+
+
+
+
+
+
+
+
+
+结果并不是非常好,但是已经出现了一些可以被认出来的数字!您可以尝试训练更长时间(例如,10或20个epoch),并调整模型配置、学习率、优化器等。此外,如果您想尝试稍微困难一点的数据集,您可以尝试一下fashionMNIST,只需要一行代码的替换就可以了。
+
+## 与 DDPM 做比较
+
+在本节中,我们将看看我们的“玩具”实现与其他笔记本中使用的基于DDPM论文的方法有何不同([扩散器简介](https://github.com/huggingface/diffusion-models-class/blob/main/unit1/01_introduction_to_diffusers.ipynb))。
+
+我们将会看到的
+
+* 模型的表现受限于随迭代周期(timesteps)变化的控制条件,在前向传到中时间步(t)是作为一个参数被传入的
+* 有很多不同的取样策略可选择,可能会比我们上面所使用的最简单的版本更好
+* diffusers`UNet2DModel`比我们的BasicUNet更先进
+* 损坏过程的处理方式不同
+* 训练目标不同,包括预测噪声而不是去噪图像
+* 该模型通过调节timestep来调节噪声水平, 其中t作为一个附加参数传入前向过程中。
+* 有许多不同的采样策略可供选择,它们应该比我们上面简单的版本更有效。
+
+自DDPM论文发表以来,已经有人提出了许多改进建议,但这个例子对于不同的可用设计决策具有指导意义。读完这篇文章后,你可能会想要深入了解这篇论文['Elucidating the Design Space of Diffusion-Based Generative Models'](https://arxiv.org/abs/2206.00364)它对所有这些组件进行了详细的探讨,并就如何获得最佳性能提出了新的建议。
+
+如果你觉得这些内容对你来说太过深奥了,请不要担心!你可以随意跳过本笔记本的其余部分或将其保存以备不时之需。
+
+### UNet
+
+diffusers中的UNet2DModel模型比上述基本UNet模型有许多改进:
+
+* GroupNorm层对每个blocks的输入进行了组标准化(group normalization)
+* Dropout层能使训练更平滑
+* 每个块有多个resnet层(如果layers_per_block未设置为1)
+* 注意机制(通常仅用于输入分辨率较低的blocks)
+* timestep的调节。
+* 具有可学习参数的下采样和上采样块
+
+让我们来创建并仔细研究一下UNet2DModel:
+
+
+```python
+model = UNet2DModel(
+ sample_size=28, # the target image resolution
+ in_channels=1, # the number of input channels, 3 for RGB images
+ out_channels=1, # the number of output channels
+ layers_per_block=2, # how many ResNet layers to use per UNet block
+ block_out_channels=(32, 64, 64), # Roughly matching our basic unet example
+ down_block_types=(
+ "DownBlock2D", # a regular ResNet downsampling block
+ "AttnDownBlock2D", # a ResNet downsampling block with spatial self-attention
+ "AttnDownBlock2D",
+ ),
+ up_block_types=(
+ "AttnUpBlock2D",
+ "AttnUpBlock2D", # a ResNet upsampling block with spatial self-attention
+ "UpBlock2D", # a regular ResNet upsampling block
+ ),
+)
+print(model)
+```
+
+ UNet2DModel(
+ (conv_in): Conv2d(1, 32, kernel_size=(3, 3), stride=(1, 1), padding=(1, 1))
+ (time_proj): Timesteps()
+ (time_embedding): TimestepEmbedding(
+ (linear_1): Linear(in_features=32, out_features=128, bias=True)
+ (act): SiLU()
+ (linear_2): Linear(in_features=128, out_features=128, bias=True)
+ )
+ (down_blocks): ModuleList(
+ (0): DownBlock2D(
+ (resnets): ModuleList(
+ (0): ResnetBlock2D(
+ (norm1): GroupNorm(32, 32, eps=1e-05, affine=True)
+ (conv1): Conv2d(32, 32, kernel_size=(3, 3), stride=(1, 1), padding=(1, 1))
+ (time_emb_proj): Linear(in_features=128, out_features=32, bias=True)
+ (norm2): GroupNorm(32, 32, eps=1e-05, affine=True)
+ (dropout): Dropout(p=0.0, inplace=False)
+ (conv2): Conv2d(32, 32, kernel_size=(3, 3), stride=(1, 1), padding=(1, 1))
+ (nonlinearity): SiLU()
+ )
+ (1): ResnetBlock2D(
+ (norm1): GroupNorm(32, 32, eps=1e-05, affine=True)
+ (conv1): Conv2d(32, 32, kernel_size=(3, 3), stride=(1, 1), padding=(1, 1))
+ (time_emb_proj): Linear(in_features=128, out_features=32, bias=True)
+ (norm2): GroupNorm(32, 32, eps=1e-05, affine=True)
+ (dropout): Dropout(p=0.0, inplace=False)
+ (conv2): Conv2d(32, 32, kernel_size=(3, 3), stride=(1, 1), padding=(1, 1))
+ (nonlinearity): SiLU()
+ )
+ )
+ (downsamplers): ModuleList(
+ (0): Downsample2D(
+ (conv): Conv2d(32, 32, kernel_size=(3, 3), stride=(2, 2), padding=(1, 1))
+ )
+ )
+ )
+ (1): AttnDownBlock2D(
+ (attentions): ModuleList(
+ (0): AttentionBlock(
+ (group_norm): GroupNorm(32, 64, eps=1e-05, affine=True)
+ (query): Linear(in_features=64, out_features=64, bias=True)
+ (key): Linear(in_features=64, out_features=64, bias=True)
+ (value): Linear(in_features=64, out_features=64, bias=True)
+ (proj_attn): Linear(in_features=64, out_features=64, bias=True)
+ )
+ (1): AttentionBlock(
+ (group_norm): GroupNorm(32, 64, eps=1e-05, affine=True)
+ (query): Linear(in_features=64, out_features=64, bias=True)
+ (key): Linear(in_features=64, out_features=64, bias=True)
+ (value): Linear(in_features=64, out_features=64, bias=True)
+ (proj_attn): Linear(in_features=64, out_features=64, bias=True)
+ )
+ )
+ (resnets): ModuleList(
+ (0): ResnetBlock2D(
+ (norm1): GroupNorm(32, 32, eps=1e-05, affine=True)
+ (conv1): Conv2d(32, 64, kernel_size=(3, 3), stride=(1, 1), padding=(1, 1))
+ (time_emb_proj): Linear(in_features=128, out_features=64, bias=True)
+ (norm2): GroupNorm(32, 64, eps=1e-05, affine=True)
+ (dropout): Dropout(p=0.0, inplace=False)
+ (conv2): Conv2d(64, 64, kernel_size=(3, 3), stride=(1, 1), padding=(1, 1))
+ (nonlinearity): SiLU()
+ (conv_shortcut): Conv2d(32, 64, kernel_size=(1, 1), stride=(1, 1))
+ )
+ (1): ResnetBlock2D(
+ (norm1): GroupNorm(32, 64, eps=1e-05, affine=True)
+ (conv1): Conv2d(64, 64, kernel_size=(3, 3), stride=(1, 1), padding=(1, 1))
+ (time_emb_proj): Linear(in_features=128, out_features=64, bias=True)
+ (norm2): GroupNorm(32, 64, eps=1e-05, affine=True)
+ (dropout): Dropout(p=0.0, inplace=False)
+ (conv2): Conv2d(64, 64, kernel_size=(3, 3), stride=(1, 1), padding=(1, 1))
+ (nonlinearity): SiLU()
+ )
+ )
+ (downsamplers): ModuleList(
+ (0): Downsample2D(
+ (conv): Conv2d(64, 64, kernel_size=(3, 3), stride=(2, 2), padding=(1, 1))
+ )
+ )
+ )
+ (2): AttnDownBlock2D(
+ (attentions): ModuleList(
+ (0): AttentionBlock(
+ (group_norm): GroupNorm(32, 64, eps=1e-05, affine=True)
+ (query): Linear(in_features=64, out_features=64, bias=True)
+ (key): Linear(in_features=64, out_features=64, bias=True)
+ (value): Linear(in_features=64, out_features=64, bias=True)
+ (proj_attn): Linear(in_features=64, out_features=64, bias=True)
+ )
+ (1): AttentionBlock(
+ (group_norm): GroupNorm(32, 64, eps=1e-05, affine=True)
+ (query): Linear(in_features=64, out_features=64, bias=True)
+ (key): Linear(in_features=64, out_features=64, bias=True)
+ (value): Linear(in_features=64, out_features=64, bias=True)
+ (proj_attn): Linear(in_features=64, out_features=64, bias=True)
+ )
+ )
+ (resnets): ModuleList(
+ (0): ResnetBlock2D(
+ (norm1): GroupNorm(32, 64, eps=1e-05, affine=True)
+ (conv1): Conv2d(64, 64, kernel_size=(3, 3), stride=(1, 1), padding=(1, 1))
+ (time_emb_proj): Linear(in_features=128, out_features=64, bias=True)
+ (norm2): GroupNorm(32, 64, eps=1e-05, affine=True)
+ (dropout): Dropout(p=0.0, inplace=False)
+ (conv2): Conv2d(64, 64, kernel_size=(3, 3), stride=(1, 1), padding=(1, 1))
+ (nonlinearity): SiLU()
+ )
+ (1): ResnetBlock2D(
+ (norm1): GroupNorm(32, 64, eps=1e-05, affine=True)
+ (conv1): Conv2d(64, 64, kernel_size=(3, 3), stride=(1, 1), padding=(1, 1))
+ (time_emb_proj): Linear(in_features=128, out_features=64, bias=True)
+ (norm2): GroupNorm(32, 64, eps=1e-05, affine=True)
+ (dropout): Dropout(p=0.0, inplace=False)
+ (conv2): Conv2d(64, 64, kernel_size=(3, 3), stride=(1, 1), padding=(1, 1))
+ (nonlinearity): SiLU()
+ )
+ )
+ )
+ )
+ (up_blocks): ModuleList(
+ (0): AttnUpBlock2D(
+ (attentions): ModuleList(
+ (0): AttentionBlock(
+ (group_norm): GroupNorm(32, 64, eps=1e-05, affine=True)
+ (query): Linear(in_features=64, out_features=64, bias=True)
+ (key): Linear(in_features=64, out_features=64, bias=True)
+ (value): Linear(in_features=64, out_features=64, bias=True)
+ (proj_attn): Linear(in_features=64, out_features=64, bias=True)
+ )
+ (1): AttentionBlock(
+ (group_norm): GroupNorm(32, 64, eps=1e-05, affine=True)
+ (query): Linear(in_features=64, out_features=64, bias=True)
+ (key): Linear(in_features=64, out_features=64, bias=True)
+ (value): Linear(in_features=64, out_features=64, bias=True)
+ (proj_attn): Linear(in_features=64, out_features=64, bias=True)
+ )
+ (2): AttentionBlock(
+ (group_norm): GroupNorm(32, 64, eps=1e-05, affine=True)
+ (query): Linear(in_features=64, out_features=64, bias=True)
+ (key): Linear(in_features=64, out_features=64, bias=True)
+ (value): Linear(in_features=64, out_features=64, bias=True)
+ (proj_attn): Linear(in_features=64, out_features=64, bias=True)
+ )
+ )
+ (resnets): ModuleList(
+ (0): ResnetBlock2D(
+ (norm1): GroupNorm(32, 128, eps=1e-05, affine=True)
+ (conv1): Conv2d(128, 64, kernel_size=(3, 3), stride=(1, 1), padding=(1, 1))
+ (time_emb_proj): Linear(in_features=128, out_features=64, bias=True)
+ (norm2): GroupNorm(32, 64, eps=1e-05, affine=True)
+ (dropout): Dropout(p=0.0, inplace=False)
+ (conv2): Conv2d(64, 64, kernel_size=(3, 3), stride=(1, 1), padding=(1, 1))
+ (nonlinearity): SiLU()
+ (conv_shortcut): Conv2d(128, 64, kernel_size=(1, 1), stride=(1, 1))
+ )
+ (1): ResnetBlock2D(
+ (norm1): GroupNorm(32, 128, eps=1e-05, affine=True)
+ (conv1): Conv2d(128, 64, kernel_size=(3, 3), stride=(1, 1), padding=(1, 1))
+ (time_emb_proj): Linear(in_features=128, out_features=64, bias=True)
+ (norm2): GroupNorm(32, 64, eps=1e-05, affine=True)
+ (dropout): Dropout(p=0.0, inplace=False)
+ (conv2): Conv2d(64, 64, kernel_size=(3, 3), stride=(1, 1), padding=(1, 1))
+ (nonlinearity): SiLU()
+ (conv_shortcut): Conv2d(128, 64, kernel_size=(1, 1), stride=(1, 1))
+ )
+ (2): ResnetBlock2D(
+ (norm1): GroupNorm(32, 128, eps=1e-05, affine=True)
+ (conv1): Conv2d(128, 64, kernel_size=(3, 3), stride=(1, 1), padding=(1, 1))
+ (time_emb_proj): Linear(in_features=128, out_features=64, bias=True)
+ (norm2): GroupNorm(32, 64, eps=1e-05, affine=True)
+ (dropout): Dropout(p=0.0, inplace=False)
+ (conv2): Conv2d(64, 64, kernel_size=(3, 3), stride=(1, 1), padding=(1, 1))
+ (nonlinearity): SiLU()
+ (conv_shortcut): Conv2d(128, 64, kernel_size=(1, 1), stride=(1, 1))
+ )
+ )
+ (upsamplers): ModuleList(
+ (0): Upsample2D(
+ (conv): Conv2d(64, 64, kernel_size=(3, 3), stride=(1, 1), padding=(1, 1))
+ )
+ )
+ )
+ (1): AttnUpBlock2D(
+ (attentions): ModuleList(
+ (0): AttentionBlock(
+ (group_norm): GroupNorm(32, 64, eps=1e-05, affine=True)
+ (query): Linear(in_features=64, out_features=64, bias=True)
+ (key): Linear(in_features=64, out_features=64, bias=True)
+ (value): Linear(in_features=64, out_features=64, bias=True)
+ (proj_attn): Linear(in_features=64, out_features=64, bias=True)
+ )
+ (1): AttentionBlock(
+ (group_norm): GroupNorm(32, 64, eps=1e-05, affine=True)
+ (query): Linear(in_features=64, out_features=64, bias=True)
+ (key): Linear(in_features=64, out_features=64, bias=True)
+ (value): Linear(in_features=64, out_features=64, bias=True)
+ (proj_attn): Linear(in_features=64, out_features=64, bias=True)
+ )
+ (2): AttentionBlock(
+ (group_norm): GroupNorm(32, 64, eps=1e-05, affine=True)
+ (query): Linear(in_features=64, out_features=64, bias=True)
+ (key): Linear(in_features=64, out_features=64, bias=True)
+ (value): Linear(in_features=64, out_features=64, bias=True)
+ (proj_attn): Linear(in_features=64, out_features=64, bias=True)
+ )
+ )
+ (resnets): ModuleList(
+ (0): ResnetBlock2D(
+ (norm1): GroupNorm(32, 128, eps=1e-05, affine=True)
+ (conv1): Conv2d(128, 64, kernel_size=(3, 3), stride=(1, 1), padding=(1, 1))
+ (time_emb_proj): Linear(in_features=128, out_features=64, bias=True)
+ (norm2): GroupNorm(32, 64, eps=1e-05, affine=True)
+ (dropout): Dropout(p=0.0, inplace=False)
+ (conv2): Conv2d(64, 64, kernel_size=(3, 3), stride=(1, 1), padding=(1, 1))
+ (nonlinearity): SiLU()
+ (conv_shortcut): Conv2d(128, 64, kernel_size=(1, 1), stride=(1, 1))
+ )
+ (1): ResnetBlock2D(
+ (norm1): GroupNorm(32, 128, eps=1e-05, affine=True)
+ (conv1): Conv2d(128, 64, kernel_size=(3, 3), stride=(1, 1), padding=(1, 1))
+ (time_emb_proj): Linear(in_features=128, out_features=64, bias=True)
+ (norm2): GroupNorm(32, 64, eps=1e-05, affine=True)
+ (dropout): Dropout(p=0.0, inplace=False)
+ (conv2): Conv2d(64, 64, kernel_size=(3, 3), stride=(1, 1), padding=(1, 1))
+ (nonlinearity): SiLU()
+ (conv_shortcut): Conv2d(128, 64, kernel_size=(1, 1), stride=(1, 1))
+ )
+ (2): ResnetBlock2D(
+ (norm1): GroupNorm(32, 96, eps=1e-05, affine=True)
+ (conv1): Conv2d(96, 64, kernel_size=(3, 3), stride=(1, 1), padding=(1, 1))
+ (time_emb_proj): Linear(in_features=128, out_features=64, bias=True)
+ (norm2): GroupNorm(32, 64, eps=1e-05, affine=True)
+ (dropout): Dropout(p=0.0, inplace=False)
+ (conv2): Conv2d(64, 64, kernel_size=(3, 3), stride=(1, 1), padding=(1, 1))
+ (nonlinearity): SiLU()
+ (conv_shortcut): Conv2d(96, 64, kernel_size=(1, 1), stride=(1, 1))
+ )
+ )
+ (upsamplers): ModuleList(
+ (0): Upsample2D(
+ (conv): Conv2d(64, 64, kernel_size=(3, 3), stride=(1, 1), padding=(1, 1))
+ )
+ )
+ )
+ (2): UpBlock2D(
+ (resnets): ModuleList(
+ (0): ResnetBlock2D(
+ (norm1): GroupNorm(32, 96, eps=1e-05, affine=True)
+ (conv1): Conv2d(96, 32, kernel_size=(3, 3), stride=(1, 1), padding=(1, 1))
+ (time_emb_proj): Linear(in_features=128, out_features=32, bias=True)
+ (norm2): GroupNorm(32, 32, eps=1e-05, affine=True)
+ (dropout): Dropout(p=0.0, inplace=False)
+ (conv2): Conv2d(32, 32, kernel_size=(3, 3), stride=(1, 1), padding=(1, 1))
+ (nonlinearity): SiLU()
+ (conv_shortcut): Conv2d(96, 32, kernel_size=(1, 1), stride=(1, 1))
+ )
+ (1): ResnetBlock2D(
+ (norm1): GroupNorm(32, 64, eps=1e-05, affine=True)
+ (conv1): Conv2d(64, 32, kernel_size=(3, 3), stride=(1, 1), padding=(1, 1))
+ (time_emb_proj): Linear(in_features=128, out_features=32, bias=True)
+ (norm2): GroupNorm(32, 32, eps=1e-05, affine=True)
+ (dropout): Dropout(p=0.0, inplace=False)
+ (conv2): Conv2d(32, 32, kernel_size=(3, 3), stride=(1, 1), padding=(1, 1))
+ (nonlinearity): SiLU()
+ (conv_shortcut): Conv2d(64, 32, kernel_size=(1, 1), stride=(1, 1))
+ )
+ (2): ResnetBlock2D(
+ (norm1): GroupNorm(32, 64, eps=1e-05, affine=True)
+ (conv1): Conv2d(64, 32, kernel_size=(3, 3), stride=(1, 1), padding=(1, 1))
+ (time_emb_proj): Linear(in_features=128, out_features=32, bias=True)
+ (norm2): GroupNorm(32, 32, eps=1e-05, affine=True)
+ (dropout): Dropout(p=0.0, inplace=False)
+ (conv2): Conv2d(32, 32, kernel_size=(3, 3), stride=(1, 1), padding=(1, 1))
+ (nonlinearity): SiLU()
+ (conv_shortcut): Conv2d(64, 32, kernel_size=(1, 1), stride=(1, 1))
+ )
+ )
+ )
+ )
+ (mid_block): UNetMidBlock2D(
+ (attentions): ModuleList(
+ (0): AttentionBlock(
+ (group_norm): GroupNorm(32, 64, eps=1e-05, affine=True)
+ (query): Linear(in_features=64, out_features=64, bias=True)
+ (key): Linear(in_features=64, out_features=64, bias=True)
+ (value): Linear(in_features=64, out_features=64, bias=True)
+ (proj_attn): Linear(in_features=64, out_features=64, bias=True)
+ )
+ )
+ (resnets): ModuleList(
+ (0): ResnetBlock2D(
+ (norm1): GroupNorm(32, 64, eps=1e-05, affine=True)
+ (conv1): Conv2d(64, 64, kernel_size=(3, 3), stride=(1, 1), padding=(1, 1))
+ (time_emb_proj): Linear(in_features=128, out_features=64, bias=True)
+ (norm2): GroupNorm(32, 64, eps=1e-05, affine=True)
+ (dropout): Dropout(p=0.0, inplace=False)
+ (conv2): Conv2d(64, 64, kernel_size=(3, 3), stride=(1, 1), padding=(1, 1))
+ (nonlinearity): SiLU()
+ )
+ (1): ResnetBlock2D(
+ (norm1): GroupNorm(32, 64, eps=1e-05, affine=True)
+ (conv1): Conv2d(64, 64, kernel_size=(3, 3), stride=(1, 1), padding=(1, 1))
+ (time_emb_proj): Linear(in_features=128, out_features=64, bias=True)
+ (norm2): GroupNorm(32, 64, eps=1e-05, affine=True)
+ (dropout): Dropout(p=0.0, inplace=False)
+ (conv2): Conv2d(64, 64, kernel_size=(3, 3), stride=(1, 1), padding=(1, 1))
+ (nonlinearity): SiLU()
+ )
+ )
+ )
+ (conv_norm_out): GroupNorm(32, 32, eps=1e-05, affine=True)
+ (conv_act): SiLU()
+ (conv_out): Conv2d(32, 1, kernel_size=(3, 3), stride=(1, 1), padding=(1, 1))
+ )
+
+
+正如你所看到的,还有更多!它比我们的BasicUNet有多得多的参数量:
+
+
+```python
+sum([p.numel() for p in model.parameters()]) # 1.7M vs the ~309k parameters of the BasicUNet
+```
+
+
+
+
+ 1707009
+
+
+
+我们可以用这个模型代替原来的模型来重复一遍上面展示的训练过程。我们需要将x和timestep传递给模型(这里我会传递t = 0,以表明它在没有timestep条件的情况下工作,并保持采样代码简单,但您也可以尝试输入 `(amount*1000)`,使timestep与噪声水平相当)。如果要检查代码,更改的行将显示为“`#<<<`。
+
+
+```python
+#@markdown Trying UNet2DModel instead of BasicUNet:
+
+# Dataloader (you can mess with batch size)
+batch_size = 128
+train_dataloader = DataLoader(dataset, batch_size=batch_size, shuffle=True)
+
+# How many runs through the data should we do?
+n_epochs = 3
+
+# Create the network
+net = UNet2DModel(
+ sample_size=28, # the target image resolution
+ in_channels=1, # the number of input channels, 3 for RGB images
+ out_channels=1, # the number of output channels
+ layers_per_block=2, # how many ResNet layers to use per UNet block
+ block_out_channels=(32, 64, 64), # Roughly matching our basic unet example
+ down_block_types=(
+ "DownBlock2D", # a regular ResNet downsampling block
+ "AttnDownBlock2D", # a ResNet downsampling block with spatial self-attention
+ "AttnDownBlock2D",
+ ),
+ up_block_types=(
+ "AttnUpBlock2D",
+ "AttnUpBlock2D", # a ResNet upsampling block with spatial self-attention
+ "UpBlock2D", # a regular ResNet upsampling block
+ ),
+) #<<<
+net.to(device)
+
+# Our loss finction
+loss_fn = nn.MSELoss()
+
+# The optimizer
+opt = torch.optim.Adam(net.parameters(), lr=1e-3)
+
+# Keeping a record of the losses for later viewing
+losses = []
+
+# The training loop
+for epoch in range(n_epochs):
+
+ for x, y in train_dataloader:
+
+ # Get some data and prepare the corrupted version
+ x = x.to(device) # Data on the GPU
+ noise_amount = torch.rand(x.shape[0]).to(device) # Pick random noise amounts
+ noisy_x = corrupt(x, noise_amount) # Create our noisy x
+
+ # Get the model prediction
+ pred = net(noisy_x, 0).sample #<<< Using timestep 0 always, adding .sample
+
+ # Calculate the loss
+ loss = loss_fn(pred, x) # How close is the output to the true 'clean' x?
+
+ # Backprop and update the params:
+ opt.zero_grad()
+ loss.backward()
+ opt.step()
+
+ # Store the loss for later
+ losses.append(loss.item())
+
+ # Print our the average of the loss values for this epoch:
+ avg_loss = sum(losses[-len(train_dataloader):])/len(train_dataloader)
+ print(f'Finished epoch {epoch}. Average loss for this epoch: {avg_loss:05f}')
+
+# Plot losses and some samples
+fig, axs = plt.subplots(1, 2, figsize=(12, 5))
+
+# Losses
+axs[0].plot(losses)
+axs[0].set_ylim(0, 0.1)
+axs[0].set_title('Loss over time')
+
+# Samples
+n_steps = 40
+x = torch.rand(64, 1, 28, 28).to(device)
+for i in range(n_steps):
+ noise_amount = torch.ones((x.shape[0], )).to(device) * (1-(i/n_steps)) # Starting high going low
+ with torch.no_grad():
+ pred = net(x, 0).sample
+ mix_factor = 1/(n_steps - i)
+ x = x*(1-mix_factor) + pred*mix_factor
+
+axs[1].imshow(torchvision.utils.make_grid(x.detach().cpu(), nrow=8)[0].clip(0, 1), cmap='Greys')
+axs[1].set_title('Generated Samples');
+```
+
+ Finished epoch 0. Average loss for this epoch: 0.018925
+ Finished epoch 1. Average loss for this epoch: 0.012785
+ Finished epoch 2. Average loss for this epoch: 0.011694
+
+
+
+
+
+
+
+
+这看起来比我们的第一组结果好多了!您可以尝试调整UNet配置或更长时间的训练,以获得更好的性能。
+
+### 损坏过程
+
+DDPM论文描述了一个为每个“timestep”添加少量噪声的损坏过程。 为某些timestep给定 $x_{t-1}$ ,我们可以得到一个噪声稍稍增加的 $x_t$:
+
+$q(\mathbf{x}_t \vert \mathbf{x}_{t-1}) = \mathcal{N}(\mathbf{x}_t; \sqrt{1 - \beta_t} \mathbf{x}_{t-1}, \beta_t\mathbf{I}) \quad
+q(\mathbf{x}_{1:T} \vert \mathbf{x}_0) = \prod^T_{t=1} q(\mathbf{x}_t \vert \mathbf{x}_{t-1})$
+
+
+这就是说,我们取 $x_{t-1}$, 给他一个$\sqrt{1 - \beta_t}$ 的系数,然后加上带有 $\beta_t$系数的噪声。 这里 $\beta$ 是根据一些管理器来为每一个t设定的,来决定每一个迭代周期中添加多少噪声。 现在,我们不想把这个推演进行500次来得到 $x_{500}$,所以我们用另一个公式来根据给出的 $x_0$ 计算得到任意t时刻的 $x_t$:
+
+$\begin{aligned}
+q(\mathbf{x}_t \vert \mathbf{x}_0) &= \mathcal{N}(\mathbf{x}_t; \sqrt{\bar{\alpha}_t} \mathbf{x}_0, \sqrt{(1 - \bar{\alpha}_t)} \mathbf{I})
+\end{aligned}$ where $\bar{\alpha}_t = \prod_{i=1}^T \alpha_i$ and $\alpha_i = 1-\beta_i$
+
+数学符号看起来总是很吓人!幸运的是,调度器为我们处理了所有这些(取消下一个单元格的注释以检查代码)。我们可以画出 $\sqrt{\bar{\alpha}_t}$ (标记为 `sqrt_alpha_prod`) 和 $\sqrt{(1 - \bar{\alpha}_t)}$ (标记为 `sqrt_one_minus_alpha_prod`) 来看一下输入(x)与噪声是如何在不同迭代周期中量化和叠加的:
+
+
+
+```python
+#??noise_scheduler.add_noise
+```
+
+
+```python
+noise_scheduler = DDPMScheduler(num_train_timesteps=1000)
+plt.plot(noise_scheduler.alphas_cumprod.cpu() ** 0.5, label=r"${\sqrt{\bar{\alpha}_t}}$")
+plt.plot((1 - noise_scheduler.alphas_cumprod.cpu()) ** 0.5, label=r"$\sqrt{(1 - \bar{\alpha}_t)}$")
+plt.legend(fontsize="x-large");
+```
+
+
+
+
+
+
+
+一开始, 噪声x里绝大部分都是x自身的值 (sqrt_alpha_prod ~= 1),但是随着时间的推移,x的成分逐渐降低而噪声的成分逐渐增加。与我们根据`amount`对x和噪声进行线性混合不同,这个噪声的增加相对较快。我们可以在一些数据上看到这一点:
+
+
+```python
+#@markdown visualize the DDPM noising process for different timesteps:
+
+# Noise a batch of images to view the effect
+fig, axs = plt.subplots(3, 1, figsize=(16, 10))
+xb, yb = next(iter(train_dataloader))
+xb = xb.to(device)[:8]
+xb = xb * 2. - 1. # Map to (-1, 1)
+print('X shape', xb.shape)
+
+# Show clean inputs
+axs[0].imshow(torchvision.utils.make_grid(xb[:8])[0].detach().cpu(), cmap='Greys')
+axs[0].set_title('Clean X')
+
+# Add noise with scheduler
+timesteps = torch.linspace(0, 999, 8).long().to(device)
+noise = torch.randn_like(xb) # << NB: randn not rand
+noisy_xb = noise_scheduler.add_noise(xb, noise, timesteps)
+print('Noisy X shape', noisy_xb.shape)
+
+# Show noisy version (with and without clipping)
+axs[1].imshow(torchvision.utils.make_grid(noisy_xb[:8])[0].detach().cpu().clip(-1, 1), cmap='Greys')
+axs[1].set_title('Noisy X (clipped to (-1, 1)')
+axs[2].imshow(torchvision.utils.make_grid(noisy_xb[:8])[0].detach().cpu(), cmap='Greys')
+axs[2].set_title('Noisy X');
+```
+
+ X shape torch.Size([8, 1, 28, 28])
+ Noisy X shape torch.Size([8, 1, 28, 28])
+
+
+
+
+
+
+
+
+在运行中的另一个变化:在DDPM版本中,加入的噪声是取自一个高斯分布(来自均值0方差1的torch.randn),而不是在我们原始 `corrupt`函数中使用的 0-1之间的均匀分布(torch.rand),当然对训练数据做正则化也可以理解。在另一篇笔记中,你会看到 `Normalize(0.5, 0.5)`函数在变化列表中,它把图片数据从(0, 1) 区间映射到 (-1, 1),对我们的目标来说也‘足够用了’。我们在此篇笔记中没使用这个方法,但在上面的可视化中为了更好的展示添加了这种做法。
+
+
+```python
+
+```
+
+### 训练目标
+
+在我们的玩具示例中,我们让模型尝试预测去噪图像。在DDPM和许多其他扩散模型实现中,模型则会预测损坏过程中使用的噪声(在缩放之前,因此是单位方差噪声)。在代码中,它看起来像使这样:
+
+```python
+noise = torch.randn_like(xb) # << NB: randn not rand
+noisy_x = noise_scheduler.add_noise(x, noise, timesteps)
+model_prediction = model(noisy_x, timesteps).sample
+loss = mse_loss(model_prediction, noise) # noise as the target
+```
+
+你可能认为预测噪声(我们可以从中得出去噪图像的样子)等同于直接预测去噪图像。那么,为什么要这么做呢?这仅仅是为了数学上的方便吗?
+
+这里其实还有另一些精妙之处。我们在训练过程中,会计算不同(随机选择)timestep的loss。这些不同的目标将导致这些loss的不同的“隐含权重”,其中预测噪声会将更多的权重放在较低的噪声水平上。你可以选择更复杂的目标来改变这种“隐性损失权重”。或者,您选择的噪声管理器将在较高的噪声水平下产生更多的示例。也许你让模型设计成预测“velocity”v,我们将其定义为由噪声水平影响的图像和噪声组合(请参阅“扩散模型快速采样的渐进蒸馏”- 'PROGRESSIVE DISTILLATION FOR FAST SAMPLING OF DIFFUSION MODELS')。也许你将模型设计成预测噪声,然后基于某些因子来对loss进行缩放:比如有些理论指出可以参考噪声水平(参见“扩散模型的感知优先训练”-'Perception Prioritized Training of Diffusion Models'),或者基于一些探索模型最佳噪声水平的实验(参见“基于扩散的生成模型的设计空间说明”-'Elucidating the Design Space of Diffusion-Based Generative Models')。
+
+一句话解释:选择目标对模型性能有影响,现在有许多研究者正在探索“最佳”选项是什么。
+目前,预测噪声(epsilon或eps)是最流行的方法,但随着时间的推移,我们很可能会看到库中支持的其他目标,并在不同的情况下使用。
+
+### 迭代周期(Timestep)调节
+
+UNet2DModel以x和timestep为输入。后者被转化为一个嵌入(embedding),并在多个地方被输入到模型中。
+
+这背后的理论支持是这样的:通过向模型提供有关噪声水平的信息,它可以更好地执行任务。虽然在没有这种timestep条件的情况下也可以训练模型,但在某些情况下,它似乎确实有助于性能,目前来说绝大多数的模型实现都包括了这一输入。
+
+### 取样(采样)
+
+有一个模型可以用来预测在带噪样本中的噪声(或者说能预测其去噪版本),我们怎么用它来生成图像呢?
+
+我们可以给入纯噪声,然后就希望模型能一步就输出一个不带噪声的好图像。但是,就我们上面所见到的来看,这通常行不通。所以,我们在模型预测的基础上使用足够多的小步,迭代着来每次去除一点点噪声。
+
+具体我们怎么走这些小步,取决于使用上面取样方法。我们不会去深入讨论太多的理论细节,但是一些顶层想法是这样:
+- 每一步你想走多大?也就是说,你遵循什么样的“噪声计划(噪声管理)”?
+- 你只使用模型当前步的预测结果来指导下一步的更新方向吗(像DDPM,DDIM或是其他的什么那样)?你是否要使用模型来多预测几次来估计一个更高阶的梯度来更新一步更大更准确的结果(更高阶的方法和一些离散ODE处理器)?或者保留历史预测值来尝试更好的指导当前步的更新(线性多步或遗传取样器)?
+- 你是否会在取样过程中额外再加一些随机噪声,或你完全已知得(deterministic)来添加噪声?许多取样器通过参数(如DDIM中的'eta')来供用户选择。
+
+对于扩散模型取样器的研究演进的很快,随之开发出了越来越多可以使用更少步就找到好结果的方法。勇敢和有好奇心的人可能会在浏览diffusers library中不同部署方法时感到非常有意思 [here](https://github.com/huggingface/diffusers/tree/main/src/diffusers/schedulers) 或看看 [docs](https://huggingface.co/docs/diffusers/api/schedulers) 这里经常有一些相关的paper.
+
+## 结语
+
+希望这可以从一些不同的角度来审视扩散模型提供一些帮助。
+这篇笔记是Jonathan Whitaker为Hugging Face 课程所写的,同时也有 [version included in his own course](https://johnowhitaker.github.io/tglcourse/dm1.html),“风景的生成”- 'The Generative Landscape'。如果你对从噪声和约束分类来生成样本的例子感兴趣。问题与bug可以通过GitHub issues 或 Discord来交流。 也同时欢迎通过Twitter联系 [@johnowhitaker](https://twitter.com/johnowhitaker).
diff --git a/markdown/unit1/02_diffusion_models_from_scratch_CN_files/02_diffusion_models_from_scratch_CN_24_1.png b/markdown/unit1/02_diffusion_models_from_scratch_CN_files/02_diffusion_models_from_scratch_CN_24_1.png
new file mode 100644
index 0000000000000000000000000000000000000000..3fdc5446bd7401de802058a4cc875f6a51df4731
--- /dev/null
+++ b/markdown/unit1/02_diffusion_models_from_scratch_CN_files/02_diffusion_models_from_scratch_CN_24_1.png
@@ -0,0 +1,3 @@
+version https://git-lfs.github.com/spec/v1
+oid sha256:1f3ec8a31b370ba4ba59dfd3072d3ab387e23b05e283f5917b69582206e136b4
+size 12507
diff --git a/markdown/unit1/02_diffusion_models_from_scratch_CN_files/02_diffusion_models_from_scratch_CN_26_0.png b/markdown/unit1/02_diffusion_models_from_scratch_CN_files/02_diffusion_models_from_scratch_CN_26_0.png
new file mode 100644
index 0000000000000000000000000000000000000000..f50733abe35352c7691f23b28a89c6cde32ea5cc
--- /dev/null
+++ b/markdown/unit1/02_diffusion_models_from_scratch_CN_files/02_diffusion_models_from_scratch_CN_26_0.png
@@ -0,0 +1,3 @@
+version https://git-lfs.github.com/spec/v1
+oid sha256:255f039788b323b65c69bac27e104edf75e9cbd3f958591d708baaa53e02ba2a
+size 119652
diff --git a/markdown/unit1/02_diffusion_models_from_scratch_CN_files/02_diffusion_models_from_scratch_CN_29_0.png b/markdown/unit1/02_diffusion_models_from_scratch_CN_files/02_diffusion_models_from_scratch_CN_29_0.png
new file mode 100644
index 0000000000000000000000000000000000000000..af3a7f816576c01be89da9e2cbbc4b58bb0254de
--- /dev/null
+++ b/markdown/unit1/02_diffusion_models_from_scratch_CN_files/02_diffusion_models_from_scratch_CN_29_0.png
@@ -0,0 +1,3 @@
+version https://git-lfs.github.com/spec/v1
+oid sha256:bf3e6c0c3c6db742b4c076f2744a0b86f92355bd9a6c3d7c9ddff2e1d4d7246d
+size 125212
diff --git a/markdown/unit1/02_diffusion_models_from_scratch_CN_files/02_diffusion_models_from_scratch_CN_31_1.png b/markdown/unit1/02_diffusion_models_from_scratch_CN_files/02_diffusion_models_from_scratch_CN_31_1.png
new file mode 100644
index 0000000000000000000000000000000000000000..7d907b091ace91390f84e97a528b1838324aae59
--- /dev/null
+++ b/markdown/unit1/02_diffusion_models_from_scratch_CN_files/02_diffusion_models_from_scratch_CN_31_1.png
@@ -0,0 +1,3 @@
+version https://git-lfs.github.com/spec/v1
+oid sha256:12d49a7db75ef5917c95fdb4a656218a8e457468b253fb58b6f8e232f627a3c8
+size 216298
diff --git a/markdown/unit1/02_diffusion_models_from_scratch_CN_files/02_diffusion_models_from_scratch_CN_39_1.png b/markdown/unit1/02_diffusion_models_from_scratch_CN_files/02_diffusion_models_from_scratch_CN_39_1.png
new file mode 100644
index 0000000000000000000000000000000000000000..1f62969442b68c9e919e0b2ea1f4762ca29d84bb
--- /dev/null
+++ b/markdown/unit1/02_diffusion_models_from_scratch_CN_files/02_diffusion_models_from_scratch_CN_39_1.png
@@ -0,0 +1,3 @@
+version https://git-lfs.github.com/spec/v1
+oid sha256:69d75b23c16679efb2d03aa4c71a24aa42ecb19d25bf61a5e729a321cd56001e
+size 81314
diff --git a/markdown/unit1/02_diffusion_models_from_scratch_CN_files/02_diffusion_models_from_scratch_CN_43_0.png b/markdown/unit1/02_diffusion_models_from_scratch_CN_files/02_diffusion_models_from_scratch_CN_43_0.png
new file mode 100644
index 0000000000000000000000000000000000000000..5a3c09eb03d06ff95c6aea71f8b32e2ce068d572
--- /dev/null
+++ b/markdown/unit1/02_diffusion_models_from_scratch_CN_files/02_diffusion_models_from_scratch_CN_43_0.png
@@ -0,0 +1,3 @@
+version https://git-lfs.github.com/spec/v1
+oid sha256:66b716d94b45e7812e7d8cf1f31b097fed9bd21b854203e80e7920c5f01115ca
+size 14564
diff --git a/markdown/unit1/02_diffusion_models_from_scratch_CN_files/02_diffusion_models_from_scratch_CN_45_1.png b/markdown/unit1/02_diffusion_models_from_scratch_CN_files/02_diffusion_models_from_scratch_CN_45_1.png
new file mode 100644
index 0000000000000000000000000000000000000000..2f156d497386fa0d26503c9d9c2fbb06615aa74d
--- /dev/null
+++ b/markdown/unit1/02_diffusion_models_from_scratch_CN_files/02_diffusion_models_from_scratch_CN_45_1.png
@@ -0,0 +1,3 @@
+version https://git-lfs.github.com/spec/v1
+oid sha256:1d18d95dcc9ab62110c54e50370bd72d7cde9c902fb40e690ab6ff0bef93c875
+size 53066
diff --git a/markdown/unit1/README_CN.md b/markdown/unit1/README_CN.md
new file mode 100644
index 0000000000000000000000000000000000000000..90c5accfe8c4c2b5aa8d56e83af0b337641e7dd7
--- /dev/null
+++ b/markdown/unit1/README_CN.md
@@ -0,0 +1,65 @@
+# 单元 1: 扩散模型简介
+
+欢迎来到 Hugging Face 扩散模型课程第一单元!在本单元中,你将学习到有关扩散模型如何运作的基础知识,同时也会学到如何使用 🤗 diffusers 库。
+
+## 开始本单元 :rocket:
+
+以下是本单元的学习步骤:
+
+- 请确保你已经 [注册了该课程](https://huggingface.us17.list-manage.com/subscribe?u=7f57e683fa28b51bfc493d048&id=ef963b4162)。这样当有新课程材料发布的时候你就会收到通知
+- 通读下面的介绍材料以及任何你感兴趣的其他资源
+- 查看下面的 _**Introduction to Diffusers**_ Notebook 链接,以使用 `diffuers` 库将理论应用到实践中
+- 使用 Notebook 或链接的训练脚本来训练和分享你自己的扩散模型
+- (可选) 如果你有兴趣看到一个极简的从头开始的项目实现,并探索所涉及的不同设计决策,你可以深入研究 _**Diffusion Models from Scratch**_ 这个 Notebook
+
+
+:loudspeaker: 请不要忘了加入我们的频道 [Discord](https://huggingface.co/join/discord), 你可以在 `#diffusion-models-class` 频道来讨论课程内容以及分享你的作品。
+
+## 什么是扩散模型?
+
+扩散模型是「生成模型」算法家族的新成员通过学习给定的训练样本,生成模型可以学会如何 **生成** 数据,比如生成图片或者声音。一个好的生成模型能生成一组 **样式不同** 的输出。这些输出会与训练数据相似,但不是一模一样的副本。扩散模型如何实现这一点?为了便于说明,让我们先看看图像生成的案例。
+
+
+
+
+ 图片来源于 DDPM paper (https://arxiv.org/abs/2006.11239)。
+
+
+扩散模型成功的秘诀在于扩散过程的迭代本质。最先生成的只是一组随机噪声,但是经过若干步的逐渐改善之后,最终会出现有意义的图像。在每一步中,模型都会估计如何从当前的输入生成完全去噪的结果。因为我们在每一步都只做了一个小小的变动,所以在早期阶段(预测最终输出实际上非常困难),这个估计中的任何 error 都可以在以后的更新中得到纠正。
+
+与其他类型的生成模型相比,训练扩散模型相对较为容易。我们只需要重复以下步骤即可:
+
+1) 从训练数据中加载一些图像
+2) 添加不同级别的噪声。请记住,我们希望模型在面对添加了极端噪声和几乎没有添加噪声的带噪图像时,都能够很好地估计如何 “修复”(去噪)。
+3) 将带噪输入送入模型中
+4) 评估模型对这些输入进行去噪的效果
+5) 使用此信息更新模型权重
+
+为了用训练好的模型生成新的图像,我们从完全随机的输入开始,反复将其输入模型,每次根据模型预测进行少量更新。我们之后会学到有许多采样方法试图简化这个过程,以便我们可以用尽可能少的步骤生成好的图像。
+我们将在第一单元的实践笔记本中详细介绍这些步骤。在第二单元中,我们将了解如何修改此过程,来通过额外的条件(例如类标签)或使用指导等技术来增加对模型输出的额外控制。第三单元和第四单元将探索一种非常强大的扩散模型,称为稳定扩散 (stable diffusion),它可以生成给定文本描述的图像。
+
+## 实践笔记本
+
+到这里,你已经足够了解如何开始使用附带的笔记本了!这里的两个笔记本以不同的方式表达了相同的想法。
+
+| Chapter | Colab | Kaggle | Gradient | Studio Lab |
+|:--------------------------------------------|:----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------|:---------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------|:---------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------|:-------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------|
+| Introduction to Diffusers | [](https://colab.research.google.com/github/darcula1993/diffusion-models-class-CN/blob/main/unit1/01_introduction_to_diffusers_CN.ipynb) | [](https://kaggle.com/kernels/welcome?src=https://github.com/huggingface/diffusion-models-class/blob/main/unit1/01_introduction_to_diffusers_CN.ipynb) | [](https://console.paperspace.com/github/darcula1993/diffusion-models-class-CN/blob/main/unit1/01_introduction_to_diffusers_CN.ipynb) | [](https://studiolab.sagemaker.aws/import/github/darcula1993/diffusion-models-class-CN/blob/main/unit1/01_introduction_to_diffusers_CN.ipynb) |
+| Diffusion Models from Scratch | [](https://colab.research.google.com/github/darcula1993/diffusion-models-class-CN/blob/main/unit1/02_diffusion_models_from_scratch_CN.ipynb) | [](https://kaggle.com/kernels/welcome?src=https://github.com/huggingface/diffusion-models-class/blob/main/unit1/02_diffusion_models_from_scratch_CN.ipynb) | [](https://console.paperspace.com/github/darcula1993/diffusion-models-class-CN/blob/main/unit1/02_diffusion_models_from_scratch_CN.ipynb) | [](https://studiolab.sagemaker.aws/import/github/darcula1993/diffusion-models-class-CN/blob/main/unit1/02_diffusion_models_from_scratch_CN.ipynb) |
+
+在 _**Introduction to Diffusers**_ 这个 Notebook 中,我们使用 diffusers 库中的构造模块显示了与上述不同的步骤。你将很快看到如何根据你选择的任何数据创建、训练和采样你自己的扩散模型。 在笔记本结束时,你将能够阅读和修改示例训练脚本,以训练扩散模型,并将其与全世界共同分享! 本笔记本还介绍了与本单元相关的主要练习,在这里,我们将共同尝试为不同规模的扩散模型找出好的「训练脚本」- 请参阅下一节了解更多信息。
+
+在 _**Diffusion Models from Scratch**_ 这个 Notebook 中,我们展示了相同的步骤(向数据添加噪声、创建模型、训练和采样),并尽可能简单地在 PyTorch 中从头开始实现。然后,我们将这个「玩具示例」与 `diffusers` 版本进行比较,并关注两者的区别以及改进之处。这里的目标是熟悉不同的组件和其中的设计决策,以便在查看新的实现时能够快速确定关键思想。
+
+## 项目时间
+
+现在,你已经掌握了基本知识,可以开始训练你自己的扩散模型了! _**Introduction to Diffusers**_ 这个 Notebook 的末尾有一些小提示,希望你能与社区分享你的成果、训练脚本和发现,以便我们能够一起找出训练这些模型的最佳方法。
+
+## 一些额外的材料
+
+- [《Hugging Face 博客: 带注释的扩散模型》](https://huggingface.co/blog/annotated-diffusion)是对 DDPM 背后的代码和理论的非常深入的介绍,其中包括数学和显示了所有不同的组件的代码。它还链接了一些论文供进一步阅读:
+- [Hugging Face 文档: 无条件图像生成 (Unconditional Image-Generation)](https://huggingface.co/docs/diffusers/training/unconditional_training),包含了有关如何使用官方训练示例脚本训练扩散模型的一些示例,包括演示如何创建自己的数据集的代码:
+- AI Coffee Break video on Diffusion Models: https://www.youtube.com/watch?v=344w5h24-h8
+- Yannic Kilcher Video on DDPMs: https://www.youtube.com/watch?v=W-O7AZNzbzQ
+
+发现了有其他任何有帮助的资源?请 [向我们提出](https://github.com/huggingface/diffusion-models-class/issues),我们会将其加入到上面的列表中。
\ No newline at end of file
diff --git a/markdown/unit2/01_finetuning_and_guidance_CN.md b/markdown/unit2/01_finetuning_and_guidance_CN.md
new file mode 100644
index 0000000000000000000000000000000000000000..800bd89d67ebb023dd1ca2d087efc6d8315fd105
--- /dev/null
+++ b/markdown/unit2/01_finetuning_and_guidance_CN.md
@@ -0,0 +1,1048 @@
+# Fine-Tuning and Guidance
+
+在这一节的笔记本中,我们将讲解两种主要的基于现有模型实现改造的方法:
+
+* 通过**微调(fine-tuning)**,我们将在新的数据集上重新训练已有的模型,来改变它原有的输出类型
+* 通过**引导(guidance)**,我们将在推理阶段引导现有模型的生成过程,以此来获取额外的控制
+
+## 你将学到:
+
+在阅读完这一节笔记本后,你将学会:
+
+- 创建一个采样循环,并使用调度器(scheduler)更快地生成样本
+- 在新数据集上微调一个现有的扩散模型,这包括:
+ - 使用累积梯度的方法去应对训练的 batch 太小所带来的一些问题
+ - 在训练过程中,将样本上传到 [Weights and Biases](https://wandb.ai/site) 来记录日志,以此来监控训练过程(通过附加的实例脚本程序)
+ - 将最终结果管线(pipeline)保存下来,并上传到Hub
+- 通过新加的损失函数来引导采样过程,以此对现有模型施加控制,这包括:
+ - 通过一个简单的基于颜色的损失来探索不同的引导方法
+ - 使用 CLIP,用文本来引导生成过程
+ - 用 Gradio 和 🤗 Spaces 来分享你的定制的采样循环
+
+❓如果你有问题,请在 Hugging Face Discord 的 `#diffusion-models-class` 频道提出。如果你还没有 Hugging Face 的账号,你可以在这里注册:https://huggingface.co/join/discord
+
+## 配置过程和需要引入的库
+
+为了将你的微调过的模型保存到 Hugging Face Hub 上,你需要使用一个**具有写权限的访问令牌**来登录。下列代码将会引导你登陆并连接上你的账号的相关令牌页。如果在模型训练过程中,你想使用训练脚本将样本记录到日志,你也需要一个 Weights and Biases 账号 —— 同样地,这些代码也会在需要的时候引导你登录。
+
+此外,你唯一需要配置的就是安装几个依赖,并在程序中引入我们需要的东西然后制定好我们将使用的计算设备。
+
+
+
+```python
+!pip install -qq diffusers datasets accelerate wandb open-clip-torch
+```
+
+
+```python
+# Code to log in to the Hugging Face Hub, needed for sharing models
+# Make sure you use a token with WRITE access
+from huggingface_hub import notebook_login
+
+notebook_login()
+```
+
+ Token is valid.
+ Your token has been saved in your configured git credential helpers (store).
+ Your token has been saved to /root/.huggingface/token
+ Login successful
+
+
+
+```python
+import numpy as np
+import torch
+import torch.nn.functional as F
+import torchvision
+from datasets import load_dataset
+from diffusers import DDIMScheduler, DDPMPipeline
+from matplotlib import pyplot as plt
+from PIL import Image
+from torchvision import transforms
+from tqdm.auto import tqdm
+```
+
+
+```python
+device = (
+ "mps"
+ if torch.backends.mps.is_available()
+ else "cuda"
+ if torch.cuda.is_available()
+ else "cpu"
+)
+```
+
+## 载入一个预训练过的管线
+
+在本节笔记本的开始,我们先载入一个现有的管线,来看看我们能用它做些什么:
+
+
+
+```python
+image_pipe = DDPMPipeline.from_pretrained("google/ddpm-celebahq-256")
+image_pipe.to(device);
+```
+
+
+ Fetching 4 files: 0%| | 0/4 [00:00, ?it/s]
+
+
+生成图像就像调用管线的[`__call__`](https://github.com/huggingface/diffusers/blob/main/src/diffusers/pipelines/ddpm/pipeline_ddpm.py#L42)方法一样简单,我们像调用函数一样来试试:
+
+
+```python
+images = image_pipe().images
+images[0]
+```
+
+
+ 0%| | 0/1000 [00:00, ?it/s]
+
+
+
+
+
+
+
+
+
+
+
+过程很简洁,但速度有点慢!所以,在我们进入今天的正题之前,我们先窥探一下实际的采样循环是什么样,并看看我们能不能用一个更炫酷点的采样器来加速这一过程:
+
+## DDIM更快的采样过程
+
+在生成的每一步中,模型都是接收一个加噪声的输入,并被要求去预测这个噪声(以此来估计完全没噪声的图片是什么样)。最初这些预测都还不算太好,因此我们把这一过程分解成很多步。但另一方面,使用1000+步来实现整个生成过程也不是必要的,因为最近的很多研究已经找到了使用尽可能少的步数来生成好的结果的方法。
+
+在 🤗 Diffusers 库中,**这些采样方法是通过调度器(scheduler)来操控的**,每次更新通过`step()`函数完成。为了生成图片,我们从随机噪声$x$开始,每一个迭代周期(timestep)我们都送入模型一个带噪声的输入$x$并把模型预测结果再次输入`step()`函数。这里返回的输出都被命名为`prev_sample` —— 之所以是“previous”,是因为我们是在时间上“后退”,即从高噪声到低噪声(这和前向扩散过程是相反的)。
+
+我们实践一下看看:先载入一个 scheduler,这里用的是 DDIMScheduler(基于这篇论文:[Denoising Diffusion Implicit Models](https://arxiv.org/abs/2010.02502))。与原版的 DDPM 相比,它可以用少得多的迭代周期来产生很不错的采样样本。
+
+
+```python
+# Create new scheduler and set num inference steps
+scheduler = DDIMScheduler.from_pretrained("google/ddpm-celebahq-256")
+scheduler.set_timesteps(num_inference_steps=40)
+```
+
+可以看到,这个模型只使用了 40 步,走一步抵得上原始 1000 步调度器走 25 步了:
+
+
+```python
+scheduler.timesteps
+```
+
+
+
+
+ tensor([975, 950, 925, 900, 875, 850, 825, 800, 775, 750, 725, 700, 675, 650,
+ 625, 600, 575, 550, 525, 500, 475, 450, 425, 400, 375, 350, 325, 300,
+ 275, 250, 225, 200, 175, 150, 125, 100, 75, 50, 25, 0])
+
+
+
+我们来造出 4 个随机噪声图片,用它们跑一跑采样循环,同时观察一下每一步的$x$和预测出的去噪版本:
+
+
+```python
+# The random starting point
+x = torch.randn(4, 3, 256, 256).to(device) # Batch of 4, 3-channel 256 x 256 px images
+
+# Loop through the sampling timesteps
+for i, t in tqdm(enumerate(scheduler.timesteps)):
+
+ # Prepare model input
+ model_input = scheduler.scale_model_input(x, t)
+
+ # Get the prediction
+ with torch.no_grad():
+ noise_pred = image_pipe.unet(model_input, t)["sample"]
+
+ # Calculate what the updated sample should look like with the scheduler
+ scheduler_output = scheduler.step(noise_pred, t, x)
+
+ # Update x
+ x = scheduler_output.prev_sample
+
+ # Occasionally display both x and the predicted denoised images
+ if i % 10 == 0 or i == len(scheduler.timesteps) - 1:
+ fig, axs = plt.subplots(1, 2, figsize=(12, 5))
+
+ grid = torchvision.utils.make_grid(x, nrow=4).permute(1, 2, 0)
+ axs[0].imshow(grid.cpu().clip(-1, 1) * 0.5 + 0.5)
+ axs[0].set_title(f"Current x (step {i})")
+
+ pred_x0 = (
+ scheduler_output.pred_original_sample
+ ) # Not available for all schedulers
+ grid = torchvision.utils.make_grid(pred_x0, nrow=4).permute(1, 2, 0)
+ axs[1].imshow(grid.cpu().clip(-1, 1) * 0.5 + 0.5)
+ axs[1].set_title(f"Predicted denoised images (step {i})")
+ plt.show()
+```
+
+
+ 0it [00:00, ?it/s]
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+可以看出,一开始预测结果并不是那么好,但随着这一过程的推进,预测的输出被一步步改善。如果你想了解`steps()`函数内的数学原理,你可以通过这行指令来看看(带详细注释的)代码:
+
+
+```python
+# ??scheduler.step
+```
+
+你也可以直接用新的调度器替换原有管线(pipeline)中的调度器,然后采样。像这样做:
+
+
+```python
+image_pipe.scheduler = scheduler
+images = image_pipe(num_inference_steps=40).images
+images[0]
+```
+
+
+ 0%| | 0/40 [00:00, ?it/s]
+
+
+
+
+
+
+
+
+
+
+
+好了!我们现在可以在可接受的时间内采样了!这样我们学习接下来的部分就能快点了:)
+
+## 微调
+
+现在玩点好玩的!给我们一个预训练过的管线(pipeline),我们怎样使用新的训练数据重训模型来生成图片?
+
+看起来这和我们从头训练模型是几乎一样的(正如我们在[第一单元](../unit1)所见的一样),除了我们这里是用现有模型作为初始化的。让我们实践一下看看,并额外考虑几点我们要注意的东西。
+
+首先,数据方面,你可以尝试用 [Vintage Faces 数据集](https://huggingface.co/datasets/Norod78/Vintage-Faces-FFHQAligned) 或者[这些动漫人脸图片](https://huggingface.co/datasets/huggan/anime-faces)来获取和这个人脸模型的原始训练数据类似的数据。但我们现在还是先用和第一单元一样的蝴蝶数据集吧。通过以下代码来下载蝴蝶数据集,并建立一个能按批(batch)采样图片的`dataloader`:
+
+
+```python
+# @markdown load and prepare a dataset:
+# Not on Colab? Comments with #@ enable UI tweaks like headings or user inputs
+# but can safely be ignored if you're working on a different platform.
+
+dataset_name = "huggan/smithsonian_butterflies_subset" # @param
+dataset = load_dataset(dataset_name, split="train")
+image_size = 256 # @param
+batch_size = 4 # @param
+preprocess = transforms.Compose(
+ [
+ transforms.Resize((image_size, image_size)),
+ transforms.RandomHorizontalFlip(),
+ transforms.ToTensor(),
+ transforms.Normalize([0.5], [0.5]),
+ ]
+)
+
+
+def transform(examples):
+ images = [preprocess(image.convert("RGB")) for image in examples["image"]]
+ return {"images": images}
+
+
+dataset.set_transform(transform)
+
+train_dataloader = torch.utils.data.DataLoader(
+ dataset, batch_size=batch_size, shuffle=True
+)
+
+print("Previewing batch:")
+batch = next(iter(train_dataloader))
+grid = torchvision.utils.make_grid(batch["images"], nrow=4)
+plt.imshow(grid.permute(1, 2, 0).cpu().clip(-1, 1) * 0.5 + 0.5);
+```
+
+ Using custom data configuration huggan--smithsonian_butterflies_subset-7665b1021a37404c
+ Found cached dataset parquet (/home/lewis_huggingface_co/.cache/huggingface/datasets/huggan___parquet/huggan--smithsonian_butterflies_subset-7665b1021a37404c/0.0.0/2a3b91fbd88a2c90d1dbbb32b460cf621d31bd5b05b934492fdef7d8d6f236ec)
+
+
+ Previewing batch:
+
+
+
+
+
+
+
+
+**考虑因素1:** 我们这里使用的 batch size 很小(只有 4),因为我们的训练是基于较大的图片尺寸的(256px),并且我们的模型也很大,如果我们的 batch size 太高,GPU 的内存可能会不够用了。你可以减小图片尺寸,换取更大的 batch size 来加速训练,但这里的模型一开始都是基于生成 256px 尺寸的图片来设计和训练的。
+
+现在我们看看训练循环。我们把要优化的目标参数设定为`image_pipe.unet.parameters()`,以此来更新预训练过的模型的权重。其它部分的代码基本上和第一单元例子中的对应部分一样。在 Colab 上跑的话,大约需要10分钟,你可以趁这个时间喝杯茶休息一下。
+
+
+```python
+num_epochs = 2 # @param
+lr = 1e-5 # 2param
+grad_accumulation_steps = 2 # @param
+
+optimizer = torch.optim.AdamW(image_pipe.unet.parameters(), lr=lr)
+
+losses = []
+
+for epoch in range(num_epochs):
+ for step, batch in tqdm(enumerate(train_dataloader), total=len(train_dataloader)):
+ clean_images = batch["images"].to(device)
+ # Sample noise to add to the images
+ noise = torch.randn(clean_images.shape).to(clean_images.device)
+ bs = clean_images.shape[0]
+
+ # Sample a random timestep for each image
+ timesteps = torch.randint(
+ 0,
+ image_pipe.scheduler.num_train_timesteps,
+ (bs,),
+ device=clean_images.device,
+ ).long()
+
+ # Add noise to the clean images according to the noise magnitude at each timestep
+ # (this is the forward diffusion process)
+ noisy_images = image_pipe.scheduler.add_noise(clean_images, noise, timesteps)
+
+ # Get the model prediction for the noise
+ noise_pred = image_pipe.unet(noisy_images, timesteps, return_dict=False)[0]
+
+ # Compare the prediction with the actual noise:
+ loss = F.mse_loss(
+ noise_pred, noise
+ ) # NB - trying to predict noise (eps) not (noisy_ims-clean_ims) or just (clean_ims)
+
+ # Store for later plotting
+ losses.append(loss.item())
+
+ # Update the model parameters with the optimizer based on this loss
+ loss.backward(loss)
+
+ # Gradient accumulation:
+ if (step + 1) % grad_accumulation_steps == 0:
+ optimizer.step()
+ optimizer.zero_grad()
+
+ print(
+ f"Epoch {epoch} average loss: {sum(losses[-len(train_dataloader):])/len(train_dataloader)}"
+ )
+
+# Plot the loss curve:
+plt.plot(losses)
+```
+
+
+ 0%| | 0/250 [00:00, ?it/s]
+
+
+ Epoch 0 average loss: 0.013324214214226231
+
+
+
+ 0%| | 0/250 [00:00, ?it/s]
+
+
+ Epoch 1 average loss: 0.014018508377484978
+
+
+
+
+
+ []
+
+
+
+
+
+
+
+
+
+**考虑因素2:** 我们的损失值曲线简直像噪声一样混乱!这是因为每一次迭代我们都只用了四个训练样本,而且加到它们上面的噪声水平还都是随机挑选的。这对于训练来讲并不理想。一种弥补的措施是,我们使用一个非常小的学习率,限制每次更新的幅度。但我们还有一个更好的方法,既能得到和使用更大的 batch size 一样的收益,又不需要让我们的内存爆掉。
+
+点击这里看看:[gradient accumulation](https://kozodoi.me/python/deep%20learning/pytorch/tutorial/2021/02/19/gradient-accumulation.html#:~:text=Simply%20speaking%2C%20gradient%20accumulation%20means,might%20find%20this%20tutorial%20useful.)。如果我们多运行几次`loss.backward()`后再调用`optimizer.step()`和`optimizer.zero_grad()`,PyTorch 就会把梯度累积(加和)起来,这样多个批次的数据产生的更新信号就会被高效地融合在一起,产出一个单独的(更好的)梯度估计用于参数更新。这样做会减少参数更新的总次数,就正如我们使用更大的 batch size 时希望看到的一样。梯度累积是一个很多框架都会替你做的事情(比如这里:[🤗 Accelerate makes this easy](https://huggingface.co/docs/accelerate/usage_guides/gradient_accumulation)),但这里我们从头实现一遍也挺好的,因为这对你在 GPU 内存受限时训练模型非常有帮助。正如你在上面代码中看到的那样(在注释 `# Gradient accumulation` 后),其实也不需要你写很多代码。
+
+
+```python
+# 练习:试试你能不能把梯度累积加到第一单元的训练循环中
+# 怎么做呢?你应该怎么基于梯度累积的步数来调整学习率?
+# 学习率应该和之前一样吗?
+```
+
+**考虑因素3:** 即使这样,我们的训练还是挺慢的,而且每遍历完一轮数据集才打印出一行更新,这也不足以让我们知道我们的训练到底怎样了。我们也许还应该:
+
+* 训练过程中时不时地生成点图像样本,供我们检查模型性能
+* 在训练过程中,把诸如损失值和生成的图片样本在内的一些东西记录到日志里。你可以使用诸如 Weights and Biases 或 tensorboard 之类的工具
+
+我创建了一个快速的脚本程序([`finetune_model.py`](https://github.com/huggingface/diffusion-models-class/blob/main/unit2/finetune_model.py)),使用了上述的训练代码并加入了少量日志记录功能。你可以[在这里看看一次训练的日志](https://wandb.ai/johnowhitaker/dm_finetune/runs/2upaa341):
+
+
+```python
+%wandb johnowhitaker/dm_finetune/2upaa341 # You'll need a W&B account for this to work - skip if you don't want to log in
+```
+
+
+
+
+
+观察随着训练进展生成的样本图片如何变化也挺好玩 —— 即使从损失值看它好像并没有改进,但我们也能看到一个从原有图像分布(卧室图片)到新的数据集(wikiart 数据集)逐渐演变的过程。在这一节笔记本最后还有一些被注释掉的用于微调的代码,可以使用该脚本程序替代你运行上面的代码块。
+
+
+```python
+# 练习: 看看你能不能修改第一单元的官方示例训练脚本程序
+# 尝试使用预训练的模型,而不是从头开始训练
+# 对比一下上面链接的最小化脚本 —— 对比一下哪些额外功能是最小化脚本没有的?
+```
+
+用这个模型生成点图片,我们可以看到这些脸看起来极其奇怪!
+
+
+```python
+# @markdown Generate and plot some images:
+x = torch.randn(8, 3, 256, 256).to(device) # Batch of 8
+for i, t in tqdm(enumerate(scheduler.timesteps)):
+ model_input = scheduler.scale_model_input(x, t)
+ with torch.no_grad():
+ noise_pred = image_pipe.unet(model_input, t)["sample"]
+ x = scheduler.step(noise_pred, t, x).prev_sample
+grid = torchvision.utils.make_grid(x, nrow=4)
+plt.imshow(grid.permute(1, 2, 0).cpu().clip(-1, 1) * 0.5 + 0.5);
+```
+
+
+ 0it [00:00, ?it/s]
+
+
+
+
+
+
+
+
+**考虑因素4:** 微调这个过程可能是难以预知的。如果我们训练很长时间,我们也许能看见一些生成得很完美的蝴蝶,但中间过程从模型自身讲也极其有趣,尤其是你对艺术风格感兴趣时!你可以试试短时间或长时间地观察一下训练过程,并试着该百年学习率,看看这会怎么影响模型的最终输出。
+
+### 代码:用我们在WikiArt上面使用最小化样例脚本去微调一个模型
+
+如果你想训练一个和我在 WikiArt 类似的模型,你可以去掉代码注释符号,运行下面的代码块。由于这很耗时间和 GPU 内存,我建议你学完这节笔记本后再做。
+
+
+```python
+## 下载微调用的脚本:
+# !wget https://github.com/huggingface/diffusion-models-class/raw/main/unit2/finetune_model.py
+```
+
+
+```python
+## 运行脚本,在Vintage Face数据集上训练脚本
+## (最好在终端里跑):
+# !python finetune_model.py --image_size 128 --batch_size 8 --num_epochs 16\
+# --grad_accumulation_steps 2 --start_model "google/ddpm-celebahq-256"\
+# --dataset_name "Norod78/Vintage-Faces-FFHQAligned" --wandb_project 'dm-finetune'\
+# --log_samples_every 100 --save_model_every 1000 --model_save_name 'vintageface'
+```
+
+### 保存和载入微调过的管线
+
+现在我们已经微调好了我们扩散模型中的 U-Net,我们可以通过下面代码将它保存到本地文件夹中:
+
+
+```python
+image_pipe.save_pretrained("my-finetuned-model")
+```
+
+和我们在第一单元见到的一样,这个过程保存了配置文件、模型、调度器三个东西。
+
+
+```python
+!ls {"my-finetuned-model"}
+```
+
+ model_index.json scheduler unet
+
+
+接下来你可以仿照第一单元 [Introduction to Diffusers](https://github.com/darcula1993/diffusion-models-class-CN/blob/main/unit1/01_introduction_to_diffusers_CN.ipynb) 中的内容把模型上传到Hub中:
+
+
+```python
+# @title Upload a locally saved pipeline to the hub
+
+# Code to upload a pipeline saved locally to the hub
+from huggingface_hub import HfApi, ModelCard, create_repo, get_full_repo_name
+
+# Set up repo and upload files
+model_name = "ddpm-celebahq-finetuned-butterflies-2epochs" # @param What you want it called on the hub
+local_folder_name = "my-finetuned-model" # @param Created by the script or one you created via image_pipe.save_pretrained('save_name')
+description = "Describe your model here" # @param
+hub_model_id = get_full_repo_name(model_name)
+create_repo(hub_model_id)
+api = HfApi()
+api.upload_folder(
+ folder_path=f"{local_folder_name}/scheduler", path_in_repo="", repo_id=hub_model_id
+)
+api.upload_folder(
+ folder_path=f"{local_folder_name}/unet", path_in_repo="", repo_id=hub_model_id
+)
+api.upload_file(
+ path_or_fileobj=f"{local_folder_name}/model_index.json",
+ path_in_repo="model_index.json",
+ repo_id=hub_model_id,
+)
+
+# Add a model card (optional but nice!)
+content = f"""
+---
+license: mit
+tags:
+- pytorch
+- diffusers
+- unconditional-image-generation
+- diffusion-models-class
+---
+
+# Example Fine-Tuned Model for Unit 2 of the [Diffusion Models Class 🧨](https://github.com/huggingface/diffusion-models-class)
+
+{description}
+
+## Usage
+
+```python
+from diffusers import DDPMPipeline
+
+pipeline = DDPMPipeline.from_pretrained('{hub_model_id}')
+image = pipeline().images[0]
+image
+```
+"""
+
+card = ModelCard(content)
+card.push_to_hub(hub_model_id)
+```
+
+
+
+
+ 'https://huggingface.co/lewtun/ddpm-celebahq-finetuned-butterflies-2epochs/blob/main/README.md'
+
+
+
+祝贺!你现在微调完了你的第一个扩散模型!
+
+剩下的部分我将使用一个从[这个在 LSUM bedrooms 数据集上训练过的模型](https://huggingface.co/google/ddpm-bedroom-256)开始在 WikiArt 数据集上微调了大约一轮的[新模型](https://huggingface.co/johnowhitaker/sd-class-wikiart-from-bedrooms)。你也可以跳过这部分代码,使用我们微调过的人脸或蝴蝶管线,或者直接从 Hub 上加载一个模型。
+
+
+```python
+# Load the pretrained pipeline
+pipeline_name = "johnowhitaker/sd-class-wikiart-from-bedrooms"
+image_pipe = DDPMPipeline.from_pretrained(pipeline_name).to(device)
+
+# Sample some images with a DDIM Scheduler over 40 steps
+scheduler = DDIMScheduler.from_pretrained(pipeline_name)
+scheduler.set_timesteps(num_inference_steps=40)
+
+# Random starting point (batch of 8 images)
+x = torch.randn(8, 3, 256, 256).to(device)
+
+# Minimal sampling loop
+for i, t in tqdm(enumerate(scheduler.timesteps)):
+ model_input = scheduler.scale_model_input(x, t)
+ with torch.no_grad():
+ noise_pred = image_pipe.unet(model_input, t)["sample"]
+ x = scheduler.step(noise_pred, t, x).prev_sample
+
+# View the results
+grid = torchvision.utils.make_grid(x, nrow=4)
+plt.imshow(grid.permute(1, 2, 0).cpu().clip(-1, 1) * 0.5 + 0.5);
+```
+
+
+ Downloading: 0%| | 0.00/180 [00:00, ?B/s]
+
+
+
+ Fetching 4 files: 0%| | 0/4 [00:00, ?it/s]
+
+
+
+ Downloading: 0%| | 0.00/938 [00:00, ?B/s]
+
+
+
+ Downloading: 0%| | 0.00/455M [00:00, ?B/s]
+
+
+
+ Downloading: 0%| | 0.00/288 [00:00, ?B/s]
+
+
+
+ 0it [00:00, ?it/s]
+
+
+
+
+
+
+
+
+**考虑因素5:** 一般而言,判断一次微调到底有多管用并不容易,而且“足够好的性能”在不同应用场景下代表什么水平也会有所不同。比如,如果你在一个很小的数据集上微调一个文本条件模型,比如stable diffusion模型,你可能希望模型极可能**保留**它原始训练所学习的东西以便于它能理解你的数据集没有涵盖的各种文本提示;同时你又希望它**适配**你的数据,以便它生成的东西和你的数据风格一致。这可能意味着,你需要使用一个很低的学习率,并配合对模型进行指数平均,就像[这个关于创建一个宝可梦版 stable diffusion 模型的博客](https://lambdalabs.com/blog/how-to-fine-tune-stable-diffusion-how-we-made-the-text-to-pokemon-model-at-lambda)中的做法一样。别的情况下,你可能还想在一个新数据集上完全重训一个模型(就像我们前面从卧室图片到 wikiart 图片微调一样),那你就需要大的学习率和长时间训练了。即使从这里的[损失值曲线](https://wandb.ai/johnowhitaker/dm_finetune/runs/2upaa341)看不出模型在进步,但生成样本已经很清楚地显示出了一个从原始数据到更有艺术范的风格迁移的过程了,虽然看着还是不太协调。
+
+这就带领我们来到了下一部分,让我们看看怎样对这种模型施加额外的引导,来更好地控制模型的输出。
+
+## 引导
+
+如果我们想对生成的样本施加点控制,那需要怎么做呢?例如,我们想让生成的图片偏向于靠近某种颜色。该怎么做呢?这里我们要介绍**引导(guidance)**,它可以用来在采样的过程中施加额外控制。
+
+第一步,我们先创建一个函数,定义我们希望优化的一个指标(损失值)。这里是一个让生成的图片趋向于某种颜色的例子,它将图片像素值和目标颜色(这里用的是一种浅蓝绿色)对比,返回平均的误差:
+
+
+```python
+def color_loss(images, target_color=(0.1, 0.9, 0.5)):
+ """Given a target color (R, G, B) return a loss for how far away on average
+ the images' pixels are from that color. Defaults to a light teal: (0.1, 0.9, 0.5)"""
+ target = (
+ torch.tensor(target_color).to(images.device) * 2 - 1
+ ) # Map target color to (-1, 1)
+ target = target[
+ None, :, None, None
+ ] # Get shape right to work with the images (b, c, h, w)
+ error = torch.abs(
+ images - target
+ ).mean() # Mean absolute difference between the image pixels and the target color
+ return error
+```
+
+接下来,我们要修改采样循环,在每一步,我们要做这些事情:
+- 创建一个新版的 x,并且 `requires_grad = True`
+- 算出去噪后的版本(x0)
+- 将预测出的x0送入我们的损失函数中
+- 找到这个损失函数对于 x 的**梯度**
+- 在我们使用调度器前,用这个梯度去修改 x ,希望 x 朝着能减低损失值的方向改进
+
+这里有两种实现方法,你可以探索一下哪一种更好。第一,我们是在从 UNet 得到噪声预测后才给 x 设置 requires_grad 的,这样对内存来讲更高效一点(因为我们不用穿过扩散模型去追踪梯度),但这样做梯度的精度会低一点。第二种方法是,我们先给 x 设置 requires_grad,然后再送入 UNet 并计算预测出的 x0。
+
+
+```python
+# Variant 1: shortcut method
+
+# The guidance scale determines the strength of the effect
+guidance_loss_scale = 40 # Explore changing this to 5, or 100
+
+x = torch.randn(8, 3, 256, 256).to(device)
+
+for i, t in tqdm(enumerate(scheduler.timesteps)):
+
+ # Prepare the model input
+ model_input = scheduler.scale_model_input(x, t)
+
+ # predict the noise residual
+ with torch.no_grad():
+ noise_pred = image_pipe.unet(model_input, t)["sample"]
+
+ # Set x.requires_grad to True
+ x = x.detach().requires_grad_()
+
+ # Get the predicted x0
+ x0 = scheduler.step(noise_pred, t, x).pred_original_sample
+
+ # Calculate loss
+ loss = color_loss(x0) * guidance_loss_scale
+ if i % 10 == 0:
+ print(i, "loss:", loss.item())
+
+ # Get gradient
+ cond_grad = -torch.autograd.grad(loss, x)[0]
+
+ # Modify x based on this gradient
+ x = x.detach() + cond_grad
+
+ # Now step with scheduler
+ x = scheduler.step(noise_pred, t, x).prev_sample
+
+# View the output
+grid = torchvision.utils.make_grid(x, nrow=4)
+im = grid.permute(1, 2, 0).cpu().clip(-1, 1) * 0.5 + 0.5
+Image.fromarray(np.array(im * 255).astype(np.uint8))
+```
+
+
+ 0it [00:00, ?it/s]
+
+
+ 0 loss: 27.279136657714844
+ 10 loss: 11.286816596984863
+ 20 loss: 10.683112144470215
+ 30 loss: 10.942476272583008
+
+
+
+
+
+
+
+
+
+
+
+这里的第二种实现方法需要几乎第一种的两倍的 GPU 内存,即使这里我们用的 batch size 是 4 而不是 8。试试看你能不能看出点不同?想想为什么这里梯度更精确?
+
+
+```python
+# Variant 2: setting x.requires_grad before calculating the model predictions
+
+guidance_loss_scale = 40
+x = torch.randn(4, 3, 256, 256).to(device)
+
+for i, t in tqdm(enumerate(scheduler.timesteps)):
+
+ # Set requires_grad before the model forward pass
+ x = x.detach().requires_grad_()
+ model_input = scheduler.scale_model_input(x, t)
+
+ # predict (with grad this time)
+ noise_pred = image_pipe.unet(model_input, t)["sample"]
+
+ # Get the predicted x0:
+ x0 = scheduler.step(noise_pred, t, x).pred_original_sample
+
+ # Calculate loss
+ loss = color_loss(x0) * guidance_loss_scale
+ if i % 10 == 0:
+ print(i, "loss:", loss.item())
+
+ # Get gradient
+ cond_grad = -torch.autograd.grad(loss, x)[0]
+
+ # Modify x based on this gradient
+ x = x.detach() + cond_grad
+
+ # Now step with scheduler
+ x = scheduler.step(noise_pred, t, x).prev_sample
+
+
+grid = torchvision.utils.make_grid(x, nrow=4)
+im = grid.permute(1, 2, 0).cpu().clip(-1, 1) * 0.5 + 0.5
+Image.fromarray(np.array(im * 255).astype(np.uint8))
+```
+
+
+ 0it [00:00, ?it/s]
+
+
+ 0 loss: 30.750328063964844
+ 10 loss: 18.550724029541016
+ 20 loss: 17.515094757080078
+ 30 loss: 17.55681037902832
+
+
+
+
+
+
+
+
+
+
+
+在第二种实现方法中,内存的需求更高了,但颜色迁移的效果却减弱了。你可能觉得这种方法其实不如第一种方法。但是,可以说这里的输出更接近于训练模型所使用的数据,你也永远可以通过增大 `guidance_loss_scale` 来加强颜色迁移的效果。使用哪种方案其实最终取决于哪种方案在实验中效果更好。
+
+
+```python
+# 练习:选出你最喜欢的颜色并在RGB空间中找出它的值
+# 修改上面代码的 color_loss(),将颜色改成你选的颜色,并检查输出 —— 输出和你期望的适配吗?
+```
+
+## CLIP 引导
+
+引导生成的图片向某种颜色倾斜确实让我们多少对生成有所控制,但如果我们能仅仅打几行字描述一下就得到我们想要的图片呢?
+
+[CLIP](https://openai.com/blog/clip/) 是一个由 OpenAI 开发的模型,它可以让我们拿图片和文字说明去作比较。这是个非常强大的功能,因为它让我们能量化一张图和一句提示语有多匹配。另外,由于这个过程是可微分的,我们可以使用它作为损失函数去引导我们的扩散模型。
+
+这里我们不深究细节。基本的方法是:
+- 给文字提示语做嵌入(embedding),为 CLIP 获取一个 512 维的 embedding
+- 对于扩散模型的生成过程的每一步:
+ - 做出多个不同版本的预测出来的去噪图片(不同的变种可以提供一个更干净的损失信号)
+ - 对每一个预测出的去噪图片,用 CLIP 给图片做嵌入(embedding),并将这个嵌入和文字的嵌入做对比(用一种叫 Great Circle Distance Squared 的度量方法)
+- 计算这个损失对于当前带噪的 x 的梯度,并在用调度器(scheduler)更新它之前用这个梯度去修改 x
+
+如果你想看关于 CLIP 的更深入的讲解,你可以看看[这个课程](https://johnowhitaker.github.io/tglcourse/clip.html)或[这个关于 OpenCLIP 的报告](https://wandb.ai/johnowhitaker/openclip-benchmarking/reports/Exploring-OpenCLIP--VmlldzoyOTIzNzIz),我们就是用 OpenCLIP 载入 CLIP 模型的。运行下列代码就可以载入一个 CLIP 模型:
+
+
+```python
+# @markdown load a CLIP model and define the loss function
+import open_clip
+
+clip_model, _, preprocess = open_clip.create_model_and_transforms(
+ "ViT-B-32", pretrained="openai"
+)
+clip_model.to(device)
+
+# Transforms to resize and augment an image + normalize to match CLIP's training data
+tfms = torchvision.transforms.Compose(
+ [
+ torchvision.transforms.RandomResizedCrop(224), # Random CROP each time
+ torchvision.transforms.RandomAffine(
+ 5
+ ), # One possible random augmentation: skews the image
+ torchvision.transforms.RandomHorizontalFlip(), # You can add additional augmentations if you like
+ torchvision.transforms.Normalize(
+ mean=(0.48145466, 0.4578275, 0.40821073),
+ std=(0.26862954, 0.26130258, 0.27577711),
+ ),
+ ]
+)
+
+# And define a loss function that takes an image, embeds it and compares with
+# the text features of the prompt
+def clip_loss(image, text_features):
+ image_features = clip_model.encode_image(
+ tfms(image)
+ ) # Note: applies the above transforms
+ input_normed = torch.nn.functional.normalize(image_features.unsqueeze(1), dim=2)
+ embed_normed = torch.nn.functional.normalize(text_features.unsqueeze(0), dim=2)
+ dists = (
+ input_normed.sub(embed_normed).norm(dim=2).div(2).arcsin().pow(2).mul(2)
+ ) # Squared Great Circle Distance
+ return dists.mean()
+```
+
+ 100%|████████████████████████████████████████| 354M/354M [00:02<00:00, 120MiB/s]
+
+
+这里也定义了一个损失函数,我们这里引导的采样循环看起来和前面的例子很像,仅仅是把`color_loss()`换成了新的基于CLIP的损失函数:
+
+
+```python
+# @markdown applying guidance using CLIP
+
+prompt = "Red Rose (still life), red flower painting" # @param
+
+# Explore changing this
+guidance_scale = 8 # @param
+n_cuts = 4 # @param
+
+# More steps -> more time for the guidance to have an effect
+scheduler.set_timesteps(50)
+
+# We embed a prompt with CLIP as our target
+text = open_clip.tokenize([prompt]).to(device)
+with torch.no_grad(), torch.cuda.amp.autocast():
+ text_features = clip_model.encode_text(text)
+
+
+x = torch.randn(4, 3, 256, 256).to(
+ device
+) # RAM usage is high, you may want only 1 image at a time
+
+for i, t in tqdm(enumerate(scheduler.timesteps)):
+
+ model_input = scheduler.scale_model_input(x, t)
+
+ # predict the noise residual
+ with torch.no_grad():
+ noise_pred = image_pipe.unet(model_input, t)["sample"]
+
+ cond_grad = 0
+
+ for cut in range(n_cuts):
+
+ # Set requires grad on x
+ x = x.detach().requires_grad_()
+
+ # Get the predicted x0:
+ x0 = scheduler.step(noise_pred, t, x).pred_original_sample
+
+ # Calculate loss
+ loss = clip_loss(x0, text_features) * guidance_scale
+
+ # Get gradient (scale by n_cuts since we want the average)
+ cond_grad -= torch.autograd.grad(loss, x)[0] / n_cuts
+
+ if i % 25 == 0:
+ print("Step:", i, ", Guidance loss:", loss.item())
+
+ # Modify x based on this gradient
+ alpha_bar = scheduler.alphas_cumprod[i]
+ x = (
+ x.detach() + cond_grad * alpha_bar.sqrt()
+ ) # Note the additional scaling factor here!
+
+ # Now step with scheduler
+ x = scheduler.step(noise_pred, t, x).prev_sample
+
+
+grid = torchvision.utils.make_grid(x.detach(), nrow=4)
+im = grid.permute(1, 2, 0).cpu().clip(-1, 1) * 0.5 + 0.5
+Image.fromarray(np.array(im * 255).astype(np.uint8))
+```
+
+
+ 0it [00:00, ?it/s]
+
+
+ Step: 0 , Guidance loss: 7.437869548797607
+ Step: 25 , Guidance loss: 7.174620628356934
+
+
+
+
+
+
+
+
+
+
+
+看起来有点像玫瑰!虽然还不够完美,但你如果接着调一调设定参数,你可以得到一些更令人满意的图片。
+
+如果你仔细看上面的代码,你会发现我在使用`alpha_bar.sqrt()`作为因子去缩放梯度。虽然理论上有所谓正确的缩放这些梯度的方法,但在实践中,你可以用实验去验证。对于有些引导来说,你可能希望大部分的引导作用都集中在刚开始的几步里,对于另一些(比如一些关注点在纹理方面的风格损失函数)来讲,你可能希望它仅在生成过程的结束部分加入进来。对此,下面展示一些可能的方案:
+
+
+```python
+# @markdown Plotting some possible schedules:
+plt.plot([1 for a in scheduler.alphas_cumprod], label="no scaling")
+plt.plot([a for a in scheduler.alphas_cumprod], label="alpha_bar")
+plt.plot([a.sqrt() for a in scheduler.alphas_cumprod], label="alpha_bar.sqrt()")
+plt.plot(
+ [(1 - a).sqrt() for a in scheduler.alphas_cumprod], label="(1-alpha_bar).sqrt()"
+)
+plt.legend()
+plt.title("Possible guidance scaling schedules");
+```
+
+
+
+
+
+
+
+你可以做点实验,针对不同的调整方案、引导规模大小(`guidance_scale`),以及任何你能想到的小技巧(比如用一个范围去截断梯度也很常见),试试你能把效果做到多好。也可以在其它模型上试试,比如我们最开始使用的那个人脸模型,你能很可靠地让它生成男性脸吗?如果你把 CLIP 引导和前面我们用过地基于颜色的损失函数结合起来用呢?
+
+如果你看[一些实操的 CLIP 引导的扩散模型代码](https://huggingface.co/spaces/EleutherAI/clip-guided-diffusion/blob/main/app.py),你会发现一种更复杂的方法:使用更好的类别去选取随机图像裁剪,并对损失函数进行许多额外的调整以获得更好的性能。在文本条件扩散模型出现前,这可是最好的文本到图像转换系统!我们这里的“玩具级”项目有很多可改进的空间,但不管怎样,它抓住了核心要点:借助于我们的引导和 CLIP 惊人的能力,我们可以给一个没有条件约束的扩散模型加上文本级的控制 🎨
+
+## 把自定义的采样训练做成 Gradio 上的展示来分享
+
+也许你现在已经想出了一个很好玩的损失函数去引导生成过程,现在你想把你的微调模型和自定义的采样策略分享给全世界......
+
+点击这里了解 [Gradio](https://gradio.app/)。Gradio 是一个免费的开源工具,让用户可以方便地通过一个简单的网页界面来创建和分享交互式的机器学习模型。使用Gradio,用户可以为自己的机器学习模型自定义接口,然后通过一个唯一的URL共享给他人。Gradio 也别集成入了 🤗 Spaces,使得创建 Demo 和共享给他人变得更容易。
+
+我们将把我们需要的核心逻辑放在一个函数中,这个函数接收一些输入然后输出一张图片作为输出。然后这个函数会被封装在一个简单的接口中,让用户能自己定义一些参数(这些参数是作为输入提供给 generate 函数的)。这里有很多[组件](https://gradio.app/docs/#components) 可以用 —— 在这个例子中我们就加入了一个滑杆来控制引导的力度(guidance scale),以及一个颜色选择器供我们定义目标颜色。
+
+
+```python
+!pip install -q gradio # Install the library
+```
+
+
+```python
+import gradio as gr
+from PIL import Image, ImageColor
+
+
+# The function that does the hard work
+def generate(color, guidance_loss_scale):
+ target_color = ImageColor.getcolor(color, "RGB") # Target color as RGB
+ target_color = [a / 255 for a in target_color] # Rescale from (0, 255) to (0, 1)
+ x = torch.randn(1, 3, 256, 256).to(device)
+ for i, t in tqdm(enumerate(scheduler.timesteps)):
+ model_input = scheduler.scale_model_input(x, t)
+ with torch.no_grad():
+ noise_pred = image_pipe.unet(model_input, t)["sample"]
+ x = x.detach().requires_grad_()
+ x0 = scheduler.step(noise_pred, t, x).pred_original_sample
+ loss = color_loss(x0, target_color) * guidance_loss_scale
+ cond_grad = -torch.autograd.grad(loss, x)[0]
+ x = x.detach() + cond_grad
+ x = scheduler.step(noise_pred, t, x).prev_sample
+ grid = torchvision.utils.make_grid(x, nrow=4)
+ im = grid.permute(1, 2, 0).cpu().clip(-1, 1) * 0.5 + 0.5
+ im = Image.fromarray(np.array(im * 255).astype(np.uint8))
+ im.save("test.jpeg")
+ return im
+
+
+# See the gradio docs for the types of inputs and outputs available
+inputs = [
+ gr.ColorPicker(label="color", value="55FFAA"), # Add any inputs you need here
+ gr.Slider(label="guidance_scale", minimum=0, maximum=30, value=3),
+]
+outputs = gr.Image(label="result")
+
+# And the minimal interface
+demo = gr.Interface(
+ fn=generate,
+ inputs=inputs,
+ outputs=outputs,
+ examples=[
+ ["#BB2266", 3],
+ ["#44CCAA", 5], # You can provide some example inputs to get people started
+ ],
+)
+demo.launch(debug=True) # debug=True allows you to see errors and output in Colab
+```
+
+当然你也可以做出点复杂得多的接口,并加入点炫酷的风格和很长的输入序列。这里我们只做最简单的演示。
+
+在 🤗 Spaces 上的演示 demo 都是默认用 CPU 跑的,所以在你移交之前,把你的接口在 Colab 上制作原型(如上所示)也是很不错的选择。当你准备好你的 demo 时,你需要创建一个 space,按照 `requirements.txt` 安装程序所需的库,然后把所都代码都放在一个名为 `app.py` 的文件里,这个文件是用来定义相关函数和接口的。
+
+
+
+幸运的是,你也可以复制一个 space。你可以在[这里](https://huggingface.co/spaces/johnowhitaker/color-guided-wikiart-diffusion)看看我的 demo,然后点击“Duplicate this Space”用我的代码作为一个模板,用于你后续修改代码来添加你自己的模型和引导函数。
+
+在设置选项中,你也可以配置你的 space,让它在更厉害的硬件上跑(当然会按小时收费)。如果你确实做出了一些很惊艳的东西、想在更好的硬件上分享它,可是你却不想花钱?那你可以在 Discord 上告诉我们,看我们能不能提供点帮助。
+
+## 总结和下一步的工作
+
+本节笔记本涵盖了好多内容啊!让我们回顾一下核心要点:
+- 载入一个现有模型并用不同的调度器(scheduler)去采样其实很容易
+- 微调(fine-tuning)看起来很像从头训练一个模型,唯一不同的是我们用已有的模型做初始化,以此我们希望能快点得到更好效果
+- 如果在大尺寸图片上微调大模型,我们可以用诸如梯度累积(gradient accumulation)的方法去应对训练时batch size太小的问题
+- 把采样的图片保存到日志里对微调很重要,而损失值曲线却可能无法反映有用的信息
+- 引导(guidance)可以让我们在使用一个没有条件约束的模型时,通过一些引导或损失函数来掌控生成过程。这里我们每步都会找一个损失对于带噪图片x的梯度,然后用梯度更新这个带噪图片,之后再进入下一个生成迭代
+- 用CLIP引导让我们可以用文字描述去控制一个没有条件约束的模型的生成过程!
+
+
+如果你想在实践中运用这些知识,你还可以做这些:
+- 微调你自己的模型并把它上传到 Hub。这包括:首先找一个起始点(比如一个在 [faces](https://huggingface.co/google/ddpm-celebahq-256)、[bedrooms](https://huggingface.co/fusing/ddpm-lsun-bedroom)、[cats](https://huggingface.co/fusing/ddpm-lsun-cat) 或 [wikiart example above](https://huggingface.co/johnowhitaker/sd-class-wikiart-from-bedrooms) 数据集上训练过的模型)和一个新的数据集(也许你可以用这个 [animal faces](https://huggingface.co/datasets/huggan/AFHQv2),或者你自己的图片);然后跑一跑这个笔记本中的代码或样例脚本程序。
+- 用你的微调过的模型探索一下引导,你可以用我们例子中的引导函数(颜色损失函数或 CLIP),也可以自己创造一个。
+- 把你的 demo 分享到 Gradio 上,你可以通过修改这里的 [space示例](https://huggingface.co/spaces/johnowhitaker/color-guided-wikiart-diffusion),也可以创建你自己的有更多功能的 space
+
+我们期待在 Discord、Twitter 或其它地方看到你的作品!🤗
+
+
+```python
+
+```
diff --git a/markdown/unit2/01_finetuning_and_guidance_CN_files/01_finetuning_and_guidance_CN_17_1.png b/markdown/unit2/01_finetuning_and_guidance_CN_files/01_finetuning_and_guidance_CN_17_1.png
new file mode 100644
index 0000000000000000000000000000000000000000..c228a19e2a0f7c91c38e05b24bd148c42551e3e2
--- /dev/null
+++ b/markdown/unit2/01_finetuning_and_guidance_CN_files/01_finetuning_and_guidance_CN_17_1.png
@@ -0,0 +1,3 @@
+version https://git-lfs.github.com/spec/v1
+oid sha256:4dcdabf9d36dea189f287d9fcf89d6f0ec610d11ffecbddabf8e53ac731a0fbf
+size 270845
diff --git a/markdown/unit2/01_finetuning_and_guidance_CN_files/01_finetuning_and_guidance_CN_17_2.png b/markdown/unit2/01_finetuning_and_guidance_CN_files/01_finetuning_and_guidance_CN_17_2.png
new file mode 100644
index 0000000000000000000000000000000000000000..a58e89bfd76d2ecb6e90e67779cddcac50c00c0c
--- /dev/null
+++ b/markdown/unit2/01_finetuning_and_guidance_CN_files/01_finetuning_and_guidance_CN_17_2.png
@@ -0,0 +1,3 @@
+version https://git-lfs.github.com/spec/v1
+oid sha256:9ff0d9e4f3ab0f7dda7364bcc13e80623932508af68a9e690f7e5de8297262b3
+size 242990
diff --git a/markdown/unit2/01_finetuning_and_guidance_CN_files/01_finetuning_and_guidance_CN_17_3.png b/markdown/unit2/01_finetuning_and_guidance_CN_files/01_finetuning_and_guidance_CN_17_3.png
new file mode 100644
index 0000000000000000000000000000000000000000..b7275d5a242335efded008cc714c7b239027b2c8
--- /dev/null
+++ b/markdown/unit2/01_finetuning_and_guidance_CN_files/01_finetuning_and_guidance_CN_17_3.png
@@ -0,0 +1,3 @@
+version https://git-lfs.github.com/spec/v1
+oid sha256:0333f5898afdad1a280462a2aa149160bf0572b1617d6de597bcf3ab55c3b72d
+size 239058
diff --git a/markdown/unit2/01_finetuning_and_guidance_CN_files/01_finetuning_and_guidance_CN_17_4.png b/markdown/unit2/01_finetuning_and_guidance_CN_files/01_finetuning_and_guidance_CN_17_4.png
new file mode 100644
index 0000000000000000000000000000000000000000..30cda52503227fc662f4a51d07ae36e6d409cfac
--- /dev/null
+++ b/markdown/unit2/01_finetuning_and_guidance_CN_files/01_finetuning_and_guidance_CN_17_4.png
@@ -0,0 +1,3 @@
+version https://git-lfs.github.com/spec/v1
+oid sha256:577cf30a7704bb7b363eefbbd9c9f50e64d5f03bad73e8365e8c25f38cd01769
+size 233565
diff --git a/markdown/unit2/01_finetuning_and_guidance_CN_files/01_finetuning_and_guidance_CN_17_5.png b/markdown/unit2/01_finetuning_and_guidance_CN_files/01_finetuning_and_guidance_CN_17_5.png
new file mode 100644
index 0000000000000000000000000000000000000000..d296ccfb032d1a946bdcc70e817493f03dd0926d
--- /dev/null
+++ b/markdown/unit2/01_finetuning_and_guidance_CN_files/01_finetuning_and_guidance_CN_17_5.png
@@ -0,0 +1,3 @@
+version https://git-lfs.github.com/spec/v1
+oid sha256:2f29b2d4140b7b45e4981e7c94aff18f9f048108046f8e22cbfb6ebb4e70f576
+size 104020
diff --git a/markdown/unit2/01_finetuning_and_guidance_CN_files/01_finetuning_and_guidance_CN_21_1.png b/markdown/unit2/01_finetuning_and_guidance_CN_files/01_finetuning_and_guidance_CN_21_1.png
new file mode 100644
index 0000000000000000000000000000000000000000..8a37ed5cd63520b727255f3b9c4f69ab86d3ae4f
--- /dev/null
+++ b/markdown/unit2/01_finetuning_and_guidance_CN_files/01_finetuning_and_guidance_CN_21_1.png
@@ -0,0 +1,3 @@
+version https://git-lfs.github.com/spec/v1
+oid sha256:d449820a0486e1f994899e3ba14082c63c4668e29e57f8111f440161a02eefe4
+size 87803
diff --git a/markdown/unit2/01_finetuning_and_guidance_CN_files/01_finetuning_and_guidance_CN_25_2.png b/markdown/unit2/01_finetuning_and_guidance_CN_files/01_finetuning_and_guidance_CN_25_2.png
new file mode 100644
index 0000000000000000000000000000000000000000..65e7bbb13082149992daef20bf912de492e06fae
--- /dev/null
+++ b/markdown/unit2/01_finetuning_and_guidance_CN_files/01_finetuning_and_guidance_CN_25_2.png
@@ -0,0 +1,3 @@
+version https://git-lfs.github.com/spec/v1
+oid sha256:bb0823471f82961aa59c6b38c91d0fdc6310e7cf72fc370ba5490a2e4043d905
+size 109317
diff --git a/markdown/unit2/01_finetuning_and_guidance_CN_files/01_finetuning_and_guidance_CN_28_5.png b/markdown/unit2/01_finetuning_and_guidance_CN_files/01_finetuning_and_guidance_CN_28_5.png
new file mode 100644
index 0000000000000000000000000000000000000000..75944bfcb04f9790651bd4e7f64d2ce50e524a2c
--- /dev/null
+++ b/markdown/unit2/01_finetuning_and_guidance_CN_files/01_finetuning_and_guidance_CN_28_5.png
@@ -0,0 +1,3 @@
+version https://git-lfs.github.com/spec/v1
+oid sha256:bcddc476caaa7391ccef6e9080dd198b56edee8de438c9225f2b5e0b0168f274
+size 40066
diff --git a/markdown/unit2/01_finetuning_and_guidance_CN_files/01_finetuning_and_guidance_CN_36_1.png b/markdown/unit2/01_finetuning_and_guidance_CN_files/01_finetuning_and_guidance_CN_36_1.png
new file mode 100644
index 0000000000000000000000000000000000000000..3a9bc84ed414f72e315a7310869f7ca7998842ae
--- /dev/null
+++ b/markdown/unit2/01_finetuning_and_guidance_CN_files/01_finetuning_and_guidance_CN_36_1.png
@@ -0,0 +1,3 @@
+version https://git-lfs.github.com/spec/v1
+oid sha256:d3f81400d08e6ea2ccfcee780e90bc105a3cd30f09129fd6e97a02c1f70ae53d
+size 268774
diff --git a/markdown/unit2/01_finetuning_and_guidance_CN_files/01_finetuning_and_guidance_CN_48_6.png b/markdown/unit2/01_finetuning_and_guidance_CN_files/01_finetuning_and_guidance_CN_48_6.png
new file mode 100644
index 0000000000000000000000000000000000000000..2374cd014b58570a423d0cbb1fc58218367310d5
--- /dev/null
+++ b/markdown/unit2/01_finetuning_and_guidance_CN_files/01_finetuning_and_guidance_CN_48_6.png
@@ -0,0 +1,3 @@
+version https://git-lfs.github.com/spec/v1
+oid sha256:366a73b8a8f0e3f37df3f493ea38e3622f237679f05c0922ed9f86aa2163e204
+size 196243
diff --git a/markdown/unit2/01_finetuning_and_guidance_CN_files/01_finetuning_and_guidance_CN_54_2.png b/markdown/unit2/01_finetuning_and_guidance_CN_files/01_finetuning_and_guidance_CN_54_2.png
new file mode 100644
index 0000000000000000000000000000000000000000..b3d2f97572d9ecdeb3ff385247bd9a55388c3272
--- /dev/null
+++ b/markdown/unit2/01_finetuning_and_guidance_CN_files/01_finetuning_and_guidance_CN_54_2.png
@@ -0,0 +1,3 @@
+version https://git-lfs.github.com/spec/v1
+oid sha256:750fd94a9f78e7b3949f2cc7c2a8ee7469756d7fa5338429f5ee806e8142ea7a
+size 862827
diff --git a/markdown/unit2/01_finetuning_and_guidance_CN_files/01_finetuning_and_guidance_CN_56_2.png b/markdown/unit2/01_finetuning_and_guidance_CN_files/01_finetuning_and_guidance_CN_56_2.png
new file mode 100644
index 0000000000000000000000000000000000000000..28860f663a19a5a76bd9461771d30a2da2e4277c
--- /dev/null
+++ b/markdown/unit2/01_finetuning_and_guidance_CN_files/01_finetuning_and_guidance_CN_56_2.png
@@ -0,0 +1,3 @@
+version https://git-lfs.github.com/spec/v1
+oid sha256:8031c0e6d6d93c7e8858789d201792c579aa0131b05123ae1641de6db844238a
+size 284275
diff --git a/markdown/unit2/01_finetuning_and_guidance_CN_files/01_finetuning_and_guidance_CN_62_2.png b/markdown/unit2/01_finetuning_and_guidance_CN_files/01_finetuning_and_guidance_CN_62_2.png
new file mode 100644
index 0000000000000000000000000000000000000000..7cd55104bbaf2b2233eaba123a8af239b5a83474
--- /dev/null
+++ b/markdown/unit2/01_finetuning_and_guidance_CN_files/01_finetuning_and_guidance_CN_62_2.png
@@ -0,0 +1,3 @@
+version https://git-lfs.github.com/spec/v1
+oid sha256:96c4fadae520fd3dfb28201758051ac414b86ab98ab215f2a0d48825ce3f4420
+size 478928
diff --git a/markdown/unit2/01_finetuning_and_guidance_CN_files/01_finetuning_and_guidance_CN_65_0.png b/markdown/unit2/01_finetuning_and_guidance_CN_files/01_finetuning_and_guidance_CN_65_0.png
new file mode 100644
index 0000000000000000000000000000000000000000..8bc9df1e832472775c3bf0bcfaebc9fa795203e4
--- /dev/null
+++ b/markdown/unit2/01_finetuning_and_guidance_CN_files/01_finetuning_and_guidance_CN_65_0.png
@@ -0,0 +1,3 @@
+version https://git-lfs.github.com/spec/v1
+oid sha256:aad9f24954016b1d845dfde5bab7dcabdd14a4b98db0b6b578f4fc6144fefb09
+size 42367
diff --git a/markdown/unit2/01_finetuning_and_guidance_CN_files/01_finetuning_and_guidance_CN_9_1.png b/markdown/unit2/01_finetuning_and_guidance_CN_files/01_finetuning_and_guidance_CN_9_1.png
new file mode 100644
index 0000000000000000000000000000000000000000..628be09542390cbf7f25defeba276f8a1fb5e51e
--- /dev/null
+++ b/markdown/unit2/01_finetuning_and_guidance_CN_files/01_finetuning_and_guidance_CN_9_1.png
@@ -0,0 +1,3 @@
+version https://git-lfs.github.com/spec/v1
+oid sha256:598a81891988e5df696b096350f4bd9e8c66474af172ad947ff24399adfc0537
+size 82947
diff --git a/markdown/unit2/02_class_conditioned_diffusion_model_example_CN.md b/markdown/unit2/02_class_conditioned_diffusion_model_example_CN.md
new file mode 100644
index 0000000000000000000000000000000000000000..7c048b56d3e6fe16a89f762be8367ee61205329a
--- /dev/null
+++ b/markdown/unit2/02_class_conditioned_diffusion_model_example_CN.md
@@ -0,0 +1,360 @@
+# 创建一个类别条件扩散模型
+
+在这节笔记本中,我们将阐述一种给扩散模型加条件信息的方法。具体来说,我们将接着[这个从头训练的例子](../unit1/02_diffusion_models_from_scratch_CN.ipynb)在 MNIST 上训练一个以类别为条件的扩散模型。这里我们可以在推理时指定我们要生成的是哪个数字。
+
+就像本单元介绍中说的那样,这只是很多给扩散模型添加额外条件信息的方法中的一种,这里用它做示例是因为它比较简单。就像第一单元中“从走训练”的例子一样,这节笔记本也是为了解释说明的目的。如果你想,你也可以安全地跳过本节。
+
+## 配置和数据准备
+
+
+```python
+!pip install -q diffusers
+```
+
+ [K |████████████████████████████████| 503 kB 7.2 MB/s
+ [K |████████████████████████████████| 182 kB 51.3 MB/s
+ [?25h
+
+
+```python
+import torch
+import torchvision
+from torch import nn
+from torch.nn import functional as F
+from torch.utils.data import DataLoader
+from diffusers import DDPMScheduler, UNet2DModel
+from matplotlib import pyplot as plt
+from tqdm.auto import tqdm
+
+device = 'mps' if torch.backends.mps.is_available() else 'cuda' if torch.cuda.is_available() else 'cpu'
+print(f'Using device: {device}')
+```
+
+ Using device: cuda
+
+
+
+```python
+# Load the dataset
+dataset = torchvision.datasets.MNIST(root="mnist/", train=True, download=True, transform=torchvision.transforms.ToTensor())
+
+# Feed it into a dataloader (batch size 8 here just for demo)
+train_dataloader = DataLoader(dataset, batch_size=8, shuffle=True)
+
+# View some examples
+x, y = next(iter(train_dataloader))
+print('Input shape:', x.shape)
+print('Labels:', y)
+plt.imshow(torchvision.utils.make_grid(x)[0], cmap='Greys');
+```
+
+ Downloading http://yann.lecun.com/exdb/mnist/train-images-idx3-ubyte.gz
+ Downloading http://yann.lecun.com/exdb/mnist/train-images-idx3-ubyte.gz to mnist/MNIST/raw/train-images-idx3-ubyte.gz
+
+
+
+ 0%| | 0/9912422 [00:00, ?it/s]
+
+
+ Extracting mnist/MNIST/raw/train-images-idx3-ubyte.gz to mnist/MNIST/raw
+
+ Downloading http://yann.lecun.com/exdb/mnist/train-labels-idx1-ubyte.gz
+ Downloading http://yann.lecun.com/exdb/mnist/train-labels-idx1-ubyte.gz to mnist/MNIST/raw/train-labels-idx1-ubyte.gz
+
+
+
+ 0%| | 0/28881 [00:00, ?it/s]
+
+
+ Extracting mnist/MNIST/raw/train-labels-idx1-ubyte.gz to mnist/MNIST/raw
+
+ Downloading http://yann.lecun.com/exdb/mnist/t10k-images-idx3-ubyte.gz
+ Downloading http://yann.lecun.com/exdb/mnist/t10k-images-idx3-ubyte.gz to mnist/MNIST/raw/t10k-images-idx3-ubyte.gz
+
+
+
+ 0%| | 0/1648877 [00:00, ?it/s]
+
+
+ Extracting mnist/MNIST/raw/t10k-images-idx3-ubyte.gz to mnist/MNIST/raw
+
+ Downloading http://yann.lecun.com/exdb/mnist/t10k-labels-idx1-ubyte.gz
+ Downloading http://yann.lecun.com/exdb/mnist/t10k-labels-idx1-ubyte.gz to mnist/MNIST/raw/t10k-labels-idx1-ubyte.gz
+
+
+
+ 0%| | 0/4542 [00:00, ?it/s]
+
+
+ Extracting mnist/MNIST/raw/t10k-labels-idx1-ubyte.gz to mnist/MNIST/raw
+
+ Input shape: torch.Size([8, 1, 28, 28])
+ Labels: tensor([8, 1, 5, 9, 7, 6, 2, 2])
+
+
+
+
+
+
+
+
+## 创建一个以类别为条件的 UNet
+
+我们输入类别这一条件的方法是:
+- 创建一个标准的 `UNet2DModel`,加入一些额外的输入通道
+- 通过一个嵌入层,把类别标签映射到一个 `(class_emb_size)` 形状的学到的向量上
+- 把这个信息作为额外通道和原有的输入向量拼接起来,用这行代码:`net_input = torch.cat((x, class_cond), 1)`
+- 把这个 `net_input` (有 `class_emb_size+1` 个通道)输入到UNet中得到最终预测
+
+在这个例子中,我把 class_emb_size 设成4,但这其实是可以任意修改的,你可以试试从把它设成1(你可以看看这有没有用)到把它设成 10(正好是类别总数),或者把需要学到的 nn.Embedding 换成简单的对类别进行独热编码(one-hot encoding)。
+
+具体实现起来就是这样:
+
+
+```python
+class ClassConditionedUnet(nn.Module):
+ def __init__(self, num_classes=10, class_emb_size=4):
+ super().__init__()
+
+ # The embedding layer will map the class label to a vector of size class_emb_size
+ self.class_emb = nn.Embedding(num_classes, class_emb_size)
+
+ # Self.model is an unconditional UNet with extra input channels to accept the conditioning information (the class embedding)
+ self.model = UNet2DModel(
+ sample_size=28, # the target image resolution
+ in_channels=1 + class_emb_size, # Additional input channels for class cond.
+ out_channels=1, # the number of output channels
+ layers_per_block=2, # how many ResNet layers to use per UNet block
+ block_out_channels=(32, 64, 64),
+ down_block_types=(
+ "DownBlock2D", # a regular ResNet downsampling block
+ "AttnDownBlock2D", # a ResNet downsampling block with spatial self-attention
+ "AttnDownBlock2D",
+ ),
+ up_block_types=(
+ "AttnUpBlock2D",
+ "AttnUpBlock2D", # a ResNet upsampling block with spatial self-attention
+ "UpBlock2D", # a regular ResNet upsampling block
+ ),
+ )
+
+ # Our forward method now takes the class labels as an additional argument
+ def forward(self, x, t, class_labels):
+ # Shape of x:
+ bs, ch, w, h = x.shape
+
+ # class conditioning in right shape to add as additional input channels
+ class_cond = self.class_emb(class_labels) # Map to embedding dinemsion
+ class_cond = class_cond.view(bs, class_cond.shape[1], 1, 1).expand(bs, class_cond.shape[1], w, h)
+ # x is shape (bs, 1, 28, 28) and class_cond is now (bs, 4, 28, 28)
+
+ # Net input is now x and class cond concatenated together along dimension 1
+ net_input = torch.cat((x, class_cond), 1) # (bs, 5, 28, 28)
+
+ # Feed this to the unet alongside the timestep and return the prediction
+ return self.model(net_input, t).sample # (bs, 1, 28, 28)
+```
+
+如果你对任何的张量形状或变换感到迷惑,你都可以在代码中加入print来看看相关形状,检查一下是不是和你预设的是一致的。这里我把一些中间变量的形状都注释上了,希望能帮你思路清晰点。
+
+## 训练和采样
+
+不同于别的地方使用的`prediction = unet(x, t)`,这里我们使用`prediction = unet(x, t, y)`,在训练时把正确的标签作为第三个输入送到模型中。在推理阶段,我们可以输入任何我们想要的标签,如果一切正常,那模型就会输出与之匹配的图片。`y`在这里时 MNIST 中的数字标签,值的范围从0到9。
+
+这里的训练循环很像[第一单元的例子](../unit1/02_diffusion_models_from_scratch_CN.ipynb)。我们这里预测的是噪声(而不是像第一单元的去噪图片),以此来匹配 DDPMScheduler 预计的目标。这里我们用 DDPMScheduler 来在训练中加噪声,并在推理时采样用。训练也需要一段时间 —— 如何加速训练也可以是个有趣的小项目。但你也可以跳过运行代码(甚至整节笔记本),因为我们这里纯粹是在讲解思路。
+
+
+```python
+# Create a scheduler
+noise_scheduler = DDPMScheduler(num_train_timesteps=1000, beta_schedule='squaredcos_cap_v2')
+```
+
+
+```python
+#@markdown Training loop (10 Epochs):
+
+# Redefining the dataloader to set the batch size higher than the demo of 8
+train_dataloader = DataLoader(dataset, batch_size=128, shuffle=True)
+
+# How many runs through the data should we do?
+n_epochs = 10
+
+# Our network
+net = ClassConditionedUnet().to(device)
+
+# Our loss finction
+loss_fn = nn.MSELoss()
+
+# The optimizer
+opt = torch.optim.Adam(net.parameters(), lr=1e-3)
+
+# Keeping a record of the losses for later viewing
+losses = []
+
+# The training loop
+for epoch in range(n_epochs):
+ for x, y in tqdm(train_dataloader):
+
+ # Get some data and prepare the corrupted version
+ x = x.to(device) * 2 - 1 # Data on the GPU (mapped to (-1, 1))
+ y = y.to(device)
+ noise = torch.randn_like(x)
+ timesteps = torch.randint(0, 999, (x.shape[0],)).long().to(device)
+ noisy_x = noise_scheduler.add_noise(x, noise, timesteps)
+
+ # Get the model prediction
+ pred = net(noisy_x, timesteps, y) # Note that we pass in the labels y
+
+ # Calculate the loss
+ loss = loss_fn(pred, noise) # How close is the output to the noise
+
+ # Backprop and update the params:
+ opt.zero_grad()
+ loss.backward()
+ opt.step()
+
+ # Store the loss for later
+ losses.append(loss.item())
+
+ # Print our the average of the last 100 loss values to get an idea of progress:
+ avg_loss = sum(losses[-100:])/100
+ print(f'Finished epoch {epoch}. Average of the last 100 loss values: {avg_loss:05f}')
+
+# View the loss curve
+plt.plot(losses)
+```
+
+
+ 0%| | 0/469 [00:00, ?it/s]
+
+
+ Finished epoch 0. Average of the last 100 loss values: 0.052451
+
+
+
+ 0%| | 0/469 [00:00, ?it/s]
+
+
+ Finished epoch 1. Average of the last 100 loss values: 0.045999
+
+
+
+ 0%| | 0/469 [00:00, ?it/s]
+
+
+ Finished epoch 2. Average of the last 100 loss values: 0.043344
+
+
+
+ 0%| | 0/469 [00:00, ?it/s]
+
+
+ Finished epoch 3. Average of the last 100 loss values: 0.042347
+
+
+
+ 0%| | 0/469 [00:00, ?it/s]
+
+
+ Finished epoch 4. Average of the last 100 loss values: 0.041174
+
+
+
+ 0%| | 0/469 [00:00, ?it/s]
+
+
+ Finished epoch 5. Average of the last 100 loss values: 0.040736
+
+
+
+ 0%| | 0/469 [00:00, ?it/s]
+
+
+ Finished epoch 6. Average of the last 100 loss values: 0.040386
+
+
+
+ 0%| | 0/469 [00:00, ?it/s]
+
+
+ Finished epoch 7. Average of the last 100 loss values: 0.039372
+
+
+
+ 0%| | 0/469 [00:00, ?it/s]
+
+
+ Finished epoch 8. Average of the last 100 loss values: 0.039056
+
+
+
+ 0%| | 0/469 [00:00, ?it/s]
+
+
+ Finished epoch 9. Average of the last 100 loss values: 0.039024
+
+
+
+
+
+ []
+
+
+
+
+
+
+
+
+
+一旦训练结束,我们就可以通过输入不同的标签作为条件,来采样图片了:
+
+
+```python
+#@markdown Sampling some different digits:
+
+# Prepare random x to start from, plus some desired labels y
+x = torch.randn(80, 1, 28, 28).to(device)
+y = torch.tensor([[i]*8 for i in range(10)]).flatten().to(device)
+
+# Sampling loop
+for i, t in tqdm(enumerate(noise_scheduler.timesteps)):
+
+ # Get model pred
+ with torch.no_grad():
+ residual = net(x, t, y) # Again, note that we pass in our labels y
+
+ # Update sample with step
+ x = noise_scheduler.step(residual, t, x).prev_sample
+
+# Show the results
+fig, ax = plt.subplots(1, 1, figsize=(12, 12))
+ax.imshow(torchvision.utils.make_grid(x.detach().cpu().clip(-1, 1), nrow=8)[0], cmap='Greys')
+```
+
+
+ 0it [00:00, ?it/s]
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+就是这么简单!我们现在已经对要生成的图片有所控制了。
+
+希望你喜欢这个例子。一如既往地,如果你有问题,你随时可以在 Discord 上提出来。
+
+
+```python
+# 练习(选做):用同样方法在 FashionMNIST 数据集上试试。调节学习率、batch size 和训练的轮数(epochs)。
+# 你能用比例子更少的训练时间得到些看起来不错的时尚相关的图片吗?
+```
diff --git a/markdown/unit2/02_class_conditioned_diffusion_model_example_CN_files/02_class_conditioned_diffusion_model_example_CN_10_21.png b/markdown/unit2/02_class_conditioned_diffusion_model_example_CN_files/02_class_conditioned_diffusion_model_example_CN_10_21.png
new file mode 100644
index 0000000000000000000000000000000000000000..d167cf29096f0b3fa2423e1aeac13c260d5f0aeb
--- /dev/null
+++ b/markdown/unit2/02_class_conditioned_diffusion_model_example_CN_files/02_class_conditioned_diffusion_model_example_CN_10_21.png
@@ -0,0 +1,3 @@
+version https://git-lfs.github.com/spec/v1
+oid sha256:c3cb5b35ca625aa0fffd64c91c1497ef4f67224b30c095c56768e531ec2ddb23
+size 6938
diff --git a/markdown/unit2/02_class_conditioned_diffusion_model_example_CN_files/02_class_conditioned_diffusion_model_example_CN_12_2.png b/markdown/unit2/02_class_conditioned_diffusion_model_example_CN_files/02_class_conditioned_diffusion_model_example_CN_12_2.png
new file mode 100644
index 0000000000000000000000000000000000000000..280c6044779f0cec43d33087bcc09bb00d63e917
--- /dev/null
+++ b/markdown/unit2/02_class_conditioned_diffusion_model_example_CN_files/02_class_conditioned_diffusion_model_example_CN_12_2.png
@@ -0,0 +1,3 @@
+version https://git-lfs.github.com/spec/v1
+oid sha256:ae4dc20c94cffe508b1324e38193a49c7d4da74dc93a2766fb64365c3a91258e
+size 184554
diff --git a/markdown/unit2/02_class_conditioned_diffusion_model_example_CN_files/02_class_conditioned_diffusion_model_example_CN_4_9.png b/markdown/unit2/02_class_conditioned_diffusion_model_example_CN_files/02_class_conditioned_diffusion_model_example_CN_4_9.png
new file mode 100644
index 0000000000000000000000000000000000000000..8f0a9bb74bbacc0e0b9bdd0377e26614e8900f00
--- /dev/null
+++ b/markdown/unit2/02_class_conditioned_diffusion_model_example_CN_files/02_class_conditioned_diffusion_model_example_CN_4_9.png
@@ -0,0 +1,3 @@
+version https://git-lfs.github.com/spec/v1
+oid sha256:79ab7b6fb12e93772e484ba67377701b0c3f08b88e9fdb122403d508308b9282
+size 10316
diff --git a/markdown/unit2/README_CN.md b/markdown/unit2/README_CN.md
new file mode 100755
index 0000000000000000000000000000000000000000..7b5f0491b48099b7d48b36f9c42927bc9a173d06
--- /dev/null
+++ b/markdown/unit2/README_CN.md
@@ -0,0 +1,72 @@
+# 单元2: 微调,引导,条件生成
+
+欢迎来到 Hugging Face 扩散模型课程的第二单元!在这一单元,你将会学到新的方法去使用和适配预训练过的扩散模型。你也会看到我们如何创建带有额外输入作为**生成条件**的扩散模型,以此控制生成过程。
+
+## 开始这一单元 :rocket:
+
+这里分几步学习这一单元:
+
+- 请首先确保你已经[注册了本课程](https://huggingface.us17.list-manage.com/subscribe?u=7f57e683fa28b51bfc493d048&id=ef963b4162),以便有新的学习资料时你会被通知到。
+- 请先通读本文,对本单元的重点有一个整体的认识。
+- 学习 **Fine-tuning and Guidance** 这节的笔记本,试着使用 🤗 Diffusers 库,在一个新数据集上微调(finetune)一个已有的扩散模型,以及用引导(guidance)这一方法修改采样过程。
+- 照着记事本中的示例,把你的自定义模型做成 Gradio 的 Demo 分享出去。
+- (可选)学习 **Class-conditioned Diffusion Model Example** 这节笔记本,看看我们如何给生成过程加入额外控制。
+
+
+:loudspeaker: 别忘了加入 [Discord](https://huggingface.co/join/discord),在这里你可以参与学习资料的讨论,在`#diffusion-models-class`频道分享你的实验成果。
+
+## 微调(Fine-Tuning)
+
+正如你在第一单元看见的,从头训练一个扩散模型耗费的时间相当长!尤其是当你使用高分辨率图片时,从头训练模型所需的时间和数据量可能多得不切实际。幸运的是,我们还有个解决方法:从一个已经被训练过的模型去开始训练!这样,我们从一个已经学过如何去噪的模型开始,希望能相比于随机初始化的模型能有一个更好的起始点。
+
+
+
+一般而言,当你的新数据和原有模型的原始训练数据多多少少有点相似的时候,微调效果会最好(比如你想生成卡通人脸,那你用于微调的模型最好是个在人脸数据上训练过的模型)。但让人吃惊的是,这些益处在图片分布变化显著时也会存在。上面的图片是通过微调一个[在 LSUN 卧室图片数据集上训练的模型](https://huggingface.co/google/ddpm-bedroom-256)而生成的,这个模型在 [WikiArt 数据集](https://huggingface.co/datasets/huggan/wikiart)被微调了500步。相关的[训练脚本](https://github.com/huggingface/diffusion-models-class/blob/main/unit2/finetune_model.py)也放在了本单元中供大家参考。
+
+## 引导(Guidance)
+
+无条件模型一般没有对生成能内容的掌控。我们可以训练一个条件模型(更过内容将会在下节讲述),接收额外输入,以此来操控生成过程。但我们如何使用一个已有的无条件模型去做这件事呢?我们可以用引导这一方法:生成过程中每一步的模型预测都将会被一些引导函数所评估,并加以修改,以此让最终的生成结果符合我们所想。
+
+
+
+这个引导函数可以是任何函数,这让我们有了很大的设计空间。在笔记本中,我们从一个简单的例子(控制颜色,如上图所示)开始,到使用一个叫CLIP的预训练模型,让生成的结果基于文字描述。
+
+## 条件生成(conditioning)
+
+引导能让我们从一个无条件扩散模型中多少得到些额外的收益,但如果我们在训练过程中就有一些额外的信息(比如图像类别或文字描述)可以输入到模型里,我们可以把这些信息输入模型,让模型使用这些信息去做预测。由此我们就创建了一个条件模型,我们可以在推理阶段通过输入什么信息作为条件来控制模型生成什么。相关的笔记本中就展示了一个例子:一个类别条件的模型,可以根据类别标签生成对应的图像。
+
+
+
+有很多种方法可以把条件信息输入到模型种,比如:
+
+* 把条件信息作为额外的通道输入给 UNet。这种情况下一般条件信息都和图片有着相同的形状,比如条件信息是图像分割的掩模(mask)、深度图或模糊版的图像(针对图像修复、超分辨率任务的模型)。这种方法在一些其它条件下也可以用,比如在相应的笔记本的例子中,类别标签就被映射成了一个嵌入(embedding),并被展开成和输入图片一样的宽度和高度,以此来作为额外的通道输入到模型里。
+* 把条件信息做成一个嵌入(embedding),然后把它映射到和模型其中一个或多个中间层输出的通道数一样,再把这个嵌入加到中间层输出上。这一般是以时间步(timestep)为条件时的做法。比如,你可以把时间步的嵌入映射到特定通道数,然后加到模型的每一个残差网络模块的输出上。这种方法在你有一个向量形式的条件时很有用,比如 CLIP 的图像嵌入。一个值得注意的例子是一个[能修改输入图片的Stable Diffusion模型](https://huggingface.co/spaces/lambdalabs/stable-diffusion-image-variations)。
+* 添加有交叉注意力机制的网络层(cross-attention)。这在当条件是某种形式的文字时最有效 —— 比如文字被一个 transformer 模型映射成了一串 embedding,那么UNet中有交叉注意力机制的网络层就会被用来把这些信息合并到去噪路径中。我们将在第三单元研究 Stable Diffusion 如何处理文字信息条件时看到这种情况。
+
+
+## 用来上手的笔记本示例
+
+| Chapter | Colab | Kaggle | Gradient | Studio Lab |
+|:--------------------------------------------|:----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------|:---------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------|:---------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------|:-------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------|
+| Fine-tuning and Guidance | [](https://colab.research.google.com/github/huggingface/diffusion-models-class/blob/main/unit2/01_finetuning_and_guidance.ipynb) | [](https://kaggle.com/kernels/welcome?src=https://github.com/huggingface/diffusion-models-class/blob/main/unit2/01_finetuning_and_guidance.ipynb) | [](https://console.paperspace.com/github/huggingface/diffusion-models-class/blob/main/unit2/01_finetuning_and_guidance.ipynb) | [](https://studiolab.sagemaker.aws/import/github/huggingface/diffusion-models-class/blob/main/unit2/01_finetuning_and_guidance.ipynb) |
+| Class-conditioned Diffusion Model Example | [](https://colab.research.google.com/github/huggingface/diffusion-models-class/blob/main/unit2/02_class_conditioned_diffusion_model_example.ipynb) | [](https://kaggle.com/kernels/welcome?src=https://github.com/huggingface/diffusion-models-class/blob/main/unit2/02_class_conditioned_diffusion_model_example.ipynb) | [](https://console.paperspace.com/github/huggingface/diffusion-models-class/blob/main/unit2/02_class_conditioned_diffusion_model_example.ipynb) | [](https://studiolab.sagemaker.aws/import/github/huggingface/diffusion-models-class/blob/main/unit2/02_class_conditioned_diffusion_model_example.ipynb) |
+
+现在你已经准备好学习这些笔记本了!通过上面的链接使用你选择的平台打开它们!微调是个计算量很大的工作,所以如果你用的是 Kaggle或 Google Colab,请确保你把运行时类型设成 GPU。
+
+本单元内容的主体在 **Fine-tuning and Guidance** 这个笔记本中,我们将通过示例探索这两个话题。笔记本将会展示给你如何在新数据上微调现有模型,添加引导,以及在 Gradio 上分享结果。这里还有一个脚本程序 [finetune_model.py](https://github.com/huggingface/diffusion-models-class/blob/main/unit2/finetune_model.py),让你更容易地实验不同的微调设置;以及一个[示例的 space](https://huggingface.co/spaces/johnowhitaker/color-guided-wikiart-diffusion),你可以以此作为目标用来在 🤗 Spaces 上分享 demo。
+
+在 **Class-conditioned Diffusion Model Example** 中,我们用 MNIST 数据集展示一个很简单的例子:创建一个以类别标签为条件的扩散模型。这里的重点在于尽可能简单地讲解核心要点:通过给模型提供额外的关于去除什么噪声的信息,我们可以在推理时控制哪种类型的图片是我们想要生成的。
+
+## 项目时间
+
+仿照 **Fine-tuning and Guidance** 笔记本中的例子,微调你自己的模型或挑选一个现有模型,创建 Gradio 的 demo 展示你的引导技巧。也不要忘了在 Discord 或 Twitter 之类的平台上分享,让我们也羡慕羡慕!
+
+## 一些其它学习资源
+
+[Denoising Diffusion Implicit Models](https://arxiv.org/abs/2010.02502) - 引出了DDIM采样方法(DDIMScheduler 用到了这个方法)
+
+[GLIDE: Towards Photorealistic Image Generation and Editing with Text-Guided Diffusion Models](https://arxiv.org/abs/2112.10741) - 介绍了如何让扩散模型基于文本类条件
+
+[eDiffi: Text-to-Image Diffusion Models with an Ensemble of Expert Denoisers](https://arxiv.org/abs/2211.01324) - 介绍了不同种类的生成条件一起使用时的情况,以此更加广泛地控制生成过程
+
+如果你找到了更好的学习资源,也别忘了告诉我们让我们加到这个列表里!
diff --git a/markdown/unit2/conditional_digit_generation.png b/markdown/unit2/conditional_digit_generation.png
new file mode 100644
index 0000000000000000000000000000000000000000..1f18a67f2627c9b4b72142ecc1a1c734495c0f1d
--- /dev/null
+++ b/markdown/unit2/conditional_digit_generation.png
@@ -0,0 +1,3 @@
+version https://git-lfs.github.com/spec/v1
+oid sha256:3a3c1b5f4583c173037028134f889507cc936c2489b6bfd75151ae02bd6e5839
+size 102391
diff --git a/markdown/unit2/finetune_model.py b/markdown/unit2/finetune_model.py
new file mode 100644
index 0000000000000000000000000000000000000000..57dc73c0726ee783b2c9d4686955ce3b419cd44f
--- /dev/null
+++ b/markdown/unit2/finetune_model.py
@@ -0,0 +1,120 @@
+import wandb
+import numpy as np
+import torch, torchvision
+import torch.nn.functional as F
+from PIL import Image
+from tqdm.auto import tqdm
+from fastcore.script import call_parse
+from torchvision import transforms
+from diffusers import DDPMPipeline
+from diffusers import DDIMScheduler
+from datasets import load_dataset
+from matplotlib import pyplot as plt
+
+@call_parse
+def train(
+ image_size = 256,
+ batch_size = 16,
+ grad_accumulation_steps = 2,
+ num_epochs = 1,
+ start_model = "google/ddpm-bedroom-256",
+ dataset_name = "huggan/wikiart",
+ device='cuda',
+ model_save_name='wikiart_1e',
+ wandb_project='dm_finetune',
+ log_samples_every = 250,
+ save_model_every = 2500,
+ ):
+
+ # Initialize wandb for logging
+ wandb.init(project=wandb_project, config=locals())
+
+
+ # Prepare pretrained model
+ image_pipe = DDPMPipeline.from_pretrained(start_model);
+ image_pipe.to(device)
+
+ # Get a scheduler for sampling
+ sampling_scheduler = DDIMScheduler.from_config(start_model)
+ sampling_scheduler.set_timesteps(num_inference_steps=50)
+
+ # Prepare dataset
+ dataset = load_dataset(dataset_name, split="train")
+ preprocess = transforms.Compose(
+ [
+ transforms.Resize((image_size, image_size)),
+ transforms.RandomHorizontalFlip(),
+ transforms.ToTensor(),
+ transforms.Normalize([0.5], [0.5]),
+ ]
+ )
+ def transform(examples):
+ images = [preprocess(image.convert("RGB")) for image in examples["image"]]
+ return {"images": images}
+ dataset.set_transform(transform)
+ train_dataloader = torch.utils.data.DataLoader(dataset, batch_size=batch_size, shuffle=True)
+
+
+ # Optimizer & lr scheduler
+ optimizer = torch.optim.AdamW(image_pipe.unet.parameters(), lr=1e-5)
+ scheduler = torch.optim.lr_scheduler.ExponentialLR(optimizer, gamma=0.9)
+
+ for epoch in range(num_epochs):
+ for step, batch in tqdm(enumerate(train_dataloader), total=len(train_dataloader)):
+
+ # Get the clean images
+ clean_images = batch['images'].to(device)
+
+ # Sample noise to add to the images
+ noise = torch.randn(clean_images.shape).to(clean_images.device)
+ bs = clean_images.shape[0]
+
+ # Sample a random timestep for each image
+ timesteps = torch.randint(0, image_pipe.scheduler.num_train_timesteps, (bs,), device=clean_images.device).long()
+
+ # Add noise to the clean images according to the noise magnitude at each timestep
+ # (this is the forward diffusion process)
+ noisy_images = image_pipe.scheduler.add_noise(clean_images, noise, timesteps)
+
+ # Get the model prediction for the noise
+ noise_pred = image_pipe.unet(noisy_images, timesteps, return_dict=False)[0]
+
+ # Compare the prediction with the actual noise:
+ loss = F.mse_loss(noise_pred, noise)
+
+ # Log the loss
+ wandb.log({'loss':loss.item()})
+
+ # Calculate the gradients
+ loss.backward()
+
+ # Gradient Acccumulation: Only update every grad_accumulation_steps
+ if (step+1)%grad_accumulation_steps == 0:
+ optimizer.step()
+ optimizer.zero_grad()
+
+ # Occasionally log samples
+ if (step+1)%log_samples_every == 0:
+ x = torch.randn(8, 3, 256, 256).to(device) # Batch of 8
+ for i, t in tqdm(enumerate(sampling_scheduler.timesteps)):
+ model_input = sampling_scheduler.scale_model_input(x, t)
+ with torch.no_grad():
+ noise_pred = image_pipe.unet(model_input, t)["sample"]
+ x = sampling_scheduler.step(noise_pred, t, x).prev_sample
+ grid = torchvision.utils.make_grid(x, nrow=4)
+ im = grid.permute(1, 2, 0).cpu().clip(-1, 1)*0.5 + 0.5
+ im = Image.fromarray(np.array(im*255).astype(np.uint8))
+ wandb.log({'Sample generations': wandb.Image(im)})
+
+ # Occasionally save model
+ if (step+1)%save_model_every == 0:
+ image_pipe.save_pretrained(model_save_name+f'step_{step+1}')
+
+ # Update the learning rate for the next epoch
+ scheduler.step()
+
+ # Save the pipeline one last time
+ image_pipe.save_pretrained(model_save_name)
+
+ # Wrap up the run
+ wandb.finish()
diff --git a/markdown/unit2/guidance_eg.png b/markdown/unit2/guidance_eg.png
new file mode 100644
index 0000000000000000000000000000000000000000..da81f4da14cfb3e7b869aa983ac76d99643b05cf
--- /dev/null
+++ b/markdown/unit2/guidance_eg.png
@@ -0,0 +1,3 @@
+version https://git-lfs.github.com/spec/v1
+oid sha256:71e0eae7cbdd4d019c1848bac3578185b1f4e9c5ecfbbd37d784bc32e5143411
+size 382274
diff --git a/markdown/unit3/01_stable_diffusion_introduction_CN.md b/markdown/unit3/01_stable_diffusion_introduction_CN.md
new file mode 100644
index 0000000000000000000000000000000000000000..a00a8630ac3e97460f375121f3c3f37639dbcbea
--- /dev/null
+++ b/markdown/unit3/01_stable_diffusion_introduction_CN.md
@@ -0,0 +1,583 @@
+# 简介
+
+本节笔记本将会涵盖一些基础性的内容,介绍如何利用现有的管线(pipeline)借助 Stable Diffusion 模型来创造和修改图片。我们也将简要一览管线内的关键组成部分,把更进一步的探究任务留给更深入介绍的一节笔记本中。特别地,我们将涵盖以下内容:
+- 利用 `StableDiffusionPipeline` 根据文字描述生成图片,并通过修改各个输入参数来进行探究实验
+- 实操了解管线的一些关键组成部分:
+ - 让这个模型成为“隐编码扩散模型(latent diffusion model)”的可变分自编码器(VAE)
+ - 处理文本提示的分词器(tokenizer)和文本编码器
+ - UNet 模型本身
+ - 使用的调度器(scheduler),以及其它不同的调度器
+- 使用管线的组成部分来复现采样循环
+- 用Img2Im管线来编辑现有图片
+- 使用inpainting管线和Depth2Img管线
+
+❓如果你有问题,请在 Hugging Face Discord 的 `#diffusion-models-class` 频道提出。如果你还没有 Hugging Face 的账号,你可以在这里注册:https://huggingface.co/join/discord
+
+
+# 配置
+
+
+```python
+!pip install -Uq diffusers ftfy accelerate
+```
+
+
+```python
+# Installing transformers from source for now since we need the latest version for Depth2Img:
+!pip install -Uq git+https://github.com/huggingface/transformers
+```
+
+
+```python
+import torch
+import requests
+from PIL import Image
+from io import BytesIO
+from matplotlib import pyplot as plt
+
+# We'll be exploring a number of pipelines today!
+from diffusers import (
+ StableDiffusionPipeline,
+ StableDiffusionImg2ImgPipeline,
+ StableDiffusionInpaintPipeline,
+ StableDiffusionDepth2ImgPipeline
+ )
+
+# We'll use a couple of demo images later in the notebook
+def download_image(url):
+ response = requests.get(url)
+ return Image.open(BytesIO(response.content)).convert("RGB")
+
+# Download images for inpainting example
+img_url = "https://raw.githubusercontent.com/CompVis/latent-diffusion/main/data/inpainting_examples/overture-creations-5sI6fQgYIuo.png"
+mask_url = "https://raw.githubusercontent.com/CompVis/latent-diffusion/main/data/inpainting_examples/overture-creations-5sI6fQgYIuo_mask.png"
+
+init_image = download_image(img_url).resize((512, 512))
+mask_image = download_image(mask_url).resize((512, 512))
+```
+
+
+```python
+# Set device
+device = (
+ "mps"
+ if torch.backends.mps.is_available()
+ else "cuda"
+ if torch.cuda.is_available()
+ else "cpu"
+)
+```
+
+# 从文本生成图像
+
+我们先载入 Stable Diffusion 的管线,看看我们能做点什么。现有的 Stable Diffusion 模型有好多不同版本,截至本文书写时的最新版本是第2.1版。如果你想探究更旧的版本,只需要在 `model_id` 处修改即可(比如你可以试试将其改成 `CompVis/stable-diffusion-v1-4` 或从[dreambooth concepts library](https://huggingface.co/sd-dreambooth-library)选一个模型)。
+
+
+```python
+# Load the pipeline
+model_id = "stabilityai/stable-diffusion-2-1-base"
+pipe = StableDiffusionPipeline.from_pretrained(model_id).to(device)
+```
+
+如果你的GPU内存不够用,这里有些办法也许可以减少内存使用:
+- 载入 FP16 精度的版本(但并不是所有的系统上都支持)。与此同时,在你对管线的某个特定部分实验时,你也需要把所有的张量换成 torch.float16 精度:
+
+ `pipe = StableDiffusionPipeline.from_pretrained(model_id, revision="fp16", torch_dtype=torch.float16).to(device)`
+
+
+- 开启注意力机制切分(attention slicing)。这会牺牲一点点速度来减少GPU内存的使用:
+
+ `pipe.enable_attention_slicing()`
+
+
+- 降低要生成的图片的尺寸
+
+当管线加载好了以后,我们可以用以下代码去使用文字提示生成图片:
+
+
+```python
+# Set up a generator for reproducibility
+generator = torch.Generator(device=device).manual_seed(42)
+
+# Run the pipeline, showing some of the available arguments
+pipe_output = pipe(
+ prompt="Palette knife painting of an autumn cityscape", # What to generate
+ negative_prompt="Oversaturated, blurry, low quality", # What NOT to generate
+ height=480, width=640, # Specify the image size
+ guidance_scale=8, # How strongly to follow the prompt
+ num_inference_steps=35, # How many steps to take
+ generator=generator # Fixed random seed
+)
+
+# View the resulting image:
+pipe_output.images[0]
+```
+
+
+ 0%| | 0/35 [00:00, ?it/s]
+
+
+
+
+
+
+
+
+
+
+
+**练习:** 花点时间去用上面的代码块实验,使用你自己的文字提示,反复调整各项设置看看这些设置如何影响生成效果。使用不同的随机种子或者移除掉 `generator` 这个输入参数,看看能获取什么不同的效果。
+
+主要的要调节参数介绍:
+- `width` 和 `height` 指定了生成图片的尺寸。它们必须是可被 8 整除的数字,只有这样我们的可变分自编码器(VAE)才能正常工作(我们在将来的章节会了解到)。
+- 步数 `num_inference_steps` 也会影响生成的质量。默认设成 50 已经很好了,但有些时候你也可以用少到像 20 步这样,这对做实验就方便多了。
+- 使用 `negative_prompt` 来强调不希望生成的内容,一般会在无分类器引导(classifier-free guidance)的过程中用到,这可以是个非常有用的添加额外控制的方式。你可以留空这个地方不管,但很多用户觉得列出一些不想要的特性对更好的生成很有帮助。
+- `guidance_scale` 这个参数决定了无分类器引导(CFG)的影响强度有多大。增大这个值会使得生成的内容更接近文字提示;但这个值如果过大,可能会使得结果变得过饱和、不好看。
+
+如果你想为文字提示找点灵感,你也可以从这里开始:[Stable Diffusion Prompt Book](https://stability.ai/sdv2-prompt-book)
+
+你可在下面看到增大`guidance_scale` 这个参数所带来的作用:
+
+
+```python
+#@markdown comparing guidance scales:
+cfg_scales = [1.1, 8, 12] #@param
+prompt = "A collie with a pink hat" #@param
+fig, axs = plt.subplots(1, len(cfg_scales), figsize=(16, 5))
+for i, ax in enumerate(axs):
+ im = pipe(prompt, height=480, width=480,
+ guidance_scale=cfg_scales[i], num_inference_steps=35,
+ generator=torch.Generator(device=device).manual_seed(42)).images[0]
+ ax.imshow(im); ax.set_title(f'CFG Scale {cfg_scales[i]}');
+```
+
+
+ 0%| | 0/35 [00:00, ?it/s]
+
+
+
+ 0%| | 0/35 [00:00, ?it/s]
+
+
+
+ 0%| | 0/35 [00:00, ?it/s]
+
+
+
+
+
+
+
+
+调一调上面的值,尝试不同的幅度和提示。当然了,如何解读这些参数是个很主观的事情,但对我来说,我觉得 8 到 12 这个值区间比其它情况产出的结果都好。
+
+# 管线的组成部分
+
+这里我们用的 `StableDiffusionPipeline` 比前面几个单元的 `DDPMPipeline` 要复杂一点。除了 UNet 和调度器之外,管线内还有很多其它的组成部分:
+
+
+```python
+print(list(pipe.components.keys())) # List components
+```
+
+ ['vae', 'text_encoder', 'tokenizer', 'unet', 'scheduler', 'safety_checker', 'feature_extractor']
+
+
+为了更好地理解管线如何工作,我们简要地一个一个看看各个组成部分,然后我们自己动手,把它们组合在一起,以此复现出整个管线功能。
+
+### 可变分自编码器(VAE)
+
+
+
+可变分自编码器(VAE)是一种模型,它可以将输入编码成一种被压缩过的表示形式,再把这个“隐式的”表示形式解码成某种接近输入的输出。当我们使用 Stable Diffusion 生成图片时,我们先在VAE的“隐空间”应用扩散过程**生成隐编码**,然后**在结尾对它们解码**来查看结果图片。
+
+这里就是一个例子,使用VAE把输入图片编码成隐式的表示形式,再对它解码:
+
+
+```python
+# Create some fake data (a random image, range (-1, 1))
+images = torch.rand(1, 3, 512, 512).to(device) * 2 - 1
+print("Input images shape:", images.shape)
+
+# Encode to latent space
+with torch.no_grad():
+ latents = 0.18215 * pipe.vae.encode(images).latent_dist.mean
+print("Encoded latents shape:", latents.shape)
+
+# Decode again
+with torch.no_grad():
+ decoded_images = pipe.vae.decode(latents / 0.18215).sample
+print("Decoded images shape:", decoded_images.shape)
+```
+
+ Input images shape: torch.Size([1, 3, 512, 512])
+ Encoded latents shape: torch.Size([1, 4, 64, 64])
+ Decoded images shape: torch.Size([1, 3, 512, 512])
+
+
+如你所见,原本 512x512 尺寸的图片被压缩到 64x64的隐式表示形式(有四个通道)中。每一空间维度上都被压缩到了原有的八分之一,这也是为什么我们设定 `width` 和 `height` 时需要它们是 8 的倍数。
+
+使用这些信息量充裕的 4x64x64 隐编码可比使用 512px 大小的图片要高效多了,可以让我们的扩散模型更快,并使用更少的资源来训练和使用。VAE的解码过程并不是完美的,但即使损失了一点点质量,总的来说也足够好了。
+
+注意:上面的代码例子包含了一个值为 0.18215 的缩放因子,以此来适配stable diffusion训练时的处理流程。
+
+### 分词器(Tokenizer)和文本编码器(Text Encoder)
+
+
+
+
+
+文本编码器的作用是将输入的字符串(文本提示)转化成数值表示形式,这样才能输入进 UNet 作为条件。文本首先要被管线中的分词器(tokenizer)转换成一系列的分词(token)。文本编码器有大约五万分词的词汇量 —— 任何不存在于这些词汇量中的词语都会被细分成多个更小的词语。这些分词然后就被送入文本编码器模型中 —— 文本编码器是一个transformer模型,最初被训练作为CLIP的文本编码器。这里我们希望这个经过了预训练的transformer模型学习到了足够好的文本表示能力,可以对我们这里的扩散任务一样有用。
+
+我们这里通过对一个文字提示进行编码,验证一下这个过程。首先,我们手动进行分词,并将它输入到文本编码器中,再使用管线的 `_encode_prompt` 方法,观察一下完成的过程,这包括补全或截断分词串的长度,使得分词串的长度等于最大长度 77 :
+
+
+```python
+# Tokenizing and encoding an example prompt manualy:
+
+# Tokenize
+input_ids = pipe.tokenizer(["A painting of a flooble"])['input_ids']
+print("Input ID -> decoded token")
+for input_id in input_ids[0]:
+ print(f"{input_id} -> {pipe.tokenizer.decode(input_id)}")
+
+# Feed through CLIP text encoder
+input_ids = torch.tensor(input_ids).to(device)
+with torch.no_grad():
+ text_embeddings = pipe.text_encoder(input_ids)['last_hidden_state']
+print("Text embeddings shape:", text_embeddings.shape)
+```
+
+ Input ID -> decoded token
+ 49406 -> <|startoftext|>
+ 320 -> a
+ 3086 -> painting
+ 539 -> of
+ 320 -> a
+ 4062 -> floo
+ 1059 -> ble
+ 49407 -> <|endoftext|>
+ Text embeddings shape: torch.Size([1, 8, 1024])
+
+
+
+```python
+# Get the final text embeddings using the pipeline's _encode_prompt function:
+text_embeddings = pipe._encode_prompt("A painting of a flooble", device, 1, False, '')
+text_embeddings.shape
+```
+
+
+
+
+ torch.Size([1, 77, 1024])
+
+
+
+这些文本嵌入(text embedding),也即文本编码器中最后一个transformer模块的“隐状态(hidden state)”,将会被送入 UNet 中作为 `forward` 函数的一个额外输入,下面部分我们会详细看到。
+
+### UNet
+
+
+
+UNet 模型接收一个带噪的输入,并预测噪声,和我们之前单元中看到的 UNet 一样。但与以往例子不同的是,这里的输入并不是图片了,而是图片的隐式表示形式(latent representation)。此外,除了把用于暗示带噪程度的timestep输入进 UNet 作为条件外,这里模型也把文字提示(prompt)的文本嵌入(text embeddings)作为了额外输入。这里我们假数据试着让它预测一下:
+
+
+```python
+# Dummy inputs:
+timestep = pipe.scheduler.timesteps[0]
+latents = torch.randn(1, 4, 64, 64).to(device)
+text_embeddings = torch.randn(1, 77, 1024).to(device)
+
+# Model prediction:
+with torch.no_grad():
+ unet_output = pipe.unet(latents, timestep, text_embeddings).sample
+print('UNet output shape:', unet_output.shape) # Same shape as the input latents
+```
+
+ UNet output shape: torch.Size([1, 4, 64, 64])
+
+
+### 调度器(Scheduler)
+
+调度器保存了如何加噪的计划安排,管理着如何基于模型的预测更新带噪样本。默认的调度器是 `PNDMScheduler` 调度器,但你也可以用其它的(比如 `LMSDiscreteScheduler` 调度器),只要它们用相同的配置初始化。
+
+我们可以画出图像来观察随着timestep添加噪声的计划安排,看看不同时间的噪声水平(基于$\bar{\alpha}$这个参数)是什么样的:
+
+
+```python
+plt.plot(pipe.scheduler.alphas_cumprod, label=r'$\bar{\alpha}$')
+plt.xlabel('Timestep (high noise to low noise ->)');
+plt.title('Noise schedule');plt.legend();
+```
+
+
+
+
+
+
+
+如果你想尝试不同的调度器,你可以像下面代码中一样换一个新的:
+
+
+```python
+from diffusers import LMSDiscreteScheduler
+
+# Replace the scheduler
+pipe.scheduler = LMSDiscreteScheduler.from_config(pipe.scheduler.config)
+
+# Print the config
+print('Scheduler config:', pipe.scheduler)
+
+# Generate an image with this new scheduler
+pipe(prompt="Palette knife painting of an winter cityscape", height=480, width=480,
+ generator=torch.Generator(device=device).manual_seed(42)).images[0]
+```
+
+ Scheduler config: LMSDiscreteScheduler {
+ "_class_name": "LMSDiscreteScheduler",
+ "_diffusers_version": "0.11.1",
+ "beta_end": 0.012,
+ "beta_schedule": "scaled_linear",
+ "beta_start": 0.00085,
+ "clip_sample": false,
+ "num_train_timesteps": 1000,
+ "prediction_type": "epsilon",
+ "set_alpha_to_one": false,
+ "skip_prk_steps": true,
+ "steps_offset": 1,
+ "trained_betas": null
+ }
+
+
+
+
+ 0%| | 0/50 [00:00, ?it/s]
+
+
+
+
+
+
+
+
+
+
+
+在[这里](https://huggingface.co/docs/diffusers/api/pipelines/stable_diffusion_2#how-to-load-and-use-different-schedulers)你可以了解更多使用不同调度器的信息。
+
+### DIY一个采样循环
+
+现在我们已经一个个看过这些组成部分了,我们可以把它们拼装到一起,来复现一下整个管线的功能:
+
+
+```python
+guidance_scale = 8 #@param
+num_inference_steps=30 #@param
+prompt = "Beautiful picture of a wave breaking" #@param
+negative_prompt = "zoomed in, blurry, oversaturated, warped" #@param
+
+# Encode the prompt
+text_embeddings = pipe._encode_prompt(prompt, device, 1, True, negative_prompt)
+
+# Create our random starting point
+latents = torch.randn((1, 4, 64, 64), device=device, generator=generator)
+latents *= pipe.scheduler.init_noise_sigma
+
+# Prepare the scheduler
+pipe.scheduler.set_timesteps(num_inference_steps, device=device)
+
+# Loop through the sampling timesteps
+for i, t in enumerate(pipe.scheduler.timesteps):
+
+ # expand the latents if we are doing classifier free guidance
+ latent_model_input = torch.cat([latents] * 2)
+
+ # Apply any scaling required by the scheduler
+ latent_model_input = pipe.scheduler.scale_model_input(latent_model_input, t)
+
+ # predict the noise residual with the unet
+ with torch.no_grad():
+ noise_pred = pipe.unet(latent_model_input, t, encoder_hidden_states=text_embeddings).sample
+
+ # perform guidance
+ noise_pred_uncond, noise_pred_text = noise_pred.chunk(2)
+ noise_pred = noise_pred_uncond + guidance_scale * (noise_pred_text - noise_pred_uncond)
+
+ # compute the previous noisy sample x_t -> x_t-1
+ latents = pipe.scheduler.step(noise_pred, t, latents).prev_sample
+
+# Decode the resulting latents into an image
+with torch.no_grad():
+ image = pipe.decode_latents(latents.detach())
+
+# View
+pipe.numpy_to_pil(image)[0]
+```
+
+
+
+
+
+
+
+
+
+
+大多数情况下,还是使用现有的管线更方便,但我们这里手动拼装采样循环会更有益于我们理解每个部分如何工作、以及如何根据需要修改这些组件。如果你想看看实际的代码以及深入了解如何修改这些组成部分,你可以参考 Stable Diffusion Deep Dive 这个[笔记本](https://github.com/fastai/diffusion-nbs/blob/master/Stable%20Diffusion%20Deep%20Dive.ipynb)和[视频](https://m.youtube.com/watch?v=0_BBRNYInx8)。这里有对相关内容更全面彻底的探究。
+
+# 其它的一些管线
+
+那我们除了从文字提示生成图片外还能做点什么呢?其实还有很多!这一部分还会向你展示几个很酷的管线,让你了解一些其它的可以应用 Stable Diffusion 的任务。这里面有几个管线需要你下载新的模型,所以你要是着急的话你也可以跳过这一部分,只看看现有的输出展示就行,不必亲自下载和运行模型。
+
+## Img2Img
+
+直到现在,我们生成的图片还都是完全从随机的隐变量来开始生成的,而且也都使用了完整的扩散模型采样循环。但其实我们不必从头开始。Img2Img 这个管线首先将一张已有的图片进行编码,编码成一系列的隐变量,然后在这些隐变量上随机加噪声,以这些作为起始点。噪声加多大量、去噪需要的步数决定了这个 img2img 过程的“强度”。只加一点点噪声(强度低)只会带来微小改变,而加入最大量的噪声并跑完完整的去噪过程又会生成出几乎完全不像原始图片的结果,即使可能在整体结构上还多少有点相似。
+
+这个管线无需什么特殊模型,只要模型的 ID 和我们的文字到图像模型一样就行,没有新的需要下载的文件。
+
+
+```python
+# Loading an Img2Img pipeline
+model_id = "stabilityai/stable-diffusion-2-1-base"
+img2img_pipe = StableDiffusionImg2ImgPipeline.from_pretrained(model_id).to(device)
+```
+
+
+ Fetching 16 files: 0%| | 0/16 [00:00, ?it/s]
+
+
+在本节的“配置”部分,我们加载了一个名为 `init_image` 的图片来用于这里的演示,当然你也可以用你自己的图片替换它。这里是使用该管线的代码:
+
+
+```python
+# Apply Img2Img
+result_image = img2img_pipe(
+ prompt="An oil painting of a man on a bench",
+ image = init_image, # The starting image
+ strength = 0.6, # 0 for no change, 1.0 for max strength
+).images[0]
+
+# View the result
+fig, axs = plt.subplots(1, 2, figsize=(12, 5))
+axs[0].imshow(init_image);axs[0].set_title('Input Image')
+axs[1].imshow(result_image);axs[1].set_title('Result');
+```
+
+
+ 0%| | 0/30 [00:00, ?it/s]
+
+
+
+
+
+
+
+
+**练习:** 用这个管线做实验,试试你自己的图片,或者试试不同的强度和文字提示。你可以使用很多和文字到图片管线相同的输入参数。所以尽可能试试不同的图片尺寸、生成步数吧。
+
+## In-Painting
+
+
+
+如果我们想在一张图中保留一部分不变而在其它部分生成新东西,那该怎么办呢?这种技术叫 inpainting 。虽然我们可以通过前面演示中的同一个模型(用 `StableDiffusionInpaintPipelineLegacy` 管线)来实现,但我们这里可以用一个自定义的微调版 Stable Diffusion 模型来得到更好的效果。这里的 Stable Diffusion 模型接收一个掩模(mask)作为额外条件性输入。这个掩模图片需要和输入图片尺寸一致,白色区域表示要被替换的部分,黑色区域表示要保留的部分。以下代码就展示了我们如何载入这个管线并如何应用到前面载入的示例图片和掩模上:
+
+
+```python
+# Load the inpainting pipeline (requires a suitable inpainting model)
+pipe = StableDiffusionInpaintPipeline.from_pretrained("runwayml/stable-diffusion-inpainting")
+pipe = pipe.to(device)
+```
+
+
+```python
+# Inpaint with a prompt for what we want the result to look like
+prompt = "A small robot, high resolution, sitting on a park bench"
+image = pipe(prompt=prompt, image=init_image, mask_image=mask_image).images[0]
+
+# View the result
+fig, axs = plt.subplots(1, 3, figsize=(16, 5))
+axs[0].imshow(init_image);axs[0].set_title('Input Image')
+axs[1].imshow(mask_image);axs[1].set_title('Mask')
+axs[2].imshow(image);axs[2].set_title('Result');
+```
+
+
+ 0%| | 0/50 [00:00, ?it/s]
+
+
+
+
+
+
+
+
+当和其它可以自动生成掩模的模型结合起来的时候,这个模型将会相当强大。比如[这个示例space](https://huggingface.co/spaces/nielsr/text-based-inpainting)就用了一个名为 CLIPSeg 的模型,它可以根据文字描述自动地用掩模去掉一个物体。
+
+### 题外话:管理你的模型缓存
+
+探索不同的管线和模型可能会占满你的硬盘空间。你可用这个指令看看你都下载了哪些模型到你的硬盘上:
+
+
+```python
+!ls ~/.cache/huggingface/diffusers/ # List the contents of the cache directory
+```
+
+ models--CompVis--stable-diffusion-v1-4
+ models--ddpm-bedroom-256
+ models--google--ddpm-bedroom-256
+ models--google--ddpm-celebahq-256
+ models--runwayml--stable-diffusion-inpainting
+ models--stabilityai--stable-diffusion-2-1-base
+
+
+看看[缓存相关文档](https://huggingface.co/docs/huggingface_hub/main/en/how-to-cache)来了解如何高效地查看和管理缓存。
+
+## Depth2Image
+
+
+
+_Input image, depth image and generated examples (image source: StabilityAI)_
+
+Img2Img 已经很厉害了,但有时我们还想用原始图片的组成成分但使用完全不同的颜色或纹理来生成新图片。通过调节 Img2Img 的“强度”来保留图片整体结构但却不保留原有颜色将会很困难。
+
+所以这里就需要另一个微调的模型了!这个模型需要输入额外的深度信息作为生成条件。相关管线使用了一个深度预测模型来预测出一个深度图,然后这个深度图会被输入微调过的 UNet 中用以生成图片。我们这里希望生成的图片能够保留原始图片的深度信息和总体结构,同时又在相关部分填入全新的内容。
+
+
+```python
+# Load the Depth2Img pipeline (requires a suitable model)
+pipe = StableDiffusionDepth2ImgPipeline.from_pretrained("stabilityai/stable-diffusion-2-depth")
+pipe = pipe.to(device)
+```
+
+
+```python
+# Inpaint with a prompt for what we want the result to look like
+prompt = "An oil painting of a man on a bench"
+image = pipe(prompt=prompt, image=init_image).images[0]
+
+# View the result
+fig, axs = plt.subplots(1, 2, figsize=(16, 5))
+axs[0].imshow(init_image);axs[0].set_title('Input Image')
+axs[1].imshow(image);axs[1].set_title('Result');
+```
+
+
+ 0%| | 0/40 [00:00, ?it/s]
+
+
+
+
+
+
+
+
+让我们和 Img2Img 的例子做个对比 —— 这里生成结果有着更丰富的颜色变化,但图片的整体结构仍然忠于原始图片。这里的例子其实并不够理想,因为为了匹配上狗的形状,这里生成的人有着怪异的身体构造。但在某些场景下,这个技术还是相当有用的,比如这个例子中的“杀手级应用软件”(查看[推特](https://twitter.com/CarsonKatri/status/1600248599254007810?s=20&t=BlzSK26sfqi2336SN0gKpQ)),它利用深度模型去给一个 3D 场景加入纹理!
+
+# 下一步?
+
+希望这节的内容让你对 Stable Diffusion 能做的事情有了一个认识!如果你玩烦了本节笔记本中的例子,你可以尝试 **DreamBooth hackathon** 这节笔记本,看看怎样自己来微调 Stable Diffusion 模型,来用在文字到图像或图像到图像的相关管线上。
+
+如果你想深入探索不同的组成部分是如何运作的,你可以看看 **Stable Diffusion Deep Dive** 这节笔记本,这里介绍了大量的细节以及额外的一些小技巧。
+
+一定要和我们以及社区分享你的创造啊!
diff --git a/markdown/unit3/01_stable_diffusion_introduction_CN_files/01_stable_diffusion_introduction_CN_10_1.png b/markdown/unit3/01_stable_diffusion_introduction_CN_files/01_stable_diffusion_introduction_CN_10_1.png
new file mode 100644
index 0000000000000000000000000000000000000000..5193fa4ec2d86da781fc7b0dcb46bde62c2aac80
--- /dev/null
+++ b/markdown/unit3/01_stable_diffusion_introduction_CN_files/01_stable_diffusion_introduction_CN_10_1.png
@@ -0,0 +1,3 @@
+version https://git-lfs.github.com/spec/v1
+oid sha256:c7220a9867c3c1aa53ab9e55cd18e757d75185efa9863dd1eb9eded925560f70
+size 680840
diff --git a/markdown/unit3/01_stable_diffusion_introduction_CN_files/01_stable_diffusion_introduction_CN_12_3.png b/markdown/unit3/01_stable_diffusion_introduction_CN_files/01_stable_diffusion_introduction_CN_12_3.png
new file mode 100644
index 0000000000000000000000000000000000000000..7e5d6ce12578ce0878d392e67210d7ff38fea902
--- /dev/null
+++ b/markdown/unit3/01_stable_diffusion_introduction_CN_files/01_stable_diffusion_introduction_CN_12_3.png
@@ -0,0 +1,3 @@
+version https://git-lfs.github.com/spec/v1
+oid sha256:754996cf287e2efe124c8c9d70abcc51081e72b94083d0a99c14f5b6ceb743e3
+size 730785
diff --git a/markdown/unit3/01_stable_diffusion_introduction_CN_files/01_stable_diffusion_introduction_CN_35_0.png b/markdown/unit3/01_stable_diffusion_introduction_CN_files/01_stable_diffusion_introduction_CN_35_0.png
new file mode 100644
index 0000000000000000000000000000000000000000..7f2a6e8af313f016b5357263ecf355f9c57ab8bd
--- /dev/null
+++ b/markdown/unit3/01_stable_diffusion_introduction_CN_files/01_stable_diffusion_introduction_CN_35_0.png
@@ -0,0 +1,3 @@
+version https://git-lfs.github.com/spec/v1
+oid sha256:9cf58376af8d73bc7d3f1dcfc411cc39055437d50036d773bbc6da880b72da54
+size 23091
diff --git a/markdown/unit3/01_stable_diffusion_introduction_CN_files/01_stable_diffusion_introduction_CN_37_2.png b/markdown/unit3/01_stable_diffusion_introduction_CN_files/01_stable_diffusion_introduction_CN_37_2.png
new file mode 100644
index 0000000000000000000000000000000000000000..d760e794dceacb0eb877e8578e791625ca159803
--- /dev/null
+++ b/markdown/unit3/01_stable_diffusion_introduction_CN_files/01_stable_diffusion_introduction_CN_37_2.png
@@ -0,0 +1,3 @@
+version https://git-lfs.github.com/spec/v1
+oid sha256:6441efcb3cae49237fdce1aefcb440b87700f09ebabe6ef54c93055842d55505
+size 530821
diff --git a/markdown/unit3/01_stable_diffusion_introduction_CN_files/01_stable_diffusion_introduction_CN_40_0.png b/markdown/unit3/01_stable_diffusion_introduction_CN_files/01_stable_diffusion_introduction_CN_40_0.png
new file mode 100644
index 0000000000000000000000000000000000000000..a8d2c07f2670e0dda8ea65b2bc8b9e91567f3b0a
--- /dev/null
+++ b/markdown/unit3/01_stable_diffusion_introduction_CN_files/01_stable_diffusion_introduction_CN_40_0.png
@@ -0,0 +1,3 @@
+version https://git-lfs.github.com/spec/v1
+oid sha256:6fa30efea8a9def3ade8f3a350cb1a2853e1f9833d66ef96dc6c92afb19aeea0
+size 412591
diff --git a/markdown/unit3/01_stable_diffusion_introduction_CN_files/01_stable_diffusion_introduction_CN_47_1.png b/markdown/unit3/01_stable_diffusion_introduction_CN_files/01_stable_diffusion_introduction_CN_47_1.png
new file mode 100644
index 0000000000000000000000000000000000000000..3e9c7ef29ca69b1582e844b59424baabb0e85a43
--- /dev/null
+++ b/markdown/unit3/01_stable_diffusion_introduction_CN_files/01_stable_diffusion_introduction_CN_47_1.png
@@ -0,0 +1,3 @@
+version https://git-lfs.github.com/spec/v1
+oid sha256:ab2a1e4cb0f45ef18ef8ef107e28aad922b9010ab4c0c99878e3add4e55e8042
+size 541569
diff --git a/markdown/unit3/01_stable_diffusion_introduction_CN_files/01_stable_diffusion_introduction_CN_53_1.png b/markdown/unit3/01_stable_diffusion_introduction_CN_files/01_stable_diffusion_introduction_CN_53_1.png
new file mode 100644
index 0000000000000000000000000000000000000000..e414f5ff94ee64bcb17e142b1748a3ac54da97db
--- /dev/null
+++ b/markdown/unit3/01_stable_diffusion_introduction_CN_files/01_stable_diffusion_introduction_CN_53_1.png
@@ -0,0 +1,3 @@
+version https://git-lfs.github.com/spec/v1
+oid sha256:7220259404c4b91b50f89464af1fbe09338f9401500e796d60fa562e69a8dc4d
+size 509033
diff --git a/markdown/unit3/01_stable_diffusion_introduction_CN_files/01_stable_diffusion_introduction_CN_62_1.png b/markdown/unit3/01_stable_diffusion_introduction_CN_files/01_stable_diffusion_introduction_CN_62_1.png
new file mode 100644
index 0000000000000000000000000000000000000000..9616149e36d00576ff764fa52d5710315ccee48e
--- /dev/null
+++ b/markdown/unit3/01_stable_diffusion_introduction_CN_files/01_stable_diffusion_introduction_CN_62_1.png
@@ -0,0 +1,3 @@
+version https://git-lfs.github.com/spec/v1
+oid sha256:f34d9df0b67bebdd41e0c273f3cc77f44e66e4e1b05ab05a6cbe4f4643598ba1
+size 547516
diff --git a/markdown/unit3/README_CN.md b/markdown/unit3/README_CN.md
new file mode 100755
index 0000000000000000000000000000000000000000..1f0757ccd6e2ad8039d91b45c07616531e054fce
--- /dev/null
+++ b/markdown/unit3/README_CN.md
@@ -0,0 +1,102 @@
+# 单元3:Stable Diffusion
+
+欢迎来到 Hugging Face 扩散模型课程的第三单元!在这一单元,你将会遇见一个非常强大的扩散模型,名叫 Stable Diffusion(SD),我们将探索它到底能干点什么。
+
+## 开始这一单元 :rocket:
+
+这里分几步学习这一单元:
+
+- 请首先确保你已经[注册了本课程](https://huggingface.us17.list-manage.com/subscribe?u=7f57e683fa28b51bfc493d048&id=ef963b4162),以便有新的学习资料时你会被通知到。
+- 请先通读本文,对本单元的重点有一个整体的认识。
+- 学习 [_**Stable Diffusion Introduction**_ notebook](#hands-on-notebook) 这节笔记本,来看看SD是如何实际应用到一些常见的应用场景中的。
+- 使用 [**hackathon** 文件夹](../hackathon)中的 **Dreambooth** 笔记本,微调你自己自定义的 Stable Diffusion 模型,并与社区分享,看看你能不能赢得点奖项和赠品。
+- (可选)观看视频 [**Stable Diffusion Deep Dive**](https://www.youtube.com/watch?app=desktop&v=0_BBRNYInx8)并学习相关 [**笔记本**](https://github.com/fastai/diffusion-nbs/blob/master/Stable%20Diffusion%20Deep%20Dive.ipynb),深入了解不同的组件以及它们如何发挥不同的作用。这些学习资料是为了最新的 FastAI 课程 [Stable Diffusion from the Foundations](https://www.fast.ai/posts/part2-2022.html)准备的 —— 前几课已经可以观看了,剩下的课程也会在近几个月内放出。这个课程也是对本教程的一个非常好的补充,适合任何对从零建立这些模型感兴趣的人。
+
+
+:loudspeaker: 别忘了加入[Discord](https://huggingface.co/join/discord),在这里你可以参与学习资料的讨论,在`#diffusion-models-class`频道分享你的实验成果。
+
+## 简介
+
+
+_示例图片,由 Stable Diffusion 生成_
+
+Stable Diffusion 是一个强大的文本条件隐式扩散模型(text-conditioned latent diffusion model)。不要担心,我们马上就会解释这些名词。它根据文字描述生成令人惊艳的图片的能力震惊了整个互联网。在本单元,我们将会一探 SD 的工作原理并了解一下它还能搞出什么其它花招。
+
+## 隐式扩散(Latent Diffusion)
+
+当图片尺寸变大时,需要的计算能力也随之增加。这种现象在自注意力机制(self-attention)这种操作的影响下尤为突出,因为操作数随着输入量的增大呈平方地增加。一个 128px 的正方形图片有着四倍于 64px 正方形图片的像素数量,所以在自注意力层就需要16倍(42)的内存和计算量。这是任何需要生成高分辨率图片的人都会遇到的问题。
+
+
+_Diagram from the [Latent Diffusion paper](http://arxiv.org/abs/2112.10752)_
+
+隐式扩散致力于克服这一难题,它使用一个独立的模型 Variational Auto-Encoder(VAE)**压缩**图片到一个更小的空间维度。这背后的原理是,图片通场都包含了大量的冗余信息 —— 给定足够的训练数据,一个 VAE 可以学会产出一个比输入图片小得多的表征,并把这个**隐式**表征重建回原图片,同时保留较高的保真度。SD 模型中的 VAE 接收一个三通道图片输入,生成出一个四通道的隐式表征,同时每一个空间维度上都减少为原来的八分之一。比如,一个 512px 的正方形图片将会被压缩到一个 4×64×64 的隐式表征上。
+
+通过把扩散过程引用到这些**隐式表征**而不是完整图像上,我们可以从中得到很多好处(更低的内存使用、更少的 UNet 层数、更快的生成速度...)。同时我们仍能把结果使用 VAE 中的解码器解码到高分辨率图片上来查看最终结果。这一创新点极大地降低了训练和运行这些模型的成本。
+
+## 以文本为生成条件
+
+在第二单元,我们展示了如何将额外信息输入到 UNet,让我们对生成的图片有了额外的控制。我们把这种方法叫做条件生成。给定一个带噪图片,我们让模型**基于额外的线索**(比如类别标签,或 Stable Diffusion 中的文字描述)去预测去噪的版本。在推理阶段,我们可以输入对期望图片的文字表述和纯噪声数据作为起始点,然后模型就开始全力对随机输入进行“去噪”,以求生成的图片能匹配上文字描述。
+
+
+_图表:文本编码过程,即将输入的文本提示转化为一系列的文本嵌入(即图中的 encoder_hidden_states),然后输入 Unet 作为生成条件_
+
+为了达成这一目的,我们首先需要为文本创建一个数值的表示形式,用来获取文字描述的相关信息。为此,SD 利用了一个名为CLIP的预训练transformer模型。CLIP 的文本编码器是用来处理图像说明文字、将其转换为可以用来对比图片和文本的形式的。所以这个模型非常适合用来从文字描述来为图像创建有用的表征信息。一个输入文字提示会首先被分词(tokenize,基于一个很大的词汇库把句中的词语或短语转化为一个个的token),然后被输入进 CLIP 的文字编码器,为每个token产出一个 768 维(针对 SD 1.X版本)或1024维(针对SD 2.X版本)的向量。为了使得输入格式一致,文本提示总是被补全或截断到含有 77 个 token 的长度,所以每个文字提示最终作为生成条件的表示形式是一个形状为 77×1024 的张量。
+
+
+
+那我们如何实际地将这些条件信息输入到 UNet 里让它预测使用呢?答案是使用交叉注意力机制(cross-attention)。交叉注意力层从头到尾贯穿了 UNet 结构。UNet 中的每个空间位置都可以“注意”文字条件中不同的token,以此从文字提示中获取到了不同位置的相互关联信息。上面的图表就展示了文字条件信息(以及基于时间周期 time-step 的条件)是如何在不同的位置点输入的。 可以看到,UNet 的每一层都由充足的机会去利用这些条件信息!
+
+## 无分类器的引导
+
+然而很多时候,即使我们付出了很多努力尽可能让文本成为生成的条件,但模型仍然会在预测时大量地基于带噪输入图片,而不是文字。在某种程度上,这其实是可以解释得通的 —— 很多说明文字和与之关联的图片相关性很弱,所以模型就学着不去过度依赖文字描述!可是这并不是我们期望的效果。如果模型不遵从文本提示,那么我们很可能得到与我们描述根本不相关的图片。
+
+
+_由描述 "An oil painting of a collie in a top hat" 生成的图片(从左到右的 CFG scale 分别是 0、1、2、10)_
+
+为了解决这一问题,我们使用了一个小技巧,叫做无分类器的引导(Classifie-free Guidance,CGF)。在训练时,我们时不时把文字条件置空,强迫模型去学着在无文字信息的情况下对图片去噪(无条件生成)。在推理阶段,我们分别做两个预测:一个有文字条件,一个没有。我们可以用这两者的差异来建立一个最终结合版的预测,让最终结果在文本条件预测所指明的方向上依据一个缩放系数(即引导尺度)去“走得更远”,希望最终生成一个更好地匹配文字提示的结果。上图就展示了在同一个文本提示下使用不同引导尺度得到的不同结果。可以看到,更高的引导尺度能让生成的图片更接近文字描述。
+
+## 其它类型的条件生成:超分辨率、图像修补、深度图到图像的转换
+
+我们也可以创建各种接收不同生成条件的 Stable Diffusion 模型。比如[深度图到图像转换模型](https://huggingface.co/stabilityai/stable-diffusion-2-depth)使用一个额外的输入通道接收要被去噪的图片的深度信息。所以在推理阶段,我们就可以输入一个目标图片的深度图(由另一个独立的模型预测出来),以此来希望模型生成一个有相似全局结构的图片。
+
+
+_基于深度的 SD 模型可以根据同一个全局结构生成不同的图片(示例来自StabilityAI)_
+
+用相似的方式,我们也可以输入一个低分辨率图片作为条件,让模型生成对应的高分辨率图片([正如Stable Diffusion Upscaler](https://huggingface.co/stabilityai/stable-diffusion-x4-upscaler)一样)。此外,我们还可以输入一个掩膜(mask),让模型知道图像相应的区域需要让模型用in-painting的方式重新生成一下:掩膜外的区域要和原图片保持一致,掩膜内的区域要生成出新的内容。
+
+## 使用 DreamBooth 微调
+
+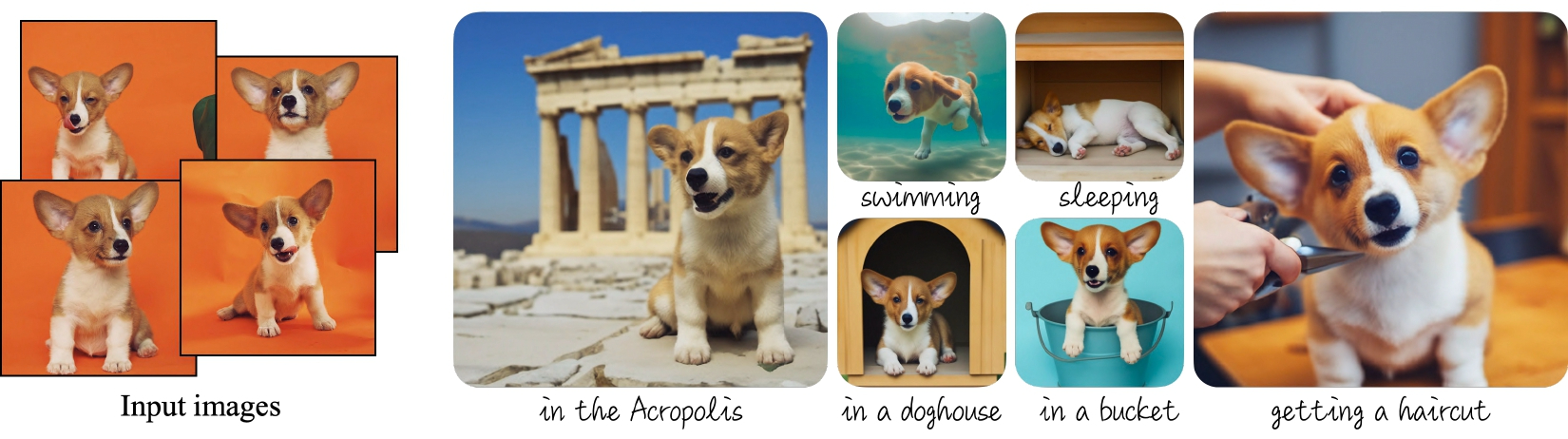
+_由 Imagen model 生成的图片,来自[dreambooth 项目页](https://dreambooth.github.io/)_
+
+DreamBooth 可以用来微调一个文字到图像的生成模型,教它一些新的概念,比如某一特定物体或某种特定风格。这一技术一开始是为 Google 的 Imagen Model 开发的,但被很快应用于 [stable diffusion](https://huggingface.co/docs/diffusers/training/dreambooth) 中。效果十分惊艳(如果你最近在社交媒体上看到谁使用了 AI 生成的头像,那这个头像有很大概率是出自于基于 DreamBooth 的服务),但该技术也对各种设置十分敏感。所以请学习我们这一单元的笔记本,并阅读[这个对不同训练参数的调研资料](https://huggingface.co/blog/dreambooth)来获取些参考,让模型尽可能地起作用。
+
+## 用来上手的笔记本示例
+
+| Chapter | Colab | Kaggle | Gradient | Studio Lab |
+|:--------------------------------------------|:----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------|:---------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------|:---------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------|:-------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------|
+| Stable Diffusion Introduction | [](https://colab.research.google.com/github/huggingface/diffusion-models-class/blob/main/unit3/01_stable_diffusion_introduction.ipynb) | [](https://kaggle.com/kernels/welcome?src=https://github.com/huggingface/diffusion-models-class/blob/main/unit3/01_stable_diffusion_introduction.ipynb) | [](https://console.paperspace.com/github/huggingface/diffusion-models-class/blob/main/unit3/01_stable_diffusion_introduction.ipynb) | [](https://studiolab.sagemaker.aws/import/github/huggingface/diffusion-models-class/blob/main/unit3/01_stable_diffusion_introduction.ipynb) |
+| DreamBooth Hackathon Notebook | [](https://colab.research.google.com/github/huggingface/diffusion-models-class/blob/main/hackathon/dreambooth.ipynb) | [](https://kaggle.com/kernels/welcome?src=https://github.com/huggingface/diffusion-models-class/blob/main/hackathon/dreambooth.ipynb) | [](https://console.paperspace.com/github/huggingface/diffusion-models-class/blob/main/hackathon/dreambooth.ipynb) | [](https://studiolab.sagemaker.aws/import/github/huggingface/diffusion-models-class/blob/main/hackathon/dreambooth.ipynb) |
+| Stable Diffusion Deep Dive | [](https://colab.research.google.com/github/fastai/diffusion-nbs/blob/master/Stable%20Diffusion%20Deep%20Dive.ipynb ) | [](https://kaggle.com/kernels/welcome?src=https://github.com/fastai/diffusion-nbs/blob/master/Stable%20Diffusion%20Deep%20Dive.ipynb ) | [](https://console.paperspace.com/github/fastai/diffusion-nbs/blob/master/Stable%20Diffusion%20Deep%20Dive.ipynb ) | [](https://studiolab.sagemaker.aws/import/github/fastai/diffusion-nbs/blob/master/Stable%20Diffusion%20Deep%20Dive.ipynb ) |
+
+至此,你已经准备好学习相关笔记本了!通过上面的链接使用你选择的平台打开它们!DreamBooth 需要的算力比较多,所以如果你是用的 Kaggle 或 Google Colab,那请确保将运行时模式设为 GPU 来获得最好效果。
+
+Stable Diffusion Introduction 这节笔记本是使用🤗 Diffusers 库对 Stable Diffusion 所作的一个简短介绍,包含了一些基本的使用管线生成和修改图片的示例。
+
+在 DreamBooth Hackathon 这节笔记本(在 [hackathon 文件夹里](../hackathon))中,我们展示了如何使用你自己的图片微调 SD,来获取一个涵盖新的风格或内容的自定义版本模型。
+
+最后,在 Stable Diffusion Deep Dive 笔记本中和视频中,我们分解了一个典型的生成管线中的每一个步骤,并提出了一些新奇的方法去对每一步进行修改,来有创造性地控制生成。
+
+## 项目时间
+
+仿照 **DreamBooth** 笔记本中的指导,针对某个特定种类的数据训练你自己的模型。请确保在提交时加入一些输出的例子,以便我们为不同的种类数据选出最好的模型!请查阅 [hackathon 信息](../hackathon),进一步了解关于奖项、GPU额度等信息。
+
+## 一些其它学习资源
+
+- [High-Resolution Image Synthesis with Latent Diffusion Models](http://arxiv.org/abs/2112.10752) - 这篇论文介绍了 Stable Diffusion 背后的方法
+
+- [CLIP](https://openai.com/blog/clip/) - CLIP 学习着去将文字和图片联系起来,CLIP 文本编码器被用来将文本提示转化为 SD 使用的信息量丰富的数值表征形式。也可查阅 [这篇关于 OpenCLIP 的文献](https://wandb.ai/johnowhitaker/openclip-benchmarking/reports/Exploring-OpenCLIP--VmlldzoyOTIzNzIz),了解最近的一些 CLIP 开源版本(其中一个被用于第二版 SD 模型)。
+
+- [GLIDE: Towards Photorealistic Image Generation and Editing with Text-Guided Diffusion Models](https://arxiv.org/abs/2112.10741) 是一篇较早的论文,介绍了把文本作为生成条件以及 CFG 相关内容。
+
+如果你找到了更好的学习资源,也别忘了告诉我们让我们加到这个列表里!
diff --git a/markdown/unit3/cfg_example_0_1_2_10.jpeg b/markdown/unit3/cfg_example_0_1_2_10.jpeg
new file mode 100644
index 0000000000000000000000000000000000000000..b685f6157517a933e19755425d207fc6dea72a35
--- /dev/null
+++ b/markdown/unit3/cfg_example_0_1_2_10.jpeg
@@ -0,0 +1,3 @@
+version https://git-lfs.github.com/spec/v1
+oid sha256:a5d9636a1381fe0e9570774ddda7a88d23fe01c52fa4ae563850686efc84a685
+size 204059
diff --git a/markdown/unit3/sd_demo_images.jpg b/markdown/unit3/sd_demo_images.jpg
new file mode 100644
index 0000000000000000000000000000000000000000..5f2770e21c196774cb52ab55d5dfee2bf9721bd6
--- /dev/null
+++ b/markdown/unit3/sd_demo_images.jpg
@@ -0,0 +1,3 @@
+version https://git-lfs.github.com/spec/v1
+oid sha256:cfd6aca118d829e505cfb60167f6020c5e9680690bd747b50e38350b8377d6a8
+size 227696
diff --git a/markdown/unit3/sd_unet_color.png b/markdown/unit3/sd_unet_color.png
new file mode 100644
index 0000000000000000000000000000000000000000..4ac89f297f2b9a6c90933a84db65d6d3380387fa
--- /dev/null
+++ b/markdown/unit3/sd_unet_color.png
@@ -0,0 +1,3 @@
+version https://git-lfs.github.com/spec/v1
+oid sha256:9a66f5943946a88a8e39ac686fb7d4d7d13acd740b2f8f146c7f1e01dec340a7
+size 190494
diff --git a/markdown/unit3/text_encoder_noborder.png b/markdown/unit3/text_encoder_noborder.png
new file mode 100644
index 0000000000000000000000000000000000000000..9c534a526fc61c0aafc0ded3eefb3d18365d5edb
--- /dev/null
+++ b/markdown/unit3/text_encoder_noborder.png
@@ -0,0 +1,3 @@
+version https://git-lfs.github.com/spec/v1
+oid sha256:befb00d52c33291fb23739e1214ab4977a1b6476d56b199b13a1e310997f6a0f
+size 55584
diff --git a/markdown/unit4/01_ddim_inversion_CN.md b/markdown/unit4/01_ddim_inversion_CN.md
new file mode 100644
index 0000000000000000000000000000000000000000..9802309d168520a4e7c6fb7b39dc6937e922a49d
--- /dev/null
+++ b/markdown/unit4/01_ddim_inversion_CN.md
@@ -0,0 +1,570 @@
+# DDIM反转
+
+在此篇笔记我们会来探索**反转**,看看它是如何影响取样的,并把它应用到扩散模型的编辑图像功能中去。
+
+## 你将会学到什么
+
+- DDIM采样是怎么工作的
+- 确定性vs随机性取样器
+- DDIM反转的理论支撑
+- 使用反转来编辑图像
+
+我们开始吧!
+
+## 设置
+
+
+```python
+# !pip install -q transformers diffusers accelerate
+```
+
+
+```python
+import torch
+import requests
+import torch.nn as nn
+import torch.nn.functional as F
+from PIL import Image
+from io import BytesIO
+from tqdm.auto import tqdm
+from matplotlib import pyplot as plt
+from torchvision import transforms as tfms
+from diffusers import StableDiffusionPipeline, DDIMScheduler
+
+# Useful function for later
+def load_image(url, size=None):
+ response = requests.get(url,timeout=0.2)
+ img = Image.open(BytesIO(response.content)).convert('RGB')
+ if size is not None:
+ img = img.resize(size)
+ return img
+```
+
+
+```python
+device = torch.device("cuda" if torch.cuda.is_available() else "cpu")
+```
+
+## 加载一个已训练的pipeline
+
+
+```python
+# Load a pipeline
+pipe = StableDiffusionPipeline.from_pretrained("runwayml/stable-diffusion-v1-5").to(device)
+```
+
+
+```python
+# Set up a DDIM scheduler:
+pipe.scheduler = DDIMScheduler.from_config(pipe.scheduler.config)
+```
+
+
+```python
+# Sample an image to make sure it is all working
+prompt = 'Beautiful DSLR Photograph of a penguin on the beach, golden hour'
+negative_prompt = 'blurry, ugly, stock photo'
+im = pipe(prompt, negative_prompt=negative_prompt).images[0]
+im.resize((256, 256)) # resize for convenient viewing
+```
+
+## DDIM取样过程
+
+在给定时间 $t$, 带噪图像 $x_t$ 是原始图像($x_0$)与一些噪声 ($\epsilon$)的叠加。这是在DDIM论文中$x_t$的定义式,我们把它引用到此节里:
+
+$$ x_t = \sqrt{\alpha_t}x_0 + \sqrt{1-\alpha_t}\epsilon $$
+
+$\epsilon$ 是一些归一方差的高斯噪声
+$\alpha_t$ ('alpha')在DDPM论文中也被叫做$\bar{\alpha}$ ('alpha_bar'),被用来定义噪声调度器(scheduler)。在扩散模型中,alpha调度器是被计算出来并排序存储在`scheduler.alphas_cumprod`中。这令人费解,我理解!我们把这些值画出来,然后在下文中我们会使用DDIM的标注方式。
+
+
+```python
+# Plot 'alpha' (alpha_bar in DDPM language, alphas_cumprod in diffusers for clarity)
+timesteps = pipe.scheduler.timesteps.cpu()
+alphas = pipe.scheduler.alphas_cumprod[timesteps]
+plt.plot(timesteps, alphas, label='alpha_t');
+plt.legend();
+```
+
+最初(timestep 0 ,图中左侧)是从一个无噪的干净图像开始,$\alpha_t = 1$。当我们到达更高的timesteps,我们得到一个几乎全是噪声的图像,$\alpha_t$也几乎下降到0。
+
+在采样过程,我们从timestep1000的纯噪声开始,慢慢地向timestep0前进。为了计算采样轨迹中的下一时刻($x_{t-1}$因为我们是从后向前移动)的值,我们预测噪声($\epsilon_\theta(x_t)$,这就是我们模型的输出),用它来预测出无噪的图片$x_0$。在这之后我们用这个预测结果朝着'$x_t$的方向'方向移动一小步。最终,我们可以加一些带$\sigma_t$系数的额外噪声。这是论文中与上述操作相关的章节内容:
+
+
+
+好,我们有了在可控量度噪声下,从$x_t$ 移动到 $x_{t-1}$的公式。今天我们所说案例是不需要再额外添加噪声的 - 即完全确定的DDIM采样。我们来看看这些是如何用代码表达的。
+
+
+```python
+# Sample function (regular DDIM)
+@torch.no_grad()
+def sample(prompt, start_step=0, start_latents=None,
+ guidance_scale=3.5, num_inference_steps=30,
+ num_images_per_prompt=1, do_classifier_free_guidance=True,
+ negative_prompt='', device=device):
+
+ # Encode prompt
+ text_embeddings = pipe._encode_prompt(
+ prompt, device, num_images_per_prompt, do_classifier_free_guidance, negative_prompt
+ )
+
+ # Set num inference steps
+ pipe.scheduler.set_timesteps(num_inference_steps, device=device)
+
+ # Create a random starting point if we don't have one already
+ if start_latents is None:
+ start_latents = torch.randn(1, 4, 64, 64, device=device)
+ start_latents *= pipe.scheduler.init_noise_sigma
+
+ latents = start_latents.clone()
+
+ for i in tqdm(range(start_step, num_inference_steps)):
+
+ t = pipe.scheduler.timesteps[i]
+
+ # expand the latents if we are doing classifier free guidance
+ latent_model_input = torch.cat([latents] * 2) if do_classifier_free_guidance else latents
+ latent_model_input = pipe.scheduler.scale_model_input(latent_model_input, t)
+
+ # predict the noise residual
+ noise_pred = pipe.unet(latent_model_input, t, encoder_hidden_states=text_embeddings).sample
+
+ # perform guidance
+ if do_classifier_free_guidance:
+ noise_pred_uncond, noise_pred_text = noise_pred.chunk(2)
+ noise_pred = noise_pred_uncond + guidance_scale * (noise_pred_text - noise_pred_uncond)
+
+
+ # Normally we'd rely on the scheduler to handle the update step:
+ # latents = pipe.scheduler.step(noise_pred, t, latents).prev_sample
+
+ # Instead, let's do it ourselves:
+ prev_t = max(1, t.item() - (1000//num_inference_steps)) # t-1
+ alpha_t = pipe.scheduler.alphas_cumprod[t.item()]
+ alpha_t_prev = pipe.scheduler.alphas_cumprod[prev_t]
+ predicted_x0 = (latents - (1-alpha_t).sqrt()*noise_pred) / alpha_t.sqrt()
+ direction_pointing_to_xt = (1-alpha_t_prev).sqrt()*noise_pred
+ latents = alpha_t_prev.sqrt()*predicted_x0 + direction_pointing_to_xt
+
+ # Post-processing
+ images = pipe.decode_latents(latents)
+ images = pipe.numpy_to_pil(images)
+
+ return images
+```
+
+
+```python
+# Test our sampling function by generating an image
+sample('Watercolor painting of a beach sunset', negative_prompt=negative_prompt, num_inference_steps=50)[0].resize((256, 256))
+```
+
+看看你是否能把这些代码和论文中的公式对应起来。注意$\sigma$=0是因为我们只注意 无-额外-噪声 的场景,所以我们略去了公式中的那部分。
+
+## 反转
+
+反转的目标就是'颠倒'取样的过程。我们想最终得到一个带噪的隐式(latent),如果把它作为我们正常取样过程的起始点,结果将生成一副原图像。
+
+这里我们先加载一个原始图像,当然你也可以生成一副图像来代替。
+
+
+```python
+# https://www.pexels.com/photo/a-beagle-on-green-grass-field-8306128/
+input_image = load_image('https://images.pexels.com/photos/8306128/pexels-photo-8306128.jpeg', size=(512, 512))
+input_image
+```
+
+我们可以用包含随意分类指引(classifier-free-guidance)的prompt来做反转操作,输入一个图片的描述:
+
+
+```python
+input_image_prompt = "Photograph of a puppy on the grass"
+```
+
+接下来我们来把这个PIL图像变成一些列隐式,它们会被用来当作反转的起点:
+
+
+```python
+# encode with VAE
+with torch.no_grad(): latent = pipe.vae.encode(tfms.functional.to_tensor(input_image).unsqueeze(0).to(device)*2-1)
+l = 0.18215 * latent.latent_dist.sample()
+```
+
+好了,到有趣的部分了。这个函数看起来和上面的取样函数很像,但我们在timesteps上是在向相反的方向移动,从t=0开始,向越来越多的噪声前进。代替更新隐式时噪声会越来越少,我们估计所预测出的噪声,用它来撤回一步更新操作,把它们从t移动到t+1。
+
+
+```python
+## Inversion
+@torch.no_grad()
+def invert(start_latents, prompt, guidance_scale=3.5, num_inference_steps=80,
+ num_images_per_prompt=1, do_classifier_free_guidance=True,
+ negative_prompt='', device=device):
+
+ # Encode prompt
+ text_embeddings = pipe._encode_prompt(
+ prompt, device, num_images_per_prompt, do_classifier_free_guidance, negative_prompt
+ )
+
+ # latents are now the specified start latents
+ latents = start_latents.clone()
+
+ # We'll keep a list of the inverted latents as the process goes on
+ intermediate_latents = []
+
+ # Set num inference steps
+ pipe.scheduler.set_timesteps(num_inference_steps, device=device)
+
+ # Reversed timesteps <<<<<<<<<<<<<<<<<<<<
+ timesteps = reversed(pipe.scheduler.timesteps)
+
+ for i in tqdm(range(1, num_inference_steps), total=num_inference_steps-1):
+
+ # We'll skip the final iteration
+ if i >= num_inference_steps - 1: continue
+
+ t = timesteps[i]
+
+ # expand the latents if we are doing classifier free guidance
+ latent_model_input = torch.cat([latents] * 2) if do_classifier_free_guidance else latents
+ latent_model_input = pipe.scheduler.scale_model_input(latent_model_input, t)
+
+ # predict the noise residual
+ noise_pred = pipe.unet(latent_model_input, t, encoder_hidden_states=text_embeddings).sample
+
+ # perform guidance
+ if do_classifier_free_guidance:
+ noise_pred_uncond, noise_pred_text = noise_pred.chunk(2)
+ noise_pred = noise_pred_uncond + guidance_scale * (noise_pred_text - noise_pred_uncond)
+
+ current_t = max(0, t.item() - (1000//num_inference_steps))#t
+ next_t = t # min(999, t.item() + (1000//num_inference_steps)) # t+1
+ alpha_t = pipe.scheduler.alphas_cumprod[current_t]
+ alpha_t_next = pipe.scheduler.alphas_cumprod[next_t]
+
+ # Inverted update step (re-arranging the update step to get x(t) (new latents) as a function of x(t-1) (current latents)
+ latents = (latents - (1-alpha_t).sqrt()*noise_pred)*(alpha_t_next.sqrt()/alpha_t.sqrt()) + (1-alpha_t_next).sqrt()*noise_pred
+
+
+ # Store
+ intermediate_latents.append(latents)
+
+ return torch.cat(intermediate_latents)
+
+```
+
+把它在小狗图片的隐式表达上运行,我们可以在反转的中间过程得到一系列的隐式:
+
+
+```python
+inverted_latents = invert(l, input_image_prompt,num_inference_steps=50)
+inverted_latents.shape
+```
+
+
+ 0%| | 0/49 [00:00, ?it/s]
+
+
+
+
+
+ torch.Size([48, 4, 64, 64])
+
+
+
+我们可以来看一下最终的隐式 - 希望这可以作为我们尝试新的取样过程的起点噪声:
+
+
+```python
+# Decode the final inverted latents:
+with torch.no_grad():
+ im = pipe.decode_latents(inverted_latents[-1].unsqueeze(0))
+pipe.numpy_to_pil(im)[0]
+```
+
+
+
+
+
+
+
+
+
+
+你可以把这个反转隐式通过正常的 __call__ 方法来传递给pipeline。
+
+
+```python
+pipe(input_image_prompt, latents=inverted_latents[-1][None], num_inference_steps=50, guidance_scale=3.5).images[0]
+
+```
+
+
+ 0%| | 0/50 [00:00, ?it/s]
+
+
+
+
+
+
+
+
+
+
+
+但这里我们遇见了第一个问题:这 **并不是我们一开始的那张图片**!这是因为DDIM的反转依赖一个重要的假设,在t时刻预测的噪声与t+1时刻会是相同的 - 这在我们只反转50或100步时是不陈立的。我们可以寄希望于更多的timesteps开得到一个更准确的反转,但我们也可以'作弊'一下,就是说直接从做对应反转过程中的第20/50步的隐式开始:
+
+
+```python
+# The reason we want to be able to specify start step
+start_step=20
+sample(input_image_prompt, start_latents=inverted_latents[-(start_step+1)][None],
+ start_step=start_step, num_inference_steps=50)[0]
+
+```
+
+
+ 0%| | 0/30 [00:00, ?it/s]
+
+
+
+
+
+
+
+
+
+
+
+距离我们的输入图像已经很接近了!我们为什么要这么做?嗯,这是因为如果我们现在若要用一个新的prompt来生成图像,我们会得到一个匹配于源图像,除了,与新prompt相关的内容。例如,把'小狗'替换为'猫',我们能看到一只猫在几乎一样草地背景上:
+
+
+```python
+# Sampling with a new prompt
+start_step=10
+new_prompt = input_image_prompt.replace('puppy', 'cat')
+sample(new_prompt, start_latents=inverted_latents[-(start_step+1)][None],
+ start_step=start_step, num_inference_steps=50)[0]
+```
+
+
+ 0%| | 0/40 [00:00, ?it/s]
+
+
+
+
+
+
+
+
+
+
+
+### 为什么不直接用 img2img?
+
+为什么要做反转,不是多此一举吗?为什么不直接对输入图片加入噪声,然后用新的promt直接来去噪呢?我们可以这么做,但这会带来一个到处都被改变得夸张得多的照片(如果我们加入了很多噪声),或哪也没怎么变的图像(如果加了太少的噪声)。来自己试试:
+
+
+```python
+start_step = 10
+num_inference_steps=50
+pipe.scheduler.set_timesteps(num_inference_steps)
+noisy_l = pipe.scheduler.add_noise(l, torch.randn_like(l), pipe.scheduler.timesteps[start_step])
+sample(new_prompt, start_latents=noisy_l, start_step=start_step, num_inference_steps=num_inference_steps)[0]
+```
+
+
+ 0%| | 0/40 [00:00, ?it/s]
+
+
+
+
+
+
+
+
+
+
+
+注意背景和草坪有着非常大的变化。
+
+# 把它们都组装起来
+
+来把我们目前所写的代码都组装在一个简单的函数里,输入一张图像和两个prompts,就会得到一个通过反转得到的修改后的图片:
+
+
+```python
+def edit(input_image, input_image_prompt, edit_prompt, num_steps=100, start_step=30, guidance_scale=3.5):
+ with torch.no_grad(): latent = pipe.vae.encode(tfms.functional.to_tensor(input_image).unsqueeze(0).to(device)*2-1)
+ l = 0.18215 * latent.latent_dist.sample()
+ inverted_latents = invert(l, input_image_prompt,num_inference_steps=num_steps)
+ final_im = sample(edit_prompt, start_latents=inverted_latents[-(start_step+1)][None],
+ start_step=start_step, num_inference_steps=num_steps, guidance_scale=guidance_scale)[0]
+ return final_im
+```
+
+And in action:
+实际操作起来:
+
+
+```python
+edit(input_image, 'A puppy on the grass', 'an old grey dog on the grass', num_steps=50, start_step=10)
+```
+
+
+ 0%| | 0/49 [00:00, ?it/s]
+
+
+
+ 0%| | 0/40 [00:00, ?it/s]
+
+
+
+
+
+
+
+
+
+
+
+
+```python
+edit(input_image, 'A puppy on the grass', 'A blue dog on the lawn', num_steps=50, start_step=12, guidance_scale=6)
+```
+
+
+ 0%| | 0/49 [00:00, ?it/s]
+
+
+
+ 0%| | 0/38 [00:00, ?it/s]
+
+
+
+
+
+
+
+
+
+
+
+
+```python
+# Exercise: Try this on some more images! Explore the different parameters
+```
+
+## 更多迭代 = 更好的表现
+
+如果你因为反转结果不准确而烦恼,你可以试试多迭代几次(代价就是更长的运行时间)。为了测试一下反转过程,你可以使用这里的edit函数并输入相同的prompt:
+
+
+```python
+# Inversion test with far more steps:
+edit(input_image, 'A puppy on the grass', 'A puppy on the grass', num_steps=350, start_step=1)
+```
+
+
+ 0%| | 0/349 [00:00, ?it/s]
+
+
+
+ 0%| | 0/349 [00:00, ?it/s]
+
+
+
+
+
+
+
+
+
+
+
+好多了!来试试用它编辑图片:
+
+
+```python
+edit(input_image, 'A photograph of a puppy', 'A photograph of a grey cat', num_steps=150, start_step=30, guidance_scale=5.5)
+```
+
+
+ 0%| | 0/149 [00:00, ?it/s]
+
+
+
+ 0%| | 0/120 [00:00, ?it/s]
+
+
+
+
+
+
+
+
+
+
+
+
+```python
+# source: https://www.pexels.com/photo/girl-taking-photo-1493111/
+face = load_image('https://images.pexels.com/photos/1493111/pexels-photo-1493111.jpeg', size=(512, 512))
+face
+```
+
+
+
+
+
+
+
+
+
+
+
+```python
+edit(face, 'A photograph of a face', 'A photograph of a face with sunglasses', num_steps=250, start_step=30, guidance_scale=3.5)
+```
+
+
+ 0%| | 0/249 [00:00, ?it/s]
+
+
+
+ 0%| | 0/220 [00:00, ?it/s]
+
+
+
+
+
+
+
+
+
+
+
+
+```python
+edit(face, 'A photograph of a face', 'Acrylic palette knife painting of a face, colorful', num_steps=250, start_step=65, guidance_scale=5.5)
+```
+
+
+ 0%| | 0/249 [00:00, ?it/s]
+
+
+
+ 0%| | 0/185 [00:00, ?it/s]
+
+
+
+
+
+
+
+
+
+
+
+# 接下来会是?
+
+有了此篇笔记的帮助,我建议你再研究下['Null-text Inversion'](https://null-text-inversion.github.io/),它是基于DDIM来优化空文本(无条件文字prompt)的反转过程,有着更准确的反转与更好的编辑效果。
diff --git a/markdown/unit4/01_ddim_inversion_CN_files/01_ddim_inversion_CN_32_0.png b/markdown/unit4/01_ddim_inversion_CN_files/01_ddim_inversion_CN_32_0.png
new file mode 100644
index 0000000000000000000000000000000000000000..d94471ae7864abaa28aac3967a6799dbd728530c
--- /dev/null
+++ b/markdown/unit4/01_ddim_inversion_CN_files/01_ddim_inversion_CN_32_0.png
@@ -0,0 +1,3 @@
+version https://git-lfs.github.com/spec/v1
+oid sha256:86d76af2b4ab1199dbb6df1e2cbb97b4906d0112b51abb7949ebbda480faa31f
+size 593387
diff --git a/markdown/unit4/01_ddim_inversion_CN_files/01_ddim_inversion_CN_34_1.png b/markdown/unit4/01_ddim_inversion_CN_files/01_ddim_inversion_CN_34_1.png
new file mode 100644
index 0000000000000000000000000000000000000000..c133b849307ea5bb40e818ecf9c2fda39d0f8e08
--- /dev/null
+++ b/markdown/unit4/01_ddim_inversion_CN_files/01_ddim_inversion_CN_34_1.png
@@ -0,0 +1,3 @@
+version https://git-lfs.github.com/spec/v1
+oid sha256:e66168a0e8a2a21c8272323e239e4bb5cf933b7b2d2b09837b1edd94a97b88da
+size 411736
diff --git a/markdown/unit4/01_ddim_inversion_CN_files/01_ddim_inversion_CN_36_1.png b/markdown/unit4/01_ddim_inversion_CN_files/01_ddim_inversion_CN_36_1.png
new file mode 100644
index 0000000000000000000000000000000000000000..47b8f29ee87ca023bad1dc1e9bab44f2370f5668
--- /dev/null
+++ b/markdown/unit4/01_ddim_inversion_CN_files/01_ddim_inversion_CN_36_1.png
@@ -0,0 +1,3 @@
+version https://git-lfs.github.com/spec/v1
+oid sha256:07d51d7ce45b25f0be9d37228dff87ab107f7db2f0be2f867b4a5079648a9abc
+size 423986
diff --git a/markdown/unit4/01_ddim_inversion_CN_files/01_ddim_inversion_CN_38_1.png b/markdown/unit4/01_ddim_inversion_CN_files/01_ddim_inversion_CN_38_1.png
new file mode 100644
index 0000000000000000000000000000000000000000..ccef4cbe8e335e3e6422e5f41f9ae69867380d52
--- /dev/null
+++ b/markdown/unit4/01_ddim_inversion_CN_files/01_ddim_inversion_CN_38_1.png
@@ -0,0 +1,3 @@
+version https://git-lfs.github.com/spec/v1
+oid sha256:b4586a074bb15e50ec5d6261d9a8e4eab02fa94fca5bae085ddc3274d37ba666
+size 418608
diff --git a/markdown/unit4/01_ddim_inversion_CN_files/01_ddim_inversion_CN_40_1.png b/markdown/unit4/01_ddim_inversion_CN_files/01_ddim_inversion_CN_40_1.png
new file mode 100644
index 0000000000000000000000000000000000000000..b3f4571c071ae896d58d87ed721f191bc327525c
--- /dev/null
+++ b/markdown/unit4/01_ddim_inversion_CN_files/01_ddim_inversion_CN_40_1.png
@@ -0,0 +1,3 @@
+version https://git-lfs.github.com/spec/v1
+oid sha256:78267d83bf9d3d5a7153d503517c2b807923d7d72db397b8af3109f2eb78a54f
+size 387302
diff --git a/markdown/unit4/01_ddim_inversion_CN_files/01_ddim_inversion_CN_45_2.png b/markdown/unit4/01_ddim_inversion_CN_files/01_ddim_inversion_CN_45_2.png
new file mode 100644
index 0000000000000000000000000000000000000000..2606f03300c5b151c0d09f6a4244c16e49ffe126
--- /dev/null
+++ b/markdown/unit4/01_ddim_inversion_CN_files/01_ddim_inversion_CN_45_2.png
@@ -0,0 +1,3 @@
+version https://git-lfs.github.com/spec/v1
+oid sha256:981edbde071a56deaa977160dc30911a13dc3368867c62ab1656c0223cbd0721
+size 425076
diff --git a/markdown/unit4/01_ddim_inversion_CN_files/01_ddim_inversion_CN_46_2.png b/markdown/unit4/01_ddim_inversion_CN_files/01_ddim_inversion_CN_46_2.png
new file mode 100644
index 0000000000000000000000000000000000000000..4855bb05e72f91640cd171e9bab313193f07859b
--- /dev/null
+++ b/markdown/unit4/01_ddim_inversion_CN_files/01_ddim_inversion_CN_46_2.png
@@ -0,0 +1,3 @@
+version https://git-lfs.github.com/spec/v1
+oid sha256:df036d0124b15296a2554b70fc48b39f45eec57b85cfdd5dfc9ec22580929551
+size 430325
diff --git a/markdown/unit4/01_ddim_inversion_CN_files/01_ddim_inversion_CN_49_2.png b/markdown/unit4/01_ddim_inversion_CN_files/01_ddim_inversion_CN_49_2.png
new file mode 100644
index 0000000000000000000000000000000000000000..ad3df74dde36c0a7d80d4db82effea085260738a
--- /dev/null
+++ b/markdown/unit4/01_ddim_inversion_CN_files/01_ddim_inversion_CN_49_2.png
@@ -0,0 +1,3 @@
+version https://git-lfs.github.com/spec/v1
+oid sha256:d9f571854f2a418a5864ceb5c3979531dc8cd80eda98c30890124fba6ac65959
+size 427293
diff --git a/markdown/unit4/01_ddim_inversion_CN_files/01_ddim_inversion_CN_51_2.png b/markdown/unit4/01_ddim_inversion_CN_files/01_ddim_inversion_CN_51_2.png
new file mode 100644
index 0000000000000000000000000000000000000000..8edcf7d7c685b5506c6fbfb44783f8855cc226e6
--- /dev/null
+++ b/markdown/unit4/01_ddim_inversion_CN_files/01_ddim_inversion_CN_51_2.png
@@ -0,0 +1,3 @@
+version https://git-lfs.github.com/spec/v1
+oid sha256:26e5e5841478cec02a0fa2e5d11b399604794e5f57ccfa4c02428d34cc26dd9b
+size 436567
diff --git a/markdown/unit4/01_ddim_inversion_CN_files/01_ddim_inversion_CN_52_0.png b/markdown/unit4/01_ddim_inversion_CN_files/01_ddim_inversion_CN_52_0.png
new file mode 100644
index 0000000000000000000000000000000000000000..5663b1eb4d9806c3c9ef0b76ea343e7d795ace13
--- /dev/null
+++ b/markdown/unit4/01_ddim_inversion_CN_files/01_ddim_inversion_CN_52_0.png
@@ -0,0 +1,3 @@
+version https://git-lfs.github.com/spec/v1
+oid sha256:0ad6233c1dcba4f19ad4361e202b99a49261db0b25a7bdb03061f427703173e1
+size 324984
diff --git a/markdown/unit4/01_ddim_inversion_CN_files/01_ddim_inversion_CN_53_2.png b/markdown/unit4/01_ddim_inversion_CN_files/01_ddim_inversion_CN_53_2.png
new file mode 100644
index 0000000000000000000000000000000000000000..2a9674696d2f19636b5cb5c29629728740793306
--- /dev/null
+++ b/markdown/unit4/01_ddim_inversion_CN_files/01_ddim_inversion_CN_53_2.png
@@ -0,0 +1,3 @@
+version https://git-lfs.github.com/spec/v1
+oid sha256:2aa6b0897da211eabd95437d99a3386f27c092e5eac3f708ea66460b73477fd4
+size 317598
diff --git a/markdown/unit4/01_ddim_inversion_CN_files/01_ddim_inversion_CN_54_2.png b/markdown/unit4/01_ddim_inversion_CN_files/01_ddim_inversion_CN_54_2.png
new file mode 100644
index 0000000000000000000000000000000000000000..aac269ef7396f3709e0b21577e05afbcb3e40001
--- /dev/null
+++ b/markdown/unit4/01_ddim_inversion_CN_files/01_ddim_inversion_CN_54_2.png
@@ -0,0 +1,3 @@
+version https://git-lfs.github.com/spec/v1
+oid sha256:f479cf2004b0119405d91dedf791bea6f2f9031857e185af9eccae922c3122f7
+size 337488
diff --git a/markdown/unit4/02_diffusion_for_audio_CN.md b/markdown/unit4/02_diffusion_for_audio_CN.md
new file mode 100644
index 0000000000000000000000000000000000000000..66e91b48132af8265dfce272cfd73ec1d35f4d8f
--- /dev/null
+++ b/markdown/unit4/02_diffusion_for_audio_CN.md
@@ -0,0 +1,632 @@
+# 音频扩散模型
+
+在此篇笔记,我们将简短地来看看使用扩散模型生成音频的过程。
+
+## 你将会学习到:
+- 音频在电脑中被如何展示
+- 源音频数据与频谱间的转换方法
+- 如何准备一个由特定的整理函数(collate function),能够把音频片段转换到频谱的数据生成器
+- 微调一个指定分类曲风的音频扩散模型
+- 把自己的pipeline上传到HFhub
+
+警告:这完全是出于教学目的 - 不能保证我们的模型一定很好听😉
+
+我们开始吧!
+
+## 设置与导入
+
+
+```python
+# !pip install -q datasets diffusers torchaudio accelerate
+```
+
+
+```python
+import torch, random
+import numpy as np
+import torch.nn.functional as F
+from tqdm.auto import tqdm
+from IPython.display import Audio
+from matplotlib import pyplot as plt
+from diffusers import DiffusionPipeline
+from torchaudio import transforms as AT
+from torchvision import transforms as IT
+
+```
+
+## 从预训练的音频Pipline开始
+
+我们通过参考[Audio Diffusion docs](https://huggingface.co/docs/diffusers/api/pipelines/audio_diffusion)来加载一个预训练的音频扩散模型pipeline:
+
+
+```python
+# Load a pre-trained audio diffusion pipeline
+device = "cuda" if torch.cuda.is_available() else "cpu"
+pipe = DiffusionPipeline.from_pretrained("teticio/audio-diffusion-instrumental-hiphop-256").to(device)
+```
+
+
+ Fetching 5 files: 0%| | 0/5 [00:00, ?it/s]
+
+
+如同我们在之前单元使用的pipelines一样,我们可以这样调用pipeline来创造几个样例:
+
+
+```python
+# Sample from the pipeline and display the outputs:
+output = pipe()
+display(output.images[0])
+display(Audio(output.audios[0], rate=pipe.mel.get_sample_rate()))
+```
+
+
+ 0%| | 0/1000 [00:00, ?it/s]
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+这里,`rate`参数明确了音频的 _采样率_ ;过会儿我们会再深入了解它。同时你也会注意到pipeline也返回了几样其他的东西。这发生了什么?我们来仔细看看这两个输出。
+
+第一项是一个数据数组,代表生成的音频:
+
+
+```python
+# The audio array:
+output.audios[0].shape
+```
+
+
+
+
+ (1, 130560)
+
+
+
+第二项看起来像是灰度图:
+
+
+```python
+# The output image (spectrogram):
+output.images[0].size
+```
+
+
+
+
+ (256, 256)
+
+
+
+这给了我们一个提示,关于这个pipeline是如何工作的。音频不是直接被扩散模型生成 - 而是,这个pipeline有着与在第一单元看到的无条件图像生成pipelines类似的2D Unet结构 [Unit 1](https://github.com/huggingface/diffusion-models-class/tree/main/unit1) 用它来生成频谱,之后再在后处理中把它变化为最终的音频。
+
+此pipe中有额外的组件来处理这个变化,我们可以通过`pipe.mel`来进行:
+
+
+```python
+pipe.mel
+```
+
+
+
+
+ Mel {
+ "_class_name": "Mel",
+ "_diffusers_version": "0.12.0.dev0",
+ "hop_length": 512,
+ "n_fft": 2048,
+ "n_iter": 32,
+ "sample_rate": 22050,
+ "top_db": 80,
+ "x_res": 256,
+ "y_res": 256
+ }
+
+
+
+## 音频到频谱的转换
+
+音频的'波形'从时间上表现出了源音频 - 比如,这可能是接收自麦克风的电信号。从这种'时域'的表达方式上做处理会有些棘手,所以有种更普遍的做法把它转换成其他形式,通常把这叫做频谱。频谱直接展示出在不同频率(y轴)与时间(x轴)上的剧烈程度。
+
+
+```python
+# Calculate and show a spectrogram for our generated audio sample using torchaudio
+spec_transform = AT.Spectrogram(power=2)
+spectrogram = spec_transform(torch.tensor(output.audios[0]))
+print(spectrogram.min(), spectrogram.max())
+log_spectrogram = spectrogram.log()
+plt.imshow(log_spectrogram[0], cmap='gray');
+```
+
+ tensor(0.) tensor(6.0842)
+
+
+
+
+
+
+
+
+我们刚刚做好的这个频谱取值范围在0.0000000000001到1之间,其中大部分内容都接近取值下限。这对于可视化与建模并不理想 - 实际上我们需要对这些值取log来得到一个可以看到更多细节的灰度图。同样也因此,我们特别使用一种专门的梅尔频谱(Mel spectrogram),这是一种通过对不同频率成分做一些变化,专门设计的一种符合人耳感知特性而利于提取重要信息的方式。
+
+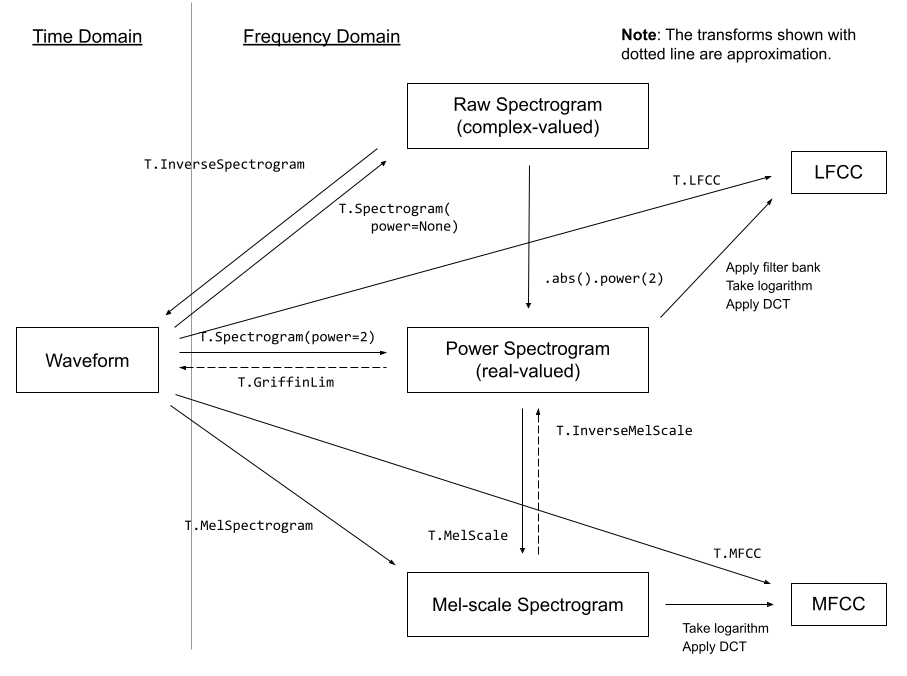
+_一些来自 [torchaudio docs](https://pytorch.org/audio/stable/transforms.html)的音频转换方法_
+
+幸运的是,我们并不需要太过于担心这些变换方法 - pipeline中的`mel`功能会为我们处理这些细节。这样操作,我们就能把频谱图像转换成音频:
+
+
+```python
+a = pipe.mel.image_to_audio(output.images[0])
+a.shape
+```
+
+
+
+
+ (130560,)
+
+
+
+我们可以先读出源音频数据然后调用 `audio_slice_to_image()`函数来把音频数组数据转化为频谱图片。更长的片段会被自动切片为能够正常输出 256x256 频谱图片的长度。
+
+
+```python
+pipe.mel.load_audio(raw_audio=a)
+im = pipe.mel.audio_slice_to_image(0)
+im
+```
+
+
+
+
+
+
+
+
+
+
+音频被表现为一串很长的数字数组。要把它播放出来的话,我们还需要一个关键信息:采样率。我们要用到多少个采样点(单个的数值),才能够播放出单位秒的音频呢?
+
+我们可以在pipeline中这样来看使用的采样率:
+
+
+```python
+sample_rate_pipeline = pipe.mel.get_sample_rate()
+sample_rate_pipeline
+```
+
+
+
+
+ 22050
+
+
+
+如果我们故意把采样率设置错误,可以得到一个可能被加速或慢放的音频:
+
+
+```python
+display(Audio(output.audios[0], rate=44100)) # 2x speed
+```
+
+
+
+
+
+
+
+## 微调pipeline
+
+现在我们已经大致理解了这个pipeline是怎么工作的,现在来在一些新音频数据上对它进行微调!
+
+这个数据集是不同类别的音频片段集合,我们可以从hub上这样加载它:
+
+
+```python
+from datasets import load_dataset
+dataset = load_dataset('lewtun/music_genres', split='train')
+dataset
+```
+
+ Using custom data configuration lewtun--music_genres-2cfa9201f94788d8
+ Found cached dataset parquet (/home/ubuntu/.cache/huggingface/datasets/lewtun___parquet/lewtun--music_genres-2cfa9201f94788d8/0.0.0/2a3b91fbd88a2c90d1dbbb32b460cf621d31bd5b05b934492fdef7d8d6f236ec)
+
+
+
+
+
+ Dataset({
+ features: ['audio', 'song_id', 'genre_id', 'genre'],
+ num_rows: 19909
+ })
+
+
+
+你可以使用下面的代码来看看在数据集中各类别样本的占比:
+
+
+```python
+for g in list(set(dataset['genre'])):
+ print(g, sum(x==g for x in dataset['genre']))
+```
+
+ Pop 945
+ Blues 58
+ Punk 2582
+ Old-Time / Historic 408
+ Experimental 1800
+ Folk 1214
+ Electronic 3071
+ Spoken 94
+ Classical 495
+ Country 142
+ Instrumental 1044
+ Chiptune / Glitch 1181
+ International 814
+ Ambient Electronic 796
+ Jazz 306
+ Soul-RnB 94
+ Hip-Hop 1757
+ Easy Listening 13
+ Rock 3095
+
+
+这个数据集把音频存储为数组:
+
+
+```python
+audio_array = dataset[0]['audio']['array']
+sample_rate_dataset = dataset[0]['audio']['sampling_rate']
+print('Audio array shape:', audio_array.shape)
+print('Sample rate:', sample_rate_dataset)
+display(Audio(audio_array, rate=sample_rate_dataset))
+```
+
+ Audio array shape: (1323119,)
+ Sample rate: 44100
+
+
+
+
+
+
+
+
+注意这条音频的采样率会更高 - 如果我们想用手头的这个pipeline,需要对它'重采样'来匹配。这个片段也比pipeline所预设的长度更长。幸运的是,当我们使用`pipe.mel`在加载音频时,会自动把它切片成更短的片区。
+
+
+```python
+a = dataset[0]['audio']['array'] # Get the audio array
+pipe.mel.load_audio(raw_audio=a) # Load it with pipe.mel
+pipe.mel.audio_slice_to_image(0) # View the first 'slice' as a spectrogram
+```
+
+
+
+
+
+
+
+
+
+
+我们要记得去调整采样率,因为此数据集的数据在每秒中有着多两倍的数据点。
+
+
+```python
+sample_rate_dataset = dataset[0]['audio']['sampling_rate']
+sample_rate_dataset
+```
+
+
+
+
+ 44100
+
+
+
+这里我们用torchaudio's transforms(导入为AT)来做音频的重采样,pipe中的`mel`把音频转换为图像,torchvision's transforms(导入为IT)来把图片转换为tensors。这个函数可以把音频片段转换为频谱tensor供训练使用:
+
+
+```python
+resampler = AT.Resample(sample_rate_dataset, sample_rate_pipeline, dtype=torch.float32)
+to_t = IT.ToTensor()
+
+def to_image(audio_array):
+ audio_tensor = torch.tensor(audio_array).to(torch.float32)
+ audio_tensor = resampler(audio_tensor)
+ pipe.mel.load_audio(raw_audio=np.array(audio_tensor))
+ num_slices = pipe.mel.get_number_of_slices()
+ slice_idx = random.randint(0, num_slices-1) # Pic a random slice each time (excluding the last short slice)
+ im = pipe.mel.audio_slice_to_image(slice_idx)
+ return im
+```
+
+来使用我们的`to_image()`函数来组成我们特定的整理函数(collate function)来把数据集转换到dataloader中来训练模型。整理函数定义了如何把一批来自数据集的样例变换为最终训练用数据。在这个例子中我们把每个音频转换为频谱图像再把他们的tensors堆叠起来:
+
+
+```python
+def collate_fn(examples):
+ # to image -> to tensor -> rescale to (-1, 1) -> stack into batch
+ audio_ims = [to_t(to_image(x['audio']['array']))*2-1 for x in examples]
+ return torch.stack(audio_ims)
+
+# Create a dataset with only the 'Chiptune / Glitch' genre of songs
+batch_size=4 # 4 on colab, 12 on A100
+chosen_genre = 'Electronic' # <<< Try training on different genres <<<
+indexes = [i for i, g in enumerate(dataset['genre']) if g == chosen_genre]
+filtered_dataset = dataset.select(indexes)
+dl = torch.utils.data.DataLoader(filtered_dataset.shuffle(), batch_size=batch_size, collate_fn=collate_fn, shuffle=True)
+batch = next(iter(dl))
+print(batch.shape)
+```
+
+ torch.Size([4, 1, 256, 256])
+
+
+**留心: 你可能要用一个更小的batchsize(比如4)除非你有足够的显存可用**
+
+## 训练循环
+
+这是一个在dataloader中读取数据的简洁训练循环,用几个周期来微调pipeline的UNet网络。你可以跳过此块,直接使用下一块代码来加载pipeline。
+
+
+```python
+epochs = 3
+lr = 1e-4
+
+pipe.unet.train()
+pipe.scheduler.set_timesteps(1000)
+optimizer = torch.optim.AdamW(pipe.unet.parameters(), lr=lr)
+
+for epoch in range(epochs):
+ for step, batch in tqdm(enumerate(dl), total=len(dl)):
+
+ # Prepare the input images
+ clean_images = batch.to(device)
+ bs = clean_images.shape[0]
+
+ # Sample a random timestep for each image
+ timesteps = torch.randint(
+ 0, pipe.scheduler.num_train_timesteps, (bs,), device=clean_images.device
+ ).long()
+
+ # Add noise to the clean images according to the noise magnitude at each timestep
+ noise = torch.randn(clean_images.shape).to(clean_images.device)
+ noisy_images = pipe.scheduler.add_noise(clean_images, noise, timesteps)
+
+ # Get the model prediction
+ noise_pred = pipe.unet(noisy_images, timesteps, return_dict=False)[0]
+
+ # Calculate the loss
+ loss = F.mse_loss(noise_pred, noise)
+ loss.backward(loss)
+
+ # Update the model parameters with the optimizer
+ optimizer.step()
+ optimizer.zero_grad()
+```
+
+
+```python
+# OR: Load the version I trained earlier:
+pipe = DiffusionPipeline.from_pretrained("johnowhitaker/Electronic_test").to(device)
+```
+
+
+ Downloading: 0%| | 0.00/282 [00:00, ?B/s]
+
+
+
+ Fetching 5 files: 0%| | 0/5 [00:00, ?it/s]
+
+
+
+ Downloading: 0%| | 0.00/192 [00:00, ?B/s]
+
+
+
+ Downloading: 0%| | 0.00/294 [00:00, ?B/s]
+
+
+
+ Downloading: 0%| | 0.00/1.03k [00:00, ?B/s]
+
+
+
+ Downloading: 0%| | 0.00/455M [00:00, ?B/s]
+
+
+
+```python
+output = pipe()
+display(output.images[0])
+display(Audio(output.audios[0], rate=22050))
+```
+
+
+ 0%| | 0/1000 [00:00, ?it/s]
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+```python
+# Make a longer sample by passing in a starting noise tensor with a different shape
+noise = torch.randn(1, 1, pipe.unet.sample_size[0],pipe.unet.sample_size[1]*4).to(device)
+output = pipe(noise=noise)
+display(output.images[0])
+display(Audio(output.audios[0], rate=22050))
+```
+
+
+ 0%| | 0/1000 [00:00, ?it/s]
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+这个输出听起来不是最美妙的,但这是一个开始 :)探索一下调整学习率和迭代周期,并在Discord上分享你的最佳结果,我们就可以来一起进步了!
+
+一些需要考虑的事情
+- 我们使用的是256像素点的方形频谱图片,这会限制住我们的batchsize。你能够从128x128的频谱中恢复出质量足够好的音频吗?
+- 为了替代随机图像增强,我们每次挑选不同的音频片段,但这种做法在训练迭代的后期是否可以用其他增强方式再优化一下?
+- 我们有什么其他办法可以用它来生成更长的音频?也许你可以先生成开头的5s音频然后再用类似图像修复的思路接着卡开头片段来继续生成后续内容...
+- 扩散模型生成的内容与图像到图像的生成有什么相同之处?
+
+## Push到Hub
+
+当你对你的模型足够满意了,你就可以把它保存下来并上传到hub上给他人来共享:
+
+
+```python
+from huggingface_hub import get_full_repo_name, HfApi, create_repo, ModelCard
+```
+
+
+```python
+# Pick a name for the model
+model_name = "audio-diffusion-electronic"
+hub_model_id = get_full_repo_name(model_name)
+```
+
+
+```python
+# Save the pipeline locally
+pipe.save_pretrained(model_name)
+```
+
+
+```python
+# Inspect the folder contents
+!ls {model_name}
+```
+
+ mel model_index.json scheduler unet
+
+
+
+```python
+# Create a repository
+create_repo(hub_model_id)
+```
+
+
+
+
+ 'https://huggingface.co/johnowhitaker/Electronic_test'
+
+
+
+
+```python
+# Upload the files
+api = HfApi()
+api.upload_folder(
+ folder_path=f"{model_name}/scheduler", path_in_repo="scheduler", repo_id=hub_model_id
+)
+api.upload_folder(
+ folder_path=f"{model_name}/mel", path_in_repo="mel", repo_id=hub_model_id
+)
+api.upload_folder(folder_path=f"{model_name}/unet", path_in_repo="unet", repo_id=hub_model_id)
+api.upload_file(
+ path_or_fileobj=f"{model_name}/model_index.json",
+ path_in_repo="model_index.json",
+ repo_id=hub_model_id,
+)
+```
+
+
+
+
+ 'https://huggingface.co/johnowhitaker/Electronic_test/blob/main/model_index.json'
+
+
+
+
+```python
+# Push a model card
+content = f"""
+---
+license: mit
+tags:
+- pytorch
+- diffusers
+- unconditional-audio-generation
+- diffusion-models-class
+---
+
+# Model Card for Unit 4 of the [Diffusion Models Class 🧨](https://github.com/huggingface/diffusion-models-class)
+
+This model is a diffusion model for unconditional audio generation of music in the genre {chosen_genre}
+
+## Usage
+
+```python
+from IPython.display import Audio
+from diffusers import DiffusionPipeline
+
+pipe = DiffusionPipeline.from_pretrained("{hub_model_id}")
+output = pipe()
+display(output.images[0])
+display(Audio(output.audios[0], rate=pipe.mel.get_sample_rate()))
+```
+"""
+
+card = ModelCard(content)
+card.push_to_hub(hub_model_id)
+```
+
+
+
+
+ 'https://huggingface.co/johnowhitaker/Electronic_test/blob/main/README.md'
+
+
+
+## 总结
+
+希望这片笔记让你浅尝到音频生成的潜力。请再留意下此单元在介绍中的一些参考链接,去看一些更酷炫的方法和它们所创造的惊艳内容!
diff --git a/markdown/unit4/02_diffusion_for_audio_CN_files/02_diffusion_for_audio_CN_17_1.png b/markdown/unit4/02_diffusion_for_audio_CN_files/02_diffusion_for_audio_CN_17_1.png
new file mode 100644
index 0000000000000000000000000000000000000000..b1db6df1bbe1e83d6b0518ed5253e8857650e00b
--- /dev/null
+++ b/markdown/unit4/02_diffusion_for_audio_CN_files/02_diffusion_for_audio_CN_17_1.png
@@ -0,0 +1,3 @@
+version https://git-lfs.github.com/spec/v1
+oid sha256:d6079cab3bab81154a55724d67efbfaca32338a9482b02b9481017846180345b
+size 40006
diff --git a/markdown/unit4/02_diffusion_for_audio_CN_files/02_diffusion_for_audio_CN_23_0.png b/markdown/unit4/02_diffusion_for_audio_CN_files/02_diffusion_for_audio_CN_23_0.png
new file mode 100644
index 0000000000000000000000000000000000000000..6729d20f7566245945f00dc079caf5101f153aae
--- /dev/null
+++ b/markdown/unit4/02_diffusion_for_audio_CN_files/02_diffusion_for_audio_CN_23_0.png
@@ -0,0 +1,3 @@
+version https://git-lfs.github.com/spec/v1
+oid sha256:147e7ceecc2953ea9ed21a97b0e93286bbaa3a2882cc00a41d1d43d85ceeb2cf
+size 43626
diff --git a/markdown/unit4/02_diffusion_for_audio_CN_files/02_diffusion_for_audio_CN_37_0.png b/markdown/unit4/02_diffusion_for_audio_CN_files/02_diffusion_for_audio_CN_37_0.png
new file mode 100644
index 0000000000000000000000000000000000000000..b217590cf1429dea04a16445ebe71b9aa9c6389c
--- /dev/null
+++ b/markdown/unit4/02_diffusion_for_audio_CN_files/02_diffusion_for_audio_CN_37_0.png
@@ -0,0 +1,3 @@
+version https://git-lfs.github.com/spec/v1
+oid sha256:9b64ae99aa9ba1edf437985809c1bbb54bf0c6ddda3146d1783c8cabe666f874
+size 40345
diff --git a/markdown/unit4/02_diffusion_for_audio_CN_files/02_diffusion_for_audio_CN_48_1.png b/markdown/unit4/02_diffusion_for_audio_CN_files/02_diffusion_for_audio_CN_48_1.png
new file mode 100644
index 0000000000000000000000000000000000000000..76e745ed5e4493c3f4a89fbf316c39f63d0f84e1
--- /dev/null
+++ b/markdown/unit4/02_diffusion_for_audio_CN_files/02_diffusion_for_audio_CN_48_1.png
@@ -0,0 +1,3 @@
+version https://git-lfs.github.com/spec/v1
+oid sha256:bb8425622f549cf0a00240a0a91aba8bb9da6263243ee9f57012a0c4f34985e8
+size 42749
diff --git a/markdown/unit4/02_diffusion_for_audio_CN_files/02_diffusion_for_audio_CN_49_1.png b/markdown/unit4/02_diffusion_for_audio_CN_files/02_diffusion_for_audio_CN_49_1.png
new file mode 100644
index 0000000000000000000000000000000000000000..52508aeaae08184c692db134a867f9bcf257dd6b
--- /dev/null
+++ b/markdown/unit4/02_diffusion_for_audio_CN_files/02_diffusion_for_audio_CN_49_1.png
@@ -0,0 +1,3 @@
+version https://git-lfs.github.com/spec/v1
+oid sha256:c5c99f6100f71ed24a99e766078294c1ced31580c86a5f8b17403d07fba4a47a
+size 163880
diff --git a/markdown/unit4/02_diffusion_for_audio_CN_files/02_diffusion_for_audio_CN_8_1.png b/markdown/unit4/02_diffusion_for_audio_CN_files/02_diffusion_for_audio_CN_8_1.png
new file mode 100644
index 0000000000000000000000000000000000000000..18033ea9795137357ea1a25a64f398c95d8f73e9
--- /dev/null
+++ b/markdown/unit4/02_diffusion_for_audio_CN_files/02_diffusion_for_audio_CN_8_1.png
@@ -0,0 +1,3 @@
+version https://git-lfs.github.com/spec/v1
+oid sha256:2babaa4b3f5d633ada48d83890881e1092044d70f51fad82208977733ad475a2
+size 43502
diff --git a/markdown/unit4/README_CN.md b/markdown/unit4/README_CN.md
new file mode 100644
index 0000000000000000000000000000000000000000..2c1fe9222207a66c95cfb701af737f8158d21fc6
--- /dev/null
+++ b/markdown/unit4/README_CN.md
@@ -0,0 +1,167 @@
+# Unit 4: 深入研究扩散模型
+
+欢迎来到 Hugging Face 扩散模型课程的第四单元!在这个单元中,我们将探讨最新研究中出现的扩散模型的许多改进和扩展。它将比以往的单元代码更少,旨在为您提供进一步研究的起点。
+
+## 开始本单元 :rocket:
+
+以下是本单元的学习步骤:
+
+- 请确保你已经 [注册了该课程](https://huggingface.us17.list-manage.com/subscribe?u=7f57e683fa28b51bfc493d048&id=ef963b4162) 以便在课程中添加其他单元时通知您
+- 阅读下面的材料,以了解本单元中涉及的不同主题的梗概
+- 通过链接的视频和资源深入了解任何您感兴趣的主题
+- 浏览演示笔记本,然后阅读“下一步”部分以获取一些项目建议
+
+:loudspeaker: 别忘了加入我们的 [Discord频道](https://huggingface.co/join/discord), 在这里,您可以讨论课程内容,并在`#diffusion-models-class` 频道中分享您的作品!
+
+## Table of Contents
+
+- [Unit 4: 深入研究扩散模型](#unit-4-going-further-with-diffusion-models)
+ - [开始本单元 :rocket:](#start-this-unit-rocket)
+ - [课程目录](#table-of-contents)
+ - [通过蒸馏进行快速采样](#faster-sampling-via-distillation)
+ - [训练改进](#training-improvements)
+ - [对生成与编辑的更多控制](#more-control-for-generation-and-editing)
+ - [视频](#video)
+ - [音频](#audio)
+ - [新的体系结构和方法 - 走向“迭代优化”](#new-architectures-and-approaches---towards-iterative-refinement)
+ - [动手笔记本](#hands-on-notebooks)
+ - [下一步?](#where-next)
+
+
+## 通过蒸馏进行快速采样
+
+渐进蒸馏是一种采用现有扩散模型并使用它来训练需要更少推理步骤的模型的新版本的技术。“学生”模型初始化自“教师”模型的权重。在训练过程中,教师模型执行两个采样步骤,学生模型尝试在一个步骤中匹配结果预测。这个过程可以重复多次,上一次迭代的学生模型成为下一阶段的教师模型。结果是,这个模型可以用比原来的教师模型少得多的步骤(通常是4或8步)生成较好的样本。核心机制如下图所示,图片来源于[这篇论文](http://arxiv.org/abs/2202.00512):
+
+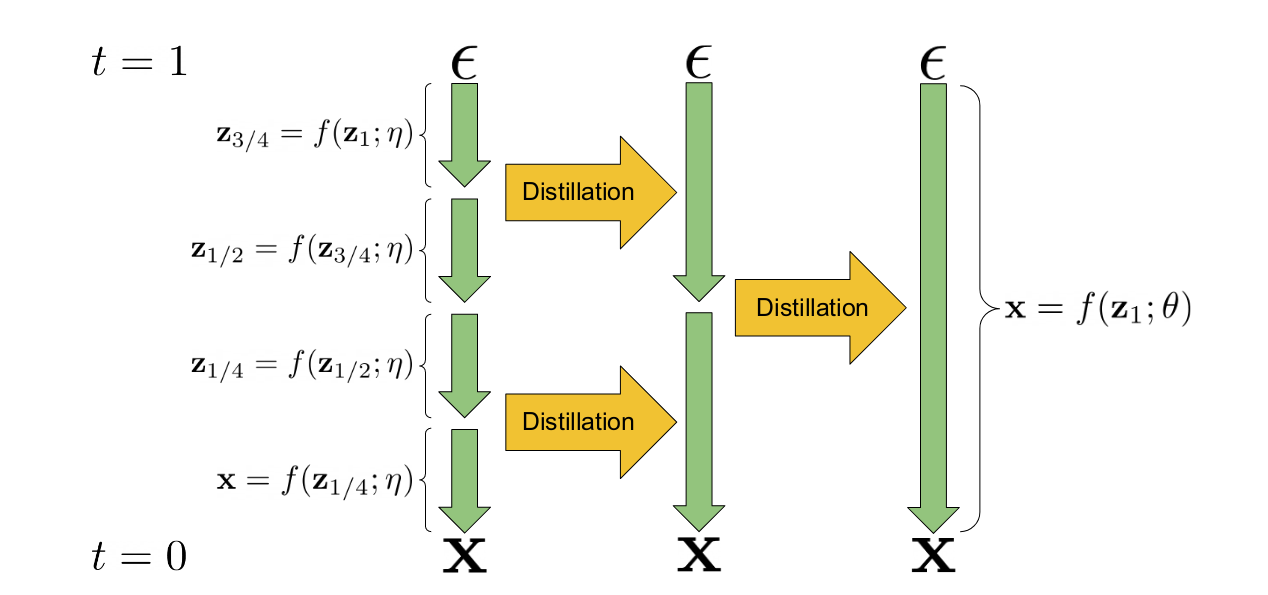
+
+_渐进蒸馏图示 (来源于[该篇论文](http://arxiv.org/abs/2202.00512))_
+
+使用现有模型“教授”新模型的想法可以扩展到创建指导模型,其中教师模型使用无分类器指导技术,学生模型必须学会在指定目标指导尺度的额外输入的基础上,在单个步骤中产生等价的输出。[这个视频](https://www.youtube.com/watch?v=ZXuK6IRJlnk) 对该方法做了一个概述。
+
+NB: Stable Diffusion的蒸馏版将很快发布。
+
+主要文献:
+- [Progressive Distillation For Fast Sampling Of Diffusion Models](http://arxiv.org/abs/2202.00512)
+- [On Distillation Of Guided Diffusion Models](http://arxiv.org/abs/2210.03142)
+
+## 训练改进
+
+研究者们现在已经开发了一些额外的技巧来改进扩散模型的训练过程。在本节中,我们试图从最近的论文中寻找一些相关的核心理念。更多关于改进方法的研究论文也是不断涌现,因此,如果你看到一篇你觉得应该添加在这里的论文,请联系我们!
+
+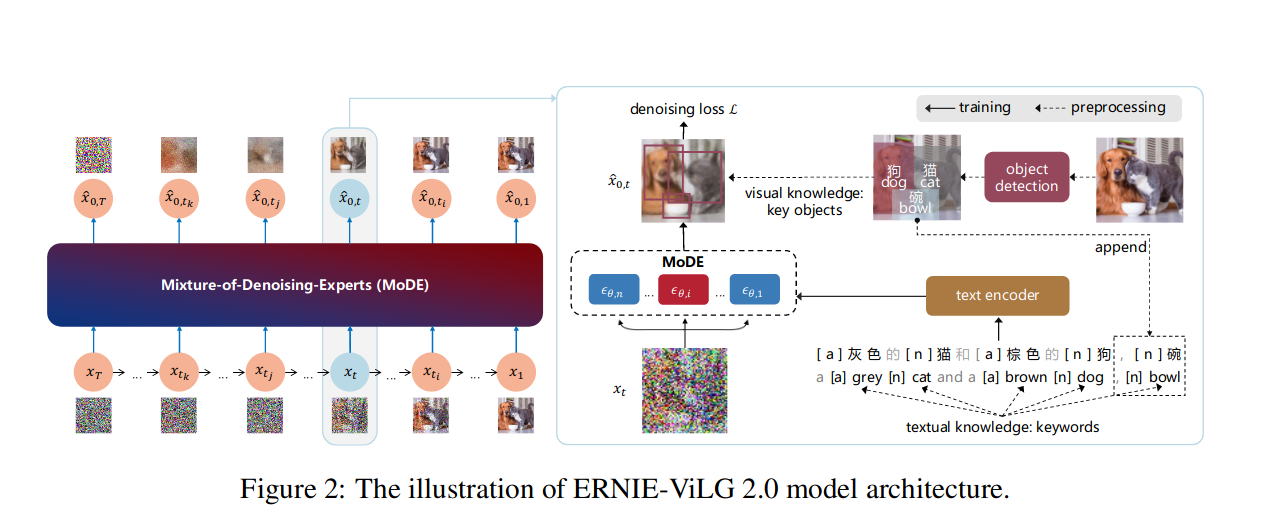
+_图像2来自于 [ERNIE-ViLG 2.0 paper](http://arxiv.org/abs/2210.15257)_
+
+关键的训练改进:
+- 调整噪声计划、损失加权和采样轨迹,以获得更有效的训练。这里是一篇探讨这些设计选择的优秀论文 [Elucidating the Design Space of Diffusion-Based Generative Models](http://arxiv.org/abs/2206.00364) by Karras et al.
+- 在不同的长宽比上进行训练。这一方法被描述在[课程启动活动提到的这个视频中](https://www.youtube.com/watch?v=g6tIUrMvOec)
+- 级联扩散模型,首先训练一个低分辨率模型,然后训练一个或多个超分辨率模型。这一方法被广泛运用于DALLE-2,Imagen等高分辨率图像生成模型中。
+- Better conditioning, incorporating rich text embeddings ([Imagen](https://arxiv.org/abs/2205.11487) uses a large language model called T5) or multiple types of conditioning ([eDiffi](http://arxiv.org/abs/2211.01324))
+- 更好的调节、结合富文本嵌入 ([Imagen](https://arxiv.org/abs/2205.11487) 使用了一个名为T-5的大语言模型) 或进行多种类型的调节 ([eDiffi](http://arxiv.org/abs/2211.01324))
+- “知识增强” - 将预先训练的图像描述和物体检测模型纳入训练过程,以创建更有信息的描述,并产生更好的表现 ([ERNIE-ViLG 2.0](http://arxiv.org/abs/2210.15257))
+- “复合降噪专家” (MoDE) - 训练模型的不同变体(“专家”)以适应不同的噪声水平,如上图所示 [ERNIE-ViLG 2.0 paper](http://arxiv.org/abs/2210.15257).
+
+主要文献:
+- [Elucidating the Design Space of Diffusion-Based Generative Models](http://arxiv.org/abs/2206.00364)
+- [eDiffi: Text-to-Image Diffusion Models with an Ensemble of Expert Denoisers](http://arxiv.org/abs/2211.01324)
+- [ERNIE-ViLG 2.0: Improving Text-to-Image Diffusion Model with Knowledge-Enhanced Mixture-of-Denoising-Experts](http://arxiv.org/abs/2210.15257)
+- [Imagen - Photorealistic Text-to-Image Diffusion Models with Deep Language Understanding](https://arxiv.org/abs/2205.11487) ([demo site](https://imagen.research.google/))
+
+## 对生成与编辑的更多控制
+
+除了训练方面的改进,在采样和推断阶段也有一些创新方法。这其中有许多甚至可以为现有扩散模型添加新的功能。
+
+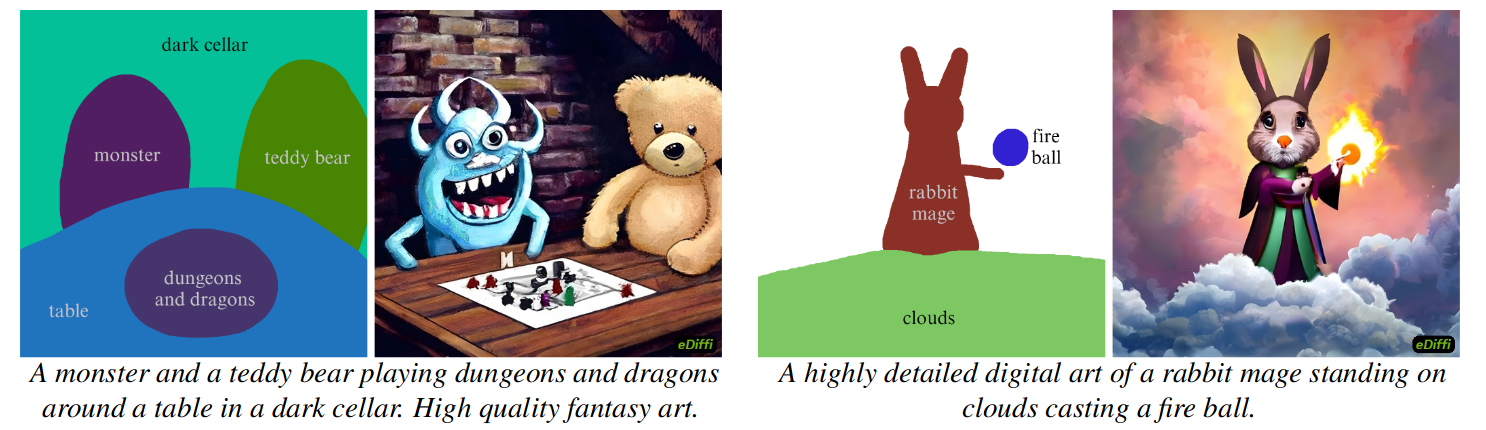
+_由'paint-with-words'生成的样本 ([eDiffi](http://arxiv.org/abs/2211.01324))_
+
+这个视频 ['使用扩散模型编辑图片'](https://www.youtube.com/watch?v=zcG7tG3xS3s) 概述了如何使用的不同的方法来通过应用扩散模型对已有的图片进行编辑。可用的技术主要分为四个主要类别:
+1) 添加噪声,然后用一个新的提示(prompt)来进行去噪. 这就是 'img2img ' 管道背后的思想,已经在各种论文中得到了扩展:
+- [SDEdit](https://sde-image-editing.github.io/) and [MagicMix](https://magicmix.github.io/) 这两篇论文都是建立在这一理念的基础上的
+- DDIM 反演 (TODO link tutorial)使用模型来“反转”采样轨迹来取代添加随机噪声, 从而提供了更多的控制
+- [Null-text Inversion](https://null-text-inversion.github.io/) 通过在每一步中优化用于无分类器引导的无条件文本嵌入,允许极高质量的基于文本的图像编辑,极大地增强了这种方法的性能。
+
+2) 扩展了(1)中的思想,但使用蒙版来控制效果的应用位置
+- [Blended Diffusion](https://omriavrahami.com/blended-diffusion-page/) 介绍了基础的理念
+- [这个 demo](https://huggingface.co/spaces/nielsr/text-based-inpainting) 使用现有的分割模型(CLIPSeg)来创建基于文本描述的掩码
+- [DiffEdit](https://arxiv.org/abs/2210.11427) 是一篇展示了如何使用扩散模型本身来生成适当的掩码,以根据文本编辑图像的优秀论文。
+- [SmartBrush: Text and Shape Guided Object Inpainting with Diffusion Model](https://arxiv.org/abs/2212.05034) 微调扩散模型以获得更准确的掩模引导图像修补。
+
+3) 交叉注意力控制:利用扩散模型中的交叉注意力机制控制编辑的空间位置,实现更细粒度的控制。
+- [Prompt-to-Prompt Image Editing with Cross Attention Control](https://arxiv.org/abs/2208.01626) 是介绍这一想法的关键论文,并且该技术[已经应用于Stable Diffusion](https://wandb.ai/wandb/cross-attention-control/reports/Improving-Generative-Images-with-Instructions-Prompt-to-Prompt-Image-Editing-with-Cross-Attention-Control--VmlldzoyNjk2MDAy)
+- 这个想法也被用于 'paint-with-words'中 ([eDiffi](http://arxiv.org/abs/2211.01324))
+
+4) 对单个图像进行微调(“过拟合”),然后使用微调模型进行图像生成。以下论文几乎在同一时间发表了非常类似的想法:
+- [Imagic: Text-Based Real Image Editing with Diffusion Models](https://arxiv.org/abs/2210.09276)
+- [UniTune: Text-Driven Image Editing by Fine Tuning an Image Generation Model on a Single Image
+](https://arxiv.org/abs/2210.09477)
+
+值得注意的是,这篇论文 [InstructPix2Pix: Learning to Follow Image Editing Instructions](https://arxiv.org/abs/2211.09800) 使用了上面描述的一些图像编辑技术来构建一个图像对的合成数据集以及图像编辑指令(由GPT3.5生成),训练生成了一个能够基于自然语言指令编辑图像的新模型。
+
+
+## 视频
+
+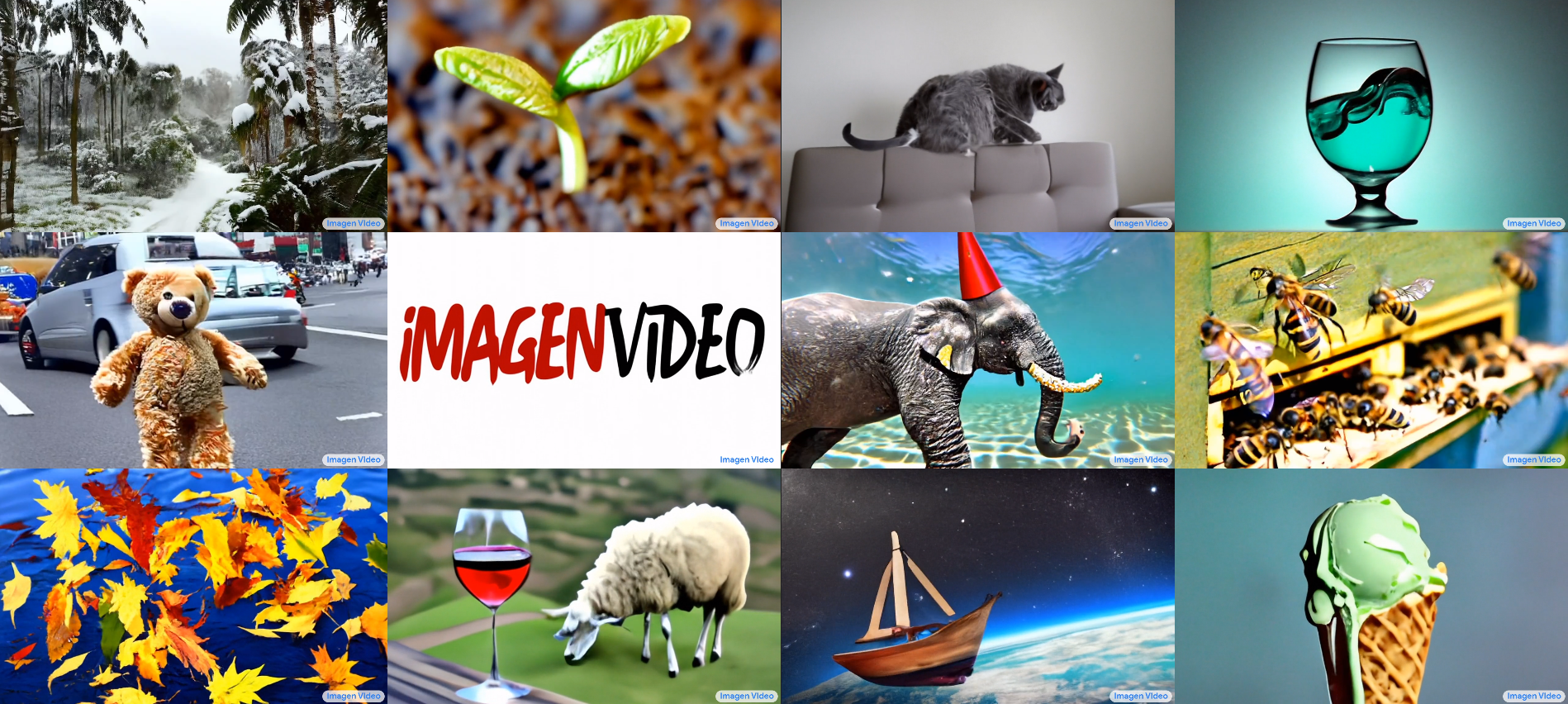
+_Still frames from [样本视频通过Imagen Video生成](https://imagen.research.google/video/)_
+
+视频可以表示为一组图像的序列,而扩散模型的核心思想可以被应用于这些序列。最近的工作主要集中在寻找合适的架构(例如“3D unet”,它可以对整个序列进行操作)并有效地处理视频数据。由于高帧率视频比静止图像涉及更多的数据,目前的方法倾向于首先生成低分辨率和低帧率视频,然后应用空间和时间超分辨率方法来生成最终的高质量视频输出。
+
+主要文献:
+- [Video Diffusion Models](https://video-diffusion.github.io/)
+- [IMAGEN VIDEO: HIGH DEFINITION VIDEO GENERATION WITH DIFFUSION MODELS](https://imagen.research.google/video/paper.pdf)
+
+## 音频
+
+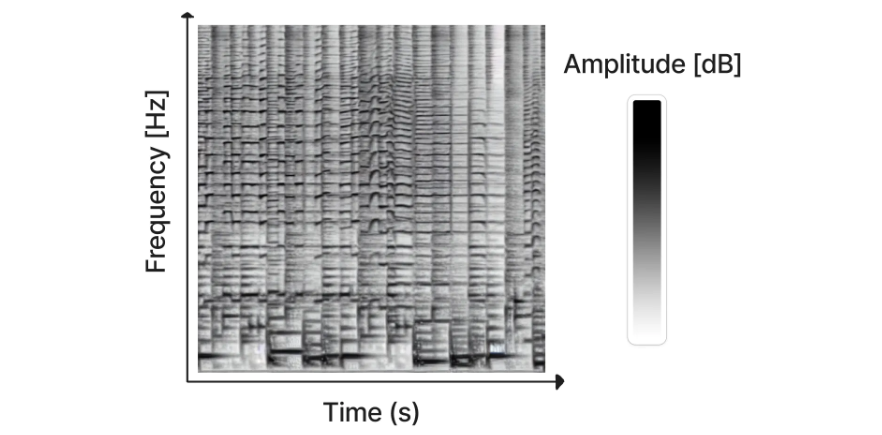
+
+_用Riffusion生成的一幅光谱图 ([图片来源](https://www.riffusion.com/about))_
+
+虽然已经有一些直接使用扩散模型生成音频的工作(e.g. [DiffWave](https://arxiv.org/abs/2009.09761)),但迄今为止最成功的方法还是将音频信号转换为一种称为频谱图的东西,这种方法有效地将音频“编码”为2D“图像”,然后可以用于训练我们用来做图像生成的扩散模型。之后就可以使用现有方法将生成的频谱图转换为音频。最近发布的Riffusion模型就是基于这样的设计思路。该模型通过微调Stable Diffusion模型来基于文字生成频谱图 - [点击这里进行尝试](https://www.riffusion.com/)。
+
+音频生成领域发展非常迅速。至少有5个新的进展在过去的一周(在撰写本文时)被发布了。在下面的列表中,我们用星号将他们标了出来:
+
+主要文献:
+- [DiffWave: A Versatile Diffusion Model for Audio Synthesis](https://arxiv.org/abs/2009.09761)
+- ['Riffusion'](https://www.riffusion.com/about) (and [code](https://github.com/riffusion/riffusion))
+- *[MusicLM](https://google-research.github.io/seanet/musiclm/examples/) 通过谷歌从文本生成一致的音频,并可以调节哼唱或吹口哨的旋律
+- *[RAVE2](https://github.com/acids-ircam/RAVE) - 一个新版本的变分自动编码器,可以被用在latent diffusion音频任务上。 这个新结构将被用在下面这篇即将发布的模型中 *[AudioLDM](https://twitter.com/LiuHaohe/status/1619119637660327936?s=20&t=jMkPWBFuAH19HI9m5Sklmg)
+- *[Noise2Music](https://noise2music.github.io/) - 该扩散模型可以基于文本描述来生成一段高质量的30秒音频片段
+- *[Make-An-Audio: Text-To-Audio Generation with Prompt-Enhanced Diffusion Models](https://text-to-audio.github.io/) - 该扩散模型可以基于文本描述来生成不同的声音
+- *[Moûsai: Text-to-Music Generation with Long-Context Latent Diffusion](https://arxiv.org/abs/2301.11757)
+
+## 新的体系结构和方法 - 走向“迭代优化”
+
+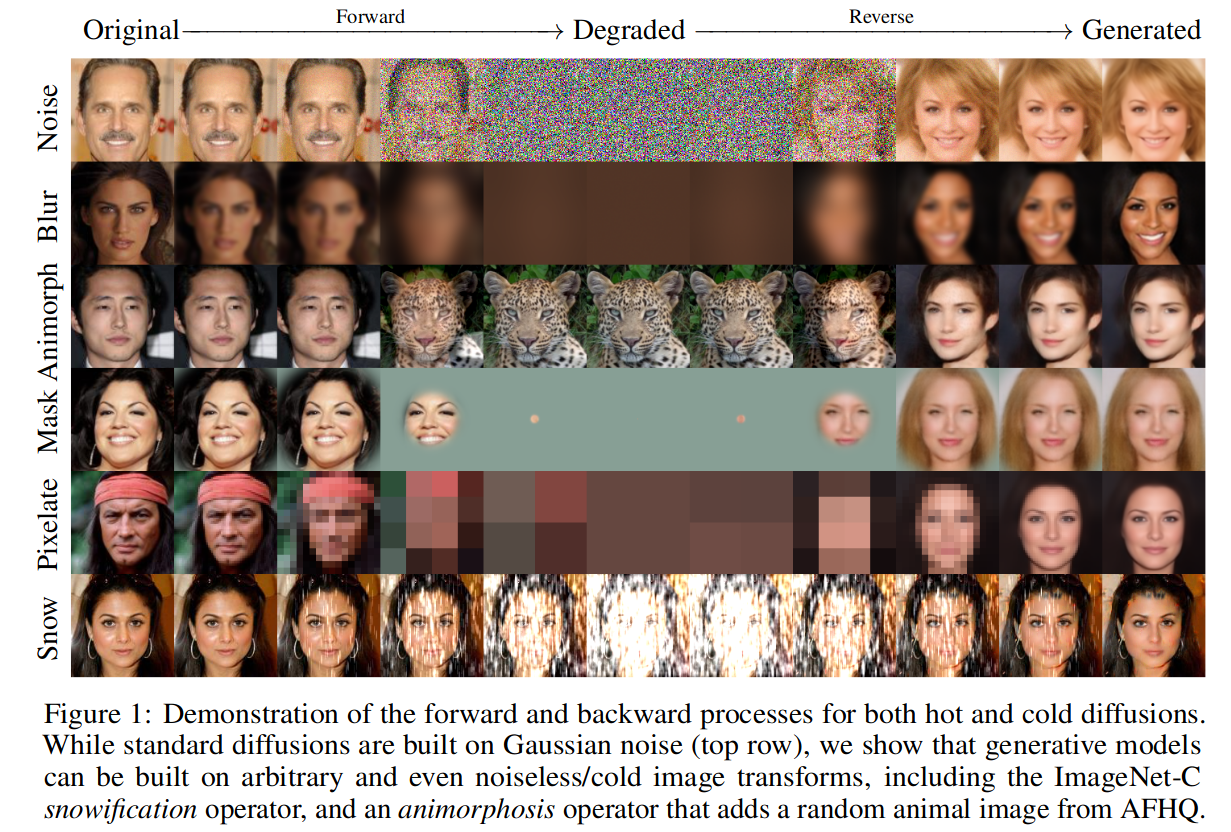
+
+_图片1来自 [Cold Diffusion](http://arxiv.org/abs/2208.09392) paper_
+
+我们正在慢慢地超越最初的“扩散”模型的狭义定义,并向更一般的、执行**迭代优化**的一类模型前进。这其中某种形式的破坏(例如在正向扩散过程中添加高斯噪声)被逐渐逆转以生成样本。这篇有关“冷扩散”的论文证明了许多其他类型的退化过程可以迭代地被“撤销”以生成图像(如上所示的示例)。同时最近基于transformer的方法也证明了符记替换(token replacement)或遮蔽(masking)作为噪声策略的有效性。
+
+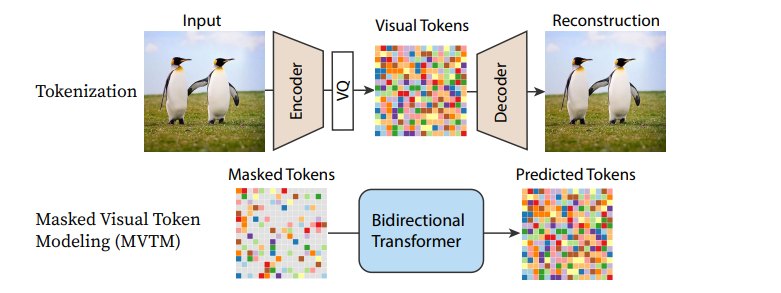
+
+_Pipeline from [MaskGIT](http://arxiv.org/abs/2202.04200)_
+
+目前许多扩散模型的核心UNet架构也正在被不同的替代方案所取代,最显著的是各种基于transformer的架构。在 [Scalable Diffusion Models with Transformers (DiT)](https://www.wpeebles.com/DiT)中,transformer结构被用来替换了一个标准扩散模型方法中的UNet结构, 并获得了相当不错的成果。 [Recurrent Interface Networks](https://arxiv.org/pdf/2212.11972.pdf) 应用一种新的基于transformer的架构和训练策略,以追求额外的效率。[MaskGIT](http://arxiv.org/abs/2202.04200) 和 [MUSE](http://arxiv.org/abs/2301.00704) 使用 transformer 模型来处理图像的标记化表示。 而[Paella](https://arxiv.org/abs/2211.07292v1)这篇文章也表示,UNet结构同样可以在这些token-based的设计中成功发挥作用。
+
+随着每一篇新论文的发表,更有效的方法正在陆续被开发出来。我们可能还需要一段时间才能看到这种迭代细化任务的巅峰性能。我们十分期待还有更多的东西被探索和发掘!
+
+主要文献
+- [Cold Diffusion: Inverting Arbitrary Image Transforms Without Noise](http://arxiv.org/abs/2208.09392)
+- [Scalable Diffusion Models with Transformers (DiT)](https://www.wpeebles.com/DiT)
+- [MaskGIT: Masked Generative Image Transformer](http://arxiv.org/abs/2202.04200)
+- [Muse: Text-To-Image Generation via Masked Generative Transformers](http://arxiv.org/abs/2301.00704)
+- [Fast Text-Conditional Discrete Denoising on Vector-Quantized Latent Spaces (Paella)](https://arxiv.org/abs/2211.07292v1)
+- [Recurrent Interface Networks](https://arxiv.org/pdf/2212.11972.pdf) - 一种很有前途的新架构,可以在不依赖latent diffusion或超分辨率模型的情况下生成高分辨率图像。另请参阅 [simple diffusion: End-to-end diffusion for high-resolution images](https://arxiv.org/abs/2301.11093) 该论文强调了噪声表(noise schedule)在高分辨率训练中的重要性。
+
+## 动手笔记本
+
+| 章节 | Colab | Kaggle | Gradient | Studio Lab |
+|:--------------------------------------------|:----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------|:---------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------|:---------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------|:-------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------|
+| DDIM Inversion | [](https://colab.research.google.com/github/huggingface/diffusion-models-class/blob/main/unit4/01_ddim_inversion.ipynb) | [](https://kaggle.com/kernels/welcome?src=https://github.com/huggingface/diffusion-models-class/blob/main/unit4/01_ddim_inversion.ipynb) | [](https://console.paperspace.com/github/huggingface/diffusion-models-class/blob/main/unit4/01_ddim_inversion.ipynb) | [](https://studiolab.sagemaker.aws/import/github/huggingface/diffusion-models-class/blob/main/unit4/01_ddim_inversion.ipynb) |
+| Diffusion for Audio | [](https://colab.research.google.com/github/huggingface/diffusion-models-class/blob/main/unit4/02_diffusion_for_audio.ipynb) | [](https://kaggle.com/kernels/welcome?src=https://github.com/huggingface/diffusion-models-class/blob/main/unit4/02_diffusion_for_audio.ipynb) | [](https://console.paperspace.com/github/huggingface/diffusion-models-class/blob/main/unit4/02_diffusion_for_audio.ipynb) | [](https://studiolab.sagemaker.aws/import/github/huggingface/diffusion-models-class/blob/main/unit4/02_diffusion_for_audio.ipynb) |
+
+在本单元中,我们已经讨论了很多不同的想法,其中许多值得在未来的后续课程中进行更详细的学习。现在,你可以通过我们准备的动手笔记本来学习其中的两个主题。
+- **DDIM Inversion** 展示了如何使用一种被称为“反转”的技术来使用已有的扩散模型编辑图像
+- **Diffusion for Audio** 介绍了频谱图的思想,并展示了如何对特定音乐风格的音频扩散模型进行微调。
+
+## 下一步?
+
+这是本课程的最后一个单元,这意味着接下来要做什么取决于你! 请记得你永远都可以来Hugging Face [discord频道](https://huggingface.co/join/discord)上来询问问题或者聊聊你自己的project。 我们期待看到你的创作! 🤗