910826444626,
0.07014613598585129,
0.0021262853406369686,
-0.04657122492790222,
-0.022287189960479736,
-0.00008005861309356987,
0.06914836913347244,
0.052596431225538254,
-0.11696965247392654,
0.03620677813887596,
0.02722635678946972,
-0.10317622125148773,
-0.047413937747478485,
0.04017644748091698,
0.05882051959633827,
-0.015092210844159126,
-0.04222790151834488,
0.03288871794939041,
-0.017060430720448494,
-0.07850735634565353,
-0.08114063739776611,
0.015986308455467224,
0.049130141735076904,
-0.039699893444776535,
-0.043375540524721146,
-0.012396907433867455,
-0.013529680669307709,
0.020965872332453728,
0.04099840670824051,
-0.01907964237034321,
0.014629007317125797,
0.013574570417404175,
0.0557689443230629,
-0.03937260061502457,
-0.005223895888775587,
0.059008531272411346,
0.00018179907056037337,
0.07953902333974838,
0.054316598922014236,
0.10470777004957199,
0.07145500928163528,
0.07257036864757538,
-0.00041511960444040596,
0.07155342400074005,
0.04033300653100014,
0.14188167452812195,
0.048177145421504974,
0.03612443432211876,
0.009551696479320526,
0.042243968695402145,
0.04885733872652054,
-0.0869387611746788,
-0.003846721490845084,
0.07481246441602707,
0.037271227687597275,
0.028784792870283127,
-0.07787173241376877,
-0.018367251381278038,
-0.06588464975357056,
-0.06670175492763519,
0.03571705147624016,
-0.10221794247627258,
0.020396947860717773,
0.03225761279463768,
-0.019428011029958725,
-0.02770017832517624,
-0.009634498506784439,
0.014141988009214401,
-0.08346899598836899,
-0.02263917587697506,
0.014977174811065197,
0.016112925484776497,
-0.019607102498412132,
-0.05548180639743805,
-0.03725787624716759,
-0.05617903172969818,
-3.0602221161872483e-33,
0.02943672426044941,
0.01839284598827362,
-0.0835631713271141,
0.05158475413918495,
-0.05417914688587189,
-0.06653233617544174,
0.007609765511006117,
0.13430950045585632,
0.0014485352439805865,
0.0579703263938427,
0.027203204110264778,
-0.04386264830827713,
0.021230187267065048,
-0.03542647510766983,
0.08480396866798401,
-0.022598953917622566,
0.013012753799557686,
-0.021184217184782028,
0.0011963321594521403,
0.037871528416872025,
-0.031500641256570816,
0.0881420373916626,
0.009098743088543415,
0.05579637736082077,
-0.00016281637363135815,
0.03662524372339249,
-0.006855715531855822,
0.05158569663763046,
0.022782472893595695,
-0.07113530486822128,
-0.021081827580928802,
-0.0045183273032307625,
-0.06445243209600449,
-0.01165973860770464,
-0.09273035824298859,
0.0013736534165218472,
0.03450005501508713,
0.03088369220495224,
-0.02900579944252968,
0.0407504141330719,
0.05537024512887001,
0.06503947824239731,
-0.13723218441009521,
0.05749116465449333,
-0.015609531663358212,
-0.02309010922908783,
-0.07503943890333176,
0.0021998744923621416,
0.0664605051279068,
-0.08438735455274582,
0.014425210654735565,
-0.03793671727180481,
-0.08871451020240784,
-0.018873892724514008,
-0.06309318542480469,
-0.09402961283922195,
0.010548761114478111,
0.02706599049270153,
-0.0039196680299937725,
-0.019654126837849617,
-0.04522402957081795,
-0.04647388309240341,
0.029423708096146584,
-0.0244191475212574,
-0.004079254809767008,
-0.07040601968765259,
-0.018120115622878075,
0.01290158275514841,
-0.004416440147906542,
0.0009713447652757168,
0.08921413123607635,
-0.0026669895742088556,
0.060396723449230194,
0.18418365716934204,
-0.040357109159231186,
-0.0006539248861372471,
-0.08175117522478104,
0.015097342431545258,
0.07581696659326553,
-0.03647267073392868,
-0.044760189950466156,
0.020926212891936302,
0.05426813289523125,
0.09408389031887054,
0.09439649432897568,
-0.044628582894802094,
0.023351013660430908,
0.08315455913543701,
0.04072597250342369,
-0.008191556669771671,
0.051477134227752686,
0.01343382615596056,
-0.0006152684800326824,
0.09911070019006729,
-0.000011453291335783433,
-4.652777008118392e-8,
-0.029247304424643517,
-0.019328800961375237,
-0.08325503766536713,
0.06196767836809158,
-0.01215765904635191,
-0.052989888936281204,
-0.030217280611395836,
0.06396995484828949,
-0.04781433567404747,
0.0662921741604805,
0.006328659597784281,
-0.03468506783246994,
-0.12267960608005524,
-0.003214627504348755,
0.047348715364933014,
0.10676403343677521,
0.05838486924767494,
0.07842548936605453,
-0.0009859659476205707,
-0.06556662917137146,
0.09433174133300781,
-0.00784111674875021,
-0.009156676940619946,
-0.07704237103462219,
0.05773666128516197,
-0.0707971379160881,
-0.08076249063014984,
0.07747934013605118,
0.021171627566218376,
-0.08217310160398483,
0.0415063202381134,
-0.008886766619980335,
-0.014156478457152843,
-0.02599666453897953,
0.07197093963623047,
0.03540549427270889,
-0.005235827993601561,
-0.028180396184325218,
0.01565971039235592,
-0.006594686768949032,
-0.029533401131629944,
-0.013506386429071426,
-0.07787341624498367,
-0.03911421447992325,
0.018627887591719627,
0.026907645165920258,
-0.06243211403489113,
-0.06900623440742493,
0.02054663747549057,
-0.01517809834331274,
0.06579858064651489,
-0.06652086973190308,
0.01782146282494068,
0.030654631555080414,
-0.026590578258037567,
0.028647512197494507,
-0.04904831200838089,
-0.0803709626197815,
-0.014750886708498001,
0.0076376814395189285,
0.06676071137189865,
0.05607517436146736,
-0.01960519142448902,
-0.009540853090584278
] |
mrm8488/GPT-2-finetuned-covid-bio-medrxiv | 9f18ece8499d11cd7e0679e14be9e32ac9148f5e | 2021-08-25T21:38:35.000Z | [
"pytorch",
"jax",
"gpt2",
"text-generation",
"en",
"transformers"
] | text-generation | false | mrm8488 | null | mrm8488/GPT-2-finetuned-covid-bio-medrxiv | 1,961 | null | transformers | ---
language: en
thumbnail:
widget:
- text: "Old people with COVID-19 tends to suffer"
---
# GPT-2 + bio/medrxiv files from CORD19: 🦠 ✍ ⚕
**GPT-2** fine-tuned on **biorxiv_medrxiv** files from [CORD-19](https://www.kaggle.com/allen-institute-for-ai/CORD-19-research-challenge) dataset.
## Datasets details:
| Dataset | # Files |
| ---------------------- | ----- |
| biorxiv_medrxiv | 885 |
## Model training:
The model was trained on a Tesla P100 GPU and 25GB of RAM with the following command:
```bash
export TRAIN_FILE=/path/to/dataset/train.txt
python run_language_modeling.py \\n --model_type gpt2 \\n --model_name_or_path gpt2 \\n --do_train \\n --train_data_file $TRAIN_FILE \\n --num_train_epochs 4 \\n --output_dir model_output \\n --overwrite_output_dir \\n --save_steps 2000 \\n --per_gpu_train_batch_size 3
```
## Model in action / Example of usage: ✒
You can get the following script [here](https://github.com/huggingface/transformers/blob/master/examples/text-generation/run_generation.py)
```bash
python run_generation.py \\n --model_type gpt2 \\n --model_name_or_path mrm8488/GPT-2-finetuned-CORD19 \\n --length 200
```
```txt
👵👴🦠
# Input: Old people with COVID-19 tends to suffer
# Output: === GENERATED SEQUENCE 1 ===
Old people with COVID-19 tends to suffer more symptom onset time and death. It is well known that many people with COVID-19 have high homozygous ZIKV infection in the face of severe symptoms in both severe and severe cases.
The origin of Wuhan Fever was investigated by Prof. Shen Jiang at the outbreak of Wuhan Fever [34]. As Huanan Province is the epicenter of this outbreak, Huanan, the epicenter of epidemic Wuhan Fever, is the most potential location for the direct transmission of infection (source: Zhongzhen et al., 2020). A negative risk ratio indicates more frequent underlying signs in the people in Huanan Province with COVID-19 patients. Further analysis of reported Huanan Fever onset data in the past two years indicated that the intensity of exposure is the key risk factor for developing MERS-CoV infection in this region, especially among children and elderly. To be continued to develop infected patients would be a very important area for
```
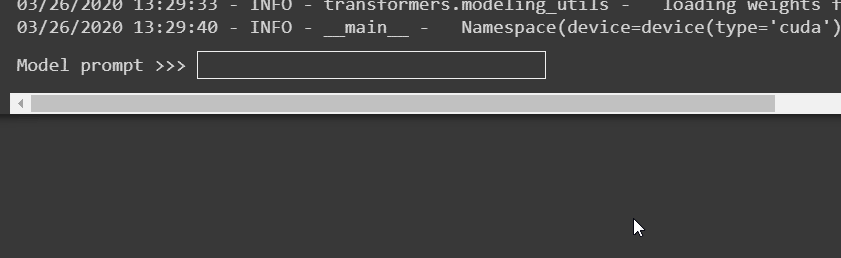
> Created by [Manuel Romero/@mrm8488](https://twitter.com/mrm8488) | [LinkedIn](https://www.linkedin.com/in/manuel-romero-cs/)
> Made with <span style="color: #e25555;">♥</span> in Spain
| [
-0.04848047345876694,
0.006547929719090462,
-0.09837093949317932,
0.019144877791404724,
0.08016050606966019,
-0.054151374846696854,
-0.02292047068476677,
0.10179144889116287,
-0.055788978934288025,
-0.08408620208501816,
0.05990283191204071,
-0.01297376025468111,
-0.027797972783446312,
0.012492181733250618,
-0.06792528182268143,
0.006111695431172848,
-0.08053026348352432,
-0.039353445172309875,
-0.05296183377504349,
-0.05418642982840538,
-0.0074276854284107685,
0.10310725122690201,
0.0669737383723259,
0.034564051777124405,
-0.02367951162159443,
0.006281619425863028,
-0.00010200170800089836,
-0.03645351529121399,
-0.0028415804263204336,
0.0026600046548992395,
0.049228280782699585,
0.08431900292634964,
0.00238189403899014,
0.04656446352601051,
0.058684270828962326,
0.10763274133205414,
-0.03623037785291672,
-0.08210471272468567,
-0.02429961785674095,
-0.006569675635546446,
0.07439596951007843,
0.010700473561882973,
-0.02534707821905613,
-0.027653668075799942,
0.052856940776109695,
-0.00969933345913887,
-0.06793481856584549,
-0.07445037364959717,
0.03508966043591499,
-0.01881321519613266,
-0.11283785104751587,
-0.0002388923312537372,
-0.013886523433029652,
0.004390704445540905,
0.03897321969270706,
-0.05951451137661934,
-0.011564003303647041,
-0.034656889736652374,
-0.0222143717110157,
-0.019288048148155212,
0.01648661494255066,
0.0001493585732532665,
-0.048054568469524384,
0.0009628994157537818,
-0.06492302566766739,
-0.05176315829157829,
0.01593666337430477,
0.05369700491428375,
-0.00077789556235075,
0.016864096745848656,
-0.03211676701903343,
0.04901420697569847,
-0.10499992221593857,
0.01842508278787136,
-0.026182223111391068,
-0.01376330479979515,
0.029847146943211555,
-0.0291881263256073,
0.05075841769576073,
-0.11582166701555252,
-0.01964060217142105,
-0.07820899039506912,
0.10239605605602264,
-0.004753562156111002,
0.024988265708088875,
-0.019502690061926842,
0.08054658770561218,
0.03275343030691147,
0.02054745890200138,
-0.046284858137369156,
-0.04357066750526428,
-0.038725435733795166,
0.05909452959895134,
0.07498202472925186,
-0.008276395499706268,
0.05535058304667473,
0.05246958136558533,
0.026082944124937057,
-0.07335121184587479,
0.030005991458892822,
0.003639929462224245,
0.00335124135017395,
0.03258255124092102,
0.11056067794561386,
-0.03326556086540222,
0.00017617622506804764,
0.04636683315038681,
0.06885369122028351,
0.019429054111242294,
-0.009522054344415665,
0.0766490250825882,
0.06978833675384521,
-0.04852449148893356,
-0.03050093539059162,
0.04878286272287369,
0.062266286462545395,
-0.10272025316953659,
0.02466476522386074,
-0.058927446603775024,
0.13272878527641296,
-0.044310685247182846,
-0.06914815306663513,
-0.03588056191802025,
-0.008902537636458874,
0.01645895093679428,
-0.0491303913295269,
-0.03893231600522995,
1.8907240722511007e-33,
0.03769400715827942,
-0.09309898316860199,
0.006546629127115011,
-0.02146996185183525,
0.07983584702014923,
0.009521842002868652,
-0.021231558173894882,
-0.028225470334291458,
-0.039423875510692596,
0.023019947111606598,
-0.11218626797199249,
-0.03253781050443649,
-0.05981829762458801,
0.06708170473575592,
0.015089933760464191,
-0.059255387634038925,
-0.07470767945051193,
0.011283972300589085,
-0.0411841943860054,
0.036096274852752686,
0.1867188811302185,
0.04078277572989464,
-0.045303698629140854,
-0.021043872460722923,
-0.016317162662744522,
-0.0038969058077782393,
-0.01557082962244749,
-0.03773048147559166,
0.01670997217297554,
0.030695324763655663,
-0.1105121523141861,
-0.025459399446845055,
0.04687044396996498,
0.029390353709459305,
-0.020242491737008095,
-0.009557466022670269,
0.01542437169700861,
-0.04627842456102371,
0.025805719196796417,
0.028821446001529694,
0.0956682488322258,
0.06499656289815903,
0.01490325853228569,
-0.08891807496547699,
-0.013532493263483047,
-0.011144143529236317,
0.046365171670913696,
-0.016697300598025322,
-0.09010084718465805,
0.07349184900522232,
-0.08213896304368973,
0.03091910481452942,
-0.027218887582421303,
-0.006584246642887592,
-0.0228949636220932,
-0.020499393343925476,
0.03652412071824074,
0.008442142978310585,
0.08395415544509888,
0.015453956089913845,
0.04550793394446373,
0.0213319044560194,
0.03676667809486389,
0.06097085773944855,
0.051472507417201996,
-0.04313422739505768,
0.03878486901521683,
0.0050290608778595924,
-0.034774117171764374,
0.08042752742767334,
-0.13358382880687714,
-0.023466147482395172,
-0.0019551278091967106,
-0.03368654102087021,
0.08274637162685394,
-0.09862333536148071,
-0.019206073135137558,
-0.016688773408532143,
-0.10838272422552109,
-0.05100720375776291,
-0.0086240004748106,
-0.00762939453125,
-0.02239244617521763,
-0.07359927892684937,
-0.0303933247923851,
-0.026907747611403465,
-0.01573745720088482,
-0.02924724854528904,
-0.08342397212982178,
-0.0009391935309395194,
-0.04556713253259659,
-0.00956423394382,
-0.02230267971754074,
-0.07997188717126846,
-0.08626118302345276,
-4.505316831054597e-33,
0.04779211804270744,
0.05316402018070221,
0.06775717437267303,
0.05714802443981171,
0.033885400742292404,
-0.02647864632308483,
0.056843847036361694,
0.04347401484847069,
0.00775220338255167,
-0.06205368414521217,
0.0462287999689579,
-0.025198234245181084,
0.020218415185809135,
-0.07763306796550751,
0.049693889915943146,
0.028446882963180542,
-0.05471140518784523,
-0.0051953149959445,
-0.049772992730140686,
0.015758996829390526,
-0.0923575684428215,
0.0713401585817337,
-0.0377606526017189,
0.03330468758940697,
-0.02312130108475685,
0.05797586217522621,
0.0427841879427433,
0.022136475890874863,
0.021645868197083473,
-0.05173252150416374,
-0.048042986541986465,
0.045184917747974396,
-0.11395374685525894,
0.006680671591311693,
-0.04001539945602417,
0.050994373857975006,
0.07746555656194687,
-0.01443476416170597,
-0.04883001744747162,
0.05516849085688591,
0.1503162831068039,
0.07469449192285538,
-0.09311558306217194,
0.05734501779079437,
0.007753666024655104,
-0.0002606181660667062,
-0.07019272446632385,
-0.049058280885219574,
-0.006105692591518164,
-0.040130458772182465,
0.04315647482872009,
-0.024525385349988937,
-0.027530120685696602,
0.04776722192764282,
-0.01840813457965851,
-0.11783477663993835,
0.04873155057430267,
-0.007596553303301334,
-0.10603684931993484,
-0.05397355929017067,
-0.011014476418495178,
-0.07411463558673859,
-0.10297903418540955,
-0.07802236825227737,
-0.02935478277504444,
-0.06397154182195663,
-0.026484815403819084,
0.022328725084662437,
0.08442947268486023,
0.06651446968317032,
0.026782415807247162,
0.06140783429145813,
0.03781098872423172,
0.006943291984498501,
-0.04235711693763733,
0.051196902990341187,
-0.08897152543067932,
-0.009640541858971119,
0.07134108245372772,
0.0235800351947546,
0.019561482593417168,
0.01661320962011814,
0.09772931784391403,
0.010664612054824829,
0.05467633903026581,
0.04516500607132912,
-0.05109599977731705,
0.0616740807890892,
0.02936173789203167,
-0.017033252865076065,
-0.05099176615476608,
-0.0409090593457222,
0.047230932861566544,
0.05659167468547821,
-0.014369338750839233,
-5.567439131937135e-8,
0.023450687527656555,
-0.031312618404626846,
-0.0388946495950222,
0.057141952216625214,
0.014655283652245998,
-0.02990816906094551,
-0.06066387519240379,
0.08188793808221817,
0.012612386606633663,
0.045746296644210815,
0.11622622609138489,
0.00988260842859745,
-0.07927646487951279,
-0.006358026992529631,
0.004839220549911261,
0.0448482409119606,
-0.026087038218975067,
0.0814935714006424,
-0.011492532677948475,
-0.004698288161307573,
-0.028260067105293274,
0.015844974666833878,
0.01901470497250557,
-0.005141465924680233,
0.04204747825860977,
-0.024578912183642387,
0.013065098784863949,
0.028226735070347786,
-0.013135130517184734,
-0.05245808884501457,
-0.021195320412516594,
-0.06177915260195732,
-0.00674221757799387,
0.0006738603115081787,
0.012041409499943256,
-0.019994083791971207,
0.05842506140470505,
0.0022982233203947544,
0.03644363582134247,
0.005699130706489086,
0.0068104201927781105,
0.020859377458691597,
-0.056161023676395416,
0.0016579048242419958,
-0.00033883523428812623,
-0.02053905837237835,
-0.030829353258013725,
-0.008356759324669838,
0.02101985178887844,
-0.03207753598690033,
0.029993252828717232,
0.02031702548265457,
-0.0430610217154026,
0.0475427582859993,
0.02950032614171505,
0.019824601709842682,
-0.07396266609430313,
-0.004292952362447977,
-0.05202058330178261,
-0.02586822211742401,
-0.022803250700235367,
-0.030647631734609604,
-0.020321056246757507,
-0.018827097490429878
] |
Jiva/xlm-roberta-large-it-mnli | c6e64469ec4aa17fedbd1b2522256f90a90b5b86 | 2021-12-10T14:56:38.000Z | [
"pytorch",
"xlm-roberta",
"text-classification",
"it",
"dataset:multi_nli",
"dataset:glue",
"arxiv:1911.02116",
"transformers",
"tensorflow",
"license:mit",
"zero-shot-classification"
] | zero-shot-classification | false | Jiva | null | Jiva/xlm-roberta-large-it-mnli | 1,960 | 4 | transformers | ---
language: it
tags:
- text-classification
- pytorch
- tensorflow
datasets:
- multi_nli
- glue
license: mit
pipeline_tag: zero-shot-classification
widget:
- text: "La seconda guerra mondiale vide contrapporsi, tra il 1939 e il 1945, le cosiddette potenze dell'Asse e gli Alleati che, come già accaduto ai belligeranti della prima guerra mondiale, si combatterono su gran parte del pianeta; il conflitto ebbe inizio il 1º settembre 1939 con l'attacco della Germania nazista alla Polonia e terminò, nel teatro europeo, l'8 maggio 1945 con la resa tedesca e, in quello asiatico, il successivo 2 settembre con la resa dell'Impero giapponese dopo i bombardamenti atomici di Hiroshima e Nagasaki."
candidate_labels: "guerra, storia, moda, cibo"
multi_class: true
---
# XLM-roBERTa-large-it-mnli
## Version 0.1
| | matched-it acc | mismatched-it acc |
| -------------------------------------------------------------------------------- |----------------|-------------------|
| XLM-roBERTa-large-it-mnli | 84.75 | 85.39 |
## Model Description
This model takes [xlm-roberta-large](https://huggingface.co/xlm-roberta-large) and fine-tunes it on a subset of NLI data taken from a automatically translated version of the MNLI corpus. It is intended to be used for zero-shot text classification, such as with the Hugging Face [ZeroShotClassificationPipeline](https://huggingface.co/transformers/master/main_classes/pipelines.html#transformers.ZeroShotClassificationPipeline).
## Intended Usage
This model is intended to be used for zero-shot text classification of italian texts.
Since the base model was pre-trained trained on 100 different languages, the
model has shown some effectiveness in languages beyond those listed above as
well. See the full list of pre-trained languages in appendix A of the
[XLM Roberata paper](https://arxiv.org/abs/1911.02116)
For English-only classification, it is recommended to use
[bart-large-mnli](https://huggingface.co/facebook/bart-large-mnli) or
[a distilled bart MNLI model](https://huggingface.co/models?filter=pipeline_tag%3Azero-shot-classification&search=valhalla).
#### With the zero-shot classification pipeline
The model can be loaded with the `zero-shot-classification` pipeline like so:
```python
from transformers import pipeline
classifier = pipeline("zero-shot-classification",
model="Jiva/xlm-roberta-large-it-mnli", device=0, use_fast=True, multi_label=True)
```
You can then classify in any of the above languages. You can even pass the labels in one language and the sequence to
classify in another:
```python
# we will classify the following wikipedia entry about Sardinia"
sequence_to_classify = "La Sardegna è una regione italiana a statuto speciale di 1 592 730 abitanti con capoluogo Cagliari, la cui denominazione bilingue utilizzata nella comunicazione ufficiale è Regione Autonoma della Sardegna / Regione Autònoma de Sardigna."
# we can specify candidate labels in Italian:
candidate_labels = ["geografia", "politica", "macchine", "cibo", "moda"]
classifier(sequence_to_classify, candidate_labels)
# {'labels': ['geografia', 'moda', 'politica', 'macchine', 'cibo'],
# 'scores': [0.38871392607688904, 0.22633370757102966, 0.19398456811904907, 0.13735772669315338, 0.13708525896072388]}
```
The default hypothesis template is the English, `This text is {}`. With this model better results are achieving when providing a translated template:
```python
sequence_to_classify = "La Sardegna è una regione italiana a statuto speciale di 1 592 730 abitanti con capoluogo Cagliari, la cui denominazione bilingue utilizzata nella comunicazione ufficiale è Regione Autonoma della Sardegna / Regione Autònoma de Sardigna."
candidate_labels = ["geografia", "politica", "macchine", "cibo", "moda"]
hypothesis_template = "si parla di {}"
# classifier(sequence_to_classify, candidate_labels, hypothesis_template=hypothesis_template)
# 'scores': [0.6068345904350281, 0.34715887904167175, 0.32433947920799255, 0.3068877160549164, 0.18744681775569916]}
```
#### With manual PyTorch
```python
# pose sequence as a NLI premise and label as a hypothesis
from transformers import AutoModelForSequenceClassification, AutoTokenizer
nli_model = AutoModelForSequenceClassification.from_pretrained('Jiva/xlm-roberta-large-it-mnli')
tokenizer = AutoTokenizer.from_pretrained('Jiva/xlm-roberta-large-it-mnli')
premise = sequence
hypothesis = f'si parla di {}.'
# run through model pre-trained on MNLI
x = tokenizer.encode(premise, hypothesis, return_tensors='pt',
truncation_strategy='only_first')
logits = nli_model(x.to(device))[0]
# we throw away "neutral" (dim 1) and take the probability of
# "entailment" (2) as the probability of the label being true
entail_contradiction_logits = logits[:,[0,2]]
probs = entail_contradiction_logits.softmax(dim=1)
prob_label_is_true = probs[:,1]
```
## Training
## Version 0.1
The model has been now retrained on the full training set. Around 1000 sentences pairs have been removed from the set because their translation was botched by the translation model.
| metric | value |
|----------------- |------- |
| learning_rate | 4e-6 |
| optimizer | AdamW |
| batch_size | 80 |
| mcc | 0.77 |
| train_loss | 0.34 |
| eval_loss | 0.40 |
| stopped_at_step | 9754 |
## Version 0.0
This model was pre-trained on set of 100 languages, as described in
[the original paper](https://arxiv.org/abs/1911.02116). It was then fine-tuned on the task of NLI on an Italian translation of the MNLI dataset (85% of the train set only so far). The model used for translating the texts is Helsinki-NLP/opus-mt-en-it, with a max output sequence lenght of 120. The model has been trained for 1 epoch with learning rate 4e-6 and batch size 80, currently it scores 82 acc. on the remaining 15% of the training. | [
-0.05990898981690407,
0.05719452723860741,
-0.09016803652048111,
-0.04915153980255127,
0.03888091444969177,
0.07147804647684097,
0.0487087219953537,
0.08475734293460846,
-0.004352279473096132,
-0.018608825281262398,
0.039780352264642715,
-0.08909304440021515,
0.02462499402463436,
0.023739490658044815,
-0.03252805024385452,
-0.005624844692647457,
-0.0018289375584572554,
-0.04203327000141144,
-0.06464546173810959,
-0.028335433453321457,
0.041564665734767914,
0.03594505786895752,
0.07817809283733368,
0.032717835158109665,
0.03516558185219765,
0.07582682371139526,
-0.09035944938659668,
0.04738038033246994,
-0.06633474677801132,
0.02175455540418625,
-0.01517684105783701,
0.12882958352565765,
0.02366855926811695,
0.035622406750917435,
0.08692582696676254,
-0.012167928740382195,
-0.015333922579884529,
-0.0007246678578667343,
0.07580676674842834,
0.05483614653348923,
-0.07970882952213287,
0.016758644953370094,
-0.038090236485004425,
0.04924580082297325,
-0.025641096755862236,
0.01002261321991682,
-0.015973933041095734,
0.003025186015293002,
-0.04486102610826492,
0.03349354490637779,
-0.07093794643878937,
0.00039105533505789936,
-0.02939143031835556,
0.06955007463693619,
-0.0295364148914814,
-0.057384192943573,
0.045360784977674484,
-0.03467679023742676,
0.02531810849905014,
-0.01211357768625021,
-0.10893801599740982,
-0.014559601433575153,
-0.029613332822918892,
0.07023964077234268,
-0.010126015171408653,
0.0013735985849052668,
0.02416905388236046,
-0.006521251983940601,
-0.07383926957845688,
0.038162194192409515,
0.08595429360866547,
0.007410421501845121,
0.04920702800154686,
0.01884831115603447,
0.006251368205994368,
0.02430211380124092,
0.012791533023118973,
0.04963022097945213,
0.036879345774650574,
-0.10295405238866806,
0.03888639807701111,
-0.027610238641500473,
0.08593543618917465,
-0.045774757862091064,
0.08317548036575317,
0.04465126618742943,
-0.058256667107343674,
-0.027775481343269348,
0.09932150691747665,
0.032005976885557175,
-0.06140599399805069,
0.026199335232377052,
0.05277125537395477,
0.09619569778442383,
-0.027980895712971687,
-0.0015836047241464257,
0.017993206158280373,
0.0012873222585767508,
0.03811020031571388,
0.11560115218162537,
0.023933226242661476,
-0.09578707069158554,
-0.026156943291425705,
0.0058529796078801155,
-0.07472895830869675,
-0.06766647845506668,
0.04893694818019867,
-0.012139157392084599,
-0.030411524698138237,
-0.04875435307621956,
-0.04283912107348442,
-0.05030079185962677,
-0.01776091940701008,
-0.08453897386789322,
0.01146865077316761,
-0.03058536909520626,
0.06538771092891693,
-0.03405533730983734,
0.0034998145420104265,
0.006314943078905344,
-0.013319278135895729,
-0.04946393147110939,
-0.11084717512130737,
-0.014751695096492767,
-0.0688081756234169,
0.02061587944626808,
-0.0543045848608017,
1.2374377603228372e-32,
0.0400601364672184,
0.015544122084975243,
-0.017510954290628433,
-0.0016368352808058262,
-0.01990014873445034,
-0.023595374077558517,
-0.08211699873209,
-0.024700289592146873,
-0.08189690858125687,
0.005974512547254562,
-0.052329305559396744,
-0.036006223410367966,
-0.041880518198013306,
0.05664695426821709,
0.01826099492609501,
-0.004919425584375858,
0.06686966121196747,
0.022400617599487305,
-0.008827815763652325,
-0.03127932548522949,
0.0014707843074575067,
0.03684283420443535,
0.025845874100923538,
-0.04165130481123924,
0.06457824259996414,
0.14783768355846405,
-0.0018178715836256742,
-0.05912299081683159,
-0.07390259206295013,
0.06338617205619812,
-0.0030161207541823387,
-0.004154794383794069,
0.04910680279135704,
-0.00691773509606719,
0.05447275564074516,
-0.07336974143981934,
-0.08986641466617584,
-0.019914617761969566,
-0.03823815658688545,
-0.003910730592906475,
-0.05155481398105621,
0.06898082792758942,
-0.03706452250480652,
0.026430662721395493,
0.05642743036150932,
-0.03808313608169556,
-0.04696691036224365,
0.027242589741945267,
0.05176561698317528,
-0.003586159087717533,
-0.026426177471876144,
-0.024505671113729477,
0.0001341192255495116,
-0.003230481641367078,
-0.015841642394661903,
0.09197482466697693,
-0.02585454098880291,
0.0484941191971302,
0.035158026963472366,
-0.0705643892288208,
-0.022493205964565277,
0.023608043789863586,
-0.002032322809100151,
0.04167144373059273,
0.07099082320928574,
0.057831086218357086,
-0.11380696296691895,
0.013900300487875938,
0.1367274522781372,
0.043803002685308456,
-0.05072619765996933,
-0.016539257019758224,
0.0117861432954669,
0.033167049288749695,
0.06690102815628052,
0.026991501450538635,
0.05407416820526123,
-0.04654025286436081,
-0.02902086079120636,
0.008774776011705399,
-0.0908566489815712,
-0.07088849693536758,
0.005534599535167217,
-0.014051340520381927,
-0.014779736287891865,
-0.026624679565429688,
0.022371510043740273,
-0.10877671092748642,
0.008789382874965668,
0.045479029417037964,
-0.018730148673057556,
0.0009719990775920451,
-0.08431461453437805,
-0.049524568021297455,
-0.09878282994031906,
-1.2558628995880073e-32,
0.023766420781612396,
0.031191224232316017,
-0.09085853397846222,
-0.02408418618142605,
-0.030322909355163574,
0.01676129549741745,
-0.07447424530982971,
0.00987040251493454,
0.04393995925784111,
0.025082096457481384,
0.04989159479737282,
-0.02252507023513317,
-0.031631194055080414,
-0.03459418937563896,
-0.01570119336247444,
0.04963252693414688,
0.02072436362504959,
0.03408493101596832,
0.04345981031656265,
0.06545058637857437,
0.048636458814144135,
-0.056033529341220856,
-0.05748182535171509,
-0.030225705355405807,
-0.04397987201809883,
-0.02200924977660179,
0.0693533644080162,
-0.011314325965940952,
0.08522261679172516,
-0.03512505069375038,
-0.030099833384156227,
-0.04920962080359459,
-0.04459463432431221,
-0.014871282503008842,
0.021005280315876007,
0.04360156133770943,
0.07222271710634232,
-0.041928332298994064,
0.011110099032521248,
0.08482189476490021,
-0.05906876176595688,
0.057241808623075485,
-0.058070652186870575,
0.007217213045805693,
-0.06635891646146774,
-0.034079018980264664,
-0.06867685914039612,
0.02571410872042179,
-0.00302844843827188,
-0.039352212101221085,
-0.014140969142317772,
0.01629454456269741,
-0.07560443878173828,
0.017695095390081406,
0.026181405410170555,
-0.08376502245664597,
-0.0036706156097352505,
-0.11121650785207748,
-0.08649352937936783,
0.005481273867189884,
-0.016811911016702652,
0.05169828236103058,
0.0007418674067594111,
-0.016531169414520264,
0.124269500374794,
-0.09149572253227234,
-0.09475240111351013,
-0.006556783802807331,
0.007500653620809317,
0.04090707004070282,
0.06028898060321808,
-0.020568478852510452,
-0.05597256124019623,
-0.008011574856936932,
-0.08233707398176193,
-0.033431652933359146,
-0.031226206570863724,
0.09195061773061752,
-0.00528757693246007,
0.05949145928025246,
-0.03699655830860138,
-0.007131324149668217,
-0.017901873216032982,
0.07990685105323792,
0.05137670040130615,
0.03191583231091499,
0.047594256699085236,
0.06368796527385712,
0.022498421370983124,
-0.017937153577804565,
0.0770476683974266,
0.0009441672009415925,
0.11031010746955872,
0.05374564602971077,
-0.032392971217632294,
-6.445257128007142e-8,
-0.01668626256287098,
0.009022960439324379,
-0.061906252056360245,
0.017799511551856995,
-0.04333309456706047,
-0.06590323895215988,
-0.039823148399591446,
-0.04617176204919815,
-0.010872460901737213,
-0.017452886328101158,
0.06444051116704941,
0.0898342877626419,
-0.018825234845280647,
-0.03752958029508591,
-0.06739000976085663,
0.03128283470869064,
-0.019599182531237602,
0.0031956308521330357,
-0.024607796221971512,
0.047800902277231216,
0.042434725910425186,
-0.07274423539638519,
-0.006087522488087416,
-0.10938528925180435,
0.015162333846092224,
0.001371189602650702,
-0.05333301052451134,
0.008243455551564693,
-0.054392632097005844,
-0.014553967863321304,
-0.05586174502968788,
0.05186533182859421,
-0.06824449449777603,
-0.10565806180238724,
-0.024330247193574905,
0.0839463546872139,
0.013270048424601555,
-0.09089595079421997,
-0.04087677597999573,
0.004709218628704548,
0.03368591144680977,
0.013692045584321022,
-0.03261655196547508,
0.01225036010146141,
0.1458093822002411,
0.03624345734715462,
0.02743583917617798,
-0.031262487173080444,
0.018158074468374252,
-0.019543243572115898,
-0.038842275738716125,
0.013139376416802406,
-0.048397015780210495,
0.07579253613948822,
0.017930472269654274,
0.030943483114242554,
0.07771115750074387,
0.031029820442199707,
0.07221411168575287,
0.0275079645216465,
0.08278237283229828,
-0.03164155036211014,
-0.03166359290480614,
-0.07138343900442123
] |
ltgoslo/norbert | 44815f7e109b53547cccdf3c6847f4c28b989816 | 2022-03-25T16:02:00.000Z | [
"pytorch",
"tf",
"jax",
"bert",
"fill-mask",
"no",
"arxiv:2104.06546",
"transformers",
"norwegian",
"license:cc-by-4.0",
"autotrain_compatible"
] | fill-mask | false | ltgoslo | null | ltgoslo/norbert | 1,956 | 6 | transformers | ---
language: no
license: cc-by-4.0
pipeline_tag: fill-mask
tags:
- norwegian
- bert
thumbnail: https://raw.githubusercontent.com/ltgoslo/NorBERT/main/Norbert.png
---
## Quickstart
**Release 1.1** (February 13, 2021)
Please check also our newer model: [NorBERT 2](https://huggingface.co/ltgoslo/norbert2), trained on a much larger corpus.
Download the model here:
* Cased Norwegian BERT Base: [216.zip](http://vectors.nlpl.eu/repository/20/216.zip)
More about NorBERT training corpora and training procedure: http://norlm.nlpl.eu/
Associated code: https://github.com/ltgoslo/NorBERT
Check this paper for more details:
_Andrey Kutuzov, Jeremy Barnes, Erik Velldal, Lilja Øvrelid, Stephan Oepen. [Large-Scale Contextualised Language Modelling for Norwegian](https://arxiv.org/abs/2104.06546), NoDaLiDa'21 (2021)_
NorBERT was trained as a part of NorLM, a joint initiative of the projects [EOSC-Nordic](https://www.eosc-nordic.eu/) (European Open Science Cloud) and [SANT](https://www.mn.uio.no/ifi/english/research/projects/sant/index.html) (Sentiment Analysis for Norwegian),
coordinated by the [Language Technology Group](https://www.mn.uio.no/ifi/english/research/groups/ltg/) (LTG) at the University of Oslo.
The computations were performed on resources provided by UNINETT Sigma2 - the National Infrastructure for High Performance Computing and Data Storage in Norway. | [
-0.07350687682628632,
-0.04478039592504501,
0.07119876146316528,
-0.04217821732163429,
0.06748258322477341,
0.0011189571814611554,
-0.04660071060061455,
0.045309215784072876,
0.010326388292014599,
-0.023361731320619583,
-0.004239283036440611,
-0.044723957777023315,
-0.015583706088364124,
0.033811479806900024,
0.04357830062508583,
0.05239760875701904,
0.021680979058146477,
0.04260355606675148,
-0.033064648509025574,
0.005951548460870981,
0.07230054587125778,
0.06366302073001862,
0.05173679441213608,
-0.13463526964187622,
0.0809081643819809,
-0.011087042279541492,
-0.02578013949096203,
-0.10276246815919876,
0.11549229174852371,
0.016365183517336845,
0.08446072041988373,
-0.0006757242372259498,
0.03613040968775749,
0.07942845672369003,
0.05274452641606331,
0.05386069416999817,
0.026680845767259598,
0.0006066481582820415,
-0.034902650862932205,
0.1452290415763855,
-0.07166086882352829,
0.018848003819584846,
-0.06169499084353447,
-0.012129134498536587,
0.09947777539491653,
0.02367531694471836,
-0.07416882365942001,
-0.007062345277518034,
-0.03102690353989601,
-0.006003784481436014,
-0.07995028048753738,
-0.09259506314992905,
0.0912816971540451,
0.024143051356077194,
-0.02695666253566742,
-0.03151102736592293,
-0.01558662112802267,
-0.052545323967933655,
0.007160349749028683,
-0.04141915589570999,
-0.061965472996234894,
-0.04876832664012909,
-0.031457044184207916,
-0.007866193540394306,
-0.06964991986751556,
0.03670896217226982,
-0.06950365751981735,
0.0569453239440918,
-0.01826011948287487,
0.026020575314760208,
-0.0024036283139139414,
0.016265522688627243,
-0.05499042570590973,
0.019624730572104454,
-0.041339389979839325,
-0.0377483032643795,
0.056462544947862625,
-0.059116873890161514,
0.05444342643022537,
-0.0770551785826683,
0.005821205675601959,
0.04809382185339928,
0.0375567227602005,
-0.03526514023542404,
0.04774341359734535,
-0.015163118951022625,
0.0804470032453537,
0.0031354259699583054,
-0.011842397041618824,
0.019894370809197426,
-0.01960902102291584,
-0.05441545322537422,
0.035844381898641586,
0.00175699673127383,
0.00651079136878252,
0.011555394157767296,
0.05646076425909996,
0.037803180515766144,
-0.024413514882326126,
0.1020088717341423,
0.008029942400753498,
0.004100071731954813,
0.017559140920639038,
-0.10860755294561386,
-0.020696520805358887,
0.02550371363759041,
-0.011771670542657375,
0.01092162448912859,
0.07324188202619553,
-0.09499888122081757,
0.009610345587134361,
-0.03804031014442444,
-0.05016014352440834,
-0.06051643192768097,
0.01437652762979269,
-0.0421481616795063,
0.03299015387892723,
-0.06422341614961624,
0.06972845643758774,
0.07449550181627274,
-0.03428197652101517,
0.027393732219934464,
-0.044864945113658905,
0.034065429121255875,
-0.019059695303440094,
0.040744006633758545,
0.009387197904288769,
3.068331190497999e-33,
0.03871249035000801,
0.0540442168712616,
-0.09522182494401932,
0.014437483623623848,
-0.028356386348605156,
0.02283320762217045,
-0.010178964585065842,
-0.03112621046602726,
-0.0733233243227005,
0.0055981832556426525,
-0.003267130348831415,
-0.009447836317121983,
-0.12298834323883057,
0.06192551553249359,
-0.07086073607206345,
-0.030508505180478096,
0.04387979209423065,
0.05811460688710213,
0.03218436241149902,
0.04933832958340645,
0.08680122345685959,
0.03637932240962982,
0.00680645601823926,
-0.019956067204475403,
0.006238373927772045,
0.01334464829415083,
0.08327436447143555,
-0.16487063467502594,
0.028183674439787865,
0.024785727262496948,
-0.06771635264158249,
0.04414444416761398,
0.02030063606798649,
0.029360737651586533,
0.018526950851082802,
-0.024486349895596504,
-0.00007028946129139513,
-0.022621752694249153,
-0.0225176140666008,
-0.08402234315872192,
-0.065773606300354,
0.009956697933375835,
0.008600446395576,
-0.04592542722821236,
-0.03664760664105415,
-0.0490766242146492,
0.039840955287218094,
0.02299487590789795,
0.034948404878377914,
-0.05354098975658417,
0.054029203951358795,
0.03175657242536545,
-0.06216597557067871,
-0.0023837629705667496,
0.018385987728834152,
-0.008782233111560345,
0.03241279348731041,
0.026684610173106194,
0.06402746587991714,
-0.00006115126598160714,
0.00013460480840876698,
-0.013147230260074139,
0.07793024182319641,
0.01364799216389656,
0.07123485952615738,
-0.08762001991271973,
-0.03642238676548004,
-0.005488880444318056,
0.06241563335061073,
-0.008425562642514706,
-0.056145116686820984,
0.01571284793317318,
0.03417064622044563,
0.030572591349482536,
0.026099979877471924,
-0.027702417224645615,
0.005767554976046085,
-0.04683729261159897,
-0.022603332996368408,
0.04619235545396805,
-0.04279463365674019,
0.000992035144008696,
-0.032078295946121216,
-0.05721963942050934,
-0.0008678013691678643,
-0.025463316589593887,
0.035703953355550766,
-0.04482468590140343,
0.007967810146510601,
0.009512534365057945,
0.06640347838401794,
-0.08173033595085144,
-0.04126286506652832,
-0.044596873223781586,
-0.0072594303637743,
-3.2268646034636313e-33,
0.024850400164723396,
0.031094806268811226,
-0.11609268933534622,
0.010909547097980976,
-0.03335554897785187,
-0.051027946174144745,
0.048987921327352524,
0.17751197516918182,
-0.02295817993581295,
-0.03124096244573593,
-0.00982342753559351,
-0.10104604810476303,
0.028216155245900154,
-0.022739753127098083,
0.06080595403909683,
-0.026366492733359337,
-0.007303216960281134,
0.0854724869132042,
0.013613706454634666,
0.03272361680865288,
0.02564873918890953,
-0.044537872076034546,
-0.08103345334529877,
0.09456156939268112,
0.009995684027671814,
0.09281737357378006,
-0.05010753497481346,
0.037555962800979614,
-0.04519457370042801,
-0.04015742242336273,
-0.05235230177640915,
0.023140160366892815,
-0.03791475296020508,
0.02505371905863285,
-0.0434238575398922,
0.05956389755010605,
0.009556173346936703,
0.02972753532230854,
-0.0015525189228355885,
-0.01142608281224966,
0.05755149573087692,
-0.005432938691228628,
-0.031221507117152214,
0.008137645199894905,
-0.04063669964671135,
-0.062099017202854156,
-0.06994634866714478,
-0.030683351680636406,
0.025880301371216774,
-0.07595042139291763,
-0.047991055995225906,
0.001726116519421339,
-0.07852606475353241,
-0.014633437618613243,
-0.028378762304782867,
-0.03884085640311241,
0.037189699709415436,
-0.13101640343666077,
-0.027516843751072884,
-0.0469590425491333,
-0.06434950977563858,
-0.01899830624461174,
0.030171366408467293,
0.02279050461947918,
0.012981707230210304,
-0.03767890855669975,
-0.04930591955780983,
0.039556220173835754,
0.003772624535486102,
0.014062212780117989,
-0.028218630701303482,
-0.03101876750588417,
0.08223196864128113,
-0.00799687672406435,
0.003961086738854647,
0.004917241167277098,
0.03565584123134613,
-0.06421740353107452,
-0.008064203895628452,
-0.05377788096666336,
-0.0465383306145668,
-0.04260069876909256,
0.02851048856973648,
0.08455944061279297,
0.08385345339775085,
0.13444678485393524,
0.06832318007946014,
0.009803160093724728,
-0.03905255347490311,
0.03833284601569176,
-0.025994043797254562,
0.044633541256189346,
-0.05237681791186333,
0.08646682649850845,
0.03687981888651848,
-5.8694414661886185e-8,
-0.07238706201314926,
0.008371264673769474,
-0.02202693186700344,
0.008646673522889614,
0.022784747183322906,
-0.07920148223638535,
-0.0017503072740510106,
0.00544793251901865,
-0.09257103502750397,
0.029979173094034195,
0.024645620957016945,
0.09984638541936874,
-0.0943744108080864,
-0.09067022800445557,
0.03705551475286484,
0.07517429441213608,
-0.0024429236073046923,
0.0643295869231224,
-0.0035120476968586445,
-0.010027504526078701,
0.004088962450623512,
0.051701392978429794,
-0.02239718846976757,
0.0038344222120940685,
-0.0014886779244989157,
-0.011314613744616508,
0.021987639367580414,
0.14809246361255646,
0.07594715058803558,
-0.02948668785393238,
0.004851360805332661,
0.05875327065587044,
-0.10480423271656036,
-0.02538437396287918,
0.08679549396038055,
0.019868046045303345,
-0.06859568506479263,
-0.0430203378200531,
-0.054225724190473557,
0.0033585417550057173,
0.07304280996322632,
0.04495823010802269,
-0.04991026967763901,
-0.026207318529486656,
0.06604167819023132,
0.03905032202601433,
-0.015871118754148483,
-0.08120077848434448,
0.014729108661413193,
0.026542576029896736,
0.03158184513449669,
-0.07696031033992767,
-0.06349301338195801,
0.10328572988510132,
0.000775723485276103,
0.0414976067841053,
-0.03583234176039696,
-0.020278995856642723,
0.02465211972594261,
0.05763585865497589,
-0.05257285758852959,
0.02659088373184204,
0.034645356237888336,
0.04301437735557556
] |
hf-internal-testing/test_dynamic_model_with_util | b731e5fae6d80a4a775461251c4388886fb7a249 | 2022-01-26T17:54:17.000Z | [
"pytorch",
"new-model",
"transformers"
] | null | false | hf-internal-testing | null | hf-internal-testing/test_dynamic_model_with_util | 1,953 | null | transformers | Entry not found | [
0.0461147278547287,
-0.038838207721710205,
-0.01049656979739666,
-0.03682169318199158,
0.011261860840022564,
0.013094935566186905,
0.0019101888174191117,
-0.013979103416204453,
0.027092741802334785,
-0.015212527476251125,
0.017284274101257324,
-0.08189476281404495,
0.03817418962717056,
-0.04920130595564842,
0.021389011293649673,
-0.015245908871293068,
-0.03203780576586723,
-0.1245758980512619,
0.03150877356529236,
0.032381657510995865,
-0.060957908630371094,
0.05409295856952667,
-0.025087490677833557,
0.01568586938083172,
0.028129950165748596,
-0.04710396006703377,
-0.018688226118683815,
0.013785239309072495,
-0.04001208767294884,
0.01173911802470684,
-0.04317743331193924,
0.05500618368387222,
0.004543041344732046,
0.02973111905157566,
0.14852192997932434,
0.02658126689493656,
0.02907961793243885,
-0.05169107764959335,
0.05803573504090309,
-0.07732241600751877,
-0.017637968063354492,
-0.04219653457403183,
0.041807834059000015,
0.023620979860424995,
0.021563321352005005,
0.016478516161441803,
-0.0021814992651343346,
-0.06400240957736969,
0.06393089145421982,
0.019599027931690216,
-0.08565037697553635,
0.00934905931353569,
-0.008718925528228283,
-0.028583496809005737,
-0.07310017943382263,
0.09416428208351135,
0.001759322709403932,
0.06184990331530571,
0.011840506456792355,
-0.035997264087200165,
0.08358278125524521,
-0.02619801089167595,
0.03736566752195358,
-0.028206506744027138,
-0.07454850524663925,
-0.08883563429117203,
-0.06279942393302917,
-0.008695344440639019,
0.014119276776909828,
-0.0825355276465416,
0.0649217739701271,
-0.00223911227658391,
-0.14716917276382446,
0.07743025571107864,
-0.03548373281955719,
-0.055201586335897446,
0.006981803569942713,
-0.012166670523583889,
0.055111464112997055,
-0.007116836030036211,
-0.023175746202468872,
-0.005835152696818113,
-0.09185640513896942,
0.055196937173604965,
0.034148022532463074,
0.03835180774331093,
0.038685429841279984,
-0.025987252593040466,
0.017804903909564018,
0.022428328171372414,
0.025005368515849113,
-0.10761535167694092,
-0.048001550137996674,
-0.04343584179878235,
0.012374646961688995,
-0.019502125680446625,
0.029218152165412903,
0.0842173621058464,
-0.011719699949026108,
0.09283553808927536,
-0.007015465293079615,
-0.03543110564351082,
-0.06936459988355637,
0.09425332397222519,
-0.010958523489534855,
-0.00805904995650053,
0.004974212497472763,
-0.0031528924591839314,
0.06105927750468254,
-0.03964288905262947,
-0.03619541600346565,
-0.019901901483535767,
0.07134733349084854,
0.039514873176813126,
-0.012729483656585217,
-0.006646515801548958,
-0.04746140539646149,
-0.014432490803301334,
-0.05157482624053955,
0.09506245702505112,
-0.049747664481401443,
-0.04591796174645424,
-0.008965466171503067,
-0.0325421579182148,
-0.08626784384250641,
-0.06624380499124527,
0.02538885548710823,
-4.303924894057984e-33,
0.01133066974580288,
0.0033434738870710135,
-0.002155609894543886,
0.04871906340122223,
-0.023564351722598076,
-0.07933273911476135,
0.0600903145968914,
0.02335330657660961,
-0.03844716399908066,
-0.020433755591511726,
-0.06952055543661118,
-0.03235611692070961,
0.0062485747039318085,
0.064804308116436,
-0.03201229125261307,
0.061689723283052444,
0.0417000837624073,
-0.00761845987290144,
0.03340127319097519,
-0.047770582139492035,
0.00887306872755289,
-0.04066338762640953,
-0.010506896302103996,
0.0106519665569067,
0.021333497017621994,
0.12854498624801636,
-0.009705503471195698,
0.010055632330477238,
-0.017507633194327354,
0.006515394430607557,
0.06334009766578674,
-0.057817306369543076,
0.013668818399310112,
-0.020286159589886665,
0.05430467426776886,
-0.023184705525636673,
0.0828516036272049,
0.0005449643940664828,
-0.10372652113437653,
-0.07634282112121582,
-0.005381610710173845,
-0.039263784885406494,
0.0006114727002568543,
-0.013281986117362976,
0.07119110971689224,
0.043696220964193344,
0.03168422728776932,
0.04338686540722847,
0.05728672817349434,
0.0832006186246872,
-0.07961414009332657,
0.015234283171594143,
0.017002005130052567,
0.047004107385873795,
-0.09794387966394424,
0.004990279674530029,
-0.07062993198633194,
-0.028000490739941597,
-0.04018733277916908,
-0.0702052190899849,
0.011351344175636768,
0.06020182743668556,
-0.03297270089387894,
0.09396500885486603,
0.03417910635471344,
-0.019825750961899757,
-0.034690454602241516,
-0.013036907650530338,
0.05896938592195511,
-0.012359356507658958,
-0.017275206744670868,
-0.07982361316680908,
0.02059139870107174,
0.06737419217824936,
0.04176458343863487,
-0.04978838190436363,
-0.05877475067973137,
-0.06289287656545639,
-0.03354167565703392,
-0.03871942684054375,
0.009898529388010502,
-0.05514208599925041,
-0.11629002541303635,
-0.011855563148856163,
0.10663620382547379,
0.037354156374931335,
-0.0065480442717671394,
-0.051189567893743515,
0.06663123518228531,
0.01874656230211258,
0.032841797918081284,
0.041593004018068314,
-0.06879369914531708,
0.04216769337654114,
-0.01628219522535801,
5.4139394340936695e-34,
0.05697013810276985,
-0.006972255185246468,
0.015711724758148193,
-0.17956365644931793,
0.02320219948887825,
0.007923615165054798,
-0.008062449283897877,
0.0074974060989916325,
0.07391711324453354,
0.0309313777834177,
0.060510627925395966,
0.058605875819921494,
0.09515274316072464,
-0.002282935893163085,
0.001603541080839932,
0.07024981826543808,
0.012629246339201927,
0.07425693422555923,
-0.038426291197538376,
0.01861148327589035,
0.030608950182795525,
-0.02449394389986992,
0.021528491750359535,
-0.003039651783183217,
-0.03676343336701393,
0.03130284696817398,
0.07998586446046829,
0.010451192036271095,
-0.07930229604244232,
-0.013543923385441303,
0.018781835213303566,
0.05168003588914871,
-0.07191970944404602,
0.15783067047595978,
0.026191607117652893,
0.01262354850769043,
0.08218053728342056,
-0.029807550832629204,
-0.07528624683618546,
-0.04250097647309303,
0.017244765534996986,
0.04411793500185013,
0.03708017244935036,
0.009233047254383564,
-0.040271829813718796,
0.022496428340673447,
0.02495843544602394,
0.07633638381958008,
0.005147108342498541,
0.013892097398638725,
0.05610476806759834,
-0.06684739887714386,
0.05862557515501976,
-0.020688841119408607,
0.05377643182873726,
0.06718500703573227,
0.005329249892383814,
-0.01388032827526331,
0.029931528493762016,
0.009508464485406876,
-0.045173756778240204,
0.11534366756677628,
-0.06510116159915924,
0.05117698386311531,
-0.0026125339791178703,
-0.08554837852716446,
-0.03784770518541336,
0.0804959163069725,
0.011298024095594883,
-0.07695550471544266,
-0.04868878796696663,
0.02515520341694355,
0.06252261996269226,
-0.04509226232767105,
-0.01246943511068821,
0.028559505939483643,
-0.030573077499866486,
0.05066261067986488,
-0.08187384903430939,
0.04469604790210724,
0.0034051244147121906,
0.04145054519176483,
-0.021858664229512215,
-0.06112268194556236,
-0.00908052921295166,
-0.05903250351548195,
0.0259539932012558,
0.059690944850444794,
-0.07613514363765717,
-0.03720718249678612,
-0.036316655576229095,
0.07058046013116837,
-0.008224100805819035,
0.041961874812841415,
-0.0285952128469944,
-1.496900736697171e-8,
-0.0014124972512945533,
0.03401879221200943,
-0.040338415652513504,
0.04116074740886688,
0.0935964286327362,
-0.05115952715277672,
0.0008746005478315055,
-0.03389839455485344,
-0.00567849725484848,
-0.010686947964131832,
-0.04789939522743225,
-0.04820054769515991,
-0.02011880651116371,
-0.03209094703197479,
-0.04211259260773659,
-0.10229527950286865,
-0.07819421589374542,
-0.031228765845298767,
-0.02154778689146042,
-0.04960230365395546,
0.08087796717882156,
-0.07801242172718048,
0.06919731199741364,
-0.04999840259552002,
0.03687043860554695,
0.03889009356498718,
-0.049989692866802216,
-0.04254625365138054,
-0.04606937617063522,
0.08682432025671005,
-0.031148413196206093,
0.11826753616333008,
0.034102488309144974,
-0.0208592489361763,
-0.0205202866345644,
0.027134142816066742,
0.09741277992725372,
0.051608603447675705,
0.013477512635290623,
-0.13649295270442963,
-0.022304272279143333,
0.02385953813791275,
0.038732077926397324,
-0.09249968826770782,
-0.04549082741141319,
0.054220106452703476,
0.01160438358783722,
0.051190607249736786,
0.07713303714990616,
-0.022097084671258926,
-0.06127818301320076,
-0.01857956498861313,
0.006740490905940533,
-0.00496308971196413,
0.024095389991998672,
0.0736224576830864,
-0.003481915919110179,
-0.0699305310845375,
-0.006629763171076775,
-0.0598808117210865,
0.05297163128852844,
-0.02902800403535366,
-0.027858933433890343,
-0.01287526823580265
] |
facebook/wav2vec2-large-960h-lv60 | 8e7d14742e8f98c6bbb24e5231406af321a8f9ce | 2022-04-05T16:42:07.000Z | [
"pytorch",
"jax",
"wav2vec2",
"automatic-speech-recognition",
"en",
"dataset:librispeech_asr",
"arxiv:2006.11477",
"transformers",
"speech",
"license:apache-2.0",
"model-index"
] | automatic-speech-recognition | false | facebook | null | facebook/wav2vec2-large-960h-lv60 | 1,947 | 5 | transformers | ---
language: en
datasets:
- librispeech_asr
tags:
- speech
license: apache-2.0
model-index:
- name: wav2vec2-large-960h-lv60
results:
- task:
name: Automatic Speech Recognition
type: automatic-speech-recognition
dataset:
name: Librispeech (clean)
type: librispeech_asr
args: en
metrics:
- name: Test WER
type: wer
value: 2.2
---
# Wav2Vec2-Large-960h-Lv60
[Facebook's Wav2Vec2](https://ai.facebook.com/blog/wav2vec-20-learning-the-structure-of-speech-from-raw-audio/)
The large model pretrained and fine-tuned on 960 hours of Libri-Light and Librispeech on 16kHz sampled speech audio. When using the model
make sure that your speech input is also sampled at 16Khz.
[Paper](https://arxiv.org/abs/2006.11477)
Authors: Alexei Baevski, Henry Zhou, Abdelrahman Mohamed, Michael Auli
**Abstract**
We show for the first time that learning powerful representations from speech audio alone followed by fine-tuning on transcribed speech can outperform the best semi-supervised methods while being conceptually simpler. wav2vec 2.0 masks the speech input in the latent space and solves a contrastive task defined over a quantization of the latent representations which are jointly learned. Experiments using all labeled data of Librispeech achieve 1.8/3.3 WER on the clean/other test sets. When lowering the amount of labeled data to one hour, wav2vec 2.0 outperforms the previous state of the art on the 100 hour subset while using 100 times less labeled data. Using just ten minutes of labeled data and pre-training on 53k hours of unlabeled data still achieves 4.8/8.2 WER. This demonstrates the feasibility of speech recognition with limited amounts of labeled data.
The original model can be found under https://github.com/pytorch/fairseq/tree/master/examples/wav2vec#wav2vec-20.
# Usage
To transcribe audio files the model can be used as a standalone acoustic model as follows:
```python
from transformers import Wav2Vec2Processor, Wav2Vec2ForCTC
from datasets import load_dataset
import torch
# load model and processor
processor = Wav2Vec2Processor.from_pretrained("facebook/wav2vec2-large-960h-lv60")
model = Wav2Vec2ForCTC.from_pretrained("facebook/wav2vec2-large-960h-lv60")
# load dummy dataset and read soundfiles
ds = load_dataset("patrickvonplaten/librispeech_asr_dummy", "clean", split="validation")
# tokenize
input_values = processor(ds[0]["audio"]["array"], return_tensors="pt", padding="longest").input_values # Batch size 1
# retrieve logits
logits = model(input_values).logits
# take argmax and decode
predicted_ids = torch.argmax(logits, dim=-1)
transcription = processor.batch_decode(predicted_ids)
```
## Evaluation
This code snippet shows how to evaluate **facebook/wav2vec2-large-960h-lv60** on LibriSpeech's "clean" and "other" test data.
```python
from datasets import load_dataset
from transformers import Wav2Vec2ForCTC, Wav2Vec2Processor
import torch
from jiwer import wer
librispeech_eval = load_dataset("librispeech_asr", "clean", split="test")
model = Wav2Vec2ForCTC.from_pretrained("facebook/wav2vec2-large-960h-lv60").to("cuda")
processor = Wav2Vec2Processor.from_pretrained("facebook/wav2vec2-large-960h-lv60")
def map_to_pred(batch):
inputs = processor(batch["audio"]["array"], return_tensors="pt", padding="longest")
input_values = inputs.input_values.to("cuda")
attention_mask = inputs.attention_mask.to("cuda")
with torch.no_grad():
logits = model(input_values, attention_mask=attention_mask).logits
predicted_ids = torch.argmax(logits, dim=-1)
transcription = processor.batch_decode(predicted_ids)
batch["transcription"] = transcription
return batch
result = librispeech_eval.map(map_to_pred, batched=True, batch_size=16, remove_columns=["speech"])
print("WER:", wer(result["text"], result["transcription"]))
```
*Result (WER)*:
| "clean" | "other" |
|---|---|
| 2.2 | 4.5 | | [
-0.10594869405031204,
-0.13361258804798126,
-0.014084266498684883,
-0.037385378032922745,
-0.01423906721174717,
-0.0208125039935112,
-0.08783972263336182,
-0.05330595001578331,
-0.04412361979484558,
-0.06937962770462036,
0.006914814468473196,
-0.07124453783035278,
-0.04296686127781868,
-0.035945355892181396,
-0.041075099259614944,
-0.01157431211322546,
0.08524638414382935,
-0.06059584394097328,
-0.022330205887556076,
-0.011747688055038452,
0.07669126242399216,
0.04573235660791397,
0.065522700548172,
-0.046318624168634415,
0.05424731597304344,
0.01889883726835251,
-0.04758954420685768,
-0.015534626320004463,
0.07013695687055588,
-0.05405040830373764,
0.1488627791404724,
0.05680746212601662,
0.053705986589193344,
-0.005044272169470787,
0.006413149181753397,
-0.040652353316545486,
-0.02879076451063156,
-0.03948523476719856,
-0.003558124415576458,
-0.008118489757180214,
0.026226434856653214,
-0.033273469656705856,
-0.01191014051437378,
-0.037606652826070786,
-0.04488586261868477,
0.017259396612644196,
0.0039808363653719425,
-0.029162725433707237,
-0.025605488568544388,
0.04032067582011223,
-0.03821420669555664,
-0.028745831921696663,
0.02043154463171959,
0.04825439676642418,
-0.12039216607809067,
-0.01594468578696251,
-0.020805256441235542,
0.027725808322429657,
0.04910263791680336,
0.015064754523336887,
-0.07915865629911423,
0.05178258568048477,
-0.0259842649102211,
0.024703484028577805,
-0.08149532973766327,
-0.0073195756413042545,
0.04803711175918579,
0.000867776689119637,
0.025831250473856926,
-0.012415544129908085,
-0.07721738517284393,
0.11096211522817612,
0.0359039269387722,
0.04233480617403984,
0.0300590917468071,
-0.03260713815689087,
0.08886422216892242,
0.003504801308736205,
0.0831887498497963,
-0.13298188149929047,
0.02057379111647606,
-0.07688935846090317,
0.024972403421998024,
-0.013961954042315483,
0.05179018899798393,
-0.029569359496235847,
0.025706101208925247,
-0.010769469663500786,
0.006426795851439238,
-0.0221711453050375,
-0.023033956065773964,
-0.02095678262412548,
-0.02997896820306778,
0.06452285498380661,
0.03628857806324959,
0.1073373332619667,
0.06137802451848984,
0.06529057770967484,
-0.0059387050569057465,
0.06924651563167572,
0.007189640309661627,
-0.010004259645938873,
0.0014906196156516671,
-0.011453689076006413,
-0.005714619066566229,
-0.09989319741725922,
-0.0069196452386677265,
0.06134968623518944,
0.05086676403880119,
0.02078724466264248,
0.09511444717645645,
0.07405879348516464,
0.030270956456661224,
0.0010553232859820127,
0.09928736835718155,
0.043570566922426224,
-0.05195637792348862,
-0.05482295900583267,
-0.004139721859246492,
0.03616926819086075,
-0.07014386355876923,
0.004909317009150982,
-0.024295587092638016,
0.007979745976626873,
0.008335831575095654,
-0.011964579112827778,
-0.012833050452172756,
4.0702450659801594e-33,
-0.011567477136850357,
0.052961159497499466,
0.010951091535389423,
-0.030977336689829826,
-0.014514288865029812,
-0.06219492480158806,
-0.0479685440659523,
0.045559756457805634,
-0.03596113994717598,
-0.017637141048908234,
-0.022673707455396652,
-0.07049866020679474,
-0.04370688647031784,
0.07317397743463516,
0.03866460174322128,
0.015302694402635098,
-0.05067150667309761,
0.021607844159007072,
-0.000011406054909457453,
0.018013419583439827,
0.1511698216199875,
-0.024731989949941635,
0.04186257719993591,
0.017526356503367424,
0.05040588229894638,
0.07393483072519302,
0.07168648391962051,
-0.030134102329611778,
-0.018774641677737236,
0.04889223724603653,
-0.030123433098196983,
-0.07889053970575333,
0.017164718359708786,
-0.026554299518465996,
0.012919225730001926,
0.028939496725797653,
0.008285201154649258,
-0.017035361379384995,
-0.08418745547533035,
-0.11200536042451859,
0.040214236825704575,
0.036126796156167984,
-0.012125159613788128,
-0.04148858040571213,
-0.03040909767150879,
-0.08817139267921448,
0.006141001358628273,
0.019823219627141953,
0.006887191440910101,
0.040108900517225266,
-0.014189849607646465,
-0.00984810572117567,
-0.09144112467765808,
0.0537305623292923,
-0.012509792111814022,
0.0006026989431120455,
0.08017485588788986,
0.04893532767891884,
0.03899814933538437,
0.024188747629523277,
0.042727548629045486,
0.022890770807862282,
0.053701918572187424,
0.01399637758731842,
0.06074349582195282,
-0.0519319586455822,
-0.0052865236066281796,
0.006028275471180677,
-0.021277297288179398,
-0.01652713678777218,
-0.0013082980876788497,
-0.07987971603870392,
0.09002082794904709,
0.04662342369556427,
0.028095467016100883,
-0.009665594436228275,
-0.00727752223610878,
-0.07472976297140121,
-0.06749866902828217,
0.038032375276088715,
-0.05066418647766113,
0.054045286029577255,
0.013674519956111908,
-0.08923894166946411,
-0.0677521675825119,
-0.04363415017724037,
0.04320446029305458,
-0.06744581460952759,
-0.072878398001194,
-0.0368766225874424,
-0.009585978463292122,
0.050640661269426346,
-0.014964752830564976,
-0.059042077511548996,
-0.05828314647078514,
-5.01520146471918e-33,
0.007897327654063702,
0.08447714895009995,
0.004886908456683159,
0.08952446281909943,
-0.004346795845776796,
-0.02065645530819893,
0.12506327033042908,
0.12349845468997955,
-0.016245270147919655,
-0.05957512557506561,
0.08172447979450226,
-0.07839612662792206,
0.056865837424993515,
-0.05354923754930496,
0.08718881756067276,
-0.010479557327926159,
0.0005196892889216542,
-0.01856769435107708,
0.08360539376735687,
0.05484117567539215,
0.03928446024656296,
0.07528672367334366,
0.0029662717133760452,
0.0783645287156105,
-0.056323420256376266,
-0.05590503290295601,
-0.03985882177948952,
0.0519380085170269,
-0.028295034542679787,
-0.01646994613111019,
-0.016820164397358894,
0.03383336588740349,
-0.07330624014139175,
0.02536558173596859,
-0.02532918006181717,
0.00334774237126112,
0.006879184860736132,
-0.04464252293109894,
-0.006875999737530947,
0.01519817765802145,
0.07726454734802246,
0.0773780569434166,
-0.11410383880138397,
-0.07391496002674103,
-0.011838016100227833,
-0.06405621767044067,
-0.09141620248556137,
-0.0600065179169178,
0.06622478365898132,
-0.0784836858510971,
0.04416045546531677,
-0.03641853109002113,
0.03075985051691532,
0.026131009683012962,
-0.023920102044939995,
-0.026096783578395844,
-0.036470748484134674,
-0.04140448197722435,
-0.06233055144548416,
-0.00042569354991428554,
0.00967047642916441,
0.051467977464199066,
-0.07308951020240784,
-0.03555513918399811,
0.0302448570728302,
0.013189858756959438,
-0.009687590412795544,
0.0337093211710453,
0.051255010068416595,
-0.010127929970622063,
-0.03540189936757088,
-0.018389616161584854,
0.03656214103102684,
0.04405134916305542,
-0.05460212379693985,
-0.04547664150595665,
-0.03303481265902519,
-0.020885523408651352,
-0.0023964743595570326,
-0.0820937231183052,
-0.04514032602310181,
0.05514153465628624,
0.04199568182229996,
0.01582748256623745,
0.03253914788365364,
0.037942372262477875,
-0.026279384270310402,
-0.002614761935546994,
-0.007218097802251577,
0.0020472873002290726,
0.0023760218173265457,
0.03189229965209961,
0.02103564515709877,
0.046259555965662,
0.008734369650483131,
-4.9436415849868354e-8,
-0.05228336527943611,
0.04900094494223595,
0.02431541495025158,
-0.015503186732530594,
-0.0158446803689003,
-0.11067239195108414,
-0.02289571985602379,
0.03075435571372509,
-0.023563863709568977,
0.029311049729585648,
0.09601990878582001,
-0.06346578150987625,
-0.033245623111724854,
0.06659127026796341,
0.08473646640777588,
0.01829901710152626,
0.0021192121785134077,
0.08731762319803238,
-0.043879177421331406,
-0.124455027282238,
0.06540316343307495,
0.024668635800480843,
0.031103556975722313,
-0.008806300349533558,
0.09283790737390518,
-0.012701934203505516,
-0.024229051545262337,
0.020925484597682953,
-0.002796686952933669,
-0.03221555054187775,
-0.09191624820232391,
0.059139933437108994,
-0.04741396754980087,
-0.05225653573870659,
0.06053778529167175,
0.04909057170152664,
-0.06909625977277756,
-0.03439866006374359,
0.021688051521778107,
0.11482381820678711,
0.03406072407960892,
0.08336509019136429,
-0.07424169778823853,
-0.04542045295238495,
0.05753948166966438,
0.034784190356731415,
-0.05162810534238815,
-0.07164575904607773,
0.03529973700642586,
0.08289065212011337,
0.023440884426236153,
0.05472107231616974,
0.011621956713497639,
-0.06469590216875076,
0.03658236563205719,
0.04318838194012642,
-0.07360845059156418,
-0.004705343395471573,
0.015067437663674355,
-0.048817917704582214,
0.06207559257745743,
-0.009877241216599941,
-0.04940216988325119,
-0.005254201125353575
] |
nikokons/gpt2-greek | b2bb85c722742ce6ea0b9e025d50425e061181c8 | 2022-07-20T09:59:03.000Z | [
"pytorch",
"jax",
"gpt2",
"text-generation",
"el",
"transformers"
] | text-generation | false | nikokons | null | nikokons/gpt2-greek | 1,944 | null | transformers | ---
language: el
---
## gpt2-greek
## Dataset:
The model is trained on a collection of almost 5GB Greek texts, with the main source to be from Greek Wikipedia. The content is extracted using the Wikiextractor tool (Attardi, 2012). The dataset is constructed as 5 sentences per sample (about 3.7 millions of samples) and the end of document is marked with the string <|endoftext|> providing the model with paragraph information, as done for the original GPT-2 training set by Radford . The input sentences are pre-processed and tokenized using 22,000 merges of byte-pair encoding.
## Model:
The model is the "small" version of GPT-2 (12-layer, 768-hidden, 12-heads) with the only difference that the maximum sequence length is set at 512 tokens instead of 1024.
## Training details:
It is trained from scratch a generative Transformer model as GPT-2 on a large corpus of Greek text so that the model can generate long stretches of contiguous coherent text. Attention dropouts with a rate of 0.1 are used for regularization on all layers and L2 weight decay of 0,01. In addition, a batch size of 4 and accumulated gradients over 8 iterations are used, resulting in an effective batch size of 32. The model uses the Adam optimization scheme with a learning rate of 1e-4 and is trained for 20 epochs. The learning rate increases linearly from zero over the first 9000 updates and decreases linearly by using a linear schedule. The implementation is based on the open-source PyTorch-transformer library (HuggingFace 2019).
| [
-0.0869232565164566,
-0.03407194837927818,
0.010280192829668522,
0.0014819958014413714,
0.09325341880321503,
-0.020575473085045815,
0.03196948021650314,
0.03946312516927719,
0.05835631489753723,
-0.04398651793599129,
0.009113320149481297,
0.05059497803449631,
-0.0412650890648365,
-0.014291197061538696,
-0.03540574386715889,
-0.041208330541849136,
0.007051841355860233,
0.03534705564379692,
-0.10382504016160965,
-0.0930730551481247,
0.07602345198392868,
0.05676839128136635,
0.08690468221902847,
0.012428969144821167,
0.03878539800643921,
0.03169400990009308,
0.008096800185739994,
-0.0804409608244896,
0.02424943447113037,
0.01938514970242977,
0.04994834214448929,
0.0030943884048610926,
-0.01084856502711773,
0.08233438432216644,
-0.08973666280508041,
0.08528684824705124,
-0.05162287876009941,
-0.03697694092988968,
0.0031563311349600554,
-0.023925624787807465,
0.0012237905757501721,
-0.0022549715358763933,
0.016915341839194298,
0.06754907965660095,
0.07787153869867325,
0.015939079225063324,
-0.06698118150234222,
-0.021901387721300125,
-0.056644078344106674,
-0.004041827749460936,
-0.06777392327785492,
-0.005316510796546936,
-0.020976601168513298,
0.05600036308169365,
-0.02967633493244648,
0.04788624122738838,
-0.031277019530534744,
0.018873896449804306,
-0.021517882123589516,
-0.03469713777303696,
-0.03662971034646034,
-0.08609416335821152,
-0.11649172753095627,
-0.026757046580314636,
-0.04736876115202904,
-0.03351727873086929,
0.05298694595694542,
0.0010603339178487659,
-0.028057768940925598,
-0.028990022838115692,
-0.037965647876262665,
0.13476230204105377,
-0.09064949303865433,
0.04479171335697174,
-0.0019132014131173491,
0.06007982790470123,
0.05487455055117607,
-0.027202073484659195,
0.02299639768898487,
-0.052240606397390366,
0.0927460715174675,
-0.025611594319343567,
0.13106995820999146,
-0.004785861819982529,
0.017245827242732048,
-0.05538465082645416,
0.03222104534506798,
0.09865579009056091,
-0.025724219158291817,
0.04693079739809036,
-0.06208755075931549,
-0.04530474916100502,
0.10629788786172867,
-0.014682125300168991,
-0.02935906872153282,
0.03445616737008095,
-0.0189349502325058,
-0.016077427193522453,
-0.016011783853173256,
0.090364471077919,
0.039797522127628326,
0.04015277698636055,
0.029219353571534157,
-0.04629595950245857,
-0.08783691376447678,
-0.0562732107937336,
-0.02840304747223854,
0.026486292481422424,
0.005402623675763607,
-0.02945133112370968,
0.07622986286878586,
-0.0062659685499966145,
-0.05018867179751396,
-0.0529962033033371,
0.006030424498021603,
0.05416416749358177,
-0.023185746744275093,
-0.0005981484428048134,
0.013583021238446236,
0.08265641331672668,
-0.05912686511874199,
0.047616004943847656,
-0.029350781813263893,
-0.030757280066609383,
-0.023369932547211647,
0.02430109493434429,
-0.05484350025653839,
4.55469530797081e-33,
0.039471741765737534,
0.07521228492259979,
-0.033119313418865204,
0.024062380194664,
-0.047413501888513565,
0.044732414186000824,
-0.023144392296671867,
-0.05120653659105301,
-0.02723008580505848,
-0.04033835977315903,
-0.06520425528287888,
-0.028279755264520645,
-0.05572423338890076,
0.06127332150936127,
0.03315357863903046,
-0.025331620126962662,
0.03081987053155899,
0.04502660408616066,
-0.03497040271759033,
0.04713568091392517,
0.046304840594530106,
0.10314439982175827,
0.04250357300043106,
-0.0452539324760437,
-0.027961652725934982,
0.03246930241584778,
-0.010189052671194077,
-0.053806766867637634,
-0.016412245109677315,
0.03514323756098747,
-0.1313113570213318,
-0.02332128770649433,
-0.010662063024938107,
0.0615001879632473,
0.07342811673879623,
-0.042401283979415894,
0.023999594151973724,
-0.1188199445605278,
0.08281689137220383,
-0.0945301428437233,
0.01776173710823059,
0.05232792720198631,
0.11645982414484024,
-0.02270919643342495,
-0.03688406944274902,
-0.03955407068133354,
0.08530860394239426,
0.006221462041139603,
-0.011876903474330902,
0.05776359513401985,
-0.021462332457304,
0.06432045996189117,
-0.08800776302814484,
0.0068810125812888145,
-0.01590067520737648,
0.07355901598930359,
0.06777867674827576,
0.03341805562376976,
0.08598220348358154,
0.09789210557937622,
0.05623365938663483,
0.06660599261522293,
0.08432337641716003,
0.07423873990774155,
0.06090651452541351,
0.04508046805858612,
-0.07787454128265381,
0.013683099299669266,
0.026980023831129074,
0.04384547099471092,
-0.009275858290493488,
-0.043579280376434326,
0.046419478952884674,
-0.013097438029944897,
0.05820730701088905,
-0.023087119683623314,
0.06237204372882843,
-0.08147339522838593,
-0.06649813801050186,
0.051088087260723114,
-0.0766383558511734,
0.0017523730639368296,
0.01448057871311903,
-0.08726947754621506,
-0.08124105632305145,
-0.04787035286426544,
0.03745328262448311,
-0.05815762281417847,
0.01882380060851574,
-0.04332394525408745,
-0.06389880180358887,
-0.02952614054083824,
-0.04764828830957413,
-0.04168824106454849,
0.013142635114490986,
-4.0792001288815175e-33,
-0.009446080774068832,
0.02672920748591423,
-0.029739243909716606,
0.0903150737285614,
-0.07176873832941055,
-0.07417495548725128,
0.011042078956961632,
0.09844492375850677,
-0.021669449284672737,
-0.020865987986326218,
0.03896905481815338,
-0.011836287565529346,
0.07959244400262833,
-0.07801046222448349,
0.0390578992664814,
-0.04327036812901497,
0.06733061373233795,
0.018808700144290924,
-0.015179538168013096,
0.05397530272603035,
0.048762060701847076,
0.018949514254927635,
-0.08788836747407913,
0.052680209279060364,
0.023998210206627846,
-0.03772588446736336,
0.0015308556612581015,
0.04504954069852829,
0.012174541130661964,
-0.03826572746038437,
-0.03464578092098236,
0.013158217072486877,
-0.04248381033539772,
-0.021305488422513008,
-0.07872166484594345,
-0.017253296449780464,
0.10568275302648544,
0.03623521327972412,
-0.07760540395975113,
0.08417560160160065,
0.09576984494924545,
-0.012977121397852898,
-0.0329156331717968,
0.06817096471786499,
-0.06888794898986816,
-0.00644717225804925,
-0.08461116254329681,
-0.002856241539120674,
-0.03716477379202843,
-0.00599440885707736,
-0.015838783234357834,
-0.007005586288869381,
-0.0342811681330204,
0.021862495690584183,
-0.006257726810872555,
-0.11199956387281418,
-0.030420402064919472,
0.005678792949765921,
-0.0696246474981308,
-0.014096226543188095,
0.03104136697947979,
-0.038643788546323776,
0.007684060372412205,
-0.0015905986074358225,
0.07051289826631546,
-0.11356622725725174,
-0.05220216140151024,
-0.0030920743010938168,
0.0055985040962696075,
0.055492497980594635,
-0.014317335560917854,
-0.036000557243824005,
0.019275622442364693,
0.05061366781592369,
-0.06135133281350136,
0.015810072422027588,
-0.04551003500819206,
-0.0703788697719574,
0.03134972229599953,
-0.06058148667216301,
0.004440782591700554,
0.03158034384250641,
0.03935667872428894,
0.03370312973856926,
0.11522460728883743,
-0.0028277477249503136,
0.029052767902612686,
0.06704060733318329,
-0.0027715228497982025,
0.024273188784718513,
-0.06258539110422134,
0.08878472447395325,
0.047754570841789246,
0.08984013646841049,
-0.04828573018312454,
-5.312269024670968e-8,
-0.03716908022761345,
-0.05278796702623367,
-0.0492546521127224,
0.03560585156083107,
-0.041129015386104584,
-0.07869132608175278,
-0.020927274599671364,
0.05964990705251694,
-0.025755625218153,
-0.05130939558148384,
0.007709062658250332,
-0.021403329446911812,
-0.08040117472410202,
-0.038675256073474884,
-0.00019044955843128264,
0.09523563832044601,
0.07952223718166351,
0.030008064582943916,
-0.01457345299422741,
0.027132393792271614,
0.029784051701426506,
0.018157539889216423,
0.015153123065829277,
-0.04718704894185066,
-0.017952432855963707,
0.011632394976913929,
-0.004646481946110725,
0.0933365598320961,
-0.008565369993448257,
-0.05737724155187607,
0.0062802196480333805,
-0.010987629182636738,
-0.06440013647079468,
-0.06573215872049332,
-0.020899804309010506,
0.06927300244569778,
0.028739402070641518,
-0.008940123952925205,
0.03483295813202858,
0.004267917480319738,
0.04349470138549805,
0.03499967232346535,
-0.06494254618883133,
0.045252084732055664,
0.009373994544148445,
-0.020365791395306587,
-0.030072975903749466,
-0.11608543246984482,
0.018593356013298035,
-0.028519686311483383,
0.0296353530138731,
0.03337819129228592,
-0.017361659556627274,
-0.007517275866121054,
0.056677717715501785,
-0.010782608762383461,
0.017969809472560883,
-0.011396381072700024,
-0.029609166085720062,
0.006537101697176695,
-0.006604933179914951,
0.015415215864777565,
-0.006623896304517984,
-0.0024758491199463606
] |
nghuyong/ernie-gram-zh | 257fee0915f1cba8dbea92c976493dcdd0491174 | 2022-04-04T06:00:26.000Z | [
"pytorch",
"bert",
"feature-extraction",
"zh",
"arxiv:2010.12148",
"transformers"
] | feature-extraction | false | nghuyong | null | nghuyong/ernie-gram-zh | 1,943 | null | transformers | ---
language: zh
---
# ERNIE-Gram-zh
## Introduction
ERNIE-Gram: Pre-Training with Explicitly N-Gram Masked Language Modeling for Natural Language Understanding
More detail: https://arxiv.org/abs/2010.12148
## Released Model Info
|Model Name|Language|Model Structure|
|:---:|:---:|:---:|
|ernie-gram-zh| Chinese |Layer:12, Hidden:768, Heads:12|
This released Pytorch model is converted from the officially released PaddlePaddle ERNIE model and
a series of experiments have been conducted to check the accuracy of the conversion.
- Official PaddlePaddle ERNIE repo: https://github.com/PaddlePaddle/ERNIE
- Pytorch Conversion repo: https://github.com/nghuyong/ERNIE-Pytorch
## How to use
```Python
from transformers import AutoTokenizer, AutoModel
tokenizer = AutoTokenizer.from_pretrained("nghuyong/ernie-gram-zh")
model = AutoModel.from_pretrained("nghuyong/ernie-gram-zh")
``` | [
-0.1279805302619934,
-0.03280772268772125,
-0.004073866177350283,
-0.02629801072180271,
-0.0890774056315422,
0.011164892464876175,
0.007379804737865925,
0.02164941094815731,
-0.021613966673612595,
-0.02596859820187092,
0.06613249331712723,
-0.054981473833322525,
0.023415373638272285,
0.03168141096830368,
0.009278042241930962,
-0.012220636010169983,
0.03530748188495636,
0.1039368286728859,
-0.09070797264575958,
-0.11831961572170258,
0.08845548331737518,
0.05472572520375252,
0.02089601196348667,
-0.02979428321123123,
0.04949883744120598,
-0.049947138875722885,
-0.022918740287423134,
-0.013882419094443321,
0.10136104375123978,
0.024974824860692024,
-0.03951859474182129,
0.0070757633075118065,
0.001126664923503995,
0.05083153396844864,
0.0448068231344223,
0.05597029998898506,
-0.06779196858406067,
0.002804332412779331,
0.045146193355321884,
-0.00963541492819786,
0.03497190400958061,
-0.04094657674431801,
0.025418493896722794,
-0.04796134680509567,
0.05093836411833763,
0.06442199647426605,
-0.023551378399133682,
-0.00022843075566925108,
-0.0663515105843544,
-0.04268287494778633,
-0.012112434022128582,
-0.04566733539104462,
0.05875037983059883,
0.05594858154654503,
-0.02513420581817627,
-0.014294934459030628,
-0.0004171405453234911,
0.0014887299621477723,
-0.005081615410745144,
-0.022575702518224716,
-0.16133050620555878,
0.04177806153893471,
-0.06158517673611641,
0.0013556889025494456,
-0.02671092562377453,
-0.0034648955333977938,
-0.04837345331907272,
-0.04194292053580284,
0.013874850235879421,
0.022654417902231216,
-0.10599778592586517,
-0.019875073805451393,
0.016233686357736588,
0.0205300971865654,
0.028762536123394966,
-0.08145331591367722,
0.08820771425962448,
0.003378588007763028,
0.04206443578004837,
-0.08427033573389053,
0.032978300005197525,
-0.01917196810245514,
0.07489796727895737,
0.06699808686971664,
0.04418317601084709,
0.0022109125275164843,
-0.029502425342798233,
0.07759298384189606,
0.019801748916506767,
0.02075204625725746,
-0.036946482956409454,
-0.08028294891119003,
0.031778961420059204,
0.055524908006191254,
0.019151752814650536,
0.06326161324977875,
-0.015061972662806511,
0.05922288820147514,
-0.03778567165136337,
0.06098566949367523,
0.01653173565864563,
0.008770066313445568,
0.055313099175691605,
-0.0892811119556427,
-0.006113533861935139,
0.011532716453075409,
-0.009124274365603924,
-0.00266531971283257,
0.0598992295563221,
-0.03695521876215935,
0.01863311231136322,
-0.02410602755844593,
-0.04567725211381912,
-0.0763755813241005,
-0.005129767116159201,
-0.016835065558552742,
-0.021022332832217216,
0.021512044593691826,
0.02598569728434086,
0.03782300651073456,
0.002179966773837805,
-0.0019035676959902048,
0.01460090558975935,
0.04897420108318329,
-0.013035128824412823,
0.04908151179552078,
-0.009160080924630165,
2.6818800758254793e-33,
-0.020728599280118942,
0.02687588892877102,
0.010143103078007698,
-0.013327168300747871,
-0.0058624339289963245,
-0.006789256352931261,
0.04523732513189316,
-0.009618515148758888,
0.03779732435941696,
0.045162126421928406,
-0.038742367178201675,
0.0036708565894514322,
-0.13136272132396698,
0.008219431154429913,
-0.06112796068191528,
0.032788265496492386,
-0.037764742970466614,
0.01369552407413721,
-0.02109985426068306,
0.041895292699337006,
0.10756046324968338,
0.08724681288003922,
-0.027566300705075264,
-0.06332696229219437,
-0.01815376803278923,
0.03412304073572159,
0.028888611122965813,
-0.13483063876628876,
-0.033942077308893204,
0.06023851037025452,
-0.06558270752429962,
-0.038872577250003815,
-0.021465569734573364,
-0.020185042172670364,
-0.06753168255090714,
-0.05882326140999794,
0.029261389747262,
-0.08237595856189728,
-0.033481307327747345,
-0.11834628880023956,
0.011886422522366047,
-0.007660060655325651,
-0.021536054089665413,
-0.027104703709483147,
-0.09181921184062958,
-0.03079318441450596,
0.011559046804904938,
0.03900780901312828,
0.029222674667835236,
0.0037008016370236874,
0.09362833946943283,
0.027984851971268654,
-0.026616467162966728,
0.006185830570757389,
0.0019545024260878563,
-0.0175576563924551,
0.0739370808005333,
0.035804085433483124,
0.062022071331739426,
0.02528674528002739,
0.006606552749872208,
0.03732822462916374,
0.058136649429798126,
0.0512503944337368,
0.06219949573278427,
-0.013879667036235332,
0.055840421468019485,
-0.03490796685218811,
0.006635480560362339,
-0.011389209888875484,
-0.10567256063222885,
-0.07799093425273895,
-0.09914557635784149,
0.000913978845346719,
0.02423057332634926,
-0.04096111282706261,
0.03545021265745163,
-0.07777564972639084,
-0.0175637174397707,
0.0029481155797839165,
-0.03326911851763725,
0.10215747356414795,
-0.014681976288557053,
-0.020235363394021988,
-0.04086783155798912,
-0.0073814187198877335,
0.059386562556028366,
-0.04140002653002739,
0.006608414929360151,
-0.0790758952498436,
0.027984555810689926,
-0.0811026319861412,
-0.005807674955576658,
0.027838755398988724,
-0.03163100406527519,
-5.203986224803224e-33,
-0.02414010651409626,
0.12190057337284088,
-0.08110102266073227,
0.08625341206789017,
-0.06864319741725922,
-0.12228135019540787,
0.09273717552423477,
0.080284483730793,
-0.002770076273009181,
-0.050229571759700775,
-0.02413896843791008,
-0.005934933666139841,
0.0012840742710977793,
0.01744324341416359,
0.1283176839351654,
0.02077099122107029,
-0.08286159485578537,
0.09300313889980316,
0.05276394262909889,
0.036339759826660156,
0.0028104770462960005,
0.03646454960107803,
-0.1069290041923523,
0.0735885500907898,
-0.062463100999593735,
0.013674967922270298,
0.004776451271027327,
0.06782195717096329,
0.015400460921227932,
0.0557326003909111,
-0.04336618632078171,
0.029595941305160522,
0.006682505831122398,
0.054834313690662384,
-0.14892688393592834,
-0.011558743193745613,
0.0033179123420268297,
0.005951788276433945,
-0.002239957917481661,
0.03410431370139122,
0.062255859375,
-0.009385133162140846,
-0.07564478367567062,
0.049715571105480194,
-0.10171034187078476,
-0.0004994949558749795,
-0.06259012222290039,
-0.015555675141513348,
0.024491673335433006,
-0.012683077715337276,
0.024320948868989944,
0.03492594137787819,
-0.05288940668106079,
-0.03934634476900101,
-0.0729137510061264,
-0.0012690955772995949,
0.011780313216149807,
-0.09681794792413712,
-0.04611086845397949,
-0.039712678641080856,
-0.10422852635383606,
-0.0512525737285614,
0.03503888100385666,
-0.024459952488541603,
0.01892382837831974,
-0.005562473554164171,
-0.01844032108783722,
0.012767426669597626,
0.01664891466498375,
-0.07349807024002075,
0.04502454400062561,
0.062186870723962784,
0.04839182272553444,
0.034039683640003204,
0.004458348732441664,
0.03229168429970741,
0.033393796533346176,
-0.04364747554063797,
0.04115056246519089,
-0.03193425014615059,
-0.12993691861629486,
0.013073112815618515,
0.031035173684358597,
0.06642965972423553,
-0.03558799996972084,
0.013416396453976631,
0.022559601813554764,
0.0835268422961235,
0.025874124839901924,
0.0066438219510018826,
0.02113467827439308,
0.09760519117116928,
0.01201560627669096,
0.09102542698383331,
-0.03582486882805824,
-5.362274180242821e-8,
-0.07347854226827621,
0.06802006810903549,
0.015157675370573997,
0.030815819278359413,
-0.04447823762893677,
-0.004132520407438278,
-0.041567008942365646,
0.01632794737815857,
-0.059941358864307404,
-0.011777748353779316,
0.007067324128001928,
-0.006169588770717382,
-0.03142121806740761,
-1.1540164024381738e-7,
-0.028479719534516335,
0.12063825130462646,
0.0052659036591649055,
0.0947633758187294,
-0.044543951749801636,
-0.016731420531868935,
-0.0030842595733702183,
0.0228780098259449,
0.0500904805958271,
-0.022228077054023743,
-0.06454794853925705,
-0.002878449624404311,
-0.08443071693181992,
0.11705834418535233,
-0.029880356043577194,
0.019590629264712334,
0.0028200780507177114,
0.04961337149143219,
-0.012185407802462578,
-0.0022317187394946814,
-0.029017316177487373,
0.09056230634450912,
-0.038083698600530624,
-0.08401970565319061,
0.040112562477588654,
0.044212456792593,
0.014593343250453472,
-0.05320346727967262,
-0.08465289324522018,
-0.009032489731907845,
0.017476527020335197,
0.003255255986005068,
-0.003456221194937825,
-0.12283571064472198,
0.002989959204569459,
0.015579133294522762,
0.048708800226449966,
0.00375764979980886,
-0.0485164113342762,
0.021915771067142487,
0.05422881990671158,
0.0038617639802396297,
-0.059170398861169815,
-0.04638662934303284,
0.03947160020470619,
0.051589012145996094,
-0.052298903465270996,
0.05322623997926712,
0.028340840712189674,
0.009151685982942581
] |
monologg/distilkobert | cfbce1328041f68781414250c9013128e77e82d2 | 2020-05-13T03:37:29.000Z | [
"pytorch",
"distilbert",
"fill-mask",
"transformers",
"autotrain_compatible"
] | fill-mask | false | monologg | null | monologg/distilkobert | 1,941 | 2 | transformers | Entry not found | [
0.0461147278547287,
-0.038838207721710205,
-0.01049656979739666,
-0.03682169318199158,
0.011261860840022564,
0.013094935566186905,
0.0019101888174191117,
-0.013979103416204453,
0.027092741802334785,
-0.015212527476251125,
0.017284274101257324,
-0.08189476281404495,
0.03817418962717056,
-0.04920130595564842,
0.021389011293649673,
-0.015245908871293068,
-0.03203780576586723,
-0.1245758980512619,
0.03150877356529236,
0.032381657510995865,
-0.060957908630371094,
0.05409295856952667,
-0.025087490677833557,
0.01568586938083172,
0.028129950165748596,
-0.04710396006703377,
-0.018688226118683815,
0.013785239309072495,
-0.04001208767294884,
0.01173911802470684,
-0.04317743331193924,
0.05500618368387222,
0.004543041344732046,
0.02973111905157566,
0.14852192997932434,
0.02658126689493656,
0.02907961793243885,
-0.05169107764959335,
0.05803573504090309,
-0.07732241600751877,
-0.017637968063354492,
-0.04219653457403183,
0.041807834059000015,
0.023620979860424995,
0.021563321352005005,
0.016478516161441803,
-0.0021814992651343346,
-0.06400240957736969,
0.06393089145421982,
0.019599027931690216,
-0.08565037697553635,
0.00934905931353569,
-0.008718925528228283,
-0.028583496809005737,
-0.07310017943382263,
0.09416428208351135,
0.001759322709403932,
0.06184990331530571,
0.011840506456792355,
-0.035997264087200165,
0.08358278125524521,
-0.02619801089167595,
0.03736566752195358,
-0.028206506744027138,
-0.07454850524663925,
-0.08883563429117203,
-0.06279942393302917,
-0.008695344440639019,
0.014119276776909828,
-0.0825355276465416,
0.0649217739701271,
-0.00223911227658391,
-0.14716917276382446,
0.07743025571107864,
-0.03548373281955719,
-0.055201586335897446,
0.006981803569942713,
-0.012166670523583889,
0.055111464112997055,
-0.007116836030036211,
-0.023175746202468872,
-0.005835152696818113,
-0.09185640513896942,
0.055196937173604965,
0.034148022532463074,
0.03835180774331093,
0.038685429841279984,
-0.025987252593040466,
0.017804903909564018,
0.022428328171372414,
0.025005368515849113,
-0.10761535167694092,
-0.048001550137996674,
-0.04343584179878235,
0.012374646961688995,
-0.019502125680446625,
0.029218152165412903,
0.0842173621058464,
-0.011719699949026108,
0.09283553808927536,
-0.007015465293079615,
-0.03543110564351082,
-0.06936459988355637,
0.09425332397222519,
-0.010958523489534855,
-0.00805904995650053,
0.004974212497472763,
-0.0031528924591839314,
0.06105927750468254,
-0.03964288905262947,
-0.03619541600346565,
-0.019901901483535767,
0.07134733349084854,
0.039514873176813126,
-0.012729483656585217,
-0.006646515801548958,
-0.04746140539646149,
-0.014432490803301334,
-0.05157482624053955,
0.09506245702505112,
-0.049747664481401443,
-0.04591796174645424,
-0.008965466171503067,
-0.0325421579182148,
-0.08626784384250641,
-0.06624380499124527,
0.02538885548710823,
-4.303924894057984e-33,
0.01133066974580288,
0.0033434738870710135,
-0.002155609894543886,
0.04871906340122223,
-0.023564351722598076,
-0.07933273911476135,
0.0600903145968914,
0.02335330657660961,
-0.03844716399908066,
-0.020433755591511726,
-0.06952055543661118,
-0.03235611692070961,
0.0062485747039318085,
0.064804308116436,
-0.03201229125261307,
0.061689723283052444,
0.0417000837624073,
-0.00761845987290144,
0.03340127319097519,
-0.047770582139492035,
0.00887306872755289,
-0.04066338762640953,
-0.010506896302103996,
0.0106519665569067,
0.021333497017621994,
0.12854498624801636,
-0.009705503471195698,
0.010055632330477238,
-0.017507633194327354,
0.006515394430607557,
0.06334009766578674,
-0.057817306369543076,
0.013668818399310112,
-0.020286159589886665,
0.05430467426776886,
-0.023184705525636673,
0.0828516036272049,
0.0005449643940664828,
-0.10372652113437653,
-0.07634282112121582,
-0.005381610710173845,
-0.039263784885406494,
0.0006114727002568543,
-0.013281986117362976,
0.07119110971689224,
0.043696220964193344,
0.03168422728776932,
0.04338686540722847,
0.05728672817349434,
0.0832006186246872,
-0.07961414009332657,
0.015234283171594143,
0.017002005130052567,
0.047004107385873795,
-0.09794387966394424,
0.004990279674530029,
-0.07062993198633194,
-0.028000490739941597,
-0.04018733277916908,
-0.0702052190899849,
0.011351344175636768,
0.06020182743668556,
-0.03297270089387894,
0.09396500885486603,
0.03417910635471344,
-0.019825750961899757,
-0.034690454602241516,
-0.013036907650530338,
0.05896938592195511,
-0.012359356507658958,
-0.017275206744670868,
-0.07982361316680908,
0.02059139870107174,
0.06737419217824936,
0.04176458343863487,
-0.04978838190436363,
-0.05877475067973137,
-0.06289287656545639,
-0.03354167565703392,
-0.03871942684054375,
0.009898529388010502,
-0.05514208599925041,
-0.11629002541303635,
-0.011855563148856163,
0.10663620382547379,
0.037354156374931335,
-0.0065480442717671394,
-0.051189567893743515,
0.06663123518228531,
0.01874656230211258,
0.032841797918081284,
0.041593004018068314,
-0.06879369914531708,
0.04216769337654114,
-0.01628219522535801,
5.4139394340936695e-34,
0.05697013810276985,
-0.006972255185246468,
0.015711724758148193,
-0.17956365644931793,
0.02320219948887825,
0.007923615165054798,
-0.008062449283897877,
0.0074974060989916325,
0.07391711324453354,
0.0309313777834177,
0.060510627925395966,
0.058605875819921494,
0.09515274316072464,
-0.002282935893163085,
0.001603541080839932,
0.07024981826543808,
0.012629246339201927,
0.07425693422555923,
-0.038426291197538376,
0.01861148327589035,
0.030608950182795525,
-0.02449394389986992,
0.021528491750359535,
-0.003039651783183217,
-0.03676343336701393,
0.03130284696817398,
0.07998586446046829,
0.010451192036271095,
-0.07930229604244232,
-0.013543923385441303,
0.018781835213303566,
0.05168003588914871,
-0.07191970944404602,
0.15783067047595978,
0.026191607117652893,
0.01262354850769043,
0.08218053728342056,
-0.029807550832629204,
-0.07528624683618546,
-0.04250097647309303,
0.017244765534996986,
0.04411793500185013,
0.03708017244935036,
0.009233047254383564,
-0.040271829813718796,
0.022496428340673447,
0.02495843544602394,
0.07633638381958008,
0.005147108342498541,
0.013892097398638725,
0.05610476806759834,
-0.06684739887714386,
0.05862557515501976,
-0.020688841119408607,
0.05377643182873726,
0.06718500703573227,
0.005329249892383814,
-0.01388032827526331,
0.029931528493762016,
0.009508464485406876,
-0.045173756778240204,
0.11534366756677628,
-0.06510116159915924,
0.05117698386311531,
-0.0026125339791178703,
-0.08554837852716446,
-0.03784770518541336,
0.0804959163069725,
0.011298024095594883,
-0.07695550471544266,
-0.04868878796696663,
0.02515520341694355,
0.06252261996269226,
-0.04509226232767105,
-0.01246943511068821,
0.028559505939483643,
-0.030573077499866486,
0.05066261067986488,
-0.08187384903430939,
0.04469604790210724,
0.0034051244147121906,
0.04145054519176483,
-0.021858664229512215,
-0.06112268194556236,
-0.00908052921295166,
-0.05903250351548195,
0.0259539932012558,
0.059690944850444794,
-0.07613514363765717,
-0.03720718249678612,
-0.036316655576229095,
0.07058046013116837,
-0.008224100805819035,
0.041961874812841415,
-0.0285952128469944,
-1.496900736697171e-8,
-0.0014124972512945533,
0.03401879221200943,
-0.040338415652513504,
0.04116074740886688,
0.0935964286327362,
-0.05115952715277672,
0.0008746005478315055,
-0.03389839455485344,
-0.00567849725484848,
-0.010686947964131832,
-0.04789939522743225,
-0.04820054769515991,
-0.02011880651116371,
-0.03209094703197479,
-0.04211259260773659,
-0.10229527950286865,
-0.07819421589374542,
-0.031228765845298767,
-0.02154778689146042,
-0.04960230365395546,
0.08087796717882156,
-0.07801242172718048,
0.06919731199741364,
-0.04999840259552002,
0.03687043860554695,
0.03889009356498718,
-0.049989692866802216,
-0.04254625365138054,
-0.04606937617063522,
0.08682432025671005,
-0.031148413196206093,
0.11826753616333008,
0.034102488309144974,
-0.0208592489361763,
-0.0205202866345644,
0.027134142816066742,
0.09741277992725372,
0.051608603447675705,
0.013477512635290623,
-0.13649295270442963,
-0.022304272279143333,
0.02385953813791275,
0.038732077926397324,
-0.09249968826770782,
-0.04549082741141319,
0.054220106452703476,
0.01160438358783722,
0.051190607249736786,
0.07713303714990616,
-0.022097084671258926,
-0.06127818301320076,
-0.01857956498861313,
0.006740490905940533,
-0.00496308971196413,
0.024095389991998672,
0.0736224576830864,
-0.003481915919110179,
-0.0699305310845375,
-0.006629763171076775,
-0.0598808117210865,
0.05297163128852844,
-0.02902800403535366,
-0.027858933433890343,
-0.01287526823580265
] |
sentence-transformers/msmarco-distilbert-base-dot-prod-v3 | 0bafe057815532ca7ee37f002d9d1413d78b6d67 | 2022-06-15T22:20:51.000Z | [
"pytorch",
"tf",
"distilbert",
"feature-extraction",
"arxiv:1908.10084",
"sentence-transformers",
"sentence-similarity",
"transformers",
"license:apache-2.0"
] | sentence-similarity | false | sentence-transformers | null | sentence-transformers/msmarco-distilbert-base-dot-prod-v3 | 1,936 | 1 | sentence-transformers | ---
pipeline_tag: sentence-similarity
license: apache-2.0
tags:
- sentence-transformers
- feature-extraction
- sentence-similarity
- transformers
---
# sentence-transformers/msmarco-distilbert-base-dot-prod-v3
This is a [sentence-transformers](https://www.SBERT.net) model: It maps sentences & paragraphs to a 768 dimensional dense vector space and can be used for tasks like clustering or semantic search.
## Usage (Sentence-Transformers)
Using this model becomes easy when you have [sentence-transformers](https://www.SBERT.net) installed:
```
pip install -U sentence-transformers
```
Then you can use the model like this:
```python
from sentence_transformers import SentenceTransformer
sentences = ["This is an example sentence", "Each sentence is converted"]
model = SentenceTransformer('sentence-transformers/msmarco-distilbert-base-dot-prod-v3')
embeddings = model.encode(sentences)
print(embeddings)
```
## Evaluation Results
For an automated evaluation of this model, see the *Sentence Embeddings Benchmark*: [https://seb.sbert.net](https://seb.sbert.net?model_name=sentence-transformers/msmarco-distilbert-base-dot-prod-v3)
## Full Model Architecture
```
SentenceTransformer(
(0): Transformer({'max_seq_length': 512, 'do_lower_case': False}) with Transformer model: DistilBertModel
(1): Pooling({'word_embedding_dimension': 768, 'pooling_mode_cls_token': False, 'pooling_mode_mean_tokens': True, 'pooling_mode_max_tokens': False, 'pooling_mode_mean_sqrt_len_tokens': False})
(2): Dense({'in_features': 768, 'out_features': 768, 'bias': False, 'activation_function': 'torch.nn.modules.linear.Identity'})
)
```
## Citing & Authors
This model was trained by [sentence-transformers](https://www.sbert.net/).
If you find this model helpful, feel free to cite our publication [Sentence-BERT: Sentence Embeddings using Siamese BERT-Networks](https://arxiv.org/abs/1908.10084):
```bibtex
@inproceedings{reimers-2019-sentence-bert,
title = "Sentence-BERT: Sentence Embeddings using Siamese BERT-Networks",
author = "Reimers, Nils and Gurevych, Iryna",
booktitle = "Proceedings of the 2019 Conference on Empirical Methods in Natural Language Processing",
month = "11",
year = "2019",
publisher = "Association for Computational Linguistics",
url = "http://arxiv.org/abs/1908.10084",
}
``` | [
-0.0664677545428276,
-0.0682670846581459,
-0.026655318215489388,
0.03809172287583351,
0.03860831633210182,
0.04941051825881004,
-0.04626838117837906,
0.057916004210710526,
0.0019445703364908695,
-0.06177717074751854,
0.0403127521276474,
-0.0071830060333013535,
0.05793493613600731,
0.04304727539420128,
0.059030864387750626,
0.04747176170349121,
0.029568126425147057,
0.09049411863088608,
-0.05163247883319855,
-0.107163205742836,
0.13494721055030823,
0.12098041921854019,
0.002731817774474621,
0.0266177486628294,
-0.014306978322565556,
0.08518534898757935,
-0.033624835312366486,
0.003180719679221511,
0.010144779458642006,
-0.017104975879192352,
0.05950415879487991,
-0.022249821573495865,
-0.026804368942975998,
0.08092588931322098,
0.06388251483440399,
0.0710522010922432,
0.008268782868981361,
-0.0006262151291593909,
-0.024546856060624123,
-0.08904323726892471,
0.012611513026058674,
-0.021275481209158897,
-0.04718044400215149,
-0.02057259902358055,
0.034356433898210526,
-0.07742823660373688,
-0.10788630694150925,
-0.01765473559498787,
-0.004357991740107536,
-0.02273569628596306,
-0.1252569556236267,
0.023164531216025352,
0.004891316872090101,
0.07123855501413345,
0.00035964863491244614,
0.0334169864654541,
0.03942669555544853,
0.0130459601059556,
0.00893437396734953,
-0.1416730135679245,
-0.07730759680271149,
-0.017298130318522453,
0.005088530946522951,
-0.018703021109104156,
-0.040474873036146164,
-0.029656056314706802,
0.02979283034801483,
0.0005861974786967039,
0.02049942873418331,
-0.012555365450680256,
-0.10854140669107437,
0.050762519240379333,
-0.046601224690675735,
-0.020215144380927086,
-0.059619441628456116,
0.03551698103547096,
0.07713446021080017,
-0.011854712851345539,
0.04094132408499718,
0.022115478292107582,
-0.015523744747042656,
-0.07438252121210098,
0.029392845928668976,
0.08351211249828339,
0.03649310767650604,
-0.06330420076847076,
0.0021546445786952972,
-0.024465326219797134,
0.013198324479162693,
-0.009479680098593235,
-0.05669139698147774,
-0.12587228417396545,
0.0052337804809212685,
-0.0358063280582428,
0.017692016437649727,
0.042081352323293686,
-0.04643911123275757,
-0.008332916535437107,
0.040837548673152924,
0.061190150678157806,
0.02564871683716774,
0.009508222341537476,
0.03275078535079956,
-0.12069713324308395,
-0.04822993278503418,
0.030284631997346878,
-0.019076185300946236,
0.025199774652719498,
0.07092364877462387,
-0.12340867519378662,
0.018209220841526985,
0.008045053109526634,
-0.03553588688373566,
-0.0234802458435297,
0.05002877116203308,
-0.045185450464487076,
0.016298504546284676,
-0.03744276985526085,
0.0007786567439325154,
0.08520057797431946,
-0.01405086275190115,
0.0702534168958664,
-0.023948656395077705,
0.018137630075216293,
-0.009394030086696148,
-0.01388720516115427,
0.0038574098143726587,
9.7018673194049e-34,
-0.034354835748672485,
-0.014487724751234055,
-0.004412449896335602,
0.013562852516770363,
0.015492460690438747,
0.030338000506162643,
0.021645741537213326,
0.055885929614305496,
-0.09146969765424728,
-0.05953315272927284,
-0.05857432633638382,
0.02678018808364868,
-0.02856198325753212,
0.06287281215190887,
0.013162738643586636,
-0.021576037630438805,
-0.025436649098992348,
-0.029244741424918175,
0.06788958609104156,
0.004975032061338425,
0.030071943998336792,
0.030088884755969048,
0.010439311154186726,
-0.024150658398866653,
-0.10528073459863663,
0.0021998975425958633,
0.058298252522945404,
-0.06829682737588882,
-0.028830746188759804,
0.01347156148403883,
-0.08342323452234268,
0.025080781430006027,
-0.013485696166753769,
0.026334580034017563,
0.00026663043536245823,
0.002523133298382163,
0.005350785795599222,
-0.04737728834152222,
-0.0275566466152668,
-0.06358933448791504,
-0.03818982094526291,
0.015406427904963493,
-0.010321958921849728,
-0.0478757806122303,
-0.010166828520596027,
0.004529439378529787,
0.029194045811891556,
0.030500931665301323,
0.11812426894903183,
-0.0005483650602400303,
0.08522573113441467,
0.004474812187254429,
0.016416028141975403,
-0.041171710938215256,
0.02198699302971363,
0.026288829743862152,
0.04433590546250343,
0.03545249253511429,
0.11796237528324127,
-0.021796131506562233,
0.02435271441936493,
-0.021002765744924545,
0.03387818858027458,
0.03039586916565895,
0.09974442422389984,
-0.03139088675379753,
0.06063935160636902,
0.04927992448210716,
0.00885539036244154,
0.09575971961021423,
-0.03036026656627655,
0.031541045755147934,
-0.05360168218612671,
0.04188045114278793,
0.0053225127048790455,
-0.019841281697154045,
0.0008136841934174299,
-0.07488624006509781,
-0.03314724192023277,
0.07657666504383087,
-0.05052131041884422,
-0.01749691553413868,
0.05135759711265564,
-0.07811238616704941,
-0.009099717251956463,
-0.035599514842033386,
0.003208337351679802,
-0.04667818918824196,
0.06678862869739532,
-0.06529540568590164,
0.06000664085149765,
-0.000007815709977876395,
0.005894732661545277,
0.06066720560193062,
0.04598283767700195,
-2.6433152285818694e-33,
-0.013265259563922882,
0.020787354558706284,
-0.0683082789182663,
0.056975435465574265,
-0.02786330133676529,
-0.04817431420087814,
0.01869594305753708,
0.05700475722551346,
-0.005211497191339731,
-0.02362082339823246,
-0.048181310296058655,
-0.038805510848760605,
0.09312043339014053,
-0.065952368080616,
0.06713267415761948,
0.08345908671617508,
-0.023759426549077034,
0.0481385737657547,
0.030072299763560295,
0.06834829598665237,
0.003945903852581978,
0.10077893733978271,
-0.10678914189338684,
0.04240656644105911,
-0.024507390335202217,
-0.023800648748874664,
-0.024907970800995827,
-0.015263134613633156,
-0.03085949830710888,
-0.03466581925749779,
-0.005807683803141117,
0.012991946190595627,
-0.062123559415340424,
-0.03313705325126648,
-0.11774259060621262,
0.014103211462497711,
-0.023047633469104767,
-0.06352370232343674,
0.052083682268857956,
0.042587872594594955,
0.017102496698498726,
0.0884568989276886,
-0.029424019157886505,
-0.0072395396418869495,
0.002187344478443265,
0.00765601173043251,
-0.06749653071165085,
-0.0727691799402237,
0.061379410326480865,
0.00719318026676774,
-0.03742232173681259,
0.04368120804429054,
-0.12174711376428604,
0.006634457968175411,
-0.04686200991272926,
-0.08374283462762833,
-0.048920467495918274,
-0.016437632963061333,
-0.10891997814178467,
-0.07585057616233826,
-0.07991256564855576,
-0.014603408053517342,
0.013797200284898281,
-0.07281865924596786,
0.08831530064344406,
-0.01769246719777584,
-0.00912017934024334,
0.024658553302288055,
-0.04625581204891205,
-0.039064209908246994,
-0.001452560885809362,
-0.039229076355695724,
0.015268848277628422,
0.043511442840099335,
0.028148429468274117,
-0.05477342754602432,
-0.012714089825749397,
0.022495334967970848,
-0.04192982614040375,
-0.04651126265525818,
0.030818918719887733,
-0.03615967556834221,
0.0307533647865057,
-0.051273901015520096,
0.017262563109397888,
-0.028094982728362083,
0.02224930189549923,
0.0920417532324791,
-0.015100928023457527,
0.04662449285387993,
-0.013985882513225079,
-0.031278304755687714,
-0.007268378045409918,
0.08473912626504898,
0.07549446076154709,
-5.128475422111478e-8,
-0.07644663751125336,
-0.024287618696689606,
-0.08088713139295578,
0.056853845715522766,
-0.08110915869474411,
-0.030644932761788368,
0.05047148838639259,
0.06621246039867401,
-0.05668272823095322,
-0.006801865994930267,
0.008874591439962387,
-0.013231009244918823,
-0.0949917882680893,
0.0017976239323616028,
-0.014519546180963516,
0.12295092642307281,
-0.02449807897210121,
0.0565696656703949,
0.022029979154467583,
-0.014579158276319504,
0.03166920691728592,
-0.007485387846827507,
-0.00947971735149622,
0.04408855736255646,
-0.008439325727522373,
0.007671753875911236,
-0.0335368774831295,
0.026183828711509705,
-0.0016291337087750435,
0.0014593026135116816,
-0.011314409784972668,
0.019474247470498085,
-0.04636992886662483,
-0.06528054177761078,
0.01478835754096508,
0.05585021898150444,
0.06791814416646957,
-0.027728937566280365,
0.04798918217420578,
0.09691836684942245,
0.05290643498301506,
0.06166788563132286,
-0.13345584273338318,
0.0005402223905548453,
0.08891358226537704,
0.0447503998875618,
-0.016542283818125725,
-0.05192333459854126,
0.012441395781934261,
0.02747306041419506,
0.09376870095729828,
-0.07006805390119553,
-0.015920205041766167,
-0.016087060794234276,
0.036791395395994186,
0.041817981749773026,
0.014066426083445549,
-0.029835084453225136,
0.05064220353960991,
-0.07965876162052155,
0.05941193178296089,
0.09582298994064331,
0.1266934871673584,
-0.08633309602737427
] |
cahya/bert-base-indonesian-522M | 7baa8f5fa385e6eff31184f11876d0d19bf5eb6c | 2021-05-19T13:38:45.000Z | [
"pytorch",
"tf",
"jax",
"bert",
"fill-mask",
"id",
"dataset:wikipedia",
"transformers",
"license:mit",
"autotrain_compatible"
] | fill-mask | false | cahya | null | cahya/bert-base-indonesian-522M | 1,934 | 3 | transformers | ---
language: "id"
license: "mit"
datasets:
- wikipedia
widget:
- text: "Ibu ku sedang bekerja [MASK] sawah."
---
# Indonesian BERT base model (uncased)
## Model description
It is BERT-base model pre-trained with indonesian Wikipedia using a masked language modeling (MLM) objective. This
model is uncased: it does not make a difference between indonesia and Indonesia.
This is one of several other language models that have been pre-trained with indonesian datasets. More detail about
its usage on downstream tasks (text classification, text generation, etc) is available at [Transformer based Indonesian Language Models](https://github.com/cahya-wirawan/indonesian-language-models/tree/master/Transformers)
## Intended uses & limitations
### How to use
You can use this model directly with a pipeline for masked language modeling:
```python
>>> from transformers import pipeline
>>> unmasker = pipeline('fill-mask', model='cahya/bert-base-indonesian-522M')
>>> unmasker("Ibu ku sedang bekerja [MASK] supermarket")
[{'sequence': '[CLS] ibu ku sedang bekerja di supermarket [SEP]',
'score': 0.7983310222625732,
'token': 1495},
{'sequence': '[CLS] ibu ku sedang bekerja. supermarket [SEP]',
'score': 0.090003103017807,
'token': 17},
{'sequence': '[CLS] ibu ku sedang bekerja sebagai supermarket [SEP]',
'score': 0.025469014421105385,
'token': 1600},
{'sequence': '[CLS] ibu ku sedang bekerja dengan supermarket [SEP]',
'score': 0.017966199666261673,
'token': 1555},
{'sequence': '[CLS] ibu ku sedang bekerja untuk supermarket [SEP]',
'score': 0.016971781849861145,
'token': 1572}]
```
Here is how to use this model to get the features of a given text in PyTorch:
```python
from transformers import BertTokenizer, BertModel
model_name='cahya/bert-base-indonesian-522M'
tokenizer = BertTokenizer.from_pretrained(model_name)
model = BertModel.from_pretrained(model_name)
text = "Silakan diganti dengan text apa saja."
encoded_input = tokenizer(text, return_tensors='pt')
output = model(**encoded_input)
```
and in Tensorflow:
```python
from transformers import BertTokenizer, TFBertModel
model_name='cahya/bert-base-indonesian-522M'
tokenizer = BertTokenizer.from_pretrained(model_name)
model = TFBertModel.from_pretrained(model_name)
text = "Silakan diganti dengan text apa saja."
encoded_input = tokenizer(text, return_tensors='tf')
output = model(encoded_input)
```
## Training data
This model was pre-trained with 522MB of indonesian Wikipedia.
The texts are lowercased and tokenized using WordPiece and a vocabulary size of 32,000. The inputs of the model are
then of the form:
```[CLS] Sentence A [SEP] Sentence B [SEP]```
| [
-0.10908884555101395,
-0.030317245051264763,
0.03746083751320839,
0.016159024089574814,
-0.05314822122454643,
0.016744349151849747,
-0.04300319403409958,
0.017528031021356583,
0.006447220221161842,
-0.01688619889318943,
0.015253201127052307,
-0.081357441842556,
-0.03760005533695221,
0.026737630367279053,
0.07591599971055984,
0.029573902487754822,
0.06089698523283005,
0.006957087200134993,
-0.03952444717288017,
-0.1517612338066101,
0.11103363335132599,
0.05274191126227379,
0.014506237581372261,
-0.04793713614344597,
0.042414478957653046,
0.001249221502803266,
0.010379228740930557,
-0.03677459806203842,
0.0801287442445755,
0.02413542941212654,
-0.008253657259047031,
0.006766212172806263,
0.005236903205513954,
0.0833665132522583,
-0.02394086867570877,
0.1031341478228569,
-0.03535589948296547,
0.025219794362783432,
0.04987170547246933,
0.030970320105552673,
-0.0055986810475587845,
-0.03418019413948059,
-0.061372555792331696,
-0.08212725818157196,
0.07631997019052505,
-0.06253419071435928,
-0.09157995879650116,
0.007229774724692106,
-0.08794483542442322,
-0.03599274903535843,
-0.06744414567947388,
-0.023905841633677483,
0.007367383688688278,
0.03416877239942551,
-0.03124404139816761,
-0.030241480097174644,
0.05286083370447159,
0.00793836172670126,
0.00398045452311635,
-0.07756143808364868,
-0.08290155977010727,
-0.032156459987163544,
0.007810194045305252,
0.022586669772863388,
-0.07546721398830414,
-0.0023509471211582422,
-0.031034769490361214,
0.06520742923021317,
0.0019282004795968533,
0.003500811057165265,
-0.05137602612376213,
0.052406661212444305,
0.04063386842608452,
0.019820062443614006,
-0.01267949678003788,
-0.0758170336484909,
0.08523331582546234,
-0.007204841822385788,
0.050173569470644,
-0.020698975771665573,
0.04994523525238037,
0.04956648126244545,
0.07809214293956757,
0.014436486177146435,
0.04797231778502464,
0.023924699053168297,
-0.0066307163797318935,
0.028622105717658997,
-0.04984069615602493,
-0.010824623517692089,
-0.015363774262368679,
-0.14424383640289307,
0.06735236942768097,
-0.002613502787426114,
-0.026394173502922058,
0.006328792776912451,
-0.0024375964421778917,
0.039445552974939346,
-0.01290690153837204,
0.03269559144973755,
0.011778276413679123,
0.002695272909477353,
0.022453097626566887,
-0.08602608740329742,
0.005996473133563995,
0.021087108179926872,
-0.04246165230870247,
-0.03331110253930092,
0.1344417929649353,
-0.044289104640483856,
-0.03242674469947815,
-0.05418996140360832,
-0.044235583394765854,
-0.08542896807193756,
-0.024832291528582573,
-0.023759707808494568,
0.010964075103402138,
-0.038923799991607666,
-0.020170297473669052,
0.08891062438488007,
0.011673507280647755,
-0.055839069187641144,
0.000902296626009047,
0.01729213260114193,
-0.05652989447116852,
0.032701488584280014,
0.015373295173048973,
3.278503335611285e-33,
0.019974183291196823,
-0.0160509143024683,
0.02549169398844242,
-0.03661255165934563,
0.03081931173801422,
-0.047943104058504105,
0.034605491906404495,
-0.03743501752614975,
-0.05970901995897293,
-0.01590687409043312,
-0.033583007752895355,
0.04925455525517464,
-0.15218152105808258,
0.05376678705215454,
-0.06235521286725998,
0.02482418157160282,
-0.00038341418257914484,
0.03197678178548813,
0.024833712726831436,
-0.026611432433128357,
0.13024741411209106,
0.05327509716153145,
-0.005036578979343176,
-0.08940308541059494,
0.00859648734331131,
0.0321904681622982,
0.030311264097690582,
-0.12357167899608612,
-0.05077434703707695,
0.056641723960638046,
-0.11074225604534149,
0.03746936842799187,
-0.032617758959531784,
0.04085586220026016,
-0.10100923478603363,
-0.05301094055175781,
0.008596253581345081,
-0.07737638801336288,
0.0009413656662218273,
-0.06061036139726639,
0.011417513713240623,
0.013624287210404873,
0.020812522619962692,
-0.04414544999599457,
-0.03513304889202118,
-0.03270323574542999,
0.025613730773329735,
-0.0624937005341053,
0.035163167864084244,
0.05929924547672272,
-0.004734664689749479,
0.033971309661865234,
-0.0260387621819973,
0.008164205588400364,
0.07433076947927475,
-0.04091889411211014,
0.08601953834295273,
0.01336115412414074,
0.09186914563179016,
-0.002562867943197489,
-0.0026891606394201517,
-0.012965240515768528,
0.023468896746635437,
0.059221185743808746,
0.05945536866784096,
-0.03088798001408577,
0.03900573402643204,
0.009925886057317257,
0.013446197845041752,
-0.030308395624160767,
-0.042740173637866974,
-0.003571238834410906,
-0.044746674597263336,
0.046473000198602676,
-0.035460468381643295,
-0.05091961845755577,
0.011522832326591015,
-0.018037835136055946,
-0.06259768456220627,
0.03504468500614166,
0.03817486763000488,
0.029621344059705734,
0.00667959451675415,
-0.06519422680139542,
-0.019989902153611183,
0.04508016258478165,
0.058360323309898376,
-0.06915246695280075,
0.011426315642893314,
-0.01232807245105505,
-0.0015046994667500257,
-0.0040740673430264,
-0.042052775621414185,
0.037391114979982376,
0.007310130167752504,
-4.874575973504356e-33,
0.026272155344486237,
0.08052194118499756,
-0.09376714378595352,
0.020697934553027153,
-0.08519184589385986,
-0.07030271738767624,
0.09785435348749161,
0.16686387360095978,
-0.0027057311963289976,
-0.05229802802205086,
-0.04323972389101982,
-0.04631313681602478,
0.031130092218518257,
-0.012957780621945858,
0.08339519053697586,
0.014018415473401546,
0.0012827487662434578,
0.04523860290646553,
0.0120516587048769,
-0.01098730880767107,
-0.001981322420760989,
0.004539701621979475,
-0.1531796157360077,
0.060502491891384125,
-0.010275560431182384,
0.010481752455234528,
-0.058757390826940536,
0.014154870994389057,
0.00577322905883193,
0.0059103816747665405,
-0.03809729218482971,
0.026093559339642525,
-0.018321184441447258,
0.052130259573459625,
-0.12769325077533722,
-0.05632070451974869,
-0.010968461632728577,
-0.0077930837869644165,
-0.01845446042716503,
0.0491703599691391,
0.054925281554460526,
0.04321666806936264,
-0.07364077121019363,
0.06692696362733841,
-0.10295680910348892,
0.00022636345238424838,
-0.05043164640665054,
-0.029200581833720207,
0.05573700740933418,
-0.11055543273687363,
0.01523969043046236,
0.023793956264853477,
-0.07798945158720016,
0.005865416023880243,
-0.0519358329474926,
-0.037622980773448944,
0.03192597255110741,
-0.04598180949687958,
-0.09905870258808136,
-0.024127298966050148,
-0.047920502722263336,
-0.024825090542435646,
0.059390634298324585,
-0.04839097335934639,
0.048529572784900665,
-0.010530740953981876,
0.02851671539247036,
0.07058533281087875,
0.010486027225852013,
-0.08656290173530579,
0.06722569465637207,
-0.013359125703573227,
0.02001590095460415,
0.04233921691775322,
0.052007149904966354,
0.021112652495503426,
-0.035166896879673004,
-0.03786122798919678,
-0.003556963987648487,
0.010595566593110561,
-0.0339970663189888,
-0.03637639060616493,
0.013026908971369267,
0.04995430260896683,
0.041507117450237274,
0.031480010598897934,
0.021663041785359383,
0.009326322004199028,
-0.014864583499729633,
0.0088282385841012,
0.022093622013926506,
0.047142449766397476,
-0.04049478471279144,
0.10572952777147293,
0.024758100509643555,
-4.8008836017743306e-8,
-0.05567013844847679,
-0.04709523916244507,
-0.03879592567682266,
0.0026883448008447886,
-0.08883669227361679,
-0.05436955764889717,
0.016101311892271042,
0.011826478876173496,
-0.05402807518839836,
-0.037953801453113556,
0.021676167845726013,
0.0391765832901001,
-0.05469464883208275,
0.04886338859796524,
0.010822351090610027,
0.03822494298219681,
0.021663857623934746,
0.13907136023044586,
0.020650221034884453,
-0.019607918336987495,
0.0047478629276156425,
0.0181757640093565,
0.026703719049692154,
-0.04577662795782089,
0.02321476861834526,
0.010440442711114883,
-0.02644823119044304,
0.08973260968923569,
0.02740226686000824,
0.0069501763209700584,
-0.0841306522488594,
0.013393372297286987,
-0.08140937983989716,
0.0677608996629715,
0.053991805762052536,
0.061987314373254776,
-0.026306448504328728,
-0.056261152029037476,
-0.05076415836811066,
0.0612821988761425,
0.17196546494960785,
-0.02449803799390793,
-0.0672241598367691,
0.007062616292387247,
0.04999866709113121,
0.06380583345890045,
0.01786285825073719,
-0.10646656900644302,
0.026607202365994453,
0.0021730035077780485,
0.028903497382998466,
-0.021256187930703163,
-0.03483743965625763,
0.01431233249604702,
-0.010121254250407219,
0.029701486229896545,
-0.05535386875271797,
0.01659262925386429,
0.05209663510322571,
0.002930045360699296,
0.043742209672927856,
0.07097204774618149,
0.05691864341497421,
0.036416202783584595
] |
phiyodr/bert-base-finetuned-squad2 | c73e3f22381ce4c230b49844ea7b8c703887385c | 2021-05-20T02:34:19.000Z | [
"pytorch",
"jax",
"bert",
"question-answering",
"en",
"dataset:squad2",
"arxiv:1810.04805",
"arxiv:1806.03822",
"transformers",
"autotrain_compatible"
] | question-answering | false | phiyodr | null | phiyodr/bert-base-finetuned-squad2 | 1,934 | null | transformers | ---
language: en
tags:
- pytorch
- question-answering
datasets:
- squad2
metrics:
- exact
- f1
widget:
- text: "What discipline did Winkelmann create?"
context: "Johann Joachim Winckelmann was a German art historian and archaeologist. He was a pioneering Hellenist who first articulated the difference between Greek, Greco-Roman and Roman art. The prophet and founding hero of modern archaeology, Winckelmann was one of the founders of scientific archaeology and first applied the categories of style on a large, systematic basis to the history of art."
---
# bert-base-finetuned-squad2
## Model description
This model is based on **[bert-base-uncased](https://huggingface.co/bert-base-uncased)** and was finetuned on **[SQuAD2.0](https://rajpurkar.github.io/SQuAD-explorer/)**. The corresponding papers you can found [here (model)](https://arxiv.org/abs/1810.04805) and [here (data)](https://arxiv.org/abs/1806.03822).
## How to use
```python
from transformers.pipelines import pipeline
model_name = "phiyodr/bert-base-finetuned-squad2"
nlp = pipeline('question-answering', model=model_name, tokenizer=model_name)
inputs = {
'question': 'What discipline did Winkelmann create?',
'context': 'Johann Joachim Winckelmann was a German art historian and archaeologist. He was a pioneering Hellenist who first articulated the difference between Greek, Greco-Roman and Roman art. "The prophet and founding hero of modern archaeology", Winckelmann was one of the founders of scientific archaeology and first applied the categories of style on a large, systematic basis to the history of art. '
}
nlp(inputs)
```
## Training procedure
```
{
"base_model": "bert-base-uncased",
"do_lower_case": True,
"learning_rate": 3e-5,
"num_train_epochs": 4,
"max_seq_length": 384,
"doc_stride": 128,
"max_query_length": 64,
"batch_size": 96
}
```
## Eval results
- Data: [dev-v2.0.json](https://rajpurkar.github.io/SQuAD-explorer/dataset/dev-v2.0.json)
- Script: [evaluate-v2.0.py](https://worksheets.codalab.org/rest/bundles/0x6b567e1cf2e041ec80d7098f031c5c9e/contents/blob/) (original script from [here](https://github.com/huggingface/transformers/blob/master/examples/question-answering/README.md))
```
{
"exact": 70.3950138970774,
"f1": 73.90527661873521,
"total": 11873,
"HasAns_exact": 71.4574898785425,
"HasAns_f1": 78.48808186475087,
"HasAns_total": 5928,
"NoAns_exact": 69.33557611438184,
"NoAns_f1": 69.33557611438184,
"NoAns_total": 5945
}
```
| [
-0.15675802528858185,
-0.007989153265953064,
0.0068563721142709255,
-0.0038506819400936365,
0.00802692398428917,
-0.0027652299031615257,
-0.02590803988277912,
0.035925496369600296,
-0.017866581678390503,
-0.03765341266989708,
0.04252910986542702,
-0.033240798860788345,
0.0048211850225925446,
0.04583992809057236,
-0.059935588389635086,
0.023921530693769455,
-0.0431741327047348,
-0.019456729292869568,
-0.04263262078166008,
0.006438800133764744,
0.012425655499100685,
0.014397555962204933,
0.0825905054807663,
-0.06353597342967987,
0.06082708388566971,
-0.0038940482772886753,
-0.01859612576663494,
-0.010510626249015331,
0.045213181525468826,
-0.022020135074853897,
0.0017678828444331884,
0.05812179297208786,
0.02920900471508503,
0.05971319228410721,
0.0193703044205904,
0.09430542588233948,
0.021104734390974045,
0.03426828235387802,
-0.03135788068175316,
0.0636724978685379,
-0.043463632464408875,
0.010632691904902458,
-0.023539703339338303,
0.0005775862955488265,
0.061657752841711044,
0.031172145158052444,
-0.03178694471716881,
0.0023328126408159733,
-0.07369396835565567,
0.027984239161014557,
-0.10668787360191345,
-0.06600906699895859,
0.017633598297834396,
-0.008143874816596508,
0.02477729693055153,
0.04001787677407265,
0.00459829019382596,
-0.030462341383099556,
-0.03692912682890892,
-0.10805952548980713,
-0.02359369955956936,
0.009511779062449932,
-0.04597518965601921,
-0.005141761619597673,
-0.062272194772958755,
0.042692918330430984,
-0.07156078517436981,
0.018117547035217285,
0.018539633601903915,
-0.002802131697535515,
0.014271137304604053,
0.0014050640165805817,
-0.02107389271259308,
-0.012630939483642578,
0.020861756056547165,
-0.015893831849098206,
0.07966557890176773,
0.01665431261062622,
0.009376327507197857,
-0.18792133033275604,
0.03526042401790619,
-0.02057056687772274,
0.04124866798520088,
0.042119722813367844,
0.04512737691402435,
0.03239460662007332,
-0.01366009283810854,
-0.024596475064754486,
0.014330990612506866,
0.013766173273324966,
0.022903213277459145,
-0.0575919933617115,
0.05378972738981247,
0.00024257069162558764,
0.057168155908584595,
0.06701476871967316,
0.0840902328491211,
0.0891069620847702,
-0.0196217093616724,
0.09828853607177734,
0.019032945856451988,
-0.0424313023686409,
0.02536378800868988,
-0.009772497229278088,
0.014385313726961613,
-0.0022899399045854807,
0.008380121551454067,
0.019891854375600815,
0.015739504247903824,
-0.06672535836696625,
0.0010098705533891916,
-0.028563736006617546,
-0.03869127109646797,
-0.03895583376288414,
-0.006087822373956442,
-0.07510261237621307,
-0.029313765466213226,
0.025789624080061913,
0.014604155905544758,
0.032782409340143204,
0.060453061014413834,
-0.013255642727017403,
0.022144658491015434,
0.045012928545475006,
-0.03474411368370056,
0.05631145462393761,
-0.04858490824699402,
4.603346815099437e-33,
0.08881866186857224,
0.035160131752491,
-0.01174646895378828,
0.07139362394809723,
0.00941465049982071,
-0.019209645688533783,
-0.027656804770231247,
-0.0019514901796355844,
-0.03951290622353554,
0.01242747437208891,
-0.07776451110839844,
0.032554417848587036,
-0.06991222500801086,
0.044340405613183975,
-0.01973838172852993,
-0.011693675071001053,
-0.017026009038090706,
0.08137441426515579,
0.043403688818216324,
0.026810508221387863,
0.04451841115951538,
0.048574574291706085,
-0.004738201387226582,
-0.10452321171760559,
0.021853847429156303,
0.07676808536052704,
0.04250134527683258,
-0.03783402219414711,
-0.05494482070207596,
0.05010053515434265,
-0.0736164078116417,
0.031118027865886688,
-0.05052094906568527,
0.02253052033483982,
-0.0040062121115624905,
-0.0025649461895227432,
0.0033665522933006287,
-0.12276056408882141,
-0.006501919124275446,
-0.03318171948194504,
0.013975955545902252,
0.07029429823160172,
0.012793260626494884,
-0.07400069385766983,
-0.04127112403512001,
-0.04581298679113388,
0.016861408948898315,
0.02187860757112503,
0.06922731548547745,
0.020875979214906693,
-0.01122166495770216,
0.030534546822309494,
0.025525733828544617,
-0.01774950698018074,
-0.0029001967050135136,
0.009199233725667,
0.034890566021203995,
0.08370055258274078,
0.02873268909752369,
0.05012109503149986,
0.07135903090238571,
0.07957983016967773,
0.05365967005491257,
0.06762158870697021,
0.034452442079782486,
0.03272256255149841,
-0.0683344379067421,
0.024733083322644234,
0.06505310535430908,
0.047700073570013046,
-0.059055522084236145,
0.013061022385954857,
0.03953808173537254,
0.017757853493094444,
0.01965430937707424,
-0.03631005063652992,
-0.014223366975784302,
-0.0610806979238987,
-0.09733244776725769,
-0.027978235855698586,
-0.11962763220071793,
0.03920149430632591,
-0.10286030173301697,
-0.06066920608282089,
-0.0846676453948021,
-0.013147356919944286,
0.10281437635421753,
-0.05079391598701477,
0.002505019074305892,
-0.0038949346635490656,
-0.022966153919696808,
-0.10575365275144577,
-0.09500986337661743,
0.005507286172360182,
-0.08864204585552216,
-4.457798206606575e-33,
-0.0017648356733843684,
0.003511338494718075,
-0.04217246174812317,
0.017335422337055206,
0.0045176539570093155,
-0.09087129682302475,
-0.00804891623556614,
0.11740516871213913,
0.03371929004788399,
0.008771127089858055,
0.09762540459632874,
0.0034425784833729267,
-0.025814777240157127,
-0.08528224378824234,
0.07774800062179565,
-0.013367535546422005,
-0.041691120713949203,
-0.06406858563423157,
-0.041608862578868866,
0.023128755390644073,
0.0678892433643341,
-0.07568952441215515,
-0.14595654606819153,
0.020809318870306015,
-0.07901936769485474,
0.09904026985168457,
-0.024103248491883278,
0.016473259776830673,
-0.0000808866461738944,
0.03311791643500328,
0.007875066250562668,
-0.07650500535964966,
-0.046015262603759766,
0.040295761078596115,
-0.05234687030315399,
0.05867251008749008,
0.04988694563508034,
-0.002512316219508648,
-0.03554728999733925,
0.01482132263481617,
0.07209204137325287,
0.04392851144075394,
-0.07032226026058197,
0.07407867163419724,
0.012268497608602047,
0.013658217154443264,
-0.07550834864377975,
0.007319561205804348,
-0.017177632078528404,
-0.11936900019645691,
0.049458906054496765,
-0.018319685012102127,
0.00499986344948411,
-0.006644866429269314,
-0.03971642255783081,
-0.027858631685376167,
-0.0122218057513237,
-0.02275151200592518,
-0.05846787616610527,
0.06329890340566635,
0.04064493626356125,
-0.03047429397702217,
-0.06885623186826706,
0.06721539795398712,
-0.025801947340369225,
-0.07263313233852386,
-0.10884024202823639,
0.07091711461544037,
-0.09287737309932709,
0.0073144384659826756,
0.018352584913372993,
-0.025014320388436317,
0.07747587561607361,
0.03153818100690842,
0.004038440994918346,
-0.03375398740172386,
0.0019498399924486876,
-0.0729917362332344,
0.07648327946662903,
-0.012519625946879387,
-0.09954998642206192,
-0.07200112193822861,
0.03048386052250862,
0.11787539720535278,
0.0036619785241782665,
0.057644251734018326,
-0.007306747604161501,
0.12522462010383606,
0.02729911170899868,
-0.05861469358205795,
0.014821456745266914,
-0.04785745218396187,
0.022987771779298782,
0.11679153889417648,
0.010251370258629322,
-6.615321268554908e-8,
-0.022899813950061798,
0.05251128971576691,
-0.002468395745381713,
0.015473157167434692,
-0.09197396039962769,
-0.05851598456501961,
-0.0504215732216835,
-0.005964966025203466,
-0.044189538806676865,
0.02242898941040039,
-0.0207543782889843,
0.07318942248821259,
-0.0752318948507309,
-0.039485327899456024,
-0.01840636506676674,
0.048224374651908875,
0.002782350406050682,
0.01913752593100071,
-0.03997392952442169,
-0.009150905534625053,
0.042213622480630875,
0.020310917869210243,
-0.002177528804168105,
-0.0779193863272667,
-0.016878293827176094,
0.003922671545296907,
-0.09982408583164215,
0.04182463884353638,
-0.04439842328429222,
0.025095269083976746,
-0.015111424960196018,
0.038826875388622284,
-0.030025165528059006,
0.014674860052764416,
0.07619740813970566,
0.0584048256278038,
-0.09077517688274384,
-0.06947042793035507,
0.034614987671375275,
0.02349141240119934,
0.05117521062493324,
-0.0013971553416922688,
-0.046185221523046494,
0.04344472661614418,
0.14959129691123962,
0.0393894724547863,
0.01372590847313404,
-0.0803312435746193,
0.00016828582738526165,
0.0009233746095560491,
0.051881853491067886,
0.012047120369970798,
-0.0465884692966938,
0.02824903465807438,
-0.07664085924625397,
0.01961860992014408,
-0.05483141541481018,
0.04567110538482666,
0.046081360429525375,
0.012275499291718006,
0.06745537370443344,
0.030869074165821075,
0.021305417641997337,
0.032174672931432724
] |
microsoft/xlm-align-base | 3e2a40ea5f9c75353ad2769bd74f7cb425fce671 | 2021-08-04T15:23:10.000Z | [
"pytorch",
"xlm-roberta",
"fill-mask",
"transformers",
"autotrain_compatible"
] | fill-mask | false | microsoft | null | microsoft/xlm-align-base | 1,932 | 3 | transformers | # XLM-Align
**XLM-Align** (ACL 2021, [paper](https://aclanthology.org/2021.acl-long.265/), [repo](https://github.com/CZWin32768/XLM-Align), [model](https://huggingface.co/microsoft/xlm-align-base)) Improving Pretrained Cross-Lingual Language Models via Self-Labeled Word Alignment
XLM-Align is a pretrained cross-lingual language model that supports 94 languages. See details in our [paper](https://aclanthology.org/2021.acl-long.265/).
## Example
```
model = AutoModel.from_pretrained("microsoft/xlm-align-base")
```
## Evaluation Results
XTREME cross-lingual understanding tasks:
| Model | POS | NER | XQuAD | MLQA | TyDiQA | XNLI | PAWS-X | Avg |
|:----:|:----:|:----:|:----:|:-----:|:----:|:-----:|:----:|:----:|
| XLM-R_base | 75.6 | 61.8 | 71.9 / 56.4 | 65.1 / 47.2 | 55.4 / 38.3 | 75.0 | 84.9 | 66.4 |
| XLM-Align | **76.0** | **63.7** | **74.7 / 59.0** | **68.1 / 49.8** | **62.1 / 44.8** | **76.2** | **86.8** | **68.9** |
## MD5
```
b9d214025837250ede2f69c9385f812c config.json
6005db708eb4bab5b85fa3976b9db85b pytorch_model.bin
bf25eb5120ad92ef5c7d8596b5dc4046 sentencepiece.bpe.model
eedbd60a7268b9fc45981b849664f747 tokenizer.json
```
## About
Contact: chizewen\@outlook.com
BibTeX:
```
@inproceedings{xlmalign,
title = "Improving Pretrained Cross-Lingual Language Models via Self-Labeled Word Alignment",
author={Zewen Chi and Li Dong and Bo Zheng and Shaohan Huang and Xian-Ling Mao and Heyan Huang and Furu Wei},
booktitle = "Proceedings of the 59th Annual Meeting of the Association for Computational Linguistics and the 11th International Joint Conference on Natural Language Processing (Volume 1: Long Papers)",
month = aug,
year = "2021",
address = "Online",
publisher = "Association for Computational Linguistics",
url = "https://aclanthology.org/2021.acl-long.265",
doi = "10.18653/v1/2021.acl-long.265",
pages = "3418--3430",}
``` | [
-0.0632518082857132,
-0.06782623380422592,
-0.0007664964650757611,
-0.027999838814139366,
-0.03313056752085686,
0.0933753103017807,
-0.09121180325746536,
0.007678649388253689,
0.021994946524500847,
-0.01039494201540947,
0.08428443223237991,
-0.1246107965707779,
0.03283899649977684,
0.008376583456993103,
0.012167949229478836,
0.03427469730377197,
-0.011022219434380531,
0.022947296500205994,
-0.07371079176664352,
-0.003007546765729785,
-0.01973199099302292,
0.04044738784432411,
0.011277327314019203,
0.00999443233013153,
0.03833505138754845,
-0.03519671410322189,
-0.00610241899266839,
-0.0063018472865223885,
0.04649472236633301,
-0.01970531791448593,
-0.00006135204603197053,
0.06357643753290176,
0.02916223369538784,
0.12414403259754181,
-0.02571840025484562,
0.01000390574336052,
-0.06265255063772202,
-0.11730753630399704,
0.05107555910944939,
0.03844476118683815,
-0.021463781595230103,
-0.020592283457517624,
0.050059471279382706,
-0.05850685387849808,
0.07991344481706619,
0.03954658657312393,
-0.023611396551132202,
0.0029800371266901493,
-0.060097090899944305,
0.06119256839156151,
-0.10200805962085724,
0.029063016176223755,
0.06204071640968323,
0.05467783287167549,
-0.03499816730618477,
-0.027244411408901215,
-0.012861309573054314,
-0.017689725384116173,
-0.0074715400114655495,
-0.022205304354429245,
-0.001029311795718968,
0.0007131677703000605,
-0.03774973750114441,
0.04421841353178024,
-0.0798204243183136,
0.017399471253156662,
-0.0012589067919179797,
0.024293169379234314,
-0.026538515463471413,
0.05921357125043869,
-0.07319127768278122,
0.003990841098129749,
0.005928033962845802,
0.013820173218846321,
0.05726794898509979,
0.060856591910123825,
0.028707129880785942,
-0.03983524441719055,
0.022630417719483376,
-0.08939247578382492,
-0.024018768221139908,
0.10485615581274033,
0.061685338616371155,
0.006906982511281967,
0.05641920492053032,
-0.07445511966943741,
0.005536532029509544,
0.045825451612472534,
0.01749080792069435,
0.004573839716613293,
0.01824350282549858,
-0.08364962041378021,
-0.0215789582580328,
0.013908686116337776,
0.013882162980735302,
0.004087530076503754,
0.09696964174509048,
0.05481451377272606,
-0.04904842749238014,
-0.04847189784049988,
0.04036538675427437,
0.08819501847028732,
0.015411500819027424,
-0.0251168180257082,
-0.046467140316963196,
-0.10173285752534866,
0.024094508960843086,
0.047297000885009766,
0.03127063810825348,
-0.1099655032157898,
0.0460234172642231,
-0.07225417345762253,
-0.06156269460916519,
-0.006663707084953785,
0.00021453379304148257,
0.011445640586316586,
0.013436289504170418,
-0.1202765554189682,
0.09202674776315689,
-0.033523447811603546,
-0.08774378895759583,
-0.04747476801276207,
0.03478872776031494,
-0.013802963308990002,
0.02254231460392475,
-0.01876804791390896,
-0.03714754432439804,
1.4969092201546486e-33,
0.01046723686158657,
0.06194595992565155,
-0.02378331869840622,
-0.0010881343623623252,
-0.0012615800369530916,
-0.03323995694518089,
-0.026956019923090935,
0.023744838312268257,
0.019949674606323242,
0.044507261365652084,
-0.07648829370737076,
0.12301934510469437,
-0.0504540354013443,
0.026961004361510277,
-0.051408857107162476,
-0.022338373586535454,
-0.04236732795834541,
0.033813171088695526,
-0.08913576602935791,
0.058408528566360474,
0.1324804127216339,
0.04862260818481445,
0.017254957929253578,
-0.05237485468387604,
-0.011235385201871395,
0.08082946389913559,
0.09476011246442795,
-0.07269900292158127,
-0.061499886214733124,
0.024480465799570084,
-0.09880296885967255,
-0.031256042420864105,
0.024961726740002632,
0.01445705909281969,
0.008969171904027462,
0.011191670782864094,
-0.03807478025555611,
-0.026354487985372543,
-0.002970292931422591,
-0.02159752883017063,
0.007393369451165199,
0.057555750012397766,
0.09264494478702545,
-0.06139489263296127,
-0.0013484236551448703,
-0.020742470398545265,
-0.01462393905967474,
-0.014989761635661125,
0.04473981261253357,
-0.029892485588788986,
0.05094392970204353,
0.0068491739220917225,
-0.06834667921066284,
-0.007479156367480755,
0.009965712204575539,
0.08361544460058212,
-0.023357395082712173,
0.10720473527908325,
-0.021391650661826134,
0.040410127490758896,
-0.01002234686166048,
-0.032575108110904694,
0.025134842842817307,
0.03595428913831711,
0.0498846210539341,
-0.003196402220055461,
0.02174181491136551,
-0.052544042468070984,
0.05387495458126068,
0.006410347297787666,
-0.02169802598655224,
-0.08016840368509293,
-0.030374236404895782,
0.011058112606406212,
0.1277034431695938,
-0.009625938721001148,
0.018506551161408424,
-0.03703445568680763,
0.046835336834192276,
-0.013221120461821556,
-0.0017802934162318707,
0.03462322801351547,
0.006139774806797504,
-0.023111019283533096,
-0.09458054602146149,
-0.07766538858413696,
0.08970458060503006,
-0.0157345999032259,
0.013042104430496693,
0.01474792417138815,
0.07094327360391617,
0.010626361705362797,
0.045477960258722305,
0.017230812460184097,
0.015621693804860115,
-3.9796849811928104e-33,
0.042565856128931046,
-0.017035042867064476,
0.0087282108142972,
0.02086649276316166,
-0.03628858923912048,
-0.021044116467237473,
0.10852927714586258,
0.11584452539682388,
-0.044468216598033905,
-0.029853038489818573,
0.07539267838001251,
-0.04640941694378853,
0.009629222564399242,
0.0967743918299675,
0.008963124826550484,
-0.010102926753461361,
0.019459404051303864,
0.028928648680448532,
0.0022218686062842607,
0.056201837956905365,
-0.03422631323337555,
0.027184177190065384,
-0.07477721571922302,
0.04578310623764992,
0.054043445736169815,
0.03142169862985611,
-0.0014707983937114477,
-0.006062494590878487,
-0.0158246923238039,
0.023958217352628708,
-0.06425149738788605,
0.030822930857539177,
0.003382219234481454,
-0.008664964698255062,
-0.07200764864683151,
0.023859022185206413,
-0.022021247074007988,
0.032101720571517944,
-0.022070102393627167,
0.027178604155778885,
0.06430187821388245,
-0.02297579124569893,
-0.11046727001667023,
0.0034681393299251795,
0.05824745446443558,
0.023905832320451736,
-0.12704387307167053,
-0.0601845383644104,
-0.017600134015083313,
0.009430190548300743,
-0.017468446865677834,
0.012458118610084057,
-0.014200855977833271,
-0.04610932618379593,
-0.021406371146440506,
-0.05164198949933052,
-0.0751243457198143,
-0.12162034958600998,
-0.11787042766809464,
-0.05662669986486435,
-0.05811377242207527,
0.006580664776265621,
0.08695808798074722,
-0.05480083450675011,
0.03433622419834137,
-0.010473186150193214,
-0.026798909530043602,
-0.05551207810640335,
-0.0005459601525217295,
-0.04731143265962601,
0.08375056087970734,
-0.005169552750885487,
-0.07425560057163239,
0.0036263824440538883,
-0.015755582600831985,
-0.04917401075363159,
0.00229081092402339,
-0.0989592969417572,
-0.0139663340523839,
-0.09538930654525757,
-0.0030642682686448097,
-0.005376489832997322,
0.02700704336166382,
0.09297464787960052,
-0.07058412581682205,
0.02799673192203045,
0.04576904699206352,
0.08747372031211853,
-0.04787577688694,
0.03937622159719467,
-0.0009711135644465685,
-0.0056893713772296906,
-0.021582119166851044,
0.07690267264842987,
-0.021098151803016663,
-4.822122434688936e-8,
-0.11360103636980057,
0.07705295085906982,
0.0022865664213895798,
0.032277047634124756,
-0.07222768664360046,
-0.061737582087516785,
-0.09253671765327454,
0.003676759544759989,
0.02312561683356762,
0.038487426936626434,
-0.03450245410203934,
0.023399358615279198,
-0.06909266114234924,
-0.00968639925122261,
-0.05244211480021477,
-0.0023329087998718023,
-0.010805192403495312,
0.04316100478172302,
-0.008756224066019058,
0.024974465370178223,
-0.02579388953745365,
0.02461952529847622,
0.012706299312412739,
-0.013989293947815895,
0.056926414370536804,
-0.06534840166568756,
-0.06327886879444122,
0.050853706896305084,
0.054320648312568665,
-0.07318115234375,
-0.03741944953799248,
0.02252751588821411,
-0.04385488107800484,
0.019816774874925613,
-0.04683712124824524,
0.033216338604688644,
0.018428275361657143,
-0.01429264061152935,
0.07291267812252045,
0.03467927500605583,
0.020076408982276917,
0.05637533962726593,
-0.15794329345226288,
-0.011809092946350574,
0.0838957205414772,
-0.03647392615675926,
-0.06058746203780174,
-0.128175750374794,
0.004636868834495544,
-0.06692519038915634,
0.043568216264247894,
0.020820515230298042,
0.050444118678569794,
-0.009965435601770878,
0.040059905499219894,
0.04299316555261612,
-0.03547806292772293,
-0.006200444884598255,
0.09763220697641373,
0.04835790395736694,
0.008412822149693966,
-0.03182760253548622,
0.024238750338554382,
-0.009561983868479729
] |
Alireza1044/albert-base-v2-sst2 | e406771b99e1913921a68fbb95d121b582d1ecb7 | 2021-07-26T14:02:35.000Z | [
"pytorch",
"tensorboard",
"albert",
"text-classification",
"en",
"dataset:glue",
"transformers",
"generated_from_trainer",
"license:apache-2.0"
] | text-classification | false | Alireza1044 | null | Alireza1044/albert-base-v2-sst2 | 1,930 | null | transformers | ---
language:
- en
license: apache-2.0
tags:
- generated_from_trainer
datasets:
- glue
metrics:
- accuracy
model_index:
- name: sst2
results:
- task:
name: Text Classification
type: text-classification
dataset:
name: GLUE SST2
type: glue
args: sst2
metric:
name: Accuracy
type: accuracy
value: 0.9231651376146789
---
<!-- This model card has been generated automatically according to the information the Trainer had access to. You
should probably proofread and complete it, then remove this comment. -->
# sst2
This model is a fine-tuned version of [albert-base-v2](https://huggingface.co/albert-base-v2) on the GLUE SST2 dataset.
It achieves the following results on the evaluation set:
- Loss: 0.3808
- Accuracy: 0.9232
## Model description
More information needed
## Intended uses & limitations
More information needed
## Training and evaluation data
More information needed
## Training procedure
### Training hyperparameters
The following hyperparameters were used during training:
- learning_rate: 1e-05
- train_batch_size: 32
- eval_batch_size: 8
- seed: 42
- optimizer: Adam with betas=(0.9,0.999) and epsilon=1e-08
- lr_scheduler_type: linear
- num_epochs: 4.0
### Training results
### Framework versions
- Transformers 4.9.0
- Pytorch 1.9.0+cu102
- Datasets 1.10.2
- Tokenizers 0.10.3
| [
-0.04976548254489899,
-0.035171810537576675,
-0.03869033232331276,
0.03656264394521713,
-0.01340805646032095,
0.05725715681910515,
-0.03857796639204025,
0.06698229908943176,
-0.048188164830207825,
-0.15046276152133942,
0.04119427129626274,
-0.05502653494477272,
-0.005924818105995655,
-0.044307127594947815,
-0.05571435019373894,
0.01833247020840645,
0.07240255922079086,
0.00788190308958292,
-0.03541015088558197,
0.00822239089757204,
0.019258132204413414,
0.04960697889328003,
-0.0014779913472011685,
-0.017550688236951828,
-0.00040446687489748,
0.0738755390048027,
-0.050874050706624985,
0.030563825741410255,
0.0299928467720747,
-0.03509048745036125,
0.033414795994758606,
-0.0232083760201931,
-0.00031540743657387793,
0.05116649344563484,
0.019359959289431572,
0.020792458206415176,
-0.020871056243777275,
-0.05046071857213974,
-0.00721097132191062,
-0.033395715057849884,
0.06074301898479462,
-0.06081409379839897,
-0.020592937245965004,
0.03413968160748482,
0.0040222397074103355,
0.018877815455198288,
-0.031835220754146576,
-0.08953789621591568,
-0.06142782047390938,
0.003190451767295599,
-0.12680816650390625,
-0.10140618681907654,
0.13192962110042572,
-0.02554706484079361,
-0.0048806332051754,
0.045835498720407486,
-0.038779888302087784,
0.012828762643039227,
-0.014030697755515575,
-0.012833775021135807,
0.11017879098653793,
-0.030651720240712166,
-0.07899057865142822,
-0.028353530913591385,
-0.061986543238162994,
-0.06586196273565292,
0.02044512704014778,
0.006157461553812027,
0.06649547070264816,
-0.02323424257338047,
0.014137537218630314,
0.05180004611611366,
-0.042962536215782166,
0.05682378634810448,
0.049305979162454605,
0.053461138159036636,
-0.04526013135910034,
-0.008210365660488605,
0.07034794241189957,
-0.07002325356006622,
-0.043202340602874756,
-0.06901540607213974,
-0.010680237784981728,
0.0023058007936924696,
0.016014231368899345,
0.020534927025437355,
0.07386530935764313,
0.045175716280937195,
-0.005876597482711077,
0.03555447980761528,
0.010700496844947338,
-0.0279876459389925,
0.011429143138229847,
0.008090241812169552,
-0.00298680760897696,
0.06435529887676239,
-0.0010767487110570073,
0.05350654199719429,
-0.10839612782001495,
0.10550849884748459,
-0.06389623135328293,
0.09483323246240616,
0.009226796217262745,
-0.006367845460772514,
0.03721565008163452,
-0.025012629106640816,
-0.06200648844242096,
0.0699382945895195,
0.061444707214832306,
-0.08656235784292221,
0.08554939180612564,
-0.07321793586015701,
-0.07180958986282349,
-0.007821735925972462,
0.0021508419886231422,
0.10032220184803009,
-0.030108850449323654,
0.01079384982585907,
-0.05313419550657272,
0.03169725090265274,
0.0026557340752333403,
0.010891136713325977,
-0.020701538771390915,
0.03722571209073067,
-0.0367274209856987,
-0.07047325372695923,
-0.11285020411014557,
4.88250688695256e-33,
0.04194805026054382,
0.06704667210578918,
-0.0054624006152153015,
0.020804185420274734,
-0.006753104273229837,
-0.09060531854629517,
-0.0459650419652462,
-0.0009964699856936932,
-0.007480766624212265,
0.004322746302932501,
-0.03812188655138016,
-0.0254171434789896,
-0.02707243524491787,
0.02922302857041359,
-0.0068710544146597385,
-0.049200475215911865,
-0.027704400941729546,
0.08624451607465744,
0.06927202641963959,
0.03422146663069725,
0.09836624562740326,
0.05553041771054268,
0.0028473760467022657,
-0.06933754682540894,
-0.02611500956118107,
0.0781790092587471,
0.03201155737042427,
-0.0634181872010231,
0.007667336612939835,
0.0427488312125206,
-0.031230853870511055,
-0.007227291818708181,
-0.0148417167365551,
-0.02751825377345085,
0.03265601769089699,
-0.0524129681289196,
0.026573412120342255,
-0.030011214315891266,
-0.07407073676586151,
-0.07376284897327423,
0.08732981234788895,
0.025983717292547226,
0.04145895689725876,
-0.026058413088321686,
0.0019596379715949297,
-0.04510976001620293,
0.07482600212097168,
0.04324718564748764,
0.08157317340373993,
0.04407354071736336,
-0.04543909803032875,
-0.002682463265955448,
0.05634647235274315,
0.0061344485729932785,
-0.04552251845598221,
-0.007149169221520424,
0.03430893272161484,
0.14412212371826172,
0.021085314452648163,
0.02161407843232155,
-0.03814991936087608,
-0.0019326219335198402,
-0.021264631301164627,
0.05489833652973175,
-0.004931477829813957,
0.012437853962182999,
-0.03795592486858368,
-0.06367519497871399,
0.0103371636942029,
0.05020196735858917,
-0.03532540798187256,
0.020270822569727898,
0.001260412740521133,
-0.027048859745264053,
0.04337290674448013,
-0.09521887451410294,
-0.004934240132570267,
0.010129706934094429,
-0.0397324338555336,
-0.010525687597692013,
-0.053454913198947906,
0.06616005301475525,
0.044904351234436035,
-0.1276387721300125,
-0.07440903782844543,
-0.0652724876999855,
-0.021370114758610725,
-0.01980392076075077,
-0.019573861733078957,
0.051355134695768356,
0.005742654670029879,
0.047223418951034546,
-0.04796883836388588,
0.03132034093141556,
-0.04061974957585335,
-5.6328554204671505e-33,
-0.024707933887839317,
-0.016431156545877457,
-0.012362447567284107,
0.07187610864639282,
0.020780907943844795,
0.03321241959929466,
-0.024594569578766823,
0.10013756155967712,
-0.05837104097008705,
-0.006640822160989046,
0.12634265422821045,
0.015725117176771164,
0.0036142345052212477,
-0.04234573617577553,
0.006872416008263826,
-0.004855831619352102,
-0.038193024694919586,
-0.05379902198910713,
0.017131924629211426,
0.02267402596771717,
0.07189702242612839,
0.13608628511428833,
-0.038663506507873535,
0.06858357042074203,
0.025882376357913017,
0.03807176277041435,
0.029576463624835014,
0.08769042044878006,
0.04254820570349693,
-0.0022391704842448235,
0.03745146468281746,
0.0015500037698075175,
-0.03834502771496773,
0.02583247609436512,
-0.06693612039089203,
0.05709055811166763,
0.0262380950152874,
0.010886150412261486,
-0.004379093647003174,
0.0890100970864296,
0.012524821795523167,
0.043895427137613297,
-0.12790469825267792,
0.0899486392736435,
0.015803266316652298,
-0.03229300305247307,
0.04871460795402527,
-0.023719538003206253,
0.05135847255587578,
-0.05999956279993057,
0.015005175024271011,
-0.06457974761724472,
-0.07982506603002548,
-0.029470117762684822,
-0.010447337292134762,
0.012342573143541813,
-0.02485089935362339,
-0.023055532947182655,
-0.09579799324274063,
0.004379873629659414,
-0.0117366723716259,
0.007031630724668503,
0.016883106902241707,
-0.024591054767370224,
0.004317052662372589,
-0.05065113306045532,
-0.07909667491912842,
0.010204944759607315,
-0.030306439846754074,
0.01758723147213459,
-0.022752048447728157,
0.057825297117233276,
0.011100390926003456,
0.0015415854286402464,
-0.0001504304527770728,
-0.02474389784038067,
0.023809975013136864,
0.04330018162727356,
-0.02760925702750683,
-0.08087483048439026,
-0.1084718331694603,
0.02999001555144787,
0.04730040207505226,
0.04151730611920357,
0.12417080253362656,
0.03634925186634064,
0.063742496073246,
0.10088633000850677,
-0.026692980900406837,
0.012251373380422592,
-0.022185729816555977,
0.04978880286216736,
-0.0012472440721467137,
0.14881078898906708,
-0.024969108402729034,
-5.7324303526229414e-8,
-0.004941237159073353,
0.0029402486979961395,
-0.09681083261966705,
0.07536230981349945,
0.0010198716772720218,
-0.04755023494362831,
-0.0340721569955349,
0.015084724873304367,
-0.07435502856969833,
-0.006332636810839176,
-0.024243127554655075,
-0.013655534945428371,
-0.10486704856157303,
-0.004860997200012207,
0.06760791689157486,
-0.050518304109573364,
-0.05339909344911575,
0.0828300267457962,
-0.0916074588894844,
-0.06909751147031784,
0.05266689509153366,
-0.09026147425174713,
0.09376668930053711,
-0.04597898945212364,
-0.004114965442568064,
-0.03750833123922348,
-0.06153982877731323,
0.07877545058727264,
-0.025250334292650223,
0.002976176096126437,
0.03865274041891098,
0.013800948858261108,
-0.03303312510251999,
-0.0037165204994380474,
0.013683891855180264,
0.037649303674697876,
-0.017615001648664474,
0.000544263340998441,
0.044459108263254166,
0.09559067338705063,
-0.02547413669526577,
0.05198870599269867,
-0.12248587608337402,
0.04008982330560684,
0.055551301687955856,
0.008443113416433334,
-0.01233852468430996,
-0.07634786516427994,
0.01259894110262394,
0.0044531309977173805,
0.025928683578968048,
-0.011461395770311356,
-0.08790944516658783,
-0.01188565231859684,
0.030311668291687965,
0.03148530423641205,
-0.013182112015783787,
-0.03767717629671097,
-0.007778484839946032,
-0.011304008774459362,
0.034980010241270065,
-0.07613453269004822,
-0.00905181746929884,
0.027978448197245598
] |
google/bert_uncased_L-2_H-256_A-4 | 4e937a8675e5afd9a4836735c186ec01695bc3ea | 2021-05-19T17:28:46.000Z | [
"pytorch",
"jax",
"bert",
"arxiv:1908.08962",
"transformers",
"license:apache-2.0"
] | null | false | google | null | google/bert_uncased_L-2_H-256_A-4 | 1,928 | 1 | transformers | ---
thumbnail: https://huggingface.co/front/thumbnails/google.png
license: apache-2.0
---
BERT Miniatures
===
This is the set of 24 BERT models referenced in [Well-Read Students Learn Better: On the Importance of Pre-training Compact Models](https://arxiv.org/abs/1908.08962) (English only, uncased, trained with WordPiece masking).
We have shown that the standard BERT recipe (including model architecture and training objective) is effective on a wide range of model sizes, beyond BERT-Base and BERT-Large. The smaller BERT models are intended for environments with restricted computational resources. They can be fine-tuned in the same manner as the original BERT models. However, they are most effective in the context of knowledge distillation, where the fine-tuning labels are produced by a larger and more accurate teacher.
Our goal is to enable research in institutions with fewer computational resources and encourage the community to seek directions of innovation alternative to increasing model capacity.
You can download the 24 BERT miniatures either from the [official BERT Github page](https://github.com/google-research/bert/), or via HuggingFace from the links below:
| |H=128|H=256|H=512|H=768|
|---|:---:|:---:|:---:|:---:|
| **L=2** |[**2/128 (BERT-Tiny)**][2_128]|[2/256][2_256]|[2/512][2_512]|[2/768][2_768]|
| **L=4** |[4/128][4_128]|[**4/256 (BERT-Mini)**][4_256]|[**4/512 (BERT-Small)**][4_512]|[4/768][4_768]|
| **L=6** |[6/128][6_128]|[6/256][6_256]|[6/512][6_512]|[6/768][6_768]|
| **L=8** |[8/128][8_128]|[8/256][8_256]|[**8/512 (BERT-Medium)**][8_512]|[8/768][8_768]|
| **L=10** |[10/128][10_128]|[10/256][10_256]|[10/512][10_512]|[10/768][10_768]|
| **L=12** |[12/128][12_128]|[12/256][12_256]|[12/512][12_512]|[**12/768 (BERT-Base)**][12_768]|
Note that the BERT-Base model in this release is included for completeness only; it was re-trained under the same regime as the original model.
Here are the corresponding GLUE scores on the test set:
|Model|Score|CoLA|SST-2|MRPC|STS-B|QQP|MNLI-m|MNLI-mm|QNLI(v2)|RTE|WNLI|AX|
|---|:---:|:---:|:---:|:---:|:---:|:---:|:---:|:---:|:---:|:---:|:---:|:---:|
|BERT-Tiny|64.2|0.0|83.2|81.1/71.1|74.3/73.6|62.2/83.4|70.2|70.3|81.5|57.2|62.3|21.0|
|BERT-Mini|65.8|0.0|85.9|81.1/71.8|75.4/73.3|66.4/86.2|74.8|74.3|84.1|57.9|62.3|26.1|
|BERT-Small|71.2|27.8|89.7|83.4/76.2|78.8/77.0|68.1/87.0|77.6|77.0|86.4|61.8|62.3|28.6|
|BERT-Medium|73.5|38.0|89.6|86.6/81.6|80.4/78.4|69.6/87.9|80.0|79.1|87.7|62.2|62.3|30.5|
For each task, we selected the best fine-tuning hyperparameters from the lists below, and trained for 4 epochs:
- batch sizes: 8, 16, 32, 64, 128
- learning rates: 3e-4, 1e-4, 5e-5, 3e-5
If you use these models, please cite the following paper:
```
@article{turc2019,
title={Well-Read Students Learn Better: On the Importance of Pre-training Compact Models},
author={Turc, Iulia and Chang, Ming-Wei and Lee, Kenton and Toutanova, Kristina},
journal={arXiv preprint arXiv:1908.08962v2 },
year={2019}
}
```
[2_128]: https://huggingface.co/google/bert_uncased_L-2_H-128_A-2
[2_256]: https://huggingface.co/google/bert_uncased_L-2_H-256_A-4
[2_512]: https://huggingface.co/google/bert_uncased_L-2_H-512_A-8
[2_768]: https://huggingface.co/google/bert_uncased_L-2_H-768_A-12
[4_128]: https://huggingface.co/google/bert_uncased_L-4_H-128_A-2
[4_256]: https://huggingface.co/google/bert_uncased_L-4_H-256_A-4
[4_512]: https://huggingface.co/google/bert_uncased_L-4_H-512_A-8
[4_768]: https://huggingface.co/google/bert_uncased_L-4_H-768_A-12
[6_128]: https://huggingface.co/google/bert_uncased_L-6_H-128_A-2
[6_256]: https://huggingface.co/google/bert_uncased_L-6_H-256_A-4
[6_512]: https://huggingface.co/google/bert_uncased_L-6_H-512_A-8
[6_768]: https://huggingface.co/google/bert_uncased_L-6_H-768_A-12
[8_128]: https://huggingface.co/google/bert_uncased_L-8_H-128_A-2
[8_256]: https://huggingface.co/google/bert_uncased_L-8_H-256_A-4
[8_512]: https://huggingface.co/google/bert_uncased_L-8_H-512_A-8
[8_768]: https://huggingface.co/google/bert_uncased_L-8_H-768_A-12
[10_128]: https://huggingface.co/google/bert_uncased_L-10_H-128_A-2
[10_256]: https://huggingface.co/google/bert_uncased_L-10_H-256_A-4
[10_512]: https://huggingface.co/google/bert_uncased_L-10_H-512_A-8
[10_768]: https://huggingface.co/google/bert_uncased_L-10_H-768_A-12
[12_128]: https://huggingface.co/google/bert_uncased_L-12_H-128_A-2
[12_256]: https://huggingface.co/google/bert_uncased_L-12_H-256_A-4
[12_512]: https://huggingface.co/google/bert_uncased_L-12_H-512_A-8
[12_768]: https://huggingface.co/google/bert_uncased_L-12_H-768_A-12
| [
-0.02777470275759697,
-0.02693094126880169,
0.07438826560974121,
0.03228488564491272,
-0.0023304771166294813,
0.018128493800759315,
-0.06253628432750702,
0.0994548574090004,
-0.014644814655184746,
0.018868697807192802,
-0.015814494341611862,
0.03585591912269592,
0.03645862638950348,
0.04551365599036217,
-0.014007769525051117,
0.02179890125989914,
0.07545263320207596,
0.024630775675177574,
-0.08102796226739883,
-0.038678135722875595,
0.04442288726568222,
0.004127463325858116,
0.035637278109788895,
-0.06602323800325394,
-0.0036878888495266438,
-0.04231955111026764,
-0.10835908353328705,
-0.10179445147514343,
0.1127767413854599,
0.017838995903730392,
0.01322801411151886,
-0.0231491569429636,
0.060954611748456955,
0.10242877900600433,
0.0375167578458786,
0.07160431146621704,
-0.007885153405368328,
0.06596683710813522,
0.08308044821023941,
0.037167150527238846,
-0.012698134407401085,
0.05730978772044182,
-0.046946585178375244,
-0.020251978188753128,
0.08908210694789886,
-0.059475671499967575,
-0.03805408999323845,
-0.05272062495350838,
-0.04246129095554352,
-0.06516197323799133,
-0.08722401410341263,
-0.0465037040412426,
-0.00350557011552155,
-0.006868511438369751,
-0.012093286029994488,
-0.017656998708844185,
-0.018602291122078896,
-0.08509580790996552,
-0.048703644424676895,
-0.05522743612527847,
-0.1006460189819336,
-0.05546271428465843,
-0.03855401650071144,
-0.02299017831683159,
-0.08375518023967743,
0.010514002293348312,
-0.0332985445857048,
0.020559493452310562,
0.02245338261127472,
0.017550311982631683,
0.02086251601576805,
0.07695921510457993,
-0.002593731041997671,
0.04768828675150871,
0.0177034679800272,
-0.08130199462175369,
0.08254873752593994,
0.01259934064000845,
0.05082662031054497,
-0.056801896542310715,
0.003977705724537373,
-0.011792338453233242,
0.061928100883960724,
-0.027844129130244255,
0.03977213054895401,
-0.01979219727218151,
0.050365421921014786,
-0.03929493576288223,
0.0031530733685940504,
-0.041712965816259384,
-0.025899091735482216,
-0.02879168465733528,
0.0234839990735054,
0.01508942898362875,
0.041859906166791916,
-0.013815062120556831,
0.07762707024812698,
-0.06824886798858643,
-0.035266585648059845,
0.06303618848323822,
0.08460132032632828,
0.05870901793241501,
0.11230025440454483,
-0.0903414711356163,
0.07434411346912384,
0.05187731981277466,
0.025597769767045975,
0.017762847244739532,
0.06019540876150131,
-0.07116957008838654,
0.025501219555735588,
0.0264898668974638,
-0.03993377089500427,
-0.02484058029949665,
0.033353839069604874,
-0.04111992195248604,
-0.012459754012525082,
-0.032413944602012634,
0.04432254657149315,
0.08561859279870987,
0.0311464574187994,
0.010137348435819149,
0.009034326300024986,
-0.013844281435012817,
-0.037362899631261826,
0.022949982434511185,
-0.04159504920244217,
3.0798436882963647e-33,
0.010033472441136837,
0.08980696648359299,
-0.015826981514692307,
0.0021228354889899492,
0.04828347638249397,
-0.012724562548100948,
0.07859385013580322,
0.013289345428347588,
-0.04710506275296211,
0.0008750183042138815,
-0.024205293506383896,
0.040203407406806946,
-0.08776650577783585,
0.1084313839673996,
0.05108625441789627,
-0.0076477923430502415,
-0.03032587841153145,
0.09285354614257812,
0.04229235649108887,
0.02342383936047554,
0.012891994789242744,
-0.03050696663558483,
0.021354084834456444,
-0.08490459620952606,
-0.04626283422112465,
-0.004968647845089436,
0.06569510698318481,
0.006347084417939186,
-0.05621005594730377,
0.04938972741365433,
-0.09828261286020279,
0.04791073501110077,
0.005325495731085539,
0.0073667350225150585,
-0.009293892420828342,
-0.030588563531637192,
-0.025204559788107872,
-0.03599413484334946,
0.06201314181089401,
-0.055159613490104675,
0.015916872769594193,
0.08668506890535355,
0.01913357712328434,
-0.03226336091756821,
0.019701041281223297,
0.016111237928271294,
0.07878092676401138,
0.027088068425655365,
-0.03437655791640282,
-0.04213705286383629,
0.038557808846235275,
0.018548857420682907,
-0.09642824530601501,
-0.02115079015493393,
0.014828594401478767,
-0.014169528149068356,
0.052391670644283295,
-0.021084407344460487,
0.018860751762986183,
0.0188959501683712,
-0.018108483403921127,
-0.017935508862137794,
-0.0007771972450427711,
0.0875239372253418,
0.05831224471330643,
-0.01666453666985035,
-0.03579762578010559,
0.019875947386026382,
-0.03154779225587845,
0.024714933708310127,
-0.04408795386552811,
-0.017733389511704445,
0.031613849103450775,
-0.034551091492176056,
0.019006161019206047,
-0.09389360249042511,
0.0749051496386528,
-0.06782030314207077,
-0.060423046350479126,
-0.0027907630428671837,
0.036781832575798035,
0.03104851022362709,
-0.06610022485256195,
-0.07133632153272629,
-0.09378468245267868,
-0.05997026711702347,
0.06689010560512543,
-0.027257995679974556,
0.019673382863402367,
0.02110666036605835,
0.0042736465111374855,
-0.07312818616628647,
0.004901031032204628,
0.009528765454888344,
-0.08911892771720886,
-2.745649909673619e-33,
0.0021529693622142076,
0.03855104371905327,
-0.10308390855789185,
0.050320789217948914,
-0.04681287705898285,
-0.04624652862548828,
0.04134273901581764,
0.15953823924064636,
-0.05114345625042915,
-0.06880908459424973,
-0.03467176482081413,
-0.01697215437889099,
-0.02391764335334301,
-0.08151818066835403,
-0.013180517591536045,
0.008677455596625805,
-0.00866649392992258,
0.0117244403809309,
0.06523464620113373,
-0.031274884939193726,
0.06625952571630478,
-0.050342388451099396,
-0.05482276901602745,
0.08445682376623154,
-0.0037109581753611565,
0.08581460267305374,
-0.1056312620639801,
-0.006267915479838848,
0.0016805074410513043,
0.03180089220404625,
-0.037861187011003494,
-0.026890192180871964,
0.029224365949630737,
0.041481297463178635,
-0.05287330225110054,
0.028274059295654297,
-0.004168998915702105,
-0.04711843654513359,
0.028253236785531044,
0.026713063940405846,
0.05356067046523094,
-0.07454729825258255,
0.01215335913002491,
0.008674802258610725,
0.002732679480686784,
-0.005528884474188089,
-0.1011095717549324,
-0.08269007503986359,
-0.00893216859549284,
-0.028915394097566605,
0.01280263438820839,
-0.03088524378836155,
-0.10103844851255417,
-0.027487996965646744,
-0.09202675521373749,
-0.08071903884410858,
-0.011788311414420605,
-0.010570026002824306,
0.040800344198942184,
0.03534208983182907,
-0.03600774705410004,
-0.08346249163150787,
-0.04663081839680672,
0.0144363883882761,
-0.0611286535859108,
-0.01945393905043602,
-0.0429740846157074,
0.06830962002277374,
-0.04516363888978958,
0.03358118236064911,
-0.04700200632214546,
-0.03670932725071907,
0.06817365437746048,
0.030344508588314056,
-0.10013546049594879,
0.05196927860379219,
-0.004978442098945379,
-0.04802384972572327,
-0.029270552098751068,
0.011249368079006672,
-0.035611048340797424,
-0.04569050669670105,
-0.007384720258414745,
0.06185262277722359,
-0.003068223362788558,
0.07179275900125504,
0.042144566774368286,
0.042808420956134796,
-0.043737392872571945,
0.1017121970653534,
-0.03529709577560425,
0.015136893838644028,
0.06037892401218414,
0.0446556992828846,
0.020039809867739677,
-5.7391801533412945e-8,
-0.020838076248764992,
0.05167875811457634,
-0.0003159099433105439,
0.032759685069322586,
-0.08053361624479294,
-0.07808814197778702,
-0.0645233765244484,
0.073664091527462,
-0.03812188282608986,
0.0739324614405632,
0.05438229441642761,
0.0640188530087471,
-0.051926061511039734,
0.03982805460691452,
0.06603474169969559,
0.08508943021297455,
-0.04874661564826965,
-0.007028104271739721,
-0.0013886261731386185,
-0.043596457690000534,
0.01172784436494112,
0.03845464810729027,
0.012406852096319199,
-0.03461853042244911,
0.06254647672176361,
-0.07115825265645981,
-0.016401374712586403,
0.15517796576023102,
-0.07044593244791031,
0.03150911629199982,
-0.028944045305252075,
0.0592564232647419,
-0.0842917189002037,
0.004482691176235676,
0.12364226579666138,
0.051830366253852844,
-0.1016145721077919,
-0.02944220043718815,
-0.0042844912968575954,
0.026145359501242638,
0.04261724650859833,
-0.0030251643620431423,
-0.05400453135371208,
-0.009814517572522163,
0.12240474671125412,
0.01839965581893921,
-0.012614627368748188,
-0.005961736664175987,
0.022503379732370377,
0.0739760547876358,
0.024917954578995705,
-0.027219194918870926,
-0.0398184210062027,
0.008865961804986,
-0.036761652678251266,
0.03012857772409916,
-0.07172215729951859,
-0.008826298639178276,
0.015618893317878246,
0.011758017353713512,
-0.004138866905122995,
0.05558526888489723,
-0.027862677350640297,
0.07714439183473587
] |
indobenchmark/indobart | 73bead20e4a67f578f6f3b3f7038040304dc7065 | 2022-06-21T17:52:16.000Z | [
"pytorch",
"mbart",
"text2text-generation",
"id",
"dataset:Indo4B+",
"arxiv:2104.08200",
"transformers",
"indogpt",
"indobenchmark",
"indonlg",
"license:mit",
"autotrain_compatible"
] | text2text-generation | false | indobenchmark | null | indobenchmark/indobart | 1,923 | 1 | transformers | ---
language: id
tags:
- indogpt
- indobenchmark
- indonlg
license: mit
inference: false
datasets:
- Indo4B+
---
# IndoBART Model
[IndoBART](https://arxiv.org/abs/2104.08200) is a state-of-the-art language model for Indonesian based on the BART model. The pretrained model is trained using the BART training objective.
## All Pre-trained Models
| Model | #params | Training data |
|--------------------------------|--------------------------------|-----------------------------------|
| `indobenchmark/indobart` | 132M | Indo4B-Plus (23.79 GB of text) |
## Authors
<b>IndoBART</b> was trained and evaluated by Samuel Cahyawijaya*, Genta Indra Winata*, Bryan Wilie*, Karissa Vincentio*, Xiaohong Li*, Adhiguna Kuncoro*, Sebastian Ruder, Zhi Yuan Lim, Syafri Bahar, Masayu Leylia Khodra, Ayu Purwarianti, Pascale Fung
## Citation
If you use our work, please cite:
```bibtex
@article{cahyawijaya2021indonlg,
title={IndoNLG: Benchmark and Resources for Evaluating Indonesian Natural Language Generation},
author={Cahyawijaya, Samuel and Winata, Genta Indra and Wilie, Bryan and Vincentio, Karissa and Li, Xiaohong and Kuncoro, Adhiguna and Ruder, Sebastian and Lim, Zhi Yuan and Bahar, Syafri and Khodra, Masayu Leylia and others},
journal={arXiv preprint arXiv:2104.08200},
year={2021}
}
```
| [
-0.013703456148505211,
-0.03858499601483345,
0.032011691480875015,
-0.036327652633190155,
-0.078417107462883,
0.08799941837787628,
-0.025135699659585953,
0.023976793512701988,
0.07147400826215744,
0.02604273334145546,
0.10672129690647125,
-0.05894240736961365,
-0.016188107430934906,
-0.03765704855322838,
-0.009259538725018501,
-0.009916143491864204,
0.042446568608284,
-0.024954527616500854,
-0.00994261633604765,
-0.1104184165596962,
0.028765954077243805,
0.03706857189536095,
0.022081933915615082,
-0.06913251429796219,
0.04079880565404892,
0.010449747554957867,
0.02811170369386673,
-0.09702451527118683,
0.01780831441283226,
-0.03155890479683876,
0.009819396771490574,
0.06864684075117111,
0.03115544281899929,
0.014130113646388054,
-0.06443211436271667,
0.01959964632987976,
-0.021225804463028908,
-0.028638429939746857,
0.09544475376605988,
0.05317527800798416,
-0.021431494504213333,
-0.009915863163769245,
-0.04996984079480171,
-0.025656087324023247,
0.11544360220432281,
-0.000303895678371191,
-0.04326274245977402,
0.004006031900644302,
-0.033122915774583817,
-0.0439448282122612,
-0.11717867851257324,
-0.03031538985669613,
-0.020700104534626007,
0.046334389597177505,
0.022950924932956696,
-0.10974714159965515,
0.002493318635970354,
0.04734928905963898,
0.021314403042197227,
0.0063075534999370575,
-0.06670732796192169,
-0.0072930241003632545,
-0.08548812568187714,
0.03361242637038231,
-0.013058027252554893,
0.025804977864027023,
-0.04156254604458809,
0.06876120716333389,
-0.042398396879434586,
0.058497171849012375,
-0.03436872735619545,
0.08047527074813843,
-0.020734364166855812,
0.056937847286462784,
-0.06040360778570175,
-0.04027707874774933,
0.07627438008785248,
0.006597556173801422,
0.06161104887723923,
-0.11649181693792343,
0.001025245408527553,
0.034806232899427414,
0.07467792928218842,
-0.010434458032250404,
0.007996827363967896,
0.004199969582259655,
0.014403562992811203,
-0.001957125263288617,
0.0024001861456781626,
-0.013662457466125488,
0.047230929136276245,
-0.03941841796040535,
-0.005673462059348822,
-0.0023833841551095247,
-0.00843162089586258,
0.056892503052949905,
-0.02192053198814392,
0.04084033519029617,
-0.0035177418030798435,
0.053862638771533966,
0.08356571942567825,
0.11852666735649109,
-0.02732706628739834,
-0.07816314697265625,
-0.047159165143966675,
-0.05175744742155075,
-0.02564893662929535,
-0.01850237511098385,
0.12208539247512817,
-0.0028558059129863977,
-0.030629487708210945,
-0.015754451975226402,
-0.05696042627096176,
-0.008740688674151897,
-0.06457503139972687,
-0.014419296756386757,
-0.021852321922779083,
-0.016327695921063423,
-0.015401095151901245,
-0.0005031803157180548,
-0.05616766959428787,
-0.007033266592770815,
0.03940653055906296,
0.025204848498106003,
-0.08406853675842285,
-0.05139414966106415,
-0.006849025841802359,
-3.289020703973283e-33,
0.03553624078631401,
-0.03331277146935463,
0.010683625005185604,
-0.04621400684118271,
-0.011698284186422825,
-0.06234809011220932,
-0.03975001350045204,
-0.0764712318778038,
-0.04492277279496193,
-0.0700010433793068,
-0.04884770140051842,
-0.040998801589012146,
-0.1075671836733818,
0.0421198345720768,
0.016128674149513245,
0.051366038620471954,
-0.08372636884450912,
0.040598466992378235,
0.012242288328707218,
-0.005167901050299406,
0.08095243573188782,
0.016404593363404274,
0.03931352496147156,
-0.06818678230047226,
0.01487488579005003,
0.028338924050331116,
0.09550817310810089,
-0.07738590240478516,
-0.06866007298231125,
0.042817458510398865,
-0.0906735509634018,
-0.02194741554558277,
-0.04549221321940422,
0.017863567918539047,
-0.09465355426073074,
-0.02456110715866089,
0.012702803127467632,
-0.07984969019889832,
-0.04708404093980789,
-0.06176694855093956,
-0.0055042146705091,
0.03573796525597572,
0.09187580645084381,
-0.043731845915317535,
-0.002316294005140662,
-0.032505687326192856,
-0.011856685392558575,
-0.06505250930786133,
-0.02681151032447815,
0.113590769469738,
-0.06626657396554947,
0.0061853923834860325,
0.040457095950841904,
0.0237212423235178,
0.027387453243136406,
0.03179841488599777,
0.0266822949051857,
0.018350008875131607,
0.0033258565235882998,
0.06380772590637207,
0.011018904857337475,
-0.03495541960000992,
0.05185871943831444,
0.02953147143125534,
0.046466827392578125,
0.04990163818001747,
-0.03591592609882355,
-0.03932150825858116,
0.05966007336974144,
-0.01883166842162609,
-0.03369227051734924,
-0.08483761548995972,
0.072164386510849,
0.04476877674460411,
-0.016265487298369408,
-0.022294895723462105,
0.02278820239007473,
-0.04056348651647568,
-0.0764651894569397,
0.03776932135224342,
-0.04101148247718811,
-0.05922519043087959,
0.0064862859435379505,
-0.04687698557972908,
0.007429081480950117,
-0.006602423265576363,
0.05549946799874306,
-0.058140505105257034,
-0.03973844647407532,
-0.0064213937148451805,
0.02541118860244751,
0.029099052771925926,
-0.05963991582393646,
0.04507782682776451,
0.011076042428612709,
-1.4518595011853384e-33,
-0.013306103646755219,
0.06130962073802948,
-0.08910821378231049,
-0.0025824757758527994,
-0.0789167732000351,
-0.05979783833026886,
0.043653227388858795,
0.12059326469898224,
-0.0345943346619606,
-0.01611519232392311,
-0.016394278034567833,
-0.09597935527563095,
0.060879360884428024,
0.04236846789717674,
0.052613310515880585,
-0.0039599076844751835,
0.01263123843818903,
0.0834924653172493,
-0.014923915266990662,
0.05419624596834183,
0.01050077099353075,
0.07079349458217621,
-0.08987176418304443,
0.09720620512962341,
0.07728685438632965,
0.028123704716563225,
-0.005646274890750647,
0.0749087780714035,
-0.09157489985227585,
-0.0032263589091598988,
0.017386484891176224,
-0.055335115641355515,
-0.07967559248209,
0.01754515804350376,
-0.10229554772377014,
-0.0427037850022316,
0.050978682935237885,
-0.009293576702475548,
-0.032350700348615646,
0.11665431410074234,
0.07805031538009644,
0.09658870846033096,
-0.08777988702058792,
-0.030898932367563248,
-0.05711040273308754,
-0.015320373699069023,
-0.061002057045698166,
-0.037651970982551575,
0.050914522260427475,
-0.12001214921474457,
0.04786771535873413,
0.02217957191169262,
0.005982370115816593,
-0.023914052173495293,
-0.04918505623936653,
-0.055403146892786026,
0.0047691017389297485,
-0.013787268660962582,
-0.030068207532167435,
-0.05471824109554291,
-0.08899640291929245,
-0.03133069723844528,
0.06439956277608871,
0.005837355274707079,
0.03780428320169449,
-0.046239420771598816,
0.04496212303638458,
0.06605193763971329,
0.01603803224861622,
-0.11904837936162949,
0.032821282744407654,
0.001394358347170055,
0.0031194298062473536,
0.07458126544952393,
0.021752389147877693,
0.009698732756078243,
-0.020404724404215813,
-0.03631832078099251,
0.01147592905908823,
-0.050758715718984604,
-0.009340545162558556,
-0.024348612874746323,
0.05646006762981415,
0.03097725473344326,
0.03734222799539566,
0.06271343678236008,
-0.030973687767982483,
0.02333296649158001,
-0.02063596434891224,
0.028298985213041306,
0.024883940815925598,
0.05024144425988197,
-0.025207163766026497,
0.08782351762056351,
-0.028017576783895493,
-4.96039085362554e-8,
-0.034405939280986786,
-0.08699502050876617,
0.021912265568971634,
0.06871746480464935,
-0.021632883697748184,
-0.04218128323554993,
-0.012886370532214642,
0.01762411929666996,
-0.10348079353570938,
-0.05268973112106323,
0.003168964758515358,
0.03973047062754631,
-0.10380364954471588,
0.05037767440080643,
-0.0071975369937717915,
0.0034424313344061375,
0.023405032232403755,
0.07339857518672943,
-0.029985999688506126,
-0.054224807769060135,
0.06478047370910645,
0.014771581627428532,
0.06208150088787079,
0.008507589809596539,
0.040142010897397995,
-0.0009944661287590861,
-0.019286097958683968,
0.07614597678184509,
0.039101265370845795,
-0.05394071340560913,
-0.04209868982434273,
0.04502837732434273,
-0.08004496246576309,
-0.014433752745389938,
0.02873837575316429,
0.05520887300372124,
0.007652274798601866,
-0.011025245301425457,
-0.0452679768204689,
0.12507747113704681,
-0.008994195610284805,
-0.053954482078552246,
-0.056635502725839615,
0.0015865161549299955,
0.09833317250013351,
0.021078046411275864,
0.0008285134099423885,
-0.057651691138744354,
0.05699273571372032,
-0.03801628202199936,
-0.022533146664500237,
0.005701057147234678,
0.03160924091935158,
-0.07486281543970108,
-0.033408015966415405,
0.02809532918035984,
-0.08313995599746704,
0.05049138888716698,
0.033450737595558167,
-0.008193252608180046,
0.12147051095962524,
-0.007974887266755104,
-0.014406641013920307,
0.038879621773958206
] |
microsoft/CodeGPT-small-py | 97ebaaa7103f64e3085e88f0ecd28d1ffeb01bea | 2021-05-23T09:01:50.000Z | [
"pytorch",
"tf",
"jax",
"gpt2",
"text-generation",
"transformers"
] | text-generation | false | microsoft | null | microsoft/CodeGPT-small-py | 1,922 | 1 | transformers | Entry not found | [
0.0461147278547287,
-0.038838207721710205,
-0.01049656979739666,
-0.03682169318199158,
0.011261860840022564,
0.013094935566186905,
0.0019101888174191117,
-0.013979103416204453,
0.027092741802334785,
-0.015212527476251125,
0.017284274101257324,
-0.08189476281404495,
0.03817418962717056,
-0.04920130595564842,
0.021389011293649673,
-0.015245908871293068,
-0.03203780576586723,
-0.1245758980512619,
0.03150877356529236,
0.032381657510995865,
-0.060957908630371094,
0.05409295856952667,
-0.025087490677833557,
0.01568586938083172,
0.028129950165748596,
-0.04710396006703377,
-0.018688226118683815,
0.013785239309072495,
-0.04001208767294884,
0.01173911802470684,
-0.04317743331193924,
0.05500618368387222,
0.004543041344732046,
0.02973111905157566,
0.14852192997932434,
0.02658126689493656,
0.02907961793243885,
-0.05169107764959335,
0.05803573504090309,
-0.07732241600751877,
-0.017637968063354492,
-0.04219653457403183,
0.041807834059000015,
0.023620979860424995,
0.021563321352005005,
0.016478516161441803,
-0.0021814992651343346,
-0.06400240957736969,
0.06393089145421982,
0.019599027931690216,
-0.08565037697553635,
0.00934905931353569,
-0.008718925528228283,
-0.028583496809005737,
-0.07310017943382263,
0.09416428208351135,
0.001759322709403932,
0.06184990331530571,
0.011840506456792355,
-0.035997264087200165,
0.08358278125524521,
-0.02619801089167595,
0.03736566752195358,
-0.028206506744027138,
-0.07454850524663925,
-0.08883563429117203,
-0.06279942393302917,
-0.008695344440639019,
0.014119276776909828,
-0.0825355276465416,
0.0649217739701271,
-0.00223911227658391,
-0.14716917276382446,
0.07743025571107864,
-0.03548373281955719,
-0.055201586335897446,
0.006981803569942713,
-0.012166670523583889,
0.055111464112997055,
-0.007116836030036211,
-0.023175746202468872,
-0.005835152696818113,
-0.09185640513896942,
0.055196937173604965,
0.034148022532463074,
0.03835180774331093,
0.038685429841279984,
-0.025987252593040466,
0.017804903909564018,
0.022428328171372414,
0.025005368515849113,
-0.10761535167694092,
-0.048001550137996674,
-0.04343584179878235,
0.012374646961688995,
-0.019502125680446625,
0.029218152165412903,
0.0842173621058464,
-0.011719699949026108,
0.09283553808927536,
-0.007015465293079615,
-0.03543110564351082,
-0.06936459988355637,
0.09425332397222519,
-0.010958523489534855,
-0.00805904995650053,
0.004974212497472763,
-0.0031528924591839314,
0.06105927750468254,
-0.03964288905262947,
-0.03619541600346565,
-0.019901901483535767,
0.07134733349084854,
0.039514873176813126,
-0.012729483656585217,
-0.006646515801548958,
-0.04746140539646149,
-0.014432490803301334,
-0.05157482624053955,
0.09506245702505112,
-0.049747664481401443,
-0.04591796174645424,
-0.008965466171503067,
-0.0325421579182148,
-0.08626784384250641,
-0.06624380499124527,
0.02538885548710823,
-4.303924894057984e-33,
0.01133066974580288,
0.0033434738870710135,
-0.002155609894543886,
0.04871906340122223,
-0.023564351722598076,
-0.07933273911476135,
0.0600903145968914,
0.02335330657660961,
-0.03844716399908066,
-0.020433755591511726,
-0.06952055543661118,
-0.03235611692070961,
0.0062485747039318085,
0.064804308116436,
-0.03201229125261307,
0.061689723283052444,
0.0417000837624073,
-0.00761845987290144,
0.03340127319097519,
-0.047770582139492035,
0.00887306872755289,
-0.04066338762640953,
-0.010506896302103996,
0.0106519665569067,
0.021333497017621994,
0.12854498624801636,
-0.009705503471195698,
0.010055632330477238,
-0.017507633194327354,
0.006515394430607557,
0.06334009766578674,
-0.057817306369543076,
0.013668818399310112,
-0.020286159589886665,
0.05430467426776886,
-0.023184705525636673,
0.0828516036272049,
0.0005449643940664828,
-0.10372652113437653,
-0.07634282112121582,
-0.005381610710173845,
-0.039263784885406494,
0.0006114727002568543,
-0.013281986117362976,
0.07119110971689224,
0.043696220964193344,
0.03168422728776932,
0.04338686540722847,
0.05728672817349434,
0.0832006186246872,
-0.07961414009332657,
0.015234283171594143,
0.017002005130052567,
0.047004107385873795,
-0.09794387966394424,
0.004990279674530029,
-0.07062993198633194,
-0.028000490739941597,
-0.04018733277916908,
-0.0702052190899849,
0.011351344175636768,
0.06020182743668556,
-0.03297270089387894,
0.09396500885486603,
0.03417910635471344,
-0.019825750961899757,
-0.034690454602241516,
-0.013036907650530338,
0.05896938592195511,
-0.012359356507658958,
-0.017275206744670868,
-0.07982361316680908,
0.02059139870107174,
0.06737419217824936,
0.04176458343863487,
-0.04978838190436363,
-0.05877475067973137,
-0.06289287656545639,
-0.03354167565703392,
-0.03871942684054375,
0.009898529388010502,
-0.05514208599925041,
-0.11629002541303635,
-0.011855563148856163,
0.10663620382547379,
0.037354156374931335,
-0.0065480442717671394,
-0.051189567893743515,
0.06663123518228531,
0.01874656230211258,
0.032841797918081284,
0.041593004018068314,
-0.06879369914531708,
0.04216769337654114,
-0.01628219522535801,
5.4139394340936695e-34,
0.05697013810276985,
-0.006972255185246468,
0.015711724758148193,
-0.17956365644931793,
0.02320219948887825,
0.007923615165054798,
-0.008062449283897877,
0.0074974060989916325,
0.07391711324453354,
0.0309313777834177,
0.060510627925395966,
0.058605875819921494,
0.09515274316072464,
-0.002282935893163085,
0.001603541080839932,
0.07024981826543808,
0.012629246339201927,
0.07425693422555923,
-0.038426291197538376,
0.01861148327589035,
0.030608950182795525,
-0.02449394389986992,
0.021528491750359535,
-0.003039651783183217,
-0.03676343336701393,
0.03130284696817398,
0.07998586446046829,
0.010451192036271095,
-0.07930229604244232,
-0.013543923385441303,
0.018781835213303566,
0.05168003588914871,
-0.07191970944404602,
0.15783067047595978,
0.026191607117652893,
0.01262354850769043,
0.08218053728342056,
-0.029807550832629204,
-0.07528624683618546,
-0.04250097647309303,
0.017244765534996986,
0.04411793500185013,
0.03708017244935036,
0.009233047254383564,
-0.040271829813718796,
0.022496428340673447,
0.02495843544602394,
0.07633638381958008,
0.005147108342498541,
0.013892097398638725,
0.05610476806759834,
-0.06684739887714386,
0.05862557515501976,
-0.020688841119408607,
0.05377643182873726,
0.06718500703573227,
0.005329249892383814,
-0.01388032827526331,
0.029931528493762016,
0.009508464485406876,
-0.045173756778240204,
0.11534366756677628,
-0.06510116159915924,
0.05117698386311531,
-0.0026125339791178703,
-0.08554837852716446,
-0.03784770518541336,
0.0804959163069725,
0.011298024095594883,
-0.07695550471544266,
-0.04868878796696663,
0.02515520341694355,
0.06252261996269226,
-0.04509226232767105,
-0.01246943511068821,
0.028559505939483643,
-0.030573077499866486,
0.05066261067986488,
-0.08187384903430939,
0.04469604790210724,
0.0034051244147121906,
0.04145054519176483,
-0.021858664229512215,
-0.06112268194556236,
-0.00908052921295166,
-0.05903250351548195,
0.0259539932012558,
0.059690944850444794,
-0.07613514363765717,
-0.03720718249678612,
-0.036316655576229095,
0.07058046013116837,
-0.008224100805819035,
0.041961874812841415,
-0.0285952128469944,
-1.496900736697171e-8,
-0.0014124972512945533,
0.03401879221200943,
-0.040338415652513504,
0.04116074740886688,
0.0935964286327362,
-0.05115952715277672,
0.0008746005478315055,
-0.03389839455485344,
-0.00567849725484848,
-0.010686947964131832,
-0.04789939522743225,
-0.04820054769515991,
-0.02011880651116371,
-0.03209094703197479,
-0.04211259260773659,
-0.10229527950286865,
-0.07819421589374542,
-0.031228765845298767,
-0.02154778689146042,
-0.04960230365395546,
0.08087796717882156,
-0.07801242172718048,
0.06919731199741364,
-0.04999840259552002,
0.03687043860554695,
0.03889009356498718,
-0.049989692866802216,
-0.04254625365138054,
-0.04606937617063522,
0.08682432025671005,
-0.031148413196206093,
0.11826753616333008,
0.034102488309144974,
-0.0208592489361763,
-0.0205202866345644,
0.027134142816066742,
0.09741277992725372,
0.051608603447675705,
0.013477512635290623,
-0.13649295270442963,
-0.022304272279143333,
0.02385953813791275,
0.038732077926397324,
-0.09249968826770782,
-0.04549082741141319,
0.054220106452703476,
0.01160438358783722,
0.051190607249736786,
0.07713303714990616,
-0.022097084671258926,
-0.06127818301320076,
-0.01857956498861313,
0.006740490905940533,
-0.00496308971196413,
0.024095389991998672,
0.0736224576830864,
-0.003481915919110179,
-0.0699305310845375,
-0.006629763171076775,
-0.0598808117210865,
0.05297163128852844,
-0.02902800403535366,
-0.027858933433890343,
-0.01287526823580265
] |
saattrupdan/nbailab-base-ner-scandi | 8635b40703c27f868a29a36d99e264facddc6610 | 2022-02-09T15:21:05.000Z | [
"pytorch",
"bert",
"token-classification",
"da",
"no",
"nb",
"nn",
"sv",
"fo",
"is",
"dataset:dane",
"dataset:norne",
"dataset:wikiann",
"dataset:suc3.0",
"arxiv:1911.12146",
"transformers",
"license:mit",
"model-index",
"autotrain_compatible"
] | token-classification | false | saattrupdan | null | saattrupdan/nbailab-base-ner-scandi | 1,921 | 8 | transformers | ---
language:
- da
- no
- nb
- nn
- sv
- fo
- is
license: mit
datasets:
- dane
- norne
- wikiann
- suc3.0
model-index:
- name: nbailab-base-ner-scandi
results: []
widget:
- "Hans er en professor på Københavns Universitetet i København, og han er en rigtig københavner. Hans kat, altså Hans' kat, Lisa, er supersød. Han fik købt en Mona Lisa på tilbud i Netto og gav den til sin kat, og nu er Mona Lisa'en Lisa's kæreste eje. Hans bror Peter og Hans besluttede, at Peterskirken skulle have fint besøg. Men nu har de begge Corona."
inference:
parameters:
aggregation_strategy: "first"
---
# ScandiNER - Named Entity Recognition model for Scandinavian Languages
This model is a fine-tuned version of [NbAiLab/nb-bert-base](https://huggingface.co/NbAiLab/nb-bert-base) for Named Entity Recognition for Danish, Norwegian (both Bokmål and Nynorsk), Swedish, Icelandic and Faroese. It has been fine-tuned on the concatenation of [DaNE](https://aclanthology.org/2020.lrec-1.565/), [NorNE](https://arxiv.org/abs/1911.12146), [SUC 3.0](https://spraakbanken.gu.se/en/resources/suc3) and the Icelandic and Faroese parts of the [WikiANN](https://aclanthology.org/P17-1178/) dataset. It also works reasonably well on English sentences, given the fact that the pretrained model is also trained on English data along with Scandinavian languages.
The model will predict the following four entities:
| **Tag** | **Name** | **Description** |
| :------ | :------- | :-------------- |
| `PER` | Person | The name of a person (e.g., *Birgitte* and *Mohammed*) |
| `LOC` | Location | The name of a location (e.g., *Tyskland* and *Djurgården*) |
| `ORG` | Organisation | The name of an organisation (e.g., *Bunnpris* and *Landsbankinn*) |
| `MISC` | Miscellaneous | A named entity of a different kind (e.g., *Ūjķnustu pund* and *Mona Lisa*) |
## Quick start
You can use this model in your scripts as follows:
```python
>>> from transformers import pipeline
>>> import pandas as pd
>>> ner = pipeline(task='ner',
... model='saattrupdan/nbailab-base-ner-scandi',
... aggregation_strategy='first')
>>> result = ner('Borghild kjøper seg inn i Bunnpris')
>>> pd.DataFrame.from_records(result)
entity_group score word start end
0 PER 0.981257 Borghild 0 8
1 ORG 0.974099 Bunnpris 26 34
```
## Performance
The following is the Micro-F1 NER performance on Scandinavian NER test datasets, compared with the current state-of-the-art. The models have been evaluated on the test set along with 9 bootstrapped versions of it, with the mean and 95% confidence interval shown here:
| **Model ID** | **DaNE** | **NorNE-NB** | **NorNE-NN** | **SUC 3.0** | **WikiANN-IS** | **WikiANN-FO** | **Average** |
| :----------- | -------: | -----------: | -----------: | ----------: | -------------: | -------------: | ----------: |
| saattrupdan/nbailab-base-ner-scandi | **87.44 ± 0.81** | **91.06 ± 0.26** | **90.42 ± 0.61** | **88.37 ± 0.17** | **88.61 ± 0.41** | **90.22 ± 0.46** | **89.08 ± 0.46** |
| chcaa/da\_dacy\_large\_trf | 83.61 ± 1.18 | 78.90 ± 0.49 | 72.62 ± 0.58 | 53.35 ± 0.17 | 50.57 ± 0.46 | 51.72 ± 0.52 | 63.00 ± 0.57 |
| RecordedFuture/Swedish-NER | 64.09 ± 0.97 | 61.74 ± 0.50 | 56.67 ± 0.79 | 66.60 ± 0.27 | 34.54 ± 0.73 | 42.16 ± 0.83 | 53.32 ± 0.69 |
| Maltehb/danish-bert-botxo-ner-dane | 69.25 ± 1.17 | 60.57 ± 0.27 | 35.60 ± 1.19 | 38.37 ± 0.26 | 21.00 ± 0.57 | 27.88 ± 0.48 | 40.92 ± 0.64 |
| Maltehb/-l-ctra-danish-electra-small-uncased-ner-dane | 70.41 ± 1.19 | 48.76 ± 0.70 | 27.58 ± 0.61 | 35.39 ± 0.38 | 26.22 ± 0.52 | 28.30 ± 0.29 | 39.70 ± 0.61 |
| radbrt/nb\_nocy\_trf | 56.82 ± 1.63 | 68.20 ± 0.75 | 69.22 ± 1.04 | 31.63 ± 0.29 | 20.32 ± 0.45 | 12.91 ± 0.50 | 38.08 ± 0.75 |
Aside from its high accuracy, it's also substantially **smaller** and **faster** than the previous state-of-the-art:
| **Model ID** | **Samples/second** | **Model size** |
| :----------- | -----------------: | -------------: |
| saattrupdan/nbailab-base-ner-scandi | 4.16 ± 0.18 | 676 MB |
| chcaa/da\_dacy\_large\_trf | 0.65 ± 0.01 | 2,090 MB |
## Training procedure
### Training hyperparameters
The following hyperparameters were used during training:
- learning_rate: 2e-05
- train_batch_size: 8
- eval_batch_size: 8
- seed: 42
- gradient_accumulation_steps: 4
- total_train_batch_size: 32
- optimizer: Adam with betas=(0.9,0.999) and epsilon=1e-08
- lr_scheduler_type: linear
- lr_scheduler_warmup_steps: 90135.90000000001
- num_epochs: 1000
### Training results
| Training Loss | Epoch | Step | Validation Loss | Micro F1 | Micro F1 No Misc |
|:-------------:|:-----:|:-----:|:---------------:|:--------:|:----------------:|
| 0.6682 | 1.0 | 2816 | 0.0872 | 0.6916 | 0.7306 |
| 0.0684 | 2.0 | 5632 | 0.0464 | 0.8167 | 0.8538 |
| 0.0444 | 3.0 | 8448 | 0.0367 | 0.8485 | 0.8783 |
| 0.0349 | 4.0 | 11264 | 0.0316 | 0.8684 | 0.8920 |
| 0.0282 | 5.0 | 14080 | 0.0290 | 0.8820 | 0.9033 |
| 0.0231 | 6.0 | 16896 | 0.0283 | 0.8854 | 0.9060 |
| 0.0189 | 7.0 | 19712 | 0.0253 | 0.8964 | 0.9156 |
| 0.0155 | 8.0 | 22528 | 0.0260 | 0.9016 | 0.9201 |
| 0.0123 | 9.0 | 25344 | 0.0266 | 0.9059 | 0.9233 |
| 0.0098 | 10.0 | 28160 | 0.0280 | 0.9091 | 0.9279 |
| 0.008 | 11.0 | 30976 | 0.0309 | 0.9093 | 0.9287 |
| 0.0065 | 12.0 | 33792 | 0.0313 | 0.9103 | 0.9284 |
| 0.0053 | 13.0 | 36608 | 0.0322 | 0.9078 | 0.9257 |
| 0.0046 | 14.0 | 39424 | 0.0343 | 0.9075 | 0.9256 |
### Framework versions
- Transformers 4.10.3
- Pytorch 1.9.0+cu102
- Datasets 1.12.1
- Tokenizers 0.10.3
| [
-0.11449264734983444,
0.01043164823204279,
-0.009990046732127666,
-0.027925102040171623,
-0.0318794846534729,
0.022546619176864624,
-0.007544053252786398,
0.0033466105815023184,
0.09138554334640503,
-0.03690354898571968,
0.055450741201639175,
-0.08400768786668777,
0.06029530242085457,
-0.03394573554396629,
-0.012074110098183155,
-0.041682831943035126,
-0.0013322620652616024,
0.002556473482400179,
-0.037712447345256805,
0.0000852960511110723,
-0.006971219088882208,
-0.011089951731264591,
0.034942157566547394,
-0.09904240816831589,
0.05836225301027298,
0.029334021732211113,
-0.015450735576450825,
-0.060508374124765396,
-0.006302736233919859,
-0.04826962947845459,
0.02191394567489624,
0.05386822670698166,
0.05541309714317322,
0.08261682838201523,
0.055655188858509064,
0.030357487499713898,
-0.047804560512304306,
-0.03719838336110115,
-0.03636256605386734,
0.08910173922777176,
-0.05847853422164917,
-0.06441935896873474,
-0.047640468925237656,
-0.03828942030668259,
0.08716749399900436,
0.11355216056108475,
-0.08663436025381088,
0.004621201194822788,
-0.07349498569965363,
0.005669206380844116,
-0.0974099338054657,
-0.009799833409488201,
0.06406378746032715,
0.06088985875248909,
-0.004444904159754515,
0.01705927401781082,
-0.0384337343275547,
-0.04069436714053154,
-0.0032194440718740225,
-0.04503283649682999,
-0.06764473766088486,
-0.024917136877775192,
0.005352787207812071,
-0.00127974315546453,
-0.013959212228655815,
-0.03085559979081154,
-0.02018645778298378,
-0.01139302272349596,
-0.05420439690351486,
0.03029446303844452,
-0.009650102816522121,
0.0033206837251782417,
-0.047124069184064865,
0.04747477546334267,
-0.0103537542745471,
0.04006163403391838,
0.04606599360704422,
-0.036952294409275055,
0.057110417634248734,
-0.09469404071569443,
-0.02003324218094349,
0.02495753951370716,
0.012054343707859516,
-0.005381861701607704,
0.010351522825658321,
0.02772649936378002,
0.05761922150850296,
-0.02613654173910618,
0.04429714381694794,
-0.005410210229456425,
-0.014090283773839474,
-0.06808152794837952,
-0.006926379632204771,
-0.007116524036973715,
0.04856467992067337,
0.018978100270032883,
0.06986118853092194,
0.09461604058742523,
0.006612004246562719,
0.09286879003047943,
-0.01218757126480341,
0.0893249660730362,
0.08099319785833359,
-0.03500719368457794,
-0.05840882286429405,
-0.07199873775243759,
-0.009450355544686317,
0.012107672169804573,
0.07589469850063324,
-0.04645533859729767,
0.0034118606708943844,
-0.06572546809911728,
-0.02533361315727234,
-0.09437325596809387,
0.02416272833943367,
-0.015725499019026756,
0.03858385607600212,
-0.07895934581756592,
0.04636998474597931,
-0.02401554211974144,
0.06643037497997284,
0.0202687606215477,
0.029704654589295387,
0.07542094588279724,
-0.015540399588644505,
0.10822892189025879,
-0.031004663556814194,
1.2705058857794067e-32,
0.02251042239367962,
0.1018921509385109,
0.011735630221664906,
-0.04309341683983803,
-0.0733153373003006,
-0.03237879276275635,
-0.0845598354935646,
0.011476007290184498,
-0.08515800535678864,
0.0006394763477146626,
-0.08395736664533615,
0.026292121037840843,
-0.08629071712493896,
-0.007650753483176231,
0.01300512719899416,
0.051323339343070984,
0.03997413441538811,
0.00832359865307808,
-0.07086232304573059,
0.04174898937344551,
0.13515734672546387,
0.0066190073266625404,
0.03758792579174042,
-0.008650480769574642,
-0.07316242158412933,
0.006953352130949497,
-0.002244579140096903,
-0.09452468901872635,
-0.0538959838449955,
0.0218551903963089,
-0.018618999049067497,
-0.060509711503982544,
-0.013052839785814285,
-0.03039541281759739,
-0.0012710954761132598,
-0.05541899800300598,
-0.013950335793197155,
-0.022394534200429916,
-0.0779985636472702,
-0.075267493724823,
-0.03622148558497429,
0.03400908038020134,
0.020654063671827316,
-0.015429284423589706,
-0.038390662521123886,
-0.020914699882268906,
0.02236304059624672,
-0.0505448542535305,
0.0004649956536013633,
0.002761021489277482,
-0.0016189386369660497,
0.01983952522277832,
-0.029290571808815002,
0.01976792886853218,
0.027651354670524597,
0.056507401168346405,
0.07625141739845276,
0.04291597381234169,
0.001715235412120819,
-0.002119959332048893,
-0.008865167386829853,
-0.022462230175733566,
0.0707399919629097,
0.032303858548402786,
0.07033190876245499,
0.005932371132075787,
-0.054733727127313614,
0.06040582060813904,
0.09990967065095901,
-0.003093003062531352,
-0.019146988168358803,
0.0033555685076862574,
0.10968238860368729,
0.034018345177173615,
0.045304302126169205,
-0.016013845801353455,
0.051455702632665634,
-0.033000290393829346,
-0.04600415751338005,
0.0006148273241706192,
-0.028628651052713394,
0.0029589503537863493,
-0.035275477916002274,
-0.0723281055688858,
-0.01272700633853674,
-0.054880280047655106,
0.07590746879577637,
-0.0879192054271698,
-0.04708178713917732,
-0.034787654876708984,
0.055885545909404755,
0.04985131695866585,
0.010049022734165192,
-0.0709778293967247,
-0.050204161554574966,
-1.1962601197451356e-32,
0.01547144539654255,
-0.0010869849938899279,
-0.06830941885709763,
0.011158079840242863,
-0.01415693573653698,
-0.03814796730875969,
0.061426736414432526,
0.08959615230560303,
-0.01975090801715851,
-0.08688747137784958,
0.023884126916527748,
-0.08855298161506653,
0.04213361069560051,
-0.01425404753535986,
0.12381816655397415,
0.026251796633005142,
-0.031985804438591,
0.050220053642988205,
0.011833103373646736,
0.11434478312730789,
0.012878078036010265,
0.015794912353157997,
-0.1291467696428299,
0.1016273945569992,
0.02036619931459427,
0.08417534083127975,
0.03677595034241676,
0.012178425677120686,
-0.025764619931578636,
-0.025461388751864433,
-0.055930718779563904,
0.0038342494517564774,
-0.03025810234248638,
0.07187998294830322,
-0.06781763583421707,
0.011998291127383709,
0.02295224368572235,
-0.0005658515729010105,
-0.0584196075797081,
0.01866287738084793,
0.004246861208230257,
0.027611173689365387,
-0.09966739267110825,
-0.006671046372503042,
0.007872496731579304,
-0.06305311620235443,
-0.020668581128120422,
0.04807692766189575,
0.023530034348368645,
-0.07226479053497314,
-0.01591627486050129,
0.012680530548095703,
-0.06150424852967262,
0.025176426395773888,
0.01827538199722767,
-0.012572837062180042,
-0.01664002425968647,
-0.049900006502866745,
-0.032096147537231445,
-0.011825175024569035,
-0.03970097005367279,
-0.019771402701735497,
0.04835359379649162,
0.04252679646015167,
0.0210232175886631,
-0.06842860579490662,
-0.07518993318080902,
-0.0020916624926030636,
-0.02851555496454239,
-0.07389888167381287,
-0.020420534536242485,
-0.03422106057405472,
0.04193229600787163,
0.004294463898986578,
0.045958153903484344,
-0.038696397095918655,
0.00358796794898808,
0.004081389866769314,
-0.03461550921201706,
-0.06694526225328445,
-0.1649877279996872,
-0.04984298348426819,
0.029344934970140457,
0.0472274124622345,
0.03996029868721962,
0.017599942162632942,
0.03234252333641052,
-0.032295163720846176,
-0.02493808977305889,
-0.016424238681793213,
-0.03380222246050835,
0.049703940749168396,
0.019093532115221024,
0.11498689651489258,
-0.011071647517383099,
-6.902004656694771e-8,
-0.02607627771794796,
-0.027853816747665405,
-0.03823114186525345,
0.010283347219228745,
0.10078584402799606,
-0.13026735186576843,
-0.03040074184536934,
-0.0150164058431983,
-0.07839254289865494,
-0.010560674592852592,
0.035203441977500916,
0.11977093666791916,
-0.088663250207901,
-0.060680340975522995,
0.0709034875035286,
0.06251552700996399,
0.043988242745399475,
0.012178843840956688,
-0.03891471028327942,
0.029191281646490097,
0.033271484076976776,
0.02171451225876808,
-0.016916325315833092,
-0.09348142892122269,
-0.02497616782784462,
0.023476967588067055,
-0.004801302682608366,
0.10730355978012085,
0.0479353666305542,
-0.11774200201034546,
-0.00022828110377304256,
0.08273763954639435,
-0.013508016243577003,
0.006984272506088018,
0.07262659817934036,
0.04390571266412735,
-0.06945332884788513,
0.004241019021719694,
-0.029799548909068108,
0.10681802034378052,
0.09678172320127487,
0.011894246563315392,
-0.08333295583724976,
0.005795092787593603,
0.028171831741929054,
0.06569275259971619,
0.001319841598160565,
-0.11209513247013092,
0.060693882405757904,
0.03334520384669304,
-0.026748590171337128,
-0.025186296552419662,
-0.021175255998969078,
0.008033737540245056,
-0.0014851418090984225,
0.06731487810611725,
0.018046455457806587,
0.0097919050604105,
0.06114359572529793,
0.03677301108837128,
0.05150828883051872,
-0.025900449603796005,
0.029114916920661926,
0.011378691531717777
] |
studio-ousia/luke-large-finetuned-conll-2003 | 2508abe6e591d7a9142d5ee9ab2eb5dccd7741fd | 2021-04-26T16:09:42.000Z | [
"pytorch",
"luke",
"transformers"
] | null | false | studio-ousia | null | studio-ousia/luke-large-finetuned-conll-2003 | 1,920 | null | transformers | Entry not found | [
0.0461147278547287,
-0.038838207721710205,
-0.01049656979739666,
-0.03682169318199158,
0.011261860840022564,
0.013094935566186905,
0.0019101888174191117,
-0.013979103416204453,
0.027092741802334785,
-0.015212527476251125,
0.017284274101257324,
-0.08189476281404495,
0.03817418962717056,
-0.04920130595564842,
0.021389011293649673,
-0.015245908871293068,
-0.03203780576586723,
-0.1245758980512619,
0.03150877356529236,
0.032381657510995865,
-0.060957908630371094,
0.05409295856952667,
-0.025087490677833557,
0.01568586938083172,
0.028129950165748596,
-0.04710396006703377,
-0.018688226118683815,
0.013785239309072495,
-0.04001208767294884,
0.01173911802470684,
-0.04317743331193924,
0.05500618368387222,
0.004543041344732046,
0.02973111905157566,
0.14852192997932434,
0.02658126689493656,
0.02907961793243885,
-0.05169107764959335,
0.05803573504090309,
-0.07732241600751877,
-0.017637968063354492,
-0.04219653457403183,
0.041807834059000015,
0.023620979860424995,
0.021563321352005005,
0.016478516161441803,
-0.0021814992651343346,
-0.06400240957736969,
0.06393089145421982,
0.019599027931690216,
-0.08565037697553635,
0.00934905931353569,
-0.008718925528228283,
-0.028583496809005737,
-0.07310017943382263,
0.09416428208351135,
0.001759322709403932,
0.06184990331530571,
0.011840506456792355,
-0.035997264087200165,
0.08358278125524521,
-0.02619801089167595,
0.03736566752195358,
-0.028206506744027138,
-0.07454850524663925,
-0.08883563429117203,
-0.06279942393302917,
-0.008695344440639019,
0.014119276776909828,
-0.0825355276465416,
0.0649217739701271,
-0.00223911227658391,
-0.14716917276382446,
0.07743025571107864,
-0.03548373281955719,
-0.055201586335897446,
0.006981803569942713,
-0.012166670523583889,
0.055111464112997055,
-0.007116836030036211,
-0.023175746202468872,
-0.005835152696818113,
-0.09185640513896942,
0.055196937173604965,
0.034148022532463074,
0.03835180774331093,
0.038685429841279984,
-0.025987252593040466,
0.017804903909564018,
0.022428328171372414,
0.025005368515849113,
-0.10761535167694092,
-0.048001550137996674,
-0.04343584179878235,
0.012374646961688995,
-0.019502125680446625,
0.029218152165412903,
0.0842173621058464,
-0.011719699949026108,
0.09283553808927536,
-0.007015465293079615,
-0.03543110564351082,
-0.06936459988355637,
0.09425332397222519,
-0.010958523489534855,
-0.00805904995650053,
0.004974212497472763,
-0.0031528924591839314,
0.06105927750468254,
-0.03964288905262947,
-0.03619541600346565,
-0.019901901483535767,
0.07134733349084854,
0.039514873176813126,
-0.012729483656585217,
-0.006646515801548958,
-0.04746140539646149,
-0.014432490803301334,
-0.05157482624053955,
0.09506245702505112,
-0.049747664481401443,
-0.04591796174645424,
-0.008965466171503067,
-0.0325421579182148,
-0.08626784384250641,
-0.06624380499124527,
0.02538885548710823,
-4.303924894057984e-33,
0.01133066974580288,
0.0033434738870710135,
-0.002155609894543886,
0.04871906340122223,
-0.023564351722598076,
-0.07933273911476135,
0.0600903145968914,
0.02335330657660961,
-0.03844716399908066,
-0.020433755591511726,
-0.06952055543661118,
-0.03235611692070961,
0.0062485747039318085,
0.064804308116436,
-0.03201229125261307,
0.061689723283052444,
0.0417000837624073,
-0.00761845987290144,
0.03340127319097519,
-0.047770582139492035,
0.00887306872755289,
-0.04066338762640953,
-0.010506896302103996,
0.0106519665569067,
0.021333497017621994,
0.12854498624801636,
-0.009705503471195698,
0.010055632330477238,
-0.017507633194327354,
0.006515394430607557,
0.06334009766578674,
-0.057817306369543076,
0.013668818399310112,
-0.020286159589886665,
0.05430467426776886,
-0.023184705525636673,
0.0828516036272049,
0.0005449643940664828,
-0.10372652113437653,
-0.07634282112121582,
-0.005381610710173845,
-0.039263784885406494,
0.0006114727002568543,
-0.013281986117362976,
0.07119110971689224,
0.043696220964193344,
0.03168422728776932,
0.04338686540722847,
0.05728672817349434,
0.0832006186246872,
-0.07961414009332657,
0.015234283171594143,
0.017002005130052567,
0.047004107385873795,
-0.09794387966394424,
0.004990279674530029,
-0.07062993198633194,
-0.028000490739941597,
-0.04018733277916908,
-0.0702052190899849,
0.011351344175636768,
0.06020182743668556,
-0.03297270089387894,
0.09396500885486603,
0.03417910635471344,
-0.019825750961899757,
-0.034690454602241516,
-0.013036907650530338,
0.05896938592195511,
-0.012359356507658958,
-0.017275206744670868,
-0.07982361316680908,
0.02059139870107174,
0.06737419217824936,
0.04176458343863487,
-0.04978838190436363,
-0.05877475067973137,
-0.06289287656545639,
-0.03354167565703392,
-0.03871942684054375,
0.009898529388010502,
-0.05514208599925041,
-0.11629002541303635,
-0.011855563148856163,
0.10663620382547379,
0.037354156374931335,
-0.0065480442717671394,
-0.051189567893743515,
0.06663123518228531,
0.01874656230211258,
0.032841797918081284,
0.041593004018068314,
-0.06879369914531708,
0.04216769337654114,
-0.01628219522535801,
5.4139394340936695e-34,
0.05697013810276985,
-0.006972255185246468,
0.015711724758148193,
-0.17956365644931793,
0.02320219948887825,
0.007923615165054798,
-0.008062449283897877,
0.0074974060989916325,
0.07391711324453354,
0.0309313777834177,
0.060510627925395966,
0.058605875819921494,
0.09515274316072464,
-0.002282935893163085,
0.001603541080839932,
0.07024981826543808,
0.012629246339201927,
0.07425693422555923,
-0.038426291197538376,
0.01861148327589035,
0.030608950182795525,
-0.02449394389986992,
0.021528491750359535,
-0.003039651783183217,
-0.03676343336701393,
0.03130284696817398,
0.07998586446046829,
0.010451192036271095,
-0.07930229604244232,
-0.013543923385441303,
0.018781835213303566,
0.05168003588914871,
-0.07191970944404602,
0.15783067047595978,
0.026191607117652893,
0.01262354850769043,
0.08218053728342056,
-0.029807550832629204,
-0.07528624683618546,
-0.04250097647309303,
0.017244765534996986,
0.04411793500185013,
0.03708017244935036,
0.009233047254383564,
-0.040271829813718796,
0.022496428340673447,
0.02495843544602394,
0.07633638381958008,
0.005147108342498541,
0.013892097398638725,
0.05610476806759834,
-0.06684739887714386,
0.05862557515501976,
-0.020688841119408607,
0.05377643182873726,
0.06718500703573227,
0.005329249892383814,
-0.01388032827526331,
0.029931528493762016,
0.009508464485406876,
-0.045173756778240204,
0.11534366756677628,
-0.06510116159915924,
0.05117698386311531,
-0.0026125339791178703,
-0.08554837852716446,
-0.03784770518541336,
0.0804959163069725,
0.011298024095594883,
-0.07695550471544266,
-0.04868878796696663,
0.02515520341694355,
0.06252261996269226,
-0.04509226232767105,
-0.01246943511068821,
0.028559505939483643,
-0.030573077499866486,
0.05066261067986488,
-0.08187384903430939,
0.04469604790210724,
0.0034051244147121906,
0.04145054519176483,
-0.021858664229512215,
-0.06112268194556236,
-0.00908052921295166,
-0.05903250351548195,
0.0259539932012558,
0.059690944850444794,
-0.07613514363765717,
-0.03720718249678612,
-0.036316655576229095,
0.07058046013116837,
-0.008224100805819035,
0.041961874812841415,
-0.0285952128469944,
-1.496900736697171e-8,
-0.0014124972512945533,
0.03401879221200943,
-0.040338415652513504,
0.04116074740886688,
0.0935964286327362,
-0.05115952715277672,
0.0008746005478315055,
-0.03389839455485344,
-0.00567849725484848,
-0.010686947964131832,
-0.04789939522743225,
-0.04820054769515991,
-0.02011880651116371,
-0.03209094703197479,
-0.04211259260773659,
-0.10229527950286865,
-0.07819421589374542,
-0.031228765845298767,
-0.02154778689146042,
-0.04960230365395546,
0.08087796717882156,
-0.07801242172718048,
0.06919731199741364,
-0.04999840259552002,
0.03687043860554695,
0.03889009356498718,
-0.049989692866802216,
-0.04254625365138054,
-0.04606937617063522,
0.08682432025671005,
-0.031148413196206093,
0.11826753616333008,
0.034102488309144974,
-0.0208592489361763,
-0.0205202866345644,
0.027134142816066742,
0.09741277992725372,
0.051608603447675705,
0.013477512635290623,
-0.13649295270442963,
-0.022304272279143333,
0.02385953813791275,
0.038732077926397324,
-0.09249968826770782,
-0.04549082741141319,
0.054220106452703476,
0.01160438358783722,
0.051190607249736786,
0.07713303714990616,
-0.022097084671258926,
-0.06127818301320076,
-0.01857956498861313,
0.006740490905940533,
-0.00496308971196413,
0.024095389991998672,
0.0736224576830864,
-0.003481915919110179,
-0.0699305310845375,
-0.006629763171076775,
-0.0598808117210865,
0.05297163128852844,
-0.02902800403535366,
-0.027858933433890343,
-0.01287526823580265
] |
m-polignano-uniba/bert_uncased_L-12_H-768_A-12_italian_alb3rt0 | 4454cfbc82952da79729e33e81c37a72dc095b4b | 2021-05-19T22:20:54.000Z | [
"pytorch",
"tf",
"jax",
"bert",
"fill-mask",
"transformers",
"autotrain_compatible"
] | fill-mask | false | m-polignano-uniba | null | m-polignano-uniba/bert_uncased_L-12_H-768_A-12_italian_alb3rt0 | 1,917 | 3 | transformers | Entry not found | [
0.0461147278547287,
-0.038838207721710205,
-0.01049656979739666,
-0.03682169318199158,
0.011261860840022564,
0.013094935566186905,
0.0019101888174191117,
-0.013979103416204453,
0.027092741802334785,
-0.015212527476251125,
0.017284274101257324,
-0.08189476281404495,
0.03817418962717056,
-0.04920130595564842,
0.021389011293649673,
-0.015245908871293068,
-0.03203780576586723,
-0.1245758980512619,
0.03150877356529236,
0.032381657510995865,
-0.060957908630371094,
0.05409295856952667,
-0.025087490677833557,
0.01568586938083172,
0.028129950165748596,
-0.04710396006703377,
-0.018688226118683815,
0.013785239309072495,
-0.04001208767294884,
0.01173911802470684,
-0.04317743331193924,
0.05500618368387222,
0.004543041344732046,
0.02973111905157566,
0.14852192997932434,
0.02658126689493656,
0.02907961793243885,
-0.05169107764959335,
0.05803573504090309,
-0.07732241600751877,
-0.017637968063354492,
-0.04219653457403183,
0.041807834059000015,
0.023620979860424995,
0.021563321352005005,
0.016478516161441803,
-0.0021814992651343346,
-0.06400240957736969,
0.06393089145421982,
0.019599027931690216,
-0.08565037697553635,
0.00934905931353569,
-0.008718925528228283,
-0.028583496809005737,
-0.07310017943382263,
0.09416428208351135,
0.001759322709403932,
0.06184990331530571,
0.011840506456792355,
-0.035997264087200165,
0.08358278125524521,
-0.02619801089167595,
0.03736566752195358,
-0.028206506744027138,
-0.07454850524663925,
-0.08883563429117203,
-0.06279942393302917,
-0.008695344440639019,
0.014119276776909828,
-0.0825355276465416,
0.0649217739701271,
-0.00223911227658391,
-0.14716917276382446,
0.07743025571107864,
-0.03548373281955719,
-0.055201586335897446,
0.006981803569942713,
-0.012166670523583889,
0.055111464112997055,
-0.007116836030036211,
-0.023175746202468872,
-0.005835152696818113,
-0.09185640513896942,
0.055196937173604965,
0.034148022532463074,
0.03835180774331093,
0.038685429841279984,
-0.025987252593040466,
0.017804903909564018,
0.022428328171372414,
0.025005368515849113,
-0.10761535167694092,
-0.048001550137996674,
-0.04343584179878235,
0.012374646961688995,
-0.019502125680446625,
0.029218152165412903,
0.0842173621058464,
-0.011719699949026108,
0.09283553808927536,
-0.007015465293079615,
-0.03543110564351082,
-0.06936459988355637,
0.09425332397222519,
-0.010958523489534855,
-0.00805904995650053,
0.004974212497472763,
-0.0031528924591839314,
0.06105927750468254,
-0.03964288905262947,
-0.03619541600346565,
-0.019901901483535767,
0.07134733349084854,
0.039514873176813126,
-0.012729483656585217,
-0.006646515801548958,
-0.04746140539646149,
-0.014432490803301334,
-0.05157482624053955,
0.09506245702505112,
-0.049747664481401443,
-0.04591796174645424,
-0.008965466171503067,
-0.0325421579182148,
-0.08626784384250641,
-0.06624380499124527,
0.02538885548710823,
-4.303924894057984e-33,
0.01133066974580288,
0.0033434738870710135,
-0.002155609894543886,
0.04871906340122223,
-0.023564351722598076,
-0.07933273911476135,
0.0600903145968914,
0.02335330657660961,
-0.03844716399908066,
-0.020433755591511726,
-0.06952055543661118,
-0.03235611692070961,
0.0062485747039318085,
0.064804308116436,
-0.03201229125261307,
0.061689723283052444,
0.0417000837624073,
-0.00761845987290144,
0.03340127319097519,
-0.047770582139492035,
0.00887306872755289,
-0.04066338762640953,
-0.010506896302103996,
0.0106519665569067,
0.021333497017621994,
0.12854498624801636,
-0.009705503471195698,
0.010055632330477238,
-0.017507633194327354,
0.006515394430607557,
0.06334009766578674,
-0.057817306369543076,
0.013668818399310112,
-0.020286159589886665,
0.05430467426776886,
-0.023184705525636673,
0.0828516036272049,
0.0005449643940664828,
-0.10372652113437653,
-0.07634282112121582,
-0.005381610710173845,
-0.039263784885406494,
0.0006114727002568543,
-0.013281986117362976,
0.07119110971689224,
0.043696220964193344,
0.03168422728776932,
0.04338686540722847,
0.05728672817349434,
0.0832006186246872,
-0.07961414009332657,
0.015234283171594143,
0.017002005130052567,
0.047004107385873795,
-0.09794387966394424,
0.004990279674530029,
-0.07062993198633194,
-0.028000490739941597,
-0.04018733277916908,
-0.0702052190899849,
0.011351344175636768,
0.06020182743668556,
-0.03297270089387894,
0.09396500885486603,
0.03417910635471344,
-0.019825750961899757,
-0.034690454602241516,
-0.013036907650530338,
0.05896938592195511,
-0.012359356507658958,
-0.017275206744670868,
-0.07982361316680908,
0.02059139870107174,
0.06737419217824936,
0.04176458343863487,
-0.04978838190436363,
-0.05877475067973137,
-0.06289287656545639,
-0.03354167565703392,
-0.03871942684054375,
0.009898529388010502,
-0.05514208599925041,
-0.11629002541303635,
-0.011855563148856163,
0.10663620382547379,
0.037354156374931335,
-0.0065480442717671394,
-0.051189567893743515,
0.06663123518228531,
0.01874656230211258,
0.032841797918081284,
0.041593004018068314,
-0.06879369914531708,
0.04216769337654114,
-0.01628219522535801,
5.4139394340936695e-34,
0.05697013810276985,
-0.006972255185246468,
0.015711724758148193,
-0.17956365644931793,
0.02320219948887825,
0.007923615165054798,
-0.008062449283897877,
0.0074974060989916325,
0.07391711324453354,
0.0309313777834177,
0.060510627925395966,
0.058605875819921494,
0.09515274316072464,
-0.002282935893163085,
0.001603541080839932,
0.07024981826543808,
0.012629246339201927,
0.07425693422555923,
-0.038426291197538376,
0.01861148327589035,
0.030608950182795525,
-0.02449394389986992,
0.021528491750359535,
-0.003039651783183217,
-0.03676343336701393,
0.03130284696817398,
0.07998586446046829,
0.010451192036271095,
-0.07930229604244232,
-0.013543923385441303,
0.018781835213303566,
0.05168003588914871,
-0.07191970944404602,
0.15783067047595978,
0.026191607117652893,
0.01262354850769043,
0.08218053728342056,
-0.029807550832629204,
-0.07528624683618546,
-0.04250097647309303,
0.017244765534996986,
0.04411793500185013,
0.03708017244935036,
0.009233047254383564,
-0.040271829813718796,
0.022496428340673447,
0.02495843544602394,
0.07633638381958008,
0.005147108342498541,
0.013892097398638725,
0.05610476806759834,
-0.06684739887714386,
0.05862557515501976,
-0.020688841119408607,
0.05377643182873726,
0.06718500703573227,
0.005329249892383814,
-0.01388032827526331,
0.029931528493762016,
0.009508464485406876,
-0.045173756778240204,
0.11534366756677628,
-0.06510116159915924,
0.05117698386311531,
-0.0026125339791178703,
-0.08554837852716446,
-0.03784770518541336,
0.0804959163069725,
0.011298024095594883,
-0.07695550471544266,
-0.04868878796696663,
0.02515520341694355,
0.06252261996269226,
-0.04509226232767105,
-0.01246943511068821,
0.028559505939483643,
-0.030573077499866486,
0.05066261067986488,
-0.08187384903430939,
0.04469604790210724,
0.0034051244147121906,
0.04145054519176483,
-0.021858664229512215,
-0.06112268194556236,
-0.00908052921295166,
-0.05903250351548195,
0.0259539932012558,
0.059690944850444794,
-0.07613514363765717,
-0.03720718249678612,
-0.036316655576229095,
0.07058046013116837,
-0.008224100805819035,
0.041961874812841415,
-0.0285952128469944,
-1.496900736697171e-8,
-0.0014124972512945533,
0.03401879221200943,
-0.040338415652513504,
0.04116074740886688,
0.0935964286327362,
-0.05115952715277672,
0.0008746005478315055,
-0.03389839455485344,
-0.00567849725484848,
-0.010686947964131832,
-0.04789939522743225,
-0.04820054769515991,
-0.02011880651116371,
-0.03209094703197479,
-0.04211259260773659,
-0.10229527950286865,
-0.07819421589374542,
-0.031228765845298767,
-0.02154778689146042,
-0.04960230365395546,
0.08087796717882156,
-0.07801242172718048,
0.06919731199741364,
-0.04999840259552002,
0.03687043860554695,
0.03889009356498718,
-0.049989692866802216,
-0.04254625365138054,
-0.04606937617063522,
0.08682432025671005,
-0.031148413196206093,
0.11826753616333008,
0.034102488309144974,
-0.0208592489361763,
-0.0205202866345644,
0.027134142816066742,
0.09741277992725372,
0.051608603447675705,
0.013477512635290623,
-0.13649295270442963,
-0.022304272279143333,
0.02385953813791275,
0.038732077926397324,
-0.09249968826770782,
-0.04549082741141319,
0.054220106452703476,
0.01160438358783722,
0.051190607249736786,
0.07713303714990616,
-0.022097084671258926,
-0.06127818301320076,
-0.01857956498861313,
0.006740490905940533,
-0.00496308971196413,
0.024095389991998672,
0.0736224576830864,
-0.003481915919110179,
-0.0699305310845375,
-0.006629763171076775,
-0.0598808117210865,
0.05297163128852844,
-0.02902800403535366,
-0.027858933433890343,
-0.01287526823580265
] |
klue/roberta-small | f360e3d753b17f3b7508154fefdb042c706db147 | 2021-10-20T16:13:01.000Z | [
"pytorch",
"roberta",
"fill-mask",
"ko",
"arxiv:2105.09680",
"transformers",
"korean",
"klue",
"autotrain_compatible"
] | fill-mask | false | klue | null | klue/roberta-small | 1,911 | null | transformers | ---
language: ko
tags:
- korean
- klue
mask_token: "[MASK]"
widget:
- text: 대한민국의 수도는 [MASK] 입니다.
---
# KLUE RoBERTa small
Pretrained RoBERTa Model on Korean Language. See [Github](https://github.com/KLUE-benchmark/KLUE) and [Paper](https://arxiv.org/abs/2105.09680) for more details.
## How to use
_NOTE:_ Use `BertTokenizer` instead of RobertaTokenizer. (`AutoTokenizer` will load `BertTokenizer`)
```python
from transformers import AutoModel, AutoTokenizer
model = AutoModel.from_pretrained("klue/roberta-small")
tokenizer = AutoTokenizer.from_pretrained("klue/roberta-small")
```
## BibTeX entry and citation info
```bibtex
@misc{park2021klue,
title={KLUE: Korean Language Understanding Evaluation},
author={Sungjoon Park and Jihyung Moon and Sungdong Kim and Won Ik Cho and Jiyoon Han and Jangwon Park and Chisung Song and Junseong Kim and Yongsook Song and Taehwan Oh and Joohong Lee and Juhyun Oh and Sungwon Lyu and Younghoon Jeong and Inkwon Lee and Sangwoo Seo and Dongjun Lee and Hyunwoo Kim and Myeonghwa Lee and Seongbo Jang and Seungwon Do and Sunkyoung Kim and Kyungtae Lim and Jongwon Lee and Kyumin Park and Jamin Shin and Seonghyun Kim and Lucy Park and Alice Oh and Jungwoo Ha and Kyunghyun Cho},
year={2021},
eprint={2105.09680},
archivePrefix={arXiv},
primaryClass={cs.CL}
}
```
| [
-0.14636178314685822,
-0.011534344404935837,
0.06657921522855759,
0.02165084145963192,
-0.042297132313251495,
0.06317547708749771,
0.02430441416800022,
0.042119383811950684,
0.000834080798085779,
-0.01416704710572958,
-0.004429937340319157,
-0.06439081579446793,
0.014072272926568985,
0.0474577434360981,
0.048516895622015,
0.06150955706834793,
0.01257176510989666,
0.019400138407945633,
-0.1024407148361206,
-0.05228295177221298,
0.08415886759757996,
0.013955292291939259,
0.08873672038316727,
-0.017345068976283073,
0.06271084398031235,
-0.05378260463476181,
-0.04495875909924507,
-0.021091993898153305,
0.049161188304424286,
0.08684474974870682,
-0.03543074429035187,
0.041090939193964005,
0.021771518513560295,
0.0437907837331295,
0.04278000816702843,
0.1005798950791359,
-0.04328589141368866,
0.050465360283851624,
0.052682701498270035,
0.01841985620558262,
-0.02822110429406166,
0.014293093234300613,
-0.07291334122419357,
-0.0666799321770668,
0.02713136002421379,
-0.06475359201431274,
-0.033614642918109894,
-0.004743543919175863,
-0.08184894174337387,
0.006280917674303055,
-0.05719539895653725,
0.010091423988342285,
0.01776302419602871,
-0.02932038903236389,
-0.06749903410673141,
-0.009681059047579765,
0.015843437984585762,
-0.009334771893918514,
0.07301445305347443,
-0.0775688961148262,
-0.0711742416024208,
-0.07182224094867706,
-0.023404907435178757,
-0.013383869081735611,
-0.05892682448029518,
-0.017260519787669182,
-0.03956688567996025,
0.06828043609857559,
0.03354541212320328,
0.03902481868863106,
0.007700042333453894,
-0.026403073221445084,
0.01811874471604824,
0.06971440464258194,
0.021872950717806816,
-0.10343381017446518,
0.04367129132151604,
0.021513676270842552,
-0.011311850510537624,
-0.07486891001462936,
0.037527214735746384,
-0.014697224833071232,
0.04923221468925476,
0.03645500913262367,
0.06839831173419952,
0.0048160068690776825,
-0.01649661920964718,
-0.010129955597221851,
-0.010707953944802284,
-0.012118994258344173,
0.02045697160065174,
-0.0786665752530098,
0.0336938239634037,
-0.01907813362777233,
-0.025267591699957848,
0.023288048803806305,
0.009325854480266571,
0.04203009605407715,
-0.03502792865037918,
0.07826443016529083,
0.06775032728910446,
0.041062697768211365,
0.054919056594371796,
-0.0015452818479388952,
0.017861051484942436,
-0.027842750772833824,
0.033393263816833496,
-0.033409275114536285,
0.04740212485194206,
-0.0284697487950325,
0.00612100912258029,
-0.0076944464817643166,
-0.004544421564787626,
-0.03134031221270561,
0.011392824351787567,
0.008712510578334332,
0.05949835106730461,
-0.07597962021827698,
0.02262534573674202,
0.11369013041257858,
0.044139500707387924,
-0.049705199897289276,
-0.07214249670505524,
0.030946576967835426,
-0.04690352454781532,
0.03882407769560814,
0.05052395164966583,
4.648221679284062e-33,
0.045150574296712875,
0.02344893105328083,
0.06616707146167755,
-0.002714726375415921,
-0.06803134828805923,
-0.02499634027481079,
-0.03500702232122421,
-0.014460671693086624,
-0.11677601933479309,
-0.07945001125335693,
-0.028780734166502953,
0.04518447816371918,
-0.06937647610902786,
0.021355096250772476,
-0.1322164684534073,
0.023929066956043243,
-0.06582489609718323,
0.0462300069630146,
0.07549820840358734,
0.031221481040120125,
0.13683180510997772,
0.022086385637521744,
-0.05140222609043121,
-0.013891802169382572,
-0.026797059923410416,
0.004610102158039808,
0.06330741941928864,
-0.1024574413895607,
-0.0427311509847641,
0.04392293095588684,
-0.07547372579574585,
0.0027349675074219704,
-0.013312733732163906,
0.0064042010344564915,
-0.11464883387088776,
-0.08408223092556,
-0.03962205350399017,
-0.034142520278692245,
-0.012237684801220894,
-0.08914078772068024,
-0.018966661766171455,
0.027345558628439903,
-0.09918848425149918,
-0.03423536941409111,
-0.007173801772296429,
-0.018624387681484222,
0.04503766447305679,
0.02796376682817936,
0.0744314193725586,
0.03159952536225319,
0.02250215783715248,
-0.009596365503966808,
-0.03360338509082794,
0.05170294642448425,
0.03020341694355011,
0.030324921011924744,
0.12078339606523514,
-0.01807207614183426,
0.07713838666677475,
-0.08033224195241928,
0.0031779534183442593,
-0.014133784919977188,
0.031834643334150314,
0.04249202460050583,
0.11658605188131332,
-0.024996889755129814,
-0.025157922878861427,
-0.0021293454337865114,
0.007532926741987467,
0.012139758095145226,
-0.09104694426059723,
-0.030257003381848335,
0.019536761566996574,
0.02458350360393524,
-0.01972622238099575,
-0.06877396255731583,
0.002843182533979416,
-0.03441068157553673,
-0.0945558100938797,
0.05308283120393753,
0.002751727355644107,
-0.0023093249183148146,
-0.058512669056653976,
-0.020870689302682877,
-0.051480408757925034,
-0.06141463667154312,
0.09388959407806396,
-0.09833326935768127,
-0.05437225103378296,
-0.040184732526540756,
0.00676035787910223,
-0.012987547554075718,
-0.0705464631319046,
0.028947435319423676,
-0.08422571420669556,
-5.4260275567819536e-33,
0.08633355796337128,
0.06974141299724579,
0.054174479097127914,
0.022528689354658127,
-0.011753343977034092,
-0.03049388714134693,
0.08445971459150314,
0.15740199387073517,
0.040360648185014725,
0.012559968046844006,
0.009713291190564632,
-0.07867540419101715,
0.013955381698906422,
-0.018076086416840553,
0.09738410264253616,
0.03144017606973648,
-0.005952177569270134,
0.10431621223688126,
0.07060329616069794,
0.028050294145941734,
-0.02783256210386753,
-0.04572596773505211,
-0.05749082565307617,
0.08997207134962082,
-0.02454603835940361,
0.03860460966825485,
0.016184180974960327,
0.010195442475378513,
-0.024427294731140137,
-0.00673733139410615,
0.008780847303569317,
0.04149049147963524,
-0.05798695608973503,
0.07399201393127441,
-0.028825514018535614,
-0.028259359300136566,
-0.02355349436402321,
-0.005199824925512075,
-0.03300365433096886,
-0.03979065641760826,
0.09657958149909973,
0.03987368568778038,
-0.012318072840571404,
0.026232659816741943,
0.005642982665449381,
-0.06082672253251076,
-0.06114054098725319,
0.003813971998170018,
-0.019232913851737976,
-0.10985580086708069,
0.05895121395587921,
-0.051854416728019714,
-0.08816779404878616,
-0.03270112723112106,
-0.0597245879471302,
-0.03252538666129112,
0.08140794187784195,
-0.035650528967380524,
-0.06705879420042038,
-0.019763855263590813,
-0.08908168971538544,
-0.042337507009506226,
0.032442156225442886,
-0.037769582122564316,
-0.010324174538254738,
-0.09470603615045547,
0.05893784761428833,
0.0737401694059372,
0.032107286155223846,
0.0013113378081470728,
0.021514663472771645,
0.038626447319984436,
0.08247462660074234,
0.0007136919302865863,
0.06758565455675125,
0.0595955029129982,
-0.014868853613734245,
-0.013398206792771816,
0.0009986074874177575,
-0.028701242059469223,
-0.06781493127346039,
0.017722392454743385,
0.054298918694257736,
0.04473830759525299,
-0.06057014316320419,
0.025328153744339943,
-0.03513610363006592,
0.10656540095806122,
0.022606203332543373,
0.01225859671831131,
-0.016926152631640434,
0.018199363723397255,
0.026204025372862816,
0.07066427916288376,
-0.0009415831882506609,
-4.8625761195353334e-8,
-0.017145060002803802,
-0.02243589609861374,
-0.036484844982624054,
0.021059362217783928,
-0.09490630775690079,
-0.04366490617394447,
-0.05169818177819252,
-0.03994183614850044,
-0.02997077815234661,
-0.01805347017943859,
0.05649824067950249,
0.02146691456437111,
-0.08599753677845001,
0.016295569017529488,
-0.06659948825836182,
-0.005921523552387953,
-0.016450749710202217,
0.07809484750032425,
0.007703572977334261,
0.017244402319192886,
0.03326788172125816,
0.022818805649876595,
0.05332966893911362,
-0.04947267472743988,
-0.013059264048933983,
0.023461494594812393,
-0.07001624256372452,
0.06176796555519104,
-0.03842105343937874,
-0.04397924244403839,
0.009607551619410515,
-0.009877467527985573,
-0.03427828475832939,
0.04220769554376602,
-0.020765289664268494,
0.01918347366154194,
-0.042930588126182556,
-0.05245254188776016,
-0.04591929540038109,
0.05281402915716171,
0.09109613299369812,
-0.021714212372899055,
-0.1010773554444313,
-0.037418752908706665,
0.018480515107512474,
-0.005924237426370382,
0.03585749864578247,
-0.07592735439538956,
0.04829352721571922,
0.005049368366599083,
0.010273924097418785,
-0.09680752456188202,
-0.06850126385688782,
-0.020704906433820724,
-0.053583983331918716,
0.030412090942263603,
-0.03974415361881256,
-0.002227504039183259,
0.055073488503694534,
-0.0439068004488945,
0.0004797331930603832,
0.06487210094928741,
0.02245965786278248,
0.042935531586408615
] |
monologg/koelectra-base-v3-naver-ner | 7fe2d3297113e0753716d7f2c85d4880d288542d | 2020-11-30T11:55:35.000Z | [
"pytorch",
"electra",
"token-classification",
"transformers",
"autotrain_compatible"
] | token-classification | false | monologg | null | monologg/koelectra-base-v3-naver-ner | 1,911 | null | transformers | Entry not found | [
0.0461147278547287,
-0.038838207721710205,
-0.01049656979739666,
-0.03682169318199158,
0.011261860840022564,
0.013094935566186905,
0.0019101888174191117,
-0.013979103416204453,
0.027092741802334785,
-0.015212527476251125,
0.017284274101257324,
-0.08189476281404495,
0.03817418962717056,
-0.04920130595564842,
0.021389011293649673,
-0.015245908871293068,
-0.03203780576586723,
-0.1245758980512619,
0.03150877356529236,
0.032381657510995865,
-0.060957908630371094,
0.05409295856952667,
-0.025087490677833557,
0.01568586938083172,
0.028129950165748596,
-0.04710396006703377,
-0.018688226118683815,
0.013785239309072495,
-0.04001208767294884,
0.01173911802470684,
-0.04317743331193924,
0.05500618368387222,
0.004543041344732046,
0.02973111905157566,
0.14852192997932434,
0.02658126689493656,
0.02907961793243885,
-0.05169107764959335,
0.05803573504090309,
-0.07732241600751877,
-0.017637968063354492,
-0.04219653457403183,
0.041807834059000015,
0.023620979860424995,
0.021563321352005005,
0.016478516161441803,
-0.0021814992651343346,
-0.06400240957736969,
0.06393089145421982,
0.019599027931690216,
-0.08565037697553635,
0.00934905931353569,
-0.008718925528228283,
-0.028583496809005737,
-0.07310017943382263,
0.09416428208351135,
0.001759322709403932,
0.06184990331530571,
0.011840506456792355,
-0.035997264087200165,
0.08358278125524521,
-0.02619801089167595,
0.03736566752195358,
-0.028206506744027138,
-0.07454850524663925,
-0.08883563429117203,
-0.06279942393302917,
-0.008695344440639019,
0.014119276776909828,
-0.0825355276465416,
0.0649217739701271,
-0.00223911227658391,
-0.14716917276382446,
0.07743025571107864,
-0.03548373281955719,
-0.055201586335897446,
0.006981803569942713,
-0.012166670523583889,
0.055111464112997055,
-0.007116836030036211,
-0.023175746202468872,
-0.005835152696818113,
-0.09185640513896942,
0.055196937173604965,
0.034148022532463074,
0.03835180774331093,
0.038685429841279984,
-0.025987252593040466,
0.017804903909564018,
0.022428328171372414,
0.025005368515849113,
-0.10761535167694092,
-0.048001550137996674,
-0.04343584179878235,
0.012374646961688995,
-0.019502125680446625,
0.029218152165412903,
0.0842173621058464,
-0.011719699949026108,
0.09283553808927536,
-0.007015465293079615,
-0.03543110564351082,
-0.06936459988355637,
0.09425332397222519,
-0.010958523489534855,
-0.00805904995650053,
0.004974212497472763,
-0.0031528924591839314,
0.06105927750468254,
-0.03964288905262947,
-0.03619541600346565,
-0.019901901483535767,
0.07134733349084854,
0.039514873176813126,
-0.012729483656585217,
-0.006646515801548958,
-0.04746140539646149,
-0.014432490803301334,
-0.05157482624053955,
0.09506245702505112,
-0.049747664481401443,
-0.04591796174645424,
-0.008965466171503067,
-0.0325421579182148,
-0.08626784384250641,
-0.06624380499124527,
0.02538885548710823,
-4.303924894057984e-33,
0.01133066974580288,
0.0033434738870710135,
-0.002155609894543886,
0.04871906340122223,
-0.023564351722598076,
-0.07933273911476135,
0.0600903145968914,
0.02335330657660961,
-0.03844716399908066,
-0.020433755591511726,
-0.06952055543661118,
-0.03235611692070961,
0.0062485747039318085,
0.064804308116436,
-0.03201229125261307,
0.061689723283052444,
0.0417000837624073,
-0.00761845987290144,
0.03340127319097519,
-0.047770582139492035,
0.00887306872755289,
-0.04066338762640953,
-0.010506896302103996,
0.0106519665569067,
0.021333497017621994,
0.12854498624801636,
-0.009705503471195698,
0.010055632330477238,
-0.017507633194327354,
0.006515394430607557,
0.06334009766578674,
-0.057817306369543076,
0.013668818399310112,
-0.020286159589886665,
0.05430467426776886,
-0.023184705525636673,
0.0828516036272049,
0.0005449643940664828,
-0.10372652113437653,
-0.07634282112121582,
-0.005381610710173845,
-0.039263784885406494,
0.0006114727002568543,
-0.013281986117362976,
0.07119110971689224,
0.043696220964193344,
0.03168422728776932,
0.04338686540722847,
0.05728672817349434,
0.0832006186246872,
-0.07961414009332657,
0.015234283171594143,
0.017002005130052567,
0.047004107385873795,
-0.09794387966394424,
0.004990279674530029,
-0.07062993198633194,
-0.028000490739941597,
-0.04018733277916908,
-0.0702052190899849,
0.011351344175636768,
0.06020182743668556,
-0.03297270089387894,
0.09396500885486603,
0.03417910635471344,
-0.019825750961899757,
-0.034690454602241516,
-0.013036907650530338,
0.05896938592195511,
-0.012359356507658958,
-0.017275206744670868,
-0.07982361316680908,
0.02059139870107174,
0.06737419217824936,
0.04176458343863487,
-0.04978838190436363,
-0.05877475067973137,
-0.06289287656545639,
-0.03354167565703392,
-0.03871942684054375,
0.009898529388010502,
-0.05514208599925041,
-0.11629002541303635,
-0.011855563148856163,
0.10663620382547379,
0.037354156374931335,
-0.0065480442717671394,
-0.051189567893743515,
0.06663123518228531,
0.01874656230211258,
0.032841797918081284,
0.041593004018068314,
-0.06879369914531708,
0.04216769337654114,
-0.01628219522535801,
5.4139394340936695e-34,
0.05697013810276985,
-0.006972255185246468,
0.015711724758148193,
-0.17956365644931793,
0.02320219948887825,
0.007923615165054798,
-0.008062449283897877,
0.0074974060989916325,
0.07391711324453354,
0.0309313777834177,
0.060510627925395966,
0.058605875819921494,
0.09515274316072464,
-0.002282935893163085,
0.001603541080839932,
0.07024981826543808,
0.012629246339201927,
0.07425693422555923,
-0.038426291197538376,
0.01861148327589035,
0.030608950182795525,
-0.02449394389986992,
0.021528491750359535,
-0.003039651783183217,
-0.03676343336701393,
0.03130284696817398,
0.07998586446046829,
0.010451192036271095,
-0.07930229604244232,
-0.013543923385441303,
0.018781835213303566,
0.05168003588914871,
-0.07191970944404602,
0.15783067047595978,
0.026191607117652893,
0.01262354850769043,
0.08218053728342056,
-0.029807550832629204,
-0.07528624683618546,
-0.04250097647309303,
0.017244765534996986,
0.04411793500185013,
0.03708017244935036,
0.009233047254383564,
-0.040271829813718796,
0.022496428340673447,
0.02495843544602394,
0.07633638381958008,
0.005147108342498541,
0.013892097398638725,
0.05610476806759834,
-0.06684739887714386,
0.05862557515501976,
-0.020688841119408607,
0.05377643182873726,
0.06718500703573227,
0.005329249892383814,
-0.01388032827526331,
0.029931528493762016,
0.009508464485406876,
-0.045173756778240204,
0.11534366756677628,
-0.06510116159915924,
0.05117698386311531,
-0.0026125339791178703,
-0.08554837852716446,
-0.03784770518541336,
0.0804959163069725,
0.011298024095594883,
-0.07695550471544266,
-0.04868878796696663,
0.02515520341694355,
0.06252261996269226,
-0.04509226232767105,
-0.01246943511068821,
0.028559505939483643,
-0.030573077499866486,
0.05066261067986488,
-0.08187384903430939,
0.04469604790210724,
0.0034051244147121906,
0.04145054519176483,
-0.021858664229512215,
-0.06112268194556236,
-0.00908052921295166,
-0.05903250351548195,
0.0259539932012558,
0.059690944850444794,
-0.07613514363765717,
-0.03720718249678612,
-0.036316655576229095,
0.07058046013116837,
-0.008224100805819035,
0.041961874812841415,
-0.0285952128469944,
-1.496900736697171e-8,
-0.0014124972512945533,
0.03401879221200943,
-0.040338415652513504,
0.04116074740886688,
0.0935964286327362,
-0.05115952715277672,
0.0008746005478315055,
-0.03389839455485344,
-0.00567849725484848,
-0.010686947964131832,
-0.04789939522743225,
-0.04820054769515991,
-0.02011880651116371,
-0.03209094703197479,
-0.04211259260773659,
-0.10229527950286865,
-0.07819421589374542,
-0.031228765845298767,
-0.02154778689146042,
-0.04960230365395546,
0.08087796717882156,
-0.07801242172718048,
0.06919731199741364,
-0.04999840259552002,
0.03687043860554695,
0.03889009356498718,
-0.049989692866802216,
-0.04254625365138054,
-0.04606937617063522,
0.08682432025671005,
-0.031148413196206093,
0.11826753616333008,
0.034102488309144974,
-0.0208592489361763,
-0.0205202866345644,
0.027134142816066742,
0.09741277992725372,
0.051608603447675705,
0.013477512635290623,
-0.13649295270442963,
-0.022304272279143333,
0.02385953813791275,
0.038732077926397324,
-0.09249968826770782,
-0.04549082741141319,
0.054220106452703476,
0.01160438358783722,
0.051190607249736786,
0.07713303714990616,
-0.022097084671258926,
-0.06127818301320076,
-0.01857956498861313,
0.006740490905940533,
-0.00496308971196413,
0.024095389991998672,
0.0736224576830864,
-0.003481915919110179,
-0.0699305310845375,
-0.006629763171076775,
-0.0598808117210865,
0.05297163128852844,
-0.02902800403535366,
-0.027858933433890343,
-0.01287526823580265
] |
hf-internal-testing/tiny-random-rembert | 917e1c9f997b17fc81d9ed84713f5de8abe57c1b | 2022-03-08T13:50:53.000Z | [
"pytorch",
"tf",
"rembert",
"feature-extraction",
"transformers",
"generated_from_keras_callback",
"model-index"
] | feature-extraction | false | hf-internal-testing | null | hf-internal-testing/tiny-random-rembert | 1,909 | null | transformers | ---
tags:
- generated_from_keras_callback
model-index:
- name: tiny-random-rembert
results: []
---
<!-- This model card has been generated automatically according to the information Keras had access to. You should
probably proofread and complete it, then remove this comment. -->
# tiny-random-rembert
This model was trained from scratch on an unknown dataset.
It achieves the following results on the evaluation set:
## Model description
More information needed
## Intended uses & limitations
More information needed
## Training and evaluation data
More information needed
## Training procedure
### Training hyperparameters
The following hyperparameters were used during training:
- optimizer: None
- training_precision: float32
### Training results
### Framework versions
- Transformers 4.18.0.dev0
- TensorFlow 2.7.0
- Datasets 1.18.3
- Tokenizers 0.11.0
| [
-0.03651832044124603,
-0.0006451349472627044,
0.009294786490499973,
0.0647704154253006,
0.034709081053733826,
0.007055279333144426,
0.0009832663927227259,
-0.03898831084370613,
-0.07697777450084686,
-0.09333143383264542,
0.04877287894487381,
-0.06799846142530441,
-0.017806848511099815,
-0.07218224555253983,
-0.1722366213798523,
-0.02507605217397213,
0.06267532706260681,
-0.041093725711107254,
-0.09724234789609909,
-0.018124110996723175,
0.04606951028108597,
0.06861347705125809,
0.0019370084628462791,
0.04217591881752014,
0.019842760637402534,
-0.04483821615576744,
0.01573764532804489,
0.03695687651634216,
0.05400178208947182,
-0.042179983109235764,
0.06122935935854912,
0.033176928758621216,
-0.030910400673747063,
0.0002121903235092759,
0.0505533367395401,
0.060481395572423935,
-0.03187599033117294,
-0.011029648594558239,
0.05563224479556084,
0.010806649923324585,
0.03140650689601898,
-0.09597361832857132,
-0.040396835654973984,
0.03842809423804283,
0.03611307963728905,
-0.006243366748094559,
-0.02387148141860962,
-0.06479164212942123,
-0.057182006537914276,
-0.022460969164967537,
-0.02000066637992859,
-0.03181373327970505,
0.0009668500279076397,
-0.011409666389226913,
-0.046204276382923126,
-0.007873881608247757,
-0.021654466167092323,
-0.05993819981813431,
0.011308527551591396,
-0.02560761198401451,
-0.0017638218123465776,
-0.030201027169823647,
-0.013591259717941284,
-0.015928441658616066,
-0.045432377606630325,
-0.06427064538002014,
-0.00424881000071764,
-0.05524429306387901,
0.07444500178098679,
-0.10236911475658417,
0.11207713186740875,
0.07406125962734222,
-0.012034377083182335,
0.09911517798900604,
0.07314382493495941,
0.020573090761899948,
0.03263741359114647,
0.0031501345802098513,
0.05739162489771843,
-0.013657618314027786,
-0.0027967907954007387,
-0.06434024125337601,
0.01529996283352375,
-0.008868958801031113,
0.005522458348423243,
-0.03326454386115074,
0.0641581267118454,
0.011541820131242275,
0.10144485533237457,
-0.07793860882520676,
-0.03187080845236778,
0.01186313759535551,
-0.04896498844027519,
0.017451340332627296,
0.0480312742292881,
0.014463691972196102,
-0.03870958834886551,
-0.009765563532710075,
-0.0549871064722538,
0.07927002757787704,
0.0005210047820582986,
0.035562582314014435,
0.0665055438876152,
0.051045700907707214,
0.0034590130671858788,
0.05300673097372055,
0.047051284462213516,
0.06347999721765518,
0.07789722830057144,
-0.03759126737713814,
0.0311661995947361,
0.02724088355898857,
-0.017405616119503975,
0.011523962020874023,
0.0739792063832283,
0.08121538162231445,
-0.06175601854920387,
-0.0014577475376427174,
-0.09441937506198883,
0.06806901842355728,
-0.07035453617572784,
0.021585775539278984,
-0.060668736696243286,
-0.035269107669591904,
-0.03896789997816086,
0.03986028954386711,
-0.08289951831102371,
6.042942787798829e-33,
0.06543289124965668,
0.025392599403858185,
0.0058987862430512905,
-0.042812954634428024,
0.07016193866729736,
-0.03363047167658806,
0.06868163496255875,
-0.051675666123628616,
-0.0054049366153776646,
0.005713785067200661,
-0.11644255369901657,
-0.030139431357383728,
-0.023327946662902832,
0.05878913402557373,
-0.024018585681915283,
-0.020196842029690742,
-0.023192549124360085,
-0.01633383147418499,
0.02461603656411171,
0.0497216135263443,
0.07073371112346649,
0.09668636322021484,
-0.009383434429764748,
-0.11493206024169922,
-0.052255891263484955,
0.03929970785975456,
0.022221095860004425,
-0.02236403338611126,
-0.009026415646076202,
0.044144872575998306,
-0.09537598490715027,
0.030582556501030922,
0.047477882355451584,
-0.02240091748535633,
-0.015399991534650326,
0.018009230494499207,
0.053523678332567215,
0.033827219158411026,
-0.04045182093977928,
-0.08106078207492828,
0.06465451419353485,
0.023115897551178932,
0.015053106471896172,
-0.1334443837404251,
-0.06062778830528259,
-0.05556739494204521,
0.03449437767267227,
0.03646838292479515,
0.04525623098015785,
0.029573049396276474,
-0.018268320709466934,
0.02580169588327408,
0.03005414642393589,
-0.034114740788936615,
-0.07299398630857468,
0.0015032184310257435,
0.028717881068587303,
0.08418527245521545,
0.024680273607373238,
0.0001785173371899873,
-0.0263191070407629,
-0.0007834975840523839,
-0.003368214936926961,
0.0524168498814106,
-0.00013528020645026118,
-0.028940018266439438,
0.021203046664595604,
-0.03086329624056816,
0.02710708975791931,
0.03689626231789589,
-0.10266672819852829,
0.020663518458604813,
-0.05493221431970596,
-0.038487330079078674,
0.06424377113580704,
-0.08048903942108154,
0.04754623770713806,
0.006317362189292908,
-0.035818930715322495,
-0.027955222874879837,
0.012417195364832878,
0.06964249163866043,
-0.017530933022499084,
-0.03664811700582504,
-0.054911766201257706,
-0.08901096880435944,
-0.03121892549097538,
-0.02435705065727234,
-0.004353006836026907,
-0.0021907477639615536,
-0.00011744568473659456,
0.002717845141887665,
-0.05174056440591812,
0.007505054585635662,
-0.06968123465776443,
-6.87275180069313e-33,
-0.026417579501867294,
0.07684178650379181,
-0.00884493999183178,
0.05398843437433243,
-0.0037853382527828217,
0.013823660090565681,
0.028004372492432594,
0.14050275087356567,
-0.07034897059202194,
-0.03419366478919983,
0.0355251170694828,
0.012334833852946758,
-0.01716437190771103,
-0.0700889304280281,
0.04829341918230057,
0.04010837897658348,
-0.07261272519826889,
-0.032802700996398926,
0.02895423397421837,
0.018334606662392616,
0.007344095502048731,
0.10354795306921005,
-0.07087486982345581,
0.06306826323270798,
-0.002751575782895088,
0.03157231956720352,
0.05558904632925987,
0.09698456525802612,
0.09663404524326324,
-0.07867583632469177,
-0.02675376459956169,
-0.03014550358057022,
-0.035727329552173615,
0.0336320661008358,
0.03570710867643356,
0.016572769731283188,
0.08576793223619461,
-0.0331617034971714,
0.04932669550180435,
0.06420840322971344,
0.02284805104136467,
0.11087774485349655,
-0.04411940649151802,
0.05381046608090401,
-0.01835019513964653,
0.009970669634640217,
0.017569471150636673,
-0.01604948751628399,
-0.014082489535212517,
-0.05699926242232323,
-0.001961587928235531,
-0.038787178695201874,
-0.12956158816814423,
-0.01547335833311081,
-0.07513604313135147,
-0.024750985205173492,
-0.02527255192399025,
0.061613284051418304,
0.02817651815712452,
0.08707563579082489,
-0.05740406736731529,
-0.06503332406282425,
-0.03953001648187637,
-0.10097551345825195,
-0.022918863222002983,
0.00781261920928955,
-0.09241020679473877,
0.031922440975904465,
-0.003252966096624732,
0.06832001358270645,
0.012728403322398663,
0.04574919864535332,
0.01783725619316101,
-0.005630875006318092,
-0.04431178793311119,
-0.05468524247407913,
-0.06488960236310959,
-0.018783096224069595,
0.032912738621234894,
-0.08086974918842316,
0.03903426602482796,
-0.023536765947937965,
0.007297603413462639,
0.01855548284947872,
0.1301685869693756,
0.03969800844788551,
0.0856151208281517,
0.08004282414913177,
0.007428277283906937,
-0.0006967770168557763,
-0.0027226691599935293,
-0.011895723640918732,
0.0339430533349514,
0.16033518314361572,
-0.03917129710316658,
-6.328985335812831e-8,
0.049906447529792786,
0.0434095673263073,
0.004603215493261814,
0.10599258542060852,
-0.00529418233782053,
0.010952957905828953,
0.03706180676817894,
0.03519119322299957,
-0.04083569720387459,
-0.047977957874536514,
0.0429210439324379,
-0.004755291156470776,
-0.06724389642477036,
0.004656727891415358,
0.05854128673672676,
0.025553107261657715,
-0.0901101678609848,
0.062131840735673904,
-0.025149671360850334,
-0.07373826950788498,
-0.003089288715273142,
-0.027609631419181824,
0.005975998938083649,
-0.024728447198867798,
0.014456924051046371,
-0.045900166034698486,
0.022784244269132614,
0.09744833409786224,
-0.07920479774475098,
-0.010423104278743267,
-0.028986552730202675,
0.037318695336580276,
-0.014556469395756721,
-0.0034731775522232056,
-0.042607467621564865,
0.1305159628391266,
-0.020111260935664177,
-0.00034529142430983484,
0.05508365109562874,
-0.023426951840519905,
-0.07913440465927124,
0.02867499180138111,
-0.02707586996257305,
-0.033196210861206055,
-0.015817951411008835,
-0.03919906169176102,
-0.014799566939473152,
-0.0878564640879631,
0.10295133292675018,
-0.026212777942419052,
-0.0038578021340072155,
-0.025411086156964302,
-0.06089997664093971,
0.005826466251164675,
0.01065769512206316,
-0.012722323648631573,
-0.06006893888115883,
-0.0468047596514225,
0.04117472469806671,
-0.032367825508117676,
0.07339277863502502,
0.01636052317917347,
-0.03285519406199455,
-0.0025617065839469433
] |
textattack/bert-base-uncased-MNLI | 3a97f689528cbd91bcc71ab29ea6c20c089d8f28 | 2021-05-20T07:31:58.000Z | [
"pytorch",
"jax",
"bert",
"text-classification",
"transformers"
] | text-classification | false | textattack | null | textattack/bert-base-uncased-MNLI | 1,908 | null | transformers | Entry not found | [
0.0461147278547287,
-0.038838207721710205,
-0.01049656979739666,
-0.03682169318199158,
0.011261860840022564,
0.013094935566186905,
0.0019101888174191117,
-0.013979103416204453,
0.027092741802334785,
-0.015212527476251125,
0.017284274101257324,
-0.08189476281404495,
0.03817418962717056,
-0.04920130595564842,
0.021389011293649673,
-0.015245908871293068,
-0.03203780576586723,
-0.1245758980512619,
0.03150877356529236,
0.032381657510995865,
-0.060957908630371094,
0.05409295856952667,
-0.025087490677833557,
0.01568586938083172,
0.028129950165748596,
-0.04710396006703377,
-0.018688226118683815,
0.013785239309072495,
-0.04001208767294884,
0.01173911802470684,
-0.04317743331193924,
0.05500618368387222,
0.004543041344732046,
0.02973111905157566,
0.14852192997932434,
0.02658126689493656,
0.02907961793243885,
-0.05169107764959335,
0.05803573504090309,
-0.07732241600751877,
-0.017637968063354492,
-0.04219653457403183,
0.041807834059000015,
0.023620979860424995,
0.021563321352005005,
0.016478516161441803,
-0.0021814992651343346,
-0.06400240957736969,
0.06393089145421982,
0.019599027931690216,
-0.08565037697553635,
0.00934905931353569,
-0.008718925528228283,
-0.028583496809005737,
-0.07310017943382263,
0.09416428208351135,
0.001759322709403932,
0.06184990331530571,
0.011840506456792355,
-0.035997264087200165,
0.08358278125524521,
-0.02619801089167595,
0.03736566752195358,
-0.028206506744027138,
-0.07454850524663925,
-0.08883563429117203,
-0.06279942393302917,
-0.008695344440639019,
0.014119276776909828,
-0.0825355276465416,
0.0649217739701271,
-0.00223911227658391,
-0.14716917276382446,
0.07743025571107864,
-0.03548373281955719,
-0.055201586335897446,
0.006981803569942713,
-0.012166670523583889,
0.055111464112997055,
-0.007116836030036211,
-0.023175746202468872,
-0.005835152696818113,
-0.09185640513896942,
0.055196937173604965,
0.034148022532463074,
0.03835180774331093,
0.038685429841279984,
-0.025987252593040466,
0.017804903909564018,
0.022428328171372414,
0.025005368515849113,
-0.10761535167694092,
-0.048001550137996674,
-0.04343584179878235,
0.012374646961688995,
-0.019502125680446625,
0.029218152165412903,
0.0842173621058464,
-0.011719699949026108,
0.09283553808927536,
-0.007015465293079615,
-0.03543110564351082,
-0.06936459988355637,
0.09425332397222519,
-0.010958523489534855,
-0.00805904995650053,
0.004974212497472763,
-0.0031528924591839314,
0.06105927750468254,
-0.03964288905262947,
-0.03619541600346565,
-0.019901901483535767,
0.07134733349084854,
0.039514873176813126,
-0.012729483656585217,
-0.006646515801548958,
-0.04746140539646149,
-0.014432490803301334,
-0.05157482624053955,
0.09506245702505112,
-0.049747664481401443,
-0.04591796174645424,
-0.008965466171503067,
-0.0325421579182148,
-0.08626784384250641,
-0.06624380499124527,
0.02538885548710823,
-4.303924894057984e-33,
0.01133066974580288,
0.0033434738870710135,
-0.002155609894543886,
0.04871906340122223,
-0.023564351722598076,
-0.07933273911476135,
0.0600903145968914,
0.02335330657660961,
-0.03844716399908066,
-0.020433755591511726,
-0.06952055543661118,
-0.03235611692070961,
0.0062485747039318085,
0.064804308116436,
-0.03201229125261307,
0.061689723283052444,
0.0417000837624073,
-0.00761845987290144,
0.03340127319097519,
-0.047770582139492035,
0.00887306872755289,
-0.04066338762640953,
-0.010506896302103996,
0.0106519665569067,
0.021333497017621994,
0.12854498624801636,
-0.009705503471195698,
0.010055632330477238,
-0.017507633194327354,
0.006515394430607557,
0.06334009766578674,
-0.057817306369543076,
0.013668818399310112,
-0.020286159589886665,
0.05430467426776886,
-0.023184705525636673,
0.0828516036272049,
0.0005449643940664828,
-0.10372652113437653,
-0.07634282112121582,
-0.005381610710173845,
-0.039263784885406494,
0.0006114727002568543,
-0.013281986117362976,
0.07119110971689224,
0.043696220964193344,
0.03168422728776932,
0.04338686540722847,
0.05728672817349434,
0.0832006186246872,
-0.07961414009332657,
0.015234283171594143,
0.017002005130052567,
0.047004107385873795,
-0.09794387966394424,
0.004990279674530029,
-0.07062993198633194,
-0.028000490739941597,
-0.04018733277916908,
-0.0702052190899849,
0.011351344175636768,
0.06020182743668556,
-0.03297270089387894,
0.09396500885486603,
0.03417910635471344,
-0.019825750961899757,
-0.034690454602241516,
-0.013036907650530338,
0.05896938592195511,
-0.012359356507658958,
-0.017275206744670868,
-0.07982361316680908,
0.02059139870107174,
0.06737419217824936,
0.04176458343863487,
-0.04978838190436363,
-0.05877475067973137,
-0.06289287656545639,
-0.03354167565703392,
-0.03871942684054375,
0.009898529388010502,
-0.05514208599925041,
-0.11629002541303635,
-0.011855563148856163,
0.10663620382547379,
0.037354156374931335,
-0.0065480442717671394,
-0.051189567893743515,
0.06663123518228531,
0.01874656230211258,
0.032841797918081284,
0.041593004018068314,
-0.06879369914531708,
0.04216769337654114,
-0.01628219522535801,
5.4139394340936695e-34,
0.05697013810276985,
-0.006972255185246468,
0.015711724758148193,
-0.17956365644931793,
0.02320219948887825,
0.007923615165054798,
-0.008062449283897877,
0.0074974060989916325,
0.07391711324453354,
0.0309313777834177,
0.060510627925395966,
0.058605875819921494,
0.09515274316072464,
-0.002282935893163085,
0.001603541080839932,
0.07024981826543808,
0.012629246339201927,
0.07425693422555923,
-0.038426291197538376,
0.01861148327589035,
0.030608950182795525,
-0.02449394389986992,
0.021528491750359535,
-0.003039651783183217,
-0.03676343336701393,
0.03130284696817398,
0.07998586446046829,
0.010451192036271095,
-0.07930229604244232,
-0.013543923385441303,
0.018781835213303566,
0.05168003588914871,
-0.07191970944404602,
0.15783067047595978,
0.026191607117652893,
0.01262354850769043,
0.08218053728342056,
-0.029807550832629204,
-0.07528624683618546,
-0.04250097647309303,
0.017244765534996986,
0.04411793500185013,
0.03708017244935036,
0.009233047254383564,
-0.040271829813718796,
0.022496428340673447,
0.02495843544602394,
0.07633638381958008,
0.005147108342498541,
0.013892097398638725,
0.05610476806759834,
-0.06684739887714386,
0.05862557515501976,
-0.020688841119408607,
0.05377643182873726,
0.06718500703573227,
0.005329249892383814,
-0.01388032827526331,
0.029931528493762016,
0.009508464485406876,
-0.045173756778240204,
0.11534366756677628,
-0.06510116159915924,
0.05117698386311531,
-0.0026125339791178703,
-0.08554837852716446,
-0.03784770518541336,
0.0804959163069725,
0.011298024095594883,
-0.07695550471544266,
-0.04868878796696663,
0.02515520341694355,
0.06252261996269226,
-0.04509226232767105,
-0.01246943511068821,
0.028559505939483643,
-0.030573077499866486,
0.05066261067986488,
-0.08187384903430939,
0.04469604790210724,
0.0034051244147121906,
0.04145054519176483,
-0.021858664229512215,
-0.06112268194556236,
-0.00908052921295166,
-0.05903250351548195,
0.0259539932012558,
0.059690944850444794,
-0.07613514363765717,
-0.03720718249678612,
-0.036316655576229095,
0.07058046013116837,
-0.008224100805819035,
0.041961874812841415,
-0.0285952128469944,
-1.496900736697171e-8,
-0.0014124972512945533,
0.03401879221200943,
-0.040338415652513504,
0.04116074740886688,
0.0935964286327362,
-0.05115952715277672,
0.0008746005478315055,
-0.03389839455485344,
-0.00567849725484848,
-0.010686947964131832,
-0.04789939522743225,
-0.04820054769515991,
-0.02011880651116371,
-0.03209094703197479,
-0.04211259260773659,
-0.10229527950286865,
-0.07819421589374542,
-0.031228765845298767,
-0.02154778689146042,
-0.04960230365395546,
0.08087796717882156,
-0.07801242172718048,
0.06919731199741364,
-0.04999840259552002,
0.03687043860554695,
0.03889009356498718,
-0.049989692866802216,
-0.04254625365138054,
-0.04606937617063522,
0.08682432025671005,
-0.031148413196206093,
0.11826753616333008,
0.034102488309144974,
-0.0208592489361763,
-0.0205202866345644,
0.027134142816066742,
0.09741277992725372,
0.051608603447675705,
0.013477512635290623,
-0.13649295270442963,
-0.022304272279143333,
0.02385953813791275,
0.038732077926397324,
-0.09249968826770782,
-0.04549082741141319,
0.054220106452703476,
0.01160438358783722,
0.051190607249736786,
0.07713303714990616,
-0.022097084671258926,
-0.06127818301320076,
-0.01857956498861313,
0.006740490905940533,
-0.00496308971196413,
0.024095389991998672,
0.0736224576830864,
-0.003481915919110179,
-0.0699305310845375,
-0.006629763171076775,
-0.0598808117210865,
0.05297163128852844,
-0.02902800403535366,
-0.027858933433890343,
-0.01287526823580265
] |
google/mt5-xxl | d4ac5e6d5125f8d30cba8763cd0ad71e5d34c17b | 2022-05-27T15:06:56.000Z | [
"pytorch",
"tf",
"mt5",
"text2text-generation",
"multilingual",
"af",
"am",
"ar",
"az",
"be",
"bg",
"bn",
"ca",
"ceb",
"co",
"cs",
"cy",
"da",
"de",
"el",
"en",
"eo",
"es",
"et",
"eu",
"fa",
"fi",
"fil",
"fr",
"fy",
"ga",
"gd",
"gl",
"gu",
"ha",
"haw",
"hi",
"hmn",
"ht",
"hu",
"hy",
"ig",
"is",
"it",
"iw",
"ja",
"jv",
"ka",
"kk",
"km",
"kn",
"ko",
"ku",
"ky",
"la",
"lb",
"lo",
"lt",
"lv",
"mg",
"mi",
"mk",
"ml",
"mn",
"mr",
"ms",
"mt",
"my",
"ne",
"nl",
"no",
"ny",
"pa",
"pl",
"ps",
"pt",
"ro",
"ru",
"sd",
"si",
"sk",
"sl",
"sm",
"sn",
"so",
"sq",
"sr",
"st",
"su",
"sv",
"sw",
"ta",
"te",
"tg",
"th",
"tr",
"uk",
"und",
"ur",
"uz",
"vi",
"xh",
"yi",
"yo",
"zh",
"zu",
"dataset:mc4",
"arxiv:2010.11934",
"transformers",
"license:apache-2.0",
"autotrain_compatible"
] | text2text-generation | false | google | null | google/mt5-xxl | 1,906 | 8 | transformers | ---
language:
- multilingual
- af
- am
- ar
- az
- be
- bg
- bn
- ca
- ceb
- co
- cs
- cy
- da
- de
- el
- en
- eo
- es
- et
- eu
- fa
- fi
- fil
- fr
- fy
- ga
- gd
- gl
- gu
- ha
- haw
- hi
- hmn
- ht
- hu
- hy
- ig
- is
- it
- iw
- ja
- jv
- ka
- kk
- km
- kn
- ko
- ku
- ky
- la
- lb
- lo
- lt
- lv
- mg
- mi
- mk
- ml
- mn
- mr
- ms
- mt
- my
- ne
- nl
- no
- ny
- pa
- pl
- ps
- pt
- ro
- ru
- sd
- si
- sk
- sl
- sm
- sn
- so
- sq
- sr
- st
- su
- sv
- sw
- ta
- te
- tg
- th
- tr
- uk
- und
- ur
- uz
- vi
- xh
- yi
- yo
- zh
- zu
datasets:
- mc4
license: apache-2.0
---
[Google's mT5](https://github.com/google-research/multilingual-t5)
mT5 is pretrained on the [mC4](https://www.tensorflow.org/datasets/catalog/c4#c4multilingual) corpus, covering 101 languages:
Afrikaans, Albanian, Amharic, Arabic, Armenian, Azerbaijani, Basque, Belarusian, Bengali, Bulgarian, Burmese, Catalan, Cebuano, Chichewa, Chinese, Corsican, Czech, Danish, Dutch, English, Esperanto, Estonian, Filipino, Finnish, French, Galician, Georgian, German, Greek, Gujarati, Haitian Creole, Hausa, Hawaiian, Hebrew, Hindi, Hmong, Hungarian, Icelandic, Igbo, Indonesian, Irish, Italian, Japanese, Javanese, Kannada, Kazakh, Khmer, Korean, Kurdish, Kyrgyz, Lao, Latin, Latvian, Lithuanian, Luxembourgish, Macedonian, Malagasy, Malay, Malayalam, Maltese, Maori, Marathi, Mongolian, Nepali, Norwegian, Pashto, Persian, Polish, Portuguese, Punjabi, Romanian, Russian, Samoan, Scottish Gaelic, Serbian, Shona, Sindhi, Sinhala, Slovak, Slovenian, Somali, Sotho, Spanish, Sundanese, Swahili, Swedish, Tajik, Tamil, Telugu, Thai, Turkish, Ukrainian, Urdu, Uzbek, Vietnamese, Welsh, West Frisian, Xhosa, Yiddish, Yoruba, Zulu.
**Note**: mT5 was only pre-trained on mC4 excluding any supervised training. Therefore, this model has to be fine-tuned before it is useable on a downstream task.
Pretraining Dataset: [mC4](https://www.tensorflow.org/datasets/catalog/c4#c4multilingual)
Other Community Checkpoints: [here](https://huggingface.co/models?search=mt5)
Paper: [mT5: A massively multilingual pre-trained text-to-text transformer](https://arxiv.org/abs/2010.11934)
Authors: *Linting Xue, Noah Constant, Adam Roberts, Mihir Kale, Rami Al-Rfou, Aditya Siddhant, Aditya Barua, Colin Raffel*
## Abstract
The recent "Text-to-Text Transfer Transformer" (T5) leveraged a unified text-to-text format and scale to attain state-of-the-art results on a wide variety of English-language NLP tasks. In this paper, we introduce mT5, a multilingual variant of T5 that was pre-trained on a new Common Crawl-based dataset covering 101 languages. We describe the design and modified training of mT5 and demonstrate its state-of-the-art performance on many multilingual benchmarks. All of the code and model checkpoints used in this work are publicly available. | [
-0.08226951956748962,
0.05192454159259796,
-0.016503630205988884,
-0.09610544890165329,
0.06950594484806061,
-0.05049009621143341,
0.05426013469696045,
-0.043981023132801056,
-0.01146115455776453,
0.004685561638325453,
0.12800902128219604,
-0.053880609571933746,
0.06841910630464554,
-0.06570184975862503,
-0.057674944400787354,
-0.0013009844115003943,
-0.05389399453997612,
0.04419076070189476,
-0.05483875051140785,
-0.004046211950480938,
0.0073880041018128395,
-0.010940438136458397,
0.01667744107544422,
0.00952968094497919,
0.03960338234901428,
0.10661116242408752,
-0.06073056533932686,
0.0827154889702797,
0.03343956172466278,
-0.09017574042081833,
-0.0011138860136270523,
0.09719036519527435,
0.060916878283023834,
0.017047910019755363,
0.09357113391160965,
0.052383407950401306,
-0.05059842765331268,
-0.03020942397415638,
-0.056635383516550064,
0.005089709535241127,
0.07504089921712875,
-0.026256386190652847,
0.05258495360612869,
-0.001976985950022936,
0.01068537775427103,
-0.010172707028687,
0.006030503660440445,
-0.06620049476623535,
0.03360452875494957,
-0.01641184650361538,
-0.08662446588277817,
0.06118741258978844,
-0.07349595427513123,
0.031867027282714844,
-0.06241359934210777,
-0.08172176033258438,
-0.06838659197092056,
0.0674639567732811,
0.07017546147108078,
0.046055398881435394,
-0.05119737237691879,
0.013286932371556759,
-0.09187904745340347,
0.06714498996734619,
-0.027266452088952065,
-0.004266867879778147,
0.05302584916353226,
-0.025762775912880898,
-0.06248275190591812,
0.04067865386605263,
0.031319379806518555,
-0.00838304590433836,
-0.07063866406679153,
0.09988468140363693,
-0.03511491045355797,
0.0714864730834961,
0.02362125925719738,
0.007606879808008671,
-0.014948847703635693,
-0.06433169543743134,
0.01354188658297062,
-0.02238389104604721,
0.10109997540712357,
-0.03373122587800026,
-0.024004042148590088,
0.011627874337136745,
-0.01733003370463848,
0.019437912851572037,
0.049975063651800156,
-0.04660605639219284,
-0.012942076660692692,
0.012130456045269966,
-0.006176701746881008,
0.06014304980635643,
-0.09442616999149323,
0.06977616250514984,
-0.009427439421415329,
0.07728400081396103,
-0.021725235506892204,
0.10221806913614273,
0.05835402384400368,
0.006102845072746277,
0.020591821521520615,
-0.031848758459091187,
-0.0419524684548378,
-0.027866890653967857,
-0.04597426950931549,
0.11782439053058624,
0.03180980682373047,
-0.014605610631406307,
-0.024843750521540642,
0.008602677844464779,
-0.016399361193180084,
-0.07798462361097336,
-0.025021182373166084,
0.013461777940392494,
-0.059679847210645676,
-0.025139303877949715,
0.04873678460717201,
0.05331956595182419,
-0.033771973103284836,
-0.04190007969737053,
-0.0833921879529953,
-0.07550914585590363,
-0.05121512711048126,
-0.01999840885400772,
-0.012782692909240723,
-5.177575070792155e-33,
0.047543082386255264,
-0.02607608772814274,
0.019410325214266777,
-0.07196234166622162,
0.01080375537276268,
-0.07022661715745926,
-0.04414408281445503,
-0.014508184045553207,
-0.12656724452972412,
0.001085383351892233,
-0.013839338906109333,
-0.0010521075455471873,
-0.06194690242409706,
0.026530306786298752,
0.03457600250840187,
-0.010319623164832592,
0.0756610780954361,
-0.034429118037223816,
0.004918399266898632,
0.056022197008132935,
0.02169242687523365,
0.08023496717214584,
0.03726128861308098,
-0.02719949185848236,
-0.06473927944898605,
0.06178782880306244,
-0.004193208646029234,
-0.05213300138711929,
0.0024899388663470745,
0.05306767672300339,
-0.04179225489497185,
-0.012370132841169834,
-0.07599841803312302,
-0.04848992079496384,
-0.010885324329137802,
-0.05308421328663826,
-0.02930128201842308,
-0.024629559367895126,
-0.04874396324157715,
-0.07331804186105728,
-0.06298509985208511,
-0.013800329528748989,
-0.05801979824900627,
0.008073466829955578,
-0.006712800357490778,
0.020526010543107986,
-0.022033000364899635,
-0.04620474949479103,
-0.0021668109111487865,
0.07867113500833511,
-0.034411605447530746,
-0.0088359909132123,
-0.015667052939534187,
0.03038167394697666,
-0.02661776915192604,
0.06920995563268661,
-0.014758666977286339,
0.018130648881196976,
0.033689241856336594,
0.02300318144261837,
-0.0014624020550400019,
0.046865444630384445,
0.015077435411512852,
0.040411219000816345,
0.09609732031822205,
-0.08579211682081223,
0.011583329178392887,
-0.03252565115690231,
0.006047783885151148,
0.0630653128027916,
-0.051646143198013306,
-0.05259852856397629,
0.07511261850595474,
0.1112237349152565,
0.023712050169706345,
-0.04496588930487633,
0.014943128451704979,
-0.04338112100958824,
0.0458889938890934,
0.04351869598031044,
-0.06638592481613159,
-0.002034885808825493,
0.0018859489355236292,
-0.08784838020801544,
-0.07015493512153625,
-0.000751463056076318,
0.03540247306227684,
-0.03321869298815727,
-0.02278093993663788,
-0.006122150458395481,
-0.11412569135427475,
0.07255146652460098,
-0.0492815338075161,
-0.08647774904966354,
-0.12023685872554779,
1.4029451608667075e-33,
-0.05966714397072792,
0.06163305789232254,
-0.0013074759626761079,
0.0737469270825386,
-0.06516411155462265,
-0.010741688311100006,
0.10451854020357132,
0.08959180861711502,
0.00855121947824955,
0.02887588180601597,
0.09541132301092148,
-0.04202093183994293,
0.06007642298936844,
-0.07749076932668686,
0.10692419111728668,
-0.014502192847430706,
-0.031121380627155304,
0.03265605494379997,
0.0017593915108591318,
0.06715693324804306,
-0.07051373273134232,
0.09467417001724243,
-0.08750158548355103,
0.11446143686771393,
0.0042797052301466465,
-0.04467932879924774,
0.0212510097771883,
0.03234926611185074,
0.030432045459747314,
0.02003355883061886,
0.053889911621809006,
-0.009858638048171997,
-0.0711526870727539,
0.017228445038199425,
-0.0018322690157219768,
-0.035425424575805664,
-0.036619916558265686,
0.08421974629163742,
0.0127663379535079,
0.05410563573241234,
-0.009932374581694603,
0.03086979314684868,
0.011450721882283688,
-0.058614786714315414,
0.011420962400734425,
-0.017519233748316765,
-0.024143872782588005,
0.00046923471381887794,
-0.03393923491239548,
-0.03125164285302162,
0.10358507931232452,
0.06307535618543625,
-0.04204455390572548,
-0.015571961179375648,
0.05310972034931183,
-0.01870930939912796,
-0.014143908396363258,
-0.04841592162847519,
-0.09805329889059067,
-0.021160781383514404,
-0.02040218748152256,
-0.1011844053864479,
-0.08104423433542252,
-0.044632766395807266,
0.07938779145479202,
0.0397975780069828,
-0.0105733098462224,
-0.020173203200101852,
0.03728258237242699,
-0.02675710618495941,
0.02452203258872032,
-0.06348703056573868,
-0.05482710525393486,
-0.04174099490046501,
0.038104914128780365,
-0.03746628761291504,
-0.026550497859716415,
0.07309646904468536,
-0.01575443707406521,
-0.055388033390045166,
0.03279346227645874,
0.03140338137745857,
0.03518225997686386,
0.021301692351698875,
0.029349278658628464,
-0.06607211381196976,
-0.09337961673736572,
0.004805712960660458,
0.033978644758462906,
0.0004536047053989023,
-0.017808105796575546,
0.022589797154068947,
-0.054385747760534286,
0.14296457171440125,
-0.0355200320482254,
-4.981630752354249e-8,
-0.04394590109586716,
-0.03739868849515915,
-0.01823284476995468,
0.04959499090909958,
0.07390817254781723,
0.028114179149270058,
-0.045683249831199646,
-0.0591815710067749,
0.024307236075401306,
-0.015005465596914291,
0.08971016854047775,
-0.02177094854414463,
-0.1302589774131775,
-0.008974679745733738,
-0.0548921599984169,
0.043083395808935165,
-0.013364007696509361,
0.116261787712574,
-0.011118242517113686,
-0.01243581809103489,
-0.0008205277263186872,
0.015747113153338432,
0.038751233369112015,
-0.03704372048377991,
0.01997259445488453,
0.044191211462020874,
0.033992599695920944,
0.05888907611370087,
0.005006789229810238,
0.010494234040379524,
-0.033356647938489914,
-0.052566271275281906,
-0.02608330361545086,
-0.04685505852103233,
-0.009877425618469715,
-0.004835542757064104,
-0.044064730405807495,
-0.020079581066966057,
0.009862438775599003,
0.030157405883073807,
0.016809897497296333,
0.09873920679092407,
0.0230304803699255,
0.03444686904549599,
0.022682776674628258,
0.009789573028683662,
0.007757692597806454,
0.058846063911914825,
0.027345245704054832,
-0.013102594763040543,
-0.013480603694915771,
0.022732805460691452,
-0.04916395619511604,
-0.03948116675019264,
0.042752355337142944,
-0.017884233966469765,
0.08941526710987091,
-0.028881100937724113,
-0.02080703340470791,
0.012945747934281826,
0.07268215715885162,
0.021486755460500717,
0.05497001111507416,
-0.013256281614303589
] |
ml6team/keyphrase-extraction-kbir-inspec | 70c7250d0cb932f4ee3332c50a73583b7cd7995d | 2022-06-16T14:51:11.000Z | [
"pytorch",
"roberta",
"token-classification",
"en",
"dataset:midas/inspec",
"arxiv:2112.08547",
"transformers",
"keyphrase-extraction",
"license:mit",
"model-index",
"autotrain_compatible"
] | token-classification | false | ml6team | null | ml6team/keyphrase-extraction-kbir-inspec | 1,906 | 2 | transformers | ---
language: en
license: mit
tags:
- keyphrase-extraction
datasets:
- midas/inspec
metrics:
- seqeval
widget:
- text: "Keyphrase extraction is a technique in text analysis where you extract the important keyphrases from a document.
Thanks to these keyphrases humans can understand the content of a text very quickly and easily without reading
it completely. Keyphrase extraction was first done primarily by human annotators, who read the text in detail
and then wrote down the most important keyphrases. The disadvantage is that if you work with a lot of documents,
this process can take a lot of time.
Here is where Artificial Intelligence comes in. Currently, classical machine learning methods, that use statistical
and linguistic features, are widely used for the extraction process. Now with deep learning, it is possible to capture
the semantic meaning of a text even better than these classical methods. Classical methods look at the frequency,
occurrence and order of words in the text, whereas these neural approaches can capture long-term semantic dependencies
and context of words in a text."
example_title: "Example 1"
- text: "In this work, we explore how to learn task specific language models aimed towards learning rich representation of keyphrases from text documents. We experiment with different masking strategies for pre-training transformer language models (LMs) in discriminative as well as generative settings. In the discriminative setting, we introduce a new pre-training objective - Keyphrase Boundary Infilling with Replacement (KBIR), showing large gains in performance (up to 9.26 points in F1) over SOTA, when LM pre-trained using KBIR is fine-tuned for the task of keyphrase extraction. In the generative setting, we introduce a new pre-training setup for BART - KeyBART, that reproduces the keyphrases related to the input text in the CatSeq format, instead of the denoised original input. This also led to gains in performance (up to 4.33 points inF1@M) over SOTA for keyphrase generation. Additionally, we also fine-tune the pre-trained language models on named entity recognition(NER), question answering (QA), relation extraction (RE), abstractive summarization and achieve comparable performance with that of the SOTA, showing that learning rich representation of keyphrases is indeed beneficial for many other fundamental NLP tasks."
example_title: "Example 2"
model-index:
- name: DeDeckerThomas/keyphrase-extraction-kbir-inspec
results:
- task:
type: keyphrase-extraction
name: Keyphrase Extraction
dataset:
type: midas/inspec
name: inspec
metrics:
- type: F1 (Seqeval)
value: 0.588
name: F1 (Seqeval)
- type: F1@M
value: 0.564
name: F1@M
---
# 🔑 Keyphrase Extraction Model: KBIR-inspec
Keyphrase extraction is a technique in text analysis where you extract the important keyphrases from a document. Thanks to these keyphrases humans can understand the content of a text very quickly and easily without reading it completely. Keyphrase extraction was first done primarily by human annotators, who read the text in detail and then wrote down the most important keyphrases. The disadvantage is that if you work with a lot of documents, this process can take a lot of time ⏳.
Here is where Artificial Intelligence 🤖 comes in. Currently, classical machine learning methods, that use statistical and linguistic features, are widely used for the extraction process. Now with deep learning, it is possible to capture the semantic meaning of a text even better than these classical methods. Classical methods look at the frequency, occurrence and order of words in the text, whereas these neural approaches can capture long-term semantic dependencies and context of words in a text.
## 📓 Model Description
This model uses [KBIR](https://huggingface.co/bloomberg/KBIR) as its base model and fine-tunes it on the [Inspec dataset](https://huggingface.co/datasets/midas/inspec). KBIR or Keyphrase Boundary Infilling with Replacement is a pre-trained model which utilizes a multi-task learning setup for optimizing a combined loss of Masked Language Modeling (MLM), Keyphrase Boundary Infilling (KBI) and Keyphrase Replacement Classification (KRC).
You can find more information about the architecture in this [paper](https://arxiv.org/abs/2112.08547).
Keyphrase extraction models are transformer models fine-tuned as a token classification problem where each word in the document is classified as being part of a keyphrase or not.
| Label | Description |
| ----- | ------------------------------- |
| B-KEY | At the beginning of a keyphrase |
| I-KEY | Inside a keyphrase |
| O | Outside a keyphrase |
Kulkarni, Mayank, Debanjan Mahata, Ravneet Arora, and Rajarshi Bhowmik. "Learning Rich Representation of Keyphrases from Text." arXiv preprint arXiv:2112.08547 (2021).
Sahrawat, Dhruva, Debanjan Mahata, Haimin Zhang, Mayank Kulkarni, Agniv Sharma, Rakesh Gosangi, Amanda Stent, Yaman Kumar, Rajiv Ratn Shah, and Roger Zimmermann. "Keyphrase extraction as sequence labeling using contextualized embeddings." In European Conference on Information Retrieval, pp. 328-335. Springer, Cham, 2020.
## ✋ Intended Uses & Limitations
### 🛑 Limitations
* This keyphrase extraction model is very domain-specific and will perform very well on abstracts of scientific papers. It's not recommended to use this model for other domains, but you are free to test it out.
* Only works for English documents.
* For a custom model, please consult the [training notebook]() for more information.
### ❓ How To Use
```python
from transformers import (
TokenClassificationPipeline,
AutoModelForTokenClassification,
AutoTokenizer,
)
from transformers.pipelines import AggregationStrategy
import numpy as np
# Define keyphrase extraction pipeline
class KeyphraseExtractionPipeline(TokenClassificationPipeline):
def __init__(self, model, *args, **kwargs):
super().__init__(
model=AutoModelForTokenClassification.from_pretrained(model),
tokenizer=AutoTokenizer.from_pretrained(model),
*args,
**kwargs
)
def postprocess(self, model_outputs):
results = super().postprocess(
model_outputs=model_outputs,
aggregation_strategy=AggregationStrategy.SIMPLE,
)
return np.unique([result.get("word").strip() for result in results])
```
```python
# Load pipeline
model_name = "ml6team/keyphrase-extraction-kbir-inspec"
extractor = KeyphraseExtractionPipeline(model=model_name)
```
```python
# Inference
text = """
Keyphrase extraction is a technique in text analysis where you extract the
important keyphrases from a document. Thanks to these keyphrases humans can
understand the content of a text very quickly and easily without reading it
completely. Keyphrase extraction was first done primarily by human annotators,
who read the text in detail and then wrote down the most important keyphrases.
The disadvantage is that if you work with a lot of documents, this process
can take a lot of time.
Here is where Artificial Intelligence comes in. Currently, classical machine
learning methods, that use statistical and linguistic features, are widely used
for the extraction process. Now with deep learning, it is possible to capture
the semantic meaning of a text even better than these classical methods.
Classical methods look at the frequency, occurrence and order of words
in the text, whereas these neural approaches can capture long-term
semantic dependencies and context of words in a text.
""".replace("\n", " ")
keyphrases = extractor(text)
print(keyphrases)
```
```
# Output
['Artificial Intelligence' 'Keyphrase extraction' 'deep learning'
'linguistic features' 'machine learning' 'semantic meaning'
'text analysis']
```
## 📚 Training Dataset
[Inspec](https://huggingface.co/datasets/midas/inspec) is a keyphrase extraction/generation dataset consisting of 2000 English scientific papers from the scientific domains of Computers and Control and Information Technology published between 1998 to 2002. The keyphrases are annotated by professional indexers or editors.
You can find more information in the [paper](https://dl.acm.org/doi/10.3115/1119355.1119383).
## 👷♂️ Training Procedure
For more in detail information, you can take a look at the [training notebook]().
### Training Parameters
| Parameter | Value |
| --------- | ------|
| Learning Rate | 1e-4 |
| Epochs | 50 |
| Early Stopping Patience | 3 |
### Preprocessing
The documents in the dataset are already preprocessed into list of words with the corresponding labels. The only thing that must be done is tokenization and the realignment of the labels so that they correspond with the right subword tokens.
```python
from datasets import load_dataset
from transformers import AutoTokenizer
# Labels
label_list = ["B", "I", "O"]
lbl2idx = {"B": 0, "I": 1, "O": 2}
idx2label = {0: "B", 1: "I", 2: "O"}
# Tokenizer
tokenizer = AutoTokenizer.from_pretrained("bloomberg/KBIR", add_prefix_space=True)
max_length = 512
# Dataset parameters
dataset_full_name = "midas/inspec"
dataset_subset = "raw"
dataset_document_column = "document"
dataset_biotags_column = "doc_bio_tags"
def preprocess_fuction(all_samples_per_split):
tokenized_samples = tokenizer.batch_encode_plus(
all_samples_per_split[dataset_document_column],
padding="max_length",
truncation=True,
is_split_into_words=True,
max_length=max_length,
)
total_adjusted_labels = []
for k in range(0, len(tokenized_samples["input_ids"])):
prev_wid = -1
word_ids_list = tokenized_samples.word_ids(batch_index=k)
existing_label_ids = all_samples_per_split[dataset_biotags_column][k]
i = -1
adjusted_label_ids = []
for wid in word_ids_list:
if wid is None:
adjusted_label_ids.append(lbl2idx["O"])
elif wid != prev_wid:
i = i + 1
adjusted_label_ids.append(lbl2idx[existing_label_ids[i]])
prev_wid = wid
else:
adjusted_label_ids.append(
lbl2idx[
f"{'I' if existing_label_ids[i] == 'B' else existing_label_ids[i]}"
]
)
total_adjusted_labels.append(adjusted_label_ids)
tokenized_samples["labels"] = total_adjusted_labels
return tokenized_samples
# Load dataset
dataset = load_dataset(dataset_full_name, dataset_subset)
# Preprocess dataset
tokenized_dataset = dataset.map(preprocess_fuction, batched=True)
```
### Postprocessing (Without Pipeline Function)
If you do not use the pipeline function, you must filter out the B and I labeled tokens. Each B and I will then be merged into a keyphrase. Finally, you need to strip the keyphrases to make sure all unnecessary spaces have been removed.
```python
# Define post_process functions
def concat_tokens_by_tag(keyphrases):
keyphrase_tokens = []
for id, label in keyphrases:
if label == "B":
keyphrase_tokens.append([id])
elif label == "I":
if len(keyphrase_tokens) > 0:
keyphrase_tokens[len(keyphrase_tokens) - 1].append(id)
return keyphrase_tokens
def extract_keyphrases(example, predictions, tokenizer, index=0):
keyphrases_list = [
(id, idx2label[label])
for id, label in zip(
np.array(example["input_ids"]).squeeze().tolist(), predictions[index]
)
if idx2label[label] in ["B", "I"]
]
processed_keyphrases = concat_tokens_by_tag(keyphrases_list)
extracted_kps = tokenizer.batch_decode(
processed_keyphrases,
skip_special_tokens=True,
clean_up_tokenization_spaces=True,
)
return np.unique([kp.strip() for kp in extracted_kps])
```
## 📝 Evaluation Results
Traditional evaluation methods are the precision, recall and F1-score @k,m where k is the number that stands for the first k predicted keyphrases and m for the average amount of predicted keyphrases.
The model achieves the following results on the Inspec test set:
| Dataset | P@5 | R@5 | F1@5 | P@10 | R@10 | F1@10 | P@M | R@M | F1@M |
|:-----------------:|:----:|:----:|:----:|:----:|:----:|:-----:|:----:|:----:|:----:|
| Inspec Test Set | 0.53 | 0.47 | 0.46 | 0.36 | 0.58 | 0.41 | 0.58 | 0.60 | 0.56 |
For more information on the evaluation process, you can take a look at the keyphrase extraction [evaluation notebook]().
## 🚨 Issues
Please feel free to start discussions in the Community Tab. | [
-0.0805460587143898,
0.008236224763095379,
0.03966188058257103,
-0.014175022952258587,
0.05817509815096855,
-0.007063322234898806,
0.04605871066451073,
0.04151735082268715,
0.06610295176506042,
-0.037019308656454086,
-0.02522243745625019,
0.05210624635219574,
0.05790720880031586,
-0.029039304703474045,
0.0029369040858000517,
0.028191957622766495,
0.05112295597791672,
0.020484987646341324,
-0.13807184994220734,
-0.09506358951330185,
0.09094663709402084,
0.005835050716996193,
0.02205318957567215,
-0.0374906063079834,
0.02843804471194744,
0.04001888260245323,
-0.01038367673754692,
0.02134895883500576,
-0.005798927042633295,
0.02458486706018448,
0.050934795290231705,
0.019210873171687126,
0.08018460869789124,
0.08462654054164886,
-0.043986618518829346,
0.09084440022706985,
-0.04151653125882149,
0.03644193708896637,
0.020643480122089386,
-0.03690282627940178,
-0.022791264578700066,
-0.0496419258415699,
-0.02059258334338665,
0.08834567666053772,
0.1185024157166481,
0.013433110900223255,
-0.079208143055439,
0.0009472669335082173,
-0.06629351526498795,
0.06894034147262573,
-0.17855983972549438,
0.04684600979089737,
-0.01184922456741333,
0.09607923030853271,
-0.03729381412267685,
0.05112900212407112,
-0.04551848769187927,
-0.03766149282455444,
-0.005291033070534468,
-0.06920194625854492,
0.0032001789659261703,
-0.07829999178647995,
0.01778949797153473,
0.0087387440726161,
-0.03575962036848068,
-0.05151093006134033,
0.0032459001522511244,
0.026779290288686752,
0.050121404230594635,
-0.008717399090528488,
0.05039451643824577,
0.058497410267591476,
0.013597344979643822,
0.0020418111234903336,
-0.04486696049571037,
0.004404130857437849,
-0.004876621067523956,
-0.00862627662718296,
0.0011373397428542376,
-0.05841110274195671,
0.018329840153455734,
0.004933347459882498,
0.11374778300523758,
0.08893048018217087,
0.03391208127140999,
-0.03704696521162987,
0.0397678017616272,
0.005320803262293339,
-0.05843105539679527,
0.04830486327409744,
0.003408692078664899,
-0.1250826120376587,
0.0949932262301445,
-0.0969439446926117,
0.08134022355079651,
0.040958747267723083,
-0.02536883018910885,
-0.1011764407157898,
0.03173643350601196,
0.07514237612485886,
0.009898502379655838,
0.07854684442281723,
-0.047661229968070984,
-0.1307535320520401,
-0.038364965468645096,
0.02177155204117298,
0.05268042907118797,
-0.020751813426613808,
0.05095198005437851,
-0.06660298258066177,
0.006512987427413464,
0.0290488563477993,
-0.039375189691782,
-0.06155719980597496,
0.04133988544344902,
-0.01196722500026226,
0.08685406297445297,
-0.00055987189989537,
0.0851379930973053,
0.07084199786186218,
-0.09487603604793549,
0.021688377484679222,
-0.00018646322132553905,
-0.017867712303996086,
-0.08877038955688477,
0.019322821870446205,
-0.035973574966192245,
5.5077313936618106e-33,
-0.015891363844275475,
0.013331733644008636,
-0.013446436263620853,
0.02226676419377327,
-0.09146186709403992,
-0.03934468328952789,
-0.0347818024456501,
-0.018042150884866714,
-0.09654757380485535,
-0.0006069045048207045,
0.03303227946162224,
0.010920621454715729,
-0.019357869401574135,
0.04002232849597931,
0.014244460500776768,
-0.03843625634908676,
-0.07370655238628387,
0.012233254499733448,
0.012320449575781822,
-0.03010452352464199,
0.03426497429609299,
-0.04044496640563011,
0.07437747716903687,
0.010585196316242218,
0.02014586329460144,
-0.016479237005114555,
0.05000642314553261,
-0.05808519944548607,
0.045671429485082626,
-0.0002340019855182618,
-0.016223590821027756,
-0.01582295261323452,
-0.03564434498548508,
-0.003871204098686576,
0.022515971213579178,
-0.020336110144853592,
0.00006794351793359965,
-0.01376278791576624,
0.010206375271081924,
-0.05821315199136734,
-0.05536464974284172,
0.005019964650273323,
0.05766046419739723,
-0.07706371694803238,
-0.022002706304192543,
0.007805423811078072,
-0.05693626403808594,
-0.03523954749107361,
0.07214104384183884,
-0.04403824359178543,
0.0029724561609327793,
0.000852600613143295,
0.0016684160800650716,
-0.05281843990087509,
0.01873171515762806,
0.08118259161710739,
0.024603165686130524,
0.01134015154093504,
0.028249768540263176,
-0.007352898828685284,
-0.04668763279914856,
-0.007978756912052631,
0.0088689299300313,
0.10009736567735672,
0.09801416099071503,
0.0267080906778574,
-0.006157508119940758,
0.0889093354344368,
0.02346513606607914,
-0.02091524377465248,
0.008757178671658039,
-0.021083462983369827,
-0.04009556770324707,
-0.04537111520767212,
0.028592383489012718,
0.030731556937098503,
-0.003692355938255787,
-0.10002878308296204,
-0.03171895071864128,
0.07793295383453369,
-0.01602942682802677,
-0.022161317989230156,
0.03848741576075554,
-0.02626180648803711,
-0.05415879562497139,
0.03707919642329216,
0.0005096224485896528,
-0.11423955112695694,
0.0710616484284401,
-0.010501290671527386,
-0.011601010337471962,
-0.0018718434730544686,
-0.08889487385749817,
-0.03702152147889137,
-0.05911986157298088,
-6.0875494923346734e-33,
-0.005313302390277386,
-0.04558006674051285,
-0.069138303399086,
0.06205340474843979,
0.022630469873547554,
0.005239446181803942,
-0.06760691851377487,
0.04834872484207153,
0.014812037348747253,
-0.024106349796056747,
-0.04915378987789154,
-0.0001895133755169809,
0.004346942529082298,
-0.057133469730615616,
0.0155134666711092,
-0.03773724287748337,
0.019391309469938278,
-0.01109821442514658,
-0.011480811983346939,
0.11266443133354187,
-0.01600414514541626,
-0.040158115327358246,
-0.07412898540496826,
0.05282500758767128,
0.0006452851230278611,
0.013473720289766788,
0.0051395404152572155,
0.07990201562643051,
-0.049337342381477356,
-0.04716874286532402,
-0.04040319100022316,
0.03761672228574753,
-0.09684503823518753,
-0.006923265289515257,
-0.08703769743442535,
-0.02688409760594368,
-0.020347900688648224,
-0.024957051500678062,
-0.04523633420467377,
0.01709498092532158,
0.056615542620420456,
0.054358769208192825,
-0.026705091819167137,
-0.10263726115226746,
-0.05332646891474724,
-0.049452029168605804,
-0.09661856293678284,
0.030548198148608208,
-0.002441172720864415,
0.011979417875409126,
0.0492822527885437,
-0.040686603635549545,
-0.08301178365945816,
-0.06411584466695786,
-0.030713537707924843,
-0.07523219287395477,
0.0023033968172967434,
-0.005435564089566469,
-0.039580781012773514,
0.022116905078291893,
-0.037807732820510864,
0.019168850034475327,
0.0312834233045578,
0.06666545569896698,
0.015820009633898735,
-0.05587188899517059,
-0.04775804281234741,
0.04036436602473259,
-0.10504120588302612,
-0.027931666001677513,
0.10851497203111649,
-0.04161826893687248,
0.009849951602518559,
0.04654518887400627,
0.009393529035151005,
-0.016256436705589294,
0.02205149456858635,
-0.026945820078253746,
-0.07112101465463638,
-0.11547897756099701,
0.0846400260925293,
-0.055806100368499756,
-0.011199413798749447,
0.043367043137550354,
0.02871469222009182,
0.04982481151819229,
0.011814062483608723,
0.014777195639908314,
0.018336940556764603,
0.003313927911221981,
-0.009008137509226799,
0.0072765774093568325,
-0.07306713610887527,
0.07534796744585037,
-0.05611306056380272,
-5.446825568355962e-8,
-0.03177030757069588,
0.055660199373960495,
-0.04311208426952362,
0.021998686715960503,
-0.031880080699920654,
-0.055951010435819626,
0.04194924980401993,
0.09203530102968216,
-0.016203250735998154,
-0.0667034238576889,
0.06604813039302826,
-0.0036869579926133156,
-0.11720463633537292,
-0.02980741299688816,
-0.014857017435133457,
0.12959924340248108,
0.06382300704717636,
0.029311558231711388,
0.05135051906108856,
-0.0315222293138504,
0.06561063230037689,
0.01892685703933239,
-0.005019431002438068,
0.02752542868256569,
0.04630458727478981,
0.016741974279284477,
-0.04497503489255905,
0.09085632115602493,
0.019637705758213997,
-0.010269547812640667,
0.03834155946969986,
0.01420969981700182,
-0.023189691826701164,
-0.045040473341941833,
0.11175977438688278,
0.08963073790073395,
0.028576385229825974,
-0.06648615747690201,
0.0005806541885249317,
0.03682245314121246,
0.021032851189374924,
0.11536245048046112,
-0.046656470745801926,
-0.026586245745420456,
-0.04086773470044136,
-0.04271315038204193,
-0.023606104776263237,
0.036804597824811935,
0.07366049289703369,
0.04700087010860443,
-0.005900242831557989,
-0.04766570404171944,
-0.027532363310456276,
0.00864469911903143,
-0.01805882900953293,
0.07487476617097855,
0.08460487425327301,
0.00037899913149885833,
0.04823409020900726,
0.008421110920608044,
0.06902813166379929,
0.02758648805320263,
0.021750258281826973,
0.027155239135026932
] |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.